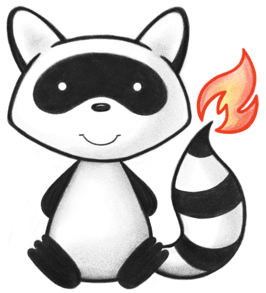
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3ObservationValue { 041 042 /** 043 * Codes specify the category of observation, evidence, or document used to assess for services, e.g., discharge planning, or to establish eligibility for coverage under a policy or program. The type of evidence is coded as observation values. 044 */ 045 _ACTCOVERAGEASSESSMENTOBSERVATIONVALUE, 046 /** 047 * Code specifying financial indicators used to assess or establish eligibility for coverage under a policy or program; e.g., pay stub; tax or income document; asset document; living expenses. 048 */ 049 _ACTFINANCIALSTATUSOBSERVATIONVALUE, 050 /** 051 * Codes specifying asset indicators used to assess or establish eligibility for coverage under a policy or program. 052 */ 053 ASSET, 054 /** 055 * Indicator of annuity ownership or status as beneficiary. 056 */ 057 ANNUITY, 058 /** 059 * Indicator of real property ownership, e.g., deed or real estate contract. 060 */ 061 PROP, 062 /** 063 * Indicator of retirement investment account ownership. 064 */ 065 RETACCT, 066 /** 067 * Indicator of status as trust beneficiary. 068 */ 069 TRUST, 070 /** 071 * Code specifying income indicators used to assess or establish eligibility for coverage under a policy or program; e.g., pay or pension check, child support payments received or provided, and taxes paid. 072 */ 073 INCOME, 074 /** 075 * Indicator of child support payments received or provided. 076 */ 077 CHILD, 078 /** 079 * Indicator of disability income replacement payment. 080 */ 081 DISABL, 082 /** 083 * Indicator of investment income, e.g., dividend check, annuity payment; real estate rent, investment divestiture proceeds; trust or endowment check. 084 */ 085 INVEST, 086 /** 087 * Indicator of paid employment, e.g., letter of hire, contract, employer letter; copy of pay check or pay stub. 088 */ 089 PAY, 090 /** 091 * Indicator of retirement payment, e.g., pension check. 092 */ 093 RETIRE, 094 /** 095 * Indicator of spousal or partner support payments received or provided; e.g., alimony payment; support stipulations in a divorce settlement. 096 */ 097 SPOUSAL, 098 /** 099 * Indicator of income supplement, e.g., gifting, parental income support; stipend, or grant. 100 */ 101 SUPPLE, 102 /** 103 * Indicator of tax obligation or payment, e.g., statement of taxable income. 104 */ 105 TAX, 106 /** 107 * Codes specifying living expense indicators used to assess or establish eligibility for coverage under a policy or program. 108 */ 109 LIVEXP, 110 /** 111 * Indicator of clothing expenses. 112 */ 113 CLOTH, 114 /** 115 * Indicator of transportation expenses. 116 */ 117 FOOD, 118 /** 119 * Indicator of health expenses; including medication costs, health service costs, financial participations, and health coverage premiums. 120 */ 121 HEALTH, 122 /** 123 * Indicator of housing expense, e.g., household appliances, fixtures, furnishings, and maintenance and repairs. 124 */ 125 HOUSE, 126 /** 127 * Indicator of legal expenses. 128 */ 129 LEGAL, 130 /** 131 * Indicator of mortgage amount, interest, and payments. 132 */ 133 MORTG, 134 /** 135 * Indicator of rental or lease payments. 136 */ 137 RENT, 138 /** 139 * Indicator of transportation expenses. 140 */ 141 SUNDRY, 142 /** 143 * Indicator of transportation expenses, e.g., vehicle payments, vehicle insurance, vehicle fuel, and vehicle maintenance and repairs. 144 */ 145 TRANS, 146 /** 147 * Indicator of transportation expenses. 148 */ 149 UTIL, 150 /** 151 * Code specifying eligibility indicators used to assess or establish eligibility for coverage under a policy or program eligibility status, e.g., certificates of creditable coverage; student enrollment; adoption, marriage or birth certificate. 152 */ 153 ELSTAT, 154 /** 155 * Indicator of adoption. 156 */ 157 ADOPT, 158 /** 159 * Indicator of birth. 160 */ 161 BTHCERT, 162 /** 163 * Indicator of creditable coverage. 164 */ 165 CCOC, 166 /** 167 * Indicator of driving status. 168 */ 169 DRLIC, 170 /** 171 * Indicator of foster child status. 172 */ 173 FOSTER, 174 /** 175 * Indicator of status as covered member under a policy or program, e.g., member id card or coverage document. 176 */ 177 MEMBER, 178 /** 179 * Indicator of military status. 180 */ 181 MIL, 182 /** 183 * Indicator of marriage status. 184 */ 185 MRGCERT, 186 /** 187 * Indicator of citizenship. 188 */ 189 PASSPORT, 190 /** 191 * Indicator of student status. 192 */ 193 STUDENRL, 194 /** 195 * Code specifying non-clinical indicators related to health status used to assess or establish eligibility for coverage under a policy or program, e.g., pregnancy, disability, drug use, mental health issues. 196 */ 197 HLSTAT, 198 /** 199 * Indication of disability. 200 */ 201 DISABLE, 202 /** 203 * Indication of drug use. 204 */ 205 DRUG, 206 /** 207 * Indication of IV drug use . 208 */ 209 IVDRG, 210 /** 211 * Non-clinical report of pregnancy. 212 */ 213 PGNT, 214 /** 215 * Code specifying observations related to living dependency, such as dependent upon spouse for activities of daily living. 216 */ 217 LIVDEP, 218 /** 219 * Continued living in private residence requires functional and health care assistance from one or more relatives. 220 */ 221 RELDEP, 222 /** 223 * Continued living in private residence requires functional and health care assistance from spouse or life partner. 224 */ 225 SPSDEP, 226 /** 227 * Continued living in private residence requires functional and health care assistance from one or more unrelated persons. 228 */ 229 URELDEP, 230 /** 231 * Code specifying observations related to living situation for a person in a private residence. 232 */ 233 LIVSIT, 234 /** 235 * Living alone. Maps to PD1-2 Living arrangement (IS) 00742 [A] 236 */ 237 ALONE, 238 /** 239 * Living with one or more dependent children requiring moderate supervision. 240 */ 241 DEPCHD, 242 /** 243 * Living with disabled spouse requiring functional and health care assistance 244 */ 245 DEPSPS, 246 /** 247 * Living with one or more dependent children requiring intensive supervision 248 */ 249 DEPYGCHD, 250 /** 251 * Living with family. Maps to PD1-2 Living arrangement (IS) 00742 [F] 252 */ 253 FAM, 254 /** 255 * Living with one or more relatives. Maps to PD1-2 Living arrangement (IS) 00742 [R] 256 */ 257 RELAT, 258 /** 259 * Living only with spouse or life partner. Maps to PD1-2 Living arrangement (IS) 00742 [S] 260 */ 261 SPS, 262 /** 263 * Living with one or more unrelated persons. 264 */ 265 UNREL, 266 /** 267 * Code specifying observations or indicators related to socio-economic status used to assess to assess for services, e.g., discharge planning, or to establish eligibility for coverage under a policy or program. 268 */ 269 SOECSTAT, 270 /** 271 * Indication of abuse victim. 272 */ 273 ABUSE, 274 /** 275 * Indication of status as homeless. 276 */ 277 HMLESS, 278 /** 279 * Indication of status as illegal immigrant. 280 */ 281 ILGIM, 282 /** 283 * Indication of status as incarcerated. 284 */ 285 INCAR, 286 /** 287 * Indication of probation status. 288 */ 289 PROB, 290 /** 291 * Indication of refugee status. 292 */ 293 REFUG, 294 /** 295 * Indication of unemployed status. 296 */ 297 UNEMPL, 298 /** 299 * Indicates the result of a particular allergy test. E.g. Negative, Mild, Moderate, Severe 300 */ 301 _ALLERGYTESTVALUE, 302 /** 303 * Description:Patient exhibits no reaction to the challenge agent. 304 */ 305 A0, 306 /** 307 * Description:Patient exhibits a minimal reaction to the challenge agent. 308 */ 309 A1, 310 /** 311 * Description:Patient exhibits a mild reaction to the challenge agent. 312 */ 313 A2, 314 /** 315 * Description:Patient exhibits moderate reaction to the challenge agent. 316 */ 317 A3, 318 /** 319 * Description:Patient exhibits a severe reaction to the challenge agent. 320 */ 321 A4, 322 /** 323 * Observation values that communicate the method used in a quality measure to combine the component measure results included in an composite measure. 324 */ 325 _COMPOSITEMEASURESCORING, 326 /** 327 * Code specifying that the measure uses all-or-nothing scoring. All-or-nothing scoring places an individual in the numerator of the composite measure if and only if they are in the numerator of all component measures in which they are in the denominator. 328 */ 329 ALLORNONESCR, 330 /** 331 * Code specifying that the measure uses linear scoring. Linear scoring computes the fraction of component measures in which the individual appears in the numerator, giving equal weight to each component measure. 332 */ 333 LINEARSCR, 334 /** 335 * Code specifying that the measure uses opportunity-based scoring. In opportunity-based scoring the measure score is determined by combining the denominator and numerator of each component measure to determine an overall composite score. 336 */ 337 OPPORSCR, 338 /** 339 * Code specifying that the measure uses weighted scoring. Weighted scoring assigns a factor to each component measure to weight that measure's contribution to the overall score. 340 */ 341 WEIGHTSCR, 342 /** 343 * Description:Coded observation values for coverage limitations, for e.g., types of claims or types of parties covered under a policy or program. 344 */ 345 _COVERAGELIMITOBSERVATIONVALUE, 346 /** 347 * Description:Coded observation values for types of covered parties under a policy or program based on their personal relationships or employment status. 348 */ 349 _COVERAGELEVELOBSERVATIONVALUE, 350 /** 351 * Description:Child over an age as specified by coverage policy or program, e.g., student, differently abled, and income dependent. 352 */ 353 ADC, 354 /** 355 * Description:Dependent biological, adopted, foster child as specified by coverage policy or program. 356 */ 357 CHD, 358 /** 359 * Description:Person requiring functional and/or financial assistance from another person as specified by coverage policy or program. 360 */ 361 DEP, 362 /** 363 * Description:Persons registered as a family unit in a domestic partner registry as specified by law and by coverage policy or program. 364 */ 365 DP, 366 /** 367 * Description:An individual employed by an employer who receive remuneration in wages, salary, commission, tips, piece-rates, or pay-in-kind through the employeraTMs payment system (i.e., not a contractor) as specified by coverage policy or program. 368 */ 369 ECH, 370 /** 371 * Description:As specified by coverage policy or program. 372 */ 373 FLY, 374 /** 375 * Description:Person as specified by coverage policy or program. 376 */ 377 IND, 378 /** 379 * Description:A pair of people of the same gender who live together as a family as specified by coverage policy or program, e.g., Naomi and Ruth from the Book of Ruth; Socrates and Alcibiades 380 */ 381 SSP, 382 /** 383 * A clinical judgment as to the worst case result of a future exposure (including substance administration). When the worst case result is assessed to have a life-threatening or organ system threatening potential, it is considered to be of high criticality. 384 */ 385 _CRITICALITYOBSERVATIONVALUE, 386 /** 387 * Worst case result of a future exposure is assessed to be life-threatening or having high potential for organ system failure. 388 */ 389 CRITH, 390 /** 391 * Worst case result of a future exposure is not assessed to be life-threatening or having high potential for organ system failure. 392 */ 393 CRITL, 394 /** 395 * Unable to assess the worst case result of a future exposure. 396 */ 397 CRITU, 398 /** 399 * Description: The domain contains genetic analysis specific observation values, e.g. Homozygote, Heterozygote, etc. 400 */ 401 _GENETICOBSERVATIONVALUE, 402 /** 403 * Description: An individual having different alleles at one or more loci regarding a specific character 404 */ 405 HOMOZYGOTE, 406 /** 407 * Observation values used to indicate the type of scoring (e.g. proportion, ratio) used by a health quality measure. 408 */ 409 _OBSERVATIONMEASURESCORING, 410 /** 411 * A measure in which either short-term cross-section or long-term longitudinal analysis is performed over a group of subjects defined by a set of common properties or defining characteristics (e.g., Male smokers between the ages of 40 and 50 years, exposure to treatment, exposure duration). 412 */ 413 COHORT, 414 /** 415 * A measure score in which each individual value for the measure can fall anywhere along a continuous scale (e.g., mean time to thrombolytics which aggregates the time in minutes from a case presenting with chest pain to the time of administration of thrombolytics). 416 */ 417 CONTVAR, 418 /** 419 * A score derived by dividing the number of cases that meet a criterion for quality (the numerator) by the number of eligible cases within a given time frame (the denominator) where the numerator cases are a subset of the denominator cases (e.g., percentage of eligible women with a mammogram performed in the last year). 420 */ 421 PROPOR, 422 /** 423 * A score that may have a value of zero or greater that is derived by dividing a count of one type of data by a count of another type of data (e.g., the number of patients with central lines who develop infection divided by the number of central line days). 424 */ 425 RATIO, 426 /** 427 * Observation values used to indicate what kind of health quality measure is used. 428 */ 429 _OBSERVATIONMEASURETYPE, 430 /** 431 * A measure that is composed from one or more other measures and indicates an overall summary of those measures. 432 */ 433 COMPOSITE, 434 /** 435 * A measure related to the efficiency of medical treatment. 436 */ 437 EFFICIENCY, 438 /** 439 * A measure related to the level of patient engagement or patient experience of care. 440 */ 441 EXPERIENCE, 442 /** 443 * A measure that indicates the result of the performance (or non-performance) of a function or process. 444 */ 445 OUTCOME, 446 /** 447 * A measure which focuses on a process which leads to a certain outcome, meaning that a scientific basis exists for believing that the process, when executed well, will increase the probability of achieving a desired outcome. 448 */ 449 PROCESS, 450 /** 451 * A measure related to the extent of use of clinical resources or cost of care. 452 */ 453 RESOURCE, 454 /** 455 * A measure related to the structure of patient care. 456 */ 457 STRUCTURE, 458 /** 459 * Observation values used to assert various populations that a subject falls into. 460 */ 461 _OBSERVATIONPOPULATIONINCLUSION, 462 /** 463 * Patients who should be removed from the eMeasure population and denominator before determining if numerator criteria are met. Denominator exclusions are used in proportion and ratio measures to help narrow the denominator. 464 */ 465 DENEX, 466 /** 467 * Denominator exceptions are those conditions that should remove a patient, procedure or unit of measurement from the denominator only if the numerator criteria are not met. Denominator exceptions allow for adjustment of the calculated score for those providers with higher risk populations. Denominator exceptions are used only in proportion eMeasures. They are not appropriate for ratio or continuous variable eMeasures. Denominator exceptions allow for the exercise of clinical judgment and should be specifically defined where capturing the information in a structured manner fits the clinical workflow. Generic denominator exception reasons used in proportion eMeasures fall into three general categories: 468 469 470 Medical reasons 471 Patient reasons 472 System reasons 473 */ 474 DENEXCEP, 475 /** 476 * It can be the same as the initial patient population or a subset of the initial patient population to further constrain the population for the purpose of the eMeasure. Different measures within an eMeasure set may have different Denominators. Continuous Variable eMeasures do not have a Denominator, but instead define a Measure Population. 477 */ 478 DENOM, 479 /** 480 * The initial population refers to all entities to be evaluated by a specific quality measure who share a common set of specified characteristics within a specific measurement set to which a given measure belongs. 481 */ 482 IP, 483 /** 484 * The initial patient population refers to all patients to be evaluated by a specific quality measure who share a common set of specified characteristics within a specific measurement set to which a given measure belongs. Details often include information based upon specific age groups, diagnoses, diagnostic and procedure codes, and enrollment periods. 485 */ 486 IPP, 487 /** 488 * Measure population is used only in continuous variable eMeasures. It is a narrative description of the eMeasure population. 489(e.g., all patients seen in the Emergency Department during the measurement period). 490 */ 491 MSRPOPL, 492 /** 493 * Numerators are used in proportion and ratio eMeasures. In proportion measures the numerator criteria are the processes or outcomes expected for each patient, procedure, or other unit of measurement defined in the denominator. In ratio measures the numerator is related, but not directly derived from the denominator (e.g., a numerator listing the number of central line blood stream infections and a denominator indicating the days per thousand of central line usage in a specific time period). 494 */ 495 NUMER, 496 /** 497 * Numerator Exclusions are used only in ratio eMeasures to define instances that should not be included in the numerator data. (e.g., if the number of central line blood stream infections per 1000 catheter days were to exclude infections with a specific bacterium, that bacterium would be listed as a numerator exclusion.) 498 */ 499 NUMEX, 500 /** 501 * PartialCompletionScale 502 */ 503 _PARTIALCOMPLETIONSCALE, 504 /** 505 * Value for Act.partialCompletionCode attribute that implies 81-99% completion 506 */ 507 G, 508 /** 509 * Value for Act.partialCompletionCode attribute that implies 61-80% completion 510 */ 511 LE, 512 /** 513 * Value for Act.partialCompletionCode attribute that implies 41-60% completion 514 */ 515 ME, 516 /** 517 * Value for Act.partialCompletionCode attribute that implies 1-20% completion 518 */ 519 MI, 520 /** 521 * Value for Act.partialCompletionCode attribute that implies 0% completion 522 */ 523 N, 524 /** 525 * Value for Act.partialCompletionCode attribute that implies 21-40% completion 526 */ 527 S, 528 /** 529 * Observation values used to indicate security observation metadata. 530 */ 531 _SECURITYOBSERVATIONVALUE, 532 /** 533 * Abstract security observation values used to indicate security integrity metadata. 534 535 536 Examples: Codes conveying integrity status, integrity confidence, and provenance. 537 */ 538 _SECINTOBV, 539 /** 540 * Abstract security metadata observation values used to indicate mechanism used for authorized alteration of an IT resource (data, information object, service, or system capability) 541 */ 542 _SECALTINTOBV, 543 /** 544 * Security metadata observation values used to indicate the use of a more abstract version of the content, e.g., replacing exact value of an age or date field with a range, or remove the left digits of a credit card number or SSN. 545 */ 546 ABSTRED, 547 /** 548 * Security metadata observation values used to indicate the use of an algorithmic combination of actual values with the result of an aggregate function, e.g., average, sum, or count in order to limit disclosure of an IT resource (data, information object, service, or system capability) to the minimum necessary. 549 */ 550 AGGRED, 551 /** 552 * Security metadata observation value conveying the alteration integrity of an IT resource (data, information object, service, or system capability) by used to indicate the mechanism by which software systems can strip portions of the resource that could allow the identification of the source of the information or the information subject. No key to relink the data is retained. 