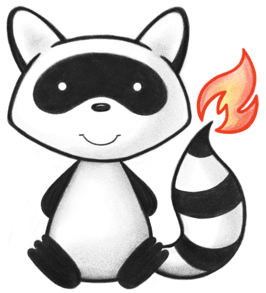
001package org.hl7.fhir.dstu3.utils.client.network; 002 003import java.io.IOException; 004import java.util.ArrayList; 005import java.util.Collections; 006import java.util.List; 007import java.util.Map; 008import java.util.concurrent.TimeUnit; 009 010import javax.annotation.Nonnull; 011 012import org.apache.commons.lang3.StringUtils; 013import org.hl7.fhir.dstu3.formats.IParser; 014import org.hl7.fhir.dstu3.formats.JsonParser; 015import org.hl7.fhir.dstu3.formats.XmlParser; 016import org.hl7.fhir.dstu3.model.Bundle; 017import org.hl7.fhir.dstu3.model.OperationOutcome; 018import org.hl7.fhir.dstu3.model.Resource; 019import org.hl7.fhir.dstu3.utils.ResourceUtilities; 020import org.hl7.fhir.dstu3.utils.client.EFhirClientException; 021import org.hl7.fhir.dstu3.utils.client.ResourceFormat; 022import org.hl7.fhir.exceptions.FHIRException; 023import org.hl7.fhir.utilities.MimeType; 024import org.hl7.fhir.utilities.ToolingClientLogger; 025import org.hl7.fhir.utilities.settings.FhirSettings; 026 027import okhttp3.Authenticator; 028import okhttp3.Credentials; 029import okhttp3.Headers; 030import okhttp3.OkHttpClient; 031import okhttp3.Request; 032import okhttp3.Response; 033 034public class FhirRequestBuilder { 035 036 protected static final String HTTP_PROXY_USER = "http.proxyUser"; 037 protected static final String HTTP_PROXY_PASS = "http.proxyPassword"; 038 protected static final String HEADER_PROXY_AUTH = "Proxy-Authorization"; 039 protected static final String LOCATION_HEADER = "location"; 040 protected static final String CONTENT_LOCATION_HEADER = "content-location"; 041 protected static final String DEFAULT_CHARSET = "UTF-8"; 042 /** 043 * The singleton instance of the HttpClient, used for all requests. 044 */ 045 private static OkHttpClient okHttpClient; 046 private final Request.Builder httpRequest; 047 private String resourceFormat = null; 048 private Headers headers = null; 049 private String message = null; 050 private int retryCount = 1; 051 /** 052 * The timeout quantity. Used in combination with {@link FhirRequestBuilder#timeoutUnit}. 053 */ 054 private long timeout = 5000; 055 /** 056 * Time unit for {@link FhirRequestBuilder#timeout}. 057 */ 058 private TimeUnit timeoutUnit = TimeUnit.MILLISECONDS; 059 /** 060 * {@link ToolingClientLogger} for log output. 061 */ 062 private ToolingClientLogger logger = null; 063 private String source; 064 065 public FhirRequestBuilder(Request.Builder httpRequest, String source) { 066 this.httpRequest = httpRequest; 067 this.source = source; 068 } 069 070 /** 071 * Adds necessary default headers, formatting headers, and any passed in {@link Headers} to the passed in 072 * {@link okhttp3.Request.Builder} 073 * 074 * @param request {@link okhttp3.Request.Builder} to add headers to. 075 * @param format Expected {@link Resource} format. 076 * @param headers Any additional {@link Headers} to add to the request. 077 */ 078 protected static void formatHeaders(Request.Builder request, String format, Headers headers) { 079 addDefaultHeaders(request, headers); 080 if (format != null) addResourceFormatHeaders(request, format); 081 if (headers != null) addHeaders(request, headers); 082 } 083 084 /** 085 * Adds necessary headers for all REST requests. 086 * <li>User-Agent : hapi-fhir-tooling-client</li> 087 * 088 * @param request {@link Request.Builder} to add default headers to. 089 */ 090 protected static void addDefaultHeaders(Request.Builder request, Headers headers) { 091 if (headers == null || !headers.names().contains("User-Agent")) { 092 request.addHeader("User-Agent", "hapi-fhir-tooling-client"); 093 } 094 } 095 096 /** 097 * Adds necessary headers for the given resource format provided. 