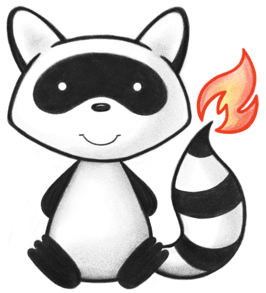
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.Date; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Child; 037import ca.uhn.fhir.model.api.annotation.DatatypeDef; 038import ca.uhn.fhir.model.api.annotation.Description; 039import org.hl7.fhir.instance.model.api.ICompositeType; 040import org.hl7.fhir.exceptions.FHIRException; 041 042/** 043 * A text note which also contains information about who made the statement and 044 * when. 045 */ 046@DatatypeDef(name = "Annotation") 047public class Annotation extends Type implements ICompositeType { 048 049 /** 050 * The individual responsible for making the annotation. 051 */ 052 @Child(name = "author", type = { Practitioner.class, Patient.class, RelatedPerson.class, 053 StringType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 054 @Description(shortDefinition = "Individual responsible for the annotation", formalDefinition = "The individual responsible for making the annotation.") 055 protected Type author; 056 057 /** 058 * Indicates when this particular annotation was made. 059 */ 060 @Child(name = "time", type = { DateTimeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 061 @Description(shortDefinition = "When the annotation was made", formalDefinition = "Indicates when this particular annotation was made.") 062 protected DateTimeType time; 063 064 /** 065 * The text of the annotation. 066 */ 067 @Child(name = "text", type = { StringType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 068 @Description(shortDefinition = "The annotation - text content", formalDefinition = "The text of the annotation.") 069 protected StringType text; 070 071 private static final long serialVersionUID = -575590381L; 072 073 /* 074 * Constructor 075 */ 076 public Annotation() { 077 super(); 078 } 079 080 /* 081 * Constructor 082 */ 083 public Annotation(StringType text) { 084 super(); 085 this.text = text; 086 } 087 088 /** 089 * @return {@link #author} (The individual responsible for making the 090 * annotation.) 091 */ 092 public Type getAuthor() { 093 return this.author; 094 } 095 096 /** 097 * @return {@link #author} (The individual responsible for making the 098 * annotation.) 099 */ 100 public Reference getAuthorReference() throws FHIRException { 101 if (!(this.author instanceof Reference)) 102 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.author.getClass().getName() 103 + " was encountered"); 104 return (Reference) this.author; 105 } 106 107 public boolean hasAuthorReference() { 108 return this.author instanceof Reference; 109 } 110 111 /** 112 * @return {@link #author} (The individual responsible for making the 113 * annotation.) 114 */ 115 public StringType getAuthorStringType() throws FHIRException { 116 if (!(this.author instanceof StringType)) 117 throw new FHIRException("Type mismatch: the type StringType was expected, but " + this.author.getClass().getName() 118 + " was encountered"); 119 return (StringType) this.author; 120 } 121 122 public boolean hasAuthorStringType() { 123 return this.author instanceof StringType; 124 } 125 126 public boolean hasAuthor() { 127 return this.author != null && !this.author.isEmpty(); 128 } 129 130 /** 131 * @param value {@link #author} (The individual responsible for making the 132 * annotation.) 133 */ 134 public Annotation setAuthor(Type value) { 135 this.author = value; 136 return this; 137 } 138 139 /** 140 * @return {@link #time} (Indicates when this particular annotation was made.). 141 * This is the underlying object with id, value and extensions. The 142 * accessor "getTime" gives direct access to the value 143 */ 144 public DateTimeType getTimeElement() { 145 if (this.time == null) 146 if (Configuration.errorOnAutoCreate()) 147 throw new Error("Attempt to auto-create Annotation.time"); 148 else if (Configuration.doAutoCreate()) 149 this.time = new DateTimeType(); // bb 150 return this.time; 151 } 152 153 public boolean hasTimeElement() { 154 return this.time != null && !this.time.isEmpty(); 155 } 156 157 public boolean hasTime() { 158 return this.time != null && !this.time.isEmpty(); 159 } 160 161 /** 162 * @param value {@link #time} (Indicates when this particular annotation was 163 * made.). This is the underlying object with id, value and 164 * extensions. The accessor "getTime" gives direct access to the 165 * value 166 */ 167 public Annotation setTimeElement(DateTimeType value) { 168 this.time = value; 169 return this; 170 } 171 172 /** 173 * @return Indicates when this particular annotation was made. 174 */ 175 public Date getTime() { 176 return