553 */ 554 ANONYED, 555 /** 556 * Security metadata observation value used to indicate that the IT resource semantic content has been transformed from one encoding to another. 557 558 559 Usage Note: "MAP" code does not indicate the semantic fidelity of the transformed content. 560 561 To indicate semantic fidelity for maps of HL7 to other code systems, this security alteration integrity observation may be further specified using an Act valued with Value Set: MapRelationship (2.16.840.1.113883.1.11.11052). 562 563 Semantic fidelity of the mapped IT Resource may also be indicated using a SecurityIntegrityConfidenceObservation. 564 */ 565 MAPPED, 566 /** 567 * Security metadata observation value conveying the alteration integrity of an IT resource (data, information object, service, or system capability) by indicating the mechanism by which software systems can make data unintelligible (that is, as unreadable and unusable by algorithmically transforming plaintext into ciphertext) such that it can only be accessed or used by authorized users. An authorized user may be provided a key to decrypt per license or "shared secret". 568 569 570 Usage Note: "MASKED" may be used, per applicable policy, as a flag to indicate to a user or receiver that some portion of an IT resource has been further encrypted, and may be accessed only by an authorized user or receiver to which a decryption key is provided. 571 */ 572 MASKED, 573 /** 574 * Security metadata observation value conveying the alteration integrity of an IT resource (data, information object, service, or system capability), by indicating the mechanism by which software systems can strip portions of the resource that could allow the identification of the source of the information or the information subject. Custodian may retain a key to relink data necessary to reidentify the information subject. 575 576 577 Rationale: Personal data which has been processed to make it impossible to know whose data it is. Used particularly for secondary use of health data. In some cases, it may be possible for authorized individuals to restore the identity of the individual, e.g.,for public health case management. Based on ISO/TS 25237:2008 Health informaticsā??Pseudonymization 578 */ 579 PSEUDED, 580 /** 581 * Security metadata observation value used to indicate the mechanism by which software systems can filter an IT resource (data, information object, service, or system capability) to remove any portion of the resource that is not authorized to be access, used, or disclosed. 582 583 584 Usage Note: "REDACTED" may be used, per applicable policy, as a flag to indicate to a user or receiver that some portion of an IT resource has filtered and not included in the content accessed or received. 585 */ 586 REDACTED, 587 /** 588 * Metadata observation used to indicate that some information has been removed from the source object when the view this object contains was constructed because of configuration options when the view was created. The content may not be suitable for use as the basis of a record update 589 590 591 Usage Note: This is not suitable to be used when information is removed for security reasons - see the code REDACTED for this use. 592 */ 593 SUBSETTED, 594 /** 595 * Security metadata observation value used to indicate that the IT resource syntax has been transformed from one syntactical representation to another. 596 597 598 Usage Note: "SYNTAC" code does not indicate the syntactical correctness of the syntactically transformed IT resource. 599 */ 600 SYNTAC, 601 /** 602 * Security metadata observation value used to indicate that the IT resource has been translated from one human language to another. 603 604 605 Usage Note: "TRSLT" does not indicate the fidelity of the translation or the languages translated. 606 607 The fidelity of the IT Resource translation may be indicated using a SecurityIntegrityConfidenceObservation. 608 609 To indicate languages, use the Value Set:HumanLanguage (2.16.840.1.113883.1.11.11526) 610 */ 611 TRSLT, 612 /** 613 * Security metadata observation value conveying the alteration integrity of an IT resource (data, information object, service, or system capability) which indicates that the resource only retains versions of an IT resource for access and use per applicable policy 614 615 616 Usage Note: When this code is used, expectation is that the system has removed historical versions of the data that falls outside the time period deemed to be the effective time of the applicable version. 617 */ 618 VERSIONED, 619 /** 620 * Abstract security observation values used to indicate data integrity metadata. 621 622 623 Examples: Codes conveying the mechanism used to preserve the accuracy and consistency of an IT resource such as a digital signature and a cryptographic hash function. 624 */ 625 _SECDATINTOBV, 626 /** 627 * Security metadata observation value used to indicate the mechanism by which software systems can establish that data was not modified in transit. 628 629 630 Rationale: This definition is intended to align with the ISO 22600-2 3.3.19 definition of cryptographic checkvalue: Information which is derived by performing a cryptographic transformation (see cryptography) on the data unit. The derivation of the checkvalue may be performed in one or more steps and is a result of a mathematical function of the key and a data unit. It is usually used to check the integrity of a data unit. 631 632 633 Examples: 634 635 636 637 SHA-1 638 SHA-2 (Secure Hash Algorithm) 639 */ 640 CRYTOHASH, 641 /** 642 * Security metadata observation value used to indicate the mechanism by which software systems use digital signature to establish that data has not been modified. 643 644 645 Rationale: This definition is intended to align with the ISO 22600-2 3.3.26 definition of digital signature: Data appended to, or a cryptographic transformation (see cryptography) of, a data unit that allows a recipient of the data unit to prove the source and integrity of the data unit and protect against forgery e.g., by the recipient. 646 */ 647 DIGSIG, 648 /** 649 * Abstract security observation value used to indicate integrity confidence metadata. 650 651 652 Examples: Codes conveying the level of reliability and trustworthiness of an IT resource. 653 */ 654 _SECINTCONOBV, 655 /** 656 * Security metadata observation value used to indicate that the veracity or trustworthiness of an IT resource (data, information object, service, or system capability) for a specified purpose of use is perceived to be or deemed by policy to be very high. 657 */ 658 HRELIABLE, 659 /** 660 * Security metadata observation value used to indicate that the veracity or trustworthiness of an IT resource (data, information object, service, or system capability) for a specified purpose of use is perceived to be or deemed by policy to be adequate. 661 */ 662 RELIABLE, 663 /** 664 * Security metadata observation value used to indicate that the veracity or trustworthiness of an IT resource (data, information object, service, or system capability) for a specified purpose of use is perceived to be or deemed by policy to be uncertain. 665 */ 666 UNCERTREL, 667 /** 668 * Security metadata observation value used to indicate that the veracity or trustworthiness of an IT resource (data, information object, service, or system capability) for a specified purpose of use is perceived to be or deemed by policy to be inadequate. 669 */ 670 UNRELIABLE, 671 /** 672 * Abstract security metadata observation value used to indicate the provenance of an IT resource (data, information object, service, or system capability). 673 674 675 Examples: Codes conveying the provenance metadata about the entity reporting an IT resource. 676 */ 677 _SECINTPRVOBV, 678 /** 679 * Abstract security provenance metadata observation value used to indicate the entity that asserted an IT resource (data, information object, service, or system capability). 680 681 682 Examples: Codes conveying the provenance metadata about the entity asserting the resource. 683 */ 684 _SECINTPRVABOBV, 685 /** 686 * Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a clinician. 687 */ 688 CLINAST, 689 /** 690 * Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a device. 691 */ 692 DEVAST, 693 /** 694 * Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a healthcare professional. 695 */ 696 HCPAST, 697 /** 698 * Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a patient acquaintance. 699 */ 700 PACQAST, 701 /** 702 * Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a patient. 703 */ 704 PATAST, 705 /** 706 * Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a payer. 707 */ 708 PAYAST, 709 /** 710 * Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a professional. 711 */ 712 PROAST, 713 /** 714 * Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a substitute decision maker. 715 */ 716 SDMAST, 717 /** 718 * Abstract security provenance metadata observation value used to indicate the entity that reported the resource (data, information object, service, or system capability). 719 720 721 Examples: Codes conveying the provenance metadata about the entity reporting an IT resource. 722 */ 723 _SECINTPRVRBOBV, 724 /** 725 * Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a clinician. 726 */ 727 CLINRPT, 728 /** 729 * Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a device. 730 */ 731 DEVRPT, 732 /** 733 * Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a healthcare professional. 734 */ 735 HCPRPT, 736 /** 737 * Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a patient acquaintance. 738 */ 739 PACQRPT, 740 /** 741 * Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a patient. 742 */ 743 PATRPT, 744 /** 745 * Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a payer. 746 */ 747 PAYRPT, 748 /** 749 * Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a professional. 750 */ 751 PRORPT, 752 /** 753 * Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a substitute decision maker. 754 */ 755 SDMRPT, 756 /** 757 * Observation value used to indicate aspects of trust applicable to an IT resource (data, information object, service, or system capability). 758 */ 759 SECTRSTOBV, 760 /** 761 * Values for security trust accreditation metadata observation made about the formal declaration by an authority or neutral third party that validates the technical, security, trust, and business practice conformance of Trust Agents to facilitate security, interoperability, and trust among participants within a security domain or trust framework. 762 */ 763 TRSTACCRDOBV, 764 /** 765 * Values for security trust agreement metadata observation made about privacy and security requirements with which a security domain must comply. [ISO IEC 10181-1] 766[ISO IEC 10181-1] 767 */ 768 TRSTAGREOBV, 769 /** 770 * Values for security trust certificate metadata observation made about a set of security-relevant data issued by a security authority or trusted third party, together with security information which is used to provide the integrity and data origin authentication services for an IT resource (data, information object, service, or system capability). [Based on ISO IEC 10181-1] 771 772 For example, a Certificate Policy (CP), which is a named set of rules that indicates the applicability of a certificate to a particular community and/or class of application with common security requirements. A particular Certificate Policy might indicate the applicability of a type of certificate to the authentication of electronic data interchange transactions for the trading of goods within a given price range. Another example is Cross Certification with Federal Bridge. 773 */ 774 TRSTCERTOBV, 775 /** 776 * Values for security trust assurance metadata observation made about the digital quality or reliability of a trust assertion, activity, capability, information exchange, mechanism, process, or protocol. 777 */ 778 TRSTLOAOBV, 779 /** 780 * The value assigned as the indicator of the digital quality or reliability of the verification and validation process used to verify the claimed identity of an entity by securely associating an identifier and its authenticator. [Based on ISO 7498-2] 781 782 For example, the degree of confidence in the vetting process used to establish the identity of the individual to whom the credential was issued, and 2) the degree of confidence that the individual who uses the credential is the individual to whom the credential was issued. [OMB M-04-04 E-Authentication Guidance for Federal Agencies] 783 */ 784 LOAAN, 785 /** 786 * Indicator of low digital quality or reliability of the digital reliability of the verification and validation process used to verify the claimed identity of an entity by securely associating an identifier and its authenticator. [Based on ISO 7498-2] 787 788 The degree of confidence in the vetting process used to establish the identity of the individual to whom the credential was issued, and 2) the degree of confidence that the individual who uses the credential is the individual to whom the credential was issued. [OMB M-04-04 E-Authentication Guidance for Federal Agencies] 789 790 Low authentication level of assurance indicates that the relying party may have little or no confidence in the asserted identity's validity. Level 1 requires little or no confidence in the asserted identity. No identity proofing is required at this level, but the authentication mechanism should provide some assurance that the same claimant is accessing the protected transaction or data. A wide range of available authentication technologies can be employed and any of the token methods of Levels 2, 3, or 4, including Personal Identification Numbers (PINs), may be used. To be authenticated, the claimant must prove control of the token through a secure authentication protocol. At Level 1, long-term shared authentication secrets may be revealed to verifiers. Assertions issued about claimants as a result of a successful authentication are either cryptographically authenticated by relying parties (using approved methods) or are obtained directly from a trusted party via a secure authentication protocol. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.] 791 */ 792 LOAAN1, 793 /** 794 * Indicator of basic digital quality or reliability of the digital reliability of the verification and validation process used to verify the claimed identity of an entity by securely associating an identifier and its authenticator. [Based on ISO 7498-2] 795 796 The degree of confidence in the vetting process used to establish the identity of the individual to whom the credential was issued, and 2) the degree of confidence that the individual who uses the credential is the individual to whom the credential was issued. [OMB M-04-04 E-Authentication Guidance for Federal Agencies] 797 798 Basic authentication level of assurance indicates that the relying party may have some confidence in the asserted identity's validity. Level 2 requires confidence that the asserted identity is accurate. Level 2 provides for single-factor remote network authentication, including identity-proofing requirements for presentation of identifying materials or information. A wide range of available authentication technologies can be employed, including any of the token methods of Levels 3 or 4, as well as passwords. Successful authentication requires that the claimant prove through a secure authentication protocol that the claimant controls the token. Eavesdropper, replay, and online guessing attacks are prevented. 799Long-term shared authentication secrets, if used, are never revealed to any party except the claimant and verifiers operated by the CSP; however, session (temporary) shared secrets may be provided to independent verifiers by the CSP. Approved cryptographic techniques are required. Assertions issued about claimants as a result of a successful authentication are either cryptographically authenticated by relying parties (using approved methods) or are obtained directly from a trusted party via a secure authentication protocol. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.] 800 */ 801 LOAAN2, 802 /** 803 * Indicator of medium digital quality or reliability of the digital reliability of verification and validation of the process used to verify the claimed identity of an entity by securely associating an identifier and its authenticator. [Based on ISO 7498-2] 804 805 The degree of confidence in the vetting process used to establish the identity of the individual to whom the credential was issued, and 2) the degree of confidence that the individual who uses the credential is the individual to whom the credential was issued. [OMB M-04-04 E-Authentication Guidance for Federal Agencies] 806 807 Medium authentication level of assurance indicates that the relying party may have high confidence in the asserted identity's validity. Level 3 is appropriate for transactions that need high confidence in the accuracy of the asserted identity. Level 3 provides multifactor remote network authentication. At this level, identity-proofing procedures require verification of identifying materials and information. Authentication is based on proof of possession of a key or password through a cryptographic protocol. Cryptographic strength mechanisms should protect the primary authentication token (a cryptographic key) against compromise by the protocol threats, including eavesdropper, replay, online guessing, verifier impersonation, and man-in-the-middle attacks. A minimum of two authentication factors is required. Three kinds of tokens may be used: 808 809 810 "soft" cryptographic token, which has the key stored on a general-purpose computer, 811 "hard" cryptographic token, which has the key stored on a special hardware device, and 812 "one-time password" device token, which has symmetric key stored on a personal hardware device that is a cryptographic module validated at FIPS 140-2 Level 1 or higher. Validation testing of cryptographic modules and algorithms for conformance to Federal Information Processing Standard (FIPS) 140-2, Security Requirements for Cryptographic Modules, is managed by NIST. 813 814 Authentication requires that the claimant prove control of the token through a secure authentication protocol. The token must be unlocked with a password or biometric representation, or a password must be used in a secure authentication protocol, to establish two-factor authentication. Long-term shared authentication secrets, if used, are never revealed to any party except the claimant and verifiers operated directly by the CSP; however, session (temporary) shared secrets may be provided to independent verifiers by the CSP. Approved cryptographic techniques are used for all operations. Assertions issued about claimants as a result of a successful authentication are either cryptographically authenticated by relying parties (using approved methods) or are obtained directly from a trusted party via a secure authentication protocol. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.] 