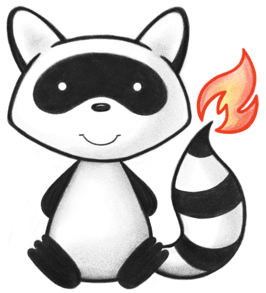
001package org.hl7.fhir.dstu3.utils.client; 002 003import java.io.IOException; 004import java.net.URI; 005import java.net.URISyntaxException; 006import java.util.*; 007 008import lombok.Getter; 009import lombok.Setter; 010import org.hl7.fhir.dstu3.model.Bundle; 011import org.hl7.fhir.dstu3.model.CapabilityStatement; 012import org.hl7.fhir.dstu3.model.CodeSystem; 013import org.hl7.fhir.dstu3.model.Coding; 014import org.hl7.fhir.dstu3.model.ConceptMap; 015import org.hl7.fhir.dstu3.model.OperationOutcome; 016import org.hl7.fhir.dstu3.model.Parameters; 017import org.hl7.fhir.dstu3.model.Parameters.ParametersParameterComponent; 018import org.hl7.fhir.dstu3.model.PrimitiveType; 019import org.hl7.fhir.dstu3.model.Resource; 020import org.hl7.fhir.dstu3.model.StringType; 021import org.hl7.fhir.dstu3.model.ValueSet; 022import org.hl7.fhir.dstu3.utils.client.network.ByteUtils; 023import org.hl7.fhir.dstu3.utils.client.network.Client; 024import org.hl7.fhir.dstu3.utils.client.network.ResourceRequest; 025import org.hl7.fhir.exceptions.FHIRException; 026import org.hl7.fhir.utilities.FHIRBaseToolingClient; 027import org.hl7.fhir.utilities.FhirPublication; 028import org.hl7.fhir.utilities.ToolingClientLogger; 029import org.hl7.fhir.utilities.Utilities; 030 031import org.hl7.fhir.utilities.http.HTTPHeader; 032 033/** 034 * Very Simple RESTful client. This is purely for use in the standalone 035 * tools jar packages. It doesn't support many features, only what the tools 036 * need. 037 * <p> 038 * To use, initialize class and set base service URI as follows: 039 * 040 * <pre><code> 041 * FHIRSimpleClient fhirClient = new FHIRSimpleClient(); 042 * fhirClient.initialize("http://my.fhir.domain/myServiceRoot"); 043 * </code></pre> 044 * <p> 045 * Default Accept and Content-Type headers are application/fhir+xml and application/fhir+json. 046 * <p> 047 * These can be changed by invoking the following setter functions: 048 * 049 * <pre><code> 050 * setPreferredResourceFormat() 051 * setPreferredFeedFormat() 052 * </code></pre> 053 * <p> 054 * TODO Review all sad paths. 055 * 056 * @author Claude Nanjo 057 */ 058public class FHIRToolingClient extends FHIRBaseToolingClient { 059 060 public static final String DATETIME_FORMAT = "yyyy-MM-dd'T'HH:mm:ssK"; 061 public static final String DATE_FORMAT = "yyyy-MM-dd"; 062 public static final String hostKey = "http.proxyHost"; 063 public static final String portKey = "http.proxyPort"; 064 065 private String base; 066 private ResourceAddress resourceAddress; 067 private ResourceFormat preferredResourceFormat; 068 private int maxResultSetSize = -1;//_count 069 private CapabilityStatement capabilities; 070 private Client client = new Client(); 071 private List<HTTPHeader> headers = new ArrayList<>(); 072 @Setter 073 @Getter 074 private String userAgent; 075 private EnumSet<FhirPublication> allowedVersions; 076 @Setter 077 @Getter 078 private String acceptLanguage; 079 @Setter 080 private String contentLanguage; 081 @Getter 082 private int useCount; 083 084 //Pass endpoint for client - URI 085 public FHIRToolingClient(String baseServiceUrl, String userAgent) throws URISyntaxException { 086 preferredResourceFormat = ResourceFormat.RESOURCE_XML; 087 this.userAgent = userAgent; 088 this.allowedVersions = supportableVersions(); 089 initialize(baseServiceUrl); 090 } 091 092 public void initialize(String baseServiceUrl) throws URISyntaxException { 093 base = baseServiceUrl; 094 client.setBase(base); 095 resourceAddress = new ResourceAddress(baseServiceUrl); 096 this.allowedVersions = supportableVersions(); 097 this.maxResultSetSize = -1; 098 } 099 100 public Client getClient() { 101 return client; 102 } 103 104 public EnumSet<FhirPublication> supportableVersions() { 105 // todo 106 return EnumSet.range(FhirPublication.STU3, FhirPublication.R5); 107 } 108 109 public void setAllowedVersions(EnumSet<FhirPublication> versions) { 110 // todo 111 } 112 113 public