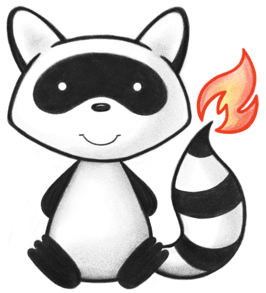
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * Risk of harmful or undesirable, physiological response which is unique to an 048 * individual and associated with exposure to a substance. 049 */ 050@ResourceDef(name = "AllergyIntolerance", profile = "http://hl7.org/fhir/Profile/AllergyIntolerance") 051public class AllergyIntolerance extends DomainResource { 052 053 public enum AllergyIntoleranceStatus { 054 /** 055 * An active record of a reaction to the identified Substance. 056 */ 057 ACTIVE, 058 /** 059 * A low level of certainty about the propensity for a reaction to the 060 * identified Substance. 061 */ 062 UNCONFIRMED, 063 /** 064 * A high level of certainty about the propensity for a reaction to the 065 * identified Substance, which may include clinical evidence by testing or 066 * rechallenge. 067 */ 068 CONFIRMED, 069 /** 070 * An inactive record of a reaction to the identified Substance. 071 */ 072 INACTIVE, 073 /** 074 * A reaction to the identified Substance has been clinically reassessed by 075 * testing or rechallenge and considered to be resolved. 076 */ 077 RESOLVED, 078 /** 079 * A propensity for a reaction to the identified Substance has been disproven 080 * with a high level of clinical certainty, which may include testing or 081 * rechallenge, and is refuted. 082 */ 083 REFUTED, 084 /** 085 * The statement was entered in error and is not valid. 086 */ 087 ENTEREDINERROR, 088 /** 089 * added to help the parsers 090 */ 091 NULL; 092 093 public static AllergyIntoleranceStatus fromCode(String codeString) throws FHIRException { 094 if (codeString == null || "".equals(codeString)) 095 return null; 096 if ("active".equals(codeString)) 097 return ACTIVE; 098 if ("unconfirmed".equals(codeString)) 099 return UNCONFIRMED; 100 if ("confirmed".equals(codeString)) 101 return CONFIRMED; 102 if ("inactive".equals(codeString)) 103 return INACTIVE; 104 if ("resolved".equals(codeString)) 105 return RESOLVED; 106 if ("refuted".equals(codeString)) 107 return REFUTED; 108 if ("entered-in-error".equals(codeString)) 109 return ENTEREDINERROR; 110 throw new FHIRException("Unknown AllergyIntoleranceStatus code '" + codeString + "'"); 111 } 112 113 public String toCode() { 114 switch (this) { 115 case ACTIVE: 116 return "active"; 117 case UNCONFIRMED: 118 return "unconfirmed"; 119 case CONFIRMED: 120 return "confirmed"; 121 case INACTIVE: 122 return "inactive"; 123 case RESOLVED: 124 return "resolved"; 125 case REFUTED: 126 return "refuted"; 127 case ENTEREDINERROR: 128 return "entered-in-error"; 129 case NULL: 130 return null; 131 default: 132 return "?"; 133 } 134 } 135 136 public String getSystem() { 137 switch (this) { 138 case ACTIVE: 139 return "http://hl7.org/fhir/allergy-intolerance-status"; 140 case UNCONFIRMED: 141 return "http://hl7.org/fhir/allergy-intolerance-status"; 142 case CONFIRMED: 143 return "http://hl7.org/fhir/allergy-intolerance-status"; 144 case INACTIVE: 145 return "http://hl7.org/fhir/allergy-intolerance-status"; 146 case RESOLVED: 147 return "http://hl7.org/fhir/allergy-intolerance-status"; 148 case REFUTED: 149 return "http://hl7.org/fhir/allergy-intolerance-status"; 150 case ENTEREDINERROR: 151 return "http://hl7.org/fhir/allergy-intolerance-status"; 152 case NULL: 153 return null; 154 default: 155 return "?"; 156 } 157 } 158 159 public String getDefinition() { 160 switch (this) { 161 case ACTIVE: 162 return "An active record of a reaction to the identified Substance."; 163 case UNCONFIRMED: 164 return "A low level of certainty about the propensity for a reaction to the identified Substance."; 165 case CONFIRMED: 166 return "A high level of certainty about the propensity for a reaction to the identified Substance, which may include clinical evidence by testing or rechallenge."; 167 case INACTIVE: 168 return "An inactive record of a reaction to the identified Substance."; 169 case RESOLVED: 170 return "A reaction to the identified Substance has been clinically reassessed by testing or rechallenge and considered to be resolved."; 171 case REFUTED: 172 return "A propensity for a reaction to the identified Substance has been disproven with a high level of clinical certainty, which may include testing or rechallenge, and is refuted."; 173 case ENTEREDINERROR: 174 return "The statement was entered in error and is not valid."; 175 case NULL: 176 return null; 177 default: 178 return "?"; 179 } 180 } 181 182 public String getDisplay() { 183 switch (this) { 184 case ACTIVE: 185 return "Active"; 186 case UNCONFIRMED: 187 return "Unconfirmed"; 188 case CONFIRMED: 189 return "Confirmed"; 190 case INACTIVE: 191 return "Inactive"; 192 case RESOLVED: 193 return "Resolved"; 194 case REFUTED: 195 return "Refuted"; 196 case ENTEREDINERROR: 197 return "Entered In Error"; 198 case NULL: 199 return null; 200 default: 201 return "?"; 202 } 203 } 204 } 205 206 public static class AllergyIntoleranceStatusEnumFactory implements EnumFactory<AllergyIntoleranceStatus> { 207 public AllergyIntoleranceStatus fromCode(String codeString) throws IllegalArgumentException { 208 if (codeString == null || "".equals(codeString)) 209 if (codeString == null || "".equals(codeString)) 210 return null; 211 if ("active".equals(codeString)) 212 return AllergyIntoleranceStatus.ACTIVE; 213 if ("unconfirmed".equals(codeString)) 214 return AllergyIntoleranceStatus.UNCONFIRMED; 215 if ("confirmed".equals(codeString)) 216 return AllergyIntoleranceStatus.CONFIRMED; 217 if ("inactive".equals(codeString)) 218 return AllergyIntoleranceStatus.INACTIVE; 219 if ("resolved".equals(codeString)) 220 return AllergyIntoleranceStatus.RESOLVED; 221 if ("refuted".equals(codeString)) 222 return AllergyIntoleranceStatus.REFUTED; 223 if ("entered-in-error".equals(codeString)) 224 return AllergyIntoleranceStatus.ENTEREDINERROR; 225 throw new IllegalArgumentException("Unknown AllergyIntoleranceStatus code '" + codeString + "'"); 226 } 227 228 public Enumeration<AllergyIntoleranceStatus> fromType(Base code) throws FHIRException { 229 if (code == null || code.isEmpty()) 230 return null; 231 String codeString = ((PrimitiveType) code).asStringValue(); 232 if (codeString == null || "".equals(codeString)) 233 return null; 234 if ("active".equals(codeString)) 235 return new Enumeration<AllergyIntoleranceStatus>(this, AllergyIntoleranceStatus.ACTIVE); 236 if ("unconfirmed".equals(codeString)) 237 return new Enumeration<AllergyIntoleranceStatus>(this, AllergyIntoleranceStatus.UNCONFIRMED); 238 if ("confirmed".equals(codeString)) 239 return new Enumeration<AllergyIntoleranceStatus>(this, AllergyIntoleranceStatus.CONFIRMED); 240 if ("inactive".equals(codeString)) 241 return new Enumeration<AllergyIntoleranceStatus>(this, AllergyIntoleranceStatus.INACTIVE); 242 if ("resolved".equals(codeString)) 243 return new Enumeration<AllergyIntoleranceStatus>(this, AllergyIntoleranceStatus.RESOLVED); 244 if ("refuted".equals(codeString)) 245 return new Enumeration<AllergyIntoleranceStatus>(this, AllergyIntoleranceStatus.REFUTED); 246 if ("entered-in-error".equals(codeString)) 247 return new Enumeration<AllergyIntoleranceStatus>(this, AllergyIntoleranceStatus.ENTEREDINERROR); 248 throw new FHIRException("Unknown AllergyIntoleranceStatus code '" + codeString + "'"); 249 } 250 251 public String toCode(AllergyIntoleranceStatus code) 252 { 253 if (code == AllergyIntoleranceStatus.NULL) 254 return null; 255 if (code == AllergyIntoleranceStatus.ACTIVE) 256 return "active"; 257 if (code == AllergyIntoleranceStatus.UNCONFIRMED) 258 return "unconfirmed"; 259 if (code == AllergyIntoleranceStatus.CONFIRMED) 260 return "confirmed"; 261 if (code == AllergyIntoleranceStatus.INACTIVE) 262 return "inactive"; 263 if (code == AllergyIntoleranceStatus.RESOLVED) 264 return "resolved"; 265 if (code == AllergyIntoleranceStatus.REFUTED) 266 return "refuted"; 267 if (code == AllergyIntoleranceStatus.ENTEREDINERROR) 268 return "entered-in-error"; 269 return "?"; 270 } 271 } 272 273 public enum AllergyIntoleranceCriticality { 274 /** 275 * The potential clinical impact of a future reaction is estimated as low risk: 276 * exposure to substance is unlikely to result in a life threatening or organ 277 * system threatening outcome. Future exposure to the Substance is considered a 278 * relative contra-indication. 279 */ 280 CRITL, 281 /** 282 * The potential clinical impact of a future reaction is estimated as high risk: 283 * exposure to substance may result in a life threatening or organ system 284 * threatening outcome. Future exposure to the Substance may be considered an 285 * absolute contra-indication. 286 */ 287 CRITH, 288 /** 289 * Unable to assess the potential clinical impact with the information 290 * available. 291 */ 292 CRITU, 293 /** 294 * added to help the parsers 295 */ 296 NULL; 297 298 public static AllergyIntoleranceCriticality fromCode(String codeString) throws FHIRException { 299 if (codeString == null || "".equals(codeString)) 300 return null; 301 if ("CRITL".equals(codeString)) 302 return CRITL; 303 if ("CRITH".equals(codeString)) 304 return CRITH; 305 if ("CRITU".equals(codeString)) 306 return CRITU; 307 throw new FHIRException("Unknown AllergyIntoleranceCriticality code '" + codeString + "'"); 308 } 309 310 public String toCode() { 311 switch (this) { 312 case CRITL: 313 return "CRITL"; 314 case CRITH: 315 return "CRITH"; 316 case CRITU: 317 return "CRITU"; 318 case NULL: 319 return null; 320 default: 321 return "?"; 322 } 323 } 324 325 public String getSystem() { 326 switch (this) { 327 case CRITL: 328 return "http://hl7.org/fhir/allergy-intolerance-criticality"; 329 case CRITH: 330 return "http://hl7.org/fhir/allergy-intolerance-criticality"; 331 case CRITU: 332 return "http://hl7.org/fhir/allergy-intolerance-criticality"; 333 case NULL: 334 return null; 335 default: 336 return "?"; 337 } 338 } 339 340 public String getDefinition() { 341 switch (this) { 342 case CRITL: 343 return "The potential clinical impact of a future reaction is estimated as low risk: exposure to substance is unlikely to result in a life threatening or organ system threatening outcome. Future exposure to the Substance is considered a relative contra-indication."; 344 case CRITH: 345 return "The potential clinical impact of a future reaction is estimated as high risk: exposure to substance may result in a life threatening or organ system threatening outcome. Future exposure to the Substance may be considered an absolute contra-indication."; 346 case CRITU: 347 return "Unable to assess the potential clinical impact with the information available."; 348 case NULL: 349 return null; 350 default: 351 return "?"; 352 } 353 } 354 355 public String getDisplay() { 356 switch (this) { 357 case CRITL: 358 return "Low Risk"; 359 case CRITH: 360 return "High Risk"; 361 case CRITU: 362 return "Unable to determine"; 363 case NULL: 364 return null; 365 default: 366 return "?"; 367 } 368 } 369 } 370 371 public static class AllergyIntoleranceCriticalityEnumFactory implements EnumFactory<AllergyIntoleranceCriticality> { 372 public AllergyIntoleranceCriticality fromCode(String codeString) throws IllegalArgumentException { 373 if (codeString == null || "".equals(codeString)) 374 if (codeString == null || "".equals(codeString)) 375 return null; 376 if ("CRITL".equals(codeString)) 377 return AllergyIntoleranceCriticality.CRITL; 378 if ("CRITH".equals(codeString)) 379 return AllergyIntoleranceCriticality.CRITH; 380 if ("CRITU".equals(codeString)) 381 return AllergyIntoleranceCriticality.CRITU; 382 throw new IllegalArgumentException("Unknown AllergyIntoleranceCriticality code '" + codeString + "'"); 383 } 384 385 public Enumeration<AllergyIntoleranceCriticality> fromType(Base code) throws FHIRException { 386 if (code == null || code.isEmpty()) 387 return null; 388 String codeString = ((PrimitiveType) code).asStringValue(); 389 if (codeString == null || "".equals(codeString)) 390 return null; 391 if ("CRITL".equals(codeString)) 392 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.CRITL); 393 if ("CRITH".equals(codeString)) 394 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.CRITH); 395 if ("CRITU".equals(codeString)) 396 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.CRITU); 397 throw new FHIRException("Unknown AllergyIntoleranceCriticality code '" + codeString + "'"); 398 } 399 400 public String toCode(AllergyIntoleranceCriticality code) 401 { 402 if (code == AllergyIntoleranceCriticality.NULL) 403 return null; 404 if (code == AllergyIntoleranceCriticality.CRITL) 405 return "CRITL"; 406 if (code == AllergyIntoleranceCriticality.CRITH) 407 return "CRITH"; 408 if (code == AllergyIntoleranceCriticality.CRITU) 409 return "CRITU"; 410 return "?"; 411 } 412 } 413 414 public enum AllergyIntoleranceType { 415 /** 416 * A propensity for hypersensitivity reaction(s) to a substance. These reactions 417 * are most typically type I hypersensitivity, plus other "allergy-like" 418 * reactions, including pseudoallergy. 