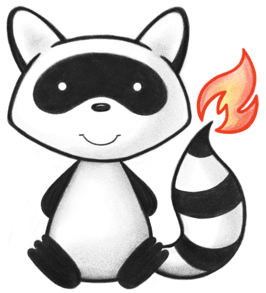
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum W3cProvenanceActivityType { 041 042 /** 043 * The completion of production of a new entity by an activity. This entity did not exist before generation and becomes available for usage after this generation. Given that a generation is the completion of production of an entity, it is instantaneous. 044 */ 045 GENERATION, 046 /** 047 * the beginning of utilizing an entity by an activity. Before usage, the activity had not begun to utilize this entity and could not have been affected by the entity. (Note: This definition is formulated for a given usage; it is permitted for an activity to have used a same entity multiple times.) Given that a usage is the beginning of utilizing an entity, it is instantaneous. 048 */ 049 USAGE, 050 /** 051 * The exchange of some unspecified entity by two activities, one activity using some entity generated by the other. A communication implies that activity a2 is dependent on another activity, a1, by way of some unspecified entity that is generated by a1 and used by a2. 052 */ 053 COMMUNICATION, 054 /** 055 * When an activity is deemed to have been started by an entity, known as trigger. The activity did not exist before its start. Any usage, generation, or invalidation involving an activity follows the activity's start. A start may refer to a trigger entity that set off the activity, or to an activity, known as starter, that generated the trigger. Given that a start is when an activity is deemed to have started, it is instantaneous. 056 */ 057 START, 058 /** 059 * When an activity is deemed to have been ended by an entity, known as trigger. The activity no longer exists after its end. Any usage, generation, or invalidation involving an activity precedes the activity's end. An end may refer to a trigger entity that terminated the activity, or to an activity, known as ender that generated the trigger. 060 */ 061 END, 062 /** 063 * The start of the destruction, cessation, or expiry of an existing entity by an activity. The entity is no longer available for use (or further invalidation) after invalidation. Any generation or usage of an entity precedes its invalidation. Given that an invalidation is the start of destruction, cessation, or expiry, it is instantaneous. 064 */ 065 INVALIDATION, 066 /** 067 * A transformation of an entity into another, an update of an entity resulting in a new one, or the construction of a new entity based on a pre-existing entity. For an entity to be transformed from, created from, or resulting from an update to another, there must be some underpinning activity or activities performing the necessary action(s) resulting in such a derivation. A derivation can be described at various levels of precision. In its simplest form, derivation relates two entities. Optionally, attributes can be added to represent further information about the derivation. If the derivation is the result of a single known activity, then this activity can also be optionally expressed. To provide a completely accurate description of the derivation, the generation and usage of the generated and used entities, respectively, can be provided, so as to make the derivation path, through usage, activity, and generation, explicit. Optional information such as activity, generation, and usage can be linked to derivations to aid analysis of provenance and to facilitate provenance-based reproducibility. 068 */ 069 DERIVATION, 070 /** 071 * A derivation for which the resulting entity is a revised version of some original. The implication here is that the resulting entity contains substantial content from the original. A revision relation is a kind of derivation relation from a revised entity to a preceding entity. 072 */ 073 REVISION, 074 /** 075 * The repeat of (some or all of) an entity, such as text or image, by someone who may or may not be its original author. A quotation relation is a kind of derivation relation, for which an entity was derived from a preceding entity by copying, or 'quoting', some or all of it. 076 */ 077 QUOTATION, 078 /** 079 * Refers to something produced by some agent with direct experience and knowledge about the topic, at the time of the topic's study, without benefit from hindsight. Because of the directness of primary sources, they 'speak for themselves' in ways that cannot be captured through the filter of secondary sources. As such, it is important for secondary sources to reference those primary sources from which they were derived, so that their reliability can be investigated. It is also important to note that a given entity might be a primary source for one entity but not another. It is the reason why Primary Source is defined as a relation as opposed to a subtype of Entity. 080 */ 081 PRIMARYSOURCE, 082 /** 083 * Ascribing of an entity (object/document) to an agent. 084 */ 085 ATTRIBUTION, 086 /** 087 * An aggregating activity that results in composition of an entity, which provides a structure to some constituents that must themselves be entities. These constituents are said to be member of the collections. 088 */ 089 COLLECTION, 090 /** 091 * added to help the parsers 092 */ 093 NULL; 094 public static W3cProvenanceActivityType fromCode(String codeString) throws FHIRException { 095 if (codeString == null || "".equals(codeString)) 096 return null; 097 if ("Generation".equals(codeString)) 098 return GENERATION; 099 if ("Usage".equals(codeString)) 100 return USAGE; 101 if ("Communication".equals(codeString)) 102 return COMMUNICATION; 103 if ("Start".equals(codeString)) 104 return START; 105 if ("End".equals(codeString)) 106 return END; 107 if ("Invalidation".equals(codeString)) 108 return INVALIDATION; 109 if ("Derivation".equals(codeString)) 110 return DERIVATION; 111 if ("Revision".equals(codeString)) 112 return REVISION; 113 if ("Quotation".equals(codeString)) 114 return QUOTATION; 115 if ("Primary-Source".equals(codeString)) 116 return PRIMARYSOURCE; 117 if ("Attribution".equals(codeString)) 118 return ATTRIBUTION; 119 if ("Collection".equals(codeString)) 120 return COLLECTION; 121 throw new FHIRException("Unknown W3cProvenanceActivityType code '"+codeString+"'"); 122 } 123 public String toCode() { 124 switch (this) { 125 case GENERATION: return "Generation"; 126 case USAGE: return "Usage"; 127 case COMMUNICATION: return "Communication"; 128 case START: return "Start"; 129 case END: return "End"; 130 case INVALIDATION: return "Invalidation"; 131 case DERIVATION: return "Derivation"; 132 case REVISION: return "Revision"; 133 case QUOTATION: return "Quotation"; 134 case PRIMARYSOURCE: return "Primary-Source"; 135 case ATTRIBUTION: return "Attribution"; 136 case COLLECTION: return "Collection"; 137 case NULL: return null; 138 default: return "?"; 139 } 140 } 141 public String getSystem() { 142 return "http://hl7.org/fhir/w3c-provenance-activity-type"; 143 } 144 public String getDefinition() { 145 switch (this) { 146 case GENERATION: return "The completion of production of a new entity by an activity. This entity did not exist before generation and becomes available for usage after this generation. Given that a generation is the completion of production of an entity, it is instantaneous."; 147 case USAGE: return "the beginning of utilizing an entity by an activity. Before usage, the activity had not begun to utilize this entity and could not have been affected by the entity. (Note: This definition is formulated for a given usage; it is permitted for an activity to have used a same entity multiple times.) Given that a usage is the beginning of utilizing an entity, it is instantaneous."; 148 case COMMUNICATION: return "The exchange of some unspecified entity by two activities, one activity using some entity generated by the other. A communication implies that activity a2 is dependent on another activity, a1, by way of some unspecified entity that is generated by a1 and used by a2."; 149 case START: return "When an activity is deemed to have been started by an entity, known as trigger. The activity did not exist before its start. Any usage, generation, or invalidation involving an activity follows the activity's start. A start may refer to a trigger entity that set off the activity, or to an activity, known as starter, that generated the trigger. Given that a start is when an activity is deemed to have started, it is instantaneous."; 150 case END: return "When an activity is deemed to have been ended by an entity, known as trigger. The activity no longer exists after its end. Any usage, generation, or invalidation involving an activity precedes the activity's end. An end may refer to a trigger entity that terminated the activity, or to an activity, known as ender that generated the trigger."; 151 case INVALIDATION: return "The start of the destruction, cessation, or expiry of an existing entity by an activity. The entity is no longer available for use (or further invalidation) after invalidation. Any generation or usage of an entity precedes its invalidation. Given that an invalidation is the start of destruction, cessation, or expiry, it is instantaneous."; 152 case DERIVATION: return "A transformation of an entity into another, an update of an entity resulting in a new one, or the construction of a new entity based on a pre-existing entity. For an entity to be transformed from, created from, or resulting from an update to another, there must be some underpinning activity or activities performing the necessary action(s) resulting in such a derivation. A derivation can be described at various levels of precision. In its simplest form, derivation relates two entities. Optionally, attributes can be added to represent further information about the derivation. If the derivation is the result of a single known activity, then this activity can also be optionally expressed. To provide a completely accurate description of the derivation, the generation and usage of the generated and used entities, respectively, can be provided, so as to make the derivation path, through usage, activity, and generation, explicit. Optional information such as activity, generation, and usage can be linked to derivations to aid analysis of provenance and to facilitate provenance-based reproducibility."; 153 case REVISION: return "A derivation for which the resulting entity is a revised version of some original. The implication here is that the resulting entity contains substantial content from the original. A revision relation is a kind of derivation relation from a revised entity to a preceding entity."; 154 case QUOTATION: return "The repeat of (some or all of) an entity, such as text or image, by someone who may or may not be its original author. A quotation relation is a kind of derivation relation, for which an entity was derived from a preceding entity by copying, or 'quoting', some or all of it."; 155 case PRIMARYSOURCE: return "Refers to something produced by some agent with direct experience and knowledge about the topic, at the time of the topic's study, without benefit from hindsight. Because of the directness of primary sources, they 'speak for themselves' in ways that cannot be captured through the filter of secondary sources. As such, it is important for secondary sources to reference those primary sources from which they were derived, so that their reliability can be investigated. It is also important to note that a given entity might be a primary source for one entity but not another. It is the reason why Primary Source is defined as a relation as opposed to a subtype of Entity."; 156 case ATTRIBUTION: return "Ascribing of an entity (object/document) to an agent."; 157 case COLLECTION: return " An aggregating activity that results in composition of an entity, which provides a structure to some constituents that must themselves be entities. These constituents are said to be member of the collections."; 158 case NULL: return null; 159 default: return "?"; 160 } 161 } 162 public String getDisplay() { 163 switch (this) { 164 case GENERATION: return "wasGeneratedBy"; 165 case USAGE: return "used"; 166 case COMMUNICATION: return "wasInformedBy"; 167 case START: return "wasStartedBy"; 168 case END: return "wasEndedBy"; 169 case INVALIDATION: return "wasInvalidatedBy"; 170 case DERIVATION: return "wasDerivedFrom"; 171 case REVISION: return "wasRevisionOf"; 172 case QUOTATION: return "wasQuotedFrom"; 173 case PRIMARYSOURCE: return "wasPrimarySourceOf"; 174 case ATTRIBUTION: return "wasAttributedTo"; 175 case COLLECTION: return "isCollectionOf"; 176 case NULL: return null; 177 default: return "?"; 178 } 179 } 180 181 182}