098 * 099 * @param request {@link Request.Builder} to add default headers to. 100 */ 101 protected static void addResourceFormatHeaders(Request.Builder request, String format) { 102 request.addHeader("Accept", format); 103 request.addHeader("Content-Type", format + ";charset=" + DEFAULT_CHARSET); 104 } 105 106 /** 107 * Iterates through the passed in {@link Headers} and adds them to the provided {@link Request.Builder}. 108 * 109 * @param request {@link Request.Builder} to add headers to. 110 * @param headers {@link Headers} to add to request. 111 */ 112 protected static void addHeaders(Request.Builder request, Headers headers) { 113 if (headers != null) { 114 headers.forEach(header -> request.addHeader(header.getFirst(), header.getSecond())); 115 } 116 } 117 118 /** 119 * Returns true if any of the {@link org.hl7.fhir.dstu3.model.OperationOutcome.OperationOutcomeIssueComponent} within the 120 * provided {@link OperationOutcome} have an {@link org.hl7.fhir.dstu3.model.OperationOutcome.IssueSeverity} of 121 * {@link org.hl7.fhir.dstu3.model.OperationOutcome.IssueSeverity#ERROR} or 122 * {@link org.hl7.fhir.dstu3.model.OperationOutcome.IssueSeverity#FATAL} 123 * 124 * @param oo {@link OperationOutcome} to evaluate. 125 * @return {@link Boolean#TRUE} if an error exists. 126 */ 127 protected static boolean hasError(OperationOutcome oo) { 128 return (oo.getIssue().stream() 129 .anyMatch(issue -> issue.getSeverity() == OperationOutcome.IssueSeverity.ERROR 130 || issue.getSeverity() == OperationOutcome.IssueSeverity.FATAL)); 131 } 132 133 /** 134 * Extracts the 'location' header from the passes in {@link Headers}. If no value for 'location' exists, the 135 * value for 'content-location' is returned. If neither header exists, we return null. 136 * 137 * @param headers {@link Headers} to evaluate 138 * @return {@link String} header value, or null if no location headers are set. 139 */ 140 protected static String getLocationHeader(Headers headers) { 141 Map<String, List<String>> headerMap = headers.toMultimap(); 142 if (headerMap.containsKey(LOCATION_HEADER)) { 143 return headerMap.get(LOCATION_HEADER).get(0); 144 } else if (headerMap.containsKey(CONTENT_LOCATION_HEADER)) { 145 return headerMap.get(CONTENT_LOCATION_HEADER).get(0); 146 } else { 147 return null; 148 } 149 } 150 151 /** 152 * We only ever want to have one copy of the HttpClient kicking around at any given time. If we need to make changes 153 * to any configuration, such as proxy settings, timeout, caches, etc, we can do a per-call configuration through 154 * the {@link OkHttpClient#newBuilder()} method. That will return a builder that shares the same connection pool, 155 * dispatcher, and configuration with the original client. 156 * </p> 157 * The {@link OkHttpClient} uses the proxy auth properties set in the current system properties. The reason we don't 158 * set the proxy address and authentication explicitly, is due to the fact that this class is often used in conjunction 159 * with other http client tools which rely on the system.properties settings to determine proxy settings. It's easier 160 * to keep the method consistent across the board. ...for now. 161 * 162 * @return {@link OkHttpClient} instance 163 */ 164 protected OkHttpClient getHttpClient() { 165 if (FhirSettings.isProhibitNetworkAccess()) { 166 throw new FHIRException("Network Access is prohibited in this context"); 167 } 168 169 if (okHttpClient == null) { 170 okHttpClient = new OkHttpClient(); 171 } 172 173 Authenticator proxyAuthenticator = getAuthenticator(); 174 175 return okHttpClient.newBuilder() 176 .addInterceptor(new RetryInterceptor(retryCount)) 177 .connectTimeout(timeout, timeoutUnit) 178 .writeTimeout(timeout, timeoutUnit) 179 .readTimeout(timeout, timeoutUnit) 180 .proxyAuthenticator(proxyAuthenticator) 181 .build(); 182 } 183 184 @Nonnull 185 private static Authenticator getAuthenticator() { 