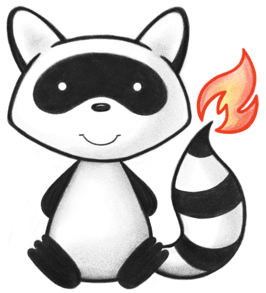
001package org.hl7.fhir.dstu3.utils; 002 003import java.io.File; 004import java.io.FileInputStream; 005import java.io.FileNotFoundException; 006import java.io.FileOutputStream; 007import java.io.IOException; 008import java.util.ArrayList; 009import java.util.HashMap; 010import java.util.List; 011import java.util.Map; 012 013import org.hl7.fhir.dstu3.formats.IParser.OutputStyle; 014import org.hl7.fhir.dstu3.formats.JsonParser; 015import org.hl7.fhir.dstu3.formats.XmlParser; 016import org.hl7.fhir.dstu3.model.Bundle; 017import org.hl7.fhir.dstu3.model.Bundle.BundleEntryComponent; 018import org.hl7.fhir.dstu3.model.DomainResource; 019import org.hl7.fhir.dstu3.model.Resource; 020import org.hl7.fhir.exceptions.FHIRFormatError; 021import org.hl7.fhir.utilities.FileUtilities; 022import org.hl7.fhir.utilities.Utilities; 023import org.hl7.fhir.utilities.filesystem.ManagedFileAccess; 024 025@Deprecated 026@SuppressWarnings("checkstyle:systemout") 027public class R3TEchnicalCorrectionProcessor { 028 029 public static void main(String[] args) throws FileNotFoundException, IOException { 030 new R3TEchnicalCorrectionProcessor().execute(args[0], args[1]); 031 032 } 033 034 private void execute(String src, String packageRoot) throws FileNotFoundException, IOException { 035 System.out.println("Loading resources from "+src); 036 List<Resource> resources = new ArrayList<>(); 037 Map<String, Resource> definitions = new HashMap<>(); 038 for (File f : ManagedFileAccess.file(src).listFiles()) { 039 if (f.getName().endsWith(".xml") && !(f.getName().endsWith("warnings.xml") || f.getName().endsWith(".diff.xml"))) { 040 try { 041 Resource r = new XmlParser().parse(ManagedFileAccess.inStream(f)); 042 if (f.getName().contains("canonical")) { 043 resources.add(r); 044 } 045 if (Utilities.existsInList(f.getName(), "conceptmaps.xml", "dataelements.xml", "extension-definitions.xml", "profiles-others.xml", "profiles-resources.xml", 046 "profiles-types.xml", "search-parameters.xml", "v2-tables.xml", "v3-codesystems.xml", "valuesets.xml")) { 047 definitions.put(f.getName(), r); 048 } 049 r.setUserData("path", f.getName().substring(0, f.getName().indexOf("."))); 050// FileUtils.copyFile(f, ManagedFileAccess.file(f.getAbsolutePath()+"1")); 051// FileUtils.copyFile(f, ManagedFileAccess.file(f.getAbsolutePath()+"2")); 052 } catch (Exception e) { 053 System.out.println("Unable to load "+f.getName()+": "+e.getMessage()); 054 } 055 } 056 if (f.getName().endsWith(".json") && !(f.getName().endsWith("schema.json") || f.getName().endsWith(".diff.json"))) { 057 try { 058// new JsonParser().parse(ManagedFileAccess.inStream(f)); 059// FileUtils.copyFile(f, ManagedFileAccess.file(f.getAbsolutePath()+"1")); 060// FileUtils.copyFile(f, ManagedFileAccess.file(f.getAbsolutePath()+"2")); 061 } catch (Exception e) { 062 System.out.println("Unable to load "+f.getName()+": "+e.getMessage()); 063 } 064 } 065 } 066 System.out.println(Integer.toString(resources.size())+" resources"); 067 System.out.println(Integer.toString(definitions.size())+" resources"); 068 produceExamplesXml(resources, src); 069 produceDefinitionsXml(definitions, src); 070 produceExamplesJson(resources, src); 071 produceDefinitionsJson(definitions, src); 072 for (Resource r : definitions.values()) { 073 if (r instanceof Bundle) { 074 Bundle bnd = (Bundle) r; 075 for (BundleEntryComponent be : bnd.getEntry()) { 076 resources.add(be.getResource()); 077 } 078 } 079 } 080 extractToPackageMaster(resources, packageRoot); 081 System.out.println("Done"); 082 } 083 084 private void produceDefinitionsXml(Map<String, Resource> definitions, String dest) throws IOException { 085 for (String n : definitions.keySet()) { 