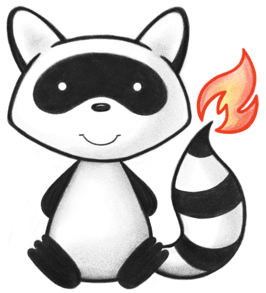
001package org.hl7.fhir.dstu2.formats; 002 003import java.io.IOException; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 035import org.hl7.fhir.dstu2.model.*; 036import org.hl7.fhir.exceptions.FHIRFormatError; 037import org.hl7.fhir.utilities.Utilities; 038 039import com.google.gson.JsonArray; 040import com.google.gson.JsonObject; 041 042public class JsonParser extends JsonParserBase { 043 044 public JsonParser() { 045 super(); 046 } 047 048 public JsonParser(boolean allowUnknownContent) { 049 super(); 050 setAllowUnknownContent(allowUnknownContent); 051 } 052 053 protected void parseElementProperties(JsonObject json, Element element) throws IOException, FHIRFormatError { 054 super.parseElementProperties(json, element); 055 if (json.has("extension")) { 056 JsonArray array = json.getAsJsonArray("extension"); 057 for (int i = 0; i < array.size(); i++) { 058 element.getExtension().add(parseExtension(array.get(i).getAsJsonObject())); 059 } 060 } 061 ; 062 } 063 064 protected void parseBackboneProperties(JsonObject json, BackboneElement element) throws IOException, FHIRFormatError { 065 parseElementProperties(json, element); 066 if (json.has("modifierExtension")) { 067 JsonArray array = json.getAsJsonArray("modifierExtension"); 068 for (int i = 0; i < array.size(); i++) { 069 element.getModifierExtension().add(parseExtension(array.get(i).getAsJsonObject())); 070 } 071 } 072 } 073 074 protected void parseTypeProperties(JsonObject json, Element element) throws IOException, FHIRFormatError { 075 parseElementProperties(json, element); 076 } 077 078 @SuppressWarnings("unchecked") 079 protected <E extends Enum<E>> Enumeration<E> parseEnumeration(String s, E item, EnumFactory e) 080 throws IOException, FHIRFormatError { 081 Enumeration<E> res = new Enumeration<E>(e); 082 if (s != null) 083 res.setValue((E) e.fromCode(s)); 084 return res; 085 } 086 087 protected DateType parseDate(String v) throws IOException, FHIRFormatError { 088 DateType res = new DateType(v); 089 return res; 090 } 091 092 protected DateTimeType parseDateTime(String v) throws IOException, FHIRFormatError { 093 DateTimeType res = new DateTimeType(v); 094 return res; 095 } 096 097 protected CodeType parseCode(String v) throws IOException, FHIRFormatError { 098 CodeType res = new CodeType(v); 099 return res; 100 } 101 102 protected StringType parseString(String v) throws IOException, FHIRFormatError { 103 StringType res = new StringType(v); 104 return res; 105 } 106 107 protected IntegerType parseInteger(java.lang.Long v) throws IOException, FHIRFormatError { 108 IntegerType res = new IntegerType(v); 109 return res; 110 } 111 112 protected OidType parseOid(String v) throws IOException, FHIRFormatError { 113 OidType res = new OidType(v); 114 return res; 115 } 116 117 protected UriType parseUri(String v) throws IOException, FHIRFormatError { 118 UriType res = new UriType(v); 119 return res; 120 } 121 122 protected UuidType parseUuid(String v) throws IOException, FHIRFormatError { 123 UuidType res = new UuidType(v); 124 return res; 125 } 126 127 protected InstantType parseInstant(String v) throws IOException, FHIRFormatError { 128 InstantType res = new InstantType(v); 129 return res; 130 } 131 132 protected BooleanType parseBoolean(java.lang.Boolean v) throws IOException, FHIRFormatError { 133 BooleanType res = new BooleanType(v); 134 return res; 135 } 136 137 protected Base64BinaryType parseBase64Binary(String v) throws IOException, FHIRFormatError { 138 Base64BinaryType res = new Base64BinaryType(v); 139 return res; 140 } 141 142 protected UnsignedIntType parseUnsignedInt(String v) throws IOException, FHIRFormatError { 143 UnsignedIntType res = new UnsignedIntType(v); 144 return res; 145 } 146 147 protected MarkdownType parseMarkdown(String v) throws IOException, FHIRFormatError { 148 MarkdownType res = new MarkdownType(v); 149 return res; 150 } 151 152 protected TimeType parseTime(String v) throws IOException, FHIRFormatError { 153 TimeType res = new TimeType(v); 154 return res; 155 } 156 157 protected IdType parseId(String v) throws IOException, FHIRFormatError { 158 IdType res = new IdType(v); 159 return res; 160 } 161 162 protected PositiveIntType parsePositiveInt(String v) throws IOException, FHIRFormatError { 163 PositiveIntType res = new PositiveIntType(v); 164 return res; 165 } 166 167 protected DecimalType parseDecimal(java.math.BigDecimal v) throws IOException, FHIRFormatError { 168 DecimalType res = new DecimalType(v); 169 return res; 170 } 171 172 protected Extension parseExtension(JsonObject json) throws IOException, FHIRFormatError { 173 Extension res = new Extension(); 174 parseElementProperties(json, res); 175 if (json.has("url")) 176 res.setUrlElement(parseUri(json.get("url").getAsString())); 177 if (json.has("_url")) 178 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 179 Type value = parseType("value", json); 180 if (value != null) 181 res.setValue(value); 182 return res; 183 } 184 185 protected Narrative parseNarrative(JsonObject json) throws IOException, FHIRFormatError { 186 Narrative res = new Narrative(); 187 parseElementProperties(json, res); 188 if (json.has("status")) 189 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Narrative.NarrativeStatus.NULL, 190 new Narrative.NarrativeStatusEnumFactory())); 191 if (json.has("_status")) 192 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 193 if (json.has("div")) 194 res.setDiv(parseXhtml(json.get("div").getAsString())); 195 return res; 196 } 197 198 protected Identifier parseIdentifier(JsonObject json) throws IOException, FHIRFormatError { 199 Identifier res = new Identifier(); 200 parseTypeProperties(json, res); 201 if (json.has("use")) 202 res.setUseElement(parseEnumeration(json.get("use").getAsString(), Identifier.IdentifierUse.NULL, 203 new Identifier.IdentifierUseEnumFactory())); 204 if (json.has("_use")) 205 parseElementProperties(json.getAsJsonObject("_use"), res.getUseElement()); 206 if (json.has("type")) 207 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 208 if (json.has("system")) 209 res.setSystemElement(parseUri(json.get("system").getAsString())); 210 if (json.has("_system")) 211 parseElementProperties(json.getAsJsonObject("_system"), res.getSystemElement()); 212 if (json.has("value")) 213 res.setValueElement(parseString(json.get("value").getAsString())); 214 if (json.has("_value")) 215 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 216 if (json.has("period")) 217 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 218 if (json.has("assigner")) 219 res.setAssigner(parseReference(json.getAsJsonObject("assigner"))); 220 return res; 221 } 222 223 protected Coding parseCoding(JsonObject json) throws IOException, FHIRFormatError { 224 Coding res = new Coding(); 225 parseTypeProperties(json, res); 226 if (json.has("system")) 227 res.setSystemElement(parseUri(json.get("system").getAsString())); 228 if (json.has("_system")) 229 parseElementProperties(json.getAsJsonObject("_system"), res.getSystemElement()); 230 if (json.has("version")) 231 res.setVersionElement(parseString(json.get("version").getAsString())); 232 if (json.has("_version")) 233 parseElementProperties(json.getAsJsonObject("_version"), res.getVersionElement()); 234 if (json.has("code")) 235 res.setCodeElement(parseCode(json.get("code").getAsString())); 236 if (json.has("_code")) 237 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 238 if (json.has("display")) 239 res.setDisplayElement(parseString(json.get("display").getAsString())); 240 if (json.has("_display")) 241 parseElementProperties(json.getAsJsonObject("_display"), res.getDisplayElement()); 242 if (json.has("userSelected")) 243 res.setUserSelectedElement(parseBoolean(json.get("userSelected").getAsBoolean())); 244 if (json.has("_userSelected")) 245 parseElementProperties(json.getAsJsonObject("_userSelected"), res.getUserSelectedElement()); 246 return res; 247 } 248 249 protected Reference parseReference(JsonObject json) throws IOException, FHIRFormatError { 250 Reference res = new Reference(); 251 parseTypeProperties(json, res); 252 if (json.has("reference")) 253 res.setReferenceElement(parseString(json.get("reference").getAsString())); 254 if (json.has("_reference")) 255 parseElementProperties(json.getAsJsonObject("_reference"), res.getReferenceElement()); 256 if (json.has("display")) 257 res.setDisplayElement(parseString(json.get("display").getAsString())); 258 if (json.has("_display")) 259 parseElementProperties(json.getAsJsonObject("_display"), res.getDisplayElement()); 260 return res; 261 } 262 263 protected Signature parseSignature(JsonObject json) throws IOException, FHIRFormatError { 264 Signature res = new Signature(); 265 parseTypeProperties(json, res); 266 if (json.has("type")) { 267 JsonArray array = json.getAsJsonArray("type"); 268 for (int i = 0; i < array.size(); i++) { 269 res.getType().add(parseCoding(array.get(i).getAsJsonObject())); 270 } 271 } 272 ; 273 if (json.has("when")) 274 res.setWhenElement(parseInstant(json.get("when").getAsString())); 275 if (json.has("_when")) 276 parseElementProperties(json.getAsJsonObject("_when"), res.getWhenElement()); 277 Type who = parseType("who", json); 278 if (who != null) 279 res.setWho(who); 280 if (json.has("contentType")) 281 res.setContentTypeElement(parseCode(json.get("contentType").getAsString())); 282 if (json.has("_contentType")) 283 parseElementProperties(json.getAsJsonObject("_contentType"), res.getContentTypeElement()); 284 if (json.has("blob")) 285 res.setBlobElement(parseBase64Binary(json.get("blob").getAsString())); 286 if (json.has("_blob")) 287 parseElementProperties(json.getAsJsonObject("_blob"), res.getBlobElement()); 288 return res; 289 } 290 291 protected SampledData parseSampledData(JsonObject json) throws IOException, FHIRFormatError { 292 SampledData res = new SampledData(); 293 parseTypeProperties(json, res); 294 if (json.has("origin")) 295 res.setOrigin(parseSimpleQuantity(json.getAsJsonObject("origin"))); 296 if (json.has("period")) 297 res.setPeriodElement(parseDecimal(json.get("period").getAsBigDecimal())); 298 if (json.has("_period")) 299 parseElementProperties(json.getAsJsonObject("_period"), res.getPeriodElement()); 300 if (json.has("factor")) 301 res.setFactorElement(parseDecimal(json.get("factor").getAsBigDecimal())); 302 if (json.has("_factor")) 303 parseElementProperties(json.getAsJsonObject("_factor"), res.getFactorElement()); 304 if (json.has("lowerLimit")) 305 res.setLowerLimitElement(parseDecimal(json.get("lowerLimit").getAsBigDecimal())); 306 if (json.has("_lowerLimit")) 307 parseElementProperties(json.getAsJsonObject("_lowerLimit"), res.getLowerLimitElement()); 308 if (json.has("upperLimit")) 309 res.setUpperLimitElement(parseDecimal(json.get("upperLimit").getAsBigDecimal())); 310 if (json.has("_upperLimit")) 311 parseElementProperties(json.getAsJsonObject("_upperLimit"), res.getUpperLimitElement()); 312 if (json.has("dimensions")) 313 res.setDimensionsElement(parsePositiveInt(json.get("dimensions").getAsString())); 314 if (json.has("_dimensions")) 315 parseElementProperties(json.getAsJsonObject("_dimensions"), res.getDimensionsElement()); 316 if (json.has("data")) 317 res.setDataElement(parseString(json.get("data").getAsString())); 318 if (json.has("_data")) 319 parseElementProperties(json.getAsJsonObject("_data"), res.getDataElement()); 320 return res; 321 } 322 323 protected Quantity parseQuantity(JsonObject json) throws IOException, FHIRFormatError { 324 Quantity res = new Quantity(); 325 parseTypeProperties(json, res); 326 if (json.has("value")) 327 res.setValueElement(parseDecimal(json.get("value").getAsBigDecimal())); 328 if (json.has("_value")) 329 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 330 if (json.has("comparator")) 331 res.setComparatorElement(parseEnumeration(json.get("comparator").getAsString(), Quantity.QuantityComparator.NULL, 332 new Quantity.QuantityComparatorEnumFactory())); 333 if (json.has("_comparator")) 334 parseElementProperties(json.getAsJsonObject("_comparator"), res.getComparatorElement()); 335 if (json.has("unit")) 336 res.setUnitElement(parseString(json.get("unit").getAsString())); 337 if (json.has("_unit")) 338 parseElementProperties(json.getAsJsonObject("_unit"), res.getUnitElement()); 339 if (json.has("system")) 340 res.setSystemElement(parseUri(json.get("system").getAsString())); 341 if (json.has("_system")) 342 parseElementProperties(json.getAsJsonObject("_system"), res.getSystemElement()); 343 if (json.has("code")) 344 res.setCodeElement(parseCode(json.get("code").getAsString())); 345 if (json.has("_code")) 346 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 347 return res; 348 } 349 350 protected Period parsePeriod(JsonObject json) throws IOException, FHIRFormatError { 351 Period res = new Period(); 352 parseTypeProperties(json, res); 353 if (json.has("start")) 354 res.setStartElement(parseDateTime(json.get("start").getAsString())); 355 if (json.has("_start")) 356 parseElementProperties(json.getAsJsonObject("_start"), res.getStartElement()); 357 if (json.has("end")) 358 res.setEndElement(parseDateTime(json.get("end").getAsString())); 359 if (json.has("_end")) 360 parseElementProperties(json.getAsJsonObject("_end"), res.getEndElement()); 361 return res; 362 } 363 364 protected Attachment parseAttachment(JsonObject json) throws IOException, FHIRFormatError { 365 Attachment res = new Attachment(); 366 parseTypeProperties(json, res); 367 if (json.has("contentType")) 368 res.setContentTypeElement(parseCode(json.get("contentType").getAsString())); 369 if (json.has("_contentType")) 370 parseElementProperties(json.getAsJsonObject("_contentType"), res.getContentTypeElement()); 371 if (json.has("language")) 372 res.setLanguageElement(parseCode(json.get("language").getAsString())); 373 if (json.has("_language")) 374 parseElementProperties(json.getAsJsonObject("_language"), res.getLanguageElement()); 375 if (json.has("data")) 376 res.setDataElement(parseBase64Binary(json.get("data").getAsString())); 377 if (json.has("_data")) 378 parseElementProperties(json.getAsJsonObject("_data"), res.getDataElement()); 379 if (json.has("url")) 380 res.setUrlElement(parseUri(json.get("url").getAsString())); 381 if (json.has("_url")) 382 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 383 if (json.has("size")) 384 res.setSizeElement(parseUnsignedInt(json.get("size").getAsString())); 385 if (json.has("_size")) 386 parseElementProperties(json.getAsJsonObject("_size"), res.getSizeElement()); 387 if (json.has("hash")) 388 res.setHashElement(parseBase64Binary(json.get("hash").getAsString())); 389 if (json.has("_hash")) 390 parseElementProperties(json.getAsJsonObject("_hash"), res.getHashElement()); 391 if (json.has("title")) 392 res.setTitleElement(parseString(json.get("title").getAsString())); 393 if (json.has("_title")) 394 parseElementProperties(json.getAsJsonObject("_title"), res.getTitleElement()); 395 if (json.has("creation")) 396 res.setCreationElement(parseDateTime(json.get("creation").getAsString())); 397 if (json.has("_creation")) 398 parseElementProperties(json.getAsJsonObject("_creation"), res.getCreationElement()); 399 return res; 400 } 401 402 protected Ratio parseRatio(JsonObject json) throws IOException, FHIRFormatError { 403 Ratio res = new Ratio(); 404 parseTypeProperties(json, res); 405 if (json.has("numerator")) 406 res.setNumerator(parseQuantity(json.getAsJsonObject("numerator"))); 407 if (json.has("denominator")) 408 res.setDenominator(parseQuantity(json.getAsJsonObject("denominator"))); 409 return res; 410 } 411 412 protected Range parseRange(JsonObject json) throws IOException, FHIRFormatError { 413 Range res = new Range(); 414 parseTypeProperties(json, res); 415 if (json.has("low")) 416 res.setLow(parseSimpleQuantity(json.getAsJsonObject("low"))); 417 if (json.has("high")) 418 res.setHigh(parseSimpleQuantity(json.getAsJsonObject("high"))); 419 return res; 420 } 421 422 protected Annotation parseAnnotation(JsonObject json) throws IOException, FHIRFormatError { 423 Annotation res = new Annotation(); 424 parseTypeProperties(json, res); 425 Type author = parseType("author", json); 426 if (author != null) 427 res.setAuthor(author); 428 if (json.has("time")) 429 res.setTimeElement(parseDateTime(json.get("time").getAsString())); 430 if (json.has("_time")) 431 parseElementProperties(json.getAsJsonObject("_time"), res.getTimeElement()); 432 if (json.has("text")) 433 res.setTextElement(parseString(json.get("text").getAsString())); 434 if (json.has("_text")) 435 parseElementProperties(json.getAsJsonObject("_text"), res.getTextElement()); 436 return res; 437 } 438 439 protected CodeableConcept parseCodeableConcept(JsonObject json) throws IOException, FHIRFormatError { 440 CodeableConcept res = new CodeableConcept(); 441 parseTypeProperties(json, res); 442 if (json.has("coding")) { 443 JsonArray array = json.getAsJsonArray("coding"); 444 for (int i = 0; i < array.size(); i++) { 445 res.getCoding().add(parseCoding(array.get(i).getAsJsonObject())); 446 } 447 } 448 ; 449 if (json.has("text")) 450 res.setTextElement(parseString(json.get("text").getAsString())); 451 if (json.has("_text")) 452 parseElementProperties(json.getAsJsonObject("_text"), res.getTextElement()); 453 return res; 454 } 455 456 protected Money parseMoney(JsonObject json) throws IOException, FHIRFormatError { 457 Money res = new Money(); 458 parseElementProperties(json, res); 459 if (json.has("value")) 460 res.setValueElement(parseDecimal(json.get("value").getAsBigDecimal())); 461 if (json.has("_value")) 462 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 463 if (json.has("comparator")) 464 res.setComparatorElement(parseEnumeration(json.get("comparator").getAsString(), Quantity.QuantityComparator.NULL, 465 new Quantity.QuantityComparatorEnumFactory())); 466 if (json.has("_comparator")) 467 parseElementProperties(json.getAsJsonObject("_comparator"), res.getComparatorElement()); 468 if (json.has("unit")) 469 res.setUnitElement(parseString(json.get("unit").getAsString())); 470 if (json.has("_unit")) 471 parseElementProperties(json.getAsJsonObject("_unit"), res.getUnitElement()); 472 if (json.has("system")) 473 res.setSystemElement(parseUri(json.get("system").getAsString())); 474 if (json.has("_system")) 475 parseElementProperties(json.getAsJsonObject("_system"), res.getSystemElement()); 476 if (json.has("code")) 477 res.setCodeElement(parseCode(json.get("code").getAsString())); 478 if (json.has("_code")) 479 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 480 return res; 481 } 482 483 protected SimpleQuantity parseSimpleQuantity(JsonObject json) throws IOException, FHIRFormatError { 484 SimpleQuantity res = new SimpleQuantity(); 485 parseElementProperties(json, res); 486 if (json.has("value")) 487 res.setValueElement(parseDecimal(json.get("value").getAsBigDecimal())); 488 if (json.has("_value")) 489 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 490 if (json.has("comparator")) 491 res.setComparatorElement(parseEnumeration(json.get("comparator").getAsString(), Quantity.QuantityComparator.NULL, 492 new Quantity.QuantityComparatorEnumFactory())); 493 if (json.has("_comparator")) 494 parseElementProperties(json.getAsJsonObject("_comparator"), res.getComparatorElement()); 495 if (json.has("unit")) 496 res.setUnitElement(parseString(json.get("unit").getAsString())); 497 if (json.has("_unit")) 498 parseElementProperties(json.getAsJsonObject("_unit"), res.getUnitElement()); 499 if (json.has("system")) 500 res.setSystemElement(parseUri(json.get("system").getAsString())); 501 if (json.has("_system")) 502 parseElementProperties(json.getAsJsonObject("_system"), res.getSystemElement()); 503 if (json.has("code")) 504 res.setCodeElement(parseCode(json.get("code").getAsString())); 505 if (json.has("_code")) 506 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 507 return res; 508 } 509 510 protected Duration parseDuration(JsonObject json) throws IOException, FHIRFormatError { 511 Duration res = new Duration(); 512 parseElementProperties(json, res); 513 if (json.has("value")) 514 res.setValueElement(parseDecimal(json.get("value").getAsBigDecimal())); 515 if (json.has("_value")) 516 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 517 if (json.has("comparator")) 518 res.setComparatorElement(parseEnumeration(json.get("comparator").getAsString(), Quantity.QuantityComparator.NULL, 519 new Quantity.QuantityComparatorEnumFactory())); 520 if (json.has("_comparator")) 521 parseElementProperties(json.getAsJsonObject("_comparator"), res.getComparatorElement()); 522 if (json.has("unit")) 523 res.setUnitElement(parseString(json.get("unit").getAsString())); 524 if (json.has("_unit")) 525 parseElementProperties(json.getAsJsonObject("_unit"), res.getUnitElement()); 526 if (json.has("system")) 527 res.setSystemElement(parseUri(json.get("system").getAsString())); 528 if (json.has("_system")) 529 parseElementProperties(json.getAsJsonObject("_system"), res.getSystemElement()); 530 if (json.has("code")) 531 res.setCodeElement(parseCode(json.get("code").getAsString())); 532 if (json.has("_code")) 533 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 534 return res; 535 } 536 537 protected Count parseCount(JsonObject json) throws IOException, FHIRFormatError { 538 Count res = new Count(); 539 parseElementProperties(json, res); 540 if (json.has("value")) 541 res.setValueElement(parseDecimal(json.get("value").getAsBigDecimal())); 542 if (json.has("_value")) 543 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 544 if (json.has("comparator")) 545 res.setComparatorElement(parseEnumeration(json.get("comparator").getAsString(), Quantity.QuantityComparator.NULL, 546 new Quantity.QuantityComparatorEnumFactory())); 547 if (json.has("_comparator")) 548 parseElementProperties(json.getAsJsonObject("_comparator"), res.getComparatorElement()); 549 if (json.has("unit")) 550 res.setUnitElement(parseString(json.get("unit").getAsString())); 551 if (json.has("_unit")) 552 parseElementProperties(json.getAsJsonObject("_unit"), res.getUnitElement()); 553 if (json.has("system")) 554 res.setSystemElement(parseUri(json.get("system").getAsString())); 555 if (json.has("_system")) 556 parseElementProperties(json.getAsJsonObject("_system"), res.getSystemElement()); 557 if (json.has("code")) 558 res.setCodeElement(parseCode(json.get("code").getAsString())); 559 if (json.has("_code")) 560 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 561 return res; 562 } 563 564 protected Distance parseDistance(JsonObject json) throws IOException, FHIRFormatError { 565 Distance res = new Distance(); 566 parseElementProperties(json, res); 567 if (json.has("value")) 568 res.setValueElement(parseDecimal(json.get("value").getAsBigDecimal())); 569 if (json.has("_value")) 570 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 571 if (json.has("comparator")) 572 res.setComparatorElement(parseEnumeration(json.get("comparator").getAsString(), Quantity.QuantityComparator.NULL, 573 new Quantity.QuantityComparatorEnumFactory())); 574 if (json.has("_comparator")) 575 parseElementProperties(json.getAsJsonObject("_comparator"), res.getComparatorElement()); 576 if (json.has("unit")) 577 res.setUnitElement(parseString(json.get("unit").getAsString())); 578 if (json.has("_unit")) 579 parseElementProperties(json.getAsJsonObject("_unit"), res.getUnitElement()); 580 if (json.has("system")) 581 res.setSystemElement(parseUri(json.get("system").getAsString())); 582 if (json.has("_system")) 583 parseElementProperties(json.getAsJsonObject("_system"), res.getSystemElement()); 584 if (json.has("code")) 585 res.setCodeElement(parseCode(json.get("code").getAsString())); 586 if (json.has("_code")) 587 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 588 return res; 589 } 590 591 protected Age parseAge(JsonObject json) throws IOException, FHIRFormatError { 592 Age res = new Age(); 593 parseElementProperties(json, res); 594 if (json.has("value")) 595 res.setValueElement(parseDecimal(json.get("value").getAsBigDecimal())); 596 if (json.has("_value")) 597 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 598 if (json.has("comparator")) 599 res.setComparatorElement(parseEnumeration(json.get("comparator").getAsString(), Quantity.QuantityComparator.NULL, 600 new Quantity.QuantityComparatorEnumFactory())); 601 if (json.has("_comparator")) 602 parseElementProperties(json.getAsJsonObject("_comparator"), res.getComparatorElement()); 603 if (json.has("unit")) 604 res.setUnitElement(parseString(json.get("unit").getAsString())); 605 if (json.has("_unit")) 606 parseElementProperties(json.getAsJsonObject("_unit"), res.getUnitElement()); 607 if (json.has("system")) 608 res.setSystemElement(parseUri(json.get("system").getAsString())); 609 if (json.has("_system")) 610 parseElementProperties(json.getAsJsonObject("_system"), res.getSystemElement()); 611 if (json.has("code")) 612 res.setCodeElement(parseCode(json.get("code").getAsString())); 613 if (json.has("_code")) 614 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 615 return res; 616 } 617 618 protected HumanName parseHumanName(JsonObject json) throws IOException, FHIRFormatError { 619 HumanName res = new HumanName(); 620 parseElementProperties(json, res); 621 if (json.has("use")) 622 res.setUseElement( 623 parseEnumeration(json.get("use").getAsString(), HumanName.NameUse.NULL, new HumanName.NameUseEnumFactory())); 624 if (json.has("_use")) 625 parseElementProperties(json.getAsJsonObject("_use"), res.getUseElement()); 626 if (json.has("text")) 627 res.setTextElement(parseString(json.get("text").getAsString())); 628 if (json.has("_text")) 629 parseElementProperties(json.getAsJsonObject("_text"), res.getTextElement()); 630 if (json.has("family")) { 631 JsonArray array = json.getAsJsonArray("family"); 632 for (int i = 0; i < array.size(); i++) { 633 res.getFamily().add(parseString(array.get(i).getAsString())); 634 } 635 } 636 ; 637 if (json.has("_family")) { 638 JsonArray array = json.getAsJsonArray("_family"); 639 for (int i = 0; i < array.size(); i++) { 640 if (i == res.getFamily().size()) 641 res.getFamily().add(parseString(null)); 642 if (array.get(i) instanceof JsonObject) 643 parseElementProperties(array.get(i).getAsJsonObject(), res.getFamily().get(i)); 644 } 645 } 646 ; 647 if (json.has("given")) { 648 JsonArray array = json.getAsJsonArray("given"); 649 for (int i = 0; i < array.size(); i++) { 650 res.getGiven().add(parseString(array.get(i).getAsString())); 651 } 652 } 653 ; 654 if (json.has("_given")) { 655 JsonArray array = json.getAsJsonArray("_given"); 656 for (int i = 0; i < array.size(); i++) { 657 if (i == res.getGiven().size()) 658 res.getGiven().add(parseString(null)); 659 if (array.get(i) instanceof JsonObject) 660 parseElementProperties(array.get(i).getAsJsonObject(), res.getGiven().get(i)); 661 } 662 } 663 ; 664 if (json.has("prefix")) { 665 JsonArray array = json.getAsJsonArray("prefix"); 666 for (int i = 0; i < array.size(); i++) { 667 res.getPrefix().add(parseString(array.get(i).getAsString())); 668 } 669 } 670 ; 671 if (json.has("_prefix")) { 672 JsonArray array = json.getAsJsonArray("_prefix"); 673 for (int i = 0; i < array.size(); i++) { 674 if (i == res.getPrefix().size()) 675 res.getPrefix().add(parseString(null)); 676 if (array.get(i) instanceof JsonObject) 677 parseElementProperties(array.get(i).getAsJsonObject(), res.getPrefix().get(i)); 678 } 679 } 680 ; 681 if (json.has("suffix")) { 682 JsonArray array = json.getAsJsonArray("suffix"); 683 for (int i = 0; i < array.size(); i++) { 684 res.getSuffix().add(parseString(array.get(i).getAsString())); 685 } 686 } 687 ; 688 if (json.has("_suffix")) { 689 JsonArray array = json.getAsJsonArray("_suffix"); 690 for (int i = 0; i < array.size(); i++) { 691 if (i == res.getSuffix().size()) 692 res.getSuffix().add(parseString(null)); 693 if (array.get(i) instanceof JsonObject) 694 parseElementProperties(array.get(i).getAsJsonObject(), res.getSuffix().get(i)); 695 } 696 } 697 ; 698 if (json.has("period")) 699 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 700 return res; 701 } 702 703 protected ContactPoint parseContactPoint(JsonObject json) throws IOException, FHIRFormatError { 704 ContactPoint res = new ContactPoint(); 705 parseElementProperties(json, res); 706 if (json.has("system")) 707 res.setSystemElement(parseEnumeration(json.get("system").getAsString(), ContactPoint.ContactPointSystem.NULL, 708 new ContactPoint.ContactPointSystemEnumFactory())); 709 if (json.has("_system")) 710 parseElementProperties(json.getAsJsonObject("_system"), res.getSystemElement()); 711 if (json.has("value")) 712 res.setValueElement(parseString(json.get("value").getAsString())); 713 if (json.has("_value")) 714 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 715 if (json.has("use")) 716 res.setUseElement(parseEnumeration(json.get("use").getAsString(), ContactPoint.ContactPointUse.NULL, 717 new ContactPoint.ContactPointUseEnumFactory())); 718 if (json.has("_use")) 719 parseElementProperties(json.getAsJsonObject("_use"), res.getUseElement()); 720 if (json.has("rank")) 721 res.setRankElement(parsePositiveInt(json.get("rank").getAsString())); 722 if (json.has("_rank")) 723 parseElementProperties(json.getAsJsonObject("_rank"), res.getRankElement()); 724 if (json.has("period")) 725 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 726 return res; 727 } 728 729 protected Meta parseMeta(JsonObject json) throws IOException, FHIRFormatError { 730 Meta res = new Meta(); 731 parseElementProperties(json, res); 732 if (json.has("versionId")) 733 res.setVersionIdElement(parseId(json.get("versionId").getAsString())); 734 if (json.has("_versionId")) 735 parseElementProperties(json.getAsJsonObject("_versionId"), res.getVersionIdElement()); 736 if (json.has("lastUpdated")) 737 res.setLastUpdatedElement(parseInstant(json.get("lastUpdated").getAsString())); 738 if (json.has("_lastUpdated")) 739 parseElementProperties(json.getAsJsonObject("_lastUpdated"), res.getLastUpdatedElement()); 740 if (json.has("profile")) { 741 JsonArray array = json.getAsJsonArray("profile"); 742 for (int i = 0; i < array.size(); i++) { 743 res.getProfile().add(parseUri(array.get(i).getAsString())); 744 } 745 } 746 ; 747 if (json.has("_profile")) { 748 JsonArray array = json.getAsJsonArray("_profile"); 749 for (int i = 0; i < array.size(); i++) { 750 if (i == res.getProfile().size()) 751 res.getProfile().add(parseUri(null)); 752 if (array.get(i) instanceof JsonObject) 753 parseElementProperties(array.get(i).getAsJsonObject(), res.getProfile().get(i)); 754 } 755 } 756 ; 757 if (json.has("security")) { 758 JsonArray array = json.getAsJsonArray("security"); 759 for (int i = 0; i < array.size(); i++) { 760 res.getSecurity().add(parseCoding(array.get(i).getAsJsonObject())); 761 } 762 } 763 ; 764 if (json.has("tag")) { 765 JsonArray array = json.getAsJsonArray("tag"); 766 for (int i = 0; i < array.size(); i++) { 767 res.getTag().add(parseCoding(array.get(i).getAsJsonObject())); 768 } 769 } 770 ; 771 return res; 772 } 773 774 protected Address parseAddress(JsonObject json) throws IOException, FHIRFormatError { 775 Address res = new Address(); 776 parseElementProperties(json, res); 777 if (json.has("use")) 778 res.setUseElement(parseEnumeration(json.get("use").getAsString(), Address.AddressUse.NULL, 779 new Address.AddressUseEnumFactory())); 780 if (json.has("_use")) 781 parseElementProperties(json.getAsJsonObject("_use"), res.getUseElement()); 782 if (json.has("type")) 783 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Address.AddressType.NULL, 784 new Address.AddressTypeEnumFactory())); 785 if (json.has("_type")) 786 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 787 if (json.has("text")) 788 res.setTextElement(parseString(json.get("text").getAsString())); 789 if (json.has("_text")) 790 parseElementProperties(json.getAsJsonObject("_text"), res.getTextElement()); 791 if (json.has("line")) { 792 JsonArray array = json.getAsJsonArray("line"); 793 for (int i = 0; i < array.size(); i++) { 794 res.getLine().add(parseString(array.get(i).getAsString())); 795 } 796 } 797 ; 798 if (json.has("_line")) { 799 JsonArray array = json.getAsJsonArray("_line"); 800 for (int i = 0; i < array.size(); i++) { 801 if (i == res.getLine().size()) 802 res.getLine().add(parseString(null)); 803 if (array.get(i) instanceof JsonObject) 804 parseElementProperties(array.get(i).getAsJsonObject(), res.getLine().get(i)); 805 } 806 } 807 ; 808 if (json.has("city")) 809 res.setCityElement(parseString(json.get("city").getAsString())); 810 if (json.has("_city")) 811 parseElementProperties(json.getAsJsonObject("_city"), res.getCityElement()); 812 if (json.has("district")) 813 res.setDistrictElement(parseString(json.get("district").getAsString())); 814 if (json.has("_district")) 815 parseElementProperties(json.getAsJsonObject("_district"), res.getDistrictElement()); 816 if (json.has("state")) 817 res.setStateElement(parseString(json.get("state").getAsString())); 818 if (json.has("_state")) 819 parseElementProperties(json.getAsJsonObject("_state"), res.getStateElement()); 820 if (json.has("postalCode")) 821 res.setPostalCodeElement(parseString(json.get("postalCode").getAsString())); 822 if (json.has("_postalCode")) 823 parseElementProperties(json.getAsJsonObject("_postalCode"), res.getPostalCodeElement()); 824 if (json.has("country")) 825 res.setCountryElement(parseString(json.get("country").getAsString())); 826 if (json.has("_country")) 827 parseElementProperties(json.getAsJsonObject("_country"), res.getCountryElement()); 828 if (json.has("period")) 829 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 830 return res; 831 } 832 833 protected Timing parseTiming(JsonObject json) throws IOException, FHIRFormatError { 834 Timing res = new Timing(); 835 parseElementProperties(json, res); 836 if (json.has("event")) { 837 JsonArray array = json.getAsJsonArray("event"); 838 for (int i = 0; i < array.size(); i++) { 839 res.getEvent().add(parseDateTime(array.get(i).getAsString())); 840 } 841 } 842 ; 843 if (json.has("_event")) { 844 JsonArray array = json.getAsJsonArray("_event"); 845 for (int i = 0; i < array.size(); i++) { 846 if (i == res.getEvent().size()) 847 res.getEvent().add(parseDateTime(null)); 848 if (array.get(i) instanceof JsonObject) 849 parseElementProperties(array.get(i).getAsJsonObject(), res.getEvent().get(i)); 850 } 851 } 852 ; 853 if (json.has("repeat")) 854 res.setRepeat(parseTimingTimingRepeatComponent(json.getAsJsonObject("repeat"), res)); 855 if (json.has("code")) 856 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 857 return res; 858 } 859 860 protected Timing.TimingRepeatComponent parseTimingTimingRepeatComponent(JsonObject json, Timing owner) 861 throws IOException, FHIRFormatError { 862 Timing.TimingRepeatComponent res = new Timing.TimingRepeatComponent(); 863 parseElementProperties(json, res); 864 Type bounds = parseType("bounds", json); 865 if (bounds != null) 866 res.setBounds(bounds); 867 if (json.has("count")) 868 res.setCountElement(parseInteger(json.get("count").getAsLong())); 869 if (json.has("_count")) 870 parseElementProperties(json.getAsJsonObject("_count"), res.getCountElement()); 871 if (json.has("duration")) 872 res.setDurationElement(parseDecimal(json.get("duration").getAsBigDecimal())); 873 if (json.has("_duration")) 874 parseElementProperties(json.getAsJsonObject("_duration"), res.getDurationElement()); 875 if (json.has("durationMax")) 876 res.setDurationMaxElement(parseDecimal(json.get("durationMax").getAsBigDecimal())); 877 if (json.has("_durationMax")) 878 parseElementProperties(json.getAsJsonObject("_durationMax"), res.getDurationMaxElement()); 879 if (json.has("durationUnits")) 880 res.setDurationUnitsElement(parseEnumeration(json.get("durationUnits").getAsString(), Timing.UnitsOfTime.NULL, 881 new Timing.UnitsOfTimeEnumFactory())); 882 if (json.has("_durationUnits")) 883 parseElementProperties(json.getAsJsonObject("_durationUnits"), res.getDurationUnitsElement()); 884 if (json.has("frequency")) 885 res.setFrequencyElement(parseInteger(json.get("frequency").getAsLong())); 886 if (json.has("_frequency")) 887 parseElementProperties(json.getAsJsonObject("_frequency"), res.getFrequencyElement()); 888 if (json.has("frequencyMax")) 889 res.setFrequencyMaxElement(parseInteger(json.get("frequencyMax").getAsLong())); 890 if (json.has("_frequencyMax")) 891 parseElementProperties(json.getAsJsonObject("_frequencyMax"), res.getFrequencyMaxElement()); 892 if (json.has("period")) 893 res.setPeriodElement(parseDecimal(json.get("period").getAsBigDecimal())); 894 if (json.has("_period")) 895 parseElementProperties(json.getAsJsonObject("_period"), res.getPeriodElement()); 896 if (json.has("periodMax")) 897 res.setPeriodMaxElement(parseDecimal(json.get("periodMax").getAsBigDecimal())); 898 if (json.has("_periodMax")) 899 parseElementProperties(json.getAsJsonObject("_periodMax"), res.getPeriodMaxElement()); 900 if (json.has("periodUnits")) 901 res.setPeriodUnitsElement(parseEnumeration(json.get("periodUnits").getAsString(), Timing.UnitsOfTime.NULL, 902 new Timing.UnitsOfTimeEnumFactory())); 903 if (json.has("_periodUnits")) 904 parseElementProperties(json.getAsJsonObject("_periodUnits"), res.getPeriodUnitsElement()); 905 if (json.has("when")) 906 res.setWhenElement(parseEnumeration(json.get("when").getAsString(), Timing.EventTiming.NULL, 907 new Timing.EventTimingEnumFactory())); 908 if (json.has("_when")) 909 parseElementProperties(json.getAsJsonObject("_when"), res.getWhenElement()); 910 return res; 911 } 912 913 protected ElementDefinition parseElementDefinition(JsonObject json) throws IOException, FHIRFormatError { 914 ElementDefinition res = new ElementDefinition(); 915 parseElementProperties(json, res); 916 if (json.has("path")) 917 res.setPathElement(parseString(json.get("path").getAsString())); 918 if (json.has("_path")) 919 parseElementProperties(json.getAsJsonObject("_path"), res.getPathElement()); 920 if (json.has("representation")) { 921 JsonArray array = json.getAsJsonArray("representation"); 922 for (int i = 0; i < array.size(); i++) { 923 res.getRepresentation().add(parseEnumeration(array.get(i).getAsString(), 924 ElementDefinition.PropertyRepresentation.NULL, new ElementDefinition.PropertyRepresentationEnumFactory())); 925 } 926 } 927 ; 928 if (json.has("_representation")) { 929 JsonArray array = json.getAsJsonArray("_representation"); 930 for (int i = 0; i < array.size(); i++) { 931 if (i == res.getRepresentation().size()) 932 res.getRepresentation().add(parseEnumeration(null, ElementDefinition.PropertyRepresentation.NULL, 933 new ElementDefinition.PropertyRepresentationEnumFactory())); 934 if (array.get(i) instanceof JsonObject) 935 parseElementProperties(array.get(i).getAsJsonObject(), res.getRepresentation().get(i)); 936 } 937 } 938 ; 939 if (json.has("name")) 940 res.setNameElement(parseString(json.get("name").getAsString())); 941 if (json.has("_name")) 942 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 943 if (json.has("label")) 944 res.setLabelElement(parseString(json.get("label").getAsString())); 945 if (json.has("_label")) 946 parseElementProperties(json.getAsJsonObject("_label"), res.getLabelElement()); 947 if (json.has("code")) { 948 JsonArray array = json.getAsJsonArray("code"); 949 for (int i = 0; i < array.size(); i++) { 950 res.getCode().add(parseCoding(array.get(i).getAsJsonObject())); 951 } 952 } 953 ; 954 if (json.has("slicing")) 955 res.setSlicing(parseElementDefinitionElementDefinitionSlicingComponent(json.getAsJsonObject("slicing"), res)); 956 if (json.has("short")) 957 res.setShortElement(parseString(json.get("short").getAsString())); 958 if (json.has("_short")) 959 parseElementProperties(json.getAsJsonObject("_short"), res.getShortElement()); 960 if (json.has("definition")) 961 res.setDefinitionElement(parseMarkdown(json.get("definition").getAsString())); 962 if (json.has("_definition")) 963 parseElementProperties(json.getAsJsonObject("_definition"), res.getDefinitionElement()); 964 if (json.has("comments")) 965 res.setCommentsElement(parseMarkdown(json.get("comments").getAsString())); 966 if (json.has("_comments")) 967 parseElementProperties(json.getAsJsonObject("_comments"), res.getCommentsElement()); 968 if (json.has("requirements")) 969 res.setRequirementsElement(parseMarkdown(json.get("requirements").getAsString())); 970 if (json.has("_requirements")) 971 parseElementProperties(json.getAsJsonObject("_requirements"), res.getRequirementsElement()); 972 if (json.has("alias")) { 973 JsonArray array = json.getAsJsonArray("alias"); 974 for (int i = 0; i < array.size(); i++) { 975 res.getAlias().add(parseString(array.get(i).getAsString())); 976 } 977 } 978 ; 979 if (json.has("_alias")) { 980 JsonArray array = json.getAsJsonArray("_alias"); 981 for (int i = 0; i < array.size(); i++) { 982 if (i == res.getAlias().size()) 983 res.getAlias().add(parseString(null)); 984 if (array.get(i) instanceof JsonObject) 985 parseElementProperties(array.get(i).getAsJsonObject(), res.getAlias().get(i)); 986 } 987 } 988 ; 989 if (json.has("min")) 990 res.setMinElement(parseInteger(json.get("min").getAsLong())); 991 if (json.has("_min")) 992 parseElementProperties(json.getAsJsonObject("_min"), res.getMinElement()); 993 if (json.has("max")) 994 res.setMaxElement(parseString(json.get("max").getAsString())); 995 if (json.has("_max")) 996 parseElementProperties(json.getAsJsonObject("_max"), res.getMaxElement()); 997 if (json.has("base")) 998 res.setBase(parseElementDefinitionElementDefinitionBaseComponent(json.getAsJsonObject("base"), res)); 999 if (json.has("type")) { 1000 JsonArray array = json.getAsJsonArray("type"); 1001 for (int i = 0; i < array.size(); i++) { 1002 res.getType().add(parseElementDefinitionTypeRefComponent(array.get(i).getAsJsonObject(), res)); 1003 } 1004 } 1005 ; 1006 if (json.has("nameReference")) 1007 res.setNameReferenceElement(parseString(json.get("nameReference").getAsString())); 1008 if (json.has("_nameReference")) 1009 parseElementProperties(json.getAsJsonObject("_nameReference"), res.getNameReferenceElement()); 1010 Type defaultValue = parseType("defaultValue", json); 1011 if (defaultValue != null) 1012 res.setDefaultValue(defaultValue); 1013 if (json.has("meaningWhenMissing")) 1014 res.setMeaningWhenMissingElement(parseMarkdown(json.get("meaningWhenMissing").getAsString())); 1015 if (json.has("_meaningWhenMissing")) 1016 parseElementProperties(json.getAsJsonObject("_meaningWhenMissing"), res.getMeaningWhenMissingElement()); 1017 Type fixed = parseType("fixed", json); 1018 if (fixed != null) 1019 res.setFixed(fixed); 1020 Type pattern = parseType("pattern", json); 1021 if (pattern != null) 1022 res.setPattern(pattern); 1023 Type example = parseType("example", json); 1024 if (example != null) 1025 res.setExample(example); 1026 Type minValue = parseType("minValue", json); 1027 if (minValue != null) 1028 res.setMinValue(minValue); 1029 Type maxValue = parseType("maxValue", json); 1030 if (maxValue != null) 1031 res.setMaxValue(maxValue); 1032 if (json.has("maxLength")) 1033 res.setMaxLengthElement(parseInteger(json.get("maxLength").getAsLong())); 1034 if (json.has("_maxLength")) 1035 parseElementProperties(json.getAsJsonObject("_maxLength"), res.getMaxLengthElement()); 1036 if (json.has("condition")) { 1037 JsonArray array = json.getAsJsonArray("condition"); 1038 for (int i = 0; i < array.size(); i++) { 1039 res.getCondition().add(parseId(array.get(i).getAsString())); 1040 } 1041 } 1042 ; 1043 if (json.has("_condition")) { 1044 JsonArray array = json.getAsJsonArray("_condition"); 1045 for (int i = 0; i < array.size(); i++) { 1046 if (i == res.getCondition().size()) 1047 res.getCondition().add(parseId(null)); 1048 if (array.get(i) instanceof JsonObject) 1049 parseElementProperties(array.get(i).getAsJsonObject(), res.getCondition().get(i)); 1050 } 1051 } 1052 ; 1053 if (json.has("constraint")) { 1054 JsonArray array = json.getAsJsonArray("constraint"); 1055 for (int i = 0; i < array.size(); i++) { 1056 res.getConstraint() 1057 .add(parseElementDefinitionElementDefinitionConstraintComponent(array.get(i).getAsJsonObject(), res)); 1058 } 1059 } 1060 ; 1061 if (json.has("mustSupport")) 1062 res.setMustSupportElement(parseBoolean(json.get("mustSupport").getAsBoolean())); 1063 if (json.has("_mustSupport")) 1064 parseElementProperties(json.getAsJsonObject("_mustSupport"), res.getMustSupportElement()); 1065 if (json.has("isModifier")) 1066 res.setIsModifierElement(parseBoolean(json.get("isModifier").getAsBoolean())); 1067 if (json.has("_isModifier")) 1068 parseElementProperties(json.getAsJsonObject("_isModifier"), res.getIsModifierElement()); 1069 if (json.has("isSummary")) 1070 res.setIsSummaryElement(parseBoolean(json.get("isSummary").getAsBoolean())); 1071 if (json.has("_isSummary")) 1072 parseElementProperties(json.getAsJsonObject("_isSummary"), res.getIsSummaryElement()); 1073 if (json.has("binding")) 1074 res.setBinding(parseElementDefinitionElementDefinitionBindingComponent(json.getAsJsonObject("binding"), res)); 1075 if (json.has("mapping")) { 1076 JsonArray array = json.getAsJsonArray("mapping"); 1077 for (int i = 0; i < array.size(); i++) { 1078 res.getMapping() 1079 .add(parseElementDefinitionElementDefinitionMappingComponent(array.get(i).getAsJsonObject(), res)); 1080 } 1081 } 1082 ; 1083 return res; 1084 } 1085 1086 protected ElementDefinition.ElementDefinitionSlicingComponent parseElementDefinitionElementDefinitionSlicingComponent( 1087 JsonObject json, ElementDefinition owner) throws IOException, FHIRFormatError { 1088 ElementDefinition.ElementDefinitionSlicingComponent res = new ElementDefinition.ElementDefinitionSlicingComponent(); 1089 parseElementProperties(json, res); 1090 if (json.has("discriminator")) { 1091 JsonArray array = json.getAsJsonArray("discriminator"); 1092 for (int i = 0; i < array.size(); i++) { 1093 res.getDiscriminator().add(parseString(array.get(i).getAsString())); 1094 } 1095 } 1096 ; 1097 if (json.has("_discriminator")) { 1098 JsonArray array = json.getAsJsonArray("_discriminator"); 1099 for (int i = 0; i < array.size(); i++) { 1100 if (i == res.getDiscriminator().size()) 1101 res.getDiscriminator().add(parseString(null)); 1102 if (array.get(i) instanceof JsonObject) 1103 parseElementProperties(array.get(i).getAsJsonObject(), res.getDiscriminator().get(i)); 1104 } 1105 } 1106 ; 1107 if (json.has("description")) 1108 res.setDescriptionElement(parseString(json.get("description").getAsString())); 1109 if (json.has("_description")) 1110 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 1111 if (json.has("ordered")) 1112 res.setOrderedElement(parseBoolean(json.get("ordered").getAsBoolean())); 1113 if (json.has("_ordered")) 1114 parseElementProperties(json.getAsJsonObject("_ordered"), res.getOrderedElement()); 1115 if (json.has("rules")) 1116 res.setRulesElement(parseEnumeration(json.get("rules").getAsString(), ElementDefinition.SlicingRules.NULL, 1117 new ElementDefinition.SlicingRulesEnumFactory())); 1118 if (json.has("_rules")) 1119 parseElementProperties(json.getAsJsonObject("_rules"), res.getRulesElement()); 1120 return res; 1121 } 1122 1123 protected ElementDefinition.ElementDefinitionBaseComponent parseElementDefinitionElementDefinitionBaseComponent( 1124 JsonObject json, ElementDefinition owner) throws IOException, FHIRFormatError { 1125 ElementDefinition.ElementDefinitionBaseComponent res = new ElementDefinition.ElementDefinitionBaseComponent(); 1126 parseElementProperties(json, res); 1127 if (json.has("path")) 1128 res.setPathElement(parseString(json.get("path").getAsString())); 1129 if (json.has("_path")) 1130 parseElementProperties(json.getAsJsonObject("_path"), res.getPathElement()); 1131 if (json.has("min")) 1132 res.setMinElement(parseInteger(json.get("min").getAsLong())); 1133 if (json.has("_min")) 1134 parseElementProperties(json.getAsJsonObject("_min"), res.getMinElement()); 1135 if (json.has("max")) 1136 res.setMaxElement(parseString(json.get("max").getAsString())); 1137 if (json.has("_max")) 1138 parseElementProperties(json.getAsJsonObject("_max"), res.getMaxElement()); 1139 return res; 1140 } 1141 1142 protected ElementDefinition.TypeRefComponent parseElementDefinitionTypeRefComponent(JsonObject json, 1143 ElementDefinition owner) throws IOException, FHIRFormatError { 1144 ElementDefinition.TypeRefComponent res = new ElementDefinition.TypeRefComponent(); 1145 parseElementProperties(json, res); 1146 if (json.has("code")) 1147 res.setCodeElement(parseCode(json.get("code").getAsString())); 1148 if (json.has("_code")) 1149 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 1150 if (json.has("profile")) { 1151 JsonArray array = json.getAsJsonArray("profile"); 1152 for (int i = 0; i < array.size(); i++) { 1153 res.getProfile().add(parseUri(array.get(i).getAsString())); 1154 } 1155 } 1156 ; 1157 if (json.has("_profile")) { 1158 JsonArray array = json.getAsJsonArray("_profile"); 1159 for (int i = 0; i < array.size(); i++) { 1160 if (i == res.getProfile().size()) 1161 res.getProfile().add(parseUri(null)); 1162 if (array.get(i) instanceof JsonObject) 1163 parseElementProperties(array.get(i).getAsJsonObject(), res.getProfile().get(i)); 1164 } 1165 } 1166 ; 1167 if (json.has("aggregation")) { 1168 JsonArray array = json.getAsJsonArray("aggregation"); 1169 for (int i = 0; i < array.size(); i++) { 1170 res.getAggregation().add(parseEnumeration(array.get(i).getAsString(), ElementDefinition.AggregationMode.NULL, 1171 new ElementDefinition.AggregationModeEnumFactory())); 1172 } 1173 } 1174 ; 1175 if (json.has("_aggregation")) { 1176 JsonArray array = json.getAsJsonArray("_aggregation"); 1177 for (int i = 0; i < array.size(); i++) { 1178 if (i == res.getAggregation().size()) 1179 res.getAggregation().add(parseEnumeration(null, ElementDefinition.AggregationMode.NULL, 1180 new ElementDefinition.AggregationModeEnumFactory())); 1181 if (array.get(i) instanceof JsonObject) 1182 parseElementProperties(array.get(i).getAsJsonObject(), res.getAggregation().get(i)); 1183 } 1184 } 1185 ; 1186 return res; 1187 } 1188 1189 protected ElementDefinition.ElementDefinitionConstraintComponent parseElementDefinitionElementDefinitionConstraintComponent( 1190 JsonObject json, ElementDefinition owner) throws IOException, FHIRFormatError { 1191 ElementDefinition.ElementDefinitionConstraintComponent res = new ElementDefinition.ElementDefinitionConstraintComponent(); 1192 parseElementProperties(json, res); 1193 if (json.has("key")) 1194 res.setKeyElement(parseId(json.get("key").getAsString())); 1195 if (json.has("_key")) 1196 parseElementProperties(json.getAsJsonObject("_key"), res.getKeyElement()); 1197 if (json.has("requirements")) 1198 res.setRequirementsElement(parseString(json.get("requirements").getAsString())); 1199 if (json.has("_requirements")) 1200 parseElementProperties(json.getAsJsonObject("_requirements"), res.getRequirementsElement()); 1201 if (json.has("severity")) 1202 res.setSeverityElement(parseEnumeration(json.get("severity").getAsString(), 1203 ElementDefinition.ConstraintSeverity.NULL, new ElementDefinition.ConstraintSeverityEnumFactory())); 1204 if (json.has("_severity")) 1205 parseElementProperties(json.getAsJsonObject("_severity"), res.getSeverityElement()); 1206 if (json.has("human")) 1207 res.setHumanElement(parseString(json.get("human").getAsString())); 1208 if (json.has("_human")) 1209 parseElementProperties(json.getAsJsonObject("_human"), res.getHumanElement()); 1210 if (json.has("xpath")) 1211 res.setXpathElement(parseString(json.get("xpath").getAsString())); 1212 if (json.has("_xpath")) 1213 parseElementProperties(json.getAsJsonObject("_xpath"), res.getXpathElement()); 1214 return res; 1215 } 1216 1217 protected ElementDefinition.ElementDefinitionBindingComponent parseElementDefinitionElementDefinitionBindingComponent( 1218 JsonObject json, ElementDefinition owner) throws IOException, FHIRFormatError { 1219 ElementDefinition.ElementDefinitionBindingComponent res = new ElementDefinition.ElementDefinitionBindingComponent(); 1220 parseElementProperties(json, res); 1221 if (json.has("strength")) 1222 res.setStrengthElement(parseEnumeration(json.get("strength").getAsString(), Enumerations.BindingStrength.NULL, 1223 new Enumerations.BindingStrengthEnumFactory())); 1224 if (json.has("_strength")) 1225 parseElementProperties(json.getAsJsonObject("_strength"), res.getStrengthElement()); 1226 if (json.has("description")) 1227 res.setDescriptionElement(parseString(json.get("description").getAsString())); 1228 if (json.has("_description")) 1229 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 1230 Type valueSet = parseType("valueSet", json); 1231 if (valueSet != null) 1232 res.setValueSet(valueSet); 1233 return res; 1234 } 1235 1236 protected ElementDefinition.ElementDefinitionMappingComponent parseElementDefinitionElementDefinitionMappingComponent( 1237 JsonObject json, ElementDefinition owner) throws IOException, FHIRFormatError { 1238 ElementDefinition.ElementDefinitionMappingComponent res = new ElementDefinition.ElementDefinitionMappingComponent(); 1239 parseElementProperties(json, res); 1240 if (json.has("identity")) 1241 res.setIdentityElement(parseId(json.get("identity").getAsString())); 1242 if (json.has("_identity")) 1243 parseElementProperties(json.getAsJsonObject("_identity"), res.getIdentityElement()); 1244 if (json.has("language")) 1245 res.setLanguageElement(parseCode(json.get("language").getAsString())); 1246 if (json.has("_language")) 1247 parseElementProperties(json.getAsJsonObject("_language"), res.getLanguageElement()); 1248 if (json.has("map")) 1249 res.setMapElement(parseString(json.get("map").getAsString())); 1250 if (json.has("_map")) 1251 parseElementProperties(json.getAsJsonObject("_map"), res.getMapElement()); 1252 return res; 1253 } 1254 1255 protected void parseDomainResourceProperties(JsonObject json, DomainResource res) 1256 throws IOException, FHIRFormatError { 1257 parseResourceProperties(json, res); 1258 if (json.has("text")) 1259 res.setText(parseNarrative(json.getAsJsonObject("text"))); 1260 if (json.has("contained")) { 1261 JsonArray array = json.getAsJsonArray("contained"); 1262 for (int i = 0; i < array.size(); i++) { 1263 res.getContained().add(parseResource(array.get(i).getAsJsonObject())); 1264 } 1265 } 1266 ; 1267 if (json.has("extension")) { 1268 JsonArray array = json.getAsJsonArray("extension"); 1269 for (int i = 0; i < array.size(); i++) { 1270 res.getExtension().add(parseExtension(array.get(i).getAsJsonObject())); 1271 } 1272 } 1273 ; 1274 if (json.has("modifierExtension")) { 1275 JsonArray array = json.getAsJsonArray("modifierExtension"); 1276 for (int i = 0; i < array.size(); i++) { 1277 res.getModifierExtension().add(parseExtension(array.get(i).getAsJsonObject())); 1278 } 1279 } 1280 ; 1281 } 1282 1283 protected Parameters parseParameters(JsonObject json) throws IOException, FHIRFormatError { 1284 Parameters res = new Parameters(); 1285 parseResourceProperties(json, res); 1286 if (json.has("parameter")) { 1287 JsonArray array = json.getAsJsonArray("parameter"); 1288 for (int i = 0; i < array.size(); i++) { 1289 res.getParameter().add(parseParametersParametersParameterComponent(array.get(i).getAsJsonObject(), res)); 1290 } 1291 } 1292 ; 1293 return res; 1294 } 1295 1296 protected Parameters.ParametersParameterComponent parseParametersParametersParameterComponent(JsonObject json, 1297 Parameters owner) throws IOException, FHIRFormatError { 1298 Parameters.ParametersParameterComponent res = new Parameters.ParametersParameterComponent(); 1299 parseBackboneProperties(json, res); 1300 if (json.has("name")) 1301 res.setNameElement(parseString(json.get("name").getAsString())); 1302 if (json.has("_name")) 1303 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 1304 Type value = parseType("value", json); 1305 if (value != null) 1306 res.setValue(value); 1307 if (json.has("resource")) 1308 res.setResource(parseResource(json.getAsJsonObject("resource"))); 1309 if (json.has("part")) { 1310 JsonArray array = json.getAsJsonArray("part"); 1311 for (int i = 0; i < array.size(); i++) { 1312 res.getPart().add(parseParametersParametersParameterComponent(array.get(i).getAsJsonObject(), owner)); 1313 } 1314 } 1315 ; 1316 return res; 1317 } 1318 1319 protected void parseResourceProperties(JsonObject json, Resource res) throws IOException, FHIRFormatError { 1320 if (json.has("id")) 1321 res.setIdElement(parseId(json.get("id").getAsString())); 1322 if (json.has("_id")) 1323 parseElementProperties(json.getAsJsonObject("_id"), res.getIdElement()); 1324 if (json.has("meta")) 1325 res.setMeta(parseMeta(json.getAsJsonObject("meta"))); 1326 if (json.has("implicitRules")) 1327 res.setImplicitRulesElement(parseUri(json.get("implicitRules").getAsString())); 1328 if (json.has("_implicitRules")) 1329 parseElementProperties(json.getAsJsonObject("_implicitRules"), res.getImplicitRulesElement()); 1330 if (json.has("language")) 1331 res.setLanguageElement(parseCode(json.get("language").getAsString())); 1332 if (json.has("_language")) 1333 parseElementProperties(json.getAsJsonObject("_language"), res.getLanguageElement()); 1334 } 1335 1336 protected Account parseAccount(JsonObject json) throws IOException, FHIRFormatError { 1337 Account res = new Account(); 1338 parseDomainResourceProperties(json, res); 1339 if (json.has("identifier")) { 1340 JsonArray array = json.getAsJsonArray("identifier"); 1341 for (int i = 0; i < array.size(); i++) { 1342 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 1343 } 1344 } 1345 ; 1346 if (json.has("name")) 1347 res.setNameElement(parseString(json.get("name").getAsString())); 1348 if (json.has("_name")) 1349 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 1350 if (json.has("type")) 1351 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 1352 if (json.has("status")) 1353 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Account.AccountStatus.NULL, 1354 new Account.AccountStatusEnumFactory())); 1355 if (json.has("_status")) 1356 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 1357 if (json.has("activePeriod")) 1358 res.setActivePeriod(parsePeriod(json.getAsJsonObject("activePeriod"))); 1359 if (json.has("currency")) 1360 res.setCurrency(parseCoding(json.getAsJsonObject("currency"))); 1361 if (json.has("balance")) 1362 res.setBalance(parseMoney(json.getAsJsonObject("balance"))); 1363 if (json.has("coveragePeriod")) 1364 res.setCoveragePeriod(parsePeriod(json.getAsJsonObject("coveragePeriod"))); 1365 if (json.has("subject")) 1366 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 1367 if (json.has("owner")) 1368 res.setOwner(parseReference(json.getAsJsonObject("owner"))); 1369 if (json.has("description")) 1370 res.setDescriptionElement(parseString(json.get("description").getAsString())); 1371 if (json.has("_description")) 1372 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 1373 return res; 1374 } 1375 1376 protected AllergyIntolerance parseAllergyIntolerance(JsonObject json) throws IOException, FHIRFormatError { 1377 AllergyIntolerance res = new AllergyIntolerance(); 1378 parseDomainResourceProperties(json, res); 1379 if (json.has("identifier")) { 1380 JsonArray array = json.getAsJsonArray("identifier"); 1381 for (int i = 0; i < array.size(); i++) { 1382 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 1383 } 1384 } 1385 ; 1386 if (json.has("onset")) 1387 res.setOnsetElement(parseDateTime(json.get("onset").getAsString())); 1388 if (json.has("_onset")) 1389 parseElementProperties(json.getAsJsonObject("_onset"), res.getOnsetElement()); 1390 if (json.has("recordedDate")) 1391 res.setRecordedDateElement(parseDateTime(json.get("recordedDate").getAsString())); 1392 if (json.has("_recordedDate")) 1393 parseElementProperties(json.getAsJsonObject("_recordedDate"), res.getRecordedDateElement()); 1394 if (json.has("recorder")) 1395 res.setRecorder(parseReference(json.getAsJsonObject("recorder"))); 1396 if (json.has("patient")) 1397 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 1398 if (json.has("reporter")) 1399 res.setReporter(parseReference(json.getAsJsonObject("reporter"))); 1400 if (json.has("substance")) 1401 res.setSubstance(parseCodeableConcept(json.getAsJsonObject("substance"))); 1402 if (json.has("status")) 1403 res.setStatusElement( 1404 parseEnumeration(json.get("status").getAsString(), AllergyIntolerance.AllergyIntoleranceStatus.NULL, 1405 new AllergyIntolerance.AllergyIntoleranceStatusEnumFactory())); 1406 if (json.has("_status")) 1407 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 1408 if (json.has("criticality")) 1409 res.setCriticalityElement( 1410 parseEnumeration(json.get("criticality").getAsString(), AllergyIntolerance.AllergyIntoleranceCriticality.NULL, 1411 new AllergyIntolerance.AllergyIntoleranceCriticalityEnumFactory())); 1412 if (json.has("_criticality")) 1413 parseElementProperties(json.getAsJsonObject("_criticality"), res.getCriticalityElement()); 1414 if (json.has("type")) 1415 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), 1416 AllergyIntolerance.AllergyIntoleranceType.NULL, new AllergyIntolerance.AllergyIntoleranceTypeEnumFactory())); 1417 if (json.has("_type")) 1418 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 1419 if (json.has("category")) 1420 res.setCategoryElement( 1421 parseEnumeration(json.get("category").getAsString(), AllergyIntolerance.AllergyIntoleranceCategory.NULL, 1422 new AllergyIntolerance.AllergyIntoleranceCategoryEnumFactory())); 1423 if (json.has("_category")) 1424 parseElementProperties(json.getAsJsonObject("_category"), res.getCategoryElement()); 1425 if (json.has("lastOccurence")) 1426 res.setLastOccurenceElement(parseDateTime(json.get("lastOccurence").getAsString())); 1427 if (json.has("_lastOccurence")) 1428 parseElementProperties(json.getAsJsonObject("_lastOccurence"), res.getLastOccurenceElement()); 1429 if (json.has("note")) 1430 res.setNote(parseAnnotation(json.getAsJsonObject("note"))); 1431 if (json.has("reaction")) { 1432 JsonArray array = json.getAsJsonArray("reaction"); 1433 for (int i = 0; i < array.size(); i++) { 1434 res.getReaction() 1435 .add(parseAllergyIntoleranceAllergyIntoleranceReactionComponent(array.get(i).getAsJsonObject(), res)); 1436 } 1437 } 1438 ; 1439 return res; 1440 } 1441 1442 protected AllergyIntolerance.AllergyIntoleranceReactionComponent parseAllergyIntoleranceAllergyIntoleranceReactionComponent( 1443 JsonObject json, AllergyIntolerance owner) throws IOException, FHIRFormatError { 1444 AllergyIntolerance.AllergyIntoleranceReactionComponent res = new AllergyIntolerance.AllergyIntoleranceReactionComponent(); 1445 parseBackboneProperties(json, res); 1446 if (json.has("substance")) 1447 res.setSubstance(parseCodeableConcept(json.getAsJsonObject("substance"))); 1448 if (json.has("certainty")) 1449 res.setCertaintyElement( 1450 parseEnumeration(json.get("certainty").getAsString(), AllergyIntolerance.AllergyIntoleranceCertainty.NULL, 1451 new AllergyIntolerance.AllergyIntoleranceCertaintyEnumFactory())); 1452 if (json.has("_certainty")) 1453 parseElementProperties(json.getAsJsonObject("_certainty"), res.getCertaintyElement()); 1454 if (json.has("manifestation")) { 1455 JsonArray array = json.getAsJsonArray("manifestation"); 1456 for (int i = 0; i < array.size(); i++) { 1457 res.getManifestation().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 1458 } 1459 } 1460 ; 1461 if (json.has("description")) 1462 res.setDescriptionElement(parseString(json.get("description").getAsString())); 1463 if (json.has("_description")) 1464 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 1465 if (json.has("onset")) 1466 res.setOnsetElement(parseDateTime(json.get("onset").getAsString())); 1467 if (json.has("_onset")) 1468 parseElementProperties(json.getAsJsonObject("_onset"), res.getOnsetElement()); 1469 if (json.has("severity")) 1470 res.setSeverityElement( 1471 parseEnumeration(json.get("severity").getAsString(), AllergyIntolerance.AllergyIntoleranceSeverity.NULL, 1472 new AllergyIntolerance.AllergyIntoleranceSeverityEnumFactory())); 1473 if (json.has("_severity")) 1474 parseElementProperties(json.getAsJsonObject("_severity"), res.getSeverityElement()); 1475 if (json.has("exposureRoute")) 1476 res.setExposureRoute(parseCodeableConcept(json.getAsJsonObject("exposureRoute"))); 1477 if (json.has("note")) 1478 res.setNote(parseAnnotation(json.getAsJsonObject("note"))); 1479 return res; 1480 } 1481 1482 protected Appointment parseAppointment(JsonObject json) throws IOException, FHIRFormatError { 1483 Appointment res = new Appointment(); 1484 parseDomainResourceProperties(json, res); 1485 if (json.has("identifier")) { 1486 JsonArray array = json.getAsJsonArray("identifier"); 1487 for (int i = 0; i < array.size(); i++) { 1488 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 1489 } 1490 } 1491 ; 1492 if (json.has("status")) 1493 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Appointment.AppointmentStatus.NULL, 1494 new Appointment.AppointmentStatusEnumFactory())); 1495 if (json.has("_status")) 1496 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 1497 if (json.has("type")) 1498 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 1499 if (json.has("reason")) 1500 res.setReason(parseCodeableConcept(json.getAsJsonObject("reason"))); 1501 if (json.has("priority")) 1502 res.setPriorityElement(parseUnsignedInt(json.get("priority").getAsString())); 1503 if (json.has("_priority")) 1504 parseElementProperties(json.getAsJsonObject("_priority"), res.getPriorityElement()); 1505 if (json.has("description")) 1506 res.setDescriptionElement(parseString(json.get("description").getAsString())); 1507 if (json.has("_description")) 1508 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 1509 if (json.has("start")) 1510 res.setStartElement(parseInstant(json.get("start").getAsString())); 1511 if (json.has("_start")) 1512 parseElementProperties(json.getAsJsonObject("_start"), res.getStartElement()); 1513 if (json.has("end")) 1514 res.setEndElement(parseInstant(json.get("end").getAsString())); 1515 if (json.has("_end")) 1516 parseElementProperties(json.getAsJsonObject("_end"), res.getEndElement()); 1517 if (json.has("minutesDuration")) 1518 res.setMinutesDurationElement(parsePositiveInt(json.get("minutesDuration").getAsString())); 1519 if (json.has("_minutesDuration")) 1520 parseElementProperties(json.getAsJsonObject("_minutesDuration"), res.getMinutesDurationElement()); 1521 if (json.has("slot")) { 1522 JsonArray array = json.getAsJsonArray("slot"); 1523 for (int i = 0; i < array.size(); i++) { 1524 res.getSlot().add(parseReference(array.get(i).getAsJsonObject())); 1525 } 1526 } 1527 ; 1528 if (json.has("comment")) 1529 res.setCommentElement(parseString(json.get("comment").getAsString())); 1530 if (json.has("_comment")) 1531 parseElementProperties(json.getAsJsonObject("_comment"), res.getCommentElement()); 1532 if (json.has("participant")) { 1533 JsonArray array = json.getAsJsonArray("participant"); 1534 for (int i = 0; i < array.size(); i++) { 1535 res.getParticipant().add(parseAppointmentAppointmentParticipantComponent(array.get(i).getAsJsonObject(), res)); 1536 } 1537 } 1538 ; 1539 return res; 1540 } 1541 1542 protected Appointment.AppointmentParticipantComponent parseAppointmentAppointmentParticipantComponent(JsonObject json, 1543 Appointment owner) throws IOException, FHIRFormatError { 1544 Appointment.AppointmentParticipantComponent res = new Appointment.AppointmentParticipantComponent(); 1545 parseBackboneProperties(json, res); 1546 if (json.has("type")) { 1547 JsonArray array = json.getAsJsonArray("type"); 1548 for (int i = 0; i < array.size(); i++) { 1549 res.getType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 1550 } 1551 } 1552 ; 1553 if (json.has("actor")) 1554 res.setActor(parseReference(json.getAsJsonObject("actor"))); 1555 if (json.has("required")) 1556 res.setRequiredElement(parseEnumeration(json.get("required").getAsString(), Appointment.ParticipantRequired.NULL, 1557 new Appointment.ParticipantRequiredEnumFactory())); 1558 if (json.has("_required")) 1559 parseElementProperties(json.getAsJsonObject("_required"), res.getRequiredElement()); 1560 if (json.has("status")) 1561 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Appointment.ParticipationStatus.NULL, 1562 new Appointment.ParticipationStatusEnumFactory())); 1563 if (json.has("_status")) 1564 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 1565 return res; 1566 } 1567 1568 protected AppointmentResponse parseAppointmentResponse(JsonObject json) throws IOException, FHIRFormatError { 1569 AppointmentResponse res = new AppointmentResponse(); 1570 parseDomainResourceProperties(json, res); 1571 if (json.has("identifier")) { 1572 JsonArray array = json.getAsJsonArray("identifier"); 1573 for (int i = 0; i < array.size(); i++) { 1574 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 1575 } 1576 } 1577 ; 1578 if (json.has("appointment")) 1579 res.setAppointment(parseReference(json.getAsJsonObject("appointment"))); 1580 if (json.has("start")) 1581 res.setStartElement(parseInstant(json.get("start").getAsString())); 1582 if (json.has("_start")) 1583 parseElementProperties(json.getAsJsonObject("_start"), res.getStartElement()); 1584 if (json.has("end")) 1585 res.setEndElement(parseInstant(json.get("end").getAsString())); 1586 if (json.has("_end")) 1587 parseElementProperties(json.getAsJsonObject("_end"), res.getEndElement()); 1588 if (json.has("participantType")) { 1589 JsonArray array = json.getAsJsonArray("participantType"); 1590 for (int i = 0; i < array.size(); i++) { 1591 res.getParticipantType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 1592 } 1593 } 1594 ; 1595 if (json.has("actor")) 1596 res.setActor(parseReference(json.getAsJsonObject("actor"))); 1597 if (json.has("participantStatus")) 1598 res.setParticipantStatusElement(parseEnumeration(json.get("participantStatus").getAsString(), 1599 AppointmentResponse.ParticipantStatus.NULL, new AppointmentResponse.ParticipantStatusEnumFactory())); 1600 if (json.has("_participantStatus")) 1601 parseElementProperties(json.getAsJsonObject("_participantStatus"), res.getParticipantStatusElement()); 1602 if (json.has("comment")) 1603 res.setCommentElement(parseString(json.get("comment").getAsString())); 1604 if (json.has("_comment")) 1605 parseElementProperties(json.getAsJsonObject("_comment"), res.getCommentElement()); 1606 return res; 1607 } 1608 1609 protected AuditEvent parseAuditEvent(JsonObject json) throws IOException, FHIRFormatError { 1610 AuditEvent res = new AuditEvent(); 1611 parseDomainResourceProperties(json, res); 1612 if (json.has("event")) 1613 res.setEvent(parseAuditEventAuditEventEventComponent(json.getAsJsonObject("event"), res)); 1614 if (json.has("participant")) { 1615 JsonArray array = json.getAsJsonArray("participant"); 1616 for (int i = 0; i < array.size(); i++) { 1617 res.getParticipant().add(parseAuditEventAuditEventParticipantComponent(array.get(i).getAsJsonObject(), res)); 1618 } 1619 } 1620 ; 1621 if (json.has("source")) 1622 res.setSource(parseAuditEventAuditEventSourceComponent(json.getAsJsonObject("source"), res)); 1623 if (json.has("object")) { 1624 JsonArray array = json.getAsJsonArray("object"); 1625 for (int i = 0; i < array.size(); i++) { 1626 res.getObject().add(parseAuditEventAuditEventObjectComponent(array.get(i).getAsJsonObject(), res)); 1627 } 1628 } 1629 ; 1630 return res; 1631 } 1632 1633 protected AuditEvent.AuditEventEventComponent parseAuditEventAuditEventEventComponent(JsonObject json, 1634 AuditEvent owner) throws IOException, FHIRFormatError { 1635 AuditEvent.AuditEventEventComponent res = new AuditEvent.AuditEventEventComponent(); 1636 parseBackboneProperties(json, res); 1637 if (json.has("type")) 1638 res.setType(parseCoding(json.getAsJsonObject("type"))); 1639 if (json.has("subtype")) { 1640 JsonArray array = json.getAsJsonArray("subtype"); 1641 for (int i = 0; i < array.size(); i++) { 1642 res.getSubtype().add(parseCoding(array.get(i).getAsJsonObject())); 1643 } 1644 } 1645 ; 1646 if (json.has("action")) 1647 res.setActionElement(parseEnumeration(json.get("action").getAsString(), AuditEvent.AuditEventAction.NULL, 1648 new AuditEvent.AuditEventActionEnumFactory())); 1649 if (json.has("_action")) 1650 parseElementProperties(json.getAsJsonObject("_action"), res.getActionElement()); 1651 if (json.has("dateTime")) 1652 res.setDateTimeElement(parseInstant(json.get("dateTime").getAsString())); 1653 if (json.has("_dateTime")) 1654 parseElementProperties(json.getAsJsonObject("_dateTime"), res.getDateTimeElement()); 1655 if (json.has("outcome")) 1656 res.setOutcomeElement(parseEnumeration(json.get("outcome").getAsString(), AuditEvent.AuditEventOutcome.NULL, 1657 new AuditEvent.AuditEventOutcomeEnumFactory())); 1658 if (json.has("_outcome")) 1659 parseElementProperties(json.getAsJsonObject("_outcome"), res.getOutcomeElement()); 1660 if (json.has("outcomeDesc")) 1661 res.setOutcomeDescElement(parseString(json.get("outcomeDesc").getAsString())); 1662 if (json.has("_outcomeDesc")) 1663 parseElementProperties(json.getAsJsonObject("_outcomeDesc"), res.getOutcomeDescElement()); 1664 if (json.has("purposeOfEvent")) { 1665 JsonArray array = json.getAsJsonArray("purposeOfEvent"); 1666 for (int i = 0; i < array.size(); i++) { 1667 res.getPurposeOfEvent().add(parseCoding(array.get(i).getAsJsonObject())); 1668 } 1669 } 1670 ; 1671 return res; 1672 } 1673 1674 protected AuditEvent.AuditEventParticipantComponent parseAuditEventAuditEventParticipantComponent(JsonObject json, 1675 AuditEvent owner) throws IOException, FHIRFormatError { 1676 AuditEvent.AuditEventParticipantComponent res = new AuditEvent.AuditEventParticipantComponent(); 1677 parseBackboneProperties(json, res); 1678 if (json.has("role")) { 1679 JsonArray array = json.getAsJsonArray("role"); 1680 for (int i = 0; i < array.size(); i++) { 1681 res.getRole().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 1682 } 1683 } 1684 ; 1685 if (json.has("reference")) 1686 res.setReference(parseReference(json.getAsJsonObject("reference"))); 1687 if (json.has("userId")) 1688 res.setUserId(parseIdentifier(json.getAsJsonObject("userId"))); 1689 if (json.has("altId")) 1690 res.setAltIdElement(parseString(json.get("altId").getAsString())); 1691 if (json.has("_altId")) 1692 parseElementProperties(json.getAsJsonObject("_altId"), res.getAltIdElement()); 1693 if (json.has("name")) 1694 res.setNameElement(parseString(json.get("name").getAsString())); 1695 if (json.has("_name")) 1696 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 1697 if (json.has("requestor")) 1698 res.setRequestorElement(parseBoolean(json.get("requestor").getAsBoolean())); 1699 if (json.has("_requestor")) 1700 parseElementProperties(json.getAsJsonObject("_requestor"), res.getRequestorElement()); 1701 if (json.has("location")) 1702 res.setLocation(parseReference(json.getAsJsonObject("location"))); 1703 if (json.has("policy")) { 1704 JsonArray array = json.getAsJsonArray("policy"); 1705 for (int i = 0; i < array.size(); i++) { 1706 res.getPolicy().add(parseUri(array.get(i).getAsString())); 1707 } 1708 } 1709 ; 1710 if (json.has("_policy")) { 1711 JsonArray array = json.getAsJsonArray("_policy"); 1712 for (int i = 0; i < array.size(); i++) { 1713 if (i == res.getPolicy().size()) 1714 res.getPolicy().add(parseUri(null)); 1715 if (array.get(i) instanceof JsonObject) 1716 parseElementProperties(array.get(i).getAsJsonObject(), res.getPolicy().get(i)); 1717 } 1718 } 1719 ; 1720 if (json.has("media")) 1721 res.setMedia(parseCoding(json.getAsJsonObject("media"))); 1722 if (json.has("network")) 1723 res.setNetwork(parseAuditEventAuditEventParticipantNetworkComponent(json.getAsJsonObject("network"), owner)); 1724 if (json.has("purposeOfUse")) { 1725 JsonArray array = json.getAsJsonArray("purposeOfUse"); 1726 for (int i = 0; i < array.size(); i++) { 1727 res.getPurposeOfUse().add(parseCoding(array.get(i).getAsJsonObject())); 1728 } 1729 } 1730 ; 1731 return res; 1732 } 1733 1734 protected AuditEvent.AuditEventParticipantNetworkComponent parseAuditEventAuditEventParticipantNetworkComponent( 1735 JsonObject json, AuditEvent owner) throws IOException, FHIRFormatError { 1736 AuditEvent.AuditEventParticipantNetworkComponent res = new AuditEvent.AuditEventParticipantNetworkComponent(); 1737 parseBackboneProperties(json, res); 1738 if (json.has("address")) 1739 res.setAddressElement(parseString(json.get("address").getAsString())); 1740 if (json.has("_address")) 1741 parseElementProperties(json.getAsJsonObject("_address"), res.getAddressElement()); 1742 if (json.has("type")) 1743 res.setTypeElement( 1744 parseEnumeration(json.get("type").getAsString(), AuditEvent.AuditEventParticipantNetworkType.NULL, 1745 new AuditEvent.AuditEventParticipantNetworkTypeEnumFactory())); 1746 if (json.has("_type")) 1747 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 1748 return res; 1749 } 1750 1751 protected AuditEvent.AuditEventSourceComponent parseAuditEventAuditEventSourceComponent(JsonObject json, 1752 AuditEvent owner) throws IOException, FHIRFormatError { 1753 AuditEvent.AuditEventSourceComponent res = new AuditEvent.AuditEventSourceComponent(); 1754 parseBackboneProperties(json, res); 1755 if (json.has("site")) 1756 res.setSiteElement(parseString(json.get("site").getAsString())); 1757 if (json.has("_site")) 1758 parseElementProperties(json.getAsJsonObject("_site"), res.getSiteElement()); 1759 if (json.has("identifier")) 1760 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 1761 if (json.has("type")) { 1762 JsonArray array = json.getAsJsonArray("type"); 1763 for (int i = 0; i < array.size(); i++) { 1764 res.getType().add(parseCoding(array.get(i).getAsJsonObject())); 1765 } 1766 } 1767 ; 1768 return res; 1769 } 1770 1771 protected AuditEvent.AuditEventObjectComponent parseAuditEventAuditEventObjectComponent(JsonObject json, 1772 AuditEvent owner) throws IOException, FHIRFormatError { 1773 AuditEvent.AuditEventObjectComponent res = new AuditEvent.AuditEventObjectComponent(); 1774 parseBackboneProperties(json, res); 1775 if (json.has("identifier")) 1776 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 1777 if (json.has("reference")) 1778 res.setReference(parseReference(json.getAsJsonObject("reference"))); 1779 if (json.has("type")) 1780 res.setType(parseCoding(json.getAsJsonObject("type"))); 1781 if (json.has("role")) 1782 res.setRole(parseCoding(json.getAsJsonObject("role"))); 1783 if (json.has("lifecycle")) 1784 res.setLifecycle(parseCoding(json.getAsJsonObject("lifecycle"))); 1785 if (json.has("securityLabel")) { 1786 JsonArray array = json.getAsJsonArray("securityLabel"); 1787 for (int i = 0; i < array.size(); i++) { 1788 res.getSecurityLabel().add(parseCoding(array.get(i).getAsJsonObject())); 1789 } 1790 } 1791 ; 1792 if (json.has("name")) 1793 res.setNameElement(parseString(json.get("name").getAsString())); 1794 if (json.has("_name")) 1795 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 1796 if (json.has("description")) 1797 res.setDescriptionElement(parseString(json.get("description").getAsString())); 1798 if (json.has("_description")) 1799 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 1800 if (json.has("query")) 1801 res.setQueryElement(parseBase64Binary(json.get("query").getAsString())); 1802 if (json.has("_query")) 1803 parseElementProperties(json.getAsJsonObject("_query"), res.getQueryElement()); 1804 if (json.has("detail")) { 1805 JsonArray array = json.getAsJsonArray("detail"); 1806 for (int i = 0; i < array.size(); i++) { 1807 res.getDetail().add(parseAuditEventAuditEventObjectDetailComponent(array.get(i).getAsJsonObject(), owner)); 1808 } 1809 } 1810 ; 1811 return res; 1812 } 1813 1814 protected AuditEvent.AuditEventObjectDetailComponent parseAuditEventAuditEventObjectDetailComponent(JsonObject json, 1815 AuditEvent owner) throws IOException, FHIRFormatError { 1816 AuditEvent.AuditEventObjectDetailComponent res = new AuditEvent.AuditEventObjectDetailComponent(); 1817 parseBackboneProperties(json, res); 1818 if (json.has("type")) 1819 res.setTypeElement(parseString(json.get("type").getAsString())); 1820 if (json.has("_type")) 1821 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 1822 if (json.has("value")) 1823 res.setValueElement(parseBase64Binary(json.get("value").getAsString())); 1824 if (json.has("_value")) 1825 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 1826 return res; 1827 } 1828 1829 protected Basic parseBasic(JsonObject json) throws IOException, FHIRFormatError { 1830 Basic res = new Basic(); 1831 parseDomainResourceProperties(json, res); 1832 if (json.has("identifier")) { 1833 JsonArray array = json.getAsJsonArray("identifier"); 1834 for (int i = 0; i < array.size(); i++) { 1835 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 1836 } 1837 } 1838 ; 1839 if (json.has("code")) 1840 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 1841 if (json.has("subject")) 1842 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 1843 if (json.has("author")) 1844 res.setAuthor(parseReference(json.getAsJsonObject("author"))); 1845 if (json.has("created")) 1846 res.setCreatedElement(parseDate(json.get("created").getAsString())); 1847 if (json.has("_created")) 1848 parseElementProperties(json.getAsJsonObject("_created"), res.getCreatedElement()); 1849 return res; 1850 } 1851 1852 protected Binary parseBinary(JsonObject json) throws IOException, FHIRFormatError { 1853 Binary res = new Binary(); 1854 parseResourceProperties(json, res); 1855 if (json.has("contentType")) 1856 res.setContentTypeElement(parseCode(json.get("contentType").getAsString())); 1857 if (json.has("_contentType")) 1858 parseElementProperties(json.getAsJsonObject("_contentType"), res.getContentTypeElement()); 1859 if (json.has("content")) 1860 res.setContentElement(parseBase64Binary(json.get("content").getAsString())); 1861 if (json.has("_content")) 1862 parseElementProperties(json.getAsJsonObject("_content"), res.getContentElement()); 1863 return res; 1864 } 1865 1866 protected BodySite parseBodySite(JsonObject json) throws IOException, FHIRFormatError { 1867 BodySite res = new BodySite(); 1868 parseDomainResourceProperties(json, res); 1869 if (json.has("patient")) 1870 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 1871 if (json.has("identifier")) { 1872 JsonArray array = json.getAsJsonArray("identifier"); 1873 for (int i = 0; i < array.size(); i++) { 1874 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 1875 } 1876 } 1877 ; 1878 if (json.has("code")) 1879 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 1880 if (json.has("modifier")) { 1881 JsonArray array = json.getAsJsonArray("modifier"); 1882 for (int i = 0; i < array.size(); i++) { 1883 res.getModifier().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 1884 } 1885 } 1886 ; 1887 if (json.has("description")) 1888 res.setDescriptionElement(parseString(json.get("description").getAsString())); 1889 if (json.has("_description")) 1890 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 1891 if (json.has("image")) { 1892 JsonArray array = json.getAsJsonArray("image"); 1893 for (int i = 0; i < array.size(); i++) { 1894 res.getImage().add(parseAttachment(array.get(i).getAsJsonObject())); 1895 } 1896 } 1897 ; 1898 return res; 1899 } 1900 1901 protected Bundle parseBundle(JsonObject json) throws IOException, FHIRFormatError { 1902 Bundle res = new Bundle(); 1903 parseResourceProperties(json, res); 1904 if (json.has("type")) 1905 res.setTypeElement( 1906 parseEnumeration(json.get("type").getAsString(), Bundle.BundleType.NULL, new Bundle.BundleTypeEnumFactory())); 1907 if (json.has("_type")) 1908 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 1909 if (json.has("total")) 1910 res.setTotalElement(parseUnsignedInt(json.get("total").getAsString())); 1911 if (json.has("_total")) 1912 parseElementProperties(json.getAsJsonObject("_total"), res.getTotalElement()); 1913 if (json.has("link")) { 1914 JsonArray array = json.getAsJsonArray("link"); 1915 for (int i = 0; i < array.size(); i++) { 1916 res.getLink().add(parseBundleBundleLinkComponent(array.get(i).getAsJsonObject(), res)); 1917 } 1918 } 1919 ; 1920 if (json.has("entry")) { 1921 JsonArray array = json.getAsJsonArray("entry"); 1922 for (int i = 0; i < array.size(); i++) { 1923 res.getEntry().add(parseBundleBundleEntryComponent(array.get(i).getAsJsonObject(), res)); 1924 } 1925 } 1926 ; 1927 if (json.has("signature")) 1928 res.setSignature(parseSignature(json.getAsJsonObject("signature"))); 1929 return res; 1930 } 1931 1932 protected Bundle.BundleLinkComponent parseBundleBundleLinkComponent(JsonObject json, Bundle owner) 1933 throws IOException, FHIRFormatError { 1934 Bundle.BundleLinkComponent res = new Bundle.BundleLinkComponent(); 1935 parseBackboneProperties(json, res); 1936 if (json.has("relation")) 1937 res.setRelationElement(parseString(json.get("relation").getAsString())); 1938 if (json.has("_relation")) 1939 parseElementProperties(json.getAsJsonObject("_relation"), res.getRelationElement()); 1940 if (json.has("url")) 1941 res.setUrlElement(parseUri(json.get("url").getAsString())); 1942 if (json.has("_url")) 1943 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 1944 return res; 1945 } 1946 1947 protected Bundle.BundleEntryComponent parseBundleBundleEntryComponent(JsonObject json, Bundle owner) 1948 throws IOException, FHIRFormatError { 1949 Bundle.BundleEntryComponent res = new Bundle.BundleEntryComponent(); 1950 parseBackboneProperties(json, res); 1951 if (json.has("link")) { 1952 JsonArray array = json.getAsJsonArray("link"); 1953 for (int i = 0; i < array.size(); i++) { 1954 res.getLink().add(parseBundleBundleLinkComponent(array.get(i).getAsJsonObject(), owner)); 1955 } 1956 } 1957 ; 1958 if (json.has("fullUrl")) 1959 res.setFullUrlElement(parseUri(json.get("fullUrl").getAsString())); 1960 if (json.has("_fullUrl")) 1961 parseElementProperties(json.getAsJsonObject("_fullUrl"), res.getFullUrlElement()); 1962 if (json.has("resource")) 1963 res.setResource(parseResource(json.getAsJsonObject("resource"))); 1964 if (json.has("search")) 1965 res.setSearch(parseBundleBundleEntrySearchComponent(json.getAsJsonObject("search"), owner)); 1966 if (json.has("request")) 1967 res.setRequest(parseBundleBundleEntryRequestComponent(json.getAsJsonObject("request"), owner)); 1968 if (json.has("response")) 1969 res.setResponse(parseBundleBundleEntryResponseComponent(json.getAsJsonObject("response"), owner)); 1970 return res; 1971 } 1972 1973 protected Bundle.BundleEntrySearchComponent parseBundleBundleEntrySearchComponent(JsonObject json, Bundle owner) 1974 throws IOException, FHIRFormatError { 1975 Bundle.BundleEntrySearchComponent res = new Bundle.BundleEntrySearchComponent(); 1976 parseBackboneProperties(json, res); 1977 if (json.has("mode")) 1978 res.setModeElement(parseEnumeration(json.get("mode").getAsString(), Bundle.SearchEntryMode.NULL, 1979 new Bundle.SearchEntryModeEnumFactory())); 1980 if (json.has("_mode")) 1981 parseElementProperties(json.getAsJsonObject("_mode"), res.getModeElement()); 1982 if (json.has("score")) 1983 res.setScoreElement(parseDecimal(json.get("score").getAsBigDecimal())); 1984 if (json.has("_score")) 1985 parseElementProperties(json.getAsJsonObject("_score"), res.getScoreElement()); 1986 return res; 1987 } 1988 1989 protected Bundle.BundleEntryRequestComponent parseBundleBundleEntryRequestComponent(JsonObject json, Bundle owner) 1990 throws IOException, FHIRFormatError { 1991 Bundle.BundleEntryRequestComponent res = new Bundle.BundleEntryRequestComponent(); 1992 parseBackboneProperties(json, res); 1993 if (json.has("method")) 1994 res.setMethodElement( 1995 parseEnumeration(json.get("method").getAsString(), Bundle.HTTPVerb.NULL, new Bundle.HTTPVerbEnumFactory())); 1996 if (json.has("_method")) 1997 parseElementProperties(json.getAsJsonObject("_method"), res.getMethodElement()); 1998 if (json.has("url")) 1999 res.setUrlElement(parseUri(json.get("url").getAsString())); 2000 if (json.has("_url")) 2001 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 2002 if (json.has("ifNoneMatch")) 2003 res.setIfNoneMatchElement(parseString(json.get("ifNoneMatch").getAsString())); 2004 if (json.has("_ifNoneMatch")) 2005 parseElementProperties(json.getAsJsonObject("_ifNoneMatch"), res.getIfNoneMatchElement()); 2006 if (json.has("ifModifiedSince")) 2007 res.setIfModifiedSinceElement(parseInstant(json.get("ifModifiedSince").getAsString())); 2008 if (json.has("_ifModifiedSince")) 2009 parseElementProperties(json.getAsJsonObject("_ifModifiedSince"), res.getIfModifiedSinceElement()); 2010 if (json.has("ifMatch")) 2011 res.setIfMatchElement(parseString(json.get("ifMatch").getAsString())); 2012 if (json.has("_ifMatch")) 2013 parseElementProperties(json.getAsJsonObject("_ifMatch"), res.getIfMatchElement()); 2014 if (json.has("ifNoneExist")) 2015 res.setIfNoneExistElement(parseString(json.get("ifNoneExist").getAsString())); 2016 if (json.has("_ifNoneExist")) 2017 parseElementProperties(json.getAsJsonObject("_ifNoneExist"), res.getIfNoneExistElement()); 2018 return res; 2019 } 2020 2021 protected Bundle.BundleEntryResponseComponent parseBundleBundleEntryResponseComponent(JsonObject json, Bundle owner) 2022 throws IOException, FHIRFormatError { 2023 Bundle.BundleEntryResponseComponent res = new Bundle.BundleEntryResponseComponent(); 2024 parseBackboneProperties(json, res); 2025 if (json.has("status")) 2026 res.setStatusElement(parseString(json.get("status").getAsString())); 2027 if (json.has("_status")) 2028 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 2029 if (json.has("location")) 2030 res.setLocationElement(parseUri(json.get("location").getAsString())); 2031 if (json.has("_location")) 2032 parseElementProperties(json.getAsJsonObject("_location"), res.getLocationElement()); 2033 if (json.has("etag")) 2034 res.setEtagElement(parseString(json.get("etag").getAsString())); 2035 if (json.has("_etag")) 2036 parseElementProperties(json.getAsJsonObject("_etag"), res.getEtagElement()); 2037 if (json.has("lastModified")) 2038 res.setLastModifiedElement(parseInstant(json.get("lastModified").getAsString())); 2039 if (json.has("_lastModified")) 2040 parseElementProperties(json.getAsJsonObject("_lastModified"), res.getLastModifiedElement()); 2041 return res; 2042 } 2043 2044 protected CarePlan parseCarePlan(JsonObject json) throws IOException, FHIRFormatError { 2045 CarePlan res = new CarePlan(); 2046 parseDomainResourceProperties(json, res); 2047 if (json.has("identifier")) { 2048 JsonArray array = json.getAsJsonArray("identifier"); 2049 for (int i = 0; i < array.size(); i++) { 2050 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 2051 } 2052 } 2053 ; 2054 if (json.has("subject")) 2055 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 2056 if (json.has("status")) 2057 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), CarePlan.CarePlanStatus.NULL, 2058 new CarePlan.CarePlanStatusEnumFactory())); 2059 if (json.has("_status")) 2060 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 2061 if (json.has("context")) 2062 res.setContext(parseReference(json.getAsJsonObject("context"))); 2063 if (json.has("period")) 2064 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 2065 if (json.has("author")) { 2066 JsonArray array = json.getAsJsonArray("author"); 2067 for (int i = 0; i < array.size(); i++) { 2068 res.getAuthor().add(parseReference(array.get(i).getAsJsonObject())); 2069 } 2070 } 2071 ; 2072 if (json.has("modified")) 2073 res.setModifiedElement(parseDateTime(json.get("modified").getAsString())); 2074 if (json.has("_modified")) 2075 parseElementProperties(json.getAsJsonObject("_modified"), res.getModifiedElement()); 2076 if (json.has("category")) { 2077 JsonArray array = json.getAsJsonArray("category"); 2078 for (int i = 0; i < array.size(); i++) { 2079 res.getCategory().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 2080 } 2081 } 2082 ; 2083 if (json.has("description")) 2084 res.setDescriptionElement(parseString(json.get("description").getAsString())); 2085 if (json.has("_description")) 2086 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 2087 if (json.has("addresses")) { 2088 JsonArray array = json.getAsJsonArray("addresses"); 2089 for (int i = 0; i < array.size(); i++) { 2090 res.getAddresses().add(parseReference(array.get(i).getAsJsonObject())); 2091 } 2092 } 2093 ; 2094 if (json.has("support")) { 2095 JsonArray array = json.getAsJsonArray("support"); 2096 for (int i = 0; i < array.size(); i++) { 2097 res.getSupport().add(parseReference(array.get(i).getAsJsonObject())); 2098 } 2099 } 2100 ; 2101 if (json.has("relatedPlan")) { 2102 JsonArray array = json.getAsJsonArray("relatedPlan"); 2103 for (int i = 0; i < array.size(); i++) { 2104 res.getRelatedPlan().add(parseCarePlanCarePlanRelatedPlanComponent(array.get(i).getAsJsonObject(), res)); 2105 } 2106 } 2107 ; 2108 if (json.has("participant")) { 2109 JsonArray array = json.getAsJsonArray("participant"); 2110 for (int i = 0; i < array.size(); i++) { 2111 res.getParticipant().add(parseCarePlanCarePlanParticipantComponent(array.get(i).getAsJsonObject(), res)); 2112 } 2113 } 2114 ; 2115 if (json.has("goal")) { 2116 JsonArray array = json.getAsJsonArray("goal"); 2117 for (int i = 0; i < array.size(); i++) { 2118 res.getGoal().add(parseReference(array.get(i).getAsJsonObject())); 2119 } 2120 } 2121 ; 2122 if (json.has("activity")) { 2123 JsonArray array = json.getAsJsonArray("activity"); 2124 for (int i = 0; i < array.size(); i++) { 2125 res.getActivity().add(parseCarePlanCarePlanActivityComponent(array.get(i).getAsJsonObject(), res)); 2126 } 2127 } 2128 ; 2129 if (json.has("note")) 2130 res.setNote(parseAnnotation(json.getAsJsonObject("note"))); 2131 return res; 2132 } 2133 2134 protected CarePlan.CarePlanRelatedPlanComponent parseCarePlanCarePlanRelatedPlanComponent(JsonObject json, 2135 CarePlan owner) throws IOException, FHIRFormatError { 2136 CarePlan.CarePlanRelatedPlanComponent res = new CarePlan.CarePlanRelatedPlanComponent(); 2137 parseBackboneProperties(json, res); 2138 if (json.has("code")) 2139 res.setCodeElement(parseEnumeration(json.get("code").getAsString(), CarePlan.CarePlanRelationship.NULL, 2140 new CarePlan.CarePlanRelationshipEnumFactory())); 2141 if (json.has("_code")) 2142 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 2143 if (json.has("plan")) 2144 res.setPlan(parseReference(json.getAsJsonObject("plan"))); 2145 return res; 2146 } 2147 2148 protected CarePlan.CarePlanParticipantComponent parseCarePlanCarePlanParticipantComponent(JsonObject json, 2149 CarePlan owner) throws IOException, FHIRFormatError { 2150 CarePlan.CarePlanParticipantComponent res = new CarePlan.CarePlanParticipantComponent(); 2151 parseBackboneProperties(json, res); 2152 if (json.has("role")) 2153 res.setRole(parseCodeableConcept(json.getAsJsonObject("role"))); 2154 if (json.has("member")) 2155 res.setMember(parseReference(json.getAsJsonObject("member"))); 2156 return res; 2157 } 2158 2159 protected CarePlan.CarePlanActivityComponent parseCarePlanCarePlanActivityComponent(JsonObject json, CarePlan owner) 2160 throws IOException, FHIRFormatError { 2161 CarePlan.CarePlanActivityComponent res = new CarePlan.CarePlanActivityComponent(); 2162 parseBackboneProperties(json, res); 2163 if (json.has("actionResulting")) { 2164 JsonArray array = json.getAsJsonArray("actionResulting"); 2165 for (int i = 0; i < array.size(); i++) { 2166 res.getActionResulting().add(parseReference(array.get(i).getAsJsonObject())); 2167 } 2168 } 2169 ; 2170 if (json.has("progress")) { 2171 JsonArray array = json.getAsJsonArray("progress"); 2172 for (int i = 0; i < array.size(); i++) { 2173 res.getProgress().add(parseAnnotation(array.get(i).getAsJsonObject())); 2174 } 2175 } 2176 ; 2177 if (json.has("reference")) 2178 res.setReference(parseReference(json.getAsJsonObject("reference"))); 2179 if (json.has("detail")) 2180 res.setDetail(parseCarePlanCarePlanActivityDetailComponent(json.getAsJsonObject("detail"), owner)); 2181 return res; 2182 } 2183 2184 protected CarePlan.CarePlanActivityDetailComponent parseCarePlanCarePlanActivityDetailComponent(JsonObject json, 2185 CarePlan owner) throws IOException, FHIRFormatError { 2186 CarePlan.CarePlanActivityDetailComponent res = new CarePlan.CarePlanActivityDetailComponent(); 2187 parseBackboneProperties(json, res); 2188 if (json.has("category")) 2189 res.setCategory(parseCodeableConcept(json.getAsJsonObject("category"))); 2190 if (json.has("code")) 2191 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 2192 if (json.has("reasonCode")) { 2193 JsonArray array = json.getAsJsonArray("reasonCode"); 2194 for (int i = 0; i < array.size(); i++) { 2195 res.getReasonCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 2196 } 2197 } 2198 ; 2199 if (json.has("reasonReference")) { 2200 JsonArray array = json.getAsJsonArray("reasonReference"); 2201 for (int i = 0; i < array.size(); i++) { 2202 res.getReasonReference().add(parseReference(array.get(i).getAsJsonObject())); 2203 } 2204 } 2205 ; 2206 if (json.has("goal")) { 2207 JsonArray array = json.getAsJsonArray("goal"); 2208 for (int i = 0; i < array.size(); i++) { 2209 res.getGoal().add(parseReference(array.get(i).getAsJsonObject())); 2210 } 2211 } 2212 ; 2213 if (json.has("status")) 2214 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), CarePlan.CarePlanActivityStatus.NULL, 2215 new CarePlan.CarePlanActivityStatusEnumFactory())); 2216 if (json.has("_status")) 2217 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 2218 if (json.has("statusReason")) 2219 res.setStatusReason(parseCodeableConcept(json.getAsJsonObject("statusReason"))); 2220 if (json.has("prohibited")) 2221 res.setProhibitedElement(parseBoolean(json.get("prohibited").getAsBoolean())); 2222 if (json.has("_prohibited")) 2223 parseElementProperties(json.getAsJsonObject("_prohibited"), res.getProhibitedElement()); 2224 Type scheduled = parseType("scheduled", json); 2225 if (scheduled != null) 2226 res.setScheduled(scheduled); 2227 if (json.has("location")) 2228 res.setLocation(parseReference(json.getAsJsonObject("location"))); 2229 if (json.has("performer")) { 2230 JsonArray array = json.getAsJsonArray("performer"); 2231 for (int i = 0; i < array.size(); i++) { 2232 res.getPerformer().add(parseReference(array.get(i).getAsJsonObject())); 2233 } 2234 } 2235 ; 2236 Type product = parseType("product", json); 2237 if (product != null) 2238 res.setProduct(product); 2239 if (json.has("dailyAmount")) 2240 res.setDailyAmount(parseSimpleQuantity(json.getAsJsonObject("dailyAmount"))); 2241 if (json.has("quantity")) 2242 res.setQuantity(parseSimpleQuantity(json.getAsJsonObject("quantity"))); 2243 if (json.has("description")) 2244 res.setDescriptionElement(parseString(json.get("description").getAsString())); 2245 if (json.has("_description")) 2246 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 2247 return res; 2248 } 2249 2250 protected Claim parseClaim(JsonObject json) throws IOException, FHIRFormatError { 2251 Claim res = new Claim(); 2252 parseDomainResourceProperties(json, res); 2253 if (json.has("type")) 2254 res.setTypeElement( 2255 parseEnumeration(json.get("type").getAsString(), Claim.ClaimType.NULL, new Claim.ClaimTypeEnumFactory())); 2256 if (json.has("_type")) 2257 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 2258 if (json.has("identifier")) { 2259 JsonArray array = json.getAsJsonArray("identifier"); 2260 for (int i = 0; i < array.size(); i++) { 2261 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 2262 } 2263 } 2264 ; 2265 if (json.has("ruleset")) 2266 res.setRuleset(parseCoding(json.getAsJsonObject("ruleset"))); 2267 if (json.has("originalRuleset")) 2268 res.setOriginalRuleset(parseCoding(json.getAsJsonObject("originalRuleset"))); 2269 if (json.has("created")) 2270 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 2271 if (json.has("_created")) 2272 parseElementProperties(json.getAsJsonObject("_created"), res.getCreatedElement()); 2273 if (json.has("target")) 2274 res.setTarget(parseReference(json.getAsJsonObject("target"))); 2275 if (json.has("provider")) 2276 res.setProvider(parseReference(json.getAsJsonObject("provider"))); 2277 if (json.has("organization")) 2278 res.setOrganization(parseReference(json.getAsJsonObject("organization"))); 2279 if (json.has("use")) 2280 res.setUseElement(parseEnumeration(json.get("use").getAsString(), Claim.Use.NULL, new Claim.UseEnumFactory())); 2281 if (json.has("_use")) 2282 parseElementProperties(json.getAsJsonObject("_use"), res.getUseElement()); 2283 if (json.has("priority")) 2284 res.setPriority(parseCoding(json.getAsJsonObject("priority"))); 2285 if (json.has("fundsReserve")) 2286 res.setFundsReserve(parseCoding(json.getAsJsonObject("fundsReserve"))); 2287 if (json.has("enterer")) 2288 res.setEnterer(parseReference(json.getAsJsonObject("enterer"))); 2289 if (json.has("facility")) 2290 res.setFacility(parseReference(json.getAsJsonObject("facility"))); 2291 if (json.has("prescription")) 2292 res.setPrescription(parseReference(json.getAsJsonObject("prescription"))); 2293 if (json.has("originalPrescription")) 2294 res.setOriginalPrescription(parseReference(json.getAsJsonObject("originalPrescription"))); 2295 if (json.has("payee")) 2296 res.setPayee(parseClaimPayeeComponent(json.getAsJsonObject("payee"), res)); 2297 if (json.has("referral")) 2298 res.setReferral(parseReference(json.getAsJsonObject("referral"))); 2299 if (json.has("diagnosis")) { 2300 JsonArray array = json.getAsJsonArray("diagnosis"); 2301 for (int i = 0; i < array.size(); i++) { 2302 res.getDiagnosis().add(parseClaimDiagnosisComponent(array.get(i).getAsJsonObject(), res)); 2303 } 2304 } 2305 ; 2306 if (json.has("condition")) { 2307 JsonArray array = json.getAsJsonArray("condition"); 2308 for (int i = 0; i < array.size(); i++) { 2309 res.getCondition().add(parseCoding(array.get(i).getAsJsonObject())); 2310 } 2311 } 2312 ; 2313 if (json.has("patient")) 2314 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 2315 if (json.has("coverage")) { 2316 JsonArray array = json.getAsJsonArray("coverage"); 2317 for (int i = 0; i < array.size(); i++) { 2318 res.getCoverage().add(parseClaimCoverageComponent(array.get(i).getAsJsonObject(), res)); 2319 } 2320 } 2321 ; 2322 if (json.has("exception")) { 2323 JsonArray array = json.getAsJsonArray("exception"); 2324 for (int i = 0; i < array.size(); i++) { 2325 res.getException().add(parseCoding(array.get(i).getAsJsonObject())); 2326 } 2327 } 2328 ; 2329 if (json.has("school")) 2330 res.setSchoolElement(parseString(json.get("school").getAsString())); 2331 if (json.has("_school")) 2332 parseElementProperties(json.getAsJsonObject("_school"), res.getSchoolElement()); 2333 if (json.has("accident")) 2334 res.setAccidentElement(parseDate(json.get("accident").getAsString())); 2335 if (json.has("_accident")) 2336 parseElementProperties(json.getAsJsonObject("_accident"), res.getAccidentElement()); 2337 if (json.has("accidentType")) 2338 res.setAccidentType(parseCoding(json.getAsJsonObject("accidentType"))); 2339 if (json.has("interventionException")) { 2340 JsonArray array = json.getAsJsonArray("interventionException"); 2341 for (int i = 0; i < array.size(); i++) { 2342 res.getInterventionException().add(parseCoding(array.get(i).getAsJsonObject())); 2343 } 2344 } 2345 ; 2346 if (json.has("item")) { 2347 JsonArray array = json.getAsJsonArray("item"); 2348 for (int i = 0; i < array.size(); i++) { 2349 res.getItem().add(parseClaimItemsComponent(array.get(i).getAsJsonObject(), res)); 2350 } 2351 } 2352 ; 2353 if (json.has("additionalMaterials")) { 2354 JsonArray array = json.getAsJsonArray("additionalMaterials"); 2355 for (int i = 0; i < array.size(); i++) { 2356 res.getAdditionalMaterials().add(parseCoding(array.get(i).getAsJsonObject())); 2357 } 2358 } 2359 ; 2360 if (json.has("missingTeeth")) { 2361 JsonArray array = json.getAsJsonArray("missingTeeth"); 2362 for (int i = 0; i < array.size(); i++) { 2363 res.getMissingTeeth().add(parseClaimMissingTeethComponent(array.get(i).getAsJsonObject(), res)); 2364 } 2365 } 2366 ; 2367 return res; 2368 } 2369 2370 protected Claim.PayeeComponent parseClaimPayeeComponent(JsonObject json, Claim owner) 2371 throws IOException, FHIRFormatError { 2372 Claim.PayeeComponent res = new Claim.PayeeComponent(); 2373 parseBackboneProperties(json, res); 2374 if (json.has("type")) 2375 res.setType(parseCoding(json.getAsJsonObject("type"))); 2376 if (json.has("provider")) 2377 res.setProvider(parseReference(json.getAsJsonObject("provider"))); 2378 if (json.has("organization")) 2379 res.setOrganization(parseReference(json.getAsJsonObject("organization"))); 2380 if (json.has("person")) 2381 res.setPerson(parseReference(json.getAsJsonObject("person"))); 2382 return res; 2383 } 2384 2385 protected Claim.DiagnosisComponent parseClaimDiagnosisComponent(JsonObject json, Claim owner) 2386 throws IOException, FHIRFormatError { 2387 Claim.DiagnosisComponent res = new Claim.DiagnosisComponent(); 2388 parseBackboneProperties(json, res); 2389 if (json.has("sequence")) 2390 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 2391 if (json.has("_sequence")) 2392 parseElementProperties(json.getAsJsonObject("_sequence"), res.getSequenceElement()); 2393 if (json.has("diagnosis")) 2394 res.setDiagnosis(parseCoding(json.getAsJsonObject("diagnosis"))); 2395 return res; 2396 } 2397 2398 protected Claim.CoverageComponent parseClaimCoverageComponent(JsonObject json, Claim owner) 2399 throws IOException, FHIRFormatError { 2400 Claim.CoverageComponent res = new Claim.CoverageComponent(); 2401 parseBackboneProperties(json, res); 2402 if (json.has("sequence")) 2403 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 2404 if (json.has("_sequence")) 2405 parseElementProperties(json.getAsJsonObject("_sequence"), res.getSequenceElement()); 2406 if (json.has("focal")) 2407 res.setFocalElement(parseBoolean(json.get("focal").getAsBoolean())); 2408 if (json.has("_focal")) 2409 parseElementProperties(json.getAsJsonObject("_focal"), res.getFocalElement()); 2410 if (json.has("coverage")) 2411 res.setCoverage(parseReference(json.getAsJsonObject("coverage"))); 2412 if (json.has("businessArrangement")) 2413 res.setBusinessArrangementElement(parseString(json.get("businessArrangement").getAsString())); 2414 if (json.has("_businessArrangement")) 2415 parseElementProperties(json.getAsJsonObject("_businessArrangement"), res.getBusinessArrangementElement()); 2416 if (json.has("relationship")) 2417 res.setRelationship(parseCoding(json.getAsJsonObject("relationship"))); 2418 if (json.has("preAuthRef")) { 2419 JsonArray array = json.getAsJsonArray("preAuthRef"); 2420 for (int i = 0; i < array.size(); i++) { 2421 res.getPreAuthRef().add(parseString(array.get(i).getAsString())); 2422 } 2423 } 2424 ; 2425 if (json.has("_preAuthRef")) { 2426 JsonArray array = json.getAsJsonArray("_preAuthRef"); 2427 for (int i = 0; i < array.size(); i++) { 2428 if (i == res.getPreAuthRef().size()) 2429 res.getPreAuthRef().add(parseString(null)); 2430 if (array.get(i) instanceof JsonObject) 2431 parseElementProperties(array.get(i).getAsJsonObject(), res.getPreAuthRef().get(i)); 2432 } 2433 } 2434 ; 2435 if (json.has("claimResponse")) 2436 res.setClaimResponse(parseReference(json.getAsJsonObject("claimResponse"))); 2437 if (json.has("originalRuleset")) 2438 res.setOriginalRuleset(parseCoding(json.getAsJsonObject("originalRuleset"))); 2439 return res; 2440 } 2441 2442 protected Claim.ItemsComponent parseClaimItemsComponent(JsonObject json, Claim owner) 2443 throws IOException, FHIRFormatError { 2444 Claim.ItemsComponent res = new Claim.ItemsComponent(); 2445 parseBackboneProperties(json, res); 2446 if (json.has("sequence")) 2447 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 2448 if (json.has("_sequence")) 2449 parseElementProperties(json.getAsJsonObject("_sequence"), res.getSequenceElement()); 2450 if (json.has("type")) 2451 res.setType(parseCoding(json.getAsJsonObject("type"))); 2452 if (json.has("provider")) 2453 res.setProvider(parseReference(json.getAsJsonObject("provider"))); 2454 if (json.has("diagnosisLinkId")) { 2455 JsonArray array = json.getAsJsonArray("diagnosisLinkId"); 2456 for (int i = 0; i < array.size(); i++) { 2457 res.getDiagnosisLinkId().add(parsePositiveInt(array.get(i).getAsString())); 2458 } 2459 } 2460 ; 2461 if (json.has("_diagnosisLinkId")) { 2462 JsonArray array = json.getAsJsonArray("_diagnosisLinkId"); 2463 for (int i = 0; i < array.size(); i++) { 2464 if (i == res.getDiagnosisLinkId().size()) 2465 res.getDiagnosisLinkId().add(parsePositiveInt(null)); 2466 if (array.get(i) instanceof JsonObject) 2467 parseElementProperties(array.get(i).getAsJsonObject(), res.getDiagnosisLinkId().get(i)); 2468 } 2469 } 2470 ; 2471 if (json.has("service")) 2472 res.setService(parseCoding(json.getAsJsonObject("service"))); 2473 if (json.has("serviceDate")) 2474 res.setServiceDateElement(parseDate(json.get("serviceDate").getAsString())); 2475 if (json.has("_serviceDate")) 2476 parseElementProperties(json.getAsJsonObject("_serviceDate"), res.getServiceDateElement()); 2477 if (json.has("quantity")) 2478 res.setQuantity(parseSimpleQuantity(json.getAsJsonObject("quantity"))); 2479 if (json.has("unitPrice")) 2480 res.setUnitPrice(parseMoney(json.getAsJsonObject("unitPrice"))); 2481 if (json.has("factor")) 2482 res.setFactorElement(parseDecimal(json.get("factor").getAsBigDecimal())); 2483 if (json.has("_factor")) 2484 parseElementProperties(json.getAsJsonObject("_factor"), res.getFactorElement()); 2485 if (json.has("points")) 2486 res.setPointsElement(parseDecimal(json.get("points").getAsBigDecimal())); 2487 if (json.has("_points")) 2488 parseElementProperties(json.getAsJsonObject("_points"), res.getPointsElement()); 2489 if (json.has("net")) 2490 res.setNet(parseMoney(json.getAsJsonObject("net"))); 2491 if (json.has("udi")) 2492 res.setUdi(parseCoding(json.getAsJsonObject("udi"))); 2493 if (json.has("bodySite")) 2494 res.setBodySite(parseCoding(json.getAsJsonObject("bodySite"))); 2495 if (json.has("subSite")) { 2496 JsonArray array = json.getAsJsonArray("subSite"); 2497 for (int i = 0; i < array.size(); i++) { 2498 res.getSubSite().add(parseCoding(array.get(i).getAsJsonObject())); 2499 } 2500 } 2501 ; 2502 if (json.has("modifier")) { 2503 JsonArray array = json.getAsJsonArray("modifier"); 2504 for (int i = 0; i < array.size(); i++) { 2505 res.getModifier().add(parseCoding(array.get(i).getAsJsonObject())); 2506 } 2507 } 2508 ; 2509 if (json.has("detail")) { 2510 JsonArray array = json.getAsJsonArray("detail"); 2511 for (int i = 0; i < array.size(); i++) { 2512 res.getDetail().add(parseClaimDetailComponent(array.get(i).getAsJsonObject(), owner)); 2513 } 2514 } 2515 ; 2516 if (json.has("prosthesis")) 2517 res.setProsthesis(parseClaimProsthesisComponent(json.getAsJsonObject("prosthesis"), owner)); 2518 return res; 2519 } 2520 2521 protected Claim.DetailComponent parseClaimDetailComponent(JsonObject json, Claim owner) 2522 throws IOException, FHIRFormatError { 2523 Claim.DetailComponent res = new Claim.DetailComponent(); 2524 parseBackboneProperties(json, res); 2525 if (json.has("sequence")) 2526 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 2527 if (json.has("_sequence")) 2528 parseElementProperties(json.getAsJsonObject("_sequence"), res.getSequenceElement()); 2529 if (json.has("type")) 2530 res.setType(parseCoding(json.getAsJsonObject("type"))); 2531 if (json.has("service")) 2532 res.setService(parseCoding(json.getAsJsonObject("service"))); 2533 if (json.has("quantity")) 2534 res.setQuantity(parseSimpleQuantity(json.getAsJsonObject("quantity"))); 2535 if (json.has("unitPrice")) 2536 res.setUnitPrice(parseMoney(json.getAsJsonObject("unitPrice"))); 2537 if (json.has("factor")) 2538 res.setFactorElement(parseDecimal(json.get("factor").getAsBigDecimal())); 2539 if (json.has("_factor")) 2540 parseElementProperties(json.getAsJsonObject("_factor"), res.getFactorElement()); 2541 if (json.has("points")) 2542 res.setPointsElement(parseDecimal(json.get("points").getAsBigDecimal())); 2543 if (json.has("_points")) 2544 parseElementProperties(json.getAsJsonObject("_points"), res.getPointsElement()); 2545 if (json.has("net")) 2546 res.setNet(parseMoney(json.getAsJsonObject("net"))); 2547 if (json.has("udi")) 2548 res.setUdi(parseCoding(json.getAsJsonObject("udi"))); 2549 if (json.has("subDetail")) { 2550 JsonArray array = json.getAsJsonArray("subDetail"); 2551 for (int i = 0; i < array.size(); i++) { 2552 res.getSubDetail().add(parseClaimSubDetailComponent(array.get(i).getAsJsonObject(), owner)); 2553 } 2554 } 2555 ; 2556 return res; 2557 } 2558 2559 protected Claim.SubDetailComponent parseClaimSubDetailComponent(JsonObject json, Claim owner) 2560 throws IOException, FHIRFormatError { 2561 Claim.SubDetailComponent res = new Claim.SubDetailComponent(); 2562 parseBackboneProperties(json, res); 2563 if (json.has("sequence")) 2564 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 2565 if (json.has("_sequence")) 2566 parseElementProperties(json.getAsJsonObject("_sequence"), res.getSequenceElement()); 2567 if (json.has("type")) 2568 res.setType(parseCoding(json.getAsJsonObject("type"))); 2569 if (json.has("service")) 2570 res.setService(parseCoding(json.getAsJsonObject("service"))); 2571 if (json.has("quantity")) 2572 res.setQuantity(parseSimpleQuantity(json.getAsJsonObject("quantity"))); 2573 if (json.has("unitPrice")) 2574 res.setUnitPrice(parseMoney(json.getAsJsonObject("unitPrice"))); 2575 if (json.has("factor")) 2576 res.setFactorElement(parseDecimal(json.get("factor").getAsBigDecimal())); 2577 if (json.has("_factor")) 2578 parseElementProperties(json.getAsJsonObject("_factor"), res.getFactorElement()); 2579 if (json.has("points")) 2580 res.setPointsElement(parseDecimal(json.get("points").getAsBigDecimal())); 2581 if (json.has("_points")) 2582 parseElementProperties(json.getAsJsonObject("_points"), res.getPointsElement()); 2583 if (json.has("net")) 2584 res.setNet(parseMoney(json.getAsJsonObject("net"))); 2585 if (json.has("udi")) 2586 res.setUdi(parseCoding(json.getAsJsonObject("udi"))); 2587 return res; 2588 } 2589 2590 protected Claim.ProsthesisComponent parseClaimProsthesisComponent(JsonObject json, Claim owner) 2591 throws IOException, FHIRFormatError { 2592 Claim.ProsthesisComponent res = new Claim.ProsthesisComponent(); 2593 parseBackboneProperties(json, res); 2594 if (json.has("initial")) 2595 res.setInitialElement(parseBoolean(json.get("initial").getAsBoolean())); 2596 if (json.has("_initial")) 2597 parseElementProperties(json.getAsJsonObject("_initial"), res.getInitialElement()); 2598 if (json.has("priorDate")) 2599 res.setPriorDateElement(parseDate(json.get("priorDate").getAsString())); 2600 if (json.has("_priorDate")) 2601 parseElementProperties(json.getAsJsonObject("_priorDate"), res.getPriorDateElement()); 2602 if (json.has("priorMaterial")) 2603 res.setPriorMaterial(parseCoding(json.getAsJsonObject("priorMaterial"))); 2604 return res; 2605 } 2606 2607 protected Claim.MissingTeethComponent parseClaimMissingTeethComponent(JsonObject json, Claim owner) 2608 throws IOException, FHIRFormatError { 2609 Claim.MissingTeethComponent res = new Claim.MissingTeethComponent(); 2610 parseBackboneProperties(json, res); 2611 if (json.has("tooth")) 2612 res.setTooth(parseCoding(json.getAsJsonObject("tooth"))); 2613 if (json.has("reason")) 2614 res.setReason(parseCoding(json.getAsJsonObject("reason"))); 2615 if (json.has("extractionDate")) 2616 res.setExtractionDateElement(parseDate(json.get("extractionDate").getAsString())); 2617 if (json.has("_extractionDate")) 2618 parseElementProperties(json.getAsJsonObject("_extractionDate"), res.getExtractionDateElement()); 2619 return res; 2620 } 2621 2622 protected ClaimResponse parseClaimResponse(JsonObject json) throws IOException, FHIRFormatError { 2623 ClaimResponse res = new ClaimResponse(); 2624 parseDomainResourceProperties(json, res); 2625 if (json.has("identifier")) { 2626 JsonArray array = json.getAsJsonArray("identifier"); 2627 for (int i = 0; i < array.size(); i++) { 2628 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 2629 } 2630 } 2631 ; 2632 if (json.has("request")) 2633 res.setRequest(parseReference(json.getAsJsonObject("request"))); 2634 if (json.has("ruleset")) 2635 res.setRuleset(parseCoding(json.getAsJsonObject("ruleset"))); 2636 if (json.has("originalRuleset")) 2637 res.setOriginalRuleset(parseCoding(json.getAsJsonObject("originalRuleset"))); 2638 if (json.has("created")) 2639 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 2640 if (json.has("_created")) 2641 parseElementProperties(json.getAsJsonObject("_created"), res.getCreatedElement()); 2642 if (json.has("organization")) 2643 res.setOrganization(parseReference(json.getAsJsonObject("organization"))); 2644 if (json.has("requestProvider")) 2645 res.setRequestProvider(parseReference(json.getAsJsonObject("requestProvider"))); 2646 if (json.has("requestOrganization")) 2647 res.setRequestOrganization(parseReference(json.getAsJsonObject("requestOrganization"))); 2648 if (json.has("outcome")) 2649 res.setOutcomeElement(parseEnumeration(json.get("outcome").getAsString(), Enumerations.RemittanceOutcome.NULL, 2650 new Enumerations.RemittanceOutcomeEnumFactory())); 2651 if (json.has("_outcome")) 2652 parseElementProperties(json.getAsJsonObject("_outcome"), res.getOutcomeElement()); 2653 if (json.has("disposition")) 2654 res.setDispositionElement(parseString(json.get("disposition").getAsString())); 2655 if (json.has("_disposition")) 2656 parseElementProperties(json.getAsJsonObject("_disposition"), res.getDispositionElement()); 2657 if (json.has("payeeType")) 2658 res.setPayeeType(parseCoding(json.getAsJsonObject("payeeType"))); 2659 if (json.has("item")) { 2660 JsonArray array = json.getAsJsonArray("item"); 2661 for (int i = 0; i < array.size(); i++) { 2662 res.getItem().add(parseClaimResponseItemsComponent(array.get(i).getAsJsonObject(), res)); 2663 } 2664 } 2665 ; 2666 if (json.has("addItem")) { 2667 JsonArray array = json.getAsJsonArray("addItem"); 2668 for (int i = 0; i < array.size(); i++) { 2669 res.getAddItem().add(parseClaimResponseAddedItemComponent(array.get(i).getAsJsonObject(), res)); 2670 } 2671 } 2672 ; 2673 if (json.has("error")) { 2674 JsonArray array = json.getAsJsonArray("error"); 2675 for (int i = 0; i < array.size(); i++) { 2676 res.getError().add(parseClaimResponseErrorsComponent(array.get(i).getAsJsonObject(), res)); 2677 } 2678 } 2679 ; 2680 if (json.has("totalCost")) 2681 res.setTotalCost(parseMoney(json.getAsJsonObject("totalCost"))); 2682 if (json.has("unallocDeductable")) 2683 res.setUnallocDeductable(parseMoney(json.getAsJsonObject("unallocDeductable"))); 2684 if (json.has("totalBenefit")) 2685 res.setTotalBenefit(parseMoney(json.getAsJsonObject("totalBenefit"))); 2686 if (json.has("paymentAdjustment")) 2687 res.setPaymentAdjustment(parseMoney(json.getAsJsonObject("paymentAdjustment"))); 2688 if (json.has("paymentAdjustmentReason")) 2689 res.setPaymentAdjustmentReason(parseCoding(json.getAsJsonObject("paymentAdjustmentReason"))); 2690 if (json.has("paymentDate")) 2691 res.setPaymentDateElement(parseDate(json.get("paymentDate").getAsString())); 2692 if (json.has("_paymentDate")) 2693 parseElementProperties(json.getAsJsonObject("_paymentDate"), res.getPaymentDateElement()); 2694 if (json.has("paymentAmount")) 2695 res.setPaymentAmount(parseMoney(json.getAsJsonObject("paymentAmount"))); 2696 if (json.has("paymentRef")) 2697 res.setPaymentRef(parseIdentifier(json.getAsJsonObject("paymentRef"))); 2698 if (json.has("reserved")) 2699 res.setReserved(parseCoding(json.getAsJsonObject("reserved"))); 2700 if (json.has("form")) 2701 res.setForm(parseCoding(json.getAsJsonObject("form"))); 2702 if (json.has("note")) { 2703 JsonArray array = json.getAsJsonArray("note"); 2704 for (int i = 0; i < array.size(); i++) { 2705 res.getNote().add(parseClaimResponseNotesComponent(array.get(i).getAsJsonObject(), res)); 2706 } 2707 } 2708 ; 2709 if (json.has("coverage")) { 2710 JsonArray array = json.getAsJsonArray("coverage"); 2711 for (int i = 0; i < array.size(); i++) { 2712 res.getCoverage().add(parseClaimResponseCoverageComponent(array.get(i).getAsJsonObject(), res)); 2713 } 2714 } 2715 ; 2716 return res; 2717 } 2718 2719 protected ClaimResponse.ItemsComponent parseClaimResponseItemsComponent(JsonObject json, ClaimResponse owner) 2720 throws IOException, FHIRFormatError { 2721 ClaimResponse.ItemsComponent res = new ClaimResponse.ItemsComponent(); 2722 parseBackboneProperties(json, res); 2723 if (json.has("sequenceLinkId")) 2724 res.setSequenceLinkIdElement(parsePositiveInt(json.get("sequenceLinkId").getAsString())); 2725 if (json.has("_sequenceLinkId")) 2726 parseElementProperties(json.getAsJsonObject("_sequenceLinkId"), res.getSequenceLinkIdElement()); 2727 if (json.has("noteNumber")) { 2728 JsonArray array = json.getAsJsonArray("noteNumber"); 2729 for (int i = 0; i < array.size(); i++) { 2730 res.getNoteNumber().add(parsePositiveInt(array.get(i).getAsString())); 2731 } 2732 } 2733 ; 2734 if (json.has("_noteNumber")) { 2735 JsonArray array = json.getAsJsonArray("_noteNumber"); 2736 for (int i = 0; i < array.size(); i++) { 2737 if (i == res.getNoteNumber().size()) 2738 res.getNoteNumber().add(parsePositiveInt(null)); 2739 if (array.get(i) instanceof JsonObject) 2740 parseElementProperties(array.get(i).getAsJsonObject(), res.getNoteNumber().get(i)); 2741 } 2742 } 2743 ; 2744 if (json.has("adjudication")) { 2745 JsonArray array = json.getAsJsonArray("adjudication"); 2746 for (int i = 0; i < array.size(); i++) { 2747 res.getAdjudication().add(parseClaimResponseItemAdjudicationComponent(array.get(i).getAsJsonObject(), owner)); 2748 } 2749 } 2750 ; 2751 if (json.has("detail")) { 2752 JsonArray array = json.getAsJsonArray("detail"); 2753 for (int i = 0; i < array.size(); i++) { 2754 res.getDetail().add(parseClaimResponseItemDetailComponent(array.get(i).getAsJsonObject(), owner)); 2755 } 2756 } 2757 ; 2758 return res; 2759 } 2760 2761 protected ClaimResponse.ItemAdjudicationComponent parseClaimResponseItemAdjudicationComponent(JsonObject json, 2762 ClaimResponse owner) throws IOException, FHIRFormatError { 2763 ClaimResponse.ItemAdjudicationComponent res = new ClaimResponse.ItemAdjudicationComponent(); 2764 parseBackboneProperties(json, res); 2765 if (json.has("code")) 2766 res.setCode(parseCoding(json.getAsJsonObject("code"))); 2767 if (json.has("amount")) 2768 res.setAmount(parseMoney(json.getAsJsonObject("amount"))); 2769 if (json.has("value")) 2770 res.setValueElement(parseDecimal(json.get("value").getAsBigDecimal())); 2771 if (json.has("_value")) 2772 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 2773 return res; 2774 } 2775 2776 protected ClaimResponse.ItemDetailComponent parseClaimResponseItemDetailComponent(JsonObject json, 2777 ClaimResponse owner) throws IOException, FHIRFormatError { 2778 ClaimResponse.ItemDetailComponent res = new ClaimResponse.ItemDetailComponent(); 2779 parseBackboneProperties(json, res); 2780 if (json.has("sequenceLinkId")) 2781 res.setSequenceLinkIdElement(parsePositiveInt(json.get("sequenceLinkId").getAsString())); 2782 if (json.has("_sequenceLinkId")) 2783 parseElementProperties(json.getAsJsonObject("_sequenceLinkId"), res.getSequenceLinkIdElement()); 2784 if (json.has("adjudication")) { 2785 JsonArray array = json.getAsJsonArray("adjudication"); 2786 for (int i = 0; i < array.size(); i++) { 2787 res.getAdjudication().add(parseClaimResponseDetailAdjudicationComponent(array.get(i).getAsJsonObject(), owner)); 2788 } 2789 } 2790 ; 2791 if (json.has("subDetail")) { 2792 JsonArray array = json.getAsJsonArray("subDetail"); 2793 for (int i = 0; i < array.size(); i++) { 2794 res.getSubDetail().add(parseClaimResponseSubDetailComponent(array.get(i).getAsJsonObject(), owner)); 2795 } 2796 } 2797 ; 2798 return res; 2799 } 2800 2801 protected ClaimResponse.DetailAdjudicationComponent parseClaimResponseDetailAdjudicationComponent(JsonObject json, 2802 ClaimResponse owner) throws IOException, FHIRFormatError { 2803 ClaimResponse.DetailAdjudicationComponent res = new ClaimResponse.DetailAdjudicationComponent(); 2804 parseBackboneProperties(json, res); 2805 if (json.has("code")) 2806 res.setCode(parseCoding(json.getAsJsonObject("code"))); 2807 if (json.has("amount")) 2808 res.setAmount(parseMoney(json.getAsJsonObject("amount"))); 2809 if (json.has("value")) 2810 res.setValueElement(parseDecimal(json.get("value").getAsBigDecimal())); 2811 if (json.has("_value")) 2812 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 2813 return res; 2814 } 2815 2816 protected ClaimResponse.SubDetailComponent parseClaimResponseSubDetailComponent(JsonObject json, ClaimResponse owner) 2817 throws IOException, FHIRFormatError { 2818 ClaimResponse.SubDetailComponent res = new ClaimResponse.SubDetailComponent(); 2819 parseBackboneProperties(json, res); 2820 if (json.has("sequenceLinkId")) 2821 res.setSequenceLinkIdElement(parsePositiveInt(json.get("sequenceLinkId").getAsString())); 2822 if (json.has("_sequenceLinkId")) 2823 parseElementProperties(json.getAsJsonObject("_sequenceLinkId"), res.getSequenceLinkIdElement()); 2824 if (json.has("adjudication")) { 2825 JsonArray array = json.getAsJsonArray("adjudication"); 2826 for (int i = 0; i < array.size(); i++) { 2827 res.getAdjudication() 2828 .add(parseClaimResponseSubdetailAdjudicationComponent(array.get(i).getAsJsonObject(), owner)); 2829 } 2830 } 2831 ; 2832 return res; 2833 } 2834 2835 protected ClaimResponse.SubdetailAdjudicationComponent parseClaimResponseSubdetailAdjudicationComponent( 2836 JsonObject json, ClaimResponse owner) throws IOException, FHIRFormatError { 2837 ClaimResponse.SubdetailAdjudicationComponent res = new ClaimResponse.SubdetailAdjudicationComponent(); 2838 parseBackboneProperties(json, res); 2839 if (json.has("code")) 2840 res.setCode(parseCoding(json.getAsJsonObject("code"))); 2841 if (json.has("amount")) 2842 res.setAmount(parseMoney(json.getAsJsonObject("amount"))); 2843 if (json.has("value")) 2844 res.setValueElement(parseDecimal(json.get("value").getAsBigDecimal())); 2845 if (json.has("_value")) 2846 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 2847 return res; 2848 } 2849 2850 protected ClaimResponse.AddedItemComponent parseClaimResponseAddedItemComponent(JsonObject json, ClaimResponse owner) 2851 throws IOException, FHIRFormatError { 2852 ClaimResponse.AddedItemComponent res = new ClaimResponse.AddedItemComponent(); 2853 parseBackboneProperties(json, res); 2854 if (json.has("sequenceLinkId")) { 2855 JsonArray array = json.getAsJsonArray("sequenceLinkId"); 2856 for (int i = 0; i < array.size(); i++) { 2857 res.getSequenceLinkId().add(parsePositiveInt(array.get(i).getAsString())); 2858 } 2859 } 2860 ; 2861 if (json.has("_sequenceLinkId")) { 2862 JsonArray array = json.getAsJsonArray("_sequenceLinkId"); 2863 for (int i = 0; i < array.size(); i++) { 2864 if (i == res.getSequenceLinkId().size()) 2865 res.getSequenceLinkId().add(parsePositiveInt(null)); 2866 if (array.get(i) instanceof JsonObject) 2867 parseElementProperties(array.get(i).getAsJsonObject(), res.getSequenceLinkId().get(i)); 2868 } 2869 } 2870 ; 2871 if (json.has("service")) 2872 res.setService(parseCoding(json.getAsJsonObject("service"))); 2873 if (json.has("fee")) 2874 res.setFee(parseMoney(json.getAsJsonObject("fee"))); 2875 if (json.has("noteNumberLinkId")) { 2876 JsonArray array = json.getAsJsonArray("noteNumberLinkId"); 2877 for (int i = 0; i < array.size(); i++) { 2878 res.getNoteNumberLinkId().add(parsePositiveInt(array.get(i).getAsString())); 2879 } 2880 } 2881 ; 2882 if (json.has("_noteNumberLinkId")) { 2883 JsonArray array = json.getAsJsonArray("_noteNumberLinkId"); 2884 for (int i = 0; i < array.size(); i++) { 2885 if (i == res.getNoteNumberLinkId().size()) 2886 res.getNoteNumberLinkId().add(parsePositiveInt(null)); 2887 if (array.get(i) instanceof JsonObject) 2888 parseElementProperties(array.get(i).getAsJsonObject(), res.getNoteNumberLinkId().get(i)); 2889 } 2890 } 2891 ; 2892 if (json.has("adjudication")) { 2893 JsonArray array = json.getAsJsonArray("adjudication"); 2894 for (int i = 0; i < array.size(); i++) { 2895 res.getAdjudication() 2896 .add(parseClaimResponseAddedItemAdjudicationComponent(array.get(i).getAsJsonObject(), owner)); 2897 } 2898 } 2899 ; 2900 if (json.has("detail")) { 2901 JsonArray array = json.getAsJsonArray("detail"); 2902 for (int i = 0; i < array.size(); i++) { 2903 res.getDetail().add(parseClaimResponseAddedItemsDetailComponent(array.get(i).getAsJsonObject(), owner)); 2904 } 2905 } 2906 ; 2907 return res; 2908 } 2909 2910 protected ClaimResponse.AddedItemAdjudicationComponent parseClaimResponseAddedItemAdjudicationComponent( 2911 JsonObject json, ClaimResponse owner) throws IOException, FHIRFormatError { 2912 ClaimResponse.AddedItemAdjudicationComponent res = new ClaimResponse.AddedItemAdjudicationComponent(); 2913 parseBackboneProperties(json, res); 2914 if (json.has("code")) 2915 res.setCode(parseCoding(json.getAsJsonObject("code"))); 2916 if (json.has("amount")) 2917 res.setAmount(parseMoney(json.getAsJsonObject("amount"))); 2918 if (json.has("value")) 2919 res.setValueElement(parseDecimal(json.get("value").getAsBigDecimal())); 2920 if (json.has("_value")) 2921 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 2922 return res; 2923 } 2924 2925 protected ClaimResponse.AddedItemsDetailComponent parseClaimResponseAddedItemsDetailComponent(JsonObject json, 2926 ClaimResponse owner) throws IOException, FHIRFormatError { 2927 ClaimResponse.AddedItemsDetailComponent res = new ClaimResponse.AddedItemsDetailComponent(); 2928 parseBackboneProperties(json, res); 2929 if (json.has("service")) 2930 res.setService(parseCoding(json.getAsJsonObject("service"))); 2931 if (json.has("fee")) 2932 res.setFee(parseMoney(json.getAsJsonObject("fee"))); 2933 if (json.has("adjudication")) { 2934 JsonArray array = json.getAsJsonArray("adjudication"); 2935 for (int i = 0; i < array.size(); i++) { 2936 res.getAdjudication() 2937 .add(parseClaimResponseAddedItemDetailAdjudicationComponent(array.get(i).getAsJsonObject(), owner)); 2938 } 2939 } 2940 ; 2941 return res; 2942 } 2943 2944 protected ClaimResponse.AddedItemDetailAdjudicationComponent parseClaimResponseAddedItemDetailAdjudicationComponent( 2945 JsonObject json, ClaimResponse owner) throws IOException, FHIRFormatError { 2946 ClaimResponse.AddedItemDetailAdjudicationComponent res = new ClaimResponse.AddedItemDetailAdjudicationComponent(); 2947 parseBackboneProperties(json, res); 2948 if (json.has("code")) 2949 res.setCode(parseCoding(json.getAsJsonObject("code"))); 2950 if (json.has("amount")) 2951 res.setAmount(parseMoney(json.getAsJsonObject("amount"))); 2952 if (json.has("value")) 2953 res.setValueElement(parseDecimal(json.get("value").getAsBigDecimal())); 2954 if (json.has("_value")) 2955 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 2956 return res; 2957 } 2958 2959 protected ClaimResponse.ErrorsComponent parseClaimResponseErrorsComponent(JsonObject json, ClaimResponse owner) 2960 throws IOException, FHIRFormatError { 2961 ClaimResponse.ErrorsComponent res = new ClaimResponse.ErrorsComponent(); 2962 parseBackboneProperties(json, res); 2963 if (json.has("sequenceLinkId")) 2964 res.setSequenceLinkIdElement(parsePositiveInt(json.get("sequenceLinkId").getAsString())); 2965 if (json.has("_sequenceLinkId")) 2966 parseElementProperties(json.getAsJsonObject("_sequenceLinkId"), res.getSequenceLinkIdElement()); 2967 if (json.has("detailSequenceLinkId")) 2968 res.setDetailSequenceLinkIdElement(parsePositiveInt(json.get("detailSequenceLinkId").getAsString())); 2969 if (json.has("_detailSequenceLinkId")) 2970 parseElementProperties(json.getAsJsonObject("_detailSequenceLinkId"), res.getDetailSequenceLinkIdElement()); 2971 if (json.has("subdetailSequenceLinkId")) 2972 res.setSubdetailSequenceLinkIdElement(parsePositiveInt(json.get("subdetailSequenceLinkId").getAsString())); 2973 if (json.has("_subdetailSequenceLinkId")) 2974 parseElementProperties(json.getAsJsonObject("_subdetailSequenceLinkId"), res.getSubdetailSequenceLinkIdElement()); 2975 if (json.has("code")) 2976 res.setCode(parseCoding(json.getAsJsonObject("code"))); 2977 return res; 2978 } 2979 2980 protected ClaimResponse.NotesComponent parseClaimResponseNotesComponent(JsonObject json, ClaimResponse owner) 2981 throws IOException, FHIRFormatError { 2982 ClaimResponse.NotesComponent res = new ClaimResponse.NotesComponent(); 2983 parseBackboneProperties(json, res); 2984 if (json.has("number")) 2985 res.setNumberElement(parsePositiveInt(json.get("number").getAsString())); 2986 if (json.has("_number")) 2987 parseElementProperties(json.getAsJsonObject("_number"), res.getNumberElement()); 2988 if (json.has("type")) 2989 res.setType(parseCoding(json.getAsJsonObject("type"))); 2990 if (json.has("text")) 2991 res.setTextElement(parseString(json.get("text").getAsString())); 2992 if (json.has("_text")) 2993 parseElementProperties(json.getAsJsonObject("_text"), res.getTextElement()); 2994 return res; 2995 } 2996 2997 protected ClaimResponse.CoverageComponent parseClaimResponseCoverageComponent(JsonObject json, ClaimResponse owner) 2998 throws IOException, FHIRFormatError { 2999 ClaimResponse.CoverageComponent res = new ClaimResponse.CoverageComponent(); 3000 parseBackboneProperties(json, res); 3001 if (json.has("sequence")) 3002 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 3003 if (json.has("_sequence")) 3004 parseElementProperties(json.getAsJsonObject("_sequence"), res.getSequenceElement()); 3005 if (json.has("focal")) 3006 res.setFocalElement(parseBoolean(json.get("focal").getAsBoolean())); 3007 if (json.has("_focal")) 3008 parseElementProperties(json.getAsJsonObject("_focal"), res.getFocalElement()); 3009 if (json.has("coverage")) 3010 res.setCoverage(parseReference(json.getAsJsonObject("coverage"))); 3011 if (json.has("businessArrangement")) 3012 res.setBusinessArrangementElement(parseString(json.get("businessArrangement").getAsString())); 3013 if (json.has("_businessArrangement")) 3014 parseElementProperties(json.getAsJsonObject("_businessArrangement"), res.getBusinessArrangementElement()); 3015 if (json.has("relationship")) 3016 res.setRelationship(parseCoding(json.getAsJsonObject("relationship"))); 3017 if (json.has("preAuthRef")) { 3018 JsonArray array = json.getAsJsonArray("preAuthRef"); 3019 for (int i = 0; i < array.size(); i++) { 3020 res.getPreAuthRef().add(parseString(array.get(i).getAsString())); 3021 } 3022 } 3023 ; 3024 if (json.has("_preAuthRef")) { 3025 JsonArray array = json.getAsJsonArray("_preAuthRef"); 3026 for (int i = 0; i < array.size(); i++) { 3027 if (i == res.getPreAuthRef().size()) 3028 res.getPreAuthRef().add(parseString(null)); 3029 if (array.get(i) instanceof JsonObject) 3030 parseElementProperties(array.get(i).getAsJsonObject(), res.getPreAuthRef().get(i)); 3031 } 3032 } 3033 ; 3034 if (json.has("claimResponse")) 3035 res.setClaimResponse(parseReference(json.getAsJsonObject("claimResponse"))); 3036 if (json.has("originalRuleset")) 3037 res.setOriginalRuleset(parseCoding(json.getAsJsonObject("originalRuleset"))); 3038 return res; 3039 } 3040 3041 protected ClinicalImpression parseClinicalImpression(JsonObject json) throws IOException, FHIRFormatError { 3042 ClinicalImpression res = new ClinicalImpression(); 3043 parseDomainResourceProperties(json, res); 3044 if (json.has("patient")) 3045 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 3046 if (json.has("assessor")) 3047 res.setAssessor(parseReference(json.getAsJsonObject("assessor"))); 3048 if (json.has("status")) 3049 res.setStatusElement( 3050 parseEnumeration(json.get("status").getAsString(), ClinicalImpression.ClinicalImpressionStatus.NULL, 3051 new ClinicalImpression.ClinicalImpressionStatusEnumFactory())); 3052 if (json.has("_status")) 3053 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 3054 if (json.has("date")) 3055 res.setDateElement(parseDateTime(json.get("date").getAsString())); 3056 if (json.has("_date")) 3057 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 3058 if (json.has("description")) 3059 res.setDescriptionElement(parseString(json.get("description").getAsString())); 3060 if (json.has("_description")) 3061 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 3062 if (json.has("previous")) 3063 res.setPrevious(parseReference(json.getAsJsonObject("previous"))); 3064 if (json.has("problem")) { 3065 JsonArray array = json.getAsJsonArray("problem"); 3066 for (int i = 0; i < array.size(); i++) { 3067 res.getProblem().add(parseReference(array.get(i).getAsJsonObject())); 3068 } 3069 } 3070 ; 3071 Type trigger = parseType("trigger", json); 3072 if (trigger != null) 3073 res.setTrigger(trigger); 3074 if (json.has("investigations")) { 3075 JsonArray array = json.getAsJsonArray("investigations"); 3076 for (int i = 0; i < array.size(); i++) { 3077 res.getInvestigations() 3078 .add(parseClinicalImpressionClinicalImpressionInvestigationsComponent(array.get(i).getAsJsonObject(), res)); 3079 } 3080 } 3081 ; 3082 if (json.has("protocol")) 3083 res.setProtocolElement(parseUri(json.get("protocol").getAsString())); 3084 if (json.has("_protocol")) 3085 parseElementProperties(json.getAsJsonObject("_protocol"), res.getProtocolElement()); 3086 if (json.has("summary")) 3087 res.setSummaryElement(parseString(json.get("summary").getAsString())); 3088 if (json.has("_summary")) 3089 parseElementProperties(json.getAsJsonObject("_summary"), res.getSummaryElement()); 3090 if (json.has("finding")) { 3091 JsonArray array = json.getAsJsonArray("finding"); 3092 for (int i = 0; i < array.size(); i++) { 3093 res.getFinding() 3094 .add(parseClinicalImpressionClinicalImpressionFindingComponent(array.get(i).getAsJsonObject(), res)); 3095 } 3096 } 3097 ; 3098 if (json.has("resolved")) { 3099 JsonArray array = json.getAsJsonArray("resolved"); 3100 for (int i = 0; i < array.size(); i++) { 3101 res.getResolved().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 3102 } 3103 } 3104 ; 3105 if (json.has("ruledOut")) { 3106 JsonArray array = json.getAsJsonArray("ruledOut"); 3107 for (int i = 0; i < array.size(); i++) { 3108 res.getRuledOut() 3109 .add(parseClinicalImpressionClinicalImpressionRuledOutComponent(array.get(i).getAsJsonObject(), res)); 3110 } 3111 } 3112 ; 3113 if (json.has("prognosis")) 3114 res.setPrognosisElement(parseString(json.get("prognosis").getAsString())); 3115 if (json.has("_prognosis")) 3116 parseElementProperties(json.getAsJsonObject("_prognosis"), res.getPrognosisElement()); 3117 if (json.has("plan")) { 3118 JsonArray array = json.getAsJsonArray("plan"); 3119 for (int i = 0; i < array.size(); i++) { 3120 res.getPlan().add(parseReference(array.get(i).getAsJsonObject())); 3121 } 3122 } 3123 ; 3124 if (json.has("action")) { 3125 JsonArray array = json.getAsJsonArray("action"); 3126 for (int i = 0; i < array.size(); i++) { 3127 res.getAction().add(parseReference(array.get(i).getAsJsonObject())); 3128 } 3129 } 3130 ; 3131 return res; 3132 } 3133 3134 protected ClinicalImpression.ClinicalImpressionInvestigationsComponent parseClinicalImpressionClinicalImpressionInvestigationsComponent( 3135 JsonObject json, ClinicalImpression owner) throws IOException, FHIRFormatError { 3136 ClinicalImpression.ClinicalImpressionInvestigationsComponent res = new ClinicalImpression.ClinicalImpressionInvestigationsComponent(); 3137 parseBackboneProperties(json, res); 3138 if (json.has("code")) 3139 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 3140 if (json.has("item")) { 3141 JsonArray array = json.getAsJsonArray("item"); 3142 for (int i = 0; i < array.size(); i++) { 3143 res.getItem().add(parseReference(array.get(i).getAsJsonObject())); 3144 } 3145 } 3146 ; 3147 return res; 3148 } 3149 3150 protected ClinicalImpression.ClinicalImpressionFindingComponent parseClinicalImpressionClinicalImpressionFindingComponent( 3151 JsonObject json, ClinicalImpression owner) throws IOException, FHIRFormatError { 3152 ClinicalImpression.ClinicalImpressionFindingComponent res = new ClinicalImpression.ClinicalImpressionFindingComponent(); 3153 parseBackboneProperties(json, res); 3154 if (json.has("item")) 3155 res.setItem(parseCodeableConcept(json.getAsJsonObject("item"))); 3156 if (json.has("cause")) 3157 res.setCauseElement(parseString(json.get("cause").getAsString())); 3158 if (json.has("_cause")) 3159 parseElementProperties(json.getAsJsonObject("_cause"), res.getCauseElement()); 3160 return res; 3161 } 3162 3163 protected ClinicalImpression.ClinicalImpressionRuledOutComponent parseClinicalImpressionClinicalImpressionRuledOutComponent( 3164 JsonObject json, ClinicalImpression owner) throws IOException, FHIRFormatError { 3165 ClinicalImpression.ClinicalImpressionRuledOutComponent res = new ClinicalImpression.ClinicalImpressionRuledOutComponent(); 3166 parseBackboneProperties(json, res); 3167 if (json.has("item")) 3168 res.setItem(parseCodeableConcept(json.getAsJsonObject("item"))); 3169 if (json.has("reason")) 3170 res.setReasonElement(parseString(json.get("reason").getAsString())); 3171 if (json.has("_reason")) 3172 parseElementProperties(json.getAsJsonObject("_reason"), res.getReasonElement()); 3173 return res; 3174 } 3175 3176 protected Communication parseCommunication(JsonObject json) throws IOException, FHIRFormatError { 3177 Communication res = new Communication(); 3178 parseDomainResourceProperties(json, res); 3179 if (json.has("identifier")) { 3180 JsonArray array = json.getAsJsonArray("identifier"); 3181 for (int i = 0; i < array.size(); i++) { 3182 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 3183 } 3184 } 3185 ; 3186 if (json.has("category")) 3187 res.setCategory(parseCodeableConcept(json.getAsJsonObject("category"))); 3188 if (json.has("sender")) 3189 res.setSender(parseReference(json.getAsJsonObject("sender"))); 3190 if (json.has("recipient")) { 3191 JsonArray array = json.getAsJsonArray("recipient"); 3192 for (int i = 0; i < array.size(); i++) { 3193 res.getRecipient().add(parseReference(array.get(i).getAsJsonObject())); 3194 } 3195 } 3196 ; 3197 if (json.has("payload")) { 3198 JsonArray array = json.getAsJsonArray("payload"); 3199 for (int i = 0; i < array.size(); i++) { 3200 res.getPayload().add(parseCommunicationCommunicationPayloadComponent(array.get(i).getAsJsonObject(), res)); 3201 } 3202 } 3203 ; 3204 if (json.has("medium")) { 3205 JsonArray array = json.getAsJsonArray("medium"); 3206 for (int i = 0; i < array.size(); i++) { 3207 res.getMedium().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 3208 } 3209 } 3210 ; 3211 if (json.has("status")) 3212 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Communication.CommunicationStatus.NULL, 3213 new Communication.CommunicationStatusEnumFactory())); 3214 if (json.has("_status")) 3215 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 3216 if (json.has("encounter")) 3217 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 3218 if (json.has("sent")) 3219 res.setSentElement(parseDateTime(json.get("sent").getAsString())); 3220 if (json.has("_sent")) 3221 parseElementProperties(json.getAsJsonObject("_sent"), res.getSentElement()); 3222 if (json.has("received")) 3223 res.setReceivedElement(parseDateTime(json.get("received").getAsString())); 3224 if (json.has("_received")) 3225 parseElementProperties(json.getAsJsonObject("_received"), res.getReceivedElement()); 3226 if (json.has("reason")) { 3227 JsonArray array = json.getAsJsonArray("reason"); 3228 for (int i = 0; i < array.size(); i++) { 3229 res.getReason().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 3230 } 3231 } 3232 ; 3233 if (json.has("subject")) 3234 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 3235 if (json.has("requestDetail")) 3236 res.setRequestDetail(parseReference(json.getAsJsonObject("requestDetail"))); 3237 return res; 3238 } 3239 3240 protected Communication.CommunicationPayloadComponent parseCommunicationCommunicationPayloadComponent(JsonObject json, 3241 Communication owner) throws IOException, FHIRFormatError { 3242 Communication.CommunicationPayloadComponent res = new Communication.CommunicationPayloadComponent(); 3243 parseBackboneProperties(json, res); 3244 Type content = parseType("content", json); 3245 if (content != null) 3246 res.setContent(content); 3247 return res; 3248 } 3249 3250 protected CommunicationRequest parseCommunicationRequest(JsonObject json) throws IOException, FHIRFormatError { 3251 CommunicationRequest res = new CommunicationRequest(); 3252 parseDomainResourceProperties(json, res); 3253 if (json.has("identifier")) { 3254 JsonArray array = json.getAsJsonArray("identifier"); 3255 for (int i = 0; i < array.size(); i++) { 3256 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 3257 } 3258 } 3259 ; 3260 if (json.has("category")) 3261 res.setCategory(parseCodeableConcept(json.getAsJsonObject("category"))); 3262 if (json.has("sender")) 3263 res.setSender(parseReference(json.getAsJsonObject("sender"))); 3264 if (json.has("recipient")) { 3265 JsonArray array = json.getAsJsonArray("recipient"); 3266 for (int i = 0; i < array.size(); i++) { 3267 res.getRecipient().add(parseReference(array.get(i).getAsJsonObject())); 3268 } 3269 } 3270 ; 3271 if (json.has("payload")) { 3272 JsonArray array = json.getAsJsonArray("payload"); 3273 for (int i = 0; i < array.size(); i++) { 3274 res.getPayload() 3275 .add(parseCommunicationRequestCommunicationRequestPayloadComponent(array.get(i).getAsJsonObject(), res)); 3276 } 3277 } 3278 ; 3279 if (json.has("medium")) { 3280 JsonArray array = json.getAsJsonArray("medium"); 3281 for (int i = 0; i < array.size(); i++) { 3282 res.getMedium().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 3283 } 3284 } 3285 ; 3286 if (json.has("requester")) 3287 res.setRequester(parseReference(json.getAsJsonObject("requester"))); 3288 if (json.has("status")) 3289 res.setStatusElement( 3290 parseEnumeration(json.get("status").getAsString(), CommunicationRequest.CommunicationRequestStatus.NULL, 3291 new CommunicationRequest.CommunicationRequestStatusEnumFactory())); 3292 if (json.has("_status")) 3293 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 3294 if (json.has("encounter")) 3295 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 3296 Type scheduled = parseType("scheduled", json); 3297 if (scheduled != null) 3298 res.setScheduled(scheduled); 3299 if (json.has("reason")) { 3300 JsonArray array = json.getAsJsonArray("reason"); 3301 for (int i = 0; i < array.size(); i++) { 3302 res.getReason().