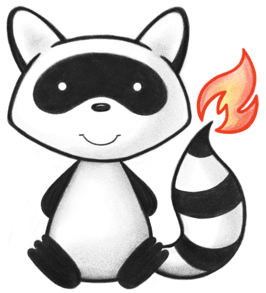
001package org.hl7.fhir.dstu3.utils; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034/* 035Copyright (c) 2011+, HL7, Inc 036All rights reserved. 037 038Redistribution and use in source and binary forms, with or without modification, 039are permitted provided that the following conditions are met: 040 041 * Redistributions of source code must retain the above copyright notice, this 042 list of conditions and the following disclaimer. 043 * Redistributions in binary form must reproduce the above copyright notice, 044 this list of conditions and the following disclaimer in the documentation 045 and/or other materials provided with the distribution. 046 * Neither the name of HL7 nor the names of its contributors may be used to 047 endorse or promote products derived from this software without specific 048 prior written permission. 049 050THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 051ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 052WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 053IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 054INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 055NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 056PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 057WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 058ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 059POSSIBILITY OF SUCH DAMAGE. 060 061 */ 062 063import java.util.ArrayList; 064import java.util.Iterator; 065import java.util.List; 066 067import org.apache.commons.lang3.StringUtils; 068import org.hl7.fhir.dstu3.model.BooleanType; 069import org.hl7.fhir.dstu3.model.CodeSystem.ConceptDefinitionComponent; 070import org.hl7.fhir.dstu3.model.CodeType; 071import org.hl7.fhir.dstu3.model.CodeableConcept; 072import org.hl7.fhir.dstu3.model.Coding; 073import org.hl7.fhir.dstu3.model.DataElement; 074import org.hl7.fhir.dstu3.model.DomainResource; 075import org.hl7.fhir.dstu3.model.Element; 076import org.hl7.fhir.dstu3.model.ElementDefinition; 077import org.hl7.fhir.dstu3.model.Extension; 078import org.hl7.fhir.dstu3.model.ExtensionHelper; 079import org.hl7.fhir.dstu3.model.Factory; 080import org.hl7.fhir.dstu3.model.Identifier; 081import org.hl7.fhir.dstu3.model.IntegerType; 082import org.hl7.fhir.dstu3.model.MarkdownType; 083import org.hl7.fhir.dstu3.model.PrimitiveType; 084import org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemComponent; 085import org.hl7.fhir.dstu3.model.Questionnaire.QuestionnaireItemType; 086import org.hl7.fhir.dstu3.model.StringType; 087import org.hl7.fhir.dstu3.model.Type; 088import org.hl7.fhir.dstu3.model.UriType; 089import org.hl7.fhir.dstu3.model.ValueSet.ConceptReferenceComponent; 090import org.hl7.fhir.dstu3.model.ValueSet.ConceptSetComponent; 091import org.hl7.fhir.dstu3.model.BackboneElement; 092import org.hl7.fhir.utilities.Utilities; 093import org.hl7.fhir.utilities.validation.ValidationMessage.Source; 094 095 096@Deprecated 097public class ToolingExtensions { 098 099 // validated 100 public static final String EXT_SUBSUMES = "http://hl7.org/fhir/StructureDefinition/codesystem-subsumes"; 101// private static final String EXT_OID = "http://hl7.org/fhir/StructureDefinition/valueset-oid"; 102// public static final String EXT_DEPRECATED = "http://hl7.org/fhir/StructureDefinition/codesystem-deprecated"; 103 public static final String EXT_DEFINITION = "http://hl7.org/fhir/StructureDefinition/valueset-definition"; 104 public static final String EXT_CS_COMMENT = "http://hl7.org/fhir/StructureDefinition/codesystem-comments"; 105 public