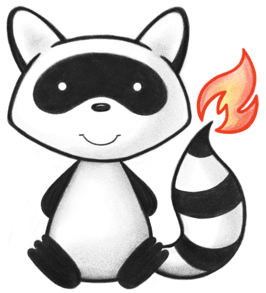
001package org.hl7.fhir.dstu3.terminologies; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034/* 035Copyright (c) 2011+, HL7, Inc 036All rights reserved. 037 038Redistribution and use in source and binary forms, with or without modification, 039are permitted provided that the following conditions are met: 040 041 * Redistributions of source code must retain the above copyright notice, this 042 list of conditions and the following disclaimer. 043 * Redistributions in binary form must reproduce the above copyright notice, 044 this list of conditions and the following disclaimer in the documentation 045 and/or other materials provided with the distribution. 046 * Neither the name of HL7 nor the names of its contributors may be used to 047 endorse or promote products derived from this software without specific 048 prior written permission. 049 050THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 051ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 052WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 053IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 054INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 055NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 056PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 057WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 058ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 059POSSIBILITY OF SUCH DAMAGE. 060 061*/ 062 063import java.io.File; 064import java.io.FileInputStream; 065import java.io.FileOutputStream; 066import java.io.IOException; 067import java.util.HashMap; 068import java.util.Map; 069 070import org.apache.commons.io.IOUtils; 071import org.hl7.fhir.dstu3.context.IWorkerContext; 072import org.hl7.fhir.dstu3.formats.IParser.OutputStyle; 073import org.hl7.fhir.dstu3.model.ExpansionProfile; 074import org.hl7.fhir.dstu3.model.OperationOutcome; 075import org.hl7.fhir.dstu3.model.Resource; 076import org.hl7.fhir.dstu3.model.ValueSet; 077import org.hl7.fhir.dstu3.terminologies.ValueSetExpander.TerminologyServiceErrorClass; 078import org.hl7.fhir.dstu3.terminologies.ValueSetExpander.ValueSetExpansionOutcome; 079import org.hl7.fhir.dstu3.utils.ToolingExtensions; 080import org.hl7.fhir.exceptions.FHIRFormatError; 081import org.hl7.fhir.utilities.Utilities; 082import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 083import org.hl7.fhir.utilities.xhtml.XhtmlComposer; 084 085@Deprecated 086public class ValueSetExpansionCache implements ValueSetExpanderFactory { 087 088 public class CacheAwareExpander implements ValueSetExpander { 089 090 @Override 091 public ValueSetExpansionOutcome expand(ValueSet source, ExpansionProfile profile) throws ETooCostly, IOException { 092 String cacheKey = makeCacheKey(source, profile); 093 if (expansions.containsKey(cacheKey)) 094 return expansions.get(cacheKey); 095 ValueSetExpander vse = new ValueSetExpanderSimple(context, ValueSetExpansionCache.this); 096 ValueSetExpansionOutcome vso = vse.expand(source, profile); 097 if (vso.getError() != null) { 098 // well, we'll see if the designated server can expand it, and if it can, we'll cache it locally 099 vso = context.expandVS(source, false, profile == null || !profile.getExcludeNested()); 100 if (cacheFolder != null) { 101 FileOutputStream s = ManagedFileAccess.outStream(Utilities.path(cacheFolder, makeFile(source.getUrl()))); 102 context.newXmlParser().setOutputStyle(OutputStyle.PRETTY).compose(s, vso.getValueset()); 103 s.close(); 104 } 105 } 106 if (vso.getValueset() != null) 107 expansions.put(cacheKey, vso); 108 return vso; 109 } 110 111 private String makeCacheKey(ValueSet source, ExpansionProfile profile) { 112 return profile == null ? source.getUrl() : source.getUrl() + " " + profile.getUrl()+" "+profile.getExcludeNested(); 113 } 114 115 private String makeFile(String url) { 116 return url.replace("$", "").replace(":", "").replace("//", "/").replace("/", "_")+".xml"; 117 } 118 } 119 120 private static final String VS_ID_EXT = "http://tools/cache"; 121 122 private final Map<String, ValueSetExpansionOutcome> expansions = new HashMap<String, ValueSetExpansionOutcome>(); 123 private final IWorkerContext context; 124 private final String cacheFolder; 125 126 public ValueSetExpansionCache(IWorkerContext context) { 127 super(); 128 cacheFolder = null; 129 this.context = context; 130 } 131 132 public ValueSetExpansionCache(IWorkerContext context, String cacheFolder) throws FHIRFormatError, IOException { 133 super(); 134 this.context = context; 135 this.cacheFolder = cacheFolder; 136 if (this.cacheFolder != null) 137 loadCache(); 138 } 139 140 private void loadCache() throws FHIRFormatError, IOException { 141 File[] files = ManagedFileAccess.file(cacheFolder).listFiles(); 142 for (File f : files) { 143 if (f.getName().endsWith(".xml")) { 144 final FileInputStream is = ManagedFileAccess.inStream(f); 145 try { 146 Resource r = context.newXmlParser().setOutputStyle(OutputStyle.PRETTY).parse(is); 147 if (r instanceof OperationOutcome) { 148 OperationOutcome oo = (OperationOutcome) r; 149 expansions.put(ToolingExtensions.getExtension(oo,VS_ID_EXT).getValue().toString(), 150 new ValueSetExpansionOutcome(new XhtmlComposer(XhtmlComposer.XML).composePlainText(oo.getText().getDiv()), TerminologyServiceErrorClass.UNKNOWN)); 151 } else { 152 ValueSet vs = (ValueSet) r; 153 expansions.put(vs.getUrl(), new ValueSetExpansionOutcome(vs)); 154 } 155 } finally { 156 IOUtils.closeQuietly(is); 157 } 158 } 159 } 160 } 161 162 @Override 163 public ValueSetExpander getExpander() { 164 return new CacheAwareExpander(); 165 // return new ValueSetExpander(valuesets, codesystems); 166 } 167 168}