815 */ 816 LOAAN3, 817 /** 818 * Indicator of high digital quality or reliability of the digital reliability of the verification and validation process used to verify the claimed identity of an entity by securely associating an identifier and its authenticator. [Based on ISO 7498-2] 819 820 The degree of confidence in the vetting process used to establish the identity of the individual to whom the credential was issued, and 2) the degree of confidence that the individual who uses the credential is the individual to whom the credential was issued. [OMB M-04-04 E-Authentication Guidance for Federal Agencies] 821 822 High authentication level of assurance indicates that the relying party may have very high confidence in the asserted identity's validity. Level 4 is for transactions that need very high confidence in the accuracy of the asserted identity. Level 4 provides the highest practical assurance of remote network authentication. Authentication is based on proof of possession of a key through a cryptographic protocol. This level is similar to Level 3 except that only ā??hardā?? cryptographic tokens are allowed, cryptographic module validation requirements are strengthened, and subsequent critical data transfers must be authenticated via a key that is bound to the authentication process. The token should be a hardware cryptographic module validated at FIPS 140-2 Level 2 or higher overall with at least FIPS 140-2 Level 3 physical security. This level requires a physical token, which cannot readily be copied, and operator authentication at Level 2 and higher, and ensures good, two-factor remote authentication. 823 824 Level 4 requires strong cryptographic authentication of all parties and all sensitive data transfers between the parties. Either public key or symmetric key technology may be used. Authentication requires that the claimant prove through a secure authentication protocol that the claimant controls the token. Eavesdropper, replay, online guessing, verifier impersonation, and man-in-the-middle attacks are prevented. Long-term shared authentication secrets, if used, are never revealed to any party except the claimant and verifiers operated directly by the CSP; however, session (temporary) shared secrets may be provided to independent verifiers by the CSP. Strong approved cryptographic techniques are used for all operations. All sensitive data transfers are cryptographically authenticated using keys bound to the authentication process. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.] 825 */ 826 LOAAN4, 827 /** 828 * The value assigned as the indicator of the digital quality or reliability of a defined sequence of messages between a Claimant and a Verifier that demonstrates that the Claimant has possession and control of a valid token to establish his/her identity, and optionally, demonstrates to the Claimant that he or she is communicating with the intended Verifier. [Based on NIST SP 800-63-2] 829 */ 830 LOAAP, 831 /** 832 * Indicator of the low digital quality or reliability of a defined sequence of messages between a Claimant and a Verifier that demonstrates that the Claimant has possession and control of a valid token to establish his/her identity, and optionally, demonstrates to the Claimant that he or she is communicating with the intended Verifier. [Based on NIST SP 800-63-2] 833 834 Low authentication process level of assurance indicates that (1) long-term shared authentication secrets may be revealed to verifiers; and (2) assertions and assertion references require protection from manufacture/modification and reuse attacks. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.] 835 */ 836 LOAAP1, 837 /** 838 * Indicator of the basic digital quality or reliability of a defined sequence of messages between a Claimant and a Verifier that demonstrates that the Claimant has possession and control of a valid token to establish his/her identity, and optionally, demonstrates to the Claimant that he or she is communicating with the intended Verifier. [Based on NIST SP 800-63-2] 839 840 Basic authentication process level of assurance indicates that long-term shared authentication secrets are never revealed to any other party except Credential Service Provider (CSP). Sessions (temporary) shared secrets may be provided to independent verifiers by CSP. Long-term shared authentication secrets, if used, are never revealed to any other party except Verifiers operated by the Credential Service Provider (CSP); however, session (temporary) shared secrets may be provided to independent Verifiers by the CSP. In addition to Level 1 requirements, assertions are resistant to disclosure, redirection, capture and substitution attacks. Approved cryptographic techniques are required. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.] 841 */ 842 LOAAP2, 843 /** 844 * Indicator of the medium digital quality or reliability of a defined sequence of messages between a Claimant and a Verifier that demonstrates that the Claimant has possession and control of a valid token to establish his/her identity, and optionally, demonstrates to the Claimant that he or she is communicating with the intended Verifier. [Based on NIST SP 800-63-2] 845 846 Medium authentication process level of assurance indicates that the token can be unlocked with password, biometric, or uses a secure multi-token authentication protocol to establish two-factor authentication. Long-term shared authentication secrets are never revealed to any party except the Claimant and Credential Service Provider (CSP). 847 848 Authentication requires that the Claimant prove, through a secure authentication protocol, that he or she controls the token. The Claimant unlocks the token with a password or biometric, or uses a secure multi-token authentication protocol to establish two-factor authentication (through proof of possession of a physical or software token in combination with some memorized secret knowledge). Long-term shared authentication secrets, if used, are never revealed to any party except the Claimant and Verifiers operated directly by the CSP; however, session (temporary) shared secrets may be provided to independent Verifiers by the CSP. In addition to Level 2 requirements, assertions are protected against repudiation by the Verifier. 849 */ 850 LOAAP3, 851 /** 852 * Indicator of the high digital quality or reliability of a defined sequence of messages between a Claimant and a Verifier that demonstrates that the Claimant has possession and control of a valid token to establish his/her identity, and optionally, demonstrates to the Claimant that he or she is communicating with the intended Verifier. [Based on NIST SP 800-63-2] 853 854 High authentication process level of assurance indicates all sensitive data transfer are cryptographically authenticated using keys bound to the authentication process. Level 4 requires strong cryptographic authentication of all communicating parties and all sensitive data transfers between the parties. Either public key or symmetric key technology may be used. Authentication requires that the Claimant prove through a secure authentication protocol that he or she controls the token. All protocol threats at Level 3 are required to be prevented at Level 4. Protocols shall also be strongly resistant to man-in-the-middle attacks. Long-term shared authentication secrets, if used, are never revealed to any party except the Claimant and Verifiers operated directly by the CSP; however, session (temporary) shared secrets may be provided to independent Verifiers by the CSP. Approved cryptographic techniques are used for all operations. All sensitive data transfers are cryptographically authenticated using keys bound to the authentication process. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.] 855 */ 856 LOAAP4, 857 /** 858 * The value assigned as the indicator of the high quality or reliability of the statement from a Verifier to a Relying Party (RP) that contains identity information about a Subscriber. Assertions may also contain verified attributes. 859 */ 860 LOAAS, 861 /** 862 * Indicator of the low quality or reliability of the statement from a Verifier to a Relying Party (RP) that contains identity information about a Subscriber. Assertions may also contain verified attributes. 863 864 Assertions and assertion references require protection from modification and reuse attacks. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.] 865 */ 866 LOAAS1, 867 /** 868 * Indicator of the basic quality or reliability of the statement from a Verifier to a Relying Party (RP) that contains identity information about a Subscriber. Assertions may also contain verified attributes. 869 870 Assertions are resistant to disclosure, redirection, capture and substitution attacks. Approved cryptographic techniques are required for all assertion protocols. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.] 871 */ 872 LOAAS2, 873 /** 874 * Indicator of the medium quality or reliability of the statement from a Verifier to a Relying Party (RP) that contains identity information about a Subscriber. Assertions may also contain verified attributes. 875 876 Assertions are protected against repudiation by the verifier. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.] 877 */ 878 LOAAS3, 879 /** 880 * Indicator of the high quality or reliability of the statement from a Verifier to a Relying Party (RP) that contains identity information about a Subscriber. Assertions may also contain verified attributes. 881 882 Strongly resistant to man-in-the-middle attacks. "Bearer" assertions are not used. "Holder-of-key" assertions may be used. RP maintains records of the assertions. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.] 883 */ 884 LOAAS4, 885 /** 886 * Indicator of the digital quality or reliability of the activities performed by the Credential Service Provider (CSP) subsequent to electronic authentication registration, identity proofing and issuance activities to manage and safeguard the integrity of an issued credential and its binding to an identity. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011] 887 */ 888 LOACM, 889 /** 890 * Indicator of the low digital quality or reliability of the activities performed by the Credential Service Provider (CSP) subsequent to electronic authentication registration, identity proofing and issuance activities to manage and safeguard the integrity of an issued credential and its binding to an identity. Little or no confidence that an individual has maintained control over a token that has been entrusted to him or her and that that token has not been compromised. Characteristics include weak identity binding to tokens and plaintext passwords or secrets not transmitted across a network. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011] 891 */ 892 LOACM1, 893 /** 894 * Indicator of the basic digital quality or reliability of the activities performed by the Credential Service Provider (CSP) subsequent to electronic authentication registration, identity proofing and issuance activities to manage and safeguard the integrity of an issued credential and its binding to an identity. Some confidence that an individual has maintained control over a token that has been entrusted to him or her and that that token has not been compromised. Characteristics include: Verification must prove claimant controls the token; token resists online guessing, replay, session hijacking, and eavesdropping attacks; and token is at least weakly resistant to man-in-the middle attacks. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011] 895 */ 896 LOACM2, 897 /** 898 * Indicator of the medium digital quality or reliability of the activities performed by the Credential Service Provider (CSP) subsequent to electronic authentication registration, identity proofing and issuance activities to manage and safeguard the integrity of an issued credential and itā??s binding to an identity. High confidence that an individual has maintained control over a token that has been entrusted to him or her and that that token has not been compromised. Characteristics include: Ownership of token verifiable through security authentication protocol and credential management protects against verifier impersonation attacks. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011] 899 */ 900 LOACM3, 901 /** 902 * Indicator of the high digital quality or reliability of the activities performed by the Credential Service Provider (CSP) subsequent to electronic authentication registration, identity proofing and issuance activities to manage and safeguard the integrity of an issued credential and itā??s binding to an identity. Very high confidence that an individual has maintained control over a token that has been entrusted to him or her and that that token has not been compromised. Characteristics include: Verifier can prove control of token through a secure protocol; credential management supports strong cryptographic authentication of all communication parties. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011] 903 */ 904 LOACM4, 905 /** 906 * Indicator of the quality or reliability in the process of ascertaining that an individual is who he or she claims to be. 907 */ 908 LOAID, 909 /** 910 * Indicator of low digital quality or reliability in the process of ascertaining that an individual is who he or she claims to be. Requires that a continuity of identity be maintained but does not require identity proofing. [Based on Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011] 911 */ 912 LOAID1, 913 /** 914 * Indicator of some digital quality or reliability in the process of ascertaining that that an individual is who he or she claims to be. Requires identity proofing via presentation of identifying material or information. [Based on Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011] 915 */ 916 LOAID2, 917 /** 918 * Indicator of high digital quality or reliability in the process of ascertaining that an individual is who he or she claims to be. Requires identity proofing procedures for verification of identifying materials and information. [Based on Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011] 919 */ 920 LOAID3, 921 /** 922 * Indicator of high digital quality or reliability in the process of ascertaining that an individual is who he or she claims to be. Requires identity proofing procedures for verification of identifying materials and information. [Based on Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011] 923 */ 924 LOAID4, 925 /** 926 * Indicator of the digital quality or reliability in the process of establishing proof of delivery and proof of origin. [Based on ISO 7498-2] 927 */ 928 LOANR, 929 /** 930 * Indicator of low digital quality or reliability in the process of establishing proof of delivery and proof of origin. [Based on ISO 7498-2] 931 */ 932 LOANR1, 933 /** 934 * Indicator of basic digital quality or reliability in the process of establishing proof of delivery and proof of origin. [Based on ISO 7498-2] 935 */ 936 LOANR2, 937 /** 938 * Indicator of medium digital quality or reliability in the process of establishing proof of delivery and proof of origin. [Based on ISO 7498-2] 939 */ 940 LOANR3, 941 /** 942 * Indicator of high digital quality or reliability in the process of establishing proof of delivery and proof of origin. [Based on ISO 7498-2] 943 */ 944 LOANR4, 945 /** 946 * Indicator of the digital quality or reliability of the information exchange between network-connected devices where the information cannot be reliably protected end-to-end by a single organizationā??s security controls. [Based on NIST SP 800-63-2] 947 */ 948 LOARA, 949 /** 950 * Indicator of low digital quality or reliability of the information exchange between network-connected devices where the information cannot be reliably protected end-to-end by a single organizationā??s security controls. [Based on NIST SP 800-63-2] 951 */ 952 LOARA1, 953 /** 954 * Indicator of basic digital quality or reliability of the information exchange between network-connected devices where the information cannot be reliably protected end-to-end by a single organizationā??s security controls. [Based on NIST SP 800-63-2] 955 */ 956 LOARA2, 957 /** 958 * Indicator of medium digital quality or reliability of the information exchange between network-connected devices where the information cannot be reliably protected end-to-end by a single organizationā??s security controls. [Based on NIST SP 800-63-2] 959 */ 960 LOARA3, 961 /** 962 * Indicator of high digital quality or reliability of the information exchange between network-connected devices where the information cannot be reliably protected end-to-end by a single organization's security controls. [Based on NIST SP 800-63-2] 963 */ 964 LOARA4, 965 /** 966 * Indicator of the digital quality or reliability of single and multi-token authentication. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011] 967 */ 968 LOATK, 969 /** 970 * Indicator of the low digital quality or reliability of single and multi-token authentication. Permits the use of any of the token methods of Levels 2, 3, or 4. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011] 971 */ 972 LOATK1, 973 /** 974 * Indicator of the basic digital quality or reliability of single and multi-token authentication. Requires single factor authentication using memorized secret tokens, pre-registered knowledge tokens, look-up secret tokens, out of band tokens, or single factor one-time password devices. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011] 975 */ 976 LOATK2, 977 /** 978 * Indicator of the medium digital quality or reliability of single and multi-token authentication. Requires two authentication factors. Provides multi-factor remote network authentication. Permits multi-factor software cryptographic token. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011] 979 */ 980 LOATK3, 981 /** 982 * Indicator of the high digital quality or reliability of single and multi-token authentication. Requires token that is a hardware cryptographic module validated at validated at Federal Information Processing Standard (FIPS) 140-2 Level 2 or higher overall with at least FIPS 140-2 Level 3 physical security. Level 4 token requirements can be met by using the PIV authentication key of a FIPS 201 compliant Personal Identity Verification (PIV) Card. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011] 983 */ 984 LOATK4, 985 /** 986 * Values for security trust mechanism metadata observation made about a security architecture system component that supports enforcement of security policies. 987 */ 988 TRSTMECOBV, 989 /** 990 * Potential values for observations of severity. 991 */ 992 _SEVERITYOBSERVATION, 993 /** 994 * Indicates the condition may be life-threatening or has the potential to cause permanent injury. 995 */ 996 H, 997 /** 998 * Indicates the condition may result in some adverse consequences but is unlikely to substantially affect the situation of the subject. 999 */ 1000 L, 1001 /** 1002 * Indicates the condition may result in noticable adverse adverse consequences but is unlikely to be life-threatening or cause permanent injury. 1003 */ 1004 M, 1005 /** 1006 * Contains codes for defining the observed, physical position of a subject, such as during an observation, assessment, collection of a specimen, etc. ECG waveforms and vital signs, such as blood pressure, are two examples where a general, observed position typically needs to be noted. 1007 */ 1008 _SUBJECTBODYPOSITION, 1009 /** 1010 * Lying on the left side. 1011 */ 1012 LLD, 1013 /** 1014 * Lying with the front or ventral surface downward; lying face down. 1015 */ 1016 PRN, 1017 /** 1018 * Lying on the right side. 1019 */ 1020 RLD, 1021 /** 1022 * A semi-sitting position in bed with the head of the bed elevated approximately 45 degrees. 1023 */ 1024 SFWL, 1025 /** 1026 * Resting the body on the buttocks, typically with upper torso erect or semi erect. 