static final String EXT_VS_COMMENT = "http://hl7.org/fhir/StructureDefinition/valueset-comments"; 106 private static final String EXT_IDENTIFIER = "http://hl7.org/fhir/StructureDefinition/identifier"; 107 public static final String EXT_TRANSLATION = "http://hl7.org/fhir/StructureDefinition/translation"; 108 public static final String EXT_ISSUE_SOURCE = "http://hl7.org/fhir/StructureDefinition/operationoutcome-issue-source"; 109 public static final String EXT_DISPLAY_HINT = "http://hl7.org/fhir/StructureDefinition/structuredefinition-display-hint"; 110 public static final String EXT_REPLACED_BY = "http://hl7.org/fhir/StructureDefinition/valueset-replacedby"; 111 public static final String EXT_JSON_TYPE = "http://hl7.org/fhir/StructureDefinition/structuredefinition-json-type"; 112 public static final String EXT_RDF_TYPE = "http://hl7.org/fhir/StructureDefinition/structuredefinition-rdf-type"; 113 public static final String EXT_XML_TYPE = "http://hl7.org/fhir/StructureDefinition/structuredefinition-xml-type"; 114 public static final String EXT_REGEX = "http://hl7.org/fhir/StructureDefinition/structuredefinition-regex"; 115 public static final String EXT_CONTROL = "http://hl7.org/fhir/StructureDefinition/questionnaire-itemControl"; 116 public static final String EXT_MINOCCURS = "http://hl7.org/fhir/StructureDefinition/questionnaire-minOccurs"; 117 public static final String EXT_MAXOCCURS = "http://hl7.org/fhir/StructureDefinition/questionnaire-maxOccurs"; 118 public static final String EXT_ALLOWEDRESOURCE = "http://hl7.org/fhir/StructureDefinition/questionnaire-allowedResource"; 119 public static final String EXT_REFERENCEFILTER = "http://hl7.org/fhir/StructureDefinition/questionnaire-referenceFilter"; 120 public static final String EXT_CODE_GENERATION_PARENT = "http://hl7.org/fhir/StructureDefinition/structuredefinition-codegen-super"; 121 122 // unregistered? 123 public static final String EXT_MAPPING_PREFIX = "http://hl7.org/fhir/tools/StructureDefinition/logical-mapping-prefix"; 124 public static final String EXT_MAPPING_SUFFIX = "http://hl7.org/fhir/tools/StructureDefinition/logical-mapping-suffix"; 125 126// public static final String EXT_FLYOVER = "http://hl7.org/fhir/Profile/questionnaire-extensions#flyover"; 127 public static final String EXT_QTYPE = "http://hl7.org/fhir/StructureDefinition/questionnnaire-baseType"; 128// private static final String EXT_QREF = "http://www.healthintersections.com.au/fhir/Profile/metadata#reference"; 129// private static final String EXTENSION_FILTER_ONLY = "http://www.healthintersections.com.au/fhir/Profile/metadata#expandNeedsFilter"; 130// private static final String EXT_TYPE = "http://www.healthintersections.com.au/fhir/Profile/metadata#type"; 131// private static final String EXT_REFERENCE = "http://www.healthintersections.com.au/fhir/Profile/metadata#reference"; 132 private static final String EXT_FHIRTYPE = "http://hl7.org/fhir/StructureDefinition/questionnaire-fhirType"; 133 private static final String EXT_ALLOWABLE_UNITS = "http://hl7.org/fhir/StructureDefinition/elementdefinition-allowedUnits"; 134 public static final String EXT_CIMI_REFERENCE = "http://hl7.org/fhir/StructureDefinition/cimi-reference"; 135 public static final String EXT_UNCLOSED = "http://hl7.org/fhir/StructureDefinition/valueset-unclosed"; 136 public static final String EXT_FMM_LEVEL = "http://hl7.org/fhir/StructureDefinition/structuredefinition-fmm"; 137 public static final String EXT_RESOURCE_CATEGORY = "http://hl7.org/fhir/StructureDefinition/structuredefinition-category"; 138 public static final String EXT_TABLE_NAME = "http://hl7.org/fhir/StructureDefinition/structuredefinition-table-name"; 139 public static final String EXT_OO_FILE = "http://hl7.org/fhir/StructureDefinition/operationoutcome-file"; 140 public static final String EXT_WORKGROUP = "http://hl7.org/fhir/StructureDefinition/structuredefinition-wg"; 141 public static final String EXT_BALLOT_STATUS = "http://hl7.org/fhir/StructureDefinition/structuredefinition-ballot-status"; 142 143 144 // specific extension helpers 145 146 public static Extension makeIssueSource(Source source) { 147 Extension ex = new Extension(); 148 // todo: write this up and get it published with the pack (and handle the redirect?) 149 ex.setUrl(ToolingExtensions.EXT_ISSUE_SOURCE); 150 CodeType c = new CodeType(); 151 c.setValue(source.toString()); 152 ex.setValue(c); 153 return ex; 154 } 155 156 public static boolean hasExtension(DomainResource de, String url) { 157 return getExtension(de, url) != null; 158 } 159 160 public static boolean hasExtension(Element e, String url) { 161 return getExtension(e, url) != null; 162 } 163 164// public static void addStringExtension(DomainResource dr, String url, String content) { 165// if (!StringUtils.isBlank(content)) { 166// Extension ex = getExtension(dr, url); 167// if (ex != null) 168// ex.setValue(new StringType(content)); 169// else 170// dr.getExtension().add(Factory.newExtension(url, new StringType(content), true)); 171// } 172// } 173 174 public static void addMarkdownExtension(DomainResource dr, String url, String content) { 175 if (!StringUtils.isBlank(content)) { 176 Extension ex = getExtension(dr, url); 177 if (ex != null) 178 ex.setValue(new StringType(content)); 179 else 180 dr.getExtension().add(Factory.newExtension(url, new MarkdownType(content), true)); 181 } 182 } 183 184 public static void addStringExtension(Element e, String url, String content) { 185 if (!StringUtils.isBlank(content)) { 186 Extension ex = getExtension(e, url); 187 if (ex != null) 188 ex.setValue(new StringType(content)); 189 else 190 e.getExtension().add(Factory.newExtension(url, new StringType(content), true)); 191 } 192 } 193 194 public static void addStringExtension(DomainResource e, String url, String content) { 195 if (!StringUtils.isBlank(content)) { 196 Extension ex = getExtension(e, url); 197 if (ex != null) 198 ex.setValue(new StringType(content)); 199 else 200 e.getExtension().add(Factory.newExtension(url, new StringType(content), true)); 201 } 202 } 203 204 public static void addIntegerExtension(DomainResource dr, String url, int value) { 205 Extension ex = getExtension(dr, url); 206 if (ex != null) 207 ex.setValue(new IntegerType(value)); 208 else 209 dr.getExtension().add(Factory.newExtension(url, new IntegerType(value), true)); 210 } 211 212 public static void addVSComment(ConceptSetComponent nc, String comment) { 213 if (!StringUtils.isBlank(comment)) 214 nc.getExtension().add(Factory.newExtension(EXT_VS_COMMENT, Factory.newString_(comment), true)); 215 } 216 public static void addVSComment(ConceptReferenceComponent nc, String comment) { 217 if (!StringUtils.isBlank(comment)) 218 nc.getExtension().add(Factory.newExtension(EXT_VS_COMMENT, Factory.newString_(comment), true)); 219 } 220 221 public static void addCSComment(ConceptDefinitionComponent nc, String comment) { 222 if (!StringUtils.isBlank(comment)) 223 nc.getExtension().add(Factory.newExtension(EXT_CS_COMMENT, Factory.newString_(comment), true)); 224 } 225 226// public static void markDeprecated(Element nc) { 227// setDeprecated(nc); 228// } 229// 230 public static void addSubsumes(ConceptDefinitionComponent nc, String code) { 231 