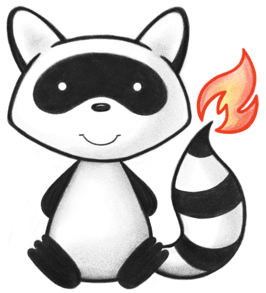
001package org.hl7.fhir.dstu3.utils; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.util.ArrayList; 035import java.util.List; 036 037import org.hl7.fhir.utilities.Utilities; 038 039 040public class TypesUtilities { 041 042 public static class WildcardInformation { 043 private String typeName; 044 private String comment; 045 public WildcardInformation(String typeName, String comment) { 046 super(); 047 this.typeName = typeName; 048 this.comment = comment; 049 } 050 public WildcardInformation(String typeName) { 051 super(); 052 this.typeName = typeName; 053 } 054 public String getTypeName() { 055 return typeName; 056 } 057 public String getComment() { 058 return comment; 059 } 060 061 } 062 063 public static List<String> wildcardTypes() { 064 List<String> res = new ArrayList<String>(); 065 for (WildcardInformation wi : wildcards()) 066 res.add(wi.getTypeName()); 067 return res; 068 } 069 070 // this is the master list for what data types are allowed where the types = * 071 // that this list is incomplete means that the following types cannot have fixed values in a profile: 072 // Narrative 073 // Meta 074 // Any of the IDMP data types 075 // You have to walk into them to profile them. 076 // 077 public static List<WildcardInformation> wildcards() { 078 List<WildcardInformation> res = new ArrayList<WildcardInformation>(); 079 080 // primitive types 081 res.add(new WildcardInformation("base64Binary")); 082 res.add(new WildcardInformation("boolean")); 083 res.add(new WildcardInformation("canonical")); 084 res.add(new WildcardInformation("code", "(only if the extension definition provides a <a href=\"terminologies.html#code\">fixed</a> binding to a suitable set of codes)")); 085 res.add(new WildcardInformation("date")); 086 res.add(new WildcardInformation("dateTime")); 087 res.add(new WildcardInformation("decimal")); 088 res.add(new WildcardInformation("id")); 089 res.add(new WildcardInformation("instant")); 090 res.add(new WildcardInformation("integer")); 091 res.add(new WildcardInformation("markdown")); 092 res.add(new WildcardInformation("oid")); 093 res.add(new WildcardInformation("positiveInt")); 094 res.add(new WildcardInformation("string")); 095 res.add(new WildcardInformation("time")); 096 res.add(new WildcardInformation("unsignedInt")); 097 res.add(new WildcardInformation("uri")); 098 res.add(new WildcardInformation("url")); 099 res.add(new WildcardInformation("uuid")); 100 101 // Complex general purpose data types 102 res.add(new WildcardInformation("Address")); 103 res.add(new WildcardInformation("Age")); 104 res.add(new WildcardInformation("Annotation")); 105 res.add(new WildcardInformation("Attachment")); 106 res.add(new WildcardInformation("CodeableConcept")); 107 res.add(new WildcardInformation("Coding")); 108 res.add(new WildcardInformation("ContactPoint")); 109 res.add(new WildcardInformation("Count")); 110 res.add(new WildcardInformation("Distance")); 111 res.add(new WildcardInformation("Duration")); 112 res.add(new WildcardInformation("HumanName")); 113 res.add(new WildcardInformation("Identifier")); 114 res.add(new WildcardInformation("Money")); 115 res.add(new WildcardInformation("Period")); 116 res.add(new WildcardInformation("Quantity")); 117 res.add(new WildcardInformation("Range")); 118 res.add(new WildcardInformation("Ratio")); 119 res.add(new WildcardInformation("Reference", " - a reference to another resource")); 120 res.add(new WildcardInformation("SampledData")); 121 res.add(new WildcardInformation("Signature")); 122 res.add(new WildcardInformation("Timing")); 123 124 // metadata types 125 res.add(new WildcardInformation("ParameterDefinition")); 126 res.add(new WildcardInformation("DataRequirement")); 127 res.add(new WildcardInformation("RelatedArtifact")); 128 res.add(new WildcardInformation("ContactDetail")); 129 res.add(new WildcardInformation("Contributor")); 130 res.add(new WildcardInformation("TriggerDefinition")); 131 res.add(new WildcardInformation("Expression")); 132 res.add(new WildcardInformation("UsageContext")); 133 134 // special cases 135 res.add(new WildcardInformation("Dosage")); 136 return res; 137 } 138 139 public static boolean isPrimitive(String code) { 140 return Utilities.existsInList(code, "boolean", "integer", "string", "decimal", "uri", "url", "canonical", "base64Binary", "instant", "date", "dateTime", "time", "code", "oid", "id", "markdown", "unsignedInt", "positiveInt", "xhtml"); 141 } 142}