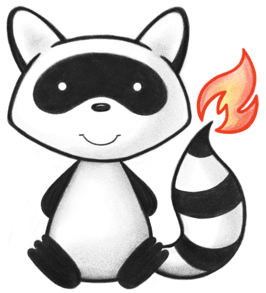
001package org.hl7.fhir.dstu3.utils.client.network; 002 003import java.util.ArrayList; 004import java.util.List; 005import java.util.stream.Collectors; 006 007import org.hl7.fhir.exceptions.FHIRException; 008import org.hl7.fhir.utilities.http.HTTPHeader; 009 010/** 011 * Generic Implementation of Client Headers. 012 * 013 * Stores a list of headers for HTTP calls to the TX server. Users can implement their own instance if they desire 014 * specific, custom behavior. 015 */ 016public class ClientHeaders { 017 018 private final ArrayList<HTTPHeader> headers; 019 020 public ClientHeaders() { 021 this.headers = new ArrayList<>(); 022 } 023 024 public ClientHeaders(ArrayList<HTTPHeader> headers) { 025 this.headers = headers; 026 } 027 028 public ArrayList<HTTPHeader> headers() { 029 return headers; 030 } 031 032 /** 033 * Add a header to the list of stored headers for network operations. 034 * 035 * @param header {@link HTTPHeader} to add to the list. 036 * @throws FHIRException if the header being added is a duplicate. 037 */ 038 public ClientHeaders addHeader(HTTPHeader header) throws FHIRException { 039 if (headers.contains(header)) { 040 throw new FHIRException("Attempting to add duplicate header, <" + header.getName() + ", " 041 + header.getValue() + ">."); 042 } 043 headers.add(header); 044 return this; 045 } 046 047 /** 048 * Add a header to the list of stored headers for network operations. 049 * 050 * @param headerList {@link List} of {@link HTTPHeader} to add. 051 * @throws FHIRException if any of the headers being added is a duplicate. 052 */ 053 public ClientHeaders addHeaders(List<HTTPHeader> headerList) throws FHIRException { 054 headerList.forEach(this::addHeader); 055 return this; 056 } 057 058 /** 059 * Removes the passed in header from the list of stored headers. 060 * @param header {@link HTTPHeader} to remove from the list. 061 * @throws FHIRException if the header passed in does not exist within the stored list. 062 */ 063 public ClientHeaders removeHeader(HTTPHeader header) throws FHIRException { 064 if (!headers.remove(header)) { 065 throw new FHIRException("Attempting to remove header, <" + header.getName() + ", " 066 + header.getValue() + ">, from GenericClientHeaders that is not currently stored."); 067 } 068 return this; 069 } 070 071 /** 072 * Removes the passed in headers from the list of stored headers. 073 * @param headerList {@link List} of {@link HTTPHeader} to remove. 074 * @throws FHIRException if any of the headers passed in does not exist within the stored list. 075 */ 076 public ClientHeaders removeHeaders(List<HTTPHeader> headerList) throws FHIRException { 077 headerList.forEach(this::removeHeader); 078 return this; 079 } 080 081 /** 082 * Clears all stored {@link HTTPHeader}. 083 */ 084 public ClientHeaders clearHeaders() { 085 headers.clear(); 086 return this; 087 } 088 089 @Override 090 public String toString() { 091 return this.headers.stream() 092 .map(header -> "\t" + header.getName() + ":" + header.getValue()) 093 .collect(Collectors.joining(",\n", "{\n", "\n}")); 094 } 095}