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 3303 } 3304 } 3305 ; 3306 if (json.has("requestedOn")) 3307 res.setRequestedOnElement(parseDateTime(json.get("requestedOn").getAsString())); 3308 if (json.has("_requestedOn")) 3309 parseElementProperties(json.getAsJsonObject("_requestedOn"), res.getRequestedOnElement()); 3310 if (json.has("subject")) 3311 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 3312 if (json.has("priority")) 3313 res.setPriority(parseCodeableConcept(json.getAsJsonObject("priority"))); 3314 return res; 3315 } 3316 3317 protected CommunicationRequest.CommunicationRequestPayloadComponent parseCommunicationRequestCommunicationRequestPayloadComponent( 3318 JsonObject json, CommunicationRequest owner) throws IOException, FHIRFormatError { 3319 CommunicationRequest.CommunicationRequestPayloadComponent res = new CommunicationRequest.CommunicationRequestPayloadComponent(); 3320 parseBackboneProperties(json, res); 3321 Type content = parseType("content", json); 3322 if (content != null) 3323 res.setContent(content); 3324 return res; 3325 } 3326 3327 protected Composition parseComposition(JsonObject json) throws IOException, FHIRFormatError { 3328 Composition res = new Composition(); 3329 parseDomainResourceProperties(json, res); 3330 if (json.has("identifier")) 3331 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 3332 if (json.has("date")) 3333 res.setDateElement(parseDateTime(json.get("date").getAsString())); 3334 if (json.has("_date")) 3335 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 3336 if (json.has("type")) 3337 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 3338 if (json.has("class")) 3339 res.setClass_(parseCodeableConcept(json.getAsJsonObject("class"))); 3340 if (json.has("title")) 3341 res.setTitleElement(parseString(json.get("title").getAsString())); 3342 if (json.has("_title")) 3343 parseElementProperties(json.getAsJsonObject("_title"), res.getTitleElement()); 3344 if (json.has("status")) 3345 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Composition.CompositionStatus.NULL, 3346 new Composition.CompositionStatusEnumFactory())); 3347 if (json.has("_status")) 3348 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 3349 if (json.has("confidentiality")) 3350 res.setConfidentialityElement(parseCode(json.get("confidentiality").getAsString())); 3351 if (json.has("_confidentiality")) 3352 parseElementProperties(json.getAsJsonObject("_confidentiality"), res.getConfidentialityElement()); 3353 if (json.has("subject")) 3354 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 3355 if (json.has("author")) { 3356 JsonArray array = json.getAsJsonArray("author"); 3357 for (int i = 0; i < array.size(); i++) { 3358 res.getAuthor().add(parseReference(array.get(i).getAsJsonObject())); 3359 } 3360 } 3361 ; 3362 if (json.has("attester")) { 3363 JsonArray array = json.getAsJsonArray("attester"); 3364 for (int i = 0; i < array.size(); i++) { 3365 res.getAttester().add(parseCompositionCompositionAttesterComponent(array.get(i).getAsJsonObject(), res)); 3366 } 3367 } 3368 ; 3369 if (json.has("custodian")) 3370 res.setCustodian(parseReference(json.getAsJsonObject("custodian"))); 3371 if (json.has("event")) { 3372 JsonArray array = json.getAsJsonArray("event"); 3373 for (int i = 0; i < array.size(); i++) { 3374 res.getEvent().add(parseCompositionCompositionEventComponent(array.get(i).getAsJsonObject(), res)); 3375 } 3376 } 3377 ; 3378 if (json.has("encounter")) 3379 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 3380 if (json.has("section")) { 3381 JsonArray array = json.getAsJsonArray("section"); 3382 for (int i = 0; i < array.size(); i++) { 3383 res.getSection().add(parseCompositionSectionComponent(array.get(i).getAsJsonObject(), res)); 3384 } 3385 } 3386 ; 3387 return res; 3388 } 3389 3390 protected Composition.CompositionAttesterComponent parseCompositionCompositionAttesterComponent(JsonObject json, 3391 Composition owner) throws IOException, FHIRFormatError { 3392 Composition.CompositionAttesterComponent res = new Composition.CompositionAttesterComponent(); 3393 parseBackboneProperties(json, res); 3394 if (json.has("mode")) { 3395 JsonArray array = json.getAsJsonArray("mode"); 3396 for (int i = 0; i < array.size(); i++) { 3397 res.getMode().add(parseEnumeration(array.get(i).getAsString(), Composition.CompositionAttestationMode.NULL, 3398 new Composition.CompositionAttestationModeEnumFactory())); 3399 } 3400 } 3401 ; 3402 if (json.has("_mode")) { 3403 JsonArray array = json.getAsJsonArray("_mode"); 3404 for (int i = 0; i < array.size(); i++) { 3405 if (i == res.getMode().size()) 3406 res.getMode().add(parseEnumeration(null, Composition.CompositionAttestationMode.NULL, 3407 new Composition.CompositionAttestationModeEnumFactory())); 3408 if (array.get(i) instanceof JsonObject) 3409 parseElementProperties(array.get(i).getAsJsonObject(), res.getMode().get(i)); 3410 } 3411 } 3412 ; 3413 if (json.has("time")) 3414 res.setTimeElement(parseDateTime(json.get("time").getAsString())); 3415 if (json.has("_time")) 3416 parseElementProperties(json.getAsJsonObject("_time"), res.getTimeElement()); 3417 if (json.has("party")) 3418 res.setParty(parseReference(json.getAsJsonObject("party"))); 3419 return res; 3420 } 3421 3422 protected Composition.CompositionEventComponent parseCompositionCompositionEventComponent(JsonObject json, 3423 Composition owner) throws IOException, FHIRFormatError { 3424 Composition.CompositionEventComponent res = new Composition.CompositionEventComponent(); 3425 parseBackboneProperties(json, res); 3426 if (json.has("code")) { 3427 JsonArray array = json.getAsJsonArray("code"); 3428 for (int i = 0; i < array.size(); i++) { 3429 res.getCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 3430 } 3431 } 3432 ; 3433 if (json.has("period")) 3434 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 3435 if (json.has("detail")) { 3436 JsonArray array = json.getAsJsonArray("detail"); 3437 for (int i = 0; i < array.size(); i++) { 3438 res.getDetail().add(parseReference(array.get(i).getAsJsonObject())); 3439 } 3440 } 3441 ; 3442 return res; 3443 } 3444 3445 protected Composition.SectionComponent parseCompositionSectionComponent(JsonObject json, Composition owner) 3446 throws IOException, FHIRFormatError { 3447 Composition.SectionComponent res = new Composition.SectionComponent(); 3448 parseBackboneProperties(json, res); 3449 if (json.has("title")) 3450 res.setTitleElement(parseString(json.get("title").getAsString())); 3451 if (json.has("_title")) 3452 parseElementProperties(json.getAsJsonObject("_title"), res.getTitleElement()); 3453 if (json.has("code")) 3454 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 3455 if (json.has("text")) 3456 res.setText(parseNarrative(json.getAsJsonObject("text"))); 3457 if (json.has("mode")) 3458 res.setModeElement(parseCode(json.get("mode").getAsString())); 3459 if (json.has("_mode")) 3460 parseElementProperties(json.getAsJsonObject("_mode"), res.getModeElement()); 3461 if (json.has("orderedBy")) 3462 res.setOrderedBy(parseCodeableConcept(json.getAsJsonObject("orderedBy"))); 3463 if (json.has("entry")) { 3464 JsonArray array = json.getAsJsonArray("entry"); 3465 for (int i = 0; i < array.size(); i++) { 3466 res.getEntry().add(parseReference(array.get(i).getAsJsonObject())); 3467 } 3468 } 3469 ; 3470 if (json.has("emptyReason")) 3471 res.setEmptyReason(parseCodeableConcept(json.getAsJsonObject("emptyReason"))); 3472 if (json.has("section")) { 3473 JsonArray array = json.getAsJsonArray("section"); 3474 for (int i = 0; i < array.size(); i++) { 3475 res.getSection().add(parseCompositionSectionComponent(array.get(i).getAsJsonObject(), owner)); 3476 } 3477 } 3478 ; 3479 return res; 3480 } 3481 3482 protected ConceptMap parseConceptMap(JsonObject json) throws IOException, FHIRFormatError { 3483 ConceptMap res = new ConceptMap(); 3484 parseDomainResourceProperties(json, res); 3485 if (json.has("url")) 3486 res.setUrlElement(parseUri(json.get("url").getAsString())); 3487 if (json.has("_url")) 3488 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 3489 if (json.has("identifier")) 3490 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 3491 if (json.has("version")) 3492 res.setVersionElement(parseString(json.get("version").getAsString())); 3493 if (json.has("_version")) 3494 parseElementProperties(json.getAsJsonObject("_version"), res.getVersionElement()); 3495 if (json.has("name")) 3496 res.setNameElement(parseString(json.get("name").getAsString())); 3497 if (json.has("_name")) 3498 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 3499 if (json.has("status")) 3500 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 3501 Enumerations.ConformanceResourceStatus.NULL, new Enumerations.ConformanceResourceStatusEnumFactory())); 3502 if (json.has("_status")) 3503 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 3504 if (json.has("experimental")) 3505 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 3506 if (json.has("_experimental")) 3507 parseElementProperties(json.getAsJsonObject("_experimental"), res.getExperimentalElement()); 3508 if (json.has("publisher")) 3509 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 3510 if (json.has("_publisher")) 3511 parseElementProperties(json.getAsJsonObject("_publisher"), res.getPublisherElement()); 3512 if (json.has("contact")) { 3513 JsonArray array = json.getAsJsonArray("contact"); 3514 for (int i = 0; i < array.size(); i++) { 3515 res.getContact().add(parseConceptMapConceptMapContactComponent(array.get(i).getAsJsonObject(), res)); 3516 } 3517 } 3518 ; 3519 if (json.has("date")) 3520 res.setDateElement(parseDateTime(json.get("date").getAsString())); 3521 if (json.has("_date")) 3522 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 3523 if (json.has("description")) 3524 res.setDescriptionElement(parseString(json.get("description").getAsString())); 3525 if (json.has("_description")) 3526 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 3527 if (json.has("useContext")) { 3528 JsonArray array = json.getAsJsonArray("useContext"); 3529 for (int i = 0; i < array.size(); i++) { 3530 res.getUseContext().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 3531 } 3532 } 3533 ; 3534 if (json.has("requirements")) 3535 res.setRequirementsElement(parseString(json.get("requirements").getAsString())); 3536 if (json.has("_requirements")) 3537 parseElementProperties(json.getAsJsonObject("_requirements"), res.getRequirementsElement()); 3538 if (json.has("copyright")) 3539 res.setCopyrightElement(parseString(json.get("copyright").getAsString())); 3540 if (json.has("_copyright")) 3541 parseElementProperties(json.getAsJsonObject("_copyright"), res.getCopyrightElement()); 3542 Type source = parseType("source", json); 3543 if (source != null) 3544 res.setSource(source); 3545 Type target = parseType("target", json); 3546 if (target != null) 3547 res.setTarget(target); 3548 if (json.has("element")) { 3549 JsonArray array = json.getAsJsonArray("element"); 3550 for (int i = 0; i < array.size(); i++) { 3551 res.getElement().add(parseConceptMapSourceElementComponent(array.get(i).getAsJsonObject(), res)); 3552 } 3553 } 3554 ; 3555 return res; 3556 } 3557 3558 protected ConceptMap.ConceptMapContactComponent parseConceptMapConceptMapContactComponent(JsonObject json, 3559 ConceptMap owner) throws IOException, FHIRFormatError { 3560 ConceptMap.ConceptMapContactComponent res = new ConceptMap.ConceptMapContactComponent(); 3561 parseBackboneProperties(json, res); 3562 if (json.has("name")) 3563 res.setNameElement(parseString(json.get("name").getAsString())); 3564 if (json.has("_name")) 3565 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 3566 if (json.has("telecom")) { 3567 JsonArray array = json.getAsJsonArray("telecom"); 3568 for (int i = 0; i < array.size(); i++) { 3569 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 3570 } 3571 } 3572 ; 3573 return res; 3574 } 3575 3576 protected ConceptMap.SourceElementComponent parseConceptMapSourceElementComponent(JsonObject json, ConceptMap owner) 3577 throws IOException, FHIRFormatError { 3578 ConceptMap.SourceElementComponent res = new ConceptMap.SourceElementComponent(); 3579 parseBackboneProperties(json, res); 3580 if (json.has("codeSystem")) 3581 res.setCodeSystemElement(parseUri(json.get("codeSystem").getAsString())); 3582 if (json.has("_codeSystem")) 3583 parseElementProperties(json.getAsJsonObject("_codeSystem"), res.getCodeSystemElement()); 3584 if (json.has("code")) 3585 res.setCodeElement(parseCode(json.get("code").getAsString())); 3586 if (json.has("_code")) 3587 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 3588 if (json.has("target")) { 3589 JsonArray array = json.getAsJsonArray("target"); 3590 for (int i = 0; i < array.size(); i++) { 3591 res.getTarget().add(parseConceptMapTargetElementComponent(array.get(i).getAsJsonObject(), owner)); 3592 } 3593 } 3594 ; 3595 return res; 3596 } 3597 3598 protected ConceptMap.TargetElementComponent parseConceptMapTargetElementComponent(JsonObject json, ConceptMap owner) 3599 throws IOException, FHIRFormatError { 3600 ConceptMap.TargetElementComponent res = new ConceptMap.TargetElementComponent(); 3601 parseBackboneProperties(json, res); 3602 if (json.has("codeSystem")) 3603 res.setCodeSystemElement(parseUri(json.get("codeSystem").getAsString())); 3604 if (json.has("_codeSystem")) 3605 parseElementProperties(json.getAsJsonObject("_codeSystem"), res.getCodeSystemElement()); 3606 if (json.has("code")) 3607 res.setCodeElement(parseCode(json.get("code").getAsString())); 3608 if (json.has("_code")) 3609 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 3610 if (json.has("equivalence")) 3611 res.setEquivalenceElement(parseEnumeration(json.get("equivalence").getAsString(), 3612 Enumerations.ConceptMapEquivalence.NULL, new Enumerations.ConceptMapEquivalenceEnumFactory())); 3613 if (json.has("_equivalence")) 3614 parseElementProperties(json.getAsJsonObject("_equivalence"), res.getEquivalenceElement()); 3615 if (json.has("comments")) 3616 res.setCommentsElement(parseString(json.get("comments").getAsString())); 3617 if (json.has("_comments")) 3618 parseElementProperties(json.getAsJsonObject("_comments"), res.getCommentsElement()); 3619 if (json.has("dependsOn")) { 3620 JsonArray array = json.getAsJsonArray("dependsOn"); 3621 for (int i = 0; i < array.size(); i++) { 3622 res.getDependsOn().add(parseConceptMapOtherElementComponent(array.get(i).getAsJsonObject(), owner)); 3623 } 3624 } 3625 ; 3626 if (json.has("product")) { 3627 JsonArray array = json.getAsJsonArray("product"); 3628 for (int i = 0; i < array.size(); i++) { 3629 res.getProduct().add(parseConceptMapOtherElementComponent(array.get(i).getAsJsonObject(), owner)); 3630 } 3631 } 3632 ; 3633 return res; 3634 } 3635 3636 protected ConceptMap.OtherElementComponent parseConceptMapOtherElementComponent(JsonObject json, ConceptMap owner) 3637 throws IOException, FHIRFormatError { 3638 ConceptMap.OtherElementComponent res = new ConceptMap.OtherElementComponent(); 3639 parseBackboneProperties(json, res); 3640 if (json.has("element")) 3641 res.setElementElement(parseUri(json.get("element").getAsString())); 3642 if (json.has("_element")) 3643 parseElementProperties(json.getAsJsonObject("_element"), res.getElementElement()); 3644 if (json.has("codeSystem")) 3645 res.setCodeSystemElement(parseUri(json.get("codeSystem").getAsString())); 3646 if (json.has("_codeSystem")) 3647 parseElementProperties(json.getAsJsonObject("_codeSystem"), res.getCodeSystemElement()); 3648 if (json.has("code")) 3649 res.setCodeElement(parseString(json.get("code").getAsString())); 3650 if (json.has("_code")) 3651 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 3652 return res; 3653 } 3654 3655 protected Condition parseCondition(JsonObject json) throws IOException, FHIRFormatError { 3656 Condition res = new Condition(); 3657 parseDomainResourceProperties(json, res); 3658 if (json.has("identifier")) { 3659 JsonArray array = json.getAsJsonArray("identifier"); 3660 for (int i = 0; i < array.size(); i++) { 3661 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 3662 } 3663 } 3664 ; 3665 if (json.has("patient")) 3666 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 3667 if (json.has("encounter")) 3668 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 3669 if (json.has("asserter")) 3670 res.setAsserter(parseReference(json.getAsJsonObject("asserter"))); 3671 if (json.has("dateRecorded")) 3672 res.setDateRecordedElement(parseDate(json.get("dateRecorded").getAsString())); 3673 if (json.has("_dateRecorded")) 3674 parseElementProperties(json.getAsJsonObject("_dateRecorded"), res.getDateRecordedElement()); 3675 if (json.has("code")) 3676 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 3677 if (json.has("category")) 3678 res.setCategory(parseCodeableConcept(json.getAsJsonObject("category"))); 3679 if (json.has("clinicalStatus")) 3680 res.setClinicalStatusElement(parseCode(json.get("clinicalStatus").getAsString())); 3681 if (json.has("_clinicalStatus")) 3682 parseElementProperties(json.getAsJsonObject("_clinicalStatus"), res.getClinicalStatusElement()); 3683 if (json.has("verificationStatus")) 3684 res.setVerificationStatusElement(parseEnumeration(json.get("verificationStatus").getAsString(), 3685 Condition.ConditionVerificationStatus.NULL, new Condition.ConditionVerificationStatusEnumFactory())); 3686 if (json.has("_verificationStatus")) 3687 parseElementProperties(json.getAsJsonObject("_verificationStatus"), res.getVerificationStatusElement()); 3688 if (json.has("severity")) 3689 res.setSeverity(parseCodeableConcept(json.getAsJsonObject("severity"))); 3690 Type onset = parseType("onset", json); 3691 if (onset != null) 3692 res.setOnset(onset); 3693 Type abatement = parseType("abatement", json); 3694 if (abatement != null) 3695 res.setAbatement(abatement); 3696 if (json.has("stage")) 3697 res.setStage(parseConditionConditionStageComponent(json.getAsJsonObject("stage"), res)); 3698 if (json.has("evidence")) { 3699 JsonArray array = json.getAsJsonArray("evidence"); 3700 for (int i = 0; i < array.size(); i++) { 3701 res.getEvidence().add(parseConditionConditionEvidenceComponent(array.get(i).getAsJsonObject(), res)); 3702 } 3703 } 3704 ; 3705 if (json.has("bodySite")) { 3706 JsonArray array = json.getAsJsonArray("bodySite"); 3707 for (int i = 0; i < array.size(); i++) { 3708 res.getBodySite().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 3709 } 3710 } 3711 ; 3712 if (json.has("notes")) 3713 res.setNotesElement(parseString(json.get("notes").getAsString())); 3714 if (json.has("_notes")) 3715 parseElementProperties(json.getAsJsonObject("_notes"), res.getNotesElement()); 3716 return res; 3717 } 3718 3719 protected Condition.ConditionStageComponent parseConditionConditionStageComponent(JsonObject json, Condition owner) 3720 throws IOException, FHIRFormatError { 3721 Condition.ConditionStageComponent res = new Condition.ConditionStageComponent(); 3722 parseBackboneProperties(json, res); 3723 if (json.has("summary")) 3724 res.setSummary(parseCodeableConcept(json.getAsJsonObject("summary"))); 3725 if (json.has("assessment")) { 3726 JsonArray array = json.getAsJsonArray("assessment"); 3727 for (int i = 0; i < array.size(); i++) { 3728 res.getAssessment().add(parseReference(array.get(i).getAsJsonObject())); 3729 } 3730 } 3731 ; 3732 return res; 3733 } 3734 3735 protected Condition.ConditionEvidenceComponent parseConditionConditionEvidenceComponent(JsonObject json, 3736 Condition owner) throws IOException, FHIRFormatError { 3737 Condition.ConditionEvidenceComponent res = new Condition.ConditionEvidenceComponent(); 3738 parseBackboneProperties(json, res); 3739 if (json.has("code")) 3740 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 3741 if (json.has("detail")) { 3742 JsonArray array = json.getAsJsonArray("detail"); 3743 for (int i = 0; i < array.size(); i++) { 3744 res.getDetail().add(parseReference(array.get(i).getAsJsonObject())); 3745 } 3746 } 3747 ; 3748 return res; 3749 } 3750 3751 protected Conformance parseConformance(JsonObject json) throws IOException, FHIRFormatError { 3752 Conformance res = new Conformance(); 3753 parseDomainResourceProperties(json, res); 3754 if (json.has("url")) 3755 res.setUrlElement(parseUri(json.get("url").getAsString())); 3756 if (json.has("_url")) 3757 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 3758 if (json.has("version")) 3759 res.setVersionElement(parseString(json.get("version").getAsString())); 3760 if (json.has("_version")) 3761 parseElementProperties(json.getAsJsonObject("_version"), res.getVersionElement()); 3762 if (json.has("name")) 3763 res.setNameElement(parseString(json.get("name").getAsString())); 3764 if (json.has("_name")) 3765 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 3766 if (json.has("status")) 3767 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 3768 Enumerations.ConformanceResourceStatus.NULL, new Enumerations.ConformanceResourceStatusEnumFactory())); 3769 if (json.has("_status")) 3770 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 3771 if (json.has("experimental")) 3772 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 3773 if (json.has("_experimental")) 3774 parseElementProperties(json.getAsJsonObject("_experimental"), res.getExperimentalElement()); 3775 if (json.has("publisher")) 3776 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 3777 if (json.has("_publisher")) 3778 parseElementProperties(json.getAsJsonObject("_publisher"), res.getPublisherElement()); 3779 if (json.has("contact")) { 3780 JsonArray array = json.getAsJsonArray("contact"); 3781 for (int i = 0; i < array.size(); i++) { 3782 res.getContact().add(parseConformanceConformanceContactComponent(array.get(i).getAsJsonObject(), res)); 3783 } 3784 } 3785 ; 3786 if (json.has("date")) 3787 res.setDateElement(parseDateTime(json.get("date").getAsString())); 3788 if (json.has("_date")) 3789 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 3790 if (json.has("description")) 3791 res.setDescriptionElement(parseString(json.get("description").getAsString())); 3792 if (json.has("_description")) 3793 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 3794 if (json.has("requirements")) 3795 res.setRequirementsElement(parseString(json.get("requirements").getAsString())); 3796 if (json.has("_requirements")) 3797 parseElementProperties(json.getAsJsonObject("_requirements"), res.getRequirementsElement()); 3798 if (json.has("copyright")) 3799 res.setCopyrightElement(parseString(json.get("copyright").getAsString())); 3800 if (json.has("_copyright")) 3801 parseElementProperties(json.getAsJsonObject("_copyright"), res.getCopyrightElement()); 3802 if (json.has("kind")) 3803 res.setKindElement(parseEnumeration(json.get("kind").getAsString(), Conformance.ConformanceStatementKind.NULL, 3804 new Conformance.ConformanceStatementKindEnumFactory())); 3805 if (json.has("_kind")) 3806 parseElementProperties(json.getAsJsonObject("_kind"), res.getKindElement()); 3807 if (json.has("software")) 3808 res.setSoftware(parseConformanceConformanceSoftwareComponent(json.getAsJsonObject("software"), res)); 3809 if (json.has("implementation")) 3810 res.setImplementation( 3811 parseConformanceConformanceImplementationComponent(json.getAsJsonObject("implementation"), res)); 3812 if (json.has("fhirVersion")) 3813 res.setFhirVersionElement(parseId(json.get("fhirVersion").getAsString())); 3814 if (json.has("_fhirVersion")) 3815 parseElementProperties(json.getAsJsonObject("_fhirVersion"), res.getFhirVersionElement()); 3816 if (json.has("acceptUnknown")) 3817 res.setAcceptUnknownElement(parseEnumeration(json.get("acceptUnknown").getAsString(), 3818 Conformance.UnknownContentCode.NULL, new Conformance.UnknownContentCodeEnumFactory())); 3819 if (json.has("_acceptUnknown")) 3820 parseElementProperties(json.getAsJsonObject("_acceptUnknown"), res.getAcceptUnknownElement()); 3821 if (json.has("format")) { 3822 JsonArray array = json.getAsJsonArray("format"); 3823 for (int i = 0; i < array.size(); i++) { 3824 res.getFormat().add(parseCode(array.get(i).getAsString())); 3825 } 3826 } 3827 ; 3828 if (json.has("_format")) { 3829 JsonArray array = json.getAsJsonArray("_format"); 3830 for (int i = 0; i < array.size(); i++) { 3831 if (i == res.getFormat().size()) 3832 res.getFormat().add(parseCode(null)); 3833 if (array.get(i) instanceof JsonObject) 3834 parseElementProperties(array.get(i).getAsJsonObject(), res.getFormat().get(i)); 3835 } 3836 } 3837 ; 3838 if (json.has("profile")) { 3839 JsonArray array = json.getAsJsonArray("profile"); 3840 for (int i = 0; i < array.size(); i++) { 3841 res.getProfile().add(parseReference(array.get(i).getAsJsonObject())); 3842 } 3843 } 3844 ; 3845 if (json.has("rest")) { 3846 JsonArray array = json.getAsJsonArray("rest"); 3847 for (int i = 0; i < array.size(); i++) { 3848 res.getRest().add(parseConformanceConformanceRestComponent(array.get(i).getAsJsonObject(), res)); 3849 } 3850 } 3851 ; 3852 if (json.has("messaging")) { 3853 JsonArray array = json.getAsJsonArray("messaging"); 3854 for (int i = 0; i < array.size(); i++) { 3855 res.getMessaging().add(parseConformanceConformanceMessagingComponent(array.get(i).getAsJsonObject(), res)); 3856 } 3857 } 3858 ; 3859 if (json.has("document")) { 3860 JsonArray array = json.getAsJsonArray("document"); 3861 for (int i = 0; i < array.size(); i++) { 3862 res.getDocument().add(parseConformanceConformanceDocumentComponent(array.get(i).getAsJsonObject(), res)); 3863 } 3864 } 3865 ; 3866 return res; 3867 } 3868 3869 protected Conformance.ConformanceContactComponent parseConformanceConformanceContactComponent(JsonObject json, 3870 Conformance owner) throws IOException, FHIRFormatError { 3871 Conformance.ConformanceContactComponent res = new Conformance.ConformanceContactComponent(); 3872 parseBackboneProperties(json, res); 3873 if (json.has("name")) 3874 res.setNameElement(parseString(json.get("name").getAsString())); 3875 if (json.has("_name")) 3876 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 3877 if (json.has("telecom")) { 3878 JsonArray array = json.getAsJsonArray("telecom"); 3879 for (int i = 0; i < array.size(); i++) { 3880 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 3881 } 3882 } 3883 ; 3884 return res; 3885 } 3886 3887 protected Conformance.ConformanceSoftwareComponent parseConformanceConformanceSoftwareComponent(JsonObject json, 3888 Conformance owner) throws IOException, FHIRFormatError { 3889 Conformance.ConformanceSoftwareComponent res = new Conformance.ConformanceSoftwareComponent(); 3890 parseBackboneProperties(json, res); 3891 if (json.has("name")) 3892 res.setNameElement(parseString(json.get("name").getAsString())); 3893 if (json.has("_name")) 3894 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 3895 if (json.has("version")) 3896 res.setVersionElement(parseString(json.get("version").getAsString())); 3897 if (json.has("_version")) 3898 parseElementProperties(json.getAsJsonObject("_version"), res.getVersionElement()); 3899 if (json.has("releaseDate")) 3900 res.setReleaseDateElement(parseDateTime(json.get("releaseDate").getAsString())); 3901 if (json.has("_releaseDate")) 3902 parseElementProperties(json.getAsJsonObject("_releaseDate"), res.getReleaseDateElement()); 3903 return res; 3904 } 3905 3906 protected Conformance.ConformanceImplementationComponent parseConformanceConformanceImplementationComponent( 3907 JsonObject json, Conformance owner) throws IOException, FHIRFormatError { 3908 Conformance.ConformanceImplementationComponent res = new Conformance.ConformanceImplementationComponent(); 3909 parseBackboneProperties(json, res); 3910 if (json.has("description")) 3911 res.setDescriptionElement(parseString(json.get("description").getAsString())); 3912 if (json.has("_description")) 3913 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 3914 if (json.has("url")) 3915 res.setUrlElement(parseUri(json.get("url").getAsString())); 3916 if (json.has("_url")) 3917 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 3918 return res; 3919 } 3920 3921 protected Conformance.ConformanceRestComponent parseConformanceConformanceRestComponent(JsonObject json, 3922 Conformance owner) throws IOException, FHIRFormatError { 3923 Conformance.ConformanceRestComponent res = new Conformance.ConformanceRestComponent(); 3924 parseBackboneProperties(json, res); 3925 if (json.has("mode")) 3926 res.setModeElement(parseEnumeration(json.get("mode").getAsString(), Conformance.RestfulConformanceMode.NULL, 3927 new Conformance.RestfulConformanceModeEnumFactory())); 3928 if (json.has("_mode")) 3929 parseElementProperties(json.getAsJsonObject("_mode"), res.getModeElement()); 3930 if (json.has("documentation")) 3931 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 3932 if (json.has("_documentation")) 3933 parseElementProperties(json.getAsJsonObject("_documentation"), res.getDocumentationElement()); 3934 if (json.has("security")) 3935 res.setSecurity(parseConformanceConformanceRestSecurityComponent(json.getAsJsonObject("security"), owner)); 3936 if (json.has("resource")) { 3937 JsonArray array = json.getAsJsonArray("resource"); 3938 for (int i = 0; i < array.size(); i++) { 3939 res.getResource().add(parseConformanceConformanceRestResourceComponent(array.get(i).getAsJsonObject(), owner)); 3940 } 3941 } 3942 ; 3943 if (json.has("interaction")) { 3944 JsonArray array = json.getAsJsonArray("interaction"); 3945 for (int i = 0; i < array.size(); i++) { 3946 res.getInteraction().add(parseConformanceSystemInteractionComponent(array.get(i).getAsJsonObject(), owner)); 3947 } 3948 } 3949 ; 3950 if (json.has("transactionMode")) 3951 res.setTransactionModeElement(parseEnumeration(json.get("transactionMode").getAsString(), 3952 Conformance.TransactionMode.NULL, new Conformance.TransactionModeEnumFactory())); 3953 if (json.has("_transactionMode")) 3954 parseElementProperties(json.getAsJsonObject("_transactionMode"), res.getTransactionModeElement()); 3955 if (json.has("searchParam")) { 3956 JsonArray array = json.getAsJsonArray("searchParam"); 3957 for (int i = 0; i < array.size(); i++) { 3958 res.getSearchParam() 3959 .add(parseConformanceConformanceRestResourceSearchParamComponent(array.get(i).getAsJsonObject(), owner)); 3960 } 3961 } 3962 ; 3963 if (json.has("operation")) { 3964 JsonArray array = json.getAsJsonArray("operation"); 3965 for (int i = 0; i < array.size(); i++) { 3966 res.getOperation() 3967 .add(parseConformanceConformanceRestOperationComponent(array.get(i).getAsJsonObject(), owner)); 3968 } 3969 } 3970 ; 3971 if (json.has("compartment")) { 3972 JsonArray array = json.getAsJsonArray("compartment"); 3973 for (int i = 0; i < array.size(); i++) { 3974 res.getCompartment().add(parseUri(array.get(i).getAsString())); 3975 } 3976 } 3977 ; 3978 if (json.has("_compartment")) { 3979 JsonArray array = json.getAsJsonArray("_compartment"); 3980 for (int i = 0; i < array.size(); i++) { 3981 if (i == res.getCompartment().size()) 3982 res.getCompartment().add(parseUri(null)); 3983 if (array.get(i) instanceof JsonObject) 3984 parseElementProperties(array.get(i).getAsJsonObject(), res.getCompartment().get(i)); 3985 } 3986 } 3987 ; 3988 return res; 3989 } 3990 3991 protected Conformance.ConformanceRestSecurityComponent parseConformanceConformanceRestSecurityComponent( 3992 JsonObject json, Conformance owner) throws IOException, FHIRFormatError { 3993 Conformance.ConformanceRestSecurityComponent res = new Conformance.ConformanceRestSecurityComponent(); 3994 parseBackboneProperties(json, res); 3995 if (json.has("cors")) 3996 res.setCorsElement(parseBoolean(json.get("cors").getAsBoolean())); 3997 if (json.has("_cors")) 3998 parseElementProperties(json.getAsJsonObject("_cors"), res.getCorsElement()); 3999 if (json.has("service")) { 4000 JsonArray array = json.getAsJsonArray("service"); 4001 for (int i = 0; i < array.size(); i++) { 4002 res.getService().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4003 } 4004 } 4005 ; 4006 if (json.has("description")) 4007 res.setDescriptionElement(parseString(json.get("description").getAsString())); 4008 if (json.has("_description")) 4009 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 4010 if (json.has("certificate")) { 4011 JsonArray array = json.getAsJsonArray("certificate"); 4012 for (int i = 0; i < array.size(); i++) { 4013 res.getCertificate() 4014 .add(parseConformanceConformanceRestSecurityCertificateComponent(array.get(i).getAsJsonObject(), owner)); 4015 } 4016 } 4017 ; 4018 return res; 4019 } 4020 4021 protected Conformance.ConformanceRestSecurityCertificateComponent parseConformanceConformanceRestSecurityCertificateComponent( 4022 JsonObject json, Conformance owner) throws IOException, FHIRFormatError { 4023 Conformance.ConformanceRestSecurityCertificateComponent res = new Conformance.ConformanceRestSecurityCertificateComponent(); 4024 parseBackboneProperties(json, res); 4025 if (json.has("type")) 4026 res.setTypeElement(parseCode(json.get("type").getAsString())); 4027 if (json.has("_type")) 4028 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 4029 if (json.has("blob")) 4030 res.setBlobElement(parseBase64Binary(json.get("blob").getAsString())); 4031 if (json.has("_blob")) 4032 parseElementProperties(json.getAsJsonObject("_blob"), res.getBlobElement()); 4033 return res; 4034 } 4035 4036 protected Conformance.ConformanceRestResourceComponent parseConformanceConformanceRestResourceComponent( 4037 JsonObject json, Conformance owner) throws IOException, FHIRFormatError { 4038 Conformance.ConformanceRestResourceComponent res = new Conformance.ConformanceRestResourceComponent(); 4039 parseBackboneProperties(json, res); 4040 if (json.has("type")) 4041 res.setTypeElement(parseCode(json.get("type").getAsString())); 4042 if (json.has("_type")) 4043 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 4044 if (json.has("profile")) 4045 res.setProfile(parseReference(json.getAsJsonObject("profile"))); 4046 if (json.has("interaction")) { 4047 JsonArray array = json.getAsJsonArray("interaction"); 4048 for (int i = 0; i < array.size(); i++) { 4049 res.getInteraction().add(parseConformanceResourceInteractionComponent(array.get(i).getAsJsonObject(), owner)); 4050 } 4051 } 4052 ; 4053 if (json.has("versioning")) 4054 res.setVersioningElement(parseEnumeration(json.get("versioning").getAsString(), 4055 Conformance.ResourceVersionPolicy.NULL, new Conformance.ResourceVersionPolicyEnumFactory())); 4056 if (json.has("_versioning")) 4057 parseElementProperties(json.getAsJsonObject("_versioning"), res.getVersioningElement()); 4058 if (json.has("readHistory")) 4059 res.setReadHistoryElement(parseBoolean(json.get("readHistory").getAsBoolean())); 4060 if (json.has("_readHistory")) 4061 parseElementProperties(json.getAsJsonObject("_readHistory"), res.getReadHistoryElement()); 4062 if (json.has("updateCreate")) 4063 res.setUpdateCreateElement(parseBoolean(json.get("updateCreate").getAsBoolean())); 4064 if (json.has("_updateCreate")) 4065 parseElementProperties(json.getAsJsonObject("_updateCreate"), res.getUpdateCreateElement()); 4066 if (json.has("conditionalCreate")) 4067 res.setConditionalCreateElement(parseBoolean(json.get("conditionalCreate").getAsBoolean())); 4068 if (json.has("_conditionalCreate")) 4069 parseElementProperties(json.getAsJsonObject("_conditionalCreate"), res.getConditionalCreateElement()); 4070 if (json.has("conditionalUpdate")) 4071 res.setConditionalUpdateElement(parseBoolean(json.get("conditionalUpdate").getAsBoolean())); 4072 if (json.has("_conditionalUpdate")) 4073 parseElementProperties(json.getAsJsonObject("_conditionalUpdate"), res.getConditionalUpdateElement()); 4074 if (json.has("conditionalDelete")) 4075 res.setConditionalDeleteElement(parseEnumeration(json.get("conditionalDelete").getAsString(), 4076 Conformance.ConditionalDeleteStatus.NULL, new Conformance.ConditionalDeleteStatusEnumFactory())); 4077 if (json.has("_conditionalDelete")) 4078 parseElementProperties(json.getAsJsonObject("_conditionalDelete"), res.getConditionalDeleteElement()); 4079 if (json.has("searchInclude")) { 4080 JsonArray array = json.getAsJsonArray("searchInclude"); 4081 for (int i = 0; i < array.size(); i++) { 4082 res.getSearchInclude().add(parseString(array.get(i).getAsString())); 4083 } 4084 } 4085 ; 4086 if (json.has("_searchInclude")) { 4087 JsonArray array = json.getAsJsonArray("_searchInclude"); 4088 for (int i = 0; i < array.size(); i++) { 4089 if (i == res.getSearchInclude().size()) 4090 res.getSearchInclude().add(parseString(null)); 4091 if (array.get(i) instanceof JsonObject) 4092 parseElementProperties(array.get(i).getAsJsonObject(), res.getSearchInclude().get(i)); 4093 } 4094 } 4095 ; 4096 if (json.has("searchRevInclude")) { 4097 JsonArray array = json.getAsJsonArray("searchRevInclude"); 4098 for (int i = 0; i < array.size(); i++) { 4099 res.getSearchRevInclude().add(parseString(array.get(i).getAsString())); 4100 } 4101 } 4102 ; 4103 if (json.has("_searchRevInclude")) { 4104 JsonArray array = json.getAsJsonArray("_searchRevInclude"); 4105 for (int i = 0; i < array.size(); i++) { 4106 if (i == res.getSearchRevInclude().size()) 4107 res.getSearchRevInclude().add(parseString(null)); 4108 if (array.get(i) instanceof JsonObject) 4109 parseElementProperties(array.get(i).getAsJsonObject(), res.getSearchRevInclude().get(i)); 4110 } 4111 } 4112 ; 4113 if (json.has("searchParam")) { 4114 JsonArray array = json.getAsJsonArray("searchParam"); 4115 for (int i = 0; i < array.size(); i++) { 4116 res.getSearchParam() 4117 .add(parseConformanceConformanceRestResourceSearchParamComponent(array.get(i).getAsJsonObject(), owner)); 4118 } 4119 } 4120 ; 4121 return res; 4122 } 4123 4124 protected Conformance.ResourceInteractionComponent parseConformanceResourceInteractionComponent(JsonObject json, 4125 Conformance owner) throws IOException, FHIRFormatError { 4126 Conformance.ResourceInteractionComponent res = new Conformance.ResourceInteractionComponent(); 4127 parseBackboneProperties(json, res); 4128 if (json.has("code")) 4129 res.setCodeElement(parseEnumeration(json.get("code").getAsString(), Conformance.TypeRestfulInteraction.NULL, 4130 new Conformance.TypeRestfulInteractionEnumFactory())); 4131 if (json.has("_code")) 4132 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 4133 if (json.has("documentation")) 4134 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 4135 if (json.has("_documentation")) 4136 parseElementProperties(json.getAsJsonObject("_documentation"), res.getDocumentationElement()); 4137 return res; 4138 } 4139 4140 protected Conformance.ConformanceRestResourceSearchParamComponent parseConformanceConformanceRestResourceSearchParamComponent( 4141 JsonObject json, Conformance owner) throws IOException, FHIRFormatError { 4142 Conformance.ConformanceRestResourceSearchParamComponent res = new Conformance.ConformanceRestResourceSearchParamComponent(); 4143 parseBackboneProperties(json, res); 4144 if (json.has("name")) 4145 res.setNameElement(parseString(json.get("name").getAsString())); 4146 if (json.has("_name")) 4147 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 4148 if (json.has("definition")) 4149 res.setDefinitionElement(parseUri(json.get("definition").getAsString())); 4150 if (json.has("_definition")) 4151 parseElementProperties(json.getAsJsonObject("_definition"), res.getDefinitionElement()); 4152 if (json.has("type")) 4153 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Enumerations.SearchParamType.NULL, 4154 new Enumerations.SearchParamTypeEnumFactory())); 4155 if (json.has("_type")) 4156 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 4157 if (json.has("documentation")) 4158 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 4159 if (json.has("_documentation")) 4160 parseElementProperties(json.getAsJsonObject("_documentation"), res.getDocumentationElement()); 4161 if (json.has("target")) { 4162 JsonArray array = json.getAsJsonArray("target"); 4163 for (int i = 0; i < array.size(); i++) { 4164 res.getTarget().add(parseCode(array.get(i).getAsString())); 4165 } 4166 } 4167 ; 4168 if (json.has("_target")) { 4169 JsonArray array = json.getAsJsonArray("_target"); 4170 for (int i = 0; i < array.size(); i++) { 4171 if (i == res.getTarget().size()) 4172 res.getTarget().add(parseCode(null)); 4173 if (array.get(i) instanceof JsonObject) 4174 parseElementProperties(array.get(i).getAsJsonObject(), res.getTarget().get(i)); 4175 } 4176 } 4177 ; 4178 if (json.has("modifier")) { 4179 JsonArray array = json.getAsJsonArray("modifier"); 4180 for (int i = 0; i < array.size(); i++) { 4181 res.getModifier().add(parseEnumeration(array.get(i).getAsString(), Conformance.SearchModifierCode.NULL, 4182 new Conformance.SearchModifierCodeEnumFactory())); 4183 } 4184 } 4185 ; 4186 if (json.has("_modifier")) { 4187 JsonArray array = json.getAsJsonArray("_modifier"); 4188 for (int i = 0; i < array.size(); i++) { 4189 if (i == res.getModifier().size()) 4190 res.getModifier().add(parseEnumeration(null, Conformance.SearchModifierCode.NULL, 4191 new Conformance.SearchModifierCodeEnumFactory())); 4192 if (array.get(i) instanceof JsonObject) 4193 parseElementProperties(array.get(i).getAsJsonObject(), res.getModifier().get(i)); 4194 } 4195 } 4196 ; 4197 if (json.has("chain")) { 4198 JsonArray array = json.getAsJsonArray("chain"); 4199 for (int i = 0; i < array.size(); i++) { 4200 res.getChain().add(parseString(array.get(i).getAsString())); 4201 } 4202 } 4203 ; 4204 if (json.has("_chain")) { 4205 JsonArray array = json.getAsJsonArray("_chain"); 4206 for (int i = 0; i < array.size(); i++) { 4207 if (i == res.getChain().size()) 4208 res.getChain().add(parseString(null)); 4209 if (array.get(i) instanceof JsonObject) 4210 parseElementProperties(array.get(i).getAsJsonObject(), res.getChain().get(i)); 4211 } 4212 } 4213 ; 4214 return res; 4215 } 4216 4217 protected Conformance.SystemInteractionComponent parseConformanceSystemInteractionComponent(JsonObject json, 4218 Conformance owner) throws IOException, FHIRFormatError { 4219 Conformance.SystemInteractionComponent res = new Conformance.SystemInteractionComponent(); 4220 parseBackboneProperties(json, res); 4221 if (json.has("code")) 4222 res.setCodeElement(parseEnumeration(json.get("code").getAsString(), Conformance.SystemRestfulInteraction.NULL, 4223 new Conformance.SystemRestfulInteractionEnumFactory())); 4224 if (json.has("_code")) 4225 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 4226 if (json.has("documentation")) 4227 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 4228 if (json.has("_documentation")) 4229 parseElementProperties(json.getAsJsonObject("_documentation"), res.getDocumentationElement()); 4230 return res; 4231 } 4232 4233 protected Conformance.ConformanceRestOperationComponent parseConformanceConformanceRestOperationComponent( 4234 JsonObject json, Conformance owner) throws IOException, FHIRFormatError { 4235 Conformance.ConformanceRestOperationComponent res = new Conformance.ConformanceRestOperationComponent(); 4236 parseBackboneProperties(json, res); 4237 if (json.has("name")) 4238 res.setNameElement(parseString(json.get("name").getAsString())); 4239 if (json.has("_name")) 4240 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 4241 if (json.has("definition")) 4242 res.setDefinition(parseReference(json.getAsJsonObject("definition"))); 4243 return res; 4244 } 4245 4246 protected Conformance.ConformanceMessagingComponent parseConformanceConformanceMessagingComponent(JsonObject json, 4247 Conformance owner) throws IOException, FHIRFormatError { 4248 Conformance.ConformanceMessagingComponent res = new Conformance.ConformanceMessagingComponent(); 4249 parseBackboneProperties(json, res); 4250 if (json.has("endpoint")) { 4251 JsonArray array = json.getAsJsonArray("endpoint"); 4252 for (int i = 0; i < array.size(); i++) { 4253 res.getEndpoint() 4254 .add(parseConformanceConformanceMessagingEndpointComponent(array.get(i).getAsJsonObject(), owner)); 4255 } 4256 } 4257 ; 4258 if (json.has("reliableCache")) 4259 res.setReliableCacheElement(parseUnsignedInt(json.get("reliableCache").getAsString())); 4260 if (json.has("_reliableCache")) 4261 parseElementProperties(json.getAsJsonObject("_reliableCache"), res.getReliableCacheElement()); 4262 if (json.has("documentation")) 4263 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 4264 if (json.has("_documentation")) 4265 parseElementProperties(json.getAsJsonObject("_documentation"), res.getDocumentationElement()); 4266 if (json.has("event")) { 4267 JsonArray array = json.getAsJsonArray("event"); 4268 for (int i = 0; i < array.size(); i++) { 4269 res.getEvent().add(parseConformanceConformanceMessagingEventComponent(array.get(i).getAsJsonObject(), owner)); 4270 } 4271 } 4272 ; 4273 return res; 4274 } 4275 4276 protected Conformance.ConformanceMessagingEndpointComponent parseConformanceConformanceMessagingEndpointComponent( 4277 JsonObject json, Conformance owner) throws IOException, FHIRFormatError { 4278 Conformance.ConformanceMessagingEndpointComponent res = new Conformance.ConformanceMessagingEndpointComponent(); 4279 parseBackboneProperties(json, res); 4280 if (json.has("protocol")) 4281 res.setProtocol(parseCoding(json.getAsJsonObject("protocol"))); 4282 if (json.has("address")) 4283 res.setAddressElement(parseUri(json.get("address").getAsString())); 4284 if (json.has("_address")) 4285 parseElementProperties(json.getAsJsonObject("_address"), res.getAddressElement()); 4286 return res; 4287 } 4288 4289 protected Conformance.ConformanceMessagingEventComponent parseConformanceConformanceMessagingEventComponent( 4290 JsonObject json, Conformance owner) throws IOException, FHIRFormatError { 4291 Conformance.ConformanceMessagingEventComponent res = new Conformance.ConformanceMessagingEventComponent(); 4292 parseBackboneProperties(json, res); 4293 if (json.has("code")) 4294 res.setCode(parseCoding(json.getAsJsonObject("code"))); 4295 if (json.has("category")) 4296 res.setCategoryElement(parseEnumeration(json.get("category").getAsString(), 4297 Conformance.MessageSignificanceCategory.NULL, new Conformance.MessageSignificanceCategoryEnumFactory())); 4298 if (json.has("_category")) 4299 parseElementProperties(json.getAsJsonObject("_category"), res.getCategoryElement()); 4300 if (json.has("mode")) 4301 res.setModeElement(parseEnumeration(json.get("mode").getAsString(), Conformance.ConformanceEventMode.NULL, 4302 new Conformance.ConformanceEventModeEnumFactory())); 4303 if (json.has("_mode")) 4304 parseElementProperties(json.getAsJsonObject("_mode"), res.getModeElement()); 4305 if (json.has("focus")) 4306 res.setFocusElement(parseCode(json.get("focus").getAsString())); 4307 if (json.has("_focus")) 4308 parseElementProperties(json.getAsJsonObject("_focus"), res.getFocusElement()); 4309 if (json.has("request")) 4310 res.setRequest(parseReference(json.getAsJsonObject("request"))); 4311 if (json.has("response")) 4312 res.setResponse(parseReference(json.getAsJsonObject("response"))); 4313 if (json.has("documentation")) 4314 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 4315 if (json.has("_documentation")) 4316 parseElementProperties(json.getAsJsonObject("_documentation"), res.getDocumentationElement()); 4317 return res; 4318 } 4319 4320 protected Conformance.ConformanceDocumentComponent parseConformanceConformanceDocumentComponent(JsonObject json, 4321 Conformance owner) throws IOException, FHIRFormatError { 4322 Conformance.ConformanceDocumentComponent res = new Conformance.ConformanceDocumentComponent(); 4323 parseBackboneProperties(json, res); 4324 if (json.has("mode")) 4325 res.setModeElement(parseEnumeration(json.get("mode").getAsString(), Conformance.DocumentMode.NULL, 4326 new Conformance.DocumentModeEnumFactory())); 4327 if (json.has("_mode")) 4328 parseElementProperties(json.getAsJsonObject("_mode"), res.getModeElement()); 4329 if (json.has("documentation")) 4330 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 4331 if (json.has("_documentation")) 4332 parseElementProperties(json.getAsJsonObject("_documentation"), res.getDocumentationElement()); 4333 if (json.has("profile")) 4334 res.setProfile(parseReference(json.getAsJsonObject("profile"))); 4335 return res; 4336 } 4337 4338 protected Contract parseContract(JsonObject json) throws IOException, FHIRFormatError { 4339 Contract res = new Contract(); 4340 parseDomainResourceProperties(json, res); 4341 if (json.has("identifier")) 4342 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 4343 if (json.has("issued")) 4344 res.setIssuedElement(parseDateTime(json.get("issued").getAsString())); 4345 if (json.has("_issued")) 4346 parseElementProperties(json.getAsJsonObject("_issued"), res.getIssuedElement()); 4347 if (json.has("applies")) 4348 res.setApplies(parsePeriod(json.getAsJsonObject("applies"))); 4349 if (json.has("subject")) { 4350 JsonArray array = json.getAsJsonArray("subject"); 4351 for (int i = 0; i < array.size(); i++) { 4352 res.getSubject().add(parseReference(array.get(i).getAsJsonObject())); 4353 } 4354 } 4355 ; 4356 if (json.has("authority")) { 4357 JsonArray array = json.getAsJsonArray("authority"); 4358 for (int i = 0; i < array.size(); i++) { 4359 res.getAuthority().add(parseReference(array.get(i).getAsJsonObject())); 4360 } 4361 } 4362 ; 4363 if (json.has("domain")) { 4364 JsonArray array = json.getAsJsonArray("domain"); 4365 for (int i = 0; i < array.size(); i++) { 4366 res.getDomain().add(parseReference(array.get(i).getAsJsonObject())); 4367 } 4368 } 4369 ; 4370 if (json.has("type")) 4371 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 4372 if (json.has("subType")) { 4373 JsonArray array = json.getAsJsonArray("subType"); 4374 for (int i = 0; i < array.size(); i++) { 4375 res.getSubType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4376 } 4377 } 4378 ; 4379 if (json.has("action")) { 4380 JsonArray array = json.getAsJsonArray("action"); 4381 for (int i = 0; i < array.size(); i++) { 4382 res.getAction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4383 } 4384 } 4385 ; 4386 if (json.has("actionReason")) { 4387 JsonArray array = json.getAsJsonArray("actionReason"); 4388 for (int i = 0; i < array.size(); i++) { 4389 res.getActionReason().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4390 } 4391 } 4392 ; 4393 if (json.has("actor")) { 4394 JsonArray array = json.getAsJsonArray("actor"); 4395 for (int i = 0; i < array.size(); i++) { 4396 res.getActor().add(parseContractActorComponent(array.get(i).getAsJsonObject(), res)); 4397 } 4398 } 4399 ; 4400 if (json.has("valuedItem")) { 4401 JsonArray array = json.getAsJsonArray("valuedItem"); 4402 for (int i = 0; i < array.size(); i++) { 4403 res.getValuedItem().add(parseContractValuedItemComponent(array.get(i).getAsJsonObject(), res)); 4404 } 4405 } 4406 ; 4407 if (json.has("signer")) { 4408 JsonArray array = json.getAsJsonArray("signer"); 4409 for (int i = 0; i < array.size(); i++) { 4410 res.getSigner().add(parseContractSignatoryComponent(array.get(i).getAsJsonObject(), res)); 4411 } 4412 } 4413 ; 4414 if (json.has("term")) { 4415 JsonArray array = json.getAsJsonArray("term"); 4416 for (int i = 0; i < array.size(); i++) { 4417 res.getTerm().add(parseContractTermComponent(array.get(i).getAsJsonObject(), res)); 4418 } 4419 } 4420 ; 4421 Type binding = parseType("binding", json); 4422 if (binding != null) 4423 res.setBinding(binding); 4424 if (json.has("friendly")) { 4425 JsonArray array = json.getAsJsonArray("friendly"); 4426 for (int i = 0; i < array.size(); i++) { 4427 res.getFriendly().add(parseContractFriendlyLanguageComponent(array.get(i).getAsJsonObject(), res)); 4428 } 4429 } 4430 ; 4431 if (json.has("legal")) { 4432 JsonArray array = json.getAsJsonArray("legal"); 4433 for (int i = 0; i < array.size(); i++) { 4434 res.getLegal().add(parseContractLegalLanguageComponent(array.get(i).getAsJsonObject(), res)); 4435 } 4436 } 4437 ; 4438 if (json.has("rule")) { 4439 JsonArray array = json.getAsJsonArray("rule"); 4440 for (int i = 0; i < array.size(); i++) { 4441 res.getRule().add(parseContractComputableLanguageComponent(array.get(i).getAsJsonObject(), res)); 4442 } 4443 } 4444 ; 4445 return res; 4446 } 4447 4448 protected Contract.ActorComponent parseContractActorComponent(JsonObject json, Contract owner) 4449 throws IOException, FHIRFormatError { 4450 Contract.ActorComponent res = new Contract.ActorComponent(); 4451 parseBackboneProperties(json, res); 4452 if (json.has("entity")) 4453 res.setEntity(parseReference(json.getAsJsonObject("entity"))); 4454 if (json.has("role")) { 4455 JsonArray array = json.getAsJsonArray("role"); 4456 for (int i = 0; i < array.size(); i++) { 4457 res.getRole().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4458 } 4459 } 4460 ; 4461 return res; 4462 } 4463 4464 protected Contract.ValuedItemComponent parseContractValuedItemComponent(JsonObject json, Contract owner) 4465 throws IOException, FHIRFormatError { 4466 Contract.ValuedItemComponent res = new Contract.ValuedItemComponent(); 4467 parseBackboneProperties(json, res); 4468 Type entity = parseType("entity", json); 4469 if (entity != null) 4470 res.setEntity(entity); 4471 if (json.has("identifier")) 4472 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 4473 if (json.has("effectiveTime")) 4474 res.setEffectiveTimeElement(parseDateTime(json.get("effectiveTime").getAsString())); 4475 if (json.has("_effectiveTime")) 4476 parseElementProperties(json.getAsJsonObject("_effectiveTime"), res.getEffectiveTimeElement()); 4477 if (json.has("quantity")) 4478 res.setQuantity(parseSimpleQuantity(json.getAsJsonObject("quantity"))); 4479 if (json.has("unitPrice")) 4480 res.setUnitPrice(parseMoney(json.getAsJsonObject("unitPrice"))); 4481 if (json.has("factor")) 4482 res.setFactorElement(parseDecimal(json.get("factor").getAsBigDecimal())); 4483 if (json.has("_factor")) 4484 parseElementProperties(json.getAsJsonObject("_factor"), res.getFactorElement()); 4485 if (json.has("points")) 4486 res.setPointsElement(parseDecimal(json.get("points").getAsBigDecimal())); 4487 if (json.has("_points")) 4488 parseElementProperties(json.getAsJsonObject("_points"), res.getPointsElement()); 4489 if (json.has("net")) 4490 res.setNet(parseMoney(json.getAsJsonObject("net"))); 4491 return res; 4492 } 4493 4494 protected Contract.SignatoryComponent parseContractSignatoryComponent(JsonObject json, Contract owner) 4495 throws IOException, FHIRFormatError { 4496 Contract.SignatoryComponent res = new Contract.SignatoryComponent(); 4497 parseBackboneProperties(json, res); 4498 if (json.has("type")) 4499 res.setType(parseCoding(json.getAsJsonObject("type"))); 4500 if (json.has("party")) 4501 res.setParty(parseReference(json.getAsJsonObject("party"))); 4502 if (json.has("signature")) 4503 res.setSignatureElement(parseString(json.get("signature").getAsString())); 4504 if (json.has("_signature")) 4505 parseElementProperties(json.getAsJsonObject("_signature"), res.getSignatureElement()); 4506 return res; 4507 } 4508 4509 protected Contract.TermComponent parseContractTermComponent(JsonObject json, Contract owner) 4510 throws IOException, FHIRFormatError { 4511 Contract.TermComponent res = new Contract.TermComponent(); 4512 parseBackboneProperties(json, res); 4513 if (json.has("identifier")) 4514 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 4515 if (json.has("issued")) 4516 res.setIssuedElement(parseDateTime(json.get("issued").getAsString())); 4517 if (json.has("_issued")) 4518 parseElementProperties(json.getAsJsonObject("_issued"), res.getIssuedElement()); 4519 if (json.has("applies")) 4520 res.setApplies(parsePeriod(json.getAsJsonObject("applies"))); 4521 if (json.has("type")) 4522 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 4523 if (json.has("subType")) 4524 res.setSubType(parseCodeableConcept(json.getAsJsonObject("subType"))); 4525 if (json.has("subject")) 4526 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 4527 if (json.has("action")) { 4528 JsonArray array = json.getAsJsonArray("action"); 4529 for (int i = 0; i < array.size(); i++) { 4530 res.getAction().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4531 } 4532 } 4533 ; 4534 if (json.has("actionReason")) { 4535 JsonArray array = json.getAsJsonArray("actionReason"); 4536 for (int i = 0; i < array.size(); i++) { 4537 res.getActionReason().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4538 } 4539 } 4540 ; 4541 if (json.has("actor")) { 4542 JsonArray array = json.getAsJsonArray("actor"); 4543 for (int i = 0; i < array.size(); i++) { 4544 res.getActor().add(parseContractTermActorComponent(array.get(i).getAsJsonObject(), owner)); 4545 } 4546 } 4547 ; 4548 if (json.has("text")) 4549 res.setTextElement(parseString(json.get("text").getAsString())); 4550 if (json.has("_text")) 4551 parseElementProperties(json.getAsJsonObject("_text"), res.getTextElement()); 4552 if (json.has("valuedItem")) { 4553 JsonArray array = json.getAsJsonArray("valuedItem"); 4554 for (int i = 0; i < array.size(); i++) { 4555 res.getValuedItem().add(parseContractTermValuedItemComponent(array.get(i).getAsJsonObject(), owner)); 4556 } 4557 } 4558 ; 4559 if (json.has("group")) { 4560 JsonArray array = json.getAsJsonArray("group"); 4561 for (int i = 0; i < array.size(); i++) { 4562 res.getGroup().add(parseContractTermComponent(array.get(i).getAsJsonObject(), owner)); 4563 } 4564 } 4565 ; 4566 return res; 4567 } 4568 4569 protected Contract.TermActorComponent parseContractTermActorComponent(JsonObject json, Contract owner) 4570 throws IOException, FHIRFormatError { 4571 Contract.TermActorComponent res = new Contract.TermActorComponent(); 4572 parseBackboneProperties(json, res); 4573 if (json.has("entity")) 4574 res.setEntity(parseReference(json.getAsJsonObject("entity"))); 4575 if (json.has("role")) { 4576 JsonArray array = json.getAsJsonArray("role"); 4577 for (int i = 0; i < array.size(); i++) { 4578 res.getRole().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4579 } 4580 } 4581 ; 4582 return res; 4583 } 4584 4585 protected Contract.TermValuedItemComponent parseContractTermValuedItemComponent(JsonObject json, Contract owner) 4586 throws IOException, FHIRFormatError { 4587 Contract.TermValuedItemComponent res = new Contract.TermValuedItemComponent(); 4588 parseBackboneProperties(json, res); 4589 Type entity = parseType("entity", json); 4590 if (entity != null) 4591 res.setEntity(entity); 4592 if (json.has("identifier")) 4593 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 4594 if (json.has("effectiveTime")) 4595 res.setEffectiveTimeElement(parseDateTime(json.get("effectiveTime").getAsString())); 4596 if (json.has("_effectiveTime")) 4597 parseElementProperties(json.getAsJsonObject("_effectiveTime"), res.getEffectiveTimeElement()); 4598 if (json.has("quantity")) 4599 res.setQuantity(parseSimpleQuantity(json.getAsJsonObject("quantity"))); 4600 if (json.has("unitPrice")) 4601 res.setUnitPrice(parseMoney(json.getAsJsonObject("unitPrice"))); 4602 if (json.has("factor")) 4603 res.setFactorElement(parseDecimal(json.get("factor").getAsBigDecimal())); 4604 if (json.has("_factor")) 4605 parseElementProperties(json.getAsJsonObject("_factor"), res.getFactorElement()); 4606 if (json.has("points")) 4607 res.setPointsElement(parseDecimal(json.get("points").getAsBigDecimal())); 4608 if (json.has("_points")) 4609 parseElementProperties(json.getAsJsonObject("_points"), res.getPointsElement()); 4610 if (json.has("net")) 4611 res.setNet(parseMoney(json.getAsJsonObject("net"))); 4612 return res; 4613 } 4614 4615 protected Contract.FriendlyLanguageComponent parseContractFriendlyLanguageComponent(JsonObject json, Contract owner) 4616 throws IOException, FHIRFormatError { 4617 Contract.FriendlyLanguageComponent res = new Contract.FriendlyLanguageComponent(); 4618 parseBackboneProperties(json, res); 4619 Type content = parseType("content", json); 4620 if (content != null) 4621 res.setContent(content); 4622 return res; 4623 } 4624 4625 protected Contract.LegalLanguageComponent parseContractLegalLanguageComponent(JsonObject json, Contract owner) 4626 throws IOException, FHIRFormatError { 4627 Contract.LegalLanguageComponent res = new Contract.LegalLanguageComponent(); 4628 parseBackboneProperties(json, res); 4629 Type content = parseType("content", json); 4630 if (content != null) 4631 res.setContent(content); 4632 return res; 4633 } 4634 4635 protected Contract.ComputableLanguageComponent parseContractComputableLanguageComponent(JsonObject json, 4636 Contract owner) throws IOException, FHIRFormatError { 4637 Contract.ComputableLanguageComponent res = new Contract.ComputableLanguageComponent(); 4638 parseBackboneProperties(json, res); 4639 Type content = parseType("content", json); 4640 if (content != null) 4641 res.setContent(content); 4642 return res; 4643 } 4644 4645 protected Coverage parseCoverage(JsonObject json) throws IOException, FHIRFormatError { 4646 Coverage res = new Coverage(); 4647 parseDomainResourceProperties(json, res); 4648 if (json.has("issuer")) 4649 res.setIssuer(parseReference(json.getAsJsonObject("issuer"))); 4650 if (json.has("bin")) 4651 res.setBin(parseIdentifier(json.getAsJsonObject("bin"))); 4652 if (json.has("period")) 4653 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 4654 if (json.has("type")) 4655 res.setType(parseCoding(json.getAsJsonObject("type"))); 4656 if (json.has("subscriberId")) 4657 res.setSubscriberId(parseIdentifier(json.getAsJsonObject("subscriberId"))); 4658 if (json.has("identifier")) { 4659 JsonArray array = json.getAsJsonArray("identifier"); 4660 for (int i = 0; i < array.size(); i++) { 4661 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 4662 } 4663 } 4664 ; 4665 if (json.has("group")) 4666 res.setGroupElement(parseString(json.get("group").getAsString())); 4667 if (json.has("_group")) 4668 parseElementProperties(json.getAsJsonObject("_group"), res.getGroupElement()); 4669 if (json.has("plan")) 4670 res.setPlanElement(parseString(json.get("plan").getAsString())); 4671 if (json.has("_plan")) 4672 parseElementProperties(json.getAsJsonObject("_plan"), res.getPlanElement()); 4673 if (json.has("subPlan")) 4674 res.setSubPlanElement(parseString(json.get("subPlan").getAsString())); 4675 if (json.has("_subPlan")) 4676 parseElementProperties(json.getAsJsonObject("_subPlan"), res.getSubPlanElement()); 4677 if (json.has("dependent")) 4678 res.setDependentElement(parsePositiveInt(json.get("dependent").getAsString())); 4679 if (json.has("_dependent")) 4680 parseElementProperties(json.getAsJsonObject("_dependent"), res.getDependentElement()); 4681 if (json.has("sequence")) 4682 res.setSequenceElement(parsePositiveInt(json.get("sequence").getAsString())); 4683 if (json.has("_sequence")) 4684 parseElementProperties(json.getAsJsonObject("_sequence"), res.getSequenceElement()); 4685 if (json.has("subscriber")) 4686 res.setSubscriber(parseReference(json.getAsJsonObject("subscriber"))); 4687 if (json.has("network")) 4688 res.setNetwork(parseIdentifier(json.getAsJsonObject("network"))); 4689 if (json.has("contract")) { 4690 JsonArray array = json.getAsJsonArray("contract"); 4691 for (int i = 0; i < array.size(); i++) { 4692 res.getContract().add(parseReference(array.get(i).getAsJsonObject())); 4693 } 4694 } 4695 ; 4696 return res; 4697 } 4698 4699 protected DataElement parseDataElement(JsonObject json) throws IOException, FHIRFormatError { 4700 DataElement res = new DataElement(); 4701 parseDomainResourceProperties(json, res); 4702 if (json.has("url")) 4703 res.setUrlElement(parseUri(json.get("url").getAsString())); 4704 if (json.has("_url")) 4705 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 4706 if (json.has("identifier")) { 4707 JsonArray array = json.getAsJsonArray("identifier"); 4708 for (int i = 0; i < array.size(); i++) { 4709 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 4710 } 4711 } 4712 ; 4713 if (json.has("version")) 4714 res.setVersionElement(parseString(json.get("version").getAsString())); 4715 if (json.has("_version")) 4716 parseElementProperties(json.getAsJsonObject("_version"), res.getVersionElement()); 4717 if (json.has("name")) 4718 res.setNameElement(parseString(json.get("name").getAsString())); 4719 if (json.has("_name")) 4720 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 4721 if (json.has("status")) 4722 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 4723 Enumerations.ConformanceResourceStatus.NULL, new Enumerations.ConformanceResourceStatusEnumFactory())); 4724 if (json.has("_status")) 4725 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 4726 if (json.has("experimental")) 4727 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 4728 if (json.has("_experimental")) 4729 parseElementProperties(json.getAsJsonObject("_experimental"), res.getExperimentalElement()); 4730 if (json.has("publisher")) 4731 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 4732 if (json.has("_publisher")) 4733 parseElementProperties(json.getAsJsonObject("_publisher"), res.getPublisherElement()); 4734 if (json.has("contact")) { 4735 JsonArray array = json.getAsJsonArray("contact"); 4736 for (int i = 0; i < array.size(); i++) { 4737 res.getContact().add(parseDataElementDataElementContactComponent(array.get(i).getAsJsonObject(), res)); 4738 } 4739 } 4740 ; 4741 if (json.has("date")) 4742 res.setDateElement(parseDateTime(json.get("date").getAsString())); 4743 if (json.has("_date")) 4744 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 4745 if (json.has("useContext")) { 4746 JsonArray array = json.getAsJsonArray("useContext"); 4747 for (int i = 0; i < array.size(); i++) { 4748 res.getUseContext().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4749 } 4750 } 4751 ; 4752 if (json.has("copyright")) 4753 res.setCopyrightElement(parseString(json.get("copyright").getAsString())); 4754 if (json.has("_copyright")) 4755 parseElementProperties(json.getAsJsonObject("_copyright"), res.getCopyrightElement()); 4756 if (json.has("stringency")) 4757 res.setStringencyElement(parseEnumeration(json.get("stringency").getAsString(), 4758 DataElement.DataElementStringency.NULL, new DataElement.DataElementStringencyEnumFactory())); 4759 if (json.has("_stringency")) 4760 parseElementProperties(json.getAsJsonObject("_stringency"), res.getStringencyElement()); 4761 if (json.has("mapping")) { 4762 JsonArray array = json.getAsJsonArray("mapping"); 4763 for (int i = 0; i < array.size(); i++) { 4764 res.getMapping().add(parseDataElementDataElementMappingComponent(array.get(i).getAsJsonObject(), res)); 4765 } 4766 } 4767 ; 4768 if (json.has("element")) { 4769 JsonArray array = json.getAsJsonArray("element"); 4770 for (int i = 0; i < array.size(); i++) { 4771 res.getElement().add(parseElementDefinition(array.get(i).getAsJsonObject())); 4772 } 4773 } 4774 ; 4775 return res; 4776 } 4777 4778 protected DataElement.DataElementContactComponent parseDataElementDataElementContactComponent(JsonObject json, 4779 DataElement owner) throws IOException, FHIRFormatError { 4780 DataElement.DataElementContactComponent res = new DataElement.DataElementContactComponent(); 4781 parseBackboneProperties(json, res); 4782 if (json.has("name")) 4783 res.setNameElement(parseString(json.get("name").getAsString())); 4784 if (json.has("_name")) 4785 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 4786 if (json.has("telecom")) { 4787 JsonArray array = json.getAsJsonArray("telecom"); 4788 for (int i = 0; i < array.size(); i++) { 4789 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 4790 } 4791 } 4792 ; 4793 return res; 4794 } 4795 4796 protected DataElement.DataElementMappingComponent parseDataElementDataElementMappingComponent(JsonObject json, 4797 DataElement owner) throws IOException, FHIRFormatError { 4798 DataElement.DataElementMappingComponent res = new DataElement.DataElementMappingComponent(); 4799 parseBackboneProperties(json, res); 4800 if (json.has("identity")) 4801 res.setIdentityElement(parseId(json.get("identity").getAsString())); 4802 if (json.has("_identity")) 4803 parseElementProperties(json.getAsJsonObject("_identity"), res.getIdentityElement()); 4804 if (json.has("uri")) 4805 res.setUriElement(parseUri(json.get("uri").getAsString())); 4806 if (json.has("_uri")) 4807 parseElementProperties(json.getAsJsonObject("_uri"), res.getUriElement()); 4808 if (json.has("name")) 4809 res.setNameElement(parseString(json.get("name").getAsString())); 4810 if (json.has("_name")) 4811 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 4812 if (json.has("comments")) 4813 res.setCommentsElement(parseString(json.get("comments").getAsString())); 4814 if (json.has("_comments")) 4815 parseElementProperties(json.getAsJsonObject("_comments"), res.getCommentsElement()); 4816 return res; 4817 } 4818 4819 protected DetectedIssue parseDetectedIssue(JsonObject json) throws IOException, FHIRFormatError { 4820 DetectedIssue res = new DetectedIssue(); 4821 parseDomainResourceProperties(json, res); 4822 if (json.has("patient")) 4823 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 4824 if (json.has("category")) 4825 res.setCategory(parseCodeableConcept(json.getAsJsonObject("category"))); 4826 if (json.has("severity")) 4827 res.setSeverityElement(parseEnumeration(json.get("severity").getAsString(), 4828 DetectedIssue.DetectedIssueSeverity.NULL, new DetectedIssue.DetectedIssueSeverityEnumFactory())); 4829 if (json.has("_severity")) 4830 parseElementProperties(json.getAsJsonObject("_severity"), res.getSeverityElement()); 4831 if (json.has("implicated")) { 4832 JsonArray array = json.getAsJsonArray("implicated"); 4833 for (int i = 0; i < array.size(); i++) { 4834 res.getImplicated().add(parseReference(array.get(i).getAsJsonObject())); 4835 } 4836 } 4837 ; 4838 if (json.has("detail")) 4839 res.setDetailElement(parseString(json.get("detail").getAsString())); 4840 if (json.has("_detail")) 4841 parseElementProperties(json.getAsJsonObject("_detail"), res.getDetailElement()); 4842 if (json.has("date")) 4843 res.setDateElement(parseDateTime(json.get("date").getAsString())); 4844 if (json.has("_date")) 4845 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 4846 if (json.has("author")) 4847 res.setAuthor(parseReference(json.getAsJsonObject("author"))); 4848 if (json.has("identifier")) 4849 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 4850 if (json.has("reference")) 4851 res.setReferenceElement(parseUri(json.get("reference").getAsString())); 4852 if (json.has("_reference")) 4853 parseElementProperties(json.getAsJsonObject("_reference"), res.getReferenceElement()); 4854 if (json.has("mitigation")) { 4855 JsonArray array = json.getAsJsonArray("mitigation"); 4856 for (int i = 0; i < array.size(); i++) { 4857 res.getMitigation() 4858 .add(parseDetectedIssueDetectedIssueMitigationComponent(array.get(i).getAsJsonObject(), res)); 4859 } 4860 } 4861 ; 4862 return res; 4863 } 4864 4865 protected DetectedIssue.DetectedIssueMitigationComponent parseDetectedIssueDetectedIssueMitigationComponent( 4866 JsonObject json, DetectedIssue owner) throws IOException, FHIRFormatError { 4867 DetectedIssue.DetectedIssueMitigationComponent res = new DetectedIssue.DetectedIssueMitigationComponent(); 4868 parseBackboneProperties(json, res); 4869 if (json.has("action")) 4870 res.setAction(parseCodeableConcept(json.getAsJsonObject("action"))); 4871 if (json.has("date")) 4872 res.setDateElement(parseDateTime(json.get("date").getAsString())); 4873 if (json.has("_date")) 4874 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 4875 if (json.has("author")) 4876 res.setAuthor(parseReference(json.getAsJsonObject("author"))); 4877 return res; 4878 } 4879 4880 protected Device parseDevice(JsonObject json) throws IOException, FHIRFormatError { 4881 Device res = new Device(); 4882 parseDomainResourceProperties(json, res); 4883 if (json.has("identifier")) { 4884 JsonArray array = json.getAsJsonArray("identifier"); 4885 for (int i = 0; i < array.size(); i++) { 4886 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 4887 } 4888 } 4889 ; 4890 if (json.has("type")) 4891 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 4892 if (json.has("note")) { 4893 JsonArray array = json.getAsJsonArray("note"); 4894 for (int i = 0; i < array.size(); i++) { 4895 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 4896 } 4897 } 4898 ; 4899 if (json.has("status")) 4900 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Device.DeviceStatus.NULL, 4901 new Device.DeviceStatusEnumFactory())); 4902 if (json.has("_status")) 4903 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 4904 if (json.has("manufacturer")) 4905 res.setManufacturerElement(parseString(json.get("manufacturer").getAsString())); 4906 if (json.has("_manufacturer")) 4907 parseElementProperties(json.getAsJsonObject("_manufacturer"), res.getManufacturerElement()); 4908 if (json.has("model")) 4909 res.setModelElement(parseString(json.get("model").getAsString())); 4910 if (json.has("_model")) 4911 parseElementProperties(json.getAsJsonObject("_model"), res.getModelElement()); 4912 if (json.has("version")) 4913 res.setVersionElement(parseString(json.get("version").getAsString())); 4914 if (json.has("_version")) 4915 parseElementProperties(json.getAsJsonObject("_version"), res.getVersionElement()); 4916 if (json.has("manufactureDate")) 4917 res.setManufactureDateElement(parseDateTime(json.get("manufactureDate").getAsString())); 4918 if (json.has("_manufactureDate")) 4919 parseElementProperties(json.getAsJsonObject("_manufactureDate"), res.getManufactureDateElement()); 4920 if (json.has("expiry")) 4921 res.setExpiryElement(parseDateTime(json.get("expiry").getAsString())); 4922 if (json.has("_expiry")) 4923 parseElementProperties(json.getAsJsonObject("_expiry"), res.getExpiryElement()); 4924 if (json.has("udi")) 4925 res.setUdiElement(parseString(json.get("udi").getAsString())); 4926 if (json.has("_udi")) 4927 parseElementProperties(json.getAsJsonObject("_udi"), res.getUdiElement()); 4928 if (json.has("lotNumber")) 4929 res.setLotNumberElement(parseString(json.get("lotNumber").getAsString())); 4930 if (json.has("_lotNumber")) 4931 parseElementProperties(json.getAsJsonObject("_lotNumber"), res.getLotNumberElement()); 4932 if (json.has("owner")) 4933 res.setOwner(parseReference(json.getAsJsonObject("owner"))); 4934 if (json.has("location")) 4935 res.setLocation(parseReference(json.getAsJsonObject("location"))); 4936 if (json.has("patient")) 4937 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 4938 if (json.has("contact")) { 4939 JsonArray array = json.getAsJsonArray("contact"); 4940 for (int i = 0; i < array.size(); i++) { 4941 res.getContact().add(parseContactPoint(array.get(i).getAsJsonObject())); 4942 } 4943 } 4944 ; 4945 if (json.has("url")) 4946 res.setUrlElement(parseUri(json.get("url").getAsString())); 4947 if (json.has("_url")) 4948 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 4949 return res; 4950 } 4951 4952 protected DeviceComponent parseDeviceComponent(JsonObject json) throws IOException, FHIRFormatError { 4953 DeviceComponent res = new DeviceComponent(); 4954 parseDomainResourceProperties(json, res); 4955 if (json.has("type")) 4956 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 4957 if (json.has("identifier")) 4958 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 4959 if (json.has("lastSystemChange")) 4960 res.setLastSystemChangeElement(parseInstant(json.get("lastSystemChange").getAsString())); 4961 if (json.has("_lastSystemChange")) 4962 parseElementProperties(json.getAsJsonObject("_lastSystemChange"), res.getLastSystemChangeElement()); 4963 if (json.has("source")) 4964 res.setSource(parseReference(json.getAsJsonObject("source"))); 4965 if (json.has("parent")) 4966 res.setParent(parseReference(json.getAsJsonObject("parent"))); 4967 if (json.has("operationalStatus")) { 4968 JsonArray array = json.getAsJsonArray("operationalStatus"); 4969 for (int i = 0; i < array.size(); i++) { 4970 res.getOperationalStatus().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 4971 } 4972 } 4973 ; 4974 if (json.has("parameterGroup")) 4975 res.setParameterGroup(parseCodeableConcept(json.getAsJsonObject("parameterGroup"))); 4976 if (json.has("measurementPrinciple")) 4977 res.setMeasurementPrincipleElement(parseEnumeration(json.get("measurementPrinciple").getAsString(), 4978 DeviceComponent.MeasmntPrinciple.NULL, new DeviceComponent.MeasmntPrincipleEnumFactory())); 4979 if (json.has("_measurementPrinciple")) 4980 parseElementProperties(json.getAsJsonObject("_measurementPrinciple"), res.getMeasurementPrincipleElement()); 4981 if (json.has("productionSpecification")) { 4982 JsonArray array = json.getAsJsonArray("productionSpecification"); 4983 for (int i = 0; i < array.size(); i++) { 4984 res.getProductionSpecification().add( 4985 parseDeviceComponentDeviceComponentProductionSpecificationComponent(array.get(i).getAsJsonObject(), res)); 4986 } 4987 } 4988 ; 4989 if (json.has("languageCode")) 4990 res.setLanguageCode(parseCodeableConcept(json.getAsJsonObject("languageCode"))); 4991 return res; 4992 } 4993 4994 protected DeviceComponent.DeviceComponentProductionSpecificationComponent parseDeviceComponentDeviceComponentProductionSpecificationComponent( 4995 JsonObject json, DeviceComponent owner) throws IOException, FHIRFormatError { 4996 DeviceComponent.DeviceComponentProductionSpecificationComponent res = new DeviceComponent.DeviceComponentProductionSpecificationComponent(); 4997 parseBackboneProperties(json, res); 4998 if (json.has("specType")) 4999 res.setSpecType(parseCodeableConcept(json.getAsJsonObject("specType"))); 5000 if (json.has("componentId")) 5001 res.setComponentId(parseIdentifier(json.getAsJsonObject("componentId"))); 5002 if (json.has("productionSpec")) 5003 res.setProductionSpecElement(parseString(json.get("productionSpec").getAsString())); 5004 if (json.has("_productionSpec")) 5005 parseElementProperties(json.getAsJsonObject("_productionSpec"), res.getProductionSpecElement()); 5006 return res; 5007 } 5008 5009 protected DeviceMetric parseDeviceMetric(JsonObject json) throws IOException, FHIRFormatError { 5010 DeviceMetric res = new DeviceMetric(); 5011 parseDomainResourceProperties(json, res); 5012 if (json.has("type")) 5013 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 5014 if (json.has("identifier")) 5015 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 5016 if (json.has("unit")) 5017 res.setUnit(parseCodeableConcept(json.getAsJsonObject("unit"))); 5018 if (json.has("source")) 5019 res.setSource(parseReference(json.getAsJsonObject("source"))); 5020 if (json.has("parent")) 5021 res.setParent(parseReference(json.getAsJsonObject("parent"))); 5022 if (json.has("operationalStatus")) 5023 res.setOperationalStatusElement( 5024 parseEnumeration(json.get("operationalStatus").getAsString(), DeviceMetric.DeviceMetricOperationalStatus.NULL, 5025 new DeviceMetric.DeviceMetricOperationalStatusEnumFactory())); 5026 if (json.has("_operationalStatus")) 5027 parseElementProperties(json.getAsJsonObject("_operationalStatus"), res.getOperationalStatusElement()); 5028 if (json.has("color")) 5029 res.setColorElement(parseEnumeration(json.get("color").getAsString(), DeviceMetric.DeviceMetricColor.NULL, 5030 new DeviceMetric.DeviceMetricColorEnumFactory())); 5031 if (json.has("_color")) 5032 parseElementProperties(json.getAsJsonObject("_color"), res.getColorElement()); 5033 if (json.has("category")) 5034 res.setCategoryElement(parseEnumeration(json.get("category").getAsString(), 5035 DeviceMetric.DeviceMetricCategory.NULL, new DeviceMetric.DeviceMetricCategoryEnumFactory())); 5036 if (json.has("_category")) 5037 parseElementProperties(json.getAsJsonObject("_category"), res.getCategoryElement()); 5038 if (json.has("measurementPeriod")) 5039 res.setMeasurementPeriod(parseTiming(json.getAsJsonObject("measurementPeriod"))); 5040 if (json.has("calibration")) { 5041 JsonArray array = json.getAsJsonArray("calibration"); 5042 for (int i = 0; i < array.size(); i++) { 5043 res.getCalibration() 5044 .add(parseDeviceMetricDeviceMetricCalibrationComponent(array.get(i).getAsJsonObject(), res)); 5045 } 5046 } 5047 ; 5048 return res; 5049 } 5050 5051 protected DeviceMetric.DeviceMetricCalibrationComponent parseDeviceMetricDeviceMetricCalibrationComponent( 5052 JsonObject json, DeviceMetric owner) throws IOException, FHIRFormatError { 5053 DeviceMetric.DeviceMetricCalibrationComponent res = new DeviceMetric.DeviceMetricCalibrationComponent(); 5054 parseBackboneProperties(json, res); 5055 if (json.has("type")) 5056 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), DeviceMetric.DeviceMetricCalibrationType.NULL, 5057 new DeviceMetric.DeviceMetricCalibrationTypeEnumFactory())); 5058 if (json.has("_type")) 5059 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 5060 if (json.has("state")) 5061 res.setStateElement(parseEnumeration(json.get("state").getAsString(), 5062 DeviceMetric.DeviceMetricCalibrationState.NULL, new DeviceMetric.DeviceMetricCalibrationStateEnumFactory())); 5063 if (json.has("_state")) 5064 parseElementProperties(json.getAsJsonObject("_state"), res.getStateElement()); 5065 if (json.has("time")) 5066 res.setTimeElement(parseInstant(json.get("time").getAsString())); 5067 if (json.has("_time")) 5068 parseElementProperties(json.getAsJsonObject("_time"), res.getTimeElement()); 5069 return res; 5070 } 5071 5072 protected DeviceUseRequest parseDeviceUseRequest(JsonObject json) throws IOException, FHIRFormatError { 5073 DeviceUseRequest res = new DeviceUseRequest(); 5074 parseDomainResourceProperties(json, res); 5075 Type bodySite = parseType("bodySite", json); 5076 if (bodySite != null) 5077 res.setBodySite(bodySite); 5078 if (json.has("status")) 5079 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 5080 DeviceUseRequest.DeviceUseRequestStatus.NULL, new DeviceUseRequest.DeviceUseRequestStatusEnumFactory())); 5081 if (json.has("_status")) 5082 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 5083 if (json.has("device")) 5084 res.setDevice(parseReference(json.getAsJsonObject("device"))); 5085 if (json.has("encounter")) 5086 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 5087 if (json.has("identifier")) { 5088 JsonArray array = json.getAsJsonArray("identifier"); 5089 for (int i = 0; i < array.size(); i++) { 5090 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 5091 } 5092 } 5093 ; 5094 if (json.has("indication")) { 5095 JsonArray array = json.getAsJsonArray("indication"); 5096 for (int i = 0; i < array.size(); i++) { 5097 res.getIndication().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5098 } 5099 } 5100 ; 5101 if (json.has("notes")) { 5102 JsonArray array = json.getAsJsonArray("notes"); 5103 for (int i = 0; i < array.size(); i++) { 5104 res.getNotes().add(parseString(array.get(i).getAsString())); 5105 } 5106 } 5107 ; 5108 if (json.has("_notes")) { 5109 JsonArray array = json.getAsJsonArray("_notes"); 5110 for (int i = 0; i < array.size(); i++) { 5111 if (i == res.getNotes().size()) 5112 res.getNotes().add(parseString(null)); 5113 if (array.get(i) instanceof JsonObject) 5114 parseElementProperties(array.get(i).getAsJsonObject(), res.getNotes().get(i)); 5115 } 5116 } 5117 ; 5118 if (json.has("prnReason")) { 5119 JsonArray array = json.getAsJsonArray("prnReason"); 5120 for (int i = 0; i < array.size(); i++) { 5121 res.getPrnReason().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5122 } 5123 } 5124 ; 5125 if (json.has("orderedOn")) 5126 res.setOrderedOnElement(parseDateTime(json.get("orderedOn").getAsString())); 5127 if (json.has("_orderedOn")) 5128 parseElementProperties(json.getAsJsonObject("_orderedOn"), res.getOrderedOnElement()); 5129 if (json.has("recordedOn")) 5130 res.setRecordedOnElement(parseDateTime(json.get("recordedOn").getAsString())); 5131 if (json.has("_recordedOn")) 5132 parseElementProperties(json.getAsJsonObject("_recordedOn"), res.getRecordedOnElement()); 5133 if (json.has("subject")) 5134 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 5135 Type timing = parseType("timing", json); 5136 if (timing != null) 5137 res.setTiming(timing); 5138 if (json.has("priority")) 5139 res.setPriorityElement(parseEnumeration(json.get("priority").getAsString(), 5140 DeviceUseRequest.DeviceUseRequestPriority.NULL, new DeviceUseRequest.DeviceUseRequestPriorityEnumFactory())); 5141 if (json.has("_priority")) 5142 parseElementProperties(json.getAsJsonObject("_priority"), res.getPriorityElement()); 5143 return res; 5144 } 5145 5146 protected DeviceUseStatement parseDeviceUseStatement(JsonObject json) throws IOException, FHIRFormatError { 5147 DeviceUseStatement res = new DeviceUseStatement(); 5148 parseDomainResourceProperties(json, res); 5149 Type bodySite = parseType("bodySite", json); 5150 if (bodySite != null) 5151 res.setBodySite(bodySite); 5152 if (json.has("whenUsed")) 5153 res.setWhenUsed(parsePeriod(json.getAsJsonObject("whenUsed"))); 5154 if (json.has("device")) 5155 res.setDevice(parseReference(json.getAsJsonObject("device"))); 5156 if (json.has("identifier")) { 5157 JsonArray array = json.getAsJsonArray("identifier"); 5158 for (int i = 0; i < array.size(); i++) { 5159 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 5160 } 5161 } 5162 ; 5163 if (json.has("indication")) { 5164 JsonArray array = json.getAsJsonArray("indication"); 5165 for (int i = 0; i < array.size(); i++) { 5166 res.getIndication().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5167 } 5168 } 5169 ; 5170 if (json.has("notes")) { 5171 JsonArray array = json.getAsJsonArray("notes"); 5172 for (int i = 0; i < array.size(); i++) { 5173 res.getNotes().add(parseString(array.get(i).getAsString())); 5174 } 5175 } 5176 ; 5177 if (json.has("_notes")) { 5178 JsonArray array = json.getAsJsonArray("_notes"); 5179 for (int i = 0; i < array.size(); i++) { 5180 if (i == res.getNotes().size()) 5181 res.getNotes().add(parseString(null)); 5182 if (array.get(i) instanceof JsonObject) 5183 parseElementProperties(array.get(i).getAsJsonObject(), res.getNotes().get(i)); 5184 } 5185 } 5186 ; 5187 if (json.has("recordedOn")) 5188 res.setRecordedOnElement(parseDateTime(json.get("recordedOn").getAsString())); 5189 if (json.has("_recordedOn")) 5190 parseElementProperties(json.getAsJsonObject("_recordedOn"), res.getRecordedOnElement()); 5191 if (json.has("subject")) 5192 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 5193 Type timing = parseType("timing", json); 5194 if (timing != null) 5195 res.setTiming(timing); 5196 return res; 5197 } 5198 5199 protected DiagnosticOrder parseDiagnosticOrder(JsonObject json) throws IOException, FHIRFormatError { 5200 DiagnosticOrder res = new DiagnosticOrder(); 5201 parseDomainResourceProperties(json, res); 5202 if (json.has("subject")) 5203 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 5204 if (json.has("orderer")) 5205 res.setOrderer(parseReference(json.getAsJsonObject("orderer"))); 5206 if (json.has("identifier")) { 5207 JsonArray array = json.getAsJsonArray("identifier"); 5208 for (int i = 0; i < array.size(); i++) { 5209 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 5210 } 5211 } 5212 ; 5213 if (json.has("encounter")) 5214 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 5215 if (json.has("reason")) { 5216 JsonArray array = json.getAsJsonArray("reason"); 5217 for (int i = 0; i < array.size(); i++) { 5218 res.getReason().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5219 } 5220 } 5221 ; 5222 if (json.has("supportingInformation")) { 5223 JsonArray array = json.getAsJsonArray("supportingInformation"); 5224 for (int i = 0; i < array.size(); i++) { 5225 res.getSupportingInformation().add(parseReference(array.get(i).getAsJsonObject())); 5226 } 5227 } 5228 ; 5229 if (json.has("specimen")) { 5230 JsonArray array = json.getAsJsonArray("specimen"); 5231 for (int i = 0; i < array.size(); i++) { 5232 res.getSpecimen().add(parseReference(array.get(i).getAsJsonObject())); 5233 } 5234 } 5235 ; 5236 if (json.has("status")) 5237 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 5238 DiagnosticOrder.DiagnosticOrderStatus.NULL, new DiagnosticOrder.DiagnosticOrderStatusEnumFactory())); 5239 if (json.has("_status")) 5240 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 5241 if (json.has("priority")) 5242 res.setPriorityElement(parseEnumeration(json.get("priority").getAsString(), 5243 DiagnosticOrder.DiagnosticOrderPriority.NULL, new DiagnosticOrder.DiagnosticOrderPriorityEnumFactory())); 5244 if (json.has("_priority")) 5245 parseElementProperties(json.getAsJsonObject("_priority"), res.getPriorityElement()); 5246 if (json.has("event")) { 5247 JsonArray array = json.getAsJsonArray("event"); 5248 for (int i = 0; i < array.size(); i++) { 5249 res.getEvent().add(parseDiagnosticOrderDiagnosticOrderEventComponent(array.get(i).getAsJsonObject(), res)); 5250 } 5251 } 5252 ; 5253 if (json.has("item")) { 5254 JsonArray array = json.getAsJsonArray("item"); 5255 for (int i = 0; i < array.size(); i++) { 5256 res.getItem().add(parseDiagnosticOrderDiagnosticOrderItemComponent(array.get(i).getAsJsonObject(), res)); 5257 } 5258 } 5259 ; 5260 if (json.has("note")) { 5261 JsonArray array = json.getAsJsonArray("note"); 5262 for (int i = 0; i < array.size(); i++) { 5263 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 5264 } 5265 } 5266 ; 5267 return res; 5268 } 5269 5270 protected DiagnosticOrder.DiagnosticOrderEventComponent parseDiagnosticOrderDiagnosticOrderEventComponent( 5271 JsonObject json, DiagnosticOrder owner) throws IOException, FHIRFormatError { 5272 DiagnosticOrder.DiagnosticOrderEventComponent res = new DiagnosticOrder.DiagnosticOrderEventComponent(); 5273 parseBackboneProperties(json, res); 5274 if (json.has("status")) 5275 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 5276 DiagnosticOrder.DiagnosticOrderStatus.NULL, new DiagnosticOrder.DiagnosticOrderStatusEnumFactory())); 5277 if (json.has("_status")) 5278 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 5279 if (json.has("description")) 5280 res.setDescription(parseCodeableConcept(json.getAsJsonObject("description"))); 5281 if (json.has("dateTime")) 5282 res.setDateTimeElement(parseDateTime(json.get("dateTime").getAsString())); 5283 if (json.has("_dateTime")) 5284 parseElementProperties(json.getAsJsonObject("_dateTime"), res.getDateTimeElement()); 5285 if (json.has("actor")) 5286 res.setActor(parseReference(json.getAsJsonObject("actor"))); 5287 return res; 5288 } 5289 5290 protected DiagnosticOrder.DiagnosticOrderItemComponent parseDiagnosticOrderDiagnosticOrderItemComponent( 5291 JsonObject json, DiagnosticOrder owner) throws IOException, FHIRFormatError { 5292 DiagnosticOrder.DiagnosticOrderItemComponent res = new DiagnosticOrder.DiagnosticOrderItemComponent(); 5293 parseBackboneProperties(json, res); 5294 if (json.has("code")) 5295 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 5296 if (json.has("specimen")) { 5297 JsonArray array = json.getAsJsonArray("specimen"); 5298 for (int i = 0; i < array.size(); i++) { 5299 res.getSpecimen().add(parseReference(array.get(i).getAsJsonObject())); 5300 } 5301 } 5302 ; 5303 if (json.has("bodySite")) 5304 res.setBodySite(parseCodeableConcept(json.getAsJsonObject("bodySite"))); 5305 if (json.has("status")) 5306 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 5307 DiagnosticOrder.DiagnosticOrderStatus.NULL, new DiagnosticOrder.DiagnosticOrderStatusEnumFactory())); 5308 if (json.has("_status")) 5309 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 5310 if (json.has("event")) { 5311 JsonArray array = json.getAsJsonArray("event"); 5312 for (int i = 0; i < array.size(); i++) { 5313 res.getEvent().add(parseDiagnosticOrderDiagnosticOrderEventComponent(array.get(i).getAsJsonObject(), owner)); 5314 } 5315 } 5316 ; 5317 return res; 5318 } 5319 5320 protected DiagnosticReport parseDiagnosticReport(JsonObject json) throws IOException, FHIRFormatError { 5321 DiagnosticReport res = new DiagnosticReport(); 5322 parseDomainResourceProperties(json, res); 5323 if (json.has("identifier")) { 5324 JsonArray array = json.getAsJsonArray("identifier"); 5325 for (int i = 0; i < array.size(); i++) { 5326 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 5327 } 5328 } 5329 ; 5330 if (json.has("status")) 5331 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 5332 DiagnosticReport.DiagnosticReportStatus.NULL, new DiagnosticReport.DiagnosticReportStatusEnumFactory())); 5333 if (json.has("_status")) 5334 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 5335 if (json.has("category")) 5336 res.setCategory(parseCodeableConcept(json.getAsJsonObject("category"))); 5337 if (json.has("code")) 5338 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 5339 if (json.has("subject")) 5340 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 5341 if (json.has("encounter")) 5342 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 5343 Type effective = parseType("effective", json); 5344 if (effective != null) 5345 res.setEffective(effective); 5346 if (json.has("issued")) 5347 res.setIssuedElement(parseInstant(json.get("issued").getAsString())); 5348 if (json.has("_issued")) 5349 parseElementProperties(json.getAsJsonObject("_issued"), res.getIssuedElement()); 5350 if (json.has("performer")) 5351 res.setPerformer(parseReference(json.getAsJsonObject("performer"))); 5352 if (json.has("request")) { 5353 JsonArray array = json.getAsJsonArray("request"); 5354 for (int i = 0; i < array.size(); i++) { 5355 res.getRequest().add(parseReference(array.get(i).getAsJsonObject())); 5356 } 5357 } 5358 ; 5359 if (json.has("specimen")) { 5360 JsonArray array = json.getAsJsonArray("specimen"); 5361 for (int i = 0; i < array.size(); i++) { 5362 res.getSpecimen().add(parseReference(array.get(i).getAsJsonObject())); 5363 } 5364 } 5365 ; 5366 if (json.has("result")) { 5367 JsonArray array = json.getAsJsonArray("result"); 5368 for (int i = 0; i < array.size(); i++) { 5369 res.getResult().add(parseReference(array.get(i).getAsJsonObject())); 5370 } 5371 } 5372 ; 5373 if (json.has("imagingStudy")) { 5374 JsonArray array = json.getAsJsonArray("imagingStudy"); 5375 for (int i = 0; i < array.size(); i++) { 5376 res.getImagingStudy().add(parseReference(array.get(i).getAsJsonObject())); 5377 } 5378 } 5379 ; 5380 if (json.has("image")) { 5381 JsonArray array = json.getAsJsonArray("image"); 5382 for (int i = 0; i < array.size(); i++) { 5383 res.getImage().add(parseDiagnosticReportDiagnosticReportImageComponent(array.get(i).getAsJsonObject(), res)); 5384 } 5385 } 5386 ; 5387 if (json.has("conclusion")) 5388 res.setConclusionElement(parseString(json.get("conclusion").getAsString())); 5389 if (json.has("_conclusion")) 5390 parseElementProperties(json.getAsJsonObject("_conclusion"), res.getConclusionElement()); 5391 if (json.has("codedDiagnosis")) { 5392 JsonArray array = json.getAsJsonArray("codedDiagnosis"); 5393 for (int i = 0; i < array.size(); i++) { 5394 res.getCodedDiagnosis().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5395 } 5396 } 5397 ; 5398 if (json.has("presentedForm")) { 5399 JsonArray array = json.getAsJsonArray("presentedForm"); 5400 for (int i = 0; i < array.size(); i++) { 5401 res.getPresentedForm().add(parseAttachment(array.get(i).getAsJsonObject())); 5402 } 5403 } 5404 ; 5405 return res; 5406 } 5407 5408 protected DiagnosticReport.DiagnosticReportImageComponent parseDiagnosticReportDiagnosticReportImageComponent( 5409 JsonObject json, DiagnosticReport owner) throws IOException, FHIRFormatError { 5410 DiagnosticReport.DiagnosticReportImageComponent res = new DiagnosticReport.DiagnosticReportImageComponent(); 5411 parseBackboneProperties(json, res); 5412 if (json.has("comment")) 5413 res.setCommentElement(parseString(json.get("comment").getAsString())); 5414 if (json.has("_comment")) 5415 parseElementProperties(json.getAsJsonObject("_comment"), res.getCommentElement()); 5416 if (json.has("link")) 5417 res.setLink(parseReference(json.getAsJsonObject("link"))); 5418 return res; 5419 } 5420 5421 protected DocumentManifest parseDocumentManifest(JsonObject json) throws IOException, FHIRFormatError { 5422 DocumentManifest res = new DocumentManifest(); 5423 parseDomainResourceProperties(json, res); 5424 if (json.has("masterIdentifier")) 5425 res.setMasterIdentifier(parseIdentifier(json.getAsJsonObject("masterIdentifier"))); 5426 if (json.has("identifier")) { 5427 JsonArray array = json.getAsJsonArray("identifier"); 5428 for (int i = 0; i < array.size(); i++) { 5429 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 5430 } 5431 } 5432 ; 5433 if (json.has("subject")) 5434 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 5435 if (json.has("recipient")) { 5436 JsonArray array = json.getAsJsonArray("recipient"); 5437 for (int i = 0; i < array.size(); i++) { 5438 res.getRecipient().add(parseReference(array.get(i).getAsJsonObject())); 5439 } 5440 } 5441 ; 5442 if (json.has("type")) 5443 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 5444 if (json.has("author")) { 5445 JsonArray array = json.getAsJsonArray("author"); 5446 for (int i = 0; i < array.size(); i++) { 5447 res.getAuthor().add(parseReference(array.get(i).getAsJsonObject())); 5448 } 5449 } 5450 ; 5451 if (json.has("created")) 5452 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 5453 if (json.has("_created")) 5454 parseElementProperties(json.getAsJsonObject("_created"), res.getCreatedElement()); 5455 if (json.has("source")) 5456 res.setSourceElement(parseUri(json.get("source").getAsString())); 5457 if (json.has("_source")) 5458 parseElementProperties(json.getAsJsonObject("_source"), res.getSourceElement()); 5459 if (json.has("status")) 5460 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.DocumentReferenceStatus.NULL, 5461 new Enumerations.DocumentReferenceStatusEnumFactory())); 5462 if (json.has("_status")) 5463 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 5464 if (json.has("description")) 5465 res.setDescriptionElement(parseString(json.get("description").getAsString())); 5466 if (json.has("_description")) 5467 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 5468 if (json.has("content")) { 5469 JsonArray array = json.getAsJsonArray("content"); 5470 for (int i = 0; i < array.size(); i++) { 5471 res.getContent() 5472 .add(parseDocumentManifestDocumentManifestContentComponent(array.get(i).getAsJsonObject(), res)); 5473 } 5474 } 5475 ; 5476 if (json.has("related")) { 5477 JsonArray array = json.getAsJsonArray("related"); 5478 for (int i = 0; i < array.size(); i++) { 5479 res.getRelated() 5480 .add(parseDocumentManifestDocumentManifestRelatedComponent(array.get(i).getAsJsonObject(), res)); 5481 } 5482 } 5483 ; 5484 return res; 5485 } 5486 5487 protected DocumentManifest.DocumentManifestContentComponent parseDocumentManifestDocumentManifestContentComponent( 5488 JsonObject json, DocumentManifest owner) throws IOException, FHIRFormatError { 5489 DocumentManifest.DocumentManifestContentComponent res = new DocumentManifest.DocumentManifestContentComponent(); 5490 parseBackboneProperties(json, res); 5491 Type p = parseType("p", json); 5492 if (p != null) 5493 res.setP(p); 5494 return res; 5495 } 5496 5497 protected DocumentManifest.DocumentManifestRelatedComponent parseDocumentManifestDocumentManifestRelatedComponent( 5498 JsonObject json, DocumentManifest owner) throws IOException, FHIRFormatError { 5499 DocumentManifest.DocumentManifestRelatedComponent res = new DocumentManifest.DocumentManifestRelatedComponent(); 5500 parseBackboneProperties(json, res); 5501 if (json.has("identifier")) 5502 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 5503 if (json.has("ref")) 5504 res.setRef(parseReference(json.getAsJsonObject("ref"))); 5505 return res; 5506 } 5507 5508 protected DocumentReference parseDocumentReference(JsonObject json) throws IOException, FHIRFormatError { 5509 DocumentReference res = new DocumentReference(); 5510 parseDomainResourceProperties(json, res); 5511 if (json.has("masterIdentifier")) 5512 res.setMasterIdentifier(parseIdentifier(json.getAsJsonObject("masterIdentifier"))); 5513 if (json.has("identifier")) { 5514 JsonArray array = json.getAsJsonArray("identifier"); 5515 for (int i = 0; i < array.size(); i++) { 5516 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 5517 } 5518 } 5519 ; 5520 if (json.has("subject")) 5521 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 5522 if (json.has("type")) 5523 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 5524 if (json.has("class")) 5525 res.setClass_(parseCodeableConcept(json.getAsJsonObject("class"))); 5526 if (json.has("author")) { 5527 JsonArray array = json.getAsJsonArray("author"); 5528 for (int i = 0; i < array.size(); i++) { 5529 res.getAuthor().add(parseReference(array.get(i).getAsJsonObject())); 5530 } 5531 } 5532 ; 5533 if (json.has("custodian")) 5534 res.setCustodian(parseReference(json.getAsJsonObject("custodian"))); 5535 if (json.has("authenticator")) 5536 res.setAuthenticator(parseReference(json.getAsJsonObject("authenticator"))); 5537 if (json.has("created")) 5538 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 5539 if (json.has("_created")) 5540 parseElementProperties(json.getAsJsonObject("_created"), res.getCreatedElement()); 5541 if (json.has("indexed")) 5542 res.setIndexedElement(parseInstant(json.get("indexed").getAsString())); 5543 if (json.has("_indexed")) 5544 parseElementProperties(json.getAsJsonObject("_indexed"), res.getIndexedElement()); 5545 if (json.has("status")) 5546 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Enumerations.DocumentReferenceStatus.NULL, 5547 new Enumerations.DocumentReferenceStatusEnumFactory())); 5548 if (json.has("_status")) 5549 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 5550 if (json.has("docStatus")) 5551 res.setDocStatus(parseCodeableConcept(json.getAsJsonObject("docStatus"))); 5552 if (json.has("relatesTo")) { 5553 JsonArray array = json.getAsJsonArray("relatesTo"); 5554 for (int i = 0; i < array.size(); i++) { 5555 res.getRelatesTo() 5556 .add(parseDocumentReferenceDocumentReferenceRelatesToComponent(array.get(i).getAsJsonObject(), res)); 5557 } 5558 } 5559 ; 5560 if (json.has("description")) 5561 res.setDescriptionElement(parseString(json.get("description").getAsString())); 5562 if (json.has("_description")) 5563 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 5564 if (json.has("securityLabel")) { 5565 JsonArray array = json.getAsJsonArray("securityLabel"); 5566 for (int i = 0; i < array.size(); i++) { 5567 res.getSecurityLabel().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5568 } 5569 } 5570 ; 5571 if (json.has("content")) { 5572 JsonArray array = json.getAsJsonArray("content"); 5573 for (int i = 0; i < array.size(); i++) { 5574 res.getContent() 5575 .add(parseDocumentReferenceDocumentReferenceContentComponent(array.get(i).getAsJsonObject(), res)); 5576 } 5577 } 5578 ; 5579 if (json.has("context")) 5580 res.setContext(parseDocumentReferenceDocumentReferenceContextComponent(json.getAsJsonObject("context"), res)); 5581 return res; 5582 } 5583 5584 protected DocumentReference.DocumentReferenceRelatesToComponent parseDocumentReferenceDocumentReferenceRelatesToComponent( 5585 JsonObject json, DocumentReference owner) throws IOException, FHIRFormatError { 5586 DocumentReference.DocumentReferenceRelatesToComponent res = new DocumentReference.DocumentReferenceRelatesToComponent(); 5587 parseBackboneProperties(json, res); 5588 if (json.has("code")) 5589 res.setCodeElement( 5590 parseEnumeration(json.get("code").getAsString(), DocumentReference.DocumentRelationshipType.NULL, 5591 new DocumentReference.DocumentRelationshipTypeEnumFactory())); 5592 if (json.has("_code")) 5593 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 5594 if (json.has("target")) 5595 res.setTarget(parseReference(json.getAsJsonObject("target"))); 5596 return res; 5597 } 5598 5599 protected DocumentReference.DocumentReferenceContentComponent parseDocumentReferenceDocumentReferenceContentComponent( 5600 JsonObject json, DocumentReference owner) throws IOException, FHIRFormatError { 5601 DocumentReference.DocumentReferenceContentComponent res = new DocumentReference.DocumentReferenceContentComponent(); 5602 parseBackboneProperties(json, res); 5603 if (json.has("attachment")) 5604 res.setAttachment(parseAttachment(json.getAsJsonObject("attachment"))); 5605 if (json.has("format")) { 5606 JsonArray array = json.getAsJsonArray("format"); 5607 for (int i = 0; i < array.size(); i++) { 5608 res.getFormat().add(parseCoding(array.get(i).getAsJsonObject())); 5609 } 5610 } 5611 ; 5612 return res; 5613 } 5614 5615 protected DocumentReference.DocumentReferenceContextComponent parseDocumentReferenceDocumentReferenceContextComponent( 5616 JsonObject json, DocumentReference owner) throws IOException, FHIRFormatError { 5617 DocumentReference.DocumentReferenceContextComponent res = new DocumentReference.DocumentReferenceContextComponent(); 5618 parseBackboneProperties(json, res); 5619 if (json.has("encounter")) 5620 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 5621 if (json.has("event")) { 5622 JsonArray array = json.getAsJsonArray("event"); 5623 for (int i = 0; i < array.size(); i++) { 5624 res.getEvent().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5625 } 5626 } 5627 ; 5628 if (json.has("period")) 5629 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 5630 if (json.has("facilityType")) 5631 res.setFacilityType(parseCodeableConcept(json.getAsJsonObject("facilityType"))); 5632 if (json.has("practiceSetting")) 5633 res.setPracticeSetting(parseCodeableConcept(json.getAsJsonObject("practiceSetting"))); 5634 if (json.has("sourcePatientInfo")) 5635 res.setSourcePatientInfo(parseReference(json.getAsJsonObject("sourcePatientInfo"))); 5636 if (json.has("related")) { 5637 JsonArray array = json.getAsJsonArray("related"); 5638 for (int i = 0; i < array.size(); i++) { 5639 res.getRelated() 5640 .add(parseDocumentReferenceDocumentReferenceContextRelatedComponent(array.get(i).getAsJsonObject(), owner)); 5641 } 5642 } 5643 ; 5644 return res; 5645 } 5646 5647 protected DocumentReference.DocumentReferenceContextRelatedComponent parseDocumentReferenceDocumentReferenceContextRelatedComponent( 5648 JsonObject json, DocumentReference owner) throws IOException, FHIRFormatError { 5649 DocumentReference.DocumentReferenceContextRelatedComponent res = new DocumentReference.DocumentReferenceContextRelatedComponent(); 5650 parseBackboneProperties(json, res); 5651 if (json.has("identifier")) 5652 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 5653 if (json.has("ref")) 5654 res.setRef(parseReference(json.getAsJsonObject("ref"))); 5655 return res; 5656 } 5657 5658 protected EligibilityRequest parseEligibilityRequest(JsonObject json) throws IOException, FHIRFormatError { 5659 EligibilityRequest res = new EligibilityRequest(); 5660 parseDomainResourceProperties(json, res); 5661 if (json.has("identifier")) { 5662 JsonArray array = json.getAsJsonArray("identifier"); 5663 for (int i = 0; i < array.size(); i++) { 5664 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 5665 } 5666 } 5667 ; 5668 if (json.has("ruleset")) 5669 res.setRuleset(parseCoding(json.getAsJsonObject("ruleset"))); 5670 if (json.has("originalRuleset")) 5671 res.setOriginalRuleset(parseCoding(json.getAsJsonObject("originalRuleset"))); 5672 if (json.has("created")) 5673 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 5674 if (json.has("_created")) 5675 parseElementProperties(json.getAsJsonObject("_created"), res.getCreatedElement()); 5676 if (json.has("target")) 5677 res.setTarget(parseReference(json.getAsJsonObject("target"))); 5678 if (json.has("provider")) 5679 res.setProvider(parseReference(json.getAsJsonObject("provider"))); 5680 if (json.has("organization")) 5681 res.setOrganization(parseReference(json.getAsJsonObject("organization"))); 5682 return res; 5683 } 5684 5685 protected EligibilityResponse parseEligibilityResponse(JsonObject json) throws IOException, FHIRFormatError { 5686 EligibilityResponse res = new EligibilityResponse(); 5687 parseDomainResourceProperties(json, res); 5688 if (json.has("identifier")) { 5689 JsonArray array = json.getAsJsonArray("identifier"); 5690 for (int i = 0; i < array.size(); i++) { 5691 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 5692 } 5693 } 5694 ; 5695 if (json.has("request")) 5696 res.setRequest(parseReference(json.getAsJsonObject("request"))); 5697 if (json.has("outcome")) 5698 res.setOutcomeElement(parseEnumeration(json.get("outcome").getAsString(), Enumerations.RemittanceOutcome.NULL, 5699 new Enumerations.RemittanceOutcomeEnumFactory())); 5700 if (json.has("_outcome")) 5701 parseElementProperties(json.getAsJsonObject("_outcome"), res.getOutcomeElement()); 5702 if (json.has("disposition")) 5703 res.setDispositionElement(parseString(json.get("disposition").getAsString())); 5704 if (json.has("_disposition")) 5705 parseElementProperties(json.getAsJsonObject("_disposition"), res.getDispositionElement()); 5706 if (json.has("ruleset")) 5707 res.setRuleset(parseCoding(json.getAsJsonObject("ruleset"))); 5708 if (json.has("originalRuleset")) 5709 res.setOriginalRuleset(parseCoding(json.getAsJsonObject("originalRuleset"))); 5710 if (json.has("created")) 5711 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 5712 if (json.has("_created")) 5713 parseElementProperties(json.getAsJsonObject("_created"), res.getCreatedElement()); 5714 if (json.has("organization")) 5715 res.setOrganization(parseReference(json.getAsJsonObject("organization"))); 5716 if (json.has("requestProvider")) 5717 res.setRequestProvider(parseReference(json.getAsJsonObject("requestProvider"))); 5718 if (json.has("requestOrganization")) 5719 res.setRequestOrganization(parseReference(json.getAsJsonObject("requestOrganization"))); 5720 return res; 5721 } 5722 5723 protected Encounter parseEncounter(JsonObject json) throws IOException, FHIRFormatError { 5724 Encounter res = new Encounter(); 5725 parseDomainResourceProperties(json, res); 5726 if (json.has("identifier")) { 5727 JsonArray array = json.getAsJsonArray("identifier"); 5728 for (int i = 0; i < array.size(); i++) { 5729 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 5730 } 5731 } 5732 ; 5733 if (json.has("status")) 5734 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Encounter.EncounterState.NULL, 5735 new Encounter.EncounterStateEnumFactory())); 5736 if (json.has("_status")) 5737 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 5738 if (json.has("statusHistory")) { 5739 JsonArray array = json.getAsJsonArray("statusHistory"); 5740 for (int i = 0; i < array.size(); i++) { 5741 res.getStatusHistory().add(parseEncounterEncounterStatusHistoryComponent(array.get(i).getAsJsonObject(), res)); 5742 } 5743 } 5744 ; 5745 if (json.has("class")) 5746 res.setClass_Element(parseEnumeration(json.get("class").getAsString(), Encounter.EncounterClass.NULL, 5747 new Encounter.EncounterClassEnumFactory())); 5748 if (json.has("_class")) 5749 parseElementProperties(json.getAsJsonObject("_class"), res.getClass_Element()); 5750 if (json.has("type")) { 5751 JsonArray array = json.getAsJsonArray("type"); 5752 for (int i = 0; i < array.size(); i++) { 5753 res.getType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5754 } 5755 } 5756 ; 5757 if (json.has("priority")) 5758 res.setPriority(parseCodeableConcept(json.getAsJsonObject("priority"))); 5759 if (json.has("patient")) 5760 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 5761 if (json.has("episodeOfCare")) { 5762 JsonArray array = json.getAsJsonArray("episodeOfCare"); 5763 for (int i = 0; i < array.size(); i++) { 5764 res.getEpisodeOfCare().add(parseReference(array.get(i).getAsJsonObject())); 5765 } 5766 } 5767 ; 5768 if (json.has("incomingReferral")) { 5769 JsonArray array = json.getAsJsonArray("incomingReferral"); 5770 for (int i = 0; i < array.size(); i++) { 5771 res.getIncomingReferral().add(parseReference(array.get(i).getAsJsonObject())); 5772 } 5773 } 5774 ; 5775 if (json.has("participant")) { 5776 JsonArray array = json.getAsJsonArray("participant"); 5777 for (int i = 0; i < array.size(); i++) { 5778 res.getParticipant().add(parseEncounterEncounterParticipantComponent(array.get(i).getAsJsonObject(), res)); 5779 } 5780 } 5781 ; 5782 if (json.has("appointment")) 5783 res.setAppointment(parseReference(json.getAsJsonObject("appointment"))); 5784 if (json.has("period")) 5785 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 5786 if (json.has("length")) 5787 res.setLength(parseDuration(json.getAsJsonObject("length"))); 5788 if (json.has("reason")) { 5789 JsonArray array = json.getAsJsonArray("reason"); 5790 for (int i = 0; i < array.size(); i++) { 5791 res.getReason().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5792 } 5793 } 5794 ; 5795 if (json.has("indication")) { 5796 JsonArray array = json.getAsJsonArray("indication"); 5797 for (int i = 0; i < array.size(); i++) { 5798 res.getIndication().add(parseReference(array.get(i).getAsJsonObject())); 5799 } 5800 } 5801 ; 5802 if (json.has("hospitalization")) 5803 res.setHospitalization( 5804 parseEncounterEncounterHospitalizationComponent(json.getAsJsonObject("hospitalization"), res)); 5805 if (json.has("location")) { 5806 JsonArray array = json.getAsJsonArray("location"); 5807 for (int i = 0; i < array.size(); i++) { 5808 res.getLocation().add(parseEncounterEncounterLocationComponent(array.get(i).getAsJsonObject(), res)); 5809 } 5810 } 5811 ; 5812 if (json.has("serviceProvider")) 5813 res.setServiceProvider(parseReference(json.getAsJsonObject("serviceProvider"))); 5814 if (json.has("partOf")) 5815 res.setPartOf(parseReference(json.getAsJsonObject("partOf"))); 5816 return res; 5817 } 5818 5819 protected Encounter.EncounterStatusHistoryComponent parseEncounterEncounterStatusHistoryComponent(JsonObject json, 5820 Encounter owner) throws IOException, FHIRFormatError { 5821 Encounter.EncounterStatusHistoryComponent res = new Encounter.EncounterStatusHistoryComponent(); 5822 parseBackboneProperties(json, res); 5823 if (json.has("status")) 5824 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Encounter.EncounterState.NULL, 5825 new Encounter.EncounterStateEnumFactory())); 5826 if (json.has("_status")) 5827 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 5828 if (json.has("period")) 5829 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 5830 return res; 5831 } 5832 5833 protected Encounter.EncounterParticipantComponent parseEncounterEncounterParticipantComponent(JsonObject json, 5834 Encounter owner) throws IOException, FHIRFormatError { 5835 Encounter.EncounterParticipantComponent res = new Encounter.EncounterParticipantComponent(); 5836 parseBackboneProperties(json, res); 5837 if (json.has("type")) { 5838 JsonArray array = json.getAsJsonArray("type"); 5839 for (int i = 0; i < array.size(); i++) { 5840 res.getType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5841 } 5842 } 5843 ; 5844 if (json.has("period")) 5845 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 5846 if (json.has("individual")) 5847 res.setIndividual(parseReference(json.getAsJsonObject("individual"))); 5848 return res; 5849 } 5850 5851 protected Encounter.EncounterHospitalizationComponent parseEncounterEncounterHospitalizationComponent(JsonObject json, 5852 Encounter owner) throws IOException, FHIRFormatError { 5853 Encounter.EncounterHospitalizationComponent res = new Encounter.EncounterHospitalizationComponent(); 5854 parseBackboneProperties(json, res); 5855 if (json.has("preAdmissionIdentifier")) 5856 res.setPreAdmissionIdentifier(parseIdentifier(json.getAsJsonObject("preAdmissionIdentifier"))); 5857 if (json.has("origin")) 5858 res.setOrigin(parseReference(json.getAsJsonObject("origin"))); 5859 if (json.has("admitSource")) 5860 res.setAdmitSource(parseCodeableConcept(json.getAsJsonObject("admitSource"))); 5861 if (json.has("admittingDiagnosis")) { 5862 JsonArray array = json.getAsJsonArray("admittingDiagnosis"); 5863 for (int i = 0; i < array.size(); i++) { 5864 res.getAdmittingDiagnosis().add(parseReference(array.get(i).getAsJsonObject())); 5865 } 5866 } 5867 ; 5868 if (json.has("reAdmission")) 5869 res.setReAdmission(parseCodeableConcept(json.getAsJsonObject("reAdmission"))); 5870 if (json.has("dietPreference")) { 5871 JsonArray array = json.getAsJsonArray("dietPreference"); 5872 for (int i = 0; i < array.size(); i++) { 5873 res.getDietPreference().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5874 } 5875 } 5876 ; 5877 if (json.has("specialCourtesy")) { 5878 JsonArray array = json.getAsJsonArray("specialCourtesy"); 5879 for (int i = 0; i < array.size(); i++) { 5880 res.getSpecialCourtesy().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5881 } 5882 } 5883 ; 5884 if (json.has("specialArrangement")) { 5885 JsonArray array = json.getAsJsonArray("specialArrangement"); 5886 for (int i = 0; i < array.size(); i++) { 5887 res.getSpecialArrangement().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 5888 } 5889 } 5890 ; 5891 if (json.has("destination")) 5892 res.setDestination(parseReference(json.getAsJsonObject("destination"))); 5893 if (json.has("dischargeDisposition")) 5894 res.setDischargeDisposition(parseCodeableConcept(json.getAsJsonObject("dischargeDisposition"))); 5895 if (json.has("dischargeDiagnosis")) { 5896 JsonArray array = json.getAsJsonArray("dischargeDiagnosis"); 5897 for (int i = 0; i < array.size(); i++) { 5898 res.getDischargeDiagnosis().add(parseReference(array.get(i).getAsJsonObject())); 5899 } 5900 } 5901 ; 5902 return res; 5903 } 5904 5905 protected Encounter.EncounterLocationComponent parseEncounterEncounterLocationComponent(JsonObject json, 5906 Encounter owner) throws IOException, FHIRFormatError { 5907 Encounter.EncounterLocationComponent res = new Encounter.EncounterLocationComponent(); 5908 parseBackboneProperties(json, res); 5909 if (json.has("location")) 5910 res.setLocation(parseReference(json.getAsJsonObject("location"))); 5911 if (json.has("status")) 5912 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Encounter.EncounterLocationStatus.NULL, 5913 new Encounter.EncounterLocationStatusEnumFactory())); 5914 if (json.has("_status")) 5915 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 5916 if (json.has("period")) 5917 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 5918 return res; 5919 } 5920 5921 protected EnrollmentRequest parseEnrollmentRequest(JsonObject json) throws IOException, FHIRFormatError { 5922 EnrollmentRequest res = new EnrollmentRequest(); 5923 parseDomainResourceProperties(json, res); 5924 if (json.has("identifier")) { 5925 JsonArray array = json.getAsJsonArray("identifier"); 5926 for (int i = 0; i < array.size(); i++) { 5927 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 5928 } 5929 } 5930 ; 5931 if (json.has("ruleset")) 5932 res.setRuleset(parseCoding(json.getAsJsonObject("ruleset"))); 5933 if (json.has("originalRuleset")) 5934 res.setOriginalRuleset(parseCoding(json.getAsJsonObject("originalRuleset"))); 5935 if (json.has("created")) 5936 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 5937 if (json.has("_created")) 5938 parseElementProperties(json.getAsJsonObject("_created"), res.getCreatedElement()); 5939 if (json.has("target")) 5940 res.setTarget(parseReference(json.getAsJsonObject("target"))); 5941 if (json.has("provider")) 5942 res.setProvider(parseReference(json.getAsJsonObject("provider"))); 5943 if (json.has("organization")) 5944 res.setOrganization(parseReference(json.getAsJsonObject("organization"))); 5945 if (json.has("subject")) 5946 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 5947 if (json.has("coverage")) 5948 res.setCoverage(parseReference(json.getAsJsonObject("coverage"))); 5949 if (json.has("relationship")) 5950 res.setRelationship(parseCoding(json.getAsJsonObject("relationship"))); 5951 return res; 5952 } 5953 5954 protected EnrollmentResponse parseEnrollmentResponse(JsonObject json) throws IOException, FHIRFormatError { 5955 EnrollmentResponse res = new EnrollmentResponse(); 5956 parseDomainResourceProperties(json, res); 5957 if (json.has("identifier")) { 5958 JsonArray array = json.getAsJsonArray("identifier"); 5959 for (int i = 0; i < array.size(); i++) { 5960 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 5961 } 5962 } 5963 ; 5964 if (json.has("request")) 5965 res.setRequest(parseReference(json.getAsJsonObject("request"))); 5966 if (json.has("outcome")) 5967 res.setOutcomeElement(parseEnumeration(json.get("outcome").getAsString(), Enumerations.RemittanceOutcome.NULL, 5968 new Enumerations.RemittanceOutcomeEnumFactory())); 5969 if (json.has("_outcome")) 5970 parseElementProperties(json.getAsJsonObject("_outcome"), res.getOutcomeElement()); 5971 if (json.has("disposition")) 5972 res.setDispositionElement(parseString(json.get("disposition").getAsString())); 5973 if (json.has("_disposition")) 5974 parseElementProperties(json.getAsJsonObject("_disposition"), res.getDispositionElement()); 5975 if (json.has("ruleset")) 5976 res.setRuleset(parseCoding(json.getAsJsonObject("ruleset"))); 5977 if (json.has("originalRuleset")) 5978 res.setOriginalRuleset(parseCoding(json.getAsJsonObject("originalRuleset"))); 5979 if (json.has("created")) 5980 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 5981 if (json.has("_created")) 5982 parseElementProperties(json.getAsJsonObject("_created"), res.getCreatedElement()); 5983 if (json.has("organization")) 5984 res.setOrganization(parseReference(json.getAsJsonObject("organization"))); 5985 if (json.has("requestProvider")) 5986 res.setRequestProvider(parseReference(json.getAsJsonObject("requestProvider"))); 5987 if (json.has("requestOrganization")) 5988 res.setRequestOrganization(parseReference(json.getAsJsonObject("requestOrganization"))); 5989 return res; 5990 } 5991 5992 protected EpisodeOfCare parseEpisodeOfCare(JsonObject json) throws IOException, FHIRFormatError { 5993 EpisodeOfCare res = new EpisodeOfCare(); 5994 parseDomainResourceProperties(json, res); 5995 if (json.has("identifier")) { 5996 JsonArray array = json.getAsJsonArray("identifier"); 5997 for (int i = 0; i < array.size(); i++) { 5998 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 5999 } 6000 } 6001 ; 6002 if (json.has("status")) 6003 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), EpisodeOfCare.EpisodeOfCareStatus.NULL, 6004 new EpisodeOfCare.EpisodeOfCareStatusEnumFactory())); 6005 if (json.has("_status")) 6006 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 6007 if (json.has("statusHistory")) { 6008 JsonArray array = json.getAsJsonArray("statusHistory"); 6009 for (int i = 0; i < array.size(); i++) { 6010 res.getStatusHistory() 6011 .add(parseEpisodeOfCareEpisodeOfCareStatusHistoryComponent(array.get(i).getAsJsonObject(), res)); 6012 } 6013 } 6014 ; 6015 if (json.has("type")) { 6016 JsonArray array = json.getAsJsonArray("type"); 6017 for (int i = 0; i < array.size(); i++) { 6018 res.getType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6019 } 6020 } 6021 ; 6022 if (json.has("condition")) { 6023 JsonArray array = json.getAsJsonArray("condition"); 6024 for (int i = 0; i < array.size(); i++) { 6025 res.getCondition().add(parseReference(array.get(i).getAsJsonObject())); 6026 } 6027 } 6028 ; 6029 if (json.has("patient")) 6030 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 6031 if (json.has("managingOrganization")) 6032 res.setManagingOrganization(parseReference(json.getAsJsonObject("managingOrganization"))); 6033 if (json.has("period")) 6034 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 6035 if (json.has("referralRequest")) { 6036 JsonArray array = json.getAsJsonArray("referralRequest"); 6037 for (int i = 0; i < array.size(); i++) { 6038 res.getReferralRequest().add(parseReference(array.get(i).getAsJsonObject())); 6039 } 6040 } 6041 ; 6042 if (json.has("careManager")) 6043 res.setCareManager(parseReference(json.getAsJsonObject("careManager"))); 6044 if (json.has("careTeam")) { 6045 JsonArray array = json.getAsJsonArray("careTeam"); 6046 for (int i = 0; i < array.size(); i++) { 6047 res.getCareTeam().add(parseEpisodeOfCareEpisodeOfCareCareTeamComponent(array.get(i).getAsJsonObject(), res)); 6048 } 6049 } 6050 ; 6051 return res; 6052 } 6053 6054 protected EpisodeOfCare.EpisodeOfCareStatusHistoryComponent parseEpisodeOfCareEpisodeOfCareStatusHistoryComponent( 6055 JsonObject json, EpisodeOfCare owner) throws IOException, FHIRFormatError { 6056 EpisodeOfCare.EpisodeOfCareStatusHistoryComponent res = new EpisodeOfCare.EpisodeOfCareStatusHistoryComponent(); 6057 parseBackboneProperties(json, res); 6058 if (json.has("status")) 6059 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), EpisodeOfCare.EpisodeOfCareStatus.NULL, 6060 new EpisodeOfCare.EpisodeOfCareStatusEnumFactory())); 6061 if (json.has("_status")) 6062 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 6063 if (json.has("period")) 6064 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 6065 return res; 6066 } 6067 6068 protected EpisodeOfCare.EpisodeOfCareCareTeamComponent parseEpisodeOfCareEpisodeOfCareCareTeamComponent( 6069 JsonObject json, EpisodeOfCare owner) throws IOException, FHIRFormatError { 6070 EpisodeOfCare.EpisodeOfCareCareTeamComponent res = new EpisodeOfCare.EpisodeOfCareCareTeamComponent(); 6071 parseBackboneProperties(json, res); 6072 if (json.has("role")) { 6073 JsonArray array = json.getAsJsonArray("role"); 6074 for (int i = 0; i < array.size(); i++) { 6075 res.getRole().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6076 } 6077 } 6078 ; 6079 if (json.has("period")) 6080 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 6081 if (json.has("member")) 6082 res.setMember(parseReference(json.getAsJsonObject("member"))); 6083 return res; 6084 } 6085 6086 protected ExplanationOfBenefit parseExplanationOfBenefit(JsonObject json) throws IOException, FHIRFormatError { 6087 ExplanationOfBenefit res = new ExplanationOfBenefit(); 6088 parseDomainResourceProperties(json, res); 6089 if (json.has("identifier")) { 6090 JsonArray array = json.getAsJsonArray("identifier"); 6091 for (int i = 0; i < array.size(); i++) { 6092 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 6093 } 6094 } 6095 ; 6096 if (json.has("request")) 6097 res.setRequest(parseReference(json.getAsJsonObject("request"))); 6098 if (json.has("outcome")) 6099 res.setOutcomeElement(parseEnumeration(json.get("outcome").getAsString(), Enumerations.RemittanceOutcome.NULL, 6100 new Enumerations.RemittanceOutcomeEnumFactory())); 6101 if (json.has("_outcome")) 6102 parseElementProperties(json.getAsJsonObject("_outcome"), res.getOutcomeElement()); 6103 if (json.has("disposition")) 6104 res.setDispositionElement(parseString(json.get("disposition").getAsString())); 6105 if (json.has("_disposition")) 6106 parseElementProperties(json.getAsJsonObject("_disposition"), res.getDispositionElement()); 6107 if (json.has("ruleset")) 6108 res.setRuleset(parseCoding(json.getAsJsonObject("ruleset"))); 6109 if (json.has("originalRuleset")) 6110 res.setOriginalRuleset(parseCoding(json.getAsJsonObject("originalRuleset"))); 6111 if (json.has("created")) 6112 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 6113 if (json.has("_created")) 6114 parseElementProperties(json.getAsJsonObject("_created"), res.getCreatedElement()); 6115 if (json.has("organization")) 6116 res.setOrganization(parseReference(json.getAsJsonObject("organization"))); 6117 if (json.has("requestProvider")) 6118 res.setRequestProvider(parseReference(json.getAsJsonObject("requestProvider"))); 6119 if (json.has("requestOrganization")) 6120 res.setRequestOrganization(parseReference(json.getAsJsonObject("requestOrganization"))); 6121 return res; 6122 } 6123 6124 protected FamilyMemberHistory parseFamilyMemberHistory(JsonObject json) throws IOException, FHIRFormatError { 6125 FamilyMemberHistory res = new FamilyMemberHistory(); 6126 parseDomainResourceProperties(json, res); 6127 if (json.has("identifier")) { 6128 JsonArray array = json.getAsJsonArray("identifier"); 6129 for (int i = 0; i < array.size(); i++) { 6130 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 6131 } 6132 } 6133 ; 6134 if (json.has("patient")) 6135 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 6136 if (json.has("date")) 6137 res.setDateElement(parseDateTime(json.get("date").getAsString())); 6138 if (json.has("_date")) 6139 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 6140 if (json.has("status")) 6141 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 6142 FamilyMemberHistory.FamilyHistoryStatus.NULL, new FamilyMemberHistory.FamilyHistoryStatusEnumFactory())); 6143 if (json.has("_status")) 6144 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 6145 if (json.has("name")) 6146 res.setNameElement(parseString(json.get("name").getAsString())); 6147 if (json.has("_name")) 6148 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 6149 if (json.has("relationship")) 6150 res.setRelationship(parseCodeableConcept(json.getAsJsonObject("relationship"))); 6151 if (json.has("gender")) 6152 res.setGenderElement(parseEnumeration(json.get("gender").getAsString(), Enumerations.AdministrativeGender.NULL, 6153 new Enumerations.AdministrativeGenderEnumFactory())); 6154 if (json.has("_gender")) 6155 parseElementProperties(json.getAsJsonObject("_gender"), res.getGenderElement()); 6156 Type born = parseType("born", json); 6157 if (born != null) 6158 res.setBorn(born); 6159 Type age = parseType("age", json); 6160 if (age != null) 6161 res.setAge(age); 6162 Type deceased = parseType("deceased", json); 6163 if (deceased != null) 6164 res.setDeceased(deceased); 6165 if (json.has("note")) 6166 res.setNote(parseAnnotation(json.getAsJsonObject("note"))); 6167 if (json.has("condition")) { 6168 JsonArray array = json.getAsJsonArray("condition"); 6169 for (int i = 0; i < array.size(); i++) { 6170 res.getCondition() 6171 .add(parseFamilyMemberHistoryFamilyMemberHistoryConditionComponent(array.get(i).getAsJsonObject(), res)); 6172 } 6173 } 6174 ; 6175 return res; 6176 } 6177 6178 protected FamilyMemberHistory.FamilyMemberHistoryConditionComponent parseFamilyMemberHistoryFamilyMemberHistoryConditionComponent( 6179 JsonObject json, FamilyMemberHistory owner) throws IOException, FHIRFormatError { 6180 FamilyMemberHistory.FamilyMemberHistoryConditionComponent res = new FamilyMemberHistory.FamilyMemberHistoryConditionComponent(); 6181 parseBackboneProperties(json, res); 6182 if (json.has("code")) 6183 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 6184 if (json.has("outcome")) 6185 res.setOutcome(parseCodeableConcept(json.getAsJsonObject("outcome"))); 6186 Type onset = parseType("onset", json); 6187 if (onset != null) 6188 res.setOnset(onset); 6189 if (json.has("note")) 6190 res.setNote(parseAnnotation(json.getAsJsonObject("note"))); 6191 return res; 6192 } 6193 6194 protected Flag parseFlag(JsonObject json) throws IOException, FHIRFormatError { 6195 Flag res = new Flag(); 6196 parseDomainResourceProperties(json, res); 6197 if (json.has("identifier")) { 6198 JsonArray array = json.getAsJsonArray("identifier"); 6199 for (int i = 0; i < array.size(); i++) { 6200 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 6201 } 6202 } 6203 ; 6204 if (json.has("category")) 6205 res.setCategory(parseCodeableConcept(json.getAsJsonObject("category"))); 6206 if (json.has("status")) 6207 res.setStatusElement( 6208 parseEnumeration(json.get("status").getAsString(), Flag.FlagStatus.NULL, new Flag.FlagStatusEnumFactory())); 6209 if (json.has("_status")) 6210 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 6211 if (json.has("period")) 6212 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 6213 if (json.has("subject")) 6214 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 6215 if (json.has("encounter")) 6216 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 6217 if (json.has("author")) 6218 res.setAuthor(parseReference(json.getAsJsonObject("author"))); 6219 if (json.has("code")) 6220 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 6221 return res; 6222 } 6223 6224 protected Goal parseGoal(JsonObject json) throws IOException, FHIRFormatError { 6225 Goal res = new Goal(); 6226 parseDomainResourceProperties(json, res); 6227 if (json.has("identifier")) { 6228 JsonArray array = json.getAsJsonArray("identifier"); 6229 for (int i = 0; i < array.size(); i++) { 6230 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 6231 } 6232 } 6233 ; 6234 if (json.has("subject")) 6235 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 6236 Type start = parseType("start", json); 6237 if (start != null) 6238 res.setStart(start); 6239 Type target = parseType("target", json); 6240 if (target != null) 6241 res.setTarget(target); 6242 if (json.has("category")) { 6243 JsonArray array = json.getAsJsonArray("category"); 6244 for (int i = 0; i < array.size(); i++) { 6245 res.getCategory().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6246 } 6247 } 6248 ; 6249 if (json.has("description")) 6250 res.setDescriptionElement(parseString(json.get("description").getAsString())); 6251 if (json.has("_description")) 6252 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 6253 if (json.has("status")) 6254 res.setStatusElement( 6255 parseEnumeration(json.get("status").getAsString(), Goal.GoalStatus.NULL, new Goal.GoalStatusEnumFactory())); 6256 if (json.has("_status")) 6257 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 6258 if (json.has("statusDate")) 6259 res.setStatusDateElement(parseDate(json.get("statusDate").getAsString())); 6260 if (json.has("_statusDate")) 6261 parseElementProperties(json.getAsJsonObject("_statusDate"), res.getStatusDateElement()); 6262 if (json.has("statusReason")) 6263 res.setStatusReason(parseCodeableConcept(json.getAsJsonObject("statusReason"))); 6264 if (json.has("author")) 6265 res.setAuthor(parseReference(json.getAsJsonObject("author"))); 6266 if (json.has("priority")) 6267 res.setPriority(parseCodeableConcept(json.getAsJsonObject("priority"))); 6268 if (json.has("addresses")) { 6269 JsonArray array = json.getAsJsonArray("addresses"); 6270 for (int i = 0; i < array.size(); i++) { 6271 res.getAddresses().add(parseReference(array.get(i).getAsJsonObject())); 6272 } 6273 } 6274 ; 6275 if (json.has("note")) { 6276 JsonArray array = json.getAsJsonArray("note"); 6277 for (int i = 0; i < array.size(); i++) { 6278 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 6279 } 6280 } 6281 ; 6282 if (json.has("outcome")) { 6283 JsonArray array = json.getAsJsonArray("outcome"); 6284 for (int i = 0; i < array.size(); i++) { 6285 res.getOutcome().add(parseGoalGoalOutcomeComponent(array.get(i).getAsJsonObject(), res)); 6286 } 6287 } 6288 ; 6289 return res; 6290 } 6291 6292 protected Goal.GoalOutcomeComponent parseGoalGoalOutcomeComponent(JsonObject json, Goal owner) 6293 throws IOException, FHIRFormatError { 6294 Goal.GoalOutcomeComponent res = new Goal.GoalOutcomeComponent(); 6295 parseBackboneProperties(json, res); 6296 Type result = parseType("result", json); 6297 if (result != null) 6298 res.setResult(result); 6299 return res; 6300 } 6301 6302 protected Group parseGroup(JsonObject json) throws IOException, FHIRFormatError { 6303 Group res = new Group(); 6304 parseDomainResourceProperties(json, res); 6305 if (json.has("identifier")) { 6306 JsonArray array = json.getAsJsonArray("identifier"); 6307 for (int i = 0; i < array.size(); i++) { 6308 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 6309 } 6310 } 6311 ; 6312 if (json.has("type")) 6313 res.setTypeElement( 6314 parseEnumeration(json.get("type").getAsString(), Group.GroupType.NULL, new Group.GroupTypeEnumFactory())); 6315 if (json.has("_type")) 6316 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 6317 if (json.has("actual")) 6318 res.setActualElement(parseBoolean(json.get("actual").getAsBoolean())); 6319 if (json.has("_actual")) 6320 parseElementProperties(json.getAsJsonObject("_actual"), res.getActualElement()); 6321 if (json.has("code")) 6322 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 6323 if (json.has("name")) 6324 res.setNameElement(parseString(json.get("name").getAsString())); 6325 if (json.has("_name")) 6326 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 6327 if (json.has("quantity")) 6328 res.setQuantityElement(parseUnsignedInt(json.get("quantity").getAsString())); 6329 if (json.has("_quantity")) 6330 parseElementProperties(json.getAsJsonObject("_quantity"), res.getQuantityElement()); 6331 if (json.has("characteristic")) { 6332 JsonArray array = json.getAsJsonArray("characteristic"); 6333 for (int i = 0; i < array.size(); i++) { 6334 res.getCharacteristic().add(parseGroupGroupCharacteristicComponent(array.get(i).getAsJsonObject(), res)); 6335 } 6336 } 6337 ; 6338 if (json.has("member")) { 6339 JsonArray array = json.getAsJsonArray("member"); 6340 for (int i = 0; i < array.size(); i++) { 6341 res.getMember().add(parseGroupGroupMemberComponent(array.get(i).getAsJsonObject(), res)); 6342 } 6343 } 6344 ; 6345 return res; 6346 } 6347 6348 protected Group.GroupCharacteristicComponent parseGroupGroupCharacteristicComponent(JsonObject json, Group owner) 6349 throws IOException, FHIRFormatError { 6350 Group.GroupCharacteristicComponent res = new Group.GroupCharacteristicComponent(); 6351 parseBackboneProperties(json, res); 6352 if (json.has("code")) 6353 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 6354 Type value = parseType("value", json); 6355 if (value != null) 6356 res.setValue(value); 6357 if (json.has("exclude")) 6358 res.setExcludeElement(parseBoolean(json.get("exclude").getAsBoolean())); 6359 if (json.has("_exclude")) 6360 parseElementProperties(json.getAsJsonObject("_exclude"), res.getExcludeElement()); 6361 if (json.has("period")) 6362 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 6363 return res; 6364 } 6365 6366 protected Group.GroupMemberComponent parseGroupGroupMemberComponent(JsonObject json, Group owner) 6367 throws IOException, FHIRFormatError { 6368 Group.GroupMemberComponent res = new Group.GroupMemberComponent(); 6369 parseBackboneProperties(json, res); 6370 if (json.has("entity")) 6371 res.setEntity(parseReference(json.getAsJsonObject("entity"))); 6372 if (json.has("period")) 6373 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 6374 if (json.has("inactive")) 6375 res.setInactiveElement(parseBoolean(json.get("inactive").getAsBoolean())); 6376 if (json.has("_inactive")) 6377 parseElementProperties(json.getAsJsonObject("_inactive"), res.getInactiveElement()); 6378 return res; 6379 } 6380 6381 protected HealthcareService parseHealthcareService(JsonObject json) throws IOException, FHIRFormatError { 6382 HealthcareService res = new HealthcareService(); 6383 parseDomainResourceProperties(json, res); 6384 if (json.has("identifier")) { 6385 JsonArray array = json.getAsJsonArray("identifier"); 6386 for (int i = 0; i < array.size(); i++) { 6387 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 6388 } 6389 } 6390 ; 6391 if (json.has("providedBy")) 6392 res.setProvidedBy(parseReference(json.getAsJsonObject("providedBy"))); 6393 if (json.has("serviceCategory")) 6394 res.setServiceCategory(parseCodeableConcept(json.getAsJsonObject("serviceCategory"))); 6395 if (json.has("serviceType")) { 6396 JsonArray array = json.getAsJsonArray("serviceType"); 6397 for (int i = 0; i < array.size(); i++) { 6398 res.getServiceType().add(parseHealthcareServiceServiceTypeComponent(array.get(i).getAsJsonObject(), res)); 6399 } 6400 } 6401 ; 6402 if (json.has("location")) 6403 res.setLocation(parseReference(json.getAsJsonObject("location"))); 6404 if (json.has("serviceName")) 6405 res.setServiceNameElement(parseString(json.get("serviceName").getAsString())); 6406 if (json.has("_serviceName")) 6407 parseElementProperties(json.getAsJsonObject("_serviceName"), res.getServiceNameElement()); 6408 if (json.has("comment")) 6409 res.setCommentElement(parseString(json.get("comment").getAsString())); 6410 if (json.has("_comment")) 6411 parseElementProperties(json.getAsJsonObject("_comment"), res.getCommentElement()); 6412 if (json.has("extraDetails")) 6413 res.setExtraDetailsElement(parseString(json.get("extraDetails").getAsString())); 6414 if (json.has("_extraDetails")) 6415 parseElementProperties(json.getAsJsonObject("_extraDetails"), res.getExtraDetailsElement()); 6416 if (json.has("photo")) 6417 res.setPhoto(parseAttachment(json.getAsJsonObject("photo"))); 6418 if (json.has("telecom")) { 6419 JsonArray array = json.getAsJsonArray("telecom"); 6420 for (int i = 0; i < array.size(); i++) { 6421 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 6422 } 6423 } 6424 ; 6425 if (json.has("coverageArea")) { 6426 JsonArray array = json.getAsJsonArray("coverageArea"); 6427 for (int i = 0; i < array.size(); i++) { 6428 res.getCoverageArea().add(parseReference(array.get(i).getAsJsonObject())); 6429 } 6430 } 6431 ; 6432 if (json.has("serviceProvisionCode")) { 6433 JsonArray array = json.getAsJsonArray("serviceProvisionCode"); 6434 for (int i = 0; i < array.size(); i++) { 6435 res.getServiceProvisionCode().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6436 } 6437 } 6438 ; 6439 if (json.has("eligibility")) 6440 res.setEligibility(parseCodeableConcept(json.getAsJsonObject("eligibility"))); 6441 if (json.has("eligibilityNote")) 6442 res.setEligibilityNoteElement(parseString(json.get("eligibilityNote").getAsString())); 6443 if (json.has("_eligibilityNote")) 6444 parseElementProperties(json.getAsJsonObject("_eligibilityNote"), res.getEligibilityNoteElement()); 6445 if (json.has("programName")) { 6446 JsonArray array = json.getAsJsonArray("programName"); 6447 for (int i = 0; i < array.size(); i++) { 6448 res.getProgramName().add(parseString(array.get(i).getAsString())); 6449 } 6450 } 6451 ; 6452 if (json.has("_programName")) { 6453 JsonArray array = json.getAsJsonArray("_programName"); 6454 for (int i = 0; i < array.size(); i++) { 6455 if (i == res.getProgramName().size()) 6456 res.getProgramName().add(parseString(null)); 6457 if (array.get(i) instanceof JsonObject) 6458 parseElementProperties(array.get(i).getAsJsonObject(), res.getProgramName().get(i)); 6459 } 6460 } 6461 ; 6462 if (json.has("characteristic")) { 6463 JsonArray array = json.getAsJsonArray("characteristic"); 6464 for (int i = 0; i < array.size(); i++) { 6465 res.getCharacteristic().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6466 } 6467 } 6468 ; 6469 if (json.has("referralMethod")) { 6470 JsonArray array = json.getAsJsonArray("referralMethod"); 6471 for (int i = 0; i < array.size(); i++) { 6472 res.getReferralMethod().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6473 } 6474 } 6475 ; 6476 if (json.has("publicKey")) 6477 res.setPublicKeyElement(parseString(json.get("publicKey").getAsString())); 6478 if (json.has("_publicKey")) 6479 parseElementProperties(json.getAsJsonObject("_publicKey"), res.getPublicKeyElement()); 6480 if (json.has("appointmentRequired")) 6481 res.setAppointmentRequiredElement(parseBoolean(json.get("appointmentRequired").getAsBoolean())); 6482 if (json.has("_appointmentRequired")) 6483 parseElementProperties(json.getAsJsonObject("_appointmentRequired"), res.getAppointmentRequiredElement()); 6484 if (json.has("availableTime")) { 6485 JsonArray array = json.getAsJsonArray("availableTime"); 6486 for (int i = 0; i < array.size(); i++) { 6487 res.getAvailableTime() 6488 .add(parseHealthcareServiceHealthcareServiceAvailableTimeComponent(array.get(i).getAsJsonObject(), res)); 6489 } 6490 } 6491 ; 6492 if (json.has("notAvailable")) { 6493 JsonArray array = json.getAsJsonArray("notAvailable"); 6494 for (int i = 0; i < array.size(); i++) { 6495 res.getNotAvailable() 6496 .add(parseHealthcareServiceHealthcareServiceNotAvailableComponent(array.get(i).getAsJsonObject(), res)); 6497 } 6498 } 6499 ; 6500 if (json.has("availabilityExceptions")) 6501 res.setAvailabilityExceptionsElement(parseString(json.get("availabilityExceptions").getAsString())); 6502 if (json.has("_availabilityExceptions")) 6503 parseElementProperties(json.getAsJsonObject("_availabilityExceptions"), res.getAvailabilityExceptionsElement()); 6504 return res; 6505 } 6506 6507 protected HealthcareService.ServiceTypeComponent parseHealthcareServiceServiceTypeComponent(JsonObject json, 6508 HealthcareService owner) throws IOException, FHIRFormatError { 6509 HealthcareService.ServiceTypeComponent res = new HealthcareService.ServiceTypeComponent(); 6510 parseBackboneProperties(json, res); 6511 if (json.has("type")) 6512 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 6513 if (json.has("specialty")) { 6514 JsonArray array = json.getAsJsonArray("specialty"); 6515 for (int i = 0; i < array.size(); i++) { 6516 res.getSpecialty().