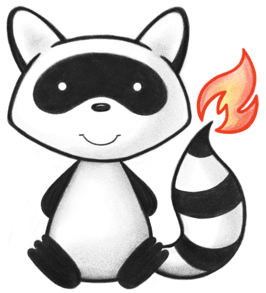
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Child; 037import ca.uhn.fhir.model.api.annotation.DatatypeDef; 038import ca.uhn.fhir.model.api.annotation.Description; 039import org.hl7.fhir.instance.model.api.ICompositeType; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.utilities.Utilities; 042 043/** 044 * There is a variety of postal address formats defined around the world. This 045 * format defines a superset that is the basis for all addresses around the 046 * world. 047 */ 048@DatatypeDef(name = "Address") 049public class Address extends Type implements ICompositeType { 050 051 public enum AddressUse { 052 /** 053 * A communication address at a home. 054 */ 055 HOME, 056 /** 057 * An office address. First choice for business related contacts during business 058 * hours. 059 */ 060 WORK, 061 /** 062 * A temporary address. The period can provide more detailed information. 063 */ 064 TEMP, 065 /** 066 * This address is no longer in use (or was never correct, but retained for 067 * records). 068 */ 069 OLD, 070 /** 071 * added to help the parsers 072 */ 073 NULL; 074 075 public static AddressUse fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("home".equals(codeString)) 079 return HOME; 080 if ("work".equals(codeString)) 081 return WORK; 082 if ("temp".equals(codeString)) 083 return TEMP; 084 if ("old".equals(codeString)) 085 return OLD; 086 throw new FHIRException("Unknown AddressUse code '" + codeString + "'"); 087 } 088 089 public String toCode() { 090 switch (this) { 091 case HOME: 092 return "home"; 093 case WORK: 094 return "work"; 095 case TEMP: 096 return "temp"; 097 case OLD: 098 return "old"; 099 case NULL: 100 return null; 101 default: 102 return "?"; 103 } 104 } 105 106 public String getSystem() { 107 switch (this) { 108 case HOME: 109 return "http://hl7.org/fhir/address-use"; 110 case WORK: 111 return "http://hl7.org/fhir/address-use"; 112 case TEMP: 113 return "http://hl7.org/fhir/address-use"; 114 case OLD: 115 return "http://hl7.org/fhir/address-use"; 116 case NULL: 117 return null; 118 default: 119 return "?"; 120 } 121 } 122 123 public String getDefinition() { 124 switch (this) { 125 case HOME: 126 return "A communication address at a home."; 127 case WORK: 128 return "An office address. First choice for business related contacts during business hours."; 129 case TEMP: 130 return "A temporary address. The period can provide more detailed information."; 131 case OLD: 132 return "This address is no longer in use (or was never correct, but retained for records)."; 133 case NULL: 134 return null; 135 default: 136 return "?"; 137 } 138 } 139 140 public String getDisplay() { 141 switch (this) { 142 case HOME: 143 return "Home"; 144 case WORK: 145 return "Work"; 146 case TEMP: 147 return "Temporary"; 148 case OLD: 149 return "Old / Incorrect"; 150 case NULL: 151 return null; 152 default: 153 return "?"; 154 } 155 } 156 } 157 158 public static class AddressUseEnumFactory implements EnumFactory<AddressUse> { 159 public AddressUse fromCode(String codeString) throws IllegalArgumentException { 160 if (codeString == null || "".equals(codeString)) 161 if (codeString == null || "".equals(codeString)) 162 return null; 163 if ("home".equals(codeString)) 164 return AddressUse.HOME; 165 if ("work".equals(codeString)) 166 return AddressUse.WORK; 167 if ("temp".equals(codeString)) 168 return AddressUse.TEMP; 169 if ("old".equals(codeString)) 170 return AddressUse.OLD; 171 throw new IllegalArgumentException("Unknown AddressUse code '" + codeString + "'"); 172 } 173 174 public Enumeration<AddressUse> fromType(Base code) throws FHIRException { 175 if (code == null || code.isEmpty()) 176 return null; 177 String codeString = ((PrimitiveType) code).asStringValue(); 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("home".equals(codeString)) 181 return new Enumeration<AddressUse>(this, AddressUse.HOME); 182 if ("work".equals(codeString)) 183 return new Enumeration<AddressUse>(this, AddressUse.WORK); 184 if ("temp".equals(codeString)) 185 return new Enumeration<AddressUse>(this, AddressUse.TEMP); 186 if ("old".equals(codeString)) 187 return new Enumeration<AddressUse>(this, AddressUse.OLD); 188 throw new FHIRException("Unknown AddressUse code '" + codeString + "'"); 189 } 190 191 public String toCode(AddressUse code) 192 { 193 if (code == AddressUse.NULL) 194 return null; 195 if (code == AddressUse.HOME) 196 return "home"; 197 if (code == AddressUse.WORK) 198 return "work"; 199 if (code == AddressUse.TEMP) 200 return "temp"; 201 if (code == AddressUse.OLD) 202 return "old"; 203 return "?"; 204 } 205 } 206 207 public enum AddressType { 208 /** 209 * Mailing addresses - PO Boxes and care-of addresses. 