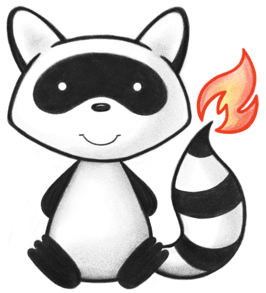
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Child; 037import ca.uhn.fhir.model.api.annotation.Description; 038import ca.uhn.fhir.model.api.annotation.ResourceDef; 039import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.utilities.Utilities; 042 043/** 044 * A financial tool for tracking value accrued for a particular purpose. In the 045 * healthcare field, used to track charges for a patient, cost centres, etc. 046 */ 047@ResourceDef(name = "Account", profile = "http://hl7.org/fhir/Profile/Account") 048public class Account extends DomainResource { 049 050 public enum AccountStatus { 051 /** 052 * This account is active and may be used. 053 */ 054 ACTIVE, 055 /** 056 * This account is inactive and should not be used to track financial 057 * information. 058 */ 059 INACTIVE, 060 /** 061 * added to help the parsers 062 */ 063 NULL; 064 065 public static AccountStatus fromCode(String codeString) throws FHIRException { 066 if (codeString == null || "".equals(codeString)) 067 return null; 068 if ("active".equals(codeString)) 069 return ACTIVE; 070 if ("inactive".equals(codeString)) 071 return INACTIVE; 072 throw new FHIRException("Unknown AccountStatus code '" + codeString + "'"); 073 } 074 075 public String toCode() { 076 switch (this) { 077 case ACTIVE: 078 return "active"; 079 case INACTIVE: 080 return "inactive"; 081 case NULL: 082 return null; 083 default: 084 return "?"; 085 } 086 } 087 088 public String getSystem() { 089 switch (this) { 090 case ACTIVE: 091 return "http://hl7.org/fhir/account-status"; 092 case INACTIVE: 093 return "http://hl7.org/fhir/account-status"; 094 case NULL: 095 return null; 096 default: 097 return "?"; 098 } 099 } 100 101 public String getDefinition() { 102 switch (this) { 103 case ACTIVE: 104 return "This account is active and may be used."; 105 case INACTIVE: 106 return "This account is inactive and should not be used to track financial information."; 107 case NULL: 108 return null; 109 default: 110 return "?"; 111 } 112 } 113 114 public String getDisplay() { 115 switch (this) { 116 case ACTIVE: 117 return "Active"; 118 case INACTIVE: 119 return "Inactive"; 120 case NULL: 121 return null; 122 default: 123 return "?"; 124 } 125 } 126 } 127 128 public static class AccountStatusEnumFactory implements EnumFactory<AccountStatus> { 129 public AccountStatus fromCode(String codeString) throws IllegalArgumentException { 130 if (codeString == null || "".equals(codeString)) 131 if (codeString == null || "".equals(codeString)) 132 return null; 133 if ("active".equals(codeString)) 134 return AccountStatus.ACTIVE; 135 if ("inactive".equals(codeString)) 136 return AccountStatus.INACTIVE; 137 throw new IllegalArgumentException("Unknown AccountStatus code '" + codeString + "'"); 138 } 139 140 public Enumeration<AccountStatus> fromType(Base code) throws FHIRException { 141 if (code == null || code.isEmpty()) 142 return null; 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return null; 146 if ("active".equals(codeString)) 147 return new Enumeration<AccountStatus>(this, AccountStatus.ACTIVE); 148 if ("inactive".equals(codeString)) 149 return new Enumeration<AccountStatus>(this, AccountStatus.INACTIVE); 150 throw new FHIRException("Unknown AccountStatus code '" + codeString + "'"); 151 } 152 153 public String toCode(AccountStatus code) 154 { 155 if (code == AccountStatus.NULL) 156 return null; 157 if (code == AccountStatus.ACTIVE) 158 return "active"; 159 if (code == AccountStatus.INACTIVE) 160 return "inactive"; 161 return "?"; 162 } 163 } 164 165 /** 166 * Unique identifier used to reference the account. May or may not be intended 167 * for human use (e.g. credit card number). 168 */ 169 @Child(name = "identifier", type = { 170 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 171 @Description(shortDefinition = "Account number", formalDefinition = "Unique identifier used to reference the account. May or may not be intended for human use (e.g. credit card number).") 