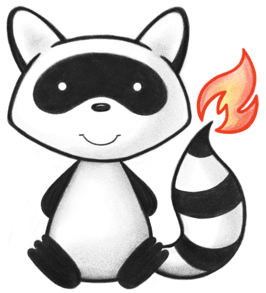
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * A booking of a healthcare event among patient(s), practitioner(s), related 048 * person(s) and/or device(s) for a specific date/time. This may result in one 049 * or more Encounter(s). 050 */ 051@ResourceDef(name = "Appointment", profile = "http://hl7.org/fhir/Profile/Appointment") 052public class Appointment extends DomainResource { 053 054 public enum AppointmentStatus { 055 /** 056 * None of the participant(s) have finalized their acceptance of the appointment 057 * request, and the start/end time may not be set yet. 058 */ 059 PROPOSED, 060 /** 061 * Some or all of the participant(s) have not finalized their acceptance of the 062 * appointment request. 063 */ 064 PENDING, 065 /** 066 * All participant(s) have been considered and the appointment is confirmed to 067 * go ahead at the date/times specified. 068 */ 069 BOOKED, 070 /** 071 * Some of the patients have arrived. 072 */ 073 ARRIVED, 074 /** 075 * This appointment has completed and may have resulted in an encounter. 076 */ 077 FULFILLED, 078 /** 079 * The appointment has been cancelled. 080 */ 081 CANCELLED, 082 /** 083 * Some or all of the participant(s) have not/did not appear for the appointment 084 * (usually the patient). 085 */ 086 NOSHOW, 087 /** 088 * added to help the parsers 089 */ 090 NULL; 091 092 public static AppointmentStatus fromCode(String codeString) throws FHIRException { 093 if (codeString == null || "".equals(codeString)) 094 return null; 095 if ("proposed".equals(codeString)) 096 return PROPOSED; 097 if ("pending".equals(codeString)) 098 return PENDING; 099 if ("booked".equals(codeString)) 100 return BOOKED; 101 if ("arrived".equals(codeString)) 102 return ARRIVED; 103 if ("fulfilled".equals(codeString)) 104 return FULFILLED; 105 if ("cancelled".equals(codeString)) 106 return CANCELLED; 107 if ("noshow".equals(codeString)) 108 return NOSHOW; 109 throw new FHIRException("Unknown AppointmentStatus code '" + codeString + "'"); 110 } 111 112 public String toCode() { 113 switch (this) { 114 case PROPOSED: 115 return "proposed"; 116 case PENDING: 117 return "pending"; 118 case BOOKED: 119 return "booked"; 120 case ARRIVED: 121 return "arrived"; 122 case FULFILLED: 123 return "fulfilled"; 124 case CANCELLED: 125 return "cancelled"; 126 case NOSHOW: 127 return "noshow"; 128 case NULL: 129 return null; 130 default: 131 return "?"; 132 } 133 } 134 135 public String getSystem() { 136 switch (this) { 137 case PROPOSED: 138 return "http://hl7.org/fhir/appointmentstatus"; 139 case PENDING: 140 return "http://hl7.org/fhir/appointmentstatus"; 141 case BOOKED: 142 return "http://hl7.org/fhir/appointmentstatus"; 143 case ARRIVED: 144 return "http://hl7.org/fhir/appointmentstatus"; 145 case FULFILLED: 146 return "http://hl7.org/fhir/appointmentstatus"; 147 case CANCELLED: 148 return "http://hl7.org/fhir/appointmentstatus"; 149 case NOSHOW: 150 return "http://hl7.org/fhir/appointmentstatus"; 151 case NULL: 152 return null; 153 default: 154 return "?"; 155 } 156 } 157 158 public String getDefinition() { 159 switch (this) { 160 case PROPOSED: 161 return "None of the participant(s) have finalized their acceptance of the appointment request, and the start/end time may not be set yet."; 162 case PENDING: 163 return "Some or all of the participant(s) have not finalized their acceptance of the appointment request."; 164 case BOOKED: 165 return "All participant(s) have been considered and the appointment is confirmed to go ahead at the date/times specified."; 166 case ARRIVED: 167 return "Some of the patients have arrived."; 168 case FULFILLED: 169 return "This appointment has completed and may have resulted in an encounter."; 170 case CANCELLED: 171 return "The appointment has been cancelled."; 172 case NOSHOW: 173 return "Some or all of the participant(s) have not/did not appear for the appointment (usually the patient)."; 174 case NULL: 175 return null; 176 default: 177 return "?"; 178 } 179 } 180 181 public String getDisplay() { 182 switch (this) { 183 case PROPOSED: 184 return "Proposed"; 185 case PENDING: 186 return "Pending"; 187 case BOOKED: 188 return "Booked"; 189 case ARRIVED: 190 return "Arrived"; 191 case FULFILLED: 192 return "Fulfilled"; 193 case CANCELLED: 194 return "Cancelled"; 195 case NOSHOW: 196 return "No Show"; 197 case NULL: 198 return null; 199 default: 200 return "?"; 201 } 202 } 203 } 204 205 public static class AppointmentStatusEnumFactory implements EnumFactory<AppointmentStatus> { 206 public AppointmentStatus fromCode(String codeString) throws IllegalArgumentException { 207 if (codeString == null || "".equals(codeString)) 208 if (codeString == null || "".equals(codeString)) 209 return null; 210 if ("proposed".equals(codeString)) 211 return AppointmentStatus.PROPOSED; 212 if ("pending".equals(codeString)) 213 return AppointmentStatus.PENDING; 214 if ("booked".equals(codeString)) 215 return AppointmentStatus.BOOKED; 216 if ("arrived".equals(codeString)) 217 return AppointmentStatus.ARRIVED; 218 if ("fulfilled".equals(codeString)) 219 return AppointmentStatus.FULFILLED; 220 if ("cancelled".equals(codeString)) 221 return AppointmentStatus.CANCELLED; 222 if ("noshow".equals(codeString)) 223 return AppointmentStatus.NOSHOW; 224 throw new IllegalArgumentException("Unknown AppointmentStatus code '" + codeString + "'"); 225 } 226 227 public Enumeration<AppointmentStatus> fromType(Base code) throws FHIRException { 228 if (code == null || code.isEmpty()) 229 return null; 230 String codeString = ((PrimitiveType) code).asStringValue(); 231 if (codeString == null || "".equals(codeString)) 232 return null; 233 if ("proposed".equals(codeString)) 234 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.PROPOSED); 235 if ("pending".equals(codeString)) 236 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.PENDING); 237 if ("booked".equals(codeString)) 238 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.BOOKED); 239 if ("arrived".equals(codeString)) 240 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.ARRIVED); 241 if ("fulfilled".equals(codeString)) 242 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.FULFILLED); 243 if ("cancelled".equals(codeString)) 244 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.CANCELLED); 245 if ("noshow".equals(codeString)) 246 return new Enumeration<AppointmentStatus>(this, AppointmentStatus.NOSHOW); 247 throw new FHIRException("Unknown AppointmentStatus code '" + codeString + "'"); 248 } 249 250 public String toCode(AppointmentStatus code) 251 { 252 if (code == AppointmentStatus.NULL) 253 return null; 254 if (code == AppointmentStatus.PROPOSED) 255 return "proposed"; 256 if (code == AppointmentStatus.PENDING) 257 return "pending"; 258 if (code == AppointmentStatus.BOOKED) 259 return "booked"; 260 if (code == AppointmentStatus.ARRIVED) 261 return "arrived"; 262 if (code == AppointmentStatus.FULFILLED) 263 return "fulfilled"; 264 if (code == AppointmentStatus.CANCELLED) 265 return "cancelled"; 266 if (code == AppointmentStatus.NOSHOW) 267 return "noshow"; 268 return "?"; 269 } 270 } 271 272 public enum ParticipantRequired { 273 /** 274 * The participant is required to attend the appointment. 