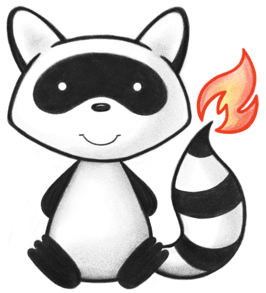
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Child; 037import ca.uhn.fhir.model.api.annotation.Description; 038import ca.uhn.fhir.model.api.annotation.ResourceDef; 039import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.utilities.Utilities; 042 043/** 044 * A financial tool for tracking value accrued for a particular purpose. In the 045 * healthcare field, used to track charges for a patient, cost centres, etc. 046 */ 047@ResourceDef(name = "Account", profile = "http://hl7.org/fhir/Profile/Account") 048public class Account extends DomainResource { 049 050 public enum AccountStatus { 051 /** 052 * This account is active and may be used. 053 */ 054 ACTIVE, 055 /** 056 * This account is inactive and should not be used to track financial 057 * information. 058 */ 059 INACTIVE, 060 /** 061 * added to help the parsers 062 */ 063 NULL; 064 065 public static AccountStatus fromCode(String codeString) throws FHIRException { 066 if (codeString == null || "".equals(codeString)) 067 return null; 068 if ("active".equals(codeString)) 069 return ACTIVE; 070 if ("inactive".equals(codeString)) 071 return INACTIVE; 072 throw new FHIRException("Unknown AccountStatus code '" + codeString + "'"); 073 } 074 075 public String toCode() { 076 switch (this) { 077 case ACTIVE: 078 return "active"; 079 case INACTIVE: 080 return "inactive"; 081 case NULL: 082 return null; 083 default: 084 return "?"; 085 } 086 } 087 088 public String getSystem() { 089 switch (this) { 090 case ACTIVE: 091 return "http://hl7.org/fhir/account-status"; 092 case INACTIVE: 093 return "http://hl7.org/fhir/account-status"; 094 case NULL: 095 return null; 096 default: 097 return "?"; 098 } 099 } 100 101 public String getDefinition() { 102 switch (this) { 103 case ACTIVE: 104 return "This account is active and may be used."; 105 case INACTIVE: 106 return "This account is inactive and should not be used to track financial information."; 107 case NULL: 108 return null; 109 default: 110 return "?"; 111 } 112 } 113 114 public String getDisplay() { 115 switch (this) { 116 case ACTIVE: 117 return "Active"; 118 case INACTIVE: 119 return "Inactive"; 120 case NULL: 121 return null; 122 default: 123 return "?"; 124 } 125 } 126 } 127 128 public static class AccountStatusEnumFactory implements EnumFactory<AccountStatus> { 129 public AccountStatus fromCode(String codeString) throws IllegalArgumentException { 130 if (codeString == null || "".equals(codeString)) 131 if (codeString == null || "".equals(codeString)) 132 return null; 133 if ("active".equals(codeString)) 134 return AccountStatus.ACTIVE; 135 if ("inactive".equals(codeString)) 136 return AccountStatus.INACTIVE; 137 throw new IllegalArgumentException("Unknown AccountStatus code '" + codeString + "'"); 138 } 139 140 public Enumeration<AccountStatus> fromType(Base code) throws FHIRException { 141 if (code == null || code.isEmpty()) 142 return null; 143 String codeString = ((PrimitiveType) code).asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return null; 146 if ("active".equals(codeString)) 147 return new Enumeration<AccountStatus>(this, AccountStatus.ACTIVE); 148 if ("inactive".equals(codeString)) 149 return new Enumeration<AccountStatus>(this, AccountStatus.INACTIVE); 150 throw new FHIRException("Unknown AccountStatus code '" + codeString + "'"); 151 } 152 153 public String toCode(AccountStatus code) { 154 if (code == AccountStatus.ACTIVE) 155 return "active"; 156 if (code == AccountStatus.INACTIVE) 157 return "inactive"; 158 return "?"; 159 } 160 } 161 162 /** 163 * Unique identifier used to reference the account. May or may not be intended 164 * for human use (e.g. credit card number). 165 */ 166 @Child(name = "identifier", type = { 167 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 168 @Description(shortDefinition = "Account number", formalDefinition = "Unique identifier used to reference the account. May or may not be intended for human use (e.g. credit card number).") 