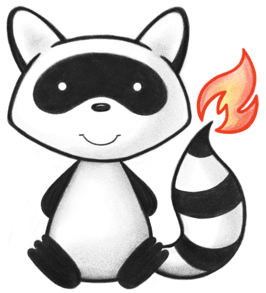
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * Risk of harmful or undesirable, physiological response which is unique to an 048 * individual and associated with exposure to a substance. 049 */ 050@ResourceDef(name = "AllergyIntolerance", profile = "http://hl7.org/fhir/Profile/AllergyIntolerance") 051public class AllergyIntolerance extends DomainResource { 052 053 public enum AllergyIntoleranceStatus { 054 /** 055 * An active record of a reaction to the identified Substance. 056 */ 057 ACTIVE, 058 /** 059 * A low level of certainty about the propensity for a reaction to the 060 * identified Substance. 061 */ 062 UNCONFIRMED, 063 /** 064 * A high level of certainty about the propensity for a reaction to the 065 * identified Substance, which may include clinical evidence by testing or 066 * rechallenge. 067 */ 068 CONFIRMED, 069 /** 070 * An inactive record of a reaction to the identified Substance. 071 */ 072 INACTIVE, 073 /** 074 * A reaction to the identified Substance has been clinically reassessed by 075 * testing or rechallenge and considered to be resolved. 076 */ 077 RESOLVED, 078 /** 079 * A propensity for a reaction to the identified Substance has been disproven 080 * with a high level of clinical certainty, which may include testing or 081 * rechallenge, and is refuted. 082 */ 083 REFUTED, 084 /** 085 * The statement was entered in error and is not valid. 086 */ 087 ENTEREDINERROR, 088 /** 089 * added to help the parsers 090 */ 091 NULL; 092 093 public static AllergyIntoleranceStatus fromCode(String codeString) throws FHIRException { 094 if (codeString == null || "".equals(codeString)) 095 return null; 096 if ("active".equals(codeString)) 097 return ACTIVE; 098 if ("unconfirmed".equals(codeString)) 099 return UNCONFIRMED; 100 if ("confirmed".equals(codeString)) 101 return CONFIRMED; 102 if ("inactive".equals(codeString)) 103 return INACTIVE; 104 if ("resolved".equals(codeString)) 105 return RESOLVED; 106 if ("refuted".equals(codeString)) 107 return REFUTED; 108 if ("entered-in-error".equals(codeString)) 109 return ENTEREDINERROR; 110 throw new FHIRException("Unknown AllergyIntoleranceStatus code '" + codeString + "'"); 111 } 112 113 public String toCode() { 114 switch (this) { 115 case ACTIVE: 116 return "active"; 117 case UNCONFIRMED: 118 return "unconfirmed"; 119 case CONFIRMED: 120 return "confirmed"; 121 case INACTIVE: 122 return "inactive"; 123 case RESOLVED: 124 return "resolved"; 125 case REFUTED: 126 return "refuted"; 127 case ENTEREDINERROR: 128 return "entered-in-error"; 129 case NULL: 130 return null; 131 default: 132 return "?"; 133 } 134 } 135 136 public String getSystem() { 137 switch (this) { 138 case ACTIVE: 139 return "http://hl7.org/fhir/allergy-intolerance-status"; 140 case UNCONFIRMED: 141 return "http://hl7.org/fhir/allergy-intolerance-status"; 142 case CONFIRMED: 143 return "http://hl7.org/fhir/allergy-intolerance-status"; 144 case INACTIVE: 145 return "http://hl7.org/fhir/allergy-intolerance-status"; 146 case RESOLVED: 147 return "http://hl7.org/fhir/allergy-intolerance-status"; 148 case REFUTED: 149 return "http://hl7.org/fhir/allergy-intolerance-status"; 150 case ENTEREDINERROR: 151 return "http://hl7.org/fhir/allergy-intolerance-status"; 152 case NULL: 153 return null; 154 default: 155 return "?"; 156 } 157 } 158 159 public String getDefinition() { 160 switch (this) { 161 case ACTIVE: 162 return "An active record of a reaction to the identified Substance."; 163 case UNCONFIRMED: 164 return "A low level of certainty about the propensity for a reaction to the identified Substance."; 165 case CONFIRMED: 166 return "A high level of certainty about the propensity for a reaction to the identified Substance, which may include clinical evidence by testing or rechallenge."; 167 case INACTIVE: 168 return "An inactive record of a reaction to the identified Substance."; 169 case RESOLVED: 170 return "A reaction to the identified Substance has been clinically reassessed by testing or rechallenge and considered to be resolved."; 171 case REFUTED: 172 return "A propensity for a reaction to the identified Substance has been disproven with a high level of clinical certainty, which may include testing or rechallenge, and is refuted."; 173 case ENTEREDINERROR: 174 return "The statement was entered in error and is not valid."; 175 case NULL: 176 return null; 177 default: 178 return "?"; 179 } 180 } 181 182 public String getDisplay() { 183 switch (this) { 184 case ACTIVE: 185 return "Active"; 186 case UNCONFIRMED: 187 return "Unconfirmed"; 188 case CONFIRMED: 189 return "Confirmed"; 190 case INACTIVE: 191 return "Inactive"; 192 case RESOLVED: 193 return "Resolved"; 194 case REFUTED: 195 return "Refuted"; 196 case ENTEREDINERROR: 197 return "Entered In Error"; 198 case NULL: 199 return null; 200 default: 201 return "?"; 202 } 203 } 204 } 205 206 public static class AllergyIntoleranceStatusEnumFactory implements EnumFactory<AllergyIntoleranceStatus> { 207 public AllergyIntoleranceStatus fromCode(String codeString) throws IllegalArgumentException { 208 if (codeString == null || "".equals(codeString)) 209 if (codeString == null || "".equals(codeString)) 210 return null; 211 if ("active".equals(codeString)) 212 return AllergyIntoleranceStatus.ACTIVE; 213 if ("unconfirmed".equals(codeString)) 214 return AllergyIntoleranceStatus.UNCONFIRMED; 215 if ("confirmed".equals(codeString)) 216 return AllergyIntoleranceStatus.CONFIRMED; 217 if ("inactive".equals(codeString)) 218 return AllergyIntoleranceStatus.INACTIVE; 219 if ("resolved".equals(codeString)) 220 return AllergyIntoleranceStatus.RESOLVED; 221 if ("refuted".equals(codeString)) 222 return AllergyIntoleranceStatus.REFUTED; 223 if ("entered-in-error".equals(codeString)) 224 return AllergyIntoleranceStatus.ENTEREDINERROR; 225 throw new IllegalArgumentException("Unknown AllergyIntoleranceStatus code '" + codeString + "'"); 226 } 227 228 public Enumeration<AllergyIntoleranceStatus> fromType(Base code) throws FHIRException { 229 if (code == null || code.isEmpty()) 230 return null; 231 String codeString = ((PrimitiveType) code).asStringValue(); 232 if (codeString == null || "".equals(codeString)) 233 return null; 234 if ("active".equals(codeString)) 235 return new Enumeration<AllergyIntoleranceStatus>(this, AllergyIntoleranceStatus.ACTIVE); 236 if ("unconfirmed".equals(codeString)) 237 return new Enumeration<AllergyIntoleranceStatus>(this, AllergyIntoleranceStatus.UNCONFIRMED); 238 if ("confirmed".equals(codeString)) 239 return new Enumeration<AllergyIntoleranceStatus>(this, AllergyIntoleranceStatus.CONFIRMED); 240 if ("inactive".equals(codeString)) 241 return new Enumeration<AllergyIntoleranceStatus>(this, AllergyIntoleranceStatus.INACTIVE); 242 if ("resolved".equals(codeString)) 243 return new Enumeration<AllergyIntoleranceStatus>(this, AllergyIntoleranceStatus.RESOLVED); 244 if ("refuted".equals(codeString)) 245 return new Enumeration<AllergyIntoleranceStatus>(this, AllergyIntoleranceStatus.REFUTED); 246 if ("entered-in-error".equals(codeString)) 247 return new Enumeration<AllergyIntoleranceStatus>(this, AllergyIntoleranceStatus.ENTEREDINERROR); 248 throw new FHIRException("Unknown AllergyIntoleranceStatus code '" + codeString + "'"); 249 } 250 251 public String toCode(AllergyIntoleranceStatus code) { 252 if (code == AllergyIntoleranceStatus.ACTIVE) 253 return "active"; 254 if (code == AllergyIntoleranceStatus.UNCONFIRMED) 255 return "unconfirmed"; 256 if (code == AllergyIntoleranceStatus.CONFIRMED) 257 return "confirmed"; 258 if (code == AllergyIntoleranceStatus.INACTIVE) 259 return "inactive"; 260 if (code == AllergyIntoleranceStatus.RESOLVED) 261 return "resolved"; 262 if (code == AllergyIntoleranceStatus.REFUTED) 263 return "refuted"; 264 if (code == AllergyIntoleranceStatus.ENTEREDINERROR) 265 return "entered-in-error"; 266 return "?"; 267 } 268 } 269 270 public enum AllergyIntoleranceCriticality { 271 /** 272 * The potential clinical impact of a future reaction is estimated as low risk: 273 * exposure to substance is unlikely to result in a life threatening or organ 274 * system threatening outcome. Future exposure to the Substance is considered a 275 * relative contra-indication. 276 */ 277 CRITL, 278 /** 279 * The potential clinical impact of a future reaction is estimated as high risk: 280 * exposure to substance may result in a life threatening or organ system 281 * threatening outcome. Future exposure to the Substance may be considered an 282 * absolute contra-indication. 283 */ 284 CRITH, 285 /** 286 * Unable to assess the potential clinical impact with the information 287 * available. 288 */ 289 CRITU, 290 /** 291 * added to help the parsers 292 */ 293 NULL; 294 295 public static AllergyIntoleranceCriticality fromCode(String codeString) throws FHIRException { 296 if (codeString == null || "".equals(codeString)) 297 return null; 298 if ("CRITL".equals(codeString)) 299 return CRITL; 300 if ("CRITH".equals(codeString)) 301 return CRITH; 302 if ("CRITU".equals(codeString)) 303 return CRITU; 304 throw new FHIRException("Unknown AllergyIntoleranceCriticality code '" + codeString + "'"); 305 } 306 307 public String toCode() { 308 switch (this) { 309 case CRITL: 310 return "CRITL"; 311 case CRITH: 312 return "CRITH"; 313 case CRITU: 314 return "CRITU"; 315 case NULL: 316 return null; 317 default: 318 return "?"; 319 } 320 } 321 322 public String getSystem() { 323 switch (this) { 324 case CRITL: 325 return "http://hl7.org/fhir/allergy-intolerance-criticality"; 326 case CRITH: 327 return "http://hl7.org/fhir/allergy-intolerance-criticality"; 328 case CRITU: 329 return "http://hl7.org/fhir/allergy-intolerance-criticality"; 330 case NULL: 331 return null; 332 default: 333 return "?"; 334 } 335 } 336 337 public String getDefinition() { 338 switch (this) { 339 case CRITL: 340 return "The potential clinical impact of a future reaction is estimated as low risk: exposure to substance is unlikely to result in a life threatening or organ system threatening outcome. Future exposure to the Substance is considered a relative contra-indication."; 341 case CRITH: 342 return "The potential clinical impact of a future reaction is estimated as high risk: exposure to substance may result in a life threatening or organ system threatening outcome. Future exposure to the Substance may be considered an absolute contra-indication."; 343 case CRITU: 344 return "Unable to assess the potential clinical impact with the information available."; 345 case NULL: 346 return null; 347 default: 348 return "?"; 349 } 350 } 351 352 public String getDisplay() { 353 switch (this) { 354 case CRITL: 355 return "Low Risk"; 356 case CRITH: 357 return "High Risk"; 358 case CRITU: 359 return "Unable to determine"; 360 case NULL: 361 return null; 362 default: 363 return "?"; 364 } 365 } 366 } 367 368 public static class AllergyIntoleranceCriticalityEnumFactory implements EnumFactory<AllergyIntoleranceCriticality> { 369 public AllergyIntoleranceCriticality fromCode(String codeString) throws IllegalArgumentException { 370 if (codeString == null || "".equals(codeString)) 371 if (codeString == null || "".equals(codeString)) 372 return null; 373 if ("CRITL".equals(codeString)) 374 return AllergyIntoleranceCriticality.CRITL; 375 if ("CRITH".equals(codeString)) 376 return AllergyIntoleranceCriticality.CRITH; 377 if ("CRITU".equals(codeString)) 378 return AllergyIntoleranceCriticality.CRITU; 379 throw new IllegalArgumentException("Unknown AllergyIntoleranceCriticality code '" + codeString + "'"); 380 } 381 382 public Enumeration<AllergyIntoleranceCriticality> fromType(Base code) throws FHIRException { 383 if (code == null || code.isEmpty()) 384 return null; 385 String codeString = ((PrimitiveType) code).asStringValue(); 386 if (codeString == null || "".equals(codeString)) 387 return null; 388 if ("CRITL".equals(codeString)) 389 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.CRITL); 390 if ("CRITH".equals(codeString)) 391 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.CRITH); 392 if ("CRITU".equals(codeString)) 393 return new Enumeration<AllergyIntoleranceCriticality>(this, AllergyIntoleranceCriticality.CRITU); 394 throw new FHIRException("Unknown AllergyIntoleranceCriticality code '" + codeString + "'"); 395 } 396 397 public String toCode(AllergyIntoleranceCriticality code) { 398 if (code == AllergyIntoleranceCriticality.CRITL) 399 return "CRITL"; 400 if (code == AllergyIntoleranceCriticality.CRITH) 401 return "CRITH"; 402 if (code == AllergyIntoleranceCriticality.CRITU) 403 return "CRITU"; 404 return "?"; 405 } 406 } 407 408 public enum AllergyIntoleranceType { 409 /** 410 * A propensity for hypersensitivity reaction(s) to a substance. These reactions 411 * are most typically type I hypersensitivity, plus other "allergy-like" 412 * reactions, including pseudoallergy. 