210 */ 211 POSTAL, 212 /** 213 * A physical address that can be visited. 214 */ 215 PHYSICAL, 216 /** 217 * An address that is both physical and postal. 218 */ 219 BOTH, 220 /** 221 * added to help the parsers 222 */ 223 NULL; 224 225 public static AddressType fromCode(String codeString) throws FHIRException { 226 if (codeString == null || "".equals(codeString)) 227 return null; 228 if ("postal".equals(codeString)) 229 return POSTAL; 230 if ("physical".equals(codeString)) 231 return PHYSICAL; 232 if ("both".equals(codeString)) 233 return BOTH; 234 throw new FHIRException("Unknown AddressType code '" + codeString + "'"); 235 } 236 237 public String toCode() { 238 switch (this) { 239 case POSTAL: 240 return "postal"; 241 case PHYSICAL: 242 return "physical"; 243 case BOTH: 244 return "both"; 245 case NULL: 246 return null; 247 default: 248 return "?"; 249 } 250 } 251 252 public String getSystem() { 253 switch (this) { 254 case POSTAL: 255 return "http://hl7.org/fhir/address-type"; 256 case PHYSICAL: 257 return "http://hl7.org/fhir/address-type"; 258 case BOTH: 259 return "http://hl7.org/fhir/address-type"; 260 case NULL: 261 return null; 262 default: 263 return "?"; 264 } 265 } 266 267 public String getDefinition() { 268 switch (this) { 269 case POSTAL: 270 return "Mailing addresses - PO Boxes and care-of addresses."; 271 case PHYSICAL: 272 return "A physical address that can be visited."; 273 case BOTH: 274 return "An address that is both physical and postal."; 275 case NULL: 276 return null; 277 default: 278 return "?"; 279 } 280 } 281 282 public String getDisplay() { 283 switch (this) { 284 case POSTAL: 285 return "Postal"; 286 case PHYSICAL: 287 return "Physical"; 288 case BOTH: 289 return "Postal & Physical"; 290 case NULL: 291 return null; 292 default: 293 return "?"; 294 } 295 } 296 } 297 298 public static class AddressTypeEnumFactory implements EnumFactory<AddressType> { 299 public AddressType fromCode(String codeString) throws IllegalArgumentException { 300 if (codeString == null || "".equals(codeString)) 301 if (codeString == null || "".equals(codeString)) 302 return null; 303 if ("postal".equals(codeString)) 304 return AddressType.POSTAL; 305 if ("physical".equals(codeString)) 306 return AddressType.PHYSICAL; 307 if ("both".equals(codeString)) 308 return AddressType.BOTH; 309 throw new IllegalArgumentException("Unknown AddressType code '" + codeString + "'"); 310 } 311 312 public Enumeration<AddressType> fromType(Base code) throws FHIRException { 313 if (code == null || code.isEmpty()) 314 return null; 315 String codeString = ((PrimitiveType) code).asStringValue(); 316 if (codeString == null || "".equals(codeString)) 317 return null; 318 if ("postal".equals(codeString)) 319 return new Enumeration<AddressType>(this, AddressType.POSTAL); 320 if ("physical".equals(codeString)) 321 return new Enumeration<AddressType>(this, AddressType.PHYSICAL); 322 if ("both".equals(codeString)) 323 return new Enumeration<AddressType>(this, AddressType.BOTH); 324 throw new FHIRException("Unknown AddressType code '" + codeString + "'"); 325 } 326 327 public String toCode(AddressType code) 328 { 329 if (code == AddressType.NULL) 330 return null; 331 if (code == AddressType.POSTAL) 332 return "postal"; 333 if (code == AddressType.PHYSICAL) 334 return "physical"; 335 if (code == AddressType.BOTH) 336 return "both"; 337 return "?"; 338 } 339 } 340 341 /** 342 * The purpose of this address. 343 */ 344 @Child(name = "use", type = { CodeType.class }, order = 0, min = 0, max = 1, modifier = true, summary = true) 345 @Description(shortDefinition = "home | work | temp | old - purpose of this address", formalDefinition = "The purpose of this address.") 346 protected Enumeration<AddressUse> use; 347 348 /** 349 * Distinguishes between physical addresses (those you can visit) and mailing 350 * addresses (e.g. PO Boxes and care-of addresses). Most addresses are both. 351 */ 352 @Child(name = "type", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 353 @Description(shortDefinition = "postal | physical | both", formalDefinition = "Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both.") 354 protected Enumeration<AddressType> type; 355 356 /** 357 * A full text representation of the address. 