169 protected List<Identifier> identifier; 170 171 /** 172 * Name used for the account when displaying it to humans in reports, etc. 173 */ 174 @Child(name = "name", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 175 @Description(shortDefinition = "Human-readable label", formalDefinition = "Name used for the account when displaying it to humans in reports, etc.") 176 protected StringType name; 177 178 /** 179 * Categorizes the account for reporting and searching purposes. 180 */ 181 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 182 @Description(shortDefinition = "E.g. patient, expense, depreciation", formalDefinition = "Categorizes the account for reporting and searching purposes.") 183 protected CodeableConcept type; 184 185 /** 186 * Indicates whether the account is presently used/useable or not. 187 */ 188 @Child(name = "status", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = true, summary = true) 189 @Description(shortDefinition = "active | inactive", formalDefinition = "Indicates whether the account is presently used/useable or not.") 190 protected Enumeration<AccountStatus> status; 191 192 /** 193 * Indicates the period of time over which the account is allowed. 194 */ 195 @Child(name = "activePeriod", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 196 @Description(shortDefinition = "Valid from..to", formalDefinition = "Indicates the period of time over which the account is allowed.") 197 protected Period activePeriod; 198 199 /** 200 * Identifies the currency to which transactions must be converted when 201 * crediting or debiting the account. 202 */ 203 @Child(name = "currency", type = { Coding.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 204 @Description(shortDefinition = "Base currency in which balance is tracked", formalDefinition = "Identifies the currency to which transactions must be converted when crediting or debiting the account.") 205 protected Coding currency; 206 207 /** 208 * Represents the sum of all credits less all debits associated with the 209 * account. Might be positive, zero or negative. 210 */ 211 @Child(name = "balance", type = { Money.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 212 @Description(shortDefinition = "How much is in account?", formalDefinition = "Represents the sum of all credits less all debits associated with the account. Might be positive, zero or negative.") 213 protected Money balance; 214 215 /** 216 * Identifies the period of time the account applies to; e.g. accounts created 217 * per fiscal year, quarter, etc. 218 */ 219 @Child(name = "coveragePeriod", type = { 220 Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 221 @Description(shortDefinition = "Transaction window", formalDefinition = "Identifies the period of time the account applies to; e.g. accounts created per fiscal year, quarter, etc.") 222 protected Period coveragePeriod; 223 224 /** 225 * Identifies the patient, device, practitioner, location or other object the 226 * account is associated with. 227 */ 228 @Child(name = "subject", type = { Patient.class, Device.class, Practitioner.class, Location.class, 229 HealthcareService.class, Organization.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 230 @Description(shortDefinition = "What is account tied to?", formalDefinition = "Identifies the patient, device, practitioner, location or other object the account is associated with.") 231 protected Reference subject; 232 233 /** 234 * The actual object that is the target of the reference (Identifies the 235 * patient, device, practitioner, location or other object the account is 236 * associated with.) 237 */ 238 protected Resource subjectTarget; 239 240 /** 241 * Indicates the organization, department, etc. with responsibility for the 242 * account. 243 */ 244 @Child(name = "owner", type = { Organization.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 245 @Description(shortDefinition = "Who is responsible?", formalDefinition = "Indicates the organization, department, etc. with responsibility for the account.") 