413 */ 414 ALLERGY, 415 /** 416 * A propensity for adverse reactions to a substance that is not judged to be 417 * allergic or "allergy-like". These reactions are typically (but not 418 * necessarily) non-immune. They are to some degree idiosyncratic and/or 419 * individually specific (i.e. are not a reaction that is expected to occur with 420 * most or all patients given similar circumstances). 421 */ 422 INTOLERANCE, 423 /** 424 * added to help the parsers 425 */ 426 NULL; 427 428 public static AllergyIntoleranceType fromCode(String codeString) throws FHIRException { 429 if (codeString == null || "".equals(codeString)) 430 return null; 431 if ("allergy".equals(codeString)) 432 return ALLERGY; 433 if ("intolerance".equals(codeString)) 434 return INTOLERANCE; 435 throw new FHIRException("Unknown AllergyIntoleranceType code '" + codeString + "'"); 436 } 437 438 public String toCode() { 439 switch (this) { 440 case ALLERGY: 441 return "allergy"; 442 case INTOLERANCE: 443 return "intolerance"; 444 case NULL: 445 return null; 446 default: 447 return "?"; 448 } 449 } 450 451 public String getSystem() { 452 switch (this) { 453 case ALLERGY: 454 return "http://hl7.org/fhir/allergy-intolerance-type"; 455 case INTOLERANCE: 456 return "http://hl7.org/fhir/allergy-intolerance-type"; 457 case NULL: 458 return null; 459 default: 460 return "?"; 461 } 462 } 463 464 public String getDefinition() { 465 switch (this) { 466 case ALLERGY: 467 return "A propensity for hypersensitivity reaction(s) to a substance. These reactions are most typically type I hypersensitivity, plus other \"allergy-like\" reactions, including pseudoallergy."; 468 case INTOLERANCE: 469 return "A propensity for adverse reactions to a substance that is not judged to be allergic or \"allergy-like\". These reactions are typically (but not necessarily) non-immune. They are to some degree idiosyncratic and/or individually specific (i.e. are not a reaction that is expected to occur with most or all patients given similar circumstances)."; 470 case NULL: 471 return null; 472 default: 473 return "?"; 474 } 475 } 476 477 public String getDisplay() { 478 switch (this) { 479 case ALLERGY: 480 return "Allergy"; 481 case INTOLERANCE: 482 return "Intolerance"; 483 case NULL: 484 return null; 485 default: 486 return "?"; 487 } 488 } 489 } 490 491 public static class AllergyIntoleranceTypeEnumFactory implements EnumFactory<AllergyIntoleranceType> { 492 public AllergyIntoleranceType fromCode(String codeString) throws IllegalArgumentException { 493 if (codeString == null || "".equals(codeString)) 494 if (codeString == null || "".equals(codeString)) 495 return null; 496 if ("allergy".equals(codeString)) 497 return AllergyIntoleranceType.ALLERGY; 498 if ("intolerance".equals(codeString)) 499 return AllergyIntoleranceType.INTOLERANCE; 500 throw new IllegalArgumentException("Unknown AllergyIntoleranceType code '" + codeString + "'"); 501 } 502 503 public Enumeration<AllergyIntoleranceType> fromType(Base code) throws FHIRException { 504 if (code == null || code.isEmpty()) 505 return null; 506 String codeString = ((PrimitiveType) code).asStringValue(); 507 if (codeString == null || "".equals(codeString)) 508 return null; 509 if ("allergy".equals(codeString)) 510 return new Enumeration<AllergyIntoleranceType>(this, AllergyIntoleranceType.ALLERGY); 511 if ("intolerance".equals(codeString)) 512 return new Enumeration<AllergyIntoleranceType>(this, AllergyIntoleranceType.INTOLERANCE); 513 throw new FHIRException("Unknown AllergyIntoleranceType code '" + codeString + "'"); 514 } 515 516 public String toCode(AllergyIntoleranceType code) { 517 if (code == AllergyIntoleranceType.ALLERGY) 518 return "allergy"; 519 if (code == AllergyIntoleranceType.INTOLERANCE) 520 return "intolerance"; 521 return "?"; 522 } 523 } 524 525 public enum AllergyIntoleranceCategory { 526 /** 527 * Any substance consumed to provide nutritional support for the body. 528 */ 529 FOOD, 530 /** 531 * Substances administered to achieve a physiological effect. 532 */ 533 MEDICATION, 534 /** 535 * Substances that are encountered in the environment. 536 */ 537 ENVIRONMENT, 538 /** 539 * Other substances that are not covered by any other category. 540 */ 541 OTHER, 542 /** 543 * added to help the parsers 544 */ 545 NULL; 546 547 public static AllergyIntoleranceCategory fromCode(String codeString) throws FHIRException { 548 if (codeString == null || "".equals(codeString)) 549 return null; 550 if ("food".equals(codeString)) 551 return FOOD; 552 if ("medication".equals(codeString)) 553 return MEDICATION; 554 if ("environment".equals(codeString)) 555 return ENVIRONMENT; 556 if ("other".equals(codeString)) 557 return OTHER; 558 throw new FHIRException("Unknown AllergyIntoleranceCategory code '" + codeString + "'"); 559 } 560 561 public String toCode() { 562 switch (this) { 563 case FOOD: 564 return "food"; 565 case MEDICATION: 566 return "medication"; 567 case ENVIRONMENT: 568 return "environment"; 569 case OTHER: 570 return "other"; 571 case NULL: 572 return null; 573 default: 574 return "?"; 575 } 576 } 577 578 public String getSystem() { 579 switch (this) { 580 case FOOD: 581 return "http://hl7.org/fhir/allergy-intolerance-category"; 582 case MEDICATION: 583 return "http://hl7.org/fhir/allergy-intolerance-category"; 584 case ENVIRONMENT: 585 return "http://hl7.org/fhir/allergy-intolerance-category"; 586 case OTHER: 587 return "http://hl7.org/fhir/allergy-intolerance-category"; 588 case NULL: 589 return null; 590 default: 591 return "?"; 592 } 593 } 594 595 public String getDefinition() { 596 switch (this) { 597 case FOOD: 598 return "Any substance consumed to provide nutritional support for the body."; 599 case MEDICATION: 600 return "Substances administered to achieve a physiological effect."; 601 case ENVIRONMENT: 602 return "Substances that are encountered in the environment."; 603 case OTHER: 604 return "Other substances that are not covered by any other category."; 605 case NULL: 606 return null; 607 default: 608 return "?"; 609 } 610 } 611 612 public String getDisplay() { 613 switch (this) { 614 case FOOD: 615 return "Food"; 616 case MEDICATION: 617 return "Medication"; 618 case ENVIRONMENT: 619 return "Environment"; 620 case OTHER: 621 return "Other"; 622 case NULL: 623 return null; 624 default: 625 return "?"; 626 } 627 } 628 } 629 630 public static class AllergyIntoleranceCategoryEnumFactory implements EnumFactory<AllergyIntoleranceCategory> { 631 public AllergyIntoleranceCategory fromCode(String codeString) throws IllegalArgumentException { 632 if (codeString == null || "".equals(codeString)) 633 if (codeString == null || "".equals(codeString)) 634 return null; 635 if ("food".equals(codeString)) 636 return AllergyIntoleranceCategory.FOOD; 637 if ("medication".equals(codeString)) 638 return AllergyIntoleranceCategory.MEDICATION; 639 if ("environment".equals(codeString)) 640 return AllergyIntoleranceCategory.ENVIRONMENT; 641 if ("other".equals(codeString)) 642 return AllergyIntoleranceCategory.OTHER; 643 throw new IllegalArgumentException("Unknown AllergyIntoleranceCategory code '" + codeString + "'"); 644 } 645 646 public Enumeration<AllergyIntoleranceCategory> fromType(Base code) throws FHIRException { 647 if (code == null || code.isEmpty()) 648 return null; 649 String codeString = ((PrimitiveType) code).asStringValue(); 650 if (codeString == null || "".equals(codeString)) 651 return null; 652 if ("food".equals(codeString)) 653 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.FOOD); 654 if ("medication".equals(codeString)) 655 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.MEDICATION); 656 if ("environment".equals(codeString)) 657 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.ENVIRONMENT); 658 if ("other".equals(codeString)) 659 return new Enumeration<AllergyIntoleranceCategory>(this, AllergyIntoleranceCategory.OTHER); 660 throw new FHIRException("Unknown AllergyIntoleranceCategory code '" + codeString + "'"); 661 } 662 663 public String toCode(AllergyIntoleranceCategory code) { 664 if (code == AllergyIntoleranceCategory.FOOD) 665 return "food"; 666 if (code == AllergyIntoleranceCategory.MEDICATION) 667 return "medication"; 668 if (code == AllergyIntoleranceCategory.ENVIRONMENT) 669 return "environment"; 670 if (code == AllergyIntoleranceCategory.OTHER) 671 return "other"; 672 return "?"; 673 } 674 } 675 676 public enum AllergyIntoleranceCertainty { 677 /** 678 * There is a low level of clinical certainty that the reaction was caused by 679 * the identified Substance. 680 */ 681 UNLIKELY, 682 /** 683 * There is a high level of clinical certainty that the reaction was caused by 684 * the identified Substance. 685 */ 686 LIKELY, 687 /** 688 * There is a very high level of clinical certainty that the reaction was due to 689 * the identified Substance, which may include clinical evidence by testing or 690 * rechallenge. 691 */ 692 CONFIRMED, 693 /** 694 * added to help the parsers 695 */ 696 NULL; 697 698 public static AllergyIntoleranceCertainty fromCode(String codeString) throws FHIRException { 699 if (codeString == null || "".equals(codeString)) 700 return null; 701 if ("unlikely".equals(codeString)) 702 return UNLIKELY; 703 if ("likely".equals(codeString)) 704 return LIKELY; 705 if ("confirmed".equals(codeString)) 706 return CONFIRMED; 707 throw new FHIRException("Unknown AllergyIntoleranceCertainty code '" + codeString + "'"); 708 } 709 710 public String toCode() { 711 switch (this) { 712 case UNLIKELY: 713 return "unlikely"; 714 case LIKELY: 715 return "likely"; 716 case CONFIRMED: 717 return "confirmed"; 718 case NULL: 719 return null; 720 default: 721 return "?"; 722 } 723 } 724 725 public String getSystem() { 726 switch (this) { 727 case UNLIKELY: 728 return "http://hl7.org/fhir/reaction-event-certainty"; 729 case LIKELY: 730 return "http://hl7.org/fhir/reaction-event-certainty"; 731 case CONFIRMED: 732 return "http://hl7.org/fhir/reaction-event-certainty"; 733 case NULL: 734 return null; 735 default: 736 return "?"; 737 } 738 } 739 740 public String getDefinition() { 741 switch (this) { 742 case UNLIKELY: 743 return "There is a low level of clinical certainty that the reaction was caused by the identified Substance."; 744 case LIKELY: 745 return "There is a high level of clinical certainty that the reaction was caused by the identified Substance."; 746 case CONFIRMED: 747 return "There is a very high level of clinical certainty that the reaction was due to the identified Substance, which may include clinical evidence by testing or rechallenge."; 748 case NULL: 749 return null; 750 default: 751 return "?"; 752 } 753 } 754 755 public String getDisplay() { 756 switch (this) { 757 case UNLIKELY: 758 return "Unlikely"; 759 case LIKELY: 760 return "Likely"; 761 case CONFIRMED: 762 return "Confirmed"; 763 case NULL: 764 return null; 765 default: 766 return "?"; 767 } 768 } 769 } 770 771 public static class AllergyIntoleranceCertaintyEnumFactory implements EnumFactory<AllergyIntoleranceCertainty> { 772 public AllergyIntoleranceCertainty fromCode(String codeString) throws IllegalArgumentException { 773 if (codeString == null || "".equals(codeString)) 774 if (codeString == null || "".equals(codeString)) 775 return null; 776 if ("unlikely".equals(codeString)) 777 return AllergyIntoleranceCertainty.UNLIKELY; 778 if ("likely".equals(codeString)) 779 return AllergyIntoleranceCertainty.LIKELY; 780 if ("confirmed".equals(codeString)) 781 return AllergyIntoleranceCertainty.CONFIRMED; 782 throw new IllegalArgumentException("Unknown AllergyIntoleranceCertainty code '" + codeString + "'"); 783 } 784 785 public Enumeration<AllergyIntoleranceCertainty> fromType(Base code) throws FHIRException { 786 if (code == null || code.isEmpty()) 787 return null; 788 String codeString = ((PrimitiveType) code).asStringValue(); 789 if (codeString == null || "".equals(codeString)) 790 return null; 791 if ("unlikely".equals(codeString)) 792 return new Enumeration<AllergyIntoleranceCertainty>(this, AllergyIntoleranceCertainty.UNLIKELY); 793 if ("likely".equals(codeString)) 794 return new Enumeration<AllergyIntoleranceCertainty>(this, AllergyIntoleranceCertainty.LIKELY); 795 if ("confirmed".equals(codeString)) 796 return new Enumeration<AllergyIntoleranceCertainty>(this, AllergyIntoleranceCertainty.CONFIRMED); 797 throw new FHIRException("Unknown AllergyIntoleranceCertainty code '" + codeString + "'"); 798 } 799 800 public String toCode(AllergyIntoleranceCertainty code) { 801 if (code == AllergyIntoleranceCertainty.UNLIKELY) 802 return "unlikely"; 803 if (code == AllergyIntoleranceCertainty.LIKELY) 804 return "likely"; 805 if (code == AllergyIntoleranceCertainty.CONFIRMED) 806 return "confirmed"; 807 return "?"; 808 } 809 } 810 811 public enum AllergyIntoleranceSeverity { 812 /** 813 * Causes mild physiological effects. 