186 return (route, response) -> { 187 final String httpProxyUser = System.getProperty(HTTP_PROXY_USER); 188 final String httpProxyPass = System.getProperty(HTTP_PROXY_PASS); 189 if (httpProxyUser != null && httpProxyPass != null) { 190 String credential = Credentials.basic(httpProxyUser, httpProxyPass); 191 return response.request().newBuilder() 192 .header(HEADER_PROXY_AUTH, credential) 193 .build(); 194 } 195 return response.request().newBuilder().build(); 196 }; 197 } 198 199 public FhirRequestBuilder withResourceFormat(String resourceFormat) { 200 this.resourceFormat = resourceFormat; 201 return this; 202 } 203 204 public FhirRequestBuilder withHeaders(Headers headers) { 205 this.headers = headers; 206 return this; 207 } 208 209 public FhirRequestBuilder withMessage(String message) { 210 this.message = message; 211 return this; 212 } 213 214 public FhirRequestBuilder withRetryCount(int retryCount) { 215 this.retryCount = retryCount; 216 return this; 217 } 218 219 public FhirRequestBuilder withLogger(ToolingClientLogger logger) { 220 this.logger = logger; 221 return this; 222 } 223 224 public FhirRequestBuilder withTimeout(long timeout, TimeUnit unit) { 225 this.timeout = timeout; 226 this.timeoutUnit = unit; 227 return this; 228 } 229 230 protected Request buildRequest() { 231 return httpRequest.build(); 232 } 233 234 public <T extends Resource> ResourceRequest<T> execute() throws IOException { 235 formatHeaders(httpRequest, resourceFormat, headers); 236 final Request request = httpRequest.build(); 237 log(request.method(), request.url().toString(), request.headers(), request.body() != null ? request.body().toString().getBytes() : null); 238 Response response = getHttpClient().newCall(request).execute(); 239 T resource = unmarshalReference(response, resourceFormat); 240 return new ResourceRequest<T>(resource, response.code(), getLocationHeader(response.headers())); 241 } 242 243 public Bundle executeAsBatch() throws IOException { 244 formatHeaders(httpRequest, resourceFormat, null); 245 final Request request = httpRequest.build(); 246 log(request.method(), request.url().toString(), request.headers(), request.body() != null ? request.body().toString().getBytes() : null); 247 248 Response response = getHttpClient().newCall(request).execute(); 249 return unmarshalFeed(response, resourceFormat); 250 } 251 252 /** 253 * Unmarshalls a resource from the response stream. 254 */ 255 @SuppressWarnings("unchecked") 256 protected <T extends Resource> T unmarshalReference(Response response, String format) { 257 T resource = null; 258 OperationOutcome error = null; 259 260 if (response.body() != null) { 261 try { 262 byte[] body = response.body().bytes(); 263 log(response.code(), response.headers(), body); 264 resource = (T) getParser(format).parse(body); 265 if (resource instanceof OperationOutcome && hasError((OperationOutcome) resource)) { 266 error = (OperationOutcome) resource; 267 } 268 } catch (IOException ioe) { 269 throw new EFhirClientException("Error reading Http Response from "+source+": " + ioe.getMessage(), ioe); 270 } catch (Exception e) { 271 throw new EFhirClientException("Error parsing response message from "+source+": " + e.getMessage(), e); 272 } 273 } 274 275 if (error != null) { 276 throw new EFhirClientException("Error from server: " + ResourceUtilities.getErrorDescription(error), error); 277 } 278 279 return resource; 280 } 281 282 /** 283 * Unmarshalls Bundle from response stream. 