172 protected List<Identifier> identifier; 173 174 /** 175 * Name used for the account when displaying it to humans in reports, etc. 176 */ 177 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 178 @Description(shortDefinition = "Human-readable label", formalDefinition = "Name used for the account when displaying it to humans in reports, etc.") 179 protected StringType name; 180 181 /** 182 * Categorizes the account for reporting and searching purposes. 183 */ 184 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 185 @Description(shortDefinition = "E.g. patient, expense, depreciation", formalDefinition = "Categorizes the account for reporting and searching purposes.") 186 protected CodeableConcept type; 187 188 /** 189 * Indicates whether the account is presently used/useable or not. 190 */ 191 @Child(name = "status", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = true, summary = true) 192 @Description(shortDefinition = "active | inactive", formalDefinition = "Indicates whether the account is presently used/useable or not.") 193 protected Enumeration<AccountStatus> status; 194 195 /** 196 * Indicates the period of time over which the account is allowed. 197 */ 198 @Child(name = "activePeriod", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 199 @Description(shortDefinition = "Valid from..to", formalDefinition = "Indicates the period of time over which the account is allowed.") 200 protected Period activePeriod; 201 202 /** 203 * Identifies the currency to which transactions must be converted when 204 * crediting or debiting the account. 205 */ 206 @Child(name = "currency", type = { Coding.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 207 @Description(shortDefinition = "Base currency in which balance is tracked", formalDefinition = "Identifies the currency to which transactions must be converted when crediting or debiting the account.") 208 protected Coding currency; 209 210 /** 211 * Represents the sum of all credits less all debits associated with the 212 * account. Might be positive, zero or negative. 213 */ 214 @Child(name = "balance", type = { Money.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 215 @Description(shortDefinition = "How much is in account?", formalDefinition = "Represents the sum of all credits less all debits associated with the account. Might be positive, zero or negative.") 216 protected Money balance; 217 218 /** 219 * Identifies the period of time the account applies to; e.g. accounts created 220 * per fiscal year, quarter, etc. 221 */ 222 @Child(name = "coveragePeriod", type = { 223 Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 224 @Description(shortDefinition = "Transaction window", formalDefinition = "Identifies the period of time the account applies to; e.g. accounts created per fiscal year, quarter, etc.") 225 protected Period coveragePeriod; 226 227 /** 228 * Identifies the patient, device, practitioner, location or other object the 229 * account is associated with. 230 */ 231 @Child(name = "subject", type = { Patient.class, Device.class, Practitioner.class, Location.class, 232 HealthcareService.class, Organization.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 233 @Description(shortDefinition = "What is account tied to?", formalDefinition = "Identifies the patient, device, practitioner, location or other object the account is associated with.") 234 protected Reference subject; 235 236 /** 237 * The actual object that is the target of the reference (Identifies the 238 * patient, device, practitioner, location or other object the account is 239 * associated with.) 240 */ 241 protected Resource subjectTarget; 242 243 /** 244 * Indicates the organization, department, etc. with responsibility for the 245 * account. 246 */ 247 @Child(name = "owner", type = { Organization.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 248 @Description(shortDefinition = "Who is responsible?", formalDefinition = "Indicates the organization, department, etc. with responsibility for the account.") 