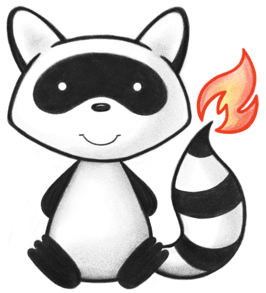
001/* 002 * #%L 003 * HAPI FHIR Structures - HL7.org DSTU2 004 * %% 005 * Copyright (C) 2014 - 2015 University Health Network 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package org.hl7.fhir.dstu2.hapi.ctx; 021 022import ca.uhn.fhir.context.ConfigurationException; 023import ca.uhn.fhir.context.FhirContext; 024import ca.uhn.fhir.context.FhirVersionEnum; 025import ca.uhn.fhir.context.RuntimeResourceDefinition; 026import ca.uhn.fhir.fhirpath.IFhirPath; 027import ca.uhn.fhir.i18n.Msg; 028import ca.uhn.fhir.model.api.IFhirVersion; 029import ca.uhn.fhir.model.base.composite.BaseCodingDt; 030import ca.uhn.fhir.rest.api.IVersionSpecificBundleFactory; 031import ca.uhn.fhir.rest.server.provider.dstu2hl7org.Dstu2Hl7OrgBundleFactory; 032import ca.uhn.fhir.util.ReflectionUtil; 033import org.apache.commons.lang3.StringUtils; 034import org.hl7.fhir.dstu2.model.IdType; 035import org.hl7.fhir.dstu2.model.Reference; 036import org.hl7.fhir.dstu2.model.Resource; 037import org.hl7.fhir.dstu2.model.StructureDefinition; 038import org.hl7.fhir.instance.model.api.IBaseResource; 039import org.hl7.fhir.instance.model.api.IIdType; 040import org.hl7.fhir.instance.model.api.IPrimitiveType; 041 042import java.io.InputStream; 043import java.util.ArrayList; 044import java.util.Date; 045 046public class FhirDstu2Hl7Org implements IFhirVersion { 047 048 private String myId; 049 050 @Override 051 public IFhirPath createFhirPathExecutor(FhirContext theFhirContext) { 052 throw new UnsupportedOperationException(Msg.code(586) + "FluentPath is not supported in DSTU2 contexts"); 053 } 054 055 @Override 056 public StructureDefinition generateProfile( 057 RuntimeResourceDefinition theRuntimeResourceDefinition, String theServerBase) { 058 StructureDefinition retVal = new StructureDefinition(); 059 060 RuntimeResourceDefinition def = theRuntimeResourceDefinition; 061 062 myId = def.getId(); 063 if (StringUtils.isBlank(myId)) { 064 myId = theRuntimeResourceDefinition.getName().toLowerCase(); 065 } 066 067 retVal.setId(myId); 068 return retVal; 069 } 070 071 @SuppressWarnings("rawtypes") 072 @Override 073 public Class<ArrayList> getContainedType() { 074 return ArrayList.class; 075 } 076 077 @Override 078 public InputStream getFhirVersionPropertiesFile() { 079 String path = "/org/hl7/fhir/instance/model/fhirversion.properties"; 080 InputStream str = FhirDstu2Hl7Org.class.getResourceAsStream(path); 081 if (str == null) { 082 str = FhirDstu2Hl7Org.class.getResourceAsStream(path.substring(1)); 083 } 084 if (str == null) { 085 throw new ConfigurationException(Msg.code(587) + "Can not find model property file on classpath: " + path); 086 } 087 return str; 088 } 089 090 @Override 091 public IPrimitiveType<Date> getLastUpdated(IBaseResource theResource) { 092 return ((Resource) theResource).getMeta().getLastUpdatedElement(); 093 } 094 095 @Override 096 public String getPathToSchemaDefinitions() { 097 return "/org/hl7/fhir/instance/model/schema"; 098 } 099 100 @Override 101 public Class<Reference> getResourceReferenceType() { 102 return Reference.class; 103 } 104 105 @Override 106 public Object getServerVersion() { 107 return ReflectionUtil.newInstanceOfFhirServerType("org.hl7.fhir.dstu2.hapi.ctx.FhirServerDstu2Hl7Org2"); 108 } 109 110 @Override 111 public FhirVersionEnum getVersion() { 112 return FhirVersionEnum.DSTU2_HL7ORG; 113 } 114 115 @Override 116 public IVersionSpecificBundleFactory newBundleFactory(FhirContext theContext) { 117 return new Dstu2Hl7OrgBundleFactory(theContext); 118 } 119 120 @Override 121 public BaseCodingDt newCodingDt() { 122 throw new UnsupportedOperationException(Msg.code(588)); 123 } 124 125 @Override 126 public IIdType newIdType() { 127 return new IdType(); 128 } 129}