this.time == null ? null : this.time.getValue(); 177 } 178 179 /** 180 * @param value Indicates when this particular annotation was made. 181 */ 182 public Annotation setTime(Date value) { 183 if (value == null) 184 this.time = null; 185 else { 186 if (this.time == null) 187 this.time = new DateTimeType(); 188 this.time.setValue(value); 189 } 190 return this; 191 } 192 193 /** 194 * @return {@link #text} (The text of the annotation.). This is the underlying 195 * object with id, value and extensions. The accessor "getText" gives 196 * direct access to the value 197 */ 198 public StringType getTextElement() { 199 if (this.text == null) 200 if (Configuration.errorOnAutoCreate()) 201 throw new Error("Attempt to auto-create Annotation.text"); 202 else if (Configuration.doAutoCreate()) 203 this.text = new StringType(); // bb 204 return this.text; 205 } 206 207 public boolean hasTextElement() { 208 return this.text != null && !this.text.isEmpty(); 209 } 210 211 public boolean hasText() { 212 return this.text != null && !this.text.isEmpty(); 213 } 214 215 /** 216 * @param value {@link #text} (The text of the annotation.). This is the 217 * underlying object with id, value and extensions. The accessor 218 * "getText" gives direct access to the value 219 */ 220 public Annotation setTextElement(StringType value) { 221 this.text = value; 222 return this; 223 } 224 225 /** 226 * @return The text of the annotation. 227 */ 228 public String getText() { 229 return this.text == null ? null : this.text.getValue(); 230 } 231 232 /** 233 * @param value The text of the annotation. 234 */ 235 public Annotation setText(String value) { 236 if (this.text == null) 237 this.text = new StringType(); 238 this.text.setValue(value); 239 return this; 240 } 241 242 protected void listChildren(List<Property> childrenList) { 243 super.listChildren(childrenList); 244 childrenList.add(new Property("author[x]", "Reference(Practitioner|Patient|RelatedPerson)|string", 245 "The individual responsible for making the annotation.", 0, java.lang.Integer.MAX_VALUE, author)); 246 childrenList.add(new Property("time", "dateTime", "Indicates when this particular annotation was made.", 0, 247 java.lang.Integer.MAX_VALUE, time)); 248 childrenList 249 .add(new Property("text", "string", "The text of the annotation.", 0, java.lang.Integer.MAX_VALUE, text)); 250 } 251 252 @Override 253 public void setProperty(String name, Base value) throws FHIRException { 254 if (name.equals("author[x]")) 255 this.author = (Type) value; // Type 256 else if (name.equals("time")) 257 this.time = castToDateTime(value); // DateTimeType 258 else if (name.equals("text")) 259 this.text = castToString(value); // StringType 260 else 261 super.setProperty(name, value); 262 } 263 264 @Override 265 public Base addChild(String name) throws FHIRException { 266 if (name.equals("authorReference")) { 267 this.author = new Reference(); 268 return this.author; 269 } else if (name.equals("authorString")) { 270 this.author = new StringType(); 271 return this.author; 272 } else if (name.equals("time")) { 273 throw new FHIRException("Cannot call addChild on a singleton property Annotation.time"); 274 } else if (name.equals("text")) { 275 throw new FHIRException("Cannot call addChild on a singleton property Annotation.text"); 276 } else 277 return super.addChild(name); 278 } 279 280 public String fhirType() { 281 return "Annotation"; 282 283 } 284 285 public Annotation copy() { 286 Annotation dst = new Annotation(); 287 copyValues(dst); 288 dst.author = author == null ? null : author.copy(); 289 dst.time = time == null ? null : time.copy(); 290 dst.text = text == null ? null : text.copy(); 291 return dst; 292 } 293 294 protected Annotation typedCopy() { 295 return copy(); 296 } 297 298 @Override 299 public boolean equalsDeep(Base other) { 300 if (!super.equalsDeep(other)) 301 return false; 302 if (!(other instanceof Annotation)) 303 return false; 304 Annotation o = (Annotation) other; 305 return compareDeep(author, o.author, true) && compareDeep(time, o.time, true) && compareDeep(text, o.text, true); 306 } 307 308 @Override 309 public boolean equalsShallow(Base other) { 310 if (!super.equalsShallow(other)) 311 return false; 312 if (!(other instanceof Annotation)) 313 return false; 314 Annotation o = (Annotation) other; 315 return compareValues(time, o.time, true) && compareValues(text, o.text, true); 316 } 317 318 public boolean isEmpty() { 319 return super.isEmpty() && (author == null || author.isEmpty()) && (time == null || time.isEmpty()) 320 && (text == null || text.isEmpty()); 321 } 322 323}