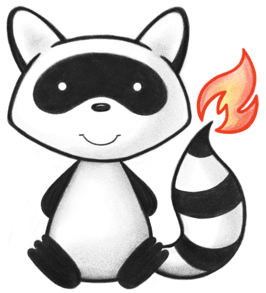
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3ParticipationMode { 041 042 /** 043 * Participation by non-human-languaged based electronic signal 044 */ 045 ELECTRONIC, 046 /** 047 * Participation by direct action where subject and actor are in the same location. (The participation involves more than communication.) 048 */ 049 PHYSICAL, 050 /** 051 * Participation by direct action where subject and actor are in separate locations, and the actions of the actor are transmitted by electronic or mechanical means. (The participation involves more than communication.) 052 */ 053 REMOTE, 054 /** 055 * Participation by voice communication 056 */ 057 VERBAL, 058 /** 059 * Participation by pre-recorded voice. Communication is limited to one direction (from the recorder to recipient). 060 */ 061 DICTATE, 062 /** 063 * Participation by voice communication where parties speak to each other directly. 064 */ 065 FACE, 066 /** 067 * Participation by voice communication where the voices of the communicating parties are transported over an electronic medium 068 */ 069 PHONE, 070 /** 071 * Participation by voice and visual communication where the voices and images of the communicating parties are transported over an electronic medium 072 */ 073 VIDEOCONF, 074 /** 075 * Participation by human language recorded on a physical material 076 */ 077 WRITTEN, 078 /** 079 * Participation by text or diagrams printed on paper that have been transmitted over a fax device 080 */ 081 FAXWRIT, 082 /** 083 * Participation by text or diagrams printed on paper or other recording medium 084 */ 085 HANDWRIT, 086 /** 087 * Participation by text or diagrams printed on paper transmitted physically (e.g. by courier service, postal service). 088 */ 089 MAILWRIT, 090 /** 091 * Participation by text or diagrams submitted by computer network, e.g. online survey. 092 */ 093 ONLINEWRIT, 094 /** 095 * Participation by text or diagrams transmitted over an electronic mail system. 096 */ 097 EMAILWRIT, 098 /** 099 * Participation by text or diagrams printed on paper or other recording medium where the recording was performed using a typewriter, typesetter, computer or similar mechanism. 100 */ 101 TYPEWRIT, 102 /** 103 * added to help the parsers 104 */ 105 NULL; 106 public static V3ParticipationMode fromCode(String codeString) throws FHIRException { 107 if (codeString == null || "".equals(codeString)) 108 return null; 109 if ("ELECTRONIC".equals(codeString)) 110 return ELECTRONIC; 111 if ("PHYSICAL".equals(codeString)) 112 return PHYSICAL; 113 if ("REMOTE".equals(codeString)) 114 return REMOTE; 115 if ("VERBAL".equals(codeString)) 116 return VERBAL; 117 if ("DICTATE".equals(codeString)) 118 return DICTATE; 119 if ("FACE".equals(codeString)) 120 return FACE; 121 if ("PHONE".equals(codeString)) 122 return PHONE; 123 if ("VIDEOCONF".equals(codeString)) 124 return VIDEOCONF; 125 if ("WRITTEN".equals(codeString)) 126 return WRITTEN; 127 if ("FAXWRIT".equals(codeString)) 128 return FAXWRIT; 129 if ("HANDWRIT".equals(codeString)) 130 return HANDWRIT; 131 if ("MAILWRIT".equals(codeString)) 132 return MAILWRIT; 133 if ("ONLINEWRIT".equals(codeString)) 134 return ONLINEWRIT; 135 if ("EMAILWRIT".equals(codeString)) 136 return EMAILWRIT; 137 if ("TYPEWRIT".equals(codeString)) 138 return TYPEWRIT; 139 throw new FHIRException("Unknown V3ParticipationMode code '"+codeString+"'"); 140 } 141 public String toCode() { 142 switch (this) { 143 case ELECTRONIC: return "ELECTRONIC"; 144 case PHYSICAL: return "PHYSICAL"; 145 case REMOTE: return "REMOTE"; 146 case VERBAL: return "VERBAL"; 147 case DICTATE: return "DICTATE"; 148 case FACE: return "FACE"; 149 case PHONE: return "PHONE"; 150 case VIDEOCONF: return "VIDEOCONF"; 151 case WRITTEN: return "WRITTEN"; 152 case FAXWRIT: return "FAXWRIT"; 153 case HANDWRIT: return "HANDWRIT"; 154 case MAILWRIT: return "MAILWRIT"; 155 case ONLINEWRIT: return "ONLINEWRIT"; 156 case EMAILWRIT: return "EMAILWRIT"; 157 case TYPEWRIT: return "TYPEWRIT"; 158 case NULL: return null; 159 default: return "?"; 160 } 161 } 162 public String getSystem() { 163 return "http://hl7.org/fhir/v3/ParticipationMode"; 164 } 165 public String getDefinition() { 166 switch (this) { 167 case ELECTRONIC: return "Participation by non-human-languaged based electronic signal"; 168 case PHYSICAL: return "Participation by direct action where subject and actor are in the same location. (The participation involves more than communication.)"; 169 case REMOTE: return "Participation by direct action where subject and actor are in separate locations, and the actions of the actor are transmitted by electronic or mechanical means. (The participation involves more than communication.)"; 170 case VERBAL: return "Participation by voice communication"; 171 case DICTATE: return "Participation by pre-recorded voice. Communication is limited to one direction (from the recorder to recipient)."; 172 case FACE: return "Participation by voice communication where parties speak to each other directly."; 173 case PHONE: return "Participation by voice communication where the voices of the communicating parties are transported over an electronic medium"; 174 case VIDEOCONF: return "Participation by voice and visual communication where the voices and images of the communicating parties are transported over an electronic medium"; 175 case WRITTEN: return "Participation by human language recorded on a physical material"; 176 case FAXWRIT: return "Participation by text or diagrams printed on paper that have been transmitted over a fax device"; 177 case HANDWRIT: return "Participation by text or diagrams printed on paper or other recording medium"; 178 case MAILWRIT: return "Participation by text or diagrams printed on paper transmitted physically (e.g. by courier service, postal service)."; 179 case ONLINEWRIT: return "Participation by text or diagrams submitted by computer network, e.g. online survey."; 180 case EMAILWRIT: return "Participation by text or diagrams transmitted over an electronic mail system."; 181 case TYPEWRIT: return "Participation by text or diagrams printed on paper or other recording medium where the recording was performed using a typewriter, typesetter, computer or similar mechanism."; 182 case NULL: return null; 183 default: return "?"; 184 } 185 } 186 public String getDisplay() { 187 switch (this) { 188 case ELECTRONIC: return "electronic data"; 189 case PHYSICAL: return "physical presence"; 190 case REMOTE: return "remote presence"; 191 case VERBAL: return "verbal"; 192 case DICTATE: return "dictated"; 193 case FACE: return "face-to-face"; 194 case PHONE: return "telephone"; 195 case VIDEOCONF: return "videoconferencing"; 196 case WRITTEN: return "written"; 197 case FAXWRIT: return "telefax"; 198 case HANDWRIT: return "handwritten"; 199 case MAILWRIT: return "mail"; 200 case ONLINEWRIT: return "online written"; 201 case EMAILWRIT: return "email"; 202 case TYPEWRIT: return "typewritten"; 203 case NULL: return null; 204 default: return "?"; 205 } 206 } 207 208 209}