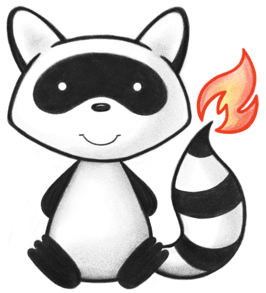
001package org.hl7.fhir.dstu3.utils; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.util.ArrayList; 035import java.util.HashMap; 036import java.util.List; 037import java.util.Map; 038 039import org.hl7.fhir.dstu3.context.IWorkerContext; 040import org.hl7.fhir.dstu3.model.ElementDefinition; 041import org.hl7.fhir.dstu3.model.ElementDefinition.TypeRefComponent; 042import org.hl7.fhir.dstu3.model.StructureDefinition; 043import org.hl7.fhir.exceptions.DefinitionException; 044 045@Deprecated 046public class DefinitionNavigator { 047 048 private IWorkerContext context; 049 private StructureDefinition structure; 050 private int index; 051 private List<DefinitionNavigator> children; 052 private List<DefinitionNavigator> typeChildren; 053 private List<DefinitionNavigator> slices; 054 private List<String> names = new ArrayList<String>(); 055 private TypeRefComponent typeOfChildren; 056 private String path; 057 058 public DefinitionNavigator(IWorkerContext context, StructureDefinition structure) throws DefinitionException { 059 if (!structure.hasSnapshot()) 060 throw new DefinitionException("Snapshot required"); 061 this.context = context; 062 this.structure = structure; 063 this.index = 0; 064 this.path = current().getPath(); 065 names.add(nameTail()); 066 } 067 068 private DefinitionNavigator(IWorkerContext context, StructureDefinition structure, int index, String path, List<String> names, String type) { 069 this.path = path; 070 this.context = context; 071 this.structure = structure; 072 this.index = index; 073 if (type == null) 074 for (String name : names) 075 this.names.add(name+"."+nameTail()); 076 else { 077 this.names.addAll(names); 078 this.names.add(type); 079 } 080 } 081 082 /** 083 * When you walk a tree, and you walk into a typed structure, an element can simultaineously 084 * be covered by multiple types at once. Take, for example, the string label for an identifer value. 085 * It has the following paths: 086 * Patient.identifier.value.value 087 * Identifier.value.value 088 * String.value 089 * value 090 * If you started in a bundle, the list might be even longer and deeper 091 * 092 * Any of these names might be relevant. This function returns the names in an ordered list 093 * in the order above 094 * @return 095 */ 096 public List<String> getNames() { 097 return names; 098 } 099 public ElementDefinition current() { 100 return structure.getSnapshot().getElement().get(index); 101 } 102 103 public List<DefinitionNavigator> slices() throws DefinitionException { 104 if (children == null) { 105 loadChildren(); 106 } 107 return slices; 108 } 109 110 public List<DefinitionNavigator> children() throws DefinitionException { 111 if (children == null) { 112 loadChildren(); 113 } 114 return children; 115 } 116 117 private void loadChildren() throws DefinitionException { 118 children = new ArrayList<DefinitionNavigator>(); 119 String prefix = current().getPath()+"."; 120 Map<String, DefinitionNavigator> nameMap = new HashMap<String, DefinitionNavigator>(); 121 122 for (int i = index + 1; i < structure.getSnapshot().getElement().size(); i++) { 123 String path = structure.getSnapshot().getElement().get(i).getPath(); 124 if (path.startsWith(prefix) && !path.substring(prefix.length()).contains(".")) { 125 DefinitionNavigator dn = new DefinitionNavigator(context, structure, i, this.path+"."+tail(path), names, null); 126 127 if (nameMap.containsKey(path)) { 128 DefinitionNavigator master = nameMap.get(path); 129 if (!master.current().hasSlicing()) 130 throw new DefinitionException("Found slices with no slicing details at "+dn.current().getPath()); 131 if (master.slices == null) 132 master.slices = new ArrayList<DefinitionNavigator>(); 133 master.slices.add(dn); 134 } else { 135 nameMap.put(path, dn); 136 children.add(dn); 137 } 138 } else if (path.length() < prefix.length()) 139 break; 140 } 141 } 142 143 public String path() { 144 return path; 145 } 146 147 private String tail(String p) { 148 if (p.contains(".")) 149 return p.substring(p.lastIndexOf('.')+1); 150 else 151 return p; 152 } 153 154 public String nameTail() { 155 return tail(path); 156 } 157 158 /** 159 * if you have a typed element, the tree might end at that point. 160 * And you may or may not want to walk into the tree of that type 161 * It depends what you are doing. So this is a choice. You can 162 * ask for the children, and then, if you get no children, you 163 * can see if there are children defined for the type, and then 164 * get them 165 * 166 * you have to provide a type if there's more than one type 167 * for current() since this library doesn't know how to choose 168 * @throws DefinitionException 169 * @ 170 */ 171 public boolean hasTypeChildren(TypeRefComponent type) throws DefinitionException { 172 if (typeChildren == null || typeOfChildren != type) { 173 loadTypedChildren(type); 174 } 175 return !typeChildren.isEmpty(); 176 } 177 178 private void loadTypedChildren(TypeRefComponent type) throws DefinitionException { 179 typeOfChildren = null; 180 StructureDefinition sd = context.fetchResource(StructureDefinition.class, type.hasProfile() ? type.getProfile() : type.getCode()); 181 if (sd != null) { 182 DefinitionNavigator dn = new DefinitionNavigator(context, sd, 0, path, names, sd.getType()); 183 typeChildren = dn.children(); 184 } else 185 throw new DefinitionException("Unable to find definition for "+type.getCode()+(type.hasProfile() ? "("+type.getProfile()+")" : "")); 186 typeOfChildren = type; 187 } 188 189 /** 190 * 191 * @return 192 * @throws DefinitionException 193 * @ 194 */ 195 public List<DefinitionNavigator> childrenFromType(TypeRefComponent type) throws DefinitionException { 196 if (typeChildren == null || typeOfChildren != type) { 197 loadTypedChildren(type); 198 } 199 return typeChildren; 200 } 201 202 203}