EnumSet<FhirPublication> getAllowedVersions() { 114 return null; // todo 115 } 116 117 public FhirPublication getActualVersion() { 118 return FhirPublication.STU3; 119 } 120 121 public void setClient(Client client) { 122 this.client = client; 123 } 124 125 private void checkCapabilities() { 126 try { 127 capabilities = getCapabilitiesStatementQuick(); 128 } catch (Throwable e) { 129 } 130 } 131 132 public String getPreferredResourceFormat() { 133 return preferredResourceFormat.getHeader(); 134 } 135 136 public void setPreferredResourceFormat(ResourceFormat resourceFormat) { 137 preferredResourceFormat = resourceFormat; 138 } 139 140 public int getMaximumRecordCount() { 141 return maxResultSetSize; 142 } 143 144 public void setMaximumRecordCount(int maxResultSetSize) { 145 this.maxResultSetSize = maxResultSetSize; 146 } 147 148 public Parameters getTerminologyCapabilities() { 149 Parameters capabilities = null; 150 try { 151 capabilities = (Parameters) client.issueGetResourceRequest(resourceAddress.resolveMetadataTxCaps(), 152 withVer(getPreferredResourceFormat(), "3.0"), 153 generateHeaders(false), 154 "TerminologyCapabilities", 155 timeoutNormal).getReference(); 156 } catch (Exception e) { 157 throw new FHIRException("Error fetching the server's terminology capabilities", e); 158 } 159 return capabilities; 160 } 161 162 public CapabilityStatement getCapabilitiesStatement() { 163 CapabilityStatement conformance = null; 164 try { 165 conformance = (CapabilityStatement) client.issueGetResourceRequest(resourceAddress.resolveMetadataUri(false), 166 withVer(getPreferredResourceFormat(), "3.0"), 167 generateHeaders(false), 168 "CapabilitiesStatement", 169 timeoutNormal).getReference(); 170 } catch (Exception e) { 171 throw new FHIRException("Error fetching the server's conformance statement", e); 172 } 173 return conformance; 174 } 175 176 public CapabilityStatement getCapabilitiesStatementQuick() throws EFhirClientException { 177 if (capabilities != null) return capabilities; 178 try { 179 capabilities = (CapabilityStatement) client.issueGetResourceRequest(resourceAddress.resolveMetadataUri(true), 180 withVer(getPreferredResourceFormat(), "3.0"), 181 generateHeaders(false), 182 "CapabilitiesStatement-Quick", 183 timeoutNormal).getReference(); 184 } catch (Exception e) { 185 throw new FHIRException("Error fetching the server's capability statement: "+e.getMessage(), e); 186 } 187 return capabilities; 188 } 189 190 public <T extends Resource> T read(Class<T> resourceClass, String id) {//TODO Change this to AddressableResource 191 recordUse(); 192 ResourceRequest<T> result = null; 193 try { 194 result = client.issueGetResourceRequest(resourceAddress.resolveGetUriFromResourceClassAndId(resourceClass, id), 195 withVer(getPreferredResourceFormat(), "3.0"), 196 generateHeaders(false), 197 "Read " + resourceClass.getName() + "/" + id, 198 timeoutNormal); 199 if (result.isUnsuccessfulRequest()) { 200 throw new EFhirClientException("Server returned error code " + result.getHttpStatus(), (OperationOutcome) result.getPayload()); 201 } 202 } catch (Exception e) { 203 throw new FHIRException(e); 204 } 205 return result.getPayload(); 206 } 207 208 public <T extends Resource> T vread(Class<T> resourceClass, String id, String version) { 209 recordUse(); 210 ResourceRequest<T> result = null; 211 try { 212 result = client.issueGetResourceRequest(resourceAddress.resolveGetUriFromResourceClassAndIdAndVersion(resourceClass, id, version), 213 withVer(getPreferredResourceFormat(), "3.0"), 214 generateHeaders(false), 215 "VRead " + resourceClass.getName() + "/" + id + "/?