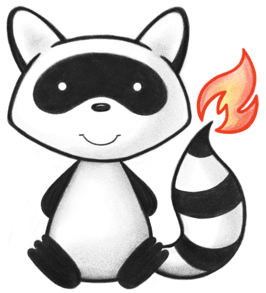
001package org.hl7.fhir.dstu3.utils; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.io.FileNotFoundException; 035import java.io.IOException; 036import java.util.HashMap; 037import java.util.Map; 038 039import javax.xml.parsers.DocumentBuilder; 040import javax.xml.parsers.DocumentBuilderFactory; 041import javax.xml.parsers.ParserConfigurationException; 042 043import org.hl7.fhir.utilities.filesystem.CSFileInputStream; 044import org.hl7.fhir.utilities.xml.XMLUtil; 045import org.w3c.dom.Document; 046import org.w3c.dom.Element; 047import org.xml.sax.SAXException; 048 049@Deprecated 050public class Translations { 051 052 private String[] lang; 053 private Map<String, Element> messages = new HashMap<String, Element>(); 054 055 /** 056 * Set a default language to use 057 * 058 * @param lang 059 */ 060 public void setLang(String lang) { 061 this.lang = lang.split("[.;]"); 062 } 063 064 /** 065 * Load from the XML translations file maintained by the FHIR project 066 * 067 * @param filename 068 * @throws IOException 069 * @throws SAXException 070 * @throws FileNotFoundException 071 * @throws ParserConfigurationException 072 * @throws Exception 073 */ 074 public void load(String filename) throws FileNotFoundException, SAXException, IOException, ParserConfigurationException { 075 DocumentBuilderFactory factory = XMLUtil.newXXEProtectedDocumentBuilderFactory(); 076 DocumentBuilder builder = factory.newDocumentBuilder(); 077 loadMessages(builder.parse(new CSFileInputStream(filename))); 078 } 079 080 private void loadMessages(Document doc) { 081 // TODO Auto-generated method stub 082 Element element = XMLUtil.getFirstChild(doc.getDocumentElement()); 083 while (element != null) { 084 messages.put(element.getAttribute("id"), element); 085 element = XMLUtil.getNextSibling(element); 086 } 087 } 088 089 public boolean hasTranslation(String id) { 090 return messages.containsKey(id); 091 } 092 093 /** 094 * use configured language 095 * 096 * @param id - the id of the message to retrieve 097 * @param defaultMsg - string to use if the message is not defined or a language match is not found (if null, then will default to english) 098 * @return the message 099 */ 100 public String getMessage(String id, String defaultMsg) { 101 return getMessage(id, lang, defaultMsg); 102 } 103 104 /** 105 * return the message in a specified language 106 * 107 * @param id - the id of the message to retrieve 108 * @param lang - a language string from a browser 109 * @param defaultMsg - string to use if the message is not defined or a language match is not found (if null, then will default to the english message) 110 * @return the message 111 */ 112 public String getMessage(String id, String lang, String defaultMsg) { 113 return getMessage(id, lang.split("[.;]"), defaultMsg); 114 } 115 116 private String getMessage(String id, String[] lang, String defaultMsg) { 117 Element msg = messages.get(id); 118 if (msg == null) 119 return defaultMsg; 120 for (String l : lang) { 121 String res = getByLang(msg, l); 122 if (res != null) 123 return res; 124 } 125 if (defaultMsg == null) { 126 String res = getByLang(msg, "en"); 127 if (res != null) 128 return res; 129 } 130 return defaultMsg; 131 } 132 133 private String getByLang(Element msg, String lang) { 134 Element c = XMLUtil.getFirstChild(msg); 135 while (c != null) { 136 if (c.getAttribute("lang").equals(lang)) 137 return c.getTextContent(); 138 c = XMLUtil.getNextSibling(c); 139 } 140 return null; 141 } 142 143 // http://en.wikipedia.org/wiki/List_of_ISO_639-1_codes 144 public String getLangDesc(String s) { 145 if (s.equals("en")) 146 return "English"; 147 if (s.equals("nl")) 148 return "Nederlands (Dutch)"; 149 if (s.equals("de")) 150 return "Deutsch (German)"; 151 if (s.equals("ru")) 152 return "\u0440\u0443\u0301\u0441\u0441\u043a\u0438\u0439 (Russian)"; 153 return "\"" + s + "\""; 154 } 155 156 157}