419 */ 420 ALLERGY, 421 /** 422 * A propensity for adverse reactions to a substance that is not judged to be 423 * allergic or "allergy-like". These reactions are typically (but not 424 * necessarily) non-immune. They are to some degree idiosyncratic and/or 425 * individually specific (i.e. are not a reaction that is expected to occur with 426 * most or all patients given similar circumstances). 427 */ 428 INTOLERANCE, 429 /** 430 * added to help the parsers 431 */ 432 NULL; 433 434 public static AllergyIntoleranceType fromCode(String codeString) throws FHIRException { 435 if (codeString == null || "".equals(codeString)) 436 return null; 437 if ("allergy".equals(codeString)) 438 return ALLERGY; 439 if ("intolerance".equals(codeString)) 440 return INTOLERANCE; 441 throw new FHIRException("Unknown AllergyIntoleranceType code '" + codeString + "'"); 442 } 443 444 public String toCode() { 445 switch (this) { 446 case ALLERGY: 447 return "allergy"; 448 case INTOLERANCE: 449 return "intolerance"; 450 case NULL: 451 return null; 452 default: 453 return "?"; 454 } 455 } 456 457 public String getSystem() { 458 switch (this) { 459 case ALLERGY: 460 return "http://hl7.org/fhir/allergy-intolerance-type"; 461 case INTOLERANCE: 462 return "http://hl7.org/fhir/allergy-intolerance-type"; 463 case NULL: 464 return null; 465 default: 466 return "?"; 467 } 468 } 469 470 public String getDefinition() { 471 switch (this) { 472 case ALLERGY: 473 return "A propensity for hypersensitivity reaction(s) to a substance. These reactions are most typically type I hypersensitivity, plus other \"allergy-like\" reactions, including pseudoallergy."; 474 case INTOLERANCE: 475 return "A propensity for adverse reactions to a substance that is not judged to be allergic or \"allergy-like\". These reactions are typically (but not necessarily) non-immune. They are to some degree idiosyncratic and/or individually specific (i.e. are not a reaction that is expected to occur with most or all patients given similar circumstances)."; 476 case NULL: 477 return null; 478 default: 479 return "?"; 480 } 481 } 482 483 public String getDisplay() { 484 switch (this) { 485 case ALLERGY: 486 return "Allergy"; 487 case INTOLERANCE: 488 return "Intolerance"; 489 case NULL: 490 return null; 491 default: 492 return "?"; 493 } 494 } 495 } 496 497 public static class AllergyIntoleranceTypeEnumFactory implements EnumFactory<AllergyIntoleranceType> { 498 public AllergyIntoleranceType fromCode(String codeString) throws IllegalArgumentException { 499 if (codeString == null || "".equals(codeString)) 500 if (codeString == null || "".equals(codeString)) 501 return null; 502 if ("allergy".equals(codeString)) 503 return AllergyIntoleranceType.ALLERGY; 504 if ("intolerance".equals(codeString)) 505 return AllergyIntoleranceType.INTOLERANCE; 506 throw new IllegalArgumentException("Unknown AllergyIntoleranceType code '" + codeString + "'"); 507 } 508 509 public Enumeration<AllergyIntoleranceType> fromType(Base code) throws FHIRException { 510 if (code == null || code.isEmpty()) 511 return null; 512 String codeString = ((PrimitiveType) code).asStringValue(); 513 if (codeString == null || "".equals(codeString)) 514 return null; 515 if ("allergy".equals(codeString)) 516 return new Enumeration<AllergyIntoleranceType>(this, AllergyIntoleranceType.ALLERGY); 517 if ("intolerance".equals(codeString)) 518 return new Enumeration<AllergyIntoleranceType>(this, AllergyIntoleranceType.INTOLERANCE); 519 throw new FHIRException("Unknown AllergyIntoleranceType code '" + codeString + "'"); 520 } 521 522 public String toCode(AllergyIntoleranceType code) 523 { 524 if (code == AllergyIntoleranceType.NULL) 525 return null; 526 if (code == AllergyIntoleranceType.ALLERGY) 527 return "allergy"; 528 if (code == AllergyIntoleranceType.INTOLERANCE) 529 return "intolerance"; 530 return "?"; 531 } 532 } 533 534 public enum AllergyIntoleranceCategory { 535 /** 536 * Any substance consumed to provide nutritional support for the body. 537 */ 538 FOOD, 539 /** 540 * Substances administered to achieve a physiological effect. 541 */ 542 MEDICATION, 543 /** 544 * Substances that are encountered in the environment. 545 */ 546 ENVIRONMENT, 547 /** 548 * Other substances that are not covered by any other category. 549 */ 550 OTHER, 551 /** 552 * added to help the parsers 553 */ 554 NULL; 555 556 public static AllergyIntoleranceCategory fromCode(String codeString) throws FHIRException { 557 if (codeString == null || "".equals(codeString)) 558 return null; 559 if ("food".equals(codeString)) 560 return FOOD; 561 if ("medication".equals(codeString)) 562 return MEDICATION; 563 if ("environment".equals(codeString)) 564 return ENVIRONMENT; 565 if ("other".equals(codeString)) 566 return OTHER; 567 throw new FHIRException("Unknown AllergyIntoleranceCategory code '" + codeString + "'"); 568 } 569 570 public String toCode() { 571 switch (this) { 572 case FOOD: 573 return "food"; 574 case MEDICATION: 575 return "medication"; 576 case ENVIRONMENT: 577 return "environment"; 578 case OTHER: 579 return "other"; 580 case NULL: 581 return null; 582 default: 583 return "?"; 584 } 585 } 586 587 public String getSystem() { 588 switch (this) { 589 case FOOD: 590 return "http://hl7.org/fhir/allergy-intolerance-category"; 591 case MEDICATION: 592 return "http://hl7.org/fhir/allergy-intolerance-category"; 593 case ENVIRONMENT: 594 return "http://hl7.org/fhir/allergy-intolerance-category"; 595 case OTHER: 596 return "http://hl7.org/fhir/allergy-intolerance-category"; 597 case NULL: 598 return null; 599 default: 600 return "?"; 601 } 602 } 603 604 public String getDefinition() { 605 switch (this) { 606 case FOOD: 607 return "Any substance consumed to provide nutritional support for the body."; 608 case MEDICATION: 609 return "Substances administered to achieve a physiological effect."; 610 case ENVIRONMENT: 611 return "Substances that are encountered in the environment."; 612 case OTHER: 613 return "Other substances that are not covered by any other category."; 614 case NULL: 615 return null; 616 default: 617 return "?"; 618 } 619 } 620 621 public String getDisplay() { 622 switch (this) { 623 case FOOD: 624 return "Food"; 625 case MEDICATION: 626 return "Medication"; 627 case ENVIRONMENT: 628 return "Environment"; 629 case OTHER: 630 return "Other"; 631 case NULL: 632 return null; 633 default: 634 return "?"; 635 } 636 } 637 } 638 639 public static class AllergyIntoleranceCategoryEnumFactory implements EnumFactory<AllergyIntoleranceCategory> { 640 public AllergyIntoleranceCategory fromCode(String codeString) throws IllegalArgumentException { 641 if (codeString == null || "".equals(codeString)) 642 if (codeString == null || "".equals(codeString)) 643 return null; 644 if ("food".equals(codeString)) 645 return AllergyIntoleranceCategory.FOOD; 646 if ("medication".equals(codeString)) 647 return AllergyIntoleranceCategory.MEDICATION; 648 if ("environment".equals(codeString)) 649 return AllergyIntoleranceCategory.ENVIRONMENT; 650 if ("other".equals(codeString)) 651 return AllergyIntoleranceCategory.OTHER; 652 throw new IllegalArgumentException("Unknown AllergyIntoleranceCategory code '" + codeString + "'"); 653 } 654 655 public Enumeration<AllergyIntoleranceCategory> fromType(Base code) throws FHIRException { 656 if (code == null || code.isEmpty()) 657 return null; 658 String codeString = ((PrimitiveType) code).asStringValue(); 659 if (codeString == null || "".equals(codeString)) 660 return null; 661 if ("food".equals(codeString)) 662 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.FOOD); 663 if ("medication".equals(codeString)) 664 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.MEDICATION); 665 if ("environment".equals(codeString)) 666 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.ENVIRONMENT); 667 if ("other".equals(codeString)) 668 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.OTHER); 669 throw new FHIRException("Unknown AllergyIntoleranceCategory code '" + codeString + "'"); 670 } 671 672 public String toCode(AllergyIntoleranceCategory code) 673 { 674 if (code == AllergyIntoleranceCategory.NULL) 675 return null; 676 if (code == AllergyIntoleranceCategory.FOOD) 677 return "food"; 678 if (code == AllergyIntoleranceCategory.MEDICATION) 679 return "medication"; 680 if (code == AllergyIntoleranceCategory.ENVIRONMENT) 681 return "environment"; 682 if (code == AllergyIntoleranceCategory.OTHER) 683 return "other"; 684 return "?"; 685 } 686 } 687 688 public enum AllergyIntoleranceCertainty { 689 /** 690 * There is a low level of clinical certainty that the reaction was caused by 691 * the identified Substance. 692 */ 693 UNLIKELY, 694 /** 695 * There is a high level of clinical certainty that the reaction was caused by 696 * the identified Substance. 697 */ 698 LIKELY, 699 /** 700 * There is a very high level of clinical certainty that the reaction was due to 701 * the identified Substance, which may include clinical evidence by testing or 702 * rechallenge. 703 */ 704 CONFIRMED, 705 /** 706 * added to help the parsers 707 */ 708 NULL; 709 710 public static AllergyIntoleranceCertainty fromCode(String codeString) throws FHIRException { 711 if (codeString == null || "".equals(codeString)) 712 return null; 713 if ("unlikely".equals(codeString)) 714 return UNLIKELY; 715 if ("likely".equals(codeString)) 716 return LIKELY; 717 if ("confirmed".equals(codeString)) 718 return CONFIRMED; 719 throw new FHIRException("Unknown AllergyIntoleranceCertainty code '" + codeString + "'"); 720 } 721 722 public String toCode() { 723 switch (this) { 724 case UNLIKELY: 725 return "unlikely"; 726 case LIKELY: 727 return "likely"; 728 case CONFIRMED: 729 return "confirmed"; 730 case NULL: 731 return null; 732 default: 733 return "?"; 734 } 735 } 736 737 public String getSystem() { 738 switch (this) { 739 case UNLIKELY: 740 return "http://hl7.org/fhir/reaction-event-certainty"; 741 case LIKELY: 742 return "http://hl7.org/fhir/reaction-event-certainty"; 743 case CONFIRMED: 744 return "http://hl7.org/fhir/reaction-event-certainty"; 745 case NULL: 746 return null; 747 default: 748 return "?"; 749 } 750 } 751 752 public String getDefinition() { 753 switch (this) { 754 case UNLIKELY: 755 return "There is a low level of clinical certainty that the reaction was caused by the identified Substance."; 756 case LIKELY: 757 return "There is a high level of clinical certainty that the reaction was caused by the identified Substance."; 758 case CONFIRMED: 759 return "There is a very high level of clinical certainty that the reaction was due to the identified Substance, which may include clinical evidence by testing or rechallenge."; 760 case NULL: 761 return null; 762 default: 763 return "?"; 764 } 765 } 766 767 public String getDisplay() { 768 switch (this) { 769 case UNLIKELY: 770 return "Unlikely"; 771 case LIKELY: 772 return "Likely"; 773 case CONFIRMED: 774 return "Confirmed"; 775 case NULL: 776 return null; 777 default: 778 return "?"; 779 } 780 } 781 } 782 783 public static class AllergyIntoleranceCertaintyEnumFactory implements EnumFactory<AllergyIntoleranceCertainty> { 784 public AllergyIntoleranceCertainty fromCode(String codeString) throws IllegalArgumentException { 785 if (codeString == null || "".equals(codeString)) 786 if (codeString == null || "".equals(codeString)) 787 return null; 788 if ("unlikely".equals(codeString)) 789 return AllergyIntoleranceCertainty.UNLIKELY; 790 if ("likely".equals(codeString)) 791 return AllergyIntoleranceCertainty.LIKELY; 792 if ("confirmed".equals(codeString)) 793 return AllergyIntoleranceCertainty.CONFIRMED; 794 throw new IllegalArgumentException("Unknown AllergyIntoleranceCertainty code '" + codeString + "'"); 795 } 796 797 public Enumeration<AllergyIntoleranceCertainty> fromType(Base code) throws FHIRException { 798 if (code == null || code.isEmpty()) 799 return null; 800 String codeString = ((PrimitiveType) code).asStringValue(); 801 if (codeString == null || "".equals(codeString)) 802 return null; 803 if ("unlikely".equals(codeString)) 804 return new Enumeration<AllergyIntoleranceCertainty>(this, AllergyIntoleranceCertainty.UNLIKELY); 805 if ("likely".equals(codeString)) 806 return new Enumeration<AllergyIntoleranceCertainty>(this, AllergyIntoleranceCertainty.LIKELY); 807 if ("confirmed".equals(codeString)) 808 return new Enumeration<AllergyIntoleranceCertainty>(this, AllergyIntoleranceCertainty.CONFIRMED); 809 throw new FHIRException("Unknown AllergyIntoleranceCertainty code '" + codeString + "'"); 810 } 811 812 public String toCode(AllergyIntoleranceCertainty code) 813 { 814 if (code == AllergyIntoleranceCertainty.NULL) 815 return null; 816 if (code == AllergyIntoleranceCertainty.UNLIKELY) 817 return "unlikely"; 818 if (code == AllergyIntoleranceCertainty.LIKELY) 819 return "likely"; 820 if (code == AllergyIntoleranceCertainty.CONFIRMED) 821 return "confirmed"; 822 return "?"; 823 } 824 } 825 826 public enum AllergyIntoleranceSeverity { 827 /** 828 * Causes mild physiological effects. 