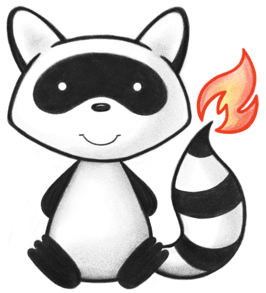
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3SubstanceAdminSubstitution { 041 042 /** 043 * Description: Substitution occurred or is permitted with another product that may potentially have different ingredients, but having the same biological and therapeutic effects. 044 */ 045 _ACTSUBSTANCEADMINSUBSTITUTIONCODE, 046 /** 047 * Description: Substitution occurred or is permitted with another bioequivalent and therapeutically equivalent product. 048 */ 049 E, 050 /** 051 * Description: 052 053 054 Substitution occurred or is permitted with another product that is a: 055 056 057 pharmaceutical alternative containing the same active ingredient but is formulated with different salt, ester 058 pharmaceutical equivalent that has the same active ingredient, strength, dosage form and route of administration 059 060 061 Examples: 062 063 064 065 066 Pharmaceutical alternative: Erythromycin Ethylsuccinate for Erythromycin Stearate 067 068 Pharmaceutical equivalent: Lisonpril for Zestril 069 */ 070 EC, 071 /** 072 * Description: 073 074 075 Substitution occurred or is permitted between equivalent Brands but not Generics 076 077 078 Examples: 079 080 081 082 Zestril for Prinivil 083 Coumadin for Jantoven 084 */ 085 BC, 086 /** 087 * Description: Substitution occurred or is permitted between equivalent Generics but not Brands 088 089 090 Examples: 091 092 093 094 Lisnopril (Lupin Corp) for Lisnopril (Wockhardt Corp) 095 */ 096 G, 097 /** 098 * Description: Substitution occurred or is permitted with another product having the same therapeutic objective and safety profile. 099 100 101 Examples: 102 103 104 105 ranitidine for Tagamet 106 */ 107 TE, 108 /** 109 * Description: Substitution occurred or is permitted between therapeutically equivalent Brands but not Generics 110> 111 Examples: 112 113 114 115 Zantac for Tagamet 116 */ 117 TB, 118 /** 119 * Description: Substitution occurred or is permitted between therapeutically equivalent Generics but not Brands 120> 121 Examples: 122 123 124 125 Ranitidine for cimetidine 126 */ 127 TG, 128 /** 129 * Description: This substitution was performed or is permitted based on formulary guidelines. 130 */ 131 F, 132 /** 133 * No substitution occurred or is permitted. 134 */ 135 N, 136 /** 137 * added to help the parsers 138 */ 139 NULL; 140 public static V3SubstanceAdminSubstitution fromCode(String codeString) throws FHIRException { 141 if (codeString == null || "".equals(codeString)) 142 return null; 143 if ("_ActSubstanceAdminSubstitutionCode".equals(codeString)) 144 return _ACTSUBSTANCEADMINSUBSTITUTIONCODE; 145 if ("E".equals(codeString)) 146 return E; 147 if ("EC".equals(codeString)) 148 return EC; 149 if ("BC".equals(codeString)) 150 return BC; 151 if ("G".equals(codeString)) 152 return G; 153 if ("TE".equals(codeString)) 154 return TE; 155 if ("TB".equals(codeString)) 156 return TB; 157 if ("TG".equals(codeString)) 158 return TG; 159 if ("F".equals(codeString)) 160 return F; 161 if ("N".equals(codeString)) 162 return N; 163 throw new FHIRException("Unknown V3SubstanceAdminSubstitution code '"+codeString+"'"); 164 } 165 public String toCode() { 166 switch (this) { 167 case _ACTSUBSTANCEADMINSUBSTITUTIONCODE: return "_ActSubstanceAdminSubstitutionCode"; 168 case E: return "E"; 169 case EC: return "EC"; 170 case BC: return "BC"; 171 case G: return "G"; 172 case TE: return "TE"; 173 case TB: return "TB"; 174 case TG: return "TG"; 175 case F: return "F"; 176 case N: return "N"; 177 case NULL: return null; 178 default: return "?"; 179 } 180 } 181 public String getSystem() { 182 return "http://hl7.org/fhir/v3/substanceAdminSubstitution"; 183 } 184 public String getDefinition() { 185 switch (this) { 186 case _ACTSUBSTANCEADMINSUBSTITUTIONCODE: return "Description: Substitution occurred or is permitted with another product that may potentially have different ingredients, but having the same biological and therapeutic effects."; 187 case E: return "Description: Substitution occurred or is permitted with another bioequivalent and therapeutically equivalent product."; 188 case EC: return "Description: \n \r\n\n Substitution occurred or is permitted with another product that is a:\r\n\n \n pharmaceutical alternative containing the same active ingredient but is formulated with different salt, ester\n pharmaceutical equivalent that has the same active ingredient, strength, dosage form and route of administration\n \n \n Examples: \n \r\n\n \n \n Pharmaceutical alternative: Erythromycin Ethylsuccinate for Erythromycin Stearate\n \n Pharmaceutical equivalent: Lisonpril for Zestril"; 189 case BC: return "Description: \n \r\n\n Substitution occurred or is permitted between equivalent Brands but not Generics\r\n\n \n Examples: \n \r\n\n \n Zestril for Prinivil\n Coumadin for Jantoven"; 190 case G: return "Description: Substitution occurred or is permitted between equivalent Generics but not Brands\r\n\n \n Examples: \n \r\n\n \n Lisnopril (Lupin Corp) for Lisnopril (Wockhardt Corp)"; 191 case TE: return "Description: Substitution occurred or is permitted with another product having the same therapeutic objective and safety profile.\r\n\n \n Examples: \n \r\n\n \n ranitidine for Tagamet"; 192 case TB: return "Description: Substitution occurred or is permitted between therapeutically equivalent Brands but not Generics\r\n>\n Examples: \n \r\n\n \n Zantac for Tagamet"; 193 case TG: return "Description: Substitution occurred or is permitted between therapeutically equivalent Generics but not Brands\r\n>\n Examples: \n \r\n\n \n Ranitidine for cimetidine"; 194 case F: return "Description: This substitution was performed or is permitted based on formulary guidelines."; 195 case N: return "No substitution occurred or is permitted."; 196 case NULL: return null; 197 default: return "?"; 198 } 199 } 200 public String getDisplay() { 201 switch (this) { 202 case _ACTSUBSTANCEADMINSUBSTITUTIONCODE: return "ActSubstanceAdminSubstitutionCode"; 203 case E: return "equivalent"; 204 case EC: return "equivalent composition"; 205 case BC: return "brand composition"; 206 case G: return "generic composition"; 207 case TE: return "therapeutic alternative"; 208 case TB: return "therapeutic brand"; 209 case TG: return "therapeutic generic"; 210 case F: return "formulary"; 211 case N: return "none"; 212 case NULL: return null; 213 default: return "?"; 214 } 215 } 216 217 218}