nc.getModifierExtension().add(Factory.newExtension(EXT_SUBSUMES, Factory.newCode(code), true)); 232 } 233 234 public static void addDefinition(Element nc, String definition) { 235 if (!StringUtils.isBlank(definition)) 236 nc.getExtension().add(Factory.newExtension(EXT_DEFINITION, Factory.newString_(definition), true)); 237 } 238 239 public static void addDisplayHint(Element def, String hint) { 240 if (!StringUtils.isBlank(hint)) 241 def.getExtension().add(Factory.newExtension(EXT_DISPLAY_HINT, Factory.newString_(hint), true)); 242 } 243 244 public static String getDisplayHint(Element def) { 245 return readStringExtension(def, EXT_DISPLAY_HINT); 246 } 247 248 public static String readStringExtension(Element c, String uri) { 249 Extension ex = ExtensionHelper.getExtension(c, uri); 250 if (ex == null) 251 return null; 252 if (ex.getValue() instanceof UriType) 253 return ((UriType) ex.getValue()).getValue(); 254 if (ex.getValue() instanceof CodeType) 255 return ((CodeType) ex.getValue()).getValue(); 256 if (ex.getValue() instanceof IntegerType) 257 return ((IntegerType) ex.getValue()).asStringValue(); 258 if ((ex.getValue() instanceof MarkdownType)) 259 return ((MarkdownType) ex.getValue()).getValue(); 260 if (!(ex.getValue() instanceof StringType)) 261 return null; 262 return ((StringType) ex.getValue()).getValue(); 263 } 264 265 public static String readStringExtension(DomainResource c, String uri) { 266 Extension ex = getExtension(c, uri); 267 if (ex == null) 268 return null; 269 if ((ex.getValue() instanceof StringType)) 270 return ((StringType) ex.getValue()).getValue(); 271 if ((ex.getValue() instanceof UriType)) 272 return ((UriType) ex.getValue()).getValue(); 273 if (ex.getValue() instanceof CodeType) 274 return ((CodeType) ex.getValue()).getValue(); 275 if (ex.getValue() instanceof IntegerType) 276 return ((IntegerType) ex.getValue()).asStringValue(); 277 if ((ex.getValue() instanceof MarkdownType)) 278 return ((MarkdownType) ex.getValue()).getValue(); 279 return null; 280 } 281 282 @SuppressWarnings("unchecked") 283 public static PrimitiveType<Type> readPrimitiveExtension(DomainResource c, String uri) { 284 Extension ex = getExtension(c, uri); 285 if (ex == null) 286 return null; 287 return (PrimitiveType<Type>) ex.getValue(); 288 } 289 290 public static boolean findStringExtension(Element c, String uri) { 291 Extension ex = ExtensionHelper.getExtension(c, uri); 292 if (ex == null) 293 return false; 294 if (!(ex.getValue() instanceof StringType)) 295 return false; 296 return !StringUtils.isBlank(((StringType) ex.getValue()).getValue()); 297 } 298 299 public static Boolean readBooleanExtension(Element c, String uri) { 300 Extension ex = ExtensionHelper.getExtension(c, uri); 301 if (ex == null) 302 return null; 303 if (!(ex.getValue() instanceof BooleanType)) 304 return null; 305 return ((BooleanType) ex.getValue()).getValue(); 306 } 307 308 public static boolean findBooleanExtension(Element c, String uri) { 309 Extension ex = ExtensionHelper.getExtension(c, uri); 310 if (ex == null) 311 return false; 312 if (!(ex.getValue() instanceof BooleanType)) 313 return false; 314 return true; 315 } 316 317 public static String getCSComment(ConceptDefinitionComponent c) { 318 return readStringExtension(c, EXT_CS_COMMENT); 319 } 320// 321// public static Boolean getDeprecated(Element c) { 322// return readBooleanExtension(c, EXT_DEPRECATED); 323// } 324 325 public static boolean hasCSComment(ConceptDefinitionComponent c) { 326 return findStringExtension(c, EXT_CS_COMMENT); 327 } 328 329// public static boolean hasDeprecated(Element c) { 330// return