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6517 } 6518 } 6519 ; 6520 return res; 6521 } 6522 6523 protected HealthcareService.HealthcareServiceAvailableTimeComponent parseHealthcareServiceHealthcareServiceAvailableTimeComponent( 6524 JsonObject json, HealthcareService owner) throws IOException, FHIRFormatError { 6525 HealthcareService.HealthcareServiceAvailableTimeComponent res = new HealthcareService.HealthcareServiceAvailableTimeComponent(); 6526 parseBackboneProperties(json, res); 6527 if (json.has("daysOfWeek")) { 6528 JsonArray array = json.getAsJsonArray("daysOfWeek"); 6529 for (int i = 0; i < array.size(); i++) { 6530 res.getDaysOfWeek().add(parseEnumeration(array.get(i).getAsString(), HealthcareService.DaysOfWeek.NULL, 6531 new HealthcareService.DaysOfWeekEnumFactory())); 6532 } 6533 } 6534 ; 6535 if (json.has("_daysOfWeek")) { 6536 JsonArray array = json.getAsJsonArray("_daysOfWeek"); 6537 for (int i = 0; i < array.size(); i++) { 6538 if (i == res.getDaysOfWeek().size()) 6539 res.getDaysOfWeek().add( 6540 parseEnumeration(null, HealthcareService.DaysOfWeek.NULL, new HealthcareService.DaysOfWeekEnumFactory())); 6541 if (array.get(i) instanceof JsonObject) 6542 parseElementProperties(array.get(i).getAsJsonObject(), res.getDaysOfWeek().get(i)); 6543 } 6544 } 6545 ; 6546 if (json.has("allDay")) 6547 res.setAllDayElement(parseBoolean(json.get("allDay").getAsBoolean())); 6548 if (json.has("_allDay")) 6549 parseElementProperties(json.getAsJsonObject("_allDay"), res.getAllDayElement()); 6550 if (json.has("availableStartTime")) 6551 res.setAvailableStartTimeElement(parseTime(json.get("availableStartTime").getAsString())); 6552 if (json.has("_availableStartTime")) 6553 parseElementProperties(json.getAsJsonObject("_availableStartTime"), res.getAvailableStartTimeElement()); 6554 if (json.has("availableEndTime")) 6555 res.setAvailableEndTimeElement(parseTime(json.get("availableEndTime").getAsString())); 6556 if (json.has("_availableEndTime")) 6557 parseElementProperties(json.getAsJsonObject("_availableEndTime"), res.getAvailableEndTimeElement()); 6558 return res; 6559 } 6560 6561 protected HealthcareService.HealthcareServiceNotAvailableComponent parseHealthcareServiceHealthcareServiceNotAvailableComponent( 6562 JsonObject json, HealthcareService owner) throws IOException, FHIRFormatError { 6563 HealthcareService.HealthcareServiceNotAvailableComponent res = new HealthcareService.HealthcareServiceNotAvailableComponent(); 6564 parseBackboneProperties(json, res); 6565 if (json.has("description")) 6566 res.setDescriptionElement(parseString(json.get("description").getAsString())); 6567 if (json.has("_description")) 6568 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 6569 if (json.has("during")) 6570 res.setDuring(parsePeriod(json.getAsJsonObject("during"))); 6571 return res; 6572 } 6573 6574 protected ImagingObjectSelection parseImagingObjectSelection(JsonObject json) throws IOException, FHIRFormatError { 6575 ImagingObjectSelection res = new ImagingObjectSelection(); 6576 parseDomainResourceProperties(json, res); 6577 if (json.has("uid")) 6578 res.setUidElement(parseOid(json.get("uid").getAsString())); 6579 if (json.has("_uid")) 6580 parseElementProperties(json.getAsJsonObject("_uid"), res.getUidElement()); 6581 if (json.has("patient")) 6582 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 6583 if (json.has("title")) 6584 res.setTitle(parseCodeableConcept(json.getAsJsonObject("title"))); 6585 if (json.has("description")) 6586 res.setDescriptionElement(parseString(json.get("description").getAsString())); 6587 if (json.has("_description")) 6588 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 6589 if (json.has("author")) 6590 res.setAuthor(parseReference(json.getAsJsonObject("author"))); 6591 if (json.has("authoringTime")) 6592 res.setAuthoringTimeElement(parseDateTime(json.get("authoringTime").getAsString())); 6593 if (json.has("_authoringTime")) 6594 parseElementProperties(json.getAsJsonObject("_authoringTime"), res.getAuthoringTimeElement()); 6595 if (json.has("study")) { 6596 JsonArray array = json.getAsJsonArray("study"); 6597 for (int i = 0; i < array.size(); i++) { 6598 res.getStudy().add(parseImagingObjectSelectionStudyComponent(array.get(i).getAsJsonObject(), res)); 6599 } 6600 } 6601 ; 6602 return res; 6603 } 6604 6605 protected ImagingObjectSelection.StudyComponent parseImagingObjectSelectionStudyComponent(JsonObject json, 6606 ImagingObjectSelection owner) throws IOException, FHIRFormatError { 6607 ImagingObjectSelection.StudyComponent res = new ImagingObjectSelection.StudyComponent(); 6608 parseBackboneProperties(json, res); 6609 if (json.has("uid")) 6610 res.setUidElement(parseOid(json.get("uid").getAsString())); 6611 if (json.has("_uid")) 6612 parseElementProperties(json.getAsJsonObject("_uid"), res.getUidElement()); 6613 if (json.has("url")) 6614 res.setUrlElement(parseUri(json.get("url").getAsString())); 6615 if (json.has("_url")) 6616 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 6617 if (json.has("imagingStudy")) 6618 res.setImagingStudy(parseReference(json.getAsJsonObject("imagingStudy"))); 6619 if (json.has("series")) { 6620 JsonArray array = json.getAsJsonArray("series"); 6621 for (int i = 0; i < array.size(); i++) { 6622 res.getSeries().add(parseImagingObjectSelectionSeriesComponent(array.get(i).getAsJsonObject(), owner)); 6623 } 6624 } 6625 ; 6626 return res; 6627 } 6628 6629 protected ImagingObjectSelection.SeriesComponent parseImagingObjectSelectionSeriesComponent(JsonObject json, 6630 ImagingObjectSelection owner) throws IOException, FHIRFormatError { 6631 ImagingObjectSelection.SeriesComponent res = new ImagingObjectSelection.SeriesComponent(); 6632 parseBackboneProperties(json, res); 6633 if (json.has("uid")) 6634 res.setUidElement(parseOid(json.get("uid").getAsString())); 6635 if (json.has("_uid")) 6636 parseElementProperties(json.getAsJsonObject("_uid"), res.getUidElement()); 6637 if (json.has("url")) 6638 res.setUrlElement(parseUri(json.get("url").getAsString())); 6639 if (json.has("_url")) 6640 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 6641 if (json.has("instance")) { 6642 JsonArray array = json.getAsJsonArray("instance"); 6643 for (int i = 0; i < array.size(); i++) { 6644 res.getInstance().add(parseImagingObjectSelectionInstanceComponent(array.get(i).getAsJsonObject(), owner)); 6645 } 6646 } 6647 ; 6648 return res; 6649 } 6650 6651 protected ImagingObjectSelection.InstanceComponent parseImagingObjectSelectionInstanceComponent(JsonObject json, 6652 ImagingObjectSelection owner) throws IOException, FHIRFormatError { 6653 ImagingObjectSelection.InstanceComponent res = new ImagingObjectSelection.InstanceComponent(); 6654 parseBackboneProperties(json, res); 6655 if (json.has("sopClass")) 6656 res.setSopClassElement(parseOid(json.get("sopClass").getAsString())); 6657 if (json.has("_sopClass")) 6658 parseElementProperties(json.getAsJsonObject("_sopClass"), res.getSopClassElement()); 6659 if (json.has("uid")) 6660 res.setUidElement(parseOid(json.get("uid").getAsString())); 6661 if (json.has("_uid")) 6662 parseElementProperties(json.getAsJsonObject("_uid"), res.getUidElement()); 6663 if (json.has("url")) 6664 res.setUrlElement(parseUri(json.get("url").getAsString())); 6665 if (json.has("_url")) 6666 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 6667 if (json.has("frames")) { 6668 JsonArray array = json.getAsJsonArray("frames"); 6669 for (int i = 0; i < array.size(); i++) { 6670 res.getFrames().add(parseImagingObjectSelectionFramesComponent(array.get(i).getAsJsonObject(), owner)); 6671 } 6672 } 6673 ; 6674 return res; 6675 } 6676 6677 protected ImagingObjectSelection.FramesComponent parseImagingObjectSelectionFramesComponent(JsonObject json, 6678 ImagingObjectSelection owner) throws IOException, FHIRFormatError { 6679 ImagingObjectSelection.FramesComponent res = new ImagingObjectSelection.FramesComponent(); 6680 parseBackboneProperties(json, res); 6681 if (json.has("frameNumbers")) { 6682 JsonArray array = json.getAsJsonArray("frameNumbers"); 6683 for (int i = 0; i < array.size(); i++) { 6684 res.getFrameNumbers().add(parseUnsignedInt(array.get(i).getAsString())); 6685 } 6686 } 6687 ; 6688 if (json.has("_frameNumbers")) { 6689 JsonArray array = json.getAsJsonArray("_frameNumbers"); 6690 for (int i = 0; i < array.size(); i++) { 6691 if (i == res.getFrameNumbers().size()) 6692 res.getFrameNumbers().add(parseUnsignedInt(null)); 6693 if (array.get(i) instanceof JsonObject) 6694 parseElementProperties(array.get(i).getAsJsonObject(), res.getFrameNumbers().get(i)); 6695 } 6696 } 6697 ; 6698 if (json.has("url")) 6699 res.setUrlElement(parseUri(json.get("url").getAsString())); 6700 if (json.has("_url")) 6701 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 6702 return res; 6703 } 6704 6705 protected ImagingStudy parseImagingStudy(JsonObject json) throws IOException, FHIRFormatError { 6706 ImagingStudy res = new ImagingStudy(); 6707 parseDomainResourceProperties(json, res); 6708 if (json.has("started")) 6709 res.setStartedElement(parseDateTime(json.get("started").getAsString())); 6710 if (json.has("_started")) 6711 parseElementProperties(json.getAsJsonObject("_started"), res.getStartedElement()); 6712 if (json.has("patient")) 6713 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 6714 if (json.has("uid")) 6715 res.setUidElement(parseOid(json.get("uid").getAsString())); 6716 if (json.has("_uid")) 6717 parseElementProperties(json.getAsJsonObject("_uid"), res.getUidElement()); 6718 if (json.has("accession")) 6719 res.setAccession(parseIdentifier(json.getAsJsonObject("accession"))); 6720 if (json.has("identifier")) { 6721 JsonArray array = json.getAsJsonArray("identifier"); 6722 for (int i = 0; i < array.size(); i++) { 6723 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 6724 } 6725 } 6726 ; 6727 if (json.has("order")) { 6728 JsonArray array = json.getAsJsonArray("order"); 6729 for (int i = 0; i < array.size(); i++) { 6730 res.getOrder().add(parseReference(array.get(i).getAsJsonObject())); 6731 } 6732 } 6733 ; 6734 if (json.has("modalityList")) { 6735 JsonArray array = json.getAsJsonArray("modalityList"); 6736 for (int i = 0; i < array.size(); i++) { 6737 res.getModalityList().add(parseCoding(array.get(i).getAsJsonObject())); 6738 } 6739 } 6740 ; 6741 if (json.has("referrer")) 6742 res.setReferrer(parseReference(json.getAsJsonObject("referrer"))); 6743 if (json.has("availability")) 6744 res.setAvailabilityElement(parseEnumeration(json.get("availability").getAsString(), 6745 ImagingStudy.InstanceAvailability.NULL, new ImagingStudy.InstanceAvailabilityEnumFactory())); 6746 if (json.has("_availability")) 6747 parseElementProperties(json.getAsJsonObject("_availability"), res.getAvailabilityElement()); 6748 if (json.has("url")) 6749 res.setUrlElement(parseUri(json.get("url").getAsString())); 6750 if (json.has("_url")) 6751 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 6752 if (json.has("numberOfSeries")) 6753 res.setNumberOfSeriesElement(parseUnsignedInt(json.get("numberOfSeries").getAsString())); 6754 if (json.has("_numberOfSeries")) 6755 parseElementProperties(json.getAsJsonObject("_numberOfSeries"), res.getNumberOfSeriesElement()); 6756 if (json.has("numberOfInstances")) 6757 res.setNumberOfInstancesElement(parseUnsignedInt(json.get("numberOfInstances").getAsString())); 6758 if (json.has("_numberOfInstances")) 6759 parseElementProperties(json.getAsJsonObject("_numberOfInstances"), res.getNumberOfInstancesElement()); 6760 if (json.has("procedure")) { 6761 JsonArray array = json.getAsJsonArray("procedure"); 6762 for (int i = 0; i < array.size(); i++) { 6763 res.getProcedure().add(parseReference(array.get(i).getAsJsonObject())); 6764 } 6765 } 6766 ; 6767 if (json.has("interpreter")) 6768 res.setInterpreter(parseReference(json.getAsJsonObject("interpreter"))); 6769 if (json.has("description")) 6770 res.setDescriptionElement(parseString(json.get("description").getAsString())); 6771 if (json.has("_description")) 6772 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 6773 if (json.has("series")) { 6774 JsonArray array = json.getAsJsonArray("series"); 6775 for (int i = 0; i < array.size(); i++) { 6776 res.getSeries().add(parseImagingStudyImagingStudySeriesComponent(array.get(i).getAsJsonObject(), res)); 6777 } 6778 } 6779 ; 6780 return res; 6781 } 6782 6783 protected ImagingStudy.ImagingStudySeriesComponent parseImagingStudyImagingStudySeriesComponent(JsonObject json, 6784 ImagingStudy owner) throws IOException, FHIRFormatError { 6785 ImagingStudy.ImagingStudySeriesComponent res = new ImagingStudy.ImagingStudySeriesComponent(); 6786 parseBackboneProperties(json, res); 6787 if (json.has("number")) 6788 res.setNumberElement(parseUnsignedInt(json.get("number").getAsString())); 6789 if (json.has("_number")) 6790 parseElementProperties(json.getAsJsonObject("_number"), res.getNumberElement()); 6791 if (json.has("modality")) 6792 res.setModality(parseCoding(json.getAsJsonObject("modality"))); 6793 if (json.has("uid")) 6794 res.setUidElement(parseOid(json.get("uid").getAsString())); 6795 if (json.has("_uid")) 6796 parseElementProperties(json.getAsJsonObject("_uid"), res.getUidElement()); 6797 if (json.has("description")) 6798 res.setDescriptionElement(parseString(json.get("description").getAsString())); 6799 if (json.has("_description")) 6800 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 6801 if (json.has("numberOfInstances")) 6802 res.setNumberOfInstancesElement(parseUnsignedInt(json.get("numberOfInstances").getAsString())); 6803 if (json.has("_numberOfInstances")) 6804 parseElementProperties(json.getAsJsonObject("_numberOfInstances"), res.getNumberOfInstancesElement()); 6805 if (json.has("availability")) 6806 res.setAvailabilityElement(parseEnumeration(json.get("availability").getAsString(), 6807 ImagingStudy.InstanceAvailability.NULL, new ImagingStudy.InstanceAvailabilityEnumFactory())); 6808 if (json.has("_availability")) 6809 parseElementProperties(json.getAsJsonObject("_availability"), res.getAvailabilityElement()); 6810 if (json.has("url")) 6811 res.setUrlElement(parseUri(json.get("url").getAsString())); 6812 if (json.has("_url")) 6813 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 6814 if (json.has("bodySite")) 6815 res.setBodySite(parseCoding(json.getAsJsonObject("bodySite"))); 6816 if (json.has("laterality")) 6817 res.setLaterality(parseCoding(json.getAsJsonObject("laterality"))); 6818 if (json.has("started")) 6819 res.setStartedElement(parseDateTime(json.get("started").getAsString())); 6820 if (json.has("_started")) 6821 parseElementProperties(json.getAsJsonObject("_started"), res.getStartedElement()); 6822 if (json.has("instance")) { 6823 JsonArray array = json.getAsJsonArray("instance"); 6824 for (int i = 0; i < array.size(); i++) { 6825 res.getInstance() 6826 .add(parseImagingStudyImagingStudySeriesInstanceComponent(array.get(i).getAsJsonObject(), owner)); 6827 } 6828 } 6829 ; 6830 return res; 6831 } 6832 6833 protected ImagingStudy.ImagingStudySeriesInstanceComponent parseImagingStudyImagingStudySeriesInstanceComponent( 6834 JsonObject json, ImagingStudy owner) throws IOException, FHIRFormatError { 6835 ImagingStudy.ImagingStudySeriesInstanceComponent res = new ImagingStudy.ImagingStudySeriesInstanceComponent(); 6836 parseBackboneProperties(json, res); 6837 if (json.has("number")) 6838 res.setNumberElement(parseUnsignedInt(json.get("number").getAsString())); 6839 if (json.has("_number")) 6840 parseElementProperties(json.getAsJsonObject("_number"), res.getNumberElement()); 6841 if (json.has("uid")) 6842 res.setUidElement(parseOid(json.get("uid").getAsString())); 6843 if (json.has("_uid")) 6844 parseElementProperties(json.getAsJsonObject("_uid"), res.getUidElement()); 6845 if (json.has("sopClass")) 6846 res.setSopClassElement(parseOid(json.get("sopClass").getAsString())); 6847 if (json.has("_sopClass")) 6848 parseElementProperties(json.getAsJsonObject("_sopClass"), res.getSopClassElement()); 6849 if (json.has("type")) 6850 res.setTypeElement(parseString(json.get("type").getAsString())); 6851 if (json.has("_type")) 6852 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 6853 if (json.has("title")) 6854 res.setTitleElement(parseString(json.get("title").getAsString())); 6855 if (json.has("_title")) 6856 parseElementProperties(json.getAsJsonObject("_title"), res.getTitleElement()); 6857 if (json.has("content")) { 6858 JsonArray array = json.getAsJsonArray("content"); 6859 for (int i = 0; i < array.size(); i++) { 6860 res.getContent().add(parseAttachment(array.get(i).getAsJsonObject())); 6861 } 6862 } 6863 ; 6864 return res; 6865 } 6866 6867 protected Immunization parseImmunization(JsonObject json) throws IOException, FHIRFormatError { 6868 Immunization res = new Immunization(); 6869 parseDomainResourceProperties(json, res); 6870 if (json.has("identifier")) { 6871 JsonArray array = json.getAsJsonArray("identifier"); 6872 for (int i = 0; i < array.size(); i++) { 6873 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 6874 } 6875 } 6876 ; 6877 if (json.has("status")) 6878 res.setStatusElement(parseCode(json.get("status").getAsString())); 6879 if (json.has("_status")) 6880 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 6881 if (json.has("date")) 6882 res.setDateElement(parseDateTime(json.get("date").getAsString())); 6883 if (json.has("_date")) 6884 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 6885 if (json.has("vaccineCode")) 6886 res.setVaccineCode(parseCodeableConcept(json.getAsJsonObject("vaccineCode"))); 6887 if (json.has("patient")) 6888 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 6889 if (json.has("wasNotGiven")) 6890 res.setWasNotGivenElement(parseBoolean(json.get("wasNotGiven").getAsBoolean())); 6891 if (json.has("_wasNotGiven")) 6892 parseElementProperties(json.getAsJsonObject("_wasNotGiven"), res.getWasNotGivenElement()); 6893 if (json.has("reported")) 6894 res.setReportedElement(parseBoolean(json.get("reported").getAsBoolean())); 6895 if (json.has("_reported")) 6896 parseElementProperties(json.getAsJsonObject("_reported"), res.getReportedElement()); 6897 if (json.has("performer")) 6898 res.setPerformer(parseReference(json.getAsJsonObject("performer"))); 6899 if (json.has("requester")) 6900 res.setRequester(parseReference(json.getAsJsonObject("requester"))); 6901 if (json.has("encounter")) 6902 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 6903 if (json.has("manufacturer")) 6904 res.setManufacturer(parseReference(json.getAsJsonObject("manufacturer"))); 6905 if (json.has("location")) 6906 res.setLocation(parseReference(json.getAsJsonObject("location"))); 6907 if (json.has("lotNumber")) 6908 res.setLotNumberElement(parseString(json.get("lotNumber").getAsString())); 6909 if (json.has("_lotNumber")) 6910 parseElementProperties(json.getAsJsonObject("_lotNumber"), res.getLotNumberElement()); 6911 if (json.has("expirationDate")) 6912 res.setExpirationDateElement(parseDate(json.get("expirationDate").getAsString())); 6913 if (json.has("_expirationDate")) 6914 parseElementProperties(json.getAsJsonObject("_expirationDate"), res.getExpirationDateElement()); 6915 if (json.has("site")) 6916 res.setSite(parseCodeableConcept(json.getAsJsonObject("site"))); 6917 if (json.has("route")) 6918 res.setRoute(parseCodeableConcept(json.getAsJsonObject("route"))); 6919 if (json.has("doseQuantity")) 6920 res.setDoseQuantity(parseSimpleQuantity(json.getAsJsonObject("doseQuantity"))); 6921 if (json.has("note")) { 6922 JsonArray array = json.getAsJsonArray("note"); 6923 for (int i = 0; i < array.size(); i++) { 6924 res.getNote().add(parseAnnotation(array.get(i).getAsJsonObject())); 6925 } 6926 } 6927 ; 6928 if (json.has("explanation")) 6929 res.setExplanation(parseImmunizationImmunizationExplanationComponent(json.getAsJsonObject("explanation"), res)); 6930 if (json.has("reaction")) { 6931 JsonArray array = json.getAsJsonArray("reaction"); 6932 for (int i = 0; i < array.size(); i++) { 6933 res.getReaction().add(parseImmunizationImmunizationReactionComponent(array.get(i).getAsJsonObject(), res)); 6934 } 6935 } 6936 ; 6937 if (json.has("vaccinationProtocol")) { 6938 JsonArray array = json.getAsJsonArray("vaccinationProtocol"); 6939 for (int i = 0; i < array.size(); i++) { 6940 res.getVaccinationProtocol() 6941 .add(parseImmunizationImmunizationVaccinationProtocolComponent(array.get(i).getAsJsonObject(), res)); 6942 } 6943 } 6944 ; 6945 return res; 6946 } 6947 6948 protected Immunization.ImmunizationExplanationComponent parseImmunizationImmunizationExplanationComponent( 6949 JsonObject json, Immunization owner) throws IOException, FHIRFormatError { 6950 Immunization.ImmunizationExplanationComponent res = new Immunization.ImmunizationExplanationComponent(); 6951 parseBackboneProperties(json, res); 6952 if (json.has("reason")) { 6953 JsonArray array = json.getAsJsonArray("reason"); 6954 for (int i = 0; i < array.size(); i++) { 6955 res.getReason().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6956 } 6957 } 6958 ; 6959 if (json.has("reasonNotGiven")) { 6960 JsonArray array = json.getAsJsonArray("reasonNotGiven"); 6961 for (int i = 0; i < array.size(); i++) { 6962 res.getReasonNotGiven().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 6963 } 6964 } 6965 ; 6966 return res; 6967 } 6968 6969 protected Immunization.ImmunizationReactionComponent parseImmunizationImmunizationReactionComponent(JsonObject json, 6970 Immunization owner) throws IOException, FHIRFormatError { 6971 Immunization.ImmunizationReactionComponent res = new Immunization.ImmunizationReactionComponent(); 6972 parseBackboneProperties(json, res); 6973 if (json.has("date")) 6974 res.setDateElement(parseDateTime(json.get("date").getAsString())); 6975 if (json.has("_date")) 6976 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 6977 if (json.has("detail")) 6978 res.setDetail(parseReference(json.getAsJsonObject("detail"))); 6979 if (json.has("reported")) 6980 res.setReportedElement(parseBoolean(json.get("reported").getAsBoolean())); 6981 if (json.has("_reported")) 6982 parseElementProperties(json.getAsJsonObject("_reported"), res.getReportedElement()); 6983 return res; 6984 } 6985 6986 protected Immunization.ImmunizationVaccinationProtocolComponent parseImmunizationImmunizationVaccinationProtocolComponent( 6987 JsonObject json, Immunization owner) throws IOException, FHIRFormatError { 6988 Immunization.ImmunizationVaccinationProtocolComponent res = new Immunization.ImmunizationVaccinationProtocolComponent(); 6989 parseBackboneProperties(json, res); 6990 if (json.has("doseSequence")) 6991 res.setDoseSequenceElement(parsePositiveInt(json.get("doseSequence").getAsString())); 6992 if (json.has("_doseSequence")) 6993 parseElementProperties(json.getAsJsonObject("_doseSequence"), res.getDoseSequenceElement()); 6994 if (json.has("description")) 6995 res.setDescriptionElement(parseString(json.get("description").getAsString())); 6996 if (json.has("_description")) 6997 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 6998 if (json.has("authority")) 6999 res.setAuthority(parseReference(json.getAsJsonObject("authority"))); 7000 if (json.has("series")) 7001 res.setSeriesElement(parseString(json.get("series").getAsString())); 7002 if (json.has("_series")) 7003 parseElementProperties(json.getAsJsonObject("_series"), res.getSeriesElement()); 7004 if (json.has("seriesDoses")) 7005 res.setSeriesDosesElement(parsePositiveInt(json.get("seriesDoses").getAsString())); 7006 if (json.has("_seriesDoses")) 7007 parseElementProperties(json.getAsJsonObject("_seriesDoses"), res.getSeriesDosesElement()); 7008 if (json.has("targetDisease")) { 7009 JsonArray array = json.getAsJsonArray("targetDisease"); 7010 for (int i = 0; i < array.size(); i++) { 7011 res.getTargetDisease().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 7012 } 7013 } 7014 ; 7015 if (json.has("doseStatus")) 7016 res.setDoseStatus(parseCodeableConcept(json.getAsJsonObject("doseStatus"))); 7017 if (json.has("doseStatusReason")) 7018 res.setDoseStatusReason(parseCodeableConcept(json.getAsJsonObject("doseStatusReason"))); 7019 return res; 7020 } 7021 7022 protected ImmunizationRecommendation parseImmunizationRecommendation(JsonObject json) 7023 throws IOException, FHIRFormatError { 7024 ImmunizationRecommendation res = new ImmunizationRecommendation(); 7025 parseDomainResourceProperties(json, res); 7026 if (json.has("identifier")) { 7027 JsonArray array = json.getAsJsonArray("identifier"); 7028 for (int i = 0; i < array.size(); i++) { 7029 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 7030 } 7031 } 7032 ; 7033 if (json.has("patient")) 7034 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 7035 if (json.has("recommendation")) { 7036 JsonArray array = json.getAsJsonArray("recommendation"); 7037 for (int i = 0; i < array.size(); i++) { 7038 res.getRecommendation().add(parseImmunizationRecommendationImmunizationRecommendationRecommendationComponent( 7039 array.get(i).getAsJsonObject(), res)); 7040 } 7041 } 7042 ; 7043 return res; 7044 } 7045 7046 protected ImmunizationRecommendation.ImmunizationRecommendationRecommendationComponent parseImmunizationRecommendationImmunizationRecommendationRecommendationComponent( 7047 JsonObject json, ImmunizationRecommendation owner) throws IOException, FHIRFormatError { 7048 ImmunizationRecommendation.ImmunizationRecommendationRecommendationComponent res = new ImmunizationRecommendation.ImmunizationRecommendationRecommendationComponent(); 7049 parseBackboneProperties(json, res); 7050 if (json.has("date")) 7051 res.setDateElement(parseDateTime(json.get("date").getAsString())); 7052 if (json.has("_date")) 7053 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 7054 if (json.has("vaccineCode")) 7055 res.setVaccineCode(parseCodeableConcept(json.getAsJsonObject("vaccineCode"))); 7056 if (json.has("doseNumber")) 7057 res.setDoseNumberElement(parsePositiveInt(json.get("doseNumber").getAsString())); 7058 if (json.has("_doseNumber")) 7059 parseElementProperties(json.getAsJsonObject("_doseNumber"), res.getDoseNumberElement()); 7060 if (json.has("forecastStatus")) 7061 res.setForecastStatus(parseCodeableConcept(json.getAsJsonObject("forecastStatus"))); 7062 if (json.has("dateCriterion")) { 7063 JsonArray array = json.getAsJsonArray("dateCriterion"); 7064 for (int i = 0; i < array.size(); i++) { 7065 res.getDateCriterion() 7066 .add(parseImmunizationRecommendationImmunizationRecommendationRecommendationDateCriterionComponent( 7067 array.get(i).getAsJsonObject(), owner)); 7068 } 7069 } 7070 ; 7071 if (json.has("protocol")) 7072 res.setProtocol(parseImmunizationRecommendationImmunizationRecommendationRecommendationProtocolComponent( 7073 json.getAsJsonObject("protocol"), owner)); 7074 if (json.has("supportingImmunization")) { 7075 JsonArray array = json.getAsJsonArray("supportingImmunization"); 7076 for (int i = 0; i < array.size(); i++) { 7077 res.getSupportingImmunization().add(parseReference(array.get(i).getAsJsonObject())); 7078 } 7079 } 7080 ; 7081 if (json.has("supportingPatientInformation")) { 7082 JsonArray array = json.getAsJsonArray("supportingPatientInformation"); 7083 for (int i = 0; i < array.size(); i++) { 7084 res.getSupportingPatientInformation().add(parseReference(array.get(i).getAsJsonObject())); 7085 } 7086 } 7087 ; 7088 return res; 7089 } 7090 7091 protected ImmunizationRecommendation.ImmunizationRecommendationRecommendationDateCriterionComponent parseImmunizationRecommendationImmunizationRecommendationRecommendationDateCriterionComponent( 7092 JsonObject json, ImmunizationRecommendation owner) throws IOException, FHIRFormatError { 7093 ImmunizationRecommendation.ImmunizationRecommendationRecommendationDateCriterionComponent res = new ImmunizationRecommendation.ImmunizationRecommendationRecommendationDateCriterionComponent(); 7094 parseBackboneProperties(json, res); 7095 if (json.has("code")) 7096 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 7097 if (json.has("value")) 7098 res.setValueElement(parseDateTime(json.get("value").getAsString())); 7099 if (json.has("_value")) 7100 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 7101 return res; 7102 } 7103 7104 protected ImmunizationRecommendation.ImmunizationRecommendationRecommendationProtocolComponent parseImmunizationRecommendationImmunizationRecommendationRecommendationProtocolComponent( 7105 JsonObject json, ImmunizationRecommendation owner) throws IOException, FHIRFormatError { 7106 ImmunizationRecommendation.ImmunizationRecommendationRecommendationProtocolComponent res = new ImmunizationRecommendation.ImmunizationRecommendationRecommendationProtocolComponent(); 7107 parseBackboneProperties(json, res); 7108 if (json.has("doseSequence")) 7109 res.setDoseSequenceElement(parseInteger(json.get("doseSequence").getAsLong())); 7110 if (json.has("_doseSequence")) 7111 parseElementProperties(json.getAsJsonObject("_doseSequence"), res.getDoseSequenceElement()); 7112 if (json.has("description")) 7113 res.setDescriptionElement(parseString(json.get("description").getAsString())); 7114 if (json.has("_description")) 7115 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 7116 if (json.has("authority")) 7117 res.setAuthority(parseReference(json.getAsJsonObject("authority"))); 7118 if (json.has("series")) 7119 res.setSeriesElement(parseString(json.get("series").getAsString())); 7120 if (json.has("_series")) 7121 parseElementProperties(json.getAsJsonObject("_series"), res.getSeriesElement()); 7122 return res; 7123 } 7124 7125 protected ImplementationGuide parseImplementationGuide(JsonObject json) throws IOException, FHIRFormatError { 7126 ImplementationGuide res = new ImplementationGuide(); 7127 parseDomainResourceProperties(json, res); 7128 if (json.has("url")) 7129 res.setUrlElement(parseUri(json.get("url").getAsString())); 7130 if (json.has("_url")) 7131 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 7132 if (json.has("version")) 7133 res.setVersionElement(parseString(json.get("version").getAsString())); 7134 if (json.has("_version")) 7135 parseElementProperties(json.getAsJsonObject("_version"), res.getVersionElement()); 7136 if (json.has("name")) 7137 res.setNameElement(parseString(json.get("name").getAsString())); 7138 if (json.has("_name")) 7139 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 7140 if (json.has("status")) 7141 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 7142 Enumerations.ConformanceResourceStatus.NULL, new Enumerations.ConformanceResourceStatusEnumFactory())); 7143 if (json.has("_status")) 7144 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 7145 if (json.has("experimental")) 7146 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 7147 if (json.has("_experimental")) 7148 parseElementProperties(json.getAsJsonObject("_experimental"), res.getExperimentalElement()); 7149 if (json.has("publisher")) 7150 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 7151 if (json.has("_publisher")) 7152 parseElementProperties(json.getAsJsonObject("_publisher"), res.getPublisherElement()); 7153 if (json.has("contact")) { 7154 JsonArray array = json.getAsJsonArray("contact"); 7155 for (int i = 0; i < array.size(); i++) { 7156 res.getContact() 7157 .add(parseImplementationGuideImplementationGuideContactComponent(array.get(i).getAsJsonObject(), res)); 7158 } 7159 } 7160 ; 7161 if (json.has("date")) 7162 res.setDateElement(parseDateTime(json.get("date").getAsString())); 7163 if (json.has("_date")) 7164 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 7165 if (json.has("description")) 7166 res.setDescriptionElement(parseString(json.get("description").getAsString())); 7167 if (json.has("_description")) 7168 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 7169 if (json.has("useContext")) { 7170 JsonArray array = json.getAsJsonArray("useContext"); 7171 for (int i = 0; i < array.size(); i++) { 7172 res.getUseContext().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 7173 } 7174 } 7175 ; 7176 if (json.has("copyright")) 7177 res.setCopyrightElement(parseString(json.get("copyright").getAsString())); 7178 if (json.has("_copyright")) 7179 parseElementProperties(json.getAsJsonObject("_copyright"), res.getCopyrightElement()); 7180 if (json.has("fhirVersion")) 7181 res.setFhirVersionElement(parseId(json.get("fhirVersion").getAsString())); 7182 if (json.has("_fhirVersion")) 7183 parseElementProperties(json.getAsJsonObject("_fhirVersion"), res.getFhirVersionElement()); 7184 if (json.has("dependency")) { 7185 JsonArray array = json.getAsJsonArray("dependency"); 7186 for (int i = 0; i < array.size(); i++) { 7187 res.getDependency() 7188 .add(parseImplementationGuideImplementationGuideDependencyComponent(array.get(i).getAsJsonObject(), res)); 7189 } 7190 } 7191 ; 7192 if (json.has("package")) { 7193 JsonArray array = json.getAsJsonArray("package"); 7194 for (int i = 0; i < array.size(); i++) { 7195 res.getPackage() 7196 .add(parseImplementationGuideImplementationGuidePackageComponent(array.get(i).getAsJsonObject(), res)); 7197 } 7198 } 7199 ; 7200 if (json.has("global")) { 7201 JsonArray array = json.getAsJsonArray("global"); 7202 for (int i = 0; i < array.size(); i++) { 7203 res.getGlobal() 7204 .add(parseImplementationGuideImplementationGuideGlobalComponent(array.get(i).getAsJsonObject(), res)); 7205 } 7206 } 7207 ; 7208 if (json.has("binary")) { 7209 JsonArray array = json.getAsJsonArray("binary"); 7210 for (int i = 0; i < array.size(); i++) { 7211 res.getBinary().add(parseUri(array.get(i).getAsString())); 7212 } 7213 } 7214 ; 7215 if (json.has("_binary")) { 7216 JsonArray array = json.getAsJsonArray("_binary"); 7217 for (int i = 0; i < array.size(); i++) { 7218 if (i == res.getBinary().size()) 7219 res.getBinary().add(parseUri(null)); 7220 if (array.get(i) instanceof JsonObject) 7221 parseElementProperties(array.get(i).getAsJsonObject(), res.getBinary().get(i)); 7222 } 7223 } 7224 ; 7225 if (json.has("page")) 7226 res.setPage(parseImplementationGuideImplementationGuidePageComponent(json.getAsJsonObject("page"), res)); 7227 return res; 7228 } 7229 7230 protected ImplementationGuide.ImplementationGuideContactComponent parseImplementationGuideImplementationGuideContactComponent( 7231 JsonObject json, ImplementationGuide owner) throws IOException, FHIRFormatError { 7232 ImplementationGuide.ImplementationGuideContactComponent res = new ImplementationGuide.ImplementationGuideContactComponent(); 7233 parseBackboneProperties(json, res); 7234 if (json.has("name")) 7235 res.setNameElement(parseString(json.get("name").getAsString())); 7236 if (json.has("_name")) 7237 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 7238 if (json.has("telecom")) { 7239 JsonArray array = json.getAsJsonArray("telecom"); 7240 for (int i = 0; i < array.size(); i++) { 7241 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 7242 } 7243 } 7244 ; 7245 return res; 7246 } 7247 7248 protected ImplementationGuide.ImplementationGuideDependencyComponent parseImplementationGuideImplementationGuideDependencyComponent( 7249 JsonObject json, ImplementationGuide owner) throws IOException, FHIRFormatError { 7250 ImplementationGuide.ImplementationGuideDependencyComponent res = new ImplementationGuide.ImplementationGuideDependencyComponent(); 7251 parseBackboneProperties(json, res); 7252 if (json.has("type")) 7253 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), ImplementationGuide.GuideDependencyType.NULL, 7254 new ImplementationGuide.GuideDependencyTypeEnumFactory())); 7255 if (json.has("_type")) 7256 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 7257 if (json.has("uri")) 7258 res.setUriElement(parseUri(json.get("uri").getAsString())); 7259 if (json.has("_uri")) 7260 parseElementProperties(json.getAsJsonObject("_uri"), res.getUriElement()); 7261 return res; 7262 } 7263 7264 protected ImplementationGuide.ImplementationGuidePackageComponent parseImplementationGuideImplementationGuidePackageComponent( 7265 JsonObject json, ImplementationGuide owner) throws IOException, FHIRFormatError { 7266 ImplementationGuide.ImplementationGuidePackageComponent res = new ImplementationGuide.ImplementationGuidePackageComponent(); 7267 parseBackboneProperties(json, res); 7268 if (json.has("name")) 7269 res.setNameElement(parseString(json.get("name").getAsString())); 7270 if (json.has("_name")) 7271 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 7272 if (json.has("description")) 7273 res.setDescriptionElement(parseString(json.get("description").getAsString())); 7274 if (json.has("_description")) 7275 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 7276 if (json.has("resource")) { 7277 JsonArray array = json.getAsJsonArray("resource"); 7278 for (int i = 0; i < array.size(); i++) { 7279 res.getResource().add( 7280 parseImplementationGuideImplementationGuidePackageResourceComponent(array.get(i).getAsJsonObject(), owner)); 7281 } 7282 } 7283 ; 7284 return res; 7285 } 7286 7287 protected ImplementationGuide.ImplementationGuidePackageResourceComponent parseImplementationGuideImplementationGuidePackageResourceComponent( 7288 JsonObject json, ImplementationGuide owner) throws IOException, FHIRFormatError { 7289 ImplementationGuide.ImplementationGuidePackageResourceComponent res = new ImplementationGuide.ImplementationGuidePackageResourceComponent(); 7290 parseBackboneProperties(json, res); 7291 if (json.has("purpose")) 7292 res.setPurposeElement(parseEnumeration(json.get("purpose").getAsString(), 7293 ImplementationGuide.GuideResourcePurpose.NULL, new ImplementationGuide.GuideResourcePurposeEnumFactory())); 7294 if (json.has("_purpose")) 7295 parseElementProperties(json.getAsJsonObject("_purpose"), res.getPurposeElement()); 7296 if (json.has("name")) 7297 res.setNameElement(parseString(json.get("name").getAsString())); 7298 if (json.has("_name")) 7299 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 7300 if (json.has("description")) 7301 res.setDescriptionElement(parseString(json.get("description").getAsString())); 7302 if (json.has("_description")) 7303 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 7304 if (json.has("acronym")) 7305 res.setAcronymElement(parseString(json.get("acronym").getAsString())); 7306 if (json.has("_acronym")) 7307 parseElementProperties(json.getAsJsonObject("_acronym"), res.getAcronymElement()); 7308 Type source = parseType("source", json); 7309 if (source != null) 7310 res.setSource(source); 7311 if (json.has("exampleFor")) 7312 res.setExampleFor(parseReference(json.getAsJsonObject("exampleFor"))); 7313 return res; 7314 } 7315 7316 protected ImplementationGuide.ImplementationGuideGlobalComponent parseImplementationGuideImplementationGuideGlobalComponent( 7317 JsonObject json, ImplementationGuide owner) throws IOException, FHIRFormatError { 7318 ImplementationGuide.ImplementationGuideGlobalComponent res = new ImplementationGuide.ImplementationGuideGlobalComponent(); 7319 parseBackboneProperties(json, res); 7320 if (json.has("type")) 7321 res.setTypeElement(parseCode(json.get("type").getAsString())); 7322 if (json.has("_type")) 7323 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 7324 if (json.has("profile")) 7325 res.setProfile(parseReference(json.getAsJsonObject("profile"))); 7326 return res; 7327 } 7328 7329 protected ImplementationGuide.ImplementationGuidePageComponent parseImplementationGuideImplementationGuidePageComponent( 7330 JsonObject json, ImplementationGuide owner) throws IOException, FHIRFormatError { 7331 ImplementationGuide.ImplementationGuidePageComponent res = new ImplementationGuide.ImplementationGuidePageComponent(); 7332 parseBackboneProperties(json, res); 7333 if (json.has("source")) 7334 res.setSourceElement(parseUri(json.get("source").getAsString())); 7335 if (json.has("_source")) 7336 parseElementProperties(json.getAsJsonObject("_source"), res.getSourceElement()); 7337 if (json.has("name")) 7338 res.setNameElement(parseString(json.get("name").getAsString())); 7339 if (json.has("_name")) 7340 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 7341 if (json.has("kind")) 7342 res.setKindElement(parseEnumeration(json.get("kind").getAsString(), ImplementationGuide.GuidePageKind.NULL, 7343 new ImplementationGuide.GuidePageKindEnumFactory())); 7344 if (json.has("_kind")) 7345 parseElementProperties(json.getAsJsonObject("_kind"), res.getKindElement()); 7346 if (json.has("type")) { 7347 JsonArray array = json.getAsJsonArray("type"); 7348 for (int i = 0; i < array.size(); i++) { 7349 res.getType().add(parseCode(array.get(i).getAsString())); 7350 } 7351 } 7352 ; 7353 if (json.has("_type")) { 7354 JsonArray array = json.getAsJsonArray("_type"); 7355 for (int i = 0; i < array.size(); i++) { 7356 if (i == res.getType().size()) 7357 res.getType().add(parseCode(null)); 7358 if (array.get(i) instanceof JsonObject) 7359 parseElementProperties(array.get(i).getAsJsonObject(), res.getType().get(i)); 7360 } 7361 } 7362 ; 7363 if (json.has("package")) { 7364 JsonArray array = json.getAsJsonArray("package"); 7365 for (int i = 0; i < array.size(); i++) { 7366 res.getPackage().add(parseString(array.get(i).getAsString())); 7367 } 7368 } 7369 ; 7370 if (json.has("_package")) { 7371 JsonArray array = json.getAsJsonArray("_package"); 7372 for (int i = 0; i < array.size(); i++) { 7373 if (i == res.getPackage().size()) 7374 res.getPackage().add(parseString(null)); 7375 if (array.get(i) instanceof JsonObject) 7376 parseElementProperties(array.get(i).getAsJsonObject(), res.getPackage().get(i)); 7377 } 7378 } 7379 ; 7380 if (json.has("format")) 7381 res.setFormatElement(parseCode(json.get("format").getAsString())); 7382 if (json.has("_format")) 7383 parseElementProperties(json.getAsJsonObject("_format"), res.getFormatElement()); 7384 if (json.has("page")) { 7385 JsonArray array = json.getAsJsonArray("page"); 7386 for (int i = 0; i < array.size(); i++) { 7387 res.getPage() 7388 .add(parseImplementationGuideImplementationGuidePageComponent(array.get(i).getAsJsonObject(), owner)); 7389 } 7390 } 7391 ; 7392 return res; 7393 } 7394 7395 protected List_ parseList_(JsonObject json) throws IOException, FHIRFormatError { 7396 List_ res = new List_(); 7397 parseDomainResourceProperties(json, res); 7398 if (json.has("identifier")) { 7399 JsonArray array = json.getAsJsonArray("identifier"); 7400 for (int i = 0; i < array.size(); i++) { 7401 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 7402 } 7403 } 7404 ; 7405 if (json.has("title")) 7406 res.setTitleElement(parseString(json.get("title").getAsString())); 7407 if (json.has("_title")) 7408 parseElementProperties(json.getAsJsonObject("_title"), res.getTitleElement()); 7409 if (json.has("code")) 7410 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 7411 if (json.has("subject")) 7412 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 7413 if (json.has("source")) 7414 res.setSource(parseReference(json.getAsJsonObject("source"))); 7415 if (json.has("encounter")) 7416 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 7417 if (json.has("status")) 7418 res.setStatusElement( 7419 parseEnumeration(json.get("status").getAsString(), List_.ListStatus.NULL, new List_.ListStatusEnumFactory())); 7420 if (json.has("_status")) 7421 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 7422 if (json.has("date")) 7423 res.setDateElement(parseDateTime(json.get("date").getAsString())); 7424 if (json.has("_date")) 7425 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 7426 if (json.has("orderedBy")) 7427 res.setOrderedBy(parseCodeableConcept(json.getAsJsonObject("orderedBy"))); 7428 if (json.has("mode")) 7429 res.setModeElement( 7430 parseEnumeration(json.get("mode").getAsString(), List_.ListMode.NULL, new List_.ListModeEnumFactory())); 7431 if (json.has("_mode")) 7432 parseElementProperties(json.getAsJsonObject("_mode"), res.getModeElement()); 7433 if (json.has("note")) 7434 res.setNoteElement(parseString(json.get("note").getAsString())); 7435 if (json.has("_note")) 7436 parseElementProperties(json.getAsJsonObject("_note"), res.getNoteElement()); 7437 if (json.has("entry")) { 7438 JsonArray array = json.getAsJsonArray("entry"); 7439 for (int i = 0; i < array.size(); i++) { 7440 res.getEntry().add(parseList_ListEntryComponent(array.get(i).getAsJsonObject(), res)); 7441 } 7442 } 7443 ; 7444 if (json.has("emptyReason")) 7445 res.setEmptyReason(parseCodeableConcept(json.getAsJsonObject("emptyReason"))); 7446 return res; 7447 } 7448 7449 protected List_.ListEntryComponent parseList_ListEntryComponent(JsonObject json, List_ owner) 7450 throws IOException, FHIRFormatError { 7451 List_.ListEntryComponent res = new List_.ListEntryComponent(); 7452 parseBackboneProperties(json, res); 7453 if (json.has("flag")) 7454 res.setFlag(parseCodeableConcept(json.getAsJsonObject("flag"))); 7455 if (json.has("deleted")) 7456 res.setDeletedElement(parseBoolean(json.get("deleted").getAsBoolean())); 7457 if (json.has("_deleted")) 7458 parseElementProperties(json.getAsJsonObject("_deleted"), res.getDeletedElement()); 7459 if (json.has("date")) 7460 res.setDateElement(parseDateTime(json.get("date").getAsString())); 7461 if (json.has("_date")) 7462 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 7463 if (json.has("item")) 7464 res.setItem(parseReference(json.getAsJsonObject("item"))); 7465 return res; 7466 } 7467 7468 protected Location parseLocation(JsonObject json) throws IOException, FHIRFormatError { 7469 Location res = new Location(); 7470 parseDomainResourceProperties(json, res); 7471 if (json.has("identifier")) { 7472 JsonArray array = json.getAsJsonArray("identifier"); 7473 for (int i = 0; i < array.size(); i++) { 7474 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 7475 } 7476 } 7477 ; 7478 if (json.has("status")) 7479 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Location.LocationStatus.NULL, 7480 new Location.LocationStatusEnumFactory())); 7481 if (json.has("_status")) 7482 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 7483 if (json.has("name")) 7484 res.setNameElement(parseString(json.get("name").getAsString())); 7485 if (json.has("_name")) 7486 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 7487 if (json.has("description")) 7488 res.setDescriptionElement(parseString(json.get("description").getAsString())); 7489 if (json.has("_description")) 7490 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 7491 if (json.has("mode")) 7492 res.setModeElement(parseEnumeration(json.get("mode").getAsString(), Location.LocationMode.NULL, 7493 new Location.LocationModeEnumFactory())); 7494 if (json.has("_mode")) 7495 parseElementProperties(json.getAsJsonObject("_mode"), res.getModeElement()); 7496 if (json.has("type")) 7497 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 7498 if (json.has("telecom")) { 7499 JsonArray array = json.getAsJsonArray("telecom"); 7500 for (int i = 0; i < array.size(); i++) { 7501 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 7502 } 7503 } 7504 ; 7505 if (json.has("address")) 7506 res.setAddress(parseAddress(json.getAsJsonObject("address"))); 7507 if (json.has("physicalType")) 7508 res.setPhysicalType(parseCodeableConcept(json.getAsJsonObject("physicalType"))); 7509 if (json.has("position")) 7510 res.setPosition(parseLocationLocationPositionComponent(json.getAsJsonObject("position"), res)); 7511 if (json.has("managingOrganization")) 7512 res.setManagingOrganization(parseReference(json.getAsJsonObject("managingOrganization"))); 7513 if (json.has("partOf")) 7514 res.setPartOf(parseReference(json.getAsJsonObject("partOf"))); 7515 return res; 7516 } 7517 7518 protected Location.LocationPositionComponent parseLocationLocationPositionComponent(JsonObject json, Location owner) 7519 throws IOException, FHIRFormatError { 7520 Location.LocationPositionComponent res = new Location.LocationPositionComponent(); 7521 parseBackboneProperties(json, res); 7522 if (json.has("longitude")) 7523 res.setLongitudeElement(parseDecimal(json.get("longitude").getAsBigDecimal())); 7524 if (json.has("_longitude")) 7525 parseElementProperties(json.getAsJsonObject("_longitude"), res.getLongitudeElement()); 7526 if (json.has("latitude")) 7527 res.setLatitudeElement(parseDecimal(json.get("latitude").getAsBigDecimal())); 7528 if (json.has("_latitude")) 7529 parseElementProperties(json.getAsJsonObject("_latitude"), res.getLatitudeElement()); 7530 if (json.has("altitude")) 7531 res.setAltitudeElement(parseDecimal(json.get("altitude").getAsBigDecimal())); 7532 if (json.has("_altitude")) 7533 parseElementProperties(json.getAsJsonObject("_altitude"), res.getAltitudeElement()); 7534 return res; 7535 } 7536 7537 protected Media parseMedia(JsonObject json) throws IOException, FHIRFormatError { 7538 Media res = new Media(); 7539 parseDomainResourceProperties(json, res); 7540 if (json.has("type")) 7541 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Media.DigitalMediaType.NULL, 7542 new Media.DigitalMediaTypeEnumFactory())); 7543 if (json.has("_type")) 7544 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 7545 if (json.has("subtype")) 7546 res.setSubtype(parseCodeableConcept(json.getAsJsonObject("subtype"))); 7547 if (json.has("identifier")) { 7548 JsonArray array = json.getAsJsonArray("identifier"); 7549 for (int i = 0; i < array.size(); i++) { 7550 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 7551 } 7552 } 7553 ; 7554 if (json.has("subject")) 7555 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 7556 if (json.has("operator")) 7557 res.setOperator(parseReference(json.getAsJsonObject("operator"))); 7558 if (json.has("view")) 7559 res.setView(parseCodeableConcept(json.getAsJsonObject("view"))); 7560 if (json.has("deviceName")) 7561 res.setDeviceNameElement(parseString(json.get("deviceName").getAsString())); 7562 if (json.has("_deviceName")) 7563 parseElementProperties(json.getAsJsonObject("_deviceName"), res.getDeviceNameElement()); 7564 if (json.has("height")) 7565 res.setHeightElement(parsePositiveInt(json.get("height").getAsString())); 7566 if (json.has("_height")) 7567 parseElementProperties(json.getAsJsonObject("_height"), res.getHeightElement()); 7568 if (json.has("width")) 7569 res.setWidthElement(parsePositiveInt(json.get("width").getAsString())); 7570 if (json.has("_width")) 7571 parseElementProperties(json.getAsJsonObject("_width"), res.getWidthElement()); 7572 if (json.has("frames")) 7573 res.setFramesElement(parsePositiveInt(json.get("frames").getAsString())); 7574 if (json.has("_frames")) 7575 parseElementProperties(json.getAsJsonObject("_frames"), res.getFramesElement()); 7576 if (json.has("duration")) 7577 res.setDurationElement(parseUnsignedInt(json.get("duration").getAsString())); 7578 if (json.has("_duration")) 7579 parseElementProperties(json.getAsJsonObject("_duration"), res.getDurationElement()); 7580 if (json.has("content")) 7581 res.setContent(parseAttachment(json.getAsJsonObject("content"))); 7582 return res; 7583 } 7584 7585 protected Medication parseMedication(JsonObject json) throws IOException, FHIRFormatError { 7586 Medication res = new Medication(); 7587 parseDomainResourceProperties(json, res); 7588 if (json.has("code")) 7589 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 7590 if (json.has("isBrand")) 7591 res.setIsBrandElement(parseBoolean(json.get("isBrand").getAsBoolean())); 7592 if (json.has("_isBrand")) 7593 parseElementProperties(json.getAsJsonObject("_isBrand"), res.getIsBrandElement()); 7594 if (json.has("manufacturer")) 7595 res.setManufacturer(parseReference(json.getAsJsonObject("manufacturer"))); 7596 if (json.has("product")) 7597 res.setProduct(parseMedicationMedicationProductComponent(json.getAsJsonObject("product"), res)); 7598 if (json.has("package")) 7599 res.setPackage(parseMedicationMedicationPackageComponent(json.getAsJsonObject("package"), res)); 7600 return res; 7601 } 7602 7603 protected Medication.MedicationProductComponent parseMedicationMedicationProductComponent(JsonObject json, 7604 Medication owner) throws IOException, FHIRFormatError { 7605 Medication.MedicationProductComponent res = new Medication.MedicationProductComponent(); 7606 parseBackboneProperties(json, res); 7607 if (json.has("form")) 7608 res.setForm(parseCodeableConcept(json.getAsJsonObject("form"))); 7609 if (json.has("ingredient")) { 7610 JsonArray array = json.getAsJsonArray("ingredient"); 7611 for (int i = 0; i < array.size(); i++) { 7612 res.getIngredient() 7613 .add(parseMedicationMedicationProductIngredientComponent(array.get(i).getAsJsonObject(), owner)); 7614 } 7615 } 7616 ; 7617 if (json.has("batch")) { 7618 JsonArray array = json.getAsJsonArray("batch"); 7619 for (int i = 0; i < array.size(); i++) { 7620 res.getBatch().add(parseMedicationMedicationProductBatchComponent(array.get(i).getAsJsonObject(), owner)); 7621 } 7622 } 7623 ; 7624 return res; 7625 } 7626 7627 protected Medication.MedicationProductIngredientComponent parseMedicationMedicationProductIngredientComponent( 7628 JsonObject json, Medication owner) throws IOException, FHIRFormatError { 7629 Medication.MedicationProductIngredientComponent res = new Medication.MedicationProductIngredientComponent(); 7630 parseBackboneProperties(json, res); 7631 if (json.has("item")) 7632 res.setItem(parseReference(json.getAsJsonObject("item"))); 7633 if (json.has("amount")) 7634 res.setAmount(parseRatio(json.getAsJsonObject("amount"))); 7635 return res; 7636 } 7637 7638 protected Medication.MedicationProductBatchComponent parseMedicationMedicationProductBatchComponent(JsonObject json, 7639 Medication owner) throws IOException, FHIRFormatError { 7640 Medication.MedicationProductBatchComponent res = new Medication.MedicationProductBatchComponent(); 7641 parseBackboneProperties(json, res); 7642 if (json.has("lotNumber")) 7643 res.setLotNumberElement(parseString(json.get("lotNumber").getAsString())); 7644 if (json.has("_lotNumber")) 7645 parseElementProperties(json.getAsJsonObject("_lotNumber"), res.getLotNumberElement()); 7646 if (json.has("expirationDate")) 7647 res.setExpirationDateElement(parseDateTime(json.get("expirationDate").getAsString())); 7648 if (json.has("_expirationDate")) 7649 parseElementProperties(json.getAsJsonObject("_expirationDate"), res.getExpirationDateElement()); 7650 return res; 7651 } 7652 7653 protected Medication.MedicationPackageComponent parseMedicationMedicationPackageComponent(JsonObject json, 7654 Medication owner) throws IOException, FHIRFormatError { 7655 Medication.MedicationPackageComponent res = new Medication.MedicationPackageComponent(); 7656 parseBackboneProperties(json, res); 7657 if (json.has("container")) 7658 res.setContainer(parseCodeableConcept(json.getAsJsonObject("container"))); 7659 if (json.has("content")) { 7660 JsonArray array = json.getAsJsonArray("content"); 7661 for (int i = 0; i < array.size(); i++) { 7662 res.getContent().add(parseMedicationMedicationPackageContentComponent(array.get(i).getAsJsonObject(), owner)); 7663 } 7664 } 7665 ; 7666 return res; 7667 } 7668 7669 protected Medication.MedicationPackageContentComponent parseMedicationMedicationPackageContentComponent( 7670 JsonObject json, Medication owner) throws IOException, FHIRFormatError { 7671 Medication.MedicationPackageContentComponent res = new Medication.MedicationPackageContentComponent(); 7672 parseBackboneProperties(json, res); 7673 if (json.has("item")) 7674 res.setItem(parseReference(json.getAsJsonObject("item"))); 7675 if (json.has("amount")) 7676 res.setAmount(parseSimpleQuantity(json.getAsJsonObject("amount"))); 7677 return res; 7678 } 7679 7680 protected MedicationAdministration parseMedicationAdministration(JsonObject json) 7681 throws IOException, FHIRFormatError { 7682 MedicationAdministration res = new MedicationAdministration(); 7683 parseDomainResourceProperties(json, res); 7684 if (json.has("identifier")) { 7685 JsonArray array = json.getAsJsonArray("identifier"); 7686 for (int i = 0; i < array.size(); i++) { 7687 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 7688 } 7689 } 7690 ; 7691 if (json.has("status")) 7692 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 7693 MedicationAdministration.MedicationAdministrationStatus.NULL, 7694 new MedicationAdministration.MedicationAdministrationStatusEnumFactory())); 7695 if (json.has("_status")) 7696 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 7697 if (json.has("patient")) 7698 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 7699 if (json.has("practitioner")) 7700 res.setPractitioner(parseReference(json.getAsJsonObject("practitioner"))); 7701 if (json.has("encounter")) 7702 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 7703 if (json.has("prescription")) 7704 res.setPrescription(parseReference(json.getAsJsonObject("prescription"))); 7705 if (json.has("wasNotGiven")) 7706 res.setWasNotGivenElement(parseBoolean(json.get("wasNotGiven").getAsBoolean())); 7707 if (json.has("_wasNotGiven")) 7708 parseElementProperties(json.getAsJsonObject("_wasNotGiven"), res.getWasNotGivenElement()); 7709 if (json.has("reasonNotGiven")) { 7710 JsonArray array = json.getAsJsonArray("reasonNotGiven"); 7711 for (int i = 0; i < array.size(); i++) { 7712 res.getReasonNotGiven().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 7713 } 7714 } 7715 ; 7716 if (json.has("reasonGiven")) { 7717 JsonArray array = json.getAsJsonArray("reasonGiven"); 7718 for (int i = 0; i < array.size(); i++) { 7719 res.getReasonGiven().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 7720 } 7721 } 7722 ; 7723 Type effectiveTime = parseType("effectiveTime", json); 7724 if (effectiveTime != null) 7725 res.setEffectiveTime(effectiveTime); 7726 Type medication = parseType("medication", json); 7727 if (medication != null) 7728 res.setMedication(medication); 7729 if (json.has("device")) { 7730 JsonArray array = json.getAsJsonArray("device"); 7731 for (int i = 0; i < array.size(); i++) { 7732 res.getDevice().add(parseReference(array.get(i).getAsJsonObject())); 7733 } 7734 } 7735 ; 7736 if (json.has("note")) 7737 res.setNoteElement(parseString(json.get("note").getAsString())); 7738 if (json.has("_note")) 7739 parseElementProperties(json.getAsJsonObject("_note"), res.getNoteElement()); 7740 if (json.has("dosage")) 7741 res.setDosage( 7742 parseMedicationAdministrationMedicationAdministrationDosageComponent(json.getAsJsonObject("dosage"), res)); 7743 return res; 7744 } 7745 7746 protected MedicationAdministration.MedicationAdministrationDosageComponent parseMedicationAdministrationMedicationAdministrationDosageComponent( 7747 JsonObject json, MedicationAdministration owner) throws IOException, FHIRFormatError { 7748 MedicationAdministration.MedicationAdministrationDosageComponent res = new MedicationAdministration.MedicationAdministrationDosageComponent(); 7749 parseBackboneProperties(json, res); 7750 if (json.has("text")) 7751 res.setTextElement(parseString(json.get("text").getAsString())); 7752 if (json.has("_text")) 7753 parseElementProperties(json.getAsJsonObject("_text"), res.getTextElement()); 7754 Type site = parseType("site", json); 7755 if (site != null) 7756 res.setSite(site); 7757 if (json.has("route")) 7758 res.setRoute(parseCodeableConcept(json.getAsJsonObject("route"))); 7759 if (json.has("method")) 7760 res.setMethod(parseCodeableConcept(json.getAsJsonObject("method"))); 7761 if (json.has("quantity")) 7762 res.setQuantity(parseSimpleQuantity(json.getAsJsonObject("quantity"))); 7763 Type rate = parseType("rate", json); 7764 if (rate != null) 7765 res.setRate(rate); 7766 return res; 7767 } 7768 7769 protected MedicationDispense parseMedicationDispense(JsonObject json) throws IOException, FHIRFormatError { 7770 MedicationDispense res = new MedicationDispense(); 7771 parseDomainResourceProperties(json, res); 7772 if (json.has("identifier")) 7773 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 7774 if (json.has("status")) 7775 res.setStatusElement( 7776 parseEnumeration(json.get("status").getAsString(), MedicationDispense.MedicationDispenseStatus.NULL, 7777 new MedicationDispense.MedicationDispenseStatusEnumFactory())); 7778 if (json.has("_status")) 7779 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 7780 if (json.has("patient")) 7781 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 7782 if (json.has("dispenser")) 7783 res.setDispenser(parseReference(json.getAsJsonObject("dispenser"))); 7784 if (json.has("authorizingPrescription")) { 7785 JsonArray array = json.getAsJsonArray("authorizingPrescription"); 7786 for (int i = 0; i < array.size(); i++) { 7787 res.getAuthorizingPrescription().add(parseReference(array.get(i).getAsJsonObject())); 7788 } 7789 } 7790 ; 7791 if (json.has("type")) 7792 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 7793 if (json.has("quantity")) 7794 res.setQuantity(parseSimpleQuantity(json.getAsJsonObject("quantity"))); 7795 if (json.has("daysSupply")) 7796 res.setDaysSupply(parseSimpleQuantity(json.getAsJsonObject("daysSupply"))); 7797 Type medication = parseType("medication", json); 7798 if (medication != null) 7799 res.setMedication(medication); 7800 if (json.has("whenPrepared")) 7801 res.setWhenPreparedElement(parseDateTime(json.get("whenPrepared").getAsString())); 7802 if (json.has("_whenPrepared")) 7803 parseElementProperties(json.getAsJsonObject("_whenPrepared"), res.getWhenPreparedElement()); 7804 if (json.has("whenHandedOver")) 7805 res.setWhenHandedOverElement(parseDateTime(json.get("whenHandedOver").getAsString())); 7806 if (json.has("_whenHandedOver")) 7807 parseElementProperties(json.getAsJsonObject("_whenHandedOver"), res.getWhenHandedOverElement()); 7808 if (json.has("destination")) 7809 res.setDestination(parseReference(json.getAsJsonObject("destination"))); 7810 if (json.has("receiver")) { 7811 JsonArray array = json.getAsJsonArray("receiver"); 7812 for (int i = 0; i < array.size(); i++) { 7813 res.getReceiver().add(parseReference(array.get(i).getAsJsonObject())); 7814 } 7815 } 7816 ; 7817 if (json.has("note")) 7818 res.setNoteElement(parseString(json.get("note").getAsString())); 7819 if (json.has("_note")) 7820 parseElementProperties(json.getAsJsonObject("_note"), res.getNoteElement()); 7821 if (json.has("dosageInstruction")) { 7822 JsonArray array = json.getAsJsonArray("dosageInstruction"); 7823 for (int i = 0; i < array.size(); i++) { 7824 res.getDosageInstruction().add( 7825 parseMedicationDispenseMedicationDispenseDosageInstructionComponent(array.get(i).getAsJsonObject(), res)); 7826 } 7827 } 7828 ; 7829 if (json.has("substitution")) 7830 res.setSubstitution( 7831 parseMedicationDispenseMedicationDispenseSubstitutionComponent(json.getAsJsonObject("substitution"), res)); 7832 return res; 7833 } 7834 7835 protected MedicationDispense.MedicationDispenseDosageInstructionComponent parseMedicationDispenseMedicationDispenseDosageInstructionComponent( 7836 JsonObject json, MedicationDispense owner) throws IOException, FHIRFormatError { 7837 MedicationDispense.MedicationDispenseDosageInstructionComponent res = new MedicationDispense.MedicationDispenseDosageInstructionComponent(); 7838 parseBackboneProperties(json, res); 7839 if (json.has("text")) 7840 res.setTextElement(parseString(json.get("text").getAsString())); 7841 if (json.has("_text")) 7842 parseElementProperties(json.getAsJsonObject("_text"), res.getTextElement()); 7843 if (json.has("additionalInstructions")) 7844 res.setAdditionalInstructions(parseCodeableConcept(json.getAsJsonObject("additionalInstructions"))); 7845 if (json.has("timing")) 7846 res.setTiming(parseTiming(json.getAsJsonObject("timing"))); 7847 Type asNeeded = parseType("asNeeded", json); 7848 if (asNeeded != null) 7849 res.setAsNeeded(asNeeded); 7850 Type site = parseType("site", json); 7851 if (site != null) 7852 res.setSite(site); 7853 if (json.has("route")) 7854 res.setRoute(parseCodeableConcept(json.getAsJsonObject("route"))); 7855 if (json.has("method")) 7856 res.setMethod(parseCodeableConcept(json.getAsJsonObject("method"))); 7857 Type dose = parseType("dose", json); 7858 if (dose != null) 7859 res.setDose(dose); 7860 Type rate = parseType("rate", json); 7861 if (rate != null) 7862 res.setRate(rate); 7863 if (json.has("maxDosePerPeriod")) 7864 res.setMaxDosePerPeriod(parseRatio(json.getAsJsonObject("maxDosePerPeriod"))); 7865 return res; 7866 } 7867 7868 protected MedicationDispense.MedicationDispenseSubstitutionComponent parseMedicationDispenseMedicationDispenseSubstitutionComponent( 7869 JsonObject json, MedicationDispense owner) throws IOException, FHIRFormatError { 7870 MedicationDispense.MedicationDispenseSubstitutionComponent res = new MedicationDispense.MedicationDispenseSubstitutionComponent(); 7871 parseBackboneProperties(json, res); 7872 if (json.has("type")) 7873 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 7874 if (json.has("reason")) { 7875 JsonArray array = json.getAsJsonArray("reason"); 7876 for (int i = 0; i < array.size(); i++) { 7877 res.getReason().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 7878 } 7879 } 7880 ; 7881 if (json.has("responsibleParty")) { 7882 JsonArray array = json.getAsJsonArray("responsibleParty"); 7883 for (int i = 0; i < array.size(); i++) { 7884 res.getResponsibleParty().add(parseReference(array.get(i).getAsJsonObject())); 7885 } 7886 } 7887 ; 7888 return res; 7889 } 7890 7891 protected MedicationOrder parseMedicationOrder(JsonObject json) throws IOException, FHIRFormatError { 7892 MedicationOrder res = new MedicationOrder(); 7893 parseDomainResourceProperties(json, res); 7894 if (json.has("identifier")) { 7895 JsonArray array = json.getAsJsonArray("identifier"); 7896 for (int i = 0; i < array.size(); i++) { 7897 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 7898 } 7899 } 7900 ; 7901 if (json.has("dateWritten")) 7902 res.setDateWrittenElement(parseDateTime(json.get("dateWritten").getAsString())); 7903 if (json.has("_dateWritten")) 7904 parseElementProperties(json.getAsJsonObject("_dateWritten"), res.getDateWrittenElement()); 7905 if (json.has("status")) 7906 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 7907 MedicationOrder.MedicationOrderStatus.NULL, new MedicationOrder.MedicationOrderStatusEnumFactory())); 7908 if (json.has("_status")) 7909 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 7910 if (json.has("dateEnded")) 7911 res.setDateEndedElement(parseDateTime(json.get("dateEnded").getAsString())); 7912 if (json.has("_dateEnded")) 7913 parseElementProperties(json.getAsJsonObject("_dateEnded"), res.getDateEndedElement()); 7914 if (json.has("reasonEnded")) 7915 res.setReasonEnded(parseCodeableConcept(json.getAsJsonObject("reasonEnded"))); 7916 if (json.has("patient")) 7917 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 7918 if (json.has("prescriber")) 7919 res.setPrescriber(parseReference(json.getAsJsonObject("prescriber"))); 7920 if (json.has("encounter")) 7921 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 7922 Type reason = parseType("reason", json); 7923 if (reason != null) 7924 res.setReason(reason); 7925 if (json.has("note")) 7926 res.setNoteElement(parseString(json.get("note").getAsString())); 7927 if (json.has("_note")) 7928 parseElementProperties(json.getAsJsonObject("_note"), res.getNoteElement()); 7929 Type medication = parseType("medication", json); 7930 if (medication != null) 7931 res.setMedication(medication); 7932 if (json.has("dosageInstruction")) { 7933 JsonArray array = json.getAsJsonArray("dosageInstruction"); 7934 for (int i = 0; i < array.size(); i++) { 7935 res.getDosageInstruction() 7936 .add(parseMedicationOrderMedicationOrderDosageInstructionComponent(array.get(i).getAsJsonObject(), res)); 7937 } 7938 } 7939 ; 7940 if (json.has("dispenseRequest")) 7941 res.setDispenseRequest( 7942 parseMedicationOrderMedicationOrderDispenseRequestComponent(json.getAsJsonObject("dispenseRequest"), res)); 7943 if (json.has("substitution")) 7944 res.setSubstitution( 7945 parseMedicationOrderMedicationOrderSubstitutionComponent(json.getAsJsonObject("substitution"), res)); 7946 if (json.has("priorPrescription")) 7947 res.setPriorPrescription(parseReference(json.getAsJsonObject("priorPrescription"))); 7948 return res; 7949 } 7950 7951 protected MedicationOrder.MedicationOrderDosageInstructionComponent parseMedicationOrderMedicationOrderDosageInstructionComponent( 7952 JsonObject json, MedicationOrder owner) throws IOException, FHIRFormatError { 7953 MedicationOrder.MedicationOrderDosageInstructionComponent res = new MedicationOrder.MedicationOrderDosageInstructionComponent(); 7954 parseBackboneProperties(json, res); 7955 if (json.has("text")) 7956 res.setTextElement(parseString(json.get("text").getAsString())); 7957 if (json.has("_text")) 7958 parseElementProperties(json.getAsJsonObject("_text"), res.getTextElement()); 7959 if (json.has("additionalInstructions")) 7960 res.setAdditionalInstructions(parseCodeableConcept(json.getAsJsonObject("additionalInstructions"))); 7961 if (json.has("timing")) 7962 res.setTiming(parseTiming(json.getAsJsonObject("timing"))); 7963 Type asNeeded = parseType("asNeeded", json); 7964 if (asNeeded != null) 7965 res.setAsNeeded(asNeeded); 7966 Type site = parseType("site", json); 7967 if (site != null) 7968 res.setSite(site); 7969 if (json.has("route")) 7970 res.setRoute(parseCodeableConcept(json.getAsJsonObject("route"))); 7971 if (json.has("method")) 7972 res.setMethod(parseCodeableConcept(json.getAsJsonObject("method"))); 7973 Type dose = parseType("dose", json); 7974 if (dose != null) 7975 res.setDose(dose); 7976 Type rate = parseType("rate", json); 7977 if (rate != null) 7978 res.setRate(rate); 7979 if (json.has("maxDosePerPeriod")) 7980 res.setMaxDosePerPeriod(parseRatio(json.getAsJsonObject("maxDosePerPeriod"))); 7981 return res; 7982 } 7983 7984 protected MedicationOrder.MedicationOrderDispenseRequestComponent parseMedicationOrderMedicationOrderDispenseRequestComponent( 7985 JsonObject json, MedicationOrder owner) throws IOException, FHIRFormatError { 7986 MedicationOrder.MedicationOrderDispenseRequestComponent res = new MedicationOrder.MedicationOrderDispenseRequestComponent(); 7987 parseBackboneProperties(json, res); 7988 Type medication = parseType("medication", json); 7989 if (medication != null) 7990 res.setMedication(medication); 7991 if (json.has("validityPeriod")) 7992 res.setValidityPeriod(parsePeriod(json.getAsJsonObject("validityPeriod"))); 7993 if (json.has("numberOfRepeatsAllowed")) 7994 res.setNumberOfRepeatsAllowedElement(parsePositiveInt(json.get("numberOfRepeatsAllowed").getAsString())); 7995 if (json.has("_numberOfRepeatsAllowed")) 7996 parseElementProperties(json.getAsJsonObject("_numberOfRepeatsAllowed"), res.getNumberOfRepeatsAllowedElement()); 7997 if (json.has("quantity")) 7998 res.setQuantity(parseSimpleQuantity(json.getAsJsonObject("quantity"))); 7999 if (json.has("expectedSupplyDuration")) 8000 res.setExpectedSupplyDuration(parseDuration(json.getAsJsonObject("expectedSupplyDuration"))); 8001 return res; 8002 } 8003 8004 protected MedicationOrder.MedicationOrderSubstitutionComponent parseMedicationOrderMedicationOrderSubstitutionComponent( 8005 JsonObject json, MedicationOrder owner) throws IOException, FHIRFormatError { 8006 MedicationOrder.MedicationOrderSubstitutionComponent res = new MedicationOrder.MedicationOrderSubstitutionComponent(); 8007 parseBackboneProperties(json, res); 8008 if (json.has("type")) 8009 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 8010 if (json.has("reason")) 8011 res.setReason(parseCodeableConcept(json.getAsJsonObject("reason"))); 8012 return res; 8013 } 8014 8015 protected MedicationStatement parseMedicationStatement(JsonObject json) throws IOException, FHIRFormatError { 8016 MedicationStatement res = new MedicationStatement(); 8017 parseDomainResourceProperties(json, res); 8018 if (json.has("identifier")) { 8019 JsonArray array = json.getAsJsonArray("identifier"); 8020 for (int i = 0; i < array.size(); i++) { 8021 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 8022 } 8023 } 8024 ; 8025 if (json.has("patient")) 8026 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 8027 if (json.has("informationSource")) 8028 res.setInformationSource(parseReference(json.getAsJsonObject("informationSource"))); 8029 if (json.has("dateAsserted")) 8030 res.setDateAssertedElement(parseDateTime(json.get("dateAsserted").getAsString())); 8031 if (json.has("_dateAsserted")) 8032 parseElementProperties(json.getAsJsonObject("_dateAsserted"), res.getDateAssertedElement()); 8033 if (json.has("status")) 8034 res.setStatusElement( 8035 parseEnumeration(json.get("status").getAsString(), MedicationStatement.MedicationStatementStatus.NULL, 8036 new MedicationStatement.MedicationStatementStatusEnumFactory())); 8037 if (json.has("_status")) 8038 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 8039 if (json.has("wasNotTaken")) 8040 res.setWasNotTakenElement(parseBoolean(json.get("wasNotTaken").getAsBoolean())); 8041 if (json.has("_wasNotTaken")) 8042 parseElementProperties(json.getAsJsonObject("_wasNotTaken"), res.getWasNotTakenElement()); 8043 if (json.has("reasonNotTaken")) { 8044 JsonArray array = json.getAsJsonArray("reasonNotTaken"); 8045 for (int i = 0; i < array.size(); i++) { 8046 res.getReasonNotTaken().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 8047 } 8048 } 8049 ; 8050 Type reasonForUse = parseType("reasonForUse", json); 8051 if (reasonForUse != null) 8052 res.setReasonForUse(reasonForUse); 8053 Type effective = parseType("effective", json); 8054 if (effective != null) 8055 res.setEffective(effective); 8056 if (json.has("note")) 8057 res.setNoteElement(parseString(json.get("note").getAsString())); 8058 if (json.has("_note")) 8059 parseElementProperties(json.getAsJsonObject("_note"), res.getNoteElement()); 8060 if (json.has("supportingInformation")) { 8061 JsonArray array = json.getAsJsonArray("supportingInformation"); 8062 for (int i = 0; i < array.size(); i++) { 8063 res.getSupportingInformation().add(parseReference(array.get(i).getAsJsonObject())); 8064 } 8065 } 8066 ; 8067 Type medication = parseType("medication", json); 8068 if (medication != null) 8069 res.setMedication(medication); 8070 if (json.has("dosage")) { 8071 JsonArray array = json.getAsJsonArray("dosage"); 8072 for (int i = 0; i < array.size(); i++) { 8073 res.getDosage() 8074 .add(parseMedicationStatementMedicationStatementDosageComponent(array.get(i).getAsJsonObject(), res)); 8075 } 8076 } 8077 ; 8078 return res; 8079 } 8080 8081 protected MedicationStatement.MedicationStatementDosageComponent parseMedicationStatementMedicationStatementDosageComponent( 8082 JsonObject json, MedicationStatement owner) throws IOException, FHIRFormatError { 8083 MedicationStatement.MedicationStatementDosageComponent res = new MedicationStatement.MedicationStatementDosageComponent(); 8084 parseBackboneProperties(json, res); 8085 if (json.has("text")) 8086 res.setTextElement(parseString(json.get("text").getAsString())); 8087 if (json.has("_text")) 8088 parseElementProperties(json.getAsJsonObject("_text"), res.getTextElement()); 8089 if (json.has("timing")) 8090 res.setTiming(parseTiming(json.getAsJsonObject("timing"))); 8091 Type asNeeded = parseType("asNeeded", json); 8092 if (asNeeded != null) 8093 res.setAsNeeded(asNeeded); 8094 Type site = parseType("site", json); 8095 if (site != null) 8096 res.setSite(site); 8097 if (json.has("route")) 8098 res.setRoute(parseCodeableConcept(json.getAsJsonObject("route"))); 8099 if (json.has("method")) 8100 res.setMethod(parseCodeableConcept(json.getAsJsonObject("method"))); 8101 Type quantity = parseType("quantity", json); 8102 if (quantity != null) 8103 res.setQuantity(quantity); 8104 Type rate = parseType("rate", json); 8105 if (rate != null) 8106 res.setRate(rate); 8107 if (json.has("maxDosePerPeriod")) 8108 res.setMaxDosePerPeriod(parseRatio(json.getAsJsonObject("maxDosePerPeriod"))); 8109 return res; 8110 } 8111 8112 protected MessageHeader parseMessageHeader(JsonObject json) throws IOException, FHIRFormatError { 8113 MessageHeader res = new MessageHeader(); 8114 parseDomainResourceProperties(json, res); 8115 if (json.has("timestamp")) 8116 res.setTimestampElement(parseInstant(json.get("timestamp").getAsString())); 8117 if (json.has("_timestamp")) 8118 parseElementProperties(json.getAsJsonObject("_timestamp"), res.getTimestampElement()); 8119 if (json.has("event")) 8120 res.setEvent(parseCoding(json.getAsJsonObject("event"))); 8121 if (json.has("response")) 8122 res.setResponse(parseMessageHeaderMessageHeaderResponseComponent(json.getAsJsonObject("response"), res)); 8123 if (json.has("source")) 8124 res.setSource(parseMessageHeaderMessageSourceComponent(json.getAsJsonObject("source"), res)); 8125 if (json.has("destination")) { 8126 JsonArray array = json.getAsJsonArray("destination"); 8127 for (int i = 0; i < array.size(); i++) { 8128 res.getDestination().add(parseMessageHeaderMessageDestinationComponent(array.get(i).getAsJsonObject(), res)); 8129 } 8130 } 8131 ; 8132 if (json.has("enterer")) 8133 res.setEnterer(parseReference(json.getAsJsonObject("enterer"))); 8134 if (json.has("author")) 8135 res.setAuthor(parseReference(json.getAsJsonObject("author"))); 8136 if (json.has("receiver")) 8137 res.setReceiver(parseReference(json.getAsJsonObject("receiver"))); 8138 if (json.has("responsible")) 8139 res.setResponsible(parseReference(json.getAsJsonObject("responsible"))); 8140 if (json.has("reason")) 8141 res.setReason(parseCodeableConcept(json.getAsJsonObject("reason"))); 8142 if (json.has("data")) { 8143 JsonArray array = json.getAsJsonArray("data"); 8144 for (int i = 0; i < array.size(); i++) { 8145 res.getData().add(parseReference(array.get(i).getAsJsonObject())); 8146 } 8147 } 8148 ; 8149 return res; 8150 } 8151 8152 protected MessageHeader.MessageHeaderResponseComponent parseMessageHeaderMessageHeaderResponseComponent( 8153 JsonObject json, MessageHeader owner) throws IOException, FHIRFormatError { 8154 MessageHeader.MessageHeaderResponseComponent res = new MessageHeader.MessageHeaderResponseComponent(); 8155 parseBackboneProperties(json, res); 8156 if (json.has("identifier")) 8157 res.setIdentifierElement(parseId(json.get("identifier").getAsString())); 8158 if (json.has("_identifier")) 8159 parseElementProperties(json.getAsJsonObject("_identifier"), res.getIdentifierElement()); 8160 if (json.has("code")) 8161 res.setCodeElement(parseEnumeration(json.get("code").getAsString(), MessageHeader.ResponseType.NULL, 8162 new MessageHeader.ResponseTypeEnumFactory())); 8163 if (json.has("_code")) 8164 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 8165 if (json.has("details")) 8166 res.setDetails(parseReference(json.getAsJsonObject("details"))); 8167 return res; 8168 } 8169 8170 protected MessageHeader.MessageSourceComponent parseMessageHeaderMessageSourceComponent(JsonObject json, 8171 MessageHeader owner) throws IOException, FHIRFormatError { 8172 MessageHeader.MessageSourceComponent res = new MessageHeader.MessageSourceComponent(); 8173 parseBackboneProperties(json, res); 8174 if (json.has("name")) 8175 res.setNameElement(parseString(json.get("name").getAsString())); 8176 if (json.has("_name")) 8177 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 8178 if (json.has("software")) 8179 res.setSoftwareElement(parseString(json.get("software").getAsString())); 8180 if (json.has("_software")) 8181 parseElementProperties(json.getAsJsonObject("_software"), res.getSoftwareElement()); 8182 if (json.has("version")) 8183 res.setVersionElement(parseString(json.get("version").getAsString())); 8184 if (json.has("_version")) 8185 parseElementProperties(json.getAsJsonObject("_version"), res.getVersionElement()); 8186 if (json.has("contact")) 8187 res.setContact(parseContactPoint(json.getAsJsonObject("contact"))); 8188 if (json.has("endpoint")) 8189 res.setEndpointElement(parseUri(json.get("endpoint").getAsString())); 8190 if (json.has("_endpoint")) 8191 parseElementProperties(json.getAsJsonObject("_endpoint"), res.getEndpointElement()); 8192 return res; 8193 } 8194 8195 protected MessageHeader.MessageDestinationComponent parseMessageHeaderMessageDestinationComponent(JsonObject json, 8196 MessageHeader owner) throws IOException, FHIRFormatError { 8197 MessageHeader.MessageDestinationComponent res = new MessageHeader.MessageDestinationComponent(); 8198 parseBackboneProperties(json, res); 8199 if (json.has("name")) 8200 res.setNameElement(parseString(json.get("name").getAsString())); 8201 if (json.has("_name")) 8202 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 8203 if (json.has("target")) 8204 res.setTarget(parseReference(json.getAsJsonObject("target"))); 8205 if (json.has("endpoint")) 8206 res.setEndpointElement(parseUri(json.get("endpoint").getAsString())); 8207 if (json.has("_endpoint")) 8208 parseElementProperties(json.getAsJsonObject("_endpoint"), res.getEndpointElement()); 8209 return res; 8210 } 8211 8212 protected NamingSystem parseNamingSystem(JsonObject json) throws IOException, FHIRFormatError { 8213 NamingSystem res = new NamingSystem(); 8214 parseDomainResourceProperties(json, res); 8215 if (json.has("name")) 8216 res.setNameElement(parseString(json.get("name").getAsString())); 8217 if (json.has("_name")) 8218 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 8219 if (json.has("status")) 8220 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 8221 Enumerations.ConformanceResourceStatus.NULL, new Enumerations.ConformanceResourceStatusEnumFactory())); 8222 if (json.has("_status")) 8223 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 8224 if (json.has("kind")) 8225 res.setKindElement(parseEnumeration(json.get("kind").getAsString(), NamingSystem.NamingSystemType.NULL, 8226 new NamingSystem.NamingSystemTypeEnumFactory())); 8227 if (json.has("_kind")) 8228 parseElementProperties(json.getAsJsonObject("_kind"), res.getKindElement()); 8229 if (json.has("publisher")) 8230 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 8231 if (json.has("_publisher")) 8232 parseElementProperties(json.getAsJsonObject("_publisher"), res.getPublisherElement()); 8233 if (json.has("contact")) { 8234 JsonArray array = json.getAsJsonArray("contact"); 8235 for (int i = 0; i < array.size(); i++) { 8236 res.getContact().add(parseNamingSystemNamingSystemContactComponent(array.get(i).getAsJsonObject(), res)); 8237 } 8238 } 8239 ; 8240 if (json.has("responsible")) 8241 res.setResponsibleElement(parseString(json.get("responsible").getAsString())); 8242 if (json.has("_responsible")) 8243 parseElementProperties(json.getAsJsonObject("_responsible"), res.getResponsibleElement()); 8244 if (json.has("date")) 8245 res.setDateElement(parseDateTime(json.get("date").getAsString())); 8246 if (json.has("_date")) 8247 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 8248 if (json.has("type")) 8249 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 8250 if (json.has("description")) 8251 res.setDescriptionElement(parseString(json.get("description").getAsString())); 8252 if (json.has("_description")) 8253 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 8254 if (json.has("useContext")) { 8255 JsonArray array = json.getAsJsonArray("useContext"); 8256 for (int i = 0; i < array.size(); i++) { 8257 res.getUseContext().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 8258 } 8259 } 8260 ; 8261 if (json.has("usage")) 8262 res.setUsageElement(parseString(json.get("usage").getAsString())); 8263 if (json.has("_usage")) 8264 parseElementProperties(json.getAsJsonObject("_usage"), res.getUsageElement()); 8265 if (json.has("uniqueId")) { 8266 JsonArray array = json.getAsJsonArray("uniqueId"); 8267 for (int i = 0; i < array.size(); i++) { 8268 res.getUniqueId().add(parseNamingSystemNamingSystemUniqueIdComponent(array.get(i).getAsJsonObject(), res)); 8269 } 8270 } 8271 ; 8272 if (json.has("replacedBy")) 8273 res.setReplacedBy(parseReference(json.getAsJsonObject("replacedBy"))); 8274 return res; 8275 } 8276 8277 protected NamingSystem.NamingSystemContactComponent parseNamingSystemNamingSystemContactComponent(JsonObject json, 8278 NamingSystem owner) throws IOException, FHIRFormatError { 8279 NamingSystem.NamingSystemContactComponent res = new NamingSystem.NamingSystemContactComponent(); 8280 parseBackboneProperties(json, res); 8281 if (json.has("name")) 8282 res.setNameElement(parseString(json.get("name").getAsString())); 8283 if (json.has("_name")) 8284 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 8285 if (json.has("telecom")) { 8286 JsonArray array = json.getAsJsonArray("telecom"); 8287 for (int i = 0; i < array.size(); i++) { 8288 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 8289 } 8290 } 8291 ; 8292 return res; 8293 } 8294 8295 protected NamingSystem.NamingSystemUniqueIdComponent parseNamingSystemNamingSystemUniqueIdComponent(JsonObject json, 8296 NamingSystem owner) throws IOException, FHIRFormatError { 8297 NamingSystem.NamingSystemUniqueIdComponent res = new NamingSystem.NamingSystemUniqueIdComponent(); 8298 parseBackboneProperties(json, res); 8299 if (json.has("type")) 8300 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), NamingSystem.NamingSystemIdentifierType.NULL, 8301 new NamingSystem.NamingSystemIdentifierTypeEnumFactory())); 8302 if (json.has("_type")) 8303 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 8304 if (json.has("value")) 8305 res.setValueElement(parseString(json.get("value").getAsString())); 8306 if (json.has("_value")) 8307 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 8308 if (json.has("preferred")) 8309 res.setPreferredElement(parseBoolean(json.get("preferred").getAsBoolean())); 8310 if (json.has("_preferred")) 8311 parseElementProperties(json.getAsJsonObject("_preferred"), res.getPreferredElement()); 8312 if (json.has("period")) 8313 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 8314 return res; 8315 } 8316 8317 protected NutritionOrder parseNutritionOrder(JsonObject json) throws IOException, FHIRFormatError { 8318 NutritionOrder res = new NutritionOrder(); 8319 parseDomainResourceProperties(json, res); 8320 if (json.has("patient")) 8321 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 8322 if (json.has("orderer")) 8323 res.setOrderer(parseReference(json.getAsJsonObject("orderer"))); 8324 if (json.has("identifier")) { 8325 JsonArray array = json.getAsJsonArray("identifier"); 8326 for (int i = 0; i < array.size(); i++) { 8327 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 8328 } 8329 } 8330 ; 8331 if (json.has("encounter")) 8332 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 8333 if (json.has("dateTime")) 8334 res.setDateTimeElement(parseDateTime(json.get("dateTime").getAsString())); 8335 if (json.has("_dateTime")) 8336 parseElementProperties(json.getAsJsonObject("_dateTime"), res.getDateTimeElement()); 8337 if (json.has("status")) 8338 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), NutritionOrder.NutritionOrderStatus.NULL, 8339 new NutritionOrder.NutritionOrderStatusEnumFactory())); 8340 if (json.has("_status")) 8341 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 8342 if (json.has("allergyIntolerance")) { 8343 JsonArray array = json.getAsJsonArray("allergyIntolerance"); 8344 for (int i = 0; i < array.size(); i++) { 8345 res.getAllergyIntolerance().add(parseReference(array.get(i).getAsJsonObject())); 8346 } 8347 } 8348 ; 8349 if (json.has("foodPreferenceModifier")) { 8350 JsonArray array = json.getAsJsonArray("foodPreferenceModifier"); 8351 for (int i = 0; i < array.size(); i++) { 8352 res.getFoodPreferenceModifier().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 8353 } 8354 } 8355 ; 8356 if (json.has("excludeFoodModifier")) { 8357 JsonArray array = json.getAsJsonArray("excludeFoodModifier"); 8358 for (int i = 0; i < array.size(); i++) { 8359 res.getExcludeFoodModifier().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 8360 } 8361 } 8362 ; 8363 if (json.has("oralDiet")) 8364 res.setOralDiet(parseNutritionOrderNutritionOrderOralDietComponent(json.getAsJsonObject("oralDiet"), res)); 8365 if (json.has("supplement")) { 8366 JsonArray array = json.getAsJsonArray("supplement"); 8367 for (int i = 0; i < array.size(); i++) { 8368 res.getSupplement() 8369 .add(parseNutritionOrderNutritionOrderSupplementComponent(array.get(i).getAsJsonObject(), res)); 8370 } 8371 } 8372 ; 8373 if (json.has("enteralFormula")) 8374 res.setEnteralFormula( 8375 parseNutritionOrderNutritionOrderEnteralFormulaComponent(json.getAsJsonObject("enteralFormula"), res)); 8376 return res; 8377 } 8378 8379 protected NutritionOrder.NutritionOrderOralDietComponent parseNutritionOrderNutritionOrderOralDietComponent( 8380 JsonObject json, NutritionOrder owner) throws IOException, FHIRFormatError { 8381 NutritionOrder.NutritionOrderOralDietComponent res = new NutritionOrder.NutritionOrderOralDietComponent(); 8382 parseBackboneProperties(json, res); 8383 if (json.has("type")) { 8384 JsonArray array = json.getAsJsonArray("type"); 8385 for (int i = 0; i < array.size(); i++) { 8386 res.getType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 8387 } 8388 } 8389 ; 8390 if (json.has("schedule")) { 8391 JsonArray array = json.getAsJsonArray("schedule"); 8392 for (int i = 0; i < array.size(); i++) { 8393 res.getSchedule().add(parseTiming(array.get(i).getAsJsonObject())); 8394 } 8395 } 8396 ; 8397 if (json.has("nutrient")) { 8398 JsonArray array = json.getAsJsonArray("nutrient"); 8399 for (int i = 0; i < array.size(); i++) { 8400 res.getNutrient() 8401 .add(parseNutritionOrderNutritionOrderOralDietNutrientComponent(array.get(i).getAsJsonObject(), owner)); 8402 } 8403 } 8404 ; 8405 if (json.has("texture")) { 8406 JsonArray array = json.getAsJsonArray("texture"); 8407 for (int i = 0; i < array.size(); i++) { 8408 res.getTexture() 8409 .add(parseNutritionOrderNutritionOrderOralDietTextureComponent(array.get(i).getAsJsonObject(), owner)); 8410 } 8411 } 8412 ; 8413 if (json.has("fluidConsistencyType")) { 8414 JsonArray array = json.getAsJsonArray("fluidConsistencyType"); 8415 for (int i = 0; i < array.size(); i++) { 8416 res.getFluidConsistencyType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 8417 } 8418 } 8419 ; 8420 if (json.has("instruction")) 8421 res.setInstructionElement(parseString(json.get("instruction").getAsString())); 8422 if (json.has("_instruction")) 8423 parseElementProperties(json.getAsJsonObject("_instruction"), res.getInstructionElement()); 8424 return res; 8425 } 8426 8427 protected NutritionOrder.NutritionOrderOralDietNutrientComponent parseNutritionOrderNutritionOrderOralDietNutrientComponent( 8428 JsonObject json, NutritionOrder owner) throws IOException, FHIRFormatError { 8429 NutritionOrder.NutritionOrderOralDietNutrientComponent res = new NutritionOrder.NutritionOrderOralDietNutrientComponent(); 8430 parseBackboneProperties(json, res); 8431 if (json.has("modifier")) 8432 res.setModifier(parseCodeableConcept(json.getAsJsonObject("modifier"))); 8433 if (json.has("amount")) 8434 res.setAmount(parseSimpleQuantity(json.getAsJsonObject("amount"))); 8435 return res; 8436 } 8437 8438 protected NutritionOrder.NutritionOrderOralDietTextureComponent parseNutritionOrderNutritionOrderOralDietTextureComponent( 8439 JsonObject json, NutritionOrder owner) throws IOException, FHIRFormatError { 8440 NutritionOrder.NutritionOrderOralDietTextureComponent res = new NutritionOrder.NutritionOrderOralDietTextureComponent(); 8441 parseBackboneProperties(json, res); 8442 if (json.has("modifier")) 8443 res.setModifier(parseCodeableConcept(json.getAsJsonObject("modifier"))); 8444 if (json.has("foodType")) 8445 res.setFoodType(parseCodeableConcept(json.getAsJsonObject("foodType"))); 8446 return res; 8447 } 8448 8449 protected NutritionOrder.NutritionOrderSupplementComponent parseNutritionOrderNutritionOrderSupplementComponent( 8450 JsonObject json, NutritionOrder owner) throws IOException, FHIRFormatError { 8451 NutritionOrder.NutritionOrderSupplementComponent res = new NutritionOrder.NutritionOrderSupplementComponent(); 8452 parseBackboneProperties(json, res); 8453 if (json.has("type")) 8454 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 8455 if (json.has("productName")) 8456 res.setProductNameElement(parseString(json.get("productName").getAsString())); 8457 if (json.has("_productName")) 8458 parseElementProperties(json.getAsJsonObject("_productName"), res.getProductNameElement()); 8459 if (json.has("schedule")) { 8460 JsonArray array = json.getAsJsonArray("schedule"); 8461 for (int i = 0; i < array.size(); i++) { 8462 res.getSchedule().add(parseTiming(array.get(i).getAsJsonObject())); 8463 } 8464 } 8465 ; 8466 if (json.has("quantity")) 8467 res.setQuantity(parseSimpleQuantity(json.getAsJsonObject("quantity"))); 8468 if (json.has("instruction")) 8469 res.setInstructionElement(parseString(json.get("instruction").getAsString())); 8470 if (json.has("_instruction")) 8471 parseElementProperties(json.getAsJsonObject("_instruction"), res.getInstructionElement()); 8472 return res; 8473 } 8474 8475 protected NutritionOrder.NutritionOrderEnteralFormulaComponent parseNutritionOrderNutritionOrderEnteralFormulaComponent( 8476 JsonObject json, NutritionOrder owner) throws IOException, FHIRFormatError { 8477 NutritionOrder.NutritionOrderEnteralFormulaComponent res = new NutritionOrder.NutritionOrderEnteralFormulaComponent(); 8478 parseBackboneProperties(json, res); 8479 if (json.has("baseFormulaType")) 8480 res.setBaseFormulaType(parseCodeableConcept(json.getAsJsonObject("baseFormulaType"))); 8481 if (json.has("baseFormulaProductName")) 8482 res.setBaseFormulaProductNameElement(parseString(json.get("baseFormulaProductName").getAsString())); 8483 if (json.has("_baseFormulaProductName")) 8484 parseElementProperties(json.getAsJsonObject("_baseFormulaProductName"), res.getBaseFormulaProductNameElement()); 8485 if (json.has("additiveType")) 8486 res.setAdditiveType(parseCodeableConcept(json.getAsJsonObject("additiveType"))); 8487 if (json.has("additiveProductName")) 8488 res.setAdditiveProductNameElement(parseString(json.get("additiveProductName").getAsString())); 8489 if (json.has("_additiveProductName")) 8490 parseElementProperties(json.getAsJsonObject("_additiveProductName"), res.getAdditiveProductNameElement()); 8491 if (json.has("caloricDensity")) 8492 res.setCaloricDensity(parseSimpleQuantity(json.getAsJsonObject("caloricDensity"))); 8493 if (json.has("routeofAdministration")) 8494 res.setRouteofAdministration(parseCodeableConcept(json.getAsJsonObject("routeofAdministration"))); 8495 if (json.has("administration")) { 8496 JsonArray array = json.getAsJsonArray("administration"); 8497 for (int i = 0; i < array.size(); i++) { 8498 res.getAdministration().add(parseNutritionOrderNutritionOrderEnteralFormulaAdministrationComponent( 8499 array.get(i).getAsJsonObject(), owner)); 8500 } 8501 } 8502 ; 8503 if (json.has("maxVolumeToDeliver")) 8504 res.setMaxVolumeToDeliver(parseSimpleQuantity(json.getAsJsonObject("maxVolumeToDeliver"))); 8505 if (json.has("administrationInstruction")) 8506 res.setAdministrationInstructionElement(parseString(json.get("administrationInstruction").getAsString())); 8507 if (json.has("_administrationInstruction")) 8508 parseElementProperties(json.getAsJsonObject("_administrationInstruction"), 8509 res.getAdministrationInstructionElement()); 8510 return res; 8511 } 8512 8513 protected NutritionOrder.NutritionOrderEnteralFormulaAdministrationComponent parseNutritionOrderNutritionOrderEnteralFormulaAdministrationComponent( 8514 JsonObject json, NutritionOrder owner) throws IOException, FHIRFormatError { 8515 NutritionOrder.NutritionOrderEnteralFormulaAdministrationComponent res = new NutritionOrder.NutritionOrderEnteralFormulaAdministrationComponent(); 8516 parseBackboneProperties(json, res); 8517 if (json.has("schedule")) 8518 res.setSchedule(parseTiming(json.getAsJsonObject("schedule"))); 8519 if (json.has("quantity")) 8520 res.setQuantity(parseSimpleQuantity(json.getAsJsonObject("quantity"))); 8521 Type rate = parseType("rate", json); 8522 if (rate != null) 8523 res.setRate(rate); 8524 return res; 8525 } 8526 8527 protected Observation parseObservation(JsonObject json) throws IOException, FHIRFormatError { 8528 Observation res = new Observation(); 8529 parseDomainResourceProperties(json, res); 8530 if (json.has("identifier")) { 8531 JsonArray array = json.getAsJsonArray("identifier"); 8532 for (int i = 0; i < array.size(); i++) { 8533 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 8534 } 8535 } 8536 ; 8537 if (json.has("status")) 8538 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Observation.ObservationStatus.NULL, 8539 new Observation.ObservationStatusEnumFactory())); 8540 if (json.has("_status")) 8541 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 8542 if (json.has("category")) 8543 res.setCategory(parseCodeableConcept(json.getAsJsonObject("category"))); 8544 if (json.has("code")) 8545 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 8546 if (json.has("subject")) 8547 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 8548 if (json.has("encounter")) 8549 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 8550 Type effective = parseType("effective", json); 8551 if (effective != null) 8552 res.setEffective(effective); 8553 if (json.has("issued")) 8554 res.setIssuedElement(parseInstant(json.get("issued").getAsString())); 8555 if (json.has("_issued")) 8556 parseElementProperties(json.getAsJsonObject("_issued"), res.getIssuedElement()); 8557 if (json.has("performer")) { 8558 JsonArray array = json.getAsJsonArray("performer"); 8559 for (int i = 0; i < array.size(); i++) { 8560 res.getPerformer().add(parseReference(array.get(i).getAsJsonObject())); 8561 } 8562 } 8563 ; 8564 Type value = parseType("value", json); 8565 if (value != null) 8566 res.setValue(value); 8567 if (json.has("dataAbsentReason")) 8568 res.setDataAbsentReason(parseCodeableConcept(json.getAsJsonObject("dataAbsentReason"))); 8569 if (json.has("interpretation")) 8570 res.setInterpretation(parseCodeableConcept(json.getAsJsonObject("interpretation"))); 8571 if (json.has("comments")) 8572 res.setCommentsElement(parseString(json.get("comments").getAsString())); 8573 if (json.has("_comments")) 8574 parseElementProperties(json.getAsJsonObject("_comments"), res.getCommentsElement()); 8575 if (json.has("bodySite")) 8576 res.setBodySite(parseCodeableConcept(json.getAsJsonObject("bodySite"))); 8577 if (json.has("method")) 8578 res.setMethod(parseCodeableConcept(json.getAsJsonObject("method"))); 8579 if (json.has("specimen")) 8580 res.setSpecimen(parseReference(json.getAsJsonObject("specimen"))); 8581 if (json.has("device")) 8582 res.setDevice(parseReference(json.getAsJsonObject("device"))); 8583 if (json.has("referenceRange")) { 8584 JsonArray array = json.getAsJsonArray("referenceRange"); 8585 for (int i = 0; i < array.size(); i++) { 8586 res.getReferenceRange() 8587 .add(parseObservationObservationReferenceRangeComponent(array.get(i).getAsJsonObject(), res)); 8588 } 8589 } 8590 ; 8591 if (json.has("related")) { 8592 JsonArray array = json.getAsJsonArray("related"); 8593 for (int i = 0; i < array.size(); i++) { 8594 res.getRelated().add(parseObservationObservationRelatedComponent(array.get(i).getAsJsonObject(), res)); 8595 } 8596 } 8597 ; 8598 if (json.has("component")) { 8599 JsonArray array = json.getAsJsonArray("component"); 8600 for (int i = 0; i < array.size(); i++) { 8601 res.getComponent().add(parseObservationObservationComponentComponent(array.get(i).getAsJsonObject(), res)); 8602 } 8603 } 8604 ; 8605 return res; 8606 } 8607 8608 protected Observation.ObservationReferenceRangeComponent parseObservationObservationReferenceRangeComponent( 8609 JsonObject json, Observation owner) throws IOException, FHIRFormatError { 8610 Observation.ObservationReferenceRangeComponent res = new Observation.ObservationReferenceRangeComponent(); 8611 parseBackboneProperties(json, res); 8612 if (json.has("low")) 8613 res.setLow(parseSimpleQuantity(json.getAsJsonObject("low"))); 8614 if (json.has("high")) 8615 res.setHigh(parseSimpleQuantity(json.getAsJsonObject("high"))); 8616 if (json.has("meaning")) 8617 res.setMeaning(parseCodeableConcept(json.getAsJsonObject("meaning"))); 8618 if (json.has("age")) 8619 res.setAge(parseRange(json.getAsJsonObject("age"))); 8620 if (json.has("text")) 8621 res.setTextElement(parseString(json.get("text").getAsString())); 8622 if (json.has("_text")) 8623 parseElementProperties(json.getAsJsonObject("_text"), res.getTextElement()); 8624 return res; 8625 } 8626 8627 protected Observation.ObservationRelatedComponent parseObservationObservationRelatedComponent(JsonObject json, 8628 Observation owner) throws IOException, FHIRFormatError { 8629 Observation.ObservationRelatedComponent res = new Observation.ObservationRelatedComponent(); 8630 parseBackboneProperties(json, res); 8631 if (json.has("type")) 8632 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Observation.ObservationRelationshipType.NULL, 8633 new Observation.ObservationRelationshipTypeEnumFactory())); 8634 if (json.has("_type")) 8635 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 8636 if (json.has("target")) 8637 res.setTarget(parseReference(json.getAsJsonObject("target"))); 8638 return res; 8639 } 8640 8641 protected Observation.ObservationComponentComponent parseObservationObservationComponentComponent(JsonObject json, 8642 Observation owner) throws IOException, FHIRFormatError { 8643 Observation.ObservationComponentComponent res = new Observation.ObservationComponentComponent(); 8644 parseBackboneProperties(json, res); 8645 if (json.has("code")) 8646 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 8647 Type value = parseType("value", json); 8648 if (value != null) 8649 res.setValue(value); 8650 if (json.has("dataAbsentReason")) 8651 res.setDataAbsentReason(parseCodeableConcept(json.getAsJsonObject("dataAbsentReason"))); 8652 if (json.has("referenceRange")) { 8653 JsonArray array = json.getAsJsonArray("referenceRange"); 8654 for (int i = 0; i < array.size(); i++) { 8655 res.getReferenceRange() 8656 .add(parseObservationObservationReferenceRangeComponent(array.get(i).getAsJsonObject(), owner)); 8657 } 8658 } 8659 ; 8660 return res; 8661 } 8662 8663 protected OperationDefinition parseOperationDefinition(JsonObject json) throws IOException, FHIRFormatError { 8664 OperationDefinition res = new OperationDefinition(); 8665 parseDomainResourceProperties(json, res); 8666 if (json.has("url")) 8667 res.setUrlElement(parseUri(json.get("url").getAsString())); 8668 if (json.has("_url")) 8669 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 8670 if (json.has("version")) 8671 res.setVersionElement(parseString(json.get("version").getAsString())); 8672 if (json.has("_version")) 8673 parseElementProperties(json.getAsJsonObject("_version"), res.getVersionElement()); 8674 if (json.has("name")) 8675 res.setNameElement(parseString(json.get("name").getAsString())); 8676 if (json.has("_name")) 8677 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 8678 if (json.has("status")) 8679 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 8680 Enumerations.ConformanceResourceStatus.NULL, new Enumerations.ConformanceResourceStatusEnumFactory())); 8681 if (json.has("_status")) 8682 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 8683 if (json.has("kind")) 8684 res.setKindElement(parseEnumeration(json.get("kind").getAsString(), OperationDefinition.OperationKind.NULL, 8685 new OperationDefinition.OperationKindEnumFactory())); 8686 if (json.has("_kind")) 8687 parseElementProperties(json.getAsJsonObject("_kind"), res.getKindElement()); 8688 if (json.has("experimental")) 8689 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 8690 if (json.has("_experimental")) 8691 parseElementProperties(json.getAsJsonObject("_experimental"), res.getExperimentalElement()); 8692 if (json.has("publisher")) 8693 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 8694 if (json.has("_publisher")) 8695 parseElementProperties(json.getAsJsonObject("_publisher"), res.getPublisherElement()); 8696 if (json.has("contact")) { 8697 JsonArray array = json.getAsJsonArray("contact"); 8698 for (int i = 0; i < array.size(); i++) { 8699 res.getContact() 8700 .add(parseOperationDefinitionOperationDefinitionContactComponent(array.get(i).getAsJsonObject(), res)); 8701 } 8702 } 8703 ; 8704 if (json.has("date")) 8705 res.setDateElement(parseDateTime(json.get("date").getAsString())); 8706 if (json.has("_date")) 8707 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 8708 if (json.has("description")) 8709 res.setDescriptionElement(parseString(json.get("description").getAsString())); 8710 if (json.has("_description")) 8711 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 8712 if (json.has("requirements")) 8713 res.setRequirementsElement(parseString(json.get("requirements").getAsString())); 8714 if (json.has("_requirements")) 8715 parseElementProperties(json.getAsJsonObject("_requirements"), res.getRequirementsElement()); 8716 if (json.has("idempotent")) 8717 res.setIdempotentElement(parseBoolean(json.get("idempotent").getAsBoolean())); 8718 if (json.has("_idempotent")) 8719 parseElementProperties(json.getAsJsonObject("_idempotent"), res.getIdempotentElement()); 8720 if (json.has("code")) 8721 res.setCodeElement(parseCode(json.get("code").getAsString())); 8722 if (json.has("_code")) 8723 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 8724 if (json.has("notes")) 8725 res.setNotesElement(parseString(json.get("notes").getAsString())); 8726 if (json.has("_notes")) 8727 parseElementProperties(json.getAsJsonObject("_notes"), res.getNotesElement()); 8728 if (json.has("base")) 8729 res.setBase(parseReference(json.getAsJsonObject("base"))); 8730 if (json.has("system")) 8731 res.setSystemElement(parseBoolean(json.get("system").getAsBoolean())); 8732 if (json.has("_system")) 8733 parseElementProperties(json.getAsJsonObject("_system"), res.getSystemElement()); 8734 if (json.has("type")) { 8735 JsonArray array = json.getAsJsonArray("type"); 8736 for (int i = 0; i < array.size(); i++) { 8737 res.getType().add(parseCode(array.get(i).getAsString())); 8738 } 8739 } 8740 ; 8741 if (json.has("_type")) { 8742 JsonArray array = json.getAsJsonArray("_type"); 8743 for (int i = 0; i < array.size(); i++) { 8744 if (i == res.getType().size()) 8745 res.getType().add(parseCode(null)); 8746 if (array.get(i) instanceof JsonObject) 8747 parseElementProperties(array.get(i).getAsJsonObject(), res.getType().get(i)); 8748 } 8749 } 8750 ; 8751 if (json.has("instance")) 8752 res.setInstanceElement(parseBoolean(json.get("instance").getAsBoolean())); 8753 if (json.has("_instance")) 8754 parseElementProperties(json.getAsJsonObject("_instance"), res.getInstanceElement()); 8755 if (json.has("parameter")) { 8756 JsonArray array = json.getAsJsonArray("parameter"); 8757 for (int i = 0; i < array.size(); i++) { 8758 res.getParameter() 8759 .add(parseOperationDefinitionOperationDefinitionParameterComponent(array.get(i).getAsJsonObject(), res)); 8760 } 8761 } 8762 ; 8763 return res; 8764 } 8765 8766 protected OperationDefinition.OperationDefinitionContactComponent parseOperationDefinitionOperationDefinitionContactComponent( 8767 JsonObject json, OperationDefinition owner) throws IOException, FHIRFormatError { 8768 OperationDefinition.OperationDefinitionContactComponent res = new OperationDefinition.OperationDefinitionContactComponent(); 8769 parseBackboneProperties(json, res); 8770 if (json.has("name")) 8771 res.setNameElement(parseString(json.get("name").getAsString())); 8772 if (json.has("_name")) 8773 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 8774 if (json.has("telecom")) { 8775 JsonArray array = json.getAsJsonArray("telecom"); 8776 for (int i = 0; i < array.size(); i++) { 8777 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 8778 } 8779 } 8780 ; 8781 return res; 8782 } 8783 8784 protected OperationDefinition.OperationDefinitionParameterComponent parseOperationDefinitionOperationDefinitionParameterComponent( 8785 JsonObject json, OperationDefinition owner) throws IOException, FHIRFormatError { 8786 OperationDefinition.OperationDefinitionParameterComponent res = new OperationDefinition.OperationDefinitionParameterComponent(); 8787 parseBackboneProperties(json, res); 8788 if (json.has("name")) 8789 res.setNameElement(parseCode(json.get("name").getAsString())); 8790 if (json.has("_name")) 8791 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 8792 if (json.has("use")) 8793 res.setUseElement(parseEnumeration(json.get("use").getAsString(), OperationDefinition.OperationParameterUse.NULL, 8794 new OperationDefinition.OperationParameterUseEnumFactory())); 8795 if (json.has("_use")) 8796 parseElementProperties(json.getAsJsonObject("_use"), res.getUseElement()); 8797 if (json.has("min")) 8798 res.setMinElement(parseInteger(json.get("min").getAsLong())); 8799 if (json.has("_min")) 8800 parseElementProperties(json.getAsJsonObject("_min"), res.getMinElement()); 8801 if (json.has("max")) 8802 res.setMaxElement(parseString(json.get("max").getAsString())); 8803 if (json.has("_max")) 8804 parseElementProperties(json.getAsJsonObject("_max"), res.getMaxElement()); 8805 if (json.has("documentation")) 8806 res.setDocumentationElement(parseString(json.get("documentation").getAsString())); 8807 if (json.has("_documentation")) 8808 parseElementProperties(json.getAsJsonObject("_documentation"), res.getDocumentationElement()); 8809 if (json.has("type")) 8810 res.setTypeElement(parseCode(json.get("type").getAsString())); 8811 if (json.has("_type")) 8812 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 8813 if (json.has("profile")) 8814 res.setProfile(parseReference(json.getAsJsonObject("profile"))); 8815 if (json.has("binding")) 8816 res.setBinding( 8817 parseOperationDefinitionOperationDefinitionParameterBindingComponent(json.getAsJsonObject("binding"), owner)); 8818 if (json.has("part")) { 8819 JsonArray array = json.getAsJsonArray("part"); 8820 for (int i = 0; i < array.size(); i++) { 8821 res.getPart() 8822 .add(parseOperationDefinitionOperationDefinitionParameterComponent(array.get(i).getAsJsonObject(), owner)); 8823 } 8824 } 8825 ; 8826 return res; 8827 } 8828 8829 protected OperationDefinition.OperationDefinitionParameterBindingComponent parseOperationDefinitionOperationDefinitionParameterBindingComponent( 8830 JsonObject json, OperationDefinition owner) throws IOException, FHIRFormatError { 8831 OperationDefinition.OperationDefinitionParameterBindingComponent res = new OperationDefinition.OperationDefinitionParameterBindingComponent(); 8832 parseBackboneProperties(json, res); 8833 if (json.has("strength")) 8834 res.setStrengthElement(parseEnumeration(json.get("strength").getAsString(), Enumerations.BindingStrength.NULL, 8835 new Enumerations.BindingStrengthEnumFactory())); 8836 if (json.has("_strength")) 8837 parseElementProperties(json.getAsJsonObject("_strength"), res.getStrengthElement()); 8838 Type valueSet = parseType("valueSet", json); 8839 if (valueSet != null) 8840 res.setValueSet(valueSet); 8841 return res; 8842 } 8843 8844 protected OperationOutcome parseOperationOutcome(JsonObject json) throws IOException, FHIRFormatError { 8845 OperationOutcome res = new OperationOutcome(); 8846 parseDomainResourceProperties(json, res); 8847 if (json.has("issue")) { 8848 JsonArray array = json.getAsJsonArray("issue"); 8849 for (int i = 0; i < array.size(); i++) { 8850 res.getIssue().add(parseOperationOutcomeOperationOutcomeIssueComponent(array.get(i).getAsJsonObject(), res)); 8851 } 8852 } 8853 ; 8854 return res; 8855 } 8856 8857 protected OperationOutcome.OperationOutcomeIssueComponent parseOperationOutcomeOperationOutcomeIssueComponent( 8858 JsonObject json, OperationOutcome owner) throws IOException, FHIRFormatError { 8859 OperationOutcome.OperationOutcomeIssueComponent res = new OperationOutcome.OperationOutcomeIssueComponent(); 8860 parseBackboneProperties(json, res); 8861 if (json.has("severity")) 8862 res.setSeverityElement(parseEnumeration(json.get("severity").getAsString(), OperationOutcome.IssueSeverity.NULL, 8863 new OperationOutcome.IssueSeverityEnumFactory())); 8864 if (json.has("_severity")) 8865 parseElementProperties(json.getAsJsonObject("_severity"), res.getSeverityElement()); 8866 if (json.has("code")) 8867 res.setCodeElement(parseEnumeration(json.get("code").getAsString(), OperationOutcome.IssueType.NULL, 8868 new OperationOutcome.IssueTypeEnumFactory())); 8869 if (json.has("_code")) 8870 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 8871 if (json.has("details")) 8872 res.setDetails(parseCodeableConcept(json.getAsJsonObject("details"))); 8873 if (json.has("diagnostics")) 8874 res.setDiagnosticsElement(parseString(json.get("diagnostics").getAsString())); 8875 if (json.has("_diagnostics")) 8876 parseElementProperties(json.getAsJsonObject("_diagnostics"), res.getDiagnosticsElement()); 8877 if (json.has("location")) { 8878 JsonArray array = json.getAsJsonArray("location"); 8879 for (int i = 0; i < array.size(); i++) { 8880 res.getLocation().add(parseString(array.get(i).getAsString())); 8881 } 8882 } 8883 ; 8884 if (json.has("_location")) { 8885 JsonArray array = json.getAsJsonArray("_location"); 8886 for (int i = 0; i < array.size(); i++) { 8887 if (i == res.getLocation().size()) 8888 res.getLocation().add(parseString(null)); 8889 if (array.get(i) instanceof JsonObject) 8890 parseElementProperties(array.get(i).getAsJsonObject(), res.getLocation().get(i)); 8891 } 8892 } 8893 ; 8894 return res; 8895 } 8896 8897 protected Order parseOrder(JsonObject json) throws IOException, FHIRFormatError { 8898 Order res = new Order(); 8899 parseDomainResourceProperties(json, res); 8900 if (json.has("identifier")) { 8901 JsonArray array = json.getAsJsonArray("identifier"); 8902 for (int i = 0; i < array.size(); i++) { 8903 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 8904 } 8905 } 8906 ; 8907 if (json.has("date")) 8908 res.setDateElement(parseDateTime(json.get("date").getAsString())); 8909 if (json.has("_date")) 8910 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 8911 if (json.has("subject")) 8912 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 8913 if (json.has("source")) 8914 res.setSource(parseReference(json.getAsJsonObject("source"))); 8915 if (json.has("target")) 8916 res.setTarget(parseReference(json.getAsJsonObject("target"))); 8917 Type reason = parseType("reason", json); 8918 if (reason != null) 8919 res.setReason(reason); 8920 if (json.has("when")) 8921 res.setWhen(parseOrderOrderWhenComponent(json.getAsJsonObject("when"), res)); 8922 if (json.has("detail")) { 8923 JsonArray array = json.getAsJsonArray("detail"); 8924 for (int i = 0; i < array.size(); i++) { 8925 res.getDetail().add(parseReference(array.get(i).getAsJsonObject())); 8926 } 8927 } 8928 ; 8929 return res; 8930 } 8931 8932 protected Order.OrderWhenComponent parseOrderOrderWhenComponent(JsonObject json, Order owner) 8933 throws IOException, FHIRFormatError { 8934 Order.OrderWhenComponent res = new Order.OrderWhenComponent(); 8935 parseBackboneProperties(json, res); 8936 if (json.has("code")) 8937 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 8938 if (json.has("schedule")) 8939 res.setSchedule(parseTiming(json.getAsJsonObject("schedule"))); 8940 return res; 8941 } 8942 8943 protected OrderResponse parseOrderResponse(JsonObject json) throws IOException, FHIRFormatError { 8944 OrderResponse res = new OrderResponse(); 8945 parseDomainResourceProperties(json, res); 8946 if (json.has("identifier")) { 8947 JsonArray array = json.getAsJsonArray("identifier"); 8948 for (int i = 0; i < array.size(); i++) { 8949 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 8950 } 8951 } 8952 ; 8953 if (json.has("request")) 8954 res.setRequest(parseReference(json.getAsJsonObject("request"))); 8955 if (json.has("date")) 8956 res.setDateElement(parseDateTime(json.get("date").getAsString())); 8957 if (json.has("_date")) 8958 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 8959 if (json.has("who")) 8960 res.setWho(parseReference(json.getAsJsonObject("who"))); 8961 if (json.has("orderStatus")) 8962 res.setOrderStatusElement(parseEnumeration(json.get("orderStatus").getAsString(), OrderResponse.OrderStatus.NULL, 8963 new OrderResponse.OrderStatusEnumFactory())); 8964 if (json.has("_orderStatus")) 8965 parseElementProperties(json.getAsJsonObject("_orderStatus"), res.getOrderStatusElement()); 8966 if (json.has("description")) 8967 res.setDescriptionElement(parseString(json.get("description").getAsString())); 8968 if (json.has("_description")) 8969 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 8970 if (json.has("fulfillment")) { 8971 JsonArray array = json.getAsJsonArray("fulfillment"); 8972 for (int i = 0; i < array.size(); i++) { 8973 res.getFulfillment().add(parseReference(array.get(i).getAsJsonObject())); 8974 } 8975 } 8976 ; 8977 return res; 8978 } 8979 8980 protected Organization parseOrganization(JsonObject json) throws IOException, FHIRFormatError { 8981 Organization res = new Organization(); 8982 parseDomainResourceProperties(json, res); 8983 if (json.has("identifier")) { 8984 JsonArray array = json.getAsJsonArray("identifier"); 8985 for (int i = 0; i < array.size(); i++) { 8986 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 8987 } 8988 } 8989 ; 8990 if (json.has("active")) 8991 res.setActiveElement(parseBoolean(json.get("active").getAsBoolean())); 8992 if (json.has("_active")) 8993 parseElementProperties(json.getAsJsonObject("_active"), res.getActiveElement()); 8994 if (json.has("type")) 8995 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 8996 if (json.has("name")) 8997 res.setNameElement(parseString(json.get("name").getAsString())); 8998 if (json.has("_name")) 8999 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 9000 if (json.has("telecom")) { 9001 JsonArray array = json.getAsJsonArray("telecom"); 9002 for (int i = 0; i < array.size(); i++) { 9003 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 9004 } 9005 } 9006 ; 9007 if (json.has("address")) { 9008 JsonArray array = json.getAsJsonArray("address"); 9009 for (int i = 0; i < array.size(); i++) { 9010 res.getAddress().add(parseAddress(array.get(i).getAsJsonObject())); 9011 } 9012 } 9013 ; 9014 if (json.has("partOf")) 9015 res.setPartOf(parseReference(json.getAsJsonObject("partOf"))); 9016 if (json.has("contact")) { 9017 JsonArray array = json.getAsJsonArray("contact"); 9018 for (int i = 0; i < array.size(); i++) { 9019 res.getContact().add(parseOrganizationOrganizationContactComponent(array.get(i).getAsJsonObject(), res)); 9020 } 9021 } 9022 ; 9023 return res; 9024 } 9025 9026 protected Organization.OrganizationContactComponent parseOrganizationOrganizationContactComponent(JsonObject json, 9027 Organization owner) throws IOException, FHIRFormatError { 9028 Organization.OrganizationContactComponent res = new Organization.OrganizationContactComponent(); 9029 parseBackboneProperties(json, res); 9030 if (json.has("purpose")) 9031 res.setPurpose(parseCodeableConcept(json.getAsJsonObject("purpose"))); 9032 if (json.has("name")) 9033 res.setName(parseHumanName(json.getAsJsonObject("name"))); 9034 if (json.has("telecom")) { 9035 JsonArray array = json.getAsJsonArray("telecom"); 9036 for (int i = 0; i < array.size(); i++) { 9037 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 9038 } 9039 } 9040 ; 9041 if (json.has("address")) 9042 res.setAddress(parseAddress(json.getAsJsonObject("address"))); 9043 return res; 9044 } 9045 9046 protected Patient parsePatient(JsonObject json) throws IOException, FHIRFormatError { 9047 Patient res = new Patient(); 9048 parseDomainResourceProperties(json, res); 9049 if (json.has("identifier")) { 9050 JsonArray array = json.getAsJsonArray("identifier"); 9051 for (int i = 0; i < array.size(); i++) { 9052 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 9053 } 9054 } 9055 ; 9056 if (json.has("active")) 9057 res.setActiveElement(parseBoolean(json.get("active").getAsBoolean())); 9058 if (json.has("_active")) 9059 parseElementProperties(json.getAsJsonObject("_active"), res.getActiveElement()); 9060 if (json.has("name")) { 9061 JsonArray array = json.getAsJsonArray("name"); 9062 for (int i = 0; i < array.size(); i++) { 9063 res.getName().add(parseHumanName(array.get(i).getAsJsonObject())); 9064 } 9065 } 9066 ; 9067 if (json.has("telecom")) { 9068 JsonArray array = json.getAsJsonArray("telecom"); 9069 for (int i = 0; i < array.size(); i++) { 9070 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 9071 } 9072 } 9073 ; 9074 if (json.has("gender")) 9075 res.setGenderElement(parseEnumeration(json.get("gender").getAsString(), Enumerations.AdministrativeGender.NULL, 9076 new Enumerations.AdministrativeGenderEnumFactory())); 9077 if (json.has("_gender")) 9078 parseElementProperties(json.getAsJsonObject("_gender"), res.getGenderElement()); 9079 if (json.has("birthDate")) 9080 res.setBirthDateElement(parseDate(json.get("birthDate").getAsString())); 9081 if (json.has("_birthDate")) 9082 parseElementProperties(json.getAsJsonObject("_birthDate"), res.getBirthDateElement()); 9083 Type deceased = parseType("deceased", json); 9084 if (deceased != null) 9085 res.setDeceased(deceased); 9086 if (json.has("address")) { 9087 JsonArray array = json.getAsJsonArray("address"); 9088 for (int i = 0; i < array.size(); i++) { 9089 res.getAddress().add(parseAddress(array.get(i).getAsJsonObject())); 9090 } 9091 } 9092 ; 9093 if (json.has("maritalStatus")) 9094 res.setMaritalStatus(parseCodeableConcept(json.getAsJsonObject("maritalStatus"))); 9095 Type multipleBirth = parseType("multipleBirth", json); 9096 if (multipleBirth != null) 9097 res.setMultipleBirth(multipleBirth); 9098 if (json.has("photo")) { 9099 JsonArray array = json.getAsJsonArray("photo"); 9100 for (int i = 0; i < array.size(); i++) { 9101 res.getPhoto().add(parseAttachment(array.get(i).getAsJsonObject())); 9102 } 9103 } 9104 ; 9105 if (json.has("contact")) { 9106 JsonArray array = json.getAsJsonArray("contact"); 9107 for (int i = 0; i < array.size(); i++) { 9108 res.getContact().add(parsePatientContactComponent(array.get(i).getAsJsonObject(), res)); 9109 } 9110 } 9111 ; 9112 if (json.has("animal")) 9113 res.setAnimal(parsePatientAnimalComponent(json.getAsJsonObject("animal"), res)); 9114 if (json.has("communication")) { 9115 JsonArray array = json.getAsJsonArray("communication"); 9116 for (int i = 0; i < array.size(); i++) { 9117 res.getCommunication().add(parsePatientPatientCommunicationComponent(array.get(i).getAsJsonObject(), res)); 9118 } 9119 } 9120 ; 9121 if (json.has("careProvider")) { 9122 JsonArray array = json.getAsJsonArray("careProvider"); 9123 for (int i = 0; i < array.size(); i++) { 9124 res.getCareProvider().add(parseReference(array.get(i).getAsJsonObject())); 9125 } 9126 } 9127 ; 9128 if (json.has("managingOrganization")) 9129 res.setManagingOrganization(parseReference(json.getAsJsonObject("managingOrganization"))); 9130 if (json.has("link")) { 9131 JsonArray array = json.getAsJsonArray("link"); 9132 for (int i = 0; i < array.size(); i++) { 9133 res.getLink().add(parsePatientPatientLinkComponent(array.get(i).getAsJsonObject(), res)); 9134 } 9135 } 9136 ; 9137 return res; 9138 } 9139 9140 protected Patient.ContactComponent parsePatientContactComponent(JsonObject json, Patient owner) 9141 throws IOException, FHIRFormatError { 9142 Patient.ContactComponent res = new Patient.ContactComponent(); 9143 parseBackboneProperties(json, res); 9144 if (json.has("relationship")) { 9145 JsonArray array = json.getAsJsonArray("relationship"); 9146 for (int i = 0; i < array.size(); i++) { 9147 res.getRelationship().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9148 } 9149 } 9150 ; 9151 if (json.has("name")) 9152 res.setName(parseHumanName(json.getAsJsonObject("name"))); 9153 if (json.has("telecom")) { 9154 JsonArray array = json.getAsJsonArray("telecom"); 9155 for (int i = 0; i < array.size(); i++) { 9156 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 9157 } 9158 } 9159 ; 9160 if (json.has("address")) 9161 res.setAddress(parseAddress(json.getAsJsonObject("address"))); 9162 if (json.has("gender")) 9163 res.setGenderElement(parseEnumeration(json.get("gender").getAsString(), Enumerations.AdministrativeGender.NULL, 9164 new Enumerations.AdministrativeGenderEnumFactory())); 9165 if (json.has("_gender")) 9166 parseElementProperties(json.getAsJsonObject("_gender"), res.getGenderElement()); 9167 if (json.has("organization")) 9168 res.setOrganization(parseReference(json.getAsJsonObject("organization"))); 9169 if (json.has("period")) 9170 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 9171 return res; 9172 } 9173 9174 protected Patient.AnimalComponent parsePatientAnimalComponent(JsonObject json, Patient owner) 9175 throws IOException, FHIRFormatError { 9176 Patient.AnimalComponent res = new Patient.AnimalComponent(); 9177 parseBackboneProperties(json, res); 9178 if (json.has("species")) 9179 res.setSpecies(parseCodeableConcept(json.getAsJsonObject("species"))); 9180 if (json.has("breed")) 9181 res.setBreed(parseCodeableConcept(json.getAsJsonObject("breed"))); 9182 if (json.has("genderStatus")) 9183 res.setGenderStatus(parseCodeableConcept(json.getAsJsonObject("genderStatus"))); 9184 return res; 9185 } 9186 9187 protected Patient.PatientCommunicationComponent parsePatientPatientCommunicationComponent(JsonObject json, 9188 Patient owner) throws IOException, FHIRFormatError { 9189 Patient.PatientCommunicationComponent res = new Patient.PatientCommunicationComponent(); 9190 parseBackboneProperties(json, res); 9191 if (json.has("language")) 9192 res.setLanguage(parseCodeableConcept(json.getAsJsonObject("language"))); 9193 if (json.has("preferred")) 9194 res.setPreferredElement(parseBoolean(json.get("preferred").getAsBoolean())); 9195 if (json.has("_preferred")) 9196 parseElementProperties(json.getAsJsonObject("_preferred"), res.getPreferredElement()); 9197 return res; 9198 } 9199 9200 protected Patient.PatientLinkComponent parsePatientPatientLinkComponent(JsonObject json, Patient owner) 9201 throws IOException, FHIRFormatError { 9202 Patient.PatientLinkComponent res = new Patient.PatientLinkComponent(); 9203 parseBackboneProperties(json, res); 9204 if (json.has("other")) 9205 res.setOther(parseReference(json.getAsJsonObject("other"))); 9206 if (json.has("type")) 9207 res.setTypeElement( 9208 parseEnumeration(json.get("type").getAsString(), Patient.LinkType.NULL, new Patient.LinkTypeEnumFactory())); 9209 if (json.has("_type")) 9210 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 9211 return res; 9212 } 9213 9214 protected PaymentNotice parsePaymentNotice(JsonObject json) throws IOException, FHIRFormatError { 9215 PaymentNotice res = new PaymentNotice(); 9216 parseDomainResourceProperties(json, res); 9217 if (json.has("identifier")) { 9218 JsonArray array = json.getAsJsonArray("identifier"); 9219 for (int i = 0; i < array.size(); i++) { 9220 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 9221 } 9222 } 9223 ; 9224 if (json.has("ruleset")) 9225 res.setRuleset(parseCoding(json.getAsJsonObject("ruleset"))); 9226 if (json.has("originalRuleset")) 9227 res.setOriginalRuleset(parseCoding(json.getAsJsonObject("originalRuleset"))); 9228 if (json.has("created")) 9229 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 9230 if (json.has("_created")) 9231 parseElementProperties(json.getAsJsonObject("_created"), res.getCreatedElement()); 9232 if (json.has("target")) 9233 res.setTarget(parseReference(json.getAsJsonObject("target"))); 9234 if (json.has("provider")) 9235 res.setProvider(parseReference(json.getAsJsonObject("provider"))); 9236 if (json.has("organization")) 9237 res.setOrganization(parseReference(json.getAsJsonObject("organization"))); 9238 if (json.has("request")) 9239 res.setRequest(parseReference(json.getAsJsonObject("request"))); 9240 if (json.has("response")) 9241 res.setResponse(parseReference(json.getAsJsonObject("response"))); 9242 if (json.has("paymentStatus")) 9243 res.setPaymentStatus(parseCoding(json.getAsJsonObject("paymentStatus"))); 9244 return res; 9245 } 9246 9247 protected PaymentReconciliation parsePaymentReconciliation(JsonObject json) throws IOException, FHIRFormatError { 9248 PaymentReconciliation res = new PaymentReconciliation(); 9249 parseDomainResourceProperties(json, res); 9250 if (json.has("identifier")) { 9251 JsonArray array = json.getAsJsonArray("identifier"); 9252 for (int i = 0; i < array.size(); i++) { 9253 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 9254 } 9255 } 9256 ; 9257 if (json.has("request")) 9258 res.setRequest(parseReference(json.getAsJsonObject("request"))); 9259 if (json.has("outcome")) 9260 res.setOutcomeElement(parseEnumeration(json.get("outcome").getAsString(), Enumerations.RemittanceOutcome.NULL, 9261 new Enumerations.RemittanceOutcomeEnumFactory())); 9262 if (json.has("_outcome")) 9263 parseElementProperties(json.getAsJsonObject("_outcome"), res.getOutcomeElement()); 9264 if (json.has("disposition")) 9265 res.setDispositionElement(parseString(json.get("disposition").getAsString())); 9266 if (json.has("_disposition")) 9267 parseElementProperties(json.getAsJsonObject("_disposition"), res.getDispositionElement()); 9268 if (json.has("ruleset")) 9269 res.setRuleset(parseCoding(json.getAsJsonObject("ruleset"))); 9270 if (json.has("originalRuleset")) 9271 res.setOriginalRuleset(parseCoding(json.getAsJsonObject("originalRuleset"))); 9272 if (json.has("created")) 9273 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 9274 if (json.has("_created")) 9275 parseElementProperties(json.getAsJsonObject("_created"), res.getCreatedElement()); 9276 if (json.has("period")) 9277 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 9278 if (json.has("organization")) 9279 res.setOrganization(parseReference(json.getAsJsonObject("organization"))); 9280 if (json.has("requestProvider")) 9281 res.setRequestProvider(parseReference(json.getAsJsonObject("requestProvider"))); 9282 if (json.has("requestOrganization")) 9283 res.setRequestOrganization(parseReference(json.getAsJsonObject("requestOrganization"))); 9284 if (json.has("detail")) { 9285 JsonArray array = json.getAsJsonArray("detail"); 9286 for (int i = 0; i < array.size(); i++) { 9287 res.getDetail().add(parsePaymentReconciliationDetailsComponent(array.get(i).getAsJsonObject(), res)); 9288 } 9289 } 9290 ; 9291 if (json.has("form")) 9292 res.setForm(parseCoding(json.getAsJsonObject("form"))); 9293 if (json.has("total")) 9294 res.setTotal(parseMoney(json.getAsJsonObject("total"))); 9295 if (json.has("note")) { 9296 JsonArray array = json.getAsJsonArray("note"); 9297 for (int i = 0; i < array.size(); i++) { 9298 res.getNote().add(parsePaymentReconciliationNotesComponent(array.get(i).getAsJsonObject(), res)); 9299 } 9300 } 9301 ; 9302 return res; 9303 } 9304 9305 protected PaymentReconciliation.DetailsComponent parsePaymentReconciliationDetailsComponent(JsonObject json, 9306 PaymentReconciliation owner) throws IOException, FHIRFormatError { 9307 PaymentReconciliation.DetailsComponent res = new PaymentReconciliation.DetailsComponent(); 9308 parseBackboneProperties(json, res); 9309 if (json.has("type")) 9310 res.setType(parseCoding(json.getAsJsonObject("type"))); 9311 if (json.has("request")) 9312 res.setRequest(parseReference(json.getAsJsonObject("request"))); 9313 if (json.has("responce")) 9314 res.setResponce(parseReference(json.getAsJsonObject("responce"))); 9315 if (json.has("submitter")) 9316 res.setSubmitter(parseReference(json.getAsJsonObject("submitter"))); 9317 if (json.has("payee")) 9318 res.setPayee(parseReference(json.getAsJsonObject("payee"))); 9319 if (json.has("date")) 9320 res.setDateElement(parseDate(json.get("date").getAsString())); 9321 if (json.has("_date")) 9322 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 9323 if (json.has("amount")) 9324 res.setAmount(parseMoney(json.getAsJsonObject("amount"))); 9325 return res; 9326 } 9327 9328 protected PaymentReconciliation.NotesComponent parsePaymentReconciliationNotesComponent(JsonObject json, 9329 PaymentReconciliation owner) throws IOException, FHIRFormatError { 9330 PaymentReconciliation.NotesComponent res = new PaymentReconciliation.NotesComponent(); 9331 parseBackboneProperties(json, res); 9332 if (json.has("type")) 9333 res.setType(parseCoding(json.getAsJsonObject("type"))); 9334 if (json.has("text")) 9335 res.setTextElement(parseString(json.get("text").getAsString())); 9336 if (json.has("_text")) 9337 parseElementProperties(json.getAsJsonObject("_text"), res.getTextElement()); 9338 return res; 9339 } 9340 9341 protected Person parsePerson(JsonObject json) throws IOException, FHIRFormatError { 9342 Person res = new Person(); 9343 parseDomainResourceProperties(json, res); 9344 if (json.has("identifier")) { 9345 JsonArray array = json.getAsJsonArray("identifier"); 9346 for (int i = 0; i < array.size(); i++) { 9347 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 9348 } 9349 } 9350 ; 9351 if (json.has("name")) { 9352 JsonArray array = json.getAsJsonArray("name"); 9353 for (int i = 0; i < array.size(); i++) { 9354 res.getName().add(parseHumanName(array.get(i).getAsJsonObject())); 9355 } 9356 } 9357 ; 9358 if (json.has("telecom")) { 9359 JsonArray array = json.getAsJsonArray("telecom"); 9360 for (int i = 0; i < array.size(); i++) { 9361 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 9362 } 9363 } 9364 ; 9365 if (json.has("gender")) 9366 res.setGenderElement(parseEnumeration(json.get("gender").getAsString(), Enumerations.AdministrativeGender.NULL, 9367 new Enumerations.AdministrativeGenderEnumFactory())); 9368 if (json.has("_gender")) 9369 parseElementProperties(json.getAsJsonObject("_gender"), res.getGenderElement()); 9370 if (json.has("birthDate")) 9371 res.setBirthDateElement(parseDate(json.get("birthDate").getAsString())); 9372 if (json.has("_birthDate")) 9373 parseElementProperties(json.getAsJsonObject("_birthDate"), res.getBirthDateElement()); 9374 if (json.has("address")) { 9375 JsonArray array = json.getAsJsonArray("address"); 9376 for (int i = 0; i < array.size(); i++) { 9377 res.getAddress().add(parseAddress(array.get(i).getAsJsonObject())); 9378 } 9379 } 9380 ; 9381 if (json.has("photo")) 9382 res.setPhoto(parseAttachment(json.getAsJsonObject("photo"))); 9383 if (json.has("managingOrganization")) 9384 res.setManagingOrganization(parseReference(json.getAsJsonObject("managingOrganization"))); 9385 if (json.has("active")) 9386 res.setActiveElement(parseBoolean(json.get("active").getAsBoolean())); 9387 if (json.has("_active")) 9388 parseElementProperties(json.getAsJsonObject("_active"), res.getActiveElement()); 9389 if (json.has("link")) { 9390 JsonArray array = json.getAsJsonArray("link"); 9391 for (int i = 0; i < array.size(); i++) { 9392 res.getLink().add(parsePersonPersonLinkComponent(array.get(i).getAsJsonObject(), res)); 9393 } 9394 } 9395 ; 9396 return res; 9397 } 9398 9399 protected Person.PersonLinkComponent parsePersonPersonLinkComponent(JsonObject json, Person owner) 9400 throws IOException, FHIRFormatError { 9401 Person.PersonLinkComponent res = new Person.PersonLinkComponent(); 9402 parseBackboneProperties(json, res); 9403 if (json.has("target")) 9404 res.setTarget(parseReference(json.getAsJsonObject("target"))); 9405 if (json.has("assurance")) 9406 res.setAssuranceElement(parseEnumeration(json.get("assurance").getAsString(), Person.IdentityAssuranceLevel.NULL, 9407 new Person.IdentityAssuranceLevelEnumFactory())); 9408 if (json.has("_assurance")) 9409 parseElementProperties(json.getAsJsonObject("_assurance"), res.getAssuranceElement()); 9410 return res; 9411 } 9412 9413 protected Practitioner parsePractitioner(JsonObject json) throws IOException, FHIRFormatError { 9414 Practitioner res = new Practitioner(); 9415 parseDomainResourceProperties(json, res); 9416 if (json.has("identifier")) { 9417 JsonArray array = json.getAsJsonArray("identifier"); 9418 for (int i = 0; i < array.size(); i++) { 9419 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 9420 } 9421 } 9422 ; 9423 if (json.has("active")) 9424 res.setActiveElement(parseBoolean(json.get("active").getAsBoolean())); 9425 if (json.has("_active")) 9426 parseElementProperties(json.getAsJsonObject("_active"), res.getActiveElement()); 9427 if (json.has("name")) 9428 res.setName(parseHumanName(json.getAsJsonObject("name"))); 9429 if (json.has("telecom")) { 9430 JsonArray array = json.getAsJsonArray("telecom"); 9431 for (int i = 0; i < array.size(); i++) { 9432 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 9433 } 9434 } 9435 ; 9436 if (json.has("address")) { 9437 JsonArray array = json.getAsJsonArray("address"); 9438 for (int i = 0; i < array.size(); i++) { 9439 res.getAddress().add(parseAddress(array.get(i).getAsJsonObject())); 9440 } 9441 } 9442 ; 9443 if (json.has("gender")) 9444 res.setGenderElement(parseEnumeration(json.get("gender").getAsString(), Enumerations.AdministrativeGender.NULL, 9445 new Enumerations.AdministrativeGenderEnumFactory())); 9446 if (json.has("_gender")) 9447 parseElementProperties(json.getAsJsonObject("_gender"), res.getGenderElement()); 9448 if (json.has("birthDate")) 9449 res.setBirthDateElement(parseDate(json.get("birthDate").getAsString())); 9450 if (json.has("_birthDate")) 9451 parseElementProperties(json.getAsJsonObject("_birthDate"), res.getBirthDateElement()); 9452 if (json.has("photo")) { 9453 JsonArray array = json.getAsJsonArray("photo"); 9454 for (int i = 0; i < array.size(); i++) { 9455 res.getPhoto().add(parseAttachment(array.get(i).getAsJsonObject())); 9456 } 9457 } 9458 ; 9459 if (json.has("practitionerRole")) { 9460 JsonArray array = json.getAsJsonArray("practitionerRole"); 9461 for (int i = 0; i < array.size(); i++) { 9462 res.getPractitionerRole() 9463 .add(parsePractitionerPractitionerPractitionerRoleComponent(array.get(i).getAsJsonObject(), res)); 9464 } 9465 } 9466 ; 9467 if (json.has("qualification")) { 9468 JsonArray array = json.getAsJsonArray("qualification"); 9469 for (int i = 0; i < array.size(); i++) { 9470 res.getQualification() 9471 .add(parsePractitionerPractitionerQualificationComponent(array.get(i).getAsJsonObject(), res)); 9472 } 9473 } 9474 ; 9475 if (json.has("communication")) { 9476 JsonArray array = json.getAsJsonArray("communication"); 9477 for (int i = 0; i < array.size(); i++) { 9478 res.getCommunication().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9479 } 9480 } 9481 ; 9482 return res; 9483 } 9484 9485 protected Practitioner.PractitionerPractitionerRoleComponent parsePractitionerPractitionerPractitionerRoleComponent( 9486 JsonObject json, Practitioner owner) throws IOException, FHIRFormatError { 9487 Practitioner.PractitionerPractitionerRoleComponent res = new Practitioner.PractitionerPractitionerRoleComponent(); 9488 parseBackboneProperties(json, res); 9489 if (json.has("managingOrganization")) 9490 res.setManagingOrganization(parseReference(json.getAsJsonObject("managingOrganization"))); 9491 if (json.has("role")) 9492 res.setRole(parseCodeableConcept(json.getAsJsonObject("role"))); 9493 if (json.has("specialty")) { 9494 JsonArray array = json.getAsJsonArray("specialty"); 9495 for (int i = 0; i < array.size(); i++) { 9496 res.getSpecialty().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9497 } 9498 } 9499 ; 9500 if (json.has("period")) 9501 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 9502 if (json.has("location")) { 9503 JsonArray array = json.getAsJsonArray("location"); 9504 for (int i = 0; i < array.size(); i++) { 9505 res.getLocation().add(parseReference(array.get(i).getAsJsonObject())); 9506 } 9507 } 9508 ; 9509 if (json.has("healthcareService")) { 9510 JsonArray array = json.getAsJsonArray("healthcareService"); 9511 for (int i = 0; i < array.size(); i++) { 9512 res.getHealthcareService().add(parseReference(array.get(i).getAsJsonObject())); 9513 } 9514 } 9515 ; 9516 return res; 9517 } 9518 9519 protected Practitioner.PractitionerQualificationComponent parsePractitionerPractitionerQualificationComponent( 9520 JsonObject json, Practitioner owner) throws IOException, FHIRFormatError { 9521 Practitioner.PractitionerQualificationComponent res = new Practitioner.PractitionerQualificationComponent(); 9522 parseBackboneProperties(json, res); 9523 if (json.has("identifier")) { 9524 JsonArray array = json.getAsJsonArray("identifier"); 9525 for (int i = 0; i < array.size(); i++) { 9526 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 9527 } 9528 } 9529 ; 9530 if (json.has("code")) 9531 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 9532 if (json.has("period")) 9533 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 9534 if (json.has("issuer")) 9535 res.setIssuer(parseReference(json.getAsJsonObject("issuer"))); 9536 return res; 9537 } 9538 9539 protected Procedure parseProcedure(JsonObject json) throws IOException, FHIRFormatError { 9540 Procedure res = new Procedure(); 9541 parseDomainResourceProperties(json, res); 9542 if (json.has("identifier")) { 9543 JsonArray array = json.getAsJsonArray("identifier"); 9544 for (int i = 0; i < array.size(); i++) { 9545 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 9546 } 9547 } 9548 ; 9549 if (json.has("subject")) 9550 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 9551 if (json.has("status")) 9552 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Procedure.ProcedureStatus.NULL, 9553 new Procedure.ProcedureStatusEnumFactory())); 9554 if (json.has("_status")) 9555 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 9556 if (json.has("category")) 9557 res.setCategory(parseCodeableConcept(json.getAsJsonObject("category"))); 9558 if (json.has("code")) 9559 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 9560 if (json.has("notPerformed")) 9561 res.setNotPerformedElement(parseBoolean(json.get("notPerformed").getAsBoolean())); 9562 if (json.has("_notPerformed")) 9563 parseElementProperties(json.getAsJsonObject("_notPerformed"), res.getNotPerformedElement()); 9564 if (json.has("reasonNotPerformed")) { 9565 JsonArray array = json.getAsJsonArray("reasonNotPerformed"); 9566 for (int i = 0; i < array.size(); i++) { 9567 res.getReasonNotPerformed().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9568 } 9569 } 9570 ; 9571 if (json.has("bodySite")) { 9572 JsonArray array = json.getAsJsonArray("bodySite"); 9573 for (int i = 0; i < array.size(); i++) { 9574 res.getBodySite().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9575 } 9576 } 9577 ; 9578 Type reason = parseType("reason", json); 9579 if (reason != null) 9580 res.setReason(reason); 9581 if (json.has("performer")) { 9582 JsonArray array = json.getAsJsonArray("performer"); 9583 for (int i = 0; i < array.size(); i++) { 9584 res.getPerformer().add(parseProcedureProcedurePerformerComponent(array.get(i).getAsJsonObject(), res)); 9585 } 9586 } 9587 ; 9588 Type performed = parseType("performed", json); 9589 if (performed != null) 9590 res.setPerformed(performed); 9591 if (json.has("encounter")) 9592 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 9593 if (json.has("location")) 9594 res.setLocation(parseReference(json.getAsJsonObject("location"))); 9595 if (json.has("outcome")) 9596 res.setOutcome(parseCodeableConcept(json.getAsJsonObject("outcome"))); 9597 if (json.has("report")) { 9598 JsonArray array = json.getAsJsonArray("report"); 9599 for (int i = 0; i < array.size(); i++) { 9600 res.getReport().add(parseReference(array.get(i).getAsJsonObject())); 9601 } 9602 } 9603 ; 9604 if (json.has("complication")) { 9605 JsonArray array = json.getAsJsonArray("complication"); 9606 for (int i = 0; i < array.size(); i++) { 9607 res.getComplication().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9608 } 9609 } 9610 ; 9611 if (json.has("followUp")) { 9612 JsonArray array = json.getAsJsonArray("followUp"); 9613 for (int i = 0; i < array.size(); i++) { 9614 res.getFollowUp().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9615 } 9616 } 9617 ; 9618 if (json.has("request")) 9619 res.setRequest(parseReference(json.getAsJsonObject("request"))); 9620 if (json.has("notes")) { 9621 JsonArray array = json.getAsJsonArray("notes"); 9622 for (int i = 0; i < array.size(); i++) { 9623 res.getNotes().add(parseAnnotation(array.get(i).getAsJsonObject())); 9624 } 9625 } 9626 ; 9627 if (json.has("focalDevice")) { 9628 JsonArray array = json.getAsJsonArray("focalDevice"); 9629 for (int i = 0; i < array.size(); i++) { 9630 res.getFocalDevice().add(parseProcedureProcedureFocalDeviceComponent(array.get(i).getAsJsonObject(), res)); 9631 } 9632 } 9633 ; 9634 if (json.has("used")) { 9635 JsonArray array = json.getAsJsonArray("used"); 9636 for (int i = 0; i < array.size(); i++) { 9637 res.getUsed().add(parseReference(array.get(i).getAsJsonObject())); 9638 } 9639 } 9640 ; 9641 return res; 9642 } 9643 9644 protected Procedure.ProcedurePerformerComponent parseProcedureProcedurePerformerComponent(JsonObject json, 9645 Procedure owner) throws IOException, FHIRFormatError { 9646 Procedure.ProcedurePerformerComponent res = new Procedure.ProcedurePerformerComponent(); 9647 parseBackboneProperties(json, res); 9648 if (json.has("actor")) 9649 res.setActor(parseReference(json.getAsJsonObject("actor"))); 9650 if (json.has("role")) 9651 res.setRole(parseCodeableConcept(json.getAsJsonObject("role"))); 9652 return res; 9653 } 9654 9655 protected Procedure.ProcedureFocalDeviceComponent parseProcedureProcedureFocalDeviceComponent(JsonObject json, 9656 Procedure owner) throws IOException, FHIRFormatError { 9657 Procedure.ProcedureFocalDeviceComponent res = new Procedure.ProcedureFocalDeviceComponent(); 9658 parseBackboneProperties(json, res); 9659 if (json.has("action")) 9660 res.setAction(parseCodeableConcept(json.getAsJsonObject("action"))); 9661 if (json.has("manipulated")) 9662 res.setManipulated(parseReference(json.getAsJsonObject("manipulated"))); 9663 return res; 9664 } 9665 9666 protected ProcedureRequest parseProcedureRequest(JsonObject json) throws IOException, FHIRFormatError { 9667 ProcedureRequest res = new ProcedureRequest(); 9668 parseDomainResourceProperties(json, res); 9669 if (json.has("identifier")) { 9670 JsonArray array = json.getAsJsonArray("identifier"); 9671 for (int i = 0; i < array.size(); i++) { 9672 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 9673 } 9674 } 9675 ; 9676 if (json.has("subject")) 9677 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 9678 if (json.has("code")) 9679 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 9680 if (json.has("bodySite")) { 9681 JsonArray array = json.getAsJsonArray("bodySite"); 9682 for (int i = 0; i < array.size(); i++) { 9683 res.getBodySite().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9684 } 9685 } 9686 ; 9687 Type reason = parseType("reason", json); 9688 if (reason != null) 9689 res.setReason(reason); 9690 Type scheduled = parseType("scheduled", json); 9691 if (scheduled != null) 9692 res.setScheduled(scheduled); 9693 if (json.has("encounter")) 9694 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 9695 if (json.has("performer")) 9696 res.setPerformer(parseReference(json.getAsJsonObject("performer"))); 9697 if (json.has("status")) 9698 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 9699 ProcedureRequest.ProcedureRequestStatus.NULL, new ProcedureRequest.ProcedureRequestStatusEnumFactory())); 9700 if (json.has("_status")) 9701 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 9702 if (json.has("notes")) { 9703 JsonArray array = json.getAsJsonArray("notes"); 9704 for (int i = 0; i < array.size(); i++) { 9705 res.getNotes().add(parseAnnotation(array.get(i).getAsJsonObject())); 9706 } 9707 } 9708 ; 9709 Type asNeeded = parseType("asNeeded", json); 9710 if (asNeeded != null) 9711 res.setAsNeeded(asNeeded); 9712 if (json.has("orderedOn")) 9713 res.setOrderedOnElement(parseDateTime(json.get("orderedOn").getAsString())); 9714 if (json.has("_orderedOn")) 9715 parseElementProperties(json.getAsJsonObject("_orderedOn"), res.getOrderedOnElement()); 9716 if (json.has("orderer")) 9717 res.setOrderer(parseReference(json.getAsJsonObject("orderer"))); 9718 if (json.has("priority")) 9719 res.setPriorityElement(parseEnumeration(json.get("priority").getAsString(), 9720 ProcedureRequest.ProcedureRequestPriority.NULL, new ProcedureRequest.ProcedureRequestPriorityEnumFactory())); 9721 if (json.has("_priority")) 9722 parseElementProperties(json.getAsJsonObject("_priority"), res.getPriorityElement()); 9723 return res; 9724 } 9725 9726 protected ProcessRequest parseProcessRequest(JsonObject json) throws IOException, FHIRFormatError { 9727 ProcessRequest res = new ProcessRequest(); 9728 parseDomainResourceProperties(json, res); 9729 if (json.has("action")) 9730 res.setActionElement(parseEnumeration(json.get("action").getAsString(), ProcessRequest.ActionList.NULL, 9731 new ProcessRequest.ActionListEnumFactory())); 9732 if (json.has("_action")) 9733 parseElementProperties(json.getAsJsonObject("_action"), res.getActionElement()); 9734 if (json.has("identifier")) { 9735 JsonArray array = json.getAsJsonArray("identifier"); 9736 for (int i = 0; i < array.size(); i++) { 9737 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 9738 } 9739 } 9740 ; 9741 if (json.has("ruleset")) 9742 res.setRuleset(parseCoding(json.getAsJsonObject("ruleset"))); 9743 if (json.has("originalRuleset")) 9744 res.setOriginalRuleset(parseCoding(json.getAsJsonObject("originalRuleset"))); 9745 if (json.has("created")) 9746 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 9747 if (json.has("_created")) 9748 parseElementProperties(json.getAsJsonObject("_created"), res.getCreatedElement()); 9749 if (json.has("target")) 9750 res.setTarget(parseReference(json.getAsJsonObject("target"))); 9751 if (json.has("provider")) 9752 res.setProvider(parseReference(json.getAsJsonObject("provider"))); 9753 if (json.has("organization")) 9754 res.setOrganization(parseReference(json.getAsJsonObject("organization"))); 9755 if (json.has("request")) 9756 res.setRequest(parseReference(json.getAsJsonObject("request"))); 9757 if (json.has("response")) 9758 res.setResponse(parseReference(json.getAsJsonObject("response"))); 9759 if (json.has("nullify")) 9760 res.setNullifyElement(parseBoolean(json.get("nullify").getAsBoolean())); 9761 if (json.has("_nullify")) 9762 parseElementProperties(json.getAsJsonObject("_nullify"), res.getNullifyElement()); 9763 if (json.has("reference")) 9764 res.setReferenceElement(parseString(json.get("reference").getAsString())); 9765 if (json.has("_reference")) 9766 parseElementProperties(json.getAsJsonObject("_reference"), res.getReferenceElement()); 9767 if (json.has("item")) { 9768 JsonArray array = json.getAsJsonArray("item"); 9769 for (int i = 0; i < array.size(); i++) { 9770 res.getItem().add(parseProcessRequestItemsComponent(array.get(i).getAsJsonObject(), res)); 9771 } 9772 } 9773 ; 9774 if (json.has("include")) { 9775 JsonArray array = json.getAsJsonArray("include"); 9776 for (int i = 0; i < array.size(); i++) { 9777 res.getInclude().add(parseString(array.get(i).getAsString())); 9778 } 9779 } 9780 ; 9781 if (json.has("_include")) { 9782 JsonArray array = json.getAsJsonArray("_include"); 9783 for (int i = 0; i < array.size(); i++) { 9784 if (i == res.getInclude().size()) 9785 res.getInclude().add(parseString(null)); 9786 if (array.get(i) instanceof JsonObject) 9787 parseElementProperties(array.get(i).getAsJsonObject(), res.getInclude().get(i)); 9788 } 9789 } 9790 ; 9791 if (json.has("exclude")) { 9792 JsonArray array = json.getAsJsonArray("exclude"); 9793 for (int i = 0; i < array.size(); i++) { 9794 res.getExclude().add(parseString(array.get(i).getAsString())); 9795 } 9796 } 9797 ; 9798 if (json.has("_exclude")) { 9799 JsonArray array = json.getAsJsonArray("_exclude"); 9800 for (int i = 0; i < array.size(); i++) { 9801 if (i == res.getExclude().size()) 9802 res.getExclude().add(parseString(null)); 9803 if (array.get(i) instanceof JsonObject) 9804 parseElementProperties(array.get(i).getAsJsonObject(), res.getExclude().get(i)); 9805 } 9806 } 9807 ; 9808 if (json.has("period")) 9809 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 9810 return res; 9811 } 9812 9813 protected ProcessRequest.ItemsComponent parseProcessRequestItemsComponent(JsonObject json, ProcessRequest owner) 9814 throws IOException, FHIRFormatError { 9815 ProcessRequest.ItemsComponent res = new ProcessRequest.ItemsComponent(); 9816 parseBackboneProperties(json, res); 9817 if (json.has("sequenceLinkId")) 9818 res.setSequenceLinkIdElement(parseInteger(json.get("sequenceLinkId").getAsLong())); 9819 if (json.has("_sequenceLinkId")) 9820 parseElementProperties(json.getAsJsonObject("_sequenceLinkId"), res.getSequenceLinkIdElement()); 9821 return res; 9822 } 9823 9824 protected ProcessResponse parseProcessResponse(JsonObject json) throws IOException, FHIRFormatError { 9825 ProcessResponse res = new ProcessResponse(); 9826 parseDomainResourceProperties(json, res); 9827 if (json.has("identifier")) { 9828 JsonArray array = json.getAsJsonArray("identifier"); 9829 for (int i = 0; i < array.size(); i++) { 9830 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 9831 } 9832 } 9833 ; 9834 if (json.has("request")) 9835 res.setRequest(parseReference(json.getAsJsonObject("request"))); 9836 if (json.has("outcome")) 9837 res.setOutcome(parseCoding(json.getAsJsonObject("outcome"))); 9838 if (json.has("disposition")) 9839 res.setDispositionElement(parseString(json.get("disposition").getAsString())); 9840 if (json.has("_disposition")) 9841 parseElementProperties(json.getAsJsonObject("_disposition"), res.getDispositionElement()); 9842 if (json.has("ruleset")) 9843 res.setRuleset(parseCoding(json.getAsJsonObject("ruleset"))); 9844 if (json.has("originalRuleset")) 9845 res.setOriginalRuleset(parseCoding(json.getAsJsonObject("originalRuleset"))); 9846 if (json.has("created")) 9847 res.setCreatedElement(parseDateTime(json.get("created").getAsString())); 9848 if (json.has("_created")) 9849 parseElementProperties(json.getAsJsonObject("_created"), res.getCreatedElement()); 9850 if (json.has("organization")) 9851 res.setOrganization(parseReference(json.getAsJsonObject("organization"))); 9852 if (json.has("requestProvider")) 9853 res.setRequestProvider(parseReference(json.getAsJsonObject("requestProvider"))); 9854 if (json.has("requestOrganization")) 9855 res.setRequestOrganization(parseReference(json.getAsJsonObject("requestOrganization"))); 9856 if (json.has("form")) 9857 res.setForm(parseCoding(json.getAsJsonObject("form"))); 9858 if (json.has("notes")) { 9859 JsonArray array = json.getAsJsonArray("notes"); 9860 for (int i = 0; i < array.size(); i++) { 9861 res.getNotes().add(parseProcessResponseProcessResponseNotesComponent(array.get(i).getAsJsonObject(), res)); 9862 } 9863 } 9864 ; 9865 if (json.has("error")) { 9866 JsonArray array = json.getAsJsonArray("error"); 9867 for (int i = 0; i < array.size(); i++) { 9868 res.getError().add(parseCoding(array.get(i).getAsJsonObject())); 9869 } 9870 } 9871 ; 9872 return res; 9873 } 9874 9875 protected ProcessResponse.ProcessResponseNotesComponent parseProcessResponseProcessResponseNotesComponent( 9876 JsonObject json, ProcessResponse owner) throws IOException, FHIRFormatError { 9877 ProcessResponse.ProcessResponseNotesComponent res = new ProcessResponse.ProcessResponseNotesComponent(); 9878 parseBackboneProperties(json, res); 9879 if (json.has("type")) 9880 res.setType(parseCoding(json.getAsJsonObject("type"))); 9881 if (json.has("text")) 9882 res.setTextElement(parseString(json.get("text").getAsString())); 9883 if (json.has("_text")) 9884 parseElementProperties(json.getAsJsonObject("_text"), res.getTextElement()); 9885 return res; 9886 } 9887 9888 protected Provenance parseProvenance(JsonObject json) throws IOException, FHIRFormatError { 9889 Provenance res = new Provenance(); 9890 parseDomainResourceProperties(json, res); 9891 if (json.has("target")) { 9892 JsonArray array = json.getAsJsonArray("target"); 9893 for (int i = 0; i < array.size(); i++) { 9894 res.getTarget().add(parseReference(array.get(i).getAsJsonObject())); 9895 } 9896 } 9897 ; 9898 if (json.has("period")) 9899 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 9900 if (json.has("recorded")) 9901 res.setRecordedElement(parseInstant(json.get("recorded").getAsString())); 9902 if (json.has("_recorded")) 9903 parseElementProperties(json.getAsJsonObject("_recorded"), res.getRecordedElement()); 9904 if (json.has("reason")) { 9905 JsonArray array = json.getAsJsonArray("reason"); 9906 for (int i = 0; i < array.size(); i++) { 9907 res.getReason().