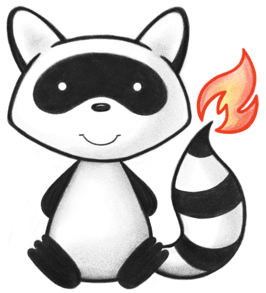
001package org.hl7.fhir.dstu3.utils.validation; 002 003import java.io.IOException; 004import java.io.InputStream; 005import java.util.List; 006 007import org.hl7.fhir.dstu3.elementmodel.Manager.FhirFormat; 008import org.hl7.fhir.dstu3.model.StructureDefinition; 009import org.hl7.fhir.dstu3.utils.ValidationProfileSet; 010import org.hl7.fhir.dstu3.utils.validation.constants.BestPracticeWarningLevel; 011import org.hl7.fhir.dstu3.utils.validation.constants.CheckDisplayOption; 012import org.hl7.fhir.dstu3.utils.validation.constants.IdStatus; 013import org.hl7.fhir.exceptions.FHIRException; 014import org.hl7.fhir.utilities.validation.ValidationMessage; 015 016/* 017 Copyright (c) 2011+, HL7, Inc. 018 All rights reserved. 019 020 Redistribution and use in source and binary forms, with or without modification, 021 are permitted provided that the following conditions are met: 022 023 * Redistributions of source code must retain the above copyright notice, this 024 list of conditions and the following disclaimer. 025 * Redistributions in binary form must reproduce the above copyright notice, 026 this list of conditions and the following disclaimer in the documentation 027 and/or other materials provided with the distribution. 028 * Neither the name of HL7 nor the names of its contributors may be used to 029 endorse or promote products derived from this software without specific 030 prior written permission. 031 032 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 033 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 034 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 035 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 036 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 037 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 038 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 039 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 040 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 041 POSSIBILITY OF SUCH DAMAGE. 042 043 */ 044 045 046import com.google.gson.JsonObject; 047 048/** 049 * Interface to the instance validator. This takes a resource, in one of many forms, and 050 * checks whether it is valid 051 * 052 * @author Grahame Grieve 053 * 054 */ 055@Deprecated 056public interface IResourceValidator { 057 058 /** 059 * how much to check displays for coded elements 060 * @return 061 */ 062 CheckDisplayOption getCheckDisplay(); 063 void setCheckDisplay(CheckDisplayOption checkDisplay); 064 065 /** 066 * whether the resource must have an id or not (depends on context) 067 * 068 * @return 069 */ 070 071 IdStatus getResourceIdRule(); 072 void setResourceIdRule(IdStatus resourceIdRule); 073 074 /** 075 * whether the validator should enforce best practice guidelines 076 * as defined by various HL7 committees 077 * 078 */ 079 BestPracticeWarningLevel getBasePracticeWarningLevel(); 080 IResourceValidator setBestPracticeWarningLevel(BestPracticeWarningLevel value); 081 082 IValidatorResourceFetcher getFetcher(); 083 IResourceValidator setFetcher(IValidatorResourceFetcher value); 084 085 IValidationPolicyAdvisor getPolicyAdvisor(); 086 IResourceValidator setPolicyAdvisor(IValidationPolicyAdvisor advisor); 087 088 boolean isNoBindingMsgSuppressed(); 089 IResourceValidator setNoBindingMsgSuppressed(boolean noBindingMsgSuppressed); 090 091 boolean isNoInvariantChecks(); 092 IResourceValidator setNoInvariantChecks(boolean value) ; 093 094 boolean isNoTerminologyChecks(); 095 IResourceValidator setNoTerminologyChecks(boolean noTerminologyChecks); 096 097 /** 098 * Whether being unable to resolve a profile in found in Resource.meta.profile or ElementDefinition.type.profile or targetProfile is an error or just a warning 099 * @return 100 */ 101 boolean isErrorForUnknownProfiles(); 102 void setErrorForUnknownProfiles(boolean errorForUnknownProfiles); 103 104 /** 105 * Validate suite 106 * 107 * you can validate one of the following representations of resources: 108 * 109 * stream - provide a format - this is the preferred choice 110 * 111 * Use one of these two if the content is known to be valid XML/JSON, and already parsed 112 * - a DOM element or Document 113 * - a Json Object 114 * 115 * In order to use these, the content must already be parsed - e.g. it must syntactically valid 116 * - a native resource 117 * - a elementmodel resource 118 * 119 * in addition, you can pass one or more profiles ti validate beyond the base standard - as structure definitions or canonical URLs 120 * @throws IOException 121 */ 122 void validate(Object Context, List<ValidationMessage> errors, org.hl7.fhir.dstu3.elementmodel.Element element) throws FHIRException, IOException; 123 void validate(Object Context, List<ValidationMessage> errors, org.hl7.fhir.dstu3.elementmodel.Element element, ValidationProfileSet profiles) throws FHIRException, IOException; 124 @Deprecated 125 void validate(Object Context, List<ValidationMessage> errors, org.hl7.fhir.dstu3.elementmodel.Element element, String profile) throws FHIRException, IOException; 126 @Deprecated 127 void validate(Object Context, List<ValidationMessage> errors, org.hl7.fhir.dstu3.elementmodel.Element element, StructureDefinition profile) throws FHIRException, IOException; 128 129 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, InputStream stream, FhirFormat format) throws FHIRException, IOException; 130 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, InputStream stream, FhirFormat format, ValidationProfileSet profiles) throws FHIRException, IOException; 131 @Deprecated 132 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, InputStream stream, FhirFormat format, String profile) throws FHIRException, IOException; 133 @Deprecated 134 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, InputStream stream, FhirFormat format, StructureDefinition profile) throws FHIRException, IOException; 135 136 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.hl7.fhir.dstu3.model.Resource resource) throws FHIRException, IOException; 137 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.hl7.fhir.dstu3.model.Resource resource, ValidationProfileSet profiles) throws FHIRException, IOException; 138 @Deprecated 139 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.hl7.fhir.dstu3.model.Resource resource, String profile) throws FHIRException, IOException; 140 @Deprecated 141 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.hl7.fhir.dstu3.model.Resource resource, StructureDefinition profile) throws FHIRException, IOException; 142 143 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.w3c.dom.Element element) throws FHIRException, IOException; 144 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.w3c.dom.Element element, ValidationProfileSet profiles) throws FHIRException, IOException; 145 @Deprecated 146 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.w3c.dom.Element element, String profile) throws FHIRException, IOException; 147 @Deprecated 148 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.w3c.dom.Element element, StructureDefinition profile) throws FHIRException, IOException; 149 150 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.w3c.dom.Document document) throws FHIRException, IOException; 151 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.w3c.dom.Document document, ValidationProfileSet profiles) throws FHIRException, IOException; 152 @Deprecated 153 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.w3c.dom.Document document, String profile) throws FHIRException, IOException; 154 @Deprecated 155 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, org.w3c.dom.Document document, StructureDefinition profile) throws FHIRException, IOException; 156 157 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, JsonObject object) throws FHIRException, IOException; 158 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, JsonObject object, ValidationProfileSet profiles) throws FHIRException, IOException; 159 @Deprecated 160 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, JsonObject object, String profile) throws FHIRException, IOException; 161 @Deprecated 162 org.hl7.fhir.dstu3.elementmodel.Element validate(Object Context, List<ValidationMessage> errors, JsonObject object, StructureDefinition profile) throws FHIRException, IOException; 163 164 165}