_history/" + version, 216 timeoutNormal); 217 if (result.isUnsuccessfulRequest()) { 218 throw new EFhirClientException("Server returned error code " + result.getHttpStatus(), (OperationOutcome) result.getPayload()); 219 } 220 } catch (Exception e) { 221 throw new FHIRException("Error trying to read this version of the resource", e); 222 } 223 return result.getPayload(); 224 } 225 226 public <T extends Resource> T getCanonical(Class<T> resourceClass, String canonicalURL) { 227 recordUse(); 228 ResourceRequest<T> result = null; 229 try { 230 result = client.issueGetResourceRequest(resourceAddress.resolveGetUriFromResourceClassAndCanonical(resourceClass, canonicalURL), 231 withVer(getPreferredResourceFormat(), "3.0"), 232 generateHeaders(false), 233 "Read " + resourceClass.getName() + "?url=" + canonicalURL, 234 timeoutNormal); 235 if (result.isUnsuccessfulRequest()) { 236 throw new EFhirClientException("Server returned error code " + result.getHttpStatus(), (OperationOutcome) result.getPayload()); 237 } 238 } catch (Exception e) { 239 handleException("An error has occurred while trying to read this version of the resource", e); 240 } 241 Bundle bnd = (Bundle) result.getPayload(); 242 if (bnd.getEntry().size() == 0) 243 throw new EFhirClientException("No matching resource found for canonical URL '" + canonicalURL + "'"); 244 if (bnd.getEntry().size() > 1) 245 throw new EFhirClientException("Multiple matching resources found for canonical URL '" + canonicalURL + "'"); 246 return (T) bnd.getEntry().get(0).getResource(); 247 } 248 249 public Resource update(Resource resource) { 250 recordUse(); 251 org.hl7.fhir.dstu3.utils.client.network.ResourceRequest<Resource> result = null; 252 try { 253 result = client.issuePutRequest(resourceAddress.resolveGetUriFromResourceClassAndId(resource.getClass(), resource.getId()), 254 ByteUtils.resourceToByteArray(resource, false, isJson(getPreferredResourceFormat()), false), 255 withVer(getPreferredResourceFormat(), "3.0"), 256 generateHeaders(true), 257 "Update " + resource.fhirType() + "/" + resource.getId(), 258 timeoutOperation); 259 if (result.isUnsuccessfulRequest()) { 260 throw new EFhirClientException("Server returned error code " + result.getHttpStatus(), (OperationOutcome) result.getPayload()); 261 } 262 } catch (Exception e) { 263 throw new EFhirClientException("An error has occurred while trying to update this resource", e); 264 } 265 // TODO oe 26.1.2015 could be made nicer if only OperationOutcome locationheader is returned with an operationOutcome would be returned (and not the resource also) we make another read 266 try { 267 OperationOutcome operationOutcome = (OperationOutcome) result.getPayload(); 268 ResourceAddress.ResourceVersionedIdentifier resVersionedIdentifier = ResourceAddress.parseCreateLocation(result.getLocation()); 269 return this.vread(resource.getClass(), resVersionedIdentifier.getId(), resVersionedIdentifier.getVersionId()); 270 } catch (ClassCastException e) { 271 // if we fall throught we have the correct type already in the create 272 } 273 274 return result.getPayload(); 275 } 276 277 public <T extends Resource> T update(Class<T> resourceClass, T resource, String id) { 278 recordUse(); 279 ResourceRequest<T> result = null; 280 try { 281 result = client.issuePutRequest(resourceAddress.resolveGetUriFromResourceClassAndId(resourceClass, id), 282 ByteUtils.resourceToByteArray(resource, false, isJson(getPreferredResourceFormat()), false), 283 withVer(getPreferredResourceFormat(), "3.0"), 284 generateHeaders(true), 285 "Update " + resource.fhirType() + "/" + id, 286 timeoutOperation); 287 if (result.isUnsuccessfulRequest()) { 288 throw new EFhirClientException("Server returned error code " + result.getHttpStatus(), (OperationOutcome) result.getPayload()); 289 } 290 } catch (Exception e) { 291 throw new EFhirClientException("An error has occurred while trying to update this resource", e); 292 } 293 // TODO oe 26.1.2015 could be made nicer if only OperationOutcome locationheader is returned with an operationOutcome would be returned (and not the resource also) we make another read 294 try { 295 OperationOutcome operationOutcome = (OperationOutcome) result.getPayload(); 296 ResourceAddress.ResourceVersionedIdentifier resVersionedIdentifier = ResourceAddress.parseCreateLocation(result.getLocation()); 297 return this.vread(resourceClass, resVersionedIdentifier.getId(), resVersionedIdentifier.getVersionId()); 298 } catch (ClassCastException e) { 299 // if we fall through we have the correct type already in the create 300 } 301 302 return result.getPayload(); 303 } 304 305 public <T extends Resource> Parameters operateType(Class<T> resourceClass, String name, Parameters params) { 306 recordUse(); 307 boolean complex = false; 308 for (ParametersParameterComponent p : params.getParameter()) 309 complex = complex || !