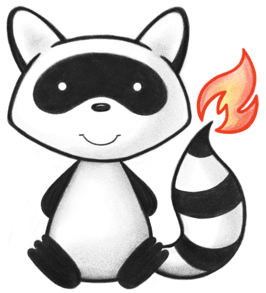
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum V3VaccineManufacturer { 041 042 /** 043 * Abbott Laboratories (includes Ross Products Division) 044 */ 045 AB, 046 /** 047 * Adams Laboratories 048 */ 049 AD, 050 /** 051 * Alpha Therapeutic Corporation 052 */ 053 ALP, 054 /** 055 * Armour [Inactive-use CEN] 056 */ 057 AR, 058 /** 059 * Aviron 060 */ 061 AVI, 062 /** 063 * Baxter Healthcare Corporation 064 */ 065 BA, 066 /** 067 * Bayer Corporation (includes Miles, Inc. and Cutter Laboratories) 068 */ 069 BAY, 070 /** 071 * Berna Products [Inactive-use BPC] 072 */ 073 BP, 074 /** 075 * Berna Products Corporation (includes Swiss Serum and Vaccine Institute Berne) 076 */ 077 BPC, 078 /** 079 * Centeon L.L.C. (includes Armour Pharmaceutical Company) 080 */ 081 CEN, 082 /** 083 * Chiron Corporation 084 */ 085 CHI, 086 /** 087 * Connaught [Inactive-use PMC] 088 */ 089 CON, 090 /** 091 * Evans Medical Limited (an affiliate of Medeva Pharmaceuticals, Inc.) 092 */ 093 EVN, 094 /** 095 * Greer Laboratories, Inc. 096 */ 097 GRE, 098 /** 099 * Immuno International AG 100 */ 101 IAG, 102 /** 103 * Merieux [Inactive-use PMC] 104 */ 105 IM, 106 /** 107 * Immuno-U.S., Inc. 108 */ 109 IUS, 110 /** 111 * The Research Foundation for Microbial Diseases of Osaka University (BIKEN) 112 */ 113 JPN, 114 /** 115 * Korea Green Cross Corporation 116 */ 117 KGC, 118 /** 119 * Lederle [Inactive-use WAL] 120 */ 121 LED, 122 /** 123 * Massachusetts Public Health Biologic Laboratories 124 */ 125 MA, 126 /** 127 * MedImmune, Inc. 128 */ 129 MED, 130 /** 131 * Miles [Inactive-use BAY] 132 */ 133 MIL, 134 /** 135 * Bioport Corporation (formerly Michigan Biologic Products Institute) 136 */ 137 MIP, 138 /** 139 * Merck & Co., Inc. 140 */ 141 MSD, 142 /** 143 * NABI (formerly North American Biologicals, Inc.) 144 */ 145 NAB, 146 /** 147 * North American Vaccine, Inc. 148 */ 149 NAV, 150 /** 151 * Novartis Pharmaceutical Corporation (includes Ciba-Geigy Limited and Sandoz Limited) 152 */ 153 NOV, 154 /** 155 * New York Blood Center 156 */ 157 NYB, 158 /** 159 * Ortho Diagnostic Systems, Inc. 160 */ 161 ORT, 162 /** 163 * Organon Teknika Corporation 164 */ 165 OTC, 166 /** 167 * Parkedale Pharmaceuticals (formerly Parke-Davis) 168 */ 169 PD, 170 /** 171 * Aventis Pasteur Inc. (formerly Pasteur Merieux Connaught; includes Connaught Laboratories and Pasteur Merieux) 172 */ 173 PMC, 174 /** 175 * Praxis Biologics [Inactive-use WAL] 176 */ 177 PRX, 178 /** 179 * Sclavo, Inc. 180 */ 181 SCL, 182 /** 183 * Swiss Serum and Vaccine Inst. [Inactive-use BPC] 184 */ 185 SI, 186 /** 187 * SmithKline Beecham 188 */ 189 SKB, 190 /** 191 * United States Army Medical Research and Materiel Command 192 */ 193 USA, 194 /** 195 * Wyeth-Ayerst [Inactive-use WAL] 196 */ 197 WA, 198 /** 199 * Wyeth-Ayerst (includes Wyeth-Lederle Vaccines and Pediatrics, Wyeth Laboratories, Lederle Laboratories, and Praxis Biologics) 200 */ 201 WAL, 202 /** 203 * added to help the parsers 204 */ 205 NULL; 206 public static V3VaccineManufacturer fromCode(String codeString) throws FHIRException { 207 if (codeString == null || "".equals(codeString)) 208 return null; 209 if ("AB".equals(codeString)) 210 return AB; 211 if ("AD".equals(codeString)) 212 return AD; 213 if ("ALP".equals(codeString)) 214 return ALP; 215 if ("AR".equals(codeString)) 216 return AR; 217 if ("AVI".equals(codeString)) 218 return AVI; 219 if ("BA".equals(codeString)) 220 return BA; 221 if ("BAY".equals(codeString)) 222 return BAY; 223 if ("BP".equals(codeString)) 224 return BP; 225 if ("BPC".equals(codeString)) 226 return BPC; 227 if ("CEN".equals(codeString)) 228 return CEN; 229 if ("CHI".equals(codeString)) 230 return CHI; 231 if ("CON".equals(codeString)) 232 return CON; 233 if ("EVN".equals(codeString)) 234 return EVN; 235 if ("GRE".equals(codeString)) 236 return GRE; 237 if ("IAG".equals(codeString)) 238 return IAG; 239 if ("IM".equals(codeString)) 240 return IM; 241 if ("IUS".equals(codeString)) 242 