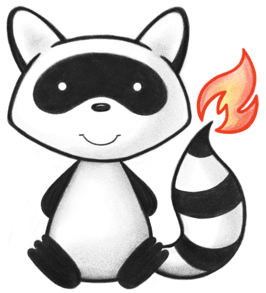
001package org.hl7.fhir.dstu3.terminologies; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.util.List; 035 036import org.hl7.fhir.dstu3.context.IWorkerContext; 037import org.hl7.fhir.dstu3.context.IWorkerContext.ValidationResult; 038import org.hl7.fhir.dstu3.model.CodeSystem; 039import org.hl7.fhir.dstu3.model.CodeSystem.CodeSystemContentMode; 040import org.hl7.fhir.dstu3.model.CodeSystem.ConceptDefinitionComponent; 041import org.hl7.fhir.dstu3.model.UriType; 042import org.hl7.fhir.dstu3.model.ValueSet; 043import org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceComponent; 044import org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent; 045import org.hl7.fhir.dstu3.model.ValueSet.ConceptSetFilterComponent; 046import org.hl7.fhir.dstu3.model.ValueSet.ValueSetExpansionContainsComponent; 047import org.hl7.fhir.dstu3.terminologies.ValueSetExpander.ValueSetExpansionOutcome; 048import org.hl7.fhir.dstu3.utils.EOperationOutcome; 049 050@Deprecated 051public class ValueSetCheckerSimple implements ValueSetChecker { 052 053 private ValueSet valueset; 054 private ValueSetExpanderFactory factory; 055 private IWorkerContext context; 056 057 public ValueSetCheckerSimple(ValueSet source, ValueSetExpanderFactory factory, IWorkerContext context) { 058 this.valueset = source; 059 this.factory = factory; 060 this.context = context; 061 } 062 063 @Override 064 public boolean codeInValueSet(String system, String code) throws EOperationOutcome, Exception { 065 066 if (valueset.hasCompose()) { 067 boolean ok = false; 068 for (ConceptSetComponent vsi : valueset.getCompose().getInclude()) { 069 ok = ok || inComponent(vsi, system, code); 070 } 071 for (ConceptSetComponent vsi : valueset.getCompose().getExclude()) { 072 ok = ok && !inComponent(vsi, system, code); 073 } 074 } 075 076 return false; 077 } 078 079 private boolean inImport(String uri, String system, String code) throws EOperationOutcome, Exception { 080 ValueSet vs = context.fetchResource(ValueSet.class, uri); 081 if (vs == null) 082 return false ; // we can't tell 083 return codeInExpansion(factory.getExpander().expand(vs, null), system, code); 084 } 085 086 private boolean codeInExpansion(ValueSetExpansionOutcome vso, String system, String code) throws EOperationOutcome, Exception { 087 if (vso.getService() != null) { 088 return vso.getService().codeInValueSet(system, code); 089 } else { 090 for (ValueSetExpansionContainsComponent c : vso.getValueset().getExpansion().getContains()) { 091 if (code.equals(c.getCode()) && (system == null || system.equals(c.getSystem()))) 092 return true; 093 if (codeinExpansion(c, system, code)) 094 return true; 095 } 096 } 097 return false; 098 } 099 100 private boolean codeinExpansion(ValueSetExpansionContainsComponent cnt, String system, String code) { 101 for (ValueSetExpansionContainsComponent c : cnt.getContains()) { 102 if (code.equals(c.getCode()) && system.equals(c.getSystem().toString())) 103 return true; 104 if (codeinExpansion(c, system, code)) 105 return true; 106 } 107 return false; 108 } 109 110 111 private boolean inComponent(ConceptSetComponent vsi, String system, String code) throws Exception { 112 if (vsi.hasSystem() && !vsi.getSystem().equals(system)) 113 return false; 114 115 for (UriType uri : vsi.getValueSet()) { 116 if (!inImport(uri.getValue(), system, code)) 117 return false; 118 } 119 120 if (!vsi.hasSystem()) 121 return false; 122 123 // whether we know the system or not, we'll accept the stated codes at face value 124 for (ConceptReferenceComponent cc : vsi.getConcept()) 125 if (cc.getCode().equals(code)) { 126 return true; 127 } 128 129 CodeSystem def = context.fetchCodeSystem(system); 130 if (def != null && def.getContent() == CodeSystemContentMode.COMPLETE) { 131 if (!def.getCaseSensitive()) { 132 // well, ok, it's not case sensitive - we'll check that too now 133 for (ConceptReferenceComponent cc : vsi.getConcept()) 134 if (cc.getCode().equalsIgnoreCase(code)) { 135 return false; 136 } 137 } 138 if (vsi.getConcept().isEmpty() && vsi.getFilter().isEmpty()) { 139 return codeInDefine(def.getConcept(), code, def.getCaseSensitive()); 140 } 141 for (ConceptSetFilterComponent f: vsi.getFilter()) 142 throw new Error("not done yet: "+f.getValue()); 143 144 return false; 145 } else if (context.supportsSystem(system)) { 146 ValidationResult vv = context.validateCode(system, code, null, vsi); 147 return vv.isOk(); 148 } else 149 // we don't know this system, and can't resolve it 150 return false; 151 } 152 153 private boolean codeInDefine(List<ConceptDefinitionComponent> concepts, String code, boolean caseSensitive) { 154 for (ConceptDefinitionComponent c : concepts) { 155 if (caseSensitive && code.equals(c.getCode())) 156 return true; 157 if (!caseSensitive && code.equalsIgnoreCase(c.getCode())) 158 return true; 159 if (codeInDefine(c.getConcept(), code, caseSensitive)) 160 return true; 161 } 162 return false; 163 } 164 165}