829 */ 830 MILD, 831 /** 832 * Causes moderate physiological effects. 833 */ 834 MODERATE, 835 /** 836 * Causes severe physiological effects. 837 */ 838 SEVERE, 839 /** 840 * added to help the parsers 841 */ 842 NULL; 843 844 public static AllergyIntoleranceSeverity fromCode(String codeString) throws FHIRException { 845 if (codeString == null || "".equals(codeString)) 846 return null; 847 if ("mild".equals(codeString)) 848 return MILD; 849 if ("moderate".equals(codeString)) 850 return MODERATE; 851 if ("severe".equals(codeString)) 852 return SEVERE; 853 throw new FHIRException("Unknown AllergyIntoleranceSeverity code '" + codeString + "'"); 854 } 855 856 public String toCode() { 857 switch (this) { 858 case MILD: 859 return "mild"; 860 case MODERATE: 861 return "moderate"; 862 case SEVERE: 863 return "severe"; 864 case NULL: 865 return null; 866 default: 867 return "?"; 868 } 869 } 870 871 public String getSystem() { 872 switch (this) { 873 case MILD: 874 return "http://hl7.org/fhir/reaction-event-severity"; 875 case MODERATE: 876 return "http://hl7.org/fhir/reaction-event-severity"; 877 case SEVERE: 878 return "http://hl7.org/fhir/reaction-event-severity"; 879 case NULL: 880 return null; 881 default: 882 return "?"; 883 } 884 } 885 886 public String getDefinition() { 887 switch (this) { 888 case MILD: 889 return "Causes mild physiological effects."; 890 case MODERATE: 891 return "Causes moderate physiological effects."; 892 case SEVERE: 893 return "Causes severe physiological effects."; 894 case NULL: 895 return null; 896 default: 897 return "?"; 898 } 899 } 900 901 public String getDisplay() { 902 switch (this) { 903 case MILD: 904 return "Mild"; 905 case MODERATE: 906 return "Moderate"; 907 case SEVERE: 908 return "Severe"; 909 case NULL: 910 return null; 911 default: 912 return "?"; 913 } 914 } 915 } 916 917 public static class AllergyIntoleranceSeverityEnumFactory implements EnumFactory<AllergyIntoleranceSeverity> { 918 public AllergyIntoleranceSeverity fromCode(String codeString) throws IllegalArgumentException { 919 if (codeString == null || "".equals(codeString)) 920 if (codeString == null || "".equals(codeString)) 921 return null; 922 if ("mild".equals(codeString)) 923 return AllergyIntoleranceSeverity.MILD; 924 if ("moderate".equals(codeString)) 925 return AllergyIntoleranceSeverity.MODERATE; 926 if ("severe".equals(codeString)) 927 return AllergyIntoleranceSeverity.SEVERE; 928 throw new IllegalArgumentException("Unknown AllergyIntoleranceSeverity code '" + codeString + "'"); 929 } 930 931 public Enumeration<AllergyIntoleranceSeverity> fromType(Base code) throws FHIRException { 932 if (code == null || code.isEmpty()) 933 return null; 934 String codeString = ((PrimitiveType) code).asStringValue(); 935 if (codeString == null || "".equals(codeString)) 936 return null; 937 if ("mild".equals(codeString)) 938 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.MILD); 939 if ("moderate".equals(codeString)) 940 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.MODERATE); 941 if ("severe".equals(codeString)) 942 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.SEVERE); 943 throw new FHIRException("Unknown AllergyIntoleranceSeverity code '" + codeString + "'"); 944 } 945 946 public String toCode(AllergyIntoleranceSeverity code) 947 { 948 if (code == AllergyIntoleranceSeverity.NULL) 949 return null; 950 if (code == AllergyIntoleranceSeverity.MILD) 951 return "mild"; 952 if (code == AllergyIntoleranceSeverity.MODERATE) 953 return "moderate"; 954 if (code == AllergyIntoleranceSeverity.SEVERE) 955 return "severe"; 956 return "?"; 957 } 958 } 959 960 @Block() 961 public static class AllergyIntoleranceReactionComponent extends BackboneElement implements IBaseBackboneElement { 962 /** 963 * Identification of the specific substance considered to be responsible for the 964 * Adverse Reaction event. Note: the substance for a specific reaction may be 965 * different to the substance identified as the cause of the risk, but must be 966 * consistent with it. For instance, it may be a more specific substance (e.g. a 967 * brand medication) or a composite substance that includes the identified 968 * substance. It must be clinically safe to only process the 969 * AllergyIntolerance.substance and ignore the 970 * AllergyIntolerance.event.substance. 971 */ 972 @Child(name = "substance", type = { 973 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 974 @Description(shortDefinition = "Specific substance considered to be responsible for event", formalDefinition = "Identification of the specific substance considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different to the substance identified as the cause of the risk, but must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite substance that includes the identified substance. It must be clinically safe to only process the AllergyIntolerance.substance and ignore the AllergyIntolerance.event.substance.") 975 protected CodeableConcept substance; 976 977 /** 978 * Statement about the degree of clinical certainty that the specific substance 979 * was the cause of the manifestation in this reaction event. 980 */ 981 @Child(name = "certainty", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 982 @Description(shortDefinition = "unlikely | likely | confirmed - clinical certainty about the specific substance", formalDefinition = "Statement about the degree of clinical certainty that the specific substance was the cause of the manifestation in this reaction event.") 983 protected Enumeration<AllergyIntoleranceCertainty> certainty; 984 985 /** 986 * Clinical symptoms and/or signs that are observed or associated with the 987 * adverse reaction event. 988 */ 989 @Child(name = "manifestation", type = { 990 CodeableConcept.class }, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 991 @Description(shortDefinition = "Clinical symptoms/signs associated with the Event", formalDefinition = "Clinical symptoms and/or signs that are observed or associated with the adverse reaction event.") 992 protected List<CodeableConcept> manifestation; 993 994 /** 995 * Text description about the reaction as a whole, including details of the 996 * manifestation if required. 997 */ 998 @Child(name = "description", type = { 999 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1000 @Description(shortDefinition = "Description of the event as a whole", formalDefinition = "Text description about the reaction as a whole, including details of the manifestation if required.") 1001 protected StringType description; 1002 1003 /** 1004 * Record of the date and/or time of the onset of the Reaction. 1005 */ 1006 @Child(name = "onset", type = { DateTimeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1007 @Description(shortDefinition = "Date(/time) when manifestations showed", formalDefinition = "Record of the date and/or time of the onset of the Reaction.") 1008 protected DateTimeType onset; 1009 1010 /** 1011 * Clinical assessment of the severity of the reaction event as a whole, 1012 * potentially considering multiple different manifestations. 1013 */ 1014 @Child(name = "severity", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1015 @Description(shortDefinition = "mild | moderate | severe (of event as a whole)", formalDefinition = "Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations.") 1016 protected Enumeration<AllergyIntoleranceSeverity> severity; 1017 1018 /** 1019 * Identification of the route by which the subject was exposed to the 1020 * substance. 1021 */ 1022 @Child(name = "exposureRoute", type = { 1023 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1024 @Description(shortDefinition = "How the subject was exposed to the substance", formalDefinition = "Identification of the route by which the subject was exposed to the substance.") 1025 protected CodeableConcept exposureRoute; 1026 1027 /** 1028 * Additional text about the adverse reaction event not captured in other 1029 * fields. 1030 */ 1031 @Child(name = "note", type = { Annotation.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1032 @Description(shortDefinition = "Text about event not captured in other fields", formalDefinition = "Additional text about the adverse reaction event not captured in other fields.") 1033 protected Annotation note; 1034 1035 private static final long serialVersionUID = -765664367L; 1036 1037 /* 1038 * Constructor 1039 */ 1040 public AllergyIntoleranceReactionComponent() { 1041 super(); 1042 } 1043 1044 /** 1045 * @return {@link #substance} (Identification of the specific substance 1046 * considered to be responsible for the Adverse Reaction event. Note: 1047 * the substance for a specific reaction may be different to the 1048 * substance identified as the cause of the risk, but must be consistent 1049 * with it. For instance, it may be a more specific substance (e.g. a 1050 * brand medication) or a composite substance that includes the 1051 * identified substance. It must be clinically safe to only process the 1052 * AllergyIntolerance.substance and ignore the 1053 * AllergyIntolerance.event.substance.) 1054 */ 1055 public CodeableConcept getSubstance() { 1056 if (this.substance == null) 1057 if (Configuration.errorOnAutoCreate()) 1058 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.substance"); 1059 else if (Configuration.doAutoCreate()) 1060 this.substance = new CodeableConcept(); // cc 1061 return this.substance; 1062 } 1063 1064 public boolean hasSubstance() { 1065 return this.substance != null && !this.substance.isEmpty(); 1066 } 1067 1068 /** 1069 * @param value {@link #substance} (Identification of the specific substance 1070 * considered to be responsible for the Adverse Reaction event. 1071 * Note: the substance for a specific reaction may be different to 1072 * the substance identified as the cause of the risk, but must be 1073 * consistent with it. For instance, it may be a more specific 1074 * substance (e.g. a brand medication) or a composite substance 1075 * that includes the identified substance. It must be clinically 1076 * safe to only process the AllergyIntolerance.substance and ignore 1077 * the AllergyIntolerance.event.substance.) 1078 */ 1079 public AllergyIntoleranceReactionComponent setSubstance(CodeableConcept value) { 1080 this.substance = value; 1081 return this; 1082 } 1083 1084 /** 1085 * @return {@link #certainty} (Statement about the degree of clinical certainty 1086 * that the specific substance was the cause of the manifestation in 1087 * this reaction event.). This is the underlying object with id, value 1088 * and extensions. The accessor "getCertainty" gives direct access to 1089 * the value 1090 */ 1091 public Enumeration<AllergyIntoleranceCertainty> getCertaintyElement() { 1092 if (this.certainty == null) 1093 if (Configuration.errorOnAutoCreate()) 1094 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.certainty"); 1095 else if (Configuration.doAutoCreate()) 1096 this.certainty = new Enumeration<AllergyIntoleranceCertainty>(new AllergyIntoleranceCertaintyEnumFactory()); // bb 1097 return this.certainty; 1098 } 1099 1100 public boolean hasCertaintyElement() { 1101 return this.certainty != null && !this.certainty.isEmpty(); 1102 } 1103 1104 public boolean hasCertainty() { 1105 return this.certainty != null && !this.certainty.isEmpty(); 1106 } 1107 1108 /** 1109 * @param value {@link #certainty} (Statement about the degree of clinical 1110 * certainty that the specific substance was the cause of the 1111 * manifestation in this reaction event.). This is the underlying 1112 * object with id, value and extensions. The accessor 1113 * "getCertainty" gives direct access to the value 1114 */ 1115 public AllergyIntoleranceReactionComponent setCertaintyElement(Enumeration<AllergyIntoleranceCertainty> value) { 1116 this.certainty = value; 1117 return this; 1118 } 1119 1120 /** 1121 * @return Statement about the degree of clinical certainty that the specific 1122 * substance was the cause of the manifestation in this reaction event. 