814 */ 815 MILD, 816 /** 817 * Causes moderate physiological effects. 818 */ 819 MODERATE, 820 /** 821 * Causes severe physiological effects. 822 */ 823 SEVERE, 824 /** 825 * added to help the parsers 826 */ 827 NULL; 828 829 public static AllergyIntoleranceSeverity fromCode(String codeString) throws FHIRException { 830 if (codeString == null || "".equals(codeString)) 831 return null; 832 if ("mild".equals(codeString)) 833 return MILD; 834 if ("moderate".equals(codeString)) 835 return MODERATE; 836 if ("severe".equals(codeString)) 837 return SEVERE; 838 throw new FHIRException("Unknown AllergyIntoleranceSeverity code '" + codeString + "'"); 839 } 840 841 public String toCode() { 842 switch (this) { 843 case MILD: 844 return "mild"; 845 case MODERATE: 846 return "moderate"; 847 case SEVERE: 848 return "severe"; 849 case NULL: 850 return null; 851 default: 852 return "?"; 853 } 854 } 855 856 public String getSystem() { 857 switch (this) { 858 case MILD: 859 return "http://hl7.org/fhir/reaction-event-severity"; 860 case MODERATE: 861 return "http://hl7.org/fhir/reaction-event-severity"; 862 case SEVERE: 863 return "http://hl7.org/fhir/reaction-event-severity"; 864 case NULL: 865 return null; 866 default: 867 return "?"; 868 } 869 } 870 871 public String getDefinition() { 872 switch (this) { 873 case MILD: 874 return "Causes mild physiological effects."; 875 case MODERATE: 876 return "Causes moderate physiological effects."; 877 case SEVERE: 878 return "Causes severe physiological effects."; 879 case NULL: 880 return null; 881 default: 882 return "?"; 883 } 884 } 885 886 public String getDisplay() { 887 switch (this) { 888 case MILD: 889 return "Mild"; 890 case MODERATE: 891 return "Moderate"; 892 case SEVERE: 893 return "Severe"; 894 case NULL: 895 return null; 896 default: 897 return "?"; 898 } 899 } 900 } 901 902 public static class AllergyIntoleranceSeverityEnumFactory implements EnumFactory<AllergyIntoleranceSeverity> { 903 public AllergyIntoleranceSeverity fromCode(String codeString) throws IllegalArgumentException { 904 if (codeString == null || "".equals(codeString)) 905 if (codeString == null || "".equals(codeString)) 906 return null; 907 if ("mild".equals(codeString)) 908 return AllergyIntoleranceSeverity.MILD; 909 if ("moderate".equals(codeString)) 910 return AllergyIntoleranceSeverity.MODERATE; 911 if ("severe".equals(codeString)) 912 return AllergyIntoleranceSeverity.SEVERE; 913 throw new IllegalArgumentException("Unknown AllergyIntoleranceSeverity code '" + codeString + "'"); 914 } 915 916 public Enumeration<AllergyIntoleranceSeverity> fromType(Base code) throws FHIRException { 917 if (code == null || code.isEmpty()) 918 return null; 919 String codeString = ((PrimitiveType) code).asStringValue(); 920 if (codeString == null || "".equals(codeString)) 921 return null; 922 if ("mild".equals(codeString)) 923 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.MILD); 924 if ("moderate".equals(codeString)) 925 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.MODERATE); 926 if ("severe".equals(codeString)) 927 return new Enumeration<AllergyIntoleranceSeverity>(this, AllergyIntoleranceSeverity.SEVERE); 928 throw new FHIRException("Unknown AllergyIntoleranceSeverity code '" + codeString + "'"); 929 } 930 931 public String toCode(AllergyIntoleranceSeverity code) { 932 if (code == AllergyIntoleranceSeverity.MILD) 933 return "mild"; 934 if (code == AllergyIntoleranceSeverity.MODERATE) 935 return "moderate"; 936 if (code == AllergyIntoleranceSeverity.SEVERE) 937 return "severe"; 938 return "?"; 939 } 940 } 941 942 @Block() 943 public static class AllergyIntoleranceReactionComponent extends BackboneElement implements IBaseBackboneElement { 944 /** 945 * Identification of the specific substance considered to be responsible for the 946 * Adverse Reaction event. Note: the substance for a specific reaction may be 947 * different to the substance identified as the cause of the risk, but must be 948 * consistent with it. For instance, it may be a more specific substance (e.g. a 949 * brand medication) or a composite substance that includes the identified 950 * substance. It must be clinically safe to only process the 951 * AllergyIntolerance.substance and ignore the 952 * AllergyIntolerance.event.substance. 953 */ 954 @Child(name = "substance", type = { 955 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 956 @Description(shortDefinition = "Specific substance considered to be responsible for event", formalDefinition = "Identification of the specific substance considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different to the substance identified as the cause of the risk, but must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite substance that includes the identified substance. It must be clinically safe to only process the AllergyIntolerance.substance and ignore the AllergyIntolerance.event.substance.") 957 protected CodeableConcept substance; 958 959 /** 960 * Statement about the degree of clinical certainty that the specific substance 961 * was the cause of the manifestation in this reaction event. 962 */ 963 @Child(name = "certainty", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 964 @Description(shortDefinition = "unlikely | likely | confirmed - clinical certainty about the specific substance", formalDefinition = "Statement about the degree of clinical certainty that the specific substance was the cause of the manifestation in this reaction event.") 965 protected Enumeration<AllergyIntoleranceCertainty> certainty; 966 967 /** 968 * Clinical symptoms and/or signs that are observed or associated with the 969 * adverse reaction event. 970 */ 971 @Child(name = "manifestation", type = { 972 CodeableConcept.class }, order = 3, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 973 @Description(shortDefinition = "Clinical symptoms/signs associated with the Event", formalDefinition = "Clinical symptoms and/or signs that are observed or associated with the adverse reaction event.") 974 protected List<CodeableConcept> manifestation; 975 976 /** 977 * Text description about the reaction as a whole, including details of the 978 * manifestation if required. 979 */ 980 @Child(name = "description", type = { 981 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 982 @Description(shortDefinition = "Description of the event as a whole", formalDefinition = "Text description about the reaction as a whole, including details of the manifestation if required.") 983 protected StringType description; 984 985 /** 986 * Record of the date and/or time of the onset of the Reaction. 987 */ 988 @Child(name = "onset", type = { DateTimeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 989 @Description(shortDefinition = "Date(/time) when manifestations showed", formalDefinition = "Record of the date and/or time of the onset of the Reaction.") 990 protected DateTimeType onset; 991 992 /** 993 * Clinical assessment of the severity of the reaction event as a whole, 994 * potentially considering multiple different manifestations. 995 */ 996 @Child(name = "severity", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 997 @Description(shortDefinition = "mild | moderate | severe (of event as a whole)", formalDefinition = "Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations.") 998 protected Enumeration<AllergyIntoleranceSeverity> severity; 999 1000 /** 1001 * Identification of the route by which the subject was exposed to the 1002 * substance. 1003 */ 1004 @Child(name = "exposureRoute", type = { 1005 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1006 @Description(shortDefinition = "How the subject was exposed to the substance", formalDefinition = "Identification of the route by which the subject was exposed to the substance.") 1007 protected CodeableConcept exposureRoute; 1008 1009 /** 1010 * Additional text about the adverse reaction event not captured in other 1011 * fields. 1012 */ 1013 @Child(name = "note", type = { Annotation.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 1014 @Description(shortDefinition = "Text about event not captured in other fields", formalDefinition = "Additional text about the adverse reaction event not captured in other fields.") 1015 protected Annotation note; 1016 1017 private static final long serialVersionUID = -765664367L; 1018 1019 /* 1020 * Constructor 1021 */ 1022 public AllergyIntoleranceReactionComponent() { 1023 super(); 1024 } 1025 1026 /** 1027 * @return {@link #substance} (Identification of the specific substance 1028 * considered to be responsible for the Adverse Reaction event. Note: 1029 * the substance for a specific reaction may be different to the 1030 * substance identified as the cause of the risk, but must be consistent 1031 * with it. For instance, it may be a more specific substance (e.g. a 1032 * brand medication) or a composite substance that includes the 1033 * identified substance. It must be clinically safe to only process the 1034 * AllergyIntolerance.substance and ignore the 1035 * AllergyIntolerance.event.substance.) 1036 */ 1037 public CodeableConcept getSubstance() { 1038 if (this.substance == null) 1039 if (Configuration.errorOnAutoCreate()) 1040 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.substance"); 1041 else if (Configuration.doAutoCreate()) 1042 this.substance = new CodeableConcept(); // cc 1043 return this.substance; 1044 } 1045 1046 public boolean hasSubstance() { 1047 return this.substance != null && !this.substance.isEmpty(); 1048 } 1049 1050 /** 1051 * @param value {@link #substance} (Identification of the specific substance 1052 * considered to be responsible for the Adverse Reaction event. 1053 * Note: the substance for a specific reaction may be different to 1054 * the substance identified as the cause of the risk, but must be 1055 * consistent with it. For instance, it may be a more specific 1056 * substance (e.g. a brand medication) or a composite substance 1057 * that includes the identified substance. It must be clinically 1058 * safe to only process the AllergyIntolerance.substance and ignore 1059 * the AllergyIntolerance.event.substance.) 1060 */ 1061 public AllergyIntoleranceReactionComponent setSubstance(CodeableConcept value) { 1062 this.substance = value; 1063 return this; 1064 } 1065 1066 /** 1067 * @return {@link #certainty} (Statement about the degree of clinical certainty 1068 * that the specific substance was the cause of the manifestation in 1069 * this reaction event.). This is the underlying object with id, value 1070 * and extensions. The accessor "getCertainty" gives direct access to 1071 * the value 1072 */ 1073 public Enumeration<AllergyIntoleranceCertainty> getCertaintyElement() { 1074 if (this.certainty == null) 1075 if (Configuration.errorOnAutoCreate()) 1076 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.certainty"); 1077 else if (Configuration.doAutoCreate()) 1078 this.certainty = new Enumeration<AllergyIntoleranceCertainty>(new AllergyIntoleranceCertaintyEnumFactory()); // bb 1079 return this.certainty; 1080 } 1081 1082 public boolean hasCertaintyElement() { 1083 return this.certainty != null && !this.certainty.isEmpty(); 1084 } 1085 1086 public boolean hasCertainty() { 1087 return this.certainty != null && !this.certainty.isEmpty(); 1088 } 1089 1090 /** 1091 * @param value {@link #certainty} (Statement about the degree of clinical 1092 * certainty that the specific substance was the cause of the 1093 * manifestation in this reaction event.). This is the underlying 1094 * object with id, value and extensions. The accessor 1095 * "getCertainty" gives direct access to the value 1096 */ 1097 public AllergyIntoleranceReactionComponent setCertaintyElement(Enumeration<AllergyIntoleranceCertainty> value) { 1098 this.certainty = value; 1099 return this; 1100 } 1101 1102 /** 1103 * @return Statement about the degree of clinical certainty that the specific 1104 * substance was the cause of the manifestation in this reaction event. 