1027 */ 1028 SIT, 1029 /** 1030 * To be stationary, upright, vertical, on one's legs. 1031 */ 1032 STN, 1033 /** 1034 * supine 1035 */ 1036 SUP, 1037 /** 1038 * Lying on the back, on an inclined plane, typically about 30-45 degrees with head raised and feet lowered. 1039 */ 1040 RTRD, 1041 /** 1042 * Lying on the back, on an inclined plane, typically about 30-45 degrees, with head lowered and feet raised. 1043 */ 1044 TRD, 1045 /** 1046 * Values for observations of verification act results 1047 1048 1049 Examples: Verified, not verified, verified with warning. 1050 */ 1051 _VERIFICATIONOUTCOMEVALUE, 1052 /** 1053 * Definition: Coverage is in effect for healthcare service(s) and/or product(s). 1054 */ 1055 ACT, 1056 /** 1057 * Definition: Coverage is in effect for healthcare service(s) and/or product(s) - Pending Investigation 1058 */ 1059 ACTPEND, 1060 /** 1061 * Definition: Coverage is in effect for healthcare service(s) and/or product(s). 1062 */ 1063 ELG, 1064 /** 1065 * Definition: Coverage is not in effect for healthcare service(s) and/or product(s). 1066 */ 1067 INACT, 1068 /** 1069 * Definition: Coverage is not in effect for healthcare service(s) and/or product(s) - Pending Investigation. 1070 */ 1071 INPNDINV, 1072 /** 1073 * Definition: Coverage is not in effect for healthcare service(s) and/or product(s) - Pending Eligibility Update. 1074 */ 1075 INPNDUPD, 1076 /** 1077 * Definition: Coverage is not in effect for healthcare service(s) and/or product(s). May optionally include reasons for the ineligibility. 1078 */ 1079 NELG, 1080 /** 1081 * AnnotationValue 1082 */ 1083 _ANNOTATIONVALUE, 1084 /** 1085 * Description:Used in a patient care message to value simple clinical (non-lab) observations. 1086 */ 1087 _COMMONCLINICALOBSERVATIONVALUE, 1088 /** 1089 * This domain is established as a parent to a variety of value domains being defined to support the communication of Individual Case Safety Reports to regulatory bodies. Arguably, this aggregation is not taxonomically pure, but the grouping will facilitate the management of these domains. 1090 */ 1091 _INDIVIDUALCASESAFETYREPORTVALUEDOMAINS, 1092 /** 1093 * Indicates the specific observation result which is the reason for the action (prescription, lab test, etc.). E.g. Headache, Ear infection, planned diagnostic image (requiring contrast agent), etc. 1094 */ 1095 _INDICATIONVALUE, 1096 /** 1097 * added to help the parsers 1098 */ 1099 NULL; 1100 public static V3ObservationValue fromCode(String codeString) throws FHIRException { 1101 if (codeString == null || "".equals(codeString)) 1102 return null; 1103 if ("_ActCoverageAssessmentObservationValue".equals(codeString)) 1104 return _ACTCOVERAGEASSESSMENTOBSERVATIONVALUE; 1105 if ("_ActFinancialStatusObservationValue".equals(codeString)) 1106 return _ACTFINANCIALSTATUSOBSERVATIONVALUE; 1107 if ("ASSET".equals(codeString)) 1108 return ASSET; 1109 if ("ANNUITY".equals(codeString)) 1110 return ANNUITY; 1111 if ("PROP".equals(codeString)) 1112 return PROP; 1113 if ("RETACCT".equals(codeString)) 1114 return RETACCT; 1115 if ("TRUST".equals(codeString)) 1116 return TRUST; 1117 if ("INCOME".equals(codeString)) 1118 return INCOME; 1119 if ("CHILD".equals(codeString)) 1120 return CHILD; 1121 if ("DISABL".equals(codeString)) 1122 return DISABL; 1123 if ("INVEST".equals(codeString)) 1124 return INVEST; 1125 if ("PAY".equals(codeString)) 1126 return PAY; 1127 if ("RETIRE".equals(codeString)) 1128 return RETIRE; 1129 if ("SPOUSAL".equals(codeString)) 1130 return SPOUSAL; 1131 if ("SUPPLE".equals(codeString)) 1132 return SUPPLE; 1133 if ("TAX".equals(codeString)) 1134 return TAX; 1135 if ("LIVEXP".equals(codeString)) 1136 return LIVEXP; 1137 if ("CLOTH".equals(codeString)) 1138 return CLOTH; 1139 if ("FOOD".equals(codeString)) 1140 return FOOD; 1141 if ("HEALTH".equals(codeString)) 1142 return HEALTH; 1143 if ("HOUSE".equals(codeString)) 1144 return HOUSE; 1145 if ("LEGAL".equals(codeString)) 1146 return LEGAL; 1147 if ("MORTG".equals(codeString)) 1148 return MORTG; 1149 if ("RENT".equals(codeString)) 1150 return RENT; 1151 if ("SUNDRY".equals(codeString)) 1152 return SUNDRY; 1153 if ("TRANS".equals(codeString)) 1154 return TRANS; 1155 if ("UTIL".equals(codeString)) 1156 return UTIL; 1157 if ("ELSTAT".equals(codeString)) 1158 return ELSTAT; 1159 if ("ADOPT".equals(codeString)) 1160 return ADOPT; 1161 if ("BTHCERT".equals(codeString)) 1162 return BTHCERT; 1163 if ("CCOC".equals(codeString)) 1164 return CCOC; 1165 if ("DRLIC".equals(codeString)) 1166 return DRLIC; 1167 if ("FOSTER".equals(codeString)) 1168 return FOSTER; 1169 if ("MEMBER".equals(codeString)) 1170 return MEMBER; 1171 if ("MIL".equals(codeString)) 1172 return MIL; 1173 if ("MRGCERT".equals(codeString)) 1174 return MRGCERT; 1175 if ("PASSPORT".equals(codeString)) 1176 return PASSPORT; 1177 if ("STUDENRL".equals(codeString)) 1178 return STUDENRL; 1179 if ("HLSTAT".equals(codeString)) 1180 return HLSTAT; 1181 if ("DISABLE".equals(codeString)) 1182 return DISABLE; 1183 if ("DRUG".equals(codeString)) 1184 return DRUG; 1185 if ("IVDRG".equals(codeString)) 1186 return IVDRG; 1187 if ("PGNT".equals(codeString)) 1188 return PGNT; 1189 if ("LIVDEP".equals(codeString)) 1190 return LIVDEP; 1191 if ("RELDEP".equals(codeString)) 1192 return RELDEP; 1193 if ("SPSDEP".equals(codeString)) 1194 return SPSDEP; 1195 if ("URELDEP".equals(codeString)) 1196 return URELDEP; 1197 if ("LIVSIT".equals(codeString)) 1198 return LIVSIT; 1199 if ("ALONE".equals(codeString)) 1200 return ALONE; 1201 if ("DEPCHD".equals(codeString)) 1202 return DEPCHD; 1203 if ("DEPSPS".equals(codeString)) 1204 return DEPSPS; 1205 if ("DEPYGCHD".equals(codeString)) 1206 return DEPYGCHD; 1207 if ("FAM".equals(codeString)) 1208 return FAM; 1209 if ("RELAT".equals(codeString)) 1210 return RELAT; 1211 if ("SPS".equals(codeString)) 1212 return SPS; 1213 if ("UNREL".equals(codeString)) 1214 return UNREL; 1215 if ("SOECSTAT".equals(codeString)) 1216 return SOECSTAT; 1217 if ("ABUSE".equals(codeString)) 1218 return ABUSE; 1219 if ("HMLESS".equals(codeString)) 1220 return HMLESS; 1221 if ("ILGIM".equals(codeString)) 1222 return ILGIM; 1223 if ("INCAR".equals(codeString)) 1224 return INCAR; 1225 if ("PROB".equals(codeString)) 1226 return PROB; 1227 if ("REFUG".equals(codeString)) 1228 return REFUG; 1229 if ("UNEMPL".equals(codeString)) 1230 return UNEMPL; 1231 if ("_AllergyTestValue".equals(codeString)) 1232 return _ALLERGYTESTVALUE; 1233 if ("A0".equals(codeString)) 1234 return A0; 1235 if ("A1".equals(codeString)) 1236 return A1; 1237 if ("A2".equals(codeString)) 1238 return A2; 1239 if ("A3".equals(codeString)) 1240 return A3; 1241 if ("A4".equals(codeString)) 1242 return A4; 1243 if ("_CompositeMeasureScoring".equals(codeString)) 1244 return _COMPOSITEMEASURESCORING; 1245 if ("ALLORNONESCR".equals(codeString)) 1246 return ALLORNONESCR; 1247 if ("LINEARSCR".equals(codeString)) 1248 return LINEARSCR; 1249 if ("OPPORSCR".equals(codeString)) 1250 return OPPORSCR; 1251 if ("WEIGHTSCR".equals(codeString)) 1252 return WEIGHTSCR; 1253 if ("_CoverageLimitObservationValue".equals(codeString)) 1254 return _COVERAGELIMITOBSERVATIONVALUE; 1255 if ("_CoverageLevelObservationValue".equals(codeString)) 1256 return _COVERAGELEVELOBSERVATIONVALUE; 1257 if ("ADC".equals(codeString)) 1258 return ADC; 1259 if ("CHD".equals(codeString)) 1260 return CHD; 1261 if ("DEP".equals(codeString)) 1262 return DEP; 1263 if ("DP".equals(codeString)) 1264 return DP; 1265 if ("ECH".equals(codeString)) 1266 return ECH; 1267 if ("FLY".equals(codeString)) 1268 return FLY; 1269 if ("IND".equals(codeString)) 1270 return IND; 1271 if ("SSP".equals(codeString)) 1272 return SSP; 1273 if ("_CriticalityObservationValue".equals(codeString)) 1274 return _CRITICALITYOBSERVATIONVALUE; 1275 if ("CRITH".equals(codeString)) 1276 return CRITH; 1277 if ("CRITL".equals(codeString)) 1278 return CRITL; 1279 if ("CRITU".equals(codeString)) 1280 return CRITU; 1281 if ("_GeneticObservationValue".equals(codeString)) 1282 return _GENETICOBSERVATIONVALUE; 1283 if ("Homozygote".equals(codeString)) 1284 return HOMOZYGOTE; 1285 if ("_ObservationMeasureScoring".equals(codeString)) 1286 return _OBSERVATIONMEASURESCORING; 1287 if ("COHORT".equals(codeString)) 1288 return COHORT; 1289 if ("CONTVAR".equals(codeString)) 1290 return CONTVAR; 1291 if ("PROPOR".equals(codeString)) 1292 return PROPOR; 1293 if ("RATIO".equals(codeString)) 1294 return RATIO; 1295 if ("_ObservationMeasureType".equals(codeString)) 1296 return _OBSERVATIONMEASURETYPE; 1297 if ("COMPOSITE".equals(codeString)) 1298 return COMPOSITE; 1299 if ("EFFICIENCY".equals(codeString)) 1300 return EFFICIENCY; 1301 if ("EXPERIENCE".equals(codeString)) 1302 return EXPERIENCE; 1303 if ("OUTCOME".equals(codeString)) 1304 return OUTCOME; 1305 if ("PROCESS".equals(codeString)) 1306 return PROCESS; 1307 if ("RESOURCE".equals(codeString)) 1308 return RESOURCE; 1309 if ("STRUCTURE".equals(codeString)) 1310 return STRUCTURE; 1311 if ("_ObservationPopulationInclusion".equals(codeString)) 1312 return _OBSERVATIONPOPULATIONINCLUSION; 1313 if ("DENEX".equals(codeString)) 1314 return DENEX; 1315 if ("DENEXCEP".equals(codeString)) 1316 return DENEXCEP; 1317 if ("DENOM".equals(codeString)) 1318 return DENOM; 1319 if ("IP".equals(codeString)) 1320 return IP; 1321 if ("IPP".equals(codeString)) 1322 return IPP; 1323 if ("MSRPOPL".equals(codeString)) 1324 return MSRPOPL; 1325 if ("NUMER".equals(codeString)) 1326 return NUMER; 1327 if ("NUMEX".equals(codeString)) 1328 return NUMEX; 1329 if ("_PartialCompletionScale".equals(codeString)) 1330 return _PARTIALCOMPLETIONSCALE; 1331 if ("G".equals(codeString)) 1332 return G; 1333 if ("LE".equals(codeString)) 1334 return LE; 1335 if ("ME".equals(codeString)) 1336 return ME; 1337 if ("MI".equals(codeString)) 1338 return MI; 1339 if ("N".equals(codeString)) 1340 return N; 1341 if ("S".equals(codeString)) 1342 return S; 1343 if ("_SecurityObservationValue".equals(codeString)) 1344 return _SECURITYOBSERVATIONVALUE; 1345 if ("_SECINTOBV".equals(codeString)) 1346 return _SECINTOBV; 1347 if ("_SECALTINTOBV".equals(codeString)) 1348 return _SECALTINTOBV; 1349 if ("ABSTRED".equals(codeString)) 1350 return ABSTRED; 1351 if ("AGGRED".equals(codeString)) 1352 return AGGRED; 1353 if ("ANONYED".equals(codeString)) 1354 return ANONYED; 1355 if ("MAPPED".equals(codeString)) 1356 return MAPPED; 1357 if ("MASKED".equals(codeString)) 1358 return MASKED; 1359 if ("PSEUDED".equals(codeString)) 1360 return PSEUDED; 1361 if ("REDACTED".equals(codeString)) 1362 return REDACTED; 1363 if ("SUBSETTED".equals(codeString)) 1364 return SUBSETTED; 1365 if ("SYNTAC".equals(codeString)) 1366 return SYNTAC; 1367 if ("TRSLT".equals(codeString)) 1368 return TRSLT; 1369 if ("VERSIONED".equals(codeString)) 1370 return VERSIONED; 1371 if ("_SECDATINTOBV".equals(codeString)) 1372 return _SECDATINTOBV; 1373 if ("CRYTOHASH".equals(codeString)) 1374 return CRYTOHASH; 1375 if ("DIGSIG".equals(codeString)) 1376 return DIGSIG; 1377 if ("_SECINTCONOBV".equals(codeString)) 1378 return _SECINTCONOBV; 1379 if ("HRELIABLE".equals(codeString)) 1380 return HRELIABLE; 1381 if ("RELIABLE".equals(codeString)) 1382 return RELIABLE; 1383 if ("UNCERTREL".equals(codeString)) 1384 return UNCERTREL; 1385 if ("UNRELIABLE".equals(codeString)) 1386 return UNRELIABLE; 1387 if ("_SECINTPRVOBV".equals(codeString)) 1388 return _SECINTPRVOBV; 1389 if ("_SECINTPRVABOBV".equals(codeString)) 1390 return _SECINTPRVABOBV; 1391 if ("CLINAST".equals(codeString)) 1392 return CLINAST; 1393 if ("DEVAST".equals(codeString)) 1394 return DEVAST; 1395 if ("HCPAST".equals(codeString)) 1396 return HCPAST; 1397 if ("PACQAST".equals(codeString)) 1398 return PACQAST; 1399 if ("PATAST".equals(codeString)) 1400 return PATAST; 1401 if ("PAYAST".equals(codeString)) 1402 return PAYAST; 1403 if ("PROAST".equals(codeString)) 1404 return PROAST; 1405 if ("SDMAST".equals(codeString)) 1406 return SDMAST; 1407 if ("_SECINTPRVRBOBV".equals(codeString)) 1408 return _SECINTPRVRBOBV; 1409 if ("CLINRPT".equals(codeString)) 1410 return CLINRPT; 1411 if ("DEVRPT".equals(codeString)) 1412 return DEVRPT; 1413 if ("HCPRPT".equals(codeString)) 1414 return HCPRPT; 1415 if ("PACQRPT".equals(codeString)) 1416 return PACQRPT; 1417 if ("PATRPT".equals(codeString)) 1418 return PATRPT; 1419 if ("PAYRPT".equals(codeString)) 1420 return PAYRPT; 1421 if ("PRORPT".equals(codeString)) 1422 return PRORPT; 1423 if ("SDMRPT".equals(codeString)) 1424 return SDMRPT; 1425 if ("SECTRSTOBV".equals(codeString)) 1426 return SECTRSTOBV; 1427 if ("TRSTACCRDOBV".equals(codeString)) 1428 return TRSTACCRDOBV; 1429 if ("TRSTAGREOBV".equals(codeString)) 1430 return TRSTAGREOBV; 1431 if ("TRSTCERTOBV".equals(codeString)) 1432 return TRSTCERTOBV; 1433 if ("TRSTLOAOBV".equals(codeString)) 1434 return TRSTLOAOBV; 1435 if ("LOAAN".equals(codeString)) 1436 return LOAAN; 1437 if ("LOAAN1".equals(codeString)) 1438 return LOAAN1; 1439 if ("LOAAN2".equals(codeString)) 1440 return LOAAN2; 1441 if ("LOAAN3".equals(codeString)) 1442 return LOAAN3; 1443 if ("LOAAN4".equals(codeString)) 1444 return LOAAN4; 1445 if ("LOAAP".equals(codeString)) 1446 return LOAAP; 1447 if ("LOAAP1".equals(codeString)) 1448 return LOAAP1; 1449 if ("LOAAP2".equals(codeString)) 1450 return LOAAP2; 1451 if ("LOAAP3".equals(codeString)) 1452 return LOAAP3; 1453 if ("LOAAP4".equals(codeString)) 1454 return LOAAP4; 1455 if ("LOAAS".equals(codeString)) 1456 return LOAAS; 1457 if ("LOAAS1".equals(codeString)) 1458 return LOAAS1; 1459 if ("LOAAS2".equals(codeString)) 1460 return LOAAS2; 1461 if ("LOAAS3".equals(codeString)) 1462 return LOAAS3; 1463 if ("LOAAS4".equals(codeString)) 1464 return LOAAS4; 1465 if ("LOACM".equals(codeString)) 1466 return LOACM; 1467 if ("LOACM1".equals(codeString)) 1468 return LOACM1; 1469 if ("LOACM2".equals(codeString)) 1470 return LOACM2; 1471 if ("LOACM3".equals(codeString)) 1472 return LOACM3; 1473 if ("LOACM4".equals(codeString)) 1474 return LOACM4; 1475 if ("LOAID".equals(codeString)) 1476 return LOAID; 1477 if ("LOAID1".equals(codeString)) 1478 return LOAID1; 1479 if ("LOAID2".equals(codeString)) 1480 return LOAID2; 1481 if ("LOAID3".equals(codeString)) 1482 return LOAID3; 1483 if ("LOAID4".equals(codeString)) 1484 return LOAID4; 1485 if ("LOANR".equals(codeString)) 1486 return LOANR; 1487 if ("LOANR1".equals(codeString)) 1488 return LOANR1; 1489 if ("LOANR2".equals(codeString)) 1490 return LOANR2; 1491 if ("LOANR3".equals(codeString)) 1492 return LOANR3; 1493 if ("LOANR4".equals(codeString)) 1494 return LOANR4; 1495 if ("LOARA".equals(codeString)) 1496 return LOARA; 1497 if ("LOARA1".equals(codeString)) 1498 return LOARA1; 1499 if ("LOARA2".equals(codeString)) 1500 return LOARA2; 1501 if ("LOARA3".equals(codeString)) 1502 return LOARA3; 1503 if ("LOARA4".equals(codeString)) 1504 return LOARA4; 1505 if ("LOATK".equals(codeString)) 1506 return LOATK; 1507 if ("LOATK1".equals(codeString)) 1508 return LOATK1; 1509 if ("LOATK2".equals(codeString)) 1510 return LOATK2; 1511 if ("LOATK3".equals(codeString)) 1512 return LOATK3; 1513 if ("LOATK4".equals(codeString)) 1514 return LOATK4; 1515 if ("TRSTMECOBV".equals(codeString)) 1516 return TRSTMECOBV; 1517 if ("_SeverityObservation".equals(codeString)) 1518 return _SEVERITYOBSERVATION; 1519 if ("H".equals(codeString)) 1520 return H; 1521 if ("L".equals(codeString)) 1522 return L; 1523 if ("M".equals(codeString)) 1524 return M; 1525 if ("_SubjectBodyPosition".equals(codeString)) 1526 return _SUBJECTBODYPOSITION; 1527 if ("LLD".equals(codeString)) 1528 return LLD; 1529 if ("PRN".equals(codeString)) 1530 return PRN; 1531 if ("RLD".equals(codeString)) 1532 return RLD; 1533 if ("SFWL".equals(codeString)) 1534 return SFWL; 1535 if ("SIT".equals(codeString)) 1536 return SIT; 1537 if ("STN".equals(codeString)) 1538 return STN; 1539 if ("SUP".equals(codeString)) 1540 return SUP; 1541 if ("RTRD".equals(codeString)) 1542 return RTRD; 1543 if ("TRD".equals(codeString)) 1544 return TRD; 1545 if ("_VerificationOutcomeValue".equals(codeString)) 1546 return _VERIFICATIONOUTCOMEVALUE; 1547 if ("ACT".equals(codeString)) 1548 return ACT; 1549 if ("ACTPEND".equals(codeString)) 1550 return ACTPEND; 1551 if ("ELG".equals(codeString)) 1552 return ELG; 1553 if ("INACT".equals(codeString)) 1554 return INACT; 1555 if ("INPNDINV".equals(codeString)) 1556 return INPNDINV; 1557 if ("INPNDUPD".equals(codeString)) 1558 return INPNDUPD; 1559 if ("NELG".equals(codeString)) 1560 return NELG; 1561 if ("_AnnotationValue".equals(codeString)) 1562 return _ANNOTATIONVALUE; 1563 if ("_CommonClinicalObservationValue".equals(codeString)) 1564 return _COMMONCLINICALOBSERVATIONVALUE; 1565 if ("_IndividualCaseSafetyReportValueDomains".equals(codeString)) 1566 return _INDIVIDUALCASESAFETYREPORTVALUEDOMAINS; 1567 if ("_IndicationValue".equals(codeString)) 1568 return _INDICATIONVALUE; 1569 throw new FHIRException("Unknown V3ObservationValue code '"+codeString+"'"); 1570 } 1571 public String toCode() { 1572 switch (this) { 1573 case _ACTCOVERAGEASSESSMENTOBSERVATIONVALUE: return "_ActCoverageAssessmentObservationValue"; 1574 case _ACTFINANCIALSTATUSOBSERVATIONVALUE: return "_ActFinancialStatusObservationValue"; 1575 case ASSET: return "ASSET"; 1576 case ANNUITY: return "ANNUITY"; 1577 case PROP: return "PROP"; 1578 case RETACCT: return "RETACCT"; 1579 case TRUST: return "TRUST"; 1580 case INCOME: return "INCOME"; 1581 case CHILD: return "CHILD"; 1582 case DISABL: return "DISABL"; 1583 case INVEST: return "INVEST"; 1584 case PAY: return "PAY"; 1585 case RETIRE: return "RETIRE"; 1586 case SPOUSAL: return "SPOUSAL"; 1587 case SUPPLE: return "SUPPLE"; 1588 case TAX: return "TAX"; 1589 case LIVEXP: return "LIVEXP"; 1590 case CLOTH: return "CLOTH"; 1591 case FOOD: return "FOOD"; 1592 case HEALTH: return "HEALTH"; 1593 case HOUSE: return "HOUSE"; 1594 case LEGAL: return "LEGAL"; 1595 case MORTG: return "MORTG"; 1596 case RENT: return "RENT"; 1597 case SUNDRY: return "SUNDRY"; 1598 case TRANS: return "TRANS"; 1599 case UTIL: return "UTIL"; 1600 case ELSTAT: return "ELSTAT"; 1601 case ADOPT: return "ADOPT"; 1602 case BTHCERT: return "BTHCERT"; 1603 case CCOC: return "CCOC"; 1604 case DRLIC: return "DRLIC"; 1605 case FOSTER: return "FOSTER"; 1606 case MEMBER: return "MEMBER"; 1607 case MIL: return "MIL"; 1608 case MRGCERT: return "MRGCERT"; 1609 case PASSPORT: return "PASSPORT"; 1610 case STUDENRL: return "STUDENRL"; 1611 case HLSTAT: return "HLSTAT"; 1612 case DISABLE: return "DISABLE"; 1613 case DRUG: return "DRUG"; 1614 case IVDRG: return "IVDRG"; 1615 case PGNT: return "PGNT"; 1616 case LIVDEP: return "LIVDEP"; 1617 case RELDEP: return "RELDEP"; 1618 case SPSDEP: return "SPSDEP"; 1619 case URELDEP: return "URELDEP"; 1620 case LIVSIT: return "LIVSIT"; 1621 case ALONE: return "ALONE"; 1622 case DEPCHD: return "DEPCHD"; 1623 case DEPSPS: return "DEPSPS"; 1624 case DEPYGCHD: return "DEPYGCHD"; 1625 case FAM: return "FAM"; 1626 case RELAT: return "RELAT"; 1627 case SPS: return "SPS"; 1628 case UNREL: return "UNREL"; 1629 case SOECSTAT: return "SOECSTAT"; 1630 case ABUSE: return "ABUSE"; 1631 case HMLESS: return "HMLESS"; 1632 case ILGIM: return "ILGIM"; 1633 case INCAR: return "INCAR"; 1634 case PROB: return "PROB"; 1635 case REFUG: return "REFUG"; 1636 case UNEMPL: return "UNEMPL"; 1637 case _ALLERGYTESTVALUE: return "_AllergyTestValue"; 1638 case A0: return "A0"; 1639 case A1: return "A1"; 1640 case A2: return "A2"; 1641 case A3: return "A3"; 1642 case A4: return "A4"; 1643 case _COMPOSITEMEASURESCORING: return "_CompositeMeasureScoring"; 1644 case ALLORNONESCR: return "ALLORNONESCR"; 1645 case LINEARSCR: return "LINEARSCR"; 1646 case OPPORSCR: return "OPPORSCR"; 1647 case WEIGHTSCR: return "WEIGHTSCR"; 1648 case _COVERAGELIMITOBSERVATIONVALUE: return "_CoverageLimitObservationValue"; 1649 case _COVERAGELEVELOBSERVATIONVALUE: return "_CoverageLevelObservationValue"; 1650 case ADC: return "ADC"; 1651 case CHD: return "CHD"; 1652 case DEP: return "DEP"; 1653 case DP: return "DP"; 1654 case ECH: return "ECH"; 1655 case FLY: return "FLY"; 1656 case IND: return "IND"; 1657 case SSP: return "SSP"; 1658 case _CRITICALITYOBSERVATIONVALUE: return "_CriticalityObservationValue"; 1659 case CRITH: return "CRITH"; 1660 case CRITL: return "CRITL"; 1661 case CRITU: return "CRITU"; 1662 case _GENETICOBSERVATIONVALUE: return "_GeneticObservationValue"; 1663 case HOMOZYGOTE: return "Homozygote"; 1664 case _OBSERVATIONMEASURESCORING: return "_ObservationMeasureScoring"; 1665 case COHORT: return "COHORT"; 1666 case CONTVAR: return "CONTVAR"; 1667 case PROPOR: return "PROPOR"; 1668 case RATIO: return "RATIO"; 1669 case _OBSERVATIONMEASURETYPE: return "_ObservationMeasureType"; 1670 case COMPOSITE: return "COMPOSITE"; 1671 case EFFICIENCY: return "EFFICIENCY"; 1672 case EXPERIENCE: return "EXPERIENCE"; 1673 case OUTCOME: return "OUTCOME"; 1674 case PROCESS: return "PROCESS"; 1675 case RESOURCE: return "RESOURCE"; 1676 case STRUCTURE: return "STRUCTURE"; 1677 case _OBSERVATIONPOPULATIONINCLUSION: return "_ObservationPopulationInclusion"; 1678 case DENEX: return "DENEX"; 1679 case DENEXCEP: return "DENEXCEP"; 1680 case DENOM: return "DENOM"; 1681 case IP: return "IP"; 1682 case IPP: return "IPP"; 1683 case MSRPOPL: return "MSRPOPL"; 1684 case NUMER: return "NUMER"; 1685 case NUMEX: return "NUMEX"; 1686 case _PARTIALCOMPLETIONSCALE: return "_PartialCompletionScale"; 1687 case G: return "G"; 1688 case LE: return "LE"; 1689 case ME: return "ME"; 1690 case MI: return "MI"; 1691 case N: return "N"; 1692 case S: return "S"; 1693 case _SECURITYOBSERVATIONVALUE: return "_SecurityObservationValue"; 1694 case _SECINTOBV: return "_SECINTOBV"; 1695 case _SECALTINTOBV: return "_SECALTINTOBV"; 1696 case ABSTRED: return "ABSTRED"; 1697 case AGGRED: return "AGGRED"; 1698 case ANONYED: return "ANONYED"; 1699 case MAPPED: return "MAPPED"; 1700 case MASKED: return "MASKED"; 1701 case PSEUDED: return "PSEUDED"; 1702 case REDACTED: return "REDACTED"; 1703 case SUBSETTED: return "SUBSETTED"; 1704 case SYNTAC: return "SYNTAC"; 1705 case TRSLT: return "TRSLT"; 1706 case VERSIONED: return "VERSIONED"; 1707 case _SECDATINTOBV: return "_SECDATINTOBV"; 1708 case CRYTOHASH: return "CRYTOHASH"; 1709 case DIGSIG: return "DIGSIG"; 1710 case _SECINTCONOBV: return "_SECINTCONOBV"; 1711 case HRELIABLE: return "HRELIABLE"; 1712 case RELIABLE: return "RELIABLE"; 1713 case UNCERTREL: return "UNCERTREL"; 1714 case UNRELIABLE: return "UNRELIABLE"; 1715 case _SECINTPRVOBV: return "_SECINTPRVOBV"; 1716 case _SECINTPRVABOBV: return "_SECINTPRVABOBV"; 1717 case CLINAST: return "CLINAST"; 1718 case DEVAST: return "DEVAST"; 1719 case HCPAST: return "HCPAST"; 1720 case PACQAST: return "PACQAST"; 1721 case PATAST: return "PATAST"; 1722 case PAYAST: return "PAYAST"; 1723 case PROAST: return "PROAST"; 1724 case SDMAST: return "SDMAST"; 1725 case _SECINTPRVRBOBV: return "_SECINTPRVRBOBV"; 1726 case CLINRPT: return "CLINRPT"; 1727 case DEVRPT: return "DEVRPT"; 1728 case HCPRPT: return "HCPRPT"; 1729 case PACQRPT: return "PACQRPT"; 1730 case PATRPT: return "PATRPT"; 1731 case PAYRPT: return "PAYRPT"; 1732 case PRORPT: return "PRORPT"; 1733 case SDMRPT: return "SDMRPT"; 1734 case SECTRSTOBV: return "SECTRSTOBV"; 1735 case TRSTACCRDOBV: return "TRSTACCRDOBV"; 1736 case TRSTAGREOBV: return "TRSTAGREOBV"; 1737 case TRSTCERTOBV: return "TRSTCERTOBV"; 1738 case TRSTLOAOBV: return "TRSTLOAOBV"; 1739 case LOAAN: return "LOAAN"; 1740 case LOAAN1: return "LOAAN1"; 1741 case LOAAN2: return "LOAAN2"; 1742 case LOAAN3: return "LOAAN3"; 1743 case LOAAN4: return "LOAAN4"; 1744 case LOAAP: return "LOAAP"; 1745 case LOAAP1: return "LOAAP1"; 1746 case LOAAP2: return "LOAAP2"; 1747 case LOAAP3: return "LOAAP3"; 1748 case LOAAP4: return "LOAAP4"; 1749 case LOAAS: return "LOAAS"; 1750 case LOAAS1: return "LOAAS1"; 1751 case LOAAS2: return "LOAAS2"; 1752 case LOAAS3: return "LOAAS3"; 1753 case LOAAS4: return "LOAAS4"; 1754 case LOACM: return "LOACM"; 1755 case LOACM1: return "LOACM1"; 1756 case LOACM2: return "LOACM2"; 1757 case LOACM3: return "LOACM3"; 1758 case LOACM4: return "LOACM4"; 1759 case LOAID: return "LOAID"; 1760 case LOAID1: return "LOAID1"; 1761 case LOAID2: return "LOAID2"; 1762 case LOAID3: return "LOAID3"; 1763 case LOAID4: return "LOAID4"; 1764 case LOANR: return "LOANR"; 1765 case LOANR1: return "LOANR1"; 1766 case LOANR2: return "LOANR2"; 1767 case LOANR3: return "LOANR3"; 1768 case LOANR4: return "LOANR4"; 1769 case LOARA: return "LOARA"; 1770 case LOARA1: return "LOARA1"; 1771 case LOARA2: return "LOARA2"; 1772 case LOARA3: return "LOARA3"; 1773 case LOARA4: return "LOARA4"; 1774 case LOATK: return "LOATK"; 1775 case LOATK1: return "LOATK1"; 1776 case LOATK2: return "LOATK2"; 1777 case LOATK3: return "LOATK3"; 1778 case LOATK4: return "LOATK4"; 1779 case TRSTMECOBV: return "TRSTMECOBV"; 1780 case _SEVERITYOBSERVATION: return "_SeverityObservation"; 1781 case H: return "H"; 1782 case L: return "L"; 1783 case M: return "M"; 1784 case _SUBJECTBODYPOSITION: return "_SubjectBodyPosition"; 1785 case LLD: return "LLD"; 1786 case PRN: return "PRN"; 1787 case RLD: return "RLD"; 1788 case SFWL: return "SFWL"; 1789 case SIT: return "SIT"; 1790 case STN: return "STN"; 1791 case SUP: return "SUP"; 1792 case RTRD: return "RTRD"; 1793 case TRD: return "TRD"; 1794 case _VERIFICATIONOUTCOMEVALUE: return "_VerificationOutcomeValue"; 1795 case ACT: return "ACT"; 1796 case ACTPEND: return "ACTPEND"; 1797 case ELG: return "ELG"; 1798 case INACT: return "INACT"; 1799 case INPNDINV: return "INPNDINV"; 1800 case INPNDUPD: return "INPNDUPD"; 1801 case NELG: return "NELG"; 1802 case _ANNOTATIONVALUE: return "_AnnotationValue"; 1803 case _COMMONCLINICALOBSERVATIONVALUE: return "_CommonClinicalObservationValue"; 1804 case _INDIVIDUALCASESAFETYREPORTVALUEDOMAINS: return "_IndividualCaseSafetyReportValueDomains"; 1805 case _INDICATIONVALUE: return "_IndicationValue"; 1806 case NULL: return null; 1807 default: return "?"; 1808 } 1809 } 1810 public String getSystem() { 1811 return "http://hl7.org/fhir/v3/ObservationValue"; 1812 } 1813 public String getDefinition() { 1814 switch (this) { 1815 case _ACTCOVERAGEASSESSMENTOBSERVATIONVALUE: return "Codes specify the category of observation, evidence, or document used to assess for services, e.g., discharge planning, or to establish eligibility for coverage under a policy or program. The type of evidence is coded as observation values."; 1816 case _ACTFINANCIALSTATUSOBSERVATIONVALUE: return "Code specifying financial indicators used to assess or establish eligibility for coverage under a policy or program; e.g., pay stub; tax or income document; asset document; living expenses."; 1817 case ASSET: return "Codes specifying asset indicators used to assess or establish eligibility for coverage under a policy or program."; 1818 case ANNUITY: return "Indicator of annuity ownership or status as beneficiary."; 1819 case PROP: return "Indicator of real property ownership, e.g., deed or real estate contract."; 1820 case RETACCT: return "Indicator of retirement investment account ownership."; 1821 case TRUST: return "Indicator of status as trust beneficiary."; 1822 case INCOME: return "Code specifying income indicators used to assess or establish eligibility for coverage under a policy or program; e.g., pay or pension check, child support payments received or provided, and taxes paid."; 1823 case CHILD: return "Indicator of child support payments received or provided."; 1824 case DISABL: return "Indicator of disability income replacement payment."; 1825 case INVEST: return "Indicator of investment income, e.g., dividend check, annuity payment; real estate rent, investment divestiture proceeds; trust or endowment check."; 1826 case PAY: return "Indicator of paid employment, e.g., letter of hire, contract, employer letter; copy of pay check or pay stub."; 1827 case RETIRE: return "Indicator of retirement payment, e.g., pension check."; 1828 case SPOUSAL: return "Indicator of spousal or partner support payments received or provided; e.g., alimony payment; support stipulations in a divorce settlement."; 1829 case SUPPLE: return "Indicator of income supplement, e.g., gifting, parental income support; stipend, or grant."; 1830 case TAX: return "Indicator of tax obligation or payment, e.g., statement of taxable income."; 1831 case LIVEXP: return "Codes specifying living expense indicators used to assess or establish eligibility for coverage under a policy or program."; 1832 case CLOTH: return "Indicator of clothing expenses."; 1833 case FOOD: return "Indicator of transportation expenses."; 1834 case HEALTH: return "Indicator of health expenses; including medication costs, health service costs, financial participations, and health coverage premiums."; 1835 case HOUSE: return "Indicator of housing expense, e.g., household appliances, fixtures, furnishings, and maintenance and repairs."; 1836 case LEGAL: return "Indicator of legal expenses."; 1837 case MORTG: return "Indicator of mortgage amount, interest, and payments."; 1838 case RENT: return "Indicator of rental or lease payments."; 1839 case SUNDRY: return "Indicator of transportation expenses."; 1840 case TRANS: return "Indicator of transportation expenses, e.g., vehicle payments, vehicle insurance, vehicle fuel, and vehicle maintenance and repairs."; 1841 case UTIL: return "Indicator of transportation expenses."; 1842 case ELSTAT: return "Code specifying eligibility indicators used to assess or establish eligibility for coverage under a policy or program eligibility status, e.g., certificates of creditable coverage; student enrollment; adoption, marriage or birth certificate."; 1843 case ADOPT: return "Indicator of adoption."; 1844 case BTHCERT: return "Indicator of birth."; 1845 case CCOC: return "Indicator of creditable coverage."; 1846 case DRLIC: return "Indicator of driving status."; 1847 case FOSTER: return "Indicator of foster child status."; 1848 case MEMBER: return "Indicator of status as covered member under a policy or program, e.g., member id card or coverage document."; 1849 case MIL: return "Indicator of military status."; 1850 case MRGCERT: return "Indicator of marriage status."; 1851 case PASSPORT: return "Indicator of citizenship."; 1852 case STUDENRL: return "Indicator of student status."; 1853 case HLSTAT: return "Code specifying non-clinical indicators related to health status used to assess or establish eligibility for coverage under a policy or program, e.g., pregnancy, disability, drug use, mental health issues."; 1854 case DISABLE: return "Indication of disability."; 1855 case DRUG: return "Indication of drug use."; 1856 case IVDRG: return "Indication of IV drug use ."; 1857 case PGNT: return "Non-clinical report of pregnancy."; 1858 case LIVDEP: return "Code specifying observations related to living dependency, such as dependent upon spouse for activities of daily living."; 1859 case RELDEP: return "Continued living in private residence requires functional and health care assistance from one or more relatives."; 1860 case SPSDEP: return "Continued living in private residence requires functional and health care assistance from spouse or life partner."; 1861 case URELDEP: return "Continued living in private residence requires functional and health care assistance from one or more unrelated persons."; 1862 case LIVSIT: return "Code specifying observations related to living situation for a person in a private residence."; 1863 case ALONE: return "Living alone. Maps to PD1-2 Living arrangement (IS) 00742 [A]"; 1864 case DEPCHD: return "Living with one or more dependent children requiring moderate supervision."; 1865 case DEPSPS: return "Living with disabled spouse requiring functional and health care assistance"; 1866 case DEPYGCHD: return "Living with one or more dependent children requiring intensive supervision"; 1867 case FAM: return "Living with family. Maps to PD1-2 Living arrangement (IS) 00742 [F]"; 1868 case RELAT: return "Living with one or more relatives. Maps to PD1-2 Living arrangement (IS) 00742 [R]"; 1869 case SPS: return "Living only with spouse or life partner. Maps to PD1-2 Living arrangement (IS) 00742 [S]"; 1870 case UNREL: return "Living with one or more unrelated persons."; 1871 case SOECSTAT: return "Code specifying observations or indicators related to socio-economic status used to assess to assess for services, e.g., discharge planning, or to establish eligibility for coverage under a policy or program."; 1872 case ABUSE: return "Indication of abuse victim."; 1873 case HMLESS: return "Indication of status as homeless."; 1874 case ILGIM: return "Indication of status as illegal immigrant."; 1875 case INCAR: return "Indication of status as incarcerated."; 1876 case PROB: return "Indication of probation status."; 1877 case REFUG: return "Indication of refugee status."; 1878 case UNEMPL: return "Indication of unemployed status."; 1879 case _ALLERGYTESTVALUE: return "Indicates the result of a particular allergy test. E.g. Negative, Mild, Moderate, Severe"; 1880 case A0: return "Description:Patient exhibits no reaction to the challenge agent."; 1881 case A1: return "Description:Patient exhibits a minimal reaction to the challenge agent."; 1882 case A2: return "Description:Patient exhibits a mild reaction to the challenge agent."; 1883 case A3: return "Description:Patient exhibits moderate reaction to the challenge agent."; 1884 case A4: return "Description:Patient exhibits a severe reaction to the challenge agent."; 1885 case _COMPOSITEMEASURESCORING: return "Observation values that communicate the method used in a quality measure to combine the component measure results included in an composite measure."; 1886 case ALLORNONESCR: return "Code specifying that the measure uses all-or-nothing scoring. All-or-nothing scoring places an individual in the numerator of the composite measure if and only if they are in the numerator of all component measures in which they are in the denominator."; 1887 case LINEARSCR: return "Code specifying that the measure uses linear scoring. Linear scoring computes the fraction of component measures in which the individual appears in the numerator, giving equal weight to each component measure."; 1888 case OPPORSCR: return "Code specifying that the measure uses opportunity-based scoring. In opportunity-based scoring the measure score is determined by combining the denominator and numerator of each component measure to determine an overall composite score."; 1889 case WEIGHTSCR: return "Code specifying that the measure uses weighted scoring. Weighted scoring assigns a factor to each component measure to weight that measure's contribution to the overall score."; 1890 case _COVERAGELIMITOBSERVATIONVALUE: return "Description:Coded observation values for coverage limitations, for e.g., types of claims or types of parties covered under a policy or program."; 1891 case _COVERAGELEVELOBSERVATIONVALUE: return "Description:Coded observation values for types of covered parties under a policy or program based on their personal relationships or employment status."; 1892 case ADC: return "Description:Child over an age as specified by coverage policy or program, e.g., student, differently abled, and income dependent."; 1893 case CHD: return "Description:Dependent biological, adopted, foster child as specified by coverage policy or program."; 1894 case DEP: return "Description:Person requiring functional and/or financial assistance from another person as specified by coverage policy or program."; 1895 case DP: return "Description:Persons registered as a family unit in a domestic partner registry as specified by law and by coverage policy or program."; 1896 case ECH: return "Description:An individual employed by an employer who receive remuneration in wages, salary, commission, tips, piece-rates, or pay-in-kind through the employeraTMs payment system (i.e., not a contractor) as specified by coverage policy or program."; 1897 case FLY: return "Description:As specified by coverage policy or program."; 1898 case IND: return "Description:Person as specified by coverage policy or program."; 1899 case SSP: return "Description:A pair of people of the same gender who live together as a family as specified by coverage policy or program, e.g., Naomi and Ruth from the Book of Ruth; Socrates and Alcibiades"; 1900 case _CRITICALITYOBSERVATIONVALUE: return "A clinical judgment as to the worst case result of a future exposure (including substance administration). When the worst case result is assessed to have a life-threatening or organ system threatening potential, it is considered to be of high criticality."; 1901 case CRITH: return "Worst case result of a future exposure is assessed to be life-threatening or having high potential for organ system failure."; 1902 case CRITL: return "Worst case result of a future exposure is not assessed to be life-threatening or having high potential for organ system failure."; 1903 case CRITU: return "Unable to assess the worst case result of a future exposure."; 1904 case _GENETICOBSERVATIONVALUE: return "Description: The domain contains genetic analysis specific observation values, e.g. Homozygote, Heterozygote, etc."; 1905 case HOMOZYGOTE: return "Description: An individual having different alleles at one or more loci regarding a specific character"; 1906 case _OBSERVATIONMEASURESCORING: return "Observation values used to indicate the type of scoring (e.g. proportion, ratio) used by a health quality measure."; 1907 case COHORT: return "A measure in which either short-term cross-section or long-term longitudinal analysis is performed over a group of subjects defined by a set of common properties or defining characteristics (e.g., Male smokers between the ages of 40 and 50 years, exposure to treatment, exposure duration)."; 1908 case CONTVAR: return "A measure score in which each individual value for the measure can fall anywhere along a continuous scale (e.g., mean time to thrombolytics which aggregates the time in minutes from a case presenting with chest pain to the time of administration of thrombolytics)."; 1909 case PROPOR: return "A score derived by dividing the number of cases that meet a criterion for quality (the numerator) by the number of eligible cases within a given time frame (the denominator) where the numerator cases are a subset of the denominator cases (e.g., percentage of eligible women with a mammogram performed in the last year)."; 1910 case RATIO: return "A score that may have a value of zero or greater that is derived by dividing a count of one type of data by a count of another type of data (e.g., the number of patients with central lines who develop infection divided by the number of central line days)."; 1911 case _OBSERVATIONMEASURETYPE: return "Observation values used to indicate what kind of health quality measure is used."; 1912 case COMPOSITE: return "A measure that is composed from one or more other measures and indicates an overall summary of those measures."; 1913 case EFFICIENCY: return "A measure related to the efficiency of medical treatment."; 1914 case EXPERIENCE: return "A measure related to the level of patient engagement or patient experience of care."; 1915 case OUTCOME: return "A measure that indicates the result of the performance (or non-performance) of a function or process."; 1916 case PROCESS: return "A measure which focuses on a process which leads to a certain outcome, meaning that a scientific basis