358 */ 359 @Child(name = "text", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 360 @Description(shortDefinition = "Text representation of the address", formalDefinition = "A full text representation of the address.") 361 protected StringType text; 362 363 /** 364 * This component contains the house number, apartment number, street name, 365 * street direction, P.O. Box number, delivery hints, and similar address 366 * information. 367 */ 368 @Child(name = "line", type = { 369 StringType.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 370 @Description(shortDefinition = "Street name, number, direction & P.O. Box etc.", formalDefinition = "This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.") 371 protected List<StringType> line; 372 373 /** 374 * The name of the city, town, village or other community or delivery center. 375 */ 376 @Child(name = "city", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 377 @Description(shortDefinition = "Name of city, town etc.", formalDefinition = "The name of the city, town, village or other community or delivery center.") 378 protected StringType city; 379 380 /** 381 * The name of the administrative area (county). 382 */ 383 @Child(name = "district", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 384 @Description(shortDefinition = "District name (aka county)", formalDefinition = "The name of the administrative area (county).") 385 protected StringType district; 386 387 /** 388 * Sub-unit of a country with limited sovereignty in a federally organized 389 * country. A code may be used if codes are in common use (i.e. US 2 letter 390 * state codes). 391 */ 392 @Child(name = "state", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 393 @Description(shortDefinition = "Sub-unit of country (abbreviations ok)", formalDefinition = "Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (i.e. US 2 letter state codes).") 394 protected StringType state; 395 396 /** 397 * A postal code designating a region defined by the postal service. 398 */ 399 @Child(name = "postalCode", type = { 400 StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 401 @Description(shortDefinition = "Postal code for area", formalDefinition = "A postal code designating a region defined by the postal service.") 402 protected StringType postalCode; 403 404 /** 405 * Country - a nation as commonly understood or generally accepted. 406 */ 407 @Child(name = "country", type = { StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 408 @Description(shortDefinition = "Country (can be ISO 3166 3 letter code)", formalDefinition = "Country - a nation as commonly understood or generally accepted.") 409 protected StringType country; 410 411 /** 412 * Time period when address was/is in use. 413 */ 414 @Child(name = "period", type = { Period.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 415 @Description(shortDefinition = "Time period when address was/is in use", formalDefinition = "Time period when address was/is in use.") 416 protected Period period; 417 418 private static final long serialVersionUID = 561490318L; 419 420 /* 421 * Constructor 422 */ 423 public Address() { 424 super(); 425 } 426 427 /** 428 * @return {@link #use} (The purpose of this address.). This is the underlying 429 * object with id, value and extensions. The accessor "getUse" gives 430 * direct access to the value 431 */ 432 public Enumeration<AddressUse> getUseElement() { 433 if (this.use == null) 434 if (Configuration.errorOnAutoCreate()) 435 throw new Error("Attempt to auto-create Address.use"); 436 else if (Configuration.doAutoCreate()) 437 this.use = new Enumeration<AddressUse>(new AddressUseEnumFactory()); // bb 438 return this.use; 439 } 440 441 public boolean hasUseElement() { 442 return this.use != null && !this.use.isEmpty(); 443 } 444 445 public boolean hasUse() { 446 return this.use != null && !this.use.isEmpty(); 447 } 448 449 /** 450 * @param value {@link #use} (The purpose of this address.). This is the 451 * underlying object with id, value and extensions. The accessor 452 * "getUse" gives direct access to the value 453 */ 454 public Address setUseElement(Enumeration<AddressUse> value) { 455 this.use = value; 456 return this; 457 } 458 459 /** 460 * @return The purpose of this address. 461 */ 462 public AddressUse getUse() { 463 return this.use == null ? null : this.use.getValue(); 464 } 465 466 /** 467 * @param value The purpose of this address. 