246 protected Reference owner; 247 248 /** 249 * The actual object that is the target of the reference (Indicates the 250 * organization, department, etc. with responsibility for the account.) 251 */ 252 protected Organization ownerTarget; 253 254 /** 255 * Provides additional information about what the account tracks and how it is 256 * used. 257 */ 258 @Child(name = "description", type = { 259 StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 260 @Description(shortDefinition = "Explanation of purpose/use", formalDefinition = "Provides additional information about what the account tracks and how it is used.") 261 protected StringType description; 262 263 private static final long serialVersionUID = -1926153194L; 264 265 /* 266 * Constructor 267 */ 268 public Account() { 269 super(); 270 } 271 272 /** 273 * @return {@link #identifier} (Unique identifier used to reference the account. 274 * May or may not be intended for human use (e.g. credit card number).) 275 */ 276 public List<Identifier> getIdentifier() { 277 if (this.identifier == null) 278 this.identifier = new ArrayList<Identifier>(); 279 return this.identifier; 280 } 281 282 public boolean hasIdentifier() { 283 if (this.identifier == null) 284 return false; 285 for (Identifier item : this.identifier) 286 if (!item.isEmpty()) 287 return true; 288 return false; 289 } 290 291 /** 292 * @return {@link #identifier} (Unique identifier used to reference the account. 293 * May or may not be intended for human use (e.g. credit card number).) 294 */ 295 // syntactic sugar 296 public Identifier addIdentifier() { // 3 297 Identifier t = new Identifier(); 298 if (this.identifier == null) 299 this.identifier = new ArrayList<Identifier>(); 300 this.identifier.add(t); 301 return t; 302 } 303 304 // syntactic sugar 305 public Account addIdentifier(Identifier t) { // 3 306 if (t == null) 307 return this; 308 if (this.identifier == null) 309 this.identifier = new ArrayList<Identifier>(); 310 this.identifier.add(t); 311 return this; 312 } 313 314 /** 315 * @return {@link #name} (Name used for the account when displaying it to humans 316 * in reports, etc.). This is the underlying object with id, value and 317 * extensions. The accessor "getName" gives direct access to the value 318 */ 319 public StringType getNameElement() { 320 if (this.name == null) 321 if (Configuration.errorOnAutoCreate()) 322 throw new Error("Attempt to auto-create Account.name"); 323 else if (Configuration.doAutoCreate()) 324 this.name = new StringType(); // bb 325 return this.name; 326 } 327 328 public boolean hasNameElement() { 329 return this.name != null && !this.name.isEmpty(); 330 } 331 332 public boolean hasName() { 333 return this.name != null && !this.name.isEmpty(); 334 } 335 336 /** 337 * @param value {@link #name} (Name used for the account when displaying it to 338 * humans in reports, etc.). This is the underlying object with id, 339 * value and extensions. The accessor "getName" gives direct access 340 * to the value 341 */ 342 public Account setNameElement(StringType value) { 343 this.name = value; 344 return this; 345 } 346 347 /** 348 * @return Name used for the account when displaying it to humans in reports, 349 * etc. 350 */ 351 public String getName() { 352 return this.name == null ? null : this.name.getValue(); 353 } 354 355 /** 356 * @param value Name used for the account when displaying it to humans in 357 * reports, etc. 358 */ 359 public Account setName(String value) { 360 if (Utilities.noString(value)) 361 this.name = null; 362 else { 363 if (this.name == null) 364 this.name = new StringType(); 365 this.name.setValue(value); 366 } 367 return this; 368 } 369 370 /** 371 * @return {@link #type} (Categorizes the account for reporting and searching 372 * purposes.) 373 */ 374 public CodeableConcept getType() { 375 if (this.type == null) 376 if (Configuration.errorOnAutoCreate()) 377 throw new Error("Attempt to auto-create Account.type"); 378 else if (Configuration.doAutoCreate()) 379 this.type = new CodeableConcept(); // cc 380 return this.type; 381 } 382 383 public boolean hasType() { 384 return this.type != null && !this.type.isEmpty(); 385 } 386 387 /** 388 * @param value {@link #type} (Categorizes the account for reporting and 389 * searching purposes.) 