1105 */ 1106 public AllergyIntoleranceCertainty getCertainty() { 1107 return this.certainty == null ? null : this.certainty.getValue(); 1108 } 1109 1110 /** 1111 * @param value Statement about the degree of clinical certainty that the 1112 * specific substance was the cause of the manifestation in this 1113 * reaction event. 1114 */ 1115 public AllergyIntoleranceReactionComponent setCertainty(AllergyIntoleranceCertainty value) { 1116 if (value == null) 1117 this.certainty = null; 1118 else { 1119 if (this.certainty == null) 1120 this.certainty = new Enumeration<AllergyIntoleranceCertainty>(new AllergyIntoleranceCertaintyEnumFactory()); 1121 this.certainty.setValue(value); 1122 } 1123 return this; 1124 } 1125 1126 /** 1127 * @return {@link #manifestation} (Clinical symptoms and/or signs that are 1128 * observed or associated with the adverse reaction event.) 1129 */ 1130 public List<CodeableConcept> getManifestation() { 1131 if (this.manifestation == null) 1132 this.manifestation = new ArrayList<CodeableConcept>(); 1133 return this.manifestation; 1134 } 1135 1136 public boolean hasManifestation() { 1137 if (this.manifestation == null) 1138 return false; 1139 for (CodeableConcept item : this.manifestation) 1140 if (!item.isEmpty()) 1141 return true; 1142 return false; 1143 } 1144 1145 /** 1146 * @return {@link #manifestation} (Clinical symptoms and/or signs that are 1147 * observed or associated with the adverse reaction event.) 1148 */ 1149 // syntactic sugar 1150 public CodeableConcept addManifestation() { // 3 1151 CodeableConcept t = new CodeableConcept(); 1152 if (this.manifestation == null) 1153 this.manifestation = new ArrayList<CodeableConcept>(); 1154 this.manifestation.add(t); 1155 return t; 1156 } 1157 1158 // syntactic sugar 1159 public AllergyIntoleranceReactionComponent addManifestation(CodeableConcept t) { // 3 1160 if (t == null) 1161 return this; 1162 if (this.manifestation == null) 1163 this.manifestation = new ArrayList<CodeableConcept>(); 1164 this.manifestation.add(t); 1165 return this; 1166 } 1167 1168 /** 1169 * @return {@link #description} (Text description about the reaction as a whole, 1170 * including details of the manifestation if required.). This is the 1171 * underlying object with id, value and extensions. The accessor 1172 * "getDescription" gives direct access to the value 1173 */ 1174 public StringType getDescriptionElement() { 1175 if (this.description == null) 1176 if (Configuration.errorOnAutoCreate()) 1177 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.description"); 1178 else if (Configuration.doAutoCreate()) 1179 this.description = new StringType(); // bb 1180 return this.description; 1181 } 1182 1183 public boolean hasDescriptionElement() { 1184 return this.description != null && !this.description.isEmpty(); 1185 } 1186 1187 public boolean hasDescription() { 1188 return this.description != null && !this.description.isEmpty(); 1189 } 1190 1191 /** 1192 * @param value {@link #description} (Text description about the reaction as a 1193 * whole, including details of the manifestation if required.). 1194 * This is the underlying object with id, value and extensions. The 1195 * accessor "getDescription" gives direct access to the value 1196 */ 1197 public AllergyIntoleranceReactionComponent setDescriptionElement(StringType value) { 1198 this.description = value; 1199 return this; 1200 } 1201 1202 /** 1203 * @return Text description about the reaction as a whole, including details of 1204 * the manifestation if required. 1205 */ 1206 public String getDescription() { 1207 return this.description == null ? null : this.description.getValue(); 1208 } 1209 1210 /** 1211 * @param value Text description about the reaction as a whole, including 1212 * details of the manifestation if required. 1213 */ 1214 public AllergyIntoleranceReactionComponent setDescription(String value) { 1215 if (Utilities.noString(value)) 1216 this.description = null; 1217 else { 1218 if (this.description == null) 1219 this.description = new StringType(); 1220 this.description.setValue(value); 1221 } 1222 return this; 1223 } 1224 1225 /** 1226 * @return {@link #onset} (Record of the date and/or time of the onset of the 1227 * Reaction.). This is the underlying object with id, value and 1228 * extensions. The accessor "getOnset" gives direct access to the value 1229 */ 1230 public DateTimeType getOnsetElement() { 1231 if (this.onset == null) 1232 if (Configuration.errorOnAutoCreate()) 1233 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.onset"); 1234 else if (Configuration.doAutoCreate()) 1235 this.onset = new DateTimeType(); // bb 1236 return this.onset; 1237 } 1238 1239 public boolean hasOnsetElement() { 1240 return this.onset != null && !this.onset.isEmpty(); 1241 } 1242 1243 public boolean hasOnset() { 1244 return this.onset != null && !this.onset.isEmpty(); 1245 } 1246 1247 /** 1248 * @param value {@link #onset} (Record of the date and/or time of the onset of 1249 * the Reaction.). This is the underlying object with id, value and 1250 * extensions. The accessor "getOnset" gives direct access to the 1251 * value 1252 */ 1253 public AllergyIntoleranceReactionComponent setOnsetElement(DateTimeType value) { 1254 this.onset = value; 1255 return this; 1256 } 1257 1258 /** 1259 * @return Record of the date and/or time of the onset of the Reaction. 1260 */ 1261 public Date getOnset() { 1262 return this.onset == null ? null : this.onset.getValue(); 1263 } 1264 1265 /** 1266 * @param value Record of the date and/or time of the onset of the Reaction. 1267 */ 1268 public AllergyIntoleranceReactionComponent setOnset(Date value) { 1269 if (value == null) 1270 this.onset = null; 1271 else { 1272 if (this.onset == null) 1273 this.onset = new DateTimeType(); 1274 this.onset.setValue(value); 1275 } 1276 return this; 1277 } 1278 1279 /** 1280 * @return {@link #severity} (Clinical assessment of the severity of the 1281 * reaction event as a whole, potentially considering multiple different 1282 * manifestations.). This is the underlying object with id, value and 1283 * extensions. The accessor "getSeverity" gives direct access to the 1284 * value 1285 */ 1286 public Enumeration<AllergyIntoleranceSeverity> getSeverityElement() { 1287 if (this.severity == null) 1288 if (Configuration.errorOnAutoCreate()) 1289 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.severity"); 1290 else if (Configuration.doAutoCreate()) 1291 this.severity = new Enumeration<AllergyIntoleranceSeverity>(new AllergyIntoleranceSeverityEnumFactory()); // bb 1292 return this.severity; 1293 } 1294 1295 public boolean hasSeverityElement() { 1296 return this.severity != null && !this.severity.isEmpty(); 1297 } 1298 1299 public boolean hasSeverity() { 1300 return this.severity != null && !this.severity.isEmpty(); 1301 } 1302 1303 /** 1304 * @param value {@link #severity} (Clinical assessment of the severity of the 1305 * reaction event as a whole, potentially considering multiple 1306 * different manifestations.). This is the underlying object with 1307 * id, value and extensions. The accessor "getSeverity" gives 1308 * direct access to the value 1309 */ 1310 public AllergyIntoleranceReactionComponent setSeverityElement(Enumeration<AllergyIntoleranceSeverity> value) { 1311 this.severity = value; 1312 return this; 1313 } 1314 1315 /** 1316 * @return Clinical assessment of the severity of the reaction event as a whole, 1317 * potentially considering multiple different manifestations. 1318 */ 1319 public AllergyIntoleranceSeverity getSeverity() { 1320 return this.severity == null ? null : this.severity.getValue(); 1321 } 1322 1323 /** 1324 * @param value Clinical assessment of the severity of the reaction event as a 1325 * whole, potentially considering multiple different 1326 * manifestations. 1327 */ 1328 public AllergyIntoleranceReactionComponent setSeverity(AllergyIntoleranceSeverity value) { 1329 if (value == null) 1330 this.severity = null; 1331 else { 1332 if (this.severity == null) 1333 this.severity = new Enumeration<AllergyIntoleranceSeverity>(new AllergyIntoleranceSeverityEnumFactory()); 1334 this.severity.setValue(value); 1335 } 1336 return this; 1337 } 1338 1339 /** 1340 * @return {@link #exposureRoute} (Identification of the route by which the 1341 * subject was exposed to the substance.) 1342 */ 1343 public CodeableConcept getExposureRoute() { 1344 if (this.exposureRoute == null) 1345 if (Configuration.errorOnAutoCreate()) 1346 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.exposureRoute"); 1347 else if (Configuration.doAutoCreate()) 1348 this.exposureRoute = new CodeableConcept(); // cc 1349 return this.exposureRoute; 1350 } 1351 1352 public boolean hasExposureRoute() { 1353 return this.exposureRoute != null && !this.exposureRoute.isEmpty(); 1354 } 1355 1356 /** 1357 * @param value {@link #exposureRoute} (Identification of the route by which the 1358 * subject was exposed to the substance.) 1359 */ 1360 public AllergyIntoleranceReactionComponent setExposureRoute(CodeableConcept value) { 1361 this.exposureRoute = value; 1362 return this; 1363 } 1364 1365 /** 1366 * @return {@link #note} (Additional text about the adverse reaction event not 1367 * captured in other fields.) 1368 */ 1369 public Annotation getNote() { 1370 if (this.note == null) 1371 if (Configuration.errorOnAutoCreate()) 1372 throw new Error("Attempt to auto-create AllergyIntoleranceReactionComponent.note"); 1373 else if (Configuration.doAutoCreate()) 1374 this.note = new Annotation(); // cc 1375 return this.note; 1376 } 1377 1378 public boolean hasNote() { 1379 return this.note != null && !this.note.isEmpty(); 1380 } 1381 1382 /** 1383 * @param value {@link #note} (Additional text about the adverse reaction event 1384 * not captured in other fields.) 1385 */ 1386 public AllergyIntoleranceReactionComponent setNote(Annotation value) { 1387 this.note = value; 1388 return this; 1389 } 1390 1391 protected void listChildren(List<Property> childrenList) { 1392 super.listChildren(childrenList); 1393 childrenList.add(new Property("substance", "CodeableConcept", 1394 "Identification of the specific substance considered to be responsible for the Adverse Reaction event. Note: the substance for a specific reaction may be different to the substance identified as the cause of the risk, but must be consistent with it. For instance, it may be a more specific substance (e.g. a brand medication) or a composite substance that includes the identified substance. It must be clinically safe to only process the AllergyIntolerance.substance and ignore the AllergyIntolerance.event.substance.", 1395 0, java.lang.Integer.MAX_VALUE, substance)); 1396 childrenList.add(new Property("certainty", "code", 1397 "Statement about the degree of clinical certainty that the specific substance was the cause of the manifestation in this reaction event.", 1398 0, java.lang.Integer.MAX_VALUE, certainty)); 1399 childrenList.add(new Property("manifestation", "CodeableConcept", 1400 "Clinical symptoms and/or signs that are observed or associated with the adverse reaction event.", 0, 1401 java.lang.Integer.MAX_VALUE, manifestation)); 1402 childrenList.add(new Property("description", "string", 1403 "Text description about the reaction as a whole, including details of the manifestation if required.", 0, 1404 java.lang.Integer.MAX_VALUE, description)); 1405 childrenList.add(new Property("onset", "dateTime", "Record of the date and/or time of the onset of the Reaction.", 1406 0, java.lang.Integer.MAX_VALUE, onset)); 1407 childrenList.add(new Property("severity", "code", 1408 "Clinical assessment of the severity of the reaction event as a whole, potentially considering multiple different manifestations.", 1409 0, java.lang.Integer.MAX_VALUE, severity)); 1410 childrenList.add(new Property("exposureRoute", "CodeableConcept", 1411 "Identification of the route by which the subject was exposed to the substance.", 0, 1412 java.lang.Integer.MAX_VALUE, exposureRoute)); 1413 