468 */ 469 public Address setUse(AddressUse value) { 470 if (value == null) 471 this.use = null; 472 else { 473 if (this.use == null) 474 this.use = new Enumeration<AddressUse>(new AddressUseEnumFactory()); 475 this.use.setValue(value); 476 } 477 return this; 478 } 479 480 /** 481 * @return {@link #type} (Distinguishes between physical addresses (those you 482 * can visit) and mailing addresses (e.g. PO Boxes and care-of 483 * addresses). Most addresses are both.). This is the underlying object 484 * with id, value and extensions. The accessor "getType" gives direct 485 * access to the value 486 */ 487 public Enumeration<AddressType> getTypeElement() { 488 if (this.type == null) 489 if (Configuration.errorOnAutoCreate()) 490 throw new Error("Attempt to auto-create Address.type"); 491 else if (Configuration.doAutoCreate()) 492 this.type = new Enumeration<AddressType>(new AddressTypeEnumFactory()); // bb 493 return this.type; 494 } 495 496 public boolean hasTypeElement() { 497 return this.type != null && !this.type.isEmpty(); 498 } 499 500 public boolean hasType() { 501 return this.type != null && !this.type.isEmpty(); 502 } 503 504 /** 505 * @param value {@link #type} (Distinguishes between physical addresses (those 506 * you can visit) and mailing addresses (e.g. PO Boxes and care-of 507 * addresses). Most addresses are both.). This is the underlying 508 * object with id, value and extensions. The accessor "getType" 509 * gives direct access to the value 510 */ 511 public Address setTypeElement(Enumeration<AddressType> value) { 512 this.type = value; 513 return this; 514 } 515 516 /** 517 * @return Distinguishes between physical addresses (those you can visit) and 518 * mailing addresses (e.g. PO Boxes and care-of addresses). Most 519 * addresses are both. 520 */ 521 public AddressType getType() { 522 return this.type == null ? null : this.type.getValue(); 523 } 524 525 /** 526 * @param value Distinguishes between physical addresses (those you can visit) 527 * and mailing addresses (e.g. PO Boxes and care-of addresses). 528 * Most addresses are both. 529 */ 530 public Address setType(AddressType value) { 531 if (value == null) 532 this.type = null; 533 else { 534 if (this.type == null) 535 this.type = new Enumeration<AddressType>(new AddressTypeEnumFactory()); 536 this.type.setValue(value); 537 } 538 return this; 539 } 540 541 /** 542 * @return {@link #text} (A full text representation of the address.). This is 543 * the underlying object with id, value and extensions. The accessor 544 * "getText" gives direct access to the value 545 */ 546 public StringType getTextElement() { 547 if (this.text == null) 548 if (Configuration.errorOnAutoCreate()) 549 throw new Error("Attempt to auto-create Address.text"); 550 else if (Configuration.doAutoCreate()) 551 this.text = new StringType(); // bb 552 return this.text; 553 } 554 555 public boolean hasTextElement() { 556 return this.text != null && !this.text.isEmpty(); 557 } 558 559 public boolean hasText() { 560 return this.text != null && !this.text.isEmpty(); 561 } 562 563 /** 564 * @param value {@link #text} (A full text representation of the address.). This 565 * is the underlying object with id, value and extensions. The 566 * accessor "getText" gives direct access to the value 567 */ 568 public Address setTextElement(StringType value) { 569 this.text = value; 570 return this; 571 } 572 573 /** 574 * @return A full text representation of the address. 575 */ 576 public String getText() { 577 return this.text == null ? null : this.text.getValue(); 578 } 579 580 /** 581 * @param value A full text representation of the address. 582 */ 583 public Address setText(String value) { 584 if (Utilities.noString(value)) 585 this.text = null; 586 else { 587 if (this.text == null) 588 this.text = new StringType(); 589 this.text.setValue(value); 590 } 591 return this; 592 } 593 594 /** 595 * @return {@link #line} (This component contains the house number, apartment 596 * number, street name, street direction, P.O. Box number, delivery 597 * hints, and similar address information.) 598 */ 599 public List<StringType> getLine() { 600 if (this.line == null) 601 this.line = new ArrayList<StringType>(); 602 return this.line; 603 } 604 605 public boolean hasLine() { 606 if (this.line == null) 607 return false; 608 for (StringType item : this.line) 609 if (!item.isEmpty()) 610 return true; 611 return false; 612 } 613 614 /** 615 * @return {@link #line} (This component contains the house number, apartment 616 * number, street name, street direction, P.O. Box number, delivery 617 * hints, and similar address information.) 