390 */ 391 public Account setType(CodeableConcept value) { 392 this.type = value; 393 return this; 394 } 395 396 /** 397 * @return {@link #status} (Indicates whether the account is presently 398 * used/useable or not.). This is the underlying object with id, value 399 * and extensions. The accessor "getStatus" gives direct access to the 400 * value 401 */ 402 public Enumeration<AccountStatus> getStatusElement() { 403 if (this.status == null) 404 if (Configuration.errorOnAutoCreate()) 405 throw new Error("Attempt to auto-create Account.status"); 406 else if (Configuration.doAutoCreate()) 407 this.status = new Enumeration<AccountStatus>(new AccountStatusEnumFactory()); // bb 408 return this.status; 409 } 410 411 public boolean hasStatusElement() { 412 return this.status != null && !this.status.isEmpty(); 413 } 414 415 public boolean hasStatus() { 416 return this.status != null && !this.status.isEmpty(); 417 } 418 419 /** 420 * @param value {@link #status} (Indicates whether the account is presently 421 * used/useable or not.). This is the underlying object with id, 422 * value and extensions. The accessor "getStatus" gives direct 423 * access to the value 424 */ 425 public Account setStatusElement(Enumeration<AccountStatus> value) { 426 this.status = value; 427 return this; 428 } 429 430 /** 431 * @return Indicates whether the account is presently used/useable or not. 432 */ 433 public AccountStatus getStatus() { 434 return this.status == null ? null : this.status.getValue(); 435 } 436 437 /** 438 * @param value Indicates whether the account is presently used/useable or not. 439 */ 440 public Account setStatus(AccountStatus value) { 441 if (value == null) 442 this.status = null; 443 else { 444 if (this.status == null) 445 this.status = new Enumeration<AccountStatus>(new AccountStatusEnumFactory()); 446 this.status.setValue(value); 447 } 448 return this; 449 } 450 451 /** 452 * @return {@link #activePeriod} (Indicates the period of time over which the 453 * account is allowed.) 454 */ 455 public Period getActivePeriod() { 456 if (this.activePeriod == null) 457 if (Configuration.errorOnAutoCreate()) 458 throw new Error("Attempt to auto-create Account.activePeriod"); 459 else if (Configuration.doAutoCreate()) 460 this.activePeriod = new Period(); // cc 461 return this.activePeriod; 462 } 463 464 public boolean hasActivePeriod() { 465 return this.activePeriod != null && !this.activePeriod.isEmpty(); 466 } 467 468 /** 469 * @param value {@link #activePeriod} (Indicates the period of time over which 470 * the account is allowed.) 471 */ 472 public Account setActivePeriod(Period value) { 473 this.activePeriod = value; 474 return this; 475 } 476 477 /** 478 * @return {@link #currency} (Identifies the currency to which transactions must 479 * be converted when crediting or debiting the account.) 480 */ 481 public Coding getCurrency() { 482 if (this.currency == null) 483 if (Configuration.errorOnAutoCreate()) 484 throw new Error("Attempt to auto-create Account.currency"); 485 else if (Configuration.doAutoCreate()) 486 this.currency = new Coding(); // cc 487 return this.currency; 488 } 489 490 public boolean hasCurrency() { 491 return this.currency != null && !this.currency.isEmpty(); 492 } 493 494 /** 495 * @param value {@link #currency} (Identifies the currency to which transactions 496 * must be converted when crediting or debiting the account.) 497 */ 498 public Account setCurrency(Coding value) { 499 this.currency = value; 500 return this; 501 } 502 503 /** 504 * @return {@link #balance} (Represents the sum of all credits less all debits 505 * associated with the account. Might be positive, zero or negative.) 