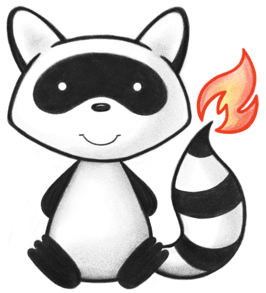
001package org.hl7.fhir.dstu3.terminologies; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import org.hl7.fhir.dstu3.model.CodeSystem.ConceptDefinitionComponent; 035import org.hl7.fhir.dstu3.model.OperationOutcome.IssueSeverity; 036import org.hl7.fhir.dstu3.model.ValueSet; 037import org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent; 038import org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionComponent; 039import org.hl7.fhir.dstu3.terminologies.ValueSetExpander.ValueSetExpansionOutcome; 040 041 042/** 043 * The value set system has a collection of value sets 044 * that define code systems, and construct value sets from 045 * them 046 * 047 * Large external terminologies - LOINC, Snomed, etc - are too big, and 048 * trying to represent their definition as a native value set is too 049 * large. (e.g. LOINC + properties ~ 500MB). So we don't try. Instead. 050 * we assume that there's some external server that provides these 051 * services, using this interface 052 * 053 * The FHIR build tool uses http://fhir.healthintersections.com.au for 054 * these services 055 * 056 * @author Grahame 057 * 058 */ 059@Deprecated 060public interface ITerminologyServices { 061 /** 062 * return true if the service handles code or value set resolution on the system 063 */ 064 public boolean supportsSystem(String system); 065 066 /** 067 * given a system|code, return a definition for it. Nil means not valid 068 */ 069 public ConceptDefinitionComponent getCodeDefinition(String system, String code); 070 071 public class ValidationResult { 072 private IssueSeverity severity; 073 private String message; 074 public ValidationResult(IssueSeverity severity, String message) { 075 super(); 076 this.severity = severity; 077 this.message = message; 078 } 079 public IssueSeverity getSeverity() { 080 return severity; 081 } 082 public String getMessage() { 083 return message; 084 } 085 086 087 } 088 089 /** 090 * for this system|code and display, validate the triple against the rules of 091 * the underlying code system 092 */ 093 public ValidationResult validateCode(String system, String code, String display); 094 095 /** 096 * Expand the value set fragment (system | codes | filters). Note that this 097 * might fail if the expansion is very large. If the expansion fails, then the 098 * checkVS will be called instead 099 */ 100 public ValueSetExpansionComponent expandVS(ConceptSetComponent inc) throws Exception; 101// public ValueSet expandVS(ValueSet vs) throws Exception; 102 public ValueSetExpansionOutcome expand(ValueSet vs); 103 104 /** 105 * Test the value set fragment (system | codes | filters). 106 */ 107 public boolean checkVS(ConceptSetComponent vsi, String system, String code); 108 109 public boolean verifiesSystem(String system); 110 111 112 113}