618 */ 619 // syntactic sugar 620 public StringType addLineElement() {// 2 621 StringType t = new StringType(); 622 if (this.line == null) 623 this.line = new ArrayList<StringType>(); 624 this.line.add(t); 625 return t; 626 } 627 628 /** 629 * @param value {@link #line} (This component contains the house number, 630 * apartment number, street name, street direction, P.O. Box 631 * number, delivery hints, and similar address information.) 632 */ 633 public Address addLine(String value) { // 1 634 StringType t = new StringType(); 635 t.setValue(value); 636 if (this.line == null) 637 this.line = new ArrayList<StringType>(); 638 this.line.add(t); 639 return this; 640 } 641 642 /** 643 * @param value {@link #line} (This component contains the house number, 644 * apartment number, street name, street direction, P.O. Box 645 * number, delivery hints, and similar address information.) 646 */ 647 public boolean hasLine(String value) { 648 if (this.line == null) 649 return false; 650 for (StringType v : this.line) 651 if (v.equals(value)) // string 652 return true; 653 return false; 654 } 655 656 /** 657 * @return {@link #city} (The name of the city, town, village or other community 658 * or delivery center.). This is the underlying object with id, value 659 * and extensions. The accessor "getCity" gives direct access to the 660 * value 661 */ 662 public StringType getCityElement() { 663 if (this.city == null) 664 if (Configuration.errorOnAutoCreate()) 665 throw new Error("Attempt to auto-create Address.city"); 666 else if (Configuration.doAutoCreate()) 667 this.city = new StringType(); // bb 668 return this.city; 669 } 670 671 public boolean hasCityElement() { 672 return this.city != null && !this.city.isEmpty(); 673 } 674 675 public boolean hasCity() { 676 return this.city != null && !this.city.isEmpty(); 677 } 678 679 /** 680 * @param value {@link #city} (The name of the city, town, village or other 681 * community or delivery center.). This is the underlying object 682 * with id, value and extensions. The accessor "getCity" gives 683 * direct access to the value 684 */ 685 public Address setCityElement(StringType value) { 686 this.city = value; 687 return this; 688 } 689 690 /** 691 * @return The name of the city, town, village or other community or delivery 692 * center. 693 */ 694 public String getCity() { 695 return this.city == null ? null : this.city.getValue(); 696 } 697 698 /** 699 * @param value The name of the city, town, village or other community or 700 * delivery center. 701 */ 702 public Address setCity(String value) { 703 if (Utilities.noString(value)) 704 this.city = null; 705 else { 706 if (this.city == null) 707 this.city = new StringType(); 708 this.city.setValue(value); 709 } 710 return this; 711 } 712 713 /** 714 * @return {@link #district} (The name of the administrative area (county).). 715 * This is the underlying object with id, value and extensions. The 716 * accessor "getDistrict" gives direct access to the value 717 */ 718 public StringType getDistrictElement() { 719 if (this.district == null) 720 if (Configuration.errorOnAutoCreate()) 721 throw new Error("Attempt to auto-create Address.district"); 722 else if (Configuration.doAutoCreate()) 723 this.district = new StringType(); // bb 724 return this.district; 725 } 726 727 public boolean hasDistrictElement() { 728 return this.district != null && !this.district.isEmpty(); 729 } 730 731 public boolean hasDistrict() { 732 return this.district != null && !this.district.isEmpty(); 733 } 734 735 /** 736 * @param value {@link #district} (The name of the administrative area 737 * (county).). This is the underlying object with id, value and 738 * extensions. The accessor "getDistrict" gives direct access to 739 * the value 740 */ 741 public Address setDistrictElement(StringType value) { 742 this.district = value; 743 return this; 744 } 745 746 /** 747 * @return The name of the administrative area (county). 748 */ 749 public String getDistrict() { 750 return this.district == null ? null : this.district.getValue(); 751 } 752 753 /** 754 * @param value The name of the administrative area (county). 