275 */ 276 REQUIRED, 277 /** 278 * The participant may optionally attend the appointment. 279 */ 280 OPTIONAL, 281 /** 282 * The participant is excluded from the appointment, and may not be informed of 283 * the appointment taking place. (Appointment is about them, not for them - such 284 * as 2 doctors discussing results about a patient's test). 285 */ 286 INFORMATIONONLY, 287 /** 288 * added to help the parsers 289 */ 290 NULL; 291 292 public static ParticipantRequired fromCode(String codeString) throws FHIRException { 293 if (codeString == null || "".equals(codeString)) 294 return null; 295 if ("required".equals(codeString)) 296 return REQUIRED; 297 if ("optional".equals(codeString)) 298 return OPTIONAL; 299 if ("information-only".equals(codeString)) 300 return INFORMATIONONLY; 301 throw new FHIRException("Unknown ParticipantRequired code '" + codeString + "'"); 302 } 303 304 public String toCode() { 305 switch (this) { 306 case REQUIRED: 307 return "required"; 308 case OPTIONAL: 309 return "optional"; 310 case INFORMATIONONLY: 311 return "information-only"; 312 case NULL: 313 return null; 314 default: 315 return "?"; 316 } 317 } 318 319 public String getSystem() { 320 switch (this) { 321 case REQUIRED: 322 return "http://hl7.org/fhir/participantrequired"; 323 case OPTIONAL: 324 return "http://hl7.org/fhir/participantrequired"; 325 case INFORMATIONONLY: 326 return "http://hl7.org/fhir/participantrequired"; 327 case NULL: 328 return null; 329 default: 330 return "?"; 331 } 332 } 333 334 public String getDefinition() { 335 switch (this) { 336 case REQUIRED: 337 return "The participant is required to attend the appointment."; 338 case OPTIONAL: 339 return "The participant may optionally attend the appointment."; 340 case INFORMATIONONLY: 341 return "The participant is excluded from the appointment, and may not be informed of the appointment taking place. (Appointment is about them, not for them - such as 2 doctors discussing results about a patient's test)."; 342 case NULL: 343 return null; 344 default: 345 return "?"; 346 } 347 } 348 349 public String getDisplay() { 350 switch (this) { 351 case REQUIRED: 352 return "Required"; 353 case OPTIONAL: 354 return "Optional"; 355 case INFORMATIONONLY: 356 return "Information Only"; 357 case NULL: 358 return null; 359 default: 360 return "?"; 361 } 362 } 363 } 364 365 public static class ParticipantRequiredEnumFactory implements EnumFactory<ParticipantRequired> { 366 public ParticipantRequired fromCode(String codeString) throws IllegalArgumentException { 367 if (codeString == null || "".equals(codeString)) 368 if (codeString == null || "".equals(codeString)) 369 return null; 370 if ("required".equals(codeString)) 371 return ParticipantRequired.REQUIRED; 372 if ("optional".equals(codeString)) 373 return ParticipantRequired.OPTIONAL; 374 if ("information-only".equals(codeString)) 375 return ParticipantRequired.INFORMATIONONLY; 376 throw new IllegalArgumentException("Unknown ParticipantRequired code '" + codeString + "'"); 377 } 378 379 public Enumeration<ParticipantRequired> fromType(Base code) throws FHIRException { 380 if (code == null || code.isEmpty()) 381 return null; 382 String codeString = ((PrimitiveType) code).asStringValue(); 383 if (codeString == null || "".equals(codeString)) 384 return null; 385 if ("required".equals(codeString)) 386 return new Enumeration<ParticipantRequired>(this, ParticipantRequired.REQUIRED); 387 if ("optional".equals(codeString)) 388 return new Enumeration<ParticipantRequired>(this, ParticipantRequired.OPTIONAL); 389 if ("information-only".equals(codeString)) 390 return new Enumeration<ParticipantRequired>(this, ParticipantRequired.INFORMATIONONLY); 391 throw new FHIRException("Unknown ParticipantRequired code '" + codeString + "'"); 392 } 393 394 public String toCode(ParticipantRequired code) 395 { 396 if (code == ParticipantRequired.NULL) 397 return null; 398 if (code == ParticipantRequired.REQUIRED) 399 return "required"; 400 if (code == ParticipantRequired.OPTIONAL) 401 return "optional"; 402 if (code == ParticipantRequired.INFORMATIONONLY) 403 return "information-only"; 404 return "?"; 405 } 406 } 407 408 public enum ParticipationStatus { 409 /** 410 * The participant has accepted the appointment. 411 */ 412 ACCEPTED, 413 /** 414 * The participant has declined the appointment and will not participate in the 415 * appointment. 416 */ 417 DECLINED, 418 /** 419 * The participant has tentatively accepted the appointment. This could be 420 * automatically created by a system and requires further processing before it 421 * can be accepted. There is no commitment that attendance will occur. 422 */ 423 TENTATIVE, 424 /** 425 * The participant needs to indicate if they accept the appointment by changing 426 * this status to one of the other statuses. 427 */ 428 NEEDSACTION, 429 /** 430 * added to help the parsers 431 */ 432 NULL; 433 434 public static ParticipationStatus fromCode(String codeString) throws FHIRException { 435 if (codeString == null || "".equals(codeString)) 436 return null; 437 if ("accepted".equals(codeString)) 438 return ACCEPTED; 439 if ("declined".equals(codeString)) 440 return DECLINED; 441 if ("tentative".equals(codeString)) 442 return TENTATIVE; 443 if ("needs-action".equals(codeString)) 444 return NEEDSACTION; 445 throw new FHIRException("Unknown ParticipationStatus code '" + codeString + "'"); 446 } 447 448 public String toCode() { 449 switch (this) { 450 case ACCEPTED: 451 return "accepted"; 452 case DECLINED: 453 return "declined"; 454 case TENTATIVE: 455 return "tentative"; 456 case NEEDSACTION: 457 return "needs-action"; 458 case NULL: 459 return null; 460 default: 461 return "?"; 462 } 463 } 464 465 public String getSystem() { 466 switch (this) { 467 case ACCEPTED: 468 return "http://hl7.org/fhir/participationstatus"; 469 case DECLINED: 470 return "http://hl7.org/fhir/participationstatus"; 471 case TENTATIVE: 472 return "http://hl7.org/fhir/participationstatus"; 473 case NEEDSACTION: 474 return "http://hl7.org/fhir/participationstatus"; 475 case NULL: 476 return null; 477 default: 478 return "?"; 479 } 480 } 481 482 public String getDefinition() { 483 switch (this) { 484 case ACCEPTED: 485 return "The participant has accepted the appointment."; 486 case DECLINED: 487 return "The participant has declined the appointment and will not participate in the appointment."; 488 case TENTATIVE: 489 return "The participant has tentatively accepted the appointment. This could be automatically created by a system and requires further processing before it can be accepted. There is no commitment that attendance will occur."; 490 case NEEDSACTION: 491 return "The participant needs to indicate if they accept the appointment by changing this status to one of the other statuses."; 492 case NULL: 493 return null; 494 default: 495 return "?"; 496 } 497 } 498 499 public String getDisplay() { 500 switch (this) { 501 case ACCEPTED: 502 return "Accepted"; 503 case DECLINED: 504 return "Declined"; 505 case TENTATIVE: 506 return "Tentative"; 507 case NEEDSACTION: 508 return "Needs Action"; 509 case NULL: 510 return null; 511 default: 512 return "?"; 513 } 514 } 515 } 516 517 public static class ParticipationStatusEnumFactory implements EnumFactory<ParticipationStatus> { 518 public ParticipationStatus fromCode(String codeString) throws IllegalArgumentException { 519 if (codeString == null || "".equals(codeString)) 520 if (codeString == null || "".equals(codeString)) 521 return null; 522 if ("accepted".equals(codeString)) 523 return ParticipationStatus.ACCEPTED; 524 if ("declined".equals(codeString)) 525 return ParticipationStatus.DECLINED; 526 if ("tentative".equals(codeString)) 527 return ParticipationStatus.TENTATIVE; 528 if ("needs-action".equals(codeString)) 529 return ParticipationStatus.NEEDSACTION; 530 throw new IllegalArgumentException("Unknown ParticipationStatus code '" + codeString + "'"); 531 } 532 533 public Enumeration<ParticipationStatus> fromType(Base code) throws FHIRException { 534 if (code == null || code.isEmpty()) 535 return null; 536 String codeString = ((PrimitiveType) code).asStringValue(); 537 if (codeString == null || "".equals(codeString)) 538 return null; 539 if ("accepted".equals(codeString)) 540 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.ACCEPTED); 541 if ("declined".equals(codeString)) 542 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.DECLINED); 543 if ("tentative".equals(codeString)) 544 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.TENTATIVE); 545 if ("needs-action".equals(codeString)) 546 return new Enumeration<ParticipationStatus>(this, ParticipationStatus.NEEDSACTION); 547 throw new FHIRException("Unknown ParticipationStatus code '" + codeString + "'"); 548 } 549 550 public String toCode(ParticipationStatus code) 551 { 552 if (code == ParticipationStatus.NULL) 553 return null; 554 if (code == ParticipationStatus.ACCEPTED) 555 return "accepted"; 556 if (code == ParticipationStatus.DECLINED) 557 return "declined"; 558 if (code == ParticipationStatus.TENTATIVE) 559 return "tentative"; 560 if (code == ParticipationStatus.NEEDSACTION) 561 return "needs-action"; 562 return "?"; 563 } 564 } 565 566 @Block() 567 public static class AppointmentParticipantComponent extends BackboneElement implements IBaseBackboneElement { 568 /** 569 * Role of participant in the appointment. 570 */ 571 @Child(name = "type", type = { 572 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 573 @Description(shortDefinition = "Role of participant in the appointment", formalDefinition = "Role of participant in the appointment.") 574 protected List<CodeableConcept> type; 575 576 /** 577 * A Person, Location/HealthcareService or Device that is participating in the 578 * appointment. 579 */ 580 @Child(name = "actor", type = { Patient.class, Practitioner.class, RelatedPerson.class, Device.class, 581 HealthcareService.class, Location.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 582 @Description(shortDefinition = "Person, Location/HealthcareService or Device", formalDefinition = "A Person, Location/HealthcareService or Device that is participating in the appointment.") 583 protected Reference actor; 584 585 /** 586 * The actual object that is the target of the reference (A Person, 587 * Location/HealthcareService or Device that is participating in the 588 * appointment.) 589 */ 590 protected Resource actorTarget; 591 592 /** 593 * Is this participant required to be present at the meeting. This covers a 594 * use-case where 2 doctors need to meet to discuss the results for a specific 595 * patient, and the patient is not required to be present. 596 */ 597 @Child(name = "required", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 598 @Description(shortDefinition = "required | optional | information-only", formalDefinition = "Is this participant required to be present at the meeting. This covers a use-case where 2 doctors need to meet to discuss the results for a specific patient, and the patient is not required to be present.") 599 protected Enumeration<ParticipantRequired> required; 600 601 /** 602 * Participation status of the Patient. 603 */ 604 @Child(name = "status", type = { CodeType.class }, order = 4, min = 1, max = 1, modifier = false, summary = false) 605 @Description(shortDefinition = "accepted | declined | tentative | needs-action", formalDefinition = "Participation status of the Patient.") 606 protected Enumeration<ParticipationStatus> status; 607 608 private static final long serialVersionUID = -1620552507L; 609 610 /* 611 * Constructor 612 */ 613 public AppointmentParticipantComponent() { 614 super(); 615 } 616 617 /* 618 * Constructor 619 */ 620 public AppointmentParticipantComponent(Enumeration<ParticipationStatus> status) { 621 super(); 622 this.status = status; 623 } 624 625 /** 626 * @return {@link #type} (Role of participant in the appointment.) 627 */ 628 public List<CodeableConcept> getType() { 629 if (this.type == null) 630 this.type = new ArrayList<CodeableConcept>(); 631 return this.type; 632 } 633 634 public boolean hasType() { 635 if (this.type == null) 636 return false; 637 for (CodeableConcept item : this.type) 638 if (!item.isEmpty()) 639 return true; 640 return false; 641 } 642 643 /** 644 * @return {@link #type} (Role of participant in the appointment.) 645 */ 646 // syntactic sugar 647 public CodeableConcept addType() { // 3 648 CodeableConcept t = new CodeableConcept(); 649 if (this.type == null) 650 this.type = new ArrayList<CodeableConcept>(); 651 this.type.add(t); 652 return t; 653 } 654 655 // syntactic sugar 656 public AppointmentParticipantComponent addType(CodeableConcept t) { // 3 657 if (t == null) 658 return this; 659 if (this.type == null) 660 this.type = new ArrayList<CodeableConcept>(); 661 this.type.add(t); 662 return this; 663 } 664 665 /** 666 * @return {@link #actor} (A Person, Location/HealthcareService or Device that 667 * is participating in the appointment.) 