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 9908 } 9909 } 9910 ; 9911 if (json.has("activity")) 9912 res.setActivity(parseCodeableConcept(json.getAsJsonObject("activity"))); 9913 if (json.has("location")) 9914 res.setLocation(parseReference(json.getAsJsonObject("location"))); 9915 if (json.has("policy")) { 9916 JsonArray array = json.getAsJsonArray("policy"); 9917 for (int i = 0; i < array.size(); i++) { 9918 res.getPolicy().add(parseUri(array.get(i).getAsString())); 9919 } 9920 } 9921 ; 9922 if (json.has("_policy")) { 9923 JsonArray array = json.getAsJsonArray("_policy"); 9924 for (int i = 0; i < array.size(); i++) { 9925 if (i == res.getPolicy().size()) 9926 res.getPolicy().add(parseUri(null)); 9927 if (array.get(i) instanceof JsonObject) 9928 parseElementProperties(array.get(i).getAsJsonObject(), res.getPolicy().get(i)); 9929 } 9930 } 9931 ; 9932 if (json.has("agent")) { 9933 JsonArray array = json.getAsJsonArray("agent"); 9934 for (int i = 0; i < array.size(); i++) { 9935 res.getAgent().add(parseProvenanceProvenanceAgentComponent(array.get(i).getAsJsonObject(), res)); 9936 } 9937 } 9938 ; 9939 if (json.has("entity")) { 9940 JsonArray array = json.getAsJsonArray("entity"); 9941 for (int i = 0; i < array.size(); i++) { 9942 res.getEntity().add(parseProvenanceProvenanceEntityComponent(array.get(i).getAsJsonObject(), res)); 9943 } 9944 } 9945 ; 9946 if (json.has("signature")) { 9947 JsonArray array = json.getAsJsonArray("signature"); 9948 for (int i = 0; i < array.size(); i++) { 9949 res.getSignature().add(parseSignature(array.get(i).getAsJsonObject())); 9950 } 9951 } 9952 ; 9953 return res; 9954 } 9955 9956 protected Provenance.ProvenanceAgentComponent parseProvenanceProvenanceAgentComponent(JsonObject json, 9957 Provenance owner) throws IOException, FHIRFormatError { 9958 Provenance.ProvenanceAgentComponent res = new Provenance.ProvenanceAgentComponent(); 9959 parseBackboneProperties(json, res); 9960 if (json.has("role")) 9961 res.setRole(parseCoding(json.getAsJsonObject("role"))); 9962 if (json.has("actor")) 9963 res.setActor(parseReference(json.getAsJsonObject("actor"))); 9964 if (json.has("userId")) 9965 res.setUserId(parseIdentifier(json.getAsJsonObject("userId"))); 9966 if (json.has("relatedAgent")) { 9967 JsonArray array = json.getAsJsonArray("relatedAgent"); 9968 for (int i = 0; i < array.size(); i++) { 9969 res.getRelatedAgent() 9970 .add(parseProvenanceProvenanceAgentRelatedAgentComponent(array.get(i).getAsJsonObject(), owner)); 9971 } 9972 } 9973 ; 9974 return res; 9975 } 9976 9977 protected Provenance.ProvenanceAgentRelatedAgentComponent parseProvenanceProvenanceAgentRelatedAgentComponent( 9978 JsonObject json, Provenance owner) throws IOException, FHIRFormatError { 9979 Provenance.ProvenanceAgentRelatedAgentComponent res = new Provenance.ProvenanceAgentRelatedAgentComponent(); 9980 parseBackboneProperties(json, res); 9981 if (json.has("type")) 9982 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 9983 if (json.has("target")) 9984 res.setTargetElement(parseUri(json.get("target").getAsString())); 9985 if (json.has("_target")) 9986 parseElementProperties(json.getAsJsonObject("_target"), res.getTargetElement()); 9987 return res; 9988 } 9989 9990 protected Provenance.ProvenanceEntityComponent parseProvenanceProvenanceEntityComponent(JsonObject json, 9991 Provenance owner) throws IOException, FHIRFormatError { 9992 Provenance.ProvenanceEntityComponent res = new Provenance.ProvenanceEntityComponent(); 9993 parseBackboneProperties(json, res); 9994 if (json.has("role")) 9995 res.setRoleElement(parseEnumeration(json.get("role").getAsString(), Provenance.ProvenanceEntityRole.NULL, 9996 new Provenance.ProvenanceEntityRoleEnumFactory())); 9997 if (json.has("_role")) 9998 parseElementProperties(json.getAsJsonObject("_role"), res.getRoleElement()); 9999 if (json.has("type")) 10000 res.setType(parseCoding(json.getAsJsonObject("type"))); 10001 if (json.has("reference")) 10002 res.setReferenceElement(parseUri(json.get("reference").getAsString())); 10003 if (json.has("_reference")) 10004 parseElementProperties(json.getAsJsonObject("_reference"), res.getReferenceElement()); 10005 if (json.has("display")) 10006 res.setDisplayElement(parseString(json.get("display").getAsString())); 10007 if (json.has("_display")) 10008 parseElementProperties(json.getAsJsonObject("_display"), res.getDisplayElement()); 10009 if (json.has("agent")) 10010 res.setAgent(parseProvenanceProvenanceAgentComponent(json.getAsJsonObject("agent"), owner)); 10011 return res; 10012 } 10013 10014 protected Questionnaire parseQuestionnaire(JsonObject json) throws IOException, FHIRFormatError { 10015 Questionnaire res = new Questionnaire(); 10016 parseDomainResourceProperties(json, res); 10017 if (json.has("identifier")) { 10018 JsonArray array = json.getAsJsonArray("identifier"); 10019 for (int i = 0; i < array.size(); i++) { 10020 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 10021 } 10022 } 10023 ; 10024 if (json.has("version")) 10025 res.setVersionElement(parseString(json.get("version").getAsString())); 10026 if (json.has("_version")) 10027 parseElementProperties(json.getAsJsonObject("_version"), res.getVersionElement()); 10028 if (json.has("status")) 10029 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Questionnaire.QuestionnaireStatus.NULL, 10030 new Questionnaire.QuestionnaireStatusEnumFactory())); 10031 if (json.has("_status")) 10032 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 10033 if (json.has("date")) 10034 res.setDateElement(parseDateTime(json.get("date").getAsString())); 10035 if (json.has("_date")) 10036 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 10037 if (json.has("publisher")) 10038 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 10039 if (json.has("_publisher")) 10040 parseElementProperties(json.getAsJsonObject("_publisher"), res.getPublisherElement()); 10041 if (json.has("telecom")) { 10042 JsonArray array = json.getAsJsonArray("telecom"); 10043 for (int i = 0; i < array.size(); i++) { 10044 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 10045 } 10046 } 10047 ; 10048 if (json.has("subjectType")) { 10049 JsonArray array = json.getAsJsonArray("subjectType"); 10050 for (int i = 0; i < array.size(); i++) { 10051 res.getSubjectType().add(parseCode(array.get(i).getAsString())); 10052 } 10053 } 10054 ; 10055 if (json.has("_subjectType")) { 10056 JsonArray array = json.getAsJsonArray("_subjectType"); 10057 for (int i = 0; i < array.size(); i++) { 10058 if (i == res.getSubjectType().size()) 10059 res.getSubjectType().add(parseCode(null)); 10060 if (array.get(i) instanceof JsonObject) 10061 parseElementProperties(array.get(i).getAsJsonObject(), res.getSubjectType().get(i)); 10062 } 10063 } 10064 ; 10065 if (json.has("group")) 10066 res.setGroup(parseQuestionnaireGroupComponent(json.getAsJsonObject("group"), res)); 10067 return res; 10068 } 10069 10070 protected Questionnaire.GroupComponent parseQuestionnaireGroupComponent(JsonObject json, Questionnaire owner) 10071 throws IOException, FHIRFormatError { 10072 Questionnaire.GroupComponent res = new Questionnaire.GroupComponent(); 10073 parseBackboneProperties(json, res); 10074 if (json.has("linkId")) 10075 res.setLinkIdElement(parseString(json.get("linkId").getAsString())); 10076 if (json.has("_linkId")) 10077 parseElementProperties(json.getAsJsonObject("_linkId"), res.getLinkIdElement()); 10078 if (json.has("title")) 10079 res.setTitleElement(parseString(json.get("title").getAsString())); 10080 if (json.has("_title")) 10081 parseElementProperties(json.getAsJsonObject("_title"), res.getTitleElement()); 10082 if (json.has("concept")) { 10083 JsonArray array = json.getAsJsonArray("concept"); 10084 for (int i = 0; i < array.size(); i++) { 10085 res.getConcept().add(parseCoding(array.get(i).getAsJsonObject())); 10086 } 10087 } 10088 ; 10089 if (json.has("text")) 10090 res.setTextElement(parseString(json.get("text").getAsString())); 10091 if (json.has("_text")) 10092 parseElementProperties(json.getAsJsonObject("_text"), res.getTextElement()); 10093 if (json.has("required")) 10094 res.setRequiredElement(parseBoolean(json.get("required").getAsBoolean())); 10095 if (json.has("_required")) 10096 parseElementProperties(json.getAsJsonObject("_required"), res.getRequiredElement()); 10097 if (json.has("repeats")) 10098 res.setRepeatsElement(parseBoolean(json.get("repeats").getAsBoolean())); 10099 if (json.has("_repeats")) 10100 parseElementProperties(json.getAsJsonObject("_repeats"), res.getRepeatsElement()); 10101 if (json.has("group")) { 10102 JsonArray array = json.getAsJsonArray("group"); 10103 for (int i = 0; i < array.size(); i++) { 10104 res.getGroup().add(parseQuestionnaireGroupComponent(array.get(i).getAsJsonObject(), owner)); 10105 } 10106 } 10107 ; 10108 if (json.has("question")) { 10109 JsonArray array = json.getAsJsonArray("question"); 10110 for (int i = 0; i < array.size(); i++) { 10111 res.getQuestion().add(parseQuestionnaireQuestionComponent(array.get(i).getAsJsonObject(), owner)); 10112 } 10113 } 10114 ; 10115 return res; 10116 } 10117 10118 protected Questionnaire.QuestionComponent parseQuestionnaireQuestionComponent(JsonObject json, Questionnaire owner) 10119 throws IOException, FHIRFormatError { 10120 Questionnaire.QuestionComponent res = new Questionnaire.QuestionComponent(); 10121 parseBackboneProperties(json, res); 10122 if (json.has("linkId")) 10123 res.setLinkIdElement(parseString(json.get("linkId").getAsString())); 10124 if (json.has("_linkId")) 10125 parseElementProperties(json.getAsJsonObject("_linkId"), res.getLinkIdElement()); 10126 if (json.has("concept")) { 10127 JsonArray array = json.getAsJsonArray("concept"); 10128 for (int i = 0; i < array.size(); i++) { 10129 res.getConcept().add(parseCoding(array.get(i).getAsJsonObject())); 10130 } 10131 } 10132 ; 10133 if (json.has("text")) 10134 res.setTextElement(parseString(json.get("text").getAsString())); 10135 if (json.has("_text")) 10136 parseElementProperties(json.getAsJsonObject("_text"), res.getTextElement()); 10137 if (json.has("type")) 10138 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Questionnaire.AnswerFormat.NULL, 10139 new Questionnaire.AnswerFormatEnumFactory())); 10140 if (json.has("_type")) 10141 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 10142 if (json.has("required")) 10143 res.setRequiredElement(parseBoolean(json.get("required").getAsBoolean())); 10144 if (json.has("_required")) 10145 parseElementProperties(json.getAsJsonObject("_required"), res.getRequiredElement()); 10146 if (json.has("repeats")) 10147 res.setRepeatsElement(parseBoolean(json.get("repeats").getAsBoolean())); 10148 if (json.has("_repeats")) 10149 parseElementProperties(json.getAsJsonObject("_repeats"), res.getRepeatsElement()); 10150 if (json.has("options")) 10151 res.setOptions(parseReference(json.getAsJsonObject("options"))); 10152 if (json.has("option")) { 10153 JsonArray array = json.getAsJsonArray("option"); 10154 for (int i = 0; i < array.size(); i++) { 10155 res.getOption().add(parseCoding(array.get(i).getAsJsonObject())); 10156 } 10157 } 10158 ; 10159 if (json.has("group")) { 10160 JsonArray array = json.getAsJsonArray("group"); 10161 for (int i = 0; i < array.size(); i++) { 10162 res.getGroup().add(parseQuestionnaireGroupComponent(array.get(i).getAsJsonObject(), owner)); 10163 } 10164 } 10165 ; 10166 return res; 10167 } 10168 10169 protected QuestionnaireResponse parseQuestionnaireResponse(JsonObject json) throws IOException, FHIRFormatError { 10170 QuestionnaireResponse res = new QuestionnaireResponse(); 10171 parseDomainResourceProperties(json, res); 10172 if (json.has("identifier")) 10173 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 10174 if (json.has("questionnaire")) 10175 res.setQuestionnaire(parseReference(json.getAsJsonObject("questionnaire"))); 10176 if (json.has("status")) 10177 res.setStatusElement( 10178 parseEnumeration(json.get("status").getAsString(), QuestionnaireResponse.QuestionnaireResponseStatus.NULL, 10179 new QuestionnaireResponse.QuestionnaireResponseStatusEnumFactory())); 10180 if (json.has("_status")) 10181 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 10182 if (json.has("subject")) 10183 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 10184 if (json.has("author")) 10185 res.setAuthor(parseReference(json.getAsJsonObject("author"))); 10186 if (json.has("authored")) 10187 res.setAuthoredElement(parseDateTime(json.get("authored").getAsString())); 10188 if (json.has("_authored")) 10189 parseElementProperties(json.getAsJsonObject("_authored"), res.getAuthoredElement()); 10190 if (json.has("source")) 10191 res.setSource(parseReference(json.getAsJsonObject("source"))); 10192 if (json.has("encounter")) 10193 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 10194 if (json.has("group")) 10195 res.setGroup(parseQuestionnaireResponseGroupComponent(json.getAsJsonObject("group"), res)); 10196 return res; 10197 } 10198 10199 protected QuestionnaireResponse.GroupComponent parseQuestionnaireResponseGroupComponent(JsonObject json, 10200 QuestionnaireResponse owner) throws IOException, FHIRFormatError { 10201 QuestionnaireResponse.GroupComponent res = new QuestionnaireResponse.GroupComponent(); 10202 parseBackboneProperties(json, res); 10203 if (json.has("linkId")) 10204 res.setLinkIdElement(parseString(json.get("linkId").getAsString())); 10205 if (json.has("_linkId")) 10206 parseElementProperties(json.getAsJsonObject("_linkId"), res.getLinkIdElement()); 10207 if (json.has("title")) 10208 res.setTitleElement(parseString(json.get("title").getAsString())); 10209 if (json.has("_title")) 10210 parseElementProperties(json.getAsJsonObject("_title"), res.getTitleElement()); 10211 if (json.has("text")) 10212 res.setTextElement(parseString(json.get("text").getAsString())); 10213 if (json.has("_text")) 10214 parseElementProperties(json.getAsJsonObject("_text"), res.getTextElement()); 10215 if (json.has("subject")) 10216 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 10217 if (json.has("group")) { 10218 JsonArray array = json.getAsJsonArray("group"); 10219 for (int i = 0; i < array.size(); i++) { 10220 res.getGroup().add(parseQuestionnaireResponseGroupComponent(array.get(i).getAsJsonObject(), owner)); 10221 } 10222 } 10223 ; 10224 if (json.has("question")) { 10225 JsonArray array = json.getAsJsonArray("question"); 10226 for (int i = 0; i < array.size(); i++) { 10227 res.getQuestion().add(parseQuestionnaireResponseQuestionComponent(array.get(i).getAsJsonObject(), owner)); 10228 } 10229 } 10230 ; 10231 return res; 10232 } 10233 10234 protected QuestionnaireResponse.QuestionComponent parseQuestionnaireResponseQuestionComponent(JsonObject json, 10235 QuestionnaireResponse owner) throws IOException, FHIRFormatError { 10236 QuestionnaireResponse.QuestionComponent res = new QuestionnaireResponse.QuestionComponent(); 10237 parseBackboneProperties(json, res); 10238 if (json.has("linkId")) 10239 res.setLinkIdElement(parseString(json.get("linkId").getAsString())); 10240 if (json.has("_linkId")) 10241 parseElementProperties(json.getAsJsonObject("_linkId"), res.getLinkIdElement()); 10242 if (json.has("text")) 10243 res.setTextElement(parseString(json.get("text").getAsString())); 10244 if (json.has("_text")) 10245 parseElementProperties(json.getAsJsonObject("_text"), res.getTextElement()); 10246 if (json.has("answer")) { 10247 JsonArray array = json.getAsJsonArray("answer"); 10248 for (int i = 0; i < array.size(); i++) { 10249 res.getAnswer().add(parseQuestionnaireResponseQuestionAnswerComponent(array.get(i).getAsJsonObject(), owner)); 10250 } 10251 } 10252 ; 10253 return res; 10254 } 10255 10256 protected QuestionnaireResponse.QuestionAnswerComponent parseQuestionnaireResponseQuestionAnswerComponent( 10257 JsonObject json, QuestionnaireResponse owner) throws IOException, FHIRFormatError { 10258 QuestionnaireResponse.QuestionAnswerComponent res = new QuestionnaireResponse.QuestionAnswerComponent(); 10259 parseBackboneProperties(json, res); 10260 Type value = parseType("value", json); 10261 if (value != null) 10262 res.setValue(value); 10263 if (json.has("group")) { 10264 JsonArray array = json.getAsJsonArray("group"); 10265 for (int i = 0; i < array.size(); i++) { 10266 res.getGroup().add(parseQuestionnaireResponseGroupComponent(array.get(i).getAsJsonObject(), owner)); 10267 } 10268 } 10269 ; 10270 return res; 10271 } 10272 10273 protected ReferralRequest parseReferralRequest(JsonObject json) throws IOException, FHIRFormatError { 10274 ReferralRequest res = new ReferralRequest(); 10275 parseDomainResourceProperties(json, res); 10276 if (json.has("status")) 10277 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), ReferralRequest.ReferralStatus.NULL, 10278 new ReferralRequest.ReferralStatusEnumFactory())); 10279 if (json.has("_status")) 10280 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 10281 if (json.has("identifier")) { 10282 JsonArray array = json.getAsJsonArray("identifier"); 10283 for (int i = 0; i < array.size(); i++) { 10284 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 10285 } 10286 } 10287 ; 10288 if (json.has("date")) 10289 res.setDateElement(parseDateTime(json.get("date").getAsString())); 10290 if (json.has("_date")) 10291 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 10292 if (json.has("type")) 10293 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 10294 if (json.has("specialty")) 10295 res.setSpecialty(parseCodeableConcept(json.getAsJsonObject("specialty"))); 10296 if (json.has("priority")) 10297 res.setPriority(parseCodeableConcept(json.getAsJsonObject("priority"))); 10298 if (json.has("patient")) 10299 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 10300 if (json.has("requester")) 10301 res.setRequester(parseReference(json.getAsJsonObject("requester"))); 10302 if (json.has("recipient")) { 10303 JsonArray array = json.getAsJsonArray("recipient"); 10304 for (int i = 0; i < array.size(); i++) { 10305 res.getRecipient().add(parseReference(array.get(i).getAsJsonObject())); 10306 } 10307 } 10308 ; 10309 if (json.has("encounter")) 10310 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 10311 if (json.has("dateSent")) 10312 res.setDateSentElement(parseDateTime(json.get("dateSent").getAsString())); 10313 if (json.has("_dateSent")) 10314 parseElementProperties(json.getAsJsonObject("_dateSent"), res.getDateSentElement()); 10315 if (json.has("reason")) 10316 res.setReason(parseCodeableConcept(json.getAsJsonObject("reason"))); 10317 if (json.has("description")) 10318 res.setDescriptionElement(parseString(json.get("description").getAsString())); 10319 if (json.has("_description")) 10320 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 10321 if (json.has("serviceRequested")) { 10322 JsonArray array = json.getAsJsonArray("serviceRequested"); 10323 for (int i = 0; i < array.size(); i++) { 10324 res.getServiceRequested().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 10325 } 10326 } 10327 ; 10328 if (json.has("supportingInformation")) { 10329 JsonArray array = json.getAsJsonArray("supportingInformation"); 10330 for (int i = 0; i < array.size(); i++) { 10331 res.getSupportingInformation().add(parseReference(array.get(i).getAsJsonObject())); 10332 } 10333 } 10334 ; 10335 if (json.has("fulfillmentTime")) 10336 res.setFulfillmentTime(parsePeriod(json.getAsJsonObject("fulfillmentTime"))); 10337 return res; 10338 } 10339 10340 protected RelatedPerson parseRelatedPerson(JsonObject json) throws IOException, FHIRFormatError { 10341 RelatedPerson res = new RelatedPerson(); 10342 parseDomainResourceProperties(json, res); 10343 if (json.has("identifier")) { 10344 JsonArray array = json.getAsJsonArray("identifier"); 10345 for (int i = 0; i < array.size(); i++) { 10346 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 10347 } 10348 } 10349 ; 10350 if (json.has("patient")) 10351 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 10352 if (json.has("relationship")) 10353 res.setRelationship(parseCodeableConcept(json.getAsJsonObject("relationship"))); 10354 if (json.has("name")) 10355 res.setName(parseHumanName(json.getAsJsonObject("name"))); 10356 if (json.has("telecom")) { 10357 JsonArray array = json.getAsJsonArray("telecom"); 10358 for (int i = 0; i < array.size(); i++) { 10359 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 10360 } 10361 } 10362 ; 10363 if (json.has("gender")) 10364 res.setGenderElement(parseEnumeration(json.get("gender").getAsString(), Enumerations.AdministrativeGender.NULL, 10365 new Enumerations.AdministrativeGenderEnumFactory())); 10366 if (json.has("_gender")) 10367 parseElementProperties(json.getAsJsonObject("_gender"), res.getGenderElement()); 10368 if (json.has("birthDate")) 10369 res.setBirthDateElement(parseDate(json.get("birthDate").getAsString())); 10370 if (json.has("_birthDate")) 10371 parseElementProperties(json.getAsJsonObject("_birthDate"), res.getBirthDateElement()); 10372 if (json.has("address")) { 10373 JsonArray array = json.getAsJsonArray("address"); 10374 for (int i = 0; i < array.size(); i++) { 10375 res.getAddress().add(parseAddress(array.get(i).getAsJsonObject())); 10376 } 10377 } 10378 ; 10379 if (json.has("photo")) { 10380 JsonArray array = json.getAsJsonArray("photo"); 10381 for (int i = 0; i < array.size(); i++) { 10382 res.getPhoto().add(parseAttachment(array.get(i).getAsJsonObject())); 10383 } 10384 } 10385 ; 10386 if (json.has("period")) 10387 res.setPeriod(parsePeriod(json.getAsJsonObject("period"))); 10388 return res; 10389 } 10390 10391 protected RiskAssessment parseRiskAssessment(JsonObject json) throws IOException, FHIRFormatError { 10392 RiskAssessment res = new RiskAssessment(); 10393 parseDomainResourceProperties(json, res); 10394 if (json.has("subject")) 10395 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 10396 if (json.has("date")) 10397 res.setDateElement(parseDateTime(json.get("date").getAsString())); 10398 if (json.has("_date")) 10399 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 10400 if (json.has("condition")) 10401 res.setCondition(parseReference(json.getAsJsonObject("condition"))); 10402 if (json.has("encounter")) 10403 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 10404 if (json.has("performer")) 10405 res.setPerformer(parseReference(json.getAsJsonObject("performer"))); 10406 if (json.has("identifier")) 10407 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 10408 if (json.has("method")) 10409 res.setMethod(parseCodeableConcept(json.getAsJsonObject("method"))); 10410 if (json.has("basis")) { 10411 JsonArray array = json.getAsJsonArray("basis"); 10412 for (int i = 0; i < array.size(); i++) { 10413 res.getBasis().add(parseReference(array.get(i).getAsJsonObject())); 10414 } 10415 } 10416 ; 10417 if (json.has("prediction")) { 10418 JsonArray array = json.getAsJsonArray("prediction"); 10419 for (int i = 0; i < array.size(); i++) { 10420 res.getPrediction() 10421 .add(parseRiskAssessmentRiskAssessmentPredictionComponent(array.get(i).getAsJsonObject(), res)); 10422 } 10423 } 10424 ; 10425 if (json.has("mitigation")) 10426 res.setMitigationElement(parseString(json.get("mitigation").getAsString())); 10427 if (json.has("_mitigation")) 10428 parseElementProperties(json.getAsJsonObject("_mitigation"), res.getMitigationElement()); 10429 return res; 10430 } 10431 10432 protected RiskAssessment.RiskAssessmentPredictionComponent parseRiskAssessmentRiskAssessmentPredictionComponent( 10433 JsonObject json, RiskAssessment owner) throws IOException, FHIRFormatError { 10434 RiskAssessment.RiskAssessmentPredictionComponent res = new RiskAssessment.RiskAssessmentPredictionComponent(); 10435 parseBackboneProperties(json, res); 10436 if (json.has("outcome")) 10437 res.setOutcome(parseCodeableConcept(json.getAsJsonObject("outcome"))); 10438 Type probability = parseType("probability", json); 10439 if (probability != null) 10440 res.setProbability(probability); 10441 if (json.has("relativeRisk")) 10442 res.setRelativeRiskElement(parseDecimal(json.get("relativeRisk").getAsBigDecimal())); 10443 if (json.has("_relativeRisk")) 10444 parseElementProperties(json.getAsJsonObject("_relativeRisk"), res.getRelativeRiskElement()); 10445 Type when = parseType("when", json); 10446 if (when != null) 10447 res.setWhen(when); 10448 if (json.has("rationale")) 10449 res.setRationaleElement(parseString(json.get("rationale").getAsString())); 10450 if (json.has("_rationale")) 10451 parseElementProperties(json.getAsJsonObject("_rationale"), res.getRationaleElement()); 10452 return res; 10453 } 10454 10455 protected Schedule parseSchedule(JsonObject json) throws IOException, FHIRFormatError { 10456 Schedule res = new Schedule(); 10457 parseDomainResourceProperties(json, res); 10458 if (json.has("identifier")) { 10459 JsonArray array = json.getAsJsonArray("identifier"); 10460 for (int i = 0; i < array.size(); i++) { 10461 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 10462 } 10463 } 10464 ; 10465 if (json.has("type")) { 10466 JsonArray array = json.getAsJsonArray("type"); 10467 for (int i = 0; i < array.size(); i++) { 10468 res.getType().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 10469 } 10470 } 10471 ; 10472 if (json.has("actor")) 10473 res.setActor(parseReference(json.getAsJsonObject("actor"))); 10474 if (json.has("planningHorizon")) 10475 res.setPlanningHorizon(parsePeriod(json.getAsJsonObject("planningHorizon"))); 10476 if (json.has("comment")) 10477 res.setCommentElement(parseString(json.get("comment").getAsString())); 10478 if (json.has("_comment")) 10479 parseElementProperties(json.getAsJsonObject("_comment"), res.getCommentElement()); 10480 return res; 10481 } 10482 10483 protected SearchParameter parseSearchParameter(JsonObject json) throws IOException, FHIRFormatError { 10484 SearchParameter res = new SearchParameter(); 10485 parseDomainResourceProperties(json, res); 10486 if (json.has("url")) 10487 res.setUrlElement(parseUri(json.get("url").getAsString())); 10488 if (json.has("_url")) 10489 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 10490 if (json.has("name")) 10491 res.setNameElement(parseString(json.get("name").getAsString())); 10492 if (json.has("_name")) 10493 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 10494 if (json.has("status")) 10495 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 10496 Enumerations.ConformanceResourceStatus.NULL, new Enumerations.ConformanceResourceStatusEnumFactory())); 10497 if (json.has("_status")) 10498 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 10499 if (json.has("experimental")) 10500 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 10501 if (json.has("_experimental")) 10502 parseElementProperties(json.getAsJsonObject("_experimental"), res.getExperimentalElement()); 10503 if (json.has("publisher")) 10504 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 10505 if (json.has("_publisher")) 10506 parseElementProperties(json.getAsJsonObject("_publisher"), res.getPublisherElement()); 10507 if (json.has("contact")) { 10508 JsonArray array = json.getAsJsonArray("contact"); 10509 for (int i = 0; i < array.size(); i++) { 10510 res.getContact().add(parseSearchParameterSearchParameterContactComponent(array.get(i).getAsJsonObject(), res)); 10511 } 10512 } 10513 ; 10514 if (json.has("date")) 10515 res.setDateElement(parseDateTime(json.get("date").getAsString())); 10516 if (json.has("_date")) 10517 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 10518 if (json.has("requirements")) 10519 res.setRequirementsElement(parseString(json.get("requirements").getAsString())); 10520 if (json.has("_requirements")) 10521 parseElementProperties(json.getAsJsonObject("_requirements"), res.getRequirementsElement()); 10522 if (json.has("code")) 10523 res.setCodeElement(parseCode(json.get("code").getAsString())); 10524 if (json.has("_code")) 10525 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 10526 if (json.has("base")) 10527 res.setBaseElement(parseCode(json.get("base").getAsString())); 10528 if (json.has("_base")) 10529 parseElementProperties(json.getAsJsonObject("_base"), res.getBaseElement()); 10530 if (json.has("type")) 10531 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Enumerations.SearchParamType.NULL, 10532 new Enumerations.SearchParamTypeEnumFactory())); 10533 if (json.has("_type")) 10534 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 10535 if (json.has("description")) 10536 res.setDescriptionElement(parseString(json.get("description").getAsString())); 10537 if (json.has("_description")) 10538 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 10539 if (json.has("xpath")) 10540 res.setXpathElement(parseString(json.get("xpath").getAsString())); 10541 if (json.has("_xpath")) 10542 parseElementProperties(json.getAsJsonObject("_xpath"), res.getXpathElement()); 10543 if (json.has("xpathUsage")) 10544 res.setXpathUsageElement(parseEnumeration(json.get("xpathUsage").getAsString(), 10545 SearchParameter.XPathUsageType.NULL, new SearchParameter.XPathUsageTypeEnumFactory())); 10546 if (json.has("_xpathUsage")) 10547 parseElementProperties(json.getAsJsonObject("_xpathUsage"), res.getXpathUsageElement()); 10548 if (json.has("target")) { 10549 JsonArray array = json.getAsJsonArray("target"); 10550 for (int i = 0; i < array.size(); i++) { 10551 res.getTarget().add(parseCode(array.get(i).getAsString())); 10552 } 10553 } 10554 ; 10555 if (json.has("_target")) { 10556 JsonArray array = json.getAsJsonArray("_target"); 10557 for (int i = 0; i < array.size(); i++) { 10558 if (i == res.getTarget().size()) 10559 res.getTarget().add(parseCode(null)); 10560 if (array.get(i) instanceof JsonObject) 10561 parseElementProperties(array.get(i).getAsJsonObject(), res.getTarget().get(i)); 10562 } 10563 } 10564 ; 10565 return res; 10566 } 10567 10568 protected SearchParameter.SearchParameterContactComponent parseSearchParameterSearchParameterContactComponent( 10569 JsonObject json, SearchParameter owner) throws IOException, FHIRFormatError { 10570 SearchParameter.SearchParameterContactComponent res = new SearchParameter.SearchParameterContactComponent(); 10571 parseBackboneProperties(json, res); 10572 if (json.has("name")) 10573 res.setNameElement(parseString(json.get("name").getAsString())); 10574 if (json.has("_name")) 10575 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 10576 if (json.has("telecom")) { 10577 JsonArray array = json.getAsJsonArray("telecom"); 10578 for (int i = 0; i < array.size(); i++) { 10579 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 10580 } 10581 } 10582 ; 10583 return res; 10584 } 10585 10586 protected Slot parseSlot(JsonObject json) throws IOException, FHIRFormatError { 10587 Slot res = new Slot(); 10588 parseDomainResourceProperties(json, res); 10589 if (json.has("identifier")) { 10590 JsonArray array = json.getAsJsonArray("identifier"); 10591 for (int i = 0; i < array.size(); i++) { 10592 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 10593 } 10594 } 10595 ; 10596 if (json.has("type")) 10597 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 10598 if (json.has("schedule")) 10599 res.setSchedule(parseReference(json.getAsJsonObject("schedule"))); 10600 if (json.has("freeBusyType")) 10601 res.setFreeBusyTypeElement(parseEnumeration(json.get("freeBusyType").getAsString(), Slot.SlotStatus.NULL, 10602 new Slot.SlotStatusEnumFactory())); 10603 if (json.has("_freeBusyType")) 10604 parseElementProperties(json.getAsJsonObject("_freeBusyType"), res.getFreeBusyTypeElement()); 10605 if (json.has("start")) 10606 res.setStartElement(parseInstant(json.get("start").getAsString())); 10607 if (json.has("_start")) 10608 parseElementProperties(json.getAsJsonObject("_start"), res.getStartElement()); 10609 if (json.has("end")) 10610 res.setEndElement(parseInstant(json.get("end").getAsString())); 10611 if (json.has("_end")) 10612 parseElementProperties(json.getAsJsonObject("_end"), res.getEndElement()); 10613 if (json.has("overbooked")) 10614 res.setOverbookedElement(parseBoolean(json.get("overbooked").getAsBoolean())); 10615 if (json.has("_overbooked")) 10616 parseElementProperties(json.getAsJsonObject("_overbooked"), res.getOverbookedElement()); 10617 if (json.has("comment")) 10618 res.setCommentElement(parseString(json.get("comment").getAsString())); 10619 if (json.has("_comment")) 10620 parseElementProperties(json.getAsJsonObject("_comment"), res.getCommentElement()); 10621 return res; 10622 } 10623 10624 protected Specimen parseSpecimen(JsonObject json) throws IOException, FHIRFormatError { 10625 Specimen res = new Specimen(); 10626 parseDomainResourceProperties(json, res); 10627 if (json.has("identifier")) { 10628 JsonArray array = json.getAsJsonArray("identifier"); 10629 for (int i = 0; i < array.size(); i++) { 10630 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 10631 } 10632 } 10633 ; 10634 if (json.has("status")) 10635 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Specimen.SpecimenStatus.NULL, 10636 new Specimen.SpecimenStatusEnumFactory())); 10637 if (json.has("_status")) 10638 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 10639 if (json.has("type")) 10640 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 10641 if (json.has("parent")) { 10642 JsonArray array = json.getAsJsonArray("parent"); 10643 for (int i = 0; i < array.size(); i++) { 10644 res.getParent().add(parseReference(array.get(i).getAsJsonObject())); 10645 } 10646 } 10647 ; 10648 if (json.has("subject")) 10649 res.setSubject(parseReference(json.getAsJsonObject("subject"))); 10650 if (json.has("accessionIdentifier")) 10651 res.setAccessionIdentifier(parseIdentifier(json.getAsJsonObject("accessionIdentifier"))); 10652 if (json.has("receivedTime")) 10653 res.setReceivedTimeElement(parseDateTime(json.get("receivedTime").getAsString())); 10654 if (json.has("_receivedTime")) 10655 parseElementProperties(json.getAsJsonObject("_receivedTime"), res.getReceivedTimeElement()); 10656 if (json.has("collection")) 10657 res.setCollection(parseSpecimenSpecimenCollectionComponent(json.getAsJsonObject("collection"), res)); 10658 if (json.has("treatment")) { 10659 JsonArray array = json.getAsJsonArray("treatment"); 10660 for (int i = 0; i < array.size(); i++) { 10661 res.getTreatment().add(parseSpecimenSpecimenTreatmentComponent(array.get(i).getAsJsonObject(), res)); 10662 } 10663 } 10664 ; 10665 if (json.has("container")) { 10666 JsonArray array = json.getAsJsonArray("container"); 10667 for (int i = 0; i < array.size(); i++) { 10668 res.getContainer().add(parseSpecimenSpecimenContainerComponent(array.get(i).getAsJsonObject(), res)); 10669 } 10670 } 10671 ; 10672 return res; 10673 } 10674 10675 protected Specimen.SpecimenCollectionComponent parseSpecimenSpecimenCollectionComponent(JsonObject json, 10676 Specimen owner) throws IOException, FHIRFormatError { 10677 Specimen.SpecimenCollectionComponent res = new Specimen.SpecimenCollectionComponent(); 10678 parseBackboneProperties(json, res); 10679 if (json.has("collector")) 10680 res.setCollector(parseReference(json.getAsJsonObject("collector"))); 10681 if (json.has("comment")) { 10682 JsonArray array = json.getAsJsonArray("comment"); 10683 for (int i = 0; i < array.size(); i++) { 10684 res.getComment().add(parseString(array.get(i).getAsString())); 10685 } 10686 } 10687 ; 10688 if (json.has("_comment")) { 10689 JsonArray array = json.getAsJsonArray("_comment"); 10690 for (int i = 0; i < array.size(); i++) { 10691 if (i == res.getComment().size()) 10692 res.getComment().add(parseString(null)); 10693 if (array.get(i) instanceof JsonObject) 10694 parseElementProperties(array.get(i).getAsJsonObject(), res.getComment().get(i)); 10695 } 10696 } 10697 ; 10698 Type collected = parseType("collected", json); 10699 if (collected != null) 10700 res.setCollected(collected); 10701 if (json.has("quantity")) 10702 res.setQuantity(parseSimpleQuantity(json.getAsJsonObject("quantity"))); 10703 if (json.has("method")) 10704 res.setMethod(parseCodeableConcept(json.getAsJsonObject("method"))); 10705 if (json.has("bodySite")) 10706 res.setBodySite(parseCodeableConcept(json.getAsJsonObject("bodySite"))); 10707 return res; 10708 } 10709 10710 protected Specimen.SpecimenTreatmentComponent parseSpecimenSpecimenTreatmentComponent(JsonObject json, Specimen owner) 10711 throws IOException, FHIRFormatError { 10712 Specimen.SpecimenTreatmentComponent res = new Specimen.SpecimenTreatmentComponent(); 10713 parseBackboneProperties(json, res); 10714 if (json.has("description")) 10715 res.setDescriptionElement(parseString(json.get("description").getAsString())); 10716 if (json.has("_description")) 10717 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 10718 if (json.has("procedure")) 10719 res.setProcedure(parseCodeableConcept(json.getAsJsonObject("procedure"))); 10720 if (json.has("additive")) { 10721 JsonArray array = json.getAsJsonArray("additive"); 10722 for (int i = 0; i < array.size(); i++) { 10723 res.getAdditive().add(parseReference(array.get(i).getAsJsonObject())); 10724 } 10725 } 10726 ; 10727 return res; 10728 } 10729 10730 protected Specimen.SpecimenContainerComponent parseSpecimenSpecimenContainerComponent(JsonObject json, Specimen owner) 10731 throws IOException, FHIRFormatError { 10732 Specimen.SpecimenContainerComponent res = new Specimen.SpecimenContainerComponent(); 10733 parseBackboneProperties(json, res); 10734 if (json.has("identifier")) { 10735 JsonArray array = json.getAsJsonArray("identifier"); 10736 for (int i = 0; i < array.size(); i++) { 10737 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 10738 } 10739 } 10740 ; 10741 if (json.has("description")) 10742 res.setDescriptionElement(parseString(json.get("description").getAsString())); 10743 if (json.has("_description")) 10744 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 10745 if (json.has("type")) 10746 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 10747 if (json.has("capacity")) 10748 res.setCapacity(parseSimpleQuantity(json.getAsJsonObject("capacity"))); 10749 if (json.has("specimenQuantity")) 10750 res.setSpecimenQuantity(parseSimpleQuantity(json.getAsJsonObject("specimenQuantity"))); 10751 Type additive = parseType("additive", json); 10752 if (additive != null) 10753 res.setAdditive(additive); 10754 return res; 10755 } 10756 10757 protected StructureDefinition parseStructureDefinition(JsonObject json) throws IOException, FHIRFormatError { 10758 StructureDefinition res = new StructureDefinition(); 10759 parseDomainResourceProperties(json, res); 10760 if (json.has("url")) 10761 res.setUrlElement(parseUri(json.get("url").getAsString())); 10762 if (json.has("_url")) 10763 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 10764 if (json.has("identifier")) { 10765 JsonArray array = json.getAsJsonArray("identifier"); 10766 for (int i = 0; i < array.size(); i++) { 10767 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 10768 } 10769 } 10770 ; 10771 if (json.has("version")) 10772 res.setVersionElement(parseString(json.get("version").getAsString())); 10773 if (json.has("_version")) 10774 parseElementProperties(json.getAsJsonObject("_version"), res.getVersionElement()); 10775 if (json.has("name")) 10776 res.setNameElement(parseString(json.get("name").getAsString())); 10777 if (json.has("_name")) 10778 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 10779 if (json.has("display")) 10780 res.setDisplayElement(parseString(json.get("display").getAsString())); 10781 if (json.has("_display")) 10782 parseElementProperties(json.getAsJsonObject("_display"), res.getDisplayElement()); 10783 if (json.has("status")) 10784 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 10785 Enumerations.ConformanceResourceStatus.NULL, new Enumerations.ConformanceResourceStatusEnumFactory())); 10786 if (json.has("_status")) 10787 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 10788 if (json.has("experimental")) 10789 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 10790 if (json.has("_experimental")) 10791 parseElementProperties(json.getAsJsonObject("_experimental"), res.getExperimentalElement()); 10792 if (json.has("publisher")) 10793 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 10794 if (json.has("_publisher")) 10795 parseElementProperties(json.getAsJsonObject("_publisher"), res.getPublisherElement()); 10796 if (json.has("contact")) { 10797 JsonArray array = json.getAsJsonArray("contact"); 10798 for (int i = 0; i < array.size(); i++) { 10799 res.getContact() 10800 .add(parseStructureDefinitionStructureDefinitionContactComponent(array.get(i).getAsJsonObject(), res)); 10801 } 10802 } 10803 ; 10804 if (json.has("date")) 10805 res.setDateElement(parseDateTime(json.get("date").getAsString())); 10806 if (json.has("_date")) 10807 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 10808 if (json.has("description")) 10809 res.setDescriptionElement(parseString(json.get("description").getAsString())); 10810 if (json.has("_description")) 10811 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 10812 if (json.has("useContext")) { 10813 JsonArray array = json.getAsJsonArray("useContext"); 10814 for (int i = 0; i < array.size(); i++) { 10815 res.getUseContext().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 10816 } 10817 } 10818 ; 10819 if (json.has("requirements")) 10820 res.setRequirementsElement(parseString(json.get("requirements").getAsString())); 10821 if (json.has("_requirements")) 10822 parseElementProperties(json.getAsJsonObject("_requirements"), res.getRequirementsElement()); 10823 if (json.has("copyright")) 10824 res.setCopyrightElement(parseString(json.get("copyright").getAsString())); 10825 if (json.has("_copyright")) 10826 parseElementProperties(json.getAsJsonObject("_copyright"), res.getCopyrightElement()); 10827 if (json.has("code")) { 10828 JsonArray array = json.getAsJsonArray("code"); 10829 for (int i = 0; i < array.size(); i++) { 10830 res.getCode().add(parseCoding(array.get(i).getAsJsonObject())); 10831 } 10832 } 10833 ; 10834 if (json.has("fhirVersion")) 10835 res.setFhirVersionElement(parseId(json.get("fhirVersion").getAsString())); 10836 if (json.has("_fhirVersion")) 10837 parseElementProperties(json.getAsJsonObject("_fhirVersion"), res.getFhirVersionElement()); 10838 if (json.has("mapping")) { 10839 JsonArray array = json.getAsJsonArray("mapping"); 10840 for (int i = 0; i < array.size(); i++) { 10841 res.getMapping() 10842 .add(parseStructureDefinitionStructureDefinitionMappingComponent(array.get(i).getAsJsonObject(), res)); 10843 } 10844 } 10845 ; 10846 if (json.has("kind")) 10847 res.setKindElement( 10848 parseEnumeration(json.get("kind").getAsString(), StructureDefinition.StructureDefinitionKind.NULL, 10849 new StructureDefinition.StructureDefinitionKindEnumFactory())); 10850 if (json.has("_kind")) 10851 parseElementProperties(json.getAsJsonObject("_kind"), res.getKindElement()); 10852 if (json.has("constrainedType")) 10853 res.setConstrainedTypeElement(parseCode(json.get("constrainedType").getAsString())); 10854 if (json.has("_constrainedType")) 10855 parseElementProperties(json.getAsJsonObject("_constrainedType"), res.getConstrainedTypeElement()); 10856 if (json.has("abstract")) 10857 res.setAbstractElement(parseBoolean(json.get("abstract").getAsBoolean())); 10858 if (json.has("_abstract")) 10859 parseElementProperties(json.getAsJsonObject("_abstract"), res.getAbstractElement()); 10860 if (json.has("contextType")) 10861 res.setContextTypeElement(parseEnumeration(json.get("contextType").getAsString(), 10862 StructureDefinition.ExtensionContext.NULL, new StructureDefinition.ExtensionContextEnumFactory())); 10863 if (json.has("_contextType")) 10864 parseElementProperties(json.getAsJsonObject("_contextType"), res.getContextTypeElement()); 10865 if (json.has("context")) { 10866 JsonArray array = json.getAsJsonArray("context"); 10867 for (int i = 0; i < array.size(); i++) { 10868 res.getContext().add(parseString(array.get(i).getAsString())); 10869 } 10870 } 10871 ; 10872 if (json.has("_context")) { 10873 JsonArray array = json.getAsJsonArray("_context"); 10874 for (int i = 0; i < array.size(); i++) { 10875 if (i == res.getContext().size()) 10876 res.getContext().add(parseString(null)); 10877 if (array.get(i) instanceof JsonObject) 10878 parseElementProperties(array.get(i).getAsJsonObject(), res.getContext().get(i)); 10879 } 10880 } 10881 ; 10882 if (json.has("base")) 10883 res.setBaseElement(parseUri(json.get("base").getAsString())); 10884 if (json.has("_base")) 10885 parseElementProperties(json.getAsJsonObject("_base"), res.getBaseElement()); 10886 if (json.has("snapshot")) 10887 res.setSnapshot( 10888 parseStructureDefinitionStructureDefinitionSnapshotComponent(json.getAsJsonObject("snapshot"), res)); 10889 if (json.has("differential")) 10890 res.setDifferential( 10891 parseStructureDefinitionStructureDefinitionDifferentialComponent(json.getAsJsonObject("differential"), res)); 10892 return res; 10893 } 10894 10895 protected StructureDefinition.StructureDefinitionContactComponent parseStructureDefinitionStructureDefinitionContactComponent( 10896 JsonObject json, StructureDefinition owner) throws IOException, FHIRFormatError { 10897 StructureDefinition.StructureDefinitionContactComponent res = new StructureDefinition.StructureDefinitionContactComponent(); 10898 parseBackboneProperties(json, res); 10899 if (json.has("name")) 10900 res.setNameElement(parseString(json.get("name").getAsString())); 10901 if (json.has("_name")) 10902 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 10903 if (json.has("telecom")) { 10904 JsonArray array = json.getAsJsonArray("telecom"); 10905 for (int i = 0; i < array.size(); i++) { 10906 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 10907 } 10908 } 10909 ; 10910 return res; 10911 } 10912 10913 protected StructureDefinition.StructureDefinitionMappingComponent parseStructureDefinitionStructureDefinitionMappingComponent( 10914 JsonObject json, StructureDefinition owner) throws IOException, FHIRFormatError { 10915 StructureDefinition.StructureDefinitionMappingComponent res = new StructureDefinition.StructureDefinitionMappingComponent(); 10916 parseBackboneProperties(json, res); 10917 if (json.has("identity")) 10918 res.setIdentityElement(parseId(json.get("identity").getAsString())); 10919 if (json.has("_identity")) 10920 parseElementProperties(json.getAsJsonObject("_identity"), res.getIdentityElement()); 10921 if (json.has("uri")) 10922 res.setUriElement(parseUri(json.get("uri").getAsString())); 10923 if (json.has("_uri")) 10924 parseElementProperties(json.getAsJsonObject("_uri"), res.getUriElement()); 10925 if (json.has("name")) 10926 res.setNameElement(parseString(json.get("name").getAsString())); 10927 if (json.has("_name")) 10928 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 10929 if (json.has("comments")) 10930 res.setCommentsElement(parseString(json.get("comments").getAsString())); 10931 if (json.has("_comments")) 10932 parseElementProperties(json.getAsJsonObject("_comments"), res.getCommentsElement()); 10933 return res; 10934 } 10935 10936 protected StructureDefinition.StructureDefinitionSnapshotComponent parseStructureDefinitionStructureDefinitionSnapshotComponent( 10937 JsonObject json, StructureDefinition owner) throws IOException, FHIRFormatError { 10938 StructureDefinition.StructureDefinitionSnapshotComponent res = new StructureDefinition.StructureDefinitionSnapshotComponent(); 10939 parseBackboneProperties(json, res); 10940 if (json.has("element")) { 10941 JsonArray array = json.getAsJsonArray("element"); 10942 for (int i = 0; i < array.size(); i++) { 10943 res.getElement().add(parseElementDefinition(array.get(i).getAsJsonObject())); 10944 } 10945 } 10946 ; 10947 return res; 10948 } 10949 10950 protected StructureDefinition.StructureDefinitionDifferentialComponent parseStructureDefinitionStructureDefinitionDifferentialComponent( 10951 JsonObject json, StructureDefinition owner) throws IOException, FHIRFormatError { 10952 StructureDefinition.StructureDefinitionDifferentialComponent res = new StructureDefinition.StructureDefinitionDifferentialComponent(); 10953 parseBackboneProperties(json, res); 10954 if (json.has("element")) { 10955 JsonArray array = json.getAsJsonArray("element"); 10956 for (int i = 0; i < array.size(); i++) { 10957 res.getElement().add(parseElementDefinition(array.get(i).getAsJsonObject())); 10958 } 10959 } 10960 ; 10961 return res; 10962 } 10963 10964 protected Subscription parseSubscription(JsonObject json) throws IOException, FHIRFormatError { 10965 Subscription res = new Subscription(); 10966 parseDomainResourceProperties(json, res); 10967 if (json.has("criteria")) 10968 res.setCriteriaElement(parseString(json.get("criteria").getAsString())); 10969 if (json.has("_criteria")) 10970 parseElementProperties(json.getAsJsonObject("_criteria"), res.getCriteriaElement()); 10971 if (json.has("contact")) { 10972 JsonArray array = json.getAsJsonArray("contact"); 10973 for (int i = 0; i < array.size(); i++) { 10974 res.getContact().add(parseContactPoint(array.get(i).getAsJsonObject())); 10975 } 10976 } 10977 ; 10978 if (json.has("reason")) 10979 res.setReasonElement(parseString(json.get("reason").getAsString())); 10980 if (json.has("_reason")) 10981 parseElementProperties(json.getAsJsonObject("_reason"), res.getReasonElement()); 10982 if (json.has("status")) 10983 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), Subscription.SubscriptionStatus.NULL, 10984 new Subscription.SubscriptionStatusEnumFactory())); 10985 if (json.has("_status")) 10986 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 10987 if (json.has("error")) 10988 res.setErrorElement(parseString(json.get("error").getAsString())); 10989 if (json.has("_error")) 10990 parseElementProperties(json.getAsJsonObject("_error"), res.getErrorElement()); 10991 if (json.has("channel")) 10992 res.setChannel(parseSubscriptionSubscriptionChannelComponent(json.getAsJsonObject("channel"), res)); 10993 if (json.has("end")) 10994 res.setEndElement(parseInstant(json.get("end").getAsString())); 10995 if (json.has("_end")) 10996 parseElementProperties(json.getAsJsonObject("_end"), res.getEndElement()); 10997 if (json.has("tag")) { 10998 JsonArray array = json.getAsJsonArray("tag"); 10999 for (int i = 0; i < array.size(); i++) { 11000 res.getTag().add(parseCoding(array.get(i).getAsJsonObject())); 11001 } 11002 } 11003 ; 11004 return res; 11005 } 11006 11007 protected Subscription.SubscriptionChannelComponent parseSubscriptionSubscriptionChannelComponent(JsonObject json, 11008 Subscription owner) throws IOException, FHIRFormatError { 11009 Subscription.SubscriptionChannelComponent res = new Subscription.SubscriptionChannelComponent(); 11010 parseBackboneProperties(json, res); 11011 if (json.has("type")) 11012 res.setTypeElement(parseEnumeration(json.get("type").getAsString(), Subscription.SubscriptionChannelType.NULL, 11013 new Subscription.SubscriptionChannelTypeEnumFactory())); 11014 if (json.has("_type")) 11015 parseElementProperties(json.getAsJsonObject("_type"), res.getTypeElement()); 11016 if (json.has("endpoint")) 11017 res.setEndpointElement(parseUri(json.get("endpoint").getAsString())); 11018 if (json.has("_endpoint")) 11019 parseElementProperties(json.getAsJsonObject("_endpoint"), res.getEndpointElement()); 11020 if (json.has("payload")) 11021 res.setPayloadElement(parseString(json.get("payload").getAsString())); 11022 if (json.has("_payload")) 11023 parseElementProperties(json.getAsJsonObject("_payload"), res.getPayloadElement()); 11024 if (json.has("header")) 11025 res.setHeaderElement(parseString(json.get("header").getAsString())); 11026 if (json.has("_header")) 11027 parseElementProperties(json.getAsJsonObject("_header"), res.getHeaderElement()); 11028 return res; 11029 } 11030 11031 protected Substance parseSubstance(JsonObject json) throws IOException, FHIRFormatError { 11032 Substance res = new Substance(); 11033 parseDomainResourceProperties(json, res); 11034 if (json.has("identifier")) { 11035 JsonArray array = json.getAsJsonArray("identifier"); 11036 for (int i = 0; i < array.size(); i++) { 11037 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 11038 } 11039 } 11040 ; 11041 if (json.has("category")) { 11042 JsonArray array = json.getAsJsonArray("category"); 11043 for (int i = 0; i < array.size(); i++) { 11044 res.getCategory().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 11045 } 11046 } 11047 ; 11048 if (json.has("code")) 11049 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 11050 if (json.has("description")) 11051 res.setDescriptionElement(parseString(json.get("description").getAsString())); 11052 if (json.has("_description")) 11053 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 11054 if (json.has("instance")) { 11055 JsonArray array = json.getAsJsonArray("instance"); 11056 for (int i = 0; i < array.size(); i++) { 11057 res.getInstance().add(parseSubstanceSubstanceInstanceComponent(array.get(i).getAsJsonObject(), res)); 11058 } 11059 } 11060 ; 11061 if (json.has("ingredient")) { 11062 JsonArray array = json.getAsJsonArray("ingredient"); 11063 for (int i = 0; i < array.size(); i++) { 11064 res.getIngredient().add(parseSubstanceSubstanceIngredientComponent(array.get(i).getAsJsonObject(), res)); 11065 } 11066 } 11067 ; 11068 return res; 11069 } 11070 11071 protected Substance.SubstanceInstanceComponent parseSubstanceSubstanceInstanceComponent(JsonObject json, 11072 Substance owner) throws IOException, FHIRFormatError { 11073 Substance.SubstanceInstanceComponent res = new Substance.SubstanceInstanceComponent(); 11074 parseBackboneProperties(json, res); 11075 if (json.has("identifier")) 11076 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 11077 if (json.has("expiry")) 11078 res.setExpiryElement(parseDateTime(json.get("expiry").getAsString())); 11079 if (json.has("_expiry")) 11080 parseElementProperties(json.getAsJsonObject("_expiry"), res.getExpiryElement()); 11081 if (json.has("quantity")) 11082 res.setQuantity(parseSimpleQuantity(json.getAsJsonObject("quantity"))); 11083 return res; 11084 } 11085 11086 protected Substance.SubstanceIngredientComponent parseSubstanceSubstanceIngredientComponent(JsonObject json, 11087 Substance owner) throws IOException, FHIRFormatError { 11088 Substance.SubstanceIngredientComponent res = new Substance.SubstanceIngredientComponent(); 11089 parseBackboneProperties(json, res); 11090 if (json.has("quantity")) 11091 res.setQuantity(parseRatio(json.getAsJsonObject("quantity"))); 11092 if (json.has("substance")) 11093 res.setSubstance(parseReference(json.getAsJsonObject("substance"))); 11094 return res; 11095 } 11096 11097 protected SupplyDelivery parseSupplyDelivery(JsonObject json) throws IOException, FHIRFormatError { 11098 SupplyDelivery res = new SupplyDelivery(); 11099 parseDomainResourceProperties(json, res); 11100 if (json.has("identifier")) 11101 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 11102 if (json.has("status")) 11103 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), SupplyDelivery.SupplyDeliveryStatus.NULL, 11104 new SupplyDelivery.SupplyDeliveryStatusEnumFactory())); 11105 if (json.has("_status")) 11106 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 11107 if (json.has("patient")) 11108 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 11109 if (json.has("type")) 11110 res.setType(parseCodeableConcept(json.getAsJsonObject("type"))); 11111 if (json.has("quantity")) 11112 res.setQuantity(parseSimpleQuantity(json.getAsJsonObject("quantity"))); 11113 if (json.has("suppliedItem")) 11114 res.setSuppliedItem(parseReference(json.getAsJsonObject("suppliedItem"))); 11115 if (json.has("supplier")) 11116 res.setSupplier(parseReference(json.getAsJsonObject("supplier"))); 11117 if (json.has("whenPrepared")) 11118 res.setWhenPrepared(parsePeriod(json.getAsJsonObject("whenPrepared"))); 11119 if (json.has("time")) 11120 res.setTimeElement(parseDateTime(json.get("time").getAsString())); 11121 if (json.has("_time")) 11122 parseElementProperties(json.getAsJsonObject("_time"), res.getTimeElement()); 11123 if (json.has("destination")) 11124 res.setDestination(parseReference(json.getAsJsonObject("destination"))); 11125 if (json.has("receiver")) { 11126 JsonArray array = json.getAsJsonArray("receiver"); 11127 for (int i = 0; i < array.size(); i++) { 11128 res.getReceiver().add(parseReference(array.get(i).getAsJsonObject())); 11129 } 11130 } 11131 ; 11132 return res; 11133 } 11134 11135 protected SupplyRequest parseSupplyRequest(JsonObject json) throws IOException, FHIRFormatError { 11136 SupplyRequest res = new SupplyRequest(); 11137 parseDomainResourceProperties(json, res); 11138 if (json.has("patient")) 11139 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 11140 if (json.has("source")) 11141 res.setSource(parseReference(json.getAsJsonObject("source"))); 11142 if (json.has("date")) 11143 res.setDateElement(parseDateTime(json.get("date").getAsString())); 11144 if (json.has("_date")) 11145 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 11146 if (json.has("identifier")) 11147 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 11148 if (json.has("status")) 11149 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), SupplyRequest.SupplyRequestStatus.NULL, 11150 new SupplyRequest.SupplyRequestStatusEnumFactory())); 11151 if (json.has("_status")) 11152 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 11153 if (json.has("kind")) 11154 res.setKind(parseCodeableConcept(json.getAsJsonObject("kind"))); 11155 if (json.has("orderedItem")) 11156 res.setOrderedItem(parseReference(json.getAsJsonObject("orderedItem"))); 11157 if (json.has("supplier")) { 11158 JsonArray array = json.getAsJsonArray("supplier"); 11159 for (int i = 0; i < array.size(); i++) { 11160 res.getSupplier().add(parseReference(array.get(i).getAsJsonObject())); 11161 } 11162 } 11163 ; 11164 Type reason = parseType("reason", json); 11165 if (reason != null) 11166 res.setReason(reason); 11167 if (json.has("when")) 11168 res.setWhen(parseSupplyRequestSupplyRequestWhenComponent(json.getAsJsonObject("when"), res)); 11169 return res; 11170 } 11171 11172 protected SupplyRequest.SupplyRequestWhenComponent parseSupplyRequestSupplyRequestWhenComponent(JsonObject json, 11173 SupplyRequest owner) throws IOException, FHIRFormatError { 11174 SupplyRequest.SupplyRequestWhenComponent res = new SupplyRequest.SupplyRequestWhenComponent(); 11175 parseBackboneProperties(json, res); 11176 if (json.has("code")) 11177 res.setCode(parseCodeableConcept(json.getAsJsonObject("code"))); 11178 if (json.has("schedule")) 11179 res.setSchedule(parseTiming(json.getAsJsonObject("schedule"))); 11180 return res; 11181 } 11182 11183 protected TestScript parseTestScript(JsonObject json) throws IOException, FHIRFormatError { 11184 TestScript res = new TestScript(); 11185 parseDomainResourceProperties(json, res); 11186 if (json.has("url")) 11187 res.setUrlElement(parseUri(json.get("url").getAsString())); 11188 if (json.has("_url")) 11189 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 11190 if (json.has("version")) 11191 res.setVersionElement(parseString(json.get("version").getAsString())); 11192 if (json.has("_version")) 11193 parseElementProperties(json.getAsJsonObject("_version"), res.getVersionElement()); 11194 if (json.has("name")) 11195 res.setNameElement(parseString(json.get("name").getAsString())); 11196 if (json.has("_name")) 11197 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 11198 if (json.has("status")) 11199 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 11200 Enumerations.ConformanceResourceStatus.NULL, new Enumerations.ConformanceResourceStatusEnumFactory())); 11201 if (json.has("_status")) 11202 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 11203 if (json.has("identifier")) 11204 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 11205 if (json.has("experimental")) 11206 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 11207 if (json.has("_experimental")) 11208 parseElementProperties(json.getAsJsonObject("_experimental"), res.getExperimentalElement()); 11209 if (json.has("publisher")) 11210 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 11211 if (json.has("_publisher")) 11212 parseElementProperties(json.getAsJsonObject("_publisher"), res.getPublisherElement()); 11213 if (json.has("contact")) { 11214 JsonArray array = json.getAsJsonArray("contact"); 11215 for (int i = 0; i < array.size(); i++) { 11216 res.getContact().add(parseTestScriptTestScriptContactComponent(array.get(i).getAsJsonObject(), res)); 11217 } 11218 } 11219 ; 11220 if (json.has("date")) 11221 res.setDateElement(parseDateTime(json.get("date").getAsString())); 11222 if (json.has("_date")) 11223 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 11224 if (json.has("description")) 11225 res.setDescriptionElement(parseString(json.get("description").getAsString())); 11226 if (json.has("_description")) 11227 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 11228 if (json.has("useContext")) { 11229 JsonArray array = json.getAsJsonArray("useContext"); 11230 for (int i = 0; i < array.size(); i++) { 11231 res.getUseContext().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 11232 } 11233 } 11234 ; 11235 if (json.has("requirements")) 11236 res.setRequirementsElement(parseString(json.get("requirements").getAsString())); 11237 if (json.has("_requirements")) 11238 parseElementProperties(json.getAsJsonObject("_requirements"), res.getRequirementsElement()); 11239 if (json.has("copyright")) 11240 res.setCopyrightElement(parseString(json.get("copyright").getAsString())); 11241 if (json.has("_copyright")) 11242 parseElementProperties(json.getAsJsonObject("_copyright"), res.getCopyrightElement()); 11243 if (json.has("metadata")) 11244 res.setMetadata(parseTestScriptTestScriptMetadataComponent(json.getAsJsonObject("metadata"), res)); 11245 if (json.has("multiserver")) 11246 res.setMultiserverElement(parseBoolean(json.get("multiserver").getAsBoolean())); 11247 if (json.has("_multiserver")) 11248 parseElementProperties(json.getAsJsonObject("_multiserver"), res.getMultiserverElement()); 11249 if (json.has("fixture")) { 11250 JsonArray array = json.getAsJsonArray("fixture"); 11251 for (int i = 0; i < array.size(); i++) { 11252 res.getFixture().add(parseTestScriptTestScriptFixtureComponent(array.get(i).getAsJsonObject(), res)); 11253 } 11254 } 11255 ; 11256 if (json.has("profile")) { 11257 JsonArray array = json.getAsJsonArray("profile"); 11258 for (int i = 0; i < array.size(); i++) { 11259 res.getProfile().add(parseReference(array.get(i).getAsJsonObject())); 11260 } 11261 } 11262 ; 11263 if (json.has("variable")) { 11264 JsonArray array = json.getAsJsonArray("variable"); 11265 for (int i = 0; i < array.size(); i++) { 11266 res.getVariable().add(parseTestScriptTestScriptVariableComponent(array.get(i).getAsJsonObject(), res)); 11267 } 11268 } 11269 ; 11270 if (json.has("setup")) 11271 res.setSetup(parseTestScriptTestScriptSetupComponent(json.getAsJsonObject("setup"), res)); 11272 if (json.has("test")) { 11273 JsonArray array = json.getAsJsonArray("test"); 11274 for (int i = 0; i < array.size(); i++) { 11275 res.getTest().add(parseTestScriptTestScriptTestComponent(array.get(i).getAsJsonObject(), res)); 11276 } 11277 } 11278 ; 11279 if (json.has("teardown")) 11280 res.setTeardown(parseTestScriptTestScriptTeardownComponent(json.getAsJsonObject("teardown"), res)); 11281 return res; 11282 } 11283 11284 protected TestScript.TestScriptContactComponent parseTestScriptTestScriptContactComponent(JsonObject json, 11285 TestScript owner) throws IOException, FHIRFormatError { 11286 TestScript.TestScriptContactComponent res = new TestScript.TestScriptContactComponent(); 11287 parseBackboneProperties(json, res); 11288 if (json.has("name")) 11289 res.setNameElement(parseString(json.get("name").getAsString())); 11290 if (json.has("_name")) 11291 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 11292 if (json.has("telecom")) { 11293 JsonArray array = json.getAsJsonArray("telecom"); 11294 for (int i = 0; i < array.size(); i++) { 11295 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 11296 } 11297 } 11298 ; 11299 return res; 11300 } 11301 11302 protected TestScript.TestScriptMetadataComponent parseTestScriptTestScriptMetadataComponent(JsonObject json, 11303 TestScript owner) throws IOException, FHIRFormatError { 11304 TestScript.TestScriptMetadataComponent res = new TestScript.TestScriptMetadataComponent(); 11305 parseBackboneProperties(json, res); 11306 if (json.has("link")) { 11307 JsonArray array = json.getAsJsonArray("link"); 11308 for (int i = 0; i < array.size(); i++) { 11309 res.getLink().add(parseTestScriptTestScriptMetadataLinkComponent(array.get(i).getAsJsonObject(), owner)); 11310 } 11311 } 11312 ; 11313 if (json.has("capability")) { 11314 JsonArray array = json.getAsJsonArray("capability"); 11315 for (int i = 0; i < array.size(); i++) { 11316 res.getCapability() 11317 .add(parseTestScriptTestScriptMetadataCapabilityComponent(array.get(i).getAsJsonObject(), owner)); 11318 } 11319 } 11320 ; 11321 return res; 11322 } 11323 11324 protected TestScript.TestScriptMetadataLinkComponent parseTestScriptTestScriptMetadataLinkComponent(JsonObject json, 11325 TestScript owner) throws IOException, FHIRFormatError { 11326 TestScript.TestScriptMetadataLinkComponent res = new TestScript.TestScriptMetadataLinkComponent(); 11327 parseBackboneProperties(json, res); 11328 if (json.has("url")) 11329 res.setUrlElement(parseUri(json.get("url").getAsString())); 11330 if (json.has("_url")) 11331 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 11332 if (json.has("description")) 11333 res.setDescriptionElement(parseString(json.get("description").getAsString())); 11334 if (json.has("_description")) 11335 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 11336 return res; 11337 } 11338 11339 protected TestScript.TestScriptMetadataCapabilityComponent parseTestScriptTestScriptMetadataCapabilityComponent( 11340 JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 11341 TestScript.TestScriptMetadataCapabilityComponent res = new TestScript.TestScriptMetadataCapabilityComponent(); 11342 parseBackboneProperties(json, res); 11343 if (json.has("required")) 11344 res.setRequiredElement(parseBoolean(json.get("required").getAsBoolean())); 11345 if (json.has("_required")) 11346 parseElementProperties(json.getAsJsonObject("_required"), res.getRequiredElement()); 11347 if (json.has("validated")) 11348 res.setValidatedElement(parseBoolean(json.get("validated").getAsBoolean())); 11349 if (json.has("_validated")) 11350 parseElementProperties(json.getAsJsonObject("_validated"), res.getValidatedElement()); 11351 if (json.has("description")) 11352 res.setDescriptionElement(parseString(json.get("description").getAsString())); 11353 if (json.has("_description")) 11354 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 11355 if (json.has("destination")) 11356 res.setDestinationElement(parseInteger(json.get("destination").getAsLong())); 11357 if (json.has("_destination")) 11358 parseElementProperties(json.getAsJsonObject("_destination"), res.getDestinationElement()); 11359 if (json.has("link")) { 11360 JsonArray array = json.getAsJsonArray("link"); 11361 for (int i = 0; i < array.size(); i++) { 11362 res.getLink().add(parseUri(array.get(i).getAsString())); 11363 } 11364 } 11365 ; 11366 if (json.has("_link")) { 11367 JsonArray array = json.getAsJsonArray("_link"); 11368 for (int i = 0; i < array.size(); i++) { 11369 if (i == res.getLink().size()) 11370 res.getLink().add(parseUri(null)); 11371 if (array.get(i) instanceof JsonObject) 11372 parseElementProperties(array.get(i).getAsJsonObject(), res.getLink().get(i)); 11373 } 11374 } 11375 ; 11376 if (json.has("conformance")) 11377 res.setConformance(parseReference(json.getAsJsonObject("conformance"))); 11378 return res; 11379 } 11380 11381 protected TestScript.TestScriptFixtureComponent parseTestScriptTestScriptFixtureComponent(JsonObject json, 11382 TestScript owner) throws IOException, FHIRFormatError { 11383 TestScript.TestScriptFixtureComponent res = new TestScript.TestScriptFixtureComponent(); 11384 parseBackboneProperties(json, res); 11385 if (json.has("autocreate")) 11386 res.setAutocreateElement(parseBoolean(json.get("autocreate").getAsBoolean())); 11387 if (json.has("_autocreate")) 11388 parseElementProperties(json.getAsJsonObject("_autocreate"), res.getAutocreateElement()); 11389 if (json.has("autodelete")) 11390 res.setAutodeleteElement(parseBoolean(json.get("autodelete").getAsBoolean())); 11391 if (json.has("_autodelete")) 11392 parseElementProperties(json.getAsJsonObject("_autodelete"), res.getAutodeleteElement()); 11393 if (json.has("resource")) 11394 res.setResource(parseReference(json.getAsJsonObject("resource"))); 11395 return res; 11396 } 11397 11398 protected TestScript.TestScriptVariableComponent parseTestScriptTestScriptVariableComponent(JsonObject json, 11399 TestScript owner) throws IOException, FHIRFormatError { 11400 TestScript.TestScriptVariableComponent res = new TestScript.TestScriptVariableComponent(); 11401 parseBackboneProperties(json, res); 11402 if (json.has("name")) 11403 res.setNameElement(parseString(json.get("name").getAsString())); 11404 if (json.has("_name")) 11405 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 11406 if (json.has("headerField")) 11407 res.setHeaderFieldElement(parseString(json.get("headerField").getAsString())); 11408 if (json.has("_headerField")) 11409 parseElementProperties(json.getAsJsonObject("_headerField"), res.getHeaderFieldElement()); 11410 if (json.has("path")) 11411 res.setPathElement(parseString(json.get("path").getAsString())); 11412 if (json.has("_path")) 11413 parseElementProperties(json.getAsJsonObject("_path"), res.getPathElement()); 11414 if (json.has("sourceId")) 11415 res.setSourceIdElement(parseId(json.get("sourceId").getAsString())); 11416 if (json.has("_sourceId")) 11417 parseElementProperties(json.getAsJsonObject("_sourceId"), res.getSourceIdElement()); 11418 return res; 11419 } 11420 11421 protected TestScript.TestScriptSetupComponent parseTestScriptTestScriptSetupComponent(JsonObject json, 11422 TestScript owner) throws IOException, FHIRFormatError { 11423 TestScript.TestScriptSetupComponent res = new TestScript.TestScriptSetupComponent(); 11424 parseBackboneProperties(json, res); 11425 if (json.has("metadata")) 11426 res.setMetadata(parseTestScriptTestScriptMetadataComponent(json.getAsJsonObject("metadata"), owner)); 11427 if (json.has("action")) { 11428 JsonArray array = json.getAsJsonArray("action"); 11429 for (int i = 0; i < array.size(); i++) { 11430 res.getAction().add(parseTestScriptTestScriptSetupActionComponent(array.get(i).getAsJsonObject(), owner)); 11431 } 11432 } 11433 ; 11434 return res; 11435 } 11436 11437 protected TestScript.TestScriptSetupActionComponent parseTestScriptTestScriptSetupActionComponent(JsonObject json, 11438 TestScript owner) throws IOException, FHIRFormatError { 11439 TestScript.TestScriptSetupActionComponent res = new TestScript.TestScriptSetupActionComponent(); 11440 parseBackboneProperties(json, res); 11441 if (json.has("operation")) 11442 res.setOperation( 11443 parseTestScriptTestScriptSetupActionOperationComponent(json.getAsJsonObject("operation"), owner)); 11444 if (json.has("assert")) 11445 res.setAssert(parseTestScriptTestScriptSetupActionAssertComponent(json.getAsJsonObject("assert"), owner)); 11446 return res; 11447 } 11448 11449 protected TestScript.TestScriptSetupActionOperationComponent parseTestScriptTestScriptSetupActionOperationComponent( 11450 JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 11451 TestScript.TestScriptSetupActionOperationComponent res = new TestScript.TestScriptSetupActionOperationComponent(); 11452 parseBackboneProperties(json, res); 11453 if (json.has("type")) 11454 res.setType(parseCoding(json.getAsJsonObject("type"))); 11455 if (json.has("resource")) 11456 res.setResourceElement(parseCode(json.get("resource").getAsString())); 11457 if (json.has("_resource")) 11458 parseElementProperties(json.getAsJsonObject("_resource"), res.getResourceElement()); 11459 if (json.has("label")) 11460 res.setLabelElement(parseString(json.get("label").getAsString())); 11461 if (json.has("_label")) 11462 parseElementProperties(json.getAsJsonObject("_label"), res.getLabelElement()); 11463 if (json.has("description")) 11464 res.setDescriptionElement(parseString(json.get("description").getAsString())); 11465 if (json.has("_description")) 11466 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 11467 if (json.has("accept")) 11468 res.setAcceptElement(parseEnumeration(json.get("accept").getAsString(), TestScript.ContentType.NULL, 11469 new TestScript.ContentTypeEnumFactory())); 11470 if (json.has("_accept")) 11471 parseElementProperties(json.getAsJsonObject("_accept"), res.getAcceptElement()); 11472 if (json.has("contentType")) 11473 res.setContentTypeElement(parseEnumeration(json.get("contentType").getAsString(), TestScript.ContentType.NULL, 11474 new TestScript.ContentTypeEnumFactory())); 11475 if (json.has("_contentType")) 11476 parseElementProperties(json.getAsJsonObject("_contentType"), res.getContentTypeElement()); 11477 if (json.has("destination")) 11478 res.setDestinationElement(parseInteger(json.get("destination").getAsLong())); 11479 if (json.has("_destination")) 11480 parseElementProperties(json.getAsJsonObject("_destination"), res.getDestinationElement()); 11481 if (json.has("encodeRequestUrl")) 11482 res.setEncodeRequestUrlElement(parseBoolean(json.get("encodeRequestUrl").getAsBoolean())); 11483 if (json.has("_encodeRequestUrl")) 11484 parseElementProperties(json.getAsJsonObject("_encodeRequestUrl"), res.getEncodeRequestUrlElement()); 11485 if (json.has("params")) 11486 res.setParamsElement(parseString(json.get("params").getAsString())); 11487 if (json.has("_params")) 11488 parseElementProperties(json.getAsJsonObject("_params"), res.getParamsElement()); 11489 if (json.has("requestHeader")) { 11490 JsonArray array = json.getAsJsonArray("requestHeader"); 11491 for (int i = 0; i < array.size(); i++) { 11492 res.getRequestHeader().add( 11493 parseTestScriptTestScriptSetupActionOperationRequestHeaderComponent(array.get(i).getAsJsonObject(), owner)); 11494 } 11495 } 11496 ; 11497 if (json.has("responseId")) 11498 res.setResponseIdElement(parseId(json.get("responseId").getAsString())); 11499 if (json.has("_responseId")) 11500 parseElementProperties(json.getAsJsonObject("_responseId"), res.getResponseIdElement()); 11501 if (json.has("sourceId")) 11502 res.setSourceIdElement(parseId(json.get("sourceId").getAsString())); 11503 if (json.has("_sourceId")) 11504 parseElementProperties(json.getAsJsonObject("_sourceId"), res.getSourceIdElement()); 11505 if (json.has("targetId")) 11506 res.setTargetIdElement(parseId(json.get("targetId").getAsString())); 11507 if (json.has("_targetId")) 11508 parseElementProperties(json.getAsJsonObject("_targetId"), res.getTargetIdElement()); 11509 if (json.has("url")) 11510 res.setUrlElement(parseString(json.get("url").getAsString())); 11511 if (json.has("_url")) 11512 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 11513 return res; 11514 } 11515 11516 protected TestScript.TestScriptSetupActionOperationRequestHeaderComponent parseTestScriptTestScriptSetupActionOperationRequestHeaderComponent( 11517 JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 11518 TestScript.TestScriptSetupActionOperationRequestHeaderComponent res = new TestScript.TestScriptSetupActionOperationRequestHeaderComponent(); 11519 parseBackboneProperties(json, res); 11520 if (json.has("field")) 11521 res.setFieldElement(parseString(json.get("field").getAsString())); 11522 if (json.has("_field")) 11523 parseElementProperties(json.getAsJsonObject("_field"), res.getFieldElement()); 11524 if (json.has("value")) 11525 res.setValueElement(parseString(json.get("value").getAsString())); 11526 if (json.has("_value")) 11527 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 11528 return res; 11529 } 11530 11531 protected TestScript.TestScriptSetupActionAssertComponent parseTestScriptTestScriptSetupActionAssertComponent( 11532 JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 11533 TestScript.TestScriptSetupActionAssertComponent res = new TestScript.TestScriptSetupActionAssertComponent(); 11534 parseBackboneProperties(json, res); 11535 if (json.has("label")) 11536 res.setLabelElement(parseString(json.get("label").getAsString())); 11537 if (json.has("_label")) 11538 parseElementProperties(json.getAsJsonObject("_label"), res.getLabelElement()); 11539 if (json.has("description")) 11540 res.setDescriptionElement(parseString(json.get("description").getAsString())); 11541 if (json.has("_description")) 11542 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 11543 if (json.has("direction")) 11544 res.setDirectionElement(parseEnumeration(json.get("direction").getAsString(), 11545 TestScript.AssertionDirectionType.NULL, new TestScript.AssertionDirectionTypeEnumFactory())); 11546 if (json.has("_direction")) 11547 parseElementProperties(json.getAsJsonObject("_direction"), res.getDirectionElement()); 11548 if (json.has("compareToSourceId")) 11549 res.setCompareToSourceIdElement(parseString(json.get("compareToSourceId").getAsString())); 11550 if (json.has("_compareToSourceId")) 11551 parseElementProperties(json.getAsJsonObject("_compareToSourceId"), res.getCompareToSourceIdElement()); 11552 if (json.has("compareToSourcePath")) 11553 res.setCompareToSourcePathElement(parseString(json.get("compareToSourcePath").getAsString())); 11554 if (json.has("_compareToSourcePath")) 11555 parseElementProperties(json.getAsJsonObject("_compareToSourcePath"), res.getCompareToSourcePathElement()); 11556 if (json.has("contentType")) 11557 res.setContentTypeElement(parseEnumeration(json.get("contentType").getAsString(), TestScript.ContentType.NULL, 11558 new TestScript.ContentTypeEnumFactory())); 11559 if (json.has("_contentType")) 11560 parseElementProperties(json.getAsJsonObject("_contentType"), res.getContentTypeElement()); 11561 if (json.has("headerField")) 11562 res.setHeaderFieldElement(parseString(json.get("headerField").getAsString())); 11563 if (json.has("_headerField")) 11564 parseElementProperties(json.getAsJsonObject("_headerField"), res.getHeaderFieldElement()); 11565 if (json.has("minimumId")) 11566 res.setMinimumIdElement(parseString(json.get("minimumId").getAsString())); 11567 if (json.has("_minimumId")) 11568 parseElementProperties(json.getAsJsonObject("_minimumId"), res.getMinimumIdElement()); 11569 if (json.has("navigationLinks")) 11570 res.setNavigationLinksElement(parseBoolean(json.get("navigationLinks").getAsBoolean())); 11571 if (json.has("_navigationLinks")) 11572 parseElementProperties(json.getAsJsonObject("_navigationLinks"), res.getNavigationLinksElement()); 11573 if (json.has("operator")) 11574 res.setOperatorElement(parseEnumeration(json.get("operator").getAsString(), TestScript.AssertionOperatorType.NULL, 11575 new TestScript.AssertionOperatorTypeEnumFactory())); 11576 if (json.has("_operator")) 11577 parseElementProperties(json.getAsJsonObject("_operator"), res.getOperatorElement()); 11578 if (json.has("path")) 11579 res.setPathElement(parseString(json.get("path").getAsString())); 11580 if (json.has("_path")) 11581 parseElementProperties(json.getAsJsonObject("_path"), res.getPathElement()); 11582 if (json.has("resource")) 11583 res.setResourceElement(parseCode(json.get("resource").getAsString())); 11584 if (json.has("_resource")) 11585 parseElementProperties(json.getAsJsonObject("_resource"), res.getResourceElement()); 11586 if (json.has("response")) 11587 res.setResponseElement(parseEnumeration(json.get("response").getAsString(), 11588 TestScript.AssertionResponseTypes.NULL, new TestScript.AssertionResponseTypesEnumFactory())); 11589 if (json.has("_response")) 11590 parseElementProperties(json.getAsJsonObject("_response"), res.getResponseElement()); 11591 if (json.has("responseCode")) 11592 res.setResponseCodeElement(parseString(json.get("responseCode").getAsString())); 11593 if (json.has("_responseCode")) 11594 parseElementProperties(json.getAsJsonObject("_responseCode"), res.getResponseCodeElement()); 11595 if (json.has("sourceId")) 11596 res.setSourceIdElement(parseId(json.get("sourceId").getAsString())); 11597 if (json.has("_sourceId")) 11598 parseElementProperties(json.getAsJsonObject("_sourceId"), res.getSourceIdElement()); 11599 if (json.has("validateProfileId")) 11600 res.setValidateProfileIdElement(parseId(json.get("validateProfileId").getAsString())); 11601 if (json.has("_validateProfileId")) 11602 parseElementProperties(json.getAsJsonObject("_validateProfileId"), res.getValidateProfileIdElement()); 11603 if (json.has("value")) 11604 res.setValueElement(parseString(json.get("value").getAsString())); 11605 if (json.has("_value")) 11606 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 11607 if (json.has("warningOnly")) 11608 res.setWarningOnlyElement(parseBoolean(json.get("warningOnly").getAsBoolean())); 11609 if (json.has("_warningOnly")) 11610 parseElementProperties(json.getAsJsonObject("_warningOnly"), res.getWarningOnlyElement()); 11611 return res; 11612 } 11613 11614 protected TestScript.TestScriptTestComponent parseTestScriptTestScriptTestComponent(JsonObject json, TestScript owner) 11615 throws IOException, FHIRFormatError { 11616 TestScript.TestScriptTestComponent res = new TestScript.TestScriptTestComponent(); 11617 parseBackboneProperties(json, res); 11618 if (json.has("name")) 11619 res.setNameElement(parseString(json.get("name").getAsString())); 11620 if (json.has("_name")) 11621 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 11622 if (json.has("description")) 11623 res.setDescriptionElement(parseString(json.get("description").getAsString())); 11624 if (json.has("_description")) 11625 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 11626 if (json.has("metadata")) 11627 res.setMetadata(parseTestScriptTestScriptMetadataComponent(json.getAsJsonObject("metadata"), owner)); 11628 if (json.has("action")) { 11629 JsonArray array = json.getAsJsonArray("action"); 11630 for (int i = 0; i < array.size(); i++) { 11631 res.getAction().add(parseTestScriptTestScriptTestActionComponent(array.get(i).getAsJsonObject(), owner)); 11632 } 11633 } 11634 ; 11635 return res; 11636 } 11637 11638 protected TestScript.TestScriptTestActionComponent parseTestScriptTestScriptTestActionComponent(JsonObject json, 11639 TestScript owner) throws IOException, FHIRFormatError { 11640 TestScript.TestScriptTestActionComponent res = new TestScript.TestScriptTestActionComponent(); 11641 parseBackboneProperties(json, res); 11642 if (json.has("operation")) 11643 res.setOperation( 11644 parseTestScriptTestScriptSetupActionOperationComponent(json.getAsJsonObject("operation"), owner)); 11645 if (json.has("assert")) 11646 res.setAssert(parseTestScriptTestScriptSetupActionAssertComponent(json.getAsJsonObject("assert"), owner)); 11647 return res; 11648 } 11649 11650 protected TestScript.TestScriptTeardownComponent parseTestScriptTestScriptTeardownComponent(JsonObject json, 11651 TestScript owner) throws IOException, FHIRFormatError { 11652 TestScript.TestScriptTeardownComponent res = new TestScript.TestScriptTeardownComponent(); 11653 parseBackboneProperties(json, res); 11654 if (json.has("action")) { 11655 JsonArray array = json.getAsJsonArray("action"); 11656 for (int i = 0; i < array.size(); i++) { 11657 res.getAction().add(parseTestScriptTestScriptTeardownActionComponent(array.get(i).getAsJsonObject(), owner)); 11658 } 11659 } 11660 ; 11661 return res; 11662 } 11663 11664 protected TestScript.TestScriptTeardownActionComponent parseTestScriptTestScriptTeardownActionComponent( 11665 JsonObject json, TestScript owner) throws IOException, FHIRFormatError { 11666 TestScript.TestScriptTeardownActionComponent res = new TestScript.TestScriptTeardownActionComponent(); 11667 parseBackboneProperties(json, res); 11668 if (json.has("operation")) 11669 res.setOperation( 11670 parseTestScriptTestScriptSetupActionOperationComponent(json.getAsJsonObject("operation"), owner)); 11671 return res; 11672 } 11673 11674 protected ValueSet parseValueSet(JsonObject json) throws IOException, FHIRFormatError { 11675 ValueSet res = new ValueSet(); 11676 parseDomainResourceProperties(json, res); 11677 if (json.has("url")) 11678 res.setUrlElement(parseUri(json.get("url").getAsString())); 11679 if (json.has("_url")) 11680 parseElementProperties(json.getAsJsonObject("_url"), res.getUrlElement()); 11681 if (json.has("identifier")) 11682 res.setIdentifier(parseIdentifier(json.getAsJsonObject("identifier"))); 11683 if (json.has("version")) 11684 res.setVersionElement(parseString(json.get("version").getAsString())); 11685 if (json.has("_version")) 11686 parseElementProperties(json.getAsJsonObject("_version"), res.getVersionElement()); 11687 if (json.has("name")) 11688 res.setNameElement(parseString(json.get("name").getAsString())); 11689 if (json.has("_name")) 11690 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 11691 if (json.has("status")) 11692 res.setStatusElement(parseEnumeration(json.get("status").getAsString(), 11693 Enumerations.ConformanceResourceStatus.NULL, new Enumerations.ConformanceResourceStatusEnumFactory())); 11694 if (json.has("_status")) 11695 parseElementProperties(json.getAsJsonObject("_status"), res.getStatusElement()); 11696 if (json.has("experimental")) 11697 res.setExperimentalElement(parseBoolean(json.get("experimental").getAsBoolean())); 11698 if (json.has("_experimental")) 11699 parseElementProperties(json.getAsJsonObject("_experimental"), res.getExperimentalElement()); 11700 if (json.has("publisher")) 11701 res.setPublisherElement(parseString(json.get("publisher").getAsString())); 11702 if (json.has("_publisher")) 11703 parseElementProperties(json.getAsJsonObject("_publisher"), res.getPublisherElement()); 11704 if (json.has("contact")) { 11705 JsonArray array = json.getAsJsonArray("contact"); 11706 for (int i = 0; i < array.size(); i++) { 11707 res.getContact().add(parseValueSetValueSetContactComponent(array.get(i).getAsJsonObject(), res)); 11708 } 11709 } 11710 ; 11711 if (json.has("date")) 11712 res.setDateElement(parseDateTime(json.get("date").getAsString())); 11713 if (json.has("_date")) 11714 parseElementProperties(json.getAsJsonObject("_date"), res.getDateElement()); 11715 if (json.has("lockedDate")) 11716 res.setLockedDateElement(parseDate(json.get("lockedDate").getAsString())); 11717 if (json.has("_lockedDate")) 11718 parseElementProperties(json.getAsJsonObject("_lockedDate"), res.getLockedDateElement()); 11719 if (json.has("description")) 11720 res.setDescriptionElement(parseString(json.get("description").getAsString())); 11721 if (json.has("_description")) 11722 parseElementProperties(json.getAsJsonObject("_description"), res.getDescriptionElement()); 11723 if (json.has("useContext")) { 11724 JsonArray array = json.getAsJsonArray("useContext"); 11725 for (int i = 0; i < array.size(); i++) { 11726 res.getUseContext().add(parseCodeableConcept(array.get(i).getAsJsonObject())); 11727 } 11728 } 11729 ; 11730 if (json.has("immutable")) 11731 res.setImmutableElement(parseBoolean(json.get("immutable").getAsBoolean())); 11732 if (json.has("_immutable")) 11733 parseElementProperties(json.getAsJsonObject("_immutable"), res.getImmutableElement()); 11734 if (json.has("requirements")) 11735 res.setRequirementsElement(parseString(json.get("requirements").getAsString())); 11736 if (json.has("_requirements")) 11737 parseElementProperties(json.getAsJsonObject("_requirements"), res.getRequirementsElement()); 11738 if (json.has("copyright")) 11739 res.setCopyrightElement(parseString(json.get("copyright").getAsString())); 11740 if (json.has("_copyright")) 11741 parseElementProperties(json.getAsJsonObject("_copyright"), res.getCopyrightElement()); 11742 if (json.has("extensible")) 11743 res.setExtensibleElement(parseBoolean(json.get("extensible").getAsBoolean())); 11744 if (json.has("_extensible")) 11745 parseElementProperties(json.getAsJsonObject("_extensible"), res.getExtensibleElement()); 11746 if (json.has("codeSystem")) 11747 res.setCodeSystem(parseValueSetValueSetCodeSystemComponent(json.getAsJsonObject("codeSystem"), res)); 11748 if (json.has("compose")) 11749 res.setCompose(parseValueSetValueSetComposeComponent(json.getAsJsonObject("compose"), res)); 11750 if (json.has("expansion")) 11751 res.setExpansion(parseValueSetValueSetExpansionComponent(json.getAsJsonObject("expansion"), res)); 11752 return res; 11753 } 11754 11755 protected ValueSet.ValueSetContactComponent parseValueSetValueSetContactComponent(JsonObject json, ValueSet owner) 11756 throws IOException, FHIRFormatError { 11757 ValueSet.ValueSetContactComponent res = new ValueSet.ValueSetContactComponent(); 11758 parseBackboneProperties(json, res); 11759 if (json.has("name")) 11760 res.setNameElement(parseString(json.get("name").getAsString())); 11761 if (json.has("_name")) 11762 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 11763 if (json.has("telecom")) { 11764 JsonArray array = json.getAsJsonArray("telecom"); 11765 for (int i = 0; i < array.size(); i++) { 11766 res.getTelecom().add(parseContactPoint(array.get(i).getAsJsonObject())); 11767 } 11768 } 11769 ; 11770 return res; 11771 } 11772 11773 protected ValueSet.ValueSetCodeSystemComponent parseValueSetValueSetCodeSystemComponent(JsonObject json, 11774 ValueSet owner) throws IOException, FHIRFormatError { 11775 ValueSet.ValueSetCodeSystemComponent res = new ValueSet.ValueSetCodeSystemComponent(); 11776 parseBackboneProperties(json, res); 11777 if (json.has("system")) 11778 res.setSystemElement(parseUri(json.get("system").getAsString())); 11779 if (json.has("_system")) 11780 parseElementProperties(json.getAsJsonObject("_system"), res.getSystemElement()); 11781 if (json.has("version")) 11782 res.setVersionElement(parseString(json.get("version").getAsString())); 11783 if (json.has("_version")) 11784 parseElementProperties(json.getAsJsonObject("_version"), res.getVersionElement()); 11785 if (json.has("caseSensitive")) 11786 res.setCaseSensitiveElement(parseBoolean(json.get("caseSensitive").getAsBoolean())); 11787 if (json.has("_caseSensitive")) 11788 parseElementProperties(json.getAsJsonObject("_caseSensitive"), res.getCaseSensitiveElement()); 11789 if (json.has("concept")) { 11790 JsonArray array = json.getAsJsonArray("concept"); 11791 for (int i = 0; i < array.size(); i++) { 11792 res.getConcept().add(parseValueSetConceptDefinitionComponent(array.get(i).getAsJsonObject(), owner)); 11793 } 11794 } 11795 ; 11796 return res; 11797 } 11798 11799 protected ValueSet.ConceptDefinitionComponent parseValueSetConceptDefinitionComponent(JsonObject json, ValueSet owner) 11800 throws IOException, FHIRFormatError { 11801 ValueSet.ConceptDefinitionComponent res = new ValueSet.ConceptDefinitionComponent(); 11802 parseBackboneProperties(json, res); 11803 if (json.has("code")) 11804 res.setCodeElement(parseCode(json.get("code").getAsString())); 11805 if (json.has("_code")) 11806 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 11807 if (json.has("abstract")) 11808 res.setAbstractElement(parseBoolean(json.get("abstract").getAsBoolean())); 11809 if (json.has("_abstract")) 11810 parseElementProperties(json.getAsJsonObject("_abstract"), res.getAbstractElement()); 11811 if (json.has("display")) 11812 res.setDisplayElement(parseString(json.get("display").getAsString())); 11813 if (json.has("_display")) 11814 parseElementProperties(json.getAsJsonObject("_display"), res.getDisplayElement()); 11815 if (json.has("definition")) 11816 res.setDefinitionElement(parseString(json.get("definition").getAsString())); 11817 if (json.has("_definition")) 11818 parseElementProperties(json.getAsJsonObject("_definition"), res.getDefinitionElement()); 11819 if (json.has("designation")) { 11820 JsonArray array = json.getAsJsonArray("designation"); 11821 for (int i = 0; i < array.size(); i++) { 11822 res.getDesignation() 11823 .add(parseValueSetConceptDefinitionDesignationComponent(array.get(i).getAsJsonObject(), owner)); 11824 } 11825 } 11826 ; 11827 if (json.has("concept")) { 11828 JsonArray array = json.getAsJsonArray("concept"); 11829 for (int i = 0; i < array.size(); i++) { 11830 res.getConcept().add(parseValueSetConceptDefinitionComponent(array.get(i).getAsJsonObject(), owner)); 11831 } 11832 } 11833 ; 11834 return res; 11835 } 11836 11837 protected ValueSet.ConceptDefinitionDesignationComponent parseValueSetConceptDefinitionDesignationComponent( 11838 JsonObject json, ValueSet owner) throws IOException, FHIRFormatError { 11839 ValueSet.ConceptDefinitionDesignationComponent res = new ValueSet.ConceptDefinitionDesignationComponent(); 11840 parseBackboneProperties(json, res); 11841 if (json.has("language")) 11842 res.setLanguageElement(parseCode(json.get("language").getAsString())); 11843 if (json.has("_language")) 11844 parseElementProperties(json.getAsJsonObject("_language"), res.getLanguageElement()); 11845 if (json.has("use")) 11846 res.setUse(parseCoding(json.getAsJsonObject("use"))); 11847 if (json.has("value")) 11848 res.setValueElement(parseString(json.get("value").getAsString())); 11849 if (json.has("_value")) 11850 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 11851 return res; 11852 } 11853 11854 protected ValueSet.ValueSetComposeComponent parseValueSetValueSetComposeComponent(JsonObject json, ValueSet owner) 11855 throws IOException, FHIRFormatError { 11856 ValueSet.ValueSetComposeComponent res = new ValueSet.ValueSetComposeComponent(); 11857 parseBackboneProperties(json, res); 11858 if (json.has("import")) { 11859 JsonArray array = json.getAsJsonArray("import"); 11860 for (int i = 0; i < array.size(); i++) { 11861 res.getImport().add(parseUri(array.get(i).getAsString())); 11862 } 11863 } 11864 ; 11865 if (json.has("_import")) { 11866 JsonArray array = json.getAsJsonArray("_import"); 11867 for (int i = 0; i < array.size(); i++) { 11868 if (i == res.getImport().size()) 11869 res.getImport().add(parseUri(null)); 11870 if (array.get(i) instanceof JsonObject) 11871 parseElementProperties(array.get(i).getAsJsonObject(), res.getImport().get(i)); 11872 } 11873 } 11874 ; 11875 if (json.has("include")) { 11876 JsonArray array = json.getAsJsonArray("include"); 11877 for (int i = 0; i < array.size(); i++) { 11878 if (!array.get(i).isJsonNull()) { 11879 res.getInclude().add(parseValueSetConceptSetComponent(array.get(i).getAsJsonObject(), owner)); 11880 } 11881 } 11882 } 11883 ; 11884 if (json.has("exclude")) { 11885 JsonArray array = json.getAsJsonArray("exclude"); 11886 for (int i = 0; i < array.size(); i++) { 11887 res.getExclude().add(parseValueSetConceptSetComponent(array.get(i).getAsJsonObject(), owner)); 11888 } 11889 } 11890 ; 11891 return res; 11892 } 11893 11894 protected ValueSet.ConceptSetComponent parseValueSetConceptSetComponent(JsonObject json, ValueSet owner) 11895 throws IOException, FHIRFormatError { 11896 ValueSet.ConceptSetComponent res = new ValueSet.ConceptSetComponent(); 11897 parseBackboneProperties(json, res); 11898 if (json.has("system")) 11899 res.setSystemElement(parseUri(json.get("system").getAsString())); 11900 if (json.has("_system")) 11901 parseElementProperties(json.getAsJsonObject("_system"), res.getSystemElement()); 11902 if (json.has("version")) 11903 res.setVersionElement(parseString(json.get("version").getAsString())); 11904 if (json.has("_version")) 11905 parseElementProperties(json.getAsJsonObject("_version"), res.getVersionElement()); 11906 if (json.has("concept")) { 11907 JsonArray array = json.getAsJsonArray("concept"); 11908 for (int i = 0; i < array.size(); i++) { 11909 res.getConcept().add(parseValueSetConceptReferenceComponent(array.get(i).getAsJsonObject(), owner)); 11910 } 11911 } 11912 ; 11913 if (json.has("filter")) { 11914 JsonArray array = json.getAsJsonArray("filter"); 11915 for (int i = 0; i < array.size(); i++) { 11916 res.getFilter().add(parseValueSetConceptSetFilterComponent(array.get(i).getAsJsonObject(), owner)); 11917 } 11918 } 11919 ; 11920 return res; 11921 } 11922 11923 protected ValueSet.ConceptReferenceComponent parseValueSetConceptReferenceComponent(JsonObject json, ValueSet owner) 11924 throws IOException, FHIRFormatError { 11925 ValueSet.ConceptReferenceComponent res = new ValueSet.ConceptReferenceComponent(); 11926 parseBackboneProperties(json, res); 11927 if (json.has("code")) 11928 res.setCodeElement(parseCode(json.get("code").getAsString())); 11929 if (json.has("_code")) 11930 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 11931 if (json.has("display")) 11932 res.setDisplayElement(parseString(json.get("display").getAsString())); 11933 if (json.has("_display")) 11934 parseElementProperties(json.getAsJsonObject("_display"), res.getDisplayElement()); 11935 if (json.has("designation")) { 11936 JsonArray array = json.getAsJsonArray("designation"); 11937 for (int i = 0; i < array.size(); i++) { 11938 res.getDesignation() 11939 .add(parseValueSetConceptDefinitionDesignationComponent(array.get(i).getAsJsonObject(), owner)); 11940 } 11941 } 11942 ; 11943 return res; 11944 } 11945 11946 protected ValueSet.ConceptSetFilterComponent parseValueSetConceptSetFilterComponent(JsonObject json, ValueSet owner) 11947 throws IOException, FHIRFormatError { 11948 ValueSet.ConceptSetFilterComponent res = new ValueSet.ConceptSetFilterComponent(); 11949 parseBackboneProperties(json, res); 11950 if (json.has("property")) 11951 res.setPropertyElement(parseCode(json.get("property").getAsString())); 11952 if (json.has("_property")) 11953 parseElementProperties(json.getAsJsonObject("_property"), res.getPropertyElement()); 11954 if (json.has("op")) 11955 res.setOpElement(parseEnumeration(json.get("op").getAsString(), ValueSet.FilterOperator.NULL, 11956 new ValueSet.FilterOperatorEnumFactory())); 11957 if (json.has("_op")) 11958 parseElementProperties(json.getAsJsonObject("_op"), res.getOpElement()); 11959 if (json.has("value")) 11960 res.setValueElement(parseCode(json.get("value").getAsString())); 11961 if (json.has("_value")) 11962 parseElementProperties(json.getAsJsonObject("_value"), res.getValueElement()); 11963 return res; 11964 } 11965 11966 protected ValueSet.ValueSetExpansionComponent parseValueSetValueSetExpansionComponent(JsonObject json, ValueSet owner) 11967 throws IOException, FHIRFormatError { 11968 ValueSet.ValueSetExpansionComponent res = new ValueSet.ValueSetExpansionComponent(); 11969 parseBackboneProperties(json, res); 11970 if (json.has("identifier")) 11971 res.setIdentifierElement(parseUri(json.get("identifier").getAsString())); 11972 if (json.has("_identifier")) 11973 parseElementProperties(json.getAsJsonObject("_identifier"), res.getIdentifierElement()); 11974 if (json.has("timestamp")) 11975 res.setTimestampElement(parseDateTime(json.get("timestamp").getAsString())); 11976 if (json.has("_timestamp")) 11977 parseElementProperties(json.getAsJsonObject("_timestamp"), res.getTimestampElement()); 11978 if (json.has("total")) 11979 res.setTotalElement(parseInteger(json.get("total").getAsLong())); 11980 if (json.has("_total")) 11981 parseElementProperties(json.getAsJsonObject("_total"), res.getTotalElement()); 11982 if (json.has("offset")) 11983 res.setOffsetElement(parseInteger(json.get("offset").getAsLong())); 11984 if (json.has("_offset")) 11985 parseElementProperties(json.getAsJsonObject("_offset"), res.getOffsetElement()); 11986 if (json.has("parameter")) { 11987 JsonArray array = json.getAsJsonArray("parameter"); 11988 for (int i = 0; i < array.size(); i++) { 11989 res.getParameter().add(parseValueSetValueSetExpansionParameterComponent(array.get(i).getAsJsonObject(), owner)); 11990 } 11991 } 11992 ; 11993 if (json.has("contains")) { 11994 JsonArray array = json.getAsJsonArray("contains"); 11995 for (int i = 0; i < array.size(); i++) { 11996 res.getContains().add(parseValueSetValueSetExpansionContainsComponent(array.get(i).getAsJsonObject(), owner)); 11997 } 11998 } 11999 ; 12000 return res; 12001 } 12002 12003 protected ValueSet.ValueSetExpansionParameterComponent parseValueSetValueSetExpansionParameterComponent( 12004 JsonObject json, ValueSet owner) throws IOException, FHIRFormatError { 12005 ValueSet.ValueSetExpansionParameterComponent res = new ValueSet.ValueSetExpansionParameterComponent(); 12006 parseBackboneProperties(json, res); 12007 if (json.has("name")) 12008 res.setNameElement(parseString(json.get("name").getAsString())); 12009 if (json.has("_name")) 12010 parseElementProperties(json.getAsJsonObject("_name"), res.getNameElement()); 12011 Type value = parseType("value", json); 12012 if (value != null) 12013 res.setValue(value); 12014 return res; 12015 } 12016 12017 protected ValueSet.ValueSetExpansionContainsComponent parseValueSetValueSetExpansionContainsComponent(JsonObject json, 12018 ValueSet owner) throws IOException, FHIRFormatError { 12019 ValueSet.ValueSetExpansionContainsComponent res = new ValueSet.ValueSetExpansionContainsComponent(); 12020 parseBackboneProperties(json, res); 12021 if (json.has("system")) 12022 res.setSystemElement(parseUri(json.get("system").getAsString())); 12023 if (json.has("_system")) 12024 parseElementProperties(json.getAsJsonObject("_system"), res.getSystemElement()); 12025 if (json.has("abstract")) 12026 res.setAbstractElement(parseBoolean(json.get("abstract").getAsBoolean())); 12027 if (json.has("_abstract")) 12028 parseElementProperties(json.getAsJsonObject("_abstract"), res.getAbstractElement()); 12029 if (json.has("version")) 12030 res.setVersionElement(parseString(json.get("version").getAsString())); 12031 if (json.has("_version")) 12032 parseElementProperties(json.getAsJsonObject("_version"), res.getVersionElement()); 12033 if (json.has("code")) 12034 res.setCodeElement(parseCode(json.get("code").getAsString())); 12035 if (json.has("_code")) 12036 parseElementProperties(json.getAsJsonObject("_code"), res.getCodeElement()); 12037 if (json.has("display")) 12038 res.setDisplayElement(parseString(json.get("display").getAsString())); 12039 if (json.has("_display")) 12040 parseElementProperties(json.getAsJsonObject("_display"), res.getDisplayElement()); 12041 if (json.has("contains")) { 12042 JsonArray array = json.getAsJsonArray("contains"); 12043 for (int i = 0; i < array.size(); i++) { 12044 res.getContains().add(parseValueSetValueSetExpansionContainsComponent(array.get(i).getAsJsonObject(), owner)); 12045 } 12046 } 12047 ; 12048 return res; 12049 } 12050 12051 protected VisionPrescription parseVisionPrescription(JsonObject json) throws IOException, FHIRFormatError { 12052 VisionPrescription res = new VisionPrescription(); 12053 parseDomainResourceProperties(json, res); 12054 if (json.has("identifier")) { 12055 JsonArray array = json.getAsJsonArray("identifier"); 12056 for (int i = 0; i < array.size(); i++) { 12057 res.getIdentifier().add(parseIdentifier(array.get(i).getAsJsonObject())); 12058 } 12059 } 12060 ; 12061 if (json.has("dateWritten")) 12062 res.setDateWrittenElement(parseDateTime(json.get("dateWritten").getAsString())); 12063 if (json.has("_dateWritten")) 12064 parseElementProperties(json.getAsJsonObject("_dateWritten"), res.getDateWrittenElement()); 12065 if (json.has("patient")) 12066 res.setPatient(parseReference(json.getAsJsonObject("patient"))); 12067 if (json.has("prescriber")) 12068 res.setPrescriber(parseReference(json.getAsJsonObject("prescriber"))); 12069 if (json.has("encounter")) 12070 res.setEncounter(parseReference(json.getAsJsonObject("encounter"))); 12071 Type reason = parseType("reason", json); 12072 if (reason != null) 12073 res.setReason(reason); 12074 if (json.has("dispense")) { 12075 JsonArray array = json.getAsJsonArray("dispense"); 12076 for (int i = 0; i < array.size(); i++) { 12077 res.getDispense() 12078 .add(parseVisionPrescriptionVisionPrescriptionDispenseComponent(array.get(i).getAsJsonObject(), res)); 12079 } 12080 } 12081 ; 12082 return res; 12083 } 12084 12085 protected VisionPrescription.VisionPrescriptionDispenseComponent parseVisionPrescriptionVisionPrescriptionDispenseComponent( 12086 JsonObject json, VisionPrescription owner) throws IOException, FHIRFormatError { 12087 VisionPrescription.VisionPrescriptionDispenseComponent res = new VisionPrescription.VisionPrescriptionDispenseComponent(); 12088 parseBackboneProperties(json, res); 12089 if (json.has("product")) 12090 res.setProduct(parseCoding(json.getAsJsonObject("product"))); 12091 if (json.has("eye")) 12092 res.setEyeElement(parseEnumeration(json.get("eye").getAsString(), VisionPrescription.VisionEyes.NULL, 12093 new VisionPrescription.VisionEyesEnumFactory())); 12094 if (json.has("_eye")) 12095 parseElementProperties(json.getAsJsonObject("_eye"), res.getEyeElement()); 12096 if (json.has("sphere")) 12097 res.setSphereElement(parseDecimal(json.get("sphere").getAsBigDecimal())); 12098 if (json.has("_sphere")) 12099 parseElementProperties(json.getAsJsonObject("_sphere"), res.getSphereElement()); 12100 if (json.has("cylinder")) 12101 res.setCylinderElement(parseDecimal(json.get("cylinder").getAsBigDecimal())); 12102 if (json.has("_cylinder")) 12103 parseElementProperties(json.getAsJsonObject("_cylinder"), res.getCylinderElement()); 12104 if (json.has("axis")) 12105 res.setAxisElement(parseInteger(json.get("axis").getAsLong())); 12106 if (json.has("_axis")) 12107 parseElementProperties(json.getAsJsonObject("_axis"), res.getAxisElement()); 12108 if (json.has("prism")) 12109 res.setPrismElement(parseDecimal(json.get("prism").getAsBigDecimal())); 12110 if (json.has("_prism")) 12111 parseElementProperties(json.getAsJsonObject("_prism"), res.getPrismElement()); 12112 if (json.has("base")) 12113 res.setBaseElement(parseEnumeration(json.get("base").getAsString(), VisionPrescription.VisionBase.NULL, 12114 new VisionPrescription.VisionBaseEnumFactory())); 12115 if (json.has("_base")) 12116 parseElementProperties(json.getAsJsonObject("_base"), res.getBaseElement()); 12117 if (json.has("add")) 12118 res.setAddElement(parseDecimal(json.get("add").getAsBigDecimal())); 12119 if (json.has("_add")) 12120 parseElementProperties(json.getAsJsonObject("_add"), res.getAddElement()); 12121 if (json.has("power")) 12122 res.setPowerElement(parseDecimal(json.get("power").getAsBigDecimal())); 12123 if (json.has("_power")) 12124 parseElementProperties(json.getAsJsonObject("_power"), res.getPowerElement()); 12125 if (json.has("backCurve")) 12126 res.setBackCurveElement(parseDecimal(json.get("backCurve").getAsBigDecimal())); 12127 if (json.has("_backCurve")) 12128 parseElementProperties(json.getAsJsonObject("_backCurve"), res.getBackCurveElement()); 12129 if (json.has("diameter")) 12130 res.setDiameterElement(parseDecimal(json.get("diameter").getAsBigDecimal())); 12131 if (json.has("_diameter")) 12132 parseElementProperties(json.getAsJsonObject("_diameter"), res.getDiameterElement()); 12133 if (json.has("duration")) 12134 res.setDuration(parseSimpleQuantity(json.getAsJsonObject("duration"))); 12135 if (json.has("color")) 12136 res.setColorElement(parseString(json.get("color").getAsString())); 12137 if (json.has("_color")) 12138 parseElementProperties(json.getAsJsonObject("_color"), res.getColorElement()); 12139 if (json.has("brand")) 12140 res.setBrandElement(parseString(json.get("brand").getAsString())); 12141 if (json.has("_brand")) 12142 parseElementProperties(json.getAsJsonObject("_brand"), res.getBrandElement()); 12143 if (json.has("notes")) 12144 res.setNotesElement(parseString(json.get("notes").getAsString())); 12145 if (json.has("_notes")) 12146 parseElementProperties(json.getAsJsonObject("_notes"), res.getNotesElement()); 12147 return res; 12148 } 12149 12150 @Override 12151 protected Resource parseResource(JsonObject json) throws IOException, FHIRFormatError { 12152 if (!json.has("resourceType")) { 12153 throw new FHIRFormatError("Unable to find resource type - maybe not a FHIR resource?"); 12154 } 12155 String t = json.get("resourceType").getAsString(); 12156 if (Utilities.noString(t)) 12157 throw new FHIRFormatError("Unable to find resource type - maybe not a FHIR resource?"); 12158 if (t.equals("Parameters")) 12159 return parseParameters(json); 12160 else if (t.equals("Account")) 12161 return parseAccount(json); 12162 else if (t.equals("AllergyIntolerance")) 12163 return parseAllergyIntolerance(json); 12164 else if (t.equals("Appointment")) 12165 return parseAppointment(json); 12166 else if (t.equals("AppointmentResponse")) 12167 return parseAppointmentResponse(json); 12168 else if (t.equals("AuditEvent")) 12169 return parseAuditEvent(json); 12170 else if (t.equals("Basic")) 12171 return parseBasic(json); 12172 else if (t.equals("Binary")) 12173 return parseBinary(json); 12174 else if (t.equals("BodySite")) 12175 return parseBodySite(json); 12176 else if (t.equals("Bundle")) 12177 return parseBundle(json); 12178 else if (t.equals("CarePlan")) 12179 return parseCarePlan(json); 12180 else if (t.equals("Claim")) 12181 return parseClaim(json); 12182 else if (t.equals("ClaimResponse")) 12183 return parseClaimResponse(json); 12184 else if (t.equals("ClinicalImpression")) 12185 return parseClinicalImpression(json); 12186 else if (t.equals("Communication")) 12187 return parseCommunication(json); 12188 else if (t.equals("CommunicationRequest")) 12189 return parseCommunicationRequest(json); 12190 else if (t.equals("Composition")) 12191 return parseComposition(json); 12192 else if (t.equals("ConceptMap")) 12193 return parseConceptMap(json); 12194 else if (t.equals("Condition")) 12195 return parseCondition(json); 12196 else if (t.equals("Conformance")) 12197 return parseConformance(json); 12198 else if (t.equals("Contract")) 12199 return parseContract(json); 12200 else if (t.equals("Coverage")) 12201 return parseCoverage(json); 12202 else if (t.equals("DataElement")) 12203 return parseDataElement(json); 12204 else if (t.equals("DetectedIssue")) 12205 return parseDetectedIssue(json); 12206 else if (t.equals("Device")) 12207 return parseDevice(json); 12208 else if (t.equals("DeviceComponent")) 12209 return parseDeviceComponent(json); 12210 else if (t.equals("DeviceMetric")) 12211 return parseDeviceMetric(json); 12212 else if (t.equals("DeviceUseRequest")) 12213 return parseDeviceUseRequest(json); 12214 else if (t.equals("DeviceUseStatement")) 12215 return parseDeviceUseStatement(json); 12216 else if (t.equals("DiagnosticOrder")) 12217 return parseDiagnosticOrder(json); 12218 else if (t.equals("DiagnosticReport")) 12219 return parseDiagnosticReport(json); 12220 else if (t.equals("DocumentManifest")) 12221 return parseDocumentManifest(json); 12222 else if (t.equals("DocumentReference")) 12223 return parseDocumentReference(json); 12224 else if (t.equals("EligibilityRequest")) 12225 return parseEligibilityRequest(json); 12226 else if (t.equals("EligibilityResponse")) 12227 return parseEligibilityResponse(json); 12228 else if (t.equals("Encounter")) 12229 return parseEncounter(json); 12230 else if (t.equals("EnrollmentRequest")) 12231 return parseEnrollmentRequest(json); 12232 else if (t.equals("EnrollmentResponse")) 12233 return parseEnrollmentResponse(json); 12234 else if (t.equals("EpisodeOfCare")) 12235 return parseEpisodeOfCare(json); 12236 else if (t.equals("ExplanationOfBenefit")) 12237 return parseExplanationOfBenefit(json); 12238 else if (t.equals("FamilyMemberHistory")) 12239 return parseFamilyMemberHistory(json); 12240 else if (t.equals("Flag")) 12241 return parseFlag(json); 12242 else if (t.equals("Goal")) 12243 return parseGoal(json); 12244 else if (t.equals("Group")) 12245 return parseGroup(json); 12246 else if (t.equals("HealthcareService")) 12247 return parseHealthcareService(json); 12248 else if (t.equals("ImagingObjectSelection")) 12249 return parseImagingObjectSelection(json); 12250 else if (t.equals("ImagingStudy")) 12251 return parseImagingStudy(json); 12252 else if (t.equals("Immunization")) 12253 return parseImmunization(json); 12254 else if (t.equals("ImmunizationRecommendation")) 12255 return parseImmunizationRecommendation(json); 12256 else if (t.equals("ImplementationGuide")) 12257 return parseImplementationGuide(json); 12258 else if (t.equals("List")) 12259 return parseList_(json); 12260 else if (t.equals("Location")) 12261 return parseLocation(json); 12262 else if (t.equals("Media")) 12263 return parseMedia(json); 12264 else if (t.equals("Medication")) 12265 return parseMedication(json); 12266 else if (t.equals("MedicationAdministration")) 12267 return parseMedicationAdministration(json); 12268 else if (t.equals("MedicationDispense")) 12269 return parseMedicationDispense(json); 12270 else if (t.equals("MedicationOrder")) 12271 return parseMedicationOrder(json); 12272 else if (t.equals("MedicationStatement")) 12273 return parseMedicationStatement(json); 12274 else if (t.equals("MessageHeader")) 12275 return parseMessageHeader(json); 12276 else if (t.equals("NamingSystem")) 12277 return parseNamingSystem(json); 12278 else if (t.equals("NutritionOrder")) 12279 return parseNutritionOrder(json); 12280 else if (t.equals("Observation")) 12281 return parseObservation(json); 12282 else if (t.equals("OperationDefinition")) 12283 return parseOperationDefinition(json); 12284 else if (t.equals("OperationOutcome")) 12285 return parseOperationOutcome(json); 12286 else if (t.equals("Order")) 12287 return parseOrder(json); 12288 else if (t.equals("OrderResponse")) 12289 return parseOrderResponse(json); 12290 else if (t.equals("Organization")) 12291 return parseOrganization(json); 12292 else if (t.equals("Patient")) 12293 return parsePatient(json); 12294 else if (t.equals("PaymentNotice")) 12295 return parsePaymentNotice(json); 12296 else if (t.equals("PaymentReconciliation")) 12297 return parsePaymentReconciliation(json); 12298 else if (t.equals("Person")) 12299 return parsePerson(json); 12300 else if (t.equals("Practitioner")) 12301 return parsePractitioner(json); 12302 else if (t.equals("Procedure")) 12303 return parseProcedure(json); 12304 else if (t.equals("ProcedureRequest")) 12305 return parseProcedureRequest(json); 12306 else if (t.equals("ProcessRequest")) 12307 return parseProcessRequest(json); 12308 else if (t.equals("ProcessResponse")) 12309 return parseProcessResponse(json); 12310 else if (t.equals("Provenance")) 12311 return parseProvenance(json); 12312 else if (t.equals("Questionnaire")) 12313 return parseQuestionnaire(json); 12314 else if (t.equals("QuestionnaireResponse")) 12315 return parseQuestionnaireResponse(json); 12316 else if (t.equals("ReferralRequest")) 12317 return parseReferralRequest(json); 12318 else if (t.equals("RelatedPerson")) 12319 return parseRelatedPerson(json); 12320 else if (t.equals("RiskAssessment")) 12321 return parseRiskAssessment(json); 12322 else if (t.equals("Schedule")) 12323 return parseSchedule(json); 12324 else if (t.equals("SearchParameter")) 12325 return parseSearchParameter(json); 12326 else if (t.equals("Slot")) 12327 return parseSlot(json); 12328 else if (t.equals("Specimen")) 12329 return parseSpecimen(json); 12330 else if (t.equals("StructureDefinition")) 12331 return parseStructureDefinition(json); 12332 else if (t.equals("Subscription")) 12333 return parseSubscription(json); 12334 else if (t.equals("Substance")) 12335 return parseSubstance(json); 12336 else if (t.equals("SupplyDelivery")) 12337 return parseSupplyDelivery(json); 12338 else if (t.equals("SupplyRequest")) 12339 return parseSupplyRequest(json); 12340 else if (t.equals("TestScript")) 12341 return parseTestScript(json); 12342 else if (t.equals("ValueSet")) 12343 return parseValueSet(json); 12344 else if (t.equals("VisionPrescription")) 12345 return parseVisionPrescription(json); 12346 else if (t.equals("Binary")) 12347 return parseBinary(json); 12348 throw new FHIRFormatError("Unknown.Unrecognised resource type '" + t + "' (in property 'resourceType')"); 12349 } 12350 12351 protected Type parseType(String prefix, JsonObject json) throws IOException, FHIRFormatError { 12352 if (json.has(prefix + "Identifier")) 12353 return parseIdentifier(json.getAsJsonObject(prefix + "Identifier")); 12354 else if (json.has(prefix + "Coding")) 12355 return parseCoding(json.getAsJsonObject(prefix + "Coding")); 12356 else if (json.has(prefix + "Reference")) 12357 return parseReference(json.getAsJsonObject(prefix + "Reference")); 12358 else if (json.has(prefix + "Signature")) 12359 return parseSignature(json.getAsJsonObject(prefix + "Signature")); 12360 else if (json.has(prefix + "SampledData")) 12361 return parseSampledData(json.getAsJsonObject(prefix + "SampledData")); 12362 else if (json.has(prefix + "Quantity")) 12363 return parseQuantity(json.getAsJsonObject(prefix + "Quantity")); 12364 else if (json.has(prefix + "Period")) 12365 return parsePeriod(json.getAsJsonObject(prefix + "Period")); 12366 else if (json.has(prefix + "Attachment")) 12367 return parseAttachment(json.getAsJsonObject(prefix + "Attachment")); 12368 else if (json.has(prefix + "Ratio")) 12369 return parseRatio(json.getAsJsonObject(prefix + "Ratio")); 12370 else if (json.has(prefix + "Range")) 12371 return parseRange(json.getAsJsonObject(prefix + "Range")); 12372 else if (json.has(prefix + "Annotation")) 12373 return parseAnnotation(json.getAsJsonObject(prefix + "Annotation")); 12374 else if (json.has(prefix + "CodeableConcept")) 12375 return parseCodeableConcept(json.getAsJsonObject(prefix + "CodeableConcept")); 12376 else if (json.has(prefix + "Money")) 12377 return parseMoney(json.getAsJsonObject(prefix + "Money")); 12378 else if (json.has(prefix + "SimpleQuantity")) 12379 return parseSimpleQuantity(json.getAsJsonObject(prefix + "SimpleQuantity")); 12380 else if (json.has(prefix + "Duration")) 12381 return parseDuration(json.getAsJsonObject(prefix + "Duration")); 12382 else if (json.has(prefix + "Count")) 12383 return parseCount(json.getAsJsonObject(prefix + "Count")); 12384 else if (json.has(prefix + "Distance")) 12385 return parseDistance(json.getAsJsonObject(prefix + "Distance")); 12386 else if (json.has(prefix + "Age")) 12387 return parseAge(json.getAsJsonObject(prefix + "Age")); 12388 else if (json.has(prefix + "HumanName")) 12389 return parseHumanName(json.getAsJsonObject(prefix + "HumanName")); 12390 else if (json.has(prefix + "ContactPoint")) 12391 return parseContactPoint(json.getAsJsonObject(prefix + "ContactPoint")); 12392 else if (json.has(prefix + "Meta")) 12393 return parseMeta(json.getAsJsonObject(prefix + "Meta")); 12394 else if (json.has(prefix + "Address")) 12395 return parseAddress(json.getAsJsonObject(prefix + "Address")); 12396 else if (json.has(prefix + "Timing")) 12397 return parseTiming(json.getAsJsonObject(prefix + "Timing")); 12398 else if (json.has(prefix + "ElementDefinition")) 12399 return parseElementDefinition(json.getAsJsonObject(prefix + "ElementDefinition")); 12400 else if (json.has(prefix + "Date") || json.has("_" + prefix + "Date")) { 12401 Type t = json.has(prefix + "Date") ? parseDate(json.get(prefix + "Date").getAsString()) : new DateType(); 12402 if (json.has("_" + prefix + "Date")) 12403 parseElementProperties(json.getAsJsonObject("_" + prefix + "Date"), t); 12404 return t; 12405 } else if (json.has(prefix + "DateTime") || json.has("_" + prefix + "DateTime")) { 12406 Type t = json.has(prefix + "DateTime") ? parseDateTime(json.get(prefix + "DateTime").getAsString()) 12407 : new DateTimeType(); 12408 if (json.has("_" + prefix + "DateTime")) 12409 parseElementProperties(json.getAsJsonObject("_" + prefix + "DateTime"), t); 12410 return t; 12411 } else if (json.has(prefix + "Code") || json.has("_" + prefix + "Code")) { 12412 Type t = json.has(prefix + "Code") ? parseCode(json.get(prefix + "Code").getAsString()) : new CodeType(); 12413 if (json.has("_" + prefix + "Code")) 12414 parseElementProperties(json.getAsJsonObject("_" + prefix + "Code"), t); 12415 return t; 12416 } else if (json.has(prefix + "String") || json.has("_" + prefix + "String")) { 12417 Type t = json.has(prefix + "String") ? parseString(json.get(prefix + "String").getAsString()) : new StringType(); 12418 if (json.has("_" + prefix + "String")) 12419 parseElementProperties(json.getAsJsonObject("_" + prefix + "String"), t); 12420 return t; 12421 } else if (json.has(prefix + "Integer") || json.has("_" + prefix + "Integer")) { 12422 Type t = json.has(prefix + "Integer") ? parseInteger(json.get(prefix + "Integer").getAsLong()) 12423 : new IntegerType(); 12424 if (json.has("_" + prefix + "Integer")) 12425 parseElementProperties(json.getAsJsonObject("_" + prefix + "Integer"), t); 12426 return t; 12427 } else if (json.has(prefix + "Oid") || json.has("_" + prefix + "Oid")) { 12428 Type t = json.has(prefix + "Oid") ? parseOid(json.get(prefix + "Oid").getAsString()) : new OidType(); 12429 if (json.has("_" + prefix + "Oid")) 12430 parseElementProperties(json.getAsJsonObject("_" + prefix + "Oid"), t); 12431 return t; 12432 } else if (json.has(prefix + "Uri") || json.has("_" + prefix + "Uri")) { 12433 Type t = json.has(prefix + "Uri") ? parseUri(json.get(prefix + "Uri").getAsString()) : new UriType(); 12434 if (json.has("_" + prefix + "Uri")) 12435 parseElementProperties(json.getAsJsonObject("_" + prefix + "Uri"), t); 12436 return t; 12437 } else if (json.has(prefix + "Uuid") || json.has("_" + prefix + "Uuid")) { 12438 Type t = json.has(prefix + "Uuid") ? parseUuid(json.get(prefix + "Uuid").getAsString()) : new UuidType(); 12439 if (json.has("_" + prefix + "Uuid")) 12440 parseElementProperties(json.getAsJsonObject("_" + prefix + "Uuid"), t); 12441 return t; 12442 } else if (json.has(prefix + "Instant") || json.has("_" + prefix + "Instant")) { 12443 Type t = json.has(prefix + "Instant") ? parseInstant(json.get(prefix + "Instant").getAsString()) 12444 : new InstantType(); 12445 if (json.has("_" + prefix + "Instant")) 12446 parseElementProperties(json.getAsJsonObject("_" + prefix + "Instant"), t); 12447 return t; 12448 } else if (json.has(prefix + "Boolean") || json.has("_" + prefix + "Boolean")) { 12449 Type t = json.has(prefix + "Boolean") ? parseBoolean(json.get(prefix + "Boolean").getAsBoolean()) 12450 : new BooleanType(); 12451 if (json.has("_" + prefix + "Boolean")) 12452 parseElementProperties(json.getAsJsonObject("_" + prefix + "Boolean"), t); 12453 return t; 12454 } else if (json.has(prefix + "Base64Binary") || json.has("_" + prefix + "Base64Binary")) { 12455 Type t = json.has(prefix + "Base64Binary") ? parseBase64Binary(json.get(prefix + "Base64Binary").getAsString()) 12456 : new Base64BinaryType(); 12457 if (json.has("_" + prefix + "Base64Binary")) 12458 parseElementProperties(json.getAsJsonObject("_" + prefix + "Base64Binary"), t); 12459 return t; 12460 } else if (json.has(prefix + "UnsignedInt") || json.has("_" + prefix + "UnsignedInt")) { 12461 Type t = json.has(prefix + "UnsignedInt") ? parseUnsignedInt(json.get(prefix + "UnsignedInt").getAsString()) 12462 : new UnsignedIntType(); 12463 if (json.has("_" + prefix + "UnsignedInt")) 12464 parseElementProperties(json.getAsJsonObject("_" + prefix + "UnsignedInt"), t); 12465 return t; 12466 } else if (json.has(prefix + "Markdown") || json.has("_" + prefix + "Markdown")) { 12467 Type t = json.has(prefix + "Markdown") ? parseMarkdown(json.get(prefix + "Markdown").getAsString()) 12468 : new MarkdownType(); 12469 if (json.has("_" + prefix + "Markdown")) 12470 parseElementProperties(json.getAsJsonObject("_" + prefix + "Markdown"), t); 12471 return t; 12472 } else if (json.has(prefix + "Time") || json.has("_" + prefix + "Time")) { 12473 Type t = json.has(prefix + "Time") ? parseTime(json.get(prefix + "Time").getAsString()) : new TimeType(); 12474 if (json.has("_" + prefix + "Time")) 12475 parseElementProperties(json.getAsJsonObject("_" + prefix + "Time"), t); 12476 return t; 12477 } else if (json.has(prefix + "Id") || json.has("_" + prefix + "Id")) { 12478 Type t = json.has(prefix + "Id") ? parseId(json.get(prefix + "Id").getAsString()) : new IdType(); 12479 if (json.has("_" + prefix + "Id")) 12480 parseElementProperties(json.getAsJsonObject("_" + prefix + "Id"), t); 12481 return t; 12482 } else if (json.has(prefix + "PositiveInt") || json.has("_" + prefix + "PositiveInt")) { 12483 Type t = json.has(prefix + "PositiveInt") ? parsePositiveInt(json.get(prefix + "PositiveInt").getAsString()) 12484 : new PositiveIntType(); 12485 if (json.has("_" + prefix + "PositiveInt")) 12486 parseElementProperties(json.getAsJsonObject("_" + prefix + "PositiveInt"), t); 12487 return t; 12488 } else if (json.has(prefix + "Decimal") || json.has("_" + prefix + "Decimal")) { 12489 Type t = json.has(prefix + "Decimal") ? parseDecimal(json.get(prefix + "Decimal").getAsBigDecimal()) 12490 : new DecimalType(); 12491 if (json.has("_" + prefix + "Decimal")) 12492 parseElementProperties(json.getAsJsonObject("_" + prefix + "Decimal"), t); 12493 return t; 12494 } 12495 return null; 12496 } 12497 12498 protected Type parseType(JsonObject json, String type) throws IOException, FHIRFormatError { 12499 if (type.equals("Identifier")) 12500 return parseIdentifier(json); 12501 else if (type.equals("Coding")) 12502 return parseCoding(json); 12503 else if (type.equals("Reference")) 12504 return parseReference(json); 12505 else if (type.equals("Signature")) 12506 return parseSignature(json); 12507 else if (type.equals("SampledData")) 12508 return parseSampledData(json); 12509 else if (type.equals("Quantity")) 12510 return parseQuantity(json); 12511 else if (type.equals("Period")) 12512 return parsePeriod(json); 12513 else if (type.equals("Attachment")) 12514 return parseAttachment(json); 12515 else if (type.equals("Ratio")) 12516 return parseRatio(json); 12517 else if (type.equals("Range")) 12518 return parseRange(json); 12519 else if (type.equals("Annotation")) 12520 return parseAnnotation(json); 12521 else if (type.equals("CodeableConcept")) 12522 return parseCodeableConcept(json); 12523 else if (type.equals("Money")) 12524 return parseMoney(json); 12525 else if (type.equals("SimpleQuantity")) 12526 return parseSimpleQuantity(json); 12527 else if (type.equals("Duration")) 12528 return parseDuration(json); 12529 else if (type.equals("Count")) 12530 return parseCount(json); 12531 else if (type.equals("Distance")) 12532 return parseDistance(json); 12533 else if (type.equals("Age")) 12534 return parseAge(json); 12535 else if (type.equals("HumanName")) 12536 return parseHumanName(json); 12537 else if (type.equals("ContactPoint")) 12538 return parseContactPoint(json); 12539 else if (type.equals("Meta")) 12540 return parseMeta(json); 12541 else if (type.equals("Address")) 12542 return parseAddress(json); 12543 else if (type.equals("Timing")) 12544 return parseTiming(json); 12545 else if (type.equals("ElementDefinition")) 12546 return parseElementDefinition(json); 12547 throw new FHIRFormatError("Unknown Type " + type); 12548 } 12549 12550 protected boolean hasTypeName(JsonObject json, String prefix) { 12551 if (json.has(prefix + "Identifier")) 12552 return true; 12553 if (json.has(prefix + "Coding")) 12554 return true; 12555 if (json.has(prefix + "Reference")) 12556 return true; 12557 if (json.has(prefix + "Signature")) 12558 return true; 12559 if (json.has(prefix + "SampledData")) 12560 return true; 12561 if (json.has(prefix + "Quantity")) 12562 return true; 12563 if (json.has(prefix + "Period")) 12564 return true; 12565 if (json.has(prefix + "Attachment")) 12566 return true; 12567 if (json.has(prefix + "Ratio")) 12568 return true; 12569 if (json.has(prefix + "Range")) 12570 return true; 12571 if (json.has(prefix + "Annotation")) 12572 return true; 12573 if (json.has(prefix + "CodeableConcept")) 12574 return true; 12575 if (json.has(prefix + "Money")) 12576 return true; 12577 if (json.has(prefix + "SimpleQuantity")) 12578 return true; 12579 if (json.has(prefix + "Duration")) 12580 return true; 12581 if (json.has(prefix + "Count")) 12582 return true; 12583 if (json.has(prefix + "Distance")) 12584 return true; 12585 if (json.has(prefix + "Age")) 12586 return true; 12587 if (json.has(prefix + "HumanName")) 12588 return true; 12589 if (json.has(prefix + "ContactPoint")) 12590 return true; 12591 if (json.has(prefix + "Meta")) 12592 return true; 12593 if (json.has(prefix + "Address")) 12594 return true; 12595 if (json.has(prefix + "Timing")) 12596 return true; 12597 if (json.has(prefix + "ElementDefinition")) 12598 return true; 12599 if (json.has(prefix + "Parameters")) 12600 return true; 12601 if (json.has(prefix + "Account")) 12602 return true; 12603 if (json.has(prefix + "AllergyIntolerance")) 12604 return true; 12605 if (json.has(prefix + "Appointment")) 12606 return true; 12607 if (json.has(prefix + "AppointmentResponse")) 12608 return true; 12609 if (json.has(prefix + "AuditEvent")) 12610 return true; 12611 if (json.has(prefix + "Basic")) 12612 return true; 12613 if (json.has(prefix + "Binary")) 12614 return true; 12615 if (json.has(prefix + "BodySite")) 12616 return true; 12617 if (json.has(prefix + "Bundle")) 12618 return true; 12619 if (json.has(prefix + "CarePlan")) 12620 return true; 12621 if (json.has(prefix + "Claim")) 12622 return true; 12623 if (json.has(prefix + "ClaimResponse")) 12624 return true; 12625 if (json.has(prefix + "ClinicalImpression")) 12626 return true; 12627 if (json.has(prefix + "Communication")) 12628 return true; 12629 if (json.has(prefix + "CommunicationRequest")) 12630 return true; 12631 if (json.has(prefix + "Composition")) 12632 return true; 12633 if (json.has(prefix + "ConceptMap")) 12634 return true; 12635 if (json.has(prefix + "Condition")) 12636 return true; 12637 if (json.has(prefix + "Conformance")) 12638 return true; 12639 if (json.has(prefix + "Contract")) 12640 return true; 12641 if (json.has(prefix + "Coverage")) 12642 return true; 12643 if (json.has(prefix + "DataElement")) 12644 return true; 12645 if (json.has(prefix + "DetectedIssue")) 12646 return true; 12647 if (json.has(prefix + "Device")) 12648 return true; 12649 if (json.has(prefix + "DeviceComponent")) 12650 return true; 12651 if (json.has(prefix + "DeviceMetric")) 12652 return true; 12653 if (json.has(prefix + "DeviceUseRequest")) 12654 return true; 12655 if (json.has(prefix + "DeviceUseStatement")) 12656 return true; 12657 if (json.has(prefix + "DiagnosticOrder")) 12658 return true; 12659 if (json.has(prefix + "DiagnosticReport")) 12660 return true; 12661 if (json.has(prefix + "DocumentManifest")) 12662 return true; 12663 if (json.has(prefix + "DocumentReference")) 12664 return true; 12665 if (json.has(prefix + "EligibilityRequest")) 12666 return true; 12667 if (json.has(prefix + "EligibilityResponse")) 12668 return true; 12669 if (json.has(prefix + "Encounter")) 12670 return true; 12671 if (json.has(prefix + "EnrollmentRequest")) 12672 return true; 12673 if (json.has(prefix + "EnrollmentResponse")) 12674 return true; 12675 if (json.has(prefix + "EpisodeOfCare")) 12676 return true; 12677 if (json.has(prefix + "ExplanationOfBenefit")) 12678 return true; 12679 if (json.has(prefix + "FamilyMemberHistory")) 12680 return true; 12681 if (json.has(prefix + "Flag")) 12682 return true; 12683 if (json.has(prefix + "Goal")) 12684 return true; 12685 if (json.has(prefix + "Group")) 12686 return true; 12687 if (json.has(prefix + "HealthcareService")) 12688 return true; 12689 if (json.has(prefix + "ImagingObjectSelection")) 12690 return true; 12691 if (json.has(prefix + "ImagingStudy")) 12692 return true; 12693 if (json.has(prefix + "Immunization")) 12694 return true; 12695 if (json.has(prefix + "ImmunizationRecommendation")) 12696 return true; 12697 if (json.has(prefix + "ImplementationGuide")) 12698 return true; 12699 if (json.has(prefix + "List")) 12700 return true; 12701 if (json.has(prefix + "Location")) 12702 return true; 12703 if (json.has(prefix + "Media")) 12704 return true; 12705 if (json.has(prefix + "Medication")) 12706 return true; 12707 if (json.has(prefix + "MedicationAdministration")) 12708 return true; 12709 if (json.has(prefix + "MedicationDispense")) 12710 return true; 12711 if (json.has(prefix + "MedicationOrder")) 12712 return true; 12713 if (json.has(prefix + "MedicationStatement")) 12714 return true; 12715 if (json.has(prefix + "MessageHeader")) 12716 return true; 12717 if (json.has(prefix + "NamingSystem")) 12718 return true; 12719 if (json.has(prefix + "NutritionOrder")) 12720 return true; 12721 if (json.has(prefix + "Observation")) 12722 return true; 12723 if (json.has(prefix + "OperationDefinition")) 12724 return true; 12725 if (json.has(prefix + "OperationOutcome")) 12726 return true; 12727 if (json.has(prefix + "Order")) 12728 return true; 12729 if (json.has(prefix + "OrderResponse")) 12730 return true; 12731 if (json.has(prefix + "Organization")) 12732 return true; 12733 if (json.has(prefix + "Patient")) 12734 return true; 12735 if (json.has(prefix + "PaymentNotice")) 12736 return true; 12737 if (json.has(prefix + "PaymentReconciliation")) 12738 return true; 12739 if (json.has(prefix + "Person")) 12740 return true; 12741 if (json.has(prefix + "Practitioner")) 12742 return true; 12743 if (json.has(prefix + "Procedure")) 12744 return true; 12745 if (json.has(prefix + "ProcedureRequest")) 12746 return true; 12747 if (json.has(prefix + "ProcessRequest")) 12748 return true; 12749 if (json.has(prefix + "ProcessResponse")) 12750 return true; 12751 if (json.has(prefix + "Provenance")) 12752 return true; 12753 if (json.has(prefix + "Questionnaire")) 12754 return true; 12755 if (json.has(prefix + "QuestionnaireResponse")) 12756 return true; 12757 if (json.has(prefix + "ReferralRequest")) 12758 return true; 12759 if (json.has(prefix + "RelatedPerson")) 12760 return true; 12761 if (json.has(prefix + "RiskAssessment")) 12762 return true; 12763 if (json.has(prefix + "Schedule")) 12764 return true; 12765 if (json.has(prefix + "SearchParameter")) 12766 return true; 12767 if (json.has(prefix + "Slot")) 12768 return true; 12769 if (json.has(prefix + "Specimen")) 12770 return true; 12771 if (json.has(prefix + "StructureDefinition")) 12772 return true; 12773 if (json.has(prefix + "Subscription")) 12774 return true; 12775 if (json.has(prefix + "Substance")) 12776 return true; 12777 if (json.has(prefix + "SupplyDelivery")) 12778 return true; 12779 if (json.has(prefix + "SupplyRequest")) 12780 return true; 12781 if (json.has(prefix + "TestScript")) 12782 return true; 12783 if (json.has(prefix + "ValueSet")) 12784 return true; 12785 if (json.has(prefix + "VisionPrescription")) 12786 return true; 12787 if (json.has(prefix + "Date") || json.has("_" + prefix + "Date")) 12788 return true; 12789 if (json.has(prefix + "DateTime") || json.has("_" + prefix + "DateTime")) 12790 return true; 12791 if (json.has(prefix + "Code") || json.has("_" + prefix + "Code")) 12792 return true; 12793 if (json.has(prefix + "String") || json.has("_" + prefix + "String")) 12794 return true; 12795 if (json.has(prefix + "Integer") || json.has("_" + prefix + "Integer")) 12796 return true; 12797 if (json.has(prefix + "Oid") || json.has("_" + prefix + "Oid")) 12798 return true; 12799 if (json.has(prefix + "Uri") || json.has("_" + prefix + "Uri")) 12800 return true; 12801 if (json.has(prefix + "Uuid") || json.has("_" + prefix + "Uuid")) 12802 return true; 12803 if (json.has(prefix + "Instant") || json.has("_" + prefix + "Instant")) 12804 return true; 12805 if (json.has(prefix + "Boolean") || json.has("_" + prefix + "Boolean")) 12806 return true; 12807 if (json.has(prefix + "Base64Binary") || json.has("_" + prefix + "Base64Binary")) 12808 return true; 12809 if (json.has(prefix + "UnsignedInt") || json.has("_" + prefix + "UnsignedInt")) 12810 return true; 12811 if (json.has(prefix + "Markdown") || json.has("_" + prefix + "Markdown")) 12812 return true; 12813 if (json.has(prefix + "Time") || json.has("_" + prefix + "Time")) 12814 return true; 12815 if (json.has(prefix + "Id") || json.has("_" + prefix + "Id")) 12816 return true; 12817 if (json.has(prefix + "PositiveInt") || json.has("_" + prefix + "PositiveInt")) 12818 return true; 12819 if (json.has(prefix + "Decimal") || json.has("_" + prefix + "Decimal")) 12820 return true; 12821 return false; 12822 } 12823 12824 protected void composeElement(Element element) throws IOException { 12825 if (element.hasId()) 12826 prop("id", element.getId()); 12827 if (makeComments(element)) { 12828 openArray("fhir_comments"); 12829 for (String s : element.getFormatCommentsPre()) 12830 prop(null, s); 12831 for (String s : element.getFormatCommentsPost()) 12832 prop(null, s); 12833 closeArray(); 12834 } 12835 if (element.hasExtension()) { 12836 openArray("extension"); 12837 for (Extension e : element.getExtension()) 12838 composeExtension(null, e); 12839 closeArray(); 12840 } 12841 } 12842 12843 protected void composeBackbone(BackboneElement element) throws IOException { 12844 composeElement(element); 12845 if (element.hasModifierExtension()) { 12846 openArray("modifierExtension"); 12847 for (Extension e : element.getModifierExtension()) 12848 composeExtension(null, e); 12849 closeArray(); 12850 } 12851 } 12852 12853 protected <E extends Enum<E>> void composeEnumerationCore(String name, Enumeration<E> value, EnumFactory e, 12854 boolean inArray) throws IOException { 12855 if (value != null && value.getValue() != null) { 12856 prop(name, e.toCode(value.getValue())); 12857 } else if (inArray) 12858 writeNull(name); 12859 } 12860 12861 protected <E extends Enum<E>> void composeEnumerationExtras(String name, Enumeration<E> value, EnumFactory e, 12862 boolean inArray) throws IOException { 12863 if (value != null 12864 && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 12865 open(inArray ? null : "_" + name); 12866 composeElement(value); 12867 close(); 12868 } else if (inArray) 12869 writeNull(name); 12870 } 12871 12872 protected void composeDateCore(String name, DateType value, boolean inArray) throws IOException { 12873 if (value != null && value.hasValue()) { 12874 prop(name, value.asStringValue()); 12875 } else if (inArray) 12876 writeNull(name); 12877 } 12878 12879 protected void composeDateExtras(String name, DateType value, boolean inArray) throws IOException { 12880 if (value != null 12881 && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 12882 open(inArray ? null : "_" + name); 12883 composeElement(value); 12884 close(); 12885 } else if (inArray) 12886 writeNull(name); 12887 } 12888 12889 protected void composeDateTimeCore(String name, DateTimeType value, boolean inArray) throws IOException { 12890 if (value != null && value.hasValue()) { 12891 prop(name, value.asStringValue()); 12892 } else if (inArray) 12893 writeNull(name); 12894 } 12895 12896 protected void composeDateTimeExtras(String name, DateTimeType value, boolean inArray) throws IOException { 12897 if (value != null 12898 && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 12899 open(inArray ? null : "_" + name); 12900 composeElement(value); 12901 close(); 12902 } else if (inArray) 12903 writeNull(name); 12904 } 12905 12906 protected void composeCodeCore(String name, CodeType value, boolean inArray) throws IOException { 12907 if (value != null && value.hasValue()) { 12908 prop(name, toString(value.getValue())); 12909 } else if (inArray) 12910 writeNull(name); 12911 } 12912 12913 protected void composeCodeExtras(String name, CodeType value, boolean inArray) throws IOException { 12914 if (value != null 12915 && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 12916 open(inArray ? null : "_" + name); 12917 composeElement(value); 12918 close(); 12919 } else if (inArray) 12920 writeNull(name); 12921 } 12922 12923 protected void composeStringCore(String name, StringType value, boolean inArray) throws IOException { 12924 if (value != null && value.hasValue()) { 12925 prop(name, toString(value.getValue())); 12926 } else if (inArray) 12927 writeNull(name); 12928 } 12929 12930 protected void composeStringExtras(String name, StringType value, boolean inArray) throws IOException { 12931 if (value != null 12932 && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 12933 open(inArray ? null : "_" + name); 12934 composeElement(value); 12935 close(); 12936 } else if (inArray) 12937 writeNull(name); 12938 } 12939 12940 protected void composeIntegerCore(String name, IntegerType value, boolean inArray) throws IOException { 12941 if (value != null && value.hasValue()) { 12942 prop(name, Integer.valueOf(value.getValue())); 12943 } else if (inArray) 12944 writeNull(name); 12945 } 12946 12947 protected void composeIntegerExtras(String name, IntegerType value, boolean inArray) throws IOException { 12948 if (value != null 12949 && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 12950 open(inArray ? null : "_" + name); 12951 composeElement(value); 12952 close(); 12953 } else if (inArray) 12954 writeNull(name); 12955 } 12956 12957 protected void composeOidCore(String name, OidType value, boolean inArray) throws IOException { 12958 if (value != null && value.hasValue()) { 12959 prop(name, toString(value.getValue())); 12960 } else if (inArray) 12961 writeNull(name); 12962 } 12963 12964 protected void composeOidExtras(String name, OidType value, boolean inArray) throws IOException { 12965 if (value != null 12966 && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 12967 open(inArray ? null : "_" + name); 12968 composeElement(value); 12969 close(); 12970 } else if (inArray) 12971 writeNull(name); 12972 } 12973 12974 protected void composeUriCore(String name, UriType value, boolean inArray) throws IOException { 12975 if (value != null && value.hasValue()) { 12976 prop(name, toString(value.getValue())); 12977 } else if (inArray) 12978 writeNull(name); 12979 } 12980 12981 protected void composeUriExtras(String name, UriType value, boolean inArray) throws IOException { 12982 if (value != null 12983 && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 12984 open(inArray ? null : "_" + name); 12985 composeElement(value); 12986 close(); 12987 } else if (inArray) 12988 writeNull(name); 12989 } 12990 12991 protected void composeUuidCore(String name, UuidType value, boolean inArray) throws IOException { 12992 if (value != null && value.hasValue()) { 12993 prop(name, toString(value.getValue())); 12994 } else if (inArray) 12995 writeNull(name); 12996 } 12997 12998 protected void composeUuidExtras(String name, UuidType value, boolean inArray) throws IOException { 12999 if (value != null 13000 && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 13001 open(inArray ? null : "_" + name); 13002 composeElement(value); 13003 close(); 13004 } else if (inArray) 13005 writeNull(name); 13006 } 13007 13008 protected void composeInstantCore(String name, InstantType value, boolean inArray) throws IOException { 13009 if (value != null && value.hasValue()) { 13010 prop(name, value.asStringValue()); 13011 } else if (inArray) 13012 writeNull(name); 13013 } 13014 13015 protected void composeInstantExtras(String name, InstantType value, boolean inArray) throws IOException { 13016 if (value != null 13017 && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 13018 open(inArray ? null : "_" + name); 13019 composeElement(value); 13020 close(); 13021 } else if (inArray) 13022 writeNull(name); 13023 } 13024 13025 protected void composeBooleanCore(String name, BooleanType value, boolean inArray) throws IOException { 13026 if (value != null && value.hasValue()) { 13027 prop(name, value.getValue()); 13028 } else if (inArray) 13029 writeNull(name); 13030 } 13031 13032 protected void composeBooleanExtras(String name, BooleanType value, boolean inArray) throws IOException { 13033 if (value != null 13034 && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 13035 open(inArray ? null : "_" + name); 13036 composeElement(value); 13037 close(); 13038 } else if (inArray) 13039 writeNull(name); 13040 } 13041 13042 protected void composeBase64BinaryCore(String name, Base64BinaryType value, boolean inArray) throws IOException { 13043 if (value != null && value.hasValue()) { 13044 prop(name, toString(value.getValue())); 13045 } else if (inArray) 13046 writeNull(name); 13047 } 13048 13049 protected void composeBase64BinaryExtras(String name, Base64BinaryType value, boolean inArray) throws IOException { 13050 if (value != null 13051 && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 13052 open(inArray ? null : "_" + name); 13053 composeElement(value); 13054 close(); 13055 } else if (inArray) 13056 writeNull(name); 13057 } 13058 13059 protected void composeUnsignedIntCore(String name, UnsignedIntType value, boolean inArray) throws IOException { 13060 if (value != null && value.hasValue()) { 13061 prop(name, Integer.valueOf(value.getValue())); 13062 } else if (inArray) 13063 writeNull(name); 13064 } 13065 13066 protected void composeUnsignedIntExtras(String name, UnsignedIntType value, boolean inArray) throws IOException { 13067 if (value != null 13068 && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 13069 open(inArray ? null : "_" + name); 13070 composeElement(value); 13071 