755 */ 756 public Address setDistrict(String value) { 757 if (Utilities.noString(value)) 758 this.district = null; 759 else { 760 if (this.district == null) 761 this.district = new StringType(); 762 this.district.setValue(value); 763 } 764 return this; 765 } 766 767 /** 768 * @return {@link #state} (Sub-unit of a country with limited sovereignty in a 769 * federally organized country. A code may be used if codes are in 770 * common use (i.e. US 2 letter state codes).). This is the underlying 771 * object with id, value and extensions. The accessor "getState" gives 772 * direct access to the value 773 */ 774 public StringType getStateElement() { 775 if (this.state == null) 776 if (Configuration.errorOnAutoCreate()) 777 throw new Error("Attempt to auto-create Address.state"); 778 else if (Configuration.doAutoCreate()) 779 this.state = new StringType(); // bb 780 return this.state; 781 } 782 783 public boolean hasStateElement() { 784 return this.state != null && !this.state.isEmpty(); 785 } 786 787 public boolean hasState() { 788 return this.state != null && !this.state.isEmpty(); 789 } 790 791 /** 792 * @param value {@link #state} (Sub-unit of a country with limited sovereignty 793 * in a federally organized country. A code may be used if codes 794 * are in common use (i.e. US 2 letter state codes).). This is the 795 * underlying object with id, value and extensions. The accessor 796 * "getState" gives direct access to the value 797 */ 798 public Address setStateElement(StringType value) { 799 this.state = value; 800 return this; 801 } 802 803 /** 804 * @return Sub-unit of a country with limited sovereignty in a federally 805 * organized country. A code may be used if codes are in common use 806 * (i.e. US 2 letter state codes). 807 */ 808 public String getState() { 809 return this.state == null ? null : this.state.getValue(); 810 } 811 812 /** 813 * @param value Sub-unit of a country with limited sovereignty in a federally 814 * organized country. A code may be used if codes are in common use 815 * (i.e. US 2 letter state codes). 816 */ 817 public Address setState(String value) { 818 if (Utilities.noString(value)) 819 this.state = null; 820 else { 821 if (this.state == null) 822 this.state = new StringType(); 823 this.state.setValue(value); 824 } 825 return this; 826 } 827 828 /** 829 * @return {@link #postalCode} (A postal code designating a region defined by 830 * the postal service.). This is the underlying object with id, value 831 * and extensions. The accessor "getPostalCode" gives direct access to 832 * the value 833 */ 834 public StringType getPostalCodeElement() { 835 if (this.postalCode == null) 836 if (Configuration.errorOnAutoCreate()) 837 throw new Error("Attempt to auto-create Address.postalCode"); 838 else if (Configuration.doAutoCreate()) 839 this.postalCode = new StringType(); // bb 840 return this.postalCode; 841 } 842 843 public boolean hasPostalCodeElement() { 844 return this.postalCode != null && !this.postalCode.isEmpty(); 845 } 846 847 public boolean hasPostalCode() { 848 return this.postalCode != null && !this.postalCode.isEmpty(); 849 } 850 851 /** 852 * @param value {@link #postalCode} (A postal code designating a region defined 853 * by the postal service.). This is the underlying object with id, 854 * value and extensions. The accessor "getPostalCode" gives direct 855 * access to the value 856 */ 857 public Address setPostalCodeElement(StringType value) { 858 this.postalCode = value; 859 return this; 860 } 861 862 /** 863 * @return A postal code designating a region defined by the postal service. 864 */ 865 public String getPostalCode() { 866 return this.postalCode == null ? null : this.postalCode.getValue(); 867 } 868 869 /** 870 * @param value A postal code designating a region defined by the postal 871 * service. 872 */ 873 public Address setPostalCode(String value) { 874 if (Utilities.noString(value)) 875 this.postalCode = null; 876 else { 877 if (this.postalCode == null) 878 this.postalCode = new StringType(); 879 this.postalCode.setValue(value); 880 } 881 return this; 882 } 883 884 /** 885 * @return {@link #country} (Country - a nation as commonly understood or 886 * generally accepted.). This is the underlying object with id, value 887 * and extensions. The accessor "getCountry" gives direct access to the 888 * value 889 */ 890 public StringType getCountryElement() { 891 if (this.country == null) 892 if (Configuration.errorOnAutoCreate()) 893 throw new Error("Attempt to auto-create Address.country"); 894 else if (Configuration.doAutoCreate()) 895 this.country = new StringType(); // bb 896 return