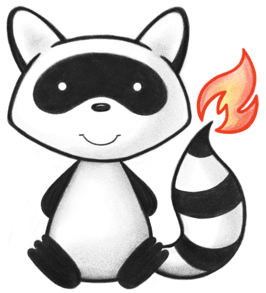
001package org.hl7.fhir.dstu2.formats; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032import java.io.ByteArrayInputStream; 033import java.io.ByteArrayOutputStream; 034import java.io.IOException; 035import java.math.BigDecimal; 036import java.nio.charset.StandardCharsets; 037import java.text.ParseException; 038import java.util.HashMap; 039import java.util.Map; 040 041import org.apache.commons.codec.binary.Base64; 042import org.hl7.fhir.dstu2.model.Resource; 043import org.hl7.fhir.dstu2.model.Type; 044import org.hl7.fhir.exceptions.FHIRFormatError; 045import org.hl7.fhir.utilities.Utilities; 046 047public abstract class ParserBase extends FormatUtilities implements IParser { 048 049 // -- implementation of variant type methods from the interface 050 // -------------------------------- 051 052 public Resource parse(String input) throws FHIRFormatError, IOException { 053 return parse(input.getBytes(StandardCharsets.UTF_8)); 054 } 055 056 public Resource parse(byte[] bytes) throws FHIRFormatError, IOException { 057 ByteArrayInputStream bi = new ByteArrayInputStream(bytes); 058 return parse(bi); 059 } 060 061 public Type parseType(String input, String typeName) throws FHIRFormatError, IOException { 062 return parseType(input.getBytes(StandardCharsets.UTF_8), typeName); 063 } 064 065 public Type parseType(byte[] bytes, String typeName) throws FHIRFormatError, IOException { 066 ByteArrayInputStream bi = new ByteArrayInputStream(bytes); 067 return parseType(bi, typeName); 068 } 069 070 public String composeString(Resource resource) throws IOException { 071 return new String(composeBytes(resource), StandardCharsets.UTF_8); 072 } 073 074 public byte[] composeBytes(Resource resource) throws IOException { 075 ByteArrayOutputStream bytes = new ByteArrayOutputStream(); 076 compose(bytes, resource); 077 bytes.close(); 078 return bytes.toByteArray(); 079 } 080 081 public String composeString(Type type, String typeName) throws IOException { 082 return new String(composeBytes(type, typeName), StandardCharsets.UTF_8); 083 } 084 085 public byte[] composeBytes(Type type, String typeName) throws IOException { 086 ByteArrayOutputStream bytes = new ByteArrayOutputStream(); 087 compose(bytes, type, typeName); 088 bytes.close(); 089 return bytes.toByteArray(); 090 } 091 092 // -- Parser Configuration -------------------------------- 093 094 protected String xhtmlMessage; 095 096 @Override 097 public IParser setSuppressXhtml(String message) { 098 xhtmlMessage = message; 099 return this; 100 } 101 102 protected boolean handleComments = true; 103 104 public boolean getHandleComments() { 105 return handleComments; 106 } 107 108 public IParser setHandleComments(boolean value) { 109 this.handleComments = value; 110 return this; 111 } 112 113 /** 114 * Whether to throw an exception if unknown content is found (or just skip it) 115 */ 116 protected boolean allowUnknownContent; 117 118 /** 119 * @return Whether to throw an exception if unknown content is found (or just 120 * skip it) 121 */ 122 public boolean isAllowUnknownContent() { 123 return allowUnknownContent; 124 } 125 126 /** 127 * @param allowUnknownContent Whether to throw an exception if unknown content 128 * is found (or just skip it) 129 */ 130 public IParser setAllowUnknownContent(boolean allowUnknownContent) { 131 this.allowUnknownContent = allowUnknownContent; 132 return this; 133 } 134 135 protected OutputStyle style = OutputStyle.NORMAL; 136 137 public OutputStyle getOutputStyle() { 138 return style; 139 } 140 141 public IParser setOutputStyle(OutputStyle style) { 142 this.style = style; 143 return this; 144 } 145 146 // -- Parser Utilities -------------------------------- 147 148 protected Map<String, Object> idMap = new HashMap<String, Object>(); 149 150 protected int parseIntegerPrimitive(String value) { 151 if (value.startsWith("+") && Utilities.isInteger(value.substring(1))) 152 value = value.substring(1); 153 return java.lang.Integer.parseInt(value); 154 } 155 156 protected int parseIntegerPrimitive(java.lang.Long value) { 157 if (value < java.lang.Integer.MIN_VALUE || value > java.lang.Integer.MAX_VALUE) { 158 throw new IllegalArgumentException(value + " cannot be cast to int without changing its value."); 159 } 160 return value.intValue(); 161 } 162 163 protected String parseCodePrimitive(String value) { 164 return value; 165 } 166 167 protected String parseTimePrimitive(String value) throws ParseException { 168 return value; 169 } 170 171 protected BigDecimal parseDecimalPrimitive(BigDecimal value) { 172 return value; 173 } 174 175 protected BigDecimal parseDecimalPrimitive(String value) { 176 return new BigDecimal(value); 177 } 178 179 protected String parseUriPrimitive(String value) { 180 return value; 181 } 182 183 protected byte[] parseBase64BinaryPrimitive(String value) { 184 return Base64.decodeBase64(value.getBytes()); 185 } 186 187 protected String parseOidPrimitive(String value) { 188 return value; 189 } 190 191 protected Boolean parseBooleanPrimitive(String value) { 192 return java.lang.Boolean.valueOf(value); 193 } 194 195 protected Boolean parseBooleanPrimitive(Boolean value) { 196 return java.lang.Boolean.valueOf(value); 197 } 198 199 protected String parseIdPrimitive(String value) { 200 return value; 201 } 202 203 protected String parseStringPrimitive(String value) { 204 return value; 205 } 206 207 protected String parseUuidPrimitive(String value) { 208 return value; 209 } 210 211}