1123 */ 1124 public AllergyIntoleranceCertainty getCertainty() { 1125 return this.certainty == null ? null : this.certainty.getValue(); 1126 } 1127 1128 /** 1129 * @param value Statement about the degree of clinical certainty that the 1130 * specific substance was the cause of the manifestation in this 1131 * reaction event. 1132 */ 1133 public AllergyIntoleranceReactionComponent setCertainty(AllergyIntoleranceCertainty value) { 1134 if (value == null) 1135 this.certainty = null; 1136 else { 1137 if (this.certainty == null) 1138 this.certainty = new Enumeration<AllergyIntoleranceCertainty>(new AllergyIntoleranceCertaintyEnumFactory()); 1139 this.certainty.setValue(value); 1140 } 1141 return this; 1142 } 1143 1144 /** 1145 * @return {@link #manifestation} (Clinical symptoms and/or signs that are 1146 * observed or associated with the adverse reaction event.) 1147 */ 1148 public List<CodeableConcept> getManifestation() { 1149 if (this.manifestation == null) 1150 this.manifestation = new ArrayList<CodeableConcept>(); 1151 return this.manifestation; 1152 } 1153 1154 public boolean hasManifestation() { 1155 if (this.manifestation == null) 1156 return false; 1157 for (CodeableConcept item : this.manifestation) 1158 if (!item.isEmpty()) 1159 return true; 1160 return false; 1161 } 1162 1163 /** 1164 * @return {@link #manifestation} (Clinical symptoms and/or signs that are 1165 * observed or associated with the adverse reaction event.) 1166 */ 1167 // syntactic sugar 1168 public CodeableConcept addManifestation() { // 3 1169 CodeableConcept t = new CodeableConcept(); 1170 if (this.manifestation == null) 1171 this.manifestation = new ArrayList<CodeableConcept>(); 1172 this.manifestation.add(t); 1173 return t; 1174 } 1175 1176 // syntactic sugar 1177 public AllergyIntoleranceReactionComponent addManifestation(CodeableConcept t) { // 3 1178 if (t == null) 1179 return this; 1180 if (this.manifestation == null) 1181 this.manifestation = new ArrayList<CodeableConcept>(); 1182 this.manifestation.add(t); 1183 return this; 1184 } 1185 1186 /** 1187 * @return {@link #description} (Text description about the reaction as a whole, 1188 * including details of the manifestation if required.). This is the 1189 * underlying object with id, value and extensions. The accessor 1190 * "getDescription" gives direct access to the value 1191 */ 1192 public StringType getDescriptionElement() { 1193 if (this.description == null) 1194 if (Configuration.errorOnAutoCreate()) 1195 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.description"); 1196 else if (Configuration.doAutoCreate()) 1197 this.description = new StringType(); // bb 1198 return this.description; 1199 } 1200 1201 public boolean hasDescriptionElement() { 1202 return this.description != null && !this.description.isEmpty(); 1203 } 1204 1205 public boolean hasDescription() { 1206 return this.description != null && !this.description.isEmpty(); 1207 } 1208 1209 /** 1210 * @param value {@link #description} (Text description about the reaction as a 1211 * whole, including details of the manifestation if required.). 1212 * This is the underlying object with id, value and extensions. The 1213 * accessor "getDescription" gives direct access to the value 1214 */ 1215 public AllergyIntoleranceReactionComponent setDescriptionElement(StringType value) { 1216 this.description = value; 1217 return this; 1218 } 1219 1220 /** 1221 * @return Text description about the reaction as a whole, including details of 1222 * the manifestation if required. 1223 */ 1224 public String getDescription() { 1225 return this.description == null ? null : this.description.getValue(); 1226 } 1227 1228 /** 1229 * @param value Text description about the reaction as a whole, including 1230 * details of the manifestation if required. 1231 */ 1232 public AllergyIntoleranceReactionComponent setDescription(String value) { 1233 if (Utilities.noString(value)) 1234 this.description = null; 1235 else { 1236 if (this.description == null) 1237 this.description = new StringType(); 1238 this.description.setValue(value); 1239 } 1240 return this; 1241 } 1242 1243 /** 1244 * @return {@link #onset} (Record of the date and/or time of the onset of the 1245 * Reaction.). This is the underlying object with id, value and 1246 * extensions. The accessor "getOnset" gives direct access to the value 1247 */ 1248 public DateTimeType getOnsetElement() { 1249 if (this.onset == null) 1250 if (Configuration.errorOnAutoCreate()) 1251 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.onset"); 1252 else if (Configuration.doAutoCreate()) 1253 this.onset = new DateTimeType(); // bb 1254 return this.onset; 1255 } 1256 1257 public boolean hasOnsetElement() { 1258 return this.onset != null && !this.onset.isEmpty(); 1259 } 1260 1261 public boolean hasOnset() { 1262 return this.onset != null && !this.onset.isEmpty(); 1263 } 1264 1265 /** 1266 * @param value {@link #onset} (Record of the date and/or time of the onset of 1267 * the Reaction.). This is the underlying object with id, value and 1268 * extensions. The accessor "getOnset" gives direct access to the 1269 * value 1270 */ 1271 public AllergyIntoleranceReactionComponent setOnsetElement(DateTimeType value) { 1272 this.onset = value; 1273 return this; 1274 } 1275 1276 /** 1277 * @return Record of the date and/or time of the onset of the Reaction. 1278 */ 1279 public Date getOnset() { 1280 return this.onset == null ? null : this.onset.getValue(); 1281 } 1282 1283 /** 1284 * @param value Record of the date and/or time of the onset of the Reaction. 1285 */ 1286 public AllergyIntoleranceReactionComponent setOnset(Date value) { 1287 if (value == null) 1288 this.onset = null; 1289 else { 1290 if (this.onset == null) 1291 this.onset = new DateTimeType(); 1292 this.onset.setValue(value); 1293 } 1294 return this; 1295 } 1296 1297 /** 1298 * @return {@link #severity} (Clinical assessment of the severity of the 1299 * reaction event as a whole, potentially considering multiple different 1300 * manifestations.). This is the underlying object with id, value and 1301 * extensions. The accessor "getSeverity" gives direct access to the 1302 * value 1303 */ 1304 public Enumeration<AllergyIntoleranceSeverity> getSeverityElement() { 1305 if (this.severity == null) 1306 if (Configuration.errorOnAutoCreate()) 1307 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.severity"); 1308 else if (Configuration.doAutoCreate()) 1309 this.severity = new Enumeration<AllergyIntoleranceSeverity>(new AllergyIntoleranceSeverityEnumFactory()); // bb 1310 return this.severity; 1311 } 1312 1313 public boolean hasSeverityElement() { 1314 return this.severity != null && !this.severity.isEmpty(); 1315 } 1316 1317 public boolean hasSeverity() { 1318 return this.severity != null && !this.severity.isEmpty(); 1319 } 1320 1321 /** 1322 * @param value {@link #severity} (Clinical assessment of the severity of the 1323 * reaction event as a whole, potentially considering multiple 1324 * different manifestations.). This is the underlying object with 1325 * id, value and extensions. The accessor "getSeverity" gives 1326 * direct access to the value 1327 */ 1328 public AllergyIntoleranceReactionComponent setSeverityElement(Enumeration<AllergyIntoleranceSeverity> value) { 1329 this.severity = value; 1330 return this; 1331 } 1332 1333 /** 1334 * @return Clinical assessment of the severity of the reaction event as a whole, 1335 * potentially considering multiple different manifestations. 1336 */ 1337 public AllergyIntoleranceSeverity getSeverity() { 1338 return this.severity == null ? null : this.severity.getValue(); 1339 } 1340 1341 /** 1342 * @param value Clinical assessment of the severity of the reaction event as a 1343 * whole, potentially considering multiple different 1344 * manifestations. 1345 */ 1346 public AllergyIntoleranceReactionComponent setSeverity(AllergyIntoleranceSeverity value) { 1347 if (value == null) 1348 this.severity = null; 1349 else { 1350 if (this.severity == null) 1351 this.severity = new Enumeration<AllergyIntoleranceSeverity>(new AllergyIntoleranceSeverityEnumFactory()); 1352 this.severity.setValue(value); 1353 } 1354 return this; 1355 } 1356 1357 /** 1358 * @return {@link #exposureRoute} (Identification of the route by which the 1359 * subject was exposed to the substance.) 1360 */ 1361 public CodeableConcept getExposureRoute() { 1362 if (this.exposureRoute == null) 1363 if (Configuration.errorOnAutoCreate()) 1364 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.exposureRoute"); 1365 else if (Configuration.doAutoCreate()) 1366 this.exposureRoute = new CodeableConcept(); // cc 1367 return this.exposureRoute; 1368 } 1369 1370 public boolean hasExposureRoute() { 1371 return this.exposureRoute != null && !this.exposureRoute.isEmpty(); 1372 } 1373 1374 /** 1375 * @param value {@link #exposureRoute} (Identification of the route by which the 1376 * subject was exposed to the substance.) 1377 */ 1378 public AllergyIntoleranceReactionComponent setExposureRoute(CodeableConcept value) { 1379 this.exposureRoute = value; 1380 return this; 1381 } 1382 1383 /** 1384 * @return {@link #note} (Additional text about the adverse reaction event not 1385 * captured in other fields.) 1386 */ 1387 public Annotation getNote() { 1388 if (this.note == null) 1389 if (Configuration.errorOnAutoCreate()) 1390 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.note"); 1391 else if (Configuration.doAutoCreate()) 1392 this.note = new Annotation(); // cc 1393 return this.note; 1394 } 1395 1396 public boolean hasNote() { 1397 return this.note != null && !this.note.isEmpty(); 1398 } 1399 1400 /** 1401 * @param value {@link #note} (Additional text about the adverse reaction event 1402 * not captured in other fields.) 1403 */ 1404 public AllergyIntoleranceReactionComponent setNote(Annotation value) { 1405 this.note = value; 1406 return this; 1407 } 1408 1409 protected void listChildren(List<Property> childrenList) { 1410 super.listChildren(childrenList); 1411 childrenList.add(new Property("substance", "CodeableConcept", 1412 "Identification of the specific substance considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different to the substance identified as the cause of the risk, but must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite substance that includes the identified substance. It must be clinically safe to only process the AllergyIntolerance.substance and ignore the AllergyIntolerance.event.substance.", 1413 0, java.lang.Integer.MAX_VALUE, substance)); 1414 childrenList.add(new Property("certainty", "code", 1415 "Statement about the degree of clinical certainty that the specific substance was the cause of the manifestation in this reaction event.", 1416 0, java.lang.Integer.MAX_VALUE, certainty)); 1417 childrenList.add(new Property("manifestation", "CodeableConcept", 1418 "Clinical symptoms and/or signs that are observed or associated with the adverse reaction event.", 0, 1419 java.lang.Integer.MAX_VALUE, manifestation)); 1420 childrenList.add(new Property("description", "string", 1421 "Text description about the reaction as a whole, including details of the manifestation if required.", 0, 1422 java.lang.Integer.MAX_VALUE, description)); 1423 childrenList.add(new Property("onset", "dateTime", "Record of the date and/or time of the onset of the Reaction.", 1424 0, java.lang.Integer.MAX_VALUE, onset)); 1425 childrenList.add(new Property("severity", "code", 1426 "Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations.", 1427 0, java.lang.Integer.MAX_VALUE, severity)); 1428 childrenList.add(new Property("exposureRoute", "CodeableConcept", 1429 "Identification