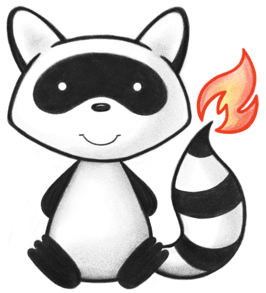
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.dstu3.model.EnumFactory; 039 040public class V3SetOperatorEnumFactory implements EnumFactory<V3SetOperator> { 041 042 public V3SetOperator fromCode(String codeString) throws IllegalArgumentException { 043 if (codeString == null || "".equals(codeString)) 044 return null; 045 if ("_ValueSetOperator".equals(codeString)) 046 return V3SetOperator._VALUESETOPERATOR; 047 if ("E".equals(codeString)) 048 return V3SetOperator.E; 049 if ("I".equals(codeString)) 050 return V3SetOperator.I; 051 if ("A".equals(codeString)) 052 return V3SetOperator.A; 053 if ("H".equals(codeString)) 054 return V3SetOperator.H; 055 if ("P".equals(codeString)) 056 return V3SetOperator.P; 057 throw new IllegalArgumentException("Unknown V3SetOperator code '"+codeString+"'"); 058 } 059 060 public String toCode(V3SetOperator code) { 061 if (code == V3SetOperator.NULL) 062 return null; 063 if (code == V3SetOperator._VALUESETOPERATOR) 064 return "_ValueSetOperator"; 065 if (code == V3SetOperator.E) 066 return "E"; 067 if (code == V3SetOperator.I) 068 return "I"; 069 if (code == V3SetOperator.A) 070 return "A"; 071 if (code == V3SetOperator.H) 072 return "H"; 073 if (code == V3SetOperator.P) 074 return "P"; 075 return "?"; 076 } 077 078 public String toSystem(V3SetOperator code) { 079 return code.getSystem(); 080 } 081 082}