childrenList.add(new Property("note", "Annotation", 1414 "Additional text about the adverse reaction event not captured in other fields.", 0, 1415 java.lang.Integer.MAX_VALUE, note)); 1416 } 1417 1418 @Override 1419 public void setProperty(String name, Base value) throws FHIRException { 1420 if (name.equals("substance")) 1421 this.substance = castToCodeableConcept(value); // CodeableConcept 1422 else if (name.equals("certainty")) 1423 this.certainty = new AllergyIntoleranceCertaintyEnumFactory().fromType(value); // Enumeration<AllergyIntoleranceCertainty> 1424 else if (name.equals("manifestation")) 1425 this.getManifestation().add(castToCodeableConcept(value)); 1426 else if (name.equals("description")) 1427 this.description = castToString(value); // StringType 1428 else if (name.equals("onset")) 1429 this.onset = castToDateTime(value); // DateTimeType 1430 else if (name.equals("severity")) 1431 this.severity = new AllergyIntoleranceSeverityEnumFactory().fromType(value); // Enumeration<AllergyIntoleranceSeverity> 1432 else if (name.equals("exposureRoute")) 1433 this.exposureRoute = castToCodeableConcept(value); // CodeableConcept 1434 else if (name.equals("note")) 1435 this.note = castToAnnotation(value); // Annotation 1436 else 1437 super.setProperty(name, value); 1438 } 1439 1440 @Override 1441 public Base addChild(String name) throws FHIRException { 1442 if (name.equals("substance")) { 1443 this.substance = new CodeableConcept(); 1444 return this.substance; 1445 } else if (name.equals("certainty")) { 1446 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.certainty"); 1447 } else if (name.equals("manifestation")) { 1448 return addManifestation(); 1449 } else if (name.equals("description")) { 1450 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.description"); 1451 } else if (name.equals("onset")) { 1452 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.onset"); 1453 } else if (name.equals("severity")) { 1454 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.severity"); 1455 } else if (name.equals("exposureRoute")) { 1456 this.exposureRoute = new CodeableConcept(); 1457 return this.exposureRoute; 1458 } else if (name.equals("note")) { 1459 this.note = new Annotation(); 1460 return this.note; 1461 } else 1462 return super.addChild(name); 1463 } 1464 1465 public AllergyIntoleranceReactionComponent copy() { 1466 AllergyIntoleranceReactionComponent dst = new AllergyIntoleranceReactionComponent(); 1467 copyValues(dst); 1468 dst.substance = substance == null ? null : substance.copy(); 1469 dst.certainty = certainty == null ? null : certainty.copy(); 1470 if (manifestation != null) { 1471 dst.manifestation = new ArrayList<CodeableConcept>(); 1472 for (CodeableConcept i : manifestation) 1473 dst.manifestation.add(i.copy()); 1474 } 1475 ; 1476 dst.description = description == null ? null : description.copy(); 1477 dst.onset = onset == null ? null : onset.copy(); 1478 dst.severity = severity == null ? null : severity.copy(); 1479 dst.exposureRoute = exposureRoute == null ? null : exposureRoute.copy(); 1480 dst.note = note == null ? null : note.copy(); 1481 return dst; 1482 } 1483 1484 @Override 1485 public boolean equalsDeep(Base other) { 1486 if (!super.equalsDeep(other)) 1487 return false; 1488 if (!(other instanceof AllergyIntoleranceReactionComponent)) 1489 return false; 1490 AllergyIntoleranceReactionComponent o = (AllergyIntoleranceReactionComponent) other; 1491 return compareDeep(substance, o.substance, true) && compareDeep(certainty, o.certainty, true) 1492 && compareDeep(manifestation, o.manifestation, true) && compareDeep(description, o.description, true) 1493 && compareDeep(onset, o.onset, true) && compareDeep(severity, o.severity, true) 1494 && compareDeep(exposureRoute, o.exposureRoute, true) && compareDeep(note, o.note, true); 1495 } 1496 1497 @Override 1498 public boolean equalsShallow(Base other) { 1499 if (!super.equalsShallow(other)) 1500 return false; 1501 if (!(other instanceof AllergyIntoleranceReactionComponent)) 1502 return false; 1503 AllergyIntoleranceReactionComponent o = (AllergyIntoleranceReactionComponent) other; 1504 return compareValues(certainty, o.certainty, true) && compareValues(description, o.description, true) 1505 && compareValues(onset, o.onset, true) && compareValues(severity, o.severity, true); 1506 } 1507 1508 public boolean isEmpty() { 1509 return super.isEmpty() && (substance == null || substance.isEmpty()) && (certainty == null || certainty.isEmpty()) 1510 && (manifestation == null || manifestation.isEmpty()) && (description == null || description.isEmpty()) 1511 && (onset == null || onset.isEmpty()) && (severity == null || severity.isEmpty()) 1512 && (exposureRoute == null || exposureRoute.isEmpty()) && (note == null || note.isEmpty()); 1513 } 1514 1515 public String fhirType() { 1516 return "AllergyIntolerance.reaction"; 1517 1518 } 1519 1520 } 1521 1522 /** 1523 * This records identifiers associated with this allergy/intolerance concern 1524 * that are defined by business processes and/or used to refer to it when a 1525 * direct URL reference to the resource itself is not appropriate (e.g. in CDA 1526 * documents, or in written / printed documentation). 1527 */ 1528 @Child(name = "identifier", type = { 1529 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1530 @Description(shortDefinition = "External ids for this item", formalDefinition = "This records identifiers associated with this allergy/intolerance concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).") 1531 protected List<Identifier> identifier; 1532 1533 /** 1534 * Record of the date and/or time of the onset of the Allergy or Intolerance. 1535 */ 1536 @Child(name = "onset", type = { DateTimeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1537 @Description(shortDefinition = "Date(/time) when manifestations showed", formalDefinition = "Record of the date and/or time of the onset of the Allergy or Intolerance.") 1538 protected DateTimeType onset; 1539 1540 /** 1541 * Date when the sensitivity was recorded. 1542 */ 1543 @Child(name = "recordedDate", type = { 1544 DateTimeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1545 @Description(shortDefinition = "When recorded", formalDefinition = "Date when the sensitivity was recorded.") 1546 protected DateTimeType recordedDate; 1547 1548 /** 1549 * Individual who recorded the record and takes responsibility for its conten. 1550 */ 1551 @Child(name = "recorder", type = { Practitioner.class, 1552 Patient.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1553 @Description(shortDefinition = "Who recorded the sensitivity", formalDefinition = "Individual who recorded the record and takes responsibility for its conten.") 1554 protected Reference recorder; 1555 1556 /** 1557 * The actual object that is the target of the reference (Individual who 1558 * recorded the record and takes responsibility for its conten.) 1559 */ 1560 protected Resource recorderTarget; 1561 1562 /** 1563 * The patient who has the allergy or intolerance. 1564 */ 1565 @Child(name = "patient", type = { Patient.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 1566 @Description(shortDefinition = "Who the sensitivity is for", formalDefinition = "The patient who has the allergy or intolerance.") 1567 protected Reference patient; 1568 1569 /** 1570 * The actual object that is the target of the reference (The patient who has 1571 * the allergy or intolerance.) 1572 */ 1573 protected Patient patientTarget; 1574 1575 /** 1576 * The source of the information about the allergy that is recorded. 1577 */ 1578 @Child(name = "reporter", type = { Patient.class, RelatedPerson.class, 1579 Practitioner.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1580 @Description(shortDefinition = "Source of the information about the allergy", formalDefinition = "The source of the information about the allergy that is recorded.") 1581 protected Reference reporter; 1582 1583 /** 1584 * The actual object that is the target of the reference (The source of the 1585 * information about the allergy that is recorded.) 1586 */ 1587 protected Resource reporterTarget; 1588 1589 /** 1590 * Identification of a substance, or a class of substances, that is considered 1591 * to be responsible for the adverse reaction risk. 1592 */ 1593 @Child(name = "substance", type = { 1594 CodeableConcept.class }, order = 6, min = 1, max = 1, modifier = false, summary = true) 1595 @Description(shortDefinition = "Substance, (or class) considered to be responsible for risk", formalDefinition = "Identification of a substance, or a class of substances, that is considered to be responsible for the adverse reaction risk.") 1596 protected CodeableConcept substance; 1597 1598 /** 1599 * Assertion about certainty associated with the propensity, or potential risk, 1600 * of a reaction to the identified Substance. 1601 */ 1602 @Child(name = "status", type = { CodeType.class }, order = 7, min = 0, max = 1, modifier = true, summary = true) 1603 @Description(shortDefinition = "active | unconfirmed | confirmed | inactive | resolved | refuted | entered-in-error", formalDefinition = "Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified Substance.") 1604 protected Enumeration<AllergyIntoleranceStatus> status; 1605 1606 /** 1607 * Estimate of the potential clinical harm, or seriousness, of the reaction to 1608 * the identified Substance. 1609 */ 1610 @Child(name = "criticality", type = { CodeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1611 @Description(shortDefinition = "CRITL | CRITH | CRITU", formalDefinition = "Estimate of the potential clinical harm, or seriousness, of the reaction to the identified Substance.") 1612 protected Enumeration<AllergyIntoleranceCriticality> criticality; 1613 1614 /** 1615 * Identification of the underlying physiological mechanism for the reaction 1616 * risk. 1617 */ 1618 @Child(name = "type", type = { CodeType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1619 @Description(shortDefinition = "allergy | intolerance - Underlying mechanism (if known)", formalDefinition = "Identification of the underlying physiological mechanism for the reaction risk.") 1620 protected Enumeration<AllergyIntoleranceType> type; 1621 1622 /** 1623 * Category of the identified Substance. 1624 */ 1625 @Child(name = "category", type = { CodeType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1626 @Description(shortDefinition = "food | medication | environment | other - Category of Substance", formalDefinition = "Category of the identified Substance.") 1627 protected Enumeration<AllergyIntoleranceCategory> category; 1628 1629 /** 1630 * Represents the date and/or time of the last known occurrence of a reaction 1631 * event. 1632 */ 1633 @Child(name = "lastOccurence", type = { 1634 DateTimeType.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 1635 @Description(shortDefinition = "Date(/time) of last known occurrence of a reaction", formalDefinition = "Represents the date and/or time of the last known occurrence of a reaction event.") 1636 protected DateTimeType lastOccurence; 1637 1638 /** 1639 * Additional narrative about the propensity for the Adverse Reaction, not 1640 * captured in other fields. 1641 */ 1642 @Child(name = "note", type = { Annotation.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 1643 @Description(shortDefinition = "Additional text not captured in other fields", formalDefinition = "Additional narrative about the propensity for the Adverse Reaction, not captured in other fields.") 1644 protected Annotation note; 1645 1646 /** 1647 * Details about each adverse reaction event linked to exposure to the 1648 * identified Substance. 1649 */ 1650 @Child(name = "reaction", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1651 @Description(shortDefinition = "Adverse Reaction Events linked to exposure to substance", formalDefinition = "Details about each adverse reaction event linked to exposure to the identified Substance.") 1652 protected List<AllergyIntoleranceReactionComponent> reaction; 1653 1654 private static final long serialVersionUID = -1657522921L; 1655 1656 /* 1657 * Constructor 1658 */ 1659 public AllergyIntolerance() { 1660 super(); 1661 } 1662 1663 /* 1664 * Constructor 1665 */ 1666 public AllergyIntolerance(Reference patient, CodeableConcept substance) { 1667 super(); 1668 this.patient = patient; 1669 this.substance = substance; 1670 } 1671 1672 /** 1673 * @return {@link #identifier} (This records identifiers associated with this 1674 * allergy/intolerance concern that are defined by business processes 1675 * and/or used to refer to it when a direct URL reference to the 1676 * resource itself is not appropriate (e.g. in CDA documents, or in 1677 * written / printed documentation).) 