this.country; 897 } 898 899 public boolean hasCountryElement() { 900 return this.country != null && !this.country.isEmpty(); 901 } 902 903 public boolean hasCountry() { 904 return this.country != null && !this.country.isEmpty(); 905 } 906 907 /** 908 * @param value {@link #country} (Country - a nation as commonly understood or 909 * generally accepted.). This is the underlying object with id, 910 * value and extensions. The accessor "getCountry" gives direct 911 * access to the value 912 */ 913 public Address setCountryElement(StringType value) { 914 this.country = value; 915 return this; 916 } 917 918 /** 919 * @return Country - a nation as commonly understood or generally accepted. 920 */ 921 public String getCountry() { 922 return this.country == null ? null : this.country.getValue(); 923 } 924 925 /** 926 * @param value Country - a nation as commonly understood or generally accepted. 927 */ 928 public Address setCountry(String value) { 929 if (Utilities.noString(value)) 930 this.country = null; 931 else { 932 if (this.country == null) 933 this.country = new StringType(); 934 this.country.setValue(value); 935 } 936 return this; 937 } 938 939 /** 940 * @return {@link #period} (Time period when address was/is in use.) 941 */ 942 public Period getPeriod() { 943 if (this.period == null) 944 if (Configuration.errorOnAutoCreate()) 945 throw new Error("Attempt to auto-create Address.period"); 946 else if (Configuration.doAutoCreate()) 947 this.period = new Period(); // cc 948 return this.period; 949 } 950 951 public boolean hasPeriod() { 952 return this.period != null && !this.period.isEmpty(); 953 } 954 955 /** 956 * @param value {@link #period} (Time period when address was/is in use.) 957 */ 958 public Address setPeriod(Period value) { 959 this.period = value; 960 return this; 961 } 962 963 protected void listChildren(List<Property> childrenList) { 964 super.listChildren(childrenList); 965 childrenList.add(new Property("use", "code", "The purpose of this address.", 0, java.lang.Integer.MAX_VALUE, use)); 966 childrenList.add(new Property("type", "code", 967 "Distinguishes between physical addresses (those you can visit) and mailing addresses (e.g. PO Boxes and care-of addresses). Most addresses are both.", 968 0, java.lang.Integer.MAX_VALUE, type)); 969 childrenList.add(new Property("text", "string", "A full text representation of the address.", 0, 970 java.lang.Integer.MAX_VALUE, text)); 971 childrenList.add(new Property("line", "string", 972 "This component contains the house number, apartment number, street name, street direction, P.O. Box number, delivery hints, and similar address information.", 973 0, java.lang.Integer.MAX_VALUE, line)); 974 childrenList.add( 975 new Property("city", "string", "The name of the city, town, village or other community or delivery center.", 0, 976 java.lang.Integer.MAX_VALUE, city)); 977 childrenList.add(new Property("district", "string", "The name of the administrative area (county).", 0, 978 java.lang.Integer.MAX_VALUE, district)); 979 childrenList.add(new Property("state", "string", 980 "Sub-unit of a country with limited sovereignty in a federally organized country. A code may be used if codes are in common use (i.e. US 2 letter state codes).", 981 0, java.lang.Integer.MAX_VALUE, state)); 982 childrenList 983 .add(new Property("postalCode", "string", "A postal code designating a region defined by the postal service.", 984 0, java.lang.Integer.MAX_VALUE, postalCode)); 985 childrenList.add(new Property("country", "string", 986 "Country - a nation as commonly understood or generally accepted.", 0, java.lang.Integer.MAX_VALUE, country)); 987 childrenList.add(new Property("period", "Period", "Time period when address was/is in use.", 0, 988 java.lang.Integer.MAX_VALUE, period)); 989 } 990 991 @Override 992 public void setProperty(String name, Base value) throws FHIRException { 993 if (name.equals("use")) 994 this.use = new AddressUseEnumFactory().fromType(value); // Enumeration<AddressUse> 995 else if (name.equals("type")) 996 this.type = new AddressTypeEnumFactory().fromType(value); // Enumeration<AddressType> 997 else if (name.equals("text")) 998 this.text = castToString(value); // StringType 999 else if (name.equals("line")) 1000 this.getLine().add(castToString(value)); 1001 else if (name.equals("city")) 1002 this.city = castToString(value); // StringType 1003 else if (name.equals("district")) 1004 this.district = castToString(value); // StringType 