086 File f = ManagedFileAccess.file(Utilities.path(dest, "definitions.xml", n)); 087 new XmlParser().setOutputStyle(OutputStyle.PRETTY).compose(ManagedFileAccess.outStream(f), definitions.get(n)); 088 } 089 } 090 091 private void produceDefinitionsJson(Map<String, Resource> definitions, String dest) throws IOException { 092 for (String n : definitions.keySet()) { 093 File f = ManagedFileAccess.file(Utilities.path(dest, "definitions.json", FileUtilities.changeFileExt(n, ".json"))); 094 new JsonParser().setOutputStyle(OutputStyle.PRETTY).compose(ManagedFileAccess.outStream(f), definitions.get(n)); 095 } 096 } 097 098 private void produceExamplesJson(List<Resource> resources, String dest) throws FileNotFoundException, IOException { 099 for (Resource r : resources) { 100 String n = r.fhirType().toLowerCase()+"-"+r.getUserString("path"); 101 if (!r.getId().equals(r.getUserString("path"))) { 102 n = n+"("+r.getId()+")"; 103 } 104 File f = ManagedFileAccess.file(Utilities.path(dest, "examples-json", n+".json")); 105 new JsonParser().setOutputStyle(OutputStyle.PRETTY).compose(ManagedFileAccess.outStream(f), r); 106 } 107 108 } 109 110 private void produceExamplesXml(List<Resource> resources, String dest) throws FileNotFoundException, IOException { 111 for (Resource r : resources) { 112 String n = r.fhirType().toLowerCase()+"-"+r.getUserString("path"); 113 if (!r.getId().equals(r.getUserString("path"))) { 114 n = n+"("+r.getId()+")"; 115 } 116 File f = ManagedFileAccess.file(Utilities.path(dest, "examples", n+".xml")); 117 new XmlParser().setOutputStyle(OutputStyle.PRETTY).compose(ManagedFileAccess.outStream(f), r); 118 } 119 } 120 121 private void extractToPackageMaster(List<Resource> list, String root) throws IOException, FHIRFormatError { 122 System.out.println("Updating Packages Master"); 123 String corePath = Utilities.path(root, "hl7.fhir.r3.core", "package"); 124 String examplesPath = Utilities.path(root, "hl7.fhir.r3.examples", "package"); 125 String elementsPath = Utilities.path(root, "hl7.fhir.r3.elements", "package"); 126 int coreTotal = ManagedFileAccess.file(corePath).list().length-1; 127 int examplesTotal = ManagedFileAccess.file(examplesPath).list().length-1; 128 int elementsTotal = ManagedFileAccess.file(elementsPath).list().length-1; 129 130 int coreCount = 0; 131 int examplesCount = 0; 132 int elementsCount = 0; 133 for (Resource r : list) { 134 String n = r.fhirType()+"-"+r.getId()+".json"; 135 FileOutputStream dst = null; 136 if (n.startsWith("DataElement-")) { 137 elementsCount++; 138 dst = ManagedFileAccess.outStream(Utilities.path(elementsPath, n)); 139 new JsonParser().setOutputStyle(OutputStyle.NORMAL).compose(dst, r); 140 } else { 141 dst = ManagedFileAccess.outStream(Utilities.path(examplesPath, n)); 142 new JsonParser().setOutputStyle(OutputStyle.NORMAL).compose(dst, r); 143 examplesCount++; 144 if (isCoreResource(r.fhirType())) { 145 coreCount++; 146 DomainResource dr = (DomainResource) r; 147 dr.setText(null); 148 new JsonParser().setOutputStyle(OutputStyle.NORMAL).compose(ManagedFileAccess.outStream(Utilities.path(corePath, n)), r); 149 } 150 } 151 } 152 System.out.println(" Core @ "+corePath+": Replaced "+coreCount+" of "+coreTotal); 153 System.out.println(" Examples @ "+examplesPath+": Replaced "+examplesCount+" of "+examplesTotal); 154 System.out.println(" Elements @ "+elementsPath+": Replaced "+elementsCount+" of "+elementsTotal); 155 } 156 157 private boolean isCoreResource(String rt) { 158 return Utilities.existsInList(rt, "CapabilityStatement", "CodeSystem", "CompartmentDefinition", "ConceptMap", "NamingSystem", "OperationDefinition", "SearchParameter", "StructureDefinition", "StructureMap", "ValueSet"); 159 } 160 161}