return IUS; 243 if ("JPN".equals(codeString)) 244 return JPN; 245 if ("KGC".equals(codeString)) 246 return KGC; 247 if ("LED".equals(codeString)) 248 return LED; 249 if ("MA".equals(codeString)) 250 return MA; 251 if ("MED".equals(codeString)) 252 return MED; 253 if ("MIL".equals(codeString)) 254 return MIL; 255 if ("MIP".equals(codeString)) 256 return MIP; 257 if ("MSD".equals(codeString)) 258 return MSD; 259 if ("NAB".equals(codeString)) 260 return NAB; 261 if ("NAV".equals(codeString)) 262 return NAV; 263 if ("NOV".equals(codeString)) 264 return NOV; 265 if ("NYB".equals(codeString)) 266 return NYB; 267 if ("ORT".equals(codeString)) 268 return ORT; 269 if ("OTC".equals(codeString)) 270 return OTC; 271 if ("PD".equals(codeString)) 272 return PD; 273 if ("PMC".equals(codeString)) 274 return PMC; 275 if ("PRX".equals(codeString)) 276 return PRX; 277 if ("SCL".equals(codeString)) 278 return SCL; 279 if ("SI".equals(codeString)) 280 return SI; 281 if ("SKB".equals(codeString)) 282 return SKB; 283 if ("USA".equals(codeString)) 284 return USA; 285 if ("WA".equals(codeString)) 286 return WA; 287 if ("WAL".equals(codeString)) 288 return WAL; 289 throw new FHIRException("Unknown V3VaccineManufacturer code '"+codeString+"'"); 290 } 291 public String toCode() { 292 switch (this) { 293 case AB: return "AB"; 294 case AD: return "AD"; 295 case ALP: return "ALP"; 296 case AR: return "AR"; 297 case AVI: return "AVI"; 298 case BA: return "BA"; 299 case BAY: return "BAY"; 300 case BP: return "BP"; 301 case BPC: return "BPC"; 302 case CEN: return "CEN"; 303 case CHI: return "CHI"; 304 case CON: return "CON"; 305 case EVN: return "EVN"; 306 case GRE: return "GRE"; 307 case IAG: return "IAG"; 308 case IM: return "IM"; 309 case IUS: return "IUS"; 310 case JPN: return "JPN"; 311 case KGC: return "KGC"; 312 case LED: return "LED"; 313 case MA: return "MA"; 314 case MED: return "MED"; 315 case MIL: return "MIL"; 316 case MIP: return "MIP"; 317 case MSD: return "MSD"; 318 case NAB: return "NAB"; 319 case NAV: return "NAV"; 320 case NOV: return "NOV"; 321 case NYB: return "NYB"; 322 case ORT: return "ORT"; 323 case OTC: return "OTC"; 324 case PD: return "PD"; 325 case PMC: return "PMC"; 326 case PRX: return "PRX"; 327 case SCL: return "SCL"; 328 case SI: return "SI"; 329 case SKB: return "SKB"; 330 case USA: return "USA"; 331 case WA: return "WA"; 332 case WAL: return "WAL"; 333 case NULL: return null; 334 default: return "?"; 335 } 336 } 337 public String getSystem() { 338 return "http://hl7.org/fhir/v3/VaccineManufacturer"; 339 } 340 public String getDefinition() { 341 switch (this) { 342 case AB: return "Abbott Laboratories (includes Ross Products Division)"; 343 case AD: return "Adams Laboratories"; 344 case ALP: return "Alpha Therapeutic Corporation"; 345 case AR: return "Armour [Inactive-use CEN]"; 346 case AVI: return "Aviron"; 347 case BA: return "Baxter Healthcare Corporation"; 348 case BAY: return "Bayer Corporation (includes Miles, Inc. and Cutter Laboratories)"; 349 case BP: return "Berna Products [Inactive-use BPC]"; 350 case BPC: return "Berna Products Corporation (includes Swiss Serum and Vaccine Institute Berne)"; 351 case CEN: return "Centeon L.L.C. (includes Armour Pharmaceutical Company)"; 352 case CHI: return "Chiron Corporation"; 353 case CON: return "Connaught [Inactive-use PMC]"; 354 case EVN: return "Evans Medical Limited (an affiliate of Medeva Pharmaceuticals, Inc.)"; 355 case GRE: return "Greer Laboratories, Inc."; 356 case IAG: return "Immuno International AG"; 357 case IM: return "Merieux [Inactive-use PMC]"; 358 case IUS: return "Immuno-U.S., Inc."; 359 case JPN: return "The Research Foundation for Microbial Diseases of Osaka University (BIKEN)"; 360 case KGC: return "Korea Green Cross Corporation"; 361 case LED: return "Lederle [Inactive-use WAL]"; 362 case MA: return "Massachusetts Public Health Biologic Laboratories"; 363 case MED: return "MedImmune, Inc."; 364 case MIL: return "Miles [Inactive-use BAY]"; 365 case MIP: return "Bioport