of the route by which the subject was exposed to the substance.", 0, 1430 java.lang.Integer.MAX_VALUE, exposureRoute)); 1431 childrenList.add(new Property("note", "Annotation", 1432 "Additional text about the adverse reaction event not captured in other fields.", 0, 1433 java.lang.Integer.MAX_VALUE, note)); 1434 } 1435 1436 @Override 1437 public void setProperty(String name, Base value) throws FHIRException { 1438 if (name.equals("substance")) 1439 this.substance = castToCodeableConcept(value); // CodeableConcept 1440 else if (name.equals("certainty")) 1441 this.certainty = new AllergyIntoleranceCertaintyEnumFactory().fromType(value); // Enumeration<AllergyIntoleranceCertainty> 1442 else if (name.equals("manifestation")) 1443 this.getManifestation().add(castToCodeableConcept(value)); 1444 else if (name.equals("description")) 1445 this.description = castToString(value); // StringType 1446 else if (name.equals("onset")) 1447 this.onset = castToDateTime(value); // DateTimeType 1448 else if (name.equals("severity")) 1449 this.severity = new AllergyIntoleranceSeverityEnumFactory().fromType(value); // Enumeration<AllergyIntoleranceSeverity> 1450 else if (name.equals("exposureRoute")) 1451 this.exposureRoute = castToCodeableConcept(value); // CodeableConcept 1452 else if (name.equals("note")) 1453 this.note = castToAnnotation(value); // Annotation 1454 else 1455 super.setProperty(name, value); 1456 } 1457 1458 @Override 1459 public Base addChild(String name) throws FHIRException { 1460 if (name.equals("substance")) { 1461 this.substance = new CodeableConcept(); 1462 return this.substance; 1463 } else if (name.equals("certainty")) { 1464 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.certainty"); 1465 } else if (name.equals("manifestation")) { 1466 return addManifestation(); 1467 } else if (name.equals("description")) { 1468 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.description"); 1469 } else if (name.equals("onset")) { 1470 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.onset"); 1471 } else if (name.equals("severity")) { 1472 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.severity"); 1473 } else if (name.equals("exposureRoute")) { 1474 this.exposureRoute = new CodeableConcept(); 1475 return this.exposureRoute; 1476 } else if (name.equals("note")) { 1477 this.note = new Annotation(); 1478 return this.note; 1479 } else 1480 return super.addChild(name); 1481 } 1482 1483 public AllergyIntoleranceReactionComponent copy() { 1484 AllergyIntoleranceReactionComponent dst = new AllergyIntoleranceReactionComponent(); 1485 copyValues(dst); 1486 dst.substance = substance == null ? null : substance.copy(); 1487 dst.certainty = certainty == null ? null : certainty.copy(); 1488 if (manifestation != null) { 1489 dst.manifestation = new ArrayList<CodeableConcept>(); 1490 for (CodeableConcept i : manifestation) 1491 dst.manifestation.add(i.copy()); 1492 } 1493 ; 1494 dst.description = description == null ? null : description.copy(); 1495 dst.onset = onset == null ? null : onset.copy(); 1496 dst.severity = severity == null ? null : severity.copy(); 1497 dst.exposureRoute = exposureRoute == null ? null : exposureRoute.copy(); 1498 dst.note = note == null ? null : note.copy(); 1499 return dst; 1500 } 1501 1502 @Override 1503 public boolean equalsDeep(Base other) { 1504 if (!super.equalsDeep(other)) 1505 return false; 1506 if (!(other instanceof AllergyIntoleranceReactionComponent)) 1507 return false; 1508 AllergyIntoleranceReactionComponent o = (AllergyIntoleranceReactionComponent) other; 1509 return compareDeep(substance, o.substance, true) && compareDeep(certainty, o.certainty, true) 1510 && compareDeep(manifestation, o.manifestation, true) && compareDeep(description, o.description, true) 1511 && compareDeep(onset, o.onset, true) && compareDeep(severity, o.severity, true) 1512 && compareDeep(exposureRoute, o.exposureRoute, true) && compareDeep(note, o.note, true); 1513 } 1514 1515 @Override 1516 public boolean equalsShallow(Base other) { 1517 if (!super.equalsShallow(other)) 1518 return false; 1519 if (!(other instanceof AllergyIntoleranceReactionComponent)) 1520 return false; 1521 AllergyIntoleranceReactionComponent o = (AllergyIntoleranceReactionComponent) other; 1522 return compareValues(certainty, o.certainty, true) && compareValues(description, o.description, true) 1523 && compareValues(onset, o.onset, true) && compareValues(severity, o.severity, true); 1524 } 1525 1526 public boolean isEmpty() { 1527 return super.isEmpty() && (substance == null || substance.isEmpty()) && (certainty == null || certainty.isEmpty()) 1528 && (manifestation == null || manifestation.isEmpty()) && (description == null || description.isEmpty()) 1529 && (onset == null || onset.isEmpty()) && (severity == null || severity.isEmpty()) 1530 && (exposureRoute == null || exposureRoute.isEmpty()) && (note == null || note.isEmpty()); 1531 } 1532 1533 public String fhirType() { 1534 return "AllergyIntolerance.reaction"; 1535 1536 } 1537 1538 } 1539 1540 /** 1541 * This records identifiers associated with this allergy/intolerance concern 1542 * that are defined by business processes and/or used to refer to it when a 1543 * direct URL reference to the resource itself is not appropriate (e.g. in CDA 1544 * documents, or in written / printed documentation). 1545 */ 1546 @Child(name = "identifier", type = { 1547 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1548 @Description(shortDefinition = "External ids for this item", formalDefinition = "This records identifiers associated with this allergy/intolerance concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).") 1549 protected List<Identifier> identifier; 1550 1551 /** 1552 * Record of the date and/or time of the onset of the Allergy or Intolerance. 1553 */ 1554 @Child(name = "onset", type = { DateTimeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1555 @Description(shortDefinition = "Date(/time) when manifestations showed", formalDefinition = "Record of the date and/or time of the onset of the Allergy or Intolerance.") 1556 protected DateTimeType onset; 1557 1558 /** 1559 * Date when the sensitivity was recorded. 1560 */ 1561 @Child(name = "recordedDate", type = { 1562 DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1563 @Description(shortDefinition = "When recorded", formalDefinition = "Date when the sensitivity was recorded.") 1564 protected DateTimeType recordedDate; 1565 1566 /** 1567 * Individual who recorded the record and takes responsibility for its conten. 1568 */ 1569 @Child(name = "recorder", type = { Practitioner.class, 1570 Patient.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1571 @Description(shortDefinition = "Who recorded the sensitivity", formalDefinition = "Individual who recorded the record and takes responsibility for its conten.") 1572 protected Reference recorder; 1573 1574 /** 1575 * The actual object that is the target of the reference (Individual who 1576 * recorded the record and takes responsibility for its conten.) 1577 */ 1578 protected Resource recorderTarget; 1579 1580 /** 1581 * The patient who has the allergy or intolerance. 1582 */ 1583 @Child(name = "patient", type = { Patient.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 1584 @Description(shortDefinition = "Who the sensitivity is for", formalDefinition = "The patient who has the allergy or intolerance.") 1585 protected Reference patient; 1586 1587 /** 1588 * The actual object that is the target of the reference (The patient who has 1589 * the allergy or intolerance.) 1590 */ 1591 protected Patient patientTarget; 1592 1593 /** 1594 * The source of the information about the allergy that is recorded. 1595 */ 1596 @Child(name = "reporter", type = { Patient.class, RelatedPerson.class, 1597 Practitioner.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1598 @Description(shortDefinition = "Source of the information about the allergy", formalDefinition = "The source of the information about the allergy that is recorded.") 1599 protected Reference reporter; 1600 1601 /** 1602 * The actual object that is the target of the reference (The source of the 1603 * information about the allergy that is recorded.) 1604 */ 1605 protected Resource reporterTarget; 1606 1607 /** 1608 * Identification of a substance, or a class of substances, that is considered 1609 * to be responsible for the adverse reaction risk. 1610 */ 1611 @Child(name = "substance", type = { 1612 CodeableConcept.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 1613 @Description(shortDefinition = "Substance, (or class) considered to be responsible for risk", formalDefinition = "Identification of a substance, or a class of substances, that is considered to be responsible for the adverse reaction risk.") 1614 protected CodeableConcept substance; 1615 1616 /** 1617 * Assertion about certainty associated with the propensity, or potential risk, 1618 * of a reaction to the identified Substance. 1619 */ 1620 @Child(name = "status", type = { CodeType.class }, order = 7, min = 0, max = 1, modifier = true, summary = true) 1621 @Description(shortDefinition = "active | unconfirmed | confirmed | inactive | resolved | refuted | entered-in-error", formalDefinition = "Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified Substance.") 1622 protected Enumeration<AllergyIntoleranceStatus> status; 1623 1624 /** 1625 * Estimate of the potential clinical harm, or seriousness, of the reaction to 1626 * the identified Substance. 1627 */ 1628 @Child(name = "criticality", type = { CodeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1629 @Description(shortDefinition = "CRITL | CRITH | CRITU", formalDefinition = "Estimate of the potential clinical harm, or seriousness, of the reaction to the identified Substance.") 1630 protected Enumeration<AllergyIntoleranceCriticality> criticality; 1631 1632 /** 1633 * Identification of the underlying physiological mechanism for the reaction 1634 * risk. 1635 */ 1636 @Child(name = "type", type = { CodeType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1637 @Description(shortDefinition = "allergy | intolerance - Underlying mechanism (if known)", formalDefinition = "Identification of the underlying physiological mechanism for the reaction risk.") 1638 protected Enumeration<AllergyIntoleranceType> type; 1639 1640 /** 1641 * Category of the identified Substance. 1642 */ 1643 @Child(name = "category", type = { CodeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1644 @Description(shortDefinition = "food | medication | environment | other - Category of Substance", formalDefinition = "Category of the identified Substance.") 1645 protected Enumeration<AllergyIntoleranceCategory> category; 1646 1647 /** 1648 * Represents the date and/or time of the last known occurrence of a reaction 1649 * event. 1650 */ 1651 @Child(name = "lastOccurence", type = { 1652 DateTimeType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 1653 @Description(shortDefinition = "Date(/time) of last known occurrence of a reaction", formalDefinition = "Represents the date and/or time of the last known occurrence of a reaction event.") 1654 protected DateTimeType lastOccurence; 1655 1656 /** 1657 * Additional narrative about the propensity for the Adverse Reaction, not 1658 * captured in other fields. 1659 */ 1660 @Child(name = "note", type = { Annotation.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 1661 @Description(shortDefinition = "Additional text not captured in other fields", formalDefinition = "Additional narrative about the propensity for the Adverse Reaction, not captured in other fields.") 1662 protected Annotation note; 1663 1664 /** 1665 * Details about each adverse reaction event linked to exposure to the 1666 * identified Substance. 1667 */ 1668 @Child(name = "reaction", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1669 @Description(shortDefinition = "Adverse Reaction Events linked to exposure to substance", formalDefinition = "Details about each adverse reaction event linked to exposure to the identified Substance.") 