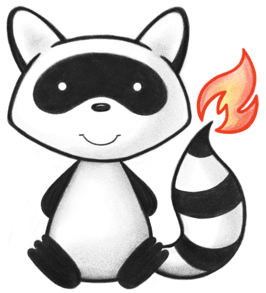
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3TimingEvent { 041 042 /** 043 * before meal (from lat. ante cibus) 044 */ 045 AC, 046 /** 047 * before lunch (from lat. ante cibus diurnus) 048 */ 049 ACD, 050 /** 051 * before breakfast (from lat. ante cibus matutinus) 052 */ 053 ACM, 054 /** 055 * before dinner (from lat. ante cibus vespertinus) 056 */ 057 ACV, 058 /** 059 * Description: meal (from lat. ante cibus) 060 */ 061 C, 062 /** 063 * Description: lunch (from lat. cibus diurnus) 064 */ 065 CD, 066 /** 067 * Description: breakfast (from lat. cibus matutinus) 068 */ 069 CM, 070 /** 071 * Description: dinner (from lat. cibus vespertinus) 072 */ 073 CV, 074 /** 075 * Description: Prior to beginning a regular period of extended sleep (this would exclude naps). Note that this might occur at different times of day depending on a person's regular sleep schedule. 076 */ 077 HS, 078 /** 079 * between meals (from lat. inter cibus) 080 */ 081 IC, 082 /** 083 * between lunch and dinner 084 */ 085 ICD, 086 /** 087 * between breakfast and lunch 088 */ 089 ICM, 090 /** 091 * between dinner and the hour of sleep 092 */ 093 ICV, 094 /** 095 * after meal (from lat. post cibus) 096 */ 097 PC, 098 /** 099 * after lunch (from lat. post cibus diurnus) 100 */ 101 PCD, 102 /** 103 * after breakfast (from lat. post cibus matutinus) 104 */ 105 PCM, 106 /** 107 * after dinner (from lat. post cibus vespertinus) 108 */ 109 PCV, 110 /** 111 * Description: Upon waking up from a regular period of sleep, in order to start regular activities (this would exclude waking up from a nap or temporarily waking up during a period of sleep) 112 113 114 Usage Notes: e.g. 115 116 Take pulse rate on waking in management of thyrotoxicosis. 117 118 Take BP on waking in management of hypertension 119 120 Take basal body temperature on waking in establishing date of ovulation 121 */ 122 WAKE, 123 /** 124 * added to help the parsers 125 */ 126 NULL; 127 public static V3TimingEvent fromCode(String codeString) throws FHIRException { 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("AC".equals(codeString)) 131 return AC; 132 if ("ACD".equals(codeString)) 133 return ACD; 134 if ("ACM".equals(codeString)) 135 return ACM; 136 if ("ACV".equals(codeString)) 137 return ACV; 138 if ("C".equals(codeString)) 139 return C; 140 if ("CD".equals(codeString)) 141 return CD; 142 if ("CM".equals(codeString)) 143 return CM; 144 if ("CV".equals(codeString)) 145 return CV; 146 if ("HS".equals(codeString)) 147 return HS; 148 if ("IC".equals(codeString)) 149 return IC; 150 if ("ICD".equals(codeString)) 151 return ICD; 152 if ("ICM".equals(codeString)) 153 return ICM; 154 if ("ICV".equals(codeString)) 155 return ICV; 156 if ("PC".equals(codeString)) 157 return PC; 158 if ("PCD".equals(codeString)) 159 return PCD; 160 if ("PCM".equals(codeString)) 161 return PCM; 162 if ("PCV".equals(codeString)) 163 return PCV; 164 if ("WAKE".equals(codeString)) 165 return WAKE; 166 throw new FHIRException("Unknown V3TimingEvent code '"+codeString+"'"); 167 } 168 public String toCode() { 169 switch (this) { 170 case AC: return "AC"; 171 case ACD: return "ACD"; 172 case ACM: return "ACM"; 173 case ACV: return "ACV"; 174 case C: return "C"; 175 case CD: return "CD"; 176 case CM: return "CM"; 177 case CV: return "CV"; 178 case HS: return "HS"; 179 case IC: return "IC"; 180 case ICD: return "ICD"; 181 case ICM: return "ICM"; 182 case ICV: return "ICV"; 183 case PC: return "PC"; 184 case PCD: return "PCD"; 185 case PCM: return "PCM"; 186 case PCV: return "PCV"; 187 case WAKE: return "WAKE"; 188 case NULL: return null; 189 default: return "?"; 190 } 191 } 192 public String getSystem() { 193 return "http://hl7.org/fhir/v3/TimingEvent"; 194 } 195 public String getDefinition() { 196 switch (this) { 197 case AC: return "before meal (from lat. ante cibus)"; 198 case ACD: return "before lunch (from lat. ante cibus diurnus)"; 199 case ACM: return "before breakfast (from lat. ante cibus matutinus)"; 200 case ACV: return "before dinner (from lat. ante cibus vespertinus)"; 201 case C: return "Description: meal (from lat. ante cibus)"; 202 case CD: return "Description: lunch (from lat. cibus diurnus)"; 203 case CM: return "Description: breakfast (from lat. cibus matutinus)"; 204 case CV: return "Description: dinner (from lat. cibus vespertinus)"; 205 case HS: return "Description: Prior to beginning a regular period of extended sleep (this would exclude naps). Note that this might occur at different times of day depending on a person's regular sleep schedule."; 206 case IC: return "between meals (from lat. inter cibus)"; 207 case ICD: return "between lunch and dinner"; 208 case ICM: return "between breakfast and lunch"; 209 case ICV: return "between dinner and the hour of sleep"; 210 case PC: return "after meal (from lat. post cibus)"; 211 case PCD: return "after lunch (from lat. post cibus diurnus)"; 212 case PCM: return "after breakfast (from lat. post cibus matutinus)"; 213 case PCV: return "after dinner (from lat. post cibus vespertinus)"; 214 case WAKE: return "Description: Upon waking up from a regular period of sleep, in order to start regular activities (this would exclude waking up from a nap or temporarily waking up during a period of sleep)\r\n\n \n Usage Notes: e.g.\r\n\n Take pulse rate on waking in management of thyrotoxicosis.\r\n\n Take BP on waking in management of hypertension\r\n\n Take basal body temperature on waking in establishing date of ovulation"; 215 case NULL: return null; 216 default: return "?"; 217 } 218 } 219 public String getDisplay() { 220 switch (this) { 221 case AC: return "AC"; 222 case ACD: return "ACD"; 223 case ACM: return "ACM"; 224 case ACV: return "ACV"; 225 case C: return "C"; 226 case CD: return "CD"; 227 case CM: return "CM"; 228 case CV: return "CV"; 229 case HS: return "HS"; 230 case IC: return "IC"; 231 case ICD: return "ICD"; 232 case ICM: return "ICM"; 233 case ICV: return "ICV"; 234 case PC: return "PC"; 235 case PCD: return "PCD"; 236 case PCM: return "PCM"; 237 case PCV: return "PCV"; 238 case WAKE: return "WAKE"; 239 case NULL: return null; 240 default: return "?"; 241 } 242 } 243 244 245}