668 */ 669 public Reference getActor() { 670 if (this.actor == null) 671 if (Configuration.errorOnAutoCreate()) 672 throw new Error("Attempt to auto-create AppointmentParticipantComponent.actor"); 673 else if (Configuration.doAutoCreate()) 674 this.actor = new Reference(); // cc 675 return this.actor; 676 } 677 678 public boolean hasActor() { 679 return this.actor != null && !this.actor.isEmpty(); 680 } 681 682 /** 683 * @param value {@link #actor} (A Person, Location/HealthcareService or Device 684 * that is participating in the appointment.) 685 */ 686 public AppointmentParticipantComponent setActor(Reference value) { 687 this.actor = value; 688 return this; 689 } 690 691 /** 692 * @return {@link #actor} The actual object that is the target of the reference. 693 * The reference library doesn't populate this, but you can use it to 694 * hold the resource if you resolve it. (A Person, 695 * Location/HealthcareService or Device that is participating in the 696 * appointment.) 697 */ 698 public Resource getActorTarget() { 699 return this.actorTarget; 700 } 701 702 /** 703 * @param value {@link #actor} The actual object that is the target of the 704 * reference. The reference library doesn't use these, but you can 705 * use it to hold the resource if you resolve it. (A Person, 706 * Location/HealthcareService or Device that is participating in 707 * the appointment.) 708 */ 709 public AppointmentParticipantComponent setActorTarget(Resource value) { 710 this.actorTarget = value; 711 return this; 712 } 713 714 /** 715 * @return {@link #required} (Is this participant required to be present at the 716 * meeting. This covers a use-case where 2 doctors need to meet to 717 * discuss the results for a specific patient, and the patient is not 718 * required to be present.). This is the underlying object with id, 719 * value and extensions. The accessor "getRequired" gives direct access 720 * to the value 721 */ 722 public Enumeration<ParticipantRequired> getRequiredElement() { 723 if (this.required == null) 724 if (Configuration.errorOnAutoCreate()) 725 throw new Error("Attempt to auto-create AppointmentParticipantComponent.required"); 726 else if (Configuration.doAutoCreate()) 727 this.required = new Enumeration<ParticipantRequired>(new ParticipantRequiredEnumFactory()); // bb 728 return this.required; 729 } 730 731 public boolean hasRequiredElement() { 732 return this.required != null && !this.required.isEmpty(); 733 } 734 735 public boolean hasRequired() { 736 return this.required != null && !this.required.isEmpty(); 737 } 738 739 /** 740 * @param value {@link #required} (Is this participant required to be present at 741 * the meeting. This covers a use-case where 2 doctors need to meet 742 * to discuss the results for a specific patient, and the patient 743 * is not required to be present.). This is the underlying object 744 * with id, value and extensions. The accessor "getRequired" gives 745 * direct access to the value 746 */ 747 public AppointmentParticipantComponent setRequiredElement(Enumeration<ParticipantRequired> value) { 748 this.required = value; 749 return this; 750 } 751 752 /** 753 * @return Is this participant required to be present at the meeting. This 754 * covers a use-case where 2 doctors need to meet to discuss the results 755 * for a specific patient, and the patient is not required to be 756 * present. 757 */ 758 public ParticipantRequired getRequired() { 759 return this.required == null ? null : this.required.getValue(); 760 } 761 762 /** 763 * @param value Is this participant required to be present at the meeting. This 764 * covers a use-case where 2 doctors need to meet to discuss the 765 * results for a specific patient, and the patient is not required 766 * to be present. 767 */ 768 public AppointmentParticipantComponent setRequired(ParticipantRequired value) { 769 if (value == null) 770 this.required = null; 771 else { 772 if (this.required == null) 773 this.required = new Enumeration<ParticipantRequired>(new ParticipantRequiredEnumFactory()); 774 this.required.setValue(value); 775 } 776 return this; 777 } 778 779 /** 780 * @return {@link #status} (Participation status of the Patient.). This is the 781 * underlying object with id, value and extensions. The accessor 782 * "getStatus" gives direct access to the value 783 */ 784 public Enumeration<ParticipationStatus> getStatusElement() { 785 if (this.status == null) 786 if (Configuration.errorOnAutoCreate()) 787 throw new Error("Attempt to auto-create AppointmentParticipantComponent.status"); 788 else if (Configuration.doAutoCreate()) 789 this.status = new Enumeration<ParticipationStatus>(new ParticipationStatusEnumFactory()); // bb 790 return this.status; 791 } 792 793 public boolean hasStatusElement() { 794 return this.status != null && !this.status.isEmpty(); 795 } 796 797 public boolean hasStatus() { 798 return this.status != null && !this.status.isEmpty(); 799 } 800 801 /** 802 * @param value {@link #status} (Participation status of the Patient.). This is 803 * the underlying object with id, value and extensions. The 804 * accessor "getStatus" gives direct access to the value 805 */ 806 public AppointmentParticipantComponent setStatusElement(Enumeration<ParticipationStatus> value) { 807 this.status = value; 808 return this; 809 } 810 811 /** 812 * @return Participation status of the Patient. 813 */ 814 public ParticipationStatus getStatus() { 815 return this.status == null ? null : this.status.getValue(); 816 } 817 818 /** 819 * @param value Participation status of the Patient. 820 */ 821 public AppointmentParticipantComponent setStatus(ParticipationStatus value) { 822 if (this.status == null) 823 this.status = new Enumeration<ParticipationStatus>(new ParticipationStatusEnumFactory()); 824 this.status.setValue(value); 825 return this; 826 } 827 828 protected void listChildren(List<Property> childrenList) { 829 super.listChildren(childrenList); 830 childrenList.add(new Property("type", "CodeableConcept", "Role of participant in the appointment.", 0, 831 java.lang.Integer.MAX_VALUE, type)); 832 childrenList 833 .add(new Property("actor", "Reference(Patient|Practitioner|RelatedPerson|Device|HealthcareService|Location)", 834 "A Person, Location/HealthcareService or Device that is participating in the appointment.", 0, 835 java.lang.Integer.MAX_VALUE, actor)); 836 childrenList.add(new Property("required", "code", 837 "Is this participant required to be present at the meeting. This covers a use-case where 2 doctors need to meet to discuss the results for a specific patient, and the patient is not required to be present.", 838 0, java.lang.Integer.MAX_VALUE, required)); 839 childrenList.add(new Property("status", "code", "Participation status of the Patient.", 0, 840 java.lang.Integer.MAX_VALUE, status)); 841 } 842 843 @Override 844 public void setProperty(String