(p.getValue() instanceof PrimitiveType); 310 String ps = ""; 311 try { 312 if (!complex) 313 for (ParametersParameterComponent p : params.getParameter()) 314 if (p.getValue() instanceof PrimitiveType) 315 ps += p.getName() + "=" + Utilities.encodeUriParam(((PrimitiveType) p.getValue()).asStringValue()) + "&"; 316 ResourceRequest<T> result; 317 URI url = resourceAddress.resolveOperationURLFromClass(resourceClass, name, ps); 318 if (complex) { 319 byte[] body = ByteUtils.resourceToByteArray(params, false, isJson(getPreferredResourceFormat()), true); 320 if (client.getLogger() != null) { 321 client.getLogger().logRequest("POST", url.toString(), null, body); 322 } 323 result = client.issuePostRequest(url, body, withVer(getPreferredResourceFormat(), "3.0"), generateHeaders(true), 324 "POST " + resourceClass.getName() + "/$" + name, timeoutLong); 325 } else { 326 if (client.getLogger() != null) { 327 client.getLogger().logRequest("GET", url.toString(), null, null); 328 } 329 result = client.issueGetResourceRequest(url, withVer(getPreferredResourceFormat(), "3.0"), generateHeaders(false), "GET " + resourceClass.getName() + "/$" + name, timeoutLong); 330 } 331 if (result.isUnsuccessfulRequest()) { 332 throw new EFhirClientException("Server returned error code " + result.getHttpStatus(), (OperationOutcome) result.getPayload()); 333 } 334 if (result.getPayload() instanceof Parameters) { 335 return (Parameters) result.getPayload(); 336 } else { 337 Parameters p_out = new Parameters(); 338 p_out.addParameter().setName("return").setResource(result.getPayload()); 339 return p_out; 340 } 341 } catch (Exception e) { 342 handleException("Error performing tx3 operation '"+name+": "+e.getMessage()+"' (parameters = \"" + ps+"\")", e); 343 } 344 return null; 345 } 346 347 348 public Bundle transaction(Bundle batch) { 349 recordUse(); 350 Bundle transactionResult = null; 351 try { 352 transactionResult = client.postBatchRequest(resourceAddress.getBaseServiceUri(), ByteUtils.resourceToByteArray(batch, false, isJson(getPreferredResourceFormat()), false), withVer(getPreferredResourceFormat(), "3.0"), "transaction", timeoutOperation + (timeoutEntry * batch.getEntry().size())); 353 } catch (Exception e) { 354 handleException("An error occurred trying to process this transaction request", e); 355 } 356 return transactionResult; 357 } 358 359 @SuppressWarnings("unchecked") 360 public <T extends Resource> OperationOutcome validate(Class<T> resourceClass, T resource, String id) { 361 recordUse(); 362 ResourceRequest<T> result = null; 363 try { 364 result = client.issuePostRequest(resourceAddress.resolveValidateUri(resourceClass, id), 365 ByteUtils.resourceToByteArray(resource, false, isJson(getPreferredResourceFormat()), false), 366 withVer(getPreferredResourceFormat(), "3.0"), generateHeaders(true), 367 "POST " + resourceClass.getName() + (id != null ? "/" + id : "") + "/$validate", timeoutLong); 368 if (result.isUnsuccessfulRequest()) { 369 throw new EFhirClientException("Server returned error code " + result.getHttpStatus(), (OperationOutcome) result.getPayload()); 370 } 371 } catch (Exception e) { 372 handleException("An error has occurred while trying to validate this resource", e); 373 } 374 return (OperationOutcome) result.getPayload(); 375 } 376 377 /** 378 * Helper method to prevent nesting of previously thrown EFhirClientExceptions. If the e param is an instance of 379 * EFhirClientException, it will be rethrown. Otherwise, a new EFhirClientException will be thrown with e as the 380 * cause. 