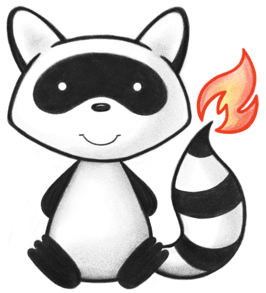
001package org.hl7.fhir.dstu3.utils.client.network; 002 003import java.io.IOException; 004import java.net.URI; 005import java.util.Collections; 006import java.util.Map; 007import java.util.concurrent.TimeUnit; 008 009import org.hl7.fhir.dstu3.model.Bundle; 010import org.hl7.fhir.dstu3.model.Resource; 011import org.hl7.fhir.dstu3.utils.client.EFhirClientException; 012import org.hl7.fhir.utilities.ToolingClientLogger; 013 014import org.hl7.fhir.utilities.http.HTTPHeader; 015import org.hl7.fhir.utilities.http.HTTPRequest; 016 017public class Client { 018 019 public static final String DEFAULT_CHARSET = "UTF-8"; 020 private static final long DEFAULT_TIMEOUT = 5000; 021 private ToolingClientLogger logger; 022 private int retryCount; 023 private long timeout = DEFAULT_TIMEOUT; 024 private String base; 025 026 public String getBase() { 027 return base; 028 } 029 030 public void setBase(String base) { 031 this.base = base; 032 } 033 034 035 public ToolingClientLogger getLogger() { 036 return logger; 037 } 038 039 public void setLogger(ToolingClientLogger logger) { 040 this.logger = logger; 041 } 042 043 public int getRetryCount() { 044 return retryCount; 045 } 046 047 public void setRetryCount(int retryCount) { 048 this.retryCount = retryCount; 049 } 050 051 public long getTimeout() { 052 return timeout; 053 } 054 055 public void setTimeout(long timeout) { 056 this.timeout = timeout; 057 } 058 059 public <T extends Resource> ResourceRequest<T> issueOptionsRequest(URI optionsUri, 060 String resourceFormat, 061 String message, 062 long timeout) throws IOException { 063 064 HTTPRequest request = new HTTPRequest() 065 .withUrl(optionsUri.toURL()) 066 .withMethod(HTTPRequest.HttpMethod.OPTIONS); 067 068 return executeFhirRequest(request, resourceFormat, Collections.emptyList(), message, retryCount, timeout); 069 } 070 071 public <T extends Resource> ResourceRequest<T> issueGetResourceRequest(URI resourceUri, 072 String resourceFormat, 073 Iterable<HTTPHeader> headers, 074 String message, 075 long timeout) throws IOException { 076 HTTPRequest request = new HTTPRequest() 077 .withUrl(resourceUri.toURL()) 078 .withMethod(HTTPRequest.HttpMethod.GET); 079 return executeFhirRequest(request, resourceFormat, headers, message, retryCount, timeout); 080 } 081 082 public int tester(int trytry) { 083 return 5; 084 } 085 public <T extends Resource> ResourceRequest<T> issuePutRequest(URI resourceUri, 086 byte[] payload, 087 String resourceFormat, 088 String message, 089 long timeout) throws IOException { 090 return issuePutRequest(resourceUri, payload, resourceFormat, Collections.emptyList(), message, timeout); 091 } 092 093 public <T extends Resource> ResourceRequest<T> issuePutRequest(URI resourceUri, 094 byte[] payload, 095 String resourceFormat, 096 Iterable<HTTPHeader> headers, 097 String message, 098 long timeout) throws IOException { 099 if (payload == null) throw new EFhirClientException("PUT requests require a non-null payload"); 100 101 HTTPRequest request = new HTTPRequest() 102 .withUrl(resourceUri.toURL()) 103 .withMethod(HTTPRequest.HttpMethod.PUT) 104 .withBody(payload); 105 return executeFhirRequest(request, resourceFormat, headers, message, retryCount, timeout); 106 } 107 108 public <T extends Resource> ResourceRequest<T> issuePostRequest(URI resourceUri, 109 byte[] payload, 110 String resourceFormat, 111 String message, 112 long timeout) throws IOException { 113 return issuePostRequest(resourceUri, payload, resourceFormat, Collections.emptyList(), message, timeout); 