1678 */ 1679 public List<Identifier> getIdentifier() { 1680 if (this.identifier == null) 1681 this.identifier = new ArrayList<Identifier>(); 1682 return this.identifier; 1683 } 1684 1685 public boolean hasIdentifier() { 1686 if (this.identifier == null) 1687 return false; 1688 for (Identifier item : this.identifier) 1689 if (!item.isEmpty()) 1690 return true; 1691 return false; 1692 } 1693 1694 /** 1695 * @return {@link #identifier} (This records identifiers associated with this 1696 * allergy/intolerance concern that are defined by business processes 1697 * and/or used to refer to it when a direct URL reference to the 1698 * resource itself is not appropriate (e.g. in CDA documents, or in 1699 * written / printed documentation).) 1700 */ 1701 // syntactic sugar 1702 public Identifier addIdentifier() { // 3 1703 Identifier t = new Identifier(); 1704 if (this.identifier == null) 1705 this.identifier = new ArrayList<Identifier>(); 1706 this.identifier.add(t); 1707 return t; 1708 } 1709 1710 // syntactic sugar 1711 public AllergyIntolerance addIdentifier(Identifier t) { // 3 1712 if (t == null) 1713 return this; 1714 if (this.identifier == null) 1715 this.identifier = new ArrayList<Identifier>(); 1716 this.identifier.add(t); 1717 return this; 1718 } 1719 1720 /** 1721 * @return {@link #onset} (Record of the date and/or time of the onset of the 1722 * Allergy or Intolerance.). This is the underlying object with id, 1723 * value and extensions. The accessor "getOnset" gives direct access to 1724 * the value 1725 */ 1726 public DateTimeType getOnsetElement() { 1727 if (this.onset == null) 1728 if (Configuration.errorOnAutoCreate()) 1729 throw new Error("Attempt to auto-create AllergyIntolerance.onset"); 1730 else if (Configuration.doAutoCreate()) 1731 this.onset = new DateTimeType(); // bb 1732 return this.onset; 1733 } 1734 1735 public boolean hasOnsetElement() { 1736 return this.onset != null && !this.onset.isEmpty(); 1737 } 1738 1739 public boolean hasOnset() { 1740 return this.onset != null && !this.onset.isEmpty(); 1741 } 1742 1743 /** 1744 * @param value {@link #onset} (Record of the date and/or time of the onset of 1745 * the Allergy or Intolerance.). This is the underlying object with 1746 * id, value and extensions. The accessor "getOnset" gives direct 1747 * access to the value 1748 */ 1749 public AllergyIntolerance setOnsetElement(DateTimeType value) { 1750 this.onset = value; 1751 return this; 1752 } 1753 1754 /** 1755 * @return Record of the date and/or time of the onset of the Allergy or 1756 * Intolerance. 1757 */ 1758 public Date getOnset() { 1759 return this.onset == null ? null : this.onset.getValue(); 1760 } 1761 1762 /** 1763 * @param value Record of the date and/or time of the onset of the Allergy or 1764 * Intolerance. 1765 */ 1766 public AllergyIntolerance setOnset(Date value) { 1767 if (value == null) 1768 this.onset = null; 1769 else { 1770 if (this.onset == null) 1771 this.onset = new DateTimeType(); 1772 this.onset.setValue(value); 1773 } 1774 return this; 1775 } 1776 1777 /** 1778 * @return {@link #recordedDate} (Date when the sensitivity was recorded.). This 1779 * is the underlying object with id, value and extensions. The accessor 1780 * "getRecordedDate" gives direct access to the value 1781 */ 1782 public DateTimeType getRecordedDateElement() { 1783 if (this.recordedDate == null) 1784 if (Configuration.errorOnAutoCreate()) 1785 throw new Error("Attempt to auto-create AllergyIntolerance.recordedDate"); 1786 else if (Configuration.doAutoCreate()) 1787 this.recordedDate = new DateTimeType(); // bb 1788 return this.recordedDate; 1789 } 1790 1791 public boolean hasRecordedDateElement() { 1792 return this.recordedDate != null && !this.recordedDate.isEmpty(); 1793 } 1794 1795 public boolean hasRecordedDate() { 1796 return this.recordedDate != null && !this.recordedDate.isEmpty(); 1797 } 1798 1799 /** 1800 * @param value {@link #recordedDate} (Date when the sensitivity was recorded.). 1801 * This is the underlying object with id, value and extensions. The 1802 * accessor "getRecordedDate" gives direct access to the value 1803 */ 1804 public AllergyIntolerance setRecordedDateElement(DateTimeType value) { 1805 this.recordedDate = value; 1806 return this; 1807 } 1808 1809 /** 1810 * @return Date when the sensitivity was recorded. 1811 */ 1812 public Date getRecordedDate() { 1813 return this.recordedDate == null ? null : this.recordedDate.getValue(); 1814 } 1815 1816 /** 1817 * @param value Date when the sensitivity was recorded. 1818 */ 1819 public AllergyIntolerance setRecordedDate(Date value) { 1820 if (value == null) 1821 this.recordedDate = null; 1822 else { 1823 if (this.recordedDate == null) 1824 this.recordedDate = new DateTimeType(); 1825 this.recordedDate.setValue(value); 1826 } 1827 return this; 1828 } 1829 1830 /** 1831 * @return {@link #recorder} (Individual who recorded the record and takes 1832 * responsibility for its conten.) 1833 */ 1834 public Reference getRecorder() { 1835 if (this.recorder == null) 1836 if (Configuration.errorOnAutoCreate()) 1837 throw new Error("Attempt to auto-create AllergyIntolerance.recorder"); 1838 else if (Configuration.doAutoCreate()) 1839 this.recorder = new Reference(); // cc 1840 return this.recorder; 1841 } 1842 1843 public boolean hasRecorder() { 1844 return this.recorder != null && !this.recorder.isEmpty(); 1845 } 1846 1847 /** 1848 * @param value {@link #recorder} (Individual who recorded the record and takes 1849 * responsibility for its conten.) 1850 */ 1851 public AllergyIntolerance setRecorder(Reference value) { 1852 this.recorder = value; 1853 return this; 1854 } 1855 1856 /** 1857 * @return {@link #recorder} The actual object that is the target of the 1858 * reference. The reference library doesn't populate this, but you can 1859 * use it to hold the resource if you resolve it. (Individual who 1860 * recorded the record and takes responsibility for its conten.) 1861 */ 1862 public Resource getRecorderTarget() { 1863 return this.recorderTarget; 1864 } 1865 1866 /** 1867 * @param value {@link #recorder} The actual object that is the target of the 1868 * reference. The reference library doesn't use these, but you can 1869 * use it to hold the resource if you resolve it. (Individual who 1870 * recorded the record and takes responsibility for its conten.) 1871 */ 1872 public AllergyIntolerance setRecorderTarget(Resource value) { 1873 this.recorderTarget = value; 1874 return this; 1875 } 1876 1877 /** 1878 * @return {@link #patient} (The patient who has the allergy or intolerance.) 1879 */ 1880 public Reference getPatient() { 1881 if (this.patient == null) 1882 if (Configuration.errorOnAutoCreate()) 1883 throw new Error("Attempt to auto-create AllergyIntolerance.patient"); 1884 else if (Configuration.doAutoCreate()) 1885 this.patient = new Reference(); // cc 1886 return this.patient; 1887 } 1888 1889 public boolean hasPatient() { 1890 return this.patient != null && !this.patient.isEmpty(); 1891 } 1892 1893 /** 1894 * @param value {@link #patient} (The patient who has the allergy or 1895 * intolerance.) 1896 */ 1897 public AllergyIntolerance setPatient(Reference value) { 1898 this.patient = value; 1899 return this; 1900 } 1901 1902 /** 1903 * @return {@link #patient} The actual object that is the target of the 1904 * reference. The reference library doesn't populate this, but you can 1905 * use it to hold the resource if you resolve it. (The patient who has 1906 * the allergy or intolerance.) 1907 */ 1908 public Patient getPatientTarget() { 1909 if (this.patientTarget == null) 1910 if (Configuration.errorOnAutoCreate()) 1911 throw new Error("Attempt to auto-create AllergyIntolerance.patient"); 1912 else if (Configuration.doAutoCreate()) 1913 this.patientTarget = new Patient(); // aa 1914 return this.patientTarget; 1915 } 1916 1917 /** 1918 * @param value {@link #patient} The actual object that is the target of the 1919 * reference. The reference library doesn't use these, but you can 1920 * use it to hold the resource if you resolve it. (The patient who 1921 * has the allergy or intolerance.) 1922 */ 1923 public AllergyIntolerance setPatientTarget(Patient value) { 1924 this.patientTarget = value; 1925 return this; 1926 } 1927 1928 /** 1929 * @return {@link #reporter} (The source of the information about the allergy 1930 * that is recorded.) 1931 */ 1932 public Reference getReporter() { 1933 if (this.reporter == null) 1934 if (Configuration.errorOnAutoCreate()) 1935 throw new Error("Attempt to auto-create AllergyIntolerance.reporter"); 1936 else if (Configuration.doAutoCreate()) 1937 this.reporter = new Reference(); // cc 1938 return this.reporter; 1939 } 1940 1941 public boolean hasReporter() { 1942 return this.reporter != null && !this.reporter.isEmpty(); 1943 } 1944 1945 /** 1946 * @param value {@link #reporter} (The source of the information about the 1947 * allergy that is recorded.) 1948 */ 1949 public AllergyIntolerance setReporter(Reference value) { 1950 this.reporter = value; 1951 return this; 1952 } 1953 1954 /** 1955 * @return {@link #reporter} The actual object that is the target of the 1956 * reference. The reference library doesn't populate this, but you can 1957 * use it to hold the resource if you resolve it. (The source of the 1958 * information about the allergy that is recorded.) 1959 */ 1960 public Resource getReporterTarget() { 1961 return this.reporterTarget; 1962 } 1963 1964 /** 1965 * @param value {@link #reporter} The actual object that is the target of the 1966 * reference. The reference library doesn't use these, but you can 1967 * use it to hold the resource if you resolve it. (The source of 1968 * the information about the allergy that is recorded.) 1969 */ 1970 public AllergyIntolerance setReporterTarget(Resource value) { 1971 this.reporterTarget = value; 1972 return this; 1973 } 1974 1975 /** 1976 * @return {@link #substance} (Identification of a substance, or a class of 1977 * substances, that is considered to be responsible for the adverse 1978 * reaction risk.) 1979 */ 1980 public CodeableConcept getSubstance() { 1981 if (this.substance == null) 1982 if (Configuration.errorOnAutoCreate()) 1983 throw new Error("Attempt to auto-create AllergyIntolerance.substance"); 1984 else if (Configuration.doAutoCreate()) 1985 this.substance = new CodeableConcept(); // cc 1986 return this.substance; 1987 } 1988 1989 public boolean hasSubstance() { 1990 return this.substance != null && !this.substance.isEmpty(); 1991 } 1992 1993 /** 1994 * @param value {@link #substance} (Identification of a substance, or a class of 1995 * substances, that is considered to be responsible for the adverse 1996 * reaction risk.) 1997 */ 1998 public AllergyIntolerance setSubstance(CodeableConcept value) { 1999 this.substance = value; 2000 return this; 2001 } 2002 2003 /** 2004 * @return {@link #status} (Assertion about certainty associated with the 2005 * propensity, or potential risk, of a reaction to the identified 2006 * Substance.). This is the underlying object with id, value and 2007 * extensions. The accessor "getStatus" gives direct access to the value 2008 */ 2009 public Enumeration<AllergyIntoleranceStatus> getStatusElement() { 2010 if (this.status == null) 2011 if (Configuration.errorOnAutoCreate()) 2012 throw new Error("Attempt to auto-create AllergyIntolerance.status"); 2013 else if (Configuration.doAutoCreate()) 2014 this.status = new Enumeration<AllergyIntoleranceStatus>(new AllergyIntoleranceStatusEnumFactory()); // bb 2015 return this.status; 2016 } 2017 2018 public boolean hasStatusElement() { 2019 return this.status != null && !this.status.isEmpty(); 2020 } 2021 2022 public boolean hasStatus() { 2023 return this.status != null && !this.status.isEmpty(); 2024 } 2025 2026 /** 2027 * @param value {@link #status} (Assertion about certainty associated with the 2028 * propensity, or potential risk, of a reaction to the identified 2029 * Substance.). This is the underlying object with id, value and 2030 * extensions. The accessor "getStatus" gives direct access to the 2031 * value 2032 */ 2033 public AllergyIntolerance setStatusElement(Enumeration<AllergyIntoleranceStatus> value) { 2034 this.status = value; 2035 return this; 2036 } 2037 2038 /** 2039 * @return Assertion about certainty associated with the propensity, or 2040 * potential risk, of a reaction to the identified Substance. 