exists for believing that the process, when executed well, will increase the probability of achieving a desired outcome."; 1917 case RESOURCE: return "A measure related to the extent of use of clinical resources or cost of care."; 1918 case STRUCTURE: return "A measure related to the structure of patient care."; 1919 case _OBSERVATIONPOPULATIONINCLUSION: return "Observation values used to assert various populations that a subject falls into."; 1920 case DENEX: return "Patients who should be removed from the eMeasure population and denominator before determining if numerator criteria are met. Denominator exclusions are used in proportion and ratio measures to help narrow the denominator."; 1921 case DENEXCEP: return "Denominator exceptions are those conditions that should remove a patient, procedure or unit of measurement from the denominator only if the numerator criteria are not met. Denominator exceptions allow for adjustment of the calculated score for those providers with higher risk populations. Denominator exceptions are used only in proportion eMeasures. They are not appropriate for ratio or continuous variable eMeasures. Denominator exceptions allow for the exercise of clinical judgment and should be specifically defined where capturing the information in a structured manner fits the clinical workflow. Generic denominator exception reasons used in proportion eMeasures fall into three general categories:\r\n\n \n Medical reasons\n Patient reasons\n System reasons"; 1922 case DENOM: return "It can be the same as the initial patient population or a subset of the initial patient population to further constrain the population for the purpose of the eMeasure. Different measures within an eMeasure set may have different Denominators. Continuous Variable eMeasures do not have a Denominator, but instead define a Measure Population."; 1923 case IP: return "The initial population refers to all entities to be evaluated by a specific quality measure who share a common set of specified characteristics within a specific measurement set to which a given measure belongs."; 1924 case IPP: return "The initial patient population refers to all patients to be evaluated by a specific quality measure who share a common set of specified characteristics within a specific measurement set to which a given measure belongs. Details often include information based upon specific age groups, diagnoses, diagnostic and procedure codes, and enrollment periods."; 1925 case MSRPOPL: return "Measure population is used only in continuous variable eMeasures. It is a narrative description of the eMeasure population. \n(e.g., all patients seen in the Emergency Department during the measurement period)."; 1926 case NUMER: return "Numerators are used in proportion and ratio eMeasures. In proportion measures the numerator criteria are the processes or outcomes expected for each patient, procedure, or other unit of measurement defined in the denominator. In ratio measures the numerator is related, but not directly derived from the denominator (e.g., a numerator listing the number of central line blood stream infections and a denominator indicating the days per thousand of central line usage in a specific time period)."; 1927 case NUMEX: return "Numerator Exclusions are used only in ratio eMeasures to define instances that should not be included in the numerator data. (e.g., if the number of central line blood stream infections per 1000 catheter days were to exclude infections with a specific bacterium, that bacterium would be listed as a numerator exclusion.)"; 1928 case _PARTIALCOMPLETIONSCALE: return "PartialCompletionScale"; 1929 case G: return "Value for Act.partialCompletionCode attribute that implies 81-99% completion"; 1930 case LE: return "Value for Act.partialCompletionCode attribute that implies 61-80% completion"; 1931 case ME: return "Value for Act.partialCompletionCode attribute that implies 41-60% completion"; 1932 case MI: return "Value for Act.partialCompletionCode attribute that implies 1-20% completion"; 1933 case N: return "Value for Act.partialCompletionCode attribute that implies 0% completion"; 1934 case S: return "Value for Act.partialCompletionCode attribute that implies 21-40% completion"; 1935 case _SECURITYOBSERVATIONVALUE: return "Observation values used to indicate security observation metadata."; 1936 case _SECINTOBV: return "Abstract security observation values used to indicate security integrity metadata.\r\n\n \n Examples: Codes conveying integrity status, integrity confidence, and provenance."; 1937 case _SECALTINTOBV: return "Abstract security metadata observation values used to indicate mechanism used for authorized alteration of an IT resource (data, information object, service, or system capability)"; 1938 case ABSTRED: return "Security metadata observation values used to indicate the use of a more abstract version of the content, e.g., replacing exact value of an age or date field with a range, or remove the left digits of a credit card number or SSN."; 1939 case AGGRED: return "Security metadata observation values used to indicate the use of an algorithmic combination of actual values with the result of an aggregate function, e.g., average, sum, or count in order to limit disclosure of an IT resource (data, information object, service, or system capability) to the minimum necessary."; 1940 case ANONYED: return "Security metadata observation value conveying the alteration integrity of an IT resource (data, information object, service, or system capability) by used to indicate the mechanism by which software systems can strip portions of the resource that could allow the identification of the source of the information or the information subject. No key to relink the data is retained."; 1941 case MAPPED: return "Security metadata observation value used to indicate that the IT resource semantic content has been transformed from one encoding to another.\r\n\n \n Usage Note: \"MAP\" code does not indicate the semantic fidelity of the transformed content.\r\n\n To indicate semantic fidelity for maps of HL7 to other code systems, this security alteration integrity observation may be further specified using an Act valued with Value Set: MapRelationship (2.16.840.1.113883.1.11.11052).\r\n\n Semantic fidelity of the mapped IT Resource may also be indicated using a SecurityIntegrityConfidenceObservation."; 1942 case MASKED: return "Security metadata observation value conveying the alteration integrity of an IT resource (data, information object, service, or system capability) by indicating the mechanism by which software systems can make data unintelligible (that is, as unreadable and unusable by algorithmically transforming plaintext into ciphertext) such that it can only be accessed or used by authorized users. An authorized user may be provided a key to decrypt per license or \"shared secret\".\r\n\n \n Usage Note: \"MASKED\" may be used, per applicable policy, as a flag to indicate to a user or receiver that some portion of an IT resource has been further encrypted, and may be accessed only by an authorized user or receiver to which a decryption key is provided."; 1943 case PSEUDED: return "Security metadata observation value conveying the alteration integrity of an IT resource (data, information object, service, or system capability), by indicating the mechanism by which software systems can strip portions of the resource that could allow the identification of the source of the information or the information subject. Custodian may retain a key to relink data necessary to reidentify the information subject.\r\n\n \n Rationale: Personal data which has been processed to make it impossible to know whose data it is. Used particularly for secondary use of health data. In some cases, it may be possible for authorized individuals to restore the identity of the individual, e.g.,for public health case management. Based on ISO/TS 25237:2008 Health informaticsā??Pseudonymization"; 1944 case REDACTED: return "Security metadata observation value used to indicate the mechanism by which software systems can filter an IT resource (data, information object, service, or system capability) to remove any portion of the resource that is not authorized to be access, used, or disclosed.\r\n\n \n Usage Note: \"REDACTED\" may be used, per applicable policy, as a flag to indicate to a user or receiver that some portion of an IT resource has filtered and not included in the content accessed or received."; 1945 case SUBSETTED: return "Metadata observation used to indicate that some information has been removed from the source object when the view this object contains was constructed because of configuration options when the view was created. The content may not be suitable for use as the basis of a record update\r\n\n \n Usage Note: This is not suitable to be used when information is removed for security reasons - see the code REDACTED for this use."; 1946 case SYNTAC: return "Security metadata observation value used to indicate that the IT resource syntax has been transformed from one syntactical representation to another. \r\n\n \n Usage Note: \"SYNTAC\" code does not indicate the syntactical correctness of the syntactically transformed IT resource."; 1947 case TRSLT: return "Security metadata observation value used to indicate that the IT resource has been translated from one human language to another. \r\n\n \n Usage Note: \"TRSLT\" does not indicate the fidelity of the translation or the languages translated.\r\n\n The fidelity of the IT Resource translation may be indicated using a SecurityIntegrityConfidenceObservation.\r\n\n To indicate languages, use the Value Set:HumanLanguage (2.16.840.1.113883.1.11.11526)"; 1948 case VERSIONED: return "Security metadata observation value conveying the alteration integrity of an IT resource (data, information object, service, or system capability) which indicates that the resource only retains versions of an IT resource for access and use per applicable policy\r\n\n \n Usage Note: When this code is used, expectation is that the system has removed historical versions of the data that falls outside the time period deemed to be the effective time of the applicable version."; 1949 case _SECDATINTOBV: return "Abstract security observation values used to indicate data integrity metadata.\r\n\n \n Examples: Codes conveying the mechanism used to preserve the accuracy and consistency of an IT resource such as a digital signature and a cryptographic hash function."; 1950 case CRYTOHASH: return "Security metadata observation value used to indicate the mechanism by which software systems can establish that data was not modified in transit.\r\n\n \n Rationale: This definition is intended to align with the ISO 22600-2 3.3.19 definition of cryptographic checkvalue: Information which is derived by performing a cryptographic transformation (see cryptography) on the data unit. The derivation of the checkvalue may be performed in one or more steps and is a result of a mathematical function of the key and a data unit. It is usually used to check the integrity of a data unit.\r\n\n \n Examples: \n \r\n\n \n SHA-1\n SHA-2 (Secure Hash Algorithm)"; 1951 case DIGSIG: return "Security metadata observation value used to indicate the mechanism by which software systems use digital signature to establish that data has not been modified. \r\n\n \n Rationale: This definition is intended to align with the ISO 22600-2 3.3.26 definition of digital signature: Data appended to, or a cryptographic transformation (see cryptography) of, a data unit that allows a recipient of the data unit to prove the source and integrity of the data unit and protect against forgery e.g., by the recipient."; 1952 case _SECINTCONOBV: return "Abstract security observation value used to indicate integrity confidence metadata.\r\n\n \n Examples: Codes conveying the level of reliability and trustworthiness of an IT resource."; 1953 case HRELIABLE: return "Security metadata observation value used to indicate that the veracity or trustworthiness of an IT resource (data, information object, service, or system capability) for a specified purpose of use is perceived to be or deemed by policy to be very high."; 1954 case RELIABLE: return "Security metadata observation value used to indicate that the veracity or trustworthiness of an IT resource (data, information object, service, or system capability) for a specified purpose of use is perceived to be or deemed by policy to be adequate."; 1955 case UNCERTREL: return "Security metadata observation value used to indicate that the veracity or trustworthiness of an IT resource (data, information object, service, or system capability) for a specified purpose of use is perceived to be or deemed by policy to be uncertain."; 1956 case UNRELIABLE: return "Security metadata observation value used to indicate that the veracity or trustworthiness of an IT resource (data, information object, service, or system capability) for a specified purpose of use is perceived to be or deemed by policy to be inadequate."; 1957 case _SECINTPRVOBV: return "Abstract security metadata observation value used to indicate the provenance of an IT resource (data, information object, service, or system capability).\r\n\n \n Examples: Codes conveying the provenance metadata about the entity reporting an IT resource."; 1958 case _SECINTPRVABOBV: return "Abstract security provenance metadata observation value used to indicate the entity that asserted an IT resource (data, information object, service, or system capability).\r\n\n \n Examples: Codes conveying the provenance metadata about the entity asserting the resource."; 1959 case CLINAST: return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a clinician."; 1960 case DEVAST: return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a device."; 1961 case HCPAST: return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a healthcare professional."; 1962 case PACQAST: return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a patient acquaintance."; 1963 case PATAST: return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a patient."; 1964 case PAYAST: return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a payer."; 1965 case PROAST: return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a professional."; 1966 case SDMAST: return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was asserted by a substitute decision maker."; 1967 case _SECINTPRVRBOBV: return "Abstract security provenance metadata observation value used to indicate the entity that reported the resource (data, information object, service, or system capability).\r\n\n \n Examples: Codes conveying the provenance metadata about the entity reporting an IT resource."; 1968 case CLINRPT: return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a clinician."; 1969 case DEVRPT: return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a device."; 1970 case HCPRPT: return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a healthcare professional."; 1971 case PACQRPT: return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a patient acquaintance."; 1972 case PATRPT: return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a patient."; 1973 case PAYRPT: return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a payer."; 1974 case PRORPT: return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a professional."; 1975 case SDMRPT: return "Security provenance metadata observation value used to indicate that an IT resource (data, information object, service, or system capability) was reported by a substitute decision maker."; 1976 case SECTRSTOBV: return "Observation value used to indicate aspects of trust applicable to an IT resource (data, information object, service, or system capability)."; 1977 case TRSTACCRDOBV: return "Values for security trust accreditation metadata observation made about the formal declaration by an authority or neutral third party that validates the technical, security, trust, and business practice conformance of Trust Agents to facilitate security, interoperability, and trust among participants within a security domain or trust framework."; 1978 case TRSTAGREOBV: return "Values for security trust agreement metadata observation made about privacy and security requirements with which a security domain must comply. [ISO IEC 10181-1]\n[ISO IEC 10181-1]"; 1979 case TRSTCERTOBV: return "Values for security trust certificate metadata observation made about a set of security-relevant data issued by a security authority or trusted third party, together with security information which is used to provide the integrity and data origin authentication services for an IT resource (data, information object, service, or system capability). [Based on ISO IEC 10181-1]\r\n\n For example, a Certificate Policy (CP), which is a named set of rules that indicates the applicability of a certificate to a particular community and/or class of application with common security requirements. A particular Certificate Policy might indicate the applicability of a type of certificate to the authentication of electronic data interchange transactions for the trading of goods within a given price range. Another example is Cross Certification with Federal Bridge."; 1980 case TRSTLOAOBV: return "Values for security trust assurance metadata observation made about the digital quality or reliability of a trust assertion, activity, capability, information exchange, mechanism, process, or protocol."; 1981 case LOAAN: return "The value assigned as the indicator of the digital quality or reliability of the verification and validation process used to verify the claimed identity of an entity by securely associating an identifier and its authenticator. [Based on ISO 7498-2]\r\n\n For example, the degree of confidence in the vetting process used to establish the identity of the individual to whom the credential was issued, and 2) the degree of confidence that the individual who uses the credential is the individual to whom the credential was issued. [OMB M-04-04 E-Authentication Guidance for Federal Agencies]"; 1982 case LOAAN1: return "Indicator of low digital quality or reliability of the digital reliability of the verification and validation process used to verify the claimed identity of an entity by securely associating an identifier and its authenticator. [Based on ISO 7498-2] \r\n\n The degree of confidence in the vetting process used to establish the identity of the individual to whom the credential was issued, and 2) the degree of confidence that the individual who uses the credential is the individual to whom the credential was issued. [OMB M-04-04 E-Authentication Guidance for Federal Agencies] \r\n\n Low authentication level of assurance indicates that the relying party may have little or no confidence in the asserted identity's validity. Level 1 requires little or no confidence in the asserted identity. No identity proofing is required at this level, but the authentication mechanism should provide some assurance that the same claimant is accessing the protected transaction or data. A wide range of available authentication technologies can be employed and any of the token methods of Levels 2, 3, or 4, including Personal Identification Numbers (PINs), may be used. To be authenticated, the claimant must prove control of the token through a secure authentication protocol. At Level 1, long-term shared authentication secrets may be revealed to verifiers. Assertions issued about claimants as a result of a successful authentication are either cryptographically authenticated by relying parties (using approved methods) or are obtained directly from a trusted party via a secure authentication protocol. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 1983 case LOAAN2: return "Indicator of basic digital quality or reliability of the digital reliability of the verification and validation process used to verify the claimed identity of an entity by securely associating an identifier and its authenticator. [Based on ISO 7498-2] \r\n\n The degree of confidence in the vetting process used to establish the identity of the individual to whom the credential was issued, and 2) the degree of confidence that the individual who uses the credential is the individual to whom the credential was issued. [OMB M-04-04 E-Authentication Guidance for Federal Agencies]\r\n\n Basic authentication level of assurance indicates that the relying party may have some confidence in the asserted identity's validity. Level 2 requires confidence that the asserted identity is accurate. Level 2 provides for single-factor remote network authentication, including identity-proofing requirements for presentation of identifying materials or information. A wide range of available authentication technologies can be employed, including any of the token methods of Levels 3 or 4, as well as passwords. Successful authentication requires that the claimant prove through a secure authentication protocol that the claimant controls the token. Eavesdropper, replay, and online guessing attacks are prevented. \nLong-term shared authentication secrets, if used, are never revealed to any party except the claimant and verifiers operated by the CSP; however, session (temporary) shared secrets may be provided to independent verifiers by the CSP. Approved cryptographic techniques are required. Assertions issued about claimants as a result of a successful authentication are either cryptographically authenticated by relying parties (using approved methods) or are obtained directly from a trusted party via a secure authentication protocol. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 1984 case LOAAN3: return "Indicator of medium digital quality or reliability of the digital reliability of verification and validation of the process used to verify the claimed identity of an entity by securely associating an identifier and its authenticator. [Based on ISO 7498-2] \r\n\n The degree of confidence in the vetting process used to establish the identity of the individual to whom the credential was issued, and 2) the degree of confidence that the individual who uses the credential is the individual to whom the credential was issued. [OMB M-04-04 E-Authentication Guidance for Federal Agencies] \r\n\n Medium authentication level of assurance indicates that the relying party may have high confidence in the asserted identity's validity. Level 3 is appropriate for transactions that need high confidence in the accuracy of the asserted identity. Level 3 provides multifactor remote network authentication. At this level, identity-proofing procedures require verification of identifying materials and information. Authentication is based on proof of possession of a key or password through a cryptographic protocol. Cryptographic strength mechanisms should protect the primary authentication token (a cryptographic key) against compromise by the protocol threats, including eavesdropper, replay, online guessing, verifier impersonation, and man-in-the-middle attacks. A minimum of two authentication factors is required. Three kinds of tokens may be used:\r\n\n \n \"soft\" cryptographic token, which has the key stored on a general-purpose computer, \n \"hard\" cryptographic token, which has the key stored on a special hardware device, and \n \"one-time password\" device token, which has symmetric key stored on a personal hardware device that is a cryptographic module validated at FIPS 140-2 Level 1 or higher. Validation testing of cryptographic modules and algorithms for conformance to Federal Information Processing Standard (FIPS) 140-2, Security Requirements for Cryptographic Modules, is managed by NIST.\n \n Authentication requires that the claimant prove control of the token through a secure authentication protocol. The token must be unlocked with a password or biometric representation, or a password must be used in a secure authentication protocol, to establish two-factor authentication. Long-term shared authentication secrets, if used, are never revealed to any party except the claimant and verifiers operated directly by the CSP; however, session (temporary) shared secrets may be provided to independent verifiers by the CSP. Approved cryptographic techniques are used for all operations. Assertions issued about claimants as a result of a successful authentication are either cryptographically authenticated by relying parties (using approved methods) or are obtained directly from a trusted party via a secure authentication protocol. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 1985 case LOAAN4: return "Indicator of high digital quality or reliability of the digital reliability of the verification and validation process used to verify the claimed identity of an entity by securely associating an identifier and its authenticator. [Based on ISO 7498-2] \r\n\n The degree of confidence in the vetting process used to establish the identity of the individual to whom the credential was issued, and 2) the degree of confidence that the individual who uses the credential is the individual to whom the credential was issued. [OMB M-04-04 E-Authentication Guidance for Federal Agencies]\r\n\n High authentication level of assurance indicates that the relying party may have very high confidence in the asserted identity's validity. Level 4 is for transactions that need very high confidence in the accuracy of the asserted identity. Level 4 provides the highest practical assurance of remote network authentication. Authentication is based on proof of possession of a key through a cryptographic protocol. This level is similar to Level 3 except that only ā??hardā?? cryptographic tokens are allowed, cryptographic module validation requirements are strengthened, and subsequent critical data transfers must be authenticated via a key that is bound to the authentication process. The token should be a hardware cryptographic module validated at FIPS 140-2 Level 2 or higher overall with at least FIPS 140-2 Level 3 physical security. This level requires a physical token, which cannot readily be copied, and operator authentication at Level 2 and higher, and ensures good, two-factor remote authentication.\r\n\n Level 4 requires strong cryptographic authentication of all parties and all sensitive data transfers between the parties. Either public key or symmetric key technology may be used. Authentication requires that the claimant prove through a secure authentication protocol that the claimant controls the token. Eavesdropper, replay, online guessing, verifier impersonation, and man-in-the-middle attacks are prevented. Long-term shared authentication secrets, if used, are never revealed to any party except the claimant and verifiers operated directly by the CSP; however, session (temporary) shared secrets may be provided to independent verifiers by the CSP. Strong approved cryptographic techniques are used for all operations. All sensitive data transfers are cryptographically authenticated using keys bound to the authentication process. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 1986 case LOAAP: return "The value assigned as the indicator of the digital quality or reliability of a defined sequence of messages between a Claimant and a Verifier that demonstrates that the Claimant has possession and control of a valid token to establish his/her identity, and optionally, demonstrates to the Claimant that he or she is communicating with the intended Verifier. [Based on NIST SP 800-63-2]"; 1987 case LOAAP1: return "Indicator of the low digital quality or reliability of a defined sequence of messages between a Claimant and a Verifier that demonstrates that the Claimant has possession and control of a valid token to establish his/her identity, and optionally, demonstrates to the Claimant that he or she is communicating with the intended Verifier. [Based on NIST SP 800-63-2]\r\n\n Low authentication process level of assurance indicates that (1) long-term shared authentication secrets may be revealed to verifiers; and (2) assertions and assertion references require protection from manufacture/modification and reuse attacks. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 1988 case LOAAP2: return "Indicator of the basic digital quality or reliability of a defined sequence of messages between a Claimant and a Verifier that demonstrates that the Claimant has possession and control of a valid token to establish his/her identity, and optionally, demonstrates to the Claimant that he or she is communicating with the intended Verifier. [Based on NIST SP 800-63-2]\r\n\n Basic authentication process level of assurance indicates that long-term shared authentication secrets are never revealed to any other party except Credential Service Provider (CSP). Sessions (temporary) shared secrets may be provided to independent verifiers by CSP. Long-term shared authentication secrets, if used, are never revealed to any other party except Verifiers operated by the Credential Service Provider (CSP); however, session (temporary) shared secrets may be provided to independent Verifiers by the CSP. In addition to Level 1 requirements, assertions are resistant to disclosure, redirection, capture and substitution attacks. Approved cryptographic techniques are required. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 1989 case LOAAP3: return "Indicator of the medium digital quality or reliability of a defined sequence of messages between a Claimant and a Verifier that demonstrates that the Claimant has possession and control of a valid token to establish his/her identity, and optionally, demonstrates to the Claimant that he or she is communicating with the intended Verifier. [Based on NIST SP 800-63-2]\r\n\n Medium authentication process level of assurance indicates that the token can be unlocked with password, biometric, or uses a secure multi-token authentication protocol to establish two-factor authentication. Long-term shared authentication secrets are never revealed to any party except the Claimant and Credential Service Provider (CSP).\r\n\n Authentication requires that the Claimant prove, through a secure authentication protocol, that he or she controls the token. The Claimant unlocks the token with a password or biometric, or uses a secure multi-token authentication protocol to establish two-factor authentication (through proof of possession of a physical or software token in combination with some memorized secret knowledge). Long-term shared authentication secrets, if used, are never revealed to any party except the Claimant and Verifiers operated directly by the CSP; however, session (temporary) shared secrets may be provided to independent Verifiers by the CSP. In addition to Level 2 requirements, assertions are protected against repudiation by the Verifier."; 1990 case LOAAP4: return "Indicator of the high digital quality or reliability of a defined sequence of messages between a Claimant and a Verifier that demonstrates that the Claimant has possession and control of a valid token to establish his/her identity, and optionally, demonstrates to the Claimant that he or she is communicating with the intended Verifier. [Based on NIST SP 800-63-2]\r\n\n High authentication process level of assurance indicates all sensitive data transfer are cryptographically authenticated using keys bound to the authentication process. Level 4 requires strong cryptographic authentication of all communicating parties and all sensitive data transfers between the parties. Either public key or symmetric key technology may be used. Authentication requires that the Claimant prove through a secure authentication protocol that he or she controls the token. All protocol threats at Level 3 are required to be prevented at Level 4. Protocols shall also be strongly resistant to man-in-the-middle attacks. Long-term shared authentication secrets, if used, are never revealed to any party except the Claimant and Verifiers operated directly by the CSP; however, session (temporary) shared secrets may be provided to independent Verifiers by the CSP. Approved cryptographic techniques are used for all operations. All sensitive data transfers are cryptographically authenticated using keys bound to the authentication process. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 1991 case LOAAS: return "The value assigned as the indicator of the high quality or reliability of the statement from a Verifier to a Relying Party (RP) that contains identity information about a Subscriber. Assertions may also contain verified attributes."; 1992 case LOAAS1: return "Indicator of the low quality or reliability of the statement from a Verifier to a Relying Party (RP) that contains identity information about a Subscriber. Assertions may also contain verified attributes.\r\n\n Assertions and assertion references require protection from modification and reuse attacks. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 1993 case LOAAS2: return "Indicator of the basic quality or reliability of the statement from a Verifier to a Relying Party (RP) that contains identity information about a Subscriber. Assertions may also contain verified attributes.\r\n\n Assertions are resistant to disclosure, redirection, capture and substitution attacks. Approved cryptographic techniques are required for all assertion protocols. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 1994 case LOAAS3: return "Indicator of the medium quality or reliability of the statement from a Verifier to a Relying Party (RP) that contains identity information about a Subscriber. Assertions may also contain verified attributes.\r\n\n Assertions are protected against repudiation by the verifier. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 1995 case LOAAS4: return "Indicator of the high quality or reliability of the statement from a Verifier to a Relying Party (RP) that contains identity information about a Subscriber. Assertions may also contain verified attributes.\r\n\n Strongly resistant to man-in-the-middle attacks. \"Bearer\" assertions are not used. \"Holder-of-key\" assertions may be used. RP maintains records of the assertions. [Summary of the technical requirements specified in NIST SP 800-63 for the four levels of assurance defined by the December 2003, the Office of Management and Budget (OMB) issued Memorandum M-04-04, E-Authentication Guidance for Federal Agencies.]"; 1996 case LOACM: return "Indicator of the digital quality or reliability of the activities performed by the Credential Service Provider (CSP) subsequent to electronic authentication registration, identity proofing and issuance activities to manage and safeguard the integrity of an issued credential and its binding to an identity. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 1997 case LOACM1: return "Indicator of the low digital quality or reliability of the activities performed by the Credential Service Provider (CSP) subsequent to electronic authentication registration, identity proofing and issuance activities to manage and safeguard the integrity of an issued credential and its binding to an identity. Little or no confidence that an individual has maintained control over a token that has been entrusted to him or her and that that token has not been compromised. Characteristics include weak identity binding to tokens and plaintext passwords or secrets not transmitted across a network. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 1998 case LOACM2: return "Indicator of the basic digital quality or reliability of the activities performed by the Credential Service Provider (CSP) subsequent to electronic authentication registration, identity proofing and issuance activities to manage and safeguard the integrity of an issued credential and its binding to an identity. Some confidence that an individual has maintained control over a token that has been entrusted to him or her and that that token has not been compromised. Characteristics include: Verification must prove claimant controls the token; token resists online guessing, replay, session hijacking, and eavesdropping attacks; and token is at least weakly resistant to man-in-the middle attacks. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 1999 case LOACM3: return "Indicator of the medium digital quality or reliability of the activities performed by the Credential Service Provider (CSP) subsequent to electronic authentication registration, identity proofing and issuance activities to manage and safeguard the integrity of an issued credential and itā??s binding to an identity. High confidence that an individual has maintained control over a token that has been entrusted to him or her and that that token has not been compromised. Characteristics include: Ownership of token verifiable through security authentication protocol and credential management protects against verifier impersonation attacks. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 2000 case LOACM4: return "Indicator of the high digital quality or reliability of the activities performed by the Credential Service Provider (CSP) subsequent to electronic authentication registration, identity proofing and issuance activities to manage and safeguard the integrity of an issued credential and itā??s binding to an identity. Very high confidence that an individual has maintained control over a token that has been entrusted to him or her and that that token has not been compromised. Characteristics include: Verifier can prove control of token through a secure protocol; credential management supports strong cryptographic authentication of all communication parties. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 2001 case LOAID: return "Indicator of the quality or reliability in the process of ascertaining that an individual is who he or she claims to be."; 2002 case LOAID1: return "Indicator of low digital quality or reliability in the process of ascertaining that an individual is who he or she claims to be. Requires that a continuity of identity be maintained but does not require identity proofing. [Based on Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 2003 case LOAID2: return "Indicator of some digital quality or reliability in the process of ascertaining that that an individual is who he or she claims to be. Requires identity proofing via presentation of identifying material or information. [Based on Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 2004 case LOAID3: return "Indicator of high digital quality or reliability in the process of ascertaining that an individual is who he or she claims to be. Requires identity proofing procedures for verification of identifying materials and information. [Based on Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 2005 case LOAID4: return "Indicator of high digital quality or reliability in the process of ascertaining that an individual is who he or she claims to be. Requires identity proofing procedures for verification of identifying materials and information. [Based on Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 2006 case LOANR: return "Indicator of the digital quality or reliability in the process of establishing proof of delivery and proof of origin. [Based on ISO 7498-2]"; 2007 case LOANR1: return "Indicator of low digital quality or reliability in the process of establishing proof of delivery and proof of origin. [Based on ISO 7498-2]"; 2008 case LOANR2: return "Indicator of basic digital quality or reliability in the process of establishing proof of delivery and proof of origin. [Based on ISO 7498-2]"; 2009 case LOANR3: return "Indicator of medium digital quality or reliability in the process of establishing proof of delivery and proof of origin. [Based on ISO 7498-2]"; 2010 case LOANR4: return "Indicator of high digital quality or reliability in the process of establishing proof of delivery and proof of origin. [Based on ISO 7498-2]"; 2011 case LOARA: return "Indicator of the digital quality or reliability of the information exchange between network-connected devices where the information cannot be reliably protected end-to-end by a single organizationā??s security controls. [Based on NIST SP 800-63-2]"; 2012 case LOARA1: return "Indicator of low digital quality or reliability of the information exchange between network-connected devices where the information cannot be reliably protected end-to-end by a single organizationā??s security controls. [Based on NIST SP 800-63-2]"; 2013 case LOARA2: return "Indicator of basic digital quality or reliability of the information exchange between network-connected devices where the information cannot be reliably protected end-to-end by a single organizationā??s security controls. [Based on NIST SP 800-63-2]"; 2014 case LOARA3: return "Indicator of medium digital quality or reliability of the information exchange between network-connected devices where the information cannot be reliably protected end-to-end by a single organizationā??s security controls. [Based on NIST SP 800-63-2]"; 2015 case LOARA4: return "Indicator of high digital quality or reliability of the information exchange between network-connected devices where the information cannot be reliably protected end-to-end by a single organization's security controls. [Based on NIST SP 800-63-2]"; 2016 case LOATK: return "Indicator of the digital quality or reliability of single and multi-token authentication. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 2017 case LOATK1: return "Indicator of the low digital quality or reliability of single and multi-token authentication. Permits the use of any of the token methods of Levels 2, 3, or 4. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 2018 case LOATK2: return "Indicator of the basic digital quality or reliability of single and multi-token authentication. Requires single factor authentication using memorized secret tokens, pre-registered knowledge tokens, look-up secret tokens, out of band tokens, or single factor one-time password devices. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 2019 case LOATK3: return "Indicator of the medium digital quality or reliability of single and multi-token authentication. Requires two authentication factors. Provides multi-factor remote network authentication. Permits multi-factor software cryptographic token. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 2020 case LOATK4: return "Indicator of the high digital quality or reliability of single and multi-token authentication. Requires token that is a hardware cryptographic module validated at validated at Federal Information Processing Standard (FIPS) 140-2 Level 2 or higher overall with at least FIPS 140-2 Level 3 physical security. Level 4 token requirements can be met by using the PIV authentication key of a FIPS 201 compliant Personal Identity Verification (PIV) Card. [Electronic Authentication Guideline - Recommendations of the National Institute of Standards and Technology, NIST Special Publication 800-63-1, Dec 2011]"; 2021 case TRSTMECOBV: return "Values for security trust mechanism metadata observation made about a security architecture system component that supports enforcement of security policies."; 2022 case _SEVERITYOBSERVATION: return "Potential values for observations of severity."; 2023 case H: return "Indicates the condition may be life-threatening or has the potential to cause permanent injury."; 2024 case L: return "Indicates the condition may result in some adverse consequences but is unlikely to substantially affect the situation of the subject."; 2025 case M: return "Indicates the condition may result in noticable adverse adverse consequences but is unlikely to be life-threatening or cause permanent injury."; 2026 case _SUBJECTBODYPOSITION: return "Contains codes for defining the observed, physical position of a subject, such as during an observation, assessment, collection of a specimen, etc. ECG waveforms and vital signs, such as blood pressure, are two examples where a general, observed position typically needs to be noted."; 2027 case LLD: return "Lying on the left side."; 2028 case PRN: return "Lying with the front or ventral surface downward; lying face down."; 2029 case RLD: return "Lying on the right side."; 2030 case SFWL: return "A semi-sitting position in bed with the head of the bed elevated approximately 45 degrees."; 2031 case SIT: return "Resting the body on the buttocks, typically with upper torso erect or semi erect."; 2032 case STN: return "To be stationary, upright, vertical, on one's legs."; 2033 case SUP: return "supine"; 2034 case RTRD: return "Lying on the back, on an inclined plane, typically about 30-45 degrees with head raised and feet lowered."; 2035 case TRD: return "Lying on the back, on an inclined plane, typically about 30-45 degrees, with head lowered and feet raised."; 2036 case _VERIFICATIONOUTCOMEVALUE: return "Values for observations of verification act results\r\n\n \n Examples: Verified, not verified, verified with warning."; 2037 case ACT: return "Definition: Coverage is in effect for healthcare service(s) and/or product(s)."; 2038 case ACTPEND: return "Definition: Coverage is in effect for healthcare service(s) and/or product(s) - Pending Investigation"; 2039 case ELG: return "Definition: Coverage is in effect for healthcare service(s) and/or product(s)."; 2040 case INACT: return "Definition: Coverage is not in effect for healthcare service(s) and/or product(s)."; 2041 case INPNDINV: return "Definition: Coverage is not in effect for healthcare service(s) and/or product(s) - Pending Investigation."; 2042 case INPNDUPD: return "Definition: Coverage is not in effect for healthcare service(s) and/or product(s) - Pending Eligibility Update."; 2043 case NELG: return "Definition: Coverage is not in effect for healthcare service(s) and/or product(s). May optionally include reasons for the ineligibility."; 2044 case _ANNOTATIONVALUE: return "AnnotationValue"; 2045 case _COMMONCLINICALOBSERVATIONVALUE: return "Description:Used in a patient care message to value simple clinical (non-lab) observations."; 2046 case _INDIVIDUALCASESAFETYREPORTVALUEDOMAINS: return "This domain is established as a parent to a variety of value domains being defined to support the communication of Individual Case Safety Reports to regulatory bodies. Arguably, this aggregation is not taxonomically pure, but the grouping will facilitate the management of these domains."; 2047 case _INDICATIONVALUE: return "Indicates the specific observation result which is the reason for the action (prescription, lab test, etc.). E.g. Headache, Ear infection, planned diagnostic image (requiring contrast agent), etc."; 2048 case NULL: return null; 2049 default: return "?"; 2050 } 2051 } 2052 public String getDisplay() { 2053 switch (this) { 2054 case _ACTCOVERAGEASSESSMENTOBSERVATIONVALUE: return "ActCoverageAssessmentObservationValue"; 2055 case _ACTFINANCIALSTATUSOBSERVATIONVALUE: return "ActFinancialStatusObservationValue"; 2056 case ASSET: return "asset"; 2057 case ANNUITY: return "annuity"; 2058 case PROP: return "real property"; 2059 case RETACCT: return "retirement investment account"; 2060 case TRUST: return "trust"; 2061 case INCOME: return "income"; 2062 case CHILD: return "child support"; 2063 case DISABL: return "disability pay"; 2064 case INVEST: return "investment income"; 2065 case PAY: return "paid employment"; 2066 case RETIRE: return "retirement pay"; 2067 case SPOUSAL: return "spousal or partner support"; 2068 case SUPPLE: return "income supplement"; 2069 case TAX: return "tax obligation"; 2070 case LIVEXP: return "living expense"; 2071 case CLOTH: return "clothing expense"; 2072 case FOOD: return "food expense"; 2073 case HEALTH: return "health expense"; 2074 case HOUSE: return "household expense"; 2075 case LEGAL: return "legal expense"; 2076 case MORTG: return "mortgage"; 2077 case RENT: return "rent"; 2078 case SUNDRY: return "sundry expense"; 2079 case TRANS: return "transportation expense"; 2080 case UTIL: return "utility expense"; 2081 case ELSTAT: return "eligibility indicator"; 2082 case ADOPT: return "adoption document"; 2083 case BTHCERT: return "birth certificate"; 2084 case CCOC: return "creditable coverage document"; 2085 case DRLIC: return "driver license"; 2086 case FOSTER: return "foster child document"; 2087 case MEMBER: return "program or policy member"; 2088 case MIL: return "military identification"; 2089 case MRGCERT: return "marriage certificate"; 2090 case PASSPORT: return "passport"; 2091 case STUDENRL: return "student enrollment"; 2092 case HLSTAT: return "health status"; 2093 case DISABLE: return "disabled"; 2094 case DRUG: return "drug use"; 2095 case IVDRG: return "IV drug use"; 2096 case PGNT: return "pregnant"; 2097 case LIVDEP: return "living dependency"; 2098 case RELDEP: return "relative dependent"; 2099 case SPSDEP: return "spouse dependent"; 2100 case URELDEP: return "unrelated person dependent"; 2101 case LIVSIT: return "living situation"; 2102 case ALONE: return "alone"; 2103 case DEPCHD: return "dependent children"; 2104 case DEPSPS: return "dependent spouse"; 2105 case DEPYGCHD: return "dependent young children"; 2106 case FAM: return "live with family"; 2107 case RELAT: return "relative"; 2108 case SPS: return "spouse only"; 2109 case UNREL: return "unrelated person"; 2110 case SOECSTAT: return "socio economic status"; 2111 case ABUSE: return "abuse victim"; 2112 case HMLESS: return "homeless"; 2113 case ILGIM: return "illegal immigrant"; 2114 case INCAR: return "incarcerated"; 2115 case PROB: return "probation"; 2116 case REFUG: return "refugee"; 2117 case UNEMPL: return "unemployed"; 2118 case _ALLERGYTESTVALUE: return "AllergyTestValue"; 2119 case A0: return "no reaction"; 2120 case A1: return "minimal reaction"; 2121 case A2: return "mild reaction"; 2122 case A3: return "moderate reaction"; 2123 case A4: return "severe reaction"; 2124 case _COMPOSITEMEASURESCORING: return "CompositeMeasureScoring"; 2125 case ALLORNONESCR: return "All-or-nothing Scoring"; 2126 case LINEARSCR: return "Linear Scoring"; 2127 case OPPORSCR: return "Opportunity Scoring"; 2128 case WEIGHTSCR: return "Weighted Scoring"; 2129 case _COVERAGELIMITOBSERVATIONVALUE: return "CoverageLimitObservationValue"; 2130 case _COVERAGELEVELOBSERVATIONVALUE: return "CoverageLevelObservationValue"; 2131 case ADC: return "adult child"; 2132 case CHD: return "child"; 2133 case DEP: return "dependent"; 2134 case DP: return "domestic partner"; 2135 case ECH: return "employee"; 2136 case FLY: return "family coverage"; 2137 case IND: return "individual"; 2138 case SSP: return "same sex partner"; 2139 case _CRITICALITYOBSERVATIONVALUE: return "CriticalityObservationValue"; 2140 case CRITH: return "high criticality"; 2141 case CRITL: return "low criticality"; 2142 case CRITU: return "unable to assess criticality"; 2143 case _GENETICOBSERVATIONVALUE: return "GeneticObservationValue"; 2144 case HOMOZYGOTE: return "HOMO"; 2145 case _OBSERVATIONMEASURESCORING: return "ObservationMeasureScoring"; 2146 case COHORT: return "cohort measure scoring"; 2147 case CONTVAR: return "continuous variable measure scoring"; 2148 case PROPOR: return "proportion measure scoring"; 2149 case RATIO: return "ratio measure scoring"; 2150 case _OBSERVATIONMEASURETYPE: return "ObservationMeasureType"; 2151 case COMPOSITE: return "composite measure type"; 2152 case EFFICIENCY: return "efficiency measure type"; 2153 case EXPERIENCE: return "experience measure type"; 2154 case OUTCOME: return "outcome measure type"; 2155 case PROCESS: return "process measure type"; 2156 case RESOURCE: return "resource use measure type"; 2157 case STRUCTURE: return "structure measure type"; 2158 case _OBSERVATIONPOPULATIONINCLUSION: return "ObservationPopulationInclusion"; 2159 case DENEX: return "denominator exclusions"; 2160 case DENEXCEP: return "denominator exceptions"; 2161 case DENOM: return "denominator"; 2162 case IP: return "initial population"; 2163 case IPP: return "initial patient population"; 2164 case MSRPOPL: return "measure population"; 2165 case NUMER: return "numerator"; 2166 case NUMEX: return "numerator exclusions"; 2167 case _PARTIALCOMPLETIONSCALE: return "PartialCompletionScale"; 2168 case G: return "Great extent"; 2169 case LE: return "Large extent"; 2170 case ME: return "Medium extent"; 2171 case MI: return "Minimal extent"; 2172 case N: return "None"; 2173 case S: return "Some extent"; 2174 case _SECURITYOBSERVATIONVALUE: return "SecurityObservationValue"; 2175 case _SECINTOBV: return "security integrity"; 2176 case _SECALTINTOBV: return "alteration integrity"; 2177 case ABSTRED: return "abstracted"; 2178 case AGGRED: return "aggregated"; 2179 case ANONYED: return "anonymized"; 2180 case MAPPED: return "mapped"; 2181 case MASKED: return "masked"; 2182 case PSEUDED: return "pseudonymized"; 2183 case REDACTED: return "redacted"; 2184 case SUBSETTED: return "subsetted"; 2185 case SYNTAC: return "syntactic transform"; 2186 case TRSLT: return "translated"; 2187 case VERSIONED: return "versioned"; 2188 case _SECDATINTOBV: return "data integrity"; 2189 case CRYTOHASH: return "cryptographic hash function"; 2190 case DIGSIG: return "digital signature"; 2191 case _SECINTCONOBV: return "integrity confidence"; 2192 case HRELIABLE: return "highly reliable"; 2193 case RELIABLE: return "reliable"; 2194 case UNCERTREL: return "uncertain reliability"; 2195 case UNRELIABLE: return "unreliable"; 2196 case _SECINTPRVOBV: return "provenance"; 2197 case _SECINTPRVABOBV: return "provenance asserted by"; 2198 case CLINAST: return "clinician asserted"; 2199 case DEVAST: return "device asserted"; 2200 case HCPAST: return "healthcare professional asserted"; 2201 case PACQAST: return "patient acquaintance asserted"; 2202 case PATAST: return "patient asserted"; 2203 case PAYAST: return "payer asserted"; 2204 case PROAST: return "professional asserted"; 2205 case SDMAST: return "substitute decision maker asserted"; 2206 case _SECINTPRVRBOBV: return "provenance reported by"; 2207 case CLINRPT: return "clinician reported"; 2208 case DEVRPT: return "device reported"; 2209 case HCPRPT: return "healthcare professional reported"; 2210 case PACQRPT: return "patient acquaintance reported"; 2211 case PATRPT: return "patient reported"; 2212 case PAYRPT: return "payer reported"; 2213 case PRORPT: return "professional reported"; 2214 case SDMRPT: return "substitute decision maker reported"; 2215 case SECTRSTOBV: return "security trust observation"; 2216 case TRSTACCRDOBV: return "trust accreditation observation"; 2217 case TRSTAGREOBV: return "trust agreement observation"; 2218 case TRSTCERTOBV: return "trust certificate observation"; 2219 case TRSTLOAOBV: return "trust assurance observation"; 2220 case LOAAN: return "authentication level of assurance value"; 2221 case LOAAN1: return "low authentication level of assurance"; 2222 case LOAAN2: return "basic authentication level of assurance"; 2223 case LOAAN3: return "medium authentication level of assurance"; 2224 case LOAAN4: return "high authentication level of assurance"; 2225 case LOAAP: return "authentication process level of assurance value"; 2226 case LOAAP1: return "low authentication process level of assurance"; 2227 case LOAAP2: return "basic authentication process level of assurance"; 2228 case LOAAP3: return "medium authentication process level of assurance"; 2229 case LOAAP4: return "high authentication process level of assurance"; 2230 case LOAAS: return "assertion level of assurance value"; 2231 case LOAAS1: return "low assertion level of assurance"; 2232 case LOAAS2: return "basic assertion level of assurance"; 2233 case LOAAS3: return "medium assertion level of assurance"; 2234 case LOAAS4: return "high assertion level of assurance"; 2235 case LOACM: return "token and credential management level of assurance value)"; 2236 case LOACM1: return "low token and credential management level of assurance"; 2237 case LOACM2: return "basic token and credential management level of assurance"; 2238 case LOACM3: return "medium token and credential management level of assurance"; 2239 case LOACM4: return "high token and credential management level of assurance"; 2240 case LOAID: return "identity proofing level of assurance"; 2241 case LOAID1: return "low identity proofing level of assurance"; 2242 case LOAID2: return "basic identity proofing level of assurance"; 2243 case LOAID3: return "medium identity proofing level of assurance"; 2244 case LOAID4: return "high identity proofing level of assurance"; 2245 case LOANR: return "non-repudiation level of assurance value"; 2246 case LOANR1: return "low non-repudiation level of assurance"; 2247 case LOANR2: return "basic non-repudiation level of assurance"; 2248 case LOANR3: return "medium non-repudiation level of assurance"; 2249 case LOANR4: return "high non-repudiation level of assurance"; 2250 case LOARA: return "remote access level of assurance value"; 2251 case LOARA1: return "low remote access level of assurance"; 2252 case LOARA2: return "basic remote access level of assurance"; 2253 case LOARA3: return "medium remote access level of assurance"; 2254 case LOARA4: return "high remote access level of assurance"; 2255 case LOATK: return "token level of assurance value"; 2256 case LOATK1: return "low token level of assurance"; 2257 case LOATK2: return "basic token level of assurance"; 2258 case LOATK3: return "medium token level of assurance"; 2259 case LOATK4: return "high token level of assurance"; 2260 case TRSTMECOBV: return "none supplied 6"; 2261 case _SEVERITYOBSERVATION: return "SeverityObservation"; 2262 case H: return "High"; 2263 case L: return "Low"; 2264 case M: return "Moderate"; 2265 case _SUBJECTBODYPOSITION: return "_SubjectBodyPosition"; 2266 case LLD: return "left lateral decubitus"; 2267 case PRN: return "prone"; 2268 case RLD: return "right lateral decubitus"; 2269 case SFWL: return "Semi-Fowler's"; 2270 case SIT: return "sitting"; 2271 case STN: return "standing"; 2272 case SUP: return "supine"; 2273 case RTRD: return "reverse trendelenburg"; 2274 case TRD: return "trendelenburg"; 2275 case _VERIFICATIONOUTCOMEVALUE: return "verification outcome"; 2276 case ACT: return "active coverage"; 2277 case ACTPEND: return "active - pending investigation"; 2278 case ELG: return "eligible"; 2279 case INACT: return "inactive"; 2280 case INPNDINV: return "inactive - pending investigation"; 2281 case INPNDUPD: return "inactive - pending eligibility update"; 2282 case NELG: return "not eligible"; 2283 case _ANNOTATIONVALUE: return "AnnotationValue"; 2284 case _COMMONCLINICALOBSERVATIONVALUE: return "common clinical observation"; 2285 case _INDIVIDUALCASESAFETYREPORTVALUEDOMAINS: return "Individual Case Safety Report Value Domains"; 2286 case _INDICATIONVALUE: return "IndicationValue"; 2287 case NULL: return null; 2288 default: return "?"; 2289 } 2290 } 2291 2292 2293}