114 } 115 116 public <T extends Resource> ResourceRequest<T> issuePostRequest(URI resourceUri, 117 byte[] payload, 118 String resourceFormat, 119 Iterable<HTTPHeader> headers, 120 String message, 121 long timeout) throws IOException { 122 if (payload == null) throw new EFhirClientException("POST requests require a non-null payload"); 123 124 HTTPRequest request = new HTTPRequest() 125 .withUrl(resourceUri.toURL()) 126 .withMethod(HTTPRequest.HttpMethod.POST) 127 .withBody(payload); 128 return executeFhirRequest(request, resourceFormat, headers, message, retryCount, timeout); 129 } 130 131 public boolean issueDeleteRequest(URI resourceUri) throws IOException { 132 HTTPRequest request = new HTTPRequest() 133 .withUrl(resourceUri.toURL()) 134 .withMethod(HTTPRequest.HttpMethod.DELETE); 135 return executeFhirRequest(request, null, Collections.emptyList(), null, retryCount, timeout).isSuccessfulRequest(); 136 } 137 138 public Bundle issueGetFeedRequest(URI resourceUri, String resourceFormat) throws IOException { 139 HTTPRequest request = new HTTPRequest() 140 .withUrl(resourceUri.toURL()) 141 .withMethod(HTTPRequest.HttpMethod.GET); 142 return executeBundleRequest(request, resourceFormat, Collections.emptyList(), null, retryCount, timeout); 143 } 144 145 public Bundle issuePostFeedRequest(URI resourceUri, 146 Map<String, String> parameters, 147 String resourceName, 148 Resource resource, 149 String resourceFormat) throws IOException { 150 String boundary = "----WebKitFormBoundarykbMUo6H8QaUnYtRy"; 151 byte[] payload = ByteUtils.encodeFormSubmission(parameters, resourceName, resource, boundary); 152 153 HTTPRequest request = new HTTPRequest() 154 .withUrl(resourceUri.toURL()) 155 .withMethod(HTTPRequest.HttpMethod.POST) 156 .withBody(payload); 157 158 return executeBundleRequest(request, resourceFormat, Collections.emptyList(), null, retryCount, timeout); 159 } 160 161 public Bundle postBatchRequest(URI resourceUri, 162 byte[] payload, 163 String resourceFormat, 164 String message, 165 int timeout) throws IOException { 166 if (payload == null) throw new EFhirClientException("POST requests require a non-null payload"); 167 168 HTTPRequest request = new HTTPRequest() 169 .withUrl(resourceUri.toURL()) 170 .withMethod(HTTPRequest.HttpMethod.POST) 171 .withBody(payload); 172 return executeBundleRequest(request, resourceFormat, Collections.emptyList(), message, retryCount, timeout); 173 } 174 175 public <T extends Resource> Bundle executeBundleRequest(HTTPRequest request, 176 String resourceFormat, 177 Iterable<HTTPHeader> headers, 178 String message, 179 int retryCount, 180 long timeout) throws IOException { 181 return new FhirRequestBuilder(request, base) 182 .withLogger(logger) 183 .withResourceFormat(resourceFormat) 184 .withRetryCount(retryCount) 185 .withMessage(message) 186 .withHeaders(headers == null ? Collections.emptyList() : headers) 187 .withTimeout(timeout, TimeUnit.MILLISECONDS) 188 .executeAsBatch(); 189 } 190 191 public <T extends Resource> ResourceRequest<T> executeFhirRequest(HTTPRequest request, 192 String resourceFormat, 193 Iterable<HTTPHeader> headers, 194 String message, 195 int retryCount, 196 long timeout) throws IOException { 197 return new FhirRequestBuilder(request, base) 198 .withLogger(logger) 199 .withResourceFormat(resourceFormat) 200 .withRetryCount(retryCount) 201 .withMessage(message) 202 .withHeaders(headers == null ? Collections.emptyList() : headers) 203 .withTimeout(timeout, TimeUnit.MILLISECONDS) 204 .execute(); 205 } 206}