name, Base value) throws FHIRException { 845 if (name.equals("type")) 846 this.getType().add(castToCodeableConcept(value)); 847 else if (name.equals("actor")) 848 this.actor = castToReference(value); // Reference 849 else if (name.equals("required")) 850 this.required = new ParticipantRequiredEnumFactory().fromType(value); // Enumeration<ParticipantRequired> 851 else if (name.equals("status")) 852 this.status = new ParticipationStatusEnumFactory().fromType(value); // Enumeration<ParticipationStatus> 853 else 854 super.setProperty(name, value); 855 } 856 857 @Override 858 public Base addChild(String name) throws FHIRException { 859 if (name.equals("type")) { 860 return addType(); 861 } else if (name.equals("actor")) { 862 this.actor = new Reference(); 863 return this.actor; 864 } else if (name.equals("required")) { 865 throw new FHIRException("Cannot call addChild on a singleton property Appointment.required"); 866 } else if (name.equals("status")) { 867 throw new FHIRException("Cannot call addChild on a singleton property Appointment.status"); 868 } else 869 return super.addChild(name); 870 } 871 872 public AppointmentParticipantComponent copy() { 873 AppointmentParticipantComponent dst = new AppointmentParticipantComponent(); 874 copyValues(dst); 875 if (type != null) { 876 dst.type = new ArrayList<CodeableConcept>(); 877 for (CodeableConcept i : type) 878 dst.type.add(i.copy()); 879 } 880 ; 881 dst.actor = actor == null ? null : actor.copy(); 882 dst.required = required == null ? null : required.copy(); 883 dst.status = status == null ? null : status.copy(); 884 return dst; 885 } 886 887 @Override 888 public boolean equalsDeep(Base other) { 889 if (!super.equalsDeep(other)) 890 return false; 891 if (!(other instanceof AppointmentParticipantComponent)) 892 return false; 893 AppointmentParticipantComponent o = (AppointmentParticipantComponent) other; 894 return compareDeep(type, o.type, true) && compareDeep(actor, o.actor, true) 895 && compareDeep(required, o.required, true) && compareDeep(status, o.status, true); 896 } 897 898 @Override 899 public boolean equalsShallow(Base other) { 900 if (!super.equalsShallow(other)) 901 return false; 902 if (!(other instanceof AppointmentParticipantComponent)) 903 return false; 904 AppointmentParticipantComponent o = (AppointmentParticipantComponent) other; 905 return compareValues(required, o.required, true) && compareValues(status, o.status, true); 906 } 907 908 public boolean isEmpty() { 909 return super.isEmpty() && (type == null || type.isEmpty()) && (actor == null || actor.isEmpty()) 910 && (required == null || required.isEmpty()) && (status == null || status.isEmpty()); 911 } 912 913 public String fhirType() { 914 return "Appointment.participant"; 915 916 } 917 918 } 919 920 /** 921 * This records identifiers associated with this appointment concern that are 922 * defined by business processes and/or used to refer to it when a direct URL 923 * reference to the resource itself is not appropriate (e.g. in CDA documents, 924 * or in written / printed documentation). 925 */ 926 @Child(name = "identifier", type = { 927 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 928 @Description(shortDefinition = "External Ids for this item", formalDefinition = "This records identifiers associated with this appointment concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).") 929 protected List<Identifier> identifier; 930 931 /** 932 * The overall status of the Appointment. Each of the participants has their own 933 * participation status which indicates their involvement in the process, 934 * however this status indicates the shared status. 935 */ 936 @Child(name = "status", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 937 @Description(shortDefinition = "proposed | pending | booked | arrived | fulfilled | cancelled | noshow", formalDefinition = "The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status.") 938 protected Enumeration<AppointmentStatus> status; 939 940 /** 941 * The type of appointment that is being booked (This may also be associated 942 * with participants for location, and/or a HealthcareService). 943 */ 944 @Child(name = "type", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 945 @Description(shortDefinition = "The type of appointment that is being booked", formalDefinition = "The type of appointment that is being booked (This may also be associated with participants for location, and/or a HealthcareService).") 946 protected CodeableConcept type; 947 948 /** 949 * The reason that this appointment is being scheduled. This is more clinical 950 * than administrative. 951 */ 952 @Child(name = "reason", type = { 953 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 954 @Description(shortDefinition = "Reason this appointment is scheduled", formalDefinition = "The reason that this appointment is being scheduled. This is more clinical than administrative.") 955 protected CodeableConcept reason; 956 957 /** 958 * The priority of the appointment. Can be used to make informed decisions if 959 * needing to re-prioritize appointments. (The iCal Standard specifies 0 as 960 * undefined, 1 as highest, 9 as lowest priority). 961 */ 962 @Child(name = "priority", type = { 963 UnsignedIntType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 964 @Description(shortDefinition = "Used to make informed decisions if needing to re-prioritize", formalDefinition = "The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority).") 965 protected UnsignedIntType priority; 966 967 /** 968 * The brief description of the appointment as would be shown on a subject line 969 * in a meeting request, or appointment list. Detailed or expanded information 970 * should be put in the comment field. 971 */ 972 @Child(name = "description", type = { 973 StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 974 @Description(shortDefinition = "Shown on a subject line in a meeting request, or appointment list", formalDefinition = "The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the comment field.") 975 protected StringType description; 976 977 /** 978 * Date/Time that the appointment is to take place. 979 */ 980 @Child(name = "start", type = { InstantType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 981 @Description(shortDefinition = "When appointment is to take place", formalDefinition = "Date/Time that the appointment is to take place.") 982 protected InstantType start; 983 984 /** 985 * Date/Time that the appointment is to conclude. 986 */ 987 @Child(name = "end", type = { InstantType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 988 @Description(shortDefinition = "When appointment is to conclude", formalDefinition = "Date/Time that the appointment is to conclude.") 