249 protected Reference owner; 250 251 /** 252 * The actual object that is the target of the reference (Indicates the 253 * organization, department, etc. with responsibility for the account.) 254 */ 255 protected Organization ownerTarget; 256 257 /** 258 * Provides additional information about what the account tracks and how it is 259 * used. 260 */ 261 @Child(name = "description", type = { 262 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 263 @Description(shortDefinition = "Explanation of purpose/use", formalDefinition = "Provides additional information about what the account tracks and how it is used.") 264 protected StringType description; 265 266 private static final long serialVersionUID = -1926153194L; 267 268 /* 269 * Constructor 270 */ 271 public Account() { 272 super(); 273 } 274 275 /** 276 * @return {@link #identifier} (Unique identifier used to reference the account. 277 * May or may not be intended for human use (e.g. credit card number).) 278 */ 279 public List<Identifier> getIdentifier() { 280 if (this.identifier == null) 281 this.identifier = new ArrayList<Identifier>(); 282 return this.identifier; 283 } 284 285 public boolean hasIdentifier() { 286 if (this.identifier == null) 287 return false; 288 for (Identifier item : this.identifier) 289 if (!item.isEmpty()) 290 return true; 291 return false; 292 } 293 294 /** 295 * @return {@link #identifier} (Unique identifier used to reference the account. 296 * May or may not be intended for human use (e.g. credit card number).) 297 */ 298 // syntactic sugar 299 public Identifier addIdentifier() { // 3 300 Identifier t = new Identifier(); 301 if (this.identifier == null) 302 this.identifier = new ArrayList<Identifier>(); 303 this.identifier.add(t); 304 return t; 305 } 306 307 // syntactic sugar 308 public Account addIdentifier(Identifier t) { // 3 309 if (t == null) 310 return this; 311 if (this.identifier == null) 312 this.identifier = new ArrayList<Identifier>(); 313 this.identifier.add(t); 314 return this; 315 } 316 317 /** 318 * @return {@link #name} (Name used for the account when displaying it to humans 319 * in reports, etc.). This is the underlying object with id, value and 320 * extensions. The accessor "getName" gives direct access to the value 321 */ 322 public StringType getNameElement() { 323 if (this.name == null) 324 if (Configuration.errorOnAutoCreate()) 325 throw new Error("Attempt to auto-create Account.name"); 326 else if (Configuration.doAutoCreate()) 327 this.name = new StringType(); // bb 328 return this.name; 329 } 330 331 public boolean hasNameElement() { 332 return this.name != null && !this.name.isEmpty(); 333 } 334 335 public boolean hasName() { 336 return this.name != null && !this.name.isEmpty(); 337 } 338 339 /** 340 * @param value {@link #name} (Name used for the account when displaying it to 341 * humans in reports, etc.). This is the underlying object with id, 342 * value and extensions. The accessor "getName" gives direct access 343 * to the value 344 */ 345 public Account setNameElement(StringType value) { 346 this.name = value; 347 return this; 348 } 349 350 /** 351 * @return Name used for the account when displaying it to humans in reports, 352 * etc. 353 */ 354 public String getName() { 355 return this.name == null ? null : this.name.getValue(); 356 } 357 358 /** 359 * @param value Name used for the account when displaying it to humans in 360 * reports, etc. 361 */ 362 public Account setName(String value) { 363 if (Utilities.noString(value)) 364 this.name = null; 365 else { 366 if (this.name == null) 367 this.name = new StringType(); 368 this.name.setValue(value); 369 } 370 return this; 371 } 372 373 /** 374 * @return {@link #type} (Categorizes the account for reporting and searching 375 * purposes.) 376 */ 377 public CodeableConcept getType() { 378 if (this.type == null) 379 if (Configuration.errorOnAutoCreate()) 380 throw new Error("Attempt to auto-create Account.type"); 381 else if (Configuration.doAutoCreate()) 382 this.type = new CodeableConcept(); // cc 383 return this.type; 384 } 385 386 public boolean hasType() { 387 return this.type != null && !this.type.isEmpty(); 388 } 389 390 /** 391 * @param value {@link #type} (Categorizes the account for reporting and 392 * searching purposes.) 