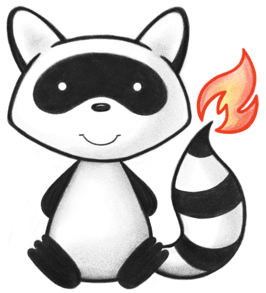
001package org.hl7.fhir.dstu3.terminologies; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.io.FileNotFoundException; 035import java.io.IOException; 036 037import org.hl7.fhir.dstu3.model.ExpansionProfile; 038import org.hl7.fhir.dstu3.model.ValueSet; 039 040@Deprecated 041public interface ValueSetExpander { 042 public enum TerminologyServiceErrorClass { 043 UNKNOWN, NOSERVICE, SERVER_ERROR, VALUESET_UNSUPPORTED; 044 045 public boolean isInfrastructure() { 046 return this == NOSERVICE || this == SERVER_ERROR || this == VALUESET_UNSUPPORTED; 047 } 048 } 049 050 public class ETooCostly extends Exception { 051 052 public ETooCostly(String msg) { 053 super(msg); 054 } 055 056 } 057 058 /** 059 * Some value sets are just too big to expand. Instead of an expanded value set, 060 * you get back an interface that can test membership - usually on a server somewhere 061 * 062 * @author Grahame 063 */ 064 public class ValueSetExpansionOutcome { 065 private ValueSet valueset; 066 private ValueSetChecker service; 067 private String error; 068 private TerminologyServiceErrorClass errorClass; 069 070 public ValueSetExpansionOutcome(ValueSet valueset) { 071 super(); 072 this.valueset = valueset; 073 this.service = null; 074 this.error = null; 075 } 076 public ValueSetExpansionOutcome(ValueSet valueset, String error, TerminologyServiceErrorClass errorClass) { 077 super(); 078 this.valueset = valueset; 079 this.service = null; 080 this.error = error; 081 this.errorClass = errorClass; 082 } 083 public ValueSetExpansionOutcome(ValueSetChecker service, String error, TerminologyServiceErrorClass errorClass) { 084 super(); 085 this.valueset = null; 086 this.service = service; 087 this.error = error; 088 this.errorClass = errorClass; 089 } 090 public ValueSetExpansionOutcome(String error, TerminologyServiceErrorClass errorClass) { 091 this.valueset = null; 092 this.service = null; 093 this.error = error; 094 this.errorClass = errorClass; 095 } 096 public ValueSet getValueset() { 097 return valueset; 098 } 099 public ValueSetChecker getService() { 100 return service; 101 } 102 public String getError() { 103 return error; 104 } 105 public TerminologyServiceErrorClass getErrorClass() { 106 return errorClass; 107 } 108 109 110 } 111/** 112 * 113 * @param source the value set definition to expand 114 * @param profile a profile affecting the outcome. If you don't supply a profile, the default internal expansion profile will be used. 115 * 116 * @return 117 * @throws ETooCostly 118 * @throws FileNotFoundException 119 * @throws IOException 120 */ 121 public ValueSetExpansionOutcome expand(ValueSet source, ExpansionProfile profile) throws ETooCostly, FileNotFoundException, IOException; 122}