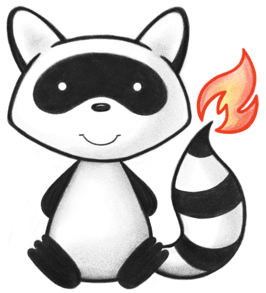
001package org.hl7.fhir.dstu3.utils; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import org.hl7.fhir.dstu3.model.CodeableConcept; 035import org.hl7.fhir.dstu3.model.OperationOutcome; 036import org.hl7.fhir.dstu3.model.OperationOutcome.IssueSeverity; 037import org.hl7.fhir.dstu3.model.OperationOutcome.IssueType; 038import org.hl7.fhir.dstu3.model.OperationOutcome.OperationOutcomeIssueComponent; 039import org.hl7.fhir.dstu3.model.StringType; 040import org.hl7.fhir.utilities.Utilities; 041import org.hl7.fhir.utilities.validation.ValidationMessage; 042 043@Deprecated 044public class OperationOutcomeUtilities { 045 046 047 public static OperationOutcomeIssueComponent convertToIssue(ValidationMessage message, OperationOutcome op) { 048 OperationOutcomeIssueComponent issue = new OperationOutcome.OperationOutcomeIssueComponent(); 049 issue.setCode(convert(message.getType())); 050 if (message.getLocation() != null) { 051 // message location has a fhirPath in it. We need to populate the expression 052 issue.addExpression(message.getLocation()); 053 // also, populate the XPath variant 054 StringType s = new StringType(); 055 s.setValue(Utilities.fhirPathToXPath(message.getLocation())+(message.getLine()>= 0 && message.getCol() >= 0 ? " (line "+Integer.toString(message.getLine())+", col"+Integer.toString(message.getCol())+")" : "") ); 056 issue.getLocation().add(s); 057 } 058 issue.setSeverity(convert(message.getLevel())); 059 CodeableConcept c = new CodeableConcept(); 060 c.setText(message.getMessage()); 061 issue.setDetails(c); 062 if (message.getSource() != null) { 063 issue.getExtension().add(ToolingExtensions.makeIssueSource(message.getSource())); 064 } 065 return issue; 066 } 067 068 private static IssueSeverity convert(org.hl7.fhir.utilities.validation.ValidationMessage.IssueSeverity level) { 069 switch (level) { 070 case FATAL : return IssueSeverity.FATAL; 071 case ERROR : return IssueSeverity.ERROR; 072 case WARNING : return IssueSeverity.WARNING; 073 case INFORMATION : return IssueSeverity.INFORMATION; 074 } 075 return IssueSeverity.NULL; 076 } 077 078 private static IssueType convert(org.hl7.fhir.utilities.validation.ValidationMessage.IssueType type) { 079 switch (type) { 080 case INVALID: 081 case STRUCTURE: return IssueType.STRUCTURE; 082 case REQUIRED: return IssueType.REQUIRED; 083 case VALUE: return IssueType.VALUE; 084 case INVARIANT: return IssueType.INVARIANT; 085 case SECURITY: return IssueType.SECURITY; 086 case LOGIN: return IssueType.LOGIN; 087 case UNKNOWN: return IssueType.UNKNOWN; 088 case EXPIRED: return IssueType.EXPIRED; 089 case FORBIDDEN: return IssueType.FORBIDDEN; 090 case SUPPRESSED: return IssueType.SUPPRESSED; 091 case PROCESSING: return IssueType.PROCESSING; 092 case NOTSUPPORTED: return IssueType.NOTSUPPORTED; 093 case DUPLICATE: return IssueType.DUPLICATE; 094 case NOTFOUND: return IssueType.NOTFOUND; 095 case TOOLONG: return IssueType.TOOLONG; 096 case CODEINVALID: return IssueType.CODEINVALID; 097 case EXTENSION: return IssueType.EXTENSION; 098 case TOOCOSTLY: return IssueType.TOOCOSTLY; 099 case BUSINESSRULE: return IssueType.BUSINESSRULE; 100 case CONFLICT: return IssueType.CONFLICT; 101 case INCOMPLETE: return IssueType.INCOMPLETE; 102 case TRANSIENT: return IssueType.TRANSIENT; 103 case LOCKERROR: return IssueType.LOCKERROR; 104 case NOSTORE: return IssueType.NOSTORE; 105 case EXCEPTION: return IssueType.EXCEPTION; 106 case TIMEOUT: return IssueType.TIMEOUT; 107 case THROTTLED: return IssueType.THROTTLED; 108 case INFORMATIONAL: return IssueType.INFORMATIONAL; 109 } 110 return IssueType.NULL; 111 } 112}