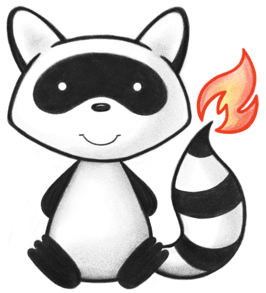
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3QueryParameterValue { 041 042 /** 043 * Description:Filter codes used to manage volume of dispenses returned by a parameter-based queries. 044 */ 045 _DISPENSEQUERYFILTERCODE, 046 /** 047 * Description:Returns all dispenses to date for a prescription. 048 */ 049 ALLDISP, 050 /** 051 * Description:Returns the most recent dispense for a prescription. 052 */ 053 LASTDISP, 054 /** 055 * Description:Returns no dispense for a prescription. 056 */ 057 NODISP, 058 /** 059 * Filter codes used to manage types of orders being returned by a parameter-based query. 060 */ 061 _ORDERFILTERCODE, 062 /** 063 * Return all orders. 064 */ 065 AO, 066 /** 067 * Return only those orders that do not have results. 068 */ 069 ONR, 070 /** 071 * Return only those orders that have results. 072 */ 073 OWR, 074 /** 075 * A "helper" vocabulary used to construct complex query filters based on how and whether a prescription has been dispensed. 076 */ 077 _PRESCRIPTIONDISPENSEFILTERCODE, 078 /** 079 * Filter to only include SubstanceAdministration orders which have no remaining quantity authorized to be dispensed. 080 */ 081 C, 082 /** 083 * Filter to only include SubstanceAdministration orders which have no fulfilling supply events performed. 084 */ 085 N, 086 /** 087 * Filter to only include SubstanceAdministration orders which have had at least one fulfilling supply event, but which still have outstanding quantity remaining to be authorized. 088 */ 089 R, 090 /** 091 * Description:Indicates how result sets should be filtered based on whether they have associated issues. 092 */ 093 _QUERYPARAMETERVALUE, 094 /** 095 * Description:Result set should not be filtered based on the presence of issues. 096 */ 097 ISSFA, 098 /** 099 * Description:Result set should be filtered to only include records with associated issues. 100 */ 101 ISSFI, 102 /** 103 * Description:Result set should be filtered to only include records with associated unmanaged issues. 104 */ 105 ISSFU, 106 /** 107 * added to help the parsers 108 */ 109 NULL; 110 public static V3QueryParameterValue fromCode(String codeString) throws FHIRException { 111 if (codeString == null || "".equals(codeString)) 112 return null; 113 if ("_DispenseQueryFilterCode".equals(codeString)) 114 return _DISPENSEQUERYFILTERCODE; 115 if ("ALLDISP".equals(codeString)) 116 return ALLDISP; 117 if ("LASTDISP".equals(codeString)) 118 return LASTDISP; 119 if ("NODISP".equals(codeString)) 120 return NODISP; 121 if ("_OrderFilterCode".equals(codeString)) 122 return _ORDERFILTERCODE; 123 if ("AO".equals(codeString)) 124 return AO; 125 if ("ONR".equals(codeString)) 126 return ONR; 127 if ("OWR".equals(codeString)) 128 return OWR; 129 if ("_PrescriptionDispenseFilterCode".equals(codeString)) 130 return _PRESCRIPTIONDISPENSEFILTERCODE; 131 if ("C".equals(codeString)) 132 return C; 133 if ("N".equals(codeString)) 134 return N; 135 if ("R".equals(codeString)) 136 return R; 137 if ("_QueryParameterValue".equals(codeString)) 138 return _QUERYPARAMETERVALUE; 139 if ("ISSFA".equals(codeString)) 140 return ISSFA; 141 if ("ISSFI".equals(codeString)) 142 return ISSFI; 143 if ("ISSFU".equals(codeString)) 144 return ISSFU; 145 throw new FHIRException("Unknown V3QueryParameterValue code '"+codeString+"'"); 146 } 147 public String toCode() { 148 switch (this) { 149 case _DISPENSEQUERYFILTERCODE: return "_DispenseQueryFilterCode"; 150 case ALLDISP: return "ALLDISP"; 151 case LASTDISP: return "LASTDISP"; 152 case NODISP: return "NODISP"; 153 case _ORDERFILTERCODE: return "_OrderFilterCode"; 154 case AO: return "AO"; 155 case ONR: return "ONR"; 156 case OWR: return "OWR"; 157 case _PRESCRIPTIONDISPENSEFILTERCODE: return "_PrescriptionDispenseFilterCode"; 158 case C: return "C"; 159 case N: return "N"; 160 case R: return "R"; 161 case _QUERYPARAMETERVALUE: return "_QueryParameterValue"; 162 case ISSFA: return "ISSFA"; 163 case ISSFI: return "ISSFI"; 164 case ISSFU: return "ISSFU"; 165 case NULL: return null; 166 default: return "?"; 167 } 168 } 169 public String getSystem() { 170 return "http://hl7.org/fhir/v3/QueryParameterValue"; 171 } 172 public String getDefinition() { 173 switch (this) { 174 case _DISPENSEQUERYFILTERCODE: return "Description:Filter codes used to manage volume of dispenses returned by a parameter-based queries."; 175 case ALLDISP: return "Description:Returns all dispenses to date for a prescription."; 176 case LASTDISP: return "Description:Returns the most recent dispense for a prescription."; 177 case NODISP: return "Description:Returns no dispense for a prescription."; 178 case _ORDERFILTERCODE: return "Filter codes used to manage types of orders being returned by a parameter-based query."; 179 case AO: return "Return all orders."; 180 case ONR: return "Return only those orders that do not have results."; 181 case OWR: return "Return only those orders that have results."; 182 case _PRESCRIPTIONDISPENSEFILTERCODE: return "A \"helper\" vocabulary used to construct complex query filters based on how and whether a prescription has been dispensed."; 183 case C: return "Filter to only include SubstanceAdministration orders which have no remaining quantity authorized to be dispensed."; 184 case N: return "Filter to only include SubstanceAdministration orders which have no fulfilling supply events performed."; 185 case R: return "Filter to only include SubstanceAdministration orders which have had at least one fulfilling supply event, but which still have outstanding quantity remaining to be authorized."; 186 case _QUERYPARAMETERVALUE: return "Description:Indicates how result sets should be filtered based on whether they have associated issues."; 187 case ISSFA: return "Description:Result set should not be filtered based on the presence of issues."; 188 case ISSFI: return "Description:Result set should be filtered to only include records with associated issues."; 189 case ISSFU: return "Description:Result set should be filtered to only include records with associated unmanaged issues."; 190 case NULL: return null; 191 default: return "?"; 192 } 193 } 194 public String getDisplay() { 195 switch (this) { 196 case _DISPENSEQUERYFILTERCODE: return "dispense query filter code"; 197 case ALLDISP: return "all dispenses"; 198 case LASTDISP: return "last dispense"; 199 case NODISP: return "no dispense"; 200 case _ORDERFILTERCODE: return "_OrderFilterCode"; 201 case AO: return "all orders"; 202 case ONR: return "orders without results"; 203 case OWR: return "orders with results"; 204 case _PRESCRIPTIONDISPENSEFILTERCODE: return "Prescription Dispense Filter Code"; 205 case C: return "Completely dispensed"; 206 case N: return "Never Dispensed"; 207 case R: return "Dispensed with remaining fills"; 208 case _QUERYPARAMETERVALUE: return "QueryParameterValue"; 209 case ISSFA: return "all"; 210 case ISSFI: return "with issues"; 211 case ISSFU: return "with unmanaged issues"; 212 case NULL: return null; 213 default: return "?"; 214 } 215 } 216 217 218}