381 * 382 383 * @param message The EFhirClientException message. 384 * @param e The exception 385 * @throws EFhirClientException EFhirClientException representing the exception. 386 */ 387 protected void handleException(String message, Exception e) throws EFhirClientException { 388 if (e instanceof EFhirClientException) { 389 throw (EFhirClientException) e; 390 } else { 391 throw new EFhirClientException(message, e); 392 } 393 } 394 395 /** 396 * Helper method to determine whether desired resource representation is Json or 397 * XML. 398 * 399 * @param format the format 400 * @return true if the format is JSON, false otherwise 401 */ 402 protected boolean isJson(String format) { 403 boolean isJson = false; 404 if (format.toLowerCase().contains("json")) { 405 isJson = true; 406 } 407 return isJson; 408 } 409 410 public Bundle fetchFeed(String url) { 411 recordUse(); 412 Bundle feed = null; 413 try { 414 feed = client.issueGetFeedRequest(new URI(url), getPreferredResourceFormat()); 415 } catch (Exception e) { 416 handleException("An error has occurred while trying to read a bundle", e); 417 } 418 return feed; 419 } 420 421 public Parameters lookupCode(Map<String, String> params) { 422 recordUse(); 423 org.hl7.fhir.dstu3.utils.client.network.ResourceRequest<Resource> result = null; 424 try { 425 result = client.issueGetResourceRequest(resourceAddress.resolveOperationUri(CodeSystem.class, "lookup", params), 426 withVer(getPreferredResourceFormat(), "3.0"), 427 generateHeaders(false), 428 "CodeSystem/$lookup", 429 timeoutNormal); 430 } catch (IOException e) { 431 e.printStackTrace(); 432 } 433 if (result.isUnsuccessfulRequest()) { 434 throw new EFhirClientException("Server returned error code " + result.getHttpStatus(), (OperationOutcome) result.getPayload()); 435 } 436 return (Parameters) result.getPayload(); 437 } 438 439 public Parameters lookupCode(Parameters p) { 440 recordUse(); 441 org.hl7.fhir.dstu3.utils.client.network.ResourceRequest<Resource> result = null; 442 try { 443 result = client.issuePostRequest(resourceAddress.resolveOperationUri(CodeSystem.class, "lookup"), 444 ByteUtils.resourceToByteArray(p, false, isJson(getPreferredResourceFormat()), true), 445 withVer(getPreferredResourceFormat(), "3.0"), 446 generateHeaders(true), 447 "CodeSystem/$lookup", 448 timeoutNormal); 449 } catch (IOException e) { 450 e.printStackTrace(); 451 } 452 if (result.isUnsuccessfulRequest()) { 453 throw new EFhirClientException("Server returned error code " + result.getHttpStatus(), (OperationOutcome) result.getPayload()); 454 } 455 return (Parameters) result.getPayload(); 456 } 457 458 public Parameters transform(Parameters p) { 459 recordUse(); 460 org.hl7.fhir.dstu3.utils.client.network.ResourceRequest<Resource> result = null; 461 try { 462 result = client.issuePostRequest(resourceAddress.resolveOperationUri(ConceptMap.class, "transform"), 463 ByteUtils.resourceToByteArray(p, false, isJson(getPreferredResourceFormat()), true), 464 withVer(getPreferredResourceFormat(), "3.0"), 465 generateHeaders(true), 466 "ConceptMap/$transform", 467 timeoutNormal); 468 } catch (IOException e) { 469 e.printStackTrace(); 470 } 471 if (result.isUnsuccessfulRequest()) { 472 throw new EFhirClientException("Server returned error code " + result.getHttpStatus(), (OperationOutcome) result.getPayload()); 473 } 474 return (Parameters) result.getPayload(); 475 } 476 477 public ValueSet expandValueset(ValueSet source, Parameters expParams) { 478 recordUse(); 479 Parameters p = expParams == null ? new Parameters() : expParams.copy(); 480 p.addParameter().setName("valueSet").setResource(source); 481 org.hl7.fhir.dstu3.utils.client.network.ResourceRequest<Resource> result = null; 482 try { 483 result = client.issuePostRequest(resourceAddress.resolveOperationUri(ValueSet.class, "expand"), 484 ByteUtils.resourceToByteArray(p, false, isJson(getPreferredResourceFormat()), true), 485 withVer(getPreferredResourceFormat(), "3.0"), 486 