1005 else if (name.equals("state")) 1006 this.state = castToString(value); // StringType 1007 else if (name.equals("postalCode")) 1008 this.postalCode = castToString(value); // StringType 1009 else if (name.equals("country")) 1010 this.country = castToString(value); // StringType 1011 else if (name.equals("period")) 1012 this.period = castToPeriod(value); // Period 1013 else 1014 super.setProperty(name, value); 1015 } 1016 1017 @Override 1018 public Base addChild(String name) throws FHIRException { 1019 if (name.equals("use")) { 1020 throw new FHIRException("Cannot call addChild on a singleton property Address.use"); 1021 } else if (name.equals("type")) { 1022 throw new FHIRException("Cannot call addChild on a singleton property Address.type"); 1023 } else if (name.equals("text")) { 1024 throw new FHIRException("Cannot call addChild on a singleton property Address.text"); 1025 } else if (name.equals("line")) { 1026 throw new FHIRException("Cannot call addChild on a singleton property Address.line"); 1027 } else if (name.equals("city")) { 1028 throw new FHIRException("Cannot call addChild on a singleton property Address.city"); 1029 } else if (name.equals("district")) { 1030 throw new FHIRException("Cannot call addChild on a singleton property Address.district"); 1031 } else if (name.equals("state")) { 1032 throw new FHIRException("Cannot call addChild on a singleton property Address.state"); 1033 } else if (name.equals("postalCode")) { 1034 throw new FHIRException("Cannot call addChild on a singleton property Address.postalCode"); 1035 } else if (name.equals("country")) { 1036 throw new FHIRException("Cannot call addChild on a singleton property Address.country"); 1037 } else if (name.equals("period")) { 1038 this.period = new Period(); 1039 return this.period; 1040 } else 1041 return super.addChild(name); 1042 } 1043 1044 public String fhirType() { 1045 return "Address"; 1046 1047 } 1048 1049 public Address copy() { 1050 Address dst = new Address(); 1051 copyValues(dst); 1052 dst.use = use == null ? null : use.copy(); 1053 dst.type = type == null ? null : type.copy(); 1054 dst.text = text == null ? null : text.copy(); 1055 if (line != null) { 1056 dst.line = new ArrayList<StringType>(); 1057 for (StringType i : line) 1058 dst.line.add(i.copy()); 1059 } 1060 ; 1061 dst.city = city == null ? null : city.copy(); 1062 dst.district = district == null ? null : district.copy(); 1063 dst.state = state == null ? null : state.copy(); 1064 dst.postalCode = postalCode == null ? null : postalCode.copy(); 1065 dst.country = country == null ? null : country.copy(); 1066 dst.period = period == null ? null : period.copy(); 1067 return dst; 1068 } 1069 1070 protected Address typedCopy() { 1071 return copy(); 1072 } 1073 1074 @Override 1075 public boolean equalsDeep(Base other) { 1076 if (!super.equalsDeep(other)) 1077 return false; 1078 if (!(other instanceof Address)) 1079 return false; 1080 Address o = (Address) other; 1081 return compareDeep(use, o.use, true) && compareDeep(type, o.type, true) && compareDeep(text, o.text, true) 1082 && compareDeep(line, o.line, true) && compareDeep(city, o.city, true) && compareDeep(district, o.district, true) 1083 && compareDeep(state, o.state, true) && compareDeep(postalCode, o.postalCode, true) 1084 && compareDeep(country, o.country, true) && compareDeep(period, o.period, true); 1085 } 1086 1087 @Override 1088 public boolean equalsShallow(Base other) { 1089 if (!super.equalsShallow(other)) 1090 return false; 1091 if (!(other instanceof Address)) 1092 return false; 1093 Address o = (Address) other; 1094 return compareValues(use, o.use, true) && compareValues(type, o.type, true) && compareValues(text, o.text, true) 1095 && compareValues(line, o.line, true) && compareValues(city, o.city, true) 1096 && compareValues(district, o.district, true) && compareValues(state, o.state, true) 1097 && compareValues(postalCode, o.postalCode, true) && compareValues(country, o.country, true); 1098 } 1099 1100 public boolean isEmpty() { 1101 return super.isEmpty() && (use == null || use.isEmpty()) && (type == null || type.isEmpty()) 1102 && (text == null || text.isEmpty()) && (line == null || line.isEmpty()) && (city == null || city.isEmpty()) 1103 && (district == null || district.isEmpty()) && (state == null || state.isEmpty()) 1104 && (postalCode == null || postalCode.isEmpty()) && (country == null || country.isEmpty()) 1105 && (period == null || period.isEmpty()); 1106 } 1107 1108}