506 */ 507 public Money getBalance() { 508 if (this.balance == null) 509 if (Configuration.errorOnAutoCreate()) 510 throw new Error("Attempt to auto-create Account.balance"); 511 else if (Configuration.doAutoCreate()) 512 this.balance = new Money(); // cc 513 return this.balance; 514 } 515 516 public boolean hasBalance() { 517 return this.balance != null && !this.balance.isEmpty(); 518 } 519 520 /** 521 * @param value {@link #balance} (Represents the sum of all credits less all 522 * debits associated with the account. Might be positive, zero or 523 * negative.) 524 */ 525 public Account setBalance(Money value) { 526 this.balance = value; 527 return this; 528 } 529 530 /** 531 * @return {@link #coveragePeriod} (Identifies the period of time the account 532 * applies to; e.g. accounts created per fiscal year, quarter, etc.) 533 */ 534 public Period getCoveragePeriod() { 535 if (this.coveragePeriod == null) 536 if (Configuration.errorOnAutoCreate()) 537 throw new Error("Attempt to auto-create Account.coveragePeriod"); 538 else if (Configuration.doAutoCreate()) 539 this.coveragePeriod = new Period(); // cc 540 return this.coveragePeriod; 541 } 542 543 public boolean hasCoveragePeriod() { 544 return this.coveragePeriod != null && !this.coveragePeriod.isEmpty(); 545 } 546 547 /** 548 * @param value {@link #coveragePeriod} (Identifies the period of time the 549 * account applies to; e.g. accounts created per fiscal year, 550 * quarter, etc.) 551 */ 552 public Account setCoveragePeriod(Period value) { 553 this.coveragePeriod = value; 554 return this; 555 } 556 557 /** 558 * @return {@link #subject} (Identifies the patient, device, practitioner, 559 * location or other object the account is associated with.) 560 */ 561 public Reference getSubject() { 562 if (this.subject == null) 563 if (Configuration.errorOnAutoCreate()) 564 throw new Error("Attempt to auto-create Account.subject"); 565 else if (Configuration.doAutoCreate()) 566 this.subject = new Reference(); // cc 567 return this.subject; 568 } 569 570 public boolean hasSubject() { 571 return this.subject != null && !this.subject.isEmpty(); 572 } 573 574 /** 575 * @param value {@link #subject} (Identifies the patient, device, practitioner, 576 * location or other object the account is associated with.) 577 */ 578 public Account setSubject(Reference value) { 579 this.subject = value; 580 return this; 581 } 582 583 /** 584 * @return {@link #subject} The actual object that is the target of the 585 * reference. The reference library doesn't populate this, but you can 586 * use it to hold the resource if you resolve it. (Identifies the 587 * patient, device, practitioner, location or other object the account 588 * is associated with.) 589 */ 590 public Resource getSubjectTarget() { 591 return this.subjectTarget; 592 } 593 594 /** 595 * @param value {@link #subject} The actual object that is the target of the 596 * reference. The reference library doesn't use these, but you can 597 * use it to hold the resource if you resolve it. (Identifies the 598 * patient, device, practitioner, location or other object the 599 * account is associated with.) 600 */ 601 public Account setSubjectTarget(Resource value) { 602 this.subjectTarget = value; 603 return this; 604 } 605 606 /** 607 * @return {@link #owner} (Indicates the organization, department, etc. with 608 * responsibility for the account.) 609 */ 610 public Reference getOwner() { 611 if (this.owner == null) 612 if (Configuration.errorOnAutoCreate()) 613 throw new Error("Attempt to auto-create Account.owner"); 614 else if (Configuration.doAutoCreate()) 615 this.owner = new Reference(); // cc 616 return this.owner; 617 } 618 619 public boolean hasOwner() { 620 return this.owner != null && !this.owner.isEmpty(); 621 } 622 623 /** 624 * @param value {@link #owner} (Indicates the organization, department, etc. 625 * with responsibility for the account.) 626 */ 627 public Account setOwner(Reference value) { 628 this.owner = value; 629 return this; 630 } 631 632 /** 633 * @return {@link #owner} The actual object that is the target of the reference. 