2041 */ 2042 public AllergyIntoleranceStatus getStatus() { 2043 return this.status == null ? null : this.status.getValue(); 2044 } 2045 2046 /** 2047 * @param value Assertion about certainty associated with the propensity, or 2048 * potential risk, of a reaction to the identified Substance. 2049 */ 2050 public AllergyIntolerance setStatus(AllergyIntoleranceStatus value) { 2051 if (value == null) 2052 this.status = null; 2053 else { 2054 if (this.status == null) 2055 this.status = new Enumeration<AllergyIntoleranceStatus>(new AllergyIntoleranceStatusEnumFactory()); 2056 this.status.setValue(value); 2057 } 2058 return this; 2059 } 2060 2061 /** 2062 * @return {@link #criticality} (Estimate of the potential clinical harm, or 2063 * seriousness, of the reaction to the identified Substance.). This is 2064 * the underlying object with id, value and extensions. The accessor 2065 * "getCriticality" gives direct access to the value 2066 */ 2067 public Enumeration<AllergyIntoleranceCriticality> getCriticalityElement() { 2068 if (this.criticality == null) 2069 if (Configuration.errorOnAutoCreate()) 2070 throw new Error("Attempt to auto-create AllergyIntolerance.criticality"); 2071 else if (Configuration.doAutoCreate()) 2072 this.criticality = new Enumeration<AllergyIntoleranceCriticality>( 2073 new AllergyIntoleranceCriticalityEnumFactory()); // bb 2074 return this.criticality; 2075 } 2076 2077 public boolean hasCriticalityElement() { 2078 return this.criticality != null && !this.criticality.isEmpty(); 2079 } 2080 2081 public boolean hasCriticality() { 2082 return this.criticality != null && !this.criticality.isEmpty(); 2083 } 2084 2085 /** 2086 * @param value {@link #criticality} (Estimate of the potential clinical harm, 2087 * or seriousness, of the reaction to the identified Substance.). 2088 * This is the underlying object with id, value and extensions. The 2089 * accessor "getCriticality" gives direct access to the value 2090 */ 2091 public AllergyIntolerance setCriticalityElement(Enumeration<AllergyIntoleranceCriticality> value) { 2092 this.criticality = value; 2093 return this; 2094 } 2095 2096 /** 2097 * @return Estimate of the potential clinical harm, or seriousness, of the 2098 * reaction to the identified Substance. 2099 */ 2100 public AllergyIntoleranceCriticality getCriticality() { 2101 return this.criticality == null ? null : this.criticality.getValue(); 2102 } 2103 2104 /** 2105 * @param value Estimate of the potential clinical harm, or seriousness, of the 2106 * reaction to the identified Substance. 2107 */ 2108 public AllergyIntolerance setCriticality(AllergyIntoleranceCriticality value) { 2109 if (value == null) 2110 this.criticality = null; 2111 else { 2112 if (this.criticality == null) 2113 this.criticality = new Enumeration<AllergyIntoleranceCriticality>( 2114 new AllergyIntoleranceCriticalityEnumFactory()); 2115 this.criticality.setValue(value); 2116 } 2117 return this; 2118 } 2119 2120 /** 2121 * @return {@link #type} (Identification of the underlying physiological 2122 * mechanism for the reaction risk.). This is the underlying object with 2123 * id, value and extensions. The accessor "getType" gives direct access 2124 * to the value 2125 */ 2126 public Enumeration<AllergyIntoleranceType> getTypeElement() { 2127 if (this.type == null) 2128 if (Configuration.errorOnAutoCreate()) 2129 throw new Error("Attempt to auto-create AllergyIntolerance.type"); 2130 else if (Configuration.doAutoCreate()) 2131 this.type = new Enumeration<AllergyIntoleranceType>(new AllergyIntoleranceTypeEnumFactory()); // bb 2132 return this.type; 2133 } 2134 2135 public boolean hasTypeElement() { 2136 return this.type != null && !this.type.isEmpty(); 2137 } 2138 2139 public boolean hasType() { 2140 return this.type != null && !this.type.isEmpty(); 2141 } 2142 2143 /** 2144 * @param value {@link #type} (Identification of the underlying physiological 2145 * mechanism for the reaction risk.). This is the underlying object 2146 * with id, value and extensions. The accessor "getType" gives 2147 * direct access to the value 2148 */ 2149 public AllergyIntolerance setTypeElement(Enumeration<AllergyIntoleranceType> value) { 2150 this.type = value; 2151 return this; 2152 } 2153 2154 /** 2155 * @return Identification of the underlying physiological mechanism for the 2156 * reaction risk. 2157 */ 2158 public AllergyIntoleranceType getType() { 2159 return this.type == null ? null : this.type.getValue(); 2160 } 2161 2162 /** 2163 * @param value Identification of the underlying physiological mechanism for the 2164 * reaction risk. 2165 */ 2166 public AllergyIntolerance setType(AllergyIntoleranceType value) { 2167 if (value == null) 2168 this.type = null; 2169 else { 2170 if (this.type == null) 2171 this.type = new Enumeration<AllergyIntoleranceType>(new AllergyIntoleranceTypeEnumFactory()); 2172 this.type.setValue(value); 2173 } 2174 return this; 2175 } 2176 2177 /** 2178 * @return {@link #category} (Category of the identified Substance.). This is 2179 * the underlying object with id, value and extensions. The accessor 2180 * "getCategory" gives direct access to the value 2181 */ 2182 public Enumeration<AllergyIntoleranceCategory> getCategoryElement() { 2183 if (this.category == null) 2184 if (Configuration.errorOnAutoCreate()) 2185 throw new Error("Attempt to auto-create AllergyIntolerance.category"); 2186 else if (Configuration.doAutoCreate()) 2187 this.category = new Enumeration<AllergyIntoleranceCategory>(new AllergyIntoleranceCategoryEnumFactory()); // bb 2188 return this.category; 2189 } 2190 2191 public boolean hasCategoryElement() { 2192 return this.category != null && !this.category.isEmpty(); 2193 } 2194 2195 public boolean hasCategory() { 2196 return this.category != null && !this.category.isEmpty(); 2197 } 2198 2199 /** 2200 * @param value {@link #category} (Category of the identified Substance.). This 2201 * is the underlying object with id, value and extensions. The 2202 * accessor "getCategory" gives direct access to the value 2203 */ 2204 public AllergyIntolerance setCategoryElement(Enumeration<AllergyIntoleranceCategory> value) { 2205 this.category = value; 2206 return this; 2207 } 2208 2209 /** 2210 * @return Category of the identified Substance. 2211 */ 2212 public AllergyIntoleranceCategory getCategory() { 2213 return this.category == null ? null : this.category.getValue(); 2214 } 2215 2216 /** 2217 * @param value Category of the identified Substance. 2218 */ 2219 public AllergyIntolerance setCategory(AllergyIntoleranceCategory value) { 2220 if (value == null) 2221 this.category = null; 2222 else { 2223 if (this.category == null) 2224 this.category = new Enumeration<AllergyIntoleranceCategory>(new AllergyIntoleranceCategoryEnumFactory()); 2225 this.category.setValue(value); 2226 } 2227 return this; 2228 } 2229 2230 /** 2231 * @return {@link #lastOccurence} (Represents the date and/or time of the last 2232 * known occurrence of a reaction event.). This is the underlying object 2233 * with id, value and extensions. The accessor "getLastOccurence" gives 2234 * direct access to the value 2235 */ 2236 public DateTimeType getLastOccurenceElement() { 2237 if (this.lastOccurence == null) 2238 if (Configuration.errorOnAutoCreate()) 2239 throw new Error("Attempt to auto-create AllergyIntolerance.lastOccurence"); 2240 else if (Configuration.doAutoCreate()) 2241 this.lastOccurence = new DateTimeType(); // bb 2242 return this.lastOccurence; 2243 } 2244 2245 public boolean hasLastOccurenceElement() { 2246 return this.lastOccurence != null && !this.lastOccurence.isEmpty(); 2247 } 2248 2249 public boolean hasLastOccurence() { 2250 return this.lastOccurence != null && !this.lastOccurence.isEmpty(); 2251 } 2252 2253 /** 2254 * @param value {@link #lastOccurence} (Represents the date and/or time of the 2255 * last known occurrence of a reaction event.). This is the 2256 * underlying object with id, value and extensions. The accessor 2257 * "getLastOccurence" gives direct access to the value 2258 */ 2259 public AllergyIntolerance setLastOccurenceElement(DateTimeType value) { 2260 this.lastOccurence = value; 2261 return this; 2262 } 2263 2264 /** 2265 * @return Represents the date and/or time of the last known occurrence of a 2266 * reaction event. 2267 */ 2268 public Date getLastOccurence() { 2269 return this.lastOccurence == null ? null : this.lastOccurence.getValue(); 2270 } 2271 2272 /** 2273 * @param value Represents the date and/or time of the last known occurrence of 2274 * a reaction event. 2275 */ 2276 public AllergyIntolerance setLastOccurence(Date value) { 2277 if (value == null) 2278 this.lastOccurence = null; 2279 else { 2280 if (this.lastOccurence == null) 2281 this.lastOccurence = new DateTimeType(); 2282 this.lastOccurence.setValue(value); 2283 } 2284 return this; 2285 } 2286 2287 /** 2288 * @return {@link #note} (Additional narrative about the propensity for the 2289 * Adverse Reaction, not captured in other fields.) 2290 */ 2291 public Annotation getNote() { 2292 if (this.note == null) 2293 if (Configuration.errorOnAutoCreate()) 2294 throw new Error("Attempt to auto-create AllergyIntolerance.note"); 2295 else if (Configuration.doAutoCreate()) 2296 this.note = new Annotation(); // cc 2297 return this.note; 2298 } 2299 2300 public boolean hasNote() { 2301 return this.note != null && !this.note.isEmpty(); 2302 } 2303 2304 /** 2305 * @param value {@link #note} (Additional narrative about the propensity for the 2306 * Adverse Reaction, not captured in other fields.) 2307 */ 2308 public AllergyIntolerance setNote(Annotation value) { 2309 this.note = value; 2310 return this; 2311 } 2312 2313 /** 2314 * @return {@link #reaction} (Details about each adverse reaction event linked 2315 * to exposure to the identified Substance.) 2316 */ 2317 public List<AllergyIntoleranceReactionComponent> getReaction() { 2318 if (this.reaction == null) 2319 this.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2320 return this.reaction; 2321 } 2322 2323 public boolean hasReaction() { 2324 if (this.reaction == null) 2325 return false; 2326 for (AllergyIntoleranceReactionComponent item : this.reaction) 2327 if (!item.isEmpty()) 2328 return true; 2329 return false; 2330 } 2331 2332 /** 2333 * @return {@link #reaction} (Details about each adverse reaction event linked 2334 * to exposure to the identified Substance.) 2335 */ 2336 // syntactic sugar 2337 public AllergyIntoleranceReactionComponent addReaction() { // 3 2338 AllergyIntoleranceReactionComponent t = new AllergyIntoleranceReactionComponent(); 2339 if (this.reaction == null) 2340 this.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2341 this.reaction.add(t); 2342 return t; 2343 } 2344 2345 // syntactic sugar 2346 public AllergyIntolerance addReaction(AllergyIntoleranceReactionComponent t) { // 3 2347 if (t == null) 2348 return this; 2349 if (this.reaction == null) 2350 this.