generateHeaders(true), 487 "ValueSet/$expand?url=" + source.getUrl(), 488 timeoutExpand); 489 if (result.isUnsuccessfulRequest()) { 490 throw new EFhirClientException("Server returned error code " + result.getHttpStatus(), (OperationOutcome) result.getPayload()); 491 } 492 } catch (IOException e) { 493 e.printStackTrace(); 494 } 495 return result == null ? null : (ValueSet) result.getPayload(); 496 } 497 498 public String getAddress() { 499 return base; 500 } 501 502 public ConceptMap initializeClosure(String name) { 503 recordUse(); 504 Parameters params = new Parameters(); 505 params.addParameter().setName("name").setValue(new StringType(name)); 506 ResourceRequest<Resource> result = null; 507 try { 508 result = client.issuePostRequest(resourceAddress.resolveOperationUri(null, "closure", new HashMap<String, String>()), 509 ByteUtils.resourceToByteArray(params, false, isJson(getPreferredResourceFormat()), true), 510 withVer(getPreferredResourceFormat(), "3.0"), 511 generateHeaders(true), 512 "Closure?name=" + name, 513 timeoutNormal); 514 if (result.isUnsuccessfulRequest()) { 515 throw new EFhirClientException("Server returned error code " + result.getHttpStatus(), (OperationOutcome) result.getPayload()); 516 } 517 } catch (IOException e) { 518 e.printStackTrace(); 519 } 520 return result == null ? null : (ConceptMap) result.getPayload(); 521 } 522 523 public ConceptMap updateClosure(String name, Coding coding) { 524 recordUse(); 525 Parameters params = new Parameters(); 526 params.addParameter().setName("name").setValue(new StringType(name)); 527 params.addParameter().setName("concept").setValue(coding); 528 org.hl7.fhir.dstu3.utils.client.network.ResourceRequest<Resource> result = null; 529 try { 530 result = client.issuePostRequest(resourceAddress.resolveOperationUri(null, "closure", new HashMap<String, String>()), 531 ByteUtils.resourceToByteArray(params, false, isJson(getPreferredResourceFormat()), true), 532 withVer(getPreferredResourceFormat(), "3.0"), 533 generateHeaders(true), 534 "UpdateClosure?name=" + name, 535 timeoutOperation); 536 if (result.isUnsuccessfulRequest()) { 537 throw new EFhirClientException("Server returned error code " + result.getHttpStatus(), (OperationOutcome) result.getPayload()); 538 } 539 } catch (IOException e) { 540 e.printStackTrace(); 541 } 542 return result == null ? null : (ConceptMap) result.getPayload(); 543 } 544 545 546 public long getTimeout() { 547 return client.getTimeout(); 548 } 549 550 public void setTimeout(long timeout) { 551 client.setTimeout(timeout); 552 } 553 554 public ToolingClientLogger getLogger() { 555 return client.getLogger(); 556 } 557 558 public void setLogger(ToolingClientLogger logger) { 559 client.setLogger(logger); 560 } 561 562 public int getRetryCount() { 563 return client.getRetryCount(); 564 } 565 566 public void setRetryCount(int retryCount) { 567 client.setRetryCount(retryCount); 568 } 569 570 public void setClientHeaders(Iterable<HTTPHeader> headers) { 571 this.headers =new ArrayList<>(); 572 headers.forEach(this.headers::add); 573 } 574 575 private Iterable<HTTPHeader> generateHeaders(boolean hasBody) { 576 // Add any other headers 577 List<HTTPHeader> headers = new ArrayList<>(this.headers); 578 if (!Utilities.noString(userAgent)) { 579 headers.add(new HTTPHeader("User-Agent",userAgent)); 580 } 581 582 if (!Utilities.noString(acceptLanguage)) { 583 headers.add(new HTTPHeader("Accept-Language", acceptLanguage)); 584 } 585 586 if (hasBody && !Utilities.noString(contentLanguage)) { 587 headers.add(new HTTPHeader("Content-Language",contentLanguage)); 588 } 589 590 return headers; 591 } 592 593 public String getServerVersion() { 594 checkCapabilities(); 595 return capabilities == null ? null : capabilities.getSoftware().getVersion(); 596 } 597 598 private void recordUse() { 599 useCount++; 600 } 601 602 public Bundle search(String type, String criteria) { 603 recordUse(); 604 return fetchFeed(Utilities.pathURL(base, type+criteria)); 605 } 606 607} 608