1670 protected List<AllergyIntoleranceReactionComponent> reaction; 1671 1672 private static final long serialVersionUID = -1657522921L; 1673 1674 /* 1675 * Constructor 1676 */ 1677 public AllergyIntolerance() { 1678 super(); 1679 } 1680 1681 /* 1682 * Constructor 1683 */ 1684 public AllergyIntolerance(Reference patient, CodeableConcept substance) { 1685 super(); 1686 this.patient = patient; 1687 this.substance = substance; 1688 } 1689 1690 /** 1691 * @return {@link #identifier} (This records identifiers associated with this 1692 * allergy/intolerance concern that are defined by business processes 1693 * and/or used to refer to it when a direct URL reference to the 1694 * resource itself is not appropriate (e.g. in CDA documents, or in 1695 * written / printed documentation).) 1696 */ 1697 public List<Identifier> getIdentifier() { 1698 if (this.identifier == null) 1699 this.identifier = new ArrayList<Identifier>(); 1700 return this.identifier; 1701 } 1702 1703 public boolean hasIdentifier() { 1704 if (this.identifier == null) 1705 return false; 1706 for (Identifier item : this.identifier) 1707 if (!item.isEmpty()) 1708 return true; 1709 return false; 1710 } 1711 1712 /** 1713 * @return {@link #identifier} (This records identifiers associated with this 1714 * allergy/intolerance concern that are defined by business processes 1715 * and/or used to refer to it when a direct URL reference to the 1716 * resource itself is not appropriate (e.g. in CDA documents, or in 1717 * written / printed documentation).) 1718 */ 1719 // syntactic sugar 1720 public Identifier addIdentifier() { // 3 1721 Identifier t = new Identifier(); 1722 if (this.identifier == null) 1723 this.identifier = new ArrayList<Identifier>(); 1724 this.identifier.add(t); 1725 return t; 1726 } 1727 1728 // syntactic sugar 1729 public AllergyIntolerance addIdentifier(Identifier t) { // 3 1730 if (t == null) 1731 return this; 1732 if (this.identifier == null) 1733 this.identifier = new ArrayList<Identifier>(); 1734 this.identifier.add(t); 1735 return this; 1736 } 1737 1738 /** 1739 * @return {@link #onset} (Record of the date and/or time of the onset of the 1740 * Allergy or Intolerance.). This is the underlying object with id, 1741 * value and extensions. The accessor "getOnset" gives direct access to 1742 * the value 1743 */ 1744 public DateTimeType getOnsetElement() { 1745 if (this.onset == null) 1746 if (Configuration.errorOnAutoCreate()) 1747 throw new Error("Attempt to auto-create AllergyIntolerance.onset"); 1748 else if (Configuration.doAutoCreate()) 1749 this.onset = new DateTimeType(); // bb 1750 return this.onset; 1751 } 1752 1753 public boolean hasOnsetElement() { 1754 return this.onset != null && !this.onset.isEmpty(); 1755 } 1756 1757 public boolean hasOnset() { 1758 return this.onset != null && !this.onset.isEmpty(); 1759 } 1760 1761 /** 1762 * @param value {@link #onset} (Record of the date and/or time of the onset of 1763 * the Allergy or Intolerance.). This is the underlying object with 1764 * id, value and extensions. The accessor "getOnset" gives direct 1765 * access to the value 1766 */ 1767 public AllergyIntolerance setOnsetElement(DateTimeType value) { 1768 this.onset = value; 1769 return this; 1770 } 1771 1772 /** 1773 * @return Record of the date and/or time of the onset of the Allergy or 1774 * Intolerance. 1775 */ 1776 public Date getOnset() { 1777 return this.onset == null ? null : this.onset.getValue(); 1778 } 1779 1780 /** 1781 * @param value Record of the date and/or time of the onset of the Allergy or 1782 * Intolerance. 1783 */ 1784 public AllergyIntolerance setOnset(Date value) { 1785 if (value == null) 1786 this.onset = null; 1787 else { 1788 if (this.onset == null) 1789 this.onset = new DateTimeType(); 1790 this.onset.setValue(value); 1791 } 1792 return this; 1793 } 1794 1795 /** 1796 * @return {@link #recordedDate} (Date when the sensitivity was recorded.). This 1797 * is the underlying object with id, value and extensions. The accessor 1798 * "getRecordedDate" gives direct access to the value 1799 */ 1800 public DateTimeType getRecordedDateElement() { 1801 if (this.recordedDate == null) 1802 if (Configuration.errorOnAutoCreate()) 1803 throw new Error("Attempt to auto-create AllergyIntolerance.recordedDate"); 1804 else if (Configuration.doAutoCreate()) 1805 this.recordedDate = new DateTimeType(); // bb 1806 return this.recordedDate; 1807 } 1808 1809 public boolean hasRecordedDateElement() { 1810 return this.recordedDate != null && !this.recordedDate.isEmpty(); 1811 } 1812 1813 public boolean hasRecordedDate() { 1814 return this.recordedDate != null && !this.recordedDate.isEmpty(); 1815 } 1816 1817 /** 1818 * @param value {@link #recordedDate} (Date when the sensitivity was recorded.). 1819 * This is the underlying object with id, value and extensions. The 1820 * accessor "getRecordedDate" gives direct access to the value 1821 */ 1822 public AllergyIntolerance setRecordedDateElement(DateTimeType value) { 1823 this.recordedDate = value; 1824 return this; 1825 } 1826 1827 /** 1828 * @return Date when the sensitivity was recorded. 1829 */ 1830 public Date getRecordedDate() { 1831 return this.recordedDate == null ? null : this.recordedDate.getValue(); 1832 } 1833 1834 /** 1835 * @param value Date when the sensitivity was recorded. 1836 */ 1837 public AllergyIntolerance setRecordedDate(Date value) { 1838 if (value == null) 1839 this.recordedDate = null; 1840 else { 1841 if (this.recordedDate == null) 1842 this.recordedDate = new DateTimeType(); 1843 this.recordedDate.setValue(value); 1844 } 1845 return this; 1846 } 1847 1848 /** 1849 * @return {@link #recorder} (Individual who recorded the record and takes 1850 * responsibility for its conten.) 1851 */ 1852 public Reference getRecorder() { 1853 if (this.recorder == null) 1854 if (Configuration.errorOnAutoCreate()) 1855 throw new Error("Attempt to auto-create AllergyIntolerance.recorder"); 1856 else if (Configuration.doAutoCreate()) 1857 this.recorder = new Reference(); // cc 1858 return this.recorder; 1859 } 1860 1861 public boolean hasRecorder() { 1862 return this.recorder != null && !this.recorder.isEmpty(); 1863 } 1864 1865 /** 1866 * @param value {@link #recorder} (Individual who recorded the record and takes 1867 * responsibility for its conten.) 1868 */ 1869 public AllergyIntolerance setRecorder(Reference value) { 1870 this.recorder = value; 1871 return this; 1872 } 1873 1874 /** 1875 * @return {@link #recorder} The actual object that is the target of the 1876 * reference. The reference library doesn't populate this, but you can 1877 * use it to hold the resource if you resolve it. (Individual who 1878 * recorded the record and takes responsibility for its conten.) 1879 */ 1880 public Resource getRecorderTarget() { 1881 return this.recorderTarget; 1882 } 1883 1884 /** 1885 * @param value {@link #recorder} The actual object that is the target of the 1886 * reference. The reference library doesn't use these, but you can 1887 * use it to hold the resource if you resolve it. (Individual who 1888 * recorded the record and takes responsibility for its conten.) 1889 */ 1890 public AllergyIntolerance setRecorderTarget(Resource value) { 1891 this.recorderTarget = value; 1892 return this; 1893 } 1894 1895 /** 1896 * @return {@link #patient} (The patient who has the allergy or intolerance.) 1897 */ 1898 public Reference getPatient() { 1899 if (this.patient == null) 1900 if (Configuration.errorOnAutoCreate()) 1901 throw new Error("Attempt to auto-create AllergyIntolerance.patient"); 1902 else if (Configuration.doAutoCreate()) 1903 this.patient = new Reference(); // cc 1904 return this.patient; 1905 } 1906 1907 public boolean hasPatient() { 1908 return this.patient != null && !this.patient.isEmpty(); 1909 } 1910 1911 /** 1912 * @param value {@link #patient} (The patient who has the allergy or 1913 * intolerance.) 1914 */ 1915 public AllergyIntolerance setPatient(Reference value) { 1916 this.patient = value; 1917 return this; 1918 } 1919 1920 /** 1921 * @return {@link #patient} The actual object that is the target of the 1922 * reference. The reference library doesn't populate this, but you can 1923 * use it to hold the resource if you resolve it. (The patient who has 1924 * the allergy or intolerance.) 1925 */ 1926 public Patient getPatientTarget() { 1927 if (this.patientTarget == null) 1928 if (Configuration.errorOnAutoCreate()) 1929 throw new Error("Attempt to auto-create AllergyIntolerance.patient"); 1930 else if (Configuration.doAutoCreate()) 1931 this.patientTarget = new Patient(); // aa 1932 return this.patientTarget; 1933 } 1934 1935 /** 1936 * @param value {@link #patient} The actual object that is the target of the 1937 * reference. The reference library doesn't use these, but you can 1938 * use it to hold the resource if you resolve it. (The patient who 1939 * has the allergy or intolerance.) 1940 */ 1941 public AllergyIntolerance setPatientTarget(Patient value) { 1942 this.patientTarget = value; 1943 return this; 1944 } 1945 1946 /** 1947 * @return {@link #reporter} (The source of the information about the allergy 1948 * that is recorded.) 1949 */ 1950 public Reference getReporter() { 1951 if (this.reporter == null) 1952 if (Configuration.errorOnAutoCreate()) 1953 throw new Error("Attempt to auto-create AllergyIntolerance.reporter"); 1954 else if (Configuration.doAutoCreate()) 1955 this.reporter = new Reference(); // cc 1956 return this.reporter; 1957 } 1958 1959 public boolean hasReporter() { 1960 return this.reporter != null && !this.reporter.isEmpty(); 1961 } 1962 1963 /** 1964 * @param value {@link #reporter} (The source of the information about the 1965 * allergy that is recorded.) 1966 */ 1967 public AllergyIntolerance setReporter(Reference value) { 1968 this.reporter = value; 1969 return this; 1970 } 1971 1972 /** 1973 * @return {@link #reporter} The actual object that is the target of the 1974 * reference. The reference library doesn't populate this, but you can 1975 * use it to hold the resource if you resolve it. (The source of the 1976 * information about the allergy that is recorded.) 1977 */ 1978 public Resource getReporterTarget() { 1979 return this.reporterTarget; 1980 } 1981 1982 /** 1983 * @param value {@link #reporter} The actual object that is the target of the 1984 * reference. The reference library doesn't use these, but you can 1985 * use it to hold the resource if you resolve it. (The source of 1986 * the information about the allergy that is recorded.) 1987 */ 1988 public AllergyIntolerance setReporterTarget(Resource value) { 1989 this.reporterTarget = value; 1990 return this; 1991 } 1992 1993 /** 1994 * @return {@link #substance} (Identification of a substance, or a class of 1995 * substances, that is considered to be responsible for the adverse 1996 * reaction risk.) 1997 */ 1998 public CodeableConcept getSubstance() { 1999 if (this.substance == null) 2000 if (Configuration.errorOnAutoCreate()) 2001 throw new Error("Attempt to auto-create AllergyIntolerance.substance"); 2002 else if (Configuration.doAutoCreate()) 2003 this.substance = new CodeableConcept(); // cc 2004 return this.substance; 2005 } 2006 2007 public boolean hasSubstance() { 2008 return this.substance != null && !this.substance.isEmpty(); 2009 } 2010 2011 /** 2012 * @param value {@link #substance} (Identification of a substance, or a class of 2013 * substances, that is considered to be responsible for the adverse 2014 * reaction risk.) 2015 */ 2016 public AllergyIntolerance setSubstance(CodeableConcept value) { 2017 this.substance = value; 2018 return this; 2019 } 2020 2021 /** 2022 * @return {@link #status} (Assertion about certainty associated with the 2023 * propensity, or potential risk, of a reaction to the identified 2024 * Substance.). This is the underlying object with id, value and 2025 * extensions. The accessor "getStatus" gives direct access to the value 2026 */ 2027 public Enumeration<AllergyIntoleranceStatus> getStatusElement() { 2028 if (this.status == null) 2029 if (Configuration.errorOnAutoCreate()) 2030 throw new Error("Attempt to auto-create AllergyIntolerance.status"); 2031 else if (Configuration.doAutoCreate()) 2032 this.status = new Enumeration<AllergyIntoleranceStatus>(new AllergyIntoleranceStatusEnumFactory()); // bb 2033 return this.status; 2034 } 2035 2036 public boolean hasStatusElement() { 2037 return this.status != null && !this.status.isEmpty(); 2038 } 2039 2040 public boolean hasStatus() { 2041 return this.status != null && !this.status.isEmpty(); 2042 } 2043 2044 /** 2045 * @param value {@link #status} (Assertion about certainty associated with the 2046 * propensity, or potential risk, of a reaction to the identified 2047 * Substance.). This is the underlying object with id, value and 2048 * extensions. The accessor "getStatus" gives direct access to the 2049 * value 2050 */ 2051 public AllergyIntolerance setStatusElement(Enumeration<AllergyIntoleranceStatus> value) { 2052 this.status = value; 2053 return this; 2054 } 2055 2056 /** 2057 * @return Assertion about certainty associated with the propensity, or 2058 * potential risk, of a reaction to the identified Substance. 