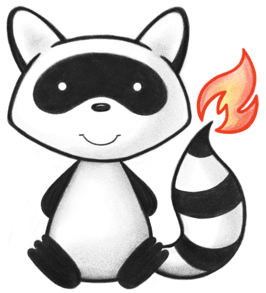
001/* 002 * #%L 003 * HAPI FHIR Structures - HL7.org DSTU2 004 * %% 005 * Copyright (C) 2014 - 2015 University Health Network 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.provider.dstu2hl7org; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.api.BundleInclusionRule; 024import ca.uhn.fhir.model.api.Include; 025import ca.uhn.fhir.model.api.ResourceMetadataKeyEnum; 026import ca.uhn.fhir.model.valueset.BundleEntrySearchModeEnum; 027import ca.uhn.fhir.model.valueset.BundleEntryTransactionMethodEnum; 028import ca.uhn.fhir.model.valueset.BundleTypeEnum; 029import ca.uhn.fhir.rest.api.BundleLinks; 030import ca.uhn.fhir.rest.api.Constants; 031import ca.uhn.fhir.rest.api.IVersionSpecificBundleFactory; 032import ca.uhn.fhir.rest.server.RestfulServerUtils; 033import ca.uhn.fhir.util.ResourceReferenceInfo; 034import jakarta.annotation.Nonnull; 035import org.hl7.fhir.dstu2.model.Bundle; 036import org.hl7.fhir.dstu2.model.Bundle.BundleEntryComponent; 037import org.hl7.fhir.dstu2.model.Bundle.BundleLinkComponent; 038import org.hl7.fhir.dstu2.model.Bundle.SearchEntryMode; 039import org.hl7.fhir.dstu2.model.DomainResource; 040import org.hl7.fhir.dstu2.model.IdType; 041import org.hl7.fhir.dstu2.model.InstantType; 042import org.hl7.fhir.dstu2.model.Resource; 043import org.hl7.fhir.instance.model.api.IAnyResource; 044import org.hl7.fhir.instance.model.api.IBaseResource; 045import org.hl7.fhir.instance.model.api.IIdType; 046import org.hl7.fhir.instance.model.api.IPrimitiveType; 047 048import java.math.BigDecimal; 049import java.util.ArrayList; 050import java.util.Date; 051import java.util.HashSet; 052import java.util.List; 053import java.util.Set; 054import java.util.UUID; 055 056import static org.apache.commons.lang3.StringUtils.isBlank; 057import static org.apache.commons.lang3.StringUtils.isNotBlank; 058 059public class Dstu2Hl7OrgBundleFactory implements IVersionSpecificBundleFactory { 060 061 private String myBase; 062 private Bundle myBundle; 063 private FhirContext myContext; 064 065 public Dstu2Hl7OrgBundleFactory(FhirContext theContext) { 066 myContext = theContext; 067 } 068 069 @Override 070 public void addResourcesToBundle( 071 List<IBaseResource> theResult, 072 BundleTypeEnum theBundleType, 073 String theServerBase, 074 BundleInclusionRule theBundleInclusionRule, 075 Set<Include> theIncludes) { 076 ensureBundle(); 077 078 List<IAnyResource> includedResources = new ArrayList<IAnyResource>(); 079 Set<IIdType> addedResourceIds = new HashSet<IIdType>(); 080 081 for (IBaseResource next : theResult) { 082 if (next.getIdElement().isEmpty() == false) { 083 addedResourceIds.add(next.getIdElement()); 084 } 085 } 086 087 for (IBaseResource next : theResult) { 088 089 Set<String> containedIds = new HashSet<String>(); 090 091 if (next instanceof DomainResource) { 092 for (Resource nextContained : ((DomainResource) next).getContained()) { 093 if (isNotBlank(nextContained.getId())) { 094 containedIds.add(nextContained.getId()); 095 } 096 } 097 } 098 099 List<ResourceReferenceInfo> references = myContext.newTerser().getAllResourceReferences(next); 100 do { 101 List<IAnyResource> addedResourcesThisPass = new ArrayList<IAnyResource>(); 102 103 for (ResourceReferenceInfo nextRefInfo : references) { 104 if (theBundleInclusionRule != null 105 && !theBundleInclusionRule.shouldIncludeReferencedResource(nextRefInfo, theIncludes)) { 106 continue; 107 } 108 109 IAnyResource nextRes = 110 (IAnyResource) nextRefInfo.getResourceReference().getResource(); 111 if (nextRes != null) { 112 if (nextRes.getIdElement().hasIdPart()) { 113 if (containedIds.contains(nextRes.getIdElement().getValue())) { 114 // Don't add contained IDs as top level resources 115 continue; 116 } 117 118 IIdType id = nextRes.getIdElement(); 119 if (id.hasResourceType() == false) { 120 String resName = myContext.getResourceType(nextRes); 121 id = id.withResourceType(resName); 122 } 123 124 if (!addedResourceIds.contains(id)) { 125 addedResourceIds.add(id); 126 addedResourcesThisPass.add(nextRes); 127 } 128 } 129 } 130 } 131 132 includedResources.addAll(addedResourcesThisPass); 133 134 // Linked resources may themselves have linked resources 135 references = new ArrayList<>(); 136 for (IAnyResource iResource : addedResourcesThisPass) { 137 List<ResourceReferenceInfo> newReferences = 138 myContext.newTerser().getAllResourceReferences(iResource); 139 references.addAll(newReferences); 140 } 141 } while (references.isEmpty() == false); 142 143 BundleEntryComponent entry = myBundle.addEntry().setResource((Resource) next); 144 Resource nextAsResource = (Resource) next; 145 IIdType id = populateBundleEntryFullUrl(next, entry); 146 BundleEntryTransactionMethodEnum httpVerb = 147 ResourceMetadataKeyEnum.ENTRY_TRANSACTION_METHOD.get(nextAsResource); 148 if (httpVerb != null) { 149 entry.getRequest().getMethodElement().setValueAsString(httpVerb.name()); 150 if (id != null) { 151 entry.getRequest().setUrl(id.getValue()); 152 } 153 } 154 if (BundleEntryTransactionMethodEnum.DELETE.equals(httpVerb)) { 155 entry.setResource(null); 156 } 157 158 // Populate Bundle.entry.response 159 if (theBundleType != null) { 160 switch (theBundleType) { 161 case BATCH_RESPONSE: 162 case TRANSACTION_RESPONSE: 163 if (id != null) { 164 if ("1".equals(id.getVersionIdPart())) { 165 entry.getResponse().setStatus("201 Created"); 166 } else if (isNotBlank(id.getVersionIdPart())) { 167 entry.getResponse().setStatus("200 OK"); 168 } 169 if (isNotBlank(id.getVersionIdPart())) { 170 entry.getResponse().setEtag(RestfulServerUtils.createEtag(id.getVersionIdPart())); 171 } 172 } 173 break; 174 } 175 } 176 177 // Populate Bundle.entry.search 178 BundleEntrySearchModeEnum searchMode = ResourceMetadataKeyEnum.ENTRY_SEARCH_MODE.get(nextAsResource); 179 if (searchMode != null) { 180 entry.getSearch().getModeElement().setValueAsString(searchMode.getCode()); 181 } 182 BigDecimal searchScore = ResourceMetadataKeyEnum.ENTRY_SEARCH_SCORE.get(nextAsResource); 183 if (searchScore != null) { 184 entry.getSearch().getScoreElement().setValue(searchScore); 185 } 186 } 187 188 /* 189 * Actually add the resources to the bundle 190 */ 191 for (IAnyResource next : includedResources) { 192 BundleEntryComponent entry = myBundle.addEntry(); 193 entry.setResource((Resource) next).getSearch().setMode(SearchEntryMode.INCLUDE); 194 populateBundleEntryFullUrl(next, entry); 195 } 196 } 197 198 @Override 199 public void addRootPropertiesToBundle( 200 String theId, 201 @Nonnull BundleLinks theBundleLinks, 202 Integer theTotalResults, 203 IPrimitiveType<Date> theLastUpdated) { 204 ensureBundle(); 205 206 if (myBundle.getIdElement().isEmpty()) { 207 