findBooleanExtension(c, EXT_DEPRECATED); 331// } 332 333 public static List<CodeType> getSubsumes(ConceptDefinitionComponent c) { 334 List<CodeType> res = new ArrayList<CodeType>(); 335 336 for (Extension e : c.getExtension()) { 337 if (EXT_SUBSUMES.equals(e.getUrl())) 338 res.add((CodeType) e.getValue()); 339 } 340 return res; 341 } 342 343 public static void addFlyOver(QuestionnaireItemComponent item, String text){ 344 if (!StringUtils.isBlank(text)) { 345 QuestionnaireItemComponent display = item.addItem(); 346 display.setType(QuestionnaireItemType.DISPLAY); 347 display.setText(text); 348 display.getExtension().add(Factory.newExtension(EXT_CONTROL, Factory.newCodeableConcept("flyover", "http://hl7.org/fhir/questionnaire-item-control", "Fly-over"), true)); 349 } 350 } 351 352 public static void addMin(QuestionnaireItemComponent item, int min) { 353 item.getExtension().add(Factory.newExtension(EXT_MINOCCURS, Factory.newInteger(min), true)); 354 } 355 356 public static void addMax(QuestionnaireItemComponent item, int max) { 357 item.getExtension().add(Factory.newExtension(EXT_MAXOCCURS, Factory.newInteger(max), true)); 358 } 359 360 public static void addFhirType(QuestionnaireItemComponent group, String value) { 361 group.getExtension().add(Factory.newExtension(EXT_FHIRTYPE, Factory.newString_(value), true)); 362 } 363 364 public static void addControl(QuestionnaireItemComponent group, String value) { 365 group.getExtension().add(Factory.newExtension(EXT_CONTROL, Factory.newCodeableConcept(value, "http://hl7.org/fhir/questionnaire-item-control", value), true)); 366 } 367 368 public static void addAllowedResource(QuestionnaireItemComponent group, String value) { 369 group.getExtension().add(Factory.newExtension(EXT_ALLOWEDRESOURCE, Factory.newCode(value), true)); 370 } 371 372 public static void addReferenceFilter(QuestionnaireItemComponent group, String value) { 373 group.getExtension().add(Factory.newExtension(EXT_REFERENCEFILTER, Factory.newString_(value), true)); 374 } 375 376 public static void addIdentifier(Element element, Identifier value) { 377 element.getExtension().add(Factory.newExtension(EXT_IDENTIFIER, value, true)); 378 } 379 380 /** 381 * @param name the identity of the extension of interest 382 * @return The extension, if on this element, else null 383 */ 384 public static Extension getExtension(DomainResource resource, String name) { 385 if (name == null) 386 return null; 387 if (!resource.hasExtension()) 388 return null; 389 for (Extension e : resource.getExtension()) { 390 if (name.equals(e.getUrl())) 391 return e; 392 } 393 return null; 394 } 395 396 public static Extension getExtension(Element el, String name) { 397 if (name == null) 398 return null; 399 if (!el.hasExtension()) 400 return null; 401 for (Extension e : el.getExtension()) { 402 if (name.equals(e.getUrl())) 403 return e; 404 } 405 return null; 406 } 407 408 public static void setStringExtension(DomainResource resource, String uri, String value) { 409 Extension ext = getExtension(resource, uri); 410 if (ext != null) 411 ext.setValue(new StringType(value)); 412 else 413 resource.getExtension().add(new Extension(new UriType(uri)).setValue(new StringType(value))); 414 } 415 416 public static void setStringExtension(Element element, String uri, String value) { 417 Extension ext = getExtension(element, uri); 418 if (ext != null) 419 ext.setValue(new StringType(value)); 420 else 421 element.getExtension().add(new Extension(new UriType(uri)).setValue(new StringType(value))); 422 } 423 424 public static void setCodeExtension(DomainResource resource, String uri, String value) { 425 Extension