Corporation (formerly Michigan Biologic Products Institute)"; 366 case MSD: return "Merck & Co., Inc."; 367 case NAB: return "NABI (formerly North American Biologicals, Inc.)"; 368 case NAV: return "North American Vaccine, Inc."; 369 case NOV: return "Novartis Pharmaceutical Corporation (includes Ciba-Geigy Limited and Sandoz Limited)"; 370 case NYB: return "New York Blood Center"; 371 case ORT: return "Ortho Diagnostic Systems, Inc."; 372 case OTC: return "Organon Teknika Corporation"; 373 case PD: return "Parkedale Pharmaceuticals (formerly Parke-Davis)"; 374 case PMC: return "Aventis Pasteur Inc. (formerly Pasteur Merieux Connaught; includes Connaught Laboratories and Pasteur Merieux)"; 375 case PRX: return "Praxis Biologics [Inactive-use WAL]"; 376 case SCL: return "Sclavo, Inc."; 377 case SI: return "Swiss Serum and Vaccine Inst. [Inactive-use BPC]"; 378 case SKB: return "SmithKline Beecham"; 379 case USA: return "United States Army Medical Research and Materiel Command"; 380 case WA: return "Wyeth-Ayerst [Inactive-use WAL]"; 381 case WAL: return "Wyeth-Ayerst (includes Wyeth-Lederle Vaccines and Pediatrics, Wyeth Laboratories, Lederle Laboratories, and Praxis Biologics)"; 382 case NULL: return null; 383 default: return "?"; 384 } 385 } 386 public String getDisplay() { 387 switch (this) { 388 case AB: return "Abbott Laboratories (includes Ross Products Division)"; 389 case AD: return "Adams Laboratories"; 390 case ALP: return "Alpha Therapeutic Corporation"; 391 case AR: return "Armour [Inactive - use CEN]"; 392 case AVI: return "Aviron"; 393 case BA: return "Baxter Healthcare Corporation"; 394 case BAY: return "Bayer Corporation (includes Miles, Inc. and Cutter Laboratories)"; 395 case BP: return "Berna Products [Inactive - use BPC]"; 396 case BPC: return "Berna Products Corporation (includes Swiss Serum and Vaccine Institute Berne)"; 397 case CEN: return "Centeon L.L.C. (includes Armour Pharmaceutical Company)"; 398 case CHI: return "Chiron Corporation"; 399 case CON: return "Connaught [Inactive - use PMC]"; 400 case EVN: return "Evans Medical Limited (an affiliate of Medeva Pharmaceuticals, Inc.)"; 401 case GRE: return "Greer Laboratories, Inc."; 402 case IAG: return "Immuno International AG"; 403 case IM: return "Merieux [Inactive - use PMC]"; 404 case IUS: return "Immuno-U.S., Inc."; 405 case JPN: return "The Research Foundation for Microbial Diseases of Osaka University (BIKEN)"; 406 case KGC: return "Korea Green Cross Corporation"; 407 case LED: return "Lederle [Inactive - use WAL]"; 408 case MA: return "Massachusetts Public Health Biologic Laboratories"; 409 case MED: return "MedImmune, Inc."; 410 case MIL: return "Miles [Inactive - use BAY]"; 411 case MIP: return "Bioport Corporation (formerly Michigan Biologic Products Institute)"; 412 case MSD: return "Merck and Co., Inc."; 413 case NAB: return "NABI (formerly North American Biologicals, Inc.)"; 414 case NAV: return "North American Vaccine, Inc."; 415 case NOV: return "Novartis Pharmaceutical Corporation (includes Ciba-Geigy Limited and Sandoz Limited)"; 416 case NYB: return "New York Blood Center"; 417 case ORT: return "Ortho Diagnostic Systems, Inc."; 418 case OTC: return "Organon Teknika Corporation"; 419 case PD: return "Parkedale Pharmaceuticals (formerly Parke-Davis)"; 420 case PMC: return "Aventis Pasteur Inc. (formerly Pasteur Merieux Connaught; includes Connaught Laboratories and Pasteur Merieux)"; 421 case PRX: return "Praxis Biologics [Inactive - use WAL]"; 422 case SCL: return "Sclavo, Inc."; 423 case SI: return "Swiss Serum and Vaccine Inst. [Inactive - use BPC]"; 424 case SKB: return "SmithKline Beecham"; 425 case USA: return "United States Army Medical Research and Materiel Command"; 426 case WA: return "Wyeth-Ayerst [Inactive - use WAL]"; 427 case WAL: return "Wyeth-Ayerst (includes Wyeth-Lederle Vaccines and Pediatrics, Wyeth Laboratories, Lederle Laboratories, and Praxis Biologics)"; 428 case NULL: return null; 429 default: return "?"; 430 } 431 } 432 433 434}