393 */ 394 public Account setType(CodeableConcept value) { 395 this.type = value; 396 return this; 397 } 398 399 /** 400 * @return {@link #status} (Indicates whether the account is presently 401 * used/useable or not.). This is the underlying object with id, value 402 * and extensions. The accessor "getStatus" gives direct access to the 403 * value 404 */ 405 public Enumeration<AccountStatus> getStatusElement() { 406 if (this.status == null) 407 if (Configuration.errorOnAutoCreate()) 408 throw new Error("Attempt to auto-create Account.status"); 409 else if (Configuration.doAutoCreate()) 410 this.status = new Enumeration<AccountStatus>(new AccountStatusEnumFactory()); // bb 411 return this.status; 412 } 413 414 public boolean hasStatusElement() { 415 return this.status != null && !this.status.isEmpty(); 416 } 417 418 public boolean hasStatus() { 419 return this.status != null && !this.status.isEmpty(); 420 } 421 422 /** 423 * @param value {@link #status} (Indicates whether the account is presently 424 * used/useable or not.). This is the underlying object with id, 425 * value and extensions. The accessor "getStatus" gives direct 426 * access to the value 427 */ 428 public Account setStatusElement(Enumeration<AccountStatus> value) { 429 this.status = value; 430 return this; 431 } 432 433 /** 434 * @return Indicates whether the account is presently used/useable or not. 435 */ 436 public AccountStatus getStatus() { 437 return this.status == null ? null : this.status.getValue(); 438 } 439 440 /** 441 * @param value Indicates whether the account is presently used/useable or not. 442 */ 443 public Account setStatus(AccountStatus value) { 444 if (value == null) 445 this.status = null; 446 else { 447 if (this.status == null) 448 this.status = new Enumeration<AccountStatus>(new AccountStatusEnumFactory()); 449 this.status.setValue(value); 450 } 451 return this; 452 } 453 454 /** 455 * @return {@link #activePeriod} (Indicates the period of time over which the 456 * account is allowed.) 457 */ 458 public Period getActivePeriod() { 459 if (this.activePeriod == null) 460 if (Configuration.errorOnAutoCreate()) 461 throw new Error("Attempt to auto-create Account.activePeriod"); 462 else if (Configuration.doAutoCreate()) 463 this.activePeriod = new Period(); // cc 464 return this.activePeriod; 465 } 466 467 public boolean hasActivePeriod() { 468 return this.activePeriod != null && !this.activePeriod.isEmpty(); 469 } 470 471 /** 472 * @param value {@link #activePeriod} (Indicates the period of time over which 473 * the account is allowed.) 474 */ 475 public Account setActivePeriod(Period value) { 476 this.activePeriod = value; 477 return this; 478 } 479 480 /** 481 * @return {@link #currency} (Identifies the currency to which transactions must 482 * be converted when crediting or debiting the account.) 483 */ 484 public Coding getCurrency() { 485 if (this.currency == null) 486 if (Configuration.errorOnAutoCreate()) 487 throw new Error("Attempt to auto-create Account.currency"); 488 else if (Configuration.doAutoCreate()) 489 this.currency = new Coding(); // cc 490 return this.currency; 491 } 492 493 public boolean hasCurrency() { 494 return this.currency != null && !this.currency.isEmpty(); 495 } 496 497 /** 498 * @param value {@link #currency} (Identifies the currency to which transactions 499 * must be converted when crediting or debiting the account.) 500 */ 501 public Account setCurrency(Coding value) { 502 this.currency = value; 503 return this; 504 } 505 506 /** 507 * @return {@link #balance} (Represents the sum of all credits less all debits 508 * associated with the account. Might be positive, zero or negative.) 