2059 */ 2060 public AllergyIntoleranceStatus getStatus() { 2061 return this.status == null ? null : this.status.getValue(); 2062 } 2063 2064 /** 2065 * @param value Assertion about certainty associated with the propensity, or 2066 * potential risk, of a reaction to the identified Substance. 2067 */ 2068 public AllergyIntolerance setStatus(AllergyIntoleranceStatus value) { 2069 if (value == null) 2070 this.status = null; 2071 else { 2072 if (this.status == null) 2073 this.status = new Enumeration<AllergyIntoleranceStatus>(new AllergyIntoleranceStatusEnumFactory()); 2074 this.status.setValue(value); 2075 } 2076 return this; 2077 } 2078 2079 /** 2080 * @return {@link #criticality} (Estimate of the potential clinical harm, or 2081 * seriousness, of the reaction to the identified Substance.). This is 2082 * the underlying object with id, value and extensions. The accessor 2083 * "getCriticality" gives direct access to the value 2084 */ 2085 public Enumeration<AllergyIntoleranceCriticality> getCriticalityElement() { 2086 if (this.criticality == null) 2087 if (Configuration.errorOnAutoCreate()) 2088 throw new Error("Attempt to auto-create AllergyIntolerance.criticality"); 2089 else if (Configuration.doAutoCreate()) 2090 this.criticality = new Enumeration<AllergyIntoleranceCriticality>( 2091 new AllergyIntoleranceCriticalityEnumFactory()); // bb 2092 return this.criticality; 2093 } 2094 2095 public boolean hasCriticalityElement() { 2096 return this.criticality != null && !this.criticality.isEmpty(); 2097 } 2098 2099 public boolean hasCriticality() { 2100 return this.criticality != null && !this.criticality.isEmpty(); 2101 } 2102 2103 /** 2104 * @param value {@link #criticality} (Estimate of the potential clinical harm, 2105 * or seriousness, of the reaction to the identified Substance.). 2106 * This is the underlying object with id, value and extensions. The 2107 * accessor "getCriticality" gives direct access to the value 2108 */ 2109 public AllergyIntolerance setCriticalityElement(Enumeration<AllergyIntoleranceCriticality> value) { 2110 this.criticality = value; 2111 return this; 2112 } 2113 2114 /** 2115 * @return Estimate of the potential clinical harm, or seriousness, of the 2116 * reaction to the identified Substance. 2117 */ 2118 public AllergyIntoleranceCriticality getCriticality() { 2119 return this.criticality == null ? null : this.criticality.getValue(); 2120 } 2121 2122 /** 2123 * @param value Estimate of the potential clinical harm, or seriousness, of the 2124 * reaction to the identified Substance. 2125 */ 2126 public AllergyIntolerance setCriticality(AllergyIntoleranceCriticality value) { 2127 if (value == null) 2128 this.criticality = null; 2129 else { 2130 if (this.criticality == null) 2131 this.criticality = new Enumeration<AllergyIntoleranceCriticality>( 2132 new AllergyIntoleranceCriticalityEnumFactory()); 2133 this.criticality.setValue(value); 2134 } 2135 return this; 2136 } 2137 2138 /** 2139 * @return {@link #type} (Identification of the underlying physiological 2140 * mechanism for the reaction risk.). This is the underlying object with 2141 * id, value and extensions. The accessor "getType" gives direct access 2142 * to the value 2143 */ 2144 public Enumeration<AllergyIntoleranceType> getTypeElement() { 2145 if (this.type == null) 2146 if (Configuration.errorOnAutoCreate()) 2147 throw new Error("Attempt to auto-create AllergyIntolerance.type"); 2148 else if (Configuration.doAutoCreate()) 2149 this.type = new Enumeration<AllergyIntoleranceType>(new AllergyIntoleranceTypeEnumFactory()); // bb 2150 return this.type; 2151 } 2152 2153 public boolean hasTypeElement() { 2154 return this.type != null && !this.type.isEmpty(); 2155 } 2156 2157 public boolean hasType() { 2158 return this.type != null && !this.type.isEmpty(); 2159 } 2160 2161 /** 2162 * @param value {@link #type} (Identification of the underlying physiological 2163 * mechanism for the reaction risk.). This is the underlying object 2164 * with id, value and extensions. The accessor "getType" gives 2165 * direct access to the value 2166 */ 2167 public AllergyIntolerance setTypeElement(Enumeration<AllergyIntoleranceType> value) { 2168 this.type = value; 2169 return this; 2170 } 2171 2172 /** 2173 * @return Identification of the underlying physiological mechanism for the 2174 * reaction risk. 2175 */ 2176 public AllergyIntoleranceType getType() { 2177 return this.type == null ? null : this.type.getValue(); 2178 } 2179 2180 /** 2181 * @param value Identification of the underlying physiological mechanism for the 2182 * reaction risk. 2183 */ 2184 public AllergyIntolerance setType(AllergyIntoleranceType value) { 2185 if (value == null) 2186 this.type = null; 2187 else { 2188 if (this.type == null) 2189 this.type = new Enumeration<AllergyIntoleranceType>(new AllergyIntoleranceTypeEnumFactory()); 2190 this.type.setValue(value); 2191 } 2192 return this; 2193 } 2194 2195 /** 2196 * @return {@link #category} (Category of the identified Substance.). This is 2197 * the underlying object with id, value and extensions. The accessor 2198 * "getCategory" gives direct access to the value 2199 */ 2200 public Enumeration<AllergyIntoleranceCategory> getCategoryElement() { 2201 if (this.category == null) 2202 if (Configuration.errorOnAutoCreate()) 2203 throw new Error("Attempt to auto-create AllergyIntolerance.category"); 2204 else if (Configuration.doAutoCreate()) 2205 this.category = new Enumeration<AllergyIntoleranceCategory>(new AllergyIntoleranceCategoryEnumFactory()); // bb 2206 return this.category; 2207 } 2208 2209 public boolean hasCategoryElement() { 2210 return this.category != null && !this.category.isEmpty(); 2211 } 2212 2213 public boolean hasCategory() { 2214 return this.category != null && !this.category.isEmpty(); 2215 } 2216 2217 /** 2218 * @param value {@link #category} (Category of the identified Substance.). This 2219 * is the underlying object with id, value and extensions. The 2220 * accessor "getCategory" gives direct access to the value 2221 */ 2222 public AllergyIntolerance setCategoryElement(Enumeration<AllergyIntoleranceCategory> value) { 2223 this.category = value; 2224 return this; 2225 } 2226 2227 /** 2228 * @return Category of the identified Substance. 2229 */ 2230 public AllergyIntoleranceCategory getCategory() { 2231 return this.category == null ? null : this.category.getValue(); 2232 } 2233 2234 /** 2235 * @param value Category of the identified Substance. 2236 */ 2237 public AllergyIntolerance setCategory(AllergyIntoleranceCategory value) { 2238 if (value == null) 2239 this.category = null; 2240 else { 2241 if (this.category == null) 2242 this.category = new Enumeration<AllergyIntoleranceCategory>(new AllergyIntoleranceCategoryEnumFactory()); 2243 this.category.setValue(value); 2244 } 2245 return this; 2246 } 2247 2248 /** 2249 * @return {@link #lastOccurence} (Represents the date and/or time of the last 2250 * known occurrence of a reaction event.). This is the underlying object 2251 * with id, value and extensions. The accessor "getLastOccurence" gives 2252 * direct access to the value 2253 */ 2254 public DateTimeType getLastOccurenceElement() { 2255 if (this.lastOccurence == null) 2256 if (Configuration.errorOnAutoCreate()) 2257 throw new Error("Attempt to auto-create AllergyIntolerance.lastOccurence"); 2258 else if (Configuration.doAutoCreate()) 2259 this.lastOccurence = new DateTimeType(); // bb 2260 return this.lastOccurence; 2261 } 2262 2263 public boolean hasLastOccurenceElement() { 2264 return this.lastOccurence != null && !this.lastOccurence.isEmpty(); 2265 } 2266 2267 public boolean hasLastOccurence() { 2268 return this.lastOccurence != null && !this.lastOccurence.isEmpty(); 2269 } 2270 2271 /** 2272 * @param value {@link #lastOccurence} (Represents the date and/or time of the 2273 * last known occurrence of a reaction event.). This is the 2274 * underlying object with id, value and extensions. The accessor 2275 * "getLastOccurence" gives direct access to the value 2276 */ 2277 public AllergyIntolerance setLastOccurenceElement(DateTimeType value) { 2278 this.lastOccurence = value; 2279 return this; 2280 } 2281 2282 /** 2283 * @return Represents the date and/or time of the last known occurrence of a 2284 * reaction event. 2285 */ 2286 public Date getLastOccurence() { 2287 return this.lastOccurence == null ? null : this.lastOccurence.getValue(); 2288 } 2289 2290 /** 2291 * @param value Represents the date and/or time of the last known occurrence of 2292 * a reaction event. 2293 */ 2294 public AllergyIntolerance setLastOccurence(Date value) { 2295 if (value == null) 2296 this.lastOccurence = null; 2297 else { 2298 if (this.lastOccurence == null) 2299 this.lastOccurence = new DateTimeType(); 2300 this.lastOccurence.setValue(value); 2301 } 2302 return this; 2303 } 2304 2305 /** 2306 * @return {@link #note} (Additional narrative about the propensity for the 2307 * Adverse Reaction, not captured in other fields.) 2308 */ 2309 public Annotation getNote() { 2310 if (this.note == null) 2311 if (Configuration.errorOnAutoCreate()) 2312 throw new Error("Attempt to auto-create AllergyIntolerance.note"); 2313 else if (Configuration.doAutoCreate()) 2314 this.note = new Annotation(); // cc 2315 return this.note; 2316 } 2317 2318 public boolean hasNote() { 2319 return this.note != null && !this.note.isEmpty(); 2320 } 2321 2322 /** 2323 * @param value {@link #note} (Additional narrative about the propensity for the 2324 * Adverse Reaction, not captured in other fields.) 2325 */ 2326 public AllergyIntolerance setNote(Annotation value) { 2327 this.note = value; 2328 return this; 2329 } 2330 2331 /** 2332 * @return {@link #reaction} (Details about each adverse reaction event linked 2333 * to exposure to the identified Substance.) 2334 */ 2335 public List<AllergyIntoleranceReactionComponent> getReaction() { 2336 if (this.reaction == null) 2337 this.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2338 return this.reaction; 2339 } 2340 2341 public boolean hasReaction() { 2342 if (this.reaction == null) 2343 return false; 2344 for (AllergyIntoleranceReactionComponent item : this.reaction) 2345 if (!item.isEmpty()) 2346 return true; 2347 return false; 2348 } 2349 2350 /** 2351 * @return {@link #reaction} (Details about each adverse reaction event linked 2352 * to exposure to the identified Substance.) 2353 */ 2354 // syntactic sugar 2355 public AllergyIntoleranceReactionComponent addReaction() { // 3 2356 AllergyIntoleranceReactionComponent t = new AllergyIntoleranceReactionComponent(); 2357 if (this.reaction == null) 2358 this.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2359 this.reaction.add(t); 2360 return t; 2361 } 2362 2363 // syntactic sugar 2364 public AllergyIntolerance addReaction(AllergyIntoleranceReactionComponent t) { // 3 2365 if (t == null) 2366 return this; 2367 if (this.reaction == null) 2368 this.