close(); 13072 } else if (inArray) 13073 writeNull(name); 13074 } 13075 13076 protected void composeMarkdownCore(String name, MarkdownType value, boolean inArray) throws IOException { 13077 if (value != null && value.hasValue()) { 13078 prop(name, toString(value.getValue())); 13079 } else if (inArray) 13080 writeNull(name); 13081 } 13082 13083 protected void composeMarkdownExtras(String name, MarkdownType value, boolean inArray) throws IOException { 13084 if (value != null 13085 && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 13086 open(inArray ? null : "_" + name); 13087 composeElement(value); 13088 close(); 13089 } else if (inArray) 13090 writeNull(name); 13091 } 13092 13093 protected void composeTimeCore(String name, TimeType value, boolean inArray) throws IOException { 13094 if (value != null && value.hasValue()) { 13095 prop(name, value.asStringValue()); 13096 } else if (inArray) 13097 writeNull(name); 13098 } 13099 13100 protected void composeTimeExtras(String name, TimeType value, boolean inArray) throws IOException { 13101 if (value != null 13102 && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 13103 open(inArray ? null : "_" + name); 13104 composeElement(value); 13105 close(); 13106 } else if (inArray) 13107 writeNull(name); 13108 } 13109 13110 protected void composeIdCore(String name, IdType value, boolean inArray) throws IOException { 13111 if (value != null && value.hasValue()) { 13112 prop(name, toString(value.getValue())); 13113 } else if (inArray) 13114 writeNull(name); 13115 } 13116 13117 protected void composeIdExtras(String name, IdType value, boolean inArray) throws IOException { 13118 if (value != null 13119 && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 13120 open(inArray ? null : "_" + name); 13121 composeElement(value); 13122 close(); 13123 } else if (inArray) 13124 writeNull(name); 13125 } 13126 13127 protected void composePositiveIntCore(String name, PositiveIntType value, boolean inArray) throws IOException { 13128 if (value != null && value.hasValue()) { 13129 prop(name, Integer.valueOf(value.getValue())); 13130 } else if (inArray) 13131 writeNull(name); 13132 } 13133 13134 protected void composePositiveIntExtras(String name, PositiveIntType value, boolean inArray) throws IOException { 13135 if (value != null 13136 && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 13137 open(inArray ? null : "_" + name); 13138 composeElement(value); 13139 close(); 13140 } else if (inArray) 13141 writeNull(name); 13142 } 13143 13144 protected void composeDecimalCore(String name, DecimalType value, boolean inArray) throws IOException { 13145 if (value != null && value.hasValue()) { 13146 prop(name, value.getValue()); 13147 } else if (inArray) 13148 writeNull(name); 13149 } 13150 13151 protected void composeDecimalExtras(String name, DecimalType value, boolean inArray) throws IOException { 13152 if (value != null 13153 && (!Utilities.noString(value.getId()) || ExtensionHelper.hasExtensions(value) || makeComments(value))) { 13154 open(inArray ? null : "_" + name); 13155 composeElement(value); 13156 close(); 13157 } else if (inArray) 13158 writeNull(name); 13159 } 13160 13161 protected void composeExtension(String name, Extension element) throws IOException { 13162 if (element != null) { 13163 open(name); 13164 composeExtensionInner(element); 13165 close(); 13166 } 13167 } 13168 13169 protected void composeExtensionInner(Extension element) throws IOException { 13170 composeElement(element); 13171 if (element.hasUrlElement()) { 13172 composeUriCore("url", element.getUrlElement(), false); 13173 composeUriExtras("url", element.getUrlElement(), false); 13174 } 13175 if (element.hasValue()) { 13176 composeType("value", element.getValue()); 13177 } 13178 } 13179 13180 protected void composeNarrative(String name, Narrative element) throws IOException { 13181 if (element != null) { 13182 open(name); 13183 composeNarrativeInner(element); 13184 close(); 13185 } 13186 } 13187 13188 protected void composeNarrativeInner(Narrative element) throws IOException { 13189 composeElement(element); 13190 if (element.hasStatusElement()) { 13191 composeEnumerationCore("status", element.getStatusElement(), new Narrative.NarrativeStatusEnumFactory(), false); 13192 composeEnumerationExtras("status", element.getStatusElement(), new Narrative.NarrativeStatusEnumFactory(), false); 13193 } 13194 if (element.hasDiv()) { 13195 composeXhtml("div", element.getDiv()); 13196 } 13197 } 13198 13199 protected void composeIdentifier(String name, Identifier element) throws IOException { 13200 if (element != null) { 13201 open(name); 13202 composeIdentifierInner(element); 13203 close(); 13204 } 13205 } 13206 13207 protected void composeIdentifierInner(Identifier element) throws IOException { 13208 composeElement(element); 13209 if (element.hasUseElement()) { 13210 composeEnumerationCore("use", element.getUseElement(), new Identifier.IdentifierUseEnumFactory(), false); 13211 composeEnumerationExtras("use", element.getUseElement(), new Identifier.IdentifierUseEnumFactory(), false); 13212 } 13213 if (element.hasType()) { 13214 composeCodeableConcept("type", element.getType()); 13215 } 13216 if (element.hasSystemElement()) { 13217 composeUriCore("system", element.getSystemElement(), false); 13218 composeUriExtras("system", element.getSystemElement(), false); 13219 } 13220 if (element.hasValueElement()) { 13221 composeStringCore("value", element.getValueElement(), false); 13222 composeStringExtras("value", element.getValueElement(), false); 13223 } 13224 if (element.hasPeriod()) { 13225 composePeriod("period", element.getPeriod()); 13226 } 13227 if (element.hasAssigner()) { 13228 composeReference("assigner", element.getAssigner()); 13229 } 13230 } 13231 13232 protected void composeCoding(String name, Coding element) throws IOException { 13233 if (element != null) { 13234 open(name); 13235 composeCodingInner(element); 13236 close(); 13237 } 13238 } 13239 13240 protected void composeCodingInner(Coding element) throws IOException { 13241 composeElement(element); 13242 if (element.hasSystemElement()) { 13243 composeUriCore("system", element.getSystemElement(), false); 13244 composeUriExtras("system", element.getSystemElement(), false); 13245 } 13246 if (element.hasVersionElement()) { 13247 composeStringCore("version", element.getVersionElement(), false); 13248 composeStringExtras("version", element.getVersionElement(), false); 13249 } 13250 if (element.hasCodeElement()) { 13251 composeCodeCore("code", element.getCodeElement(), false); 13252 composeCodeExtras("code", element.getCodeElement(), false); 13253 } 13254 if (element.hasDisplayElement()) { 13255 composeStringCore("display", element.getDisplayElement(), false); 13256 composeStringExtras("display", element.getDisplayElement(), false); 13257 } 13258 if (element.hasUserSelectedElement()) { 13259 composeBooleanCore("userSelected", element.getUserSelectedElement(), false); 13260 composeBooleanExtras("userSelected", element.getUserSelectedElement(), false); 13261 } 13262 } 13263 13264 protected void composeReference(String name, Reference element) throws IOException { 13265 if (element != null) { 13266 open(name); 13267 composeReferenceInner(element); 13268 close(); 13269 } 13270 } 13271 13272 protected void composeReferenceInner(Reference element) throws IOException { 13273 composeElement(element); 13274 if (element.hasReferenceElement()) { 13275 composeStringCore("reference", element.getReferenceElement(), false); 13276 composeStringExtras("reference", element.getReferenceElement(), false); 13277 } 13278 if (element.hasDisplayElement()) { 13279 composeStringCore("display", element.getDisplayElement(), false); 13280 composeStringExtras("display", element.getDisplayElement(), false); 13281 } 13282 } 13283 13284 protected void composeSignature(String name, Signature element) throws IOException { 13285 if (element != null) { 13286 open(name); 13287 composeSignatureInner(element); 13288 close(); 13289 } 13290 } 13291 13292 protected void composeSignatureInner(Signature element) throws IOException { 13293 composeElement(element); 13294 if (element.hasType()) { 13295 openArray("type"); 13296 for (Coding e : element.getType()) 13297 composeCoding(null, e); 13298 closeArray(); 13299 } 13300 ; 13301 if (element.hasWhenElement()) { 13302 composeInstantCore("when", element.getWhenElement(), false); 13303 composeInstantExtras("when", element.getWhenElement(), false); 13304 } 13305 if (element.hasWho()) { 13306 composeType("who", element.getWho()); 13307 } 13308 if (element.hasContentTypeElement()) { 13309 composeCodeCore("contentType", element.getContentTypeElement(), false); 13310 composeCodeExtras("contentType", element.getContentTypeElement(), false); 13311 } 13312 if (element.hasBlobElement()) { 13313 composeBase64BinaryCore("blob", element.getBlobElement(), false); 13314 composeBase64BinaryExtras("blob", element.getBlobElement(), false); 13315 } 13316 } 13317 13318 protected void composeSampledData(String name, SampledData element) throws IOException { 13319 if (element != null) { 13320 open(name); 13321 composeSampledDataInner(element); 13322 close(); 13323 } 13324 } 13325 13326 protected void composeSampledDataInner(SampledData element) throws IOException { 13327 composeElement(element); 13328 if (element.hasOrigin()) { 13329 composeSimpleQuantity("origin", element.getOrigin()); 13330 } 13331 if (element.hasPeriodElement()) { 13332 composeDecimalCore("period", element.getPeriodElement(), false); 13333 composeDecimalExtras("period", element.getPeriodElement(), false); 13334 } 13335 if (element.hasFactorElement()) { 13336 composeDecimalCore("factor", element.getFactorElement(), false); 13337 composeDecimalExtras("factor", element.getFactorElement(), false); 13338 } 13339 if (element.hasLowerLimitElement()) { 13340 composeDecimalCore("lowerLimit", element.getLowerLimitElement(), false); 13341 composeDecimalExtras("lowerLimit", element.getLowerLimitElement(), false); 13342 } 13343 if (element.hasUpperLimitElement()) { 13344 composeDecimalCore("upperLimit", element.getUpperLimitElement(), false); 13345 composeDecimalExtras("upperLimit", element.getUpperLimitElement(), false); 13346 } 13347 if (element.hasDimensionsElement()) { 13348 composePositiveIntCore("dimensions", element.getDimensionsElement(), false); 13349 composePositiveIntExtras("dimensions", element.getDimensionsElement(), false); 13350 } 13351 if (element.hasDataElement()) { 13352 composeStringCore("data", element.getDataElement(), false); 13353 composeStringExtras("data", element.getDataElement(), false); 13354 } 13355 } 13356 13357 protected void composeQuantity(String name, Quantity element) throws IOException { 13358 if (element != null) { 13359 open(name); 13360 composeQuantityInner(element); 13361 close(); 13362 } 13363 } 13364 13365 protected void composeQuantityInner(Quantity element) throws IOException { 13366 composeElement(element); 13367 if (element.hasValueElement()) { 13368 composeDecimalCore("value", element.getValueElement(), false); 13369 composeDecimalExtras("value", element.getValueElement(), false); 13370 } 13371 if (element.hasComparatorElement()) { 13372 composeEnumerationCore("comparator", element.getComparatorElement(), new Quantity.QuantityComparatorEnumFactory(), 13373 false); 13374 composeEnumerationExtras("comparator", element.getComparatorElement(), 13375 new Quantity.QuantityComparatorEnumFactory(), false); 13376 } 13377 if (element.hasUnitElement()) { 13378 composeStringCore("unit", element.getUnitElement(), false); 13379 composeStringExtras("unit", element.getUnitElement(), false); 13380 } 13381 if (element.hasSystemElement()) { 13382 composeUriCore("system", element.getSystemElement(), false); 13383 composeUriExtras("system", element.getSystemElement(), false); 13384 } 13385 if (element.hasCodeElement()) { 13386 composeCodeCore("code", element.getCodeElement(), false); 13387 composeCodeExtras("code", element.getCodeElement(), false); 13388 } 13389 } 13390 13391 protected void composePeriod(String name, Period element) throws IOException { 13392 if (element != null) { 13393 open(name); 13394 composePeriodInner(element); 13395 close(); 13396 } 13397 } 13398 13399 protected void composePeriodInner(Period element) throws IOException { 13400 composeElement(element); 13401 if (element.hasStartElement()) { 13402 composeDateTimeCore("start", element.getStartElement(), false); 13403 composeDateTimeExtras("start", element.getStartElement(), false); 13404 } 13405 if (element.hasEndElement()) { 13406 composeDateTimeCore("end", element.getEndElement(), false); 13407 composeDateTimeExtras("end", element.getEndElement(), false); 13408 } 13409 } 13410 13411 protected void composeAttachment(String name, Attachment element) throws IOException { 13412 if (element != null) { 13413 open(name); 13414 composeAttachmentInner(element); 13415 close(); 13416 } 13417 } 13418 13419 protected void composeAttachmentInner(Attachment element) throws IOException { 13420 composeElement(element); 13421 if (element.hasContentTypeElement()) { 13422 composeCodeCore("contentType", element.getContentTypeElement(), false); 13423 composeCodeExtras("contentType", element.getContentTypeElement(), false); 13424 } 13425 if (element.hasLanguageElement()) { 13426 composeCodeCore("language", element.getLanguageElement(), false); 13427 composeCodeExtras("language", element.getLanguageElement(), false); 13428 } 13429 if (element.hasDataElement()) { 13430 composeBase64BinaryCore("data", element.getDataElement(), false); 13431 composeBase64BinaryExtras("data", element.getDataElement(), false); 13432 } 13433 if (element.hasUrlElement()) { 13434 composeUriCore("url", element.getUrlElement(), false); 13435 composeUriExtras("url", element.getUrlElement(), false); 13436 } 13437 if (element.hasSizeElement()) { 13438 composeUnsignedIntCore("size", element.getSizeElement(), false); 13439 composeUnsignedIntExtras("size", element.getSizeElement(), false); 13440 } 13441 if (element.hasHashElement()) { 13442 composeBase64BinaryCore("hash", element.getHashElement(), false); 13443 composeBase64BinaryExtras("hash", element.getHashElement(), false); 13444 } 13445 if (element.hasTitleElement()) { 13446 composeStringCore("title", element.getTitleElement(), false); 13447 composeStringExtras("title", element.getTitleElement(), false); 13448 } 13449 if (element.hasCreationElement()) { 13450 composeDateTimeCore("creation", element.getCreationElement(), false); 13451 composeDateTimeExtras("creation", element.getCreationElement(), false); 13452 } 13453 } 13454 13455 protected void composeRatio(String name, Ratio element) throws IOException { 13456 if (element != null) { 13457 open(name); 13458 composeRatioInner(element); 13459 close(); 13460 } 13461 } 13462 13463 protected void composeRatioInner(Ratio element) throws IOException { 13464 composeElement(element); 13465 if (element.hasNumerator()) { 13466 composeQuantity("numerator", element.getNumerator()); 13467 } 13468 if (element.hasDenominator()) { 13469 composeQuantity("denominator", element.getDenominator()); 13470 } 13471 } 13472 13473 protected void composeRange(String name, Range element) throws IOException { 13474 if (element != null) { 13475 open(name); 13476 composeRangeInner(element); 13477 close(); 13478 } 13479 } 13480 13481 protected void composeRangeInner(Range element) throws IOException { 13482 composeElement(element); 13483 if (element.hasLow()) { 13484 composeSimpleQuantity("low", element.getLow()); 13485 } 13486 if (element.hasHigh()) { 13487 composeSimpleQuantity("high", element.getHigh()); 13488 } 13489 } 13490 13491 protected void composeAnnotation(String name, Annotation element) throws IOException { 13492 if (element != null) { 13493 open(name); 13494 composeAnnotationInner(element); 13495 close(); 13496 } 13497 } 13498 13499 protected void composeAnnotationInner(Annotation element) throws IOException { 13500 composeElement(element); 13501 if (element.hasAuthor()) { 13502 composeType("author", element.getAuthor()); 13503 } 13504 if (element.hasTimeElement()) { 13505 composeDateTimeCore("time", element.getTimeElement(), false); 13506 composeDateTimeExtras("time", element.getTimeElement(), false); 13507 } 13508 if (element.hasTextElement()) { 13509 composeStringCore("text", element.getTextElement(), false); 13510 composeStringExtras("text", element.getTextElement(), false); 13511 } 13512 } 13513 13514 protected void composeCodeableConcept(String name, CodeableConcept element) throws IOException { 13515 if (element != null) { 13516 open(name); 13517 composeCodeableConceptInner(element); 13518 close(); 13519 } 13520 } 13521 13522 protected void composeCodeableConceptInner(CodeableConcept element) throws IOException { 13523 composeElement(element); 13524 if (element.hasCoding()) { 13525 openArray("coding"); 13526 for (Coding e : element.getCoding()) 13527 composeCoding(null, e); 13528 closeArray(); 13529 } 13530 ; 13531 if (element.hasTextElement()) { 13532 composeStringCore("text", element.getTextElement(), false); 13533 composeStringExtras("text", element.getTextElement(), false); 13534 } 13535 } 13536 13537 protected void composeMoney(String name, Money element) throws IOException { 13538 if (element != null) { 13539 open(name); 13540 composeMoneyInner(element); 13541 close(); 13542 } 13543 } 13544 13545 protected void composeMoneyInner(Money element) throws IOException { 13546 composeElement(element); 13547 if (element.hasValueElement()) { 13548 composeDecimalCore("value", element.getValueElement(), false); 13549 composeDecimalExtras("value", element.getValueElement(), false); 13550 } 13551 if (element.hasComparatorElement()) { 13552 composeEnumerationCore("comparator", element.getComparatorElement(), new Money.QuantityComparatorEnumFactory(), 13553 false); 13554 composeEnumerationExtras("comparator", element.getComparatorElement(), new Money.QuantityComparatorEnumFactory(), 13555 false); 13556 } 13557 if (element.hasUnitElement()) { 13558 composeStringCore("unit", element.getUnitElement(), false); 13559 composeStringExtras("unit", element.getUnitElement(), false); 13560 } 13561 if (element.hasSystemElement()) { 13562 composeUriCore("system", element.getSystemElement(), false); 13563 composeUriExtras("system", element.getSystemElement(), false); 13564 } 13565 if (element.hasCodeElement()) { 13566 composeCodeCore("code", element.getCodeElement(), false); 13567 composeCodeExtras("code", element.getCodeElement(), false); 13568 } 13569 } 13570 13571 protected void composeSimpleQuantity(String name, SimpleQuantity element) throws IOException { 13572 if (element != null) { 13573 open(name); 13574 composeSimpleQuantityInner(element); 13575 close(); 13576 } 13577 } 13578 13579 protected void composeSimpleQuantityInner(SimpleQuantity element) throws IOException { 13580 composeElement(element); 13581 if (element.hasValueElement()) { 13582 composeDecimalCore("value", element.getValueElement(), false); 13583 composeDecimalExtras("value", element.getValueElement(), false); 13584 } 13585 if (element.hasComparatorElement()) { 13586 composeEnumerationCore("comparator", element.getComparatorElement(), 13587 new SimpleQuantity.QuantityComparatorEnumFactory(), false); 13588 composeEnumerationExtras("comparator", element.getComparatorElement(), 13589 new SimpleQuantity.QuantityComparatorEnumFactory(), false); 13590 } 13591 if (element.hasUnitElement()) { 13592 composeStringCore("unit", element.getUnitElement(), false); 13593 composeStringExtras("unit", element.getUnitElement(), false); 13594 } 13595 if (element.hasSystemElement()) { 13596 composeUriCore("system", element.getSystemElement(), false); 13597 composeUriExtras("system", element.getSystemElement(), false); 13598 } 13599 if (element.hasCodeElement()) { 13600 composeCodeCore("code", element.getCodeElement(), false); 13601 composeCodeExtras("code", element.getCodeElement(), false); 13602 } 13603 } 13604 13605 protected void composeDuration(String name, Duration element) throws IOException { 13606 if (element != null) { 13607 open(name); 13608 composeDurationInner(element); 13609 close(); 13610 } 13611 } 13612 13613 protected void composeDurationInner(Duration element) throws IOException { 13614 composeElement(element); 13615 if (element.hasValueElement()) { 13616 composeDecimalCore("value", element.getValueElement(), false); 13617 composeDecimalExtras("value", element.getValueElement(), false); 13618 } 13619 if (element.hasComparatorElement()) { 13620 composeEnumerationCore("comparator", element.getComparatorElement(), new Duration.QuantityComparatorEnumFactory(), 13621 false); 13622 composeEnumerationExtras("comparator", element.getComparatorElement(), 13623 new Duration.QuantityComparatorEnumFactory(), false); 13624 } 13625 if (element.hasUnitElement()) { 13626 composeStringCore("unit", element.getUnitElement(), false); 13627 composeStringExtras("unit", element.getUnitElement(), false); 13628 } 13629 if (element.hasSystemElement()) { 13630 composeUriCore("system", element.getSystemElement(), false); 13631 composeUriExtras("system", element.getSystemElement(), false); 13632 } 13633 if (element.hasCodeElement()) { 13634 composeCodeCore("code", element.getCodeElement(), false); 13635 composeCodeExtras("code", element.getCodeElement(), false); 13636 } 13637 } 13638 13639 protected void composeCount(String name, Count element) throws IOException { 13640 if (element != null) { 13641 open(name); 13642 composeCountInner(element); 13643 close(); 13644 } 13645 } 13646 13647 protected void composeCountInner(Count element) throws IOException { 13648 composeElement(element); 13649 if (element.hasValueElement()) { 13650 composeDecimalCore("value", element.getValueElement(), false); 13651 composeDecimalExtras("value", element.getValueElement(), false); 13652 } 13653 if (element.hasComparatorElement()) { 13654 composeEnumerationCore("comparator", element.getComparatorElement(), new Count.QuantityComparatorEnumFactory(), 13655 false); 13656 composeEnumerationExtras("comparator", element.getComparatorElement(), new Count.QuantityComparatorEnumFactory(), 13657 false); 13658 } 13659 if (element.hasUnitElement()) { 13660 composeStringCore("unit", element.getUnitElement(), false); 13661 composeStringExtras("unit", element.getUnitElement(), false); 13662 } 13663 if (element.hasSystemElement()) { 13664 composeUriCore("system", element.getSystemElement(), false); 13665 composeUriExtras("system", element.getSystemElement(), false); 13666 } 13667 if (element.hasCodeElement()) { 13668 composeCodeCore("code", element.getCodeElement(), false); 13669 composeCodeExtras("code", element.getCodeElement(), false); 13670 } 13671 } 13672 13673 protected void composeDistance(String name, Distance element) throws IOException { 13674 if (element != null) { 13675 open(name); 13676 composeDistanceInner(element); 13677 close(); 13678 } 13679 } 13680 13681 protected void composeDistanceInner(Distance element) throws IOException { 13682 composeElement(element); 13683 if (element.hasValueElement()) { 13684 composeDecimalCore("value", element.getValueElement(), false); 13685 composeDecimalExtras("value", element.getValueElement(), false); 13686 } 13687 if (element.hasComparatorElement()) { 13688 composeEnumerationCore("comparator", element.getComparatorElement(), new Distance.QuantityComparatorEnumFactory(), 13689 false); 13690 composeEnumerationExtras("comparator", element.getComparatorElement(), 13691 new Distance.QuantityComparatorEnumFactory(), false); 13692 } 13693 if (element.hasUnitElement()) { 13694 composeStringCore("unit", element.getUnitElement(), false); 13695 composeStringExtras("unit", element.getUnitElement(), false); 13696 } 13697 if (element.hasSystemElement()) { 13698 composeUriCore("system", element.getSystemElement(), false); 13699 composeUriExtras("system", element.getSystemElement(), false); 13700 } 13701 if (element.hasCodeElement()) { 13702 composeCodeCore("code", element.getCodeElement(), false); 13703 composeCodeExtras("code", element.getCodeElement(), false); 13704 } 13705 } 13706 13707 protected void composeAge(String name, Age element) throws IOException { 13708 if (element != null) { 13709 open(name); 13710 composeAgeInner(element); 13711 close(); 13712 } 13713 } 13714 13715 protected void composeAgeInner(Age element) throws IOException { 13716 composeElement(element); 13717 if (element.hasValueElement()) { 13718 composeDecimalCore("value", element.getValueElement(), false); 13719 composeDecimalExtras("value", element.getValueElement(), false); 13720 } 13721 if (element.hasComparatorElement()) { 13722 composeEnumerationCore("comparator", element.getComparatorElement(), new Age.QuantityComparatorEnumFactory(), 13723 false); 13724 composeEnumerationExtras("comparator", element.getComparatorElement(), new Age.QuantityComparatorEnumFactory(), 13725 false); 13726 } 13727 if (element.hasUnitElement()) { 13728 composeStringCore("unit", element.getUnitElement(), false); 13729 composeStringExtras("unit", element.getUnitElement(), false); 13730 } 13731 if (element.hasSystemElement()) { 13732 composeUriCore("system", element.getSystemElement(), false); 13733 composeUriExtras("system", element.getSystemElement(), false); 13734 } 13735 if (element.hasCodeElement()) { 13736 composeCodeCore("code", element.getCodeElement(), false); 13737 composeCodeExtras("code", element.getCodeElement(), false); 13738 } 13739 } 13740 13741 protected void composeHumanName(String name, HumanName element) throws IOException { 13742 if (element != null) { 13743 open(name); 13744 composeHumanNameInner(element); 13745 close(); 13746 } 13747 } 13748 13749 protected void composeHumanNameInner(HumanName element) throws IOException { 13750 composeElement(element); 13751 if (element.hasUseElement()) { 13752 composeEnumerationCore("use", element.getUseElement(), new HumanName.NameUseEnumFactory(), false); 13753 composeEnumerationExtras("use", element.getUseElement(), new HumanName.NameUseEnumFactory(), false); 13754 } 13755 if (element.hasTextElement()) { 13756 composeStringCore("text", element.getTextElement(), false); 13757 composeStringExtras("text", element.getTextElement(), false); 13758 } 13759 if (element.hasFamily()) { 13760 openArray("family"); 13761 for (StringType e : element.getFamily()) 13762 composeStringCore(null, e, true); 13763 closeArray(); 13764 if (anyHasExtras(element.getFamily())) { 13765 openArray("_family"); 13766 for (StringType e : element.getFamily()) 13767 composeStringExtras(null, e, true); 13768 closeArray(); 13769 } 13770 } 13771 ; 13772 if (element.hasGiven()) { 13773 openArray("given"); 13774 for (StringType e : element.getGiven()) 13775 composeStringCore(null, e, true); 13776 closeArray(); 13777 if (anyHasExtras(element.getGiven())) { 13778 openArray("_given"); 13779 for (StringType e : element.getGiven()) 13780 composeStringExtras(null, e, true); 13781 closeArray(); 13782 } 13783 } 13784 ; 13785 if (element.hasPrefix()) { 13786 openArray("prefix"); 13787 for (StringType e : element.getPrefix()) 13788 composeStringCore(null, e, true); 13789 closeArray(); 13790 if (anyHasExtras(element.getPrefix())) { 13791 openArray("_prefix"); 13792 for (StringType e : element.getPrefix()) 13793 composeStringExtras(null, e, true); 13794 closeArray(); 13795 } 13796 } 13797 ; 13798 if (element.hasSuffix()) { 13799 openArray("suffix"); 13800 for (StringType e : element.getSuffix()) 13801 composeStringCore(null, e, true); 13802 closeArray(); 13803 if (anyHasExtras(element.getSuffix())) { 13804 openArray("_suffix"); 13805 for (StringType e : element.getSuffix()) 13806 composeStringExtras(null, e, true); 13807 closeArray(); 13808 } 13809 } 13810 ; 13811 if (element.hasPeriod()) { 13812 composePeriod("period", element.getPeriod()); 13813 } 13814 } 13815 13816 protected void composeContactPoint(String name, ContactPoint element) throws IOException { 13817 if (element != null) { 13818 open(name); 13819 composeContactPointInner(element); 13820 close(); 13821 } 13822 } 13823 13824 protected void composeContactPointInner(ContactPoint element) throws IOException { 13825 composeElement(element); 13826 if (element.hasSystemElement()) { 13827 composeEnumerationCore("system", element.getSystemElement(), new ContactPoint.ContactPointSystemEnumFactory(), 13828 false); 13829 composeEnumerationExtras("system", element.getSystemElement(), new ContactPoint.ContactPointSystemEnumFactory(), 13830 false); 13831 } 13832 if (element.hasValueElement()) { 13833 composeStringCore("value", element.getValueElement(), false); 13834 composeStringExtras("value", element.getValueElement(), false); 13835 } 13836 if (element.hasUseElement()) { 13837 composeEnumerationCore("use", element.getUseElement(), new ContactPoint.ContactPointUseEnumFactory(), false); 13838 composeEnumerationExtras("use", element.getUseElement(), new ContactPoint.ContactPointUseEnumFactory(), false); 13839 } 13840 if (element.hasRankElement()) { 13841 composePositiveIntCore("rank", element.getRankElement(), false); 13842 composePositiveIntExtras("rank", element.getRankElement(), false); 13843 } 13844 if (element.hasPeriod()) { 13845 composePeriod("period", element.getPeriod()); 13846 } 13847 } 13848 13849 protected void composeMeta(String name, Meta element) throws IOException { 13850 if (element != null) { 13851 open(name); 13852 composeMetaInner(element); 13853 close(); 13854 } 13855 } 13856 13857 protected void composeMetaInner(Meta element) throws IOException { 13858 composeElement(element); 13859 if (element.hasVersionIdElement()) { 13860 composeIdCore("versionId", element.getVersionIdElement(), false); 13861 composeIdExtras("versionId", element.getVersionIdElement(), false); 13862 } 13863 if (element.hasLastUpdatedElement()) { 13864 composeInstantCore("lastUpdated", element.getLastUpdatedElement(), false); 13865 composeInstantExtras("lastUpdated", element.getLastUpdatedElement(), false); 13866 } 13867 if (element.hasProfile()) { 13868 openArray("profile"); 13869 for (UriType e : element.getProfile()) 13870 composeUriCore(null, e, true); 13871 closeArray(); 13872 if (anyHasExtras(element.getProfile())) { 13873 openArray("_profile"); 13874 for (UriType e : element.getProfile()) 13875 composeUriExtras(null, e, true); 13876 closeArray(); 13877 } 13878 } 13879 ; 13880 if (element.hasSecurity()) { 13881 openArray("security"); 13882 for (Coding e : element.getSecurity()) 13883 composeCoding(null, e); 13884 closeArray(); 13885 } 13886 ; 13887 if (element.hasTag()) { 13888 openArray("tag"); 13889 for (Coding e : element.getTag()) 13890 composeCoding(null, e); 13891 closeArray(); 13892 } 13893 ; 13894 } 13895 13896 protected void composeAddress(String name, Address element) throws IOException { 13897 if (element != null) { 13898 open(name); 13899 composeAddressInner(element); 13900 close(); 13901 } 13902 } 13903 13904 protected void composeAddressInner(Address element) throws IOException { 13905 composeElement(element); 13906 if (element.hasUseElement()) { 13907 composeEnumerationCore("use", element.getUseElement(), new Address.AddressUseEnumFactory(), false); 13908 composeEnumerationExtras("use", element.getUseElement(), new Address.AddressUseEnumFactory(), false); 13909 } 13910 if (element.hasTypeElement()) { 13911 composeEnumerationCore("type", element.getTypeElement(), new Address.AddressTypeEnumFactory(), false); 13912 composeEnumerationExtras("type", element.getTypeElement(), new Address.AddressTypeEnumFactory(), false); 13913 } 13914 if (element.hasTextElement()) { 13915 composeStringCore("text", element.getTextElement(), false); 13916 composeStringExtras("text", element.getTextElement(), false); 13917 } 13918 if (element.hasLine()) { 13919 openArray("line"); 13920 for (StringType e : element.getLine()) 13921 composeStringCore(null, e, true); 13922 closeArray(); 13923 if (anyHasExtras(element.getLine())) { 13924 openArray("_line"); 13925 for (StringType e : element.getLine()) 13926 composeStringExtras(null, e, true); 13927 closeArray(); 13928 } 13929 } 13930 ; 13931 if (element.hasCityElement()) { 13932 composeStringCore("city", element.getCityElement(), false); 13933 composeStringExtras("city", element.getCityElement(), false); 13934 } 13935 if (element.hasDistrictElement()) { 13936 composeStringCore("district", element.getDistrictElement(), false); 13937 composeStringExtras("district", element.getDistrictElement(), false); 13938 } 13939 if (element.hasStateElement()) { 13940 composeStringCore("state", element.getStateElement(), false); 13941 composeStringExtras("state", element.getStateElement(), false); 13942 } 13943 if (element.hasPostalCodeElement()) { 13944 composeStringCore("postalCode", element.getPostalCodeElement(), false); 13945 composeStringExtras("postalCode", element.getPostalCodeElement(), false); 13946 } 13947 if (element.hasCountryElement()) { 13948 composeStringCore("country", element.getCountryElement(), false); 13949 composeStringExtras("country", element.getCountryElement(), false); 13950 } 13951 if (element.hasPeriod()) { 13952 composePeriod("period", element.getPeriod()); 13953 } 13954 } 13955 13956 protected void composeTiming(String name, Timing element) throws IOException { 13957 if (element != null) { 13958 open(name); 13959 composeTimingInner(element); 13960 close(); 13961 } 13962 } 13963 13964 protected void composeTimingInner(Timing element) throws IOException { 13965 composeElement(element); 13966 if (element.hasEvent()) { 13967 openArray("event"); 13968 for (DateTimeType e : element.getEvent()) 13969 composeDateTimeCore(null, e, true); 13970 closeArray(); 13971 if (anyHasExtras(element.getEvent())) { 13972 openArray("_event"); 13973 for (DateTimeType e : element.getEvent()) 13974 composeDateTimeExtras(null, e, true); 13975 closeArray(); 13976 } 13977 } 13978 ; 13979 if (element.hasRepeat()) { 13980 composeTimingTimingRepeatComponent("repeat", element.getRepeat()); 13981 } 13982 if (element.hasCode()) { 13983 composeCodeableConcept("code", element.getCode()); 13984 } 13985 } 13986 13987 protected void composeTimingTimingRepeatComponent(String name, Timing.TimingRepeatComponent element) 13988 throws IOException { 13989 if (element != null) { 13990 open(name); 13991 composeTimingTimingRepeatComponentInner(element); 13992 close(); 13993 } 13994 } 13995 13996 protected void composeTimingTimingRepeatComponentInner(Timing.TimingRepeatComponent element) throws IOException { 13997 composeElement(element); 13998 if (element.hasBounds()) { 13999 composeType("bounds", element.getBounds()); 14000 } 14001 if (element.hasCountElement()) { 14002 composeIntegerCore("count", element.getCountElement(), false); 14003 composeIntegerExtras("count", element.getCountElement(), false); 14004 } 14005 if (element.hasDurationElement()) { 14006 composeDecimalCore("duration", element.getDurationElement(), false); 14007 composeDecimalExtras("duration", element.getDurationElement(), false); 14008 } 14009 if (element.hasDurationMaxElement()) { 14010 composeDecimalCore("durationMax", element.getDurationMaxElement(), false); 14011 composeDecimalExtras("durationMax", element.getDurationMaxElement(), false); 14012 } 14013 if (element.hasDurationUnitsElement()) { 14014 composeEnumerationCore("durationUnits", element.getDurationUnitsElement(), new Timing.UnitsOfTimeEnumFactory(), 14015 false); 14016 composeEnumerationExtras("durationUnits", element.getDurationUnitsElement(), new Timing.UnitsOfTimeEnumFactory(), 14017 false); 14018 } 14019 if (element.hasFrequencyElement()) { 14020 composeIntegerCore("frequency", element.getFrequencyElement(), false); 14021 composeIntegerExtras("frequency", element.getFrequencyElement(), false); 14022 } 14023 if (element.hasFrequencyMaxElement()) { 14024 composeIntegerCore("frequencyMax", element.getFrequencyMaxElement(), false); 14025 composeIntegerExtras("frequencyMax", element.getFrequencyMaxElement(), false); 14026 } 14027 if (element.hasPeriodElement()) { 14028 composeDecimalCore("period", element.getPeriodElement(), false); 14029 composeDecimalExtras("period", element.getPeriodElement(), false); 14030 } 14031 if (element.hasPeriodMaxElement()) { 14032 composeDecimalCore("periodMax", element.getPeriodMaxElement(), false); 14033 composeDecimalExtras("periodMax", element.getPeriodMaxElement(), false); 14034 } 14035 if (element.hasPeriodUnitsElement()) { 14036 composeEnumerationCore("periodUnits", element.getPeriodUnitsElement(), new Timing.UnitsOfTimeEnumFactory(), 14037 false); 14038 composeEnumerationExtras("periodUnits", element.getPeriodUnitsElement(), new Timing.UnitsOfTimeEnumFactory(), 14039 false); 14040 } 14041 if (element.hasWhenElement()) { 14042 composeEnumerationCore("when", element.getWhenElement(), new Timing.EventTimingEnumFactory(), false); 14043 composeEnumerationExtras("when", element.getWhenElement(), new Timing.EventTimingEnumFactory(), false); 14044 } 14045 } 14046 14047 protected void composeElementDefinition(String name, ElementDefinition element) throws IOException { 14048 if (element != null) { 14049 open(name); 14050 composeElementDefinitionInner(element); 14051 close(); 14052 } 14053 } 14054 14055 protected void composeElementDefinitionInner(ElementDefinition element) throws IOException { 14056 composeElement(element); 14057 if (element.hasPathElement()) { 14058 composeStringCore("path", element.getPathElement(), false); 14059 composeStringExtras("path", element.getPathElement(), false); 14060 } 14061 if (element.hasRepresentation()) { 14062 openArray("representation"); 14063 for (Enumeration<ElementDefinition.PropertyRepresentation> e : element.getRepresentation()) 14064 composeEnumerationCore(null, e, new ElementDefinition.PropertyRepresentationEnumFactory(), true); 14065 closeArray(); 14066 if (anyHasExtras(element.getRepresentation())) { 14067 openArray("_representation"); 14068 for (Enumeration<ElementDefinition.PropertyRepresentation> e : element.getRepresentation()) 14069 composeEnumerationExtras(null, e, new ElementDefinition.PropertyRepresentationEnumFactory(), true); 14070 closeArray(); 14071 } 14072 } 14073 ; 14074 if (element.hasNameElement()) { 14075 composeStringCore("name", element.getNameElement(), false); 14076 composeStringExtras("name", element.getNameElement(), false); 14077 } 14078 if (element.hasLabelElement()) { 14079 composeStringCore("label", element.getLabelElement(), false); 14080 composeStringExtras("label", element.getLabelElement(), false); 14081 } 14082 if (element.hasCode()) { 14083 openArray("code"); 14084 for (Coding e : element.getCode()) 14085 composeCoding(null, e); 14086 closeArray(); 14087 } 14088 ; 14089 if (element.hasSlicing()) { 14090 composeElementDefinitionElementDefinitionSlicingComponent("slicing", element.getSlicing()); 14091 } 14092 if (element.hasShortElement()) { 14093 composeStringCore("short", element.getShortElement(), false); 14094 composeStringExtras("short", element.getShortElement(), false); 14095 } 14096 if (element.hasDefinitionElement()) { 14097 composeMarkdownCore("definition", element.getDefinitionElement(), false); 14098 composeMarkdownExtras("definition", element.getDefinitionElement(), false); 14099 } 14100 if (element.hasCommentsElement()) { 14101 composeMarkdownCore("comments", element.getCommentsElement(), false); 14102 composeMarkdownExtras("comments", element.getCommentsElement(), false); 14103 } 14104 if (element.hasRequirementsElement()) { 14105 composeMarkdownCore("requirements", element.getRequirementsElement(), false); 14106 composeMarkdownExtras("requirements", element.getRequirementsElement(), false); 14107 } 14108 if (element.hasAlias()) { 14109 openArray("alias"); 14110 for (StringType e : element.getAlias()) 14111 composeStringCore(null, e, true); 14112 closeArray(); 14113 if (anyHasExtras(element.getAlias())) { 14114 openArray("_alias"); 14115 for (StringType e : element.getAlias()) 14116 composeStringExtras(null, e, true); 14117 closeArray(); 14118 } 14119 } 14120 ; 14121 if (element.hasMinElement()) { 14122 composeIntegerCore("min", element.getMinElement(), false); 14123 composeIntegerExtras("min", element.getMinElement(), false); 14124 } 14125 if (element.hasMaxElement()) { 14126 composeStringCore("max", element.getMaxElement(), false); 14127 composeStringExtras("max", element.getMaxElement(), false); 14128 } 14129 if (element.hasBase()) { 14130 composeElementDefinitionElementDefinitionBaseComponent("base", element.getBase()); 14131 } 14132 if (element.hasType()) { 14133 openArray("type"); 14134 for (ElementDefinition.TypeRefComponent e : element.getType()) 14135 composeElementDefinitionTypeRefComponent(null, e); 14136 closeArray(); 14137 } 14138 ; 14139 if (element.hasNameReferenceElement()) { 14140 composeStringCore("nameReference", element.getNameReferenceElement(), false); 14141 composeStringExtras("nameReference", element.getNameReferenceElement(), false); 14142 } 14143 if (element.hasDefaultValue()) { 14144 composeType("defaultValue", element.getDefaultValue()); 14145 } 14146 if (element.hasMeaningWhenMissingElement()) { 14147 composeMarkdownCore("meaningWhenMissing", element.getMeaningWhenMissingElement(), false); 14148 composeMarkdownExtras("meaningWhenMissing", element.getMeaningWhenMissingElement(), false); 14149 } 14150 if (element.hasFixed()) { 14151 composeType("fixed", element.getFixed()); 14152 } 14153 if (element.hasPattern()) { 14154 composeType("pattern", element.getPattern()); 14155 } 14156 if (element.hasExample()) { 14157 composeType("example", element.getExample()); 14158 } 14159 if (element.hasMinValue()) { 14160 composeType("minValue", element.getMinValue()); 14161 } 14162 if (element.hasMaxValue()) { 14163 composeType("maxValue", element.getMaxValue()); 14164 } 14165 if (element.hasMaxLengthElement()) { 14166 composeIntegerCore("maxLength", element.getMaxLengthElement(), false); 14167 composeIntegerExtras("maxLength", element.getMaxLengthElement(), false); 14168 } 14169 if (element.hasCondition()) { 14170 openArray("condition"); 14171 for (IdType e : element.getCondition()) 14172 composeIdCore(null, e, true); 14173 closeArray(); 14174 if (anyHasExtras(element.getCondition())) { 14175 openArray("_condition"); 14176 for (IdType e : element.getCondition()) 14177 composeIdExtras(null, e, true); 14178 closeArray(); 14179 } 14180 } 14181 ; 14182 if (element.hasConstraint()) { 14183 openArray("constraint"); 14184 for (ElementDefinition.ElementDefinitionConstraintComponent e : element.getConstraint()) 14185 composeElementDefinitionElementDefinitionConstraintComponent(null, e); 14186 closeArray(); 14187 } 14188 ; 14189 if (element.hasMustSupportElement()) { 14190 composeBooleanCore("mustSupport", element.getMustSupportElement(), false); 14191 composeBooleanExtras("mustSupport", element.getMustSupportElement(), false); 14192 } 14193 if (element.hasIsModifierElement()) { 14194 composeBooleanCore("isModifier", element.getIsModifierElement(), false); 14195 composeBooleanExtras("isModifier", element.getIsModifierElement(), false); 14196 } 14197 if (element.hasIsSummaryElement()) { 14198 composeBooleanCore("isSummary", element.getIsSummaryElement(), false); 14199 composeBooleanExtras("isSummary", element.getIsSummaryElement(), false); 14200 } 14201 if (element.hasBinding()) { 14202 composeElementDefinitionElementDefinitionBindingComponent("binding", element.getBinding()); 14203 } 14204 if (element.hasMapping()) { 14205 openArray("mapping"); 14206 for (ElementDefinition.ElementDefinitionMappingComponent e : element.getMapping()) 14207 composeElementDefinitionElementDefinitionMappingComponent(null, e); 14208 closeArray(); 14209 } 14210 ; 14211 } 14212 14213 protected void composeElementDefinitionElementDefinitionSlicingComponent(String name, 14214 ElementDefinition.ElementDefinitionSlicingComponent element) throws IOException { 14215 if (element != null) { 14216 open(name); 14217 composeElementDefinitionElementDefinitionSlicingComponentInner(element); 14218 close(); 14219 } 14220 } 14221 14222 protected void composeElementDefinitionElementDefinitionSlicingComponentInner( 14223 ElementDefinition.ElementDefinitionSlicingComponent element) throws IOException { 14224 composeElement(element); 14225 if (element.hasDiscriminator()) { 14226 openArray("discriminator"); 14227 for (StringType e : element.getDiscriminator()) 14228 composeStringCore(null, e, true); 14229 closeArray(); 14230 if (anyHasExtras(element.getDiscriminator())) { 14231 openArray("_discriminator"); 14232 for (StringType e : element.getDiscriminator()) 14233 composeStringExtras(null, e, true); 14234 closeArray(); 14235 } 14236 } 14237 ; 14238 if (element.hasDescriptionElement()) { 14239 composeStringCore("description", element.getDescriptionElement(), false); 14240 composeStringExtras("description", element.getDescriptionElement(), false); 14241 } 14242 if (element.hasOrderedElement()) { 14243 composeBooleanCore("ordered", element.getOrderedElement(), false); 14244 composeBooleanExtras("ordered", element.getOrderedElement(), false); 14245 } 14246 if (element.hasRulesElement()) { 14247 composeEnumerationCore("rules", element.getRulesElement(), new ElementDefinition.SlicingRulesEnumFactory(), 14248 false); 14249 composeEnumerationExtras("rules", element.getRulesElement(), new ElementDefinition.SlicingRulesEnumFactory(), 14250 false); 14251 } 14252 } 14253 14254 protected void composeElementDefinitionElementDefinitionBaseComponent(String name, 14255 ElementDefinition.ElementDefinitionBaseComponent element) throws IOException { 14256 if (element != null) { 14257 open(name); 14258 composeElementDefinitionElementDefinitionBaseComponentInner(element); 14259 close(); 14260 } 14261 } 14262 14263 protected void composeElementDefinitionElementDefinitionBaseComponentInner( 14264 ElementDefinition.ElementDefinitionBaseComponent element) throws IOException { 14265 composeElement(element); 14266 if (element.hasPathElement()) { 14267 composeStringCore("path", element.getPathElement(), false); 14268 composeStringExtras("path", element.getPathElement(), false); 14269 } 14270 if (element.hasMinElement()) { 14271 composeIntegerCore("min", element.getMinElement(), false); 14272 composeIntegerExtras("min", element.getMinElement(), false); 14273 } 14274 if (element.hasMaxElement()) { 14275 composeStringCore("max", element.getMaxElement(), false); 14276 composeStringExtras("max", element.getMaxElement(), false); 14277 } 14278 } 14279 14280 protected void composeElementDefinitionTypeRefComponent(String name, ElementDefinition.TypeRefComponent element) 14281 throws IOException { 14282 if (element != null) { 14283 open(name); 14284 composeElementDefinitionTypeRefComponentInner(element); 14285 close(); 14286 } 14287 } 14288 14289 protected void composeElementDefinitionTypeRefComponentInner(ElementDefinition.TypeRefComponent element) 14290 throws IOException { 14291 composeElement(element); 14292 if (element.hasCodeElement()) { 14293 composeCodeCore("code", element.getCodeElement(), false); 14294 composeCodeExtras("code", element.getCodeElement(), false); 14295 } 14296 if (element.hasProfile()) { 14297 openArray("profile"); 14298 for (UriType e : element.getProfile()) 14299 composeUriCore(null, e, true); 14300 closeArray(); 14301 if (anyHasExtras(element.getProfile())) { 14302 openArray("_profile"); 14303 for (UriType e : element.getProfile()) 14304 composeUriExtras(null, e, true); 14305 closeArray(); 14306 } 14307 } 14308 ; 14309 if (element.hasAggregation()) { 14310 openArray("aggregation"); 14311 for (Enumeration<ElementDefinition.AggregationMode> e : element.getAggregation()) 14312 composeEnumerationCore(null, e, new ElementDefinition.AggregationModeEnumFactory(), true); 14313 closeArray(); 14314 if (anyHasExtras(element.getAggregation())) { 14315 openArray("_aggregation"); 14316 for (Enumeration<ElementDefinition.AggregationMode> e : element.getAggregation()) 14317 composeEnumerationExtras(null, e, new ElementDefinition.AggregationModeEnumFactory(), true); 14318 closeArray(); 14319 } 14320 } 14321 ; 14322 } 14323 14324 protected void composeElementDefinitionElementDefinitionConstraintComponent(String name, 14325 ElementDefinition.ElementDefinitionConstraintComponent element) throws IOException { 14326 if (element != null) { 14327 open(name); 14328 composeElementDefinitionElementDefinitionConstraintComponentInner(element); 14329 close(); 14330 } 14331 } 14332 14333 protected void composeElementDefinitionElementDefinitionConstraintComponentInner( 14334 ElementDefinition.ElementDefinitionConstraintComponent element) throws IOException { 14335 composeElement(element); 14336 if (element.hasKeyElement()) { 14337 composeIdCore("key", element.getKeyElement(), false); 14338 composeIdExtras("key", element.getKeyElement(), false); 14339 } 14340 if (element.hasRequirementsElement()) { 14341 composeStringCore("requirements", element.getRequirementsElement(), false); 14342 composeStringExtras("requirements", element.getRequirementsElement(), false); 14343 } 14344 if (element.hasSeverityElement()) { 14345 composeEnumerationCore("severity", element.getSeverityElement(), 14346 new ElementDefinition.ConstraintSeverityEnumFactory(), false); 14347 composeEnumerationExtras("severity", element.getSeverityElement(), 14348 new ElementDefinition.ConstraintSeverityEnumFactory(), false); 14349 } 14350 if (element.hasHumanElement()) { 14351 composeStringCore("human", element.getHumanElement(), false); 14352 composeStringExtras("human", element.getHumanElement(), false); 14353 } 14354 if (element.hasXpathElement()) { 14355 composeStringCore("xpath", element.getXpathElement(), false); 14356 composeStringExtras("xpath", element.getXpathElement(), false); 14357 } 14358 } 14359 14360 protected void composeElementDefinitionElementDefinitionBindingComponent(String name, 14361 ElementDefinition.ElementDefinitionBindingComponent element) throws IOException { 14362 if (element != null) { 14363 open(name); 14364 composeElementDefinitionElementDefinitionBindingComponentInner(element); 14365 close(); 14366 } 14367 } 14368 14369 protected void composeElementDefinitionElementDefinitionBindingComponentInner( 14370 ElementDefinition.ElementDefinitionBindingComponent element) throws IOException { 14371 composeElement(element); 14372 if (element.hasStrengthElement()) { 14373 composeEnumerationCore("strength", element.getStrengthElement(), new Enumerations.BindingStrengthEnumFactory(), 14374 false); 14375 composeEnumerationExtras("strength", element.getStrengthElement(), new Enumerations.BindingStrengthEnumFactory(), 14376 false); 14377 } 14378 if (element.hasDescriptionElement()) { 14379 composeStringCore("description", element.getDescriptionElement(), false); 14380 composeStringExtras("description", element.getDescriptionElement(), false); 14381 } 14382 if (element.hasValueSet()) { 14383 composeType("valueSet", element.getValueSet()); 14384 } 14385 } 14386 14387 protected void composeElementDefinitionElementDefinitionMappingComponent(String name, 14388 ElementDefinition.ElementDefinitionMappingComponent element) throws IOException { 14389 if (element != null) { 14390 open(name); 14391 composeElementDefinitionElementDefinitionMappingComponentInner(element); 14392 close(); 14393 } 14394 } 14395 14396 protected void composeElementDefinitionElementDefinitionMappingComponentInner( 14397 ElementDefinition.ElementDefinitionMappingComponent element) throws IOException { 14398 composeElement(element); 14399 if (element.hasIdentityElement()) { 14400 composeIdCore("identity", element.getIdentityElement(), false); 14401 composeIdExtras("identity", element.getIdentityElement(), false); 14402 } 14403 if (element.hasLanguageElement()) { 14404 composeCodeCore("language", element.getLanguageElement(), false); 14405 composeCodeExtras("language", element.getLanguageElement(), false); 14406 } 14407 if (element.hasMapElement()) { 14408 composeStringCore("map", element.getMapElement(), false); 14409 composeStringExtras("map", element.getMapElement(), false); 14410 } 14411 } 14412 14413 protected void composeDomainResourceElements(DomainResource element) throws IOException { 14414 composeResourceElements(element); 14415 if (element.hasText()) { 14416 composeNarrative("text", element.getText()); 14417 } 14418 if (element.hasContained()) { 14419 openArray("contained"); 14420 for (Resource e : element.getContained()) { 14421 open(null); 14422 composeResource(e); 14423 close(); 14424 } 14425 closeArray(); 14426 } 14427 ; 14428 if (element.hasExtension()) { 14429 openArray("extension"); 14430 for (Extension e : element.getExtension()) 14431 composeExtension(null, e); 14432 closeArray(); 14433 } 14434 ; 14435 if (element.hasModifierExtension()) { 14436 openArray("modifierExtension"); 14437 for (Extension e : element.getModifierExtension()) 14438 composeExtension(null, e); 14439 closeArray(); 14440 } 14441 ; 14442 } 14443 14444 protected void composeParameters(String name, Parameters element) throws IOException { 14445 if (element != null) { 14446 prop("resourceType", name); 14447 composeParametersInner(element); 14448 } 14449 } 14450 14451 protected void composeParametersInner(Parameters element) throws IOException { 14452 composeResourceElements(element); 14453 if (element.hasParameter()) { 14454 openArray("parameter"); 14455 for (Parameters.ParametersParameterComponent e : element.getParameter()) 14456 composeParametersParametersParameterComponent(null, e); 14457 closeArray(); 14458 } 14459 ; 14460 } 14461 14462 protected void composeParametersParametersParameterComponent(String name, 14463 Parameters.ParametersParameterComponent element) throws IOException { 14464 if (element != null) { 14465 open(name); 14466 composeParametersParametersParameterComponentInner(element); 14467 close(); 14468 } 14469 } 14470 14471 protected void composeParametersParametersParameterComponentInner(Parameters.ParametersParameterComponent element) 14472 throws IOException { 14473 composeBackbone(element); 14474 if (element.hasNameElement()) { 14475 composeStringCore("name", element.getNameElement(), false); 14476 composeStringExtras("name", element.getNameElement(), false); 14477 } 14478 if (element.hasValue()) { 14479 composeType("value", element.getValue()); 14480 } 14481 if (element.hasResource()) { 14482 open("resource"); 14483 composeResource(element.getResource()); 14484 close(); 14485 } 14486 if (element.hasPart()) { 14487 openArray("part"); 14488 for (Parameters.ParametersParameterComponent e : element.getPart()) 14489 composeParametersParametersParameterComponent(null, e); 14490 closeArray(); 14491 } 14492 ; 14493 } 14494 14495 protected void composeResourceElements(Resource element) throws IOException { 14496 if (element.hasIdElement()) { 14497 composeIdCore("id", element.getIdElement(), false); 14498 composeIdExtras("id", element.getIdElement(), false); 14499 } 14500 if (element.hasMeta()) { 14501 composeMeta("meta", element.getMeta()); 14502 } 14503 if (element.hasImplicitRulesElement()) { 14504 composeUriCore("implicitRules", element.getImplicitRulesElement(), false); 14505 composeUriExtras("implicitRules", element.getImplicitRulesElement(), false); 14506 } 14507 if (element.hasLanguageElement()) { 14508 composeCodeCore("language", element.getLanguageElement(), false); 14509 composeCodeExtras("language", element.getLanguageElement(), false); 14510 } 14511 } 14512 14513 protected void composeAccount(String name, Account element) throws IOException { 14514 if (element != null) { 14515 prop("resourceType", name); 14516 composeAccountInner(element); 14517 } 14518 } 14519 14520 protected void composeAccountInner(Account element) throws IOException { 14521 composeDomainResourceElements(element); 14522 if (element.hasIdentifier()) { 14523 openArray("identifier"); 14524 for (Identifier e : element.getIdentifier()) 14525 composeIdentifier(null, e); 14526 closeArray(); 14527 } 14528 ; 14529 if (element.hasNameElement()) { 14530 composeStringCore("name", element.getNameElement(), false); 14531 composeStringExtras("name", element.getNameElement(), false); 14532 } 14533 if (element.hasType()) { 14534 composeCodeableConcept("type", element.getType()); 14535 } 14536 if (element.hasStatusElement()) { 14537 composeEnumerationCore("status", element.getStatusElement(), new Account.AccountStatusEnumFactory(), false); 14538 composeEnumerationExtras("status", element.getStatusElement(), new Account.AccountStatusEnumFactory(), false); 14539 } 14540 if (element.hasActivePeriod()) { 14541 composePeriod("activePeriod", element.getActivePeriod()); 14542 } 14543 if (element.hasCurrency()) { 14544 composeCoding("currency", element.getCurrency()); 14545 } 14546 if (element.hasBalance()) { 14547 composeMoney("balance", element.getBalance()); 14548 } 14549 if (element.hasCoveragePeriod()) { 14550 composePeriod("coveragePeriod", element.getCoveragePeriod()); 14551 } 14552 if (element.hasSubject()) { 14553 composeReference("subject", element.getSubject()); 14554 } 14555 if (element.hasOwner()) { 14556 composeReference("owner", element.getOwner()); 14557 } 14558 if (element.hasDescriptionElement()) { 14559 composeStringCore("description", element.getDescriptionElement(), false); 14560 composeStringExtras("description", element.getDescriptionElement(), false); 14561 } 14562 } 14563 14564 protected void composeAllergyIntolerance(String name, AllergyIntolerance element) throws IOException { 14565 if (element != null) { 14566 prop("resourceType", name); 14567 composeAllergyIntoleranceInner(element); 14568 } 14569 } 14570 14571 protected void composeAllergyIntoleranceInner(AllergyIntolerance element) throws IOException { 14572 composeDomainResourceElements(element); 14573 if (element.hasIdentifier()) { 14574 openArray("identifier"); 14575 for (Identifier e : element.getIdentifier()) 14576 composeIdentifier(null, e); 14577 closeArray(); 14578 } 14579 ; 14580 if (element.hasOnsetElement()) { 14581 composeDateTimeCore("onset", element.getOnsetElement(), false); 14582 composeDateTimeExtras("onset", element.getOnsetElement(), false); 14583 } 14584 if (element.hasRecordedDateElement()) { 14585 composeDateTimeCore("recordedDate", element.getRecordedDateElement(), false); 14586 composeDateTimeExtras("recordedDate", element.getRecordedDateElement(), false); 14587 } 14588 if (element.hasRecorder()) { 14589 composeReference("recorder", element.getRecorder()); 14590 } 14591 if (element.hasPatient()) { 14592 composeReference("patient", element.getPatient()); 14593 } 14594 if (element.hasReporter()) { 14595 composeReference("reporter", element.getReporter()); 14596 } 14597 if (element.hasSubstance()) { 14598 composeCodeableConcept("substance", element.getSubstance()); 14599 } 14600 if (element.hasStatusElement()) { 14601 composeEnumerationCore("status", element.getStatusElement(), 14602 new AllergyIntolerance.AllergyIntoleranceStatusEnumFactory(), false); 14603 composeEnumerationExtras("status", element.getStatusElement(), 14604 new AllergyIntolerance.AllergyIntoleranceStatusEnumFactory(), false); 14605 } 14606 if (element.hasCriticalityElement()) { 14607 composeEnumerationCore("criticality", element.getCriticalityElement(), 14608 new AllergyIntolerance.AllergyIntoleranceCriticalityEnumFactory(), false); 14609 composeEnumerationExtras("criticality", element.getCriticalityElement(), 14610 new AllergyIntolerance.AllergyIntoleranceCriticalityEnumFactory(), false); 14611 } 14612 if (element.hasTypeElement()) { 14613 composeEnumerationCore("type", element.getTypeElement(), 14614 new AllergyIntolerance.AllergyIntoleranceTypeEnumFactory(), false); 14615 composeEnumerationExtras("type", element.getTypeElement(), 14616 new AllergyIntolerance.AllergyIntoleranceTypeEnumFactory(), false); 14617 } 14618 if (element.hasCategoryElement()) { 14619 composeEnumerationCore("category", element.getCategoryElement(), 14620 new AllergyIntolerance.AllergyIntoleranceCategoryEnumFactory(), false); 14621 composeEnumerationExtras("category", element.getCategoryElement(), 14622 new AllergyIntolerance.AllergyIntoleranceCategoryEnumFactory(), false); 14623 } 14624 if (element.hasLastOccurenceElement()) { 14625 composeDateTimeCore("lastOccurence", element.getLastOccurenceElement(), false); 14626 composeDateTimeExtras("lastOccurence", element.getLastOccurenceElement(), false); 14627 } 14628 if (element.hasNote()) { 14629 composeAnnotation("note", element.getNote()); 14630 } 14631 if (element.hasReaction()) { 14632 openArray("reaction"); 14633 for (AllergyIntolerance.AllergyIntoleranceReactionComponent e : element.getReaction()) 14634 composeAllergyIntoleranceAllergyIntoleranceReactionComponent(null, e); 14635 closeArray(); 14636 } 14637 ; 14638 } 14639 14640 protected void composeAllergyIntoleranceAllergyIntoleranceReactionComponent(String name, 14641 AllergyIntolerance.AllergyIntoleranceReactionComponent element) throws IOException { 14642 if (element != null) { 14643 open(name); 14644 composeAllergyIntoleranceAllergyIntoleranceReactionComponentInner(element); 14645 close(); 14646 } 14647 } 14648 14649 protected void composeAllergyIntoleranceAllergyIntoleranceReactionComponentInner( 14650 AllergyIntolerance.AllergyIntoleranceReactionComponent element) throws IOException { 14651 composeBackbone(element); 14652 if (element.hasSubstance()) { 14653 composeCodeableConcept("substance", element.getSubstance()); 14654 } 14655 if (element.hasCertaintyElement()) { 14656 composeEnumerationCore("certainty", element.getCertaintyElement(), 14657 new AllergyIntolerance.AllergyIntoleranceCertaintyEnumFactory(), false); 14658 composeEnumerationExtras("certainty", element.getCertaintyElement(), 14659 new AllergyIntolerance.AllergyIntoleranceCertaintyEnumFactory(), false); 14660 } 14661 if (element.hasManifestation()) { 14662 openArray("manifestation"); 14663 for (CodeableConcept e : element.getManifestation()) 14664 composeCodeableConcept(null, e); 14665 closeArray(); 14666 } 14667 ; 14668 if (element.hasDescriptionElement()) { 14669 composeStringCore("description", element.getDescriptionElement(), false); 14670 composeStringExtras("description", element.getDescriptionElement(), false); 14671 } 14672 if (element.hasOnsetElement()) { 14673 composeDateTimeCore("onset", element.getOnsetElement(), false); 14674 composeDateTimeExtras("onset", element.getOnsetElement(), false); 14675 } 14676 if (element.hasSeverityElement()) { 14677 composeEnumerationCore("severity", element.getSeverityElement(), 14678 new AllergyIntolerance.AllergyIntoleranceSeverityEnumFactory(), false); 14679 composeEnumerationExtras("severity", element.getSeverityElement(), 14680 new AllergyIntolerance.AllergyIntoleranceSeverityEnumFactory(), false); 14681 } 14682 if (element.hasExposureRoute()) { 14683 composeCodeableConcept("exposureRoute", element.getExposureRoute()); 14684 } 14685 if (element.hasNote()) { 14686 composeAnnotation("note", element.getNote()); 14687 } 14688 } 14689 14690 protected void composeAppointment(String name, Appointment element) throws IOException { 14691 if (element != null) { 14692 prop("resourceType", name); 14693 composeAppointmentInner(element); 14694 } 14695 } 14696 14697 protected void composeAppointmentInner(Appointment element) throws IOException { 14698 composeDomainResourceElements(element); 14699 if (element.hasIdentifier()) { 14700 openArray("identifier"); 14701 for (Identifier e : element.getIdentifier()) 14702 composeIdentifier(null, e); 14703 closeArray(); 14704 } 14705 ; 14706 if (element.hasStatusElement()) { 14707 composeEnumerationCore("status", element.getStatusElement(), new Appointment.AppointmentStatusEnumFactory(), 14708 false); 14709 composeEnumerationExtras("status", element.getStatusElement(), new Appointment.AppointmentStatusEnumFactory(), 14710 false); 14711 } 14712 if (element.hasType()) { 14713 composeCodeableConcept("type", element.getType()); 14714 } 14715 if (element.hasReason()) { 14716 composeCodeableConcept("reason", element.getReason()); 14717 } 14718 if (element.hasPriorityElement()) { 14719 composeUnsignedIntCore("priority", element.getPriorityElement(), false); 14720 composeUnsignedIntExtras("priority", element.getPriorityElement(), false); 14721 } 14722 if (element.hasDescriptionElement()) { 14723 composeStringCore("description", element.getDescriptionElement(), false); 14724 composeStringExtras("description", element.getDescriptionElement(), false); 14725 } 14726 if (element.hasStartElement()) { 14727 composeInstantCore("start", element.getStartElement(), false); 14728 composeInstantExtras("start", element.getStartElement(), false); 14729 } 14730 if (element.hasEndElement()) { 14731 composeInstantCore("end", element.getEndElement(), false); 14732 composeInstantExtras("end", element.getEndElement(), false); 14733 } 14734 if (element.hasMinutesDurationElement()) { 14735 composePositiveIntCore("minutesDuration", element.getMinutesDurationElement(), false); 14736 composePositiveIntExtras("minutesDuration", element.getMinutesDurationElement(), false); 14737 } 14738 if (element.hasSlot()) { 14739 openArray("slot"); 14740 for (Reference e : element.getSlot()) 14741 composeReference(null, e); 14742 closeArray(); 14743 } 14744 ; 14745 if (element.hasCommentElement()) { 14746 composeStringCore("comment", element.getCommentElement(), false); 14747 composeStringExtras("comment", element.getCommentElement(), false); 14748 } 14749 if (element.hasParticipant()) { 14750 openArray("participant"); 14751 for (Appointment.AppointmentParticipantComponent e : element.getParticipant()) 14752 composeAppointmentAppointmentParticipantComponent(null, e); 14753 closeArray(); 14754 } 14755 ; 14756 } 14757 14758 protected void composeAppointmentAppointmentParticipantComponent(String name, 14759 Appointment.AppointmentParticipantComponent element) throws IOException { 14760 if (element != null) { 14761 open(name); 14762 composeAppointmentAppointmentParticipantComponentInner(element); 14763 close(); 14764 } 14765 } 14766 14767 protected void composeAppointmentAppointmentParticipantComponentInner( 14768 Appointment.AppointmentParticipantComponent element) throws IOException { 14769 composeBackbone(element); 14770 if (element.hasType()) { 14771 openArray("type"); 14772 for (CodeableConcept e : element.getType()) 14773 composeCodeableConcept(null, e); 14774 closeArray(); 14775 } 14776 ; 14777 if (element.hasActor()) { 14778 composeReference("actor", element.getActor()); 14779 } 14780 if (element.hasRequiredElement()) { 14781 composeEnumerationCore("required", element.getRequiredElement(), new Appointment.ParticipantRequiredEnumFactory(), 14782 false); 14783 composeEnumerationExtras("required", element.getRequiredElement(), 14784 new Appointment.ParticipantRequiredEnumFactory(), false); 14785 } 14786 if (element.hasStatusElement()) { 14787 composeEnumerationCore("status", element.getStatusElement(), new Appointment.ParticipationStatusEnumFactory(), 14788 false); 14789 composeEnumerationExtras("status", element.getStatusElement(), new Appointment.ParticipationStatusEnumFactory(), 14790 false); 14791 } 14792 } 14793 14794 protected void composeAppointmentResponse(String name, AppointmentResponse element) throws IOException { 14795 if (element != null) { 14796 prop("resourceType", name); 14797 composeAppointmentResponseInner(element); 14798 } 14799 } 14800 14801 protected void composeAppointmentResponseInner(AppointmentResponse element) throws IOException { 14802 composeDomainResourceElements(element); 14803 if (element.hasIdentifier()) { 14804 openArray("identifier"); 14805 for (Identifier e : element.getIdentifier()) 14806 composeIdentifier(null, e); 14807 closeArray(); 14808 } 14809 ; 14810 if (element.hasAppointment()) { 14811 composeReference("appointment", element.getAppointment()); 14812 } 14813 if (element.hasStartElement()) { 14814 composeInstantCore("start", element.getStartElement(), false); 14815 composeInstantExtras("start", element.getStartElement(), false); 14816 } 14817 if (element.hasEndElement()) { 14818 composeInstantCore("end", element.getEndElement(), false); 14819 composeInstantExtras("end", element.getEndElement(), false); 14820 } 14821 if (element.hasParticipantType()) { 14822 openArray("participantType"); 14823 for (CodeableConcept e : element.getParticipantType()) 14824 composeCodeableConcept(null, e); 14825 closeArray(); 14826 } 14827 ; 14828 if (element.hasActor()) { 14829 composeReference("actor", element.getActor()); 14830 } 14831 if (element.hasParticipantStatusElement()) { 14832 composeEnumerationCore("participantStatus", element.getParticipantStatusElement(), 14833 new AppointmentResponse.ParticipantStatusEnumFactory(), false); 14834 composeEnumerationExtras("participantStatus", element.getParticipantStatusElement(), 14835 new AppointmentResponse.ParticipantStatusEnumFactory(), false); 14836 } 14837 if (element.hasCommentElement()) { 14838 composeStringCore("comment", element.getCommentElement(), false); 14839 composeStringExtras("comment", element.getCommentElement(), false); 14840 } 14841 } 14842 14843 protected void composeAuditEvent(String name, AuditEvent element) throws IOException { 14844 if (element != null) { 14845 prop("resourceType", name); 14846 composeAuditEventInner(element); 14847 } 14848 } 14849 14850 protected void composeAuditEventInner(AuditEvent element) throws IOException { 14851 composeDomainResourceElements(element); 14852 if (element.hasEvent()) { 14853 composeAuditEventAuditEventEventComponent("event", element.getEvent()); 14854 } 14855 if (element.hasParticipant()) { 14856 openArray("participant"); 14857 for (AuditEvent.AuditEventParticipantComponent e : element.getParticipant()) 14858 composeAuditEventAuditEventParticipantComponent(null, e); 14859 closeArray(); 14860 } 14861 ; 14862 if (element.hasSource()) { 14863 composeAuditEventAuditEventSourceComponent("source", element.getSource()); 14864 } 14865 if (element.hasObject()) { 14866 openArray("object"); 14867 for (AuditEvent.AuditEventObjectComponent e : element.getObject()) 14868 composeAuditEventAuditEventObjectComponent(null, e); 14869 closeArray(); 14870 } 14871 ; 14872 } 14873 14874 protected void composeAuditEventAuditEventEventComponent(String name, AuditEvent.AuditEventEventComponent element) 14875 throws IOException { 14876 if (element != null) { 14877 open(name); 14878 composeAuditEventAuditEventEventComponentInner(element); 14879 close(); 14880 } 14881 } 14882 14883 protected void composeAuditEventAuditEventEventComponentInner(AuditEvent.AuditEventEventComponent element) 14884 throws IOException { 14885 composeBackbone(element); 14886 if (element.hasType()) { 14887 composeCoding("type", element.getType()); 14888 } 14889 if (element.hasSubtype()) { 14890 openArray("subtype"); 14891 for (Coding e : element.getSubtype()) 14892 composeCoding(null, e); 14893 closeArray(); 14894 } 14895 ; 14896 if (element.hasActionElement()) { 14897 composeEnumerationCore("action", element.getActionElement(), new AuditEvent.AuditEventActionEnumFactory(), false); 14898 composeEnumerationExtras("action", element.getActionElement(), new AuditEvent.AuditEventActionEnumFactory(), 14899 false); 14900 } 14901 if (element.hasDateTimeElement()) { 14902 composeInstantCore("dateTime", element.getDateTimeElement(), false); 14903 composeInstantExtras("dateTime", element.getDateTimeElement(), false); 14904 } 14905 if (element.hasOutcomeElement()) { 14906 composeEnumerationCore("outcome", element.getOutcomeElement(), new AuditEvent.AuditEventOutcomeEnumFactory(), 14907 false); 14908 composeEnumerationExtras("outcome", element.getOutcomeElement(), new AuditEvent.AuditEventOutcomeEnumFactory(), 14909 false); 14910 } 14911 if (element.hasOutcomeDescElement()) { 14912 composeStringCore("outcomeDesc", element.getOutcomeDescElement(), false); 14913 composeStringExtras("outcomeDesc", element.getOutcomeDescElement(), false); 14914 } 14915 if (element.hasPurposeOfEvent()) { 14916 openArray("purposeOfEvent"); 14917 for (Coding e : element.getPurposeOfEvent()) 14918 composeCoding(null, e); 14919 closeArray(); 14920 } 14921 ; 14922 } 14923 14924 protected void composeAuditEventAuditEventParticipantComponent(String name, 14925 AuditEvent.AuditEventParticipantComponent element) throws IOException { 14926 if (element != null) { 14927 open(name); 14928 composeAuditEventAuditEventParticipantComponentInner(element); 14929 close(); 14930 } 14931 } 14932 14933 protected void composeAuditEventAuditEventParticipantComponentInner(AuditEvent.AuditEventParticipantComponent element) 14934 throws IOException { 14935 composeBackbone(element); 14936 if (element.hasRole()) { 14937 openArray("role"); 14938 for (CodeableConcept e : element.getRole()) 14939 composeCodeableConcept(null, e); 14940 closeArray(); 14941 } 14942 ; 14943 if (element.hasReference()) { 14944 composeReference("reference", element.getReference()); 14945 } 14946 if (element.hasUserId()) { 14947 composeIdentifier("userId", element.getUserId()); 14948 } 14949 if (element.hasAltIdElement()) { 14950 composeStringCore("altId", element.getAltIdElement(), false); 14951 composeStringExtras("altId", element.getAltIdElement(), false); 14952 } 14953 if (element.hasNameElement()) { 14954 composeStringCore("name", element.getNameElement(), false); 14955 composeStringExtras("name", element.getNameElement(), false); 14956 } 14957 if (element.hasRequestorElement()) { 14958 composeBooleanCore("requestor", element.getRequestorElement(), false); 14959 composeBooleanExtras("requestor", element.getRequestorElement(), false); 14960 } 14961 if (element.hasLocation()) { 14962 composeReference("location", element.getLocation()); 14963 } 14964 if (element.hasPolicy()) { 14965 openArray("policy"); 14966 for (UriType e : element.getPolicy()) 14967 composeUriCore(null, e, true); 14968 closeArray(); 14969 if (anyHasExtras(element.getPolicy())) { 14970 openArray("_policy"); 14971 for (UriType e : element.getPolicy()) 14972 composeUriExtras(null, e, true); 14973 closeArray(); 14974 } 14975 } 14976 ; 14977 if (element.hasMedia()) { 14978 composeCoding("media", element.getMedia()); 14979 } 14980 if (element.hasNetwork()) { 14981 composeAuditEventAuditEventParticipantNetworkComponent("network", element.getNetwork()); 14982 } 14983 if (element.hasPurposeOfUse()) { 14984 openArray("purposeOfUse"); 14985 for (Coding e : element.getPurposeOfUse()) 14986 composeCoding(null, e); 14987 closeArray(); 14988 } 14989 ; 14990 } 14991 14992 protected void composeAuditEventAuditEventParticipantNetworkComponent(String name, 14993 AuditEvent.AuditEventParticipantNetworkComponent element) throws IOException { 14994 if (element != null) { 14995 open(name); 14996 composeAuditEventAuditEventParticipantNetworkComponentInner(element); 14997 close(); 14998 } 14999 } 15000 15001 protected void composeAuditEventAuditEventParticipantNetworkComponentInner( 15002 AuditEvent.AuditEventParticipantNetworkComponent element) throws IOException { 15003 composeBackbone(element); 15004 if (element.hasAddressElement()) { 15005 composeStringCore("address", element.getAddressElement(), false); 15006 composeStringExtras("address", element.getAddressElement(), false); 15007 } 15008 if (element.hasTypeElement()) { 15009 composeEnumerationCore("type", element.getTypeElement(), 15010 new AuditEvent.AuditEventParticipantNetworkTypeEnumFactory(), false); 15011 composeEnumerationExtras("type", element.getTypeElement(), 15012 new AuditEvent.AuditEventParticipantNetworkTypeEnumFactory(), false); 15013 } 15014 } 15015 15016 protected void composeAuditEventAuditEventSourceComponent(String name, AuditEvent.AuditEventSourceComponent element) 15017 throws IOException { 15018 if (element != null) { 15019 open(name); 15020 composeAuditEventAuditEventSourceComponentInner(element); 15021 close(); 15022 } 15023 } 15024 15025 protected void composeAuditEventAuditEventSourceComponentInner(AuditEvent.AuditEventSourceComponent element) 15026 throws IOException { 15027 composeBackbone(element); 15028 if (element.hasSiteElement()) { 15029 composeStringCore("site", element.getSiteElement(), false); 15030 composeStringExtras("site", element.getSiteElement(), false); 15031 } 15032 if (element.hasIdentifier()) { 15033 composeIdentifier("identifier", element.getIdentifier()); 15034 } 15035 if (element.hasType()) { 15036 openArray("type"); 15037 for (Coding e : element.getType()) 15038 composeCoding(null, e); 15039 closeArray(); 15040 } 15041 ; 15042 } 15043 15044 protected void composeAuditEventAuditEventObjectComponent(String name, AuditEvent.AuditEventObjectComponent element) 15045 throws IOException { 15046 if (element != null) { 15047 open(name); 15048 composeAuditEventAuditEventObjectComponentInner(element); 15049 close(); 15050 } 15051 } 15052 15053 protected void composeAuditEventAuditEventObjectComponentInner(AuditEvent.AuditEventObjectComponent element) 15054 throws IOException { 15055 composeBackbone(element); 15056 if (element.hasIdentifier()) { 15057 composeIdentifier("identifier", element.getIdentifier()); 15058 } 15059 if (element.hasReference()) { 15060 composeReference("reference", element.getReference()); 15061 } 15062 if (element.hasType()) { 15063 composeCoding("type", element.getType()); 15064 } 15065 if (element.hasRole()) { 15066 composeCoding("role", element.getRole()); 15067 } 15068 if (element.hasLifecycle()) { 15069 composeCoding("lifecycle", element.getLifecycle()); 15070 } 15071 if (element.hasSecurityLabel()) { 15072 openArray("securityLabel"); 15073 for (Coding e : element.getSecurityLabel()) 15074 composeCoding(null, e); 15075 closeArray(); 15076 } 15077 ; 15078 if (element.hasNameElement()) { 15079 composeStringCore("name", element.getNameElement(), false); 15080 composeStringExtras("name", element.getNameElement(), false); 15081 } 15082 if (element.hasDescriptionElement()) { 15083 composeStringCore("description", element.getDescriptionElement(), false); 15084 composeStringExtras("description", element.getDescriptionElement(), false); 15085 } 15086 if (element.hasQueryElement()) { 15087 composeBase64BinaryCore("query", element.getQueryElement(), false); 15088 composeBase64BinaryExtras("query", element.getQueryElement(), false); 15089 } 15090 if (element.hasDetail()) { 15091 openArray("detail"); 15092 for (AuditEvent.AuditEventObjectDetailComponent e : element.getDetail()) 15093 composeAuditEventAuditEventObjectDetailComponent(null, e); 15094 closeArray(); 15095 } 15096 ; 15097 } 15098 15099 protected void composeAuditEventAuditEventObjectDetailComponent(String name, 15100 AuditEvent.AuditEventObjectDetailComponent element) throws IOException { 15101 if (element != null) { 15102 open(name); 15103 composeAuditEventAuditEventObjectDetailComponentInner(element); 15104 close(); 15105 } 15106 } 15107 15108 protected void composeAuditEventAuditEventObjectDetailComponentInner( 15109 AuditEvent.AuditEventObjectDetailComponent element) throws IOException { 15110 composeBackbone(element); 15111 if (element.hasTypeElement()) { 15112 composeStringCore("type", element.getTypeElement(), false); 15113 composeStringExtras("type", element.getTypeElement(), false); 15114 } 15115 if (element.hasValueElement()) { 15116 composeBase64BinaryCore("value", element.getValueElement(), false); 15117 composeBase64BinaryExtras("value", element.getValueElement(), false); 15118 } 15119 } 15120 15121 protected void composeBasic(String name, Basic element) throws IOException { 15122 if (element != null) { 15123 prop("resourceType", name); 15124 composeBasicInner(element); 15125 } 15126 } 15127 15128 protected void composeBasicInner(Basic element) throws IOException { 15129 composeDomainResourceElements(element); 15130 if (element.hasIdentifier()) { 15131 openArray("identifier"); 15132 for (Identifier e : element.getIdentifier()) 15133 composeIdentifier(null, e); 15134 closeArray(); 15135 } 15136 ; 15137 if (element.hasCode()) { 15138 composeCodeableConcept("code", element.getCode()); 15139 } 15140 if (element.hasSubject()) { 15141 composeReference("subject", element.getSubject()); 15142 } 15143 if (element.hasAuthor()) { 15144 composeReference("author", element.getAuthor()); 15145 } 15146 if (element.hasCreatedElement()) { 15147 composeDateCore("created", element.getCreatedElement(), false); 15148 composeDateExtras("created", element.getCreatedElement(), false); 15149 } 15150 } 15151 15152 protected void composeBinary(String name, Binary element) throws IOException { 15153 if (element != null) { 15154 prop("resourceType", name); 15155 composeBinaryInner(element); 15156 } 15157 } 15158 15159 protected void composeBinaryInner(Binary element) throws IOException { 15160 composeResourceElements(element); 15161 if (element.hasContentTypeElement()) { 15162 composeCodeCore("contentType", element.getContentTypeElement(), false); 15163 composeCodeExtras("contentType", element.getContentTypeElement(), false); 15164 } 15165 if (element.hasContentElement()) { 15166 composeBase64BinaryCore("content", element.getContentElement(), false); 15167 composeBase64BinaryExtras("content", element.getContentElement(), false); 15168 } 15169 } 15170 15171 protected void composeBodySite(String name, BodySite element) throws IOException { 15172 if (element != null) { 15173 prop("resourceType", name); 15174 composeBodySiteInner(element); 15175 } 15176 } 15177 15178 protected void composeBodySiteInner(BodySite element) throws IOException { 15179 composeDomainResourceElements(element); 15180 if (element.hasPatient()) { 15181 composeReference("patient", element.getPatient()); 15182 } 15183 if (element.hasIdentifier()) { 15184 openArray("identifier"); 15185 for (Identifier e : element.getIdentifier()) 15186 composeIdentifier(null, e); 15187 closeArray(); 15188 } 15189 ; 15190 if (element.hasCode()) { 15191 composeCodeableConcept("code", element.getCode()); 15192 } 15193 if (element.hasModifier()) { 15194 openArray("modifier"); 15195 for (CodeableConcept e : element.getModifier()) 15196 composeCodeableConcept(null, e); 15197 closeArray(); 15198 } 15199 ; 15200 if (element.hasDescriptionElement()) { 15201 composeStringCore("description", element.getDescriptionElement(), false); 15202 composeStringExtras("description", element.getDescriptionElement(), false); 15203 } 15204 if (element.hasImage()) { 15205 openArray("image"); 15206 for (Attachment e : element.getImage()) 15207 composeAttachment(null, e); 15208 closeArray(); 15209 } 15210 ; 15211 } 15212 15213 protected void composeBundle(String name, Bundle element) throws IOException { 15214 if (element != null) { 15215 prop("resourceType", name); 15216 composeBundleInner(element); 15217 } 15218 } 15219 15220 protected void composeBundleInner(Bundle element) throws IOException { 15221 composeResourceElements(element); 15222 if (element.hasTypeElement()) { 15223 composeEnumerationCore("type", element.getTypeElement(), new Bundle.BundleTypeEnumFactory(), false); 15224 composeEnumerationExtras("type", element.getTypeElement(), new Bundle.BundleTypeEnumFactory(), false); 15225 } 15226 if (element.hasTotalElement()) { 15227 composeUnsignedIntCore("total", element.getTotalElement(), false); 15228 composeUnsignedIntExtras("total", element.getTotalElement(), false); 15229 } 15230 if (element.hasLink()) { 15231 openArray("link"); 15232 for (Bundle.BundleLinkComponent e : element.getLink()) 15233 composeBundleBundleLinkComponent(null, e); 15234 closeArray(); 15235 } 15236 ; 15237 if (element.hasEntry()) { 15238 openArray("entry"); 15239 for (Bundle.BundleEntryComponent e : element.getEntry()) 15240 composeBundleBundleEntryComponent(null, e); 15241 closeArray(); 15242 } 15243 ; 15244 if (element.hasSignature()) { 15245 composeSignature("signature", element.getSignature()); 15246 } 15247 } 15248 15249 protected void composeBundleBundleLinkComponent(String name, Bundle.BundleLinkComponent element) throws IOException { 15250 if (element != null) { 15251 open(name); 15252 composeBundleBundleLinkComponentInner(element); 15253 close(); 15254 } 15255 } 15256 15257 protected void composeBundleBundleLinkComponentInner(Bundle.BundleLinkComponent element) throws IOException { 15258 composeBackbone(element); 15259 if (element.hasRelationElement()) { 15260 composeStringCore("relation", element.getRelationElement(), false); 15261 composeStringExtras("relation", element.getRelationElement(), false); 15262 } 15263 if (element.hasUrlElement()) { 15264 composeUriCore("url", element.getUrlElement(), false); 15265 composeUriExtras("url", element.getUrlElement(), false); 15266 } 15267 } 15268 15269 protected void composeBundleBundleEntryComponent(String name, Bundle.BundleEntryComponent element) 15270 throws IOException { 15271 if (element != null) { 15272 open(name); 15273 composeBundleBundleEntryComponentInner(element); 15274 close(); 15275 } 15276 } 15277 15278 protected void composeBundleBundleEntryComponentInner(Bundle.BundleEntryComponent element) throws IOException { 15279 composeBackbone(element); 15280 if (element.hasLink()) { 15281 openArray("link"); 15282 for (Bundle.BundleLinkComponent e : element.getLink()) 15283 composeBundleBundleLinkComponent(null, e); 15284 closeArray(); 15285 } 15286 ; 15287 if (element.hasFullUrlElement()) { 15288 composeUriCore("fullUrl", element.getFullUrlElement(), false); 15289 composeUriExtras("fullUrl", element.getFullUrlElement(), false); 15290 } 15291 if (element.hasResource()) { 15292 open("resource"); 15293 composeResource(element.getResource()); 15294 close(); 15295 } 15296 if (element.hasSearch()) { 15297 composeBundleBundleEntrySearchComponent("search", element.getSearch()); 15298 } 15299 if (element.hasRequest()) { 15300 composeBundleBundleEntryRequestComponent("request", element.getRequest()); 15301 } 15302 if (element.hasResponse()) { 15303 composeBundleBundleEntryResponseComponent("response", element.getResponse()); 15304 } 15305 } 15306 15307 protected void composeBundleBundleEntrySearchComponent(String name, Bundle.BundleEntrySearchComponent element) 15308 throws IOException { 15309 if (element != null) { 15310 open(name); 15311 composeBundleBundleEntrySearchComponentInner(element); 15312 close(); 15313 } 15314 } 15315 15316 protected void composeBundleBundleEntrySearchComponentInner(Bundle.BundleEntrySearchComponent element) 15317 throws IOException { 15318 composeBackbone(element); 15319 if (element.hasModeElement()) { 15320 composeEnumerationCore("mode", element.getModeElement(), new Bundle.SearchEntryModeEnumFactory(), false); 15321 composeEnumerationExtras("mode", element.getModeElement(), new Bundle.SearchEntryModeEnumFactory(), false); 15322 } 15323 if (element.hasScoreElement()) { 15324 composeDecimalCore("score", element.getScoreElement(), false); 15325 composeDecimalExtras("score", element.getScoreElement(), false); 15326 } 15327 } 15328 15329 protected void composeBundleBundleEntryRequestComponent(String name, Bundle.BundleEntryRequestComponent element) 15330 throws IOException { 15331 if (element != null) { 15332 open(name); 15333 composeBundleBundleEntryRequestComponentInner(element); 15334 close(); 15335 } 15336 } 15337 15338 protected void composeBundleBundleEntryRequestComponentInner(Bundle.BundleEntryRequestComponent element) 15339 throws IOException { 15340 composeBackbone(element); 15341 if (element.hasMethodElement()) { 15342 composeEnumerationCore("method", element.getMethodElement(), new Bundle.HTTPVerbEnumFactory(), false); 15343 composeEnumerationExtras("method", element.getMethodElement(), new Bundle.HTTPVerbEnumFactory(), false); 15344 } 15345 if (element.hasUrlElement()) { 15346 composeUriCore("url", element.getUrlElement(), false); 15347 composeUriExtras("url", element.getUrlElement(), false); 15348 } 15349 if (element.hasIfNoneMatchElement()) { 15350 composeStringCore("ifNoneMatch", element.getIfNoneMatchElement(), false); 15351 composeStringExtras("ifNoneMatch", element.getIfNoneMatchElement(), false); 15352 } 15353 if (element.hasIfModifiedSinceElement()) { 15354 composeInstantCore("ifModifiedSince", element.getIfModifiedSinceElement(), false); 15355 composeInstantExtras("ifModifiedSince", element.getIfModifiedSinceElement(), false); 15356 } 15357 if (element.hasIfMatchElement()) { 15358 composeStringCore("ifMatch", element.getIfMatchElement(), false); 15359 composeStringExtras("ifMatch", element.getIfMatchElement(), false); 15360 } 15361 if (element.hasIfNoneExistElement()) { 15362 composeStringCore("ifNoneExist", element.getIfNoneExistElement(), false); 15363 composeStringExtras("ifNoneExist", element.getIfNoneExistElement(), false); 15364 } 15365 } 15366 15367 protected void composeBundleBundleEntryResponseComponent(String name, Bundle.BundleEntryResponseComponent element) 15368 throws IOException { 15369 if (element != null) { 15370 open(name); 15371 composeBundleBundleEntryResponseComponentInner(element); 15372 close(); 15373 } 15374 } 15375 15376 protected void composeBundleBundleEntryResponseComponentInner(Bundle.BundleEntryResponseComponent element) 15377 throws IOException { 15378 composeBackbone(element); 15379 if (element.hasStatusElement()) { 15380 composeStringCore("status", element.getStatusElement(), false); 15381 composeStringExtras("status", element.getStatusElement(), false); 15382 } 15383 if (element.hasLocationElement()) { 15384 composeUriCore("location", element.getLocationElement(), false); 15385 composeUriExtras("location", element.getLocationElement(), false); 15386 } 15387 if (element.hasEtagElement()) { 15388 composeStringCore("etag", element.getEtagElement(), false); 15389 composeStringExtras("etag", element.getEtagElement(), false); 15390 } 15391 if (element.hasLastModifiedElement()) { 15392 composeInstantCore("lastModified", element.getLastModifiedElement(), false); 15393 composeInstantExtras("lastModified", element.getLastModifiedElement(), false); 15394 } 15395 } 15396 15397 protected void composeCarePlan(String name, CarePlan element) throws IOException { 15398 if (element != null) { 15399 prop("resourceType", name); 15400 composeCarePlanInner(element); 15401 } 15402 } 15403 15404 protected void composeCarePlanInner(CarePlan element) throws IOException { 15405 composeDomainResourceElements(element); 15406 if (element.hasIdentifier()) { 15407 openArray("identifier"); 15408 for (Identifier e : element.getIdentifier()) 15409 composeIdentifier(null, e); 15410 closeArray(); 15411 } 15412 ; 15413 if (element.hasSubject()) { 15414 composeReference("subject", element.getSubject()); 15415 } 15416 if (element.hasStatusElement()) { 15417 composeEnumerationCore("status", element.getStatusElement(), new CarePlan.CarePlanStatusEnumFactory(), false); 15418 composeEnumerationExtras("status", element.getStatusElement(), new CarePlan.CarePlanStatusEnumFactory(), false); 15419 } 15420 if (element.hasContext()) { 15421 composeReference("context", element.getContext()); 15422 } 15423 if (element.hasPeriod()) { 15424 composePeriod("period", element.getPeriod()); 15425 } 15426 if (element.hasAuthor()) { 15427 openArray("author"); 15428 for (Reference e : element.getAuthor()) 15429 composeReference(null, e); 15430 closeArray(); 15431 } 15432 ; 15433 if (element.hasModifiedElement()) { 15434 composeDateTimeCore("modified", element.getModifiedElement(), false); 15435 composeDateTimeExtras("modified", element.getModifiedElement(), false); 15436 } 15437 if (element.hasCategory()) { 15438 openArray("category"); 15439 for (CodeableConcept e : element.getCategory()) 15440 composeCodeableConcept(null, e); 15441 closeArray(); 15442 } 15443 ; 15444 if (element.hasDescriptionElement()) { 15445 composeStringCore("description", element.getDescriptionElement(), false); 15446 composeStringExtras("description", element.getDescriptionElement(), false); 15447 } 15448 if (element.hasAddresses()) { 15449 openArray("addresses"); 15450 for (Reference e : element.getAddresses()) 15451 composeReference(null, e); 15452 closeArray(); 15453 } 15454 ; 15455 if (element.hasSupport()) { 15456 openArray("support"); 15457 for (Reference e : element.getSupport()) 15458 composeReference(null, e); 15459 closeArray(); 15460 } 15461 ; 15462 if (element.hasRelatedPlan()) { 15463 openArray("relatedPlan"); 15464 for (CarePlan.CarePlanRelatedPlanComponent e : element.getRelatedPlan()) 15465 composeCarePlanCarePlanRelatedPlanComponent(null, e); 15466 closeArray(); 15467 } 15468 ; 15469 if (element.hasParticipant()) { 15470 openArray("participant"); 15471 for (CarePlan.CarePlanParticipantComponent e : element.getParticipant()) 15472 composeCarePlanCarePlanParticipantComponent(null, e); 15473 closeArray(); 15474 } 15475 ; 15476 if (element.hasGoal()) { 15477 openArray("goal"); 15478 for (Reference e : element.getGoal()) 15479 composeReference(null, e); 15480 closeArray(); 15481 } 15482 ; 15483 if (element.hasActivity()) { 15484 openArray("activity"); 15485 for (CarePlan.CarePlanActivityComponent e : element.getActivity()) 15486 composeCarePlanCarePlanActivityComponent(null, e); 15487 closeArray(); 15488 } 15489 ; 15490 if (element.hasNote()) { 15491 composeAnnotation("note", element.getNote()); 15492 } 15493 } 15494 15495 protected void composeCarePlanCarePlanRelatedPlanComponent(String name, CarePlan.CarePlanRelatedPlanComponent element) 15496 throws IOException { 15497 if (element != null) { 15498 open(name); 15499 composeCarePlanCarePlanRelatedPlanComponentInner(element); 15500 close(); 15501 } 15502 } 15503 15504 protected void composeCarePlanCarePlanRelatedPlanComponentInner(CarePlan.CarePlanRelatedPlanComponent element) 15505 throws IOException { 15506 composeBackbone(element); 15507 if (element.hasCodeElement()) { 15508 composeEnumerationCore("code", element.getCodeElement(), new CarePlan.CarePlanRelationshipEnumFactory(), false); 15509 composeEnumerationExtras("code", element.getCodeElement(), new CarePlan.CarePlanRelationshipEnumFactory(), false); 15510 } 15511 if (element.hasPlan()) { 15512 composeReference("plan", element.getPlan()); 15513 } 15514 } 15515 15516 protected void composeCarePlanCarePlanParticipantComponent(String name, CarePlan.CarePlanParticipantComponent element) 15517 throws IOException { 15518 if (element != null) { 15519 open(name); 15520 composeCarePlanCarePlanParticipantComponentInner(element); 15521 close(); 15522 } 15523 } 15524 15525 protected void composeCarePlanCarePlanParticipantComponentInner(CarePlan.CarePlanParticipantComponent element) 15526 throws IOException { 15527 composeBackbone(element); 15528 if (element.hasRole()) { 15529 composeCodeableConcept("role", element.getRole()); 15530 } 15531 if (element.hasMember()) { 15532 composeReference("member", element.getMember()); 15533 } 15534 } 15535 15536 protected void composeCarePlanCarePlanActivityComponent(String name, CarePlan.CarePlanActivityComponent element) 15537 throws IOException { 15538 if (element != null) { 15539 open(name); 15540 composeCarePlanCarePlanActivityComponentInner(element); 15541 close(); 15542 } 15543 } 15544 15545 protected void composeCarePlanCarePlanActivityComponentInner(CarePlan.CarePlanActivityComponent element) 15546 throws IOException { 15547 composeBackbone(element); 15548 if (element.hasActionResulting()) { 15549 openArray("actionResulting"); 15550 for (Reference e : element.getActionResulting()) 15551 composeReference(null, e); 15552 closeArray(); 15553 } 15554 ; 15555 if (element.hasProgress()) { 15556 openArray("progress"); 15557 for (Annotation e : element.getProgress()) 15558 composeAnnotation(null, e); 15559 closeArray(); 15560 } 15561 ; 15562 if (element.hasReference()) { 15563 composeReference("reference", element.getReference()); 15564 } 15565 if (element.hasDetail()) { 15566 composeCarePlanCarePlanActivityDetailComponent("detail", element.getDetail()); 15567 } 15568 } 15569 15570 protected void composeCarePlanCarePlanActivityDetailComponent(String name, 15571 CarePlan.CarePlanActivityDetailComponent element) throws IOException { 15572 if (element != null) { 15573 open(name); 15574 composeCarePlanCarePlanActivityDetailComponentInner(element); 15575 close(); 15576 } 15577 } 15578 15579 protected void composeCarePlanCarePlanActivityDetailComponentInner(CarePlan.CarePlanActivityDetailComponent element) 15580 throws IOException { 15581 composeBackbone(element); 15582 if (element.hasCategory()) { 15583 composeCodeableConcept("category", element.getCategory()); 15584 } 15585 if (element.hasCode()) { 15586 composeCodeableConcept("code", element.getCode()); 15587 } 15588 if (element.hasReasonCode()) { 15589 openArray("reasonCode"); 15590 for (CodeableConcept e : element.getReasonCode()) 15591 composeCodeableConcept(null, e); 15592 closeArray(); 15593 } 15594 ; 15595 if (element.hasReasonReference()) { 15596 openArray("reasonReference"); 15597 for (Reference e : element.getReasonReference()) 15598 composeReference(null, e); 15599 closeArray(); 15600 } 15601 ; 15602 if (element.hasGoal()) { 15603 openArray("goal"); 15604 for (Reference e : element.getGoal()) 15605 composeReference(null, e); 15606 closeArray(); 15607 } 15608 ; 15609 if (element.hasStatusElement()) { 15610 composeEnumerationCore("status", element.getStatusElement(), new CarePlan.CarePlanActivityStatusEnumFactory(), 15611 false); 15612 composeEnumerationExtras("status", element.getStatusElement(), new CarePlan.CarePlanActivityStatusEnumFactory(), 15613 false); 15614 } 15615 if (element.hasStatusReason()) { 15616 composeCodeableConcept("statusReason", element.getStatusReason()); 15617 } 15618 if (element.hasProhibitedElement()) { 15619 composeBooleanCore("prohibited", element.getProhibitedElement(), false); 15620 composeBooleanExtras("prohibited", element.getProhibitedElement(), false); 15621 } 15622 if (element.hasScheduled()) { 15623 composeType("scheduled", element.getScheduled()); 15624 } 15625 if (element.hasLocation()) { 15626 composeReference("location", element.getLocation()); 15627 } 15628 if (element.hasPerformer()) { 15629 openArray("performer"); 15630 for (Reference e : element.getPerformer()) 15631 composeReference(null, e); 15632 closeArray(); 15633 } 15634 ; 15635 if (element.hasProduct()) { 15636 composeType("product", element.getProduct()); 15637 } 15638 if (element.hasDailyAmount()) { 15639 composeSimpleQuantity("dailyAmount", element.getDailyAmount()); 15640 } 15641 if (element.hasQuantity()) { 15642 composeSimpleQuantity("quantity", element.getQuantity()); 15643 } 15644 if (element.hasDescriptionElement()) { 15645 composeStringCore("description", element.getDescriptionElement(), false); 15646 composeStringExtras("description", element.getDescriptionElement(), false); 15647 } 15648 } 15649 15650 protected void composeClaim(String name, Claim element) throws IOException { 15651 if (element != null) { 15652 prop("resourceType", name); 15653 composeClaimInner(element); 15654 } 15655 } 15656 15657 protected void composeClaimInner(Claim element) throws IOException { 15658 composeDomainResourceElements(element); 15659 if (element.hasTypeElement()) { 15660 composeEnumerationCore("type", element.getTypeElement(), new Claim.ClaimTypeEnumFactory(), false); 15661 composeEnumerationExtras("type", element.getTypeElement(), new Claim.ClaimTypeEnumFactory(), false); 15662 } 15663 if (element.hasIdentifier()) { 15664 openArray("identifier"); 15665 for (Identifier e : element.getIdentifier()) 15666 composeIdentifier(null, e); 15667 closeArray(); 15668 } 15669 ; 15670 if (element.hasRuleset()) { 15671 composeCoding("ruleset", element.getRuleset()); 15672 } 15673 if (element.hasOriginalRuleset()) { 15674 composeCoding("originalRuleset", element.getOriginalRuleset()); 15675 } 15676 if (element.hasCreatedElement()) { 15677 composeDateTimeCore("created", element.getCreatedElement(), false); 15678 composeDateTimeExtras("created", element.getCreatedElement(), false); 15679 } 15680 if (element.hasTarget()) { 15681 composeReference("target", element.getTarget()); 15682 } 15683 if (element.hasProvider()) { 15684 composeReference("provider", element.getProvider()); 15685 } 15686 if (element.hasOrganization()) { 15687 composeReference("organization", element.getOrganization()); 15688 } 15689 if (element.hasUseElement()) { 15690 composeEnumerationCore("use", element.getUseElement(), new Claim.UseEnumFactory(), false); 15691 composeEnumerationExtras("use", element.getUseElement(), new Claim.UseEnumFactory(), false); 15692 } 15693 if (element.hasPriority()) { 15694 composeCoding("priority", element.getPriority()); 15695 } 15696 if (element.hasFundsReserve()) { 15697 composeCoding("fundsReserve", element.getFundsReserve()); 15698 } 15699 if (element.hasEnterer()) { 15700 composeReference("enterer", element.getEnterer()); 15701 } 15702 if (element.hasFacility()) { 15703 composeReference("facility", element.getFacility()); 15704 } 15705 if (element.hasPrescription()) { 15706 composeReference("prescription", element.getPrescription()); 15707 } 15708 if (element.hasOriginalPrescription()) { 15709 composeReference("originalPrescription", element.getOriginalPrescription()); 15710 } 15711 if (element.hasPayee()) { 15712 composeClaimPayeeComponent("payee", element.getPayee()); 15713 } 15714 if (element.hasReferral()) { 15715 composeReference("referral", element.getReferral()); 15716 } 15717 if (element.hasDiagnosis()) { 15718 openArray("diagnosis"); 15719 for (Claim.DiagnosisComponent e : element.getDiagnosis()) 15720 composeClaimDiagnosisComponent(null, e); 15721 closeArray(); 15722 } 15723 ; 15724 if (element.hasCondition()) { 15725 openArray("condition"); 15726 for (Coding e : element.getCondition()) 15727 composeCoding(null, e); 15728 closeArray(); 15729 } 15730 ; 15731 if (element.hasPatient()) { 15732 composeReference("patient", element.getPatient()); 15733 } 15734 if (element.hasCoverage()) { 15735 openArray("coverage"); 15736 for (Claim.CoverageComponent e : element.getCoverage()) 15737 composeClaimCoverageComponent(null, e); 15738 closeArray(); 15739 } 15740 ; 15741 if (element.hasException()) { 15742 openArray("exception"); 15743 for (Coding e : element.getException()) 15744 composeCoding(null, e); 15745 closeArray(); 15746 } 15747 ; 15748 if (element.hasSchoolElement()) { 15749 composeStringCore("school", element.getSchoolElement(), false); 15750 composeStringExtras("school", element.getSchoolElement(), false); 15751 } 15752 if (element.hasAccidentElement()) { 15753 composeDateCore("accident", element.getAccidentElement(), false); 15754 composeDateExtras("accident", element.getAccidentElement(), false); 15755 } 15756 if (element.hasAccidentType()) { 15757 composeCoding("accidentType", element.getAccidentType()); 15758 } 15759 if (element.hasInterventionException()) { 15760 openArray("interventionException"); 15761 for (Coding e : element.getInterventionException()) 15762 composeCoding(null, e); 15763 closeArray(); 15764 } 15765 ; 15766 if (element.hasItem()) { 15767 openArray("item"); 15768 for (Claim.ItemsComponent e : element.getItem()) 15769 composeClaimItemsComponent(null, e); 15770 closeArray(); 15771 } 15772 ; 15773 if (element.hasAdditionalMaterials()) { 15774 openArray("additionalMaterials"); 15775 for (Coding e : element.getAdditionalMaterials()) 15776 composeCoding(null, e); 15777 closeArray(); 15778 } 15779 ; 15780 if (element.hasMissingTeeth()) { 15781 openArray("missingTeeth"); 15782 for (Claim.MissingTeethComponent e : element.getMissingTeeth()) 15783 composeClaimMissingTeethComponent(null, e); 15784 closeArray(); 15785 } 15786 ; 15787 } 15788 15789 protected void composeClaimPayeeComponent(String name, Claim.PayeeComponent element) throws IOException { 15790 if (element != null) { 15791 open(name); 15792 composeClaimPayeeComponentInner(element); 15793 close(); 15794 } 15795 } 15796 15797 protected void composeClaimPayeeComponentInner(Claim.PayeeComponent element) throws IOException { 15798 composeBackbone(element); 15799 if (element.hasType()) { 15800 composeCoding("type", element.getType()); 15801 } 15802 if (element.hasProvider()) { 15803 composeReference("provider", element.getProvider()); 15804 } 15805 if (element.hasOrganization()) { 15806 composeReference("organization", element.getOrganization()); 15807 } 15808 if (element.hasPerson()) { 15809 composeReference("person", element.getPerson()); 15810 } 15811 } 15812 15813 protected void composeClaimDiagnosisComponent(String name, Claim.DiagnosisComponent element) throws IOException { 15814 if (element != null) { 15815 open(name); 15816 composeClaimDiagnosisComponentInner(element); 15817 close(); 15818 } 15819 } 15820 15821 protected void composeClaimDiagnosisComponentInner(Claim.DiagnosisComponent element) throws IOException { 15822 composeBackbone(element); 15823 if (element.hasSequenceElement()) { 15824 composePositiveIntCore("sequence", element.getSequenceElement(), false); 15825 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 15826 } 15827 if (element.hasDiagnosis()) { 15828 composeCoding("diagnosis", element.getDiagnosis()); 15829 } 15830 } 15831 15832 protected void composeClaimCoverageComponent(String name, Claim.CoverageComponent element) throws IOException { 15833 if (element != null) { 15834 open(name); 15835 composeClaimCoverageComponentInner(element); 15836 close(); 15837 } 15838 } 15839 15840 protected void composeClaimCoverageComponentInner(Claim.CoverageComponent element) throws IOException { 15841 composeBackbone(element); 15842 if (element.hasSequenceElement()) { 15843 composePositiveIntCore("sequence", element.getSequenceElement(), false); 15844 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 15845 } 15846 if (element.hasFocalElement()) { 15847 composeBooleanCore("focal", element.getFocalElement(), false); 15848 composeBooleanExtras("focal", element.getFocalElement(), false); 15849 } 15850 if (element.hasCoverage()) { 15851 composeReference("coverage", element.getCoverage()); 15852 } 15853 if (element.hasBusinessArrangementElement()) { 15854 composeStringCore("businessArrangement", element.getBusinessArrangementElement(), false); 15855 composeStringExtras("businessArrangement", element.getBusinessArrangementElement(), false); 15856 } 15857 if (element.hasRelationship()) { 15858 composeCoding("relationship", element.getRelationship()); 15859 } 15860 if (element.hasPreAuthRef()) { 15861 openArray("preAuthRef"); 15862 for (StringType e : element.getPreAuthRef()) 15863 composeStringCore(null, e, true); 15864 closeArray(); 15865 if (anyHasExtras(element.getPreAuthRef())) { 15866 openArray("_preAuthRef"); 15867 for (StringType e : element.getPreAuthRef()) 15868 composeStringExtras(null, e, true); 15869 closeArray(); 15870 } 15871 } 15872 ; 15873 if (element.hasClaimResponse()) { 15874 composeReference("claimResponse", element.getClaimResponse()); 15875 } 15876 if (element.hasOriginalRuleset()) { 15877 composeCoding("originalRuleset", element.getOriginalRuleset()); 15878 } 15879 } 15880 15881 protected void composeClaimItemsComponent(String name, Claim.ItemsComponent element) throws IOException { 15882 if (element != null) { 15883 open(name); 15884 composeClaimItemsComponentInner(element); 15885 close(); 15886 } 15887 } 15888 15889 protected void composeClaimItemsComponentInner(Claim.ItemsComponent element) throws IOException { 15890 composeBackbone(element); 15891 if (element.hasSequenceElement()) { 15892 composePositiveIntCore("sequence", element.getSequenceElement(), false); 15893 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 15894 } 15895 if (element.hasType()) { 15896 composeCoding("type", element.getType()); 15897 } 15898 if (element.hasProvider()) { 15899 composeReference("provider", element.getProvider()); 15900 } 15901 if (element.hasDiagnosisLinkId()) { 15902 openArray("diagnosisLinkId"); 15903 for (PositiveIntType e : element.getDiagnosisLinkId()) 15904 composePositiveIntCore(null, e, true); 15905 closeArray(); 15906 if (anyHasExtras(element.getDiagnosisLinkId())) { 15907 openArray("_diagnosisLinkId"); 15908 for (PositiveIntType e : element.getDiagnosisLinkId()) 15909 composePositiveIntExtras(null, e, true); 15910 closeArray(); 15911 } 15912 } 15913 ; 15914 if (element.hasService()) { 15915 composeCoding("service", element.getService()); 15916 } 15917 if (element.hasServiceDateElement()) { 15918 composeDateCore("serviceDate", element.getServiceDateElement(), false); 15919 composeDateExtras("serviceDate", element.getServiceDateElement(), false); 15920 } 15921 if (element.hasQuantity()) { 15922 composeSimpleQuantity("quantity", element.getQuantity()); 15923 } 15924 if (element.hasUnitPrice()) { 15925 composeMoney("unitPrice", element.getUnitPrice()); 15926 } 15927 if (element.hasFactorElement()) { 15928 composeDecimalCore("factor", element.getFactorElement(), false); 15929 composeDecimalExtras("factor", element.getFactorElement(), false); 15930 } 15931 if (element.hasPointsElement()) { 15932 composeDecimalCore("points", element.getPointsElement(), false); 15933 composeDecimalExtras("points", element.getPointsElement(), false); 15934 } 15935 if (element.hasNet()) { 15936 composeMoney("net", element.getNet()); 15937 } 15938 if (element.hasUdi()) { 15939 composeCoding("udi", element.getUdi()); 15940 } 15941 if (element.hasBodySite()) { 15942 composeCoding("bodySite", element.getBodySite()); 15943 } 15944 if (element.hasSubSite()) { 15945 openArray("subSite"); 15946 for (Coding e : element.getSubSite()) 15947 composeCoding(null, e); 15948 closeArray(); 15949 } 15950 ; 15951 if (element.hasModifier()) { 15952 openArray("modifier"); 15953 for (Coding e : element.getModifier()) 15954 composeCoding(null, e); 15955 closeArray(); 15956 } 15957 ; 15958 if (element.hasDetail()) { 15959 openArray("detail"); 15960 for (Claim.DetailComponent e : element.getDetail()) 15961 composeClaimDetailComponent(null, e); 15962 closeArray(); 15963 } 15964 ; 15965 if (element.hasProsthesis()) { 15966 composeClaimProsthesisComponent("prosthesis", element.getProsthesis()); 15967 } 15968 } 15969 15970 protected void composeClaimDetailComponent(String name, Claim.DetailComponent element) throws IOException { 15971 if (element != null) { 15972 open(name); 15973 composeClaimDetailComponentInner(element); 15974 close(); 15975 } 15976 } 15977 15978 protected void composeClaimDetailComponentInner(Claim.DetailComponent element) throws IOException { 15979 composeBackbone(element); 15980 if (element.hasSequenceElement()) { 15981 composePositiveIntCore("sequence", element.getSequenceElement(), false); 15982 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 15983 } 15984 if (element.hasType()) { 15985 composeCoding("type", element.getType()); 15986 } 15987 if (element.hasService()) { 15988 composeCoding("service", element.getService()); 15989 } 15990 if (element.hasQuantity()) { 15991 composeSimpleQuantity("quantity", element.getQuantity()); 15992 } 15993 if (element.hasUnitPrice()) { 15994 composeMoney("unitPrice", element.getUnitPrice()); 15995 } 15996 if (element.hasFactorElement()) { 15997 composeDecimalCore("factor", element.getFactorElement(), false); 15998 composeDecimalExtras("factor", element.getFactorElement(), false); 15999 } 16000 if (element.hasPointsElement()) { 16001 composeDecimalCore("points", element.getPointsElement(), false); 16002 composeDecimalExtras("points", element.getPointsElement(), false); 16003 } 16004 if (element.hasNet()) { 16005 composeMoney("net", element.getNet()); 16006 } 16007 if (element.hasUdi()) { 16008 composeCoding("udi", element.getUdi()); 16009 } 16010 if (element.hasSubDetail()) { 16011 openArray("subDetail"); 16012 for (Claim.SubDetailComponent e : element.getSubDetail()) 16013 composeClaimSubDetailComponent(null, e); 16014 closeArray(); 16015 } 16016 ; 16017 } 16018 16019 protected void composeClaimSubDetailComponent(String name, Claim.SubDetailComponent element) throws IOException { 16020 if (element != null) { 16021 open(name); 16022 composeClaimSubDetailComponentInner(element); 16023 close(); 16024 } 16025 } 16026 16027 protected void composeClaimSubDetailComponentInner(Claim.SubDetailComponent element) throws IOException { 16028 composeBackbone(element); 16029 if (element.hasSequenceElement()) { 16030 composePositiveIntCore("sequence", element.getSequenceElement(), false); 16031 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 16032 } 16033 if (element.hasType()) { 16034 composeCoding("type", element.getType()); 16035 } 16036 if (element.hasService()) { 16037 composeCoding("service", element.getService()); 16038 } 16039 if (element.hasQuantity()) { 16040 composeSimpleQuantity("quantity", element.getQuantity()); 16041 } 16042 if (element.hasUnitPrice()) { 16043 composeMoney("unitPrice", element.getUnitPrice()); 16044 } 16045 if (element.hasFactorElement()) { 16046 composeDecimalCore("factor", element.getFactorElement(), false); 16047 composeDecimalExtras("factor", element.getFactorElement(), false); 16048 } 16049 if (element.hasPointsElement()) { 16050 composeDecimalCore("points", element.getPointsElement(), false); 16051 composeDecimalExtras("points", element.getPointsElement(), false); 16052 } 16053 if (element.hasNet()) { 16054 composeMoney("net", element.getNet()); 16055 } 16056 if (element.hasUdi()) { 16057 composeCoding("udi", element.getUdi()); 16058 } 16059 } 16060 16061 protected void composeClaimProsthesisComponent(String name, Claim.ProsthesisComponent element) throws IOException { 16062 if (element != null) { 16063 open(name); 16064 composeClaimProsthesisComponentInner(element); 16065 close(); 16066 } 16067 } 16068 16069 protected void composeClaimProsthesisComponentInner(Claim.ProsthesisComponent element) throws IOException { 16070 composeBackbone(element); 16071 if (element.hasInitialElement()) { 16072 composeBooleanCore("initial", element.getInitialElement(), false); 16073 composeBooleanExtras("initial", element.getInitialElement(), false); 16074 } 16075 if (element.hasPriorDateElement()) { 16076 composeDateCore("priorDate", element.getPriorDateElement(), false); 16077 composeDateExtras("priorDate", element.getPriorDateElement(), false); 16078 } 16079 if (element.hasPriorMaterial()) { 16080 composeCoding("priorMaterial", element.getPriorMaterial()); 16081 } 16082 } 16083 16084 protected void composeClaimMissingTeethComponent(String name, Claim.MissingTeethComponent element) 16085 throws IOException { 16086 if (element != null) { 16087 open(name); 16088 composeClaimMissingTeethComponentInner(element); 16089 close(); 16090 } 16091 } 16092 16093 protected void composeClaimMissingTeethComponentInner(Claim.MissingTeethComponent element) throws IOException { 16094 composeBackbone(element); 16095 if (element.hasTooth()) { 16096 composeCoding("tooth", element.getTooth()); 16097 } 16098 if (element.hasReason()) { 16099 composeCoding("reason", element.getReason()); 16100 } 16101 if (element.hasExtractionDateElement()) { 16102 composeDateCore("extractionDate", element.getExtractionDateElement(), false); 16103 composeDateExtras("extractionDate", element.getExtractionDateElement(), false); 16104 } 16105 } 16106 16107 protected void composeClaimResponse(String name, ClaimResponse element) throws IOException { 16108 if (element != null) { 16109 prop("resourceType", name); 16110 composeClaimResponseInner(element); 16111 } 16112 } 16113 16114 protected void composeClaimResponseInner(ClaimResponse element) throws IOException { 16115 composeDomainResourceElements(element); 16116 if (element.hasIdentifier()) { 16117 openArray("identifier"); 16118 for (Identifier e : element.getIdentifier()) 16119 composeIdentifier(null, e); 16120 closeArray(); 16121 } 16122 ; 16123 if (element.hasRequest()) { 16124 composeReference("request", element.getRequest()); 16125 } 16126 if (element.hasRuleset()) { 16127 composeCoding("ruleset", element.getRuleset()); 16128 } 16129 if (element.hasOriginalRuleset()) { 16130 composeCoding("originalRuleset", element.getOriginalRuleset()); 16131 } 16132 if (element.hasCreatedElement()) { 16133 composeDateTimeCore("created", element.getCreatedElement(), false); 16134 composeDateTimeExtras("created", element.getCreatedElement(), false); 16135 } 16136 if (element.hasOrganization()) { 16137 composeReference("organization", element.getOrganization()); 16138 } 16139 if (element.hasRequestProvider()) { 16140 composeReference("requestProvider", element.getRequestProvider()); 16141 } 16142 if (element.hasRequestOrganization()) { 16143 composeReference("requestOrganization", element.getRequestOrganization()); 16144 } 16145 if (element.hasOutcomeElement()) { 16146 composeEnumerationCore("outcome", element.getOutcomeElement(), new Enumerations.RemittanceOutcomeEnumFactory(), 16147 false); 16148 composeEnumerationExtras("outcome", element.getOutcomeElement(), new Enumerations.RemittanceOutcomeEnumFactory(), 16149 false); 16150 } 16151 if (element.hasDispositionElement()) { 16152 composeStringCore("disposition", element.getDispositionElement(), false); 16153 composeStringExtras("disposition", element.getDispositionElement(), false); 16154 } 16155 if (element.hasPayeeType()) { 16156 composeCoding("payeeType", element.getPayeeType()); 16157 } 16158 if (element.hasItem()) { 16159 openArray("item"); 16160 for (ClaimResponse.ItemsComponent e : element.getItem()) 16161 composeClaimResponseItemsComponent(null, e); 16162 closeArray(); 16163 } 16164 ; 16165 if (element.hasAddItem()) { 16166 openArray("addItem"); 16167 for (ClaimResponse.AddedItemComponent e : element.getAddItem()) 16168 composeClaimResponseAddedItemComponent(null, e); 16169 closeArray(); 16170 } 16171 ; 16172 if (element.hasError()) { 16173 openArray("error"); 16174 for (ClaimResponse.ErrorsComponent e : element.getError()) 16175 composeClaimResponseErrorsComponent(null, e); 16176 closeArray(); 16177 } 16178 ; 16179 if (element.hasTotalCost()) { 16180 composeMoney("totalCost", element.getTotalCost()); 16181 } 16182 if (element.hasUnallocDeductable()) { 16183 composeMoney("unallocDeductable", element.getUnallocDeductable()); 16184 } 16185 if (element.hasTotalBenefit()) { 16186 composeMoney("totalBenefit", element.getTotalBenefit()); 16187 } 16188 if (element.hasPaymentAdjustment()) { 16189 composeMoney("paymentAdjustment", element.getPaymentAdjustment()); 16190 } 16191 if (element.hasPaymentAdjustmentReason()) { 16192 composeCoding("paymentAdjustmentReason", element.getPaymentAdjustmentReason()); 16193 } 16194 if (element.hasPaymentDateElement()) { 16195 composeDateCore("paymentDate", element.getPaymentDateElement(), false); 16196 composeDateExtras("paymentDate", element.getPaymentDateElement(), false); 16197 } 16198 if (element.hasPaymentAmount()) { 16199 composeMoney("paymentAmount", element.getPaymentAmount()); 16200 } 16201 if (element.hasPaymentRef()) { 16202 composeIdentifier("paymentRef", element.getPaymentRef()); 16203 } 16204 if (element.hasReserved()) { 16205 composeCoding("reserved", element.getReserved()); 16206 } 16207 if (element.hasForm()) { 16208 composeCoding("form", element.getForm()); 16209 } 16210 if (element.hasNote()) { 16211 openArray("note"); 16212 for (ClaimResponse.NotesComponent e : element.getNote()) 16213 composeClaimResponseNotesComponent(null, e); 16214 closeArray(); 16215 } 16216 ; 16217 if (element.hasCoverage()) { 16218 openArray("coverage"); 16219 for (ClaimResponse.CoverageComponent e : element.getCoverage()) 16220 composeClaimResponseCoverageComponent(null, e); 16221 closeArray(); 16222 } 16223 ; 16224 } 16225 16226 protected void composeClaimResponseItemsComponent(String name, ClaimResponse.ItemsComponent element) 16227 throws IOException { 16228 if (element != null) { 16229 open(name); 16230 composeClaimResponseItemsComponentInner(element); 16231 close(); 16232 } 16233 } 16234 16235 protected void composeClaimResponseItemsComponentInner(ClaimResponse.ItemsComponent element) throws IOException { 16236 composeBackbone(element); 16237 if (element.hasSequenceLinkIdElement()) { 16238 composePositiveIntCore("sequenceLinkId", element.getSequenceLinkIdElement(), false); 16239 composePositiveIntExtras("sequenceLinkId", element.getSequenceLinkIdElement(), false); 16240 } 16241 if (element.hasNoteNumber()) { 16242 openArray("noteNumber"); 16243 for (PositiveIntType e : element.getNoteNumber()) 16244 composePositiveIntCore(null, e, true); 16245 closeArray(); 16246 if (anyHasExtras(element.getNoteNumber())) { 16247 openArray("_noteNumber"); 16248 for (PositiveIntType e : element.getNoteNumber()) 16249 composePositiveIntExtras(null, e, true); 16250 closeArray(); 16251 } 16252 } 16253 ; 16254 if (element.hasAdjudication()) { 16255 openArray("adjudication"); 16256 for (ClaimResponse.ItemAdjudicationComponent e : element.getAdjudication()) 16257 composeClaimResponseItemAdjudicationComponent(null, e); 16258 closeArray(); 16259 } 16260 ; 16261 if (element.hasDetail()) { 16262 openArray("detail"); 16263 for (ClaimResponse.ItemDetailComponent e : element.getDetail()) 16264 composeClaimResponseItemDetailComponent(null, e); 16265 closeArray(); 16266 } 16267 ; 16268 } 16269 16270 protected void composeClaimResponseItemAdjudicationComponent(String name, 16271 ClaimResponse.ItemAdjudicationComponent element) throws IOException { 16272 if (element != null) { 16273 open(name); 16274 composeClaimResponseItemAdjudicationComponentInner(element); 16275 close(); 16276 } 16277 } 16278 16279 protected void composeClaimResponseItemAdjudicationComponentInner(ClaimResponse.ItemAdjudicationComponent element) 16280 throws IOException { 16281 composeBackbone(element); 16282 if (element.hasCode()) { 16283 composeCoding("code", element.getCode()); 16284 } 16285 if (element.hasAmount()) { 16286 composeMoney("amount", element.getAmount()); 16287 } 16288 if (element.hasValueElement()) { 16289 composeDecimalCore("value", element.getValueElement(), false); 16290 composeDecimalExtras("value", element.getValueElement(), false); 16291 } 16292 } 16293 16294 protected void composeClaimResponseItemDetailComponent(String name, ClaimResponse.ItemDetailComponent element) 16295 throws IOException { 16296 if (element != null) { 16297 open(name); 16298 composeClaimResponseItemDetailComponentInner(element); 16299 close(); 16300 } 16301 } 16302 16303 protected void composeClaimResponseItemDetailComponentInner(ClaimResponse.ItemDetailComponent element) 16304 throws IOException { 16305 composeBackbone(element); 16306 if (element.hasSequenceLinkIdElement()) { 16307 composePositiveIntCore("sequenceLinkId", element.getSequenceLinkIdElement(), false); 16308 composePositiveIntExtras("sequenceLinkId", element.getSequenceLinkIdElement(), false); 16309 } 16310 if (element.hasAdjudication()) { 16311 openArray("adjudication"); 16312 for (ClaimResponse.DetailAdjudicationComponent e : element.getAdjudication()) 16313 composeClaimResponseDetailAdjudicationComponent(null, e); 16314 closeArray(); 16315 } 16316 ; 16317 if (element.hasSubDetail()) { 16318 openArray("subDetail"); 16319 for (ClaimResponse.SubDetailComponent e : element.getSubDetail()) 16320 composeClaimResponseSubDetailComponent(null, e); 16321 closeArray(); 16322 } 16323 ; 16324 } 16325 16326 protected void composeClaimResponseDetailAdjudicationComponent(String name, 16327 ClaimResponse.DetailAdjudicationComponent element) throws IOException { 16328 if (element != null) { 16329 open(name); 16330 composeClaimResponseDetailAdjudicationComponentInner(element); 16331 close(); 16332 } 16333 } 16334 16335 protected void composeClaimResponseDetailAdjudicationComponentInner(ClaimResponse.DetailAdjudicationComponent element) 16336 throws IOException { 16337 composeBackbone(element); 16338 if (element.hasCode()) { 16339 composeCoding("code", element.getCode()); 16340 } 16341 if (element.hasAmount()) { 16342 composeMoney("amount", element.getAmount()); 16343 } 16344 if (element.hasValueElement()) { 16345 composeDecimalCore("value", element.getValueElement(), false); 16346 composeDecimalExtras("value", element.getValueElement(), false); 16347 } 16348 } 16349 16350 protected void composeClaimResponseSubDetailComponent(String name, ClaimResponse.SubDetailComponent element) 16351 throws IOException { 16352 if (element != null) { 16353 open(name); 16354 composeClaimResponseSubDetailComponentInner(element); 16355 close(); 16356 } 16357 } 16358 16359 protected void composeClaimResponseSubDetailComponentInner(ClaimResponse.SubDetailComponent element) 16360 throws IOException { 16361 composeBackbone(element); 16362 if (element.hasSequenceLinkIdElement()) { 16363 composePositiveIntCore("sequenceLinkId", element.getSequenceLinkIdElement(), false); 16364 composePositiveIntExtras("sequenceLinkId", element.getSequenceLinkIdElement(), false); 16365 } 16366 if (element.hasAdjudication()) { 16367 openArray("adjudication"); 16368 for (ClaimResponse.SubdetailAdjudicationComponent e : element.getAdjudication()) 16369 composeClaimResponseSubdetailAdjudicationComponent(null, e); 16370 closeArray(); 16371 } 16372 ; 16373 } 16374 16375 protected void composeClaimResponseSubdetailAdjudicationComponent(String name, 16376 ClaimResponse.SubdetailAdjudicationComponent element) throws IOException { 16377 if (element != null) { 16378 open(name); 16379 composeClaimResponseSubdetailAdjudicationComponentInner(element); 16380 close(); 16381 } 16382 } 16383 16384 protected void composeClaimResponseSubdetailAdjudicationComponentInner( 16385 ClaimResponse.SubdetailAdjudicationComponent element) throws IOException { 16386 composeBackbone(element); 16387 if (element.hasCode()) { 16388 composeCoding("code", element.getCode()); 16389 } 16390 if (element.hasAmount()) { 16391 composeMoney("amount", element.getAmount()); 16392 } 16393 if (element.hasValueElement()) { 16394 composeDecimalCore("value", element.getValueElement(), false); 16395 composeDecimalExtras("value", element.getValueElement(), false); 16396 } 16397 } 16398 16399 protected void composeClaimResponseAddedItemComponent(String name, ClaimResponse.AddedItemComponent element) 16400 throws IOException { 16401 if (element != null) { 16402 open(name); 16403 composeClaimResponseAddedItemComponentInner(element); 16404 close(); 16405 } 16406 } 16407 16408 protected void composeClaimResponseAddedItemComponentInner(ClaimResponse.AddedItemComponent element) 16409 throws IOException { 16410 composeBackbone(element); 16411 if (element.hasSequenceLinkId()) { 16412 openArray("sequenceLinkId"); 16413 for (PositiveIntType e : element.getSequenceLinkId()) 16414 composePositiveIntCore(null, e, true); 16415 closeArray(); 16416 if (anyHasExtras(element.getSequenceLinkId())) { 16417 openArray("_sequenceLinkId"); 16418 for (PositiveIntType e : element.getSequenceLinkId()) 16419 composePositiveIntExtras(null, e, true); 16420 closeArray(); 16421 } 16422 } 16423 ; 16424 if (element.hasService()) { 16425 composeCoding("service", element.getService()); 16426 } 16427 if (element.hasFee()) { 16428 composeMoney("fee", element.getFee()); 16429 } 16430 if (element.hasNoteNumberLinkId()) { 16431 openArray("noteNumberLinkId"); 16432 for (PositiveIntType e : element.getNoteNumberLinkId()) 16433 composePositiveIntCore(null, e, true); 16434 closeArray(); 16435 if (anyHasExtras(element.getNoteNumberLinkId())) { 16436 openArray("_noteNumberLinkId"); 16437 for (PositiveIntType e : element.getNoteNumberLinkId()) 16438 composePositiveIntExtras(null, e, true); 16439 closeArray(); 16440 } 16441 } 16442 ; 16443 if (element.hasAdjudication()) { 16444 openArray("adjudication"); 16445 for (ClaimResponse.AddedItemAdjudicationComponent e : element.getAdjudication()) 16446 composeClaimResponseAddedItemAdjudicationComponent(null, e); 16447 closeArray(); 16448 } 16449 ; 16450 if (element.hasDetail()) { 16451 openArray("detail"); 16452 for (ClaimResponse.AddedItemsDetailComponent e : element.getDetail()) 16453 composeClaimResponseAddedItemsDetailComponent(null, e); 16454 closeArray(); 16455 } 16456 ; 16457 } 16458 16459 protected void composeClaimResponseAddedItemAdjudicationComponent(String name, 16460 ClaimResponse.AddedItemAdjudicationComponent element) throws IOException { 16461 if (element != null) { 16462 open(name); 16463 composeClaimResponseAddedItemAdjudicationComponentInner(element); 16464 close(); 16465 } 16466 } 16467 16468 protected void composeClaimResponseAddedItemAdjudicationComponentInner( 16469 ClaimResponse.AddedItemAdjudicationComponent element) throws IOException { 16470 composeBackbone(element); 16471 if (element.hasCode()) { 16472 composeCoding("code", element.getCode()); 16473 } 16474 if (element.hasAmount()) { 16475 composeMoney("amount", element.getAmount()); 16476 } 16477 if (element.hasValueElement()) { 16478 composeDecimalCore("value", element.getValueElement(), false); 16479 composeDecimalExtras("value", element.getValueElement(), false); 16480 } 16481 } 16482 16483 protected void composeClaimResponseAddedItemsDetailComponent(String name, 16484 ClaimResponse.AddedItemsDetailComponent element) throws IOException { 16485 if (element != null) { 16486 open(name); 16487 composeClaimResponseAddedItemsDetailComponentInner(element); 16488 close(); 16489 } 16490 } 16491 16492 protected void composeClaimResponseAddedItemsDetailComponentInner(ClaimResponse.AddedItemsDetailComponent element) 16493 throws IOException { 16494 composeBackbone(element); 16495 if (element.hasService()) { 16496 composeCoding("service", element.getService()); 16497 } 16498 if (element.hasFee()) { 16499 composeMoney("fee", element.getFee()); 16500 } 16501 if (element.hasAdjudication()) { 16502 openArray("adjudication"); 16503 for (ClaimResponse.AddedItemDetailAdjudicationComponent e : element.getAdjudication()) 16504 composeClaimResponseAddedItemDetailAdjudicationComponent(null, e); 16505 closeArray(); 16506 } 16507 ; 16508 } 16509 16510 protected void composeClaimResponseAddedItemDetailAdjudicationComponent(String name, 16511 ClaimResponse.AddedItemDetailAdjudicationComponent element) throws IOException { 16512 if (element != null) { 16513 open(name); 16514 composeClaimResponseAddedItemDetailAdjudicationComponentInner(element); 16515 close(); 16516 } 16517 } 16518 16519 protected void composeClaimResponseAddedItemDetailAdjudicationComponentInner( 16520 ClaimResponse.AddedItemDetailAdjudicationComponent element) throws IOException { 16521 composeBackbone(element); 16522 if (element.hasCode()) { 16523 composeCoding("code", element.getCode()); 16524 } 16525 if (element.hasAmount()) { 16526 composeMoney("amount", element.getAmount()); 16527 } 16528 if (element.hasValueElement()) { 16529 composeDecimalCore("value", element.getValueElement(), false); 16530 composeDecimalExtras("value", element.getValueElement(), false); 16531 } 16532 } 16533 16534 protected void composeClaimResponseErrorsComponent(String name, ClaimResponse.ErrorsComponent element) 16535 throws IOException { 16536 if (element != null) { 16537 open(name); 16538 composeClaimResponseErrorsComponentInner(element); 16539 close(); 16540 } 16541 } 16542 16543 protected void composeClaimResponseErrorsComponentInner(ClaimResponse.ErrorsComponent element) throws IOException { 16544 composeBackbone(element); 16545 if (element.hasSequenceLinkIdElement()) { 16546 composePositiveIntCore("sequenceLinkId", element.getSequenceLinkIdElement(), false); 16547 composePositiveIntExtras("sequenceLinkId", element.getSequenceLinkIdElement(), false); 16548 } 16549 if (element.hasDetailSequenceLinkIdElement()) { 16550 composePositiveIntCore("detailSequenceLinkId", element.getDetailSequenceLinkIdElement(), false); 16551 composePositiveIntExtras("detailSequenceLinkId", element.getDetailSequenceLinkIdElement(), false); 16552 } 16553 if (element.hasSubdetailSequenceLinkIdElement()) { 16554 composePositiveIntCore("subdetailSequenceLinkId", element.getSubdetailSequenceLinkIdElement(), false); 16555 composePositiveIntExtras("subdetailSequenceLinkId", element.getSubdetailSequenceLinkIdElement(), false); 16556 } 16557 if (element.hasCode()) { 16558 composeCoding("code", element.getCode()); 16559 } 16560 } 16561 16562 protected void composeClaimResponseNotesComponent(String name, ClaimResponse.NotesComponent element) 16563 throws IOException { 16564 if (element != null) { 16565 open(name); 16566 composeClaimResponseNotesComponentInner(element); 16567 close(); 16568 } 16569 } 16570 16571 protected void composeClaimResponseNotesComponentInner(ClaimResponse.NotesComponent element) throws IOException { 16572 composeBackbone(element); 16573 if (element.hasNumberElement()) { 16574 composePositiveIntCore("number", element.getNumberElement(), false); 16575 composePositiveIntExtras("number", element.getNumberElement(), false); 16576 } 16577 if (element.hasType()) { 16578 composeCoding("type", element.getType()); 16579 } 16580 if (element.hasTextElement()) { 16581 composeStringCore("text", element.getTextElement(), false); 16582 composeStringExtras("text", element.getTextElement(), false); 16583 } 16584 } 16585 16586 protected void composeClaimResponseCoverageComponent(String name, ClaimResponse.CoverageComponent element) 16587 throws IOException { 16588 if (element != null) { 16589 open(name); 16590 composeClaimResponseCoverageComponentInner(element); 16591 close(); 16592 } 16593 } 16594 16595 protected void composeClaimResponseCoverageComponentInner(ClaimResponse.CoverageComponent element) 16596 throws IOException { 16597 composeBackbone(element); 16598 if (element.hasSequenceElement()) { 16599 composePositiveIntCore("sequence", element.getSequenceElement(), false); 16600 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 16601 } 16602 if (element.hasFocalElement()) { 16603 composeBooleanCore("focal", element.getFocalElement(), false); 16604 composeBooleanExtras("focal", element.getFocalElement(), false); 16605 } 16606 if (element.hasCoverage()) { 16607 composeReference("coverage", element.getCoverage()); 16608 } 16609 if (element.hasBusinessArrangementElement()) { 16610 composeStringCore("businessArrangement", element.getBusinessArrangementElement(), false); 16611 composeStringExtras("businessArrangement", element.getBusinessArrangementElement(), false); 16612 } 16613 if (element.hasRelationship()) { 16614 composeCoding("relationship", element.getRelationship()); 16615 } 16616 if (element.hasPreAuthRef()) { 16617 openArray("preAuthRef"); 16618 for (StringType e : element.getPreAuthRef()) 16619 composeStringCore(null, e, true); 16620 closeArray(); 16621 if (anyHasExtras(element.getPreAuthRef())) { 16622 openArray("_preAuthRef"); 16623 for (StringType e : element.getPreAuthRef()) 16624 composeStringExtras(null, e, true); 16625 closeArray(); 16626 } 16627 } 16628 ; 16629 if (element.hasClaimResponse()) { 16630 composeReference("claimResponse", element.getClaimResponse()); 16631 } 16632 if (element.hasOriginalRuleset()) { 16633 composeCoding("originalRuleset", element.getOriginalRuleset()); 16634 } 16635 } 16636 16637 protected void composeClinicalImpression(String name, ClinicalImpression element) throws IOException { 16638 if (element != null) { 16639 prop("resourceType", name); 16640 composeClinicalImpressionInner(element); 16641 } 16642 } 16643 16644 protected void composeClinicalImpressionInner(ClinicalImpression element) throws IOException { 16645 composeDomainResourceElements(element); 16646 if (element.hasPatient()) { 16647 composeReference("patient", element.getPatient()); 16648 } 16649 if (element.hasAssessor()) { 16650 composeReference("assessor", element.getAssessor()); 16651 } 16652 if (element.hasStatusElement()) { 16653 composeEnumerationCore("status", element.getStatusElement(), 16654 new ClinicalImpression.ClinicalImpressionStatusEnumFactory(), false); 16655 composeEnumerationExtras("status", element.getStatusElement(), 16656 new ClinicalImpression.ClinicalImpressionStatusEnumFactory(), false); 16657 } 16658 if (element.hasDateElement()) { 16659 composeDateTimeCore("date", element.getDateElement(), false); 16660 composeDateTimeExtras("date", element.getDateElement(), false); 16661 } 16662 if (element.hasDescriptionElement()) { 16663 composeStringCore("description", element.getDescriptionElement(), false); 16664 composeStringExtras("description", element.getDescriptionElement(), false); 16665 } 16666 if (element.hasPrevious()) { 16667 composeReference("previous", element.getPrevious()); 16668 } 16669 if (element.hasProblem()) { 16670 openArray("problem"); 16671 for (Reference e : element.getProblem()) 16672 composeReference(null, e); 16673 closeArray(); 16674 } 16675 ; 16676 if (element.hasTrigger()) { 16677 composeType("trigger", element.getTrigger()); 16678 } 16679 if (element.hasInvestigations()) { 16680 openArray("investigations"); 16681 for (ClinicalImpression.ClinicalImpressionInvestigationsComponent e : element.getInvestigations()) 16682 composeClinicalImpressionClinicalImpressionInvestigationsComponent(null, e); 16683 closeArray(); 16684 } 16685 ; 16686 if (element.hasProtocolElement()) { 16687 composeUriCore("protocol", element.getProtocolElement(), false); 16688 composeUriExtras("protocol", element.getProtocolElement(), false); 16689 } 16690 if (element.hasSummaryElement()) { 16691 composeStringCore("summary", element.getSummaryElement(), false); 16692 composeStringExtras("summary", element.getSummaryElement(), false); 16693 } 16694 if (element.hasFinding()) { 16695 openArray("finding"); 16696 for (ClinicalImpression.ClinicalImpressionFindingComponent e : element.getFinding()) 16697 composeClinicalImpressionClinicalImpressionFindingComponent(null, e); 16698 closeArray(); 16699 } 16700 ; 16701 if (element.hasResolved()) { 16702 openArray("resolved"); 16703 for (CodeableConcept e : element.getResolved()) 16704 composeCodeableConcept(null, e); 16705 closeArray(); 16706 } 16707 ; 16708 if (element.hasRuledOut()) { 16709 openArray("ruledOut"); 16710 for (ClinicalImpression.ClinicalImpressionRuledOutComponent e : element.getRuledOut()) 16711 composeClinicalImpressionClinicalImpressionRuledOutComponent(null, e); 16712 closeArray(); 16713 } 16714 ; 16715 if (element.hasPrognosisElement()) { 16716 composeStringCore("prognosis", element.getPrognosisElement(), false); 16717 composeStringExtras("prognosis", element.getPrognosisElement(), false); 16718 } 16719 if (element.hasPlan()) { 16720 openArray("plan"); 16721 for (Reference e : element.getPlan()) 16722 composeReference(null, e); 16723 closeArray(); 16724 } 16725 ; 16726 if (element.hasAction()) { 16727 openArray("action"); 16728 for (Reference e : element.getAction()) 16729 composeReference(null, e); 16730 closeArray(); 16731 } 16732 ; 16733 } 16734 16735 protected void composeClinicalImpressionClinicalImpressionInvestigationsComponent(String name, 16736 ClinicalImpression.ClinicalImpressionInvestigationsComponent element) throws IOException { 16737 if (element != null) { 16738 open(name); 16739 composeClinicalImpressionClinicalImpressionInvestigationsComponentInner(element); 16740 close(); 16741 } 16742 } 16743 16744 protected void composeClinicalImpressionClinicalImpressionInvestigationsComponentInner( 16745 ClinicalImpression.ClinicalImpressionInvestigationsComponent element) throws IOException { 16746 composeBackbone(element); 16747 if (element.hasCode()) { 16748 composeCodeableConcept("code", element.getCode()); 16749 } 16750 if (element.hasItem()) { 16751 openArray("item"); 16752 for (Reference e : element.getItem()) 16753 composeReference(null, e); 16754 closeArray(); 16755 } 16756 ; 16757 } 16758 16759 protected void composeClinicalImpressionClinicalImpressionFindingComponent(String name, 16760 ClinicalImpression.ClinicalImpressionFindingComponent element) throws IOException { 16761 if (element != null) { 16762 open(name); 16763 composeClinicalImpressionClinicalImpressionFindingComponentInner(element); 16764 close(); 16765 } 16766 } 16767 16768 protected void composeClinicalImpressionClinicalImpressionFindingComponentInner( 16769 ClinicalImpression.ClinicalImpressionFindingComponent element) throws IOException { 16770 composeBackbone(element); 16771 if (element.hasItem()) { 16772 composeCodeableConcept("item", element.getItem()); 16773 } 16774 if (element.hasCauseElement()) { 16775 composeStringCore("cause", element.getCauseElement(), false); 16776 composeStringExtras("cause", element.getCauseElement(), false); 16777 } 16778 } 16779 16780 protected void composeClinicalImpressionClinicalImpressionRuledOutComponent(String name, 16781 ClinicalImpression.ClinicalImpressionRuledOutComponent element) throws IOException { 16782 if (element != null) { 16783 open(name); 16784 composeClinicalImpressionClinicalImpressionRuledOutComponentInner(element); 16785 close(); 16786 } 16787 } 16788 16789 protected void composeClinicalImpressionClinicalImpressionRuledOutComponentInner( 16790 ClinicalImpression.ClinicalImpressionRuledOutComponent element) throws IOException { 16791 composeBackbone(element); 16792 if (element.hasItem()) { 16793 composeCodeableConcept("item", element.getItem()); 16794 } 16795 if (element.hasReasonElement()) { 16796 composeStringCore("reason", element.getReasonElement(), false); 16797 composeStringExtras("reason", element.getReasonElement(), false); 16798 } 16799 } 16800 16801 protected void composeCommunication(String name, Communication element) throws IOException { 16802 if (element != null) { 16803 prop("resourceType", name); 16804 composeCommunicationInner(element); 16805 } 16806 } 16807 16808 protected void composeCommunicationInner(Communication element) throws IOException { 16809 composeDomainResourceElements(element); 16810 if (element.hasIdentifier()) { 16811 openArray("identifier"); 16812 for (Identifier e : element.getIdentifier()) 16813 composeIdentifier(null, e); 16814 closeArray(); 16815 } 16816 ; 16817 if (element.hasCategory()) { 16818 composeCodeableConcept("category", element.getCategory()); 16819 } 16820 if (element.hasSender()) { 16821 composeReference("sender", element.getSender()); 16822 } 16823 if (element.hasRecipient()) { 16824 openArray("recipient"); 16825 for (Reference e : element.getRecipient()) 16826 composeReference(null, e); 16827 closeArray(); 16828 } 16829 ; 16830 if (element.hasPayload()) { 16831 openArray("payload"); 16832 for (Communication.CommunicationPayloadComponent e : element.getPayload()) 16833 composeCommunicationCommunicationPayloadComponent(null, e); 16834 closeArray(); 16835 } 16836 ; 16837 if (element.hasMedium()) { 16838 openArray("medium"); 16839 for (CodeableConcept e : element.getMedium()) 16840 composeCodeableConcept(null, e); 16841 closeArray(); 16842 } 16843 ; 16844 if (element.hasStatusElement()) { 16845 composeEnumerationCore("status", element.getStatusElement(), new Communication.CommunicationStatusEnumFactory(), 16846 false); 16847 composeEnumerationExtras("status", element.getStatusElement(), new Communication.CommunicationStatusEnumFactory(), 16848 false); 16849 } 16850 if (element.hasEncounter()) { 16851 composeReference("encounter", element.getEncounter()); 16852 } 16853 if (element.hasSentElement()) { 16854 composeDateTimeCore("sent", element.getSentElement(), false); 16855 composeDateTimeExtras("sent", element.getSentElement(), false); 16856 } 16857 if (element.hasReceivedElement()) { 16858 composeDateTimeCore("received", element.getReceivedElement(), false); 16859 composeDateTimeExtras("received", element.getReceivedElement(), false); 16860 } 16861 if (element.hasReason()) { 16862 openArray("reason"); 16863 for (CodeableConcept e : element.getReason()) 16864 composeCodeableConcept(null, e); 16865 closeArray(); 16866 } 16867 ; 16868 if (element.hasSubject()) { 16869 composeReference("subject", element.getSubject()); 16870 } 16871 if (element.hasRequestDetail()) { 16872 composeReference("requestDetail", element.getRequestDetail()); 16873 } 16874 } 16875 16876 protected void composeCommunicationCommunicationPayloadComponent(String name, 16877 Communication.CommunicationPayloadComponent element) throws IOException { 16878 if (element != null) { 16879 open(name); 16880 composeCommunicationCommunicationPayloadComponentInner(element); 16881 close(); 16882 } 16883 } 16884 16885 protected void composeCommunicationCommunicationPayloadComponentInner( 16886 Communication.CommunicationPayloadComponent element) throws IOException { 16887 composeBackbone(element); 16888 if (element.hasContent()) { 16889 composeType("content", element.getContent()); 16890 } 16891 } 16892 16893 protected void composeCommunicationRequest(String name, CommunicationRequest element) throws IOException { 16894 if (element != null) { 16895 prop("resourceType", name); 16896 composeCommunicationRequestInner(element); 16897 } 16898 } 16899 16900 protected void composeCommunicationRequestInner(CommunicationRequest element) throws IOException { 16901 composeDomainResourceElements(element); 16902 if (element.hasIdentifier()) { 16903 openArray("identifier"); 16904 for (Identifier e : element.getIdentifier()) 16905 composeIdentifier(null, e); 16906 closeArray(); 16907 } 16908 ; 16909 if (element.hasCategory()) { 16910 composeCodeableConcept("category", element.getCategory()); 16911 } 16912 if (element.hasSender()) { 16913 composeReference("sender", element.getSender()); 16914 } 16915 if (element.hasRecipient()) { 16916 openArray("recipient"); 16917 for (Reference e : element.getRecipient()) 16918 composeReference(null, e); 16919 closeArray(); 16920 } 16921 ; 16922 if (element.hasPayload()) { 16923 openArray("payload"); 16924 for (CommunicationRequest.CommunicationRequestPayloadComponent e : element.getPayload()) 16925 composeCommunicationRequestCommunicationRequestPayloadComponent(null, e); 16926 closeArray(); 16927 } 16928 ; 16929 if (element.hasMedium()) { 16930 openArray("medium"); 16931 for (CodeableConcept e : element.getMedium()) 16932 composeCodeableConcept(null, e); 16933 closeArray(); 16934 } 16935 ; 16936 if (element.hasRequester()) { 16937 composeReference("requester", element.getRequester()); 16938 } 16939 if (element.hasStatusElement()) { 16940 composeEnumerationCore("status", element.getStatusElement(), 16941 new CommunicationRequest.CommunicationRequestStatusEnumFactory(), false); 16942 composeEnumerationExtras("status", element.getStatusElement(), 16943 new CommunicationRequest.CommunicationRequestStatusEnumFactory(), false); 16944 } 16945 if (element.hasEncounter()) { 16946 composeReference("encounter", element.getEncounter()); 16947 } 16948 if (element.hasScheduled()) { 16949 composeType("scheduled", element.getScheduled()); 16950 } 16951 if (element.hasReason()) { 16952 openArray("reason"); 16953 for (CodeableConcept e : element.getReason()) 16954 composeCodeableConcept(null, e); 16955 closeArray(); 16956 } 16957 ; 16958 if (element.hasRequestedOnElement()) { 16959 composeDateTimeCore("requestedOn", element.getRequestedOnElement(), false); 16960 composeDateTimeExtras("requestedOn", element.getRequestedOnElement(), false); 16961 } 16962 if (element.hasSubject()) { 16963 composeReference("subject", element.getSubject()); 16964 } 16965 if (element.hasPriority()) { 16966 composeCodeableConcept("priority", element.getPriority()); 16967 } 16968 } 16969 16970 protected void composeCommunicationRequestCommunicationRequestPayloadComponent(String name, 16971 CommunicationRequest.CommunicationRequestPayloadComponent element) throws IOException { 16972 if (element != null) { 16973 open(name); 16974 composeCommunicationRequestCommunicationRequestPayloadComponentInner(element); 16975 close(); 16976 } 16977 } 16978 16979 protected void composeCommunicationRequestCommunicationRequestPayloadComponentInner( 16980 CommunicationRequest.CommunicationRequestPayloadComponent element) throws IOException { 16981 composeBackbone(element); 16982 if (element.hasContent()) { 16983 composeType("content", element.getContent()); 16984 } 16985 } 16986 16987 protected void composeComposition(String name, Composition element) throws IOException { 16988 if (element != null) { 16989 prop("resourceType", name); 16990 composeCompositionInner(element); 16991 } 16992 } 16993 16994 protected void composeCompositionInner(Composition element) throws IOException { 16995 composeDomainResourceElements(element); 16996 if (element.hasIdentifier()) { 16997 composeIdentifier("identifier", element.getIdentifier()); 16998 } 16999 if (element.hasDateElement()) { 17000 composeDateTimeCore("date", element.getDateElement(), false); 17001 composeDateTimeExtras("date", element.getDateElement(), false); 17002 } 17003 if (element.hasType()) { 17004 composeCodeableConcept("type", element.getType()); 17005 } 17006 if (element.hasClass_()) { 17007 composeCodeableConcept("class", element.getClass_()); 17008 } 17009 if (element.hasTitleElement()) { 17010 composeStringCore("title", element.getTitleElement(), false); 17011 composeStringExtras("title", element.getTitleElement(), false); 17012 } 17013 if (element.hasStatusElement()) { 17014 composeEnumerationCore("status", element.getStatusElement(), new Composition.CompositionStatusEnumFactory(), 17015 false); 17016 composeEnumerationExtras("status", element.getStatusElement(), new Composition.CompositionStatusEnumFactory(), 17017 false); 17018 } 17019 if (element.hasConfidentialityElement()) { 17020 composeCodeCore("confidentiality", element.getConfidentialityElement(), false); 17021 composeCodeExtras("confidentiality", element.getConfidentialityElement(), false); 17022 } 17023 if (element.hasSubject()) { 17024 composeReference("subject", element.getSubject()); 17025 } 17026 if (element.hasAuthor()) { 17027 openArray("author"); 17028 for (Reference e : element.getAuthor()) 17029 composeReference(null, e); 17030 closeArray(); 17031 } 17032 ; 17033 if (element.hasAttester()) { 17034 openArray("attester"); 17035 for (Composition.CompositionAttesterComponent e : element.getAttester()) 17036 composeCompositionCompositionAttesterComponent(null, e); 17037 closeArray(); 17038 } 17039 ; 17040 if (element.hasCustodian()) { 17041 composeReference("custodian", element.getCustodian()); 17042 } 17043 if (element.hasEvent()) { 17044 openArray("event"); 17045 for (Composition.CompositionEventComponent e : element.getEvent()) 17046 composeCompositionCompositionEventComponent(null, e); 17047 closeArray(); 17048 } 17049 ; 17050 if (element.hasEncounter()) { 17051 composeReference("encounter", element.getEncounter()); 17052 } 17053 if (element.hasSection()) { 17054 openArray("section"); 17055 for (Composition.SectionComponent e : element.getSection()) 17056 composeCompositionSectionComponent(null, e); 17057 closeArray(); 17058 } 17059 ; 17060 } 17061 17062 protected void composeCompositionCompositionAttesterComponent(String name, 17063 Composition.CompositionAttesterComponent element) throws IOException { 17064 if (element != null) { 17065 open(name); 17066 composeCompositionCompositionAttesterComponentInner(element); 17067 close(); 17068 } 17069 } 17070 17071 protected void composeCompositionCompositionAttesterComponentInner(Composition.CompositionAttesterComponent element) 17072 throws IOException { 17073 composeBackbone(element); 17074 if (element.hasMode()) { 17075 openArray("mode"); 17076 for (Enumeration<Composition.CompositionAttestationMode> e : element.getMode()) 17077 composeEnumerationCore(null, e, new Composition.CompositionAttestationModeEnumFactory(), true); 17078 closeArray(); 17079 if (anyHasExtras(element.getMode())) { 17080 openArray("_mode"); 17081 for (Enumeration<Composition.CompositionAttestationMode> e : element.getMode()) 17082 composeEnumerationExtras(null, e, new Composition.CompositionAttestationModeEnumFactory(), true); 17083 closeArray(); 17084 } 17085 } 17086 ; 17087 if (element.hasTimeElement()) { 17088 composeDateTimeCore("time", element.getTimeElement(), false); 17089 composeDateTimeExtras("time", element.getTimeElement(), false); 17090 } 17091 if (element.hasParty()) { 17092 composeReference("party", element.getParty()); 17093 } 17094 } 17095 17096 protected void composeCompositionCompositionEventComponent(String name, Composition.CompositionEventComponent element) 17097 throws IOException { 17098 if (element != null) { 17099 open(name); 17100 composeCompositionCompositionEventComponentInner(element); 17101 close(); 17102 } 17103 } 17104 17105 protected void composeCompositionCompositionEventComponentInner(Composition.CompositionEventComponent element) 17106 throws IOException { 17107 composeBackbone(element); 17108 if (element.hasCode()) { 17109 openArray("code"); 17110 for (CodeableConcept e : element.getCode()) 17111 composeCodeableConcept(null, e); 17112 closeArray(); 17113 } 17114 ; 17115 if (element.hasPeriod()) { 17116 composePeriod("period", element.getPeriod()); 17117 } 17118 if (element.hasDetail()) { 17119 openArray("detail"); 17120 for (Reference e : element.getDetail()) 17121 composeReference(null, e); 17122 closeArray(); 17123 } 17124 ; 17125 } 17126 17127 protected void composeCompositionSectionComponent(String name, Composition.SectionComponent element) 17128 throws IOException { 17129 if (element != null) { 17130 open(name); 17131 composeCompositionSectionComponentInner(element); 17132 close(); 17133 } 17134 } 17135 17136 protected void composeCompositionSectionComponentInner(Composition.SectionComponent element) throws IOException { 17137 composeBackbone(element); 17138 if (element.hasTitleElement()) { 17139 composeStringCore("title", element.getTitleElement(), false); 17140 composeStringExtras("title", element.getTitleElement(), false); 17141 } 17142 if (element.hasCode()) { 17143 composeCodeableConcept("code", element.getCode()); 17144 } 17145 if (element.hasText()) { 17146 composeNarrative("text", element.getText()); 17147 } 17148 if (element.hasModeElement()) { 17149 composeCodeCore("mode", element.getModeElement(), false); 17150 composeCodeExtras("mode", element.getModeElement(), false); 17151 } 17152 if (element.hasOrderedBy()) { 17153 composeCodeableConcept("orderedBy", element.getOrderedBy()); 17154 } 17155 if (element.hasEntry()) { 17156 openArray("entry"); 17157 for (Reference e : element.getEntry()) 17158 composeReference(null, e); 17159 closeArray(); 17160 } 17161 ; 17162 if (element.hasEmptyReason()) { 17163 composeCodeableConcept("emptyReason", element.getEmptyReason()); 17164 } 17165 if (element.hasSection()) { 17166 openArray("section"); 17167 for (Composition.SectionComponent e : element.getSection()) 17168 composeCompositionSectionComponent(null, e); 17169 closeArray(); 17170 } 17171 ; 17172 } 17173 17174 protected void composeConceptMap(String name, ConceptMap element) throws IOException { 17175 if (element != null) { 17176 prop("resourceType", name); 17177 composeConceptMapInner(element); 17178 } 17179 } 17180 17181 protected void composeConceptMapInner(ConceptMap element) throws IOException { 17182 composeDomainResourceElements(element); 17183 if (element.hasUrlElement()) { 17184 composeUriCore("url", element.getUrlElement(), false); 17185 composeUriExtras("url", element.getUrlElement(), false); 17186 } 17187 if (element.hasIdentifier()) { 17188 composeIdentifier("identifier", element.getIdentifier()); 17189 } 17190 if (element.hasVersionElement()) { 17191 composeStringCore("version", element.getVersionElement(), false); 17192 composeStringExtras("version", element.getVersionElement(), false); 17193 } 17194 if (element.hasNameElement()) { 17195 composeStringCore("name", element.getNameElement(), false); 17196 composeStringExtras("name", element.getNameElement(), false); 17197 } 17198 if (element.hasStatusElement()) { 17199 composeEnumerationCore("status", element.getStatusElement(), 17200 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 17201 composeEnumerationExtras("status", element.getStatusElement(), 17202 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 17203 } 17204 if (element.hasExperimentalElement()) { 17205 composeBooleanCore("experimental", element.getExperimentalElement(), false); 17206 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 17207 } 17208 if (element.hasPublisherElement()) { 17209 composeStringCore("publisher", element.getPublisherElement(), false); 17210 composeStringExtras("publisher", element.getPublisherElement(), false); 17211 } 17212 if (element.hasContact()) { 17213 openArray("contact"); 17214 for (ConceptMap.ConceptMapContactComponent e : element.getContact()) 17215 composeConceptMapConceptMapContactComponent(null, e); 17216 closeArray(); 17217 } 17218 ; 17219 if (element.hasDateElement()) { 17220 composeDateTimeCore("date", element.getDateElement(), false); 17221 composeDateTimeExtras("date", element.getDateElement(), false); 17222 } 17223 if (element.hasDescriptionElement()) { 17224 composeStringCore("description", element.getDescriptionElement(), false); 17225 composeStringExtras("description", element.getDescriptionElement(), false); 17226 } 17227 if (element.hasUseContext()) { 17228 openArray("useContext"); 17229 for (CodeableConcept e : element.getUseContext()) 17230 composeCodeableConcept(null, e); 17231 closeArray(); 17232 } 17233 ; 17234 if (element.hasRequirementsElement()) { 17235 composeStringCore("requirements", element.getRequirementsElement(), false); 17236 composeStringExtras("requirements", element.getRequirementsElement(), false); 17237 } 17238 if (element.hasCopyrightElement()) { 17239 composeStringCore("copyright", element.getCopyrightElement(), false); 17240 composeStringExtras("copyright", element.getCopyrightElement(), false); 17241 } 17242 if (element.hasSource()) { 17243 composeType("source", element.getSource()); 17244 } 17245 if (element.hasTarget()) { 17246 composeType("target", element.getTarget()); 17247 } 17248 if (element.hasElement()) { 17249 openArray("element"); 17250 for (ConceptMap.SourceElementComponent e : element.getElement()) 17251 composeConceptMapSourceElementComponent(null, e); 17252 closeArray(); 17253 } 17254 ; 17255 } 17256 17257 protected void composeConceptMapConceptMapContactComponent(String name, ConceptMap.ConceptMapContactComponent element) 17258 throws IOException { 17259 if (element != null) { 17260 open(name); 17261 composeConceptMapConceptMapContactComponentInner(element); 17262 close(); 17263 } 17264 } 17265 17266 protected void composeConceptMapConceptMapContactComponentInner(ConceptMap.ConceptMapContactComponent element) 17267 throws IOException { 17268 composeBackbone(element); 17269 if (element.hasNameElement()) { 17270 composeStringCore("name", element.getNameElement(), false); 17271 composeStringExtras("name", element.getNameElement(), false); 17272 } 17273 if (element.hasTelecom()) { 17274 openArray("telecom"); 17275 for (ContactPoint e : element.getTelecom()) 17276 composeContactPoint(null, e); 17277 closeArray(); 17278 } 17279 ; 17280 } 17281 17282 protected void composeConceptMapSourceElementComponent(String name, ConceptMap.SourceElementComponent element) 17283 throws IOException { 17284 if (element != null) { 17285 open(name); 17286 composeConceptMapSourceElementComponentInner(element); 17287 close(); 17288 } 17289 } 17290 17291 protected void composeConceptMapSourceElementComponentInner(ConceptMap.SourceElementComponent element) 17292 throws IOException { 17293 composeBackbone(element); 17294 if (element.hasCodeSystemElement()) { 17295 composeUriCore("codeSystem", element.getCodeSystemElement(), false); 17296 composeUriExtras("codeSystem", element.getCodeSystemElement(), false); 17297 } 17298 if (element.hasCodeElement()) { 17299 composeCodeCore("code", element.getCodeElement(), false); 17300 composeCodeExtras("code", element.getCodeElement(), false); 17301 } 17302 if (element.hasTarget()) { 17303 openArray("target"); 17304 for (ConceptMap.TargetElementComponent e : element.getTarget()) 17305 composeConceptMapTargetElementComponent(null, e); 17306 closeArray(); 17307 } 17308 ; 17309 } 17310 17311 protected void composeConceptMapTargetElementComponent(String name, ConceptMap.TargetElementComponent element) 17312 throws IOException { 17313 if (element != null) { 17314 open(name); 17315 composeConceptMapTargetElementComponentInner(element); 17316 close(); 17317 } 17318 } 17319 17320 protected void composeConceptMapTargetElementComponentInner(ConceptMap.TargetElementComponent element) 17321 throws IOException { 17322 composeBackbone(element); 17323 if (element.hasCodeSystemElement()) { 17324 composeUriCore("codeSystem", element.getCodeSystemElement(), false); 17325 composeUriExtras("codeSystem", element.getCodeSystemElement(), false); 17326 } 17327 if (element.hasCodeElement()) { 17328 composeCodeCore("code", element.getCodeElement(), false); 17329 composeCodeExtras("code", element.getCodeElement(), false); 17330 } 17331 if (element.hasEquivalenceElement()) { 17332 composeEnumerationCore("equivalence", element.getEquivalenceElement(), 17333 new Enumerations.ConceptMapEquivalenceEnumFactory(), false); 17334 composeEnumerationExtras("equivalence", element.getEquivalenceElement(), 17335 new Enumerations.ConceptMapEquivalenceEnumFactory(), false); 17336 } 17337 if (element.hasCommentsElement()) { 17338 composeStringCore("comments", element.getCommentsElement(), false); 17339 composeStringExtras("comments", element.getCommentsElement(), false); 17340 } 17341 if (element.hasDependsOn()) { 17342 openArray("dependsOn"); 17343 for (ConceptMap.OtherElementComponent e : element.getDependsOn()) 17344 composeConceptMapOtherElementComponent(null, e); 17345 closeArray(); 17346 } 17347 ; 17348 if (element.hasProduct()) { 17349 openArray("product"); 17350 for (ConceptMap.OtherElementComponent e : element.getProduct()) 17351 composeConceptMapOtherElementComponent(null, e); 17352 closeArray(); 17353 } 17354 ; 17355 } 17356 17357 protected void composeConceptMapOtherElementComponent(String name, ConceptMap.OtherElementComponent element) 17358 throws IOException { 17359 if (element != null) { 17360 open(name); 17361 composeConceptMapOtherElementComponentInner(element); 17362 close(); 17363 } 17364 } 17365 17366 protected void composeConceptMapOtherElementComponentInner(ConceptMap.OtherElementComponent element) 17367 throws IOException { 17368 composeBackbone(element); 17369 if (element.hasElementElement()) { 17370 composeUriCore("element", element.getElementElement(), false); 17371 composeUriExtras("element", element.getElementElement(), false); 17372 } 17373 if (element.hasCodeSystemElement()) { 17374 composeUriCore("codeSystem", element.getCodeSystemElement(), false); 17375 composeUriExtras("codeSystem", element.getCodeSystemElement(), false); 17376 } 17377 if (element.hasCodeElement()) { 17378 composeStringCore("code", element.getCodeElement(), false); 17379 composeStringExtras("code", element.getCodeElement(), false); 17380 } 17381 } 17382 17383 protected void composeCondition(String name, Condition element) throws IOException { 17384 if (element != null) { 17385 prop("resourceType", name); 17386 composeConditionInner(element); 17387 } 17388 } 17389 17390 protected void composeConditionInner(Condition element) throws IOException { 17391 composeDomainResourceElements(element); 17392 if (element.hasIdentifier()) { 17393 openArray("identifier"); 17394 for (Identifier e : element.getIdentifier()) 17395 composeIdentifier(null, e); 17396 closeArray(); 17397 } 17398 ; 17399 if (element.hasPatient()) { 17400 composeReference("patient", element.getPatient()); 17401 } 17402 if (element.hasEncounter()) { 17403 composeReference("encounter", element.getEncounter()); 17404 } 17405 if (element.hasAsserter()) { 17406 composeReference("asserter", element.getAsserter()); 17407 } 17408 if (element.hasDateRecordedElement()) { 17409 composeDateCore("dateRecorded", element.getDateRecordedElement(), false); 17410 composeDateExtras("dateRecorded", element.getDateRecordedElement(), false); 17411 } 17412 if (element.hasCode()) { 17413 composeCodeableConcept("code", element.getCode()); 17414 } 17415 if (element.hasCategory()) { 17416 composeCodeableConcept("category", element.getCategory()); 17417 } 17418 if (element.hasClinicalStatusElement()) { 17419 composeCodeCore("clinicalStatus", element.getClinicalStatusElement(), false); 17420 composeCodeExtras("clinicalStatus", element.getClinicalStatusElement(), false); 17421 } 17422 if (element.hasVerificationStatusElement()) { 17423 composeEnumerationCore("verificationStatus", element.getVerificationStatusElement(), 17424 new Condition.ConditionVerificationStatusEnumFactory(), false); 17425 composeEnumerationExtras("verificationStatus", element.getVerificationStatusElement(), 17426 new Condition.ConditionVerificationStatusEnumFactory(), false); 17427 } 17428 if (element.hasSeverity()) { 17429 composeCodeableConcept("severity", element.getSeverity()); 17430 } 17431 if (element.hasOnset()) { 17432 composeType("onset", element.getOnset()); 17433 } 17434 if (element.hasAbatement()) { 17435 composeType("abatement", element.getAbatement()); 17436 } 17437 if (element.hasStage()) { 17438 composeConditionConditionStageComponent("stage", element.getStage()); 17439 } 17440 if (element.hasEvidence()) { 17441 openArray("evidence"); 17442 for (Condition.ConditionEvidenceComponent e : element.getEvidence()) 17443 composeConditionConditionEvidenceComponent(null, e); 17444 closeArray(); 17445 } 17446 ; 17447 if (element.hasBodySite()) { 17448 openArray("bodySite"); 17449 for (CodeableConcept e : element.getBodySite()) 17450 composeCodeableConcept(null, e); 17451 closeArray(); 17452 } 17453 ; 17454 if (element.hasNotesElement()) { 17455 composeStringCore("notes", element.getNotesElement(), false); 17456 composeStringExtras("notes", element.getNotesElement(), false); 17457 } 17458 } 17459 17460 protected void composeConditionConditionStageComponent(String name, Condition.ConditionStageComponent element) 17461 throws IOException { 17462 if (element != null) { 17463 open(name); 17464 composeConditionConditionStageComponentInner(element); 17465 close(); 17466 } 17467 } 17468 17469 protected void composeConditionConditionStageComponentInner(Condition.ConditionStageComponent element) 17470 throws IOException { 17471 composeBackbone(element); 17472 if (element.hasSummary()) { 17473 composeCodeableConcept("summary", element.getSummary()); 17474 } 17475 if (element.hasAssessment()) { 17476 openArray("assessment"); 17477 for (Reference e : element.getAssessment()) 17478 composeReference(null, e); 17479 closeArray(); 17480 } 17481 ; 17482 } 17483 17484 protected void composeConditionConditionEvidenceComponent(String name, Condition.ConditionEvidenceComponent element) 17485 throws IOException { 17486 if (element != null) { 17487 open(name); 17488 composeConditionConditionEvidenceComponentInner(element); 17489 close(); 17490 } 17491 } 17492 17493 protected void composeConditionConditionEvidenceComponentInner(Condition.ConditionEvidenceComponent element) 17494 throws IOException { 17495 composeBackbone(element); 17496 if (element.hasCode()) { 17497 composeCodeableConcept("code", element.getCode()); 17498 } 17499 if (element.hasDetail()) { 17500 openArray("detail"); 17501 for (Reference e : element.getDetail()) 17502 composeReference(null, e); 17503 closeArray(); 17504 } 17505 ; 17506 } 17507 17508 protected void composeConformance(String name, Conformance element) throws IOException { 17509 if (element != null) { 17510 prop("resourceType", name); 17511 composeConformanceInner(element); 17512 } 17513 } 17514 17515 protected void composeConformanceInner(Conformance element) throws IOException { 17516 composeDomainResourceElements(element); 17517 if (element.hasUrlElement()) { 17518 composeUriCore("url", element.getUrlElement(), false); 17519 composeUriExtras("url", element.getUrlElement(), false); 17520 } 17521 if (element.hasVersionElement()) { 17522 composeStringCore("version", element.getVersionElement(), false); 17523 composeStringExtras("version", element.getVersionElement(), false); 17524 } 17525 if (element.hasNameElement()) { 17526 composeStringCore("name", element.getNameElement(), false); 17527 composeStringExtras("name", element.getNameElement(), false); 17528 } 17529 if (element.hasStatusElement()) { 17530 composeEnumerationCore("status", element.getStatusElement(), 17531 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 17532 composeEnumerationExtras("status", element.getStatusElement(), 17533 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 17534 } 17535 if (element.hasExperimentalElement()) { 17536 composeBooleanCore("experimental", element.getExperimentalElement(), false); 17537 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 17538 } 17539 if (element.hasPublisherElement()) { 17540 composeStringCore("publisher", element.getPublisherElement(), false); 17541 composeStringExtras("publisher", element.getPublisherElement(), false); 17542 } 17543 if (element.hasContact()) { 17544 openArray("contact"); 17545 for (Conformance.ConformanceContactComponent e : element.getContact()) 17546 composeConformanceConformanceContactComponent(null, e); 17547 closeArray(); 17548 } 17549 ; 17550 if (element.hasDateElement()) { 17551 composeDateTimeCore("date", element.getDateElement(), false); 17552 composeDateTimeExtras("date", element.getDateElement(), false); 17553 } 17554 if (element.hasDescriptionElement()) { 17555 composeStringCore("description", element.getDescriptionElement(), false); 17556 composeStringExtras("description", element.getDescriptionElement(), false); 17557 } 17558 if (element.hasRequirementsElement()) { 17559 composeStringCore("requirements", element.getRequirementsElement(), false); 17560 composeStringExtras("requirements", element.getRequirementsElement(), false); 17561 } 17562 if (element.hasCopyrightElement()) { 17563 composeStringCore("copyright", element.getCopyrightElement(), false); 17564 composeStringExtras("copyright", element.getCopyrightElement(), false); 17565 } 17566 if (element.hasKindElement()) { 17567 composeEnumerationCore("kind", element.getKindElement(), new Conformance.ConformanceStatementKindEnumFactory(), 17568 false); 17569 composeEnumerationExtras("kind", element.getKindElement(), new Conformance.ConformanceStatementKindEnumFactory(), 17570 false); 17571 } 17572 if (element.hasSoftware()) { 17573 composeConformanceConformanceSoftwareComponent("software", element.getSoftware()); 17574 } 17575 if (element.hasImplementation()) { 17576 composeConformanceConformanceImplementationComponent("implementation", element.getImplementation()); 17577 } 17578 if (element.hasFhirVersionElement()) { 17579 composeIdCore("fhirVersion", element.getFhirVersionElement(), false); 17580 composeIdExtras("fhirVersion", element.getFhirVersionElement(), false); 17581 } 17582 if (element.hasAcceptUnknownElement()) { 17583 composeEnumerationCore("acceptUnknown", element.getAcceptUnknownElement(), 17584 new Conformance.UnknownContentCodeEnumFactory(), false); 17585 composeEnumerationExtras("acceptUnknown", element.getAcceptUnknownElement(), 17586 new Conformance.UnknownContentCodeEnumFactory(), false); 17587 } 17588 if (element.hasFormat()) { 17589 openArray("format"); 17590 for (CodeType e : element.getFormat()) 17591 composeCodeCore(null, e, true); 17592 closeArray(); 17593 if (anyHasExtras(element.getFormat())) { 17594 openArray("_format"); 17595 for (CodeType e : element.getFormat()) 17596 composeCodeExtras(null, e, true); 17597 closeArray(); 17598 } 17599 } 17600 ; 17601 if (element.hasProfile()) { 17602 openArray("profile"); 17603 for (Reference e : element.getProfile()) 17604 composeReference(null, e); 17605 closeArray(); 17606 } 17607 ; 17608 if (element.hasRest()) { 17609 openArray("rest"); 17610 for (Conformance.ConformanceRestComponent e : element.getRest()) 17611 composeConformanceConformanceRestComponent(null, e); 17612 closeArray(); 17613 } 17614 ; 17615 if (element.hasMessaging()) { 17616 openArray("messaging"); 17617 for (Conformance.ConformanceMessagingComponent e : element.getMessaging()) 17618 composeConformanceConformanceMessagingComponent(null, e); 17619 closeArray(); 17620 } 17621 ; 17622 if (element.hasDocument()) { 17623 openArray("document"); 17624 for (Conformance.ConformanceDocumentComponent e : element.getDocument()) 17625 composeConformanceConformanceDocumentComponent(null, e); 17626 closeArray(); 17627 } 17628 ; 17629 } 17630 17631 protected void composeConformanceConformanceContactComponent(String name, 17632 Conformance.ConformanceContactComponent element) throws IOException { 17633 if (element != null) { 17634 open(name); 17635 composeConformanceConformanceContactComponentInner(element); 17636 close(); 17637 } 17638 } 17639 17640 protected void composeConformanceConformanceContactComponentInner(Conformance.ConformanceContactComponent element) 17641 throws IOException { 17642 composeBackbone(element); 17643 if (element.hasNameElement()) { 17644 composeStringCore("name", element.getNameElement(), false); 17645 composeStringExtras("name", element.getNameElement(), false); 17646 } 17647 if (element.hasTelecom()) { 17648 openArray("telecom"); 17649 for (ContactPoint e : element.getTelecom()) 17650 composeContactPoint(null, e); 17651 closeArray(); 17652 } 17653 ; 17654 } 17655 17656 protected void composeConformanceConformanceSoftwareComponent(String name, 17657 Conformance.ConformanceSoftwareComponent element) throws IOException { 17658 if (element != null) { 17659 open(name); 17660 composeConformanceConformanceSoftwareComponentInner(element); 17661 close(); 17662 } 17663 } 17664 17665 protected void composeConformanceConformanceSoftwareComponentInner(Conformance.ConformanceSoftwareComponent element) 17666 throws IOException { 17667 composeBackbone(element); 17668 if (element.hasNameElement()) { 17669 composeStringCore("name", element.getNameElement(), false); 17670 composeStringExtras("name", element.getNameElement(), false); 17671 } 17672 if (element.hasVersionElement()) { 17673 composeStringCore("version", element.getVersionElement(), false); 17674 composeStringExtras("version", element.getVersionElement(), false); 17675 } 17676 if (element.hasReleaseDateElement()) { 17677 composeDateTimeCore("releaseDate", element.getReleaseDateElement(), false); 17678 composeDateTimeExtras("releaseDate", element.getReleaseDateElement(), false); 17679 } 17680 } 17681 17682 protected void composeConformanceConformanceImplementationComponent(String name, 17683 Conformance.ConformanceImplementationComponent element) throws IOException { 17684 if (element != null) { 17685 open(name); 17686 composeConformanceConformanceImplementationComponentInner(element); 17687 close(); 17688 } 17689 } 17690 17691 protected void composeConformanceConformanceImplementationComponentInner( 17692 Conformance.ConformanceImplementationComponent element) throws IOException { 17693 composeBackbone(element); 17694 if (element.hasDescriptionElement()) { 17695 composeStringCore("description", element.getDescriptionElement(), false); 17696 composeStringExtras("description", element.getDescriptionElement(), false); 17697 } 17698 if (element.hasUrlElement()) { 17699 composeUriCore("url", element.getUrlElement(), false); 17700 composeUriExtras("url", element.getUrlElement(), false); 17701 } 17702 } 17703 17704 protected void composeConformanceConformanceRestComponent(String name, Conformance.ConformanceRestComponent element) 17705 throws IOException { 17706 if (element != null) { 17707 open(name); 17708 composeConformanceConformanceRestComponentInner(element); 17709 close(); 17710 } 17711 } 17712 17713 protected void composeConformanceConformanceRestComponentInner(Conformance.ConformanceRestComponent element) 17714 throws IOException { 17715 composeBackbone(element); 17716 if (element.hasModeElement()) { 17717 composeEnumerationCore("mode", element.getModeElement(), new Conformance.RestfulConformanceModeEnumFactory(), 17718 false); 17719 composeEnumerationExtras("mode", element.getModeElement(), new Conformance.RestfulConformanceModeEnumFactory(), 17720 false); 17721 } 17722 if (element.hasDocumentationElement()) { 17723 composeStringCore("documentation", element.getDocumentationElement(), false); 17724 composeStringExtras("documentation", element.getDocumentationElement(), false); 17725 } 17726 if (element.hasSecurity()) { 17727 composeConformanceConformanceRestSecurityComponent("security", element.getSecurity()); 17728 } 17729 if (element.hasResource()) { 17730 openArray("resource"); 17731 for (Conformance.ConformanceRestResourceComponent e : element.getResource()) 17732 composeConformanceConformanceRestResourceComponent(null, e); 17733 closeArray(); 17734 } 17735 ; 17736 if (element.hasInteraction()) { 17737 openArray("interaction"); 17738 for (Conformance.SystemInteractionComponent e : element.getInteraction()) 17739 composeConformanceSystemInteractionComponent(null, e); 17740 closeArray(); 17741 } 17742 ; 17743 if (element.hasTransactionModeElement()) { 17744 composeEnumerationCore("transactionMode", element.getTransactionModeElement(), 17745 new Conformance.TransactionModeEnumFactory(), false); 17746 composeEnumerationExtras("transactionMode", element.getTransactionModeElement(), 17747 new Conformance.TransactionModeEnumFactory(), false); 17748 } 17749 if (element.hasSearchParam()) { 17750 openArray("searchParam"); 17751 for (Conformance.ConformanceRestResourceSearchParamComponent e : element.getSearchParam()) 17752 composeConformanceConformanceRestResourceSearchParamComponent(null, e); 17753 closeArray(); 17754 } 17755 ; 17756 if (element.hasOperation()) { 17757 openArray("operation"); 17758 for (Conformance.ConformanceRestOperationComponent e : element.getOperation()) 17759 composeConformanceConformanceRestOperationComponent(null, e); 17760 closeArray(); 17761 } 17762 ; 17763 if (element.hasCompartment()) { 17764 openArray("compartment"); 17765 for (UriType e : element.getCompartment()) 17766 composeUriCore(null, e, true); 17767 closeArray(); 17768 if (anyHasExtras(element.getCompartment())) { 17769 openArray("_compartment"); 17770 for (UriType e : element.getCompartment()) 17771 composeUriExtras(null, e, true); 17772 closeArray(); 17773 } 17774 } 17775 ; 17776 } 17777 17778 protected void composeConformanceConformanceRestSecurityComponent(String name, 17779 Conformance.ConformanceRestSecurityComponent element) throws IOException { 17780 if (element != null) { 17781 open(name); 17782 composeConformanceConformanceRestSecurityComponentInner(element); 17783 close(); 17784 } 17785 } 17786 17787 protected void composeConformanceConformanceRestSecurityComponentInner( 17788 Conformance.ConformanceRestSecurityComponent element) throws IOException { 17789 composeBackbone(element); 17790 if (element.hasCorsElement()) { 17791 composeBooleanCore("cors", element.getCorsElement(), false); 17792 composeBooleanExtras("cors", element.getCorsElement(), false); 17793 } 17794 if (element.hasService()) { 17795 openArray("service"); 17796 for (CodeableConcept e : element.getService()) 17797 composeCodeableConcept(null, e); 17798 closeArray(); 17799 } 17800 ; 17801 if (element.hasDescriptionElement()) { 17802 composeStringCore("description", element.getDescriptionElement(), false); 17803 composeStringExtras("description", element.getDescriptionElement(), false); 17804 } 17805 if (element.hasCertificate()) { 17806 openArray("certificate"); 17807 for (Conformance.ConformanceRestSecurityCertificateComponent e : element.getCertificate()) 17808 composeConformanceConformanceRestSecurityCertificateComponent(null, e); 17809 closeArray(); 17810 } 17811 ; 17812 } 17813 17814 protected void composeConformanceConformanceRestSecurityCertificateComponent(String name, 17815 Conformance.ConformanceRestSecurityCertificateComponent element) throws IOException { 17816 if (element != null) { 17817 open(name); 17818 composeConformanceConformanceRestSecurityCertificateComponentInner(element); 17819 close(); 17820 } 17821 } 17822 17823 protected void composeConformanceConformanceRestSecurityCertificateComponentInner( 17824 Conformance.ConformanceRestSecurityCertificateComponent element) throws IOException { 17825 composeBackbone(element); 17826 if (element.hasTypeElement()) { 17827 composeCodeCore("type", element.getTypeElement(), false); 17828 composeCodeExtras("type", element.getTypeElement(), false); 17829 } 17830 if (element.hasBlobElement()) { 17831 composeBase64BinaryCore("blob", element.getBlobElement(), false); 17832 composeBase64BinaryExtras("blob", element.getBlobElement(), false); 17833 } 17834 } 17835 17836 protected void composeConformanceConformanceRestResourceComponent(String name, 17837 Conformance.ConformanceRestResourceComponent element) throws IOException { 17838 if (element != null) { 17839 open(name); 17840 composeConformanceConformanceRestResourceComponentInner(element); 17841 close(); 17842 } 17843 } 17844 17845 protected void composeConformanceConformanceRestResourceComponentInner( 17846 Conformance.ConformanceRestResourceComponent element) throws IOException { 17847 composeBackbone(element); 17848 if (element.hasTypeElement()) { 17849 composeCodeCore("type", element.getTypeElement(), false); 17850 composeCodeExtras("type", element.getTypeElement(), false); 17851 } 17852 if (element.hasProfile()) { 17853 composeReference("profile", element.getProfile()); 17854 } 17855 if (element.hasInteraction()) { 17856 openArray("interaction"); 17857 for (Conformance.ResourceInteractionComponent e : element.getInteraction()) 17858 composeConformanceResourceInteractionComponent(null, e); 17859 closeArray(); 17860 } 17861 ; 17862 if (element.hasVersioningElement()) { 17863 composeEnumerationCore("versioning", element.getVersioningElement(), 17864 new Conformance.ResourceVersionPolicyEnumFactory(), false); 17865 composeEnumerationExtras("versioning", element.getVersioningElement(), 17866 new Conformance.ResourceVersionPolicyEnumFactory(), false); 17867 } 17868 if (element.hasReadHistoryElement()) { 17869 composeBooleanCore("readHistory", element.getReadHistoryElement(), false); 17870 composeBooleanExtras("readHistory", element.getReadHistoryElement(), false); 17871 } 17872 if (element.hasUpdateCreateElement()) { 17873 composeBooleanCore("updateCreate", element.getUpdateCreateElement(), false); 17874 composeBooleanExtras("updateCreate", element.getUpdateCreateElement(), false); 17875 } 17876 if (element.hasConditionalCreateElement()) { 17877 composeBooleanCore("conditionalCreate", element.getConditionalCreateElement(), false); 17878 composeBooleanExtras("conditionalCreate", element.getConditionalCreateElement(), false); 17879 } 17880 if (element.hasConditionalUpdateElement()) { 17881 composeBooleanCore("conditionalUpdate", element.getConditionalUpdateElement(), false); 17882 composeBooleanExtras("conditionalUpdate", element.getConditionalUpdateElement(), false); 17883 } 17884 if (element.hasConditionalDeleteElement()) { 17885 composeEnumerationCore("conditionalDelete", element.getConditionalDeleteElement(), 17886 new Conformance.ConditionalDeleteStatusEnumFactory(), false); 17887 composeEnumerationExtras("conditionalDelete", element.getConditionalDeleteElement(), 17888 new Conformance.ConditionalDeleteStatusEnumFactory(), false); 17889 } 17890 if (element.hasSearchInclude()) { 17891 openArray("searchInclude"); 17892 for (StringType e : element.getSearchInclude()) 17893 composeStringCore(null, e, true); 17894 closeArray(); 17895 if (anyHasExtras(element.getSearchInclude())) { 17896 openArray("_searchInclude"); 17897 for (StringType e : element.getSearchInclude()) 17898 composeStringExtras(null, e, true); 17899 closeArray(); 17900 } 17901 } 17902 ; 17903 if (element.hasSearchRevInclude()) { 17904 openArray("searchRevInclude"); 17905 for (StringType e : element.getSearchRevInclude()) 17906 composeStringCore(null, e, true); 17907 closeArray(); 17908 if (anyHasExtras(element.getSearchRevInclude())) { 17909 openArray("_searchRevInclude"); 17910 for (StringType e : element.getSearchRevInclude()) 17911 composeStringExtras(null, e, true); 17912 closeArray(); 17913 } 17914 } 17915 ; 17916 if (element.hasSearchParam()) { 17917 openArray("searchParam"); 17918 for (Conformance.ConformanceRestResourceSearchParamComponent e : element.getSearchParam()) 17919 composeConformanceConformanceRestResourceSearchParamComponent(null, e); 17920 closeArray(); 17921 } 17922 ; 17923 } 17924 17925 protected void composeConformanceResourceInteractionComponent(String name, 17926 Conformance.ResourceInteractionComponent element) throws IOException { 17927 if (element != null) { 17928 open(name); 17929 composeConformanceResourceInteractionComponentInner(element); 17930 close(); 17931 } 17932 } 17933 17934 protected void composeConformanceResourceInteractionComponentInner(Conformance.ResourceInteractionComponent element) 17935 throws IOException { 17936 composeBackbone(element); 17937 if (element.hasCodeElement()) { 17938 composeEnumerationCore("code", element.getCodeElement(), new Conformance.TypeRestfulInteractionEnumFactory(), 17939 false); 17940 composeEnumerationExtras("code", element.getCodeElement(), new Conformance.TypeRestfulInteractionEnumFactory(), 17941 false); 17942 } 17943 if (element.hasDocumentationElement()) { 17944 composeStringCore("documentation", element.getDocumentationElement(), false); 17945 composeStringExtras("documentation", element.getDocumentationElement(), false); 17946 } 17947 } 17948 17949 protected void composeConformanceConformanceRestResourceSearchParamComponent(String name, 17950 Conformance.ConformanceRestResourceSearchParamComponent element) throws IOException { 17951 if (element != null) { 17952 open(name); 17953 composeConformanceConformanceRestResourceSearchParamComponentInner(element); 17954 close(); 17955 } 17956 } 17957 17958 protected void composeConformanceConformanceRestResourceSearchParamComponentInner( 17959 Conformance.ConformanceRestResourceSearchParamComponent element) throws IOException { 17960 composeBackbone(element); 17961 if (element.hasNameElement()) { 17962 composeStringCore("name", element.getNameElement(), false); 17963 composeStringExtras("name", element.getNameElement(), false); 17964 } 17965 if (element.hasDefinitionElement()) { 17966 composeUriCore("definition", element.getDefinitionElement(), false); 17967 composeUriExtras("definition", element.getDefinitionElement(), false); 17968 } 17969 if (element.hasTypeElement()) { 17970 composeEnumerationCore("type", element.getTypeElement(), new Enumerations.SearchParamTypeEnumFactory(), false); 17971 composeEnumerationExtras("type", element.getTypeElement(), new Enumerations.SearchParamTypeEnumFactory(), false); 17972 } 17973 if (element.hasDocumentationElement()) { 17974 composeStringCore("documentation", element.getDocumentationElement(), false); 17975 composeStringExtras("documentation", element.getDocumentationElement(), false); 17976 } 17977 if (element.hasTarget()) { 17978 openArray("target"); 17979 for (CodeType e : element.getTarget()) 17980 composeCodeCore(null, e, true); 17981 closeArray(); 17982 if (anyHasExtras(element.getTarget())) { 17983 openArray("_target"); 17984 for (CodeType e : element.getTarget()) 17985 composeCodeExtras(null, e, true); 17986 closeArray(); 17987 } 17988 } 17989 ; 17990 if (element.hasModifier()) { 17991 openArray("modifier"); 17992 for (Enumeration<Conformance.SearchModifierCode> e : element.getModifier()) 17993 composeEnumerationCore(null, e, new Conformance.SearchModifierCodeEnumFactory(), true); 17994 closeArray(); 17995 if (anyHasExtras(element.getModifier())) { 17996 openArray("_modifier"); 17997 for (Enumeration<Conformance.SearchModifierCode> e : element.getModifier()) 17998 composeEnumerationExtras(null, e, new Conformance.SearchModifierCodeEnumFactory(), true); 17999 closeArray(); 18000 } 18001 } 18002 ; 18003 if (element.hasChain()) { 18004 openArray("chain"); 18005 for (StringType e : element.getChain()) 18006 composeStringCore(null, e, true); 18007 closeArray(); 18008 if (anyHasExtras(element.getChain())) { 18009 openArray("_chain"); 18010 for (StringType e : element.getChain()) 18011 composeStringExtras(null, e, true); 18012 closeArray(); 18013 } 18014 } 18015 ; 18016 } 18017 18018 protected void composeConformanceSystemInteractionComponent(String name, 18019 Conformance.SystemInteractionComponent element) throws IOException { 18020 if (element != null) { 18021 open(name); 18022 composeConformanceSystemInteractionComponentInner(element); 18023 close(); 18024 } 18025 } 18026 18027 protected void composeConformanceSystemInteractionComponentInner(Conformance.SystemInteractionComponent element) 18028 throws IOException { 18029 composeBackbone(element); 18030 if (element.hasCodeElement()) { 18031 composeEnumerationCore("code", element.getCodeElement(), new Conformance.SystemRestfulInteractionEnumFactory(), 18032 false); 18033 composeEnumerationExtras("code", element.getCodeElement(), new Conformance.SystemRestfulInteractionEnumFactory(), 18034 false); 18035 } 18036 if (element.hasDocumentationElement()) { 18037 composeStringCore("documentation", element.getDocumentationElement(), false); 18038 composeStringExtras("documentation", element.getDocumentationElement(), false); 18039 } 18040 } 18041 18042 protected void composeConformanceConformanceRestOperationComponent(String name, 18043 Conformance.ConformanceRestOperationComponent element) throws IOException { 18044 if (element != null) { 18045 open(name); 18046 composeConformanceConformanceRestOperationComponentInner(element); 18047 close(); 18048 } 18049 } 18050 18051 protected void composeConformanceConformanceRestOperationComponentInner( 18052 Conformance.ConformanceRestOperationComponent element) throws IOException { 18053 composeBackbone(element); 18054 if (element.hasNameElement()) { 18055 composeStringCore("name", element.getNameElement(), false); 18056 composeStringExtras("name", element.getNameElement(), false); 18057 } 18058 if (element.hasDefinition()) { 18059 composeReference("definition", element.getDefinition()); 18060 } 18061 } 18062 18063 protected void composeConformanceConformanceMessagingComponent(String name, 18064 Conformance.ConformanceMessagingComponent element) throws IOException { 18065 if (element != null) { 18066 open(name); 18067 composeConformanceConformanceMessagingComponentInner(element); 18068 close(); 18069 } 18070 } 18071 18072 protected void composeConformanceConformanceMessagingComponentInner(Conformance.ConformanceMessagingComponent element) 18073 throws IOException { 18074 composeBackbone(element); 18075 if (element.hasEndpoint()) { 18076 openArray("endpoint"); 18077 for (Conformance.ConformanceMessagingEndpointComponent e : element.getEndpoint()) 18078 composeConformanceConformanceMessagingEndpointComponent(null, e); 18079 closeArray(); 18080 } 18081 ; 18082 if (element.hasReliableCacheElement()) { 18083 composeUnsignedIntCore("reliableCache", element.getReliableCacheElement(), false); 18084 composeUnsignedIntExtras("reliableCache", element.getReliableCacheElement(), false); 18085 } 18086 if (element.hasDocumentationElement()) { 18087 composeStringCore("documentation", element.getDocumentationElement(), false); 18088 composeStringExtras("documentation", element.getDocumentationElement(), false); 18089 } 18090 if (element.hasEvent()) { 18091 openArray("event"); 18092 for (Conformance.ConformanceMessagingEventComponent e : element.getEvent()) 18093 composeConformanceConformanceMessagingEventComponent(null, e); 18094 closeArray(); 18095 } 18096 ; 18097 } 18098 18099 protected void composeConformanceConformanceMessagingEndpointComponent(String name, 18100 Conformance.ConformanceMessagingEndpointComponent element) throws IOException { 18101 if (element != null) { 18102 open(name); 18103 composeConformanceConformanceMessagingEndpointComponentInner(element); 18104 close(); 18105 } 18106 } 18107 18108 protected void composeConformanceConformanceMessagingEndpointComponentInner( 18109 Conformance.ConformanceMessagingEndpointComponent element) throws IOException { 18110 composeBackbone(element); 18111 if (element.hasProtocol()) { 18112 composeCoding("protocol", element.getProtocol()); 18113 } 18114 if (element.hasAddressElement()) { 18115 composeUriCore("address", element.getAddressElement(), false); 18116 composeUriExtras("address", element.getAddressElement(), false); 18117 } 18118 } 18119 18120 protected void composeConformanceConformanceMessagingEventComponent(String name, 18121 Conformance.ConformanceMessagingEventComponent element) throws IOException { 18122 if (element != null) { 18123 open(name); 18124 composeConformanceConformanceMessagingEventComponentInner(element); 18125 close(); 18126 } 18127 } 18128 18129 protected void composeConformanceConformanceMessagingEventComponentInner( 18130 Conformance.ConformanceMessagingEventComponent element) throws IOException { 18131 composeBackbone(element); 18132 if (element.hasCode()) { 18133 composeCoding("code", element.getCode()); 18134 } 18135 if (element.hasCategoryElement()) { 18136 composeEnumerationCore("category", element.getCategoryElement(), 18137 new Conformance.MessageSignificanceCategoryEnumFactory(), false); 18138 composeEnumerationExtras("category", element.getCategoryElement(), 18139 new Conformance.MessageSignificanceCategoryEnumFactory(), false); 18140 } 18141 if (element.hasModeElement()) { 18142 composeEnumerationCore("mode", element.getModeElement(), new Conformance.ConformanceEventModeEnumFactory(), 18143 false); 18144 composeEnumerationExtras("mode", element.getModeElement(), new Conformance.ConformanceEventModeEnumFactory(), 18145 false); 18146 } 18147 if (element.hasFocusElement()) { 18148 composeCodeCore("focus", element.getFocusElement(), false); 18149 composeCodeExtras("focus", element.getFocusElement(), false); 18150 } 18151 if (element.hasRequest()) { 18152 composeReference("request", element.getRequest()); 18153 } 18154 if (element.hasResponse()) { 18155 composeReference("response", element.getResponse()); 18156 } 18157 if (element.hasDocumentationElement()) { 18158 composeStringCore("documentation", element.getDocumentationElement(), false); 18159 composeStringExtras("documentation", element.getDocumentationElement(), false); 18160 } 18161 } 18162 18163 protected void composeConformanceConformanceDocumentComponent(String name, 18164 Conformance.ConformanceDocumentComponent element) throws IOException { 18165 if (element != null) { 18166 open(name); 18167 composeConformanceConformanceDocumentComponentInner(element); 18168 close(); 18169 } 18170 } 18171 18172 protected void composeConformanceConformanceDocumentComponentInner(Conformance.ConformanceDocumentComponent element) 18173 throws IOException { 18174 composeBackbone(element); 18175 if (element.hasModeElement()) { 18176 composeEnumerationCore("mode", element.getModeElement(), new Conformance.DocumentModeEnumFactory(), false); 18177 composeEnumerationExtras("mode", element.getModeElement(), new Conformance.DocumentModeEnumFactory(), false); 18178 } 18179 if (element.hasDocumentationElement()) { 18180 composeStringCore("documentation", element.getDocumentationElement(), false); 18181 composeStringExtras("documentation", element.getDocumentationElement(), false); 18182 } 18183 if (element.hasProfile()) { 18184 composeReference("profile", element.getProfile()); 18185 } 18186 } 18187 18188 protected void composeContract(String name, Contract element) throws IOException { 18189 if (element != null) { 18190 prop("resourceType", name); 18191 composeContractInner(element); 18192 } 18193 } 18194 18195 protected void composeContractInner(Contract element) throws IOException { 18196 composeDomainResourceElements(element); 18197 if (element.hasIdentifier()) { 18198 composeIdentifier("identifier", element.getIdentifier()); 18199 } 18200 if (element.hasIssuedElement()) { 18201 composeDateTimeCore("issued", element.getIssuedElement(), false); 18202 composeDateTimeExtras("issued", element.getIssuedElement(), false); 18203 } 18204 if (element.hasApplies()) { 18205 composePeriod("applies", element.getApplies()); 18206 } 18207 if (element.hasSubject()) { 18208 openArray("subject"); 18209 for (Reference e : element.getSubject()) 18210 composeReference(null, e); 18211 closeArray(); 18212 } 18213 ; 18214 if (element.hasAuthority()) { 18215 openArray("authority"); 18216 for (Reference e : element.getAuthority()) 18217 composeReference(null, e); 18218 closeArray(); 18219 } 18220 ; 18221 if (element.hasDomain()) { 18222 openArray("domain"); 18223 for (Reference e : element.getDomain()) 18224 composeReference(null, e); 18225 closeArray(); 18226 } 18227 ; 18228 if (element.hasType()) { 18229 composeCodeableConcept("type", element.getType()); 18230 } 18231 if (element.hasSubType()) { 18232 openArray("subType"); 18233 for (CodeableConcept e : element.getSubType()) 18234 composeCodeableConcept(null, e); 18235 closeArray(); 18236 } 18237 ; 18238 if (element.hasAction()) { 18239 openArray("action"); 18240 for (CodeableConcept e : element.getAction()) 18241 composeCodeableConcept(null, e); 18242 closeArray(); 18243 } 18244 ; 18245 if (element.hasActionReason()) { 18246 openArray("actionReason"); 18247 for (CodeableConcept e : element.getActionReason()) 18248 composeCodeableConcept(null, e); 18249 closeArray(); 18250 } 18251 ; 18252 if (element.hasActor()) { 18253 openArray("actor"); 18254 for (Contract.ActorComponent e : element.getActor()) 18255 composeContractActorComponent(null, e); 18256 closeArray(); 18257 } 18258 ; 18259 if (element.hasValuedItem()) { 18260 openArray("valuedItem"); 18261 for (Contract.ValuedItemComponent e : element.getValuedItem()) 18262 composeContractValuedItemComponent(null, e); 18263 closeArray(); 18264 } 18265 ; 18266 if (element.hasSigner()) { 18267 openArray("signer"); 18268 for (Contract.SignatoryComponent e : element.getSigner()) 18269 composeContractSignatoryComponent(null, e); 18270 closeArray(); 18271 } 18272 ; 18273 if (element.hasTerm()) { 18274 openArray("term"); 18275 for (Contract.TermComponent e : element.getTerm()) 18276 composeContractTermComponent(null, e); 18277 closeArray(); 18278 } 18279 ; 18280 if (element.hasBinding()) { 18281 composeType("binding", element.getBinding()); 18282 } 18283 if (element.hasFriendly()) { 18284 openArray("friendly"); 18285 for (Contract.FriendlyLanguageComponent e : element.getFriendly()) 18286 composeContractFriendlyLanguageComponent(null, e); 18287 closeArray(); 18288 } 18289 ; 18290 if (element.hasLegal()) { 18291 openArray("legal"); 18292 for (Contract.LegalLanguageComponent e : element.getLegal()) 18293 composeContractLegalLanguageComponent(null, e); 18294 closeArray(); 18295 } 18296 ; 18297 if (element.hasRule()) { 18298 openArray("rule"); 18299 for (Contract.ComputableLanguageComponent e : element.getRule()) 18300 composeContractComputableLanguageComponent(null, e); 18301 closeArray(); 18302 } 18303 ; 18304 } 18305 18306 protected void composeContractActorComponent(String name, Contract.ActorComponent element) throws IOException { 18307 if (element != null) { 18308 open(name); 18309 composeContractActorComponentInner(element); 18310 close(); 18311 } 18312 } 18313 18314 protected void composeContractActorComponentInner(Contract.ActorComponent element) throws IOException { 18315 composeBackbone(element); 18316 if (element.hasEntity()) { 18317 composeReference("entity", element.getEntity()); 18318 } 18319 if (element.hasRole()) { 18320 openArray("role"); 18321 for (CodeableConcept e : element.getRole()) 18322 composeCodeableConcept(null, e); 18323 closeArray(); 18324 } 18325 ; 18326 } 18327 18328 protected void composeContractValuedItemComponent(String name, Contract.ValuedItemComponent element) 18329 throws IOException { 18330 if (element != null) { 18331 open(name); 18332 composeContractValuedItemComponentInner(element); 18333 close(); 18334 } 18335 } 18336 18337 protected void composeContractValuedItemComponentInner(Contract.ValuedItemComponent element) throws IOException { 18338 composeBackbone(element); 18339 if (element.hasEntity()) { 18340 composeType("entity", element.getEntity()); 18341 } 18342 if (element.hasIdentifier()) { 18343 composeIdentifier("identifier", element.getIdentifier()); 18344 } 18345 if (element.hasEffectiveTimeElement()) { 18346 composeDateTimeCore("effectiveTime", element.getEffectiveTimeElement(), false); 18347 composeDateTimeExtras("effectiveTime", element.getEffectiveTimeElement(), false); 18348 } 18349 if (element.hasQuantity()) { 18350 composeSimpleQuantity("quantity", element.getQuantity()); 18351 } 18352 if (element.hasUnitPrice()) { 18353 composeMoney("unitPrice", element.getUnitPrice()); 18354 } 18355 if (element.hasFactorElement()) { 18356 composeDecimalCore("factor", element.getFactorElement(), false); 18357 composeDecimalExtras("factor", element.getFactorElement(), false); 18358 } 18359 if (element.hasPointsElement()) { 18360 composeDecimalCore("points", element.getPointsElement(), false); 18361 composeDecimalExtras("points", element.getPointsElement(), false); 18362 } 18363 if (element.hasNet()) { 18364 composeMoney("net", element.getNet()); 18365 } 18366 } 18367 18368 protected void composeContractSignatoryComponent(String name, Contract.SignatoryComponent element) 18369 throws IOException { 18370 if (element != null) { 18371 open(name); 18372 composeContractSignatoryComponentInner(element); 18373 close(); 18374 } 18375 } 18376 18377 protected void composeContractSignatoryComponentInner(Contract.SignatoryComponent element) throws IOException { 18378 composeBackbone(element); 18379 if (element.hasType()) { 18380 composeCoding("type", element.getType()); 18381 } 18382 if (element.hasParty()) { 18383 composeReference("party", element.getParty()); 18384 } 18385 if (element.hasSignatureElement()) { 18386 composeStringCore("signature", element.getSignatureElement(), false); 18387 composeStringExtras("signature", element.getSignatureElement(), false); 18388 } 18389 } 18390 18391 protected void composeContractTermComponent(String name, Contract.TermComponent element) throws IOException { 18392 if (element != null) { 18393 open(name); 18394 composeContractTermComponentInner(element); 18395 close(); 18396 } 18397 } 18398 18399 protected void composeContractTermComponentInner(Contract.TermComponent element) throws IOException { 18400 composeBackbone(element); 18401 if (element.hasIdentifier()) { 18402 composeIdentifier("identifier", element.getIdentifier()); 18403 } 18404 if (element.hasIssuedElement()) { 18405 composeDateTimeCore("issued", element.getIssuedElement(), false); 18406 composeDateTimeExtras("issued", element.getIssuedElement(), false); 18407 } 18408 if (element.hasApplies()) { 18409 composePeriod("applies", element.getApplies()); 18410 } 18411 if (element.hasType()) { 18412 composeCodeableConcept("type", element.getType()); 18413 } 18414 if (element.hasSubType()) { 18415 composeCodeableConcept("subType", element.getSubType()); 18416 } 18417 if (element.hasSubject()) { 18418 composeReference("subject", element.getSubject()); 18419 } 18420 if (element.hasAction()) { 18421 openArray("action"); 18422 for (CodeableConcept e : element.getAction()) 18423 composeCodeableConcept(null, e); 18424 closeArray(); 18425 } 18426 ; 18427 if (element.hasActionReason()) { 18428 openArray("actionReason"); 18429 for (CodeableConcept e : element.getActionReason()) 18430 composeCodeableConcept(null, e); 18431 closeArray(); 18432 } 18433 ; 18434 if (element.hasActor()) { 18435 openArray("actor"); 18436 for (Contract.TermActorComponent e : element.getActor()) 18437 composeContractTermActorComponent(null, e); 18438 closeArray(); 18439 } 18440 ; 18441 if (element.hasTextElement()) { 18442 composeStringCore("text", element.getTextElement(), false); 18443 composeStringExtras("text", element.getTextElement(), false); 18444 } 18445 if (element.hasValuedItem()) { 18446 openArray("valuedItem"); 18447 for (Contract.TermValuedItemComponent e : element.getValuedItem()) 18448 composeContractTermValuedItemComponent(null, e); 18449 closeArray(); 18450 } 18451 ; 18452 if (element.hasGroup()) { 18453 openArray("group"); 18454 for (Contract.TermComponent e : element.getGroup()) 18455 composeContractTermComponent(null, e); 18456 closeArray(); 18457 } 18458 ; 18459 } 18460 18461 protected void composeContractTermActorComponent(String name, Contract.TermActorComponent element) 18462 throws IOException { 18463 if (element != null) { 18464 open(name); 18465 composeContractTermActorComponentInner(element); 18466 close(); 18467 } 18468 } 18469 18470 protected void composeContractTermActorComponentInner(Contract.TermActorComponent element) throws IOException { 18471 composeBackbone(element); 18472 if (element.hasEntity()) { 18473 composeReference("entity", element.getEntity()); 18474 } 18475 if (element.hasRole()) { 18476 openArray("role"); 18477 for (CodeableConcept e : element.getRole()) 18478 composeCodeableConcept(null, e); 18479 closeArray(); 18480 } 18481 ; 18482 } 18483 18484 protected void composeContractTermValuedItemComponent(String name, Contract.TermValuedItemComponent element) 18485 throws IOException { 18486 if (element != null) { 18487 open(name); 18488 composeContractTermValuedItemComponentInner(element); 18489 close(); 18490 } 18491 } 18492 18493 protected void composeContractTermValuedItemComponentInner(Contract.TermValuedItemComponent element) 18494 throws IOException { 18495 composeBackbone(element); 18496 if (element.hasEntity()) { 18497 composeType("entity", element.getEntity()); 18498 } 18499 if (element.hasIdentifier()) { 18500 composeIdentifier("identifier", element.getIdentifier()); 18501 } 18502 if (element.hasEffectiveTimeElement()) { 18503 composeDateTimeCore("effectiveTime", element.getEffectiveTimeElement(), false); 18504 composeDateTimeExtras("effectiveTime", element.getEffectiveTimeElement(), false); 18505 } 18506 if (element.hasQuantity()) { 18507 composeSimpleQuantity("quantity", element.getQuantity()); 18508 } 18509 if (element.hasUnitPrice()) { 18510 composeMoney("unitPrice", element.getUnitPrice()); 18511 } 18512 if (element.hasFactorElement()) { 18513 composeDecimalCore("factor", element.getFactorElement(), false); 18514 composeDecimalExtras("factor", element.getFactorElement(), false); 18515 } 18516 if (element.hasPointsElement()) { 18517 composeDecimalCore("points", element.getPointsElement(), false); 18518 composeDecimalExtras("points", element.getPointsElement(), false); 18519 } 18520 if (element.hasNet()) { 18521 composeMoney("net", element.getNet()); 18522 } 18523 } 18524 18525 protected void composeContractFriendlyLanguageComponent(String name, Contract.FriendlyLanguageComponent element) 18526 throws IOException { 18527 if (element != null) { 18528 open(name); 18529 composeContractFriendlyLanguageComponentInner(element); 18530 close(); 18531 } 18532 } 18533 18534 protected void composeContractFriendlyLanguageComponentInner(Contract.FriendlyLanguageComponent element) 18535 throws IOException { 18536 composeBackbone(element); 18537 if (element.hasContent()) { 18538 composeType("content", element.getContent()); 18539 } 18540 } 18541 18542 protected void composeContractLegalLanguageComponent(String name, Contract.LegalLanguageComponent element) 18543 throws IOException { 18544 if (element != null) { 18545 open(name); 18546 composeContractLegalLanguageComponentInner(element); 18547 close(); 18548 } 18549 } 18550 18551 protected void composeContractLegalLanguageComponentInner(Contract.LegalLanguageComponent element) 18552 throws IOException { 18553 composeBackbone(element); 18554 if (element.hasContent()) { 18555 composeType("content", element.getContent()); 18556 } 18557 } 18558 18559 protected void composeContractComputableLanguageComponent(String name, Contract.ComputableLanguageComponent element) 18560 throws IOException { 18561 if (element != null) { 18562 open(name); 18563 composeContractComputableLanguageComponentInner(element); 18564 close(); 18565 } 18566 } 18567 18568 protected void composeContractComputableLanguageComponentInner(Contract.ComputableLanguageComponent element) 18569 throws IOException { 18570 composeBackbone(element); 18571 if (element.hasContent()) { 18572 composeType("content", element.getContent()); 18573 } 18574 } 18575 18576 protected void composeCoverage(String name, Coverage element) throws IOException { 18577 if (element != null) { 18578 prop("resourceType", name); 18579 composeCoverageInner(element); 18580 } 18581 } 18582 18583 protected void composeCoverageInner(Coverage element) throws IOException { 18584 composeDomainResourceElements(element); 18585 if (element.hasIssuer()) { 18586 composeReference("issuer", element.getIssuer()); 18587 } 18588 if (element.hasBin()) { 18589 composeIdentifier("bin", element.getBin()); 18590 } 18591 if (element.hasPeriod()) { 18592 composePeriod("period", element.getPeriod()); 18593 } 18594 if (element.hasType()) { 18595 composeCoding("type", element.getType()); 18596 } 18597 if (element.hasSubscriberId()) { 18598 composeIdentifier("subscriberId", element.getSubscriberId()); 18599 } 18600 if (element.hasIdentifier()) { 18601 openArray("identifier"); 18602 for (Identifier e : element.getIdentifier()) 18603 composeIdentifier(null, e); 18604 closeArray(); 18605 } 18606 ; 18607 if (element.hasGroupElement()) { 18608 composeStringCore("group", element.getGroupElement(), false); 18609 composeStringExtras("group", element.getGroupElement(), false); 18610 } 18611 if (element.hasPlanElement()) { 18612 composeStringCore("plan", element.getPlanElement(), false); 18613 composeStringExtras("plan", element.getPlanElement(), false); 18614 } 18615 if (element.hasSubPlanElement()) { 18616 composeStringCore("subPlan", element.getSubPlanElement(), false); 18617 composeStringExtras("subPlan", element.getSubPlanElement(), false); 18618 } 18619 if (element.hasDependentElement()) { 18620 composePositiveIntCore("dependent", element.getDependentElement(), false); 18621 composePositiveIntExtras("dependent", element.getDependentElement(), false); 18622 } 18623 if (element.hasSequenceElement()) { 18624 composePositiveIntCore("sequence", element.getSequenceElement(), false); 18625 composePositiveIntExtras("sequence", element.getSequenceElement(), false); 18626 } 18627 if (element.hasSubscriber()) { 18628 composeReference("subscriber", element.getSubscriber()); 18629 } 18630 if (element.hasNetwork()) { 18631 composeIdentifier("network", element.getNetwork()); 18632 } 18633 if (element.hasContract()) { 18634 openArray("contract"); 18635 for (Reference e : element.getContract()) 18636 composeReference(null, e); 18637 closeArray(); 18638 } 18639 ; 18640 } 18641 18642 protected void composeDataElement(String name, DataElement element) throws IOException { 18643 if (element != null) { 18644 prop("resourceType", name); 18645 composeDataElementInner(element); 18646 } 18647 } 18648 18649 protected void composeDataElementInner(DataElement element) throws IOException { 18650 composeDomainResourceElements(element); 18651 if (element.hasUrlElement()) { 18652 composeUriCore("url", element.getUrlElement(), false); 18653 composeUriExtras("url", element.getUrlElement(), false); 18654 } 18655 if (element.hasIdentifier()) { 18656 openArray("identifier"); 18657 for (Identifier e : element.getIdentifier()) 18658 composeIdentifier(null, e); 18659 closeArray(); 18660 } 18661 ; 18662 if (element.hasVersionElement()) { 18663 composeStringCore("version", element.getVersionElement(), false); 18664 composeStringExtras("version", element.getVersionElement(), false); 18665 } 18666 if (element.hasNameElement()) { 18667 composeStringCore("name", element.getNameElement(), false); 18668 composeStringExtras("name", element.getNameElement(), false); 18669 } 18670 if (element.hasStatusElement()) { 18671 composeEnumerationCore("status", element.getStatusElement(), 18672 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 18673 composeEnumerationExtras("status", element.getStatusElement(), 18674 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 18675 } 18676 if (element.hasExperimentalElement()) { 18677 composeBooleanCore("experimental", element.getExperimentalElement(), false); 18678 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 18679 } 18680 if (element.hasPublisherElement()) { 18681 composeStringCore("publisher", element.getPublisherElement(), false); 18682 composeStringExtras("publisher", element.getPublisherElement(), false); 18683 } 18684 if (element.hasContact()) { 18685 openArray("contact"); 18686 for (DataElement.DataElementContactComponent e : element.getContact()) 18687 composeDataElementDataElementContactComponent(null, e); 18688 closeArray(); 18689 } 18690 ; 18691 if (element.hasDateElement()) { 18692 composeDateTimeCore("date", element.getDateElement(), false); 18693 composeDateTimeExtras("date", element.getDateElement(), false); 18694 } 18695 if (element.hasUseContext()) { 18696 openArray("useContext"); 18697 for (CodeableConcept e : element.getUseContext()) 18698 composeCodeableConcept(null, e); 18699 closeArray(); 18700 } 18701 ; 18702 if (element.hasCopyrightElement()) { 18703 composeStringCore("copyright", element.getCopyrightElement(), false); 18704 composeStringExtras("copyright", element.getCopyrightElement(), false); 18705 } 18706 if (element.hasStringencyElement()) { 18707 composeEnumerationCore("stringency", element.getStringencyElement(), 18708 new DataElement.DataElementStringencyEnumFactory(), false); 18709 composeEnumerationExtras("stringency", element.getStringencyElement(), 18710 new DataElement.DataElementStringencyEnumFactory(), false); 18711 } 18712 if (element.hasMapping()) { 18713 openArray("mapping"); 18714 for (DataElement.DataElementMappingComponent e : element.getMapping()) 18715 composeDataElementDataElementMappingComponent(null, e); 18716 closeArray(); 18717 } 18718 ; 18719 if (element.hasElement()) { 18720 openArray("element"); 18721 for (ElementDefinition e : element.getElement()) 18722 composeElementDefinition(null, e); 18723 closeArray(); 18724 } 18725 ; 18726 } 18727 18728 protected void composeDataElementDataElementContactComponent(String name, 18729 DataElement.DataElementContactComponent element) throws IOException { 18730 if (element != null) { 18731 open(name); 18732 composeDataElementDataElementContactComponentInner(element); 18733 close(); 18734 } 18735 } 18736 18737 protected void composeDataElementDataElementContactComponentInner(DataElement.DataElementContactComponent element) 18738 throws IOException { 18739 composeBackbone(element); 18740 if (element.hasNameElement()) { 18741 composeStringCore("name", element.getNameElement(), false); 18742 composeStringExtras("name", element.getNameElement(), false); 18743 } 18744 if (element.hasTelecom()) { 18745 openArray("telecom"); 18746 for (ContactPoint e : element.getTelecom()) 18747 composeContactPoint(null, e); 18748 closeArray(); 18749 } 18750 ; 18751 } 18752 18753 protected void composeDataElementDataElementMappingComponent(String name, 18754 DataElement.DataElementMappingComponent element) throws IOException { 18755 if (element != null) { 18756 open(name); 18757 composeDataElementDataElementMappingComponentInner(element); 18758 close(); 18759 } 18760 } 18761 18762 protected void composeDataElementDataElementMappingComponentInner(DataElement.DataElementMappingComponent element) 18763 throws IOException { 18764 composeBackbone(element); 18765 if (element.hasIdentityElement()) { 18766 composeIdCore("identity", element.getIdentityElement(), false); 18767 composeIdExtras("identity", element.getIdentityElement(), false); 18768 } 18769 if (element.hasUriElement()) { 18770 composeUriCore("uri", element.getUriElement(), false); 18771 composeUriExtras("uri", element.getUriElement(), false); 18772 } 18773 if (element.hasNameElement()) { 18774 composeStringCore("name", element.getNameElement(), false); 18775 composeStringExtras("name", element.getNameElement(), false); 18776 } 18777 if (element.hasCommentsElement()) { 18778 composeStringCore("comments", element.getCommentsElement(), false); 18779 composeStringExtras("comments", element.getCommentsElement(), false); 18780 } 18781 } 18782 18783 protected void composeDetectedIssue(String name, DetectedIssue element) throws IOException { 18784 if (element != null) { 18785 prop("resourceType", name); 18786 composeDetectedIssueInner(element); 18787 } 18788 } 18789 18790 protected void composeDetectedIssueInner(DetectedIssue element) throws IOException { 18791 composeDomainResourceElements(element); 18792 if (element.hasPatient()) { 18793 composeReference("patient", element.getPatient()); 18794 } 18795 if (element.hasCategory()) { 18796 composeCodeableConcept("category", element.getCategory()); 18797 } 18798 if (element.hasSeverityElement()) { 18799 composeEnumerationCore("severity", element.getSeverityElement(), 18800 new DetectedIssue.DetectedIssueSeverityEnumFactory(), false); 18801 composeEnumerationExtras("severity", element.getSeverityElement(), 18802 new DetectedIssue.DetectedIssueSeverityEnumFactory(), false); 18803 } 18804 if (element.hasImplicated()) { 18805 openArray("implicated"); 18806 for (Reference e : element.getImplicated()) 18807 composeReference(null, e); 18808 closeArray(); 18809 } 18810 ; 18811 if (element.hasDetailElement()) { 18812 composeStringCore("detail", element.getDetailElement(), false); 18813 composeStringExtras("detail", element.getDetailElement(), false); 18814 } 18815 if (element.hasDateElement()) { 18816 composeDateTimeCore("date", element.getDateElement(), false); 18817 composeDateTimeExtras("date", element.getDateElement(), false); 18818 } 18819 if (element.hasAuthor()) { 18820 composeReference("author", element.getAuthor()); 18821 } 18822 if (element.hasIdentifier()) { 18823 composeIdentifier("identifier", element.getIdentifier()); 18824 } 18825 if (element.hasReferenceElement()) { 18826 composeUriCore("reference", element.getReferenceElement(), false); 18827 composeUriExtras("reference", element.getReferenceElement(), false); 18828 } 18829 if (element.hasMitigation()) { 18830 openArray("mitigation"); 18831 for (DetectedIssue.DetectedIssueMitigationComponent e : element.getMitigation()) 18832 composeDetectedIssueDetectedIssueMitigationComponent(null, e); 18833 closeArray(); 18834 } 18835 ; 18836 } 18837 18838 protected void composeDetectedIssueDetectedIssueMitigationComponent(String name, 18839 DetectedIssue.DetectedIssueMitigationComponent element) throws IOException { 18840 if (element != null) { 18841 open(name); 18842 composeDetectedIssueDetectedIssueMitigationComponentInner(element); 18843 close(); 18844 } 18845 } 18846 18847 protected void composeDetectedIssueDetectedIssueMitigationComponentInner( 18848 DetectedIssue.DetectedIssueMitigationComponent element) throws IOException { 18849 composeBackbone(element); 18850 if (element.hasAction()) { 18851 composeCodeableConcept("action", element.getAction()); 18852 } 18853 if (element.hasDateElement()) { 18854 composeDateTimeCore("date", element.getDateElement(), false); 18855 composeDateTimeExtras("date", element.getDateElement(), false); 18856 } 18857 if (element.hasAuthor()) { 18858 composeReference("author", element.getAuthor()); 18859 } 18860 } 18861 18862 protected void composeDevice(String name, Device element) throws IOException { 18863 if (element != null) { 18864 prop("resourceType", name); 18865 composeDeviceInner(element); 18866 } 18867 } 18868 18869 protected void composeDeviceInner(Device element) throws IOException { 18870 composeDomainResourceElements(element); 18871 if (element.hasIdentifier()) { 18872 openArray("identifier"); 18873 for (Identifier e : element.getIdentifier()) 18874 composeIdentifier(null, e); 18875 closeArray(); 18876 } 18877 ; 18878 if (element.hasType()) { 18879 composeCodeableConcept("type", element.getType()); 18880 } 18881 if (element.hasNote()) { 18882 openArray("note"); 18883 for (Annotation e : element.getNote()) 18884 composeAnnotation(null, e); 18885 closeArray(); 18886 } 18887 ; 18888 if (element.hasStatusElement()) { 18889 composeEnumerationCore("status", element.getStatusElement(), new Device.DeviceStatusEnumFactory(), false); 18890 composeEnumerationExtras("status", element.getStatusElement(), new Device.DeviceStatusEnumFactory(), false); 18891 } 18892 if (element.hasManufacturerElement()) { 18893 composeStringCore("manufacturer", element.getManufacturerElement(), false); 18894 composeStringExtras("manufacturer", element.getManufacturerElement(), false); 18895 } 18896 if (element.hasModelElement()) { 18897 composeStringCore("model", element.getModelElement(), false); 18898 composeStringExtras("model", element.getModelElement(), false); 18899 } 18900 if (element.hasVersionElement()) { 18901 composeStringCore("version", element.getVersionElement(), false); 18902 composeStringExtras("version", element.getVersionElement(), false); 18903 } 18904 if (element.hasManufactureDateElement()) { 18905 composeDateTimeCore("manufactureDate", element.getManufactureDateElement(), false); 18906 composeDateTimeExtras("manufactureDate", element.getManufactureDateElement(), false); 18907 } 18908 if (element.hasExpiryElement()) { 18909 composeDateTimeCore("expiry", element.getExpiryElement(), false); 18910 composeDateTimeExtras("expiry", element.getExpiryElement(), false); 18911 } 18912 if (element.hasUdiElement()) { 18913 composeStringCore("udi", element.getUdiElement(), false); 18914 composeStringExtras("udi", element.getUdiElement(), false); 18915 } 18916 if (element.hasLotNumberElement()) { 18917 composeStringCore("lotNumber", element.getLotNumberElement(), false); 18918 composeStringExtras("lotNumber", element.getLotNumberElement(), false); 18919 } 18920 if (element.hasOwner()) { 18921 composeReference("owner", element.getOwner()); 18922 } 18923 if (element.hasLocation()) { 18924 composeReference("location", element.getLocation()); 18925 } 18926 if (element.hasPatient()) { 18927 composeReference("patient", element.getPatient()); 18928 } 18929 if (element.hasContact()) { 18930 openArray("contact"); 18931 for (ContactPoint e : element.getContact()) 18932 composeContactPoint(null, e); 18933 closeArray(); 18934 } 18935 ; 18936 if (element.hasUrlElement()) { 18937 composeUriCore("url", element.getUrlElement(), false); 18938 composeUriExtras("url", element.getUrlElement(), false); 18939 } 18940 } 18941 18942 protected void composeDeviceComponent(String name, DeviceComponent element) throws IOException { 18943 if (element != null) { 18944 prop("resourceType", name); 18945 composeDeviceComponentInner(element); 18946 } 18947 } 18948 18949 protected void composeDeviceComponentInner(DeviceComponent element) throws IOException { 18950 composeDomainResourceElements(element); 18951 if (element.hasType()) { 18952 composeCodeableConcept("type", element.getType()); 18953 } 18954 if (element.hasIdentifier()) { 18955 composeIdentifier("identifier", element.getIdentifier()); 18956 } 18957 if (element.hasLastSystemChangeElement()) { 18958 composeInstantCore("lastSystemChange", element.getLastSystemChangeElement(), false); 18959 composeInstantExtras("lastSystemChange", element.getLastSystemChangeElement(), false); 18960 } 18961 if (element.hasSource()) { 18962 composeReference("source", element.getSource()); 18963 } 18964 if (element.hasParent()) { 18965 composeReference("parent", element.getParent()); 18966 } 18967 if (element.hasOperationalStatus()) { 18968 openArray("operationalStatus"); 18969 for (CodeableConcept e : element.getOperationalStatus()) 18970 composeCodeableConcept(null, e); 18971 closeArray(); 18972 } 18973 ; 18974 if (element.hasParameterGroup()) { 18975 composeCodeableConcept("parameterGroup", element.getParameterGroup()); 18976 } 18977 if (element.hasMeasurementPrincipleElement()) { 18978 composeEnumerationCore("measurementPrinciple", element.getMeasurementPrincipleElement(), 18979 new DeviceComponent.MeasmntPrincipleEnumFactory(), false); 18980 composeEnumerationExtras("measurementPrinciple", element.getMeasurementPrincipleElement(), 18981 new DeviceComponent.MeasmntPrincipleEnumFactory(), false); 18982 } 18983 if (element.hasProductionSpecification()) { 18984 openArray("productionSpecification"); 18985 for (DeviceComponent.DeviceComponentProductionSpecificationComponent e : element.getProductionSpecification()) 18986 composeDeviceComponentDeviceComponentProductionSpecificationComponent(null, e); 18987 closeArray(); 18988 } 18989 ; 18990 if (element.hasLanguageCode()) { 18991 composeCodeableConcept("languageCode", element.getLanguageCode()); 18992 } 18993 } 18994 18995 protected void composeDeviceComponentDeviceComponentProductionSpecificationComponent(String name, 18996 DeviceComponent.DeviceComponentProductionSpecificationComponent element) throws IOException { 18997 if (element != null) { 18998 open(name); 18999 composeDeviceComponentDeviceComponentProductionSpecificationComponentInner(element); 19000 close(); 19001 } 19002 } 19003 19004 protected void composeDeviceComponentDeviceComponentProductionSpecificationComponentInner( 19005 DeviceComponent.DeviceComponentProductionSpecificationComponent element) throws IOException { 19006 composeBackbone(element); 19007 if (element.hasSpecType()) { 19008 composeCodeableConcept("specType", element.getSpecType()); 19009 } 19010 if (element.hasComponentId()) { 19011 composeIdentifier("componentId", element.getComponentId()); 19012 } 19013 if (element.hasProductionSpecElement()) { 19014 composeStringCore("productionSpec", element.getProductionSpecElement(), false); 19015 composeStringExtras("productionSpec", element.getProductionSpecElement(), false); 19016 } 19017 } 19018 19019 protected void composeDeviceMetric(String name, DeviceMetric element) throws IOException { 19020 if (element != null) { 19021 prop("resourceType", name); 19022 composeDeviceMetricInner(element); 19023 } 19024 } 19025 19026 protected void composeDeviceMetricInner(DeviceMetric element) throws IOException { 19027 composeDomainResourceElements(element); 19028 if (element.hasType()) { 19029 composeCodeableConcept("type", element.getType()); 19030 } 19031 if (element.hasIdentifier()) { 19032 composeIdentifier("identifier", element.getIdentifier()); 19033 } 19034 if (element.hasUnit()) { 19035 composeCodeableConcept("unit", element.getUnit()); 19036 } 19037 if (element.hasSource()) { 19038 composeReference("source", element.getSource()); 19039 } 19040 if (element.hasParent()) { 19041 composeReference("parent", element.getParent()); 19042 } 19043 if (element.hasOperationalStatusElement()) { 19044 composeEnumerationCore("operationalStatus", element.getOperationalStatusElement(), 19045 new DeviceMetric.DeviceMetricOperationalStatusEnumFactory(), false); 19046 composeEnumerationExtras("operationalStatus", element.getOperationalStatusElement(), 19047 new DeviceMetric.DeviceMetricOperationalStatusEnumFactory(), false); 19048 } 19049 if (element.hasColorElement()) { 19050 composeEnumerationCore("color", element.getColorElement(), new DeviceMetric.DeviceMetricColorEnumFactory(), 19051 false); 19052 composeEnumerationExtras("color", element.getColorElement(), new DeviceMetric.DeviceMetricColorEnumFactory(), 19053 false); 19054 } 19055 if (element.hasCategoryElement()) { 19056 composeEnumerationCore("category", element.getCategoryElement(), 19057 new DeviceMetric.DeviceMetricCategoryEnumFactory(), false); 19058 composeEnumerationExtras("category", element.getCategoryElement(), 19059 new DeviceMetric.DeviceMetricCategoryEnumFactory(), false); 19060 } 19061 if (element.hasMeasurementPeriod()) { 19062 composeTiming("measurementPeriod", element.getMeasurementPeriod()); 19063 } 19064 if (element.hasCalibration()) { 19065 openArray("calibration"); 19066 for (DeviceMetric.DeviceMetricCalibrationComponent e : element.getCalibration()) 19067 composeDeviceMetricDeviceMetricCalibrationComponent(null, e); 19068 closeArray(); 19069 } 19070 ; 19071 } 19072 19073 protected void composeDeviceMetricDeviceMetricCalibrationComponent(String name, 19074 DeviceMetric.DeviceMetricCalibrationComponent element) throws IOException { 19075 if (element != null) { 19076 open(name); 19077 composeDeviceMetricDeviceMetricCalibrationComponentInner(element); 19078 close(); 19079 } 19080 } 19081 19082 protected void composeDeviceMetricDeviceMetricCalibrationComponentInner( 19083 DeviceMetric.DeviceMetricCalibrationComponent element) throws IOException { 19084 composeBackbone(element); 19085 if (element.hasTypeElement()) { 19086 composeEnumerationCore("type", element.getTypeElement(), 19087 new DeviceMetric.DeviceMetricCalibrationTypeEnumFactory(), false); 19088 composeEnumerationExtras("type", element.getTypeElement(), 19089 new DeviceMetric.DeviceMetricCalibrationTypeEnumFactory(), false); 19090 } 19091 if (element.hasStateElement()) { 19092 composeEnumerationCore("state", element.getStateElement(), 19093 new DeviceMetric.DeviceMetricCalibrationStateEnumFactory(), false); 19094 composeEnumerationExtras("state", element.getStateElement(), 19095 new DeviceMetric.DeviceMetricCalibrationStateEnumFactory(), false); 19096 } 19097 if (element.hasTimeElement()) { 19098 composeInstantCore("time", element.getTimeElement(), false); 19099 composeInstantExtras("time", element.getTimeElement(), false); 19100 } 19101 } 19102 19103 protected void composeDeviceUseRequest(String name, DeviceUseRequest element) throws IOException { 19104 if (element != null) { 19105 prop("resourceType", name); 19106 composeDeviceUseRequestInner(element); 19107 } 19108 } 19109 19110 protected void composeDeviceUseRequestInner(DeviceUseRequest element) throws IOException { 19111 composeDomainResourceElements(element); 19112 if (element.hasBodySite()) { 19113 composeType("bodySite", element.getBodySite()); 19114 } 19115 if (element.hasStatusElement()) { 19116 composeEnumerationCore("status", element.getStatusElement(), 19117 new DeviceUseRequest.DeviceUseRequestStatusEnumFactory(), false); 19118 composeEnumerationExtras("status", element.getStatusElement(), 19119 new DeviceUseRequest.DeviceUseRequestStatusEnumFactory(), false); 19120 } 19121 if (element.hasDevice()) { 19122 composeReference("device", element.getDevice()); 19123 } 19124 if (element.hasEncounter()) { 19125 composeReference("encounter", element.getEncounter()); 19126 } 19127 if (element.hasIdentifier()) { 19128 openArray("identifier"); 19129 for (Identifier e : element.getIdentifier()) 19130 composeIdentifier(null, e); 19131 closeArray(); 19132 } 19133 ; 19134 if (element.hasIndication()) { 19135 openArray("indication"); 19136 for (CodeableConcept e : element.getIndication()) 19137 composeCodeableConcept(null, e); 19138 closeArray(); 19139 } 19140 ; 19141 if (element.hasNotes()) { 19142 openArray("notes"); 19143 for (StringType e : element.getNotes()) 19144 composeStringCore(null, e, true); 19145 closeArray(); 19146 if (anyHasExtras(element.getNotes())) { 19147 openArray("_notes"); 19148 for (StringType e : element.getNotes()) 19149 composeStringExtras(null, e, true); 19150 closeArray(); 19151 } 19152 } 19153 ; 19154 if (element.hasPrnReason()) { 19155 openArray("prnReason"); 19156 for (CodeableConcept e : element.getPrnReason()) 19157 composeCodeableConcept(null, e); 19158 closeArray(); 19159 } 19160 ; 19161 if (element.hasOrderedOnElement()) { 19162 composeDateTimeCore("orderedOn", element.getOrderedOnElement(), false); 19163 composeDateTimeExtras("orderedOn", element.getOrderedOnElement(), false); 19164 } 19165 if (element.hasRecordedOnElement()) { 19166 composeDateTimeCore("recordedOn", element.getRecordedOnElement(), false); 19167 composeDateTimeExtras("recordedOn", element.getRecordedOnElement(), false); 19168 } 19169 if (element.hasSubject()) { 19170 composeReference("subject", element.getSubject()); 19171 } 19172 if (element.hasTiming()) { 19173 composeType("timing", element.getTiming()); 19174 } 19175 if (element.hasPriorityElement()) { 19176 composeEnumerationCore("priority", element.getPriorityElement(), 19177 new DeviceUseRequest.DeviceUseRequestPriorityEnumFactory(), false); 19178 composeEnumerationExtras("priority", element.getPriorityElement(), 19179 new DeviceUseRequest.DeviceUseRequestPriorityEnumFactory(), false); 19180 } 19181 } 19182 19183 protected void composeDeviceUseStatement(String name, DeviceUseStatement element) throws IOException { 19184 if (element != null) { 19185 prop("resourceType", name); 19186 composeDeviceUseStatementInner(element); 19187 } 19188 } 19189 19190 protected void composeDeviceUseStatementInner(DeviceUseStatement element) throws IOException { 19191 composeDomainResourceElements(element); 19192 if (element.hasBodySite()) { 19193 composeType("bodySite", element.getBodySite()); 19194 } 19195 if (element.hasWhenUsed()) { 19196 composePeriod("whenUsed", element.getWhenUsed()); 19197 } 19198 if (element.hasDevice()) { 19199 composeReference("device", element.getDevice()); 19200 } 19201 if (element.hasIdentifier()) { 19202 openArray("identifier"); 19203 for (Identifier e : element.getIdentifier()) 19204 composeIdentifier(null, e); 19205 closeArray(); 19206 } 19207 ; 19208 if (element.hasIndication()) { 19209 openArray("indication"); 19210 for (CodeableConcept e : element.getIndication()) 19211 composeCodeableConcept(null, e); 19212 closeArray(); 19213 } 19214 ; 19215 if (element.hasNotes()) { 19216 openArray("notes"); 19217 for (StringType e : element.getNotes()) 19218 composeStringCore(null, e, true); 19219 closeArray(); 19220 if (anyHasExtras(element.getNotes())) { 19221 openArray("_notes"); 19222 for (StringType e : element.getNotes()) 19223 composeStringExtras(null, e, true); 19224 closeArray(); 19225 } 19226 } 19227 ; 19228 if (element.hasRecordedOnElement()) { 19229 composeDateTimeCore("recordedOn", element.getRecordedOnElement(), false); 19230 composeDateTimeExtras("recordedOn", element.getRecordedOnElement(), false); 19231 } 19232 if (element.hasSubject()) { 19233 composeReference("subject", element.getSubject()); 19234 } 19235 if (element.hasTiming()) { 19236 composeType("timing", element.getTiming()); 19237 } 19238 } 19239 19240 protected void composeDiagnosticOrder(String name, DiagnosticOrder element) throws IOException { 19241 if (element != null) { 19242 prop("resourceType", name); 19243 composeDiagnosticOrderInner(element); 19244 } 19245 } 19246 19247 protected void composeDiagnosticOrderInner(DiagnosticOrder element) throws IOException { 19248 composeDomainResourceElements(element); 19249 if (element.hasSubject()) { 19250 composeReference("subject", element.getSubject()); 19251 } 19252 if (element.hasOrderer()) { 19253 composeReference("orderer", element.getOrderer()); 19254 } 19255 if (element.hasIdentifier()) { 19256 openArray("identifier"); 19257 for (Identifier e : element.getIdentifier()) 19258 composeIdentifier(null, e); 19259 closeArray(); 19260 } 19261 ; 19262 if (element.hasEncounter()) { 19263 composeReference("encounter", element.getEncounter()); 19264 } 19265 if (element.hasReason()) { 19266 openArray("reason"); 19267 for (CodeableConcept e : element.getReason()) 19268 composeCodeableConcept(null, e); 19269 closeArray(); 19270 } 19271 ; 19272 if (element.hasSupportingInformation()) { 19273 openArray("supportingInformation"); 19274 for (Reference e : element.getSupportingInformation()) 19275 composeReference(null, e); 19276 closeArray(); 19277 } 19278 ; 19279 if (element.hasSpecimen()) { 19280 openArray("specimen"); 19281 for (Reference e : element.getSpecimen()) 19282 composeReference(null, e); 19283 closeArray(); 19284 } 19285 ; 19286 if (element.hasStatusElement()) { 19287 composeEnumerationCore("status", element.getStatusElement(), 19288 new DiagnosticOrder.DiagnosticOrderStatusEnumFactory(), false); 19289 composeEnumerationExtras("status", element.getStatusElement(), 19290 new DiagnosticOrder.DiagnosticOrderStatusEnumFactory(), false); 19291 } 19292 if (element.hasPriorityElement()) { 19293 composeEnumerationCore("priority", element.getPriorityElement(), 19294 new DiagnosticOrder.DiagnosticOrderPriorityEnumFactory(), false); 19295 composeEnumerationExtras("priority", element.getPriorityElement(), 19296 new DiagnosticOrder.DiagnosticOrderPriorityEnumFactory(), false); 19297 } 19298 if (element.hasEvent()) { 19299 openArray("event"); 19300 for (DiagnosticOrder.DiagnosticOrderEventComponent e : element.getEvent()) 19301 composeDiagnosticOrderDiagnosticOrderEventComponent(null, e); 19302 closeArray(); 19303 } 19304 ; 19305 if (element.hasItem()) { 19306 openArray("item"); 19307 for (DiagnosticOrder.DiagnosticOrderItemComponent e : element.getItem()) 19308 composeDiagnosticOrderDiagnosticOrderItemComponent(null, e); 19309 closeArray(); 19310 } 19311 ; 19312 if (element.hasNote()) { 19313 openArray("note"); 19314 for (Annotation e : element.getNote()) 19315 composeAnnotation(null, e); 19316 closeArray(); 19317 } 19318 ; 19319 } 19320 19321 protected void composeDiagnosticOrderDiagnosticOrderEventComponent(String name, 19322 DiagnosticOrder.DiagnosticOrderEventComponent element) throws IOException { 19323 if (element != null) { 19324 open(name); 19325 composeDiagnosticOrderDiagnosticOrderEventComponentInner(element); 19326 close(); 19327 } 19328 } 19329 19330 protected void composeDiagnosticOrderDiagnosticOrderEventComponentInner( 19331 DiagnosticOrder.DiagnosticOrderEventComponent element) throws IOException { 19332 composeBackbone(element); 19333 if (element.hasStatusElement()) { 19334 composeEnumerationCore("status", element.getStatusElement(), 19335 new DiagnosticOrder.DiagnosticOrderStatusEnumFactory(), false); 19336 composeEnumerationExtras("status", element.getStatusElement(), 19337 new DiagnosticOrder.DiagnosticOrderStatusEnumFactory(), false); 19338 } 19339 if (element.hasDescription()) { 19340 composeCodeableConcept("description", element.getDescription()); 19341 } 19342 if (element.hasDateTimeElement()) { 19343 composeDateTimeCore("dateTime", element.getDateTimeElement(), false); 19344 composeDateTimeExtras("dateTime", element.getDateTimeElement(), false); 19345 } 19346 if (element.hasActor()) { 19347 composeReference("actor", element.getActor()); 19348 } 19349 } 19350 19351 protected void composeDiagnosticOrderDiagnosticOrderItemComponent(String name, 19352 DiagnosticOrder.DiagnosticOrderItemComponent element) throws IOException { 19353 if (element != null) { 19354 open(name); 19355 composeDiagnosticOrderDiagnosticOrderItemComponentInner(element); 19356 close(); 19357 } 19358 } 19359 19360 protected void composeDiagnosticOrderDiagnosticOrderItemComponentInner( 19361 DiagnosticOrder.DiagnosticOrderItemComponent element) throws IOException { 19362 composeBackbone(element); 19363 if (element.hasCode()) { 19364 composeCodeableConcept("code", element.getCode()); 19365 } 19366 if (element.hasSpecimen()) { 19367 openArray("specimen"); 19368 for (Reference e : element.getSpecimen()) 19369 composeReference(null, e); 19370 closeArray(); 19371 } 19372 ; 19373 if (element.hasBodySite()) { 19374 composeCodeableConcept("bodySite", element.getBodySite()); 19375 } 19376 if (element.hasStatusElement()) { 19377 composeEnumerationCore("status", element.getStatusElement(), 19378 new DiagnosticOrder.DiagnosticOrderStatusEnumFactory(), false); 19379 composeEnumerationExtras("status", element.getStatusElement(), 19380 new DiagnosticOrder.DiagnosticOrderStatusEnumFactory(), false); 19381 } 19382 if (element.hasEvent()) { 19383 openArray("event"); 19384 for (DiagnosticOrder.DiagnosticOrderEventComponent e : element.getEvent()) 19385 composeDiagnosticOrderDiagnosticOrderEventComponent(null, e); 19386 closeArray(); 19387 } 19388 ; 19389 } 19390 19391 protected void composeDiagnosticReport(String name, DiagnosticReport element) throws IOException { 19392 if (element != null) { 19393 prop("resourceType", name); 19394 composeDiagnosticReportInner(element); 19395 } 19396 } 19397 19398 protected void composeDiagnosticReportInner(DiagnosticReport element) throws IOException { 19399 composeDomainResourceElements(element); 19400 if (element.hasIdentifier()) { 19401 openArray("identifier"); 19402 for (Identifier e : element.getIdentifier()) 19403 composeIdentifier(null, e); 19404 closeArray(); 19405 } 19406 ; 19407 if (element.hasStatusElement()) { 19408 composeEnumerationCore("status", element.getStatusElement(), 19409 new DiagnosticReport.DiagnosticReportStatusEnumFactory(), false); 19410 composeEnumerationExtras("status", element.getStatusElement(), 19411 new DiagnosticReport.DiagnosticReportStatusEnumFactory(), false); 19412 } 19413 if (element.hasCategory()) { 19414 composeCodeableConcept("category", element.getCategory()); 19415 } 19416 if (element.hasCode()) { 19417 composeCodeableConcept("code", element.getCode()); 19418 } 19419 if (element.hasSubject()) { 19420 composeReference("subject", element.getSubject()); 19421 } 19422 if (element.hasEncounter()) { 19423 composeReference("encounter", element.getEncounter()); 19424 } 19425 if (element.hasEffective()) { 19426 composeType("effective", element.getEffective()); 19427 } 19428 if (element.hasIssuedElement()) { 19429 composeInstantCore("issued", element.getIssuedElement(), false); 19430 composeInstantExtras("issued", element.getIssuedElement(), false); 19431 } 19432 if (element.hasPerformer()) { 19433 composeReference("performer", element.getPerformer()); 19434 } 19435 if (element.hasRequest()) { 19436 openArray("request"); 19437 for (Reference e : element.getRequest()) 19438 composeReference(null, e); 19439 closeArray(); 19440 } 19441 ; 19442 if (element.hasSpecimen()) { 19443 openArray("specimen"); 19444 for (Reference e : element.getSpecimen()) 19445 composeReference(null, e); 19446 closeArray(); 19447 } 19448 ; 19449 if (element.hasResult()) { 19450 openArray("result"); 19451 for (Reference e : element.getResult()) 19452 composeReference(null, e); 19453 closeArray(); 19454 } 19455 ; 19456 if (element.hasImagingStudy()) { 19457 openArray("imagingStudy"); 19458 for (Reference e : element.getImagingStudy()) 19459 composeReference(null, e); 19460 closeArray(); 19461 } 19462 ; 19463 if (element.hasImage()) { 19464 openArray("image"); 19465 for (DiagnosticReport.DiagnosticReportImageComponent e : element.getImage()) 19466 composeDiagnosticReportDiagnosticReportImageComponent(null, e); 19467 closeArray(); 19468 } 19469 ; 19470 if (element.hasConclusionElement()) { 19471 composeStringCore("conclusion", element.getConclusionElement(), false); 19472 composeStringExtras("conclusion", element.getConclusionElement(), false); 19473 } 19474 if (element.hasCodedDiagnosis()) { 19475 openArray("codedDiagnosis"); 19476 for (CodeableConcept e : element.getCodedDiagnosis()) 19477 composeCodeableConcept(null, e); 19478 closeArray(); 19479 } 19480 ; 19481 if (element.hasPresentedForm()) { 19482 openArray("presentedForm"); 19483 for (Attachment e : element.getPresentedForm()) 19484 composeAttachment(null, e); 19485 closeArray(); 19486 } 19487 ; 19488 } 19489 19490 protected void composeDiagnosticReportDiagnosticReportImageComponent(String name, 19491 DiagnosticReport.DiagnosticReportImageComponent element) throws IOException { 19492 if (element != null) { 19493 open(name); 19494 composeDiagnosticReportDiagnosticReportImageComponentInner(element); 19495 close(); 19496 } 19497 } 19498 19499 protected void composeDiagnosticReportDiagnosticReportImageComponentInner( 19500 DiagnosticReport.DiagnosticReportImageComponent element) throws IOException { 19501 composeBackbone(element); 19502 if (element.hasCommentElement()) { 19503 composeStringCore("comment", element.getCommentElement(), false); 19504 composeStringExtras("comment", element.getCommentElement(), false); 19505 } 19506 if (element.hasLink()) { 19507 composeReference("link", element.getLink()); 19508 } 19509 } 19510 19511 protected void composeDocumentManifest(String name, DocumentManifest element) throws IOException { 19512 if (element != null) { 19513 prop("resourceType", name); 19514 composeDocumentManifestInner(element); 19515 } 19516 } 19517 19518 protected void composeDocumentManifestInner(DocumentManifest element) throws IOException { 19519 composeDomainResourceElements(element); 19520 if (element.hasMasterIdentifier()) { 19521 composeIdentifier("masterIdentifier", element.getMasterIdentifier()); 19522 } 19523 if (element.hasIdentifier()) { 19524 openArray("identifier"); 19525 for (Identifier e : element.getIdentifier()) 19526 composeIdentifier(null, e); 19527 closeArray(); 19528 } 19529 ; 19530 if (element.hasSubject()) { 19531 composeReference("subject", element.getSubject()); 19532 } 19533 if (element.hasRecipient()) { 19534 openArray("recipient"); 19535 for (Reference e : element.getRecipient()) 19536 composeReference(null, e); 19537 closeArray(); 19538 } 19539 ; 19540 if (element.hasType()) { 19541 composeCodeableConcept("type", element.getType()); 19542 } 19543 if (element.hasAuthor()) { 19544 openArray("author"); 19545 for (Reference e : element.getAuthor()) 19546 composeReference(null, e); 19547 closeArray(); 19548 } 19549 ; 19550 if (element.hasCreatedElement()) { 19551 composeDateTimeCore("created", element.getCreatedElement(), false); 19552 composeDateTimeExtras("created", element.getCreatedElement(), false); 19553 } 19554 if (element.hasSourceElement()) { 19555 composeUriCore("source", element.getSourceElement(), false); 19556 composeUriExtras("source", element.getSourceElement(), false); 19557 } 19558 if (element.hasStatusElement()) { 19559 composeEnumerationCore("status", element.getStatusElement(), 19560 new Enumerations.DocumentReferenceStatusEnumFactory(), false); 19561 composeEnumerationExtras("status", element.getStatusElement(), 19562 new Enumerations.DocumentReferenceStatusEnumFactory(), false); 19563 } 19564 if (element.hasDescriptionElement()) { 19565 composeStringCore("description", element.getDescriptionElement(), false); 19566 composeStringExtras("description", element.getDescriptionElement(), false); 19567 } 19568 if (element.hasContent()) { 19569 openArray("content"); 19570 for (DocumentManifest.DocumentManifestContentComponent e : element.getContent()) 19571 composeDocumentManifestDocumentManifestContentComponent(null, e); 19572 closeArray(); 19573 } 19574 ; 19575 if (element.hasRelated()) { 19576 openArray("related"); 19577 for (DocumentManifest.DocumentManifestRelatedComponent e : element.getRelated()) 19578 composeDocumentManifestDocumentManifestRelatedComponent(null, e); 19579 closeArray(); 19580 } 19581 ; 19582 } 19583 19584 protected void composeDocumentManifestDocumentManifestContentComponent(String name, 19585 DocumentManifest.DocumentManifestContentComponent element) throws IOException { 19586 if (element != null) { 19587 open(name); 19588 composeDocumentManifestDocumentManifestContentComponentInner(element); 19589 close(); 19590 } 19591 } 19592 19593 protected void composeDocumentManifestDocumentManifestContentComponentInner( 19594 DocumentManifest.DocumentManifestContentComponent element) throws IOException { 19595 composeBackbone(element); 19596 if (element.hasP()) { 19597 composeType("p", element.getP()); 19598 } 19599 } 19600 19601 protected void composeDocumentManifestDocumentManifestRelatedComponent(String name, 19602 DocumentManifest.DocumentManifestRelatedComponent element) throws IOException { 19603 if (element != null) { 19604 open(name); 19605 composeDocumentManifestDocumentManifestRelatedComponentInner(element); 19606 close(); 19607 } 19608 } 19609 19610 protected void composeDocumentManifestDocumentManifestRelatedComponentInner( 19611 DocumentManifest.DocumentManifestRelatedComponent element) throws IOException { 19612 composeBackbone(element); 19613 if (element.hasIdentifier()) { 19614 composeIdentifier("identifier", element.getIdentifier()); 19615 } 19616 if (element.hasRef()) { 19617 composeReference("ref", element.getRef()); 19618 } 19619 } 19620 19621 protected void composeDocumentReference(String name, DocumentReference element) throws IOException { 19622 if (element != null) { 19623 prop("resourceType", name); 19624 composeDocumentReferenceInner(element); 19625 } 19626 } 19627 19628 protected void composeDocumentReferenceInner(DocumentReference element) throws IOException { 19629 composeDomainResourceElements(element); 19630 if (element.hasMasterIdentifier()) { 19631 composeIdentifier("masterIdentifier", element.getMasterIdentifier()); 19632 } 19633 if (element.hasIdentifier()) { 19634 openArray("identifier"); 19635 for (Identifier e : element.getIdentifier()) 19636 composeIdentifier(null, e); 19637 closeArray(); 19638 } 19639 ; 19640 if (element.hasSubject()) { 19641 composeReference("subject", element.getSubject()); 19642 } 19643 if (element.hasType()) { 19644 composeCodeableConcept("type", element.getType()); 19645 } 19646 if (element.hasClass_()) { 19647 composeCodeableConcept("class", element.getClass_()); 19648 } 19649 if (element.hasAuthor()) { 19650 openArray("author"); 19651 for (Reference e : element.getAuthor()) 19652 composeReference(null, e); 19653 closeArray(); 19654 } 19655 ; 19656 if (element.hasCustodian()) { 19657 composeReference("custodian", element.getCustodian()); 19658 } 19659 if (element.hasAuthenticator()) { 19660 composeReference("authenticator", element.getAuthenticator()); 19661 } 19662 if (element.hasCreatedElement()) { 19663 composeDateTimeCore("created", element.getCreatedElement(), false); 19664 composeDateTimeExtras("created", element.getCreatedElement(), false); 19665 } 19666 if (element.hasIndexedElement()) { 19667 composeInstantCore("indexed", element.getIndexedElement(), false); 19668 composeInstantExtras("indexed", element.getIndexedElement(), false); 19669 } 19670 if (element.hasStatusElement()) { 19671 composeEnumerationCore("status", element.getStatusElement(), 19672 new Enumerations.DocumentReferenceStatusEnumFactory(), false); 19673 composeEnumerationExtras("status", element.getStatusElement(), 19674 new Enumerations.DocumentReferenceStatusEnumFactory(), false); 19675 } 19676 if (element.hasDocStatus()) { 19677 composeCodeableConcept("docStatus", element.getDocStatus()); 19678 } 19679 if (element.hasRelatesTo()) { 19680 openArray("relatesTo"); 19681 for (DocumentReference.DocumentReferenceRelatesToComponent e : element.getRelatesTo()) 19682 composeDocumentReferenceDocumentReferenceRelatesToComponent(null, e); 19683 closeArray(); 19684 } 19685 ; 19686 if (element.hasDescriptionElement()) { 19687 composeStringCore("description", element.getDescriptionElement(), false); 19688 composeStringExtras("description", element.getDescriptionElement(), false); 19689 } 19690 if (element.hasSecurityLabel()) { 19691 openArray("securityLabel"); 19692 for (CodeableConcept e : element.getSecurityLabel()) 19693 composeCodeableConcept(null, e); 19694 closeArray(); 19695 } 19696 ; 19697 if (element.hasContent()) { 19698 openArray("content"); 19699 for (DocumentReference.DocumentReferenceContentComponent e : element.getContent()) 19700 composeDocumentReferenceDocumentReferenceContentComponent(null, e); 19701 closeArray(); 19702 } 19703 ; 19704 if (element.hasContext()) { 19705 composeDocumentReferenceDocumentReferenceContextComponent("context", element.getContext()); 19706 } 19707 } 19708 19709 protected void composeDocumentReferenceDocumentReferenceRelatesToComponent(String name, 19710 DocumentReference.DocumentReferenceRelatesToComponent element) throws IOException { 19711 if (element != null) { 19712 open(name); 19713 composeDocumentReferenceDocumentReferenceRelatesToComponentInner(element); 19714 close(); 19715 } 19716 } 19717 19718 protected void composeDocumentReferenceDocumentReferenceRelatesToComponentInner( 19719 DocumentReference.DocumentReferenceRelatesToComponent element) throws IOException { 19720 composeBackbone(element); 19721 if (element.hasCodeElement()) { 19722 composeEnumerationCore("code", element.getCodeElement(), 19723 new DocumentReference.DocumentRelationshipTypeEnumFactory(), false); 19724 composeEnumerationExtras("code", element.getCodeElement(), 19725 new DocumentReference.DocumentRelationshipTypeEnumFactory(), false); 19726 } 19727 if (element.hasTarget()) { 19728 composeReference("target", element.getTarget()); 19729 } 19730 } 19731 19732 protected void composeDocumentReferenceDocumentReferenceContentComponent(String name, 19733 DocumentReference.DocumentReferenceContentComponent element) throws IOException { 19734 if (element != null) { 19735 open(name); 19736 composeDocumentReferenceDocumentReferenceContentComponentInner(element); 19737 close(); 19738 } 19739 } 19740 19741 protected void composeDocumentReferenceDocumentReferenceContentComponentInner( 19742 DocumentReference.DocumentReferenceContentComponent element) throws IOException { 19743 composeBackbone(element); 19744 if (element.hasAttachment()) { 19745 composeAttachment("attachment", element.getAttachment()); 19746 } 19747 if (element.hasFormat()) { 19748 openArray("format"); 19749 for (Coding e : element.getFormat()) 19750 composeCoding(null, e); 19751 closeArray(); 19752 } 19753 ; 19754 } 19755 19756 protected void composeDocumentReferenceDocumentReferenceContextComponent(String name, 19757 DocumentReference.DocumentReferenceContextComponent element) throws IOException { 19758 if (element != null) { 19759 open(name); 19760 composeDocumentReferenceDocumentReferenceContextComponentInner(element); 19761 close(); 19762 } 19763 } 19764 19765 protected void composeDocumentReferenceDocumentReferenceContextComponentInner( 19766 DocumentReference.DocumentReferenceContextComponent element) throws IOException { 19767 composeBackbone(element); 19768 if (element.hasEncounter()) { 19769 composeReference("encounter", element.getEncounter()); 19770 } 19771 if (element.hasEvent()) { 19772 openArray("event"); 19773 for (CodeableConcept e : element.getEvent()) 19774 composeCodeableConcept(null, e); 19775 closeArray(); 19776 } 19777 ; 19778 if (element.hasPeriod()) { 19779 composePeriod("period", element.getPeriod()); 19780 } 19781 if (element.hasFacilityType()) { 19782 composeCodeableConcept("facilityType", element.getFacilityType()); 19783 } 19784 if (element.hasPracticeSetting()) { 19785 composeCodeableConcept("practiceSetting", element.getPracticeSetting()); 19786 } 19787 if (element.hasSourcePatientInfo()) { 19788 composeReference("sourcePatientInfo", element.getSourcePatientInfo()); 19789 } 19790 if (element.hasRelated()) { 19791 openArray("related"); 19792 for (DocumentReference.DocumentReferenceContextRelatedComponent e : element.getRelated()) 19793 composeDocumentReferenceDocumentReferenceContextRelatedComponent(null, e); 19794 closeArray(); 19795 } 19796 ; 19797 } 19798 19799 protected void composeDocumentReferenceDocumentReferenceContextRelatedComponent(String name, 19800 DocumentReference.DocumentReferenceContextRelatedComponent element) throws IOException { 19801 if (element != null) { 19802 open(name); 19803 composeDocumentReferenceDocumentReferenceContextRelatedComponentInner(element); 19804 close(); 19805 } 19806 } 19807 19808 protected void composeDocumentReferenceDocumentReferenceContextRelatedComponentInner( 19809 DocumentReference.DocumentReferenceContextRelatedComponent element) throws IOException { 19810 composeBackbone(element); 19811 if (element.hasIdentifier()) { 19812 composeIdentifier("identifier", element.getIdentifier()); 19813 } 19814 if (element.hasRef()) { 19815 composeReference("ref", element.getRef()); 19816 } 19817 } 19818 19819 protected void composeEligibilityRequest(String name, EligibilityRequest element) throws IOException { 19820 if (element != null) { 19821 prop("resourceType", name); 19822 composeEligibilityRequestInner(element); 19823 } 19824 } 19825 19826 protected void composeEligibilityRequestInner(EligibilityRequest element) throws IOException { 19827 composeDomainResourceElements(element); 19828 if (element.hasIdentifier()) { 19829 openArray("identifier"); 19830 for (Identifier e : element.getIdentifier()) 19831 composeIdentifier(null, e); 19832 closeArray(); 19833 } 19834 ; 19835 if (element.hasRuleset()) { 19836 composeCoding("ruleset", element.getRuleset()); 19837 } 19838 if (element.hasOriginalRuleset()) { 19839 composeCoding("originalRuleset", element.getOriginalRuleset()); 19840 } 19841 if (element.hasCreatedElement()) { 19842 composeDateTimeCore("created", element.getCreatedElement(), false); 19843 composeDateTimeExtras("created", element.getCreatedElement(), false); 19844 } 19845 if (element.hasTarget()) { 19846 composeReference("target", element.getTarget()); 19847 } 19848 if (element.hasProvider()) { 19849 composeReference("provider", element.getProvider()); 19850 } 19851 if (element.hasOrganization()) { 19852 composeReference("organization", element.getOrganization()); 19853 } 19854 } 19855 19856 protected void composeEligibilityResponse(String name, EligibilityResponse element) throws IOException { 19857 if (element != null) { 19858 prop("resourceType", name); 19859 composeEligibilityResponseInner(element); 19860 } 19861 } 19862 19863 protected void composeEligibilityResponseInner(EligibilityResponse element) throws IOException { 19864 composeDomainResourceElements(element); 19865 if (element.hasIdentifier()) { 19866 openArray("identifier"); 19867 for (Identifier e : element.getIdentifier()) 19868 composeIdentifier(null, e); 19869 closeArray(); 19870 } 19871 ; 19872 if (element.hasRequest()) { 19873 composeReference("request", element.getRequest()); 19874 } 19875 if (element.hasOutcomeElement()) { 19876 composeEnumerationCore("outcome", element.getOutcomeElement(), new Enumerations.RemittanceOutcomeEnumFactory(), 19877 false); 19878 composeEnumerationExtras("outcome", element.getOutcomeElement(), new Enumerations.RemittanceOutcomeEnumFactory(), 19879 false); 19880 } 19881 if (element.hasDispositionElement()) { 19882 composeStringCore("disposition", element.getDispositionElement(), false); 19883 composeStringExtras("disposition", element.getDispositionElement(), false); 19884 } 19885 if (element.hasRuleset()) { 19886 composeCoding("ruleset", element.getRuleset()); 19887 } 19888 if (element.hasOriginalRuleset()) { 19889 composeCoding("originalRuleset", element.getOriginalRuleset()); 19890 } 19891 if (element.hasCreatedElement()) { 19892 composeDateTimeCore("created", element.getCreatedElement(), false); 19893 composeDateTimeExtras("created", element.getCreatedElement(), false); 19894 } 19895 if (element.hasOrganization()) { 19896 composeReference("organization", element.getOrganization()); 19897 } 19898 if (element.hasRequestProvider()) { 19899 composeReference("requestProvider", element.getRequestProvider()); 19900 } 19901 if (element.hasRequestOrganization()) { 19902 composeReference("requestOrganization", element.getRequestOrganization()); 19903 } 19904 } 19905 19906 protected void composeEncounter(String name, Encounter element) throws IOException { 19907 if (element != null) { 19908 prop("resourceType", name); 19909 composeEncounterInner(element); 19910 } 19911 } 19912 19913 protected void composeEncounterInner(Encounter element) throws IOException { 19914 composeDomainResourceElements(element); 19915 if (element.hasIdentifier()) { 19916 openArray("identifier"); 19917 for (Identifier e : element.getIdentifier()) 19918 composeIdentifier(null, e); 19919 closeArray(); 19920 } 19921 ; 19922 if (element.hasStatusElement()) { 19923 composeEnumerationCore("status", element.getStatusElement(), new Encounter.EncounterStateEnumFactory(), false); 19924 composeEnumerationExtras("status", element.getStatusElement(), new Encounter.EncounterStateEnumFactory(), false); 19925 } 19926 if (element.hasStatusHistory()) { 19927 openArray("statusHistory"); 19928 for (Encounter.EncounterStatusHistoryComponent e : element.getStatusHistory()) 19929 composeEncounterEncounterStatusHistoryComponent(null, e); 19930 closeArray(); 19931 } 19932 ; 19933 if (element.hasClass_Element()) { 19934 composeEnumerationCore("class", element.getClass_Element(), new Encounter.EncounterClassEnumFactory(), false); 19935 composeEnumerationExtras("class", element.getClass_Element(), new Encounter.EncounterClassEnumFactory(), false); 19936 } 19937 if (element.hasType()) { 19938 openArray("type"); 19939 for (CodeableConcept e : element.getType()) 19940 composeCodeableConcept(null, e); 19941 closeArray(); 19942 } 19943 ; 19944 if (element.hasPriority()) { 19945 composeCodeableConcept("priority", element.getPriority()); 19946 } 19947 if (element.hasPatient()) { 19948 composeReference("patient", element.getPatient()); 19949 } 19950 if (element.hasEpisodeOfCare()) { 19951 openArray("episodeOfCare"); 19952 for (Reference e : element.getEpisodeOfCare()) 19953 composeReference(null, e); 19954 closeArray(); 19955 } 19956 ; 19957 if (element.hasIncomingReferral()) { 19958 openArray("incomingReferral"); 19959 for (Reference e : element.getIncomingReferral()) 19960 composeReference(null, e); 19961 closeArray(); 19962 } 19963 ; 19964 if (element.hasParticipant()) { 19965 openArray("participant"); 19966 for (Encounter.EncounterParticipantComponent e : element.getParticipant()) 19967 composeEncounterEncounterParticipantComponent(null, e); 19968 closeArray(); 19969 } 19970 ; 19971 if (element.hasAppointment()) { 19972 composeReference("appointment", element.getAppointment()); 19973 } 19974 if (element.hasPeriod()) { 19975 composePeriod("period", element.getPeriod()); 19976 } 19977 if (element.hasLength()) { 19978 composeDuration("length", element.getLength()); 19979 } 19980 if (element.hasReason()) { 19981 openArray("reason"); 19982 for (CodeableConcept e : element.getReason()) 19983 composeCodeableConcept(null, e); 19984 closeArray(); 19985 } 19986 ; 19987 if (element.hasIndication()) { 19988 openArray("indication"); 19989 for (Reference e : element.getIndication()) 19990 composeReference(null, e); 19991 closeArray(); 19992 } 19993 ; 19994 if (element.hasHospitalization()) { 19995 composeEncounterEncounterHospitalizationComponent("hospitalization", element.getHospitalization()); 19996 } 19997 if (element.hasLocation()) { 19998 openArray("location"); 19999 for (Encounter.EncounterLocationComponent e : element.getLocation()) 20000 composeEncounterEncounterLocationComponent(null, e); 20001 closeArray(); 20002 } 20003 ; 20004 if (element.hasServiceProvider()) { 20005 composeReference("serviceProvider", element.getServiceProvider()); 20006 } 20007 if (element.hasPartOf()) { 20008 composeReference("partOf", element.getPartOf()); 20009 } 20010 } 20011 20012 protected void composeEncounterEncounterStatusHistoryComponent(String name, 20013 Encounter.EncounterStatusHistoryComponent element) throws IOException { 20014 if (element != null) { 20015 open(name); 20016 composeEncounterEncounterStatusHistoryComponentInner(element); 20017 close(); 20018 } 20019 } 20020 20021 protected void composeEncounterEncounterStatusHistoryComponentInner(Encounter.EncounterStatusHistoryComponent element) 20022 throws IOException { 20023 composeBackbone(element); 20024 if (element.hasStatusElement()) { 20025 composeEnumerationCore("status", element.getStatusElement(), new Encounter.EncounterStateEnumFactory(), false); 20026 composeEnumerationExtras("status", element.getStatusElement(), new Encounter.EncounterStateEnumFactory(), false); 20027 } 20028 if (element.hasPeriod()) { 20029 composePeriod("period", element.getPeriod()); 20030 } 20031 } 20032 20033 protected void composeEncounterEncounterParticipantComponent(String name, 20034 Encounter.EncounterParticipantComponent element) throws IOException { 20035 if (element != null) { 20036 open(name); 20037 composeEncounterEncounterParticipantComponentInner(element); 20038 close(); 20039 } 20040 } 20041 20042 protected void composeEncounterEncounterParticipantComponentInner(Encounter.EncounterParticipantComponent element) 20043 throws IOException { 20044 composeBackbone(element); 20045 if (element.hasType()) { 20046 openArray("type"); 20047 for (CodeableConcept e : element.getType()) 20048 composeCodeableConcept(null, e); 20049 closeArray(); 20050 } 20051 ; 20052 if (element.hasPeriod()) { 20053 composePeriod("period", element.getPeriod()); 20054 } 20055 if (element.hasIndividual()) { 20056 composeReference("individual", element.getIndividual()); 20057 } 20058 } 20059 20060 protected void composeEncounterEncounterHospitalizationComponent(String name, 20061 Encounter.EncounterHospitalizationComponent element) throws IOException { 20062 if (element != null) { 20063 open(name); 20064 composeEncounterEncounterHospitalizationComponentInner(element); 20065 close(); 20066 } 20067 } 20068 20069 protected void composeEncounterEncounterHospitalizationComponentInner( 20070 Encounter.EncounterHospitalizationComponent element) throws IOException { 20071 composeBackbone(element); 20072 if (element.hasPreAdmissionIdentifier()) { 20073 composeIdentifier("preAdmissionIdentifier", element.getPreAdmissionIdentifier()); 20074 } 20075 if (element.hasOrigin()) { 20076 composeReference("origin", element.getOrigin()); 20077 } 20078 if (element.hasAdmitSource()) { 20079 composeCodeableConcept("admitSource", element.getAdmitSource()); 20080 } 20081 if (element.hasAdmittingDiagnosis()) { 20082 openArray("admittingDiagnosis"); 20083 for (Reference e : element.getAdmittingDiagnosis()) 20084 composeReference(null, e); 20085 closeArray(); 20086 } 20087 ; 20088 if (element.hasReAdmission()) { 20089 composeCodeableConcept("reAdmission", element.getReAdmission()); 20090 } 20091 if (element.hasDietPreference()) { 20092 openArray("dietPreference"); 20093 for (CodeableConcept e : element.getDietPreference()) 20094 composeCodeableConcept(null, e); 20095 closeArray(); 20096 } 20097 ; 20098 if (element.hasSpecialCourtesy()) { 20099 openArray("specialCourtesy"); 20100 for (CodeableConcept e : element.getSpecialCourtesy()) 20101 composeCodeableConcept(null, e); 20102 closeArray(); 20103 } 20104 ; 20105 if (element.hasSpecialArrangement()) { 20106 openArray("specialArrangement"); 20107 for (CodeableConcept e : element.getSpecialArrangement()) 20108 composeCodeableConcept(null, e); 20109 closeArray(); 20110 } 20111 ; 20112 if (element.hasDestination()) { 20113 composeReference("destination", element.getDestination()); 20114 } 20115 if (element.hasDischargeDisposition()) { 20116 composeCodeableConcept("dischargeDisposition", element.getDischargeDisposition()); 20117 } 20118 if (element.hasDischargeDiagnosis()) { 20119 openArray("dischargeDiagnosis"); 20120 for (Reference e : element.getDischargeDiagnosis()) 20121 composeReference(null, e); 20122 closeArray(); 20123 } 20124 ; 20125 } 20126 20127 protected void composeEncounterEncounterLocationComponent(String name, Encounter.EncounterLocationComponent element) 20128 throws IOException { 20129 if (element != null) { 20130 open(name); 20131 composeEncounterEncounterLocationComponentInner(element); 20132 close(); 20133 } 20134 } 20135 20136 protected void composeEncounterEncounterLocationComponentInner(Encounter.EncounterLocationComponent element) 20137 throws IOException { 20138 composeBackbone(element); 20139 if (element.hasLocation()) { 20140 composeReference("location", element.getLocation()); 20141 } 20142 if (element.hasStatusElement()) { 20143 composeEnumerationCore("status", element.getStatusElement(), new Encounter.EncounterLocationStatusEnumFactory(), 20144 false); 20145 composeEnumerationExtras("status", element.getStatusElement(), new Encounter.EncounterLocationStatusEnumFactory(), 20146 false); 20147 } 20148 if (element.hasPeriod()) { 20149 composePeriod("period", element.getPeriod()); 20150 } 20151 } 20152 20153 protected void composeEnrollmentRequest(String name, EnrollmentRequest element) throws IOException { 20154 if (element != null) { 20155 prop("resourceType", name); 20156 composeEnrollmentRequestInner(element); 20157 } 20158 } 20159 20160 protected void composeEnrollmentRequestInner(EnrollmentRequest element) throws IOException { 20161 composeDomainResourceElements(element); 20162 if (element.hasIdentifier()) { 20163 openArray("identifier"); 20164 for (Identifier e : element.getIdentifier()) 20165 composeIdentifier(null, e); 20166 closeArray(); 20167 } 20168 ; 20169 if (element.hasRuleset()) { 20170 composeCoding("ruleset", element.getRuleset()); 20171 } 20172 if (element.hasOriginalRuleset()) { 20173 composeCoding("originalRuleset", element.getOriginalRuleset()); 20174 } 20175 if (element.hasCreatedElement()) { 20176 composeDateTimeCore("created", element.getCreatedElement(), false); 20177 composeDateTimeExtras("created", element.getCreatedElement(), false); 20178 } 20179 if (element.hasTarget()) { 20180 composeReference("target", element.getTarget()); 20181 } 20182 if (element.hasProvider()) { 20183 composeReference("provider", element.getProvider()); 20184 } 20185 if (element.hasOrganization()) { 20186 composeReference("organization", element.getOrganization()); 20187 } 20188 if (element.hasSubject()) { 20189 composeReference("subject", element.getSubject()); 20190 } 20191 if (element.hasCoverage()) { 20192 composeReference("coverage", element.getCoverage()); 20193 } 20194 if (element.hasRelationship()) { 20195 composeCoding("relationship", element.getRelationship()); 20196 } 20197 } 20198 20199 protected void composeEnrollmentResponse(String name, EnrollmentResponse element) throws IOException { 20200 if (element != null) { 20201 prop("resourceType", name); 20202 composeEnrollmentResponseInner(element); 20203 } 20204 } 20205 20206 protected void composeEnrollmentResponseInner(EnrollmentResponse element) throws IOException { 20207 composeDomainResourceElements(element); 20208 if (element.hasIdentifier()) { 20209 openArray("identifier"); 20210 for (Identifier e : element.getIdentifier()) 20211 composeIdentifier(null, e); 20212 closeArray(); 20213 } 20214 ; 20215 if (element.hasRequest()) { 20216 composeReference("request", element.getRequest()); 20217 } 20218 if (element.hasOutcomeElement()) { 20219 composeEnumerationCore("outcome", element.getOutcomeElement(), new Enumerations.RemittanceOutcomeEnumFactory(), 20220 false); 20221 composeEnumerationExtras("outcome", element.getOutcomeElement(), new Enumerations.RemittanceOutcomeEnumFactory(), 20222 false); 20223 } 20224 if (element.hasDispositionElement()) { 20225 composeStringCore("disposition", element.getDispositionElement(), false); 20226 composeStringExtras("disposition", element.getDispositionElement(), false); 20227 } 20228 if (element.hasRuleset()) { 20229 composeCoding("ruleset", element.getRuleset()); 20230 } 20231 if (element.hasOriginalRuleset()) { 20232 composeCoding("originalRuleset", element.getOriginalRuleset()); 20233 } 20234 if (element.hasCreatedElement()) { 20235 composeDateTimeCore("created", element.getCreatedElement(), false); 20236 composeDateTimeExtras("created", element.getCreatedElement(), false); 20237 } 20238 if (element.hasOrganization()) { 20239 composeReference("organization", element.getOrganization()); 20240 } 20241 if (element.hasRequestProvider()) { 20242 composeReference("requestProvider", element.getRequestProvider()); 20243 } 20244 if (element.hasRequestOrganization()) { 20245 composeReference("requestOrganization", element.getRequestOrganization()); 20246 } 20247 } 20248 20249 protected void composeEpisodeOfCare(String name, EpisodeOfCare element) throws IOException { 20250 if (element != null) { 20251 prop("resourceType", name); 20252 composeEpisodeOfCareInner(element); 20253 } 20254 } 20255 20256 protected void composeEpisodeOfCareInner(EpisodeOfCare element) throws IOException { 20257 composeDomainResourceElements(element); 20258 if (element.hasIdentifier()) { 20259 openArray("identifier"); 20260 for (Identifier e : element.getIdentifier()) 20261 composeIdentifier(null, e); 20262 closeArray(); 20263 } 20264 ; 20265 if (element.hasStatusElement()) { 20266 composeEnumerationCore("status", element.getStatusElement(), new EpisodeOfCare.EpisodeOfCareStatusEnumFactory(), 20267 false); 20268 composeEnumerationExtras("status", element.getStatusElement(), new EpisodeOfCare.EpisodeOfCareStatusEnumFactory(), 20269 false); 20270 } 20271 if (element.hasStatusHistory()) { 20272 openArray("statusHistory"); 20273 for (EpisodeOfCare.EpisodeOfCareStatusHistoryComponent e : element.getStatusHistory()) 20274 composeEpisodeOfCareEpisodeOfCareStatusHistoryComponent(null, e); 20275 closeArray(); 20276 } 20277 ; 20278 if (element.hasType()) { 20279 openArray("type"); 20280 for (CodeableConcept e : element.getType()) 20281 composeCodeableConcept(null, e); 20282 closeArray(); 20283 } 20284 ; 20285 if (element.hasCondition()) { 20286 openArray("condition"); 20287 for (Reference e : element.getCondition()) 20288 composeReference(null, e); 20289 closeArray(); 20290 } 20291 ; 20292 if (element.hasPatient()) { 20293 composeReference("patient", element.getPatient()); 20294 } 20295 if (element.hasManagingOrganization()) { 20296 composeReference("managingOrganization", element.getManagingOrganization()); 20297 } 20298 if (element.hasPeriod()) { 20299 composePeriod("period", element.getPeriod()); 20300 } 20301 if (element.hasReferralRequest()) { 20302 openArray("referralRequest"); 20303 for (Reference e : element.getReferralRequest()) 20304 composeReference(null, e); 20305 closeArray(); 20306 } 20307 ; 20308 if (element.hasCareManager()) { 20309 composeReference("careManager", element.getCareManager()); 20310 } 20311 if (element.hasCareTeam()) { 20312 openArray("careTeam"); 20313 for (EpisodeOfCare.EpisodeOfCareCareTeamComponent e : element.getCareTeam()) 20314 composeEpisodeOfCareEpisodeOfCareCareTeamComponent(null, e); 20315 closeArray(); 20316 } 20317 ; 20318 } 20319 20320 protected void composeEpisodeOfCareEpisodeOfCareStatusHistoryComponent(String name, 20321 EpisodeOfCare.EpisodeOfCareStatusHistoryComponent element) throws IOException { 20322 if (element != null) { 20323 open(name); 20324 composeEpisodeOfCareEpisodeOfCareStatusHistoryComponentInner(element); 20325 close(); 20326 } 20327 } 20328 20329 protected void composeEpisodeOfCareEpisodeOfCareStatusHistoryComponentInner( 20330 EpisodeOfCare.EpisodeOfCareStatusHistoryComponent element) throws IOException { 20331 composeBackbone(element); 20332 if (element.hasStatusElement()) { 20333 composeEnumerationCore("status", element.getStatusElement(), new EpisodeOfCare.EpisodeOfCareStatusEnumFactory(), 20334 false); 20335 composeEnumerationExtras("status", element.getStatusElement(), new EpisodeOfCare.EpisodeOfCareStatusEnumFactory(), 20336 false); 20337 } 20338 if (element.hasPeriod()) { 20339 composePeriod("period", element.getPeriod()); 20340 } 20341 } 20342 20343 protected void composeEpisodeOfCareEpisodeOfCareCareTeamComponent(String name, 20344 EpisodeOfCare.EpisodeOfCareCareTeamComponent element) throws IOException { 20345 if (element != null) { 20346 open(name); 20347 composeEpisodeOfCareEpisodeOfCareCareTeamComponentInner(element); 20348 close(); 20349 } 20350 } 20351 20352 protected void composeEpisodeOfCareEpisodeOfCareCareTeamComponentInner( 20353 EpisodeOfCare.EpisodeOfCareCareTeamComponent element) throws IOException { 20354 composeBackbone(element); 20355 if (element.hasRole()) { 20356 openArray("role"); 20357 for (CodeableConcept e : element.getRole()) 20358 composeCodeableConcept(null, e); 20359 closeArray(); 20360 } 20361 ; 20362 if (element.hasPeriod()) { 20363 composePeriod("period", element.getPeriod()); 20364 } 20365 if (element.hasMember()) { 20366 composeReference("member", element.getMember()); 20367 } 20368 } 20369 20370 protected void composeExplanationOfBenefit(String name, ExplanationOfBenefit element) throws IOException { 20371 if (element != null) { 20372 prop("resourceType", name); 20373 composeExplanationOfBenefitInner(element); 20374 } 20375 } 20376 20377 protected void composeExplanationOfBenefitInner(ExplanationOfBenefit element) throws IOException { 20378 composeDomainResourceElements(element); 20379 if (element.hasIdentifier()) { 20380 openArray("identifier"); 20381 for (Identifier e : element.getIdentifier()) 20382 composeIdentifier(null, e); 20383 closeArray(); 20384 } 20385 ; 20386 if (element.hasRequest()) { 20387 composeReference("request", element.getRequest()); 20388 } 20389 if (element.hasOutcomeElement()) { 20390 composeEnumerationCore("outcome", element.getOutcomeElement(), new Enumerations.RemittanceOutcomeEnumFactory(), 20391 false); 20392 composeEnumerationExtras("outcome", element.getOutcomeElement(), new Enumerations.RemittanceOutcomeEnumFactory(), 20393 false); 20394 } 20395 if (element.hasDispositionElement()) { 20396 composeStringCore("disposition", element.getDispositionElement(), false); 20397 composeStringExtras("disposition", element.getDispositionElement(), false); 20398 } 20399 if (element.hasRuleset()) { 20400 composeCoding("ruleset", element.getRuleset()); 20401 } 20402 if (element.hasOriginalRuleset()) { 20403 composeCoding("originalRuleset", element.getOriginalRuleset()); 20404 } 20405 if (element.hasCreatedElement()) { 20406 composeDateTimeCore("created", element.getCreatedElement(), false); 20407 composeDateTimeExtras("created", element.getCreatedElement(), false); 20408 } 20409 if (element.hasOrganization()) { 20410 composeReference("organization", element.getOrganization()); 20411 } 20412 if (element.hasRequestProvider()) { 20413 composeReference("requestProvider", element.getRequestProvider()); 20414 } 20415 if (element.hasRequestOrganization()) { 20416 composeReference("requestOrganization", element.getRequestOrganization()); 20417 } 20418 } 20419 20420 protected void composeFamilyMemberHistory(String name, FamilyMemberHistory element) throws IOException { 20421 if (element != null) { 20422 prop("resourceType", name); 20423 composeFamilyMemberHistoryInner(element); 20424 } 20425 } 20426 20427 protected void composeFamilyMemberHistoryInner(FamilyMemberHistory element) throws IOException { 20428 composeDomainResourceElements(element); 20429 if (element.hasIdentifier()) { 20430 openArray("identifier"); 20431 for (Identifier e : element.getIdentifier()) 20432 composeIdentifier(null, e); 20433 closeArray(); 20434 } 20435 ; 20436 if (element.hasPatient()) { 20437 composeReference("patient", element.getPatient()); 20438 } 20439 if (element.hasDateElement()) { 20440 composeDateTimeCore("date", element.getDateElement(), false); 20441 composeDateTimeExtras("date", element.getDateElement(), false); 20442 } 20443 if (element.hasStatusElement()) { 20444 composeEnumerationCore("status", element.getStatusElement(), 20445 new FamilyMemberHistory.FamilyHistoryStatusEnumFactory(), false); 20446 composeEnumerationExtras("status", element.getStatusElement(), 20447 new FamilyMemberHistory.FamilyHistoryStatusEnumFactory(), false); 20448 } 20449 if (element.hasNameElement()) { 20450 composeStringCore("name", element.getNameElement(), false); 20451 composeStringExtras("name", element.getNameElement(), false); 20452 } 20453 if (element.hasRelationship()) { 20454 composeCodeableConcept("relationship", element.getRelationship()); 20455 } 20456 if (element.hasGenderElement()) { 20457 composeEnumerationCore("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), 20458 false); 20459 composeEnumerationExtras("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), 20460 false); 20461 } 20462 if (element.hasBorn()) { 20463 composeType("born", element.getBorn()); 20464 } 20465 if (element.hasAge()) { 20466 composeType("age", element.getAge()); 20467 } 20468 if (element.hasDeceased()) { 20469 composeType("deceased", element.getDeceased()); 20470 } 20471 if (element.hasNote()) { 20472 composeAnnotation("note", element.getNote()); 20473 } 20474 if (element.hasCondition()) { 20475 openArray("condition"); 20476 for (FamilyMemberHistory.FamilyMemberHistoryConditionComponent e : element.getCondition()) 20477 composeFamilyMemberHistoryFamilyMemberHistoryConditionComponent(null, e); 20478 closeArray(); 20479 } 20480 ; 20481 } 20482 20483 protected void composeFamilyMemberHistoryFamilyMemberHistoryConditionComponent(String name, 20484 FamilyMemberHistory.FamilyMemberHistoryConditionComponent element) throws IOException { 20485 if (element != null) { 20486 open(name); 20487 composeFamilyMemberHistoryFamilyMemberHistoryConditionComponentInner(element); 20488 close(); 20489 } 20490 } 20491 20492 protected void composeFamilyMemberHistoryFamilyMemberHistoryConditionComponentInner( 20493 FamilyMemberHistory.FamilyMemberHistoryConditionComponent element) throws IOException { 20494 composeBackbone(element); 20495 if (element.hasCode()) { 20496 composeCodeableConcept("code", element.getCode()); 20497 } 20498 if (element.hasOutcome()) { 20499 composeCodeableConcept("outcome", element.getOutcome()); 20500 } 20501 if (element.hasOnset()) { 20502 composeType("onset", element.getOnset()); 20503 } 20504 if (element.hasNote()) { 20505 composeAnnotation("note", element.getNote()); 20506 } 20507 } 20508 20509 protected void composeFlag(String name, Flag element) throws IOException { 20510 if (element != null) { 20511 prop("resourceType", name); 20512 composeFlagInner(element); 20513 } 20514 } 20515 20516 protected void composeFlagInner(Flag element) throws IOException { 20517 composeDomainResourceElements(element); 20518 if (element.hasIdentifier()) { 20519 openArray("identifier"); 20520 for (Identifier e : element.getIdentifier()) 20521 composeIdentifier(null, e); 20522 closeArray(); 20523 } 20524 ; 20525 if (element.hasCategory()) { 20526 composeCodeableConcept("category", element.getCategory()); 20527 } 20528 if (element.hasStatusElement()) { 20529 composeEnumerationCore("status", element.getStatusElement(), new Flag.FlagStatusEnumFactory(), false); 20530 composeEnumerationExtras("status", element.getStatusElement(), new Flag.FlagStatusEnumFactory(), false); 20531 } 20532 if (element.hasPeriod()) { 20533 composePeriod("period", element.getPeriod()); 20534 } 20535 if (element.hasSubject()) { 20536 composeReference("subject", element.getSubject()); 20537 } 20538 if (element.hasEncounter()) { 20539 composeReference("encounter", element.getEncounter()); 20540 } 20541 if (element.hasAuthor()) { 20542 composeReference("author", element.getAuthor()); 20543 } 20544 if (element.hasCode()) { 20545 composeCodeableConcept("code", element.getCode()); 20546 } 20547 } 20548 20549 protected void composeGoal(String name, Goal element) throws IOException { 20550 if (element != null) { 20551 prop("resourceType", name); 20552 composeGoalInner(element); 20553 } 20554 } 20555 20556 protected void composeGoalInner(Goal element) throws IOException { 20557 composeDomainResourceElements(element); 20558 if (element.hasIdentifier()) { 20559 openArray("identifier"); 20560 for (Identifier e : element.getIdentifier()) 20561 composeIdentifier(null, e); 20562 closeArray(); 20563 } 20564 ; 20565 if (element.hasSubject()) { 20566 composeReference("subject", element.getSubject()); 20567 } 20568 if (element.hasStart()) { 20569 composeType("start", element.getStart()); 20570 } 20571 if (element.hasTarget()) { 20572 composeType("target", element.getTarget()); 20573 } 20574 if (element.hasCategory()) { 20575 openArray("category"); 20576 for (CodeableConcept e : element.getCategory()) 20577 composeCodeableConcept(null, e); 20578 closeArray(); 20579 } 20580 ; 20581 if (element.hasDescriptionElement()) { 20582 composeStringCore("description", element.getDescriptionElement(), false); 20583 composeStringExtras("description", element.getDescriptionElement(), false); 20584 } 20585 if (element.hasStatusElement()) { 20586 composeEnumerationCore("status", element.getStatusElement(), new Goal.GoalStatusEnumFactory(), false); 20587 composeEnumerationExtras("status", element.getStatusElement(), new Goal.GoalStatusEnumFactory(), false); 20588 } 20589 if (element.hasStatusDateElement()) { 20590 composeDateCore("statusDate", element.getStatusDateElement(), false); 20591 composeDateExtras("statusDate", element.getStatusDateElement(), false); 20592 } 20593 if (element.hasStatusReason()) { 20594 composeCodeableConcept("statusReason", element.getStatusReason()); 20595 } 20596 if (element.hasAuthor()) { 20597 composeReference("author", element.getAuthor()); 20598 } 20599 if (element.hasPriority()) { 20600 composeCodeableConcept("priority", element.getPriority()); 20601 } 20602 if (element.hasAddresses()) { 20603 openArray("addresses"); 20604 for (Reference e : element.getAddresses()) 20605 composeReference(null, e); 20606 closeArray(); 20607 } 20608 ; 20609 if (element.hasNote()) { 20610 openArray("note"); 20611 for (Annotation e : element.getNote()) 20612 composeAnnotation(null, e); 20613 closeArray(); 20614 } 20615 ; 20616 if (element.hasOutcome()) { 20617 openArray("outcome"); 20618 for (Goal.GoalOutcomeComponent e : element.getOutcome()) 20619 composeGoalGoalOutcomeComponent(null, e); 20620 closeArray(); 20621 } 20622 ; 20623 } 20624 20625 protected void composeGoalGoalOutcomeComponent(String name, Goal.GoalOutcomeComponent element) throws IOException { 20626 if (element != null) { 20627 open(name); 20628 composeGoalGoalOutcomeComponentInner(element); 20629 close(); 20630 } 20631 } 20632 20633 protected void composeGoalGoalOutcomeComponentInner(Goal.GoalOutcomeComponent element) throws IOException { 20634 composeBackbone(element); 20635 if (element.hasResult()) { 20636 composeType("result", element.getResult()); 20637 } 20638 } 20639 20640 protected void composeGroup(String name, Group element) throws IOException { 20641 if (element != null) { 20642 prop("resourceType", name); 20643 composeGroupInner(element); 20644 } 20645 } 20646 20647 protected void composeGroupInner(Group element) throws IOException { 20648 composeDomainResourceElements(element); 20649 if (element.hasIdentifier()) { 20650 openArray("identifier"); 20651 for (Identifier e : element.getIdentifier()) 20652 composeIdentifier(null, e); 20653 closeArray(); 20654 } 20655 ; 20656 if (element.hasTypeElement()) { 20657 composeEnumerationCore("type", element.getTypeElement(), new Group.GroupTypeEnumFactory(), false); 20658 composeEnumerationExtras("type", element.getTypeElement(), new Group.GroupTypeEnumFactory(), false); 20659 } 20660 if (element.hasActualElement()) { 20661 composeBooleanCore("actual", element.getActualElement(), false); 20662 composeBooleanExtras("actual", element.getActualElement(), false); 20663 } 20664 if (element.hasCode()) { 20665 composeCodeableConcept("code", element.getCode()); 20666 } 20667 if (element.hasNameElement()) { 20668 composeStringCore("name", element.getNameElement(), false); 20669 composeStringExtras("name", element.getNameElement(), false); 20670 } 20671 if (element.hasQuantityElement()) { 20672 composeUnsignedIntCore("quantity", element.getQuantityElement(), false); 20673 composeUnsignedIntExtras("quantity", element.getQuantityElement(), false); 20674 } 20675 if (element.hasCharacteristic()) { 20676 openArray("characteristic"); 20677 for (Group.GroupCharacteristicComponent e : element.getCharacteristic()) 20678 composeGroupGroupCharacteristicComponent(null, e); 20679 closeArray(); 20680 } 20681 ; 20682 if (element.hasMember()) { 20683 openArray("member"); 20684 for (Group.GroupMemberComponent e : element.getMember()) 20685 composeGroupGroupMemberComponent(null, e); 20686 closeArray(); 20687 } 20688 ; 20689 } 20690 20691 protected void composeGroupGroupCharacteristicComponent(String name, Group.GroupCharacteristicComponent element) 20692 throws IOException { 20693 if (element != null) { 20694 open(name); 20695 composeGroupGroupCharacteristicComponentInner(element); 20696 close(); 20697 } 20698 } 20699 20700 protected void composeGroupGroupCharacteristicComponentInner(Group.GroupCharacteristicComponent element) 20701 throws IOException { 20702 composeBackbone(element); 20703 if (element.hasCode()) { 20704 composeCodeableConcept("code", element.getCode()); 20705 } 20706 if (element.hasValue()) { 20707 composeType("value", element.getValue()); 20708 } 20709 if (element.hasExcludeElement()) { 20710 composeBooleanCore("exclude", element.getExcludeElement(), false); 20711 composeBooleanExtras("exclude", element.getExcludeElement(), false); 20712 } 20713 if (element.hasPeriod()) { 20714 composePeriod("period", element.getPeriod()); 20715 } 20716 } 20717 20718 protected void composeGroupGroupMemberComponent(String name, Group.GroupMemberComponent element) throws IOException { 20719 if (element != null) { 20720 open(name); 20721 composeGroupGroupMemberComponentInner(element); 20722 close(); 20723 } 20724 } 20725 20726 protected void composeGroupGroupMemberComponentInner(Group.GroupMemberComponent element) throws IOException { 20727 composeBackbone(element); 20728 if (element.hasEntity()) { 20729 composeReference("entity", element.getEntity()); 20730 } 20731 if (element.hasPeriod()) { 20732 composePeriod("period", element.getPeriod()); 20733 } 20734 if (element.hasInactiveElement()) { 20735 composeBooleanCore("inactive", element.getInactiveElement(), false); 20736 composeBooleanExtras("inactive", element.getInactiveElement(), false); 20737 } 20738 } 20739 20740 protected void composeHealthcareService(String name, HealthcareService element) throws IOException { 20741 if (element != null) { 20742 prop("resourceType", name); 20743 composeHealthcareServiceInner(element); 20744 } 20745 } 20746 20747 protected void composeHealthcareServiceInner(HealthcareService element) throws IOException { 20748 composeDomainResourceElements(element); 20749 if (element.hasIdentifier()) { 20750 openArray("identifier"); 20751 for (Identifier e : element.getIdentifier()) 20752 composeIdentifier(null, e); 20753 closeArray(); 20754 } 20755 ; 20756 if (element.hasProvidedBy()) { 20757 composeReference("providedBy", element.getProvidedBy()); 20758 } 20759 if (element.hasServiceCategory()) { 20760 composeCodeableConcept("serviceCategory", element.getServiceCategory()); 20761 } 20762 if (element.hasServiceType()) { 20763 openArray("serviceType"); 20764 for (HealthcareService.ServiceTypeComponent e : element.getServiceType()) 20765 composeHealthcareServiceServiceTypeComponent(null, e); 20766 closeArray(); 20767 } 20768 ; 20769 if (element.hasLocation()) { 20770 composeReference("location", element.getLocation()); 20771 } 20772 if (element.hasServiceNameElement()) { 20773 composeStringCore("serviceName", element.getServiceNameElement(), false); 20774 composeStringExtras("serviceName", element.getServiceNameElement(), false); 20775 } 20776 if (element.hasCommentElement()) { 20777 composeStringCore("comment", element.getCommentElement(), false); 20778 composeStringExtras("comment", element.getCommentElement(), false); 20779 } 20780 if (element.hasExtraDetailsElement()) { 20781 composeStringCore("extraDetails", element.getExtraDetailsElement(), false); 20782 composeStringExtras("extraDetails", element.getExtraDetailsElement(), false); 20783 } 20784 if (element.hasPhoto()) { 20785 composeAttachment("photo", element.getPhoto()); 20786 } 20787 if (element.hasTelecom()) { 20788 openArray("telecom"); 20789 for (ContactPoint e : element.getTelecom()) 20790 composeContactPoint(null, e); 20791 closeArray(); 20792 } 20793 ; 20794 if (element.hasCoverageArea()) { 20795 openArray("coverageArea"); 20796 for (Reference e : element.getCoverageArea()) 20797 composeReference(null, e); 20798 closeArray(); 20799 } 20800 ; 20801 if (element.hasServiceProvisionCode()) { 20802 openArray("serviceProvisionCode"); 20803 for (CodeableConcept e : element.getServiceProvisionCode()) 20804 composeCodeableConcept(null, e); 20805 closeArray(); 20806 } 20807 ; 20808 if (element.hasEligibility()) { 20809 composeCodeableConcept("eligibility", element.getEligibility()); 20810 } 20811 if (element.hasEligibilityNoteElement()) { 20812 composeStringCore("eligibilityNote", element.getEligibilityNoteElement(), false); 20813 composeStringExtras("eligibilityNote", element.getEligibilityNoteElement(), false); 20814 } 20815 if (element.hasProgramName()) { 20816 openArray("programName"); 20817 for (StringType e : element.getProgramName()) 20818 composeStringCore(null, e, true); 20819 closeArray(); 20820 if (anyHasExtras(element.getProgramName())) { 20821 openArray("_programName"); 20822 for (StringType e : element.getProgramName()) 20823 composeStringExtras(null, e, true); 20824 closeArray(); 20825 } 20826 } 20827 ; 20828 if (element.hasCharacteristic()) { 20829 openArray("characteristic"); 20830 for (CodeableConcept e : element.getCharacteristic()) 20831 composeCodeableConcept(null, e); 20832 closeArray(); 20833 } 20834 ; 20835 if (element.hasReferralMethod()) { 20836 openArray("referralMethod"); 20837 for (CodeableConcept e : element.getReferralMethod()) 20838 composeCodeableConcept(null, e); 20839 closeArray(); 20840 } 20841 ; 20842 if (element.hasPublicKeyElement()) { 20843 composeStringCore("publicKey", element.getPublicKeyElement(), false); 20844 composeStringExtras("publicKey", element.getPublicKeyElement(), false); 20845 } 20846 if (element.hasAppointmentRequiredElement()) { 20847 composeBooleanCore("appointmentRequired", element.getAppointmentRequiredElement(), false); 20848 composeBooleanExtras("appointmentRequired", element.getAppointmentRequiredElement(), false); 20849 } 20850 if (element.hasAvailableTime()) { 20851 openArray("availableTime"); 20852 for (HealthcareService.HealthcareServiceAvailableTimeComponent e : element.getAvailableTime()) 20853 composeHealthcareServiceHealthcareServiceAvailableTimeComponent(null, e); 20854 closeArray(); 20855 } 20856 ; 20857 if (element.hasNotAvailable()) { 20858 openArray("notAvailable"); 20859 for (HealthcareService.HealthcareServiceNotAvailableComponent e : element.getNotAvailable()) 20860 composeHealthcareServiceHealthcareServiceNotAvailableComponent(null, e); 20861 closeArray(); 20862 } 20863 ; 20864 if (element.hasAvailabilityExceptionsElement()) { 20865 composeStringCore("availabilityExceptions", element.getAvailabilityExceptionsElement(), false); 20866 composeStringExtras("availabilityExceptions", element.getAvailabilityExceptionsElement(), false); 20867 } 20868 } 20869 20870 protected void composeHealthcareServiceServiceTypeComponent(String name, 20871 HealthcareService.ServiceTypeComponent element) throws IOException { 20872 if (element != null) { 20873 open(name); 20874 composeHealthcareServiceServiceTypeComponentInner(element); 20875 close(); 20876 } 20877 } 20878 20879 protected void composeHealthcareServiceServiceTypeComponentInner(HealthcareService.ServiceTypeComponent element) 20880 throws IOException { 20881 composeBackbone(element); 20882 if (element.hasType()) { 20883 composeCodeableConcept("type", element.getType()); 20884 } 20885 if (element.hasSpecialty()) { 20886 openArray("specialty"); 20887 for (CodeableConcept e : element.getSpecialty()) 20888 composeCodeableConcept(null, e); 20889 closeArray(); 20890 } 20891 ; 20892 } 20893 20894 protected void composeHealthcareServiceHealthcareServiceAvailableTimeComponent(String name, 20895 HealthcareService.HealthcareServiceAvailableTimeComponent element) throws IOException { 20896 if (element != null) { 20897 open(name); 20898 composeHealthcareServiceHealthcareServiceAvailableTimeComponentInner(element); 20899 close(); 20900 } 20901 } 20902 20903 protected void composeHealthcareServiceHealthcareServiceAvailableTimeComponentInner( 20904 HealthcareService.HealthcareServiceAvailableTimeComponent element) throws IOException { 20905 composeBackbone(element); 20906 if (element.hasDaysOfWeek()) { 20907 openArray("daysOfWeek"); 20908 for (Enumeration<HealthcareService.DaysOfWeek> e : element.getDaysOfWeek()) 20909 composeEnumerationCore(null, e, new HealthcareService.DaysOfWeekEnumFactory(), true); 20910 closeArray(); 20911 if (anyHasExtras(element.getDaysOfWeek())) { 20912 openArray("_daysOfWeek"); 20913 for (Enumeration<HealthcareService.DaysOfWeek> e : element.getDaysOfWeek()) 20914 composeEnumerationExtras(null, e, new HealthcareService.DaysOfWeekEnumFactory(), true); 20915 closeArray(); 20916 } 20917 } 20918 ; 20919 if (element.hasAllDayElement()) { 20920 composeBooleanCore("allDay", element.getAllDayElement(), false); 20921 composeBooleanExtras("allDay", element.getAllDayElement(), false); 20922 } 20923 if (element.hasAvailableStartTimeElement()) { 20924 composeTimeCore("availableStartTime", element.getAvailableStartTimeElement(), false); 20925 composeTimeExtras("availableStartTime", element.getAvailableStartTimeElement(), false); 20926 } 20927 if (element.hasAvailableEndTimeElement()) { 20928 composeTimeCore("availableEndTime", element.getAvailableEndTimeElement(), false); 20929 composeTimeExtras("availableEndTime", element.getAvailableEndTimeElement(), false); 20930 } 20931 } 20932 20933 protected void composeHealthcareServiceHealthcareServiceNotAvailableComponent(String name, 20934 HealthcareService.HealthcareServiceNotAvailableComponent element) throws IOException { 20935 if (element != null) { 20936 open(name); 20937 