284 */ 285 protected Bundle unmarshalFeed(Response response, String format) { 286 Bundle feed = null; 287 OperationOutcome error = null; 288 try { 289 byte[] body = response.body().bytes(); 290 log(response.code(), response.headers(), body); 291 String contentType = response.header("Content-Type"); 292 if (body != null) { 293 if (contentType.contains(ResourceFormat.RESOURCE_XML.getHeader()) || contentType.contains(ResourceFormat.RESOURCE_JSON.getHeader()) || contentType.contains("text/xml+fhir")) { 294 Resource rf = getParser(format).parse(body); 295 if (rf instanceof Bundle) 296 feed = (Bundle) rf; 297 else if (rf instanceof OperationOutcome && hasError((OperationOutcome) rf)) { 298 error = (OperationOutcome) rf; 299 } else { 300 throw new EFhirClientException("Error reading server response: a resource was returned instead"); 301 } 302 } 303 } 304 } catch (IOException ioe) { 305 throw new EFhirClientException("Error reading Http Response from "+source+": "+ioe.getMessage(), ioe); 306 } catch (Exception e) { 307 throw new EFhirClientException("Error parsing response message from "+source+":"+e.getMessage(), e); 308 } 309 if (error != null) { 310 throw new EFhirClientException("Error from "+source+": " + ResourceUtilities.getErrorDescription(error), error); 311 } 312 return feed; 313 } 314 315 /** 316 * Returns the appropriate parser based on the format type passed in. Defaults to XML parser if a blank format is 317 * provided...because reasons. 318 * <p> 319 * Currently supports only "json" and "xml" formats. 320 * 321 * @param format One of "json" or "xml". 322 * @return {@link JsonParser} or {@link XmlParser} 323 */ 324 protected IParser getParser(String format) { 325 if (StringUtils.isBlank(format)) { 326 format = ResourceFormat.RESOURCE_XML.getHeader(); 327 } 328 MimeType mt = new MimeType(format); 329 if (mt.getBase().equalsIgnoreCase(ResourceFormat.RESOURCE_JSON.getHeader())) { 330 return new JsonParser(); 331 } else if (mt.getBase().equalsIgnoreCase(ResourceFormat.RESOURCE_XML.getHeader())) { 332 return new XmlParser(); 333 } else { 334 throw new EFhirClientException("Invalid format: " + format); 335 } 336 } 337 338 /** 339 * Logs the given {@link Request}, using the current {@link ToolingClientLogger}. If the current 340 * {@link FhirRequestBuilder#logger} is null, no action is taken. 341 * 342 * @param method HTTP request method 343 * @param url request URL 344 * @param requestHeaders {@link Headers} for request 345 * @param requestBody Byte array request 346 */ 347 protected void log(String method, String url, Headers requestHeaders, byte[] requestBody) { 348 if (logger != null) { 349 List<String> headerList = new ArrayList<>(Collections.emptyList()); 350 Map<String, List<String>> headerMap = requestHeaders.toMultimap(); 351 headerMap.keySet().forEach(key -> headerMap.get(key).forEach(value -> headerList.add(key + ":" + value))); 352 353 logger.logRequest(method, url, headerList, requestBody); 354 } 355 356 } 357 358 /** 359 * Logs the given {@link Response}, using the current {@link ToolingClientLogger}. If the current 360 * {@link FhirRequestBuilder#logger} is null, no action is taken. 361 * 362 * @param responseCode HTTP response code 363 * @param responseHeaders {@link Headers} from response 364 * @param responseBody Byte array response 365 */ 366 protected void log(int responseCode, Headers responseHeaders, byte[] responseBody) { 367 if (logger != null) { 368 List<String> headerList = new ArrayList<>(Collections.emptyList()); 369 Map<String, List<String>> headerMap = responseHeaders.toMultimap(); 370 headerMap.keySet().forEach(key -> headerMap.get(key).forEach(value -> headerList.add(key + ":" + value))); 371 372 try { 373 if (logger != null) { 374 logger.logResponse(Integer.toString(responseCode), headerList, responseBody, 0); 375 } 376 } catch (Exception e) { 377 System.out.println("Error parsing response body passed in to logger ->\n" + e.getLocalizedMessage()); 378 } 379 } 380// else { // TODO fix logs 381// System.out.println("Call to log HTTP response with null ToolingClientLogger set... are you forgetting to " + 382// "initialize your logger?"); 383// } 384 } 385}