989 protected InstantType end; 990 991 /** 992 * Number of minutes that the appointment is to take. This can be less than the 993 * duration between the start and end times (where actual time of appointment is 994 * only an estimate or is a planned appointment request). 995 */ 996 @Child(name = "minutesDuration", type = { 997 PositiveIntType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 998 @Description(shortDefinition = "Can be less than start/end (e.g. estimate)", formalDefinition = "Number of minutes that the appointment is to take. This can be less than the duration between the start and end times (where actual time of appointment is only an estimate or is a planned appointment request).") 999 protected PositiveIntType minutesDuration; 1000 1001 /** 1002 * The slot that this appointment is filling. If provided then the schedule will 1003 * not be provided as slots are not recursive, and the start/end values MUST be 1004 * the same as from the slot. 1005 */ 1006 @Child(name = "slot", type = { 1007 Slot.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1008 @Description(shortDefinition = "If provided, then no schedule and start/end values MUST match slot", formalDefinition = "The slot that this appointment is filling. If provided then the schedule will not be provided as slots are not recursive, and the start/end values MUST be the same as from the slot.") 1009 protected List<Reference> slot; 1010 /** 1011 * The actual objects that are the target of the reference (The slot that this 1012 * appointment is filling. If provided then the schedule will not be provided as 1013 * slots are not recursive, and the start/end values MUST be the same as from 1014 * the slot.) 1015 */ 1016 protected List<Slot> slotTarget; 1017 1018 /** 1019 * Additional comments about the appointment. 1020 */ 1021 @Child(name = "comment", type = { StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1022 @Description(shortDefinition = "Additional comments", formalDefinition = "Additional comments about the appointment.") 1023 protected StringType comment; 1024 1025 /** 1026 * List of participants involved in the appointment. 1027 */ 1028 @Child(name = "participant", type = {}, order = 11, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1029 @Description(shortDefinition = "Participants involved in appointment", formalDefinition = "List of participants involved in the appointment.") 1030 protected List<AppointmentParticipantComponent> participant; 1031 1032 private static final long serialVersionUID = -1403944125L; 1033 1034 /* 1035 * Constructor 1036 */ 1037 public Appointment() { 1038 super(); 1039 } 1040 1041 /* 1042 * Constructor 1043 */ 1044 public Appointment(Enumeration<AppointmentStatus> status) { 1045 super(); 1046 this.status = status; 1047 } 1048 1049 /** 1050 * @return {@link #identifier} (This records identifiers associated with this 1051 * appointment concern that are defined by business processes and/or 1052 * used to refer to it when a direct URL reference to the resource 1053 * itself is not appropriate (e.g. in CDA documents, or in written / 1054 * printed documentation).) 1055 */ 1056 public List<Identifier> getIdentifier() { 1057 if (this.identifier == null) 1058 this.identifier = new ArrayList<Identifier>(); 1059 return this.identifier; 1060 } 1061 1062 public boolean hasIdentifier() { 1063 if (this.identifier == null) 1064 return false; 1065 for (Identifier item : this.identifier) 1066 if (!item.isEmpty()) 1067 return true; 1068 return false; 1069 } 1070 1071 /** 1072 * @return {@link #identifier} (This records identifiers associated with this 1073 * appointment concern that are defined by business processes and/or 1074 * used to refer to it when a direct URL reference to the resource 1075 * itself is not appropriate (e.g. in CDA documents, or in written / 1076 * printed documentation).) 1077 */ 1078 // syntactic sugar 1079 public Identifier addIdentifier() { // 3 1080 Identifier t = new Identifier(); 1081 if (this.identifier == null) 1082 this.identifier = new ArrayList<Identifier>(); 1083 this.identifier.add(t); 1084 return t; 1085 } 1086 1087 // syntactic sugar 1088 public Appointment addIdentifier(Identifier t) { // 3 1089 if (t == null) 1090 return this; 1091 if (this.identifier == null) 1092 this.identifier = new ArrayList<Identifier>(); 1093 this.identifier.add(t); 1094 return this; 1095 } 1096 1097 /** 1098 * @return {@link #status} (The overall status of the Appointment. Each of the 1099 * participants has their own participation status which indicates their 1100 * involvement in the process, however this status indicates the shared 1101 * status.). This is the underlying object with id, value and 1102 * extensions. The accessor "getStatus" gives direct access to the value 1103 */ 1104 public Enumeration<AppointmentStatus> getStatusElement() { 1105 if (this.status == null) 1106 if (Configuration.errorOnAutoCreate()) 1107 throw new Error("Attempt to auto-create Appointment.status"); 1108 else if (Configuration.doAutoCreate()) 1109 this.status = new Enumeration<AppointmentStatus>(new AppointmentStatusEnumFactory()); // bb 1110 return this.status; 1111 } 1112 1113 public boolean hasStatusElement() { 1114 return this.status != null && !this.status.isEmpty(); 1115 } 1116 1117 public boolean hasStatus() { 1118 return this.status != null && !this.status.isEmpty(); 1119 } 1120 1121 /** 1122 * @param value {@link #status} (The overall status of the Appointment. Each of 1123 * the participants has their own participation status which 1124 * indicates their involvement in the process, however this status 1125 * indicates the shared status.). This is the underlying object 1126 * with id, value and extensions. The accessor "getStatus" gives 1127 * direct access to the value 1128 */ 1129 public Appointment setStatusElement(Enumeration<AppointmentStatus> value) { 1130 this.status = value; 1131 return this; 1132 } 1133 1134 /** 1135 * @return The overall status of the Appointment. Each of the participants has 1136 * their own participation status which indicates their involvement in 1137 * the process, however this status indicates the shared status. 1138 */ 1139 public AppointmentStatus getStatus() { 1140 return this.status == null ? null : this.status.getValue(); 1141 } 1142 1143 /** 1144 * @param value The overall status of the Appointment. Each of the participants 1145 * has their own participation status which indicates their 1146 * involvement in the process, however this status indicates the 1147 * shared status. 1148 */ 1149 public Appointment setStatus(AppointmentStatus value) { 1150 if (this.status == null) 1151 this.status = new Enumeration<AppointmentStatus>(new AppointmentStatusEnumFactory()); 1152 this.status.setValue(value); 1153 return this; 1154 } 1155 1156 /** 1157 * @return {@link #type} (The type of appointment that is being booked (This may 1158 * also be associated with participants for location, and/or a 1159 * HealthcareService).) 