myBundle.setId(theId); 208 } 209 210 if (myBundle.getMeta().getLastUpdated() == null && theLastUpdated != null) { 211 InstantType instantType = new InstantType(); 212 instantType.setValueAsString(theLastUpdated.getValueAsString()); 213 myBundle.getMeta().setLastUpdatedElement(instantType); 214 } 215 216 if (!hasLink(Constants.LINK_SELF, myBundle) && isNotBlank(theBundleLinks.getSelf())) { 217 myBundle.addLink().setRelation(Constants.LINK_SELF).setUrl(theBundleLinks.getSelf()); 218 } 219 if (!hasLink(Constants.LINK_NEXT, myBundle) && isNotBlank(theBundleLinks.getNext())) { 220 myBundle.addLink().setRelation(Constants.LINK_NEXT).setUrl(theBundleLinks.getNext()); 221 } 222 if (!hasLink(Constants.LINK_SELF, myBundle) && isNotBlank(theBundleLinks.getPrev())) { 223 myBundle.addLink().setRelation(Constants.LINK_PREVIOUS).setUrl(theBundleLinks.getPrev()); 224 } 225 226 myBase = theBundleLinks.serverBase; 227 228 addTotalResultsToBundle(theTotalResults, theBundleLinks.bundleType); 229 } 230 231 @Override 232 public void addTotalResultsToBundle(Integer theTotalResults, BundleTypeEnum theBundleType) { 233 ensureBundle(); 234 235 if (isBlank(myBundle.getId())) { 236 myBundle.setId(UUID.randomUUID().toString()); 237 } 238 239 if (myBundle.getTypeElement().isEmpty() && theBundleType != null) { 240 myBundle.getTypeElement().setValueAsString(theBundleType.getCode()); 241 } 242 243 if (myBundle.getTotalElement().isEmpty() && theTotalResults != null) { 244 myBundle.getTotalElement().setValue(theTotalResults); 245 } 246 } 247 248 private void ensureBundle() { 249 if (myBundle == null) { 250 myBundle = new Bundle(); 251 } 252 } 253 254 @Override 255 public IBaseResource getResourceBundle() { 256 return myBundle; 257 } 258 259 private boolean hasLink(String theLinkType, Bundle theBundle) { 260 for (BundleLinkComponent next : theBundle.getLink()) { 261 if (theLinkType.equals(next.getRelation())) { 262 return true; 263 } 264 } 265 return false; 266 } 267 268 @Override 269 public void initializeWithBundleResource(IBaseResource theBundle) { 270 myBundle = (Bundle) theBundle; 271 } 272 273 private IIdType populateBundleEntryFullUrl(IBaseResource next, BundleEntryComponent entry) { 274 IIdType idElement = null; 275 if (next.getIdElement().hasBaseUrl()) { 276 idElement = next.getIdElement(); 277 entry.setFullUrl(idElement.toVersionless().getValue()); 278 } else { 279 if (isNotBlank(myBase) && next.getIdElement().hasIdPart()) { 280 idElement = next.getIdElement(); 281 idElement = idElement.withServerBase(myBase, myContext.getResourceType(next)); 282 entry.setFullUrl(idElement.toVersionless().getValue()); 283 } 284 } 285 return idElement; 286 } 287 288 @Override 289 public List<IBaseResource> toListOfResources() { 290 ArrayList<IBaseResource> retVal = new ArrayList<IBaseResource>(); 291 for (BundleEntryComponent next : myBundle.getEntry()) { 292 if (next.getResource() != null) { 293 retVal.add(next.getResource()); 294 } else if (next.getResponse().getLocationElement().isEmpty() == false) { 295 IdType id = new IdType(next.getResponse().getLocation()); 296 String resourceType = id.getResourceType(); 297 if (isNotBlank(resourceType)) { 298 IBaseResource res = (IBaseResource) 299 myContext.getResourceDefinition(resourceType).newInstance(); 300 res.setId(id); 301 retVal.add(res); 302 } 303 } 304 } 305 return retVal; 306 } 307}