composeHealthcareServiceHealthcareServiceNotAvailableComponentInner(element); 20938 close(); 20939 } 20940 } 20941 20942 protected void composeHealthcareServiceHealthcareServiceNotAvailableComponentInner( 20943 HealthcareService.HealthcareServiceNotAvailableComponent element) throws IOException { 20944 composeBackbone(element); 20945 if (element.hasDescriptionElement()) { 20946 composeStringCore("description", element.getDescriptionElement(), false); 20947 composeStringExtras("description", element.getDescriptionElement(), false); 20948 } 20949 if (element.hasDuring()) { 20950 composePeriod("during", element.getDuring()); 20951 } 20952 } 20953 20954 protected void composeImagingObjectSelection(String name, ImagingObjectSelection element) throws IOException { 20955 if (element != null) { 20956 prop("resourceType", name); 20957 composeImagingObjectSelectionInner(element); 20958 } 20959 } 20960 20961 protected void composeImagingObjectSelectionInner(ImagingObjectSelection element) throws IOException { 20962 composeDomainResourceElements(element); 20963 if (element.hasUidElement()) { 20964 composeOidCore("uid", element.getUidElement(), false); 20965 composeOidExtras("uid", element.getUidElement(), false); 20966 } 20967 if (element.hasPatient()) { 20968 composeReference("patient", element.getPatient()); 20969 } 20970 if (element.hasTitle()) { 20971 composeCodeableConcept("title", element.getTitle()); 20972 } 20973 if (element.hasDescriptionElement()) { 20974 composeStringCore("description", element.getDescriptionElement(), false); 20975 composeStringExtras("description", element.getDescriptionElement(), false); 20976 } 20977 if (element.hasAuthor()) { 20978 composeReference("author", element.getAuthor()); 20979 } 20980 if (element.hasAuthoringTimeElement()) { 20981 composeDateTimeCore("authoringTime", element.getAuthoringTimeElement(), false); 20982 composeDateTimeExtras("authoringTime", element.getAuthoringTimeElement(), false); 20983 } 20984 if (element.hasStudy()) { 20985 openArray("study"); 20986 for (ImagingObjectSelection.StudyComponent e : element.getStudy()) 20987 composeImagingObjectSelectionStudyComponent(null, e); 20988 closeArray(); 20989 } 20990 ; 20991 } 20992 20993 protected void composeImagingObjectSelectionStudyComponent(String name, ImagingObjectSelection.StudyComponent element) 20994 throws IOException { 20995 if (element != null) { 20996 open(name); 20997 composeImagingObjectSelectionStudyComponentInner(element); 20998 close(); 20999 } 21000 } 21001 21002 protected void composeImagingObjectSelectionStudyComponentInner(ImagingObjectSelection.StudyComponent element) 21003 throws IOException { 21004 composeBackbone(element); 21005 if (element.hasUidElement()) { 21006 composeOidCore("uid", element.getUidElement(), false); 21007 composeOidExtras("uid", element.getUidElement(), false); 21008 } 21009 if (element.hasUrlElement()) { 21010 composeUriCore("url", element.getUrlElement(), false); 21011 composeUriExtras("url", element.getUrlElement(), false); 21012 } 21013 if (element.hasImagingStudy()) { 21014 composeReference("imagingStudy", element.getImagingStudy()); 21015 } 21016 if (element.hasSeries()) { 21017 openArray("series"); 21018 for (ImagingObjectSelection.SeriesComponent e : element.getSeries()) 21019 composeImagingObjectSelectionSeriesComponent(null, e); 21020 closeArray(); 21021 } 21022 ; 21023 } 21024 21025 protected void composeImagingObjectSelectionSeriesComponent(String name, 21026 ImagingObjectSelection.SeriesComponent element) throws IOException { 21027 if (element != null) { 21028 open(name); 21029 composeImagingObjectSelectionSeriesComponentInner(element); 21030 close(); 21031 } 21032 } 21033 21034 protected void composeImagingObjectSelectionSeriesComponentInner(ImagingObjectSelection.SeriesComponent element) 21035 throws IOException { 21036 composeBackbone(element); 21037 if (element.hasUidElement()) { 21038 composeOidCore("uid", element.getUidElement(), false); 21039 composeOidExtras("uid", element.getUidElement(), false); 21040 } 21041 if (element.hasUrlElement()) { 21042 composeUriCore("url", element.getUrlElement(), false); 21043 composeUriExtras("url", element.getUrlElement(), false); 21044 } 21045 if (element.hasInstance()) { 21046 openArray("instance"); 21047 for (ImagingObjectSelection.InstanceComponent e : element.getInstance()) 21048 composeImagingObjectSelectionInstanceComponent(null, e); 21049 closeArray(); 21050 } 21051 ; 21052 } 21053 21054 protected void composeImagingObjectSelectionInstanceComponent(String name, 21055 ImagingObjectSelection.InstanceComponent element) throws IOException { 21056 if (element != null) { 21057 open(name); 21058 composeImagingObjectSelectionInstanceComponentInner(element); 21059 close(); 21060 } 21061 } 21062 21063 protected void composeImagingObjectSelectionInstanceComponentInner(ImagingObjectSelection.InstanceComponent element) 21064 throws IOException { 21065 composeBackbone(element); 21066 if (element.hasSopClassElement()) { 21067 composeOidCore("sopClass", element.getSopClassElement(), false); 21068 composeOidExtras("sopClass", element.getSopClassElement(), false); 21069 } 21070 if (element.hasUidElement()) { 21071 composeOidCore("uid", element.getUidElement(), false); 21072 composeOidExtras("uid", element.getUidElement(), false); 21073 } 21074 if (element.hasUrlElement()) { 21075 composeUriCore("url", element.getUrlElement(), false); 21076 composeUriExtras("url", element.getUrlElement(), false); 21077 } 21078 if (element.hasFrames()) { 21079 openArray("frames"); 21080 for (ImagingObjectSelection.FramesComponent e : element.getFrames()) 21081 composeImagingObjectSelectionFramesComponent(null, e); 21082 closeArray(); 21083 } 21084 ; 21085 } 21086 21087 protected void composeImagingObjectSelectionFramesComponent(String name, 21088 ImagingObjectSelection.FramesComponent element) throws IOException { 21089 if (element != null) { 21090 open(name); 21091 composeImagingObjectSelectionFramesComponentInner(element); 21092 close(); 21093 } 21094 } 21095 21096 protected void composeImagingObjectSelectionFramesComponentInner(ImagingObjectSelection.FramesComponent element) 21097 throws IOException { 21098 composeBackbone(element); 21099 if (element.hasFrameNumbers()) { 21100 openArray("frameNumbers"); 21101 for (UnsignedIntType e : element.getFrameNumbers()) 21102 composeUnsignedIntCore(null, e, true); 21103 closeArray(); 21104 if (anyHasExtras(element.getFrameNumbers())) { 21105 openArray("_frameNumbers"); 21106 for (UnsignedIntType e : element.getFrameNumbers()) 21107 composeUnsignedIntExtras(null, e, true); 21108 closeArray(); 21109 } 21110 } 21111 ; 21112 if (element.hasUrlElement()) { 21113 composeUriCore("url", element.getUrlElement(), false); 21114 composeUriExtras("url", element.getUrlElement(), false); 21115 } 21116 } 21117 21118 protected void composeImagingStudy(String name, ImagingStudy element) throws IOException { 21119 if (element != null) { 21120 prop("resourceType", name); 21121 composeImagingStudyInner(element); 21122 } 21123 } 21124 21125 protected void composeImagingStudyInner(ImagingStudy element) throws IOException { 21126 composeDomainResourceElements(element); 21127 if (element.hasStartedElement()) { 21128 composeDateTimeCore("started", element.getStartedElement(), false); 21129 composeDateTimeExtras("started", element.getStartedElement(), false); 21130 } 21131 if (element.hasPatient()) { 21132 composeReference("patient", element.getPatient()); 21133 } 21134 if (element.hasUidElement()) { 21135 composeOidCore("uid", element.getUidElement(), false); 21136 composeOidExtras("uid", element.getUidElement(), false); 21137 } 21138 if (element.hasAccession()) { 21139 composeIdentifier("accession", element.getAccession()); 21140 } 21141 if (element.hasIdentifier()) { 21142 openArray("identifier"); 21143 for (Identifier e : element.getIdentifier()) 21144 composeIdentifier(null, e); 21145 closeArray(); 21146 } 21147 ; 21148 if (element.hasOrder()) { 21149 openArray("order"); 21150 for (Reference e : element.getOrder()) 21151 composeReference(null, e); 21152 closeArray(); 21153 } 21154 ; 21155 if (element.hasModalityList()) { 21156 openArray("modalityList"); 21157 for (Coding e : element.getModalityList()) 21158 composeCoding(null, e); 21159 closeArray(); 21160 } 21161 ; 21162 if (element.hasReferrer()) { 21163 composeReference("referrer", element.getReferrer()); 21164 } 21165 if (element.hasAvailabilityElement()) { 21166 composeEnumerationCore("availability", element.getAvailabilityElement(), 21167 new ImagingStudy.InstanceAvailabilityEnumFactory(), false); 21168 composeEnumerationExtras("availability", element.getAvailabilityElement(), 21169 new ImagingStudy.InstanceAvailabilityEnumFactory(), false); 21170 } 21171 if (element.hasUrlElement()) { 21172 composeUriCore("url", element.getUrlElement(), false); 21173 composeUriExtras("url", element.getUrlElement(), false); 21174 } 21175 if (element.hasNumberOfSeriesElement()) { 21176 composeUnsignedIntCore("numberOfSeries", element.getNumberOfSeriesElement(), false); 21177 composeUnsignedIntExtras("numberOfSeries", element.getNumberOfSeriesElement(), false); 21178 } 21179 if (element.hasNumberOfInstancesElement()) { 21180 composeUnsignedIntCore("numberOfInstances", element.getNumberOfInstancesElement(), false); 21181 composeUnsignedIntExtras("numberOfInstances", element.getNumberOfInstancesElement(), false); 21182 } 21183 if (element.hasProcedure()) { 21184 openArray("procedure"); 21185 for (Reference e : element.getProcedure()) 21186 composeReference(null, e); 21187 closeArray(); 21188 } 21189 ; 21190 if (element.hasInterpreter()) { 21191 composeReference("interpreter", element.getInterpreter()); 21192 } 21193 if (element.hasDescriptionElement()) { 21194 composeStringCore("description", element.getDescriptionElement(), false); 21195 composeStringExtras("description", element.getDescriptionElement(), false); 21196 } 21197 if (element.hasSeries()) { 21198 openArray("series"); 21199 for (ImagingStudy.ImagingStudySeriesComponent e : element.getSeries()) 21200 composeImagingStudyImagingStudySeriesComponent(null, e); 21201 closeArray(); 21202 } 21203 ; 21204 } 21205 21206 protected void composeImagingStudyImagingStudySeriesComponent(String name, 21207 ImagingStudy.ImagingStudySeriesComponent element) throws IOException { 21208 if (element != null) { 21209 open(name); 21210 composeImagingStudyImagingStudySeriesComponentInner(element); 21211 close(); 21212 } 21213 } 21214 21215 protected void composeImagingStudyImagingStudySeriesComponentInner(ImagingStudy.ImagingStudySeriesComponent element) 21216 throws IOException { 21217 composeBackbone(element); 21218 if (element.hasNumberElement()) { 21219 composeUnsignedIntCore("number", element.getNumberElement(), false); 21220 composeUnsignedIntExtras("number", element.getNumberElement(), false); 21221 } 21222 if (element.hasModality()) { 21223 composeCoding("modality", element.getModality()); 21224 } 21225 if (element.hasUidElement()) { 21226 composeOidCore("uid", element.getUidElement(), false); 21227 composeOidExtras("uid", element.getUidElement(), false); 21228 } 21229 if (element.hasDescriptionElement()) { 21230 composeStringCore("description", element.getDescriptionElement(), false); 21231 composeStringExtras("description", element.getDescriptionElement(), false); 21232 } 21233 if (element.hasNumberOfInstancesElement()) { 21234 composeUnsignedIntCore("numberOfInstances", element.getNumberOfInstancesElement(), false); 21235 composeUnsignedIntExtras("numberOfInstances", element.getNumberOfInstancesElement(), false); 21236 } 21237 if (element.hasAvailabilityElement()) { 21238 composeEnumerationCore("availability", element.getAvailabilityElement(), 21239 new ImagingStudy.InstanceAvailabilityEnumFactory(), false); 21240 composeEnumerationExtras("availability", element.getAvailabilityElement(), 21241 new ImagingStudy.InstanceAvailabilityEnumFactory(), false); 21242 } 21243 if (element.hasUrlElement()) { 21244 composeUriCore("url", element.getUrlElement(), false); 21245 composeUriExtras("url", element.getUrlElement(), false); 21246 } 21247 if (element.hasBodySite()) { 21248 composeCoding("bodySite", element.getBodySite()); 21249 } 21250 if (element.hasLaterality()) { 21251 composeCoding("laterality", element.getLaterality()); 21252 } 21253 if (element.hasStartedElement()) { 21254 composeDateTimeCore("started", element.getStartedElement(), false); 21255 composeDateTimeExtras("started", element.getStartedElement(), false); 21256 } 21257 if (element.hasInstance()) { 21258 openArray("instance"); 21259 for (ImagingStudy.ImagingStudySeriesInstanceComponent e : element.getInstance()) 21260 composeImagingStudyImagingStudySeriesInstanceComponent(null, e); 21261 closeArray(); 21262 } 21263 ; 21264 } 21265 21266 protected void composeImagingStudyImagingStudySeriesInstanceComponent(String name, 21267 ImagingStudy.ImagingStudySeriesInstanceComponent element) throws IOException { 21268 if (element != null) { 21269 open(name); 21270 composeImagingStudyImagingStudySeriesInstanceComponentInner(element); 21271 close(); 21272 } 21273 } 21274 21275 protected void composeImagingStudyImagingStudySeriesInstanceComponentInner( 21276 ImagingStudy.ImagingStudySeriesInstanceComponent element) throws IOException { 21277 composeBackbone(element); 21278 if (element.hasNumberElement()) { 21279 composeUnsignedIntCore("number", element.getNumberElement(), false); 21280 composeUnsignedIntExtras("number", element.getNumberElement(), false); 21281 } 21282 if (element.hasUidElement()) { 21283 composeOidCore("uid", element.getUidElement(), false); 21284 composeOidExtras("uid", element.getUidElement(), false); 21285 } 21286 if (element.hasSopClassElement()) { 21287 composeOidCore("sopClass", element.getSopClassElement(), false); 21288 composeOidExtras("sopClass", element.getSopClassElement(), false); 21289 } 21290 if (element.hasTypeElement()) { 21291 composeStringCore("type", element.getTypeElement(), false); 21292 composeStringExtras("type", element.getTypeElement(), false); 21293 } 21294 if (element.hasTitleElement()) { 21295 composeStringCore("title", element.getTitleElement(), false); 21296 composeStringExtras("title", element.getTitleElement(), false); 21297 } 21298 if (element.hasContent()) { 21299 openArray("content"); 21300 for (Attachment e : element.getContent()) 21301 composeAttachment(null, e); 21302 closeArray(); 21303 } 21304 ; 21305 } 21306 21307 protected void composeImmunization(String name, Immunization element) throws IOException { 21308 if (element != null) { 21309 prop("resourceType", name); 21310 composeImmunizationInner(element); 21311 } 21312 } 21313 21314 protected void composeImmunizationInner(Immunization element) throws IOException { 21315 composeDomainResourceElements(element); 21316 if (element.hasIdentifier()) { 21317 openArray("identifier"); 21318 for (Identifier e : element.getIdentifier()) 21319 composeIdentifier(null, e); 21320 closeArray(); 21321 } 21322 ; 21323 if (element.hasStatusElement()) { 21324 composeCodeCore("status", element.getStatusElement(), false); 21325 composeCodeExtras("status", element.getStatusElement(), false); 21326 } 21327 if (element.hasDateElement()) { 21328 composeDateTimeCore("date", element.getDateElement(), false); 21329 composeDateTimeExtras("date", element.getDateElement(), false); 21330 } 21331 if (element.hasVaccineCode()) { 21332 composeCodeableConcept("vaccineCode", element.getVaccineCode()); 21333 } 21334 if (element.hasPatient()) { 21335 composeReference("patient", element.getPatient()); 21336 } 21337 if (element.hasWasNotGivenElement()) { 21338 composeBooleanCore("wasNotGiven", element.getWasNotGivenElement(), false); 21339 composeBooleanExtras("wasNotGiven", element.getWasNotGivenElement(), false); 21340 } 21341 if (element.hasReportedElement()) { 21342 composeBooleanCore("reported", element.getReportedElement(), false); 21343 composeBooleanExtras("reported", element.getReportedElement(), false); 21344 } 21345 if (element.hasPerformer()) { 21346 composeReference("performer", element.getPerformer()); 21347 } 21348 if (element.hasRequester()) { 21349 composeReference("requester", element.getRequester()); 21350 } 21351 if (element.hasEncounter()) { 21352 composeReference("encounter", element.getEncounter()); 21353 } 21354 if (element.hasManufacturer()) { 21355 composeReference("manufacturer", element.getManufacturer()); 21356 } 21357 if (element.hasLocation()) { 21358 composeReference("location", element.getLocation()); 21359 } 21360 if (element.hasLotNumberElement()) { 21361 composeStringCore("lotNumber", element.getLotNumberElement(), false); 21362 composeStringExtras("lotNumber", element.getLotNumberElement(), false); 21363 } 21364 if (element.hasExpirationDateElement()) { 21365 composeDateCore("expirationDate", element.getExpirationDateElement(), false); 21366 composeDateExtras("expirationDate", element.getExpirationDateElement(), false); 21367 } 21368 if (element.hasSite()) { 21369 composeCodeableConcept("site", element.getSite()); 21370 } 21371 if (element.hasRoute()) { 21372 composeCodeableConcept("route", element.getRoute()); 21373 } 21374 if (element.hasDoseQuantity()) { 21375 composeSimpleQuantity("doseQuantity", element.getDoseQuantity()); 21376 } 21377 if (element.hasNote()) { 21378 openArray("note"); 21379 for (Annotation e : element.getNote()) 21380 composeAnnotation(null, e); 21381 closeArray(); 21382 } 21383 ; 21384 if (element.hasExplanation()) { 21385 composeImmunizationImmunizationExplanationComponent("explanation", element.getExplanation()); 21386 } 21387 if (element.hasReaction()) { 21388 openArray("reaction"); 21389 for (Immunization.ImmunizationReactionComponent e : element.getReaction()) 21390 composeImmunizationImmunizationReactionComponent(null, e); 21391 closeArray(); 21392 } 21393 ; 21394 if (element.hasVaccinationProtocol()) { 21395 openArray("vaccinationProtocol"); 21396 for (Immunization.ImmunizationVaccinationProtocolComponent e : element.getVaccinationProtocol()) 21397 composeImmunizationImmunizationVaccinationProtocolComponent(null, e); 21398 closeArray(); 21399 } 21400 ; 21401 } 21402 21403 protected void composeImmunizationImmunizationExplanationComponent(String name, 21404 Immunization.ImmunizationExplanationComponent element) throws IOException { 21405 if (element != null) { 21406 open(name); 21407 composeImmunizationImmunizationExplanationComponentInner(element); 21408 close(); 21409 } 21410 } 21411 21412 protected void composeImmunizationImmunizationExplanationComponentInner( 21413 Immunization.ImmunizationExplanationComponent element) throws IOException { 21414 composeBackbone(element); 21415 if (element.hasReason()) { 21416 openArray("reason"); 21417 for (CodeableConcept e : element.getReason()) 21418 composeCodeableConcept(null, e); 21419 closeArray(); 21420 } 21421 ; 21422 if (element.hasReasonNotGiven()) { 21423 openArray("reasonNotGiven"); 21424 for (CodeableConcept e : element.getReasonNotGiven()) 21425 composeCodeableConcept(null, e); 21426 closeArray(); 21427 } 21428 ; 21429 } 21430 21431 protected void composeImmunizationImmunizationReactionComponent(String name, 21432 Immunization.ImmunizationReactionComponent element) throws IOException { 21433 if (element != null) { 21434 open(name); 21435 composeImmunizationImmunizationReactionComponentInner(element); 21436 close(); 21437 } 21438 } 21439 21440 protected void composeImmunizationImmunizationReactionComponentInner( 21441 Immunization.ImmunizationReactionComponent element) throws IOException { 21442 composeBackbone(element); 21443 if (element.hasDateElement()) { 21444 composeDateTimeCore("date", element.getDateElement(), false); 21445 composeDateTimeExtras("date", element.getDateElement(), false); 21446 } 21447 if (element.hasDetail()) { 21448 composeReference("detail", element.getDetail()); 21449 } 21450 if (element.hasReportedElement()) { 21451 composeBooleanCore("reported", element.getReportedElement(), false); 21452 composeBooleanExtras("reported", element.getReportedElement(), false); 21453 } 21454 } 21455 21456 protected void composeImmunizationImmunizationVaccinationProtocolComponent(String name, 21457 Immunization.ImmunizationVaccinationProtocolComponent element) throws IOException { 21458 if (element != null) { 21459 open(name); 21460 composeImmunizationImmunizationVaccinationProtocolComponentInner(element); 21461 close(); 21462 } 21463 } 21464 21465 protected void composeImmunizationImmunizationVaccinationProtocolComponentInner( 21466 Immunization.ImmunizationVaccinationProtocolComponent element) throws IOException { 21467 composeBackbone(element); 21468 if (element.hasDoseSequenceElement()) { 21469 composePositiveIntCore("doseSequence", element.getDoseSequenceElement(), false); 21470 composePositiveIntExtras("doseSequence", element.getDoseSequenceElement(), false); 21471 } 21472 if (element.hasDescriptionElement()) { 21473 composeStringCore("description", element.getDescriptionElement(), false); 21474 composeStringExtras("description", element.getDescriptionElement(), false); 21475 } 21476 if (element.hasAuthority()) { 21477 composeReference("authority", element.getAuthority()); 21478 } 21479 if (element.hasSeriesElement()) { 21480 composeStringCore("series", element.getSeriesElement(), false); 21481 composeStringExtras("series", element.getSeriesElement(), false); 21482 } 21483 if (element.hasSeriesDosesElement()) { 21484 composePositiveIntCore("seriesDoses", element.getSeriesDosesElement(), false); 21485 composePositiveIntExtras("seriesDoses", element.getSeriesDosesElement(), false); 21486 } 21487 if (element.hasTargetDisease()) { 21488 openArray("targetDisease"); 21489 for (CodeableConcept e : element.getTargetDisease()) 21490 composeCodeableConcept(null, e); 21491 closeArray(); 21492 } 21493 ; 21494 if (element.hasDoseStatus()) { 21495 composeCodeableConcept("doseStatus", element.getDoseStatus()); 21496 } 21497 if (element.hasDoseStatusReason()) { 21498 composeCodeableConcept("doseStatusReason", element.getDoseStatusReason()); 21499 } 21500 } 21501 21502 protected void composeImmunizationRecommendation(String name, ImmunizationRecommendation element) throws IOException { 21503 if (element != null) { 21504 prop("resourceType", name); 21505 composeImmunizationRecommendationInner(element); 21506 } 21507 } 21508 21509 protected void composeImmunizationRecommendationInner(ImmunizationRecommendation element) throws IOException { 21510 composeDomainResourceElements(element); 21511 if (element.hasIdentifier()) { 21512 openArray("identifier"); 21513 for (Identifier e : element.getIdentifier()) 21514 composeIdentifier(null, e); 21515 closeArray(); 21516 } 21517 ; 21518 if (element.hasPatient()) { 21519 composeReference("patient", element.getPatient()); 21520 } 21521 if (element.hasRecommendation()) { 21522 openArray("recommendation"); 21523 for (ImmunizationRecommendation.ImmunizationRecommendationRecommendationComponent e : element.getRecommendation()) 21524 composeImmunizationRecommendationImmunizationRecommendationRecommendationComponent(null, e); 21525 closeArray(); 21526 } 21527 ; 21528 } 21529 21530 protected void composeImmunizationRecommendationImmunizationRecommendationRecommendationComponent(String name, 21531 ImmunizationRecommendation.ImmunizationRecommendationRecommendationComponent element) throws IOException { 21532 if (element != null) { 21533 open(name); 21534 composeImmunizationRecommendationImmunizationRecommendationRecommendationComponentInner(element); 21535 close(); 21536 } 21537 } 21538 21539 protected void composeImmunizationRecommendationImmunizationRecommendationRecommendationComponentInner( 21540 ImmunizationRecommendation.ImmunizationRecommendationRecommendationComponent element) throws IOException { 21541 composeBackbone(element); 21542 if (element.hasDateElement()) { 21543 composeDateTimeCore("date", element.getDateElement(), false); 21544 composeDateTimeExtras("date", element.getDateElement(), false); 21545 } 21546 if (element.hasVaccineCode()) { 21547 composeCodeableConcept("vaccineCode", element.getVaccineCode()); 21548 } 21549 if (element.hasDoseNumberElement()) { 21550 composePositiveIntCore("doseNumber", element.getDoseNumberElement(), false); 21551 composePositiveIntExtras("doseNumber", element.getDoseNumberElement(), false); 21552 } 21553 if (element.hasForecastStatus()) { 21554 composeCodeableConcept("forecastStatus", element.getForecastStatus()); 21555 } 21556 if (element.hasDateCriterion()) { 21557 openArray("dateCriterion"); 21558 for (ImmunizationRecommendation.ImmunizationRecommendationRecommendationDateCriterionComponent e : element 21559 .getDateCriterion()) 21560 composeImmunizationRecommendationImmunizationRecommendationRecommendationDateCriterionComponent(null, e); 21561 closeArray(); 21562 } 21563 ; 21564 if (element.hasProtocol()) { 21565 composeImmunizationRecommendationImmunizationRecommendationRecommendationProtocolComponent("protocol", 21566 element.getProtocol()); 21567 } 21568 if (element.hasSupportingImmunization()) { 21569 openArray("supportingImmunization"); 21570 for (Reference e : element.getSupportingImmunization()) 21571 composeReference(null, e); 21572 closeArray(); 21573 } 21574 ; 21575 if (element.hasSupportingPatientInformation()) { 21576 openArray("supportingPatientInformation"); 21577 for (Reference e : element.getSupportingPatientInformation()) 21578 composeReference(null, e); 21579 closeArray(); 21580 } 21581 ; 21582 } 21583 21584 protected void composeImmunizationRecommendationImmunizationRecommendationRecommendationDateCriterionComponent( 21585 String name, ImmunizationRecommendation.ImmunizationRecommendationRecommendationDateCriterionComponent element) 21586 throws IOException { 21587 if (element != null) { 21588 open(name); 21589 composeImmunizationRecommendationImmunizationRecommendationRecommendationDateCriterionComponentInner(element); 21590 close(); 21591 } 21592 } 21593 21594 protected void composeImmunizationRecommendationImmunizationRecommendationRecommendationDateCriterionComponentInner( 21595 ImmunizationRecommendation.ImmunizationRecommendationRecommendationDateCriterionComponent element) 21596 throws IOException { 21597 composeBackbone(element); 21598 if (element.hasCode()) { 21599 composeCodeableConcept("code", element.getCode()); 21600 } 21601 if (element.hasValueElement()) { 21602 composeDateTimeCore("value", element.getValueElement(), false); 21603 composeDateTimeExtras("value", element.getValueElement(), false); 21604 } 21605 } 21606 21607 protected void composeImmunizationRecommendationImmunizationRecommendationRecommendationProtocolComponent(String name, 21608 ImmunizationRecommendation.ImmunizationRecommendationRecommendationProtocolComponent element) throws IOException { 21609 if (element != null) { 21610 open(name); 21611 composeImmunizationRecommendationImmunizationRecommendationRecommendationProtocolComponentInner(element); 21612 close(); 21613 } 21614 } 21615 21616 protected void composeImmunizationRecommendationImmunizationRecommendationRecommendationProtocolComponentInner( 21617 ImmunizationRecommendation.ImmunizationRecommendationRecommendationProtocolComponent element) throws IOException { 21618 composeBackbone(element); 21619 if (element.hasDoseSequenceElement()) { 21620 composeIntegerCore("doseSequence", element.getDoseSequenceElement(), false); 21621 composeIntegerExtras("doseSequence", element.getDoseSequenceElement(), false); 21622 } 21623 if (element.hasDescriptionElement()) { 21624 composeStringCore("description", element.getDescriptionElement(), false); 21625 composeStringExtras("description", element.getDescriptionElement(), false); 21626 } 21627 if (element.hasAuthority()) { 21628 composeReference("authority", element.getAuthority()); 21629 } 21630 if (element.hasSeriesElement()) { 21631 composeStringCore("series", element.getSeriesElement(), false); 21632 composeStringExtras("series", element.getSeriesElement(), false); 21633 } 21634 } 21635 21636 protected void composeImplementationGuide(String name, ImplementationGuide element) throws IOException { 21637 if (element != null) { 21638 prop("resourceType", name); 21639 composeImplementationGuideInner(element); 21640 } 21641 } 21642 21643 protected void composeImplementationGuideInner(ImplementationGuide element) throws IOException { 21644 composeDomainResourceElements(element); 21645 if (element.hasUrlElement()) { 21646 composeUriCore("url", element.getUrlElement(), false); 21647 composeUriExtras("url", element.getUrlElement(), false); 21648 } 21649 if (element.hasVersionElement()) { 21650 composeStringCore("version", element.getVersionElement(), false); 21651 composeStringExtras("version", element.getVersionElement(), false); 21652 } 21653 if (element.hasNameElement()) { 21654 composeStringCore("name", element.getNameElement(), false); 21655 composeStringExtras("name", element.getNameElement(), false); 21656 } 21657 if (element.hasStatusElement()) { 21658 composeEnumerationCore("status", element.getStatusElement(), 21659 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 21660 composeEnumerationExtras("status", element.getStatusElement(), 21661 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 21662 } 21663 if (element.hasExperimentalElement()) { 21664 composeBooleanCore("experimental", element.getExperimentalElement(), false); 21665 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 21666 } 21667 if (element.hasPublisherElement()) { 21668 composeStringCore("publisher", element.getPublisherElement(), false); 21669 composeStringExtras("publisher", element.getPublisherElement(), false); 21670 } 21671 if (element.hasContact()) { 21672 openArray("contact"); 21673 for (ImplementationGuide.ImplementationGuideContactComponent e : element.getContact()) 21674 composeImplementationGuideImplementationGuideContactComponent(null, e); 21675 closeArray(); 21676 } 21677 ; 21678 if (element.hasDateElement()) { 21679 composeDateTimeCore("date", element.getDateElement(), false); 21680 composeDateTimeExtras("date", element.getDateElement(), false); 21681 } 21682 if (element.hasDescriptionElement()) { 21683 composeStringCore("description", element.getDescriptionElement(), false); 21684 composeStringExtras("description", element.getDescriptionElement(), false); 21685 } 21686 if (element.hasUseContext()) { 21687 openArray("useContext"); 21688 for (CodeableConcept e : element.getUseContext()) 21689 composeCodeableConcept(null, e); 21690 closeArray(); 21691 } 21692 ; 21693 if (element.hasCopyrightElement()) { 21694 composeStringCore("copyright", element.getCopyrightElement(), false); 21695 composeStringExtras("copyright", element.getCopyrightElement(), false); 21696 } 21697 if (element.hasFhirVersionElement()) { 21698 composeIdCore("fhirVersion", element.getFhirVersionElement(), false); 21699 composeIdExtras("fhirVersion", element.getFhirVersionElement(), false); 21700 } 21701 if (element.hasDependency()) { 21702 openArray("dependency"); 21703 for (ImplementationGuide.ImplementationGuideDependencyComponent e : element.getDependency()) 21704 composeImplementationGuideImplementationGuideDependencyComponent(null, e); 21705 closeArray(); 21706 } 21707 ; 21708 if (element.hasPackage()) { 21709 openArray("package"); 21710 for (ImplementationGuide.ImplementationGuidePackageComponent e : element.getPackage()) 21711 composeImplementationGuideImplementationGuidePackageComponent(null, e); 21712 closeArray(); 21713 } 21714 ; 21715 if (element.hasGlobal()) { 21716 openArray("global"); 21717 for (ImplementationGuide.ImplementationGuideGlobalComponent e : element.getGlobal()) 21718 composeImplementationGuideImplementationGuideGlobalComponent(null, e); 21719 closeArray(); 21720 } 21721 ; 21722 if (element.hasBinary()) { 21723 openArray("binary"); 21724 for (UriType e : element.getBinary()) 21725 composeUriCore(null, e, true); 21726 closeArray(); 21727 if (anyHasExtras(element.getBinary())) { 21728 openArray("_binary"); 21729 for (UriType e : element.getBinary()) 21730 composeUriExtras(null, e, true); 21731 closeArray(); 21732 } 21733 } 21734 ; 21735 if (element.hasPage()) { 21736 composeImplementationGuideImplementationGuidePageComponent("page", element.getPage()); 21737 } 21738 } 21739 21740 protected void composeImplementationGuideImplementationGuideContactComponent(String name, 21741 ImplementationGuide.ImplementationGuideContactComponent element) throws IOException { 21742 if (element != null) { 21743 open(name); 21744 composeImplementationGuideImplementationGuideContactComponentInner(element); 21745 close(); 21746 } 21747 } 21748 21749 protected void composeImplementationGuideImplementationGuideContactComponentInner( 21750 ImplementationGuide.ImplementationGuideContactComponent element) throws IOException { 21751 composeBackbone(element); 21752 if (element.hasNameElement()) { 21753 composeStringCore("name", element.getNameElement(), false); 21754 composeStringExtras("name", element.getNameElement(), false); 21755 } 21756 if (element.hasTelecom()) { 21757 openArray("telecom"); 21758 for (ContactPoint e : element.getTelecom()) 21759 composeContactPoint(null, e); 21760 closeArray(); 21761 } 21762 ; 21763 } 21764 21765 protected void composeImplementationGuideImplementationGuideDependencyComponent(String name, 21766 ImplementationGuide.ImplementationGuideDependencyComponent element) throws IOException { 21767 if (element != null) { 21768 open(name); 21769 composeImplementationGuideImplementationGuideDependencyComponentInner(element); 21770 close(); 21771 } 21772 } 21773 21774 protected void composeImplementationGuideImplementationGuideDependencyComponentInner( 21775 ImplementationGuide.ImplementationGuideDependencyComponent element) throws IOException { 21776 composeBackbone(element); 21777 if (element.hasTypeElement()) { 21778 composeEnumerationCore("type", element.getTypeElement(), new ImplementationGuide.GuideDependencyTypeEnumFactory(), 21779 false); 21780 composeEnumerationExtras("type", element.getTypeElement(), 21781 new ImplementationGuide.GuideDependencyTypeEnumFactory(), false); 21782 } 21783 if (element.hasUriElement()) { 21784 composeUriCore("uri", element.getUriElement(), false); 21785 composeUriExtras("uri", element.getUriElement(), false); 21786 } 21787 } 21788 21789 protected void composeImplementationGuideImplementationGuidePackageComponent(String name, 21790 ImplementationGuide.ImplementationGuidePackageComponent element) throws IOException { 21791 if (element != null) { 21792 open(name); 21793 composeImplementationGuideImplementationGuidePackageComponentInner(element); 21794 close(); 21795 } 21796 } 21797 21798 protected void composeImplementationGuideImplementationGuidePackageComponentInner( 21799 ImplementationGuide.ImplementationGuidePackageComponent element) throws IOException { 21800 composeBackbone(element); 21801 if (element.hasNameElement()) { 21802 composeStringCore("name", element.getNameElement(), false); 21803 composeStringExtras("name", element.getNameElement(), false); 21804 } 21805 if (element.hasDescriptionElement()) { 21806 composeStringCore("description", element.getDescriptionElement(), false); 21807 composeStringExtras("description", element.getDescriptionElement(), false); 21808 } 21809 if (element.hasResource()) { 21810 openArray("resource"); 21811 for (ImplementationGuide.ImplementationGuidePackageResourceComponent e : element.getResource()) 21812 composeImplementationGuideImplementationGuidePackageResourceComponent(null, e); 21813 closeArray(); 21814 } 21815 ; 21816 } 21817 21818 protected void composeImplementationGuideImplementationGuidePackageResourceComponent(String name, 21819 ImplementationGuide.ImplementationGuidePackageResourceComponent element) throws IOException { 21820 if (element != null) { 21821 open(name); 21822 composeImplementationGuideImplementationGuidePackageResourceComponentInner(element); 21823 close(); 21824 } 21825 } 21826 21827 protected void composeImplementationGuideImplementationGuidePackageResourceComponentInner( 21828 ImplementationGuide.ImplementationGuidePackageResourceComponent element) throws IOException { 21829 composeBackbone(element); 21830 if (element.hasPurposeElement()) { 21831 composeEnumerationCore("purpose", element.getPurposeElement(), 21832 new ImplementationGuide.GuideResourcePurposeEnumFactory(), false); 21833 composeEnumerationExtras("purpose", element.getPurposeElement(), 21834 new ImplementationGuide.GuideResourcePurposeEnumFactory(), false); 21835 } 21836 if (element.hasNameElement()) { 21837 composeStringCore("name", element.getNameElement(), false); 21838 composeStringExtras("name", element.getNameElement(), false); 21839 } 21840 if (element.hasDescriptionElement()) { 21841 composeStringCore("description", element.getDescriptionElement(), false); 21842 composeStringExtras("description", element.getDescriptionElement(), false); 21843 } 21844 if (element.hasAcronymElement()) { 21845 composeStringCore("acronym", element.getAcronymElement(), false); 21846 composeStringExtras("acronym", element.getAcronymElement(), false); 21847 } 21848 if (element.hasSource()) { 21849 composeType("source", element.getSource()); 21850 } 21851 if (element.hasExampleFor()) { 21852 composeReference("exampleFor", element.getExampleFor()); 21853 } 21854 } 21855 21856 protected void composeImplementationGuideImplementationGuideGlobalComponent(String name, 21857 ImplementationGuide.ImplementationGuideGlobalComponent element) throws IOException { 21858 if (element != null) { 21859 open(name); 21860 composeImplementationGuideImplementationGuideGlobalComponentInner(element); 21861 close(); 21862 } 21863 } 21864 21865 protected void composeImplementationGuideImplementationGuideGlobalComponentInner( 21866 ImplementationGuide.ImplementationGuideGlobalComponent element) throws IOException { 21867 composeBackbone(element); 21868 if (element.hasTypeElement()) { 21869 composeCodeCore("type", element.getTypeElement(), false); 21870 composeCodeExtras("type", element.getTypeElement(), false); 21871 } 21872 if (element.hasProfile()) { 21873 composeReference("profile", element.getProfile()); 21874 } 21875 } 21876 21877 protected void composeImplementationGuideImplementationGuidePageComponent(String name, 21878 ImplementationGuide.ImplementationGuidePageComponent element) throws IOException { 21879 if (element != null) { 21880 open(name); 21881 composeImplementationGuideImplementationGuidePageComponentInner(element); 21882 close(); 21883 } 21884 } 21885 21886 protected void composeImplementationGuideImplementationGuidePageComponentInner( 21887 ImplementationGuide.ImplementationGuidePageComponent element) throws IOException { 21888 composeBackbone(element); 21889 if (element.hasSourceElement()) { 21890 composeUriCore("source", element.getSourceElement(), false); 21891 composeUriExtras("source", element.getSourceElement(), false); 21892 } 21893 if (element.hasNameElement()) { 21894 composeStringCore("name", element.getNameElement(), false); 21895 composeStringExtras("name", element.getNameElement(), false); 21896 } 21897 if (element.hasKindElement()) { 21898 composeEnumerationCore("kind", element.getKindElement(), new ImplementationGuide.GuidePageKindEnumFactory(), 21899 false); 21900 composeEnumerationExtras("kind", element.getKindElement(), new ImplementationGuide.GuidePageKindEnumFactory(), 21901 false); 21902 } 21903 if (element.hasType()) { 21904 openArray("type"); 21905 for (CodeType e : element.getType()) 21906 composeCodeCore(null, e, true); 21907 closeArray(); 21908 if (anyHasExtras(element.getType())) { 21909 openArray("_type"); 21910 for (CodeType e : element.getType()) 21911 composeCodeExtras(null, e, true); 21912 closeArray(); 21913 } 21914 } 21915 ; 21916 if (element.hasPackage()) { 21917 openArray("package"); 21918 for (StringType e : element.getPackage()) 21919 composeStringCore(null, e, true); 21920 closeArray(); 21921 if (anyHasExtras(element.getPackage())) { 21922 openArray("_package"); 21923 for (StringType e : element.getPackage()) 21924 composeStringExtras(null, e, true); 21925 closeArray(); 21926 } 21927 } 21928 ; 21929 if (element.hasFormatElement()) { 21930 composeCodeCore("format", element.getFormatElement(), false); 21931 composeCodeExtras("format", element.getFormatElement(), false); 21932 } 21933 if (element.hasPage()) { 21934 openArray("page"); 21935 for (ImplementationGuide.ImplementationGuidePageComponent e : element.getPage()) 21936 composeImplementationGuideImplementationGuidePageComponent(null, e); 21937 closeArray(); 21938 } 21939 ; 21940 } 21941 21942 protected void composeList_(String name, List_ element) throws IOException { 21943 if (element != null) { 21944 prop("resourceType", name); 21945 composeList_Inner(element); 21946 } 21947 } 21948 21949 protected void composeList_Inner(List_ element) throws IOException { 21950 composeDomainResourceElements(element); 21951 if (element.hasIdentifier()) { 21952 openArray("identifier"); 21953 for (Identifier e : element.getIdentifier()) 21954 composeIdentifier(null, e); 21955 closeArray(); 21956 } 21957 ; 21958 if (element.hasTitleElement()) { 21959 composeStringCore("title", element.getTitleElement(), false); 21960 composeStringExtras("title", element.getTitleElement(), false); 21961 } 21962 if (element.hasCode()) { 21963 composeCodeableConcept("code", element.getCode()); 21964 } 21965 if (element.hasSubject()) { 21966 composeReference("subject", element.getSubject()); 21967 } 21968 if (element.hasSource()) { 21969 composeReference("source", element.getSource()); 21970 } 21971 if (element.hasEncounter()) { 21972 composeReference("encounter", element.getEncounter()); 21973 } 21974 if (element.hasStatusElement()) { 21975 composeEnumerationCore("status", element.getStatusElement(), new List_.ListStatusEnumFactory(), false); 21976 composeEnumerationExtras("status", element.getStatusElement(), new List_.ListStatusEnumFactory(), false); 21977 } 21978 if (element.hasDateElement()) { 21979 composeDateTimeCore("date", element.getDateElement(), false); 21980 composeDateTimeExtras("date", element.getDateElement(), false); 21981 } 21982 if (element.hasOrderedBy()) { 21983 composeCodeableConcept("orderedBy", element.getOrderedBy()); 21984 } 21985 if (element.hasModeElement()) { 21986 composeEnumerationCore("mode", element.getModeElement(), new List_.ListModeEnumFactory(), false); 21987 composeEnumerationExtras("mode", element.getModeElement(), new List_.ListModeEnumFactory(), false); 21988 } 21989 if (element.hasNoteElement()) { 21990 composeStringCore("note", element.getNoteElement(), false); 21991 composeStringExtras("note", element.getNoteElement(), false); 21992 } 21993 if (element.hasEntry()) { 21994 openArray("entry"); 21995 for (List_.ListEntryComponent e : element.getEntry()) 21996 composeList_ListEntryComponent(null, e); 21997 closeArray(); 21998 } 21999 ; 22000 if (element.hasEmptyReason()) { 22001 composeCodeableConcept("emptyReason", element.getEmptyReason()); 22002 } 22003 } 22004 22005 protected void composeList_ListEntryComponent(String name, List_.ListEntryComponent element) throws IOException { 22006 if (element != null) { 22007 open(name); 22008 composeList_ListEntryComponentInner(element); 22009 close(); 22010 } 22011 } 22012 22013 protected void composeList_ListEntryComponentInner(List_.ListEntryComponent element) throws IOException { 22014 composeBackbone(element); 22015 if (element.hasFlag()) { 22016 composeCodeableConcept("flag", element.getFlag()); 22017 } 22018 if (element.hasDeletedElement()) { 22019 composeBooleanCore("deleted", element.getDeletedElement(), false); 22020 composeBooleanExtras("deleted", element.getDeletedElement(), false); 22021 } 22022 if (element.hasDateElement()) { 22023 composeDateTimeCore("date", element.getDateElement(), false); 22024 composeDateTimeExtras("date", element.getDateElement(), false); 22025 } 22026 if (element.hasItem()) { 22027 composeReference("item", element.getItem()); 22028 } 22029 } 22030 22031 protected void composeLocation(String name, Location element) throws IOException { 22032 if (element != null) { 22033 prop("resourceType", name); 22034 composeLocationInner(element); 22035 } 22036 } 22037 22038 protected void composeLocationInner(Location element) throws IOException { 22039 composeDomainResourceElements(element); 22040 if (element.hasIdentifier()) { 22041 openArray("identifier"); 22042 for (Identifier e : element.getIdentifier()) 22043 composeIdentifier(null, e); 22044 closeArray(); 22045 } 22046 ; 22047 if (element.hasStatusElement()) { 22048 composeEnumerationCore("status", element.getStatusElement(), new Location.LocationStatusEnumFactory(), false); 22049 composeEnumerationExtras("status", element.getStatusElement(), new Location.LocationStatusEnumFactory(), false); 22050 } 22051 if (element.hasNameElement()) { 22052 composeStringCore("name", element.getNameElement(), false); 22053 composeStringExtras("name", element.getNameElement(), false); 22054 } 22055 if (element.hasDescriptionElement()) { 22056 composeStringCore("description", element.getDescriptionElement(), false); 22057 composeStringExtras("description", element.getDescriptionElement(), false); 22058 } 22059 if (element.hasModeElement()) { 22060 composeEnumerationCore("mode", element.getModeElement(), new Location.LocationModeEnumFactory(), false); 22061 composeEnumerationExtras("mode", element.getModeElement(), new Location.LocationModeEnumFactory(), false); 22062 } 22063 if (element.hasType()) { 22064 composeCodeableConcept("type", element.getType()); 22065 } 22066 if (element.hasTelecom()) { 22067 openArray("telecom"); 22068 for (ContactPoint e : element.getTelecom()) 22069 composeContactPoint(null, e); 22070 closeArray(); 22071 } 22072 ; 22073 if (element.hasAddress()) { 22074 composeAddress("address", element.getAddress()); 22075 } 22076 if (element.hasPhysicalType()) { 22077 composeCodeableConcept("physicalType", element.getPhysicalType()); 22078 } 22079 if (element.hasPosition()) { 22080 composeLocationLocationPositionComponent("position", element.getPosition()); 22081 } 22082 if (element.hasManagingOrganization()) { 22083 composeReference("managingOrganization", element.getManagingOrganization()); 22084 } 22085 if (element.hasPartOf()) { 22086 composeReference("partOf", element.getPartOf()); 22087 } 22088 } 22089 22090 protected void composeLocationLocationPositionComponent(String name, Location.LocationPositionComponent element) 22091 throws IOException { 22092 if (element != null) { 22093 open(name); 22094 composeLocationLocationPositionComponentInner(element); 22095 close(); 22096 } 22097 } 22098 22099 protected void composeLocationLocationPositionComponentInner(Location.LocationPositionComponent element) 22100 throws IOException { 22101 composeBackbone(element); 22102 if (element.hasLongitudeElement()) { 22103 composeDecimalCore("longitude", element.getLongitudeElement(), false); 22104 composeDecimalExtras("longitude", element.getLongitudeElement(), false); 22105 } 22106 if (element.hasLatitudeElement()) { 22107 composeDecimalCore("latitude", element.getLatitudeElement(), false); 22108 composeDecimalExtras("latitude", element.getLatitudeElement(), false); 22109 } 22110 if (element.hasAltitudeElement()) { 22111 composeDecimalCore("altitude", element.getAltitudeElement(), false); 22112 composeDecimalExtras("altitude", element.getAltitudeElement(), false); 22113 } 22114 } 22115 22116 protected void composeMedia(String name, Media element) throws IOException { 22117 if (element != null) { 22118 prop("resourceType", name); 22119 composeMediaInner(element); 22120 } 22121 } 22122 22123 protected void composeMediaInner(Media element) throws IOException { 22124 composeDomainResourceElements(element); 22125 if (element.hasTypeElement()) { 22126 composeEnumerationCore("type", element.getTypeElement(), new Media.DigitalMediaTypeEnumFactory(), false); 22127 composeEnumerationExtras("type", element.getTypeElement(), new Media.DigitalMediaTypeEnumFactory(), false); 22128 } 22129 if (element.hasSubtype()) { 22130 composeCodeableConcept("subtype", element.getSubtype()); 22131 } 22132 if (element.hasIdentifier()) { 22133 openArray("identifier"); 22134 for (Identifier e : element.getIdentifier()) 22135 composeIdentifier(null, e); 22136 closeArray(); 22137 } 22138 ; 22139 if (element.hasSubject()) { 22140 composeReference("subject", element.getSubject()); 22141 } 22142 if (element.hasOperator()) { 22143 composeReference("operator", element.getOperator()); 22144 } 22145 if (element.hasView()) { 22146 composeCodeableConcept("view", element.getView()); 22147 } 22148 if (element.hasDeviceNameElement()) { 22149 composeStringCore("deviceName", element.getDeviceNameElement(), false); 22150 composeStringExtras("deviceName", element.getDeviceNameElement(), false); 22151 } 22152 if (element.hasHeightElement()) { 22153 composePositiveIntCore("height", element.getHeightElement(), false); 22154 composePositiveIntExtras("height", element.getHeightElement(), false); 22155 } 22156 if (element.hasWidthElement()) { 22157 composePositiveIntCore("width", element.getWidthElement(), false); 22158 composePositiveIntExtras("width", element.getWidthElement(), false); 22159 } 22160 if (element.hasFramesElement()) { 22161 composePositiveIntCore("frames", element.getFramesElement(), false); 22162 composePositiveIntExtras("frames", element.getFramesElement(), false); 22163 } 22164 if (element.hasDurationElement()) { 22165 composeUnsignedIntCore("duration", element.getDurationElement(), false); 22166 composeUnsignedIntExtras("duration", element.getDurationElement(), false); 22167 } 22168 if (element.hasContent()) { 22169 composeAttachment("content", element.getContent()); 22170 } 22171 } 22172 22173 protected void composeMedication(String name, Medication element) throws IOException { 22174 if (element != null) { 22175 prop("resourceType", name); 22176 composeMedicationInner(element); 22177 } 22178 } 22179 22180 protected void composeMedicationInner(Medication element) throws IOException { 22181 composeDomainResourceElements(element); 22182 if (element.hasCode()) { 22183 composeCodeableConcept("code", element.getCode()); 22184 } 22185 if (element.hasIsBrandElement()) { 22186 composeBooleanCore("isBrand", element.getIsBrandElement(), false); 22187 composeBooleanExtras("isBrand", element.getIsBrandElement(), false); 22188 } 22189 if (element.hasManufacturer()) { 22190 composeReference("manufacturer", element.getManufacturer()); 22191 } 22192 if (element.hasProduct()) { 22193 composeMedicationMedicationProductComponent("product", element.getProduct()); 22194 } 22195 if (element.hasPackage()) { 22196 composeMedicationMedicationPackageComponent("package", element.getPackage()); 22197 } 22198 } 22199 22200 protected void composeMedicationMedicationProductComponent(String name, Medication.MedicationProductComponent element) 22201 throws IOException { 22202 if (element != null) { 22203 open(name); 22204 composeMedicationMedicationProductComponentInner(element); 22205 close(); 22206 } 22207 } 22208 22209 protected void composeMedicationMedicationProductComponentInner(Medication.MedicationProductComponent element) 22210 throws IOException { 22211 composeBackbone(element); 22212 if (element.hasForm()) { 22213 composeCodeableConcept("form", element.getForm()); 22214 } 22215 if (element.hasIngredient()) { 22216 openArray("ingredient"); 22217 for (Medication.MedicationProductIngredientComponent e : element.getIngredient()) 22218 composeMedicationMedicationProductIngredientComponent(null, e); 22219 closeArray(); 22220 } 22221 ; 22222 if (element.hasBatch()) { 22223 openArray("batch"); 22224 for (Medication.MedicationProductBatchComponent e : element.getBatch()) 22225 composeMedicationMedicationProductBatchComponent(null, e); 22226 closeArray(); 22227 } 22228 ; 22229 } 22230 22231 protected void composeMedicationMedicationProductIngredientComponent(String name, 22232 Medication.MedicationProductIngredientComponent element) throws IOException { 22233 if (element != null) { 22234 open(name); 22235 composeMedicationMedicationProductIngredientComponentInner(element); 22236 close(); 22237 } 22238 } 22239 22240 protected void composeMedicationMedicationProductIngredientComponentInner( 22241 Medication.MedicationProductIngredientComponent element) throws IOException { 22242 composeBackbone(element); 22243 if (element.hasItem()) { 22244 composeReference("item", element.getItem()); 22245 } 22246 if (element.hasAmount()) { 22247 composeRatio("amount", element.getAmount()); 22248 } 22249 } 22250 22251 protected void composeMedicationMedicationProductBatchComponent(String name, 22252 Medication.MedicationProductBatchComponent element) throws IOException { 22253 if (element != null) { 22254 open(name); 22255 composeMedicationMedicationProductBatchComponentInner(element); 22256 close(); 22257 } 22258 } 22259 22260 protected void composeMedicationMedicationProductBatchComponentInner( 22261 Medication.MedicationProductBatchComponent element) throws IOException { 22262 composeBackbone(element); 22263 if (element.hasLotNumberElement()) { 22264 composeStringCore("lotNumber", element.getLotNumberElement(), false); 22265 composeStringExtras("lotNumber", element.getLotNumberElement(), false); 22266 } 22267 if (element.hasExpirationDateElement()) { 22268 composeDateTimeCore("expirationDate", element.getExpirationDateElement(), false); 22269 composeDateTimeExtras("expirationDate", element.getExpirationDateElement(), false); 22270 } 22271 } 22272 22273 protected void composeMedicationMedicationPackageComponent(String name, Medication.MedicationPackageComponent element) 22274 throws IOException { 22275 if (element != null) { 22276 open(name); 22277 composeMedicationMedicationPackageComponentInner(element); 22278 close(); 22279 } 22280 } 22281 22282 protected void composeMedicationMedicationPackageComponentInner(Medication.MedicationPackageComponent element) 22283 throws IOException { 22284 composeBackbone(element); 22285 if (element.hasContainer()) { 22286 composeCodeableConcept("container", element.getContainer()); 22287 } 22288 if (element.hasContent()) { 22289 openArray("content"); 22290 for (Medication.MedicationPackageContentComponent e : element.getContent()) 22291 composeMedicationMedicationPackageContentComponent(null, e); 22292 closeArray(); 22293 } 22294 ; 22295 } 22296 22297 protected void composeMedicationMedicationPackageContentComponent(String name, 22298 Medication.MedicationPackageContentComponent element) throws IOException { 22299 if (element != null) { 22300 open(name); 22301 composeMedicationMedicationPackageContentComponentInner(element); 22302 close(); 22303 } 22304 } 22305 22306 protected void composeMedicationMedicationPackageContentComponentInner( 22307 Medication.MedicationPackageContentComponent element) throws IOException { 22308 composeBackbone(element); 22309 if (element.hasItem()) { 22310 composeReference("item", element.getItem()); 22311 } 22312 if (element.hasAmount()) { 22313 composeSimpleQuantity("amount", element.getAmount()); 22314 } 22315 } 22316 22317 protected void composeMedicationAdministration(String name, MedicationAdministration element) throws IOException { 22318 if (element != null) { 22319 prop("resourceType", name); 22320 composeMedicationAdministrationInner(element); 22321 } 22322 } 22323 22324 protected void composeMedicationAdministrationInner(MedicationAdministration element) throws IOException { 22325 composeDomainResourceElements(element); 22326 if (element.hasIdentifier()) { 22327 openArray("identifier"); 22328 for (Identifier e : element.getIdentifier()) 22329 composeIdentifier(null, e); 22330 closeArray(); 22331 } 22332 ; 22333 if (element.hasStatusElement()) { 22334 composeEnumerationCore("status", element.getStatusElement(), 22335 new MedicationAdministration.MedicationAdministrationStatusEnumFactory(), false); 22336 composeEnumerationExtras("status", element.getStatusElement(), 22337 new MedicationAdministration.MedicationAdministrationStatusEnumFactory(), false); 22338 } 22339 if (element.hasPatient()) { 22340 composeReference("patient", element.getPatient()); 22341 } 22342 if (element.hasPractitioner()) { 22343 composeReference("practitioner", element.getPractitioner()); 22344 } 22345 if (element.hasEncounter()) { 22346 composeReference("encounter", element.getEncounter()); 22347 } 22348 if (element.hasPrescription()) { 22349 composeReference("prescription", element.getPrescription()); 22350 } 22351 if (element.hasWasNotGivenElement()) { 22352 composeBooleanCore("wasNotGiven", element.getWasNotGivenElement(), false); 22353 composeBooleanExtras("wasNotGiven", element.getWasNotGivenElement(), false); 22354 } 22355 if (element.hasReasonNotGiven()) { 22356 openArray("reasonNotGiven"); 22357 for (CodeableConcept e : element.getReasonNotGiven()) 22358 composeCodeableConcept(null, e); 22359 closeArray(); 22360 } 22361 ; 22362 if (element.hasReasonGiven()) { 22363 openArray("reasonGiven"); 22364 for (CodeableConcept e : element.getReasonGiven()) 22365 composeCodeableConcept(null, e); 22366 closeArray(); 22367 } 22368 ; 22369 if (element.hasEffectiveTime()) { 22370 composeType("effectiveTime", element.getEffectiveTime()); 22371 } 22372 if (element.hasMedication()) { 22373 composeType("medication", element.getMedication()); 22374 } 22375 if (element.hasDevice()) { 22376 openArray("device"); 22377 for (Reference e : element.getDevice()) 22378 composeReference(null, e); 22379 closeArray(); 22380 } 22381 ; 22382 if (element.hasNoteElement()) { 22383 composeStringCore("note", element.getNoteElement(), false); 22384 composeStringExtras("note", element.getNoteElement(), false); 22385 } 22386 if (element.hasDosage()) { 22387 composeMedicationAdministrationMedicationAdministrationDosageComponent("dosage", element.getDosage()); 22388 } 22389 } 22390 22391 protected void composeMedicationAdministrationMedicationAdministrationDosageComponent(String name, 22392 MedicationAdministration.MedicationAdministrationDosageComponent element) throws IOException { 22393 if (element != null) { 22394 open(name); 22395 composeMedicationAdministrationMedicationAdministrationDosageComponentInner(element); 22396 close(); 22397 } 22398 } 22399 22400 protected void composeMedicationAdministrationMedicationAdministrationDosageComponentInner( 22401 MedicationAdministration.MedicationAdministrationDosageComponent element) throws IOException { 22402 composeBackbone(element); 22403 if (element.hasTextElement()) { 22404 composeStringCore("text", element.getTextElement(), false); 22405 composeStringExtras("text", element.getTextElement(), false); 22406 } 22407 if (element.hasSite()) { 22408 composeType("site", element.getSite()); 22409 } 22410 if (element.hasRoute()) { 22411 composeCodeableConcept("route", element.getRoute()); 22412 } 22413 if (element.hasMethod()) { 22414 composeCodeableConcept("method", element.getMethod()); 22415 } 22416 if (element.hasQuantity()) { 22417 composeSimpleQuantity("quantity", element.getQuantity()); 22418 } 22419 if (element.hasRate()) { 22420 composeType("rate", element.getRate()); 22421 } 22422 } 22423 22424 protected void composeMedicationDispense(String name, MedicationDispense element) throws IOException { 22425 if (element != null) { 22426 prop("resourceType", name); 22427 composeMedicationDispenseInner(element); 22428 } 22429 } 22430 22431 protected void composeMedicationDispenseInner(MedicationDispense element) throws IOException { 22432 composeDomainResourceElements(element); 22433 if (element.hasIdentifier()) { 22434 composeIdentifier("identifier", element.getIdentifier()); 22435 } 22436 if (element.hasStatusElement()) { 22437 composeEnumerationCore("status", element.getStatusElement(), 22438 new MedicationDispense.MedicationDispenseStatusEnumFactory(), false); 22439 composeEnumerationExtras("status", element.getStatusElement(), 22440 new MedicationDispense.MedicationDispenseStatusEnumFactory(), false); 22441 } 22442 if (element.hasPatient()) { 22443 composeReference("patient", element.getPatient()); 22444 } 22445 if (element.hasDispenser()) { 22446 composeReference("dispenser", element.getDispenser()); 22447 } 22448 if (element.hasAuthorizingPrescription()) { 22449 openArray("authorizingPrescription"); 22450 for (Reference e : element.getAuthorizingPrescription()) 22451 composeReference(null, e); 22452 closeArray(); 22453 } 22454 ; 22455 if (element.hasType()) { 22456 composeCodeableConcept("type", element.getType()); 22457 } 22458 if (element.hasQuantity()) { 22459 composeSimpleQuantity("quantity", element.getQuantity()); 22460 } 22461 if (element.hasDaysSupply()) { 22462 composeSimpleQuantity("daysSupply", element.getDaysSupply()); 22463 } 22464 if (element.hasMedication()) { 22465 composeType("medication", element.getMedication()); 22466 } 22467 if (element.hasWhenPreparedElement()) { 22468 composeDateTimeCore("whenPrepared", element.getWhenPreparedElement(), false); 22469 composeDateTimeExtras("whenPrepared", element.getWhenPreparedElement(), false); 22470 } 22471 if (element.hasWhenHandedOverElement()) { 22472 composeDateTimeCore("whenHandedOver", element.getWhenHandedOverElement(), false); 22473 composeDateTimeExtras("whenHandedOver", element.getWhenHandedOverElement(), false); 22474 } 22475 if (element.hasDestination()) { 22476 composeReference("destination", element.getDestination()); 22477 } 22478 if (element.hasReceiver()) { 22479 openArray("receiver"); 22480 for (Reference e : element.getReceiver()) 22481 composeReference(null, e); 22482 closeArray(); 22483 } 22484 ; 22485 if (element.hasNoteElement()) { 22486 composeStringCore("note", element.getNoteElement(), false); 22487 composeStringExtras("note", element.getNoteElement(), false); 22488 } 22489 if (element.hasDosageInstruction()) { 22490 openArray("dosageInstruction"); 22491 for (MedicationDispense.MedicationDispenseDosageInstructionComponent e : element.getDosageInstruction()) 22492 composeMedicationDispenseMedicationDispenseDosageInstructionComponent(null, e); 22493 closeArray(); 22494 } 22495 ; 22496 if (element.hasSubstitution()) { 22497 composeMedicationDispenseMedicationDispenseSubstitutionComponent("substitution", element.getSubstitution()); 22498 } 22499 } 22500 22501 protected void composeMedicationDispenseMedicationDispenseDosageInstructionComponent(String name, 22502 MedicationDispense.MedicationDispenseDosageInstructionComponent element) throws IOException { 22503 if (element != null) { 22504 open(name); 22505 composeMedicationDispenseMedicationDispenseDosageInstructionComponentInner(element); 22506 close(); 22507 } 22508 } 22509 22510 protected void composeMedicationDispenseMedicationDispenseDosageInstructionComponentInner( 22511 MedicationDispense.MedicationDispenseDosageInstructionComponent element) throws IOException { 22512 composeBackbone(element); 22513 if (element.hasTextElement()) { 22514 composeStringCore("text", element.getTextElement(), false); 22515 composeStringExtras("text", element.getTextElement(), false); 22516 } 22517 if (element.hasAdditionalInstructions()) { 22518 composeCodeableConcept("additionalInstructions", element.getAdditionalInstructions()); 22519 } 22520 if (element.hasTiming()) { 22521 composeTiming("timing", element.getTiming()); 22522 } 22523 if (element.hasAsNeeded()) { 22524 composeType("asNeeded", element.getAsNeeded()); 22525 } 22526 if (element.hasSite()) { 22527 composeType("site", element.getSite()); 22528 } 22529 if (element.hasRoute()) { 22530 composeCodeableConcept("route", element.getRoute()); 22531 } 22532 if (element.hasMethod()) { 22533 composeCodeableConcept("method", element.getMethod()); 22534 } 22535 if (element.hasDose()) { 22536 composeType("dose", element.getDose()); 22537 } 22538 if (element.hasRate()) { 22539 composeType("rate", element.getRate()); 22540 } 22541 if (element.hasMaxDosePerPeriod()) { 22542 composeRatio("maxDosePerPeriod", element.getMaxDosePerPeriod()); 22543 } 22544 } 22545 22546 protected void composeMedicationDispenseMedicationDispenseSubstitutionComponent(String name, 22547 MedicationDispense.MedicationDispenseSubstitutionComponent element) throws IOException { 22548 if (element != null) { 22549 open(name); 22550 composeMedicationDispenseMedicationDispenseSubstitutionComponentInner(element); 22551 close(); 22552 } 22553 } 22554 22555 protected void composeMedicationDispenseMedicationDispenseSubstitutionComponentInner( 22556 MedicationDispense.MedicationDispenseSubstitutionComponent element) throws IOException { 22557 composeBackbone(element); 22558 if (element.hasType()) { 22559 composeCodeableConcept("type", element.getType()); 22560 } 22561 if (element.hasReason()) { 22562 openArray("reason"); 22563 for (CodeableConcept e : element.getReason()) 22564 composeCodeableConcept(null, e); 22565 closeArray(); 22566 } 22567 ; 22568 if (element.hasResponsibleParty()) { 22569 openArray("responsibleParty"); 22570 for (Reference e : element.getResponsibleParty()) 22571 composeReference(null, e); 22572 closeArray(); 22573 } 22574 ; 22575 } 22576 22577 protected void composeMedicationOrder(String name, MedicationOrder element) throws IOException { 22578 if (element != null) { 22579 prop("resourceType", name); 22580 composeMedicationOrderInner(element); 22581 } 22582 } 22583 22584 protected void composeMedicationOrderInner(MedicationOrder element) throws IOException { 22585 composeDomainResourceElements(element); 22586 if (element.hasIdentifier()) { 22587 openArray("identifier"); 22588 for (Identifier e : element.getIdentifier()) 22589 composeIdentifier(null, e); 22590 closeArray(); 22591 } 22592 ; 22593 if (element.hasDateWrittenElement()) { 22594 composeDateTimeCore("dateWritten", element.getDateWrittenElement(), false); 22595 composeDateTimeExtras("dateWritten", element.getDateWrittenElement(), false); 22596 } 22597 if (element.hasStatusElement()) { 22598 composeEnumerationCore("status", element.getStatusElement(), 22599 new MedicationOrder.MedicationOrderStatusEnumFactory(), false); 22600 composeEnumerationExtras("status", element.getStatusElement(), 22601 new MedicationOrder.MedicationOrderStatusEnumFactory(), false); 22602 } 22603 if (element.hasDateEndedElement()) { 22604 composeDateTimeCore("dateEnded", element.getDateEndedElement(), false); 22605 composeDateTimeExtras("dateEnded", element.getDateEndedElement(), false); 22606 } 22607 if (element.hasReasonEnded()) { 22608 composeCodeableConcept("reasonEnded", element.getReasonEnded()); 22609 } 22610 if (element.hasPatient()) { 22611 composeReference("patient", element.getPatient()); 22612 } 22613 if (element.hasPrescriber()) { 22614 composeReference("prescriber", element.getPrescriber()); 22615 } 22616 if (element.hasEncounter()) { 22617 composeReference("encounter", element.getEncounter()); 22618 } 22619 if (element.hasReason()) { 22620 composeType("reason", element.getReason()); 22621 } 22622 if (element.hasNoteElement()) { 22623 composeStringCore("note", element.getNoteElement(), false); 22624 composeStringExtras("note", element.getNoteElement(), false); 22625 } 22626 if (element.hasMedication()) { 22627 composeType("medication", element.getMedication()); 22628 } 22629 if (element.hasDosageInstruction()) { 22630 openArray("dosageInstruction"); 22631 for (MedicationOrder.MedicationOrderDosageInstructionComponent e : element.getDosageInstruction()) 22632 composeMedicationOrderMedicationOrderDosageInstructionComponent(null, e); 22633 closeArray(); 22634 } 22635 ; 22636 if (element.hasDispenseRequest()) { 22637 composeMedicationOrderMedicationOrderDispenseRequestComponent("dispenseRequest", element.getDispenseRequest()); 22638 } 22639 if (element.hasSubstitution()) { 22640 composeMedicationOrderMedicationOrderSubstitutionComponent("substitution", element.getSubstitution()); 22641 } 22642 if (element.hasPriorPrescription()) { 22643 composeReference("priorPrescription", element.getPriorPrescription()); 22644 } 22645 } 22646 22647 protected void composeMedicationOrderMedicationOrderDosageInstructionComponent(String name, 22648 MedicationOrder.MedicationOrderDosageInstructionComponent element) throws IOException { 22649 if (element != null) { 22650 open(name); 22651 composeMedicationOrderMedicationOrderDosageInstructionComponentInner(element); 22652 close(); 22653 } 22654 } 22655 22656 protected void composeMedicationOrderMedicationOrderDosageInstructionComponentInner( 22657 MedicationOrder.MedicationOrderDosageInstructionComponent element) throws IOException { 22658 composeBackbone(element); 22659 if (element.hasTextElement()) { 22660 composeStringCore("text", element.getTextElement(), false); 22661 composeStringExtras("text", element.getTextElement(), false); 22662 } 22663 if (element.hasAdditionalInstructions()) { 22664 composeCodeableConcept("additionalInstructions", element.getAdditionalInstructions()); 22665 } 22666 if (element.hasTiming()) { 22667 composeTiming("timing", element.getTiming()); 22668 } 22669 if (element.hasAsNeeded()) { 22670 composeType("asNeeded", element.getAsNeeded()); 22671 } 22672 if (element.hasSite()) { 22673 composeType("site", element.getSite()); 22674 } 22675 if (element.hasRoute()) { 22676 composeCodeableConcept("route", element.getRoute()); 22677 } 22678 if (element.hasMethod()) { 22679 composeCodeableConcept("method", element.getMethod()); 22680 } 22681 if (element.hasDose()) { 22682 composeType("dose", element.getDose()); 22683 } 22684 if (element.hasRate()) { 22685 composeType("rate", element.getRate()); 22686 } 22687 if (element.hasMaxDosePerPeriod()) { 22688 composeRatio("maxDosePerPeriod", element.getMaxDosePerPeriod()); 22689 } 22690 } 22691 22692 protected void composeMedicationOrderMedicationOrderDispenseRequestComponent(String name, 22693 MedicationOrder.MedicationOrderDispenseRequestComponent element) throws IOException { 22694 if (element != null) { 22695 open(name); 22696 composeMedicationOrderMedicationOrderDispenseRequestComponentInner(element); 22697 close(); 22698 } 22699 } 22700 22701 protected void composeMedicationOrderMedicationOrderDispenseRequestComponentInner( 22702 MedicationOrder.MedicationOrderDispenseRequestComponent element) throws IOException { 22703 composeBackbone(element); 22704 if (element.hasMedication()) { 22705 composeType("medication", element.getMedication()); 22706 } 22707 if (element.hasValidityPeriod()) { 22708 composePeriod("validityPeriod", element.getValidityPeriod()); 22709 } 22710 if (element.hasNumberOfRepeatsAllowedElement()) { 22711 composePositiveIntCore("numberOfRepeatsAllowed", element.getNumberOfRepeatsAllowedElement(), false); 22712 composePositiveIntExtras("numberOfRepeatsAllowed", element.getNumberOfRepeatsAllowedElement(), false); 22713 } 22714 if (element.hasQuantity()) { 22715 composeSimpleQuantity("quantity", element.getQuantity()); 22716 } 22717 if (element.hasExpectedSupplyDuration()) { 22718 composeDuration("expectedSupplyDuration", element.getExpectedSupplyDuration()); 22719 } 22720 } 22721 22722 protected void composeMedicationOrderMedicationOrderSubstitutionComponent(String name, 22723 MedicationOrder.MedicationOrderSubstitutionComponent element) throws IOException { 22724 if (element != null) { 22725 open(name); 22726 composeMedicationOrderMedicationOrderSubstitutionComponentInner(element); 22727 close(); 22728 } 22729 } 22730 22731 protected void composeMedicationOrderMedicationOrderSubstitutionComponentInner( 22732 MedicationOrder.MedicationOrderSubstitutionComponent element) throws IOException { 22733 composeBackbone(element); 22734 if (element.hasType()) { 22735 composeCodeableConcept("type", element.getType()); 22736 } 22737 if (element.hasReason()) { 22738 composeCodeableConcept("reason", element.getReason()); 22739 } 22740 } 22741 22742 protected void composeMedicationStatement(String name, MedicationStatement element) throws IOException { 22743 if (element != null) { 22744 prop("resourceType", name); 22745 composeMedicationStatementInner(element); 22746 } 22747 } 22748 22749 protected void composeMedicationStatementInner(MedicationStatement element) throws IOException { 22750 composeDomainResourceElements(element); 22751 if (element.hasIdentifier()) { 22752 openArray("identifier"); 22753 for (Identifier e : element.getIdentifier()) 22754 composeIdentifier(null, e); 22755 closeArray(); 22756 } 22757 ; 22758 if (element.hasPatient()) { 22759 composeReference("patient", element.getPatient()); 22760 } 22761 if (element.hasInformationSource()) { 22762 composeReference("informationSource", element.getInformationSource()); 22763 } 22764 if (element.hasDateAssertedElement()) { 22765 composeDateTimeCore("dateAsserted", element.getDateAssertedElement(), false); 22766 composeDateTimeExtras("dateAsserted", element.getDateAssertedElement(), false); 22767 } 22768 if (element.hasStatusElement()) { 22769 composeEnumerationCore("status", element.getStatusElement(), 22770 new MedicationStatement.MedicationStatementStatusEnumFactory(), false); 22771 composeEnumerationExtras("status", element.getStatusElement(), 22772 new MedicationStatement.MedicationStatementStatusEnumFactory(), false); 22773 } 22774 if (element.hasWasNotTakenElement()) { 22775 composeBooleanCore("wasNotTaken", element.getWasNotTakenElement(), false); 22776 composeBooleanExtras("wasNotTaken", element.getWasNotTakenElement(), false); 22777 } 22778 if (element.hasReasonNotTaken()) { 22779 openArray("reasonNotTaken"); 22780 for (CodeableConcept e : element.getReasonNotTaken()) 22781 composeCodeableConcept(null, e); 22782 closeArray(); 22783 } 22784 ; 22785 if (element.hasReasonForUse()) { 22786 composeType("reasonForUse", element.getReasonForUse()); 22787 } 22788 if (element.hasEffective()) { 22789 composeType("effective", element.getEffective()); 22790 } 22791 if (element.hasNoteElement()) { 22792 composeStringCore("note", element.getNoteElement(), false); 22793 composeStringExtras("note", element.getNoteElement(), false); 22794 } 22795 if (element.hasSupportingInformation()) { 22796 openArray("supportingInformation"); 22797 for (Reference e : element.getSupportingInformation()) 22798 composeReference(null, e); 22799 closeArray(); 22800 } 22801 ; 22802 if (element.hasMedication()) { 22803 composeType("medication", element.getMedication()); 22804 } 22805 if (element.hasDosage()) { 22806 openArray("dosage"); 22807 for (MedicationStatement.MedicationStatementDosageComponent e : element.getDosage()) 22808 composeMedicationStatementMedicationStatementDosageComponent(null, e); 22809 closeArray(); 22810 } 22811 ; 22812 } 22813 22814 protected void composeMedicationStatementMedicationStatementDosageComponent(String name, 22815 MedicationStatement.MedicationStatementDosageComponent element) throws IOException { 22816 if (element != null) { 22817 open(name); 22818 composeMedicationStatementMedicationStatementDosageComponentInner(element); 22819 close(); 22820 } 22821 } 22822 22823 protected void composeMedicationStatementMedicationStatementDosageComponentInner( 22824 MedicationStatement.MedicationStatementDosageComponent element) throws IOException { 22825 composeBackbone(element); 22826 if (element.hasTextElement()) { 22827 composeStringCore("text", element.getTextElement(), false); 22828 composeStringExtras("text", element.getTextElement(), false); 22829 } 22830 if (element.hasTiming()) { 22831 composeTiming("timing", element.getTiming()); 22832 } 22833 if (element.hasAsNeeded()) { 22834 composeType("asNeeded", element.getAsNeeded()); 22835 } 22836 if (element.hasSite()) { 22837 composeType("site", element.getSite()); 22838 } 22839 if (element.hasRoute()) { 22840 composeCodeableConcept("route", element.getRoute()); 22841 } 22842 if (element.hasMethod()) { 22843 composeCodeableConcept("method", element.getMethod()); 22844 } 22845 if (element.hasQuantity()) { 22846 composeType("quantity", element.getQuantity()); 22847 } 22848 if (element.hasRate()) { 22849 composeType("rate", element.getRate()); 22850 } 22851 if (element.hasMaxDosePerPeriod()) { 22852 composeRatio("maxDosePerPeriod", element.getMaxDosePerPeriod()); 22853 } 22854 } 22855 22856 protected void composeMessageHeader(String name, MessageHeader element) throws IOException { 22857 if (element != null) { 22858 prop("resourceType", name); 22859 composeMessageHeaderInner(element); 22860 } 22861 } 22862 22863 protected void composeMessageHeaderInner(MessageHeader element) throws IOException { 22864 composeDomainResourceElements(element); 22865 if (element.hasTimestampElement()) { 22866 composeInstantCore("timestamp", element.getTimestampElement(), false); 22867 composeInstantExtras("timestamp", element.getTimestampElement(), false); 22868 } 22869 if (element.hasEvent()) { 22870 composeCoding("event", element.getEvent()); 22871 } 22872 if (element.hasResponse()) { 22873 composeMessageHeaderMessageHeaderResponseComponent("response", element.getResponse()); 22874 } 22875 if (element.hasSource()) { 22876 composeMessageHeaderMessageSourceComponent("source", element.getSource()); 22877 } 22878 if (element.hasDestination()) { 22879 openArray("destination"); 22880 for (MessageHeader.MessageDestinationComponent e : element.getDestination()) 22881 composeMessageHeaderMessageDestinationComponent(null, e); 22882 closeArray(); 22883 } 22884 ; 22885 if (element.hasEnterer()) { 22886 composeReference("enterer", element.getEnterer()); 22887 } 22888 if (element.hasAuthor()) { 22889 composeReference("author", element.getAuthor()); 22890 } 22891 if (element.hasReceiver()) { 22892 composeReference("receiver", element.getReceiver()); 22893 } 22894 if (element.hasResponsible()) { 22895 composeReference("responsible", element.getResponsible()); 22896 } 22897 if (element.hasReason()) { 22898 composeCodeableConcept("reason", element.getReason()); 22899 } 22900 if (element.hasData()) { 22901 openArray("data"); 22902 for (Reference e : element.getData()) 22903 composeReference(null, e); 22904 closeArray(); 22905 } 22906 ; 22907 } 22908 22909 protected void composeMessageHeaderMessageHeaderResponseComponent(String name, 22910 MessageHeader.MessageHeaderResponseComponent element) throws IOException { 22911 if (element != null) { 22912 open(name); 22913 composeMessageHeaderMessageHeaderResponseComponentInner(element); 22914 close(); 22915 } 22916 } 22917 22918 protected void composeMessageHeaderMessageHeaderResponseComponentInner( 22919 MessageHeader.MessageHeaderResponseComponent element) throws IOException { 22920 composeBackbone(element); 22921 if (element.hasIdentifierElement()) { 22922 composeIdCore("identifier", element.getIdentifierElement(), false); 22923 composeIdExtras("identifier", element.getIdentifierElement(), false); 22924 } 22925 if (element.hasCodeElement()) { 22926 composeEnumerationCore("code", element.getCodeElement(), new MessageHeader.ResponseTypeEnumFactory(), false); 22927 composeEnumerationExtras("code", element.getCodeElement(), new MessageHeader.ResponseTypeEnumFactory(), false); 22928 } 22929 if (element.hasDetails()) { 22930 composeReference("details", element.getDetails()); 22931 } 22932 } 22933 22934 protected void composeMessageHeaderMessageSourceComponent(String name, MessageHeader.MessageSourceComponent element) 22935 throws IOException { 22936 if (element != null) { 22937 open(name); 22938 composeMessageHeaderMessageSourceComponentInner(element); 22939 close(); 22940 } 22941 } 22942 22943 protected void composeMessageHeaderMessageSourceComponentInner(MessageHeader.MessageSourceComponent element) 22944 throws IOException { 22945 composeBackbone(element); 22946 if (element.hasNameElement()) { 22947 composeStringCore("name", element.getNameElement(), false); 22948 composeStringExtras("name", element.getNameElement(), false); 22949 } 22950 if (element.hasSoftwareElement()) { 22951 composeStringCore("software", element.getSoftwareElement(), false); 22952 composeStringExtras("software", element.getSoftwareElement(), false); 22953 } 22954 if (element.hasVersionElement()) { 22955 composeStringCore("version", element.getVersionElement(), false); 22956 composeStringExtras("version", element.getVersionElement(), false); 22957 } 22958 if (element.hasContact()) { 22959 composeContactPoint("contact", element.getContact()); 22960 } 22961 if (element.hasEndpointElement()) { 22962 composeUriCore("endpoint", element.getEndpointElement(), false); 22963 composeUriExtras("endpoint", element.getEndpointElement(), false); 22964 } 22965 } 22966 22967 protected void composeMessageHeaderMessageDestinationComponent(String name, 22968 MessageHeader.MessageDestinationComponent element) throws IOException { 22969 if (element != null) { 22970 open(name); 22971 composeMessageHeaderMessageDestinationComponentInner(element); 22972 close(); 22973 } 22974 } 22975 22976 protected void composeMessageHeaderMessageDestinationComponentInner(MessageHeader.MessageDestinationComponent element) 22977 throws IOException { 22978 composeBackbone(element); 22979 if (element.hasNameElement()) { 22980 composeStringCore("name", element.getNameElement(), false); 22981 composeStringExtras("name", element.getNameElement(), false); 22982 } 22983 if (element.hasTarget()) { 22984 composeReference("target", element.getTarget()); 22985 } 22986 if (element.hasEndpointElement()) { 22987 composeUriCore("endpoint", element.getEndpointElement(), false); 22988 composeUriExtras("endpoint", element.getEndpointElement(), false); 22989 } 22990 } 22991 22992 protected void composeNamingSystem(String name, NamingSystem element) throws IOException { 22993 if (element != null) { 22994 prop("resourceType", name); 22995 composeNamingSystemInner(element); 22996 } 22997 } 22998 22999 protected void composeNamingSystemInner(NamingSystem element) throws IOException { 23000 composeDomainResourceElements(element); 23001 if (element.hasNameElement()) { 23002 composeStringCore("name", element.getNameElement(), false); 23003 composeStringExtras("name", element.getNameElement(), false); 23004 } 23005 if (element.hasStatusElement()) { 23006 composeEnumerationCore("status", element.getStatusElement(), 23007 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 23008 composeEnumerationExtras("status", element.getStatusElement(), 23009 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 23010 } 23011 if (element.hasKindElement()) { 23012 composeEnumerationCore("kind", element.getKindElement(), new NamingSystem.NamingSystemTypeEnumFactory(), false); 23013 composeEnumerationExtras("kind", element.getKindElement(), new NamingSystem.NamingSystemTypeEnumFactory(), false); 23014 } 23015 if (element.hasPublisherElement()) { 23016 composeStringCore("publisher", element.getPublisherElement(), false); 23017 composeStringExtras("publisher", element.getPublisherElement(), false); 23018 } 23019 if (element.hasContact()) { 23020 openArray("contact"); 23021 for (NamingSystem.NamingSystemContactComponent e : element.getContact()) 23022 composeNamingSystemNamingSystemContactComponent(null, e); 23023 closeArray(); 23024 } 23025 ; 23026 if (element.hasResponsibleElement()) { 23027 composeStringCore("responsible", element.getResponsibleElement(), false); 23028 composeStringExtras("responsible", element.getResponsibleElement(), false); 23029 } 23030 if (element.hasDateElement()) { 23031 composeDateTimeCore("date", element.getDateElement(), false); 23032 composeDateTimeExtras("date", element.getDateElement(), false); 23033 } 23034 if (element.hasType()) { 23035 composeCodeableConcept("type", element.getType()); 23036 } 23037 if (element.hasDescriptionElement()) { 23038 composeStringCore("description", element.getDescriptionElement(), false); 23039 composeStringExtras("description", element.getDescriptionElement(), false); 23040 } 23041 if (element.hasUseContext()) { 23042 openArray("useContext"); 23043 for (CodeableConcept e : element.getUseContext()) 23044 composeCodeableConcept(null, e); 23045 closeArray(); 23046 } 23047 ; 23048 if (element.hasUsageElement()) { 23049 composeStringCore("usage", element.getUsageElement(), false); 23050 composeStringExtras("usage", element.getUsageElement(), false); 23051 } 23052 if (element.hasUniqueId()) { 23053 openArray("uniqueId"); 23054 for (NamingSystem.NamingSystemUniqueIdComponent e : element.getUniqueId()) 23055 composeNamingSystemNamingSystemUniqueIdComponent(null, e); 23056 closeArray(); 23057 } 23058 ; 23059 if (element.hasReplacedBy()) { 23060 composeReference("replacedBy", element.getReplacedBy()); 23061 } 23062 } 23063 23064 protected void composeNamingSystemNamingSystemContactComponent(String name, 23065 NamingSystem.NamingSystemContactComponent element) throws IOException { 23066 if (element != null) { 23067 open(name); 23068 composeNamingSystemNamingSystemContactComponentInner(element); 23069 close(); 23070 } 23071 } 23072 23073 protected void composeNamingSystemNamingSystemContactComponentInner(NamingSystem.NamingSystemContactComponent element) 23074 throws IOException { 23075 composeBackbone(element); 23076 if (element.hasNameElement()) { 23077 composeStringCore("name", element.getNameElement(), false); 23078 composeStringExtras("name", element.getNameElement(), false); 23079 } 23080 if (element.hasTelecom()) { 23081 openArray("telecom"); 23082 for (ContactPoint e : element.getTelecom()) 23083 composeContactPoint(null, e); 23084 closeArray(); 23085 } 23086 ; 23087 } 23088 23089 protected void composeNamingSystemNamingSystemUniqueIdComponent(String name, 23090 NamingSystem.NamingSystemUniqueIdComponent element) throws IOException { 23091 if (element != null) { 23092 open(name); 23093 composeNamingSystemNamingSystemUniqueIdComponentInner(element); 23094 close(); 23095 } 23096 } 23097 23098 protected void composeNamingSystemNamingSystemUniqueIdComponentInner( 23099 NamingSystem.NamingSystemUniqueIdComponent element) throws IOException { 23100 composeBackbone(element); 23101 if (element.hasTypeElement()) { 23102 composeEnumerationCore("type", element.getTypeElement(), new NamingSystem.NamingSystemIdentifierTypeEnumFactory(), 23103 false); 23104 composeEnumerationExtras("type", element.getTypeElement(), 23105 new NamingSystem.NamingSystemIdentifierTypeEnumFactory(), false); 23106 } 23107 if (element.hasValueElement()) { 23108 composeStringCore("value", element.getValueElement(), false); 23109 composeStringExtras("value", element.getValueElement(), false); 23110 } 23111 if (element.hasPreferredElement()) { 23112 composeBooleanCore("preferred", element.getPreferredElement(), false); 23113 composeBooleanExtras("preferred", element.getPreferredElement(), false); 23114 } 23115 if (element.hasPeriod()) { 23116 composePeriod("period", element.getPeriod()); 23117 } 23118 } 23119 23120 protected void composeNutritionOrder(String name, NutritionOrder element) throws IOException { 23121 if (element != null) { 23122 prop("resourceType", name); 23123 composeNutritionOrderInner(element); 23124 } 23125 } 23126 23127 protected void composeNutritionOrderInner(NutritionOrder element) throws IOException { 23128 composeDomainResourceElements(element); 23129 if (element.hasPatient()) { 23130 composeReference("patient", element.getPatient()); 23131 } 23132 if (element.hasOrderer()) { 23133 composeReference("orderer", element.getOrderer()); 23134 } 23135 if (element.hasIdentifier()) { 23136 openArray("identifier"); 23137 for (Identifier e : element.getIdentifier()) 23138 composeIdentifier(null, e); 23139 closeArray(); 23140 } 23141 ; 23142 if (element.hasEncounter()) { 23143 composeReference("encounter", element.getEncounter()); 23144 } 23145 if (element.hasDateTimeElement()) { 23146 composeDateTimeCore("dateTime", element.getDateTimeElement(), false); 23147 composeDateTimeExtras("dateTime", element.getDateTimeElement(), false); 23148 } 23149 if (element.hasStatusElement()) { 23150 composeEnumerationCore("status", element.getStatusElement(), new NutritionOrder.NutritionOrderStatusEnumFactory(), 23151 false); 23152 composeEnumerationExtras("status", element.getStatusElement(), 23153 new NutritionOrder.NutritionOrderStatusEnumFactory(), false); 23154 } 23155 if (element.hasAllergyIntolerance()) { 23156 openArray("allergyIntolerance"); 23157 for (Reference e : element.getAllergyIntolerance()) 23158 composeReference(null, e); 23159 closeArray(); 23160 } 23161 ; 23162 if (element.hasFoodPreferenceModifier()) { 23163 openArray("foodPreferenceModifier"); 23164 for (CodeableConcept e : element.getFoodPreferenceModifier()) 23165 composeCodeableConcept(null, e); 23166 closeArray(); 23167 } 23168 ; 23169 if (element.hasExcludeFoodModifier()) { 23170 openArray("excludeFoodModifier"); 23171 for (CodeableConcept e : element.getExcludeFoodModifier()) 23172 composeCodeableConcept(null, e); 23173 closeArray(); 23174 } 23175 ; 23176 if (element.hasOralDiet()) { 23177 composeNutritionOrderNutritionOrderOralDietComponent("oralDiet", element.getOralDiet()); 23178 } 23179 if (element.hasSupplement()) { 23180 openArray("supplement"); 23181 for (NutritionOrder.NutritionOrderSupplementComponent e : element.getSupplement()) 23182 composeNutritionOrderNutritionOrderSupplementComponent(null, e); 23183 closeArray(); 23184 } 23185 ; 23186 if (element.hasEnteralFormula()) { 23187 composeNutritionOrderNutritionOrderEnteralFormulaComponent("enteralFormula", element.getEnteralFormula()); 23188 } 23189 } 23190 23191 protected void composeNutritionOrderNutritionOrderOralDietComponent(String name, 23192 NutritionOrder.NutritionOrderOralDietComponent element) throws IOException { 23193 if (element != null) { 23194 open(name); 23195 composeNutritionOrderNutritionOrderOralDietComponentInner(element); 23196 close(); 23197 } 23198 } 23199 23200 protected void composeNutritionOrderNutritionOrderOralDietComponentInner( 23201 NutritionOrder.NutritionOrderOralDietComponent element) throws IOException { 23202 composeBackbone(element); 23203 if (element.hasType()) { 23204 openArray("type"); 23205 for (CodeableConcept e : element.getType()) 23206 composeCodeableConcept(null, e); 23207 closeArray(); 23208 } 23209 ; 23210 if (element.hasSchedule()) { 23211 openArray("schedule"); 23212 for (Timing e : element.getSchedule()) 23213 composeTiming(null, e); 23214 closeArray(); 23215 } 23216 ; 23217 if (element.hasNutrient()) { 23218 openArray("nutrient"); 23219 for (NutritionOrder.NutritionOrderOralDietNutrientComponent e : element.getNutrient()) 23220 composeNutritionOrderNutritionOrderOralDietNutrientComponent(null, e); 23221 closeArray(); 23222 } 23223 ; 23224 if (element.hasTexture()) { 23225 openArray("texture"); 23226 for (NutritionOrder.NutritionOrderOralDietTextureComponent e : element.getTexture()) 23227 composeNutritionOrderNutritionOrderOralDietTextureComponent(null, e); 23228 closeArray(); 23229 } 23230 ; 23231 if (element.hasFluidConsistencyType()) { 23232 openArray("fluidConsistencyType"); 23233 for (CodeableConcept e : element.getFluidConsistencyType()) 23234 composeCodeableConcept(null, e); 23235 closeArray(); 23236 } 23237 ; 23238 if (element.hasInstructionElement()) { 23239 composeStringCore("instruction", element.getInstructionElement(), false); 23240 composeStringExtras("instruction", element.getInstructionElement(), false); 23241 } 23242 } 23243 23244 protected void composeNutritionOrderNutritionOrderOralDietNutrientComponent(String name, 23245 NutritionOrder.NutritionOrderOralDietNutrientComponent element) throws IOException { 23246 if (element != null) { 23247 open(name); 23248 composeNutritionOrderNutritionOrderOralDietNutrientComponentInner(element); 23249 close(); 23250 } 23251 } 23252 23253 protected void composeNutritionOrderNutritionOrderOralDietNutrientComponentInner( 23254 NutritionOrder.NutritionOrderOralDietNutrientComponent element) throws IOException { 23255 composeBackbone(element); 23256 if (element.hasModifier()) { 23257 composeCodeableConcept("modifier", element.getModifier()); 23258 } 23259 if (element.hasAmount()) { 23260 composeSimpleQuantity("amount", element.getAmount()); 23261 } 23262 } 23263 23264 protected void composeNutritionOrderNutritionOrderOralDietTextureComponent(String name, 23265 NutritionOrder.NutritionOrderOralDietTextureComponent element) throws IOException { 23266 if (element != null) { 23267 open(name); 23268 composeNutritionOrderNutritionOrderOralDietTextureComponentInner(element); 23269 close(); 23270 } 23271 } 23272 23273 protected void composeNutritionOrderNutritionOrderOralDietTextureComponentInner( 23274 NutritionOrder.NutritionOrderOralDietTextureComponent element) throws IOException { 23275 composeBackbone(element); 23276 if (element.hasModifier()) { 23277 composeCodeableConcept("modifier", element.getModifier()); 23278 } 23279 if (element.hasFoodType()) { 23280 composeCodeableConcept("foodType", element.getFoodType()); 23281 } 23282 } 23283 23284 protected void composeNutritionOrderNutritionOrderSupplementComponent(String name, 23285 NutritionOrder.NutritionOrderSupplementComponent element) throws IOException { 23286 if (element != null) { 23287 open(name); 23288 composeNutritionOrderNutritionOrderSupplementComponentInner(element); 23289 close(); 23290 } 23291 } 23292 23293 protected void composeNutritionOrderNutritionOrderSupplementComponentInner( 23294 NutritionOrder.NutritionOrderSupplementComponent element) throws IOException { 23295 composeBackbone(element); 23296 if (element.hasType()) { 23297 composeCodeableConcept("type", element.getType()); 23298 } 23299 if (element.hasProductNameElement()) { 23300 composeStringCore("productName", element.getProductNameElement(), false); 23301 composeStringExtras("productName", element.getProductNameElement(), false); 23302 } 23303 if (element.hasSchedule()) { 23304 openArray("schedule"); 23305 for (Timing e : element.getSchedule()) 23306 composeTiming(null, e); 23307 closeArray(); 23308 } 23309 ; 23310 if (element.hasQuantity()) { 23311 composeSimpleQuantity("quantity", element.getQuantity()); 23312 } 23313 if (element.hasInstructionElement()) { 23314 composeStringCore("instruction", element.getInstructionElement(), false); 23315 composeStringExtras("instruction", element.getInstructionElement(), false); 23316 } 23317 } 23318 23319 protected void composeNutritionOrderNutritionOrderEnteralFormulaComponent(String name, 23320 NutritionOrder.NutritionOrderEnteralFormulaComponent element) throws IOException { 23321 if (element != null) { 23322 open(name); 23323 composeNutritionOrderNutritionOrderEnteralFormulaComponentInner(element); 23324 close(); 23325 } 23326 } 23327 23328 protected void composeNutritionOrderNutritionOrderEnteralFormulaComponentInner( 23329 NutritionOrder.NutritionOrderEnteralFormulaComponent element) throws IOException { 23330 composeBackbone(element); 23331 if (element.hasBaseFormulaType()) { 23332 composeCodeableConcept("baseFormulaType", element.getBaseFormulaType()); 23333 } 23334 if (element.hasBaseFormulaProductNameElement()) { 23335 composeStringCore("baseFormulaProductName", element.getBaseFormulaProductNameElement(), false); 23336 composeStringExtras("baseFormulaProductName", element.getBaseFormulaProductNameElement(), false); 23337 } 23338 if (element.hasAdditiveType()) { 23339 composeCodeableConcept("additiveType", element.getAdditiveType()); 23340 } 23341 if (element.hasAdditiveProductNameElement()) { 23342 composeStringCore("additiveProductName", element.getAdditiveProductNameElement(), false); 23343 composeStringExtras("additiveProductName", element.getAdditiveProductNameElement(), false); 23344 } 23345 if (element.hasCaloricDensity()) { 23346 composeSimpleQuantity("caloricDensity", element.getCaloricDensity()); 23347 } 23348 if (element.hasRouteofAdministration()) { 23349 composeCodeableConcept("routeofAdministration", element.getRouteofAdministration()); 23350 } 23351 if (element.hasAdministration()) { 23352 openArray("administration"); 23353 for (NutritionOrder.NutritionOrderEnteralFormulaAdministrationComponent e : element.getAdministration()) 23354 composeNutritionOrderNutritionOrderEnteralFormulaAdministrationComponent(null, e); 23355 closeArray(); 23356 } 23357 ; 23358 if (element.hasMaxVolumeToDeliver()) { 23359 composeSimpleQuantity("maxVolumeToDeliver", element.getMaxVolumeToDeliver()); 23360 } 23361 if (element.hasAdministrationInstructionElement()) { 23362 composeStringCore("administrationInstruction", element.getAdministrationInstructionElement(), false); 23363 composeStringExtras("administrationInstruction", element.getAdministrationInstructionElement(), false); 23364 } 23365 } 23366 23367 protected void composeNutritionOrderNutritionOrderEnteralFormulaAdministrationComponent(String name, 23368 NutritionOrder.NutritionOrderEnteralFormulaAdministrationComponent element) throws IOException { 23369 if (element != null) { 23370 open(name); 23371 composeNutritionOrderNutritionOrderEnteralFormulaAdministrationComponentInner(element); 23372 close(); 23373 } 23374 } 23375 23376 protected void composeNutritionOrderNutritionOrderEnteralFormulaAdministrationComponentInner( 23377 NutritionOrder.NutritionOrderEnteralFormulaAdministrationComponent element) throws IOException { 23378 composeBackbone(element); 23379 if (element.hasSchedule()) { 23380 composeTiming("schedule", element.getSchedule()); 23381 } 23382 if (element.hasQuantity()) { 23383 composeSimpleQuantity("quantity", element.getQuantity()); 23384 } 23385 if (element.hasRate()) { 23386 composeType("rate", element.getRate()); 23387 } 23388 } 23389 23390 protected void composeObservation(String name, Observation element) throws IOException { 23391 if (element != null) { 23392 prop("resourceType", name); 23393 composeObservationInner(element); 23394 } 23395 } 23396 23397 protected void composeObservationInner(Observation element) throws IOException { 23398 composeDomainResourceElements(element); 23399 if (element.hasIdentifier()) { 23400 openArray("identifier"); 23401 for (Identifier e : element.getIdentifier()) 23402 composeIdentifier(null, e); 23403 closeArray(); 23404 } 23405 ; 23406 if (element.hasStatusElement()) { 23407 composeEnumerationCore("status", element.getStatusElement(), new Observation.ObservationStatusEnumFactory(), 23408 false); 23409 composeEnumerationExtras("status", element.getStatusElement(), new Observation.ObservationStatusEnumFactory(), 23410 false); 23411 } 23412 if (element.hasCategory()) { 23413 composeCodeableConcept("category", element.getCategory()); 23414 } 23415 if (element.hasCode()) { 23416 composeCodeableConcept("code", element.getCode()); 23417 } 23418 if (element.hasSubject()) { 23419 composeReference("subject", element.getSubject()); 23420 } 23421 if (element.hasEncounter()) { 23422 composeReference("encounter", element.getEncounter()); 23423 } 23424 if (element.hasEffective()) { 23425 composeType("effective", element.getEffective()); 23426 } 23427 if (element.hasIssuedElement()) { 23428 composeInstantCore("issued", element.getIssuedElement(), false); 23429 composeInstantExtras("issued", element.getIssuedElement(), false); 23430 } 23431 if (element.hasPerformer()) { 23432 openArray("performer"); 23433 for (Reference e : element.getPerformer()) 23434 composeReference(null, e); 23435 closeArray(); 23436 } 23437 ; 23438 if (element.hasValue()) { 23439 composeType("value", element.getValue()); 23440 } 23441 if (element.hasDataAbsentReason()) { 23442 composeCodeableConcept("dataAbsentReason", element.getDataAbsentReason()); 23443 } 23444 if (element.hasInterpretation()) { 23445 composeCodeableConcept("interpretation", element.getInterpretation()); 23446 } 23447 if (element.hasCommentsElement()) { 23448 composeStringCore("comments", element.getCommentsElement(), false); 23449 composeStringExtras("comments", element.getCommentsElement(), false); 23450 } 23451 if (element.hasBodySite()) { 23452 composeCodeableConcept("bodySite", element.getBodySite()); 23453 } 23454 if (element.hasMethod()) { 23455 composeCodeableConcept("method", element.getMethod()); 23456 } 23457 if (element.hasSpecimen()) { 23458 composeReference("specimen", element.getSpecimen()); 23459 } 23460 if (element.hasDevice()) { 23461 composeReference("device", element.getDevice()); 23462 } 23463 if (element.hasReferenceRange()) { 23464 openArray("referenceRange"); 23465 for (Observation.ObservationReferenceRangeComponent e : element.getReferenceRange()) 23466 composeObservationObservationReferenceRangeComponent(null, e); 23467 closeArray(); 23468 } 23469 ; 23470 if (element.hasRelated()) { 23471 openArray("related"); 23472 for (Observation.ObservationRelatedComponent e : element.getRelated()) 23473 composeObservationObservationRelatedComponent(null, e); 23474 closeArray(); 23475 } 23476 ; 23477 if (element.hasComponent()) { 23478 openArray("component"); 23479 for (Observation.ObservationComponentComponent e : element.getComponent()) 23480 composeObservationObservationComponentComponent(null, e); 23481 closeArray(); 23482 } 23483 ; 23484 } 23485 23486 protected void composeObservationObservationReferenceRangeComponent(String name, 23487 Observation.ObservationReferenceRangeComponent element) throws IOException { 23488 if (element != null) { 23489 open(name); 23490 composeObservationObservationReferenceRangeComponentInner(element); 23491 close(); 23492 } 23493 } 23494 23495 protected void composeObservationObservationReferenceRangeComponentInner( 23496 Observation.ObservationReferenceRangeComponent element) throws IOException { 23497 composeBackbone(element); 23498 if (element.hasLow()) { 23499 composeSimpleQuantity("low", element.getLow()); 23500 } 23501 if (element.hasHigh()) { 23502 composeSimpleQuantity("high", element.getHigh()); 23503 } 23504 if (element.hasMeaning()) { 23505 composeCodeableConcept("meaning", element.getMeaning()); 23506 } 23507 if (element.hasAge()) { 23508 composeRange("age", element.getAge()); 23509 } 23510 if (element.hasTextElement()) { 23511 composeStringCore("text", element.getTextElement(), false); 23512 composeStringExtras("text", element.getTextElement(), false); 23513 } 23514 } 23515 23516 protected void composeObservationObservationRelatedComponent(String name, 23517 Observation.ObservationRelatedComponent element) throws IOException { 23518 if (element != null) { 23519 open(name); 23520 composeObservationObservationRelatedComponentInner(element); 23521 close(); 23522 } 23523 } 23524 23525 protected void composeObservationObservationRelatedComponentInner(Observation.ObservationRelatedComponent element) 23526 throws IOException { 23527 composeBackbone(element); 23528 if (element.hasTypeElement()) { 23529 composeEnumerationCore("type", element.getTypeElement(), new Observation.ObservationRelationshipTypeEnumFactory(), 23530 false); 23531 composeEnumerationExtras("type", element.getTypeElement(), 23532 new Observation.ObservationRelationshipTypeEnumFactory(), false); 23533 } 23534 if (element.hasTarget()) { 23535 composeReference("target", element.getTarget()); 23536 } 23537 } 23538 23539 protected void composeObservationObservationComponentComponent(String name, 23540 Observation.ObservationComponentComponent element) throws IOException { 23541 if (element != null) { 23542 open(name); 23543 composeObservationObservationComponentComponentInner(element); 23544 close(); 23545 } 23546 } 23547 23548 protected void composeObservationObservationComponentComponentInner(Observation.ObservationComponentComponent element) 23549 throws IOException { 23550 composeBackbone(element); 23551 if (element.hasCode()) { 23552 composeCodeableConcept("code", element.getCode()); 23553 } 23554 if (element.hasValue()) { 23555 composeType("value", element.getValue()); 23556 } 23557 if (element.hasDataAbsentReason()) { 23558 composeCodeableConcept("dataAbsentReason", element.getDataAbsentReason()); 23559 } 23560 if (element.hasReferenceRange()) { 23561 openArray("referenceRange"); 23562 for (Observation.ObservationReferenceRangeComponent e : element.getReferenceRange()) 23563 composeObservationObservationReferenceRangeComponent(null, e); 23564 closeArray(); 23565 } 23566 ; 23567 } 23568 23569 protected void composeOperationDefinition(String name, OperationDefinition element) throws IOException { 23570 if (element != null) { 23571 prop("resourceType", name); 23572 composeOperationDefinitionInner(element); 23573 } 23574 } 23575 23576 protected void composeOperationDefinitionInner(OperationDefinition element) throws IOException { 23577 composeDomainResourceElements(element); 23578 if (element.hasUrlElement()) { 23579 composeUriCore("url", element.getUrlElement(), false); 23580 composeUriExtras("url", element.getUrlElement(), false); 23581 } 23582 if (element.hasVersionElement()) { 23583 composeStringCore("version", element.getVersionElement(), false); 23584 composeStringExtras("version", element.getVersionElement(), false); 23585 } 23586 if (element.hasNameElement()) { 23587 composeStringCore("name", element.getNameElement(), false); 23588 composeStringExtras("name", element.getNameElement(), false); 23589 } 23590 if (element.hasStatusElement()) { 23591 composeEnumerationCore("status", element.getStatusElement(), 23592 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 23593 composeEnumerationExtras("status", element.getStatusElement(), 23594 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 23595 } 23596 if (element.hasKindElement()) { 23597 composeEnumerationCore("kind", element.getKindElement(), new OperationDefinition.OperationKindEnumFactory(), 23598 false); 23599 composeEnumerationExtras("kind", element.getKindElement(), new OperationDefinition.OperationKindEnumFactory(), 23600 false); 23601 } 23602 if (element.hasExperimentalElement()) { 23603 composeBooleanCore("experimental", element.getExperimentalElement(), false); 23604 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 23605 } 23606 if (element.hasPublisherElement()) { 23607 composeStringCore("publisher", element.getPublisherElement(), false); 23608 composeStringExtras("publisher", element.getPublisherElement(), false); 23609 } 23610 if (element.hasContact()) { 23611 openArray("contact"); 23612 for (OperationDefinition.OperationDefinitionContactComponent e : element.getContact()) 23613 composeOperationDefinitionOperationDefinitionContactComponent(null, e); 23614 closeArray(); 23615 } 23616 ; 23617 if (element.hasDateElement()) { 23618 composeDateTimeCore("date", element.getDateElement(), false); 23619 composeDateTimeExtras("date", element.getDateElement(), false); 23620 } 23621 if (element.hasDescriptionElement()) { 23622 composeStringCore("description", element.getDescriptionElement(), false); 23623 composeStringExtras("description", element.getDescriptionElement(), false); 23624 } 23625 if (element.hasRequirementsElement()) { 23626 composeStringCore("requirements", element.getRequirementsElement(), false); 23627 composeStringExtras("requirements", element.getRequirementsElement(), false); 23628 } 23629 if (element.hasIdempotentElement()) { 23630 composeBooleanCore("idempotent", element.getIdempotentElement(), false); 23631 composeBooleanExtras("idempotent", element.getIdempotentElement(), false); 23632 } 23633 if (element.hasCodeElement()) { 23634 composeCodeCore("code", element.getCodeElement(), false); 23635 composeCodeExtras("code", element.getCodeElement(), false); 23636 } 23637 if (element.hasNotesElement()) { 23638 composeStringCore("notes", element.getNotesElement(), false); 23639 composeStringExtras("notes", element.getNotesElement(), false); 23640 } 23641 if (element.hasBase()) { 23642 composeReference("base", element.getBase()); 23643 } 23644 if (element.hasSystemElement()) { 23645 composeBooleanCore("system", element.getSystemElement(), false); 23646 composeBooleanExtras("system", element.getSystemElement(), false); 23647 } 23648 if (element.hasType()) { 23649 openArray("type"); 23650 for (CodeType e : element.getType()) 23651 composeCodeCore(null, e, true); 23652 closeArray(); 23653 if (anyHasExtras(element.getType())) { 23654 openArray("_type"); 23655 for (CodeType e : element.getType()) 23656 composeCodeExtras(null, e, true); 23657 closeArray(); 23658 } 23659 } 23660 ; 23661 if (element.hasInstanceElement()) { 23662 composeBooleanCore("instance", element.getInstanceElement(), false); 23663 composeBooleanExtras("instance", element.getInstanceElement(), false); 23664 } 23665 if (element.hasParameter()) { 23666 openArray("parameter"); 23667 for (OperationDefinition.OperationDefinitionParameterComponent e : element.getParameter()) 23668 composeOperationDefinitionOperationDefinitionParameterComponent(null, e); 23669 closeArray(); 23670 } 23671 ; 23672 } 23673 23674 protected void composeOperationDefinitionOperationDefinitionContactComponent(String name, 23675 OperationDefinition.OperationDefinitionContactComponent element) throws IOException { 23676 if (element != null) { 23677 open(name); 23678 composeOperationDefinitionOperationDefinitionContactComponentInner(element); 23679 close(); 23680 } 23681 } 23682 23683 protected void composeOperationDefinitionOperationDefinitionContactComponentInner( 23684 OperationDefinition.OperationDefinitionContactComponent element) throws IOException { 23685 composeBackbone(element); 23686 if (element.hasNameElement()) { 23687 composeStringCore("name", element.getNameElement(), false); 23688 composeStringExtras("name", element.getNameElement(), false); 23689 } 23690 if (element.hasTelecom()) { 23691 openArray("telecom"); 23692 for (ContactPoint e : element.getTelecom()) 23693 composeContactPoint(null, e); 23694 closeArray(); 23695 } 23696 ; 23697 } 23698 23699 protected void composeOperationDefinitionOperationDefinitionParameterComponent(String name, 23700 OperationDefinition.OperationDefinitionParameterComponent element) throws IOException { 23701 if (element != null) { 23702 open(name); 23703 composeOperationDefinitionOperationDefinitionParameterComponentInner(element); 23704 close(); 23705 } 23706 } 23707 23708 protected void composeOperationDefinitionOperationDefinitionParameterComponentInner( 23709 OperationDefinition.OperationDefinitionParameterComponent element) throws IOException { 23710 composeBackbone(element); 23711 if (element.hasNameElement()) { 23712 composeCodeCore("name", element.getNameElement(), false); 23713 composeCodeExtras("name", element.getNameElement(), false); 23714 } 23715 if (element.hasUseElement()) { 23716 composeEnumerationCore("use", element.getUseElement(), new OperationDefinition.OperationParameterUseEnumFactory(), 23717 false); 23718 composeEnumerationExtras("use", element.getUseElement(), 23719 new OperationDefinition.OperationParameterUseEnumFactory(), false); 23720 } 23721 if (element.hasMinElement()) { 23722 composeIntegerCore("min", element.getMinElement(), false); 23723 composeIntegerExtras("min", element.getMinElement(), false); 23724 } 23725 if (element.hasMaxElement()) { 23726 composeStringCore("max", element.getMaxElement(), false); 23727 composeStringExtras("max", element.getMaxElement(), false); 23728 } 23729 if (element.hasDocumentationElement()) { 23730 composeStringCore("documentation", element.getDocumentationElement(), false); 23731 composeStringExtras("documentation", element.getDocumentationElement(), false); 23732 } 23733 if (element.hasTypeElement()) { 23734 composeCodeCore("type", element.getTypeElement(), false); 23735 composeCodeExtras("type", element.getTypeElement(), false); 23736 } 23737 if (element.hasProfile()) { 23738 composeReference("profile", element.getProfile()); 23739 } 23740 if (element.hasBinding()) { 23741 composeOperationDefinitionOperationDefinitionParameterBindingComponent("binding", element.getBinding()); 23742 } 23743 if (element.hasPart()) { 23744 openArray("part"); 23745 for (OperationDefinition.OperationDefinitionParameterComponent e : element.getPart()) 23746 composeOperationDefinitionOperationDefinitionParameterComponent(null, e); 23747 closeArray(); 23748 } 23749 ; 23750 } 23751 23752 protected void composeOperationDefinitionOperationDefinitionParameterBindingComponent(String name, 23753 OperationDefinition.OperationDefinitionParameterBindingComponent element) throws IOException { 23754 if (element != null) { 23755 open(name); 23756 composeOperationDefinitionOperationDefinitionParameterBindingComponentInner(element); 23757 close(); 23758 } 23759 } 23760 23761 protected void composeOperationDefinitionOperationDefinitionParameterBindingComponentInner( 23762 OperationDefinition.OperationDefinitionParameterBindingComponent element) throws IOException { 23763 composeBackbone(element); 23764 if (element.hasStrengthElement()) { 23765 composeEnumerationCore("strength", element.getStrengthElement(), new Enumerations.BindingStrengthEnumFactory(), 23766 false); 23767 composeEnumerationExtras("strength", element.getStrengthElement(), new Enumerations.BindingStrengthEnumFactory(), 23768 false); 23769 } 23770 if (element.hasValueSet()) { 23771 composeType("valueSet", element.getValueSet()); 23772 } 23773 } 23774 23775 protected void composeOperationOutcome(String name, OperationOutcome element) throws IOException { 23776 if (element != null) { 23777 prop("resourceType", name); 23778 composeOperationOutcomeInner(element); 23779 } 23780 } 23781 23782 protected void composeOperationOutcomeInner(OperationOutcome element) throws IOException { 23783 composeDomainResourceElements(element); 23784 if (element.hasIssue()) { 23785 openArray("issue"); 23786 for (OperationOutcome.OperationOutcomeIssueComponent e : element.getIssue()) 23787 composeOperationOutcomeOperationOutcomeIssueComponent(null, e); 23788 closeArray(); 23789 } 23790 ; 23791 } 23792 23793 protected void composeOperationOutcomeOperationOutcomeIssueComponent(String name, 23794 OperationOutcome.OperationOutcomeIssueComponent element) throws IOException { 23795 if (element != null) { 23796 open(name); 23797 composeOperationOutcomeOperationOutcomeIssueComponentInner(element); 23798 close(); 23799 } 23800 } 23801 23802 protected void composeOperationOutcomeOperationOutcomeIssueComponentInner( 23803 OperationOutcome.OperationOutcomeIssueComponent element) throws IOException { 23804 composeBackbone(element); 23805 if (element.hasSeverityElement()) { 23806 composeEnumerationCore("severity", element.getSeverityElement(), new OperationOutcome.IssueSeverityEnumFactory(), 23807 false); 23808 composeEnumerationExtras("severity", element.getSeverityElement(), 23809 new OperationOutcome.IssueSeverityEnumFactory(), false); 23810 } 23811 if (element.hasCodeElement()) { 23812 composeEnumerationCore("code", element.getCodeElement(), new OperationOutcome.IssueTypeEnumFactory(), false); 23813 composeEnumerationExtras("code", element.getCodeElement(), new OperationOutcome.IssueTypeEnumFactory(), false); 23814 } 23815 if (element.hasDetails()) { 23816 composeCodeableConcept("details", element.getDetails()); 23817 } 23818 if (element.hasDiagnosticsElement()) { 23819 composeStringCore("diagnostics", element.getDiagnosticsElement(), false); 23820 composeStringExtras("diagnostics", element.getDiagnosticsElement(), false); 23821 } 23822 if (element.hasLocation()) { 23823 openArray("location"); 23824 for (StringType e : element.getLocation()) 23825 composeStringCore(null, e, true); 23826 closeArray(); 23827 if (anyHasExtras(element.getLocation())) { 23828 openArray("_location"); 23829 for (StringType e : element.getLocation()) 23830 composeStringExtras(null, e, true); 23831 closeArray(); 23832 } 23833 } 23834 ; 23835 } 23836 23837 protected void composeOrder(String name, Order element) throws IOException { 23838 if (element != null) { 23839 prop("resourceType", name); 23840 composeOrderInner(element); 23841 } 23842 } 23843 23844 protected void composeOrderInner(Order element) throws IOException { 23845 composeDomainResourceElements(element); 23846 if (element.hasIdentifier()) { 23847 openArray("identifier"); 23848 for (Identifier e : element.getIdentifier()) 23849 composeIdentifier(null, e); 23850 closeArray(); 23851 } 23852 ; 23853 if (element.hasDateElement()) { 23854 composeDateTimeCore("date", element.getDateElement(), false); 23855 composeDateTimeExtras("date", element.getDateElement(), false); 23856 } 23857 if (element.hasSubject()) { 23858 composeReference("subject", element.getSubject()); 23859 } 23860 if (element.hasSource()) { 23861 composeReference("source", element.getSource()); 23862 } 23863 if (element.hasTarget()) { 23864 composeReference("target", element.getTarget()); 23865 } 23866 if (element.hasReason()) { 23867 composeType("reason", element.getReason()); 23868 } 23869 if (element.hasWhen()) { 23870 composeOrderOrderWhenComponent("when", element.getWhen()); 23871 } 23872 if (element.hasDetail()) { 23873 openArray("detail"); 23874 for (Reference e : element.getDetail()) 23875 composeReference(null, e); 23876 closeArray(); 23877 } 23878 ; 23879 } 23880 23881 protected void composeOrderOrderWhenComponent(String name, Order.OrderWhenComponent element) throws IOException { 23882 if (element != null) { 23883 open(name); 23884 composeOrderOrderWhenComponentInner(element); 23885 close(); 23886 } 23887 } 23888 23889 protected void composeOrderOrderWhenComponentInner(Order.OrderWhenComponent element) throws IOException { 23890 composeBackbone(element); 23891 if (element.hasCode()) { 23892 composeCodeableConcept("code", element.getCode()); 23893 } 23894 if (element.hasSchedule()) { 23895 composeTiming("schedule", element.getSchedule()); 23896 } 23897 } 23898 23899 protected void composeOrderResponse(String name, OrderResponse element) throws IOException { 23900 if (element != null) { 23901 prop("resourceType", name); 23902 composeOrderResponseInner(element); 23903 } 23904 } 23905 23906 protected void composeOrderResponseInner(OrderResponse element) throws IOException { 23907 composeDomainResourceElements(element); 23908 if (element.hasIdentifier()) { 23909 openArray("identifier"); 23910 for (Identifier e : element.getIdentifier()) 23911 composeIdentifier(null, e); 23912 closeArray(); 23913 } 23914 ; 23915 if (element.hasRequest()) { 23916 composeReference("request", element.getRequest()); 23917 } 23918 if (element.hasDateElement()) { 23919 composeDateTimeCore("date", element.getDateElement(), false); 23920 composeDateTimeExtras("date", element.getDateElement(), false); 23921 } 23922 if (element.hasWho()) { 23923 composeReference("who", element.getWho()); 23924 } 23925 if (element.hasOrderStatusElement()) { 23926 composeEnumerationCore("orderStatus", element.getOrderStatusElement(), new OrderResponse.OrderStatusEnumFactory(), 23927 false); 23928 composeEnumerationExtras("orderStatus", element.getOrderStatusElement(), 23929 new OrderResponse.OrderStatusEnumFactory(), false); 23930 } 23931 if (element.hasDescriptionElement()) { 23932 composeStringCore("description", element.getDescriptionElement(), false); 23933 composeStringExtras("description", element.getDescriptionElement(), false); 23934 } 23935 if (element.hasFulfillment()) { 23936 openArray("fulfillment"); 23937 for (Reference e : element.getFulfillment()) 23938 composeReference(null, e); 23939 closeArray(); 23940 } 23941 ; 23942 } 23943 23944 protected void composeOrganization(String name, Organization element) throws IOException { 23945 if (element != null) { 23946 prop("resourceType", name); 23947 composeOrganizationInner(element); 23948 } 23949 } 23950 23951 protected void composeOrganizationInner(Organization element) throws IOException { 23952 composeDomainResourceElements(element); 23953 if (element.hasIdentifier()) { 23954 openArray("identifier"); 23955 for (Identifier e : element.getIdentifier()) 23956 composeIdentifier(null, e); 23957 closeArray(); 23958 } 23959 ; 23960 if (element.hasActiveElement()) { 23961 composeBooleanCore("active", element.getActiveElement(), false); 23962 composeBooleanExtras("active", element.getActiveElement(), false); 23963 } 23964 if (element.hasType()) { 23965 composeCodeableConcept("type", element.getType()); 23966 } 23967 if (element.hasNameElement()) { 23968 composeStringCore("name", element.getNameElement(), false); 23969 composeStringExtras("name", element.getNameElement(), false); 23970 } 23971 if (element.hasTelecom()) { 23972 openArray("telecom"); 23973 for (ContactPoint e : element.getTelecom()) 23974 composeContactPoint(null, e); 23975 closeArray(); 23976 } 23977 ; 23978 if (element.hasAddress()) { 23979 openArray("address"); 23980 for (Address e : element.getAddress()) 23981 composeAddress(null, e); 23982 closeArray(); 23983 } 23984 ; 23985 if (element.hasPartOf()) { 23986 composeReference("partOf", element.getPartOf()); 23987 } 23988 if (element.hasContact()) { 23989 openArray("contact"); 23990 for (Organization.OrganizationContactComponent e : element.getContact()) 23991 composeOrganizationOrganizationContactComponent(null, e); 23992 closeArray(); 23993 } 23994 ; 23995 } 23996 23997 protected void composeOrganizationOrganizationContactComponent(String name, 23998 Organization.OrganizationContactComponent element) throws IOException { 23999 if (element != null) { 24000 open(name); 24001 composeOrganizationOrganizationContactComponentInner(element); 24002 close(); 24003 } 24004 } 24005 24006 protected void composeOrganizationOrganizationContactComponentInner(Organization.OrganizationContactComponent element) 24007 throws IOException { 24008 composeBackbone(element); 24009 if (element.hasPurpose()) { 24010 composeCodeableConcept("purpose", element.getPurpose()); 24011 } 24012 if (element.hasName()) { 24013 composeHumanName("name", element.getName()); 24014 } 24015 if (element.hasTelecom()) { 24016 openArray("telecom"); 24017 for (ContactPoint e : element.getTelecom()) 24018 composeContactPoint(null, e); 24019 closeArray(); 24020 } 24021 ; 24022 if (element.hasAddress()) { 24023 composeAddress("address", element.getAddress()); 24024 } 24025 } 24026 24027 protected void composePatient(String name, Patient element) throws IOException { 24028 if (element != null) { 24029 prop("resourceType", name); 24030 composePatientInner(element); 24031 } 24032 } 24033 24034 protected void composePatientInner(Patient element) throws IOException { 24035 composeDomainResourceElements(element); 24036 if (element.hasIdentifier()) { 24037 openArray("identifier"); 24038 for (Identifier e : element.getIdentifier()) 24039 composeIdentifier(null, e); 24040 closeArray(); 24041 } 24042 ; 24043 if (element.hasActiveElement()) { 24044 composeBooleanCore("active", element.getActiveElement(), false); 24045 composeBooleanExtras("active", element.getActiveElement(), false); 24046 } 24047 if (element.hasName()) { 24048 openArray("name"); 24049 for (HumanName e : element.getName()) 24050 composeHumanName(null, e); 24051 closeArray(); 24052 } 24053 ; 24054 if (element.hasTelecom()) { 24055 openArray("telecom"); 24056 for (ContactPoint e : element.getTelecom()) 24057 composeContactPoint(null, e); 24058 closeArray(); 24059 } 24060 ; 24061 if (element.hasGenderElement()) { 24062 composeEnumerationCore("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), 24063 false); 24064 composeEnumerationExtras("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), 24065 false); 24066 } 24067 if (element.hasBirthDateElement()) { 24068 composeDateCore("birthDate", element.getBirthDateElement(), false); 24069 composeDateExtras("birthDate", element.getBirthDateElement(), false); 24070 } 24071 if (element.hasDeceased()) { 24072 composeType("deceased", element.getDeceased()); 24073 } 24074 if (element.hasAddress()) { 24075 openArray("address"); 24076 for (Address e : element.getAddress()) 24077 composeAddress(null, e); 24078 closeArray(); 24079 } 24080 ; 24081 if (element.hasMaritalStatus()) { 24082 composeCodeableConcept("maritalStatus", element.getMaritalStatus()); 24083 } 24084 if (element.hasMultipleBirth()) { 24085 composeType("multipleBirth", element.getMultipleBirth()); 24086 } 24087 if (element.hasPhoto()) { 24088 openArray("photo"); 24089 for (Attachment e : element.getPhoto()) 24090 composeAttachment(null, e); 24091 closeArray(); 24092 } 24093 ; 24094 if (element.hasContact()) { 24095 openArray("contact"); 24096 for (Patient.ContactComponent e : element.getContact()) 24097 composePatientContactComponent(null, e); 24098 closeArray(); 24099 } 24100 ; 24101 if (element.hasAnimal()) { 24102 composePatientAnimalComponent("animal", element.getAnimal()); 24103 } 24104 if (element.hasCommunication()) { 24105 openArray("communication"); 24106 for (Patient.PatientCommunicationComponent e : element.getCommunication()) 24107 composePatientPatientCommunicationComponent(null, e); 24108 closeArray(); 24109 } 24110 ; 24111 if (element.hasCareProvider()) { 24112 openArray("careProvider"); 24113 for (Reference e : element.getCareProvider()) 24114 composeReference(null, e); 24115 closeArray(); 24116 } 24117 ; 24118 if (element.hasManagingOrganization()) { 24119 composeReference("managingOrganization", element.getManagingOrganization()); 24120 } 24121 if (element.hasLink()) { 24122 openArray("link"); 24123 for (Patient.PatientLinkComponent e : element.getLink()) 24124 composePatientPatientLinkComponent(null, e); 24125 closeArray(); 24126 } 24127 ; 24128 } 24129 24130 protected void composePatientContactComponent(String name, Patient.ContactComponent element) throws IOException { 24131 if (element != null) { 24132 open(name); 24133 composePatientContactComponentInner(element); 24134 close(); 24135 } 24136 } 24137 24138 protected void composePatientContactComponentInner(Patient.ContactComponent element) throws IOException { 24139 composeBackbone(element); 24140 if (element.hasRelationship()) { 24141 openArray("relationship"); 24142 for (CodeableConcept e : element.getRelationship()) 24143 composeCodeableConcept(null, e); 24144 closeArray(); 24145 } 24146 ; 24147 if (element.hasName()) { 24148 composeHumanName("name", element.getName()); 24149 } 24150 if (element.hasTelecom()) { 24151 openArray("telecom"); 24152 for (ContactPoint e : element.getTelecom()) 24153 composeContactPoint(null, e); 24154 closeArray(); 24155 } 24156 ; 24157 if (element.hasAddress()) { 24158 composeAddress("address", element.getAddress()); 24159 } 24160 if (element.hasGenderElement()) { 24161 composeEnumerationCore("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), 24162 false); 24163 composeEnumerationExtras("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), 24164 false); 24165 } 24166 if (element.hasOrganization()) { 24167 composeReference("organization", element.getOrganization()); 24168 } 24169 if (element.hasPeriod()) { 24170 composePeriod("period", element.getPeriod()); 24171 } 24172 } 24173 24174 protected void composePatientAnimalComponent(String name, Patient.AnimalComponent element) throws IOException { 24175 if (element != null) { 24176 open(name); 24177 composePatientAnimalComponentInner(element); 24178 close(); 24179 } 24180 } 24181 24182 protected void composePatientAnimalComponentInner(Patient.AnimalComponent element) throws IOException { 24183 composeBackbone(element); 24184 if (element.hasSpecies()) { 24185 composeCodeableConcept("species", element.getSpecies()); 24186 } 24187 if (element.hasBreed()) { 24188 composeCodeableConcept("breed", element.getBreed()); 24189 } 24190 if (element.hasGenderStatus()) { 24191 composeCodeableConcept("genderStatus", element.getGenderStatus()); 24192 } 24193 } 24194 24195 protected void composePatientPatientCommunicationComponent(String name, Patient.PatientCommunicationComponent element) 24196 throws IOException { 24197 if (element != null) { 24198 open(name); 24199 composePatientPatientCommunicationComponentInner(element); 24200 close(); 24201 } 24202 } 24203 24204 protected void composePatientPatientCommunicationComponentInner(Patient.PatientCommunicationComponent element) 24205 throws IOException { 24206 composeBackbone(element); 24207 if (element.hasLanguage()) { 24208 composeCodeableConcept("language", element.getLanguage()); 24209 } 24210 if (element.hasPreferredElement()) { 24211 composeBooleanCore("preferred", element.getPreferredElement(), false); 24212 composeBooleanExtras("preferred", element.getPreferredElement(), false); 24213 } 24214 } 24215 24216 protected void composePatientPatientLinkComponent(String name, Patient.PatientLinkComponent element) 24217 throws IOException { 24218 if (element != null) { 24219 open(name); 24220 composePatientPatientLinkComponentInner(element); 24221 close(); 24222 } 24223 } 24224 24225 protected void composePatientPatientLinkComponentInner(Patient.PatientLinkComponent element) throws IOException { 24226 composeBackbone(element); 24227 if (element.hasOther()) { 24228 composeReference("other", element.getOther()); 24229 } 24230 if (element.hasTypeElement()) { 24231 composeEnumerationCore("type", element.getTypeElement(), new Patient.LinkTypeEnumFactory(), false); 24232 composeEnumerationExtras("type", element.getTypeElement(), new Patient.LinkTypeEnumFactory(), false); 24233 } 24234 } 24235 24236 protected void composePaymentNotice(String name, PaymentNotice element) throws IOException { 24237 if (element != null) { 24238 prop("resourceType", name); 24239 composePaymentNoticeInner(element); 24240 } 24241 } 24242 24243 protected void composePaymentNoticeInner(PaymentNotice element) throws IOException { 24244 composeDomainResourceElements(element); 24245 if (element.hasIdentifier()) { 24246 openArray("identifier"); 24247 for (Identifier e : element.getIdentifier()) 24248 composeIdentifier(null, e); 24249 closeArray(); 24250 } 24251 ; 24252 if (element.hasRuleset()) { 24253 composeCoding("ruleset", element.getRuleset()); 24254 } 24255 if (element.hasOriginalRuleset()) { 24256 composeCoding("originalRuleset", element.getOriginalRuleset()); 24257 } 24258 if (element.hasCreatedElement()) { 24259 composeDateTimeCore("created", element.getCreatedElement(), false); 24260 composeDateTimeExtras("created", element.getCreatedElement(), false); 24261 } 24262 if (element.hasTarget()) { 24263 composeReference("target", element.getTarget()); 24264 } 24265 if (element.hasProvider()) { 24266 composeReference("provider", element.getProvider()); 24267 } 24268 if (element.hasOrganization()) { 24269 composeReference("organization", element.getOrganization()); 24270 } 24271 if (element.hasRequest()) { 24272 composeReference("request", element.getRequest()); 24273 } 24274 if (element.hasResponse()) { 24275 composeReference("response", element.getResponse()); 24276 } 24277 if (element.hasPaymentStatus()) { 24278 composeCoding("paymentStatus", element.getPaymentStatus()); 24279 } 24280 } 24281 24282 protected void composePaymentReconciliation(String name, PaymentReconciliation element) throws IOException { 24283 if (element != null) { 24284 prop("resourceType", name); 24285 composePaymentReconciliationInner(element); 24286 } 24287 } 24288 24289 protected void composePaymentReconciliationInner(PaymentReconciliation element) throws IOException { 24290 composeDomainResourceElements(element); 24291 if (element.hasIdentifier()) { 24292 openArray("identifier"); 24293 for (Identifier e : element.getIdentifier()) 24294 composeIdentifier(null, e); 24295 closeArray(); 24296 } 24297 ; 24298 if (element.hasRequest()) { 24299 composeReference("request", element.getRequest()); 24300 } 24301 if (element.hasOutcomeElement()) { 24302 composeEnumerationCore("outcome", element.getOutcomeElement(), new Enumerations.RemittanceOutcomeEnumFactory(), 24303 false); 24304 composeEnumerationExtras("outcome", element.getOutcomeElement(), new Enumerations.RemittanceOutcomeEnumFactory(), 24305 false); 24306 } 24307 if (element.hasDispositionElement()) { 24308 composeStringCore("disposition", element.getDispositionElement(), false); 24309 composeStringExtras("disposition", element.getDispositionElement(), false); 24310 } 24311 if (element.hasRuleset()) { 24312 composeCoding("ruleset", element.getRuleset()); 24313 } 24314 if (element.hasOriginalRuleset()) { 24315 composeCoding("originalRuleset", element.getOriginalRuleset()); 24316 } 24317 if (element.hasCreatedElement()) { 24318 composeDateTimeCore("created", element.getCreatedElement(), false); 24319 composeDateTimeExtras("created", element.getCreatedElement(), false); 24320 } 24321 if (element.hasPeriod()) { 24322 composePeriod("period", element.getPeriod()); 24323 } 24324 if (element.hasOrganization()) { 24325 composeReference("organization", element.getOrganization()); 24326 } 24327 if (element.hasRequestProvider()) { 24328 composeReference("requestProvider", element.getRequestProvider()); 24329 } 24330 if (element.hasRequestOrganization()) { 24331 composeReference("requestOrganization", element.getRequestOrganization()); 24332 } 24333 if (element.hasDetail()) { 24334 openArray("detail"); 24335 for (PaymentReconciliation.DetailsComponent e : element.getDetail()) 24336 composePaymentReconciliationDetailsComponent(null, e); 24337 closeArray(); 24338 } 24339 ; 24340 if (element.hasForm()) { 24341 composeCoding("form", element.getForm()); 24342 } 24343 if (element.hasTotal()) { 24344 composeMoney("total", element.getTotal()); 24345 } 24346 if (element.hasNote()) { 24347 openArray("note"); 24348 for (PaymentReconciliation.NotesComponent e : element.getNote()) 24349 composePaymentReconciliationNotesComponent(null, e); 24350 closeArray(); 24351 } 24352 ; 24353 } 24354 24355 protected void composePaymentReconciliationDetailsComponent(String name, 24356 PaymentReconciliation.DetailsComponent element) throws IOException { 24357 if (element != null) { 24358 open(name); 24359 composePaymentReconciliationDetailsComponentInner(element); 24360 close(); 24361 } 24362 } 24363 24364 protected void composePaymentReconciliationDetailsComponentInner(PaymentReconciliation.DetailsComponent element) 24365 throws IOException { 24366 composeBackbone(element); 24367 if (element.hasType()) { 24368 composeCoding("type", element.getType()); 24369 } 24370 if (element.hasRequest()) { 24371 composeReference("request", element.getRequest()); 24372 } 24373 if (element.hasResponce()) { 24374 composeReference("responce", element.getResponce()); 24375 } 24376 if (element.hasSubmitter()) { 24377 composeReference("submitter", element.getSubmitter()); 24378 } 24379 if (element.hasPayee()) { 24380 composeReference("payee", element.getPayee()); 24381 } 24382 if (element.hasDateElement()) { 24383 composeDateCore("date", element.getDateElement(), false); 24384 composeDateExtras("date", element.getDateElement(), false); 24385 } 24386 if (element.hasAmount()) { 24387 composeMoney("amount", element.getAmount()); 24388 } 24389 } 24390 24391 protected void composePaymentReconciliationNotesComponent(String name, PaymentReconciliation.NotesComponent element) 24392 throws IOException { 24393 if (element != null) { 24394 open(name); 24395 composePaymentReconciliationNotesComponentInner(element); 24396 close(); 24397 } 24398 } 24399 24400 protected void composePaymentReconciliationNotesComponentInner(PaymentReconciliation.NotesComponent element) 24401 throws IOException { 24402 composeBackbone(element); 24403 if (element.hasType()) { 24404 composeCoding("type", element.getType()); 24405 } 24406 if (element.hasTextElement()) { 24407 composeStringCore("text", element.getTextElement(), false); 24408 composeStringExtras("text", element.getTextElement(), false); 24409 } 24410 } 24411 24412 protected void composePerson(String name, Person element) throws IOException { 24413 if (element != null) { 24414 prop("resourceType", name); 24415 composePersonInner(element); 24416 } 24417 } 24418 24419 protected void composePersonInner(Person element) throws IOException { 24420 composeDomainResourceElements(element); 24421 if (element.hasIdentifier()) { 24422 openArray("identifier"); 24423 for (Identifier e : element.getIdentifier()) 24424 composeIdentifier(null, e); 24425 closeArray(); 24426 } 24427 ; 24428 if (element.hasName()) { 24429 openArray("name"); 24430 for (HumanName e : element.getName()) 24431 composeHumanName(null, e); 24432 closeArray(); 24433 } 24434 ; 24435 if (element.hasTelecom()) { 24436 openArray("telecom"); 24437 for (ContactPoint e : element.getTelecom()) 24438 composeContactPoint(null, e); 24439 closeArray(); 24440 } 24441 ; 24442 if (element.hasGenderElement()) { 24443 composeEnumerationCore("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), 24444 false); 24445 composeEnumerationExtras("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), 24446 false); 24447 } 24448 if (element.hasBirthDateElement()) { 24449 composeDateCore("birthDate", element.getBirthDateElement(), false); 24450 composeDateExtras("birthDate", element.getBirthDateElement(), false); 24451 } 24452 if (element.hasAddress()) { 24453 openArray("address"); 24454 for (Address e : element.getAddress()) 24455 composeAddress(null, e); 24456 closeArray(); 24457 } 24458 ; 24459 if (element.hasPhoto()) { 24460 composeAttachment("photo", element.getPhoto()); 24461 } 24462 if (element.hasManagingOrganization()) { 24463 composeReference("managingOrganization", element.getManagingOrganization()); 24464 } 24465 if (element.hasActiveElement()) { 24466 composeBooleanCore("active", element.getActiveElement(), false); 24467 composeBooleanExtras("active", element.getActiveElement(), false); 24468 } 24469 if (element.hasLink()) { 24470 openArray("link"); 24471 for (Person.PersonLinkComponent e : element.getLink()) 24472 composePersonPersonLinkComponent(null, e); 24473 closeArray(); 24474 } 24475 ; 24476 } 24477 24478 protected void composePersonPersonLinkComponent(String name, Person.PersonLinkComponent element) throws IOException { 24479 if (element != null) { 24480 open(name); 24481 composePersonPersonLinkComponentInner(element); 24482 close(); 24483 } 24484 } 24485 24486 protected void composePersonPersonLinkComponentInner(Person.PersonLinkComponent element) throws IOException { 24487 composeBackbone(element); 24488 if (element.hasTarget()) { 24489 composeReference("target", element.getTarget()); 24490 } 24491 if (element.hasAssuranceElement()) { 24492 composeEnumerationCore("assurance", element.getAssuranceElement(), new Person.IdentityAssuranceLevelEnumFactory(), 24493 false); 24494 composeEnumerationExtras("assurance", element.getAssuranceElement(), 24495 new Person.IdentityAssuranceLevelEnumFactory(), false); 24496 } 24497 } 24498 24499 protected void composePractitioner(String name, Practitioner element) throws IOException { 24500 if (element != null) { 24501 prop("resourceType", name); 24502 composePractitionerInner(element); 24503 } 24504 } 24505 24506 protected void composePractitionerInner(Practitioner element) throws IOException { 24507 composeDomainResourceElements(element); 24508 if (element.hasIdentifier()) { 24509 openArray("identifier"); 24510 for (Identifier e : element.getIdentifier()) 24511 composeIdentifier(null, e); 24512 closeArray(); 24513 } 24514 ; 24515 if (element.hasActiveElement()) { 24516 composeBooleanCore("active", element.getActiveElement(), false); 24517 composeBooleanExtras("active", element.getActiveElement(), false); 24518 } 24519 if (element.hasName()) { 24520 composeHumanName("name", element.getName()); 24521 } 24522 if (element.hasTelecom()) { 24523 openArray("telecom"); 24524 for (ContactPoint e : element.getTelecom()) 24525 composeContactPoint(null, e); 24526 closeArray(); 24527 } 24528 ; 24529 if (element.hasAddress()) { 24530 openArray("address"); 24531 for (Address e : element.getAddress()) 24532 composeAddress(null, e); 24533 closeArray(); 24534 } 24535 ; 24536 if (element.hasGenderElement()) { 24537 composeEnumerationCore("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), 24538 false); 24539 composeEnumerationExtras("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), 24540 false); 24541 } 24542 if (element.hasBirthDateElement()) { 24543 composeDateCore("birthDate", element.getBirthDateElement(), false); 24544 composeDateExtras("birthDate", element.getBirthDateElement(), false); 24545 } 24546 if (element.hasPhoto()) { 24547 openArray("photo"); 24548 for (Attachment e : element.getPhoto()) 24549 composeAttachment(null, e); 24550 closeArray(); 24551 } 24552 ; 24553 if (element.hasPractitionerRole()) { 24554 openArray("practitionerRole"); 24555 for (Practitioner.PractitionerPractitionerRoleComponent e : element.getPractitionerRole()) 24556 composePractitionerPractitionerPractitionerRoleComponent(null, e); 24557 closeArray(); 24558 } 24559 ; 24560 if (element.hasQualification()) { 24561 openArray("qualification"); 24562 for (Practitioner.PractitionerQualificationComponent e : element.getQualification()) 24563 composePractitionerPractitionerQualificationComponent(null, e); 24564 closeArray(); 24565 } 24566 ; 24567 if (element.hasCommunication()) { 24568 openArray("communication"); 24569 for (CodeableConcept e : element.getCommunication()) 24570 composeCodeableConcept(null, e); 24571 closeArray(); 24572 } 24573 ; 24574 } 24575 24576 protected void composePractitionerPractitionerPractitionerRoleComponent(String name, 24577 Practitioner.PractitionerPractitionerRoleComponent element) throws IOException { 24578 if (element != null) { 24579 open(name); 24580 composePractitionerPractitionerPractitionerRoleComponentInner(element); 24581 close(); 24582 } 24583 } 24584 24585 protected void composePractitionerPractitionerPractitionerRoleComponentInner( 24586 Practitioner.PractitionerPractitionerRoleComponent element) throws IOException { 24587 composeBackbone(element); 24588 if (element.hasManagingOrganization()) { 24589 composeReference("managingOrganization", element.getManagingOrganization()); 24590 } 24591 if (element.hasRole()) { 24592 composeCodeableConcept("role", element.getRole()); 24593 } 24594 if (element.hasSpecialty()) { 24595 openArray("specialty"); 24596 for (CodeableConcept e : element.getSpecialty()) 24597 composeCodeableConcept(null, e); 24598 closeArray(); 24599 } 24600 ; 24601 if (element.hasPeriod()) { 24602 composePeriod("period", element.getPeriod()); 24603 } 24604 if (element.hasLocation()) { 24605 openArray("location"); 24606 for (Reference e : element.getLocation()) 24607 composeReference(null, e); 24608 closeArray(); 24609 } 24610 ; 24611 if (element.hasHealthcareService()) { 24612 openArray("healthcareService"); 24613 for (Reference e : element.getHealthcareService()) 24614 composeReference(null, e); 24615 closeArray(); 24616 } 24617 ; 24618 } 24619 24620 protected void composePractitionerPractitionerQualificationComponent(String name, 24621 Practitioner.PractitionerQualificationComponent element) throws IOException { 24622 if (element != null) { 24623 open(name); 24624 composePractitionerPractitionerQualificationComponentInner(element); 24625 close(); 24626 } 24627 } 24628 24629 protected void composePractitionerPractitionerQualificationComponentInner( 24630 Practitioner.PractitionerQualificationComponent element) throws IOException { 24631 composeBackbone(element); 24632 if (element.hasIdentifier()) { 24633 openArray("identifier"); 24634 for (Identifier e : element.getIdentifier()) 24635 composeIdentifier(null, e); 24636 closeArray(); 24637 } 24638 ; 24639 if (element.hasCode()) { 24640 composeCodeableConcept("code", element.getCode()); 24641 } 24642 if (element.hasPeriod()) { 24643 composePeriod("period", element.getPeriod()); 24644 } 24645 if (element.hasIssuer()) { 24646 composeReference("issuer", element.getIssuer()); 24647 } 24648 } 24649 24650 protected void composeProcedure(String name, Procedure element) throws IOException { 24651 if (element != null) { 24652 prop("resourceType", name); 24653 composeProcedureInner(element); 24654 } 24655 } 24656 24657 protected void composeProcedureInner(Procedure element) throws IOException { 24658 composeDomainResourceElements(element); 24659 if (element.hasIdentifier()) { 24660 openArray("identifier"); 24661 for (Identifier e : element.getIdentifier()) 24662 composeIdentifier(null, e); 24663 closeArray(); 24664 } 24665 ; 24666 if (element.hasSubject()) { 24667 composeReference("subject", element.getSubject()); 24668 } 24669 if (element.hasStatusElement()) { 24670 composeEnumerationCore("status", element.getStatusElement(), new Procedure.ProcedureStatusEnumFactory(), false); 24671 composeEnumerationExtras("status", element.getStatusElement(), new Procedure.ProcedureStatusEnumFactory(), false); 24672 } 24673 if (element.hasCategory()) { 24674 composeCodeableConcept("category", element.getCategory()); 24675 } 24676 if (element.hasCode()) { 24677 composeCodeableConcept("code", element.getCode()); 24678 } 24679 if (element.hasNotPerformedElement()) { 24680 composeBooleanCore("notPerformed", element.getNotPerformedElement(), false); 24681 composeBooleanExtras("notPerformed", element.getNotPerformedElement(), false); 24682 } 24683 if (element.hasReasonNotPerformed()) { 24684 openArray("reasonNotPerformed"); 24685 for (CodeableConcept e : element.getReasonNotPerformed()) 24686 composeCodeableConcept(null, e); 24687 closeArray(); 24688 } 24689 ; 24690 if (element.hasBodySite()) { 24691 openArray("bodySite"); 24692 for (CodeableConcept e : element.getBodySite()) 24693 composeCodeableConcept(null, e); 24694 closeArray(); 24695 } 24696 ; 24697 if (element.hasReason()) { 24698 composeType("reason", element.getReason()); 24699 } 24700 if (element.hasPerformer()) { 24701 openArray("performer"); 24702 for (Procedure.ProcedurePerformerComponent e : element.getPerformer()) 24703 composeProcedureProcedurePerformerComponent(null, e); 24704 closeArray(); 24705 } 24706 ; 24707 if (element.hasPerformed()) { 24708 composeType("performed", element.getPerformed()); 24709 } 24710 if (element.hasEncounter()) { 24711 composeReference("encounter", element.getEncounter()); 24712 } 24713 if (element.hasLocation()) { 24714 composeReference("location", element.getLocation()); 24715 } 24716 if (element.hasOutcome()) { 24717 composeCodeableConcept("outcome", element.getOutcome()); 24718 } 24719 if (element.hasReport()) { 24720 openArray("report"); 24721 for (Reference e : element.getReport()) 24722 composeReference(null, e); 24723 closeArray(); 24724 } 24725 ; 24726 if (element.hasComplication()) { 24727 openArray("complication"); 24728 for (CodeableConcept e : element.getComplication()) 24729 composeCodeableConcept(null, e); 24730 closeArray(); 24731 } 24732 ; 24733 if (element.hasFollowUp()) { 24734 openArray("followUp"); 24735 for (CodeableConcept e : element.getFollowUp()) 24736 composeCodeableConcept(null, e); 24737 closeArray(); 24738 } 24739 ; 24740 if (element.hasRequest()) { 24741 composeReference("request", element.getRequest()); 24742 } 24743 if (element.hasNotes()) { 24744 openArray("notes"); 24745 for (Annotation e : element.getNotes()) 24746 composeAnnotation(null, e); 24747 closeArray(); 24748 } 24749 ; 24750 if (element.hasFocalDevice()) { 24751 openArray("focalDevice"); 24752 for (Procedure.ProcedureFocalDeviceComponent e : element.getFocalDevice()) 24753 composeProcedureProcedureFocalDeviceComponent(null, e); 24754 closeArray(); 24755 } 24756 ; 24757 if (element.hasUsed()) { 24758 openArray("used"); 24759 for (Reference e : element.getUsed()) 24760 composeReference(null, e); 24761 closeArray(); 24762 } 24763 ; 24764 } 24765 24766 protected void composeProcedureProcedurePerformerComponent(String name, Procedure.ProcedurePerformerComponent element) 24767 throws IOException { 24768 if (element != null) { 24769 open(name); 24770 composeProcedureProcedurePerformerComponentInner(element); 24771 close(); 24772 } 24773 } 24774 24775 protected void composeProcedureProcedurePerformerComponentInner(Procedure.ProcedurePerformerComponent element) 24776 throws IOException { 24777 composeBackbone(element); 24778 if (element.hasActor()) { 24779 composeReference("actor", element.getActor()); 24780 } 24781 if (element.hasRole()) { 24782 composeCodeableConcept("role", element.getRole()); 24783 } 24784 } 24785 24786 protected void composeProcedureProcedureFocalDeviceComponent(String name, 24787 Procedure.ProcedureFocalDeviceComponent element) throws IOException { 24788 if (element != null) { 24789 open(name); 24790 composeProcedureProcedureFocalDeviceComponentInner(element); 24791 close(); 24792 } 24793 } 24794 24795 protected void composeProcedureProcedureFocalDeviceComponentInner(Procedure.ProcedureFocalDeviceComponent element) 24796 throws IOException { 24797 composeBackbone(element); 24798 if (element.hasAction()) { 