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2351 this.reaction.add(t); 2352 return this; 2353 } 2354 2355 protected void listChildren(List<Property> childrenList) { 2356 super.listChildren(childrenList); 2357 childrenList.add(new Property("identifier", "Identifier", 2358 "This records identifiers associated with this allergy/intolerance concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 2359 0, java.lang.Integer.MAX_VALUE, identifier)); 2360 childrenList.add( 2361 new Property("onset", "dateTime", "Record of the date and/or time of the onset of the Allergy or Intolerance.", 2362 0, java.lang.Integer.MAX_VALUE, onset)); 2363 childrenList.add(new Property("recordedDate", "dateTime", "Date when the sensitivity was recorded.", 0, 2364 java.lang.Integer.MAX_VALUE, recordedDate)); 2365 childrenList.add(new Property("recorder", "Reference(Practitioner|Patient)", 2366 "Individual who recorded the record and takes responsibility for its conten.", 0, java.lang.Integer.MAX_VALUE, 2367 recorder)); 2368 childrenList.add(new Property("patient", "Reference(Patient)", "The patient who has the allergy or intolerance.", 0, 2369 java.lang.Integer.MAX_VALUE, patient)); 2370 childrenList.add(new Property("reporter", "Reference(Patient|RelatedPerson|Practitioner)", 2371 "The source of the information about the allergy that is recorded.", 0, java.lang.Integer.MAX_VALUE, reporter)); 2372 childrenList.add(new Property("substance", "CodeableConcept", 2373 "Identification of a substance, or a class of substances, that is considered to be responsible for the adverse reaction risk.", 2374 0, java.lang.Integer.MAX_VALUE, substance)); 2375 childrenList.add(new Property("status", "code", 2376 "Assertion about certainty associated with the propensity, or potential risk, of a reaction to the identified Substance.", 2377 0, java.lang.Integer.MAX_VALUE, status)); 2378 childrenList.add(new Property("criticality", "code", 2379 "Estimate of the potential clinical harm, or seriousness, of the reaction to the identified Substance.", 0, 2380 java.lang.Integer.MAX_VALUE, criticality)); 2381 childrenList.add( 2382 new Property("type", "code", "Identification of the underlying physiological mechanism for the reaction risk.", 2383 0, java.lang.Integer.MAX_VALUE, type)); 2384 childrenList.add(new Property("category", "code", "Category of the identified Substance.", 0, 2385 java.lang.Integer.MAX_VALUE, category)); 2386 childrenList.add(new Property("lastOccurence", "dateTime", 2387 "Represents the date and/or time of the last known occurrence of a reaction event.", 0, 2388 java.lang.Integer.MAX_VALUE, lastOccurence)); 2389 childrenList.add(new Property("note", "Annotation", 2390 "Additional narrative about the propensity for the Adverse Reaction, not captured in other fields.", 0, 2391 java.lang.Integer.MAX_VALUE, note)); 2392 childrenList.add(new Property("reaction", "", 2393 "Details about each adverse reaction event linked to exposure to the identified Substance.", 0, 2394 java.lang.Integer.MAX_VALUE, reaction)); 2395 } 2396 2397 @Override 2398 public void setProperty(String name, Base value) throws FHIRException { 2399 if (name.equals("identifier")) 2400 this.getIdentifier().add(castToIdentifier(value)); 2401 else if (name.equals("onset")) 2402 this.onset = castToDateTime(value); // DateTimeType 2403 else if (name.equals("recordedDate")) 2404 this.recordedDate = castToDateTime(value); // DateTimeType 2405 else if (name.equals("recorder")) 2406 this.recorder = castToReference(value); // Reference 2407 else if (name.equals("patient")) 2408 this.patient = castToReference(value); // Reference 2409 else if (name.equals("reporter")) 2410 this.reporter = castToReference(value); // Reference 2411 else if (name.equals("substance")) 2412 this.substance = castToCodeableConcept(value); // CodeableConcept 2413 else if (name.equals("status")) 2414 this.status = new AllergyIntoleranceStatusEnumFactory().fromType(value); // Enumeration<AllergyIntoleranceStatus> 2415 else if (name.equals("criticality")) 2416 this.criticality = new AllergyIntoleranceCriticalityEnumFactory().fromType(value); // Enumeration<AllergyIntoleranceCriticality> 2417 else if (name.equals("type")) 2418 this.type = new AllergyIntoleranceTypeEnumFactory().fromType(value); // Enumeration<AllergyIntoleranceType> 2419 else if (name.equals("category")) 2420 this.category = new AllergyIntoleranceCategoryEnumFactory().fromType(value); // Enumeration<AllergyIntoleranceCategory> 2421 else if (name.equals("lastOccurence")) 2422 this.lastOccurence = castToDateTime(value); // DateTimeType 2423 else if (name.equals("note")) 2424 this.note = castToAnnotation(value); // Annotation 2425 else if (name.equals("reaction")) 2426 this.getReaction().add((AllergyIntoleranceReactionComponent) value); 2427 else 2428 super.setProperty(name, value); 2429 } 2430 2431 @Override 2432 public Base addChild(String name) throws FHIRException { 2433 if (name.equals("identifier")) { 2434 return addIdentifier(); 2435 } else if (name.equals("onset")) { 2436 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.onset"); 2437 } else if (name.equals("recordedDate")) { 2438 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.recordedDate"); 2439 } else if (name.equals("recorder")) { 2440 this.recorder = new Reference(); 2441 return this.recorder; 2442 } else if (name.equals("patient")) { 2443 this.patient = new Reference(); 2444 return this.patient; 2445 } else if (name.equals("reporter")) { 2446 this.reporter = new Reference(); 2447 return this.reporter; 2448 } else if (name.equals("substance")) { 2449 this.substance = new CodeableConcept(); 2450 return this.substance; 2451 } else if (name.equals("status")) { 2452 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.status"); 2453 } else if (name.equals("criticality")) { 2454 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.criticality"); 2455 } else if (name.equals("type")) { 2456 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.type"); 2457 } else if (name.equals("category")) { 2458 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.category"); 2459 } else if (name.equals("lastOccurence")) { 2460 throw new FHIRException("Cannot call addChild on a singleton property AllergyIntolerance.lastOccurence"); 2461 } else if (name.equals("note")) { 2462 this.note = new Annotation(); 2463 return this.note; 2464 } else if (name.equals("reaction")) { 2465 return addReaction(); 2466 } else 2467 return super.addChild(name); 2468 } 2469 2470 public String fhirType() { 2471 return "AllergyIntolerance"; 2472 2473 } 2474 2475 public AllergyIntolerance copy() { 2476 AllergyIntolerance dst = new AllergyIntolerance(); 2477 copyValues(dst); 2478 if (identifier != null) { 2479 dst.identifier = new ArrayList<Identifier>(); 2480 for (Identifier i : identifier) 2481 dst.identifier.add(i.copy()); 2482 } 2483 ; 2484 dst.onset = onset == null ? null : onset.copy(); 2485 dst.recordedDate = recordedDate == null ? null : recordedDate.copy(); 2486 dst.recorder = recorder == null ? null : recorder.copy(); 2487 dst.patient = patient == null ? null : patient.copy(); 2488 dst.reporter = reporter == null ? null : reporter.copy(); 2489 dst.substance = substance == null ? null : substance.copy(); 2490 dst.status = status == null ? null : status.copy(); 2491 dst.criticality = criticality == null ? null : criticality.copy(); 2492 dst.type = type == null ? null : type.copy(); 2493 dst.category = category == null ? null : category.copy(); 2494 dst.lastOccurence = lastOccurence == null ? null : lastOccurence.copy(); 2495 dst.note = note == null ? null : note.copy(); 2496 if (reaction != null) { 2497 dst.reaction = new ArrayList<AllergyIntoleranceReactionComponent>(); 2498 for (AllergyIntoleranceReactionComponent i : reaction) 2499 dst.reaction.add(i.copy()); 2500 } 2501 ; 2502 return dst; 2503 } 2504 2505 protected AllergyIntolerance typedCopy() { 2506 return copy(); 2507 } 2508 2509 @Override 2510 public boolean equalsDeep(Base other) { 2511 if (!super.equalsDeep(other)) 2512 return false; 2513 if (!(other instanceof AllergyIntolerance)) 2514 return false; 2515 AllergyIntolerance o = (AllergyIntolerance) other; 2516 return compareDeep(identifier, o.identifier, true) && compareDeep(onset, o.onset, true) 2517 && compareDeep(recordedDate, o.recordedDate, true) && compareDeep(recorder, o.recorder, true) 2518 && compareDeep(patient, o.patient, true) && compareDeep(reporter, o.reporter, true) 2519 && compareDeep(substance, o.substance, true) && compareDeep(status, o.status, true) 2520 && compareDeep(criticality, o.criticality, true) && compareDeep(type, o.type, true) 2521 && compareDeep(category, o.category, true) && compareDeep(lastOccurence, o.lastOccurence, true) 2522 && compareDeep(note, o.note, true) && compareDeep(reaction, o.reaction, true); 2523 } 2524 2525 @Override 2526 public boolean equalsShallow(Base other) { 2527 if (!super.equalsShallow(other)) 2528 return false; 2529 if (!(other instanceof AllergyIntolerance)) 2530 return false; 2531 AllergyIntolerance o = (AllergyIntolerance) other; 2532 return compareValues(onset, o.onset, true) && compareValues(recordedDate, o.recordedDate, true) 2533 && compareValues(status, o.status, true) && compareValues(criticality, o.criticality, true) 2534 && compareValues(type, o.type, true) && compareValues(category, o.category, true) 2535 && compareValues(lastOccurence, o.lastOccurence, true); 2536 } 2537 2538 public boolean isEmpty() { 2539 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (onset == null || onset.isEmpty()) 2540 && (recordedDate == null || recordedDate.isEmpty()) && (recorder == null || recorder.isEmpty()) 2541 && (patient == null || patient.isEmpty()) && (reporter == null || reporter.isEmpty()) 2542 && (substance == null || substance.isEmpty()) && (status == null || status.isEmpty()) 2543 && (criticality == null || criticality.isEmpty()) && (type == null || type.isEmpty()) 2544 && (category == null || category.isEmpty()) && (lastOccurence == null || lastOccurence.isEmpty()) 2545 && (note == null || note.isEmpty()) && (reaction == null || reaction.isEmpty()); 2546 } 2547 2548 @Override 2549 public ResourceType getResourceType() { 2550 return ResourceType.AllergyIntolerance; 2551 } 2552 2553 @SearchParamDefinition(name = "severity", path = "AllergyIntolerance.reaction.severity", description = "mild | moderate | severe (of event as a whole)", type = "token") 2554 public static final String SP_SEVERITY = "severity"; 2555 @SearchParamDefinition(name = "date", path = "AllergyIntolerance.recordedDate", description = "When recorded", type = "date") 2556 public static final String SP_DATE = "date"; 2557 @SearchParamDefinition(name = "identifier", path = "AllergyIntolerance.identifier", description = "External ids for this item", type = "token") 2558 public static final String SP_IDENTIFIER = "identifier"; 2559 @SearchParamDefinition(name = "manifestation", path = "AllergyIntolerance.reaction.manifestation", description = "Clinical symptoms/signs associated with the Event", type = "token") 2560 public static final String SP_MANIFESTATION = "manifestation"; 2561 @SearchParamDefinition(name = "recorder", path = "AllergyIntolerance.recorder", description = "Who recorded the sensitivity", type = "reference") 2562 public static final String SP_RECORDER = "recorder"; 2563 @SearchParamDefinition(name = "substance", path = "AllergyIntolerance.substance | AllergyIntolerance.reaction.substance", description = "Substance, (or class) considered to be responsible for risk", type = "token") 2564 public static final String SP_SUBSTANCE = "substance"; 2565 @SearchParamDefinition(name = "criticality", path = "AllergyIntolerance.criticality", description = "CRITL | CRITH | CRITU", type = "token") 2566 public static final String SP_CRITICALITY = "criticality"; 2567 @SearchParamDefinition(name = "reporter", path = "AllergyIntolerance.reporter", description = "Source of the information about the allergy", type = "reference") 2568 public static final String SP_REPORTER = "reporter"; 2569 @SearchParamDefinition(name = "type", path = "AllergyIntolerance.type", description = "allergy | intolerance - Underlying mechanism (if known)", type = "token") 2570 public static final String SP_TYPE = "type"; 2571 @SearchParamDefinition(name = "onset", path = "AllergyIntolerance.reaction.onset", description = "Date(/time) when manifestations showed", type = "date") 2572 public static final String SP_ONSET = "onset"; 2573 @SearchParamDefinition(name = "route", path = "AllergyIntolerance.reaction.exposureRoute", description = "How the subject was exposed to the substance", type = "token") 2574 public static final String SP_ROUTE = "route"; 2575 @SearchParamDefinition(name = "patient", path = "AllergyIntolerance.patient", description = "Who the sensitivity is for", type = "reference") 2576 public static final String SP_PATIENT = "patient"; 2577 @SearchParamDefinition(name = "category", path = "AllergyIntolerance.category", description = "food | medication | environment | other - Category of Substance", type = "token") 2578 public static final String SP_CATEGORY = "category"; 2579 @SearchParamDefinition(name = "last-date", path = "AllergyIntolerance.lastOccurence", description = "Date(/time) of last known occurrence of a reaction", type = "date") 2580 public static final String SP_LASTDATE = "last-date"; 2581 @SearchParamDefinition(name = "status", path = "AllergyIntolerance.status", description = "active | unconfirmed | confirmed | inactive | resolved | refuted | entered-in-error", type = "token") 2582 public static final String SP_STATUS = "status"; 2583 2584}