1160 */ 1161 public CodeableConcept getType() { 1162 if (this.type == null) 1163 if (Configuration.errorOnAutoCreate()) 1164 throw new Error("Attempt to auto-create Appointment.type"); 1165 else if (Configuration.doAutoCreate()) 1166 this.type = new CodeableConcept(); // cc 1167 return this.type; 1168 } 1169 1170 public boolean hasType() { 1171 return this.type != null && !this.type.isEmpty(); 1172 } 1173 1174 /** 1175 * @param value {@link #type} (The type of appointment that is being booked 1176 * (This may also be associated with participants for location, 1177 * and/or a HealthcareService).) 1178 */ 1179 public Appointment setType(CodeableConcept value) { 1180 this.type = value; 1181 return this; 1182 } 1183 1184 /** 1185 * @return {@link #reason} (The reason that this appointment is being scheduled. 1186 * This is more clinical than administrative.) 1187 */ 1188 public CodeableConcept getReason() { 1189 if (this.reason == null) 1190 if (Configuration.errorOnAutoCreate()) 1191 throw new Error("Attempt to auto-create Appointment.reason"); 1192 else if (Configuration.doAutoCreate()) 1193 this.reason = new CodeableConcept(); // cc 1194 return this.reason; 1195 } 1196 1197 public boolean hasReason() { 1198 return this.reason != null && !this.reason.isEmpty(); 1199 } 1200 1201 /** 1202 * @param value {@link #reason} (The reason that this appointment is being 1203 * scheduled. This is more clinical than administrative.) 1204 */ 1205 public Appointment setReason(CodeableConcept value) { 1206 this.reason = value; 1207 return this; 1208 } 1209 1210 /** 1211 * @return {@link #priority} (The priority of the appointment. Can be used to 1212 * make informed decisions if needing to re-prioritize appointments. 1213 * (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as 1214 * lowest priority).). This is the underlying object with id, value and 1215 * extensions. The accessor "getPriority" gives direct access to the 1216 * value 1217 */ 1218 public UnsignedIntType getPriorityElement() { 1219 if (this.priority == null) 1220 if (Configuration.errorOnAutoCreate()) 1221 throw new Error("Attempt to auto-create Appointment.priority"); 1222 else if (Configuration.doAutoCreate()) 1223 this.priority = new UnsignedIntType(); // bb 1224 return this.priority; 1225 } 1226 1227 public boolean hasPriorityElement() { 1228 return this.priority != null && !this.priority.isEmpty(); 1229 } 1230 1231 public boolean hasPriority() { 1232 return this.priority != null && !this.priority.isEmpty(); 1233 } 1234 1235 /** 1236 * @param value {@link #priority} (The priority of the appointment. Can be used 1237 * to make informed decisions if needing to re-prioritize 1238 * appointments. (The iCal Standard specifies 0 as undefined, 1 as 1239 * highest, 9 as lowest priority).). This is the underlying object 1240 * with id, value and extensions. The accessor "getPriority" gives 1241 * direct access to the value 1242 */ 1243 public Appointment setPriorityElement(UnsignedIntType value) { 1244 this.priority = value; 1245 return this; 1246 } 1247 1248 /** 1249 * @return The priority of the appointment. Can be used to make informed 1250 * decisions if needing to re-prioritize appointments. (The iCal 1251 * Standard specifies 0 as undefined, 1 as highest, 9 as lowest 1252 * priority). 1253 */ 1254 public int getPriority() { 1255 return this.priority == null || this.priority.isEmpty() ? 0 : this.priority.getValue(); 1256 } 1257 1258 /** 1259 * @param value The priority of the appointment. Can be used to make informed 1260 * decisions if needing to re-prioritize appointments. (The iCal 1261 * Standard specifies 0 as undefined, 1 as highest, 9 as lowest 1262 * priority). 1263 */ 1264 public Appointment setPriority(int value) { 1265 if (this.priority == null) 1266 this.priority = new UnsignedIntType(); 1267 this.priority.setValue(value); 1268 return this; 1269 } 1270 1271 /** 1272 * @return {@link #description} (The brief description of the appointment as 1273 * would be shown on a subject line in a meeting request, or appointment 1274 * list. Detailed or expanded information should be put in the comment 1275 * field.). This is the underlying object with id, value and extensions. 1276 * The accessor "getDescription" gives direct access to the value 1277 */ 1278 public StringType getDescriptionElement() { 1279 if (this.description == null) 1280 if (Configuration.errorOnAutoCreate()) 1281 throw new Error("Attempt to auto-create Appointment.description"); 1282 else if (Configuration.doAutoCreate()) 1283 this.description = new StringType(); // bb 1284 return this.description; 1285 } 1286 1287 public boolean hasDescriptionElement() { 1288 return this.description != null && !this.description.isEmpty(); 1289 } 1290 1291 public boolean hasDescription() { 1292 return this.description != null && !this.description.isEmpty(); 1293 } 1294 1295 /** 1296 * @param value {@link #description} (The brief description of the appointment 1297 * as would be shown on a subject line in a meeting request, or 1298 * appointment list. Detailed or expanded information should be put 1299 * in the comment field.). This is the underlying object with id, 1300 * value and extensions. The accessor "getDescription" gives direct 1301 * access to the value 1302 */ 1303 public Appointment setDescriptionElement(StringType value) { 1304 this.description = value; 1305 return this; 1306 } 1307 1308 /** 1309 * @return The brief description of the appointment as would be shown on a 1310 * subject line in a meeting request, or appointment list. Detailed or 1311 * expanded information should be put in the comment field. 1312 */ 1313 public String getDescription() { 1314 return this.description == null ? null : this.description.getValue(); 1315 } 1316 1317 /** 1318 * @param value The brief description of the appointment as would be shown on a 1319 * subject line in a meeting request, or appointment list. Detailed 1320 * or expanded information should be put in the comment field. 1321 */ 1322 public Appointment setDescription(String value) { 1323 if (Utilities.noString(value)) 1324 this.description = null; 1325 else { 1326 if (this.description == null) 1327 this.description = new StringType(); 1328 this.description.setValue(value); 1329 } 1330 return this; 1331 } 1332 1333 /** 1334 * @return {@link #start} (Date/Time that the appointment is to take place.). 