24799 composeCodeableConcept("action", element.getAction()); 24800 } 24801 if (element.hasManipulated()) { 24802 composeReference("manipulated", element.getManipulated()); 24803 } 24804 } 24805 24806 protected void composeProcedureRequest(String name, ProcedureRequest element) throws IOException { 24807 if (element != null) { 24808 prop("resourceType", name); 24809 composeProcedureRequestInner(element); 24810 } 24811 } 24812 24813 protected void composeProcedureRequestInner(ProcedureRequest element) throws IOException { 24814 composeDomainResourceElements(element); 24815 if (element.hasIdentifier()) { 24816 openArray("identifier"); 24817 for (Identifier e : element.getIdentifier()) 24818 composeIdentifier(null, e); 24819 closeArray(); 24820 } 24821 ; 24822 if (element.hasSubject()) { 24823 composeReference("subject", element.getSubject()); 24824 } 24825 if (element.hasCode()) { 24826 composeCodeableConcept("code", element.getCode()); 24827 } 24828 if (element.hasBodySite()) { 24829 openArray("bodySite"); 24830 for (CodeableConcept e : element.getBodySite()) 24831 composeCodeableConcept(null, e); 24832 closeArray(); 24833 } 24834 ; 24835 if (element.hasReason()) { 24836 composeType("reason", element.getReason()); 24837 } 24838 if (element.hasScheduled()) { 24839 composeType("scheduled", element.getScheduled()); 24840 } 24841 if (element.hasEncounter()) { 24842 composeReference("encounter", element.getEncounter()); 24843 } 24844 if (element.hasPerformer()) { 24845 composeReference("performer", element.getPerformer()); 24846 } 24847 if (element.hasStatusElement()) { 24848 composeEnumerationCore("status", element.getStatusElement(), 24849 new ProcedureRequest.ProcedureRequestStatusEnumFactory(), false); 24850 composeEnumerationExtras("status", element.getStatusElement(), 24851 new ProcedureRequest.ProcedureRequestStatusEnumFactory(), false); 24852 } 24853 if (element.hasNotes()) { 24854 openArray("notes"); 24855 for (Annotation e : element.getNotes()) 24856 composeAnnotation(null, e); 24857 closeArray(); 24858 } 24859 ; 24860 if (element.hasAsNeeded()) { 24861 composeType("asNeeded", element.getAsNeeded()); 24862 } 24863 if (element.hasOrderedOnElement()) { 24864 composeDateTimeCore("orderedOn", element.getOrderedOnElement(), false); 24865 composeDateTimeExtras("orderedOn", element.getOrderedOnElement(), false); 24866 } 24867 if (element.hasOrderer()) { 24868 composeReference("orderer", element.getOrderer()); 24869 } 24870 if (element.hasPriorityElement()) { 24871 composeEnumerationCore("priority", element.getPriorityElement(), 24872 new ProcedureRequest.ProcedureRequestPriorityEnumFactory(), false); 24873 composeEnumerationExtras("priority", element.getPriorityElement(), 24874 new ProcedureRequest.ProcedureRequestPriorityEnumFactory(), false); 24875 } 24876 } 24877 24878 protected void composeProcessRequest(String name, ProcessRequest element) throws IOException { 24879 if (element != null) { 24880 prop("resourceType", name); 24881 composeProcessRequestInner(element); 24882 } 24883 } 24884 24885 protected void composeProcessRequestInner(ProcessRequest element) throws IOException { 24886 composeDomainResourceElements(element); 24887 if (element.hasActionElement()) { 24888 composeEnumerationCore("action", element.getActionElement(), new ProcessRequest.ActionListEnumFactory(), false); 24889 composeEnumerationExtras("action", element.getActionElement(), new ProcessRequest.ActionListEnumFactory(), false); 24890 } 24891 if (element.hasIdentifier()) { 24892 openArray("identifier"); 24893 for (Identifier e : element.getIdentifier()) 24894 composeIdentifier(null, e); 24895 closeArray(); 24896 } 24897 ; 24898 if (element.hasRuleset()) { 24899 composeCoding("ruleset", element.getRuleset()); 24900 } 24901 if (element.hasOriginalRuleset()) { 24902 composeCoding("originalRuleset", element.getOriginalRuleset()); 24903 } 24904 if (element.hasCreatedElement()) { 24905 composeDateTimeCore("created", element.getCreatedElement(), false); 24906 composeDateTimeExtras("created", element.getCreatedElement(), false); 24907 } 24908 if (element.hasTarget()) { 24909 composeReference("target", element.getTarget()); 24910 } 24911 if (element.hasProvider()) { 24912 composeReference("provider", element.getProvider()); 24913 } 24914 if (element.hasOrganization()) { 24915 composeReference("organization", element.getOrganization()); 24916 } 24917 if (element.hasRequest()) { 24918 composeReference("request", element.getRequest()); 24919 } 24920 if (element.hasResponse()) { 24921 composeReference("response", element.getResponse()); 24922 } 24923 if (element.hasNullifyElement()) { 24924 composeBooleanCore("nullify", element.getNullifyElement(), false); 24925 composeBooleanExtras("nullify", element.getNullifyElement(), false); 24926 } 24927 if (element.hasReferenceElement()) { 24928 composeStringCore("reference", element.getReferenceElement(), false); 24929 composeStringExtras("reference", element.getReferenceElement(), false); 24930 } 24931 if (element.hasItem()) { 24932 openArray("item"); 24933 for (ProcessRequest.ItemsComponent e : element.getItem()) 24934 composeProcessRequestItemsComponent(null, e); 24935 closeArray(); 24936 } 24937 ; 24938 if (element.hasInclude()) { 24939 openArray("include"); 24940 for (StringType e : element.getInclude()) 24941 composeStringCore(null, e, true); 24942 closeArray(); 24943 if (anyHasExtras(element.getInclude())) { 24944 openArray("_include"); 24945 for (StringType e : element.getInclude()) 24946 composeStringExtras(null, e, true); 24947 closeArray(); 24948 } 24949 } 24950 ; 24951 if (element.hasExclude()) { 24952 openArray("exclude"); 24953 for (StringType e : element.getExclude()) 24954 composeStringCore(null, e, true); 24955 closeArray(); 24956 if (anyHasExtras(element.getExclude())) { 24957 openArray("_exclude"); 24958 for (StringType e : element.getExclude()) 24959 composeStringExtras(null, e, true); 24960 closeArray(); 24961 } 24962 } 24963 ; 24964 if (element.hasPeriod()) { 24965 composePeriod("period", element.getPeriod()); 24966 } 24967 } 24968 24969 protected void composeProcessRequestItemsComponent(String name, ProcessRequest.ItemsComponent element) 24970 throws IOException { 24971 if (element != null) { 24972 open(name); 24973 composeProcessRequestItemsComponentInner(element); 24974 close(); 24975 } 24976 } 24977 24978 protected void composeProcessRequestItemsComponentInner(ProcessRequest.ItemsComponent element) throws IOException { 24979 composeBackbone(element); 24980 if (element.hasSequenceLinkIdElement()) { 24981 composeIntegerCore("sequenceLinkId", element.getSequenceLinkIdElement(), false); 24982 composeIntegerExtras("sequenceLinkId", element.getSequenceLinkIdElement(), false); 24983 } 24984 } 24985 24986 protected void composeProcessResponse(String name, ProcessResponse element) throws IOException { 24987 if (element != null) { 24988 prop("resourceType", name); 24989 composeProcessResponseInner(element); 24990 } 24991 } 24992 24993 protected void composeProcessResponseInner(ProcessResponse element) throws IOException { 24994 composeDomainResourceElements(element); 24995 if (element.hasIdentifier()) { 24996 openArray("identifier"); 24997 for (Identifier e : element.getIdentifier()) 24998 composeIdentifier(null, e); 24999 closeArray(); 25000 } 25001 ; 25002 if (element.hasRequest()) { 25003 composeReference("request", element.getRequest()); 25004 } 25005 if (element.hasOutcome()) { 25006 composeCoding("outcome", element.getOutcome()); 25007 } 25008 if (element.hasDispositionElement()) { 25009 composeStringCore("disposition", element.getDispositionElement(), false); 25010 composeStringExtras("disposition", element.getDispositionElement(), false); 25011 } 25012 if (element.hasRuleset()) { 25013 composeCoding("ruleset", element.getRuleset()); 25014 } 25015 if (element.hasOriginalRuleset()) { 25016 composeCoding("originalRuleset", element.getOriginalRuleset()); 25017 } 25018 if (element.hasCreatedElement()) { 25019 composeDateTimeCore("created", element.getCreatedElement(), false); 25020 composeDateTimeExtras("created", element.getCreatedElement(), false); 25021 } 25022 if (element.hasOrganization()) { 25023 composeReference("organization", element.getOrganization()); 25024 } 25025 if (element.hasRequestProvider()) { 25026 composeReference("requestProvider", element.getRequestProvider()); 25027 } 25028 if (element.hasRequestOrganization()) { 25029 composeReference("requestOrganization", element.getRequestOrganization()); 25030 } 25031 if (element.hasForm()) { 25032 composeCoding("form", element.getForm()); 25033 } 25034 if (element.hasNotes()) { 25035 openArray("notes"); 25036 for (ProcessResponse.ProcessResponseNotesComponent e : element.getNotes()) 25037 composeProcessResponseProcessResponseNotesComponent(null, e); 25038 closeArray(); 25039 } 25040 ; 25041 if (element.hasError()) { 25042 openArray("error"); 25043 for (Coding e : element.getError()) 25044 composeCoding(null, e); 25045 closeArray(); 25046 } 25047 ; 25048 } 25049 25050 protected void composeProcessResponseProcessResponseNotesComponent(String name, 25051 ProcessResponse.ProcessResponseNotesComponent element) throws IOException { 25052 if (element != null) { 25053 open(name); 25054 composeProcessResponseProcessResponseNotesComponentInner(element); 25055 close(); 25056 } 25057 } 25058 25059 protected void composeProcessResponseProcessResponseNotesComponentInner( 25060 ProcessResponse.ProcessResponseNotesComponent element) throws IOException { 25061 composeBackbone(element); 25062 if (element.hasType()) { 25063 composeCoding("type", element.getType()); 25064 } 25065 if (element.hasTextElement()) { 25066 composeStringCore("text", element.getTextElement(), false); 25067 composeStringExtras("text", element.getTextElement(), false); 25068 } 25069 } 25070 25071 protected void composeProvenance(String name, Provenance element) throws IOException { 25072 if (element != null) { 25073 prop("resourceType", name); 25074 composeProvenanceInner(element); 25075 } 25076 } 25077 25078 protected void composeProvenanceInner(Provenance element) throws IOException { 25079 composeDomainResourceElements(element); 25080 if (element.hasTarget()) { 25081 openArray("target"); 25082 for (Reference e : element.getTarget()) 25083 composeReference(null, e); 25084 closeArray(); 25085 } 25086 ; 25087 if (element.hasPeriod()) { 25088 composePeriod("period", element.getPeriod()); 25089 } 25090 if (element.hasRecordedElement()) { 25091 composeInstantCore("recorded", element.getRecordedElement(), false); 25092 composeInstantExtras("recorded", element.getRecordedElement(), false); 25093 } 25094 if (element.hasReason()) { 25095 openArray("reason"); 25096 for (CodeableConcept e : element.getReason()) 25097 composeCodeableConcept(null, e); 25098 closeArray(); 25099 } 25100 ; 25101 if (element.hasActivity()) { 25102 composeCodeableConcept("activity", element.getActivity()); 25103 } 25104 if (element.hasLocation()) { 25105 composeReference("location", element.getLocation()); 25106 } 25107 if (element.hasPolicy()) { 25108 openArray("policy"); 25109 for (UriType e : element.getPolicy()) 25110 composeUriCore(null, e, true); 25111 closeArray(); 25112 if (anyHasExtras(element.getPolicy())) { 25113 openArray("_policy"); 25114 for (UriType e : element.getPolicy()) 25115 composeUriExtras(null, e, true); 25116 closeArray(); 25117 } 25118 } 25119 ; 25120 if (element.hasAgent()) { 25121 openArray("agent"); 25122 for (Provenance.ProvenanceAgentComponent e : element.getAgent()) 25123 composeProvenanceProvenanceAgentComponent(null, e); 25124 closeArray(); 25125 } 25126 ; 25127 if (element.hasEntity()) { 25128 openArray("entity"); 25129 for (Provenance.ProvenanceEntityComponent e : element.getEntity()) 25130 composeProvenanceProvenanceEntityComponent(null, e); 25131 closeArray(); 25132 } 25133 ; 25134 if (element.hasSignature()) { 25135 openArray("signature"); 25136 for (Signature e : element.getSignature()) 25137 composeSignature(null, e); 25138 closeArray(); 25139 } 25140 ; 25141 } 25142 25143 protected void composeProvenanceProvenanceAgentComponent(String name, Provenance.ProvenanceAgentComponent element) 25144 throws IOException { 25145 if (element != null) { 25146 open(name); 25147 composeProvenanceProvenanceAgentComponentInner(element); 25148 close(); 25149 } 25150 } 25151 25152 protected void composeProvenanceProvenanceAgentComponentInner(Provenance.ProvenanceAgentComponent element) 25153 throws IOException { 25154 composeBackbone(element); 25155 if (element.hasRole()) { 25156 composeCoding("role", element.getRole()); 25157 } 25158 if (element.hasActor()) { 25159 composeReference("actor", element.getActor()); 25160 } 25161 if (element.hasUserId()) { 25162 composeIdentifier("userId", element.getUserId()); 25163 } 25164 if (element.hasRelatedAgent()) { 25165 openArray("relatedAgent"); 25166 for (Provenance.ProvenanceAgentRelatedAgentComponent e : element.getRelatedAgent()) 25167 composeProvenanceProvenanceAgentRelatedAgentComponent(null, e); 25168 closeArray(); 25169 } 25170 ; 25171 } 25172 25173 protected void composeProvenanceProvenanceAgentRelatedAgentComponent(String name, 25174 Provenance.ProvenanceAgentRelatedAgentComponent element) throws IOException { 25175 if (element != null) { 25176 open(name); 25177 composeProvenanceProvenanceAgentRelatedAgentComponentInner(element); 25178 close(); 25179 } 25180 } 25181 25182 protected void composeProvenanceProvenanceAgentRelatedAgentComponentInner( 25183 Provenance.ProvenanceAgentRelatedAgentComponent element) throws IOException { 25184 composeBackbone(element); 25185 if (element.hasType()) { 25186 composeCodeableConcept("type", element.getType()); 25187 } 25188 if (element.hasTargetElement()) { 25189 composeUriCore("target", element.getTargetElement(), false); 25190 composeUriExtras("target", element.getTargetElement(), false); 25191 } 25192 } 25193 25194 protected void composeProvenanceProvenanceEntityComponent(String name, Provenance.ProvenanceEntityComponent element) 25195 throws IOException { 25196 if (element != null) { 25197 open(name); 25198 composeProvenanceProvenanceEntityComponentInner(element); 25199 close(); 25200 } 25201 } 25202 25203 protected void composeProvenanceProvenanceEntityComponentInner(Provenance.ProvenanceEntityComponent element) 25204 throws IOException { 25205 composeBackbone(element); 25206 if (element.hasRoleElement()) { 25207 composeEnumerationCore("role", element.getRoleElement(), new Provenance.ProvenanceEntityRoleEnumFactory(), false); 25208 composeEnumerationExtras("role", element.getRoleElement(), new Provenance.ProvenanceEntityRoleEnumFactory(), 25209 false); 25210 } 25211 if (element.hasType()) { 25212 composeCoding("type", element.getType()); 25213 } 25214 if (element.hasReferenceElement()) { 25215 composeUriCore("reference", element.getReferenceElement(), false); 25216 composeUriExtras("reference", element.getReferenceElement(), false); 25217 } 25218 if (element.hasDisplayElement()) { 25219 composeStringCore("display", element.getDisplayElement(), false); 25220 composeStringExtras("display", element.getDisplayElement(), false); 25221 } 25222 if (element.hasAgent()) { 25223 composeProvenanceProvenanceAgentComponent("agent", element.getAgent()); 25224 } 25225 } 25226 25227 protected void composeQuestionnaire(String name, Questionnaire element) throws IOException { 25228 if (element != null) { 25229 prop("resourceType", name); 25230 composeQuestionnaireInner(element); 25231 } 25232 } 25233 25234 protected void composeQuestionnaireInner(Questionnaire element) throws IOException { 25235 composeDomainResourceElements(element); 25236 if (element.hasIdentifier()) { 25237 openArray("identifier"); 25238 for (Identifier e : element.getIdentifier()) 25239 composeIdentifier(null, e); 25240 closeArray(); 25241 } 25242 ; 25243 if (element.hasVersionElement()) { 25244 composeStringCore("version", element.getVersionElement(), false); 25245 composeStringExtras("version", element.getVersionElement(), false); 25246 } 25247 if (element.hasStatusElement()) { 25248 composeEnumerationCore("status", element.getStatusElement(), new Questionnaire.QuestionnaireStatusEnumFactory(), 25249 false); 25250 composeEnumerationExtras("status", element.getStatusElement(), new Questionnaire.QuestionnaireStatusEnumFactory(), 25251 false); 25252 } 25253 if (element.hasDateElement()) { 25254 composeDateTimeCore("date", element.getDateElement(), false); 25255 composeDateTimeExtras("date", element.getDateElement(), false); 25256 } 25257 if (element.hasPublisherElement()) { 25258 composeStringCore("publisher", element.getPublisherElement(), false); 25259 composeStringExtras("publisher", element.getPublisherElement(), false); 25260 } 25261 if (element.hasTelecom()) { 25262 openArray("telecom"); 25263 for (ContactPoint e : element.getTelecom()) 25264 composeContactPoint(null, e); 25265 closeArray(); 25266 } 25267 ; 25268 if (element.hasSubjectType()) { 25269 openArray("subjectType"); 25270 for (CodeType e : element.getSubjectType()) 25271 composeCodeCore(null, e, true); 25272 closeArray(); 25273 if (anyHasExtras(element.getSubjectType())) { 25274 openArray("_subjectType"); 25275 for (CodeType e : element.getSubjectType()) 25276 composeCodeExtras(null, e, true); 25277 closeArray(); 25278 } 25279 } 25280 ; 25281 if (element.hasGroup()) { 25282 composeQuestionnaireGroupComponent("group", element.getGroup()); 25283 } 25284 } 25285 25286 protected void composeQuestionnaireGroupComponent(String name, Questionnaire.GroupComponent element) 25287 throws IOException { 25288 if (element != null) { 25289 open(name); 25290 composeQuestionnaireGroupComponentInner(element); 25291 close(); 25292 } 25293 } 25294 25295 protected void composeQuestionnaireGroupComponentInner(Questionnaire.GroupComponent element) throws IOException { 25296 composeBackbone(element); 25297 if (element.hasLinkIdElement()) { 25298 composeStringCore("linkId", element.getLinkIdElement(), false); 25299 composeStringExtras("linkId", element.getLinkIdElement(), false); 25300 } 25301 if (element.hasTitleElement()) { 25302 composeStringCore("title", element.getTitleElement(), false); 25303 composeStringExtras("title", element.getTitleElement(), false); 25304 } 25305 if (element.hasConcept()) { 25306 openArray("concept"); 25307 for (Coding e : element.getConcept()) 25308 composeCoding(null, e); 25309 closeArray(); 25310 } 25311 ; 25312 if (element.hasTextElement()) { 25313 composeStringCore("text", element.getTextElement(), false); 25314 composeStringExtras("text", element.getTextElement(), false); 25315 } 25316 if (element.hasRequiredElement()) { 25317 composeBooleanCore("required", element.getRequiredElement(), false); 25318 composeBooleanExtras("required", element.getRequiredElement(), false); 25319 } 25320 if (element.hasRepeatsElement()) { 25321 composeBooleanCore("repeats", element.getRepeatsElement(), false); 25322 composeBooleanExtras("repeats", element.getRepeatsElement(), false); 25323 } 25324 if (element.hasGroup()) { 25325 openArray("group"); 25326 for (Questionnaire.GroupComponent e : element.getGroup()) 25327 composeQuestionnaireGroupComponent(null, e); 25328 closeArray(); 25329 } 25330 ; 25331 if (element.hasQuestion()) { 25332 openArray("question"); 25333 for (Questionnaire.QuestionComponent e : element.getQuestion()) 25334 composeQuestionnaireQuestionComponent(null, e); 25335 closeArray(); 25336 } 25337 ; 25338 } 25339 25340 protected void composeQuestionnaireQuestionComponent(String name, Questionnaire.QuestionComponent element) 25341 throws IOException { 25342 if (element != null) { 25343 open(name); 25344 composeQuestionnaireQuestionComponentInner(element); 25345 close(); 25346 } 25347 } 25348 25349 protected void composeQuestionnaireQuestionComponentInner(Questionnaire.QuestionComponent element) 25350 throws IOException { 25351 composeBackbone(element); 25352 if (element.hasLinkIdElement()) { 25353 composeStringCore("linkId", element.getLinkIdElement(), false); 25354 composeStringExtras("linkId", element.getLinkIdElement(), false); 25355 } 25356 if (element.hasConcept()) { 25357 openArray("concept"); 25358 for (Coding e : element.getConcept()) 25359 composeCoding(null, e); 25360 closeArray(); 25361 } 25362 ; 25363 if (element.hasTextElement()) { 25364 composeStringCore("text", element.getTextElement(), false); 25365 composeStringExtras("text", element.getTextElement(), false); 25366 } 25367 if (element.hasTypeElement()) { 25368 composeEnumerationCore("type", element.getTypeElement(), new Questionnaire.AnswerFormatEnumFactory(), false); 25369 composeEnumerationExtras("type", element.getTypeElement(), new Questionnaire.AnswerFormatEnumFactory(), false); 25370 } 25371 if (element.hasRequiredElement()) { 25372 composeBooleanCore("required", element.getRequiredElement(), false); 25373 composeBooleanExtras("required", element.getRequiredElement(), false); 25374 } 25375 if (element.hasRepeatsElement()) { 25376 composeBooleanCore("repeats", element.getRepeatsElement(), false); 25377 composeBooleanExtras("repeats", element.getRepeatsElement(), false); 25378 } 25379 if (element.hasOptions()) { 25380 composeReference("options", element.getOptions()); 25381 } 25382 if (element.hasOption()) { 25383 openArray("option"); 25384 for (Coding e : element.getOption()) 25385 composeCoding(null, e); 25386 closeArray(); 25387 } 25388 ; 25389 if (element.hasGroup()) { 25390 openArray("group"); 25391 for (Questionnaire.GroupComponent e : element.getGroup()) 25392 composeQuestionnaireGroupComponent(null, e); 25393 closeArray(); 25394 } 25395 ; 25396 } 25397 25398 protected void composeQuestionnaireResponse(String name, QuestionnaireResponse element) throws IOException { 25399 if (element != null) { 25400 prop("resourceType", name); 25401 composeQuestionnaireResponseInner(element); 25402 } 25403 } 25404 25405 protected void composeQuestionnaireResponseInner(QuestionnaireResponse element) throws IOException { 25406 composeDomainResourceElements(element); 25407 if (element.hasIdentifier()) { 25408 composeIdentifier("identifier", element.getIdentifier()); 25409 } 25410 if (element.hasQuestionnaire()) { 25411 composeReference("questionnaire", element.getQuestionnaire()); 25412 } 25413 if (element.hasStatusElement()) { 25414 composeEnumerationCore("status", element.getStatusElement(), 25415 new QuestionnaireResponse.QuestionnaireResponseStatusEnumFactory(), false); 25416 composeEnumerationExtras("status", element.getStatusElement(), 25417 new QuestionnaireResponse.QuestionnaireResponseStatusEnumFactory(), false); 25418 } 25419 if (element.hasSubject()) { 25420 composeReference("subject", element.getSubject()); 25421 } 25422 if (element.hasAuthor()) { 25423 composeReference("author", element.getAuthor()); 25424 } 25425 if (element.hasAuthoredElement()) { 25426 composeDateTimeCore("authored", element.getAuthoredElement(), false); 25427 composeDateTimeExtras("authored", element.getAuthoredElement(), false); 25428 } 25429 if (element.hasSource()) { 25430 composeReference("source", element.getSource()); 25431 } 25432 if (element.hasEncounter()) { 25433 composeReference("encounter", element.getEncounter()); 25434 } 25435 if (element.hasGroup()) { 25436 composeQuestionnaireResponseGroupComponent("group", element.getGroup()); 25437 } 25438 } 25439 25440 protected void composeQuestionnaireResponseGroupComponent(String name, QuestionnaireResponse.GroupComponent element) 25441 throws IOException { 25442 if (element != null) { 25443 open(name); 25444 composeQuestionnaireResponseGroupComponentInner(element); 25445 close(); 25446 } 25447 } 25448 25449 protected void composeQuestionnaireResponseGroupComponentInner(QuestionnaireResponse.GroupComponent element) 25450 throws IOException { 25451 composeBackbone(element); 25452 if (element.hasLinkIdElement()) { 25453 composeStringCore("linkId", element.getLinkIdElement(), false); 25454 composeStringExtras("linkId", element.getLinkIdElement(), false); 25455 } 25456 if (element.hasTitleElement()) { 25457 composeStringCore("title", element.getTitleElement(), false); 25458 composeStringExtras("title", element.getTitleElement(), false); 25459 } 25460 if (element.hasTextElement()) { 25461 composeStringCore("text", element.getTextElement(), false); 25462 composeStringExtras("text", element.getTextElement(), false); 25463 } 25464 if (element.hasSubject()) { 25465 composeReference("subject", element.getSubject()); 25466 } 25467 if (element.hasGroup()) { 25468 openArray("group"); 25469 for (QuestionnaireResponse.GroupComponent e : element.getGroup()) 25470 composeQuestionnaireResponseGroupComponent(null, e); 25471 closeArray(); 25472 } 25473 ; 25474 if (element.hasQuestion()) { 25475 openArray("question"); 25476 for (QuestionnaireResponse.QuestionComponent e : element.getQuestion()) 25477 composeQuestionnaireResponseQuestionComponent(null, e); 25478 closeArray(); 25479 } 25480 ; 25481 } 25482 25483 protected void composeQuestionnaireResponseQuestionComponent(String name, 25484 QuestionnaireResponse.QuestionComponent element) throws IOException { 25485 if (element != null) { 25486 open(name); 25487 composeQuestionnaireResponseQuestionComponentInner(element); 25488 close(); 25489 } 25490 } 25491 25492 protected void composeQuestionnaireResponseQuestionComponentInner(QuestionnaireResponse.QuestionComponent element) 25493 throws IOException { 25494 composeBackbone(element); 25495 if (element.hasLinkIdElement()) { 25496 composeStringCore("linkId", element.getLinkIdElement(), false); 25497 composeStringExtras("linkId", element.getLinkIdElement(), false); 25498 } 25499 if (element.hasTextElement()) { 25500 composeStringCore("text", element.getTextElement(), false); 25501 composeStringExtras("text", element.getTextElement(), false); 25502 } 25503 if (element.hasAnswer()) { 25504 openArray("answer"); 25505 for (QuestionnaireResponse.QuestionAnswerComponent e : element.getAnswer()) 25506 composeQuestionnaireResponseQuestionAnswerComponent(null, e); 25507 closeArray(); 25508 } 25509 ; 25510 } 25511 25512 protected void composeQuestionnaireResponseQuestionAnswerComponent(String name, 25513 QuestionnaireResponse.QuestionAnswerComponent element) throws IOException { 25514 if (element != null) { 25515 open(name); 25516 composeQuestionnaireResponseQuestionAnswerComponentInner(element); 25517 close(); 25518 } 25519 } 25520 25521 protected void composeQuestionnaireResponseQuestionAnswerComponentInner( 25522 QuestionnaireResponse.QuestionAnswerComponent element) throws IOException { 25523 composeBackbone(element); 25524 if (element.hasValue()) { 25525 composeType("value", element.getValue()); 25526 } 25527 if (element.hasGroup()) { 25528 openArray("group"); 25529 for (QuestionnaireResponse.GroupComponent e : element.getGroup()) 25530 composeQuestionnaireResponseGroupComponent(null, e); 25531 closeArray(); 25532 } 25533 ; 25534 } 25535 25536 protected void composeReferralRequest(String name, ReferralRequest element) throws IOException { 25537 if (element != null) { 25538 prop("resourceType", name); 25539 composeReferralRequestInner(element); 25540 } 25541 } 25542 25543 protected void composeReferralRequestInner(ReferralRequest element) throws IOException { 25544 composeDomainResourceElements(element); 25545 if (element.hasStatusElement()) { 25546 composeEnumerationCore("status", element.getStatusElement(), new ReferralRequest.ReferralStatusEnumFactory(), 25547 false); 25548 composeEnumerationExtras("status", element.getStatusElement(), new ReferralRequest.ReferralStatusEnumFactory(), 25549 false); 25550 } 25551 if (element.hasIdentifier()) { 25552 openArray("identifier"); 25553 for (Identifier e : element.getIdentifier()) 25554 composeIdentifier(null, e); 25555 closeArray(); 25556 } 25557 ; 25558 if (element.hasDateElement()) { 25559 composeDateTimeCore("date", element.getDateElement(), false); 25560 composeDateTimeExtras("date", element.getDateElement(), false); 25561 } 25562 if (element.hasType()) { 25563 composeCodeableConcept("type", element.getType()); 25564 } 25565 if (element.hasSpecialty()) { 25566 composeCodeableConcept("specialty", element.getSpecialty()); 25567 } 25568 if (element.hasPriority()) { 25569 composeCodeableConcept("priority", element.getPriority()); 25570 } 25571 if (element.hasPatient()) { 25572 composeReference("patient", element.getPatient()); 25573 } 25574 if (element.hasRequester()) { 25575 composeReference("requester", element.getRequester()); 25576 } 25577 if (element.hasRecipient()) { 25578 openArray("recipient"); 25579 for (Reference e : element.getRecipient()) 25580 composeReference(null, e); 25581 closeArray(); 25582 } 25583 ; 25584 if (element.hasEncounter()) { 25585 composeReference("encounter", element.getEncounter()); 25586 } 25587 if (element.hasDateSentElement()) { 25588 composeDateTimeCore("dateSent", element.getDateSentElement(), false); 25589 composeDateTimeExtras("dateSent", element.getDateSentElement(), false); 25590 } 25591 if (element.hasReason()) { 25592 composeCodeableConcept("reason", element.getReason()); 25593 } 25594 if (element.hasDescriptionElement()) { 25595 composeStringCore("description", element.getDescriptionElement(), false); 25596 composeStringExtras("description", element.getDescriptionElement(), false); 25597 } 25598 if (element.hasServiceRequested()) { 25599 openArray("serviceRequested"); 25600 for (CodeableConcept e : element.getServiceRequested()) 25601 composeCodeableConcept(null, e); 25602 closeArray(); 25603 } 25604 ; 25605 if (element.hasSupportingInformation()) { 25606 openArray("supportingInformation"); 25607 for (Reference e : element.getSupportingInformation()) 25608 composeReference(null, e); 25609 closeArray(); 25610 } 25611 ; 25612 if (element.hasFulfillmentTime()) { 25613 composePeriod("fulfillmentTime", element.getFulfillmentTime()); 25614 } 25615 } 25616 25617 protected void composeRelatedPerson(String name, RelatedPerson element) throws IOException { 25618 if (element != null) { 25619 prop("resourceType", name); 25620 composeRelatedPersonInner(element); 25621 } 25622 } 25623 25624 protected void composeRelatedPersonInner(RelatedPerson element) throws IOException { 25625 composeDomainResourceElements(element); 25626 if (element.hasIdentifier()) { 25627 openArray("identifier"); 25628 for (Identifier e : element.getIdentifier()) 25629 composeIdentifier(null, e); 25630 closeArray(); 25631 } 25632 ; 25633 if (element.hasPatient()) { 25634 composeReference("patient", element.getPatient()); 25635 } 25636 if (element.hasRelationship()) { 25637 composeCodeableConcept("relationship", element.getRelationship()); 25638 } 25639 if (element.hasName()) { 25640 composeHumanName("name", element.getName()); 25641 } 25642 if (element.hasTelecom()) { 25643 openArray("telecom"); 25644 for (ContactPoint e : element.getTelecom()) 25645 composeContactPoint(null, e); 25646 closeArray(); 25647 } 25648 ; 25649 if (element.hasGenderElement()) { 25650 composeEnumerationCore("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), 25651 false); 25652 composeEnumerationExtras("gender", element.getGenderElement(), new Enumerations.AdministrativeGenderEnumFactory(), 25653 false); 25654 } 25655 if (element.hasBirthDateElement()) { 25656 composeDateCore("birthDate", element.getBirthDateElement(), false); 25657 composeDateExtras("birthDate", element.getBirthDateElement(), false); 25658 } 25659 if (element.hasAddress()) { 25660 openArray("address"); 25661 for (Address e : element.getAddress()) 25662 composeAddress(null, e); 25663 closeArray(); 25664 } 25665 ; 25666 if (element.hasPhoto()) { 25667 openArray("photo"); 25668 for (Attachment e : element.getPhoto()) 25669 composeAttachment(null, e); 25670 closeArray(); 25671 } 25672 ; 25673 if (element.hasPeriod()) { 25674 composePeriod("period", element.getPeriod()); 25675 } 25676 } 25677 25678 protected void composeRiskAssessment(String name, RiskAssessment element) throws IOException { 25679 if (element != null) { 25680 prop("resourceType", name); 25681 composeRiskAssessmentInner(element); 25682 } 25683 } 25684 25685 protected void composeRiskAssessmentInner(RiskAssessment element) throws IOException { 25686 composeDomainResourceElements(element); 25687 if (element.hasSubject()) { 25688 composeReference("subject", element.getSubject()); 25689 } 25690 if (element.hasDateElement()) { 25691 composeDateTimeCore("date", element.getDateElement(), false); 25692 composeDateTimeExtras("date", element.getDateElement(), false); 25693 } 25694 if (element.hasCondition()) { 25695 composeReference("condition", element.getCondition()); 25696 } 25697 if (element.hasEncounter()) { 25698 composeReference("encounter", element.getEncounter()); 25699 } 25700 if (element.hasPerformer()) { 25701 composeReference("performer", element.getPerformer()); 25702 } 25703 if (element.hasIdentifier()) { 25704 composeIdentifier("identifier", element.getIdentifier()); 25705 } 25706 if (element.hasMethod()) { 25707 composeCodeableConcept("method", element.getMethod()); 25708 } 25709 if (element.hasBasis()) { 25710 openArray("basis"); 25711 for (Reference e : element.getBasis()) 25712 composeReference(null, e); 25713 closeArray(); 25714 } 25715 ; 25716 if (element.hasPrediction()) { 25717 openArray("prediction"); 25718 for (RiskAssessment.RiskAssessmentPredictionComponent e : element.getPrediction()) 25719 composeRiskAssessmentRiskAssessmentPredictionComponent(null, e); 25720 closeArray(); 25721 } 25722 ; 25723 if (element.hasMitigationElement()) { 25724 composeStringCore("mitigation", element.getMitigationElement(), false); 25725 composeStringExtras("mitigation", element.getMitigationElement(), false); 25726 } 25727 } 25728 25729 protected void composeRiskAssessmentRiskAssessmentPredictionComponent(String name, 25730 RiskAssessment.RiskAssessmentPredictionComponent element) throws IOException { 25731 if (element != null) { 25732 open(name); 25733 composeRiskAssessmentRiskAssessmentPredictionComponentInner(element); 25734 close(); 25735 } 25736 } 25737 25738 protected void composeRiskAssessmentRiskAssessmentPredictionComponentInner( 25739 RiskAssessment.RiskAssessmentPredictionComponent element) throws IOException { 25740 composeBackbone(element); 25741 if (element.hasOutcome()) { 25742 composeCodeableConcept("outcome", element.getOutcome()); 25743 } 25744 if (element.hasProbability()) { 25745 composeType("probability", element.getProbability()); 25746 } 25747 if (element.hasRelativeRiskElement()) { 25748 composeDecimalCore("relativeRisk", element.getRelativeRiskElement(), false); 25749 composeDecimalExtras("relativeRisk", element.getRelativeRiskElement(), false); 25750 } 25751 if (element.hasWhen()) { 25752 composeType("when", element.getWhen()); 25753 } 25754 if (element.hasRationaleElement()) { 25755 composeStringCore("rationale", element.getRationaleElement(), false); 25756 composeStringExtras("rationale", element.getRationaleElement(), false); 25757 } 25758 } 25759 25760 protected void composeSchedule(String name, Schedule element) throws IOException { 25761 if (element != null) { 25762 prop("resourceType", name); 25763 composeScheduleInner(element); 25764 } 25765 } 25766 25767 protected void composeScheduleInner(Schedule element) throws IOException { 25768 composeDomainResourceElements(element); 25769 if (element.hasIdentifier()) { 25770 openArray("identifier"); 25771 for (Identifier e : element.getIdentifier()) 25772 composeIdentifier(null, e); 25773 closeArray(); 25774 } 25775 ; 25776 if (element.hasType()) { 25777 openArray("type"); 25778 for (CodeableConcept e : element.getType()) 25779 composeCodeableConcept(null, e); 25780 closeArray(); 25781 } 25782 ; 25783 if (element.hasActor()) { 25784 composeReference("actor", element.getActor()); 25785 } 25786 if (element.hasPlanningHorizon()) { 25787 composePeriod("planningHorizon", element.getPlanningHorizon()); 25788 } 25789 if (element.hasCommentElement()) { 25790 composeStringCore("comment", element.getCommentElement(), false); 25791 composeStringExtras("comment", element.getCommentElement(), false); 25792 } 25793 } 25794 25795 protected void composeSearchParameter(String name, SearchParameter element) throws IOException { 25796 if (element != null) { 25797 prop("resourceType", name); 25798 composeSearchParameterInner(element); 25799 } 25800 } 25801 25802 protected void composeSearchParameterInner(SearchParameter element) throws IOException { 25803 composeDomainResourceElements(element); 25804 if (element.hasUrlElement()) { 25805 composeUriCore("url", element.getUrlElement(), false); 25806 composeUriExtras("url", element.getUrlElement(), false); 25807 } 25808 if (element.hasNameElement()) { 25809 composeStringCore("name", element.getNameElement(), false); 25810 composeStringExtras("name", element.getNameElement(), false); 25811 } 25812 if (element.hasStatusElement()) { 25813 composeEnumerationCore("status", element.getStatusElement(), 25814 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 25815 composeEnumerationExtras("status", element.getStatusElement(), 25816 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 25817 } 25818 if (element.hasExperimentalElement()) { 25819 composeBooleanCore("experimental", element.getExperimentalElement(), false); 25820 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 25821 } 25822 if (element.hasPublisherElement()) { 25823 composeStringCore("publisher", element.getPublisherElement(), false); 25824 composeStringExtras("publisher", element.getPublisherElement(), false); 25825 } 25826 if (element.hasContact()) { 25827 openArray("contact"); 25828 for (SearchParameter.SearchParameterContactComponent e : element.getContact()) 25829 composeSearchParameterSearchParameterContactComponent(null, e); 25830 closeArray(); 25831 } 25832 ; 25833 if (element.hasDateElement()) { 25834 composeDateTimeCore("date", element.getDateElement(), false); 25835 composeDateTimeExtras("date", element.getDateElement(), false); 25836 } 25837 if (element.hasRequirementsElement()) { 25838 composeStringCore("requirements", element.getRequirementsElement(), false); 25839 composeStringExtras("requirements", element.getRequirementsElement(), false); 25840 } 25841 if (element.hasCodeElement()) { 25842 composeCodeCore("code", element.getCodeElement(), false); 25843 composeCodeExtras("code", element.getCodeElement(), false); 25844 } 25845 if (element.hasBaseElement()) { 25846 composeCodeCore("base", element.getBaseElement(), false); 25847 composeCodeExtras("base", element.getBaseElement(), false); 25848 } 25849 if (element.hasTypeElement()) { 25850 composeEnumerationCore("type", element.getTypeElement(), new Enumerations.SearchParamTypeEnumFactory(), false); 25851 composeEnumerationExtras("type", element.getTypeElement(), new Enumerations.SearchParamTypeEnumFactory(), false); 25852 } 25853 if (element.hasDescriptionElement()) { 25854 composeStringCore("description", element.getDescriptionElement(), false); 25855 composeStringExtras("description", element.getDescriptionElement(), false); 25856 } 25857 if (element.hasXpathElement()) { 25858 composeStringCore("xpath", element.getXpathElement(), false); 25859 composeStringExtras("xpath", element.getXpathElement(), false); 25860 } 25861 if (element.hasXpathUsageElement()) { 25862 composeEnumerationCore("xpathUsage", element.getXpathUsageElement(), 25863 new SearchParameter.XPathUsageTypeEnumFactory(), false); 25864 composeEnumerationExtras("xpathUsage", element.getXpathUsageElement(), 25865 new SearchParameter.XPathUsageTypeEnumFactory(), false); 25866 } 25867 if (element.hasTarget()) { 25868 openArray("target"); 25869 for (CodeType e : element.getTarget()) 25870 composeCodeCore(null, e, true); 25871 closeArray(); 25872 if (anyHasExtras(element.getTarget())) { 25873 openArray("_target"); 25874 for (CodeType e : element.getTarget()) 25875 composeCodeExtras(null, e, true); 25876 closeArray(); 25877 } 25878 } 25879 ; 25880 } 25881 25882 protected void composeSearchParameterSearchParameterContactComponent(String name, 25883 SearchParameter.SearchParameterContactComponent element) throws IOException { 25884 if (element != null) { 25885 open(name); 25886 composeSearchParameterSearchParameterContactComponentInner(element); 25887 close(); 25888 } 25889 } 25890 25891 protected void composeSearchParameterSearchParameterContactComponentInner( 25892 SearchParameter.SearchParameterContactComponent element) throws IOException { 25893 composeBackbone(element); 25894 if (element.hasNameElement()) { 25895 composeStringCore("name", element.getNameElement(), false); 25896 composeStringExtras("name", element.getNameElement(), false); 25897 } 25898 if (element.hasTelecom()) { 25899 openArray("telecom"); 25900 for (ContactPoint e : element.getTelecom()) 25901 composeContactPoint(null, e); 25902 closeArray(); 25903 } 25904 ; 25905 } 25906 25907 protected void composeSlot(String name, Slot element) throws IOException { 25908 if (element != null) { 25909 prop("resourceType", name); 25910 composeSlotInner(element); 25911 } 25912 } 25913 25914 protected void composeSlotInner(Slot element) throws IOException { 25915 composeDomainResourceElements(element); 25916 if (element.hasIdentifier()) { 25917 openArray("identifier"); 25918 for (Identifier e : element.getIdentifier()) 25919 composeIdentifier(null, e); 25920 closeArray(); 25921 } 25922 ; 25923 if (element.hasType()) { 25924 composeCodeableConcept("type", element.getType()); 25925 } 25926 if (element.hasSchedule()) { 25927 composeReference("schedule", element.getSchedule()); 25928 } 25929 if (element.hasFreeBusyTypeElement()) { 25930 composeEnumerationCore("freeBusyType", element.getFreeBusyTypeElement(), new Slot.SlotStatusEnumFactory(), false); 25931 composeEnumerationExtras("freeBusyType", element.getFreeBusyTypeElement(), new Slot.SlotStatusEnumFactory(), 25932 false); 25933 } 25934 if (element.hasStartElement()) { 25935 composeInstantCore("start", element.getStartElement(), false); 25936 composeInstantExtras("start", element.getStartElement(), false); 25937 } 25938 if (element.hasEndElement()) { 25939 composeInstantCore("end", element.getEndElement(), false); 25940 composeInstantExtras("end", element.getEndElement(), false); 25941 } 25942 if (element.hasOverbookedElement()) { 25943 composeBooleanCore("overbooked", element.getOverbookedElement(), false); 25944 composeBooleanExtras("overbooked", element.getOverbookedElement(), false); 25945 } 25946 if (element.hasCommentElement()) { 25947 composeStringCore("comment", element.getCommentElement(), false); 25948 composeStringExtras("comment", element.getCommentElement(), false); 25949 } 25950 } 25951 25952 protected void composeSpecimen(String name, Specimen element) throws IOException { 25953 if (element != null) { 25954 prop("resourceType", name); 25955 composeSpecimenInner(element); 25956 } 25957 } 25958 25959 protected void composeSpecimenInner(Specimen element) throws IOException { 25960 composeDomainResourceElements(element); 25961 if (element.hasIdentifier()) { 25962 openArray("identifier"); 25963 for (Identifier e : element.getIdentifier()) 25964 composeIdentifier(null, e); 25965 closeArray(); 25966 } 25967 ; 25968 if (element.hasStatusElement()) { 25969 composeEnumerationCore("status", element.getStatusElement(), new Specimen.SpecimenStatusEnumFactory(), false); 25970 composeEnumerationExtras("status", element.getStatusElement(), new Specimen.SpecimenStatusEnumFactory(), false); 25971 } 25972 if (element.hasType()) { 25973 composeCodeableConcept("type", element.getType()); 25974 } 25975 if (element.hasParent()) { 25976 openArray("parent"); 25977 for (Reference e : element.getParent()) 25978 composeReference(null, e); 25979 closeArray(); 25980 } 25981 ; 25982 if (element.hasSubject()) { 25983 composeReference("subject", element.getSubject()); 25984 } 25985 if (element.hasAccessionIdentifier()) { 25986 composeIdentifier("accessionIdentifier", element.getAccessionIdentifier()); 25987 } 25988 if (element.hasReceivedTimeElement()) { 25989 composeDateTimeCore("receivedTime", element.getReceivedTimeElement(), false); 25990 composeDateTimeExtras("receivedTime", element.getReceivedTimeElement(), false); 25991 } 25992 if (element.hasCollection()) { 25993 composeSpecimenSpecimenCollectionComponent("collection", element.getCollection()); 25994 } 25995 if (element.hasTreatment()) { 25996 openArray("treatment"); 25997 for (Specimen.SpecimenTreatmentComponent e : element.getTreatment()) 25998 composeSpecimenSpecimenTreatmentComponent(null, e); 25999 closeArray(); 26000 } 26001 ; 26002 if (element.hasContainer()) { 26003 openArray("container"); 26004 for (Specimen.SpecimenContainerComponent e : element.getContainer()) 26005 composeSpecimenSpecimenContainerComponent(null, e); 26006 closeArray(); 26007 } 26008 ; 26009 } 26010 26011 protected void composeSpecimenSpecimenCollectionComponent(String name, Specimen.SpecimenCollectionComponent element) 26012 throws IOException { 26013 if (element != null) { 26014 open(name); 26015 composeSpecimenSpecimenCollectionComponentInner(element); 26016 close(); 26017 } 26018 } 26019 26020 protected void composeSpecimenSpecimenCollectionComponentInner(Specimen.SpecimenCollectionComponent element) 26021 throws IOException { 26022 composeBackbone(element); 26023 if (element.hasCollector()) { 26024 composeReference("collector", element.getCollector()); 26025 } 26026 if (element.hasComment()) { 26027 openArray("comment"); 26028 for (StringType e : element.getComment()) 26029 composeStringCore(null, e, true); 26030 closeArray(); 26031 if (anyHasExtras(element.getComment())) { 26032 openArray("_comment"); 26033 for (StringType e : element.getComment()) 26034 composeStringExtras(null, e, true); 26035 closeArray(); 26036 } 26037 } 26038 ; 26039 if (element.hasCollected()) { 26040 composeType("collected", element.getCollected()); 26041 } 26042 if (element.hasQuantity()) { 26043 composeSimpleQuantity("quantity", element.getQuantity()); 26044 } 26045 if (element.hasMethod()) { 26046 composeCodeableConcept("method", element.getMethod()); 26047 } 26048 if (element.hasBodySite()) { 26049 composeCodeableConcept("bodySite", element.getBodySite()); 26050 } 26051 } 26052 26053 protected void composeSpecimenSpecimenTreatmentComponent(String name, Specimen.SpecimenTreatmentComponent element) 26054 throws IOException { 26055 if (element != null) { 26056 open(name); 26057 composeSpecimenSpecimenTreatmentComponentInner(element); 26058 close(); 26059 } 26060 } 26061 26062 protected void composeSpecimenSpecimenTreatmentComponentInner(Specimen.SpecimenTreatmentComponent element) 26063 throws IOException { 26064 composeBackbone(element); 26065 if (element.hasDescriptionElement()) { 26066 composeStringCore("description", element.getDescriptionElement(), false); 26067 composeStringExtras("description", element.getDescriptionElement(), false); 26068 } 26069 if (element.hasProcedure()) { 26070 composeCodeableConcept("procedure", element.getProcedure()); 26071 } 26072 if (element.hasAdditive()) { 26073 openArray("additive"); 26074 for (Reference e : element.getAdditive()) 26075 composeReference(null, e); 26076 closeArray(); 26077 } 26078 ; 26079 } 26080 26081 protected void composeSpecimenSpecimenContainerComponent(String name, Specimen.SpecimenContainerComponent element) 26082 throws IOException { 26083 if (element != null) { 26084 open(name); 26085 composeSpecimenSpecimenContainerComponentInner(element); 26086 close(); 26087 } 26088 } 26089 26090 protected void composeSpecimenSpecimenContainerComponentInner(Specimen.SpecimenContainerComponent element) 26091 throws IOException { 26092 composeBackbone(element); 26093 if (element.hasIdentifier()) { 26094 openArray("identifier"); 26095 for (Identifier e : element.getIdentifier()) 26096 composeIdentifier(null, e); 26097 closeArray(); 26098 } 26099 ; 26100 if (element.hasDescriptionElement()) { 26101 composeStringCore("description", element.getDescriptionElement(), false); 26102 composeStringExtras("description", element.getDescriptionElement(), false); 26103 } 26104 if (element.hasType()) { 26105 composeCodeableConcept("type", element.getType()); 26106 } 26107 if (element.hasCapacity()) { 26108 composeSimpleQuantity("capacity", element.getCapacity()); 26109 } 26110 if (element.hasSpecimenQuantity()) { 26111 composeSimpleQuantity("specimenQuantity", element.getSpecimenQuantity()); 26112 } 26113 if (element.hasAdditive()) { 26114 composeType("additive", element.getAdditive()); 26115 } 26116 } 26117 26118 protected void composeStructureDefinition(String name, StructureDefinition element) throws IOException { 26119 if (element != null) { 26120 prop("resourceType", name); 26121 composeStructureDefinitionInner(element); 26122 } 26123 } 26124 26125 protected void composeStructureDefinitionInner(StructureDefinition element) throws IOException { 26126 composeDomainResourceElements(element); 26127 if (element.hasUrlElement()) { 26128 composeUriCore("url", element.getUrlElement(), false); 26129 composeUriExtras("url", element.getUrlElement(), false); 26130 } 26131 if (element.hasIdentifier()) { 26132 openArray("identifier"); 26133 for (Identifier e : element.getIdentifier()) 26134 composeIdentifier(null, e); 26135 closeArray(); 26136 } 26137 ; 26138 if (element.hasVersionElement()) { 26139 composeStringCore("version", element.getVersionElement(), false); 26140 composeStringExtras("version", element.getVersionElement(), false); 26141 } 26142 if (element.hasNameElement()) { 26143 composeStringCore("name", element.getNameElement(), false); 26144 composeStringExtras("name", element.getNameElement(), false); 26145 } 26146 if (element.hasDisplayElement()) { 26147 composeStringCore("display", element.getDisplayElement(), false); 26148 composeStringExtras("display", element.getDisplayElement(), false); 26149 } 26150 if (element.hasStatusElement()) { 26151 composeEnumerationCore("status", element.getStatusElement(), 26152 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 26153 composeEnumerationExtras("status", element.getStatusElement(), 26154 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 26155 } 26156 if (element.hasExperimentalElement()) { 26157 composeBooleanCore("experimental", element.getExperimentalElement(), false); 26158 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 26159 } 26160 if (element.hasPublisherElement()) { 26161 composeStringCore("publisher", element.getPublisherElement(), false); 26162 composeStringExtras("publisher", element.getPublisherElement(), false); 26163 } 26164 if (element.hasContact()) { 26165 openArray("contact"); 26166 for (StructureDefinition.StructureDefinitionContactComponent e : element.getContact()) 26167 composeStructureDefinitionStructureDefinitionContactComponent(null, e); 26168 closeArray(); 26169 } 26170 ; 26171 if (element.hasDateElement()) { 26172 composeDateTimeCore("date", element.getDateElement(), false); 26173 composeDateTimeExtras("date", element.getDateElement(), false); 26174 } 26175 if (element.hasDescriptionElement()) { 26176 composeStringCore("description", element.getDescriptionElement(), false); 26177 composeStringExtras("description", element.getDescriptionElement(), false); 26178 } 26179 if (element.hasUseContext()) { 26180 openArray("useContext"); 26181 for (CodeableConcept e : element.getUseContext()) 26182 composeCodeableConcept(null, e); 26183 closeArray(); 26184 } 26185 ; 26186 if (element.hasRequirementsElement()) { 26187 composeStringCore("requirements", element.getRequirementsElement(), false); 26188 composeStringExtras("requirements", element.getRequirementsElement(), false); 26189 } 26190 if (element.hasCopyrightElement()) { 26191 composeStringCore("copyright", element.getCopyrightElement(), false); 26192 composeStringExtras("copyright", element.getCopyrightElement(), false); 26193 } 26194 if (element.hasCode()) { 26195 openArray("code"); 26196 for (Coding e : element.getCode()) 26197 composeCoding(null, e); 26198 closeArray(); 26199 } 26200 ; 26201 if (element.hasFhirVersionElement()) { 26202 composeIdCore("fhirVersion", element.getFhirVersionElement(), false); 26203 composeIdExtras("fhirVersion", element.getFhirVersionElement(), false); 26204 } 26205 if (element.hasMapping()) { 26206 openArray("mapping"); 26207 for (StructureDefinition.StructureDefinitionMappingComponent e : element.getMapping()) 26208 composeStructureDefinitionStructureDefinitionMappingComponent(null, e); 26209 closeArray(); 26210 } 26211 ; 26212 if (element.hasKindElement()) { 26213 composeEnumerationCore("kind", element.getKindElement(), 26214 new StructureDefinition.StructureDefinitionKindEnumFactory(), false); 26215 composeEnumerationExtras("kind", element.getKindElement(), 26216 new StructureDefinition.StructureDefinitionKindEnumFactory(), false); 26217 } 26218 if (element.hasConstrainedTypeElement()) { 26219 composeCodeCore("constrainedType", element.getConstrainedTypeElement(), false); 26220 composeCodeExtras("constrainedType", element.getConstrainedTypeElement(), false); 26221 } 26222 if (element.hasAbstractElement()) { 26223 composeBooleanCore("abstract", element.getAbstractElement(), false); 26224 composeBooleanExtras("abstract", element.getAbstractElement(), false); 26225 } 26226 if (element.hasContextTypeElement()) { 26227 composeEnumerationCore("contextType", element.getContextTypeElement(), 26228 new StructureDefinition.ExtensionContextEnumFactory(), false); 26229 composeEnumerationExtras("contextType", element.getContextTypeElement(), 26230 new StructureDefinition.ExtensionContextEnumFactory(), false); 26231 } 26232 if (element.hasContext()) { 26233 openArray("context"); 26234 for (StringType e : element.getContext()) 26235 composeStringCore(null, e, true); 26236 closeArray(); 26237 if (anyHasExtras(element.getContext())) { 26238 openArray("_context"); 26239 for (StringType e : element.getContext()) 26240 composeStringExtras(null, e, true); 26241 closeArray(); 26242 } 26243 } 26244 ; 26245 if (element.hasBaseElement()) { 26246 composeUriCore("base", element.getBaseElement(), false); 26247 composeUriExtras("base", element.getBaseElement(), false); 26248 } 26249 if (element.hasSnapshot()) { 26250 composeStructureDefinitionStructureDefinitionSnapshotComponent("snapshot", element.getSnapshot()); 26251 } 26252 if (element.hasDifferential()) { 26253 composeStructureDefinitionStructureDefinitionDifferentialComponent("differential", element.getDifferential()); 26254 } 26255 } 26256 26257 protected void composeStructureDefinitionStructureDefinitionContactComponent(String name, 26258 StructureDefinition.StructureDefinitionContactComponent element) throws IOException { 26259 if (element != null) { 26260 open(name); 26261 composeStructureDefinitionStructureDefinitionContactComponentInner(element); 26262 close(); 26263 } 26264 } 26265 26266 protected void composeStructureDefinitionStructureDefinitionContactComponentInner( 26267 StructureDefinition.StructureDefinitionContactComponent element) throws IOException { 26268 composeBackbone(element); 26269 if (element.hasNameElement()) { 26270 composeStringCore("name", element.getNameElement(), false); 26271 composeStringExtras("name", element.getNameElement(), false); 26272 } 26273 if (element.hasTelecom()) { 26274 openArray("telecom"); 26275 for (ContactPoint e : element.getTelecom()) 26276 composeContactPoint(null, e); 26277 closeArray(); 26278 } 26279 ; 26280 } 26281 26282 protected void composeStructureDefinitionStructureDefinitionMappingComponent(String name, 26283 StructureDefinition.StructureDefinitionMappingComponent element) throws IOException { 26284 if (element != null) { 26285 open(name); 26286 composeStructureDefinitionStructureDefinitionMappingComponentInner(element); 26287 close(); 26288 } 26289 } 26290 26291 protected void composeStructureDefinitionStructureDefinitionMappingComponentInner( 26292 StructureDefinition.StructureDefinitionMappingComponent element) throws IOException { 26293 composeBackbone(element); 26294 if (element.hasIdentityElement()) { 26295 composeIdCore("identity", element.getIdentityElement(), false); 26296 composeIdExtras("identity", element.getIdentityElement(), false); 26297 } 26298 if (element.hasUriElement()) { 26299 composeUriCore("uri", element.getUriElement(), false); 26300 composeUriExtras("uri", element.getUriElement(), false); 26301 } 26302 if (element.hasNameElement()) { 26303 composeStringCore("name", element.getNameElement(), false); 26304 composeStringExtras("name", element.getNameElement(), false); 26305 } 26306 if (element.hasCommentsElement()) { 26307 composeStringCore("comments", element.getCommentsElement(), false); 26308 composeStringExtras("comments", element.getCommentsElement(), false); 26309 } 26310 } 26311 26312 protected void composeStructureDefinitionStructureDefinitionSnapshotComponent(String name, 26313 StructureDefinition.StructureDefinitionSnapshotComponent element) throws IOException { 26314 if (element != null) { 26315 open(name); 26316 composeStructureDefinitionStructureDefinitionSnapshotComponentInner(element); 26317 close(); 26318 } 26319 } 26320 26321 protected void composeStructureDefinitionStructureDefinitionSnapshotComponentInner( 26322 StructureDefinition.StructureDefinitionSnapshotComponent element) throws IOException { 26323 composeBackbone(element); 26324 if (element.hasElement()) { 26325 openArray("element"); 26326 for (ElementDefinition e : element.getElement()) 26327 composeElementDefinition(null, e); 26328 closeArray(); 26329 } 26330 ; 26331 } 26332 26333 protected void composeStructureDefinitionStructureDefinitionDifferentialComponent(String name, 26334 StructureDefinition.StructureDefinitionDifferentialComponent element) throws IOException { 26335 if (element != null) { 26336 open(name); 26337 composeStructureDefinitionStructureDefinitionDifferentialComponentInner(element); 26338 close(); 26339 } 26340 } 26341 26342 protected void composeStructureDefinitionStructureDefinitionDifferentialComponentInner( 26343 StructureDefinition.StructureDefinitionDifferentialComponent element) throws IOException { 26344 composeBackbone(element); 26345 if (element.hasElement()) { 26346 openArray("element"); 26347 for (ElementDefinition e : element.getElement()) 26348 composeElementDefinition(null, e); 26349 closeArray(); 26350 } 26351 ; 26352 } 26353 26354 protected void composeSubscription(String name, Subscription element) throws IOException { 26355 if (element != null) { 26356 prop("resourceType", name); 26357 composeSubscriptionInner(element); 26358 } 26359 } 26360 26361 protected void composeSubscriptionInner(Subscription element) throws IOException { 26362 composeDomainResourceElements(element); 26363 if (element.hasCriteriaElement()) { 26364 composeStringCore("criteria", element.getCriteriaElement(), false); 26365 composeStringExtras("criteria", element.getCriteriaElement(), false); 26366 } 26367 if (element.hasContact()) { 26368 openArray("contact"); 26369 for (ContactPoint e : element.getContact()) 26370 composeContactPoint(null, e); 26371 closeArray(); 26372 } 26373 ; 26374 if (element.hasReasonElement()) { 26375 composeStringCore("reason", element.getReasonElement(), false); 26376 composeStringExtras("reason", element.getReasonElement(), false); 26377 } 26378 if (element.hasStatusElement()) { 26379 composeEnumerationCore("status", element.getStatusElement(), new Subscription.SubscriptionStatusEnumFactory(), 26380 false); 26381 composeEnumerationExtras("status", element.getStatusElement(), new Subscription.SubscriptionStatusEnumFactory(), 26382 false); 26383 } 26384 if (element.hasErrorElement()) { 26385 composeStringCore("error", element.getErrorElement(), false); 26386 composeStringExtras("error", element.getErrorElement(), false); 26387 } 26388 if (element.hasChannel()) { 26389 composeSubscriptionSubscriptionChannelComponent("channel", element.getChannel()); 26390 } 26391 if (element.hasEndElement()) { 26392 composeInstantCore("end", element.getEndElement(), false); 26393 composeInstantExtras("end", element.getEndElement(), false); 26394 } 26395 if (element.hasTag()) { 26396 openArray("tag"); 26397 for (Coding e : element.getTag()) 26398 composeCoding(null, e); 26399 closeArray(); 26400 } 26401 ; 26402 } 26403 26404 protected void composeSubscriptionSubscriptionChannelComponent(String name, 26405 Subscription.SubscriptionChannelComponent element) throws IOException { 26406 if (element != null) { 26407 open(name); 26408 composeSubscriptionSubscriptionChannelComponentInner(element); 26409 close(); 26410 } 26411 } 26412 26413 protected void composeSubscriptionSubscriptionChannelComponentInner(Subscription.SubscriptionChannelComponent element) 26414 throws IOException { 26415 composeBackbone(element); 26416 if (element.hasTypeElement()) { 26417 composeEnumerationCore("type", element.getTypeElement(), new Subscription.SubscriptionChannelTypeEnumFactory(), 26418 false); 26419 composeEnumerationExtras("type", element.getTypeElement(), new Subscription.SubscriptionChannelTypeEnumFactory(), 26420 false); 26421 } 26422 if (element.hasEndpointElement()) { 26423 composeUriCore("endpoint", element.getEndpointElement(), false); 26424 composeUriExtras("endpoint", element.getEndpointElement(), false); 26425 } 26426 if (element.hasPayloadElement()) { 26427 composeStringCore("payload", element.getPayloadElement(), false); 26428 composeStringExtras("payload", element.getPayloadElement(), false); 26429 } 26430 if (element.hasHeaderElement()) { 26431 composeStringCore("header", element.getHeaderElement(), false); 26432 composeStringExtras("header", element.getHeaderElement(), false); 26433 } 26434 } 26435 26436 protected void composeSubstance(String name, Substance element) throws IOException { 26437 if (element != null) { 26438 prop("resourceType", name); 26439 composeSubstanceInner(element); 26440 } 26441 } 26442 26443 protected void composeSubstanceInner(Substance element) throws IOException { 26444 composeDomainResourceElements(element); 26445 if (element.hasIdentifier()) { 26446 openArray("identifier"); 26447 for (Identifier e : element.getIdentifier()) 26448 composeIdentifier(null, e); 26449 closeArray(); 26450 } 26451 ; 26452 if (element.hasCategory()) { 26453 openArray("category"); 26454 for (CodeableConcept e : element.getCategory()) 26455 composeCodeableConcept(null, e); 26456 closeArray(); 26457 } 26458 ; 26459 if (element.hasCode()) { 26460 composeCodeableConcept("code", element.getCode()); 26461 } 26462 if (element.hasDescriptionElement()) { 26463 composeStringCore("description", element.getDescriptionElement(), false); 26464 composeStringExtras("description", element.getDescriptionElement(), false); 26465 } 26466 if (element.hasInstance()) { 26467 openArray("instance"); 26468 for (Substance.SubstanceInstanceComponent e : element.getInstance()) 26469 composeSubstanceSubstanceInstanceComponent(null, e); 26470 closeArray(); 26471 } 26472 ; 26473 if (element.hasIngredient()) { 26474 openArray("ingredient"); 26475 for (Substance.SubstanceIngredientComponent e : element.getIngredient()) 26476 composeSubstanceSubstanceIngredientComponent(null, e); 26477 closeArray(); 26478 } 26479 ; 26480 } 26481 26482 protected void composeSubstanceSubstanceInstanceComponent(String name, Substance.SubstanceInstanceComponent element) 26483 throws IOException { 26484 if (element != null) { 26485 open(name); 26486 composeSubstanceSubstanceInstanceComponentInner(element); 26487 close(); 26488 } 26489 } 26490 26491 protected void composeSubstanceSubstanceInstanceComponentInner(Substance.SubstanceInstanceComponent element) 26492 throws IOException { 26493 composeBackbone(element); 26494 if (element.hasIdentifier()) { 26495 composeIdentifier("identifier", element.getIdentifier()); 26496 } 26497 if (element.hasExpiryElement()) { 26498 composeDateTimeCore("expiry", element.getExpiryElement(), false); 26499 composeDateTimeExtras("expiry", element.getExpiryElement(), false); 26500 } 26501 if (element.hasQuantity()) { 26502 composeSimpleQuantity("quantity", element.getQuantity()); 26503 } 26504 } 26505 26506 protected void composeSubstanceSubstanceIngredientComponent(String name, 26507 Substance.SubstanceIngredientComponent element) throws IOException { 26508 if (element != null) { 26509 open(name); 26510 composeSubstanceSubstanceIngredientComponentInner(element); 26511 close(); 26512 } 26513 } 26514 26515 protected void composeSubstanceSubstanceIngredientComponentInner(Substance.SubstanceIngredientComponent element) 26516 throws IOException { 26517 composeBackbone(element); 26518 if (element.hasQuantity()) { 26519 composeRatio("quantity", element.getQuantity()); 26520 } 26521 if (element.hasSubstance()) { 26522 composeReference("substance", element.getSubstance()); 26523 } 26524 } 26525 26526 protected void composeSupplyDelivery(String name, SupplyDelivery element) throws IOException { 26527 if (element != null) { 26528 prop("resourceType", name); 26529 composeSupplyDeliveryInner(element); 26530 } 26531 } 26532 26533 protected void composeSupplyDeliveryInner(SupplyDelivery element) throws IOException { 26534 composeDomainResourceElements(element); 26535 if (element.hasIdentifier()) { 26536 composeIdentifier("identifier", element.getIdentifier()); 26537 } 26538 if (element.hasStatusElement()) { 26539 composeEnumerationCore("status", element.getStatusElement(), new SupplyDelivery.SupplyDeliveryStatusEnumFactory(), 26540 false); 26541 composeEnumerationExtras("status", element.getStatusElement(), 26542 new SupplyDelivery.SupplyDeliveryStatusEnumFactory(), false); 26543 } 26544 if (element.hasPatient()) { 26545 composeReference("patient", element.getPatient()); 26546 } 26547 if (element.hasType()) { 26548 composeCodeableConcept("type", element.getType()); 26549 } 26550 if (element.hasQuantity()) { 26551 composeSimpleQuantity("quantity", element.getQuantity()); 26552 } 26553 if (element.hasSuppliedItem()) { 26554 composeReference("suppliedItem", element.getSuppliedItem()); 26555 } 26556 if (element.hasSupplier()) { 26557 composeReference("supplier", element.getSupplier()); 26558 } 26559 if (element.hasWhenPrepared()) { 26560 composePeriod("whenPrepared", element.getWhenPrepared()); 26561 } 26562 if (element.hasTimeElement()) { 26563 composeDateTimeCore("time", element.getTimeElement(), false); 26564 composeDateTimeExtras("time", element.getTimeElement(), false); 26565 } 26566 if (element.hasDestination()) { 26567 composeReference("destination", element.getDestination()); 26568 } 26569 if (element.hasReceiver()) { 26570 openArray("receiver"); 26571 for (Reference e : element.getReceiver()) 26572 composeReference(null, e); 26573 closeArray(); 26574 } 26575 ; 26576 } 26577 26578 protected void composeSupplyRequest(String name, SupplyRequest element) throws IOException { 26579 if (element != null) { 26580 prop("resourceType", name); 26581 composeSupplyRequestInner(element); 26582 } 26583 } 26584 26585 protected void composeSupplyRequestInner(SupplyRequest element) throws IOException { 26586 composeDomainResourceElements(element); 26587 if (element.hasPatient()) { 26588 composeReference("patient", element.getPatient()); 26589 } 26590 if (element.hasSource()) { 26591 composeReference("source", element.getSource()); 26592 } 26593 if (element.hasDateElement()) { 26594 composeDateTimeCore("date", element.getDateElement(), false); 26595 composeDateTimeExtras("date", element.getDateElement(), false); 26596 } 26597 if (element.hasIdentifier()) { 26598 composeIdentifier("identifier", element.getIdentifier()); 26599 } 26600 if (element.hasStatusElement()) { 26601 composeEnumerationCore("status", element.getStatusElement(), new SupplyRequest.SupplyRequestStatusEnumFactory(), 26602 false); 26603 composeEnumerationExtras("status", element.getStatusElement(), new SupplyRequest.SupplyRequestStatusEnumFactory(), 26604 false); 26605 } 26606 if (element.hasKind()) { 26607 composeCodeableConcept("kind", element.getKind()); 26608 } 26609 if (element.hasOrderedItem()) { 26610 composeReference("orderedItem", element.getOrderedItem()); 26611 } 26612 if (element.hasSupplier()) { 26613 openArray("supplier"); 26614 for (Reference e : element.getSupplier()) 26615 composeReference(null, e); 26616 closeArray(); 26617 } 26618 ; 26619 if (element.hasReason()) { 26620 composeType("reason", element.getReason()); 26621 } 26622 if (element.hasWhen()) { 26623 composeSupplyRequestSupplyRequestWhenComponent("when", element.getWhen()); 26624 } 26625 } 26626 26627 protected void composeSupplyRequestSupplyRequestWhenComponent(String name, 26628 SupplyRequest.SupplyRequestWhenComponent element) throws IOException { 26629 if (element != null) { 26630 open(name); 26631 composeSupplyRequestSupplyRequestWhenComponentInner(element); 26632 close(); 26633 } 26634 } 26635 26636 protected void composeSupplyRequestSupplyRequestWhenComponentInner(SupplyRequest.SupplyRequestWhenComponent element) 26637 throws IOException { 26638 composeBackbone(element); 26639 if (element.hasCode()) { 26640 composeCodeableConcept("code", element.getCode()); 26641 } 26642 if (element.hasSchedule()) { 26643 composeTiming("schedule", element.getSchedule()); 26644 } 26645 } 26646 26647 protected void composeTestScript(String name, TestScript element) throws IOException { 26648 if (element != null) { 26649 prop("resourceType", name); 26650 composeTestScriptInner(element); 26651 } 26652 } 26653 26654 protected void composeTestScriptInner(TestScript element) throws IOException { 26655 composeDomainResourceElements(element); 26656 if (element.hasUrlElement()) { 26657 composeUriCore("url", element.getUrlElement(), false); 26658 composeUriExtras("url", element.getUrlElement(), false); 26659 } 26660 if (element.hasVersionElement()) { 26661 composeStringCore("version", element.getVersionElement(), false); 26662 composeStringExtras("version", element.getVersionElement(), false); 26663 } 26664 if (element.hasNameElement()) { 26665 composeStringCore("name", element.getNameElement(), false); 26666 composeStringExtras("name", element.getNameElement(), false); 26667 } 26668 if (element.hasStatusElement()) { 26669 composeEnumerationCore("status", element.getStatusElement(), 26670 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 26671 composeEnumerationExtras("status", element.getStatusElement(), 26672 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 26673 } 26674 if (element.hasIdentifier()) { 26675 composeIdentifier("identifier", element.getIdentifier()); 26676 } 26677 if (element.hasExperimentalElement()) { 26678 composeBooleanCore("experimental", element.getExperimentalElement(), false); 26679 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 26680 } 26681 if (element.hasPublisherElement()) { 26682 composeStringCore("publisher", element.getPublisherElement(), false); 26683 composeStringExtras("publisher", element.getPublisherElement(), false); 26684 } 26685 if (element.hasContact()) { 26686 openArray("contact"); 26687 for (TestScript.TestScriptContactComponent e : element.getContact()) 26688 composeTestScriptTestScriptContactComponent(null, e); 26689 closeArray(); 26690 } 26691 ; 26692 if (element.hasDateElement()) { 26693 composeDateTimeCore("date", element.getDateElement(), false); 26694 composeDateTimeExtras("date", element.getDateElement(), false); 26695 } 26696 if (element.hasDescriptionElement()) { 26697 composeStringCore("description", element.getDescriptionElement(), false); 26698 composeStringExtras("description", element.getDescriptionElement(), false); 26699 } 26700 if (element.hasUseContext()) { 26701 openArray("useContext"); 26702 for (CodeableConcept e : element.getUseContext()) 26703 composeCodeableConcept(null, e); 26704 closeArray(); 26705 } 26706 ; 26707 if (element.hasRequirementsElement()) { 26708 composeStringCore("requirements", element.getRequirementsElement(), false); 26709 composeStringExtras("requirements", element.getRequirementsElement(), false); 26710 } 26711 if (element.hasCopyrightElement()) { 26712 composeStringCore("copyright", element.getCopyrightElement(), false); 26713 composeStringExtras("copyright", element.getCopyrightElement(), false); 26714 } 26715 if (element.hasMetadata()) { 26716 composeTestScriptTestScriptMetadataComponent("metadata", element.getMetadata()); 26717 } 26718 if (element.hasMultiserverElement()) { 26719 composeBooleanCore("multiserver", element.getMultiserverElement(), false); 26720 composeBooleanExtras("multiserver", element.getMultiserverElement(), false); 26721 } 26722 if (element.hasFixture()) { 26723 openArray("fixture"); 26724 for (TestScript.TestScriptFixtureComponent e : element.getFixture()) 26725 composeTestScriptTestScriptFixtureComponent(null, e); 26726 closeArray(); 26727 } 26728 ; 26729 if (element.hasProfile()) { 26730 openArray("profile"); 26731 for (Reference e : element.getProfile()) 26732 composeReference(null, e); 26733 closeArray(); 26734 } 26735 ; 26736 if (element.hasVariable()) { 26737 openArray("variable"); 26738 for (TestScript.TestScriptVariableComponent e : element.getVariable()) 26739 composeTestScriptTestScriptVariableComponent(null, e); 26740 closeArray(); 26741 } 26742 ; 26743 if (element.hasSetup()) { 26744 composeTestScriptTestScriptSetupComponent("setup", element.getSetup()); 26745 } 26746 if (element.hasTest()) { 26747 openArray("test"); 26748 for (TestScript.TestScriptTestComponent e : element.getTest()) 26749 composeTestScriptTestScriptTestComponent(null, e); 26750 closeArray(); 26751 } 26752 ; 26753 if (element.hasTeardown()) { 26754 composeTestScriptTestScriptTeardownComponent("teardown", element.getTeardown()); 26755 } 26756 } 26757 26758 protected void composeTestScriptTestScriptContactComponent(String name, TestScript.TestScriptContactComponent element) 26759 throws IOException { 26760 if (element != null) { 26761 open(name); 26762 composeTestScriptTestScriptContactComponentInner(element); 26763 close(); 26764 } 26765 } 26766 26767 protected void composeTestScriptTestScriptContactComponentInner(TestScript.TestScriptContactComponent element) 26768 throws IOException { 26769 composeBackbone(element); 26770 if (element.hasNameElement()) { 26771 composeStringCore("name", element.getNameElement(), false); 26772 composeStringExtras("name", element.getNameElement(), false); 26773 } 26774 if (element.hasTelecom()) { 26775 openArray("telecom"); 26776 for (ContactPoint e : element.getTelecom()) 26777 composeContactPoint(null, e); 26778 closeArray(); 26779 } 26780 ; 26781 } 26782 26783 protected void composeTestScriptTestScriptMetadataComponent(String name, 26784 TestScript.TestScriptMetadataComponent element) throws IOException { 26785 if (element != null) { 26786 open(name); 26787 composeTestScriptTestScriptMetadataComponentInner(element); 26788 close(); 26789 } 26790 } 26791 26792 protected void composeTestScriptTestScriptMetadataComponentInner(TestScript.TestScriptMetadataComponent element) 26793 throws IOException { 26794 composeBackbone(element); 26795 if (element.hasLink()) { 26796 openArray("link"); 26797 for (TestScript.TestScriptMetadataLinkComponent e : element.getLink()) 26798 composeTestScriptTestScriptMetadataLinkComponent(null, e); 26799 closeArray(); 26800 } 26801 ; 26802 if (element.hasCapability()) { 26803 openArray("capability"); 26804 for (TestScript.TestScriptMetadataCapabilityComponent e : element.getCapability()) 26805 composeTestScriptTestScriptMetadataCapabilityComponent(null, e); 26806 closeArray(); 26807 } 26808 ; 26809 } 26810 26811 protected void composeTestScriptTestScriptMetadataLinkComponent(String name, 26812 TestScript.TestScriptMetadataLinkComponent element) throws IOException { 26813 if (element != null) { 26814 open(name); 26815 composeTestScriptTestScriptMetadataLinkComponentInner(element); 26816 close(); 26817 } 26818 } 26819 26820 protected void composeTestScriptTestScriptMetadataLinkComponentInner( 26821 TestScript.TestScriptMetadataLinkComponent element) throws IOException { 26822 composeBackbone(element); 26823 if (element.hasUrlElement()) { 26824 composeUriCore("url", element.getUrlElement(), false); 26825 composeUriExtras("url", element.getUrlElement(), false); 26826 } 26827 if (element.hasDescriptionElement()) { 26828 composeStringCore("description", element.getDescriptionElement(), false); 26829 composeStringExtras("description", element.getDescriptionElement(), false); 26830 } 26831 } 26832 26833 protected void composeTestScriptTestScriptMetadataCapabilityComponent(String name, 26834 TestScript.TestScriptMetadataCapabilityComponent element) throws IOException { 26835 if (element != null) { 26836 open(name); 26837 composeTestScriptTestScriptMetadataCapabilityComponentInner(element); 26838 close(); 26839 } 26840 } 26841 26842 protected void composeTestScriptTestScriptMetadataCapabilityComponentInner( 26843 TestScript.TestScriptMetadataCapabilityComponent element) throws IOException { 26844 composeBackbone(element); 26845 if (element.hasRequiredElement()) { 26846 composeBooleanCore("required", element.getRequiredElement(), false); 26847 composeBooleanExtras("required", element.getRequiredElement(), false); 26848 } 26849 if (element.hasValidatedElement()) { 26850 composeBooleanCore("validated", element.getValidatedElement(), false); 26851 composeBooleanExtras("validated", element.getValidatedElement(), false); 26852 } 26853 if (element.hasDescriptionElement()) { 26854 composeStringCore("description", element.getDescriptionElement(), false); 26855 composeStringExtras("description", element.getDescriptionElement(), false); 26856 } 26857 if (element.hasDestinationElement()) { 26858 composeIntegerCore("destination", element.getDestinationElement(), false); 26859 composeIntegerExtras("destination", element.getDestinationElement(), false); 26860 } 26861 if (element.hasLink()) { 26862 openArray("link"); 26863 for (UriType e : element.getLink()) 26864 composeUriCore(null, e, true); 26865 closeArray(); 26866 if (anyHasExtras(element.getLink())) { 26867 openArray("_link"); 26868 for (UriType e : element.getLink()) 26869 composeUriExtras(null, e, true); 26870 closeArray(); 26871 } 26872 } 26873 ; 26874 if (element.hasConformance()) { 26875 composeReference("conformance", element.getConformance()); 26876 } 26877 } 26878 26879 protected void composeTestScriptTestScriptFixtureComponent(String name, TestScript.TestScriptFixtureComponent element) 26880 throws IOException { 26881 if (element != null) { 26882 open(name); 26883 composeTestScriptTestScriptFixtureComponentInner(element); 26884 close(); 26885 } 26886 } 26887 26888 protected void composeTestScriptTestScriptFixtureComponentInner(TestScript.TestScriptFixtureComponent element) 26889 throws IOException { 26890 composeBackbone(element); 26891 if (element.hasAutocreateElement()) { 26892 composeBooleanCore("autocreate", element.getAutocreateElement(), false); 26893 composeBooleanExtras("autocreate", element.getAutocreateElement(), false); 26894 } 26895 if (element.hasAutodeleteElement()) { 26896 composeBooleanCore("autodelete", element.getAutodeleteElement(), false); 26897 composeBooleanExtras("autodelete", element.getAutodeleteElement(), false); 26898 } 26899 if (element.hasResource()) { 26900 composeReference("resource", element.getResource()); 26901 } 26902 } 26903 26904 protected void composeTestScriptTestScriptVariableComponent(String name, 26905 TestScript.TestScriptVariableComponent element) throws IOException { 26906 if (element != null) { 26907 open(name); 26908 composeTestScriptTestScriptVariableComponentInner(element); 26909 close(); 26910 } 26911 } 26912 26913 protected void composeTestScriptTestScriptVariableComponentInner(TestScript.TestScriptVariableComponent element) 26914 throws IOException { 26915 composeBackbone(element); 26916 if (element.hasNameElement()) { 26917 composeStringCore("name", element.getNameElement(), false); 26918 composeStringExtras("name", element.getNameElement(), false); 26919 } 26920 if (element.hasHeaderFieldElement()) { 26921 composeStringCore("headerField", element.getHeaderFieldElement(), false); 26922 composeStringExtras("headerField", element.getHeaderFieldElement(), false); 26923 } 26924 if (element.hasPathElement()) { 26925 composeStringCore("path", element.getPathElement(), false); 26926 composeStringExtras("path", element.getPathElement(), false); 26927 } 26928 if (element.hasSourceIdElement()) { 26929 composeIdCore("sourceId", element.getSourceIdElement(), false); 26930 composeIdExtras("sourceId", element.getSourceIdElement(), false); 26931 } 26932 } 26933 26934 protected void composeTestScriptTestScriptSetupComponent(String name, TestScript.TestScriptSetupComponent element) 26935 throws IOException { 26936 if (element != null) { 26937 open(name); 26938 composeTestScriptTestScriptSetupComponentInner(element); 26939 close(); 26940 } 26941 } 26942 26943 protected void composeTestScriptTestScriptSetupComponentInner(TestScript.TestScriptSetupComponent element) 26944 throws IOException { 26945 composeBackbone(element); 26946 if (element.hasMetadata()) { 26947 composeTestScriptTestScriptMetadataComponent("metadata", element.getMetadata()); 26948 } 26949 if (element.hasAction()) { 26950 openArray("action"); 26951 for (TestScript.TestScriptSetupActionComponent e : element.getAction()) 26952 composeTestScriptTestScriptSetupActionComponent(null, e); 26953 closeArray(); 26954 } 26955 ; 26956 } 26957 26958 protected void composeTestScriptTestScriptSetupActionComponent(String name, 26959 TestScript.TestScriptSetupActionComponent element) throws IOException { 26960 if (element != null) { 26961 open(name); 26962 composeTestScriptTestScriptSetupActionComponentInner(element); 26963 close(); 26964 } 26965 } 26966 26967 protected void composeTestScriptTestScriptSetupActionComponentInner(TestScript.TestScriptSetupActionComponent element) 26968 throws IOException { 26969 composeBackbone(element); 26970 if (element.hasOperation()) { 26971 composeTestScriptTestScriptSetupActionOperationComponent("operation", element.getOperation()); 26972 } 26973 if (element.hasAssert()) { 26974 composeTestScriptTestScriptSetupActionAssertComponent("assert", element.getAssert()); 26975 } 26976 } 26977 26978 protected void composeTestScriptTestScriptSetupActionOperationComponent(String name, 26979 TestScript.TestScriptSetupActionOperationComponent element) throws IOException { 26980 if (element != null) { 26981 open(name); 26982 composeTestScriptTestScriptSetupActionOperationComponentInner(element); 26983 close(); 26984 } 26985 } 26986 26987 protected void composeTestScriptTestScriptSetupActionOperationComponentInner( 26988 TestScript.TestScriptSetupActionOperationComponent element) throws IOException { 26989 composeBackbone(element); 26990 if (element.hasType()) { 26991 composeCoding("type", element.getType()); 26992 } 26993 if (element.hasResourceElement()) { 26994 composeCodeCore("resource", element.getResourceElement(), false); 26995 composeCodeExtras("resource", element.getResourceElement(), false); 26996 } 26997 if (element.hasLabelElement()) { 26998 composeStringCore("label", element.getLabelElement(), false); 26999 composeStringExtras("label", element.getLabelElement(), false); 27000 } 27001 if (element.hasDescriptionElement()) { 27002 composeStringCore("description", element.getDescriptionElement(), false); 27003 composeStringExtras("description", element.getDescriptionElement(), false); 27004 } 27005 if (element.hasAcceptElement()) { 27006 composeEnumerationCore("accept", element.getAcceptElement(), new TestScript.ContentTypeEnumFactory(), false); 27007 composeEnumerationExtras("accept", element.getAcceptElement(), new TestScript.ContentTypeEnumFactory(), false); 27008 } 27009 if (element.hasContentTypeElement()) { 27010 composeEnumerationCore("contentType", element.getContentTypeElement(), new TestScript.ContentTypeEnumFactory(), 27011 false); 27012 composeEnumerationExtras("contentType", element.getContentTypeElement(), new TestScript.ContentTypeEnumFactory(), 27013 false); 27014 } 27015 if (element.hasDestinationElement()) { 27016 composeIntegerCore("destination", element.getDestinationElement(), false); 27017 composeIntegerExtras("destination", element.getDestinationElement(), false); 27018 } 27019 if (element.hasEncodeRequestUrlElement()) { 27020 composeBooleanCore("encodeRequestUrl", element.getEncodeRequestUrlElement(), false); 27021 composeBooleanExtras("encodeRequestUrl", element.getEncodeRequestUrlElement(), false); 27022 } 27023 if (element.hasParamsElement()) { 27024 composeStringCore("params", element.getParamsElement(), false); 27025 composeStringExtras("params", element.getParamsElement(), false); 27026 } 27027 if (element.hasRequestHeader()) { 27028 openArray("requestHeader"); 27029 for (TestScript.TestScriptSetupActionOperationRequestHeaderComponent e : element.getRequestHeader()) 27030 composeTestScriptTestScriptSetupActionOperationRequestHeaderComponent(null, e); 27031 closeArray(); 27032 } 27033 ; 27034 if (element.hasResponseIdElement()) { 27035 composeIdCore("responseId", element.getResponseIdElement(), false); 27036 composeIdExtras("responseId", element.getResponseIdElement(), false); 27037 } 27038 if (element.hasSourceIdElement()) { 27039 composeIdCore("sourceId", element.getSourceIdElement(), false); 27040 composeIdExtras("sourceId", element.getSourceIdElement(), false); 27041 } 27042 if (element.hasTargetIdElement()) { 27043 composeIdCore("targetId", element.getTargetIdElement(), false); 27044 composeIdExtras("targetId", element.getTargetIdElement(), false); 27045 } 27046 if (element.hasUrlElement()) { 27047 composeStringCore("url", element.getUrlElement(), false); 27048 composeStringExtras("url", element.getUrlElement(), false); 27049 } 27050 } 27051 27052 protected void composeTestScriptTestScriptSetupActionOperationRequestHeaderComponent(String name, 27053 TestScript.TestScriptSetupActionOperationRequestHeaderComponent element) throws IOException { 27054 if (element != null) { 27055 open(name); 27056 composeTestScriptTestScriptSetupActionOperationRequestHeaderComponentInner(element); 27057 close(); 27058 } 27059 } 27060 27061 protected void composeTestScriptTestScriptSetupActionOperationRequestHeaderComponentInner( 27062 TestScript.TestScriptSetupActionOperationRequestHeaderComponent element) throws IOException { 27063 composeBackbone(element); 27064 if (element.hasFieldElement()) { 27065 composeStringCore("field", element.getFieldElement(), false); 27066 composeStringExtras("field", element.getFieldElement(), false); 27067 } 27068 if (element.hasValueElement()) { 27069 composeStringCore("value", element.getValueElement(), false); 27070 composeStringExtras("value", element.getValueElement(), false); 27071 } 27072 } 27073 27074 protected void composeTestScriptTestScriptSetupActionAssertComponent(String name, 27075 TestScript.TestScriptSetupActionAssertComponent element) throws IOException { 27076 if (element != null) { 27077 open(name); 27078 composeTestScriptTestScriptSetupActionAssertComponentInner(element); 27079 close(); 27080 } 27081 } 27082 27083 protected void composeTestScriptTestScriptSetupActionAssertComponentInner( 27084 TestScript.TestScriptSetupActionAssertComponent element) throws IOException { 27085 composeBackbone(element); 27086 if (element.hasLabelElement()) { 27087 composeStringCore("label", element.getLabelElement(), false); 27088 composeStringExtras("label", element.getLabelElement(), false); 27089 } 27090 if (element.hasDescriptionElement()) { 27091 composeStringCore("description", element.getDescriptionElement(), false); 27092 composeStringExtras("description", element.getDescriptionElement(), false); 27093 } 27094 if (element.hasDirectionElement()) { 27095 composeEnumerationCore("direction", element.getDirectionElement(), 27096 new TestScript.AssertionDirectionTypeEnumFactory(), false); 27097 composeEnumerationExtras("direction", element.getDirectionElement(), 27098 new TestScript.AssertionDirectionTypeEnumFactory(), false); 27099 } 27100 if (element.hasCompareToSourceIdElement()) { 27101 composeStringCore("compareToSourceId", element.getCompareToSourceIdElement(), false); 27102 composeStringExtras("compareToSourceId", element.getCompareToSourceIdElement(), false); 27103 } 27104 if (element.hasCompareToSourcePathElement()) { 27105 composeStringCore("compareToSourcePath", element.getCompareToSourcePathElement(), false); 27106 composeStringExtras("compareToSourcePath", element.getCompareToSourcePathElement(), false); 27107 } 27108 if (element.hasContentTypeElement()) { 27109 composeEnumerationCore("contentType", element.getContentTypeElement(), new TestScript.ContentTypeEnumFactory(), 27110 false); 27111 composeEnumerationExtras("contentType", element.getContentTypeElement(), new TestScript.ContentTypeEnumFactory(), 27112 false); 27113 } 27114 if (element.hasHeaderFieldElement()) { 27115 composeStringCore("headerField", element.getHeaderFieldElement(), false); 27116 composeStringExtras("headerField", element.getHeaderFieldElement(), false); 27117 } 27118 if (element.hasMinimumIdElement()) { 27119 composeStringCore("minimumId", element.getMinimumIdElement(), false); 27120 composeStringExtras("minimumId", element.getMinimumIdElement(), false); 27121 } 27122 if (element.hasNavigationLinksElement()) { 27123 composeBooleanCore("navigationLinks", element.getNavigationLinksElement(), false); 27124 composeBooleanExtras("navigationLinks", element.getNavigationLinksElement(), false); 27125 } 27126 if (element.hasOperatorElement()) { 27127 composeEnumerationCore("operator", element.getOperatorElement(), 27128 new TestScript.AssertionOperatorTypeEnumFactory(), false); 27129 composeEnumerationExtras("operator", element.getOperatorElement(), 27130 new TestScript.AssertionOperatorTypeEnumFactory(), false); 27131 } 27132 if (element.hasPathElement()) { 27133 composeStringCore("path", element.getPathElement(), false); 27134 composeStringExtras("path", element.getPathElement(), false); 27135 } 27136 if (element.hasResourceElement()) { 27137 composeCodeCore("resource", element.getResourceElement(), false); 27138 composeCodeExtras("resource", element.getResourceElement(), false); 27139 } 27140 if (element.hasResponseElement()) { 27141 composeEnumerationCore("response", element.getResponseElement(), 27142 new TestScript.AssertionResponseTypesEnumFactory(), false); 27143 composeEnumerationExtras("response", element.getResponseElement(), 27144 new TestScript.AssertionResponseTypesEnumFactory(), false); 27145 } 27146 if (element.hasResponseCodeElement()) { 27147 composeStringCore("responseCode", element.getResponseCodeElement(), false); 27148 composeStringExtras("responseCode", element.getResponseCodeElement(), false); 27149 } 27150 if (element.hasSourceIdElement()) { 27151 composeIdCore("sourceId", element.getSourceIdElement(), false); 27152 composeIdExtras("sourceId", element.getSourceIdElement(), false); 27153 } 27154 if (element.hasValidateProfileIdElement()) { 27155 composeIdCore("validateProfileId", element.getValidateProfileIdElement(), false); 27156 composeIdExtras("validateProfileId", element.getValidateProfileIdElement(), false); 27157 } 27158 if (element.hasValueElement()) { 27159 composeStringCore("value", element.getValueElement(), false); 27160 composeStringExtras("value", element.getValueElement(), false); 27161 } 27162 if (element.hasWarningOnlyElement()) { 27163 composeBooleanCore("warningOnly", element.getWarningOnlyElement(), false); 27164 composeBooleanExtras("warningOnly", element.getWarningOnlyElement(), false); 27165 } 27166 } 27167 27168 protected void composeTestScriptTestScriptTestComponent(String name, TestScript.TestScriptTestComponent element) 27169 throws IOException { 27170 if (element != null) { 27171 open(name); 27172 composeTestScriptTestScriptTestComponentInner(element); 27173 close(); 27174 } 27175 } 27176 27177 protected void composeTestScriptTestScriptTestComponentInner(TestScript.TestScriptTestComponent element) 27178 throws IOException { 27179 composeBackbone(element); 27180 if (element.hasNameElement()) { 27181 composeStringCore("name", element.getNameElement(), false); 27182 composeStringExtras("name", element.getNameElement(), false); 27183 } 27184 if (element.hasDescriptionElement()) { 27185 composeStringCore("description", element.getDescriptionElement(), false); 27186 composeStringExtras("description", element.getDescriptionElement(), false); 27187 } 27188 if (element.hasMetadata()) { 27189 composeTestScriptTestScriptMetadataComponent("metadata", element.getMetadata()); 27190 } 27191 if (element.hasAction()) { 27192 openArray("action"); 27193 for (TestScript.TestScriptTestActionComponent e : element.getAction()) 27194 composeTestScriptTestScriptTestActionComponent(null, e); 27195 closeArray(); 27196 } 27197 ; 27198 } 27199 27200 protected void composeTestScriptTestScriptTestActionComponent(String name, 27201 TestScript.TestScriptTestActionComponent element) throws IOException { 27202 if (element != null) { 27203 open(name); 27204 composeTestScriptTestScriptTestActionComponentInner(element); 27205 close(); 27206 } 27207 } 27208 27209 protected void composeTestScriptTestScriptTestActionComponentInner(TestScript.TestScriptTestActionComponent element) 27210 throws IOException { 27211 composeBackbone(element); 27212 if (element.hasOperation()) { 27213 composeTestScriptTestScriptSetupActionOperationComponent("operation", element.getOperation()); 27214 } 27215 if (element.hasAssert()) { 27216 composeTestScriptTestScriptSetupActionAssertComponent("assert", element.getAssert()); 27217 } 27218 } 27219 27220 protected void composeTestScriptTestScriptTeardownComponent(String name, 27221 TestScript.TestScriptTeardownComponent element) throws IOException { 27222 if (element != null) { 27223 open(name); 27224 composeTestScriptTestScriptTeardownComponentInner(element); 27225 close(); 27226 } 27227 } 27228 27229 protected void composeTestScriptTestScriptTeardownComponentInner(TestScript.TestScriptTeardownComponent element) 27230 throws IOException { 27231 composeBackbone(element); 27232 if (element.hasAction()) { 27233 openArray("action"); 27234 for (TestScript.TestScriptTeardownActionComponent e : element.getAction()) 27235 composeTestScriptTestScriptTeardownActionComponent(null, e); 27236 closeArray(); 27237 } 27238 ; 27239 } 27240 27241 protected void composeTestScriptTestScriptTeardownActionComponent(String name, 27242 TestScript.TestScriptTeardownActionComponent element) throws IOException { 27243 if (element != null) { 27244 open(name); 27245 composeTestScriptTestScriptTeardownActionComponentInner(element); 27246 close(); 27247 } 27248 } 27249 27250 protected void composeTestScriptTestScriptTeardownActionComponentInner( 27251 TestScript.TestScriptTeardownActionComponent element) throws IOException { 27252 composeBackbone(element); 27253 if (element.hasOperation()) { 27254 composeTestScriptTestScriptSetupActionOperationComponent("operation", element.getOperation()); 27255 } 27256 } 27257 27258 protected void composeValueSet(String name, ValueSet element) throws IOException { 27259 if (element != null) { 27260 prop("resourceType", name); 27261 composeValueSetInner(element); 27262 } 27263 } 27264 27265 protected void composeValueSetInner(ValueSet element) throws IOException { 27266 composeDomainResourceElements(element); 27267 if (element.hasUrlElement()) { 27268 composeUriCore("url", element.getUrlElement(), false); 27269 composeUriExtras("url", element.getUrlElement(), false); 27270 } 27271 if (element.hasIdentifier()) { 27272 composeIdentifier("identifier", element.getIdentifier()); 27273 } 27274 if (element.hasVersionElement()) { 27275 composeStringCore("version", element.getVersionElement(), false); 27276 composeStringExtras("version", element.getVersionElement(), false); 27277 } 27278 if (element.hasNameElement()) { 27279 composeStringCore("name", element.getNameElement(), false); 27280 composeStringExtras("name", element.getNameElement(), false); 27281 } 27282 if (element.hasStatusElement()) { 27283 composeEnumerationCore("status", element.getStatusElement(), 27284 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 27285 composeEnumerationExtras("status", element.getStatusElement(), 27286 new Enumerations.ConformanceResourceStatusEnumFactory(), false); 27287 } 27288 if (element.hasExperimentalElement()) { 27289 composeBooleanCore("experimental", element.getExperimentalElement(), false); 27290 composeBooleanExtras("experimental", element.getExperimentalElement(), false); 27291 } 27292 if (element.hasPublisherElement()) { 27293 composeStringCore("publisher", element.getPublisherElement(), false); 27294 composeStringExtras("publisher", element.getPublisherElement(), false); 27295 } 27296 if (element.hasContact()) { 27297 openArray("contact"); 27298 for (ValueSet.ValueSetContactComponent e : element.getContact()) 27299 composeValueSetValueSetContactComponent(null, e); 27300 closeArray(); 27301 } 27302 ; 27303 if (element.hasDateElement()) { 27304 composeDateTimeCore("date", element.getDateElement(), false); 27305 composeDateTimeExtras("date", element.getDateElement(), false); 27306 } 27307 if (element.hasLockedDateElement()) { 27308 composeDateCore("lockedDate", element.getLockedDateElement(), false); 27309 composeDateExtras("lockedDate", element.getLockedDateElement(), false); 27310 } 27311 if (element.hasDescriptionElement()) { 27312 composeStringCore("description", element.getDescriptionElement(), false); 27313 composeStringExtras("description", element.getDescriptionElement(), false); 27314 } 27315 if (element.hasUseContext()) { 27316 openArray("useContext"); 27317 for (CodeableConcept e : element.getUseContext()) 27318 composeCodeableConcept(null, e); 27319 closeArray(); 27320 } 27321 ; 27322 if (element.hasImmutableElement()) { 27323 composeBooleanCore("immutable", element.getImmutableElement(), false); 27324 composeBooleanExtras("immutable", element.getImmutableElement(), false); 27325 } 27326 if (element.hasRequirementsElement()) { 27327 composeStringCore("requirements", element.getRequirementsElement(), false); 27328 composeStringExtras("requirements", element.getRequirementsElement(), false); 27329 } 27330 if (element.hasCopyrightElement()) { 27331 composeStringCore("copyright", element.getCopyrightElement(), false); 27332 composeStringExtras("copyright", element.getCopyrightElement(), false); 27333 } 27334 if (element.hasExtensibleElement()) { 27335 composeBooleanCore("extensible", element.getExtensibleElement(), false); 27336 composeBooleanExtras("extensible", element.getExtensibleElement(), false); 27337 } 27338 if (element.hasCodeSystem()) { 27339 composeValueSetValueSetCodeSystemComponent("codeSystem", element.getCodeSystem()); 27340 } 27341 if (element.hasCompose()) { 27342 composeValueSetValueSetComposeComponent("compose", element.getCompose()); 27343 } 27344 if (element.hasExpansion()) { 27345 composeValueSetValueSetExpansionComponent("expansion", element.getExpansion()); 27346 } 27347 } 27348 27349 protected void composeValueSetValueSetContactComponent(String name, ValueSet.ValueSetContactComponent element) 27350 throws IOException { 27351 if (element != null) { 27352 open(name); 27353 composeValueSetValueSetContactComponentInner(element); 27354 close(); 27355 } 27356 } 27357 27358 protected void composeValueSetValueSetContactComponentInner(ValueSet.ValueSetContactComponent element) 27359 throws IOException { 27360 composeBackbone(element); 27361 if (element.hasNameElement()) { 27362 composeStringCore("name", element.getNameElement(), false); 27363 composeStringExtras("name", element.getNameElement(), false); 27364 } 27365 if (element.hasTelecom()) { 27366 openArray("telecom"); 27367 for (ContactPoint e : element.getTelecom()) 27368 composeContactPoint(null, e); 27369 closeArray(); 27370 } 27371 ; 27372 } 27373 27374 protected void composeValueSetValueSetCodeSystemComponent(String name, ValueSet.ValueSetCodeSystemComponent element) 27375 throws IOException { 27376 if (element != null) { 27377 open(name); 27378 composeValueSetValueSetCodeSystemComponentInner(element); 27379 close(); 27380 } 27381 } 27382 27383 protected void composeValueSetValueSetCodeSystemComponentInner(ValueSet.ValueSetCodeSystemComponent element) 27384 throws IOException { 27385 composeBackbone(element); 27386 if (element.hasSystemElement()) { 27387 composeUriCore("system", element.getSystemElement(), false); 27388 composeUriExtras("system", element.getSystemElement(), false); 27389 } 27390 if (element.hasVersionElement()) { 27391 composeStringCore("version", element.getVersionElement(), false); 27392 composeStringExtras("version", element.getVersionElement(), false); 27393 } 27394 if (element.hasCaseSensitiveElement()) { 27395 composeBooleanCore("caseSensitive", element.getCaseSensitiveElement(), false); 27396 composeBooleanExtras("caseSensitive", element.getCaseSensitiveElement(), false); 27397 } 27398 if (element.hasConcept()) { 27399 openArray("concept"); 27400 for (ValueSet.ConceptDefinitionComponent e : element.getConcept()) 27401 composeValueSetConceptDefinitionComponent(null, e); 27402 closeArray(); 27403 } 27404 ; 27405 } 27406 27407 protected void composeValueSetConceptDefinitionComponent(String name, ValueSet.ConceptDefinitionComponent element) 27408 throws IOException { 27409 if (element != null) { 27410 open(name); 27411 composeValueSetConceptDefinitionComponentInner(element); 27412 close(); 27413 } 27414 } 27415 27416 protected void composeValueSetConceptDefinitionComponentInner(ValueSet.ConceptDefinitionComponent element) 27417 throws IOException { 27418 composeBackbone(element); 27419 if (element.hasCodeElement()) { 27420 composeCodeCore("code", element.getCodeElement(), false); 27421 composeCodeExtras("code", element.getCodeElement(), false); 27422 } 27423 if (element.hasAbstractElement()) { 27424 composeBooleanCore("abstract", element.getAbstractElement(), false); 27425 composeBooleanExtras("abstract", element.getAbstractElement(), false); 27426 } 27427 if (element.hasDisplayElement()) { 27428 composeStringCore("display", element.getDisplayElement(), false); 27429 composeStringExtras("display", element.getDisplayElement(), false); 27430 } 27431 if (element.hasDefinitionElement()) { 27432 composeStringCore("definition", element.getDefinitionElement(), false); 27433 composeStringExtras("definition", element.getDefinitionElement(), false); 27434 } 27435 if (element.hasDesignation()) { 27436 openArray("designation"); 27437 for (ValueSet.ConceptDefinitionDesignationComponent e : element.getDesignation()) 27438 composeValueSetConceptDefinitionDesignationComponent(null, e); 27439 closeArray(); 27440 } 27441 ; 27442 if (element.hasConcept()) { 27443 openArray("concept"); 27444 for (ValueSet.ConceptDefinitionComponent e : element.getConcept()) 27445 composeValueSetConceptDefinitionComponent(null, e); 27446 closeArray(); 27447 } 27448 ; 27449 } 27450 27451 protected void composeValueSetConceptDefinitionDesignationComponent(String name, 27452 ValueSet.ConceptDefinitionDesignationComponent element) throws IOException { 27453 if (element != null) { 27454 open(name); 27455 composeValueSetConceptDefinitionDesignationComponentInner(element); 27456 close(); 27457 } 27458 } 27459 27460 protected void composeValueSetConceptDefinitionDesignationComponentInner( 27461 ValueSet.ConceptDefinitionDesignationComponent element) throws IOException { 27462 composeBackbone(element); 27463 if (element.hasLanguageElement()) { 27464 composeCodeCore("language", element.getLanguageElement(), false); 27465 composeCodeExtras("language", element.getLanguageElement(), false); 27466 } 27467 if (element.hasUse()) { 27468 composeCoding("use", element.getUse()); 27469 } 27470 if (element.hasValueElement()) { 27471 composeStringCore("value", element.getValueElement(), false); 27472 composeStringExtras("value", element.getValueElement(), false); 27473 } 27474 } 27475 27476 protected void composeValueSetValueSetComposeComponent(String name, ValueSet.ValueSetComposeComponent element) 27477 throws IOException { 27478 if (element != null) { 27479 open(name); 27480 composeValueSetValueSetComposeComponentInner(element); 27481 close(); 27482 } 27483 } 27484 27485 protected void composeValueSetValueSetComposeComponentInner(ValueSet.ValueSetComposeComponent element) 27486 throws IOException { 27487 composeBackbone(element); 27488 if (element.hasImport()) { 27489 openArray("import"); 27490 for (UriType e : element.getImport()) 27491 composeUriCore(null, e, true); 27492 closeArray(); 27493 if (anyHasExtras(element.getImport())) { 27494 openArray("_import"); 27495 for (UriType e : element.getImport()) 27496 composeUriExtras(null, e, true); 27497 closeArray(); 27498 } 27499 } 27500 ; 27501 if (element.hasInclude()) { 27502 openArray("include"); 27503 for (ValueSet.ConceptSetComponent e : element.getInclude()) 27504 composeValueSetConceptSetComponent(null, e); 27505 closeArray(); 27506 } 27507 ; 27508 if (element.hasExclude()) { 27509 openArray("exclude"); 27510 for (ValueSet.ConceptSetComponent e : element.getExclude()) 27511 composeValueSetConceptSetComponent(null, e); 27512 closeArray(); 27513 } 27514 ; 27515 } 27516 27517 protected void composeValueSetConceptSetComponent(String name, ValueSet.ConceptSetComponent element) 27518 throws IOException { 27519 if (element != null) { 27520 open(name); 27521 composeValueSetConceptSetComponentInner(element); 27522 close(); 27523 } 27524 } 27525 27526 protected void composeValueSetConceptSetComponentInner(ValueSet.ConceptSetComponent element) throws IOException { 27527 composeBackbone(element); 27528 if (element.hasSystemElement()) { 27529 composeUriCore("system", element.getSystemElement(), false); 27530 composeUriExtras("system", element.getSystemElement(), false); 27531 } 27532 if (element.hasVersionElement()) { 27533 composeStringCore("version", element.getVersionElement(), false); 27534 composeStringExtras("version", element.getVersionElement(), false); 27535 } 27536 if (element.hasConcept()) { 27537 openArray("concept"); 27538 for (ValueSet.ConceptReferenceComponent e : element.getConcept()) 27539 composeValueSetConceptReferenceComponent(null, e); 27540 closeArray(); 27541 } 27542 ; 27543 if (element.hasFilter()) { 27544 openArray("filter"); 27545 for (ValueSet.ConceptSetFilterComponent e : element.getFilter()) 27546 composeValueSetConceptSetFilterComponent(null, e); 27547 closeArray(); 27548 } 27549 ; 27550 } 27551 27552 protected void composeValueSetConceptReferenceComponent(String name, ValueSet.ConceptReferenceComponent element) 27553 throws IOException { 27554 if (element != null) { 27555 open(name); 27556 composeValueSetConceptReferenceComponentInner(element); 27557 close(); 27558 } 27559 } 27560 27561 protected void composeValueSetConceptReferenceComponentInner(ValueSet.ConceptReferenceComponent element) 27562 throws IOException { 27563 composeBackbone(element); 27564 if (element.hasCodeElement()) { 27565 composeCodeCore("code", element.getCodeElement(), false); 27566 composeCodeExtras("code", element.getCodeElement(), false); 27567 } 27568 if (element.hasDisplayElement()) { 27569 composeStringCore("display", element.getDisplayElement(), false); 27570 composeStringExtras("display", element.getDisplayElement(), false); 27571 } 27572 if (element.hasDesignation()) { 27573 openArray("designation"); 27574 for (ValueSet.ConceptDefinitionDesignationComponent e : element.getDesignation()) 27575 composeValueSetConceptDefinitionDesignationComponent(null, e); 27576 closeArray(); 27577 } 27578 ; 27579 } 27580 27581 protected void composeValueSetConceptSetFilterComponent(String name, ValueSet.ConceptSetFilterComponent element) 27582 throws IOException { 27583 if (element != null) { 27584 open(name); 27585 composeValueSetConceptSetFilterComponentInner(element); 27586 close(); 27587 } 27588 } 27589 27590 protected void composeValueSetConceptSetFilterComponentInner(ValueSet.ConceptSetFilterComponent element) 27591 throws IOException { 27592 composeBackbone(element); 27593 if (element.hasPropertyElement()) { 27594 composeCodeCore("property", element.getPropertyElement(), false); 27595 composeCodeExtras("property", element.getPropertyElement(), false); 27596 } 27597 if (element.hasOpElement()) { 27598 composeEnumerationCore("op", element.getOpElement(), new ValueSet.FilterOperatorEnumFactory(), false); 27599 composeEnumerationExtras("op", element.getOpElement(), new ValueSet.FilterOperatorEnumFactory(), false); 27600 } 27601 if (element.hasValueElement()) { 27602 composeCodeCore("value", element.getValueElement(), false); 27603 composeCodeExtras("value", element.getValueElement(), false); 27604 } 27605 } 27606 27607 protected void composeValueSetValueSetExpansionComponent(String name, ValueSet.ValueSetExpansionComponent element) 27608 throws IOException { 27609 if (element != null) { 27610 open(name); 27611 composeValueSetValueSetExpansionComponentInner(element); 27612 close(); 27613 } 27614 } 27615 27616 protected void composeValueSetValueSetExpansionComponentInner(ValueSet.ValueSetExpansionComponent element) 27617 throws IOException { 27618 composeBackbone(element); 27619 if (element.hasIdentifierElement()) { 27620 composeUriCore("identifier", element.getIdentifierElement(), false); 27621 composeUriExtras("identifier", element.getIdentifierElement(), false); 27622 } 27623 if (element.hasTimestampElement()) { 27624 composeDateTimeCore("timestamp", element.getTimestampElement(), false); 27625 composeDateTimeExtras("timestamp", element.getTimestampElement(), false); 27626 } 27627 if (element.hasTotalElement()) { 27628 composeIntegerCore("total", element.getTotalElement(), false); 27629 composeIntegerExtras("total", element.getTotalElement(), false); 27630 } 27631 if (element.hasOffsetElement()) { 27632 composeIntegerCore("offset", element.getOffsetElement(), false); 27633 composeIntegerExtras("offset", element.getOffsetElement(), false); 27634 } 27635 if (element.hasParameter()) { 27636 openArray("parameter"); 27637 for (ValueSet.ValueSetExpansionParameterComponent e : element.getParameter()) 27638 composeValueSetValueSetExpansionParameterComponent(null, e); 27639 closeArray(); 27640 } 27641 ; 27642 if (element.hasContains()) { 27643 openArray("contains"); 27644 for (ValueSet.ValueSetExpansionContainsComponent e : element.getContains()) 27645 composeValueSetValueSetExpansionContainsComponent(null, e); 27646 closeArray(); 27647 } 27648 ; 27649 } 27650 27651 protected void composeValueSetValueSetExpansionParameterComponent(String name, 27652 ValueSet.ValueSetExpansionParameterComponent element) throws IOException { 27653 if (element != null) { 27654 open(name); 27655 composeValueSetValueSetExpansionParameterComponentInner(element); 27656 close(); 27657 } 27658 } 27659 27660 protected void composeValueSetValueSetExpansionParameterComponentInner( 27661 ValueSet.ValueSetExpansionParameterComponent element) throws IOException { 27662 composeBackbone(element); 27663 if (element.hasNameElement()) { 27664 composeStringCore("name", element.getNameElement(), false); 27665 composeStringExtras("name", element.getNameElement(), false); 27666 } 27667 if (element.hasValue()) { 27668 composeType("value", element.getValue()); 27669 } 27670 } 27671 27672 protected void composeValueSetValueSetExpansionContainsComponent(String name, 27673 ValueSet.ValueSetExpansionContainsComponent element) throws IOException { 27674 if (element != null) { 27675 open(name); 27676 composeValueSetValueSetExpansionContainsComponentInner(element); 27677 close(); 27678 } 27679 } 27680 27681 protected void composeValueSetValueSetExpansionContainsComponentInner( 27682 ValueSet.ValueSetExpansionContainsComponent element) throws IOException { 27683 composeBackbone(element); 27684 if (element.hasSystemElement()) { 27685 composeUriCore("system", element.getSystemElement(), false); 27686 composeUriExtras("system", element.getSystemElement(), false); 27687 } 27688 if (element.hasAbstractElement()) { 27689 composeBooleanCore("abstract", element.getAbstractElement(), false); 27690 composeBooleanExtras("abstract", element.getAbstractElement(), false); 27691 } 27692 if (element.hasVersionElement()) { 27693 composeStringCore("version", element.getVersionElement(), false); 27694 composeStringExtras("version", element.getVersionElement(), false); 27695 } 27696 if (element.hasCodeElement()) { 27697 composeCodeCore("code", element.getCodeElement(), false); 27698 composeCodeExtras("code", element.getCodeElement(), false); 27699 } 27700 if (element.hasDisplayElement()) { 27701 composeStringCore("display", element.getDisplayElement(), false); 27702 composeStringExtras("display", element.getDisplayElement(), false); 27703 } 27704 if (element.hasContains()) { 27705 openArray("contains"); 27706 for (ValueSet.ValueSetExpansionContainsComponent e : element.getContains()) 27707 composeValueSetValueSetExpansionContainsComponent(null, e); 27708 closeArray(); 27709 } 27710 ; 27711 } 27712 27713 protected void composeVisionPrescription(String name, VisionPrescription element) throws IOException { 27714 if (element != null) { 27715 prop("resourceType", name); 27716 composeVisionPrescriptionInner(element); 27717 } 27718 } 27719 27720 protected void composeVisionPrescriptionInner(VisionPrescription element) throws IOException { 27721 composeDomainResourceElements(element); 27722 if (element.hasIdentifier()) { 27723 openArray("identifier"); 27724 for (Identifier e : element.getIdentifier()) 27725 composeIdentifier(null, e); 27726 closeArray(); 27727 } 27728 ; 27729 if (element.hasDateWrittenElement()) { 27730 composeDateTimeCore("dateWritten", element.getDateWrittenElement(), false); 27731 composeDateTimeExtras("dateWritten", element.getDateWrittenElement(), false); 27732 } 27733 if (element.hasPatient()) { 27734 composeReference("patient", element.getPatient()); 27735 } 27736 if (element.hasPrescriber()) { 27737 composeReference("prescriber", element.getPrescriber()); 27738 } 27739 if (element.hasEncounter()) { 27740 composeReference("encounter", element.getEncounter()); 27741 } 27742 if (element.hasReason()) { 27743 composeType("reason", element.getReason()); 27744 } 27745 if (element.hasDispense()) { 27746 openArray("dispense"); 27747 for (VisionPrescription.VisionPrescriptionDispenseComponent e : element.getDispense()) 27748 composeVisionPrescriptionVisionPrescriptionDispenseComponent(null, e); 27749 closeArray(); 27750 } 27751 ; 27752 } 27753 27754 protected void composeVisionPrescriptionVisionPrescriptionDispenseComponent(String name, 27755 VisionPrescription.VisionPrescriptionDispenseComponent element) throws IOException { 27756 if (element != null) { 27757 open(name); 27758 composeVisionPrescriptionVisionPrescriptionDispenseComponentInner(element); 27759 close(); 27760 } 27761 } 27762 27763 protected void composeVisionPrescriptionVisionPrescriptionDispenseComponentInner( 27764 VisionPrescription.VisionPrescriptionDispenseComponent element) throws IOException { 27765 composeBackbone(element); 27766 if (element.hasProduct()) { 27767 composeCoding("product", element.getProduct()); 27768 } 27769 if (element.hasEyeElement()) { 27770 composeEnumerationCore("eye", element.getEyeElement(), new VisionPrescription.VisionEyesEnumFactory(), false); 27771 composeEnumerationExtras("eye", element.getEyeElement(), new VisionPrescription.VisionEyesEnumFactory(), false); 27772 } 27773 if (element.hasSphereElement()) { 27774 composeDecimalCore("sphere", element.getSphereElement(), false); 27775 composeDecimalExtras("sphere", element.getSphereElement(), false); 27776 } 27777 if (element.hasCylinderElement()) { 27778 composeDecimalCore("cylinder", element.getCylinderElement(), false); 27779 composeDecimalExtras("cylinder", element.getCylinderElement(), false); 27780 } 27781 if (element.hasAxisElement()) { 27782 composeIntegerCore("axis", element.getAxisElement(), false); 27783 composeIntegerExtras("axis", element.getAxisElement(), false); 27784 } 27785 if (element.hasPrismElement()) { 27786 composeDecimalCore("prism", element.getPrismElement(), false); 27787 composeDecimalExtras("prism", element.getPrismElement(), false); 27788 } 27789 if (element.hasBaseElement()) { 27790 composeEnumerationCore("base", element.getBaseElement(), new VisionPrescription.VisionBaseEnumFactory(), false); 27791 composeEnumerationExtras("base", element.getBaseElement(), new VisionPrescription.VisionBaseEnumFactory(), false); 27792 } 27793 if (element.hasAddElement()) { 27794 composeDecimalCore("add", element.getAddElement(), false); 27795 composeDecimalExtras("add", element.getAddElement(), false); 27796 } 27797 if (element.hasPowerElement()) { 27798 composeDecimalCore("power", element.getPowerElement(), false); 27799 composeDecimalExtras("power", element.getPowerElement(), false); 27800 } 27801 if (element.hasBackCurveElement()) { 27802 composeDecimalCore("backCurve", element.getBackCurveElement(), false); 27803 composeDecimalExtras("backCurve", element.getBackCurveElement(), false); 27804 } 27805 if (element.hasDiameterElement()) { 27806 composeDecimalCore("diameter", element.getDiameterElement(), false); 27807 composeDecimalExtras("diameter", element.getDiameterElement(), false); 27808 } 27809 if (element.hasDuration()) { 27810 composeSimpleQuantity("duration", element.getDuration()); 27811 } 27812 if (element.hasColorElement()) { 27813 composeStringCore("color", element.getColorElement(), false); 27814 composeStringExtras("color", element.getColorElement(), false); 27815 } 27816 if (element.hasBrandElement()) { 27817 composeStringCore("brand", element.getBrandElement(), false); 27818 composeStringExtras("brand", element.getBrandElement(), false); 27819 } 27820 if (element.hasNotesElement()) { 27821 composeStringCore("notes", element.getNotesElement(), false); 27822 composeStringExtras("notes", element.getNotesElement(), false); 27823 } 27824 } 27825 27826 @Override 27827 protected void composeResource(Resource resource) throws IOException { 27828 if (resource instanceof Parameters) 27829 composeParameters("Parameters", (Parameters) resource); 27830 else if (resource instanceof Account) 27831 composeAccount("Account", (Account) resource); 27832 else if (resource instanceof AllergyIntolerance) 27833 composeAllergyIntolerance("AllergyIntolerance", (AllergyIntolerance) resource); 27834 else if (resource instanceof Appointment) 27835 composeAppointment("Appointment", (Appointment) resource); 27836 else if (resource instanceof AppointmentResponse) 27837 composeAppointmentResponse("AppointmentResponse", (AppointmentResponse) resource); 27838 else if (resource instanceof AuditEvent) 27839 composeAuditEvent("AuditEvent", (AuditEvent) resource); 27840 else if (resource instanceof Basic) 27841 composeBasic("Basic", (Basic) resource); 27842 else if (resource instanceof Binary) 27843 composeBinary("Binary", (Binary) resource); 27844 else if (resource instanceof BodySite) 27845 composeBodySite("BodySite", (BodySite) resource); 27846 else if (resource instanceof Bundle) 27847 composeBundle("Bundle", (Bundle) resource); 27848 else if (resource instanceof CarePlan) 27849 composeCarePlan("CarePlan", (CarePlan) resource); 27850 else if (resource instanceof Claim) 27851 composeClaim("Claim", (Claim) resource); 27852 else if (resource instanceof ClaimResponse) 27853 composeClaimResponse("ClaimResponse", (ClaimResponse) resource); 27854 else if (resource instanceof ClinicalImpression) 27855 composeClinicalImpression("ClinicalImpression", (ClinicalImpression) resource); 27856 else if (resource instanceof Communication) 27857 composeCommunication("Communication", (Communication) resource); 27858 else if (resource instanceof CommunicationRequest) 27859 composeCommunicationRequest("CommunicationRequest", (CommunicationRequest) resource); 27860 else if (resource instanceof Composition) 27861 composeComposition("Composition", (Composition) resource); 27862 else if (resource instanceof ConceptMap) 27863 composeConceptMap("ConceptMap", (ConceptMap) resource); 27864 else if (resource instanceof Condition) 27865 composeCondition("Condition", (Condition) resource); 27866 else if (resource instanceof Conformance) 27867 composeConformance("Conformance", (Conformance) resource); 27868 else if (resource instanceof Contract) 27869 composeContract("Contract", (Contract) resource); 27870 else if (resource instanceof Coverage) 27871 composeCoverage("Coverage", (Coverage) resource); 27872 else if (resource instanceof DataElement) 27873 composeDataElement("DataElement", (DataElement) resource); 27874 else if (resource instanceof DetectedIssue) 27875 composeDetectedIssue("DetectedIssue", (DetectedIssue) resource); 27876 else if (resource instanceof Device) 27877 composeDevice("Device", (Device) resource); 27878 else if (resource instanceof DeviceComponent) 27879 composeDeviceComponent("DeviceComponent", (DeviceComponent) resource); 27880 else if (resource instanceof DeviceMetric) 27881 composeDeviceMetric("DeviceMetric", (DeviceMetric) resource); 27882 else if (resource instanceof DeviceUseRequest) 27883 composeDeviceUseRequest("DeviceUseRequest", (DeviceUseRequest) resource); 27884 else if (resource instanceof DeviceUseStatement) 27885 composeDeviceUseStatement("DeviceUseStatement", (DeviceUseStatement) resource); 27886 else if (resource instanceof DiagnosticOrder) 27887 composeDiagnosticOrder("DiagnosticOrder", (DiagnosticOrder) resource); 27888 else if (resource instanceof DiagnosticReport) 27889 composeDiagnosticReport("DiagnosticReport", (DiagnosticReport) resource); 27890 else if (resource instanceof DocumentManifest) 27891 composeDocumentManifest("DocumentManifest", (DocumentManifest) resource); 27892 else if (resource instanceof DocumentReference) 27893 composeDocumentReference("DocumentReference", (DocumentReference) resource); 27894 else if (resource instanceof EligibilityRequest) 27895 composeEligibilityRequest("EligibilityRequest", (EligibilityRequest) resource); 27896 else if (resource instanceof EligibilityResponse) 27897 composeEligibilityResponse("EligibilityResponse", (EligibilityResponse) resource); 27898 else if (resource instanceof Encounter) 27899 composeEncounter("Encounter", (Encounter) resource); 27900 else if (resource instanceof EnrollmentRequest) 27901 composeEnrollmentRequest("EnrollmentRequest", (EnrollmentRequest) resource); 27902 else if (resource instanceof EnrollmentResponse) 27903 composeEnrollmentResponse("EnrollmentResponse", (EnrollmentResponse) resource); 27904 else if (resource instanceof EpisodeOfCare) 27905 composeEpisodeOfCare("EpisodeOfCare", (EpisodeOfCare) resource); 27906 else if (resource instanceof ExplanationOfBenefit) 27907 composeExplanationOfBenefit("ExplanationOfBenefit", (ExplanationOfBenefit) resource); 27908 else if (resource instanceof FamilyMemberHistory) 27909 composeFamilyMemberHistory("FamilyMemberHistory", (FamilyMemberHistory) resource); 27910 else if (resource instanceof Flag) 27911 composeFlag("Flag", (Flag) resource); 27912 else if (resource instanceof Goal) 27913 composeGoal("Goal", (Goal) resource); 27914 else if (resource instanceof Group) 27915 composeGroup("Group", (Group) resource); 27916 else if (resource instanceof HealthcareService) 27917 composeHealthcareService("HealthcareService", (HealthcareService) resource); 27918 else if (resource instanceof ImagingObjectSelection) 27919 composeImagingObjectSelection("ImagingObjectSelection", (ImagingObjectSelection) resource); 27920 else if (resource instanceof ImagingStudy) 27921 composeImagingStudy("ImagingStudy", (ImagingStudy) resource); 27922 else if (resource instanceof Immunization) 27923 composeImmunization("Immunization", (Immunization) resource); 27924 else if (resource instanceof ImmunizationRecommendation) 27925 composeImmunizationRecommendation("ImmunizationRecommendation", (ImmunizationRecommendation) resource); 27926 else if (resource instanceof ImplementationGuide) 27927 composeImplementationGuide("ImplementationGuide", (ImplementationGuide) resource); 27928 else if (resource instanceof List_) 27929 composeList_("List", (List_) resource); 27930 else if (resource instanceof Location) 27931 composeLocation("Location", (Location) resource); 27932 else if (resource instanceof Media) 27933 composeMedia("Media", (Media) resource); 27934 else if (resource instanceof Medication) 27935 composeMedication("Medication", (Medication) resource); 27936 else if (resource instanceof MedicationAdministration) 27937 composeMedicationAdministration("MedicationAdministration", (MedicationAdministration) resource); 27938 else if (resource instanceof MedicationDispense) 27939 composeMedicationDispense("MedicationDispense", (MedicationDispense) resource); 27940 else if (resource instanceof MedicationOrder) 27941 composeMedicationOrder("MedicationOrder", (MedicationOrder) resource); 27942 else if (resource instanceof MedicationStatement) 27943 composeMedicationStatement("MedicationStatement", (MedicationStatement) resource); 27944 else if (resource instanceof MessageHeader) 27945 composeMessageHeader("MessageHeader", (MessageHeader) resource); 27946 else if (resource instanceof NamingSystem) 27947 composeNamingSystem("NamingSystem", (NamingSystem) resource); 27948 else if (resource instanceof NutritionOrder) 27949 composeNutritionOrder("NutritionOrder", (NutritionOrder) resource); 27950 else if (resource instanceof Observation) 27951 composeObservation("Observation", (Observation) resource); 27952 else if (resource instanceof OperationDefinition) 27953 composeOperationDefinition("OperationDefinition", (OperationDefinition) resource); 27954 else if (resource instanceof OperationOutcome) 27955 composeOperationOutcome("OperationOutcome", (OperationOutcome) resource); 27956 else if (resource instanceof Order) 27957 composeOrder("Order", (Order) resource); 27958 else if (resource instanceof OrderResponse) 27959 composeOrderResponse("OrderResponse", (OrderResponse) resource); 27960 else if (resource instanceof Organization) 27961 composeOrganization("Organization", (Organization) resource); 27962 else if (resource instanceof Patient) 27963 composePatient("Patient", (Patient) resource); 27964 else if (resource instanceof PaymentNotice) 27965 composePaymentNotice("PaymentNotice", (PaymentNotice) resource); 27966 else if (resource instanceof PaymentReconciliation) 27967 composePaymentReconciliation("PaymentReconciliation", (PaymentReconciliation) resource); 27968 else if (resource instanceof Person) 27969 composePerson("Person", (Person) resource); 27970 else if (resource instanceof Practitioner) 27971 composePractitioner("Practitioner", (Practitioner) resource); 27972 else if (resource instanceof Procedure) 27973 composeProcedure("Procedure", (Procedure) resource); 27974 else if (resource instanceof ProcedureRequest) 27975 composeProcedureRequest("ProcedureRequest", (ProcedureRequest) resource); 27976 else if (resource instanceof ProcessRequest) 27977 composeProcessRequest("ProcessRequest", (ProcessRequest) resource); 27978 else if (resource instanceof ProcessResponse) 27979 composeProcessResponse("ProcessResponse", (ProcessResponse) resource); 27980 else if (resource instanceof Provenance) 27981 composeProvenance("Provenance", (Provenance) resource); 27982 else if (resource instanceof Questionnaire) 27983 composeQuestionnaire("Questionnaire", (Questionnaire) resource); 27984 else if (resource instanceof QuestionnaireResponse) 27985 composeQuestionnaireResponse("QuestionnaireResponse", (QuestionnaireResponse) resource); 27986 else if (resource instanceof ReferralRequest) 27987 composeReferralRequest("ReferralRequest", (ReferralRequest) resource); 27988 else if (resource instanceof RelatedPerson) 27989 composeRelatedPerson("RelatedPerson", (RelatedPerson) resource); 27990 else if (resource instanceof RiskAssessment) 27991 composeRiskAssessment("RiskAssessment", (RiskAssessment) resource); 27992 else if (resource instanceof Schedule) 27993 composeSchedule("Schedule", (Schedule) resource); 27994 else if (resource instanceof SearchParameter) 27995 composeSearchParameter("SearchParameter", (SearchParameter) resource); 27996 else if (resource instanceof Slot) 27997 composeSlot("Slot", (Slot) resource); 27998 else if (resource instanceof Specimen) 27999 composeSpecimen("Specimen", (Specimen) resource); 28000 else if (resource instanceof StructureDefinition) 28001 composeStructureDefinition("StructureDefinition", (StructureDefinition) resource); 28002 else if (resource instanceof Subscription) 28003 composeSubscription("Subscription", (Subscription) resource); 28004 else if (resource instanceof Substance) 28005 composeSubstance("Substance", (Substance) resource); 28006 else if (resource instanceof SupplyDelivery) 28007 composeSupplyDelivery("SupplyDelivery", (SupplyDelivery) resource); 28008 else if (resource instanceof SupplyRequest) 28009 composeSupplyRequest("SupplyRequest", (SupplyRequest) resource); 28010 else if (resource instanceof TestScript) 28011 composeTestScript("TestScript", (TestScript) resource); 28012 else if (resource instanceof ValueSet) 28013 composeValueSet("ValueSet", (ValueSet) resource); 28014 else if (resource instanceof VisionPrescription) 28015 composeVisionPrescription("VisionPrescription", (VisionPrescription) resource); 28016 else if (resource instanceof Binary) 28017 composeBinary("Binary", (Binary) resource); 28018 else 28019 throw new Error("Unhandled resource type " + resource.getClass().getName()); 28020 } 28021 28022 protected void composeNamedReference(String name, Resource resource) throws IOException { 28023 if (resource instanceof Parameters) 28024 composeParameters(name, (Parameters) resource); 28025 else if (resource instanceof Account) 28026 composeAccount(name, (Account) resource); 28027 else if (resource instanceof AllergyIntolerance) 28028 composeAllergyIntolerance(name, (AllergyIntolerance) resource); 28029 else if (resource instanceof Appointment) 28030 composeAppointment(name, (Appointment) resource); 28031 else if (resource instanceof AppointmentResponse) 28032 composeAppointmentResponse(name, (AppointmentResponse) resource); 28033 else if (resource instanceof AuditEvent) 28034 composeAuditEvent(name, (AuditEvent) resource); 28035 else if (resource instanceof Basic) 28036 composeBasic(name, (Basic) resource); 28037 else if (resource instanceof Binary) 28038 composeBinary(name, (Binary) resource); 28039 else if (resource instanceof BodySite) 28040 composeBodySite(name, (BodySite) resource); 28041 else if (resource instanceof Bundle) 28042 composeBundle(name, (Bundle) resource); 28043 else if (resource instanceof CarePlan) 28044 composeCarePlan(name, (CarePlan) resource); 28045 else if (resource instanceof Claim) 28046 composeClaim(name, (Claim) resource); 28047 else if (resource instanceof ClaimResponse) 28048 composeClaimResponse(name, (ClaimResponse) resource); 28049 else if (resource instanceof ClinicalImpression) 28050 composeClinicalImpression(name, (ClinicalImpression) resource); 28051 else if (resource instanceof Communication) 28052 composeCommunication(name, (Communication) resource); 28053 else if (resource instanceof CommunicationRequest) 28054 composeCommunicationRequest(name, (CommunicationRequest) resource); 28055 else if (resource instanceof Composition) 28056 composeComposition(name, (Composition) resource); 28057 else if (resource instanceof ConceptMap) 28058 composeConceptMap(name, (ConceptMap) resource); 28059 else if (resource instanceof Condition) 28060 composeCondition(name, (Condition) resource); 28061 else if (resource instanceof Conformance) 28062 composeConformance(name, (Conformance) resource); 28063 else if (resource instanceof Contract) 28064 composeContract(name, (Contract) resource); 28065 else if (resource instanceof Coverage) 28066 composeCoverage(name, (Coverage) resource); 28067 else if (resource instanceof DataElement) 28068 composeDataElement(name, (DataElement) resource); 28069 else if (resource instanceof DetectedIssue) 28070 composeDetectedIssue(name, (DetectedIssue) resource); 28071 else if (resource instanceof Device) 28072 composeDevice(name, (Device) resource); 28073 else if (resource instanceof DeviceComponent) 28074 composeDeviceComponent(name, (DeviceComponent) resource); 28075 else if (resource instanceof DeviceMetric) 28076 composeDeviceMetric(name, (DeviceMetric) resource); 28077 else if (resource instanceof DeviceUseRequest) 28078 composeDeviceUseRequest(name, (DeviceUseRequest) resource); 28079 else if (resource instanceof DeviceUseStatement) 28080 composeDeviceUseStatement(name, (DeviceUseStatement) resource); 28081 else if (resource instanceof DiagnosticOrder) 28082 composeDiagnosticOrder(name, (DiagnosticOrder) resource); 28083 else if (resource instanceof DiagnosticReport) 28084 composeDiagnosticReport(name, (DiagnosticReport) resource); 28085 else if (resource instanceof DocumentManifest) 28086 composeDocumentManifest(name, (DocumentManifest) resource); 28087 else if (resource instanceof DocumentReference) 28088 composeDocumentReference(name, (DocumentReference) resource); 28089 else if (resource instanceof EligibilityRequest) 28090 composeEligibilityRequest(name, (EligibilityRequest) resource); 28091 else if (resource instanceof EligibilityResponse) 28092 composeEligibilityResponse(name, (EligibilityResponse) resource); 28093 else if (resource instanceof Encounter) 28094 composeEncounter(name, (Encounter) resource); 28095 else if (resource instanceof EnrollmentRequest) 28096 composeEnrollmentRequest(name, (EnrollmentRequest) resource); 28097 else if (resource instanceof EnrollmentResponse) 28098 composeEnrollmentResponse(name, (EnrollmentResponse) resource); 28099 else if (resource instanceof EpisodeOfCare) 28100 composeEpisodeOfCare(name, (EpisodeOfCare) resource); 28101 else if (resource instanceof ExplanationOfBenefit) 28102 composeExplanationOfBenefit(name, (ExplanationOfBenefit) resource); 28103 else if (resource instanceof FamilyMemberHistory) 28104 composeFamilyMemberHistory(name, (FamilyMemberHistory) resource); 28105 else if (resource instanceof Flag) 28106 composeFlag(name, (Flag) resource); 28107 else if (resource instanceof Goal) 28108 composeGoal(name, (Goal) resource); 28109 else if (resource instanceof Group) 28110 composeGroup(name, (Group) resource); 28111 else if (resource instanceof HealthcareService) 28112 composeHealthcareService(name, (HealthcareService) resource); 28113 else if (resource instanceof ImagingObjectSelection) 28114 composeImagingObjectSelection(name, (ImagingObjectSelection) resource); 28115 else if (resource instanceof ImagingStudy) 28116 composeImagingStudy(name, (ImagingStudy) resource); 28117 else if (resource instanceof Immunization) 28118 composeImmunization(name, (Immunization) resource); 28119 else if (resource instanceof ImmunizationRecommendation) 28120 composeImmunizationRecommendation(name, (ImmunizationRecommendation) resource); 28121 else if (resource instanceof ImplementationGuide) 28122 composeImplementationGuide(name, (ImplementationGuide) resource); 28123 else if (resource instanceof List_) 28124 composeList_(name, (List_) resource); 28125 else if (resource instanceof Location) 28126 composeLocation(name, (Location) resource); 28127 else if (resource instanceof Media) 28128 composeMedia(name, (Media) resource); 28129 else if (resource instanceof Medication) 28130 composeMedication(name, (Medication) resource); 28131 else if (resource instanceof MedicationAdministration) 28132 composeMedicationAdministration(name, (MedicationAdministration) resource); 28133 else if (resource instanceof MedicationDispense) 28134 composeMedicationDispense(name, (MedicationDispense) resource); 28135 else if (resource instanceof MedicationOrder) 28136 composeMedicationOrder(name, (MedicationOrder) resource); 28137 else if (resource instanceof MedicationStatement) 28138 composeMedicationStatement(name, (MedicationStatement) resource); 28139 else if (resource instanceof MessageHeader) 28140 composeMessageHeader(name, (MessageHeader) resource); 28141 else if (resource instanceof NamingSystem) 28142 composeNamingSystem(name, (NamingSystem) resource); 28143 else if (resource instanceof NutritionOrder) 28144 composeNutritionOrder(name, (NutritionOrder) resource); 28145 else if (resource instanceof Observation) 28146 composeObservation(name, (Observation) resource); 28147 else if (resource instanceof OperationDefinition) 28148 composeOperationDefinition(name, (OperationDefinition) resource); 28149 else if (resource instanceof OperationOutcome) 28150 composeOperationOutcome(name, (OperationOutcome) resource); 28151 else if (resource instanceof Order) 28152 composeOrder(name, (Order) resource); 28153 else if (resource instanceof OrderResponse) 28154 composeOrderResponse(name, (OrderResponse) resource); 28155 else if (resource instanceof Organization) 28156 composeOrganization(name, (Organization) resource); 28157 else if (resource instanceof Patient) 28158 composePatient(name, (Patient) resource); 28159 else if (resource instanceof PaymentNotice) 28160 composePaymentNotice(name, (PaymentNotice) resource); 28161 else if (resource instanceof PaymentReconciliation) 28162 composePaymentReconciliation(name, (PaymentReconciliation) resource); 28163 else if (resource instanceof Person) 28164 composePerson(name, (Person) resource); 28165 else if (resource instanceof Practitioner) 28166 composePractitioner(name, (Practitioner) resource); 28167 else if (resource instanceof Procedure) 28168 composeProcedure(name, (Procedure) resource); 28169 else if (resource instanceof ProcedureRequest) 28170 composeProcedureRequest(name, (ProcedureRequest) resource); 28171 else if (resource instanceof ProcessRequest) 28172 composeProcessRequest(name, (ProcessRequest) resource); 28173 else if (resource instanceof ProcessResponse) 28174 composeProcessResponse(name, (ProcessResponse) resource); 28175 else if (resource instanceof Provenance) 28176 composeProvenance(name, (Provenance) resource); 28177 else if (resource instanceof Questionnaire) 28178 composeQuestionnaire(name, (Questionnaire) resource); 28179 else if (resource instanceof QuestionnaireResponse) 28180 composeQuestionnaireResponse(name, (QuestionnaireResponse) resource); 28181 else if (resource instanceof ReferralRequest) 28182 composeReferralRequest(name, (ReferralRequest) resource); 28183 else if (resource instanceof RelatedPerson) 28184 composeRelatedPerson(name, (RelatedPerson) resource); 28185 else if (resource instanceof RiskAssessment) 28186 composeRiskAssessment(name, (RiskAssessment) resource); 28187 else if (resource instanceof Schedule) 28188 composeSchedule(name, (Schedule) resource); 28189 else if (resource instanceof SearchParameter) 28190 composeSearchParameter(name, (SearchParameter) resource); 28191 else if (resource instanceof Slot) 28192 composeSlot(name, (Slot) resource); 28193 else if (resource instanceof Specimen) 28194 composeSpecimen(name, (Specimen) resource); 28195 else if (resource instanceof StructureDefinition) 28196 composeStructureDefinition(name, (StructureDefinition) resource); 28197 else if (resource instanceof Subscription) 28198 composeSubscription(name, (Subscription) resource); 28199 else if (resource instanceof Substance) 28200 composeSubstance(name, (Substance) resource); 28201 else if (resource instanceof SupplyDelivery) 28202 composeSupplyDelivery(name, (SupplyDelivery) resource); 28203 else if (resource instanceof SupplyRequest) 28204 composeSupplyRequest(name, (SupplyRequest) resource); 28205 else if (resource instanceof TestScript) 28206 composeTestScript(name, (TestScript) resource); 28207 else if (resource instanceof ValueSet) 28208 composeValueSet(name, (ValueSet) resource); 28209 else if (resource instanceof VisionPrescription) 28210 composeVisionPrescription(name, (VisionPrescription) resource); 28211 else if (resource instanceof Binary) 28212 composeBinary(name, (Binary) resource); 28213 else 28214 throw new Error("Unhandled resource type " + resource.getClass().getName()); 28215 } 28216 28217 protected void composeType(String prefix, Type type) throws IOException { 28218 if (type == null) 28219 ; 28220 else if (type instanceof Money) 28221 composeMoney(prefix + "Money", (Money) type); 28222 else if (type instanceof SimpleQuantity) 28223 composeSimpleQuantity(prefix + "SimpleQuantity", (SimpleQuantity) type); 28224 else if (type instanceof Duration) 28225 composeDuration(prefix + "Duration", (Duration) type); 28226 else if (type instanceof Count) 28227 composeCount(prefix + "Count", (Count) type); 28228 else if (type instanceof Distance) 28229 composeDistance(prefix + "Distance", (Distance) type); 28230 else if (type instanceof Age) 28231 composeAge(prefix + "Age", (Age) type); 28232 else if (type instanceof Identifier) 28233 composeIdentifier(prefix + "Identifier", (Identifier) type); 28234 else if (type instanceof Coding) 28235 composeCoding(prefix + "Coding", (Coding) type); 28236 else if (type instanceof Reference) 28237 composeReference(prefix + "Reference", (Reference) type); 28238 else if (type instanceof Signature) 28239 composeSignature(prefix + "Signature", (Signature) type); 28240 else if (type instanceof SampledData) 28241 composeSampledData(prefix + "SampledData", (SampledData) type); 28242 else if (type instanceof Quantity) 28243 composeQuantity(prefix + "Quantity", (Quantity) type); 28244 else if (type instanceof Period) 28245 composePeriod(prefix + "Period", (Period) type); 28246 else if (type instanceof Attachment) 28247 composeAttachment(prefix + "Attachment", (Attachment) type); 28248 else if (type instanceof Ratio) 28249 composeRatio(prefix + "Ratio", (Ratio) type); 28250 else if (type instanceof Range) 28251 composeRange(prefix + "Range", (Range) type); 28252 else if (type instanceof Annotation) 28253 composeAnnotation(prefix + "Annotation", (Annotation) type); 28254 else if (type instanceof CodeableConcept) 28255 composeCodeableConcept(prefix + "CodeableConcept", (CodeableConcept) type); 28256 else if (type instanceof HumanName) 28257 composeHumanName(prefix + "HumanName", (HumanName) type); 28258 else if (type instanceof ContactPoint) 28259 composeContactPoint(prefix + "ContactPoint", (ContactPoint) type); 28260 else if (type instanceof Meta) 28261 composeMeta(prefix + "Meta", (Meta) type); 28262 else if (type instanceof Address) 28263 composeAddress(prefix + "Address", (Address) type); 28264 else if (type instanceof Timing) 28265 composeTiming(prefix + "Timing", (Timing) type); 28266 else if (type instanceof ElementDefinition) 28267 composeElementDefinition(prefix + "ElementDefinition", (ElementDefinition) type); 28268 else if (type instanceof DateType) { 28269 composeDateCore(prefix + "Date", (DateType) type, false); 28270 composeDateExtras(prefix + "Date", (DateType) type, false); 28271 } else if (type instanceof DateTimeType) { 28272 composeDateTimeCore(prefix + "DateTime", (DateTimeType) type, false); 28273 composeDateTimeExtras(prefix + "DateTime", (DateTimeType) type, false); 28274 } else if (type instanceof CodeType) { 28275 composeCodeCore(prefix + "Code", (CodeType) type, false); 28276 composeCodeExtras(prefix + "Code", (CodeType) type, false); 28277 } else if (type instanceof StringType) { 28278 composeStringCore(prefix + "String", (StringType) type, false); 28279 composeStringExtras(prefix + "String", (StringType) type, false); 28280 } else if (type instanceof IntegerType) { 28281 composeIntegerCore(prefix + "Integer", (IntegerType) type, false); 28282 composeIntegerExtras(prefix + "Integer", (IntegerType) type, false); 28283 } else if (type instanceof OidType) { 28284 composeOidCore(prefix + "Oid", (OidType) type, false); 28285 composeOidExtras(prefix + "Oid", (OidType) type, false); 28286 } else if (type instanceof UriType) { 28287 composeUriCore(prefix + "Uri", (UriType) type, false); 28288 composeUriExtras(prefix + "Uri", (UriType) type, false); 28289 } else if (type instanceof UuidType) { 28290 composeUuidCore(prefix + "Uuid", (UuidType) type, false); 28291 composeUuidExtras(prefix + "Uuid", (UuidType) type, false); 28292 } else if (type instanceof InstantType) { 28293 composeInstantCore(prefix + "Instant", (InstantType) type, false); 28294 composeInstantExtras(prefix + "Instant", (InstantType) type, false); 28295 } else if (type instanceof BooleanType) { 28296 composeBooleanCore(prefix + "Boolean", (BooleanType) type, false); 28297 composeBooleanExtras(prefix + "Boolean", (BooleanType) type, false); 28298 } else if (type instanceof Base64BinaryType) { 28299 composeBase64BinaryCore(prefix + "Base64Binary", (Base64BinaryType) type, false); 28300 composeBase64BinaryExtras(prefix + "Base64Binary", (Base64BinaryType) type, false); 28301 } else if (type instanceof UnsignedIntType) { 28302 composeUnsignedIntCore(prefix + "UnsignedInt", (UnsignedIntType) type, false); 28303 composeUnsignedIntExtras(prefix + "UnsignedInt", (UnsignedIntType) type, false); 28304 } else if (type instanceof MarkdownType) { 28305 composeMarkdownCore(prefix + "Markdown", (MarkdownType) type, false); 28306 composeMarkdownExtras(prefix + "Markdown", (MarkdownType) type, false); 28307 } else if (type instanceof TimeType) { 28308 composeTimeCore(prefix + "Time", (TimeType) type, false); 28309 composeTimeExtras(prefix + "Time", (TimeType) type, false); 28310 } else if (type instanceof IdType) { 28311 composeIdCore(prefix + "Id", (IdType) type, false); 28312 composeIdExtras(prefix + "Id", (IdType) type, false); 28313 } else if (type instanceof PositiveIntType) { 28314 composePositiveIntCore(prefix + "PositiveInt", (PositiveIntType) type, false); 28315 composePositiveIntExtras(prefix + "PositiveInt", (PositiveIntType) type, false); 28316 } else if (type instanceof DecimalType) { 28317 composeDecimalCore(prefix + "Decimal", (DecimalType) type, false); 28318 composeDecimalExtras(prefix + "Decimal", (DecimalType) type, false); 28319 } else 28320 throw new Error("Unhandled type"); 28321 } 28322 28323 protected void composeTypeInner(Type type) throws IOException { 28324 if (type == null) 28325 ; 28326 else if (type instanceof Identifier) 28327 composeIdentifierInner((Identifier) type); 28328 else if (type instanceof Coding) 28329 composeCodingInner((Coding) type); 28330 else if (type instanceof Reference) 28331 composeReferenceInner((Reference) type); 28332 else if (type instanceof Signature) 28333 composeSignatureInner((Signature) type); 28334 else if (type instanceof SampledData) 28335 composeSampledDataInner((SampledData) type); 28336 else if (type instanceof Quantity) 28337 composeQuantityInner((Quantity) type); 28338 else if (type instanceof Period) 28339 composePeriodInner((Period) type); 28340 else if (type instanceof Attachment) 28341 composeAttachmentInner((Attachment) type); 28342 else if (type instanceof Ratio) 28343 composeRatioInner((Ratio) type); 28344 else if (type instanceof Range) 28345 composeRangeInner((Range) type); 28346 else if (type instanceof Annotation) 28347 composeAnnotationInner((Annotation) type); 28348 else if (type instanceof CodeableConcept) 28349 composeCodeableConceptInner((CodeableConcept) type); 28350 else if (type instanceof Money) 28351 composeMoneyInner((Money) type); 28352 else if (type instanceof SimpleQuantity) 28353 composeSimpleQuantityInner((SimpleQuantity) type); 28354 else if (type instanceof Duration) 28355 composeDurationInner((Duration) type); 28356 else if (type instanceof Count) 28357 composeCountInner((Count) type); 28358 else if (type instanceof Distance) 28359 composeDistanceInner((Distance) type); 28360 else if (type instanceof Age) 28361 composeAgeInner((Age) type); 28362 else if (type instanceof HumanName) 28363 composeHumanNameInner((HumanName) type); 28364 else if (type instanceof ContactPoint) 28365 composeContactPointInner((ContactPoint) type); 28366 else if (type instanceof Meta) 28367 composeMetaInner((Meta) type); 28368 else if (type instanceof Address) 28369 composeAddressInner((Address) type); 28370 else if (type instanceof Timing) 28371 composeTimingInner((Timing) type); 28372 else if (type instanceof ElementDefinition) 28373 composeElementDefinitionInner((ElementDefinition) type); 28374 else 28375 throw new Error("Unhandled type"); 28376 } 28377 28378}