509 */ 510 public Money getBalance() { 511 if (this.balance == null) 512 if (Configuration.errorOnAutoCreate()) 513 throw new Error("Attempt to auto-create Account.balance"); 514 else if (Configuration.doAutoCreate()) 515 this.balance = new Money(); // cc 516 return this.balance; 517 } 518 519 public boolean hasBalance() { 520 return this.balance != null && !this.balance.isEmpty(); 521 } 522 523 /** 524 * @param value {@link #balance} (Represents the sum of all credits less all 525 * debits associated with the account. Might be positive, zero or 526 * negative.) 527 */ 528 public Account setBalance(Money value) { 529 this.balance = value; 530 return this; 531 } 532 533 /** 534 * @return {@link #coveragePeriod} (Identifies the period of time the account 535 * applies to; e.g. accounts created per fiscal year, quarter, etc.) 536 */ 537 public Period getCoveragePeriod() { 538 if (this.coveragePeriod == null) 539 if (Configuration.errorOnAutoCreate()) 540 throw new Error("Attempt to auto-create Account.coveragePeriod"); 541 else if (Configuration.doAutoCreate()) 542 this.coveragePeriod = new Period(); // cc 543 return this.coveragePeriod; 544 } 545 546 public boolean hasCoveragePeriod() { 547 return this.coveragePeriod != null && !this.coveragePeriod.isEmpty(); 548 } 549 550 /** 551 * @param value {@link #coveragePeriod} (Identifies the period of time the 552 * account applies to; e.g. accounts created per fiscal year, 553 * quarter, etc.) 554 */ 555 public Account setCoveragePeriod(Period value) { 556 this.coveragePeriod = value; 557 return this; 558 } 559 560 /** 561 * @return {@link #subject} (Identifies the patient, device, practitioner, 562 * location or other object the account is associated with.) 563 */ 564 public Reference getSubject() { 565 if (this.subject == null) 566 if (Configuration.errorOnAutoCreate()) 567 throw new Error("Attempt to auto-create Account.subject"); 568 else if (Configuration.doAutoCreate()) 569 this.subject = new Reference(); // cc 570 return this.subject; 571 } 572 573 public boolean hasSubject() { 574 return this.subject != null && !this.subject.isEmpty(); 575 } 576 577 /** 578 * @param value {@link #subject} (Identifies the patient, device, practitioner, 579 * location or other object the account is associated with.) 580 */ 581 public Account setSubject(Reference value) { 582 this.subject = value; 583 return this; 584 } 585 586 /** 587 * @return {@link #subject} The actual object that is the target of the 588 * reference. The reference library doesn't populate this, but you can 589 * use it to hold the resource if you resolve it. (Identifies the 590 * patient, device, practitioner, location or other object the account 591 * is associated with.) 592 */ 593 public Resource getSubjectTarget() { 594 return this.subjectTarget; 595 } 596 597 /** 598 * @param value {@link #subject} The actual object that is the target of the 599 * reference. The reference library doesn't use these, but you can 600 * use it to hold the resource if you resolve it. (Identifies the 601 * patient, device, practitioner, location or other object the 602 * account is associated with.) 603 */ 604 public Account setSubjectTarget(Resource value) { 605 this.subjectTarget = value; 606 return this; 607 } 608 609 /** 610 * @return {@link #owner} (Indicates the organization, department, etc. with 611 * responsibility for the account.) 612 */ 613 public Reference getOwner() { 614 if (this.owner == null) 615 if (Configuration.errorOnAutoCreate()) 616 throw new Error("Attempt to auto-create Account.owner"); 617 else if (Configuration.doAutoCreate()) 618 this.owner = new Reference(); // cc 619 return this.owner; 620 } 621 622 public boolean hasOwner() { 623 return this.owner != null && !this.owner.isEmpty(); 624 } 625 626 /** 627 * @param value {@link #owner} (Indicates the organization, department, etc. 628 * with responsibility for the account.) 629 */ 630 public Account setOwner(Reference value) { 631 this.owner = value; 632 return this; 633 } 634 635 /** 636 * @return {@link #owner} The actual object that is the target of the reference. 