634 * The reference library doesn't populate this, but you can use it to 635 * hold the resource if you resolve it. (Indicates the organization, 636 * department, etc. with responsibility for the account.) 637 */ 638 public Organization getOwnerTarget() { 639 if (this.ownerTarget == null) 640 if (Configuration.errorOnAutoCreate()) 641 throw new Error("Attempt to auto-create Account.owner"); 642 else if (Configuration.doAutoCreate()) 643 this.ownerTarget = new Organization(); // aa 644 return this.ownerTarget; 645 } 646 647 /** 648 * @param value {@link #owner} The actual object that is the target of the 649 * reference. The reference library doesn't use these, but you can 650 * use it to hold the resource if you resolve it. (Indicates the 651 * organization, department, etc. with responsibility for the 652 * account.) 653 */ 654 public Account setOwnerTarget(Organization value) { 655 this.ownerTarget = value; 656 return this; 657 } 658 659 /** 660 * @return {@link #description} (Provides additional information about what the 661 * account tracks and how it is used.). This is the underlying object 662 * with id, value and extensions. The accessor "getDescription" gives 663 * direct access to the value 664 */ 665 public StringType getDescriptionElement() { 666 if (this.description == null) 667 if (Configuration.errorOnAutoCreate()) 668 throw new Error("Attempt to auto-create Account.description"); 669 else if (Configuration.doAutoCreate()) 670 this.description = new StringType(); // bb 671 return this.description; 672 } 673 674 public boolean hasDescriptionElement() { 675 return this.description != null && !this.description.isEmpty(); 676 } 677 678 public boolean hasDescription() { 679 return this.description != null && !this.description.isEmpty(); 680 } 681 682 /** 683 * @param value {@link #description} (Provides additional information about what 684 * the account tracks and how it is used.). This is the underlying 685 * object with id, value and extensions. The accessor 686 * "getDescription" gives direct access to the value 687 */ 688 public Account setDescriptionElement(StringType value) { 689 this.description = value; 690 return this; 691 } 692 693 /** 694 * @return Provides additional information about what the account tracks and how 695 * it is used. 696 */ 697 public String getDescription() { 698 return this.description == null ? null : this.description.getValue(); 699 } 700 701 /** 702 * @param value Provides additional information about what the account tracks 703 * and how it is used. 704 */ 705 public Account setDescription(String value) { 706 if (Utilities.noString(value)) 707 this.description = null; 708 else { 709 if (this.description == null) 710 this.description = new StringType(); 711 this.description.setValue(value); 712 } 713 return this; 714 } 715 716 protected void listChildren(List<Property> childrenList) { 717 super.listChildren(childrenList); 718 childrenList.add(new Property("identifier", "Identifier", 719 "Unique identifier used to reference the account. May or may not be intended for human use (e.g. credit card number).", 720 0, java.lang.Integer.MAX_VALUE, identifier)); 721 childrenList 722 .add(new Property("name", "string", "Name used for the account when displaying it to humans in reports, etc.", 723 0, java.lang.Integer.MAX_VALUE, name)); 724 childrenList.add(new Property("type", "CodeableConcept", 725 "Categorizes the account for reporting and searching purposes.", 0, java.lang.Integer.MAX_VALUE, type)); 726 childrenList.add(new Property("status", "code", "Indicates whether the account is presently used/useable or not.", 727 0, java.lang.Integer.MAX_VALUE, status)); 728 childrenList 729 .add(new Property("activePeriod", "Period", "Indicates the period of time over which the account is allowed.", 730 0, java.lang.Integer.MAX_VALUE, activePeriod)); 731 childrenList.add(new Property("currency", "Coding", 732 "Identifies the currency to which transactions must be converted when crediting or debiting the account.", 0, 733 java.lang.Integer.MAX_VALUE, currency)); 