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2369 this.reaction.add(t); 2370 return this; 2371 } 2372 2373 protected void listChildren(List<Property> childrenList) { 2374 super.listChildren(childrenList); 2375 childrenList.add(new Property("identifier", "Identifier", 2376 "This records identifiers associated with this allergy/intolerance concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 2377 0, java.lang.Integer.MAX_VALUE, identifier)); 2378 childrenList.add( 2379 new Property("onset", "dateTime", "Record of the date and/or time of the onset of the Allergy or Intolerance.", 2380 0, java.lang.Integer.MAX_VALUE, onset)); 2381 childrenList.add(new Property("recordedDate", "dateTime", "Date when the sensitivity was recorded.", 0, 2382 java.lang.Integer.MAX_VALUE, recordedDate)); 2383 childrenList.add(new Property("recorder", "Reference(Practitioner|Patient)", 2384 "Individual who recorded the record and takes responsibility for its conten.", 0, java.lang.Integer.MAX_VALUE, 2385 recorder)); 2386 childrenList.add(new Property("patient", "Reference(Patient)", "The patient who has the allergy or intolerance.", 0, 2387 java.lang.Integer.MAX_VALUE, patient)); 2388 childrenList.add(new Property("reporter", "Reference(Patient|RelatedPerson|Practitioner)", 2389 "The source of the information about the allergy that is recorded.", 0, java.lang.Integer.MAX_VALUE, reporter)); 2390 childrenList.add(new Property("substance", "CodeableConcept", 2391 "Identification of a substance, or a class of substances, that is considered to be responsible for the adverse reaction risk.", 2392 0, java.lang.Integer.MAX_VALUE, substance)); 2393 childrenList.add(new Property("status", "code", 2394 "Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified Substance.", 2395 0, java.lang.Integer.MAX_VALUE, status)); 2396 childrenList.add(new Property("criticality", "code", 2397 "Estimate of the potential clinical harm, or seriousness, of the reaction to the identified Substance.", 0, 2398 java.lang.Integer.MAX_VALUE, criticality)); 2399 childrenList.add( 2400 new Property("type", "code", "Identification of the underlying physiological mechanism for the reaction risk.", 2401 0, java.lang.Integer.MAX_VALUE, type)); 2402 childrenList.add(new Property("category", "code", "Category of the identified Substance.", 0, 2403 java.lang.Integer.MAX_VALUE, category)); 2404 childrenList.add(new Property("lastOccurence", "dateTime", 2405 "Represents the date and/or time of the last known occurrence of a reaction event.", 0, 2406 java.lang.Integer.MAX_VALUE, lastOccurence)); 2407 childrenList.add(new Property("note", "Annotation", 2408 "Additional narrative about the propensity for the Adverse Reaction, not captured in other fields.", 0, 2409 java.lang.Integer.MAX_VALUE, note)); 2410 childrenList.add(new Property("reaction", "", 2411 "Details about each adverse reaction event linked to exposure to the identified Substance.", 0, 2412 java.lang.Integer.MAX_VALUE, reaction)); 2413 } 2414 2415 @Override 2416 public void setProperty(String name, Base value) throws FHIRException { 2417 if (name.equals("identifier")) 2418 this.getIdentifier().add(castToIdentifier(value)); 2419 else if (name.equals("onset")) 2420 this.onset = castToDateTime(value); // DateTimeType 2421 else if (name.equals("recordedDate")) 2422 this.recordedDate = castToDateTime(value); // DateTimeType 2423 else if (name.equals("recorder")) 2424 this.recorder = castToReference(value); // Reference 2425 else if (name.equals("patient")) 2426 this.patient = castToReference(value); // Reference 2427 else if (name.equals("reporter")) 2428 this.reporter = castToReference(value); // Reference 2429 else if (name.equals("substance")) 2430 this.substance = castToCodeableConcept(value); // CodeableConcept 2431 else if (name.equals("status")) 2432 this.status = new AllergyIntoleranceStatusEnumFactory().fromType(value); // Enumeration<AllergyIntoleranceStatus> 2433 else if (name.equals("criticality")) 2434 this.criticality = new AllergyIntoleranceCriticalityEnumFactory().fromType(value); // Enumeration<AllergyIntoleranceCriticality> 2435 else if (name.equals("type")) 2436 this.type = new AllergyIntoleranceTypeEnumFactory().fromType(value); // Enumeration<AllergyIntoleranceType> 2437 else if (name.equals("category")) 2438 this.category = new AllergyIntoleranceCategoryEnumFactory().fromType(value); // Enumeration<AllergyIntoleranceCategory> 2439 else if (name.equals("lastOccurence")) 2440 this.lastOccurence = castToDateTime(value); // DateTimeType 2441 else if (name.equals("note")) 2442 this.note = castToAnnotation(value); // Annotation 2443 else if (name.equals("reaction")) 2444 this.getReaction().add((AllergyIntoleranceReactionComponent) value); 2445 else 2446 super.setProperty(name, value); 2447 } 2448 2449 @Override 2450 public Base addChild(String name) throws FHIRException { 2451 if (name.equals("identifier")) { 2452 return addIdentifier(); 2453 } else if (name.equals("onset")) { 2454 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.onset"); 2455 } else if (name.equals("recordedDate")) { 2456 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.recordedDate"); 2457 } else if (name.equals("recorder")) { 2458 this.recorder = new Reference(); 2459 return this.recorder; 2460 } else if (name.equals("patient")) { 2461 this.patient = new Reference(); 2462 return this.patient; 2463 } else if (name.equals("reporter")) { 2464 this.reporter = new Reference(); 2465 return this.reporter; 2466 } else if (name.equals("substance")) { 2467 this.substance = new CodeableConcept(); 2468 return this.substance; 2469 } else if (name.equals("status")) { 2470 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.status"); 2471 } else if (name.equals("criticality")) { 2472 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.criticality"); 2473 } else if (name.equals("type")) { 2474 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.type"); 2475 } else if (name.equals("category")) { 2476 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.category"); 2477 } else if (name.equals("lastOccurence")) { 2478 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.lastOccurence"); 2479 } else if (name.equals("note")) { 2480 this.note = new Annotation(); 2481 return this.note; 2482 } else if (name.equals("reaction")) { 2483 return addReaction(); 2484 } else 2485 return super.addChild(name); 2486 } 2487 2488 public String fhirType() { 2489 return "AllergyIntolerance"; 2490 2491 } 2492 2493 public AllergyIntolerance copy() { 2494 AllergyIntolerance dst = new AllergyIntolerance(); 2495 copyValues(dst); 2496 if (identifier != null) { 2497 dst.identifier = new ArrayList<Identifier>(); 2498 for (Identifier i : identifier) 2499 dst.identifier.add(i.copy()); 2500 } 2501 ; 2502 dst.onset = onset == null ? null : onset.copy(); 2503 dst.recordedDate = recordedDate == null ? null : recordedDate.copy(); 2504 dst.recorder = recorder == null ? null : recorder.copy(); 2505 dst.patient = patient == null ? null : patient.copy(); 2506 dst.reporter = reporter == null ? null : reporter.copy(); 2507 dst.substance = substance == null ? null : substance.copy(); 2508 dst.status = status == null ? null : status.copy(); 2509 dst.criticality = criticality == null ? null : criticality.copy(); 2510 dst.type = type == null ? null : type.copy(); 2511 dst.category = category == null ? null : category.copy(); 2512 dst.lastOccurence = lastOccurence == null ? null : lastOccurence.copy(); 2513 dst.note = note == null ? null : note.copy(); 2514 if (reaction != null) { 2515 dst.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2516 for (AllergyIntoleranceReactionComponent i : reaction) 2517 dst.reaction.add(i.copy()); 2518 } 2519 ; 2520 return dst; 2521 } 2522 2523 protected AllergyIntolerance typedCopy() { 2524 return copy(); 2525 } 2526 2527 @Override 2528 public boolean equalsDeep(Base other) { 2529 if (!super.equalsDeep(other)) 2530 return false; 2531 if (!(other instanceof AllergyIntolerance)) 2532 return false; 2533 AllergyIntolerance o = (AllergyIntolerance) other; 2534 return compareDeep(identifier, o.identifier, true) && compareDeep(onset, o.onset, true) 2535 && compareDeep(recordedDate, o.recordedDate, true) && compareDeep(recorder, o.recorder, true) 2536 && compareDeep(patient, o.patient, true) && compareDeep(reporter, o.reporter, true) 2537 && compareDeep(substance, o.substance, true) && compareDeep(status, o.status, true) 2538 && compareDeep(criticality, o.criticality, true) && compareDeep(type, o.type, true) 2539 && compareDeep(category, o.category, true) && compareDeep(lastOccurence, o.lastOccurence, true) 2540 && compareDeep(note, o.note, true) && compareDeep(reaction, o.reaction, true); 2541 } 2542 2543 @Override 2544 public boolean equalsShallow(Base other) { 2545 if (!super.equalsShallow(other)) 2546 return false; 2547 if (!(other instanceof AllergyIntolerance)) 2548 return false; 2549 AllergyIntolerance o = (AllergyIntolerance) other; 2550 return compareValues(onset, o.onset, true) && compareValues(recordedDate, o.recordedDate, true) 2551 && compareValues(status, o.status, true) && compareValues(criticality, o.criticality, true) 2552 && compareValues(type, o.type, true) && compareValues(category, o.category, true) 2553 && compareValues(lastOccurence, o.lastOccurence, true); 2554 } 2555 2556 public boolean isEmpty() { 2557 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (onset == null || onset.isEmpty()) 2558 && (recordedDate == null || recordedDate.isEmpty()) && (recorder == null || recorder.isEmpty()) 2559 && (patient == null || patient.isEmpty()) && (reporter == null || reporter.isEmpty()) 2560 && (substance == null || substance.isEmpty()) && (status == null || status.isEmpty()) 2561 && (criticality == null || criticality.isEmpty()) && (type == null || type.isEmpty()) 2562 && (category == null || category.isEmpty()) && (lastOccurence == null || lastOccurence.isEmpty()) 2563 && (note == null || note.isEmpty()) && (reaction == null || reaction.isEmpty()); 2564 } 2565 2566 @Override 2567 public ResourceType getResourceType() { 2568 return ResourceType.AllergyIntolerance; 2569 } 2570 2571 @SearchParamDefinition(name = "severity", path = "AllergyIntolerance.reaction.severity", description = "mild | moderate | severe (of event as a whole)", type = "token") 2572 public static final String SP_SEVERITY = "severity"; 2573 @SearchParamDefinition(name = "date", path = "AllergyIntolerance.recordedDate", description = "When recorded", type = "date") 2574 public static final String SP_DATE = "date"; 2575 @SearchParamDefinition(name = "identifier", path = "AllergyIntolerance.identifier", description = "External ids for this item", type = "token") 2576 public static final String SP_IDENTIFIER = "identifier"; 2577 @SearchParamDefinition(name = "manifestation", path = "AllergyIntolerance.reaction.manifestation", description = "Clinical symptoms/signs associated with the Event", type = "token") 2578 public static final String SP_MANIFESTATION = "manifestation"; 2579 @SearchParamDefinition(name = "recorder", path = "AllergyIntolerance.recorder", description = "Who recorded the sensitivity", type = "reference") 2580 public static final String SP_RECORDER = "recorder"; 2581 @SearchParamDefinition(name = "substance", path = "AllergyIntolerance.substance | AllergyIntolerance.reaction.substance", description = "Substance, (or class) considered to be responsible for risk", type = "token") 2582 public static final String SP_SUBSTANCE = "substance"; 2583 @SearchParamDefinition(name = "criticality", path = "AllergyIntolerance.criticality", description = "CRITL | CRITH | CRITU", type = "token") 2584 public static final String SP_CRITICALITY = "criticality"; 2585 @SearchParamDefinition(name = "reporter", path = "AllergyIntolerance.reporter", description = "Source of the information about the allergy", type = "reference") 2586 public static final String SP_REPORTER = "reporter"; 2587 @SearchParamDefinition(name = "type", path = "AllergyIntolerance.type", description = "allergy | intolerance - Underlying mechanism (if known)", type = "token") 2588 public static final String SP_TYPE = "type"; 2589 @SearchParamDefinition(name = "onset", path = "AllergyIntolerance.reaction.onset", description = "Date(/time) when manifestations showed", type = "date") 2590 public static final String SP_ONSET = "onset"; 2591 @SearchParamDefinition(name = "route", path = "AllergyIntolerance.reaction.exposureRoute", description = "How the subject was exposed to the substance", type = "token") 2592 public static final String SP_ROUTE = "route"; 2593 @SearchParamDefinition(name = "patient", path = "AllergyIntolerance.patient", description = "Who the sensitivity is for", type = "reference") 2594 public static final String SP_PATIENT = "patient"; 2595 @SearchParamDefinition(name = "category", path = "AllergyIntolerance.category", description = "food | medication | environment | other - Category of Substance", type = "token") 2596 public static final String SP_CATEGORY = "category"; 2597 @SearchParamDefinition(name = "last-date", path = "AllergyIntolerance.lastOccurence", description = "Date(/time) of last known occurrence of a reaction", type = "date") 2598 public static final String SP_LASTDATE = "last-date"; 2599 @SearchParamDefinition(name = "status", path = "AllergyIntolerance.status", description = "active | unconfirmed | confirmed | inactive | resolved | refuted | entered-in-error", type = "token") 2600 public static final String SP_STATUS = "status"; 2601 2602}