637 * The reference library doesn't populate this, but you can use it to 638 * hold the resource if you resolve it. (Indicates the organization, 639 * department, etc. with responsibility for the account.) 640 */ 641 public Organization getOwnerTarget() { 642 if (this.ownerTarget == null) 643 if (Configuration.errorOnAutoCreate()) 644 throw new Error("Attempt to auto-create Account.owner"); 645 else if (Configuration.doAutoCreate()) 646 this.ownerTarget = new Organization(); // aa 647 return this.ownerTarget; 648 } 649 650 /** 651 * @param value {@link #owner} The actual object that is the target of the 652 * reference. The reference library doesn't use these, but you can 653 * use it to hold the resource if you resolve it. (Indicates the 654 * organization, department, etc. with responsibility for the 655 * account.) 656 */ 657 public Account setOwnerTarget(Organization value) { 658 this.ownerTarget = value; 659 return this; 660 } 661 662 /** 663 * @return {@link #description} (Provides additional information about what the 664 * account tracks and how it is used.). This is the underlying object 665 * with id, value and extensions. The accessor "getDescription" gives 666 * direct access to the value 667 */ 668 public StringType getDescriptionElement() { 669 if (this.description == null) 670 if (Configuration.errorOnAutoCreate()) 671 throw new Error("Attempt to auto-create Account.description"); 672 else if (Configuration.doAutoCreate()) 673 this.description = new StringType(); // bb 674 return this.description; 675 } 676 677 public boolean hasDescriptionElement() { 678 return this.description != null && !this.description.isEmpty(); 679 } 680 681 public boolean hasDescription() { 682 return this.description != null && !this.description.isEmpty(); 683 } 684 685 /** 686 * @param value {@link #description} (Provides additional information about what 687 * the account tracks and how it is used.). This is the underlying 688 * object with id, value and extensions. The accessor 689 * "getDescription" gives direct access to the value 690 */ 691 public Account setDescriptionElement(StringType value) { 692 this.description = value; 693 return this; 694 } 695 696 /** 697 * @return Provides additional information about what the account tracks and how 698 * it is used. 699 */ 700 public String getDescription() { 701 return this.description == null ? null : this.description.getValue(); 702 } 703 704 /** 705 * @param value Provides additional information about what the account tracks 706 * and how it is used. 707 */ 708 public Account setDescription(String value) { 709 if (Utilities.noString(value)) 710 this.description = null; 711 else { 712 if (this.description == null) 713 this.description = new StringType(); 714 this.description.setValue(value); 715 } 716 return this; 717 } 718 719 protected void listChildren(List<Property> childrenList) { 720 super.listChildren(childrenList); 721 childrenList.add(new Property("identifier", "Identifier", 722 "Unique identifier used to reference the account. May or may not be intended for human use (e.g. credit card number).", 723 0, java.lang.Integer.MAX_VALUE, identifier)); 724 childrenList 725 .add(new Property("name", "string", "Name used for the account when displaying it to humans in reports, etc.", 726 0, java.lang.Integer.MAX_VALUE, name)); 727 childrenList.add(new Property("type", "CodeableConcept", 728 "Categorizes the account for reporting and searching purposes.", 0, java.lang.Integer.MAX_VALUE, type)); 729 childrenList.add(new Property("status", "code", "Indicates whether the account is presently used/useable or not.", 730 0, java.lang.Integer.MAX_VALUE, status)); 731 childrenList 732 .add(new Property("activePeriod", "Period", "Indicates the period of time over which the account is allowed.", 733 0, java.lang.Integer.MAX_VALUE, activePeriod)); 734 childrenList.add(new Property("currency", "Coding", 735 "Identifies the currency to which transactions must be converted when crediting or debiting the account.", 0, 736 java.lang.Integer.MAX_VALUE, currency)); 737 