ext = getExtension(resource, uri); 426 if (ext != null) 427 ext.setValue(new CodeType(value)); 428 else 429 resource.getExtension().add(new Extension(new UriType(uri)).setValue(new CodeType(value))); 430 } 431 432 public static void setIntegerExtension(DomainResource resource, String uri, int value) { 433 Extension ext = getExtension(resource, uri); 434 if (ext != null) 435 ext.setValue(new IntegerType(value)); 436 else 437 resource.getExtension().add(new Extension(new UriType(uri)).setValue(new IntegerType(value))); 438 } 439 440 441 public static void setCodeExtensionMod(DomainResource resource, String uri, String value) { 442 if (Utilities.noString(value)) 443 return; 444 445 Extension ext = getExtension(resource, uri); 446 if (ext != null) 447 ext.setValue(new CodeType(value)); 448 else 449 resource.getModifierExtension().add(new Extension(uri).setValue(new CodeType(value))); 450 } 451 452 public static void setCodeExtensionMod(BackboneElement resource, String uri, String value) { 453 if (Utilities.noString(value)) 454 return; 455 456 Extension ext = getExtension(resource, uri); 457 if (ext != null) 458 ext.setValue(new CodeType(value)); 459 else 460 resource.getModifierExtension().add(new Extension(uri).setValue(new CodeType(value))); 461 } 462 463// public static String getOID(CodeSystem define) { 464// return readStringExtension(define, EXT_OID); 465// } 466// 467// public static String getOID(ValueSet vs) { 468// return readStringExtension(vs, EXT_OID); 469// } 470// 471// public static void setOID(CodeSystem define, String oid) throws FHIRFormatError, URISyntaxException { 472// if (!oid.startsWith("urn:oid:")) 473// throw new FHIRFormatError("Error in OID format"); 474// if (oid.startsWith("urn:oid:urn:oid:")) 475// throw new FHIRFormatError("Error in OID format"); 476// if (!hasExtension(define, EXT_OID)) 477// define.getExtension().add(Factory.newExtension(EXT_OID, Factory.newUri(oid), false)); 478// else if (!oid.equals(readStringExtension(define, EXT_OID))) 479// throw new Error("Attempt to assign multiple OIDs to a code system"); 480// } 481// public static void setOID(ValueSet vs, String oid) throws FHIRFormatError, URISyntaxException { 482// if (!oid.startsWith("urn:oid:")) 483// throw new FHIRFormatError("Error in OID format"); 484// if (oid.startsWith("urn:oid:urn:oid:")) 485// throw new FHIRFormatError("Error in OID format"); 486// if (!hasExtension(vs, EXT_OID)) 487// vs.getExtension().add(Factory.newExtension(EXT_OID, Factory.newUri(oid), false)); 488// else if (!oid.equals(readStringExtension(vs, EXT_OID))) 489// throw new Error("Attempt to assign multiple OIDs to value set "+vs.getName()+" ("+vs.getUrl()+"). Has "+readStringExtension(vs, EXT_OID)+", trying to add "+oid); 490// } 491 492 public static boolean hasLanguageTranslation(Element element, String lang) { 493 for (Extension e : element.getExtension()) { 494 if (e.getUrl().equals(EXT_TRANSLATION)) { 495 Extension e1 = ExtensionHelper.getExtension(e, "lang"); 496 497 if (e1 != null && e1.getValue() instanceof CodeType && ((CodeType) e.getValue()).getValue().equals(lang)) 498 return true; 499 } 500 } 501 return false; 502 } 503 504 public static String getLanguageTranslation(Element element, String lang) { 505 for (Extension e : element.getExtension()) { 506 if (e.getUrl().equals(EXT_TRANSLATION)) { 507 Extension e1 = ExtensionHelper.getExtension(e, "lang"); 508 509 if (e1 != null && e1.getValue() instanceof CodeType && ((CodeType) e.getValue()).getValue().equals(lang)) { 510 e1 = ExtensionHelper.getExtension(e, "content"); 511 return ((StringType) e.getValue()).getValue(); 