734 childrenList.add(new Property("balance", "Money", 735 "Represents the sum of all credits less all debits associated with the account. Might be positive, zero or negative.", 736 0, java.lang.Integer.MAX_VALUE, balance)); 737 childrenList.add(new Property("coveragePeriod", "Period", 738 "Identifies the period of time the account applies to; e.g. accounts created per fiscal year, quarter, etc.", 0, 739 java.lang.Integer.MAX_VALUE, coveragePeriod)); 740 childrenList 741 .add(new Property("subject", "Reference(Patient|Device|Practitioner|Location|HealthcareService|Organization)", 742 "Identifies the patient, device, practitioner, location or other object the account is associated with.", 0, 743 java.lang.Integer.MAX_VALUE, subject)); 744 childrenList.add(new Property("owner", "Reference(Organization)", 745 "Indicates the organization, department, etc. with responsibility for the account.", 0, 746 java.lang.Integer.MAX_VALUE, owner)); 747 childrenList.add(new Property("description", "string", 748 "Provides additional information about what the account tracks and how it is used.", 0, 749 java.lang.Integer.MAX_VALUE, description)); 750 } 751 752 @Override 753 public void setProperty(String name, Base value) throws FHIRException { 754 if (name.equals("identifier")) 755 this.getIdentifier().add(castToIdentifier(value)); 756 else if (name.equals("name")) 757 this.name = castToString(value); // StringType 758 else if (name.equals("type")) 759 this.type = castToCodeableConcept(value); // CodeableConcept 760 else if (name.equals("status")) 761 this.status = new AccountStatusEnumFactory().fromType(value); // Enumeration<AccountStatus> 762 else if (name.equals("activePeriod")) 763 this.activePeriod = castToPeriod(value); // Period 764 else if (name.equals("currency")) 765 this.currency = castToCoding(value); // Coding 766 else if (name.equals("balance")) 767 this.balance = castToMoney(value); // Money 768 else if (name.equals("coveragePeriod")) 769 this.coveragePeriod = castToPeriod(value); // Period 770 else if (name.equals("subject")) 771 this.subject = castToReference(value); // Reference 772 else if (name.equals("owner")) 773 this.owner = castToReference(value); // Reference 774 else if (name.equals("description")) 775 this.description = castToString(value); // StringType 776 else 777 super.setProperty(name, value); 778 } 779 780 @Override 781 public Base addChild(String name) throws FHIRException { 782 if (name.equals("identifier")) { 783 return addIdentifier(); 784 } else if (name.equals("name")) { 785 throw new FHIRException("Cannot call addChild on a singleton property Account.name"); 786 } else if (name.equals("type")) { 787 this.type = new CodeableConcept(); 788 return this.type; 789 } else if (name.equals("status")) { 790 throw new FHIRException("Cannot call addChild on a singleton property Account.status"); 791 } else if (name.equals("activePeriod")) { 792 this.activePeriod = new Period(); 793 return this.activePeriod; 794 } else if (name.equals("currency")) { 795 this.currency = new Coding(); 796 return this.currency; 797 } else if (name.equals("balance")) { 798 this.balance = new Money(); 799 return this.balance; 800 } else if (name.equals("coveragePeriod")) { 801 this.coveragePeriod = new Period(); 802 return this.coveragePeriod; 803 } else if (name.equals("subject")) { 804 this.subject = new Reference(); 805 return this.subject; 806 } else if (name.equals("owner")) { 807 this.owner = new Reference(); 808 return this.owner; 809 } else if (name.equals("description")) { 810 throw new FHIRException("Cannot call addChild on a singleton property Account.description"); 811 } else 812 return super.addChild(name); 813 } 814 815 public String fhirType() { 816 return "Account"; 817 818 } 819 820 public Account copy() { 821 Account dst = new Account(); 822 copyValues(dst); 823 if (identifier != null) { 824 dst.identifier = new ArrayList<Identifier>(); 825 for (Identifier i : identifier) 826 dst.identifier.add(i.copy()); 827 } 828 ; 829 dst.name = name == null ? null : name.copy(); 830 dst.type = type == null ? null : type.copy(); 831 dst.status = status == null ? null : status.copy(); 832 dst.activePeriod = activePeriod == null ? null : activePeriod.copy(); 833 dst.currency = currency == null ? null : currency.copy(); 834 dst.balance = balance == null ? null : balance.copy(); 835 dst.coveragePeriod = coveragePeriod == null ? null : coveragePeriod.copy(); 836 dst.subject = subject == null ? null : subject.copy(); 837 dst.owner = owner == null ? null : owner.copy(); 838 dst.description = description == null ? null : description.copy(); 839 return dst; 840 } 841 842 protected Account typedCopy() { 843 return copy(); 844 } 845 846 @Override 847 public boolean equalsDeep(Base other) { 848 if (!super.equalsDeep(other)) 849 return false; 850 if (!(other instanceof Account)) 851 return false; 852 Account o = (Account) other; 853 return compareDeep(identifier, o.identifier, true) && compareDeep(name, o.name, true) 854 && compareDeep(type, o.type, true) && compareDeep(status, o.status, true) 855 && compareDeep(activePeriod, o.activePeriod, true) && compareDeep(currency, o.currency, true) 856 && compareDeep(balance, o.balance, true) && compareDeep(coveragePeriod, o.coveragePeriod, true) 857 && compareDeep(subject, o.subject, true) && compareDeep(owner, o.owner, true) 858 && compareDeep(description, o.description, true); 859 } 860 861 @Override 862 public boolean equalsShallow(Base other) { 863 if (!super.equalsShallow(other)) 864 return false; 865 if (!(other instanceof Account)) 866 return false; 867 Account o = (Account) other; 868 return compareValues(name, o.name, true) && compareValues(status, o.status, true) 869 && compareValues(description, o.description, true); 870 } 871 872 public boolean isEmpty() { 873 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (name == null || name.isEmpty()) 874 && (type == null || type.isEmpty()) && (status == null || status.isEmpty()) 875 && (activePeriod == null || activePeriod.isEmpty()) && (currency == null || currency.isEmpty()) 876 && (balance == null || balance.isEmpty()) && (coveragePeriod == null || coveragePeriod.isEmpty()) 877 && (subject == null || subject.isEmpty()) && (owner == null || owner.isEmpty()) 878 && (description == null || description.isEmpty()); 879 } 880 881 @Override 882 public ResourceType getResourceType() { 883 return ResourceType.Account; 884 } 885 886 @SearchParamDefinition(name = "owner", path = "Account.owner", description = "Who is responsible?", type = "reference") 887 public static final String SP_OWNER = "owner"; 888 @SearchParamDefinition(name = "identifier", path = "Account.identifier", description = "Account number", type = "token") 889 public static final String SP_IDENTIFIER = "identifier"; 890 @SearchParamDefinition(name = "period", path = "Account.coveragePeriod", description = "Transaction window", type = "date") 891 public static final String SP_PERIOD = "period"; 892 @SearchParamDefinition(name = "balance", path = "Account.balance", description = "How much is in account?", type = "quantity") 893 public static final String SP_BALANCE = "balance"; 894 @SearchParamDefinition(name = "subject", path = "Account.subject", description = "What is account tied to?", type = "reference") 895 public static final String SP_SUBJECT = "subject"; 896 @SearchParamDefinition(name = "patient", path = "Account.subject", description = "What is account tied to?", type = "reference") 897 public static final String SP_PATIENT = "patient"; 898 @SearchParamDefinition(name = "name", path = "Account.name", description = "Human-readable label", type = "string") 899 public static final String SP_NAME = "name"; 900 @SearchParamDefinition(name = "type", path = "Account.type", description = "E.g. patient, expense, depreciation", type = "token") 901 public static final String SP_TYPE = "type"; 902 @SearchParamDefinition(name = "status", path = "Account.status", description = "active | inactive", type = "token") 903 public static final String SP_STATUS = "status"; 904 905}