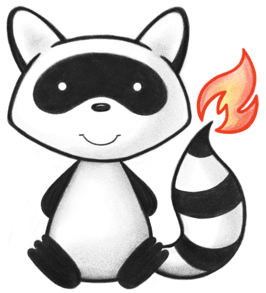
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3RoleLinkType { 041 042 /** 043 * An action taken with respect to a subject Entity by a regulatory or authoritative body with supervisory capacity over that entity. The action is taken in response to behavior by the subject Entity that body finds to be undesirable. 044 045 Suspension, license restrictions, monetary fine, letter of reprimand, mandated training, mandated supervision, etc.Examples: 046 */ 047 REL, 048 /** 049 * This relationship indicates the source Role is available to the target Role as a backup. An entity in a backup role will be available as a substitute or replacement in the event that the entity assigned the role is unavailable. In medical roles where it is critical that the function be performed and there is a possibility that the individual assigned may be ill or otherwise indisposed, another individual is assigned to cover for the individual originally assigned the role. A backup may be required to be identified, but unless the backup is actually used, he/she would not assume the assigned entity role. 050 */ 051 BACKUP, 052 /** 053 * This relationship indicates the target Role provides or receives information regarding the target role. For example, AssignedEntity is a contact for a ServiceDeliveryLocation. 054 */ 055 CONT, 056 /** 057 * The source Role has direct authority over the target role in a chain of authority. 058 */ 059 DIRAUTH, 060 /** 061 * Description: The source role provides identification for the target role. The source role must be IDENT. The player entity of the source role is constrained to be the same as (i.e. the equivalent of, or equal to) the player of the target role if present. If the player is absent from the source role, then it is assumed to be the same as the player of the target role. 062 */ 063 IDENT, 064 /** 065 * The source Role has indirect authority over the target role in a chain of authority. 066 */ 067 INDAUTH, 068 /** 069 * The target Role is part of the Source Role. 070 */ 071 PART, 072 /** 073 * This relationship indicates that the source Role replaces (or subsumes) the target Role. Allows for new identifiers and/or new effective time for a registry entry or a certification, etc. 074 */ 075 REPL, 076 /** 077 * added to help the parsers 078 */ 079 NULL; 080 public static V3RoleLinkType fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("REL".equals(codeString)) 084 return REL; 085 if ("BACKUP".equals(codeString)) 086 return BACKUP; 087 if ("CONT".equals(codeString)) 088 return CONT; 089 if ("DIRAUTH".equals(codeString)) 090 return DIRAUTH; 091 if ("IDENT".equals(codeString)) 092 return IDENT; 093 if ("INDAUTH".equals(codeString)) 094 return INDAUTH; 095 if ("PART".equals(codeString)) 096 return PART; 097 if ("REPL".equals(codeString)) 098 return REPL; 099 throw new FHIRException("Unknown V3RoleLinkType code '"+codeString+"'"); 100 } 101 public String toCode() { 102 switch (this) { 103 case REL: return "REL"; 104 case BACKUP: return "BACKUP"; 105 case CONT: return "CONT"; 106 case DIRAUTH: return "DIRAUTH"; 107 case IDENT: return "IDENT"; 108 case INDAUTH: return "INDAUTH"; 109 case PART: return "PART"; 110 case REPL: return "REPL"; 111 case NULL: return null; 112 default: return "?"; 113 } 114 } 115 public String getSystem() { 116 return "http://hl7.org/fhir/v3/RoleLinkType"; 117 } 118 public String getDefinition() { 119 switch (this) { 120 case REL: return "An action taken with respect to a subject Entity by a regulatory or authoritative body with supervisory capacity over that entity. The action is taken in response to behavior by the subject Entity that body finds to be undesirable.\r\n\n Suspension, license restrictions, monetary fine, letter of reprimand, mandated training, mandated supervision, etc.Examples:"; 121 case BACKUP: return "This relationship indicates the source Role is available to the target Role as a backup. An entity in a backup role will be available as a substitute or replacement in the event that the entity assigned the role is unavailable. In medical roles where it is critical that the function be performed and there is a possibility that the individual assigned may be ill or otherwise indisposed, another individual is assigned to cover for the individual originally assigned the role. A backup may be required to be identified, but unless the backup is actually used, he/she would not assume the assigned entity role."; 122 case CONT: return "This relationship indicates the target Role provides or receives information regarding the target role. For example, AssignedEntity is a contact for a ServiceDeliveryLocation."; 123 case DIRAUTH: return "The source Role has direct authority over the target role in a chain of authority."; 124 case IDENT: return "Description: The source role provides identification for the target role. The source role must be IDENT. The player entity of the source role is constrained to be the same as (i.e. the equivalent of, or equal to) the player of the target role if present. If the player is absent from the source role, then it is assumed to be the same as the player of the target role."; 125 case INDAUTH: return "The source Role has indirect authority over the target role in a chain of authority."; 126 case PART: return "The target Role is part of the Source Role."; 127 case REPL: return "This relationship indicates that the source Role replaces (or subsumes) the target Role. Allows for new identifiers and/or new effective time for a registry entry or a certification, etc."; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 public String getDisplay() { 133 switch (this) { 134 case REL: return "related"; 135 case BACKUP: return "is backup for"; 136 case CONT: return "has contact"; 137 case DIRAUTH: return "has direct authority over"; 138 case IDENT: return "Identification"; 139 case INDAUTH: return "has indirect authority over"; 140 case PART: return "has part"; 141 case REPL: return "replaces"; 142 case NULL: return null; 143 default: return "?"; 144 } 145 } 146 147 148}