512 } 513 } 514 } 515 return null; 516 } 517 518 public static void addLanguageTranslation(Element element, String lang, String value) { 519 Extension extension = new Extension().setUrl(EXT_TRANSLATION); 520 extension.addExtension().setUrl("lang").setValue(new StringType(lang)); 521 extension.addExtension().setUrl("content").setValue(new StringType(value)); 522 element.getExtension().add(extension); 523 } 524 525 public static Type getAllowedUnits(ElementDefinition eld) { 526 for (Extension e : eld.getExtension()) 527 if (e.getUrl().equals(EXT_ALLOWABLE_UNITS)) 528 return e.getValue(); 529 return null; 530 } 531 532 public static void setAllowableUnits(ElementDefinition eld, CodeableConcept cc) { 533 for (Extension e : eld.getExtension()) 534 if (e.getUrl().equals(EXT_ALLOWABLE_UNITS)) { 535 e.setValue(cc); 536 return; 537 } 538 eld.getExtension().add(new Extension().setUrl(EXT_ALLOWABLE_UNITS).setValue(cc)); 539 } 540 541 public static List<Extension> getExtensions(Element element, String url) { 542 List<Extension> results = new ArrayList<Extension>(); 543 for (Extension ex : element.getExtension()) 544 if (ex.getUrl().equals(url)) 545 results.add(ex); 546 return results; 547 } 548 549 public static List<Extension> getExtensions(DomainResource resource, String url) { 550 List<Extension> results = new ArrayList<Extension>(); 551 for (Extension ex : resource.getExtension()) 552 if (ex.getUrl().equals(url)) 553 results.add(ex); 554 return results; 555 } 556 557 public static void addDEReference(DataElement de, String value) { 558 for (Extension e : de.getExtension()) 559 if (e.getUrl().equals(EXT_CIMI_REFERENCE)) { 560 e.setValue(new UriType(value)); 561 return; 562 } 563 de.getExtension().add(new Extension().setUrl(EXT_CIMI_REFERENCE).setValue(new UriType(value))); 564 } 565 566// public static void setDeprecated(Element nc) { 567// for (Extension e : nc.getExtension()) 568// if (e.getUrl().equals(EXT_DEPRECATED)) { 569// e.setValue(new BooleanType(true)); 570// return; 571// } 572// nc.getExtension().add(new Extension().setUrl(EXT_DEPRECATED).setValue(new BooleanType(true))); 573// } 574 575 public static void setExtension(Element focus, String url, Coding c) { 576 for (Extension e : focus.getExtension()) 577 if (e.getUrl().equals(url)) { 578 e.setValue(c); 579 return; 580 } 581 focus.getExtension().add(new Extension().setUrl(url).setValue(c)); 582 } 583 584 public static void removeExtension(DomainResource focus, String url) { 585 Iterator<Extension> i = focus.getExtension().iterator(); 586 while (i.hasNext()) { 587 Extension e = i.next(); // must be called before you can call i.remove() 588 if (e.getUrl().equals(url)) { 589 i.remove(); 590 } 591 } 592 } 593 594 public static void removeExtension(Element focus, String url) { 595 Iterator<Extension> i = focus.getExtension().iterator(); 596 while (i.hasNext()) { 597 Extension e = i.next(); // must be called before you can call i.remove() 598 if (e.getUrl().equals(url)) { 599 i.remove(); 600 } 601 } 602 } 603 604 public static int readIntegerExtension(DomainResource dr, String uri, int defaultValue) { 605 Extension ex = ExtensionHelper.getExtension(dr, uri); 606 if (ex == null) 607 return defaultValue; 608 if (ex.getValue() instanceof IntegerType) 609 return ((IntegerType) ex.getValue()).getValue(); 610 throw new Error("Unable to read extension "+uri+" as an integer"); 611 } 612 613// public static boolean hasOID(ValueSet vs) { 614// return hasExtension(vs, EXT_OID); 615// } 616// 617// public static boolean hasOID(CodeSystem cs) { 618// return hasExtension(cs, EXT_OID); 619// } 620// 621 622}