childrenList.add(new Property("balance", "Money", 738 "Represents the sum of all credits less all debits associated with the account. Might be positive, zero or negative.", 739 0, java.lang.Integer.MAX_VALUE, balance)); 740 childrenList.add(new Property("coveragePeriod", "Period", 741 "Identifies the period of time the account applies to; e.g. accounts created per fiscal year, quarter, etc.", 0, 742 java.lang.Integer.MAX_VALUE, coveragePeriod)); 743 childrenList 744 .add(new Property("subject", "Reference(Patient|Device|Practitioner|Location|HealthcareService|Organization)", 745 "Identifies the patient, device, practitioner, location or other object the account is associated with.", 0, 746 java.lang.Integer.MAX_VALUE, subject)); 747 childrenList.add(new Property("owner", "Reference(Organization)", 748 "Indicates the organization, department, etc. with responsibility for the account.", 0, 749 java.lang.Integer.MAX_VALUE, owner)); 750 childrenList.add(new Property("description", "string", 751 "Provides additional information about what the account tracks and how it is used.", 0, 752 java.lang.Integer.MAX_VALUE, description)); 753 } 754 755 @Override 756 public void setProperty(String name, Base value) throws FHIRException { 757 if (name.equals("identifier")) 758 this.getIdentifier().add(castToIdentifier(value)); 759 else if (name.equals("name")) 760 this.name = castToString(value); // StringType 761 else if (name.equals("type")) 762 this.type = castToCodeableConcept(value); // CodeableConcept 763 else if (name.equals("status")) 764 this.status = new AccountStatusEnumFactory().fromType(value); // Enumeration<AccountStatus> 765 else if (name.equals("activePeriod")) 766 this.activePeriod = castToPeriod(value); // Period 767 else if (name.equals("currency")) 768 this.currency = castToCoding(value); // Coding 769 else if (name.equals("balance")) 770 this.balance = castToMoney(value); // Money 771 else if (name.equals("coveragePeriod")) 772 this.coveragePeriod = castToPeriod(value); // Period 773 else if (name.equals("subject")) 774 this.subject = castToReference(value); // Reference 775 else if (name.equals("owner")) 776 this.owner = castToReference(value); // Reference 777 else if (name.equals("description")) 778 this.description = castToString(value); // StringType 779 else 780 super.setProperty(name, value); 781 } 782 783 @Override 784 public Base addChild(String name) throws FHIRException { 785 if (name.equals("identifier")) { 786 return addIdentifier(); 787 } else if (name.equals("name")) { 788 throw new FHIRException("Cannot call addChild on a singleton property Account.name"); 789 } else if (name.equals("type")) { 790 this.type = new CodeableConcept(); 791 return this.type; 792 } else if (name.equals("status")) { 793 throw new FHIRException("Cannot call addChild on a singleton property Account.status"); 794 } else if (name.equals("activePeriod")) { 795 this.activePeriod = new Period(); 796 return this.activePeriod; 797 } else if (name.equals("currency")) { 798 this.currency = new Coding(); 799 return this.currency; 800 } else if (name.equals("balance")) { 801 this.balance = new Money(); 802 return this.balance; 803 } else if (name.equals("coveragePeriod")) { 804 this.coveragePeriod = new Period(); 805 return this.coveragePeriod; 806 } else if (name.equals("subject")) { 807 this.subject = new Reference(); 808 return this.subject; 809 } else if (name.equals("owner")) { 810 this.owner = new Reference(); 811 return this.owner; 812 } else if (name.equals("description")) { 813 throw new FHIRException("Cannot call addChild on a singleton property Account.description"); 814 } else 815 return super.addChild(name); 816 } 817 818 public String fhirType() { 819 return "Account"; 820 821 } 822 823 public Account copy() { 824 Account dst = new Account(); 825 copyValues(dst); 826 if (identifier != null) { 827 dst.identifier = new ArrayList<Identifier>(); 828 for (Identifier i : identifier) 829 dst.identifier.add(i.copy()); 830 } 831 ; 832 dst.name = name == null ? null : name.copy(); 833 dst.type = type == null ? null : type.copy(); 834 dst.status = status == null ? null : status.copy(); 835 dst.activePeriod = activePeriod == null ? null : activePeriod.copy(); 836 dst.currency = currency == null ? null : currency.copy(); 837 dst.balance = balance == null ? null : balance.copy(); 838 dst.coveragePeriod = coveragePeriod == null ? null : coveragePeriod.copy(); 839 dst.subject = subject == null ? null : subject.copy(); 840 dst.owner = owner == null ? null : owner.copy(); 841 dst.description = description == null ? null : description.copy(); 842 return dst; 843 } 844 845 protected Account typedCopy() { 846 return copy(); 847 } 848 849 @Override 850 public boolean equalsDeep(Base other) { 851 if (!super.equalsDeep(other)) 852 return false; 853 if (!(other instanceof Account)) 854 return false; 855 Account o = (Account) other; 856 return compareDeep(identifier, o.identifier, true) && compareDeep(name, o.name, true) 857 && compareDeep(type, o.type, true) && compareDeep(status, o.status, true) 858 && compareDeep(activePeriod, o.activePeriod, true) && compareDeep(currency, o.currency, true) 859 && compareDeep(balance, o.balance, true) && compareDeep(coveragePeriod, o.coveragePeriod, true) 860 && compareDeep(subject, o.subject, true) && compareDeep(owner, o.owner, true) 861 && compareDeep(description, o.description, true); 862 } 863 864 @Override 865 public boolean equalsShallow(Base other) { 866 if (!super.equalsShallow(other)) 867 return false; 868 if (!(other instanceof Account)) 869 return false; 870 Account o = (Account) other; 871 return compareValues(name, o.name, true) && compareValues(status, o.status, true) 872 && compareValues(description, o.description, true); 873 } 874 875 public boolean isEmpty() { 876 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (name == null || name.isEmpty()) 877 && (type == null || type.isEmpty()) && (status == null || status.isEmpty()) 878 && (activePeriod == null || activePeriod.isEmpty()) && (currency == null || currency.isEmpty()) 879 && (balance == null || balance.isEmpty()) && (coveragePeriod == null || coveragePeriod.isEmpty()) 880 && (subject == null || subject.isEmpty()) && (owner == null || owner.isEmpty()) 881 && (description == null || description.isEmpty()); 882 } 883 884 @Override 885 public ResourceType getResourceType() { 886 return ResourceType.Account; 887 } 888 889 @SearchParamDefinition(name = "owner", path = "Account.owner", description = "Who is responsible?", type = "reference") 890 public static final String SP_OWNER = "owner"; 891 @SearchParamDefinition(name = "identifier", path = "Account.identifier", description = "Account number", type = "token") 892 public static final String SP_IDENTIFIER = "identifier"; 893 @SearchParamDefinition(name = "period", path = "Account.coveragePeriod", description = "Transaction window", type = "date") 894 public static final String SP_PERIOD = "period"; 895 @SearchParamDefinition(name = "balance", path = "Account.balance", description = "How much is in account?", type = "quantity") 896 public static final String SP_BALANCE = "balance"; 897 @SearchParamDefinition(name = "subject", path = "Account.subject", description = "What is account tied to?", type = "reference") 898 public static final String SP_SUBJECT = "subject"; 899 @SearchParamDefinition(name = "patient", path = "Account.subject", description = "What is account tied to?", type = "reference") 900 public static final String SP_PATIENT = "patient"; 901 @SearchParamDefinition(name = "name", path = "Account.name", description = "Human-readable label", type = "string") 902 public static final String SP_NAME = "name"; 903 @SearchParamDefinition(name = "type", path = "Account.type", description = "E.g. patient, expense, depreciation", type = "token") 904 public static final String SP_TYPE = "type"; 905 @SearchParamDefinition(name = "status", path = "Account.status", description = "active | inactive", type = "token") 906 public static final String SP_STATUS = "status"; 907 908}