1335 * This is the underlying object with id, value and extensions. The 1336 * accessor "getStart" gives direct access to the value 1337 */ 1338 public InstantType getStartElement() { 1339 if (this.start == null) 1340 if (Configuration.errorOnAutoCreate()) 1341 throw new Error("Attempt to auto-create Appointment.start"); 1342 else if (Configuration.doAutoCreate()) 1343 this.start = new InstantType(); // bb 1344 return this.start; 1345 } 1346 1347 public boolean hasStartElement() { 1348 return this.start != null && !this.start.isEmpty(); 1349 } 1350 1351 public boolean hasStart() { 1352 return this.start != null && !this.start.isEmpty(); 1353 } 1354 1355 /** 1356 * @param value {@link #start} (Date/Time that the appointment is to take 1357 * place.). This is the underlying object with id, value and 1358 * extensions. The accessor "getStart" gives direct access to the 1359 * value 1360 */ 1361 public Appointment setStartElement(InstantType value) { 1362 this.start = value; 1363 return this; 1364 } 1365 1366 /** 1367 * @return Date/Time that the appointment is to take place. 1368 */ 1369 public Date getStart() { 1370 return this.start == null ? null : this.start.getValue(); 1371 } 1372 1373 /** 1374 * @param value Date/Time that the appointment is to take place. 1375 */ 1376 public Appointment setStart(Date value) { 1377 if (value == null) 1378 this.start = null; 1379 else { 1380 if (this.start == null) 1381 this.start = new InstantType(); 1382 this.start.setValue(value); 1383 } 1384 return this; 1385 } 1386 1387 /** 1388 * @return {@link #end} (Date/Time that the appointment is to conclude.). This 1389 * is the underlying object with id, value and extensions. The accessor 1390 * "getEnd" gives direct access to the value 1391 */ 1392 public InstantType getEndElement() { 1393 if (this.end == null) 1394 if (Configuration.errorOnAutoCreate()) 1395 throw new Error("Attempt to auto-create Appointment.end"); 1396 else if (Configuration.doAutoCreate()) 1397 this.end = new InstantType(); // bb 1398 return this.end; 1399 } 1400 1401 public boolean hasEndElement() { 1402 return this.end != null && !this.end.isEmpty(); 1403 } 1404 1405 public boolean hasEnd() { 1406 return this.end != null && !this.end.isEmpty(); 1407 } 1408 1409 /** 1410 * @param value {@link #end} (Date/Time that the appointment is to conclude.). 1411 * This is the underlying object with id, value and extensions. The 1412 * accessor "getEnd" gives direct access to the value 1413 */ 1414 public Appointment setEndElement(InstantType value) { 1415 this.end = value; 1416 return this; 1417 } 1418 1419 /** 1420 * @return Date/Time that the appointment is to conclude. 1421 */ 1422 public Date getEnd() { 1423 return this.end == null ? null : this.end.getValue(); 1424 } 1425 1426 /** 1427 * @param value Date/Time that the appointment is to conclude. 1428 */ 1429 public Appointment setEnd(Date value) { 1430 if (value == null) 1431 this.end = null; 1432 else { 1433 if (this.end == null) 1434 this.end = new InstantType(); 1435 this.end.setValue(value); 1436 } 1437 return this; 1438 } 1439 1440 /** 1441 * @return {@link #minutesDuration} (Number of minutes that the appointment is 1442 * to take. This can be less than the duration between the start and end 1443 * times (where actual time of appointment is only an estimate or is a 1444 * planned appointment request).). This is the underlying object with 1445 * id, value and extensions. The accessor "getMinutesDuration" gives 1446 * direct access to the value 1447 */ 1448 public PositiveIntType getMinutesDurationElement() { 1449 if (this.minutesDuration == null) 1450 if (Configuration.errorOnAutoCreate()) 1451 throw new Error("Attempt to auto-create Appointment.minutesDuration"); 1452 else if (Configuration.doAutoCreate()) 1453 this.minutesDuration = new PositiveIntType(); // bb 1454 return this.minutesDuration; 1455 } 1456 1457 public boolean hasMinutesDurationElement() { 1458 return this.minutesDuration != null && !this.minutesDuration.isEmpty(); 1459 } 1460 1461 public boolean hasMinutesDuration() { 1462 return this.minutesDuration != null && !this.minutesDuration.isEmpty(); 1463 } 1464 1465 /** 1466 * @param value {@link #minutesDuration} (Number of minutes that the appointment 1467 * is to take. This can be less than the duration between the start 1468 * and end times (where actual time of appointment is only an 1469 * estimate or is a planned appointment request).). This is the 1470 * underlying object with id, value and extensions. The accessor 1471 * "getMinutesDuration" gives direct access to the value 1472 */ 1473 public Appointment setMinutesDurationElement(PositiveIntType value) { 1474 this.minutesDuration = value; 1475 return this; 1476 } 1477 1478 /** 1479 * @return Number of minutes that the appointment is to take. This can be less 1480 * than the duration between the start and end times (where actual time 1481 * of appointment is only an estimate or is a planned appointment 1482 * request). 1483 */ 1484 public int getMinutesDuration() { 1485 return this.minutesDuration == null || this.minutesDuration.isEmpty() ? 0 : this.minutesDuration.getValue(); 1486 } 1487 1488 /** 1489 * @param value Number of minutes that the appointment is to take. This can be 1490 * less than the duration between the start and end times (where 1491 * actual time of appointment is only an estimate or is a planned 1492 * appointment request). 1493 */ 1494 public Appointment setMinutesDuration(int value) { 1495 if (this.minutesDuration == null) 1496 this.minutesDuration = new PositiveIntType(); 1497 this.minutesDuration.setValue(value); 1498 return this; 1499 } 1500 1501 /** 1502 * @return {@link #slot} (The slot that this appointment is filling. If provided 1503 * then the schedule will not be provided as slots are not recursive, 1504 * and the start/end values MUST be the same as from the slot.) 1505 */ 1506 public List<Reference> getSlot() { 1507 if (this.slot == null) 1508 this.slot = new ArrayList<Reference>(); 1509 return this.slot; 1510 } 1511 1512 public boolean hasSlot() { 1513 if (this.slot == null) 1514 return false; 1515 for (Reference item : this.slot) 1516 if (!item.isEmpty()) 1517 return true; 1518 return false; 1519 } 1520 1521 /** 1522 * @return {@link #slot} (The slot that this appointment is filling. If provided 1523 * then the schedule will not be provided as slots are not recursive, 1524 * and the start/end values MUST be the same as from the slot.) 1525 */ 1526 // syntactic sugar 1527 public Reference addSlot() { // 3 1528 Reference t = new Reference(); 1529 if (this.slot == null) 1530 this.slot = new ArrayList<Reference>(); 1531 this.slot.add(t); 1532 return t; 1533 } 1534 1535 // syntactic sugar 1536 public Appointment addSlot(Reference t) { // 3 1537 if (t == null) 1538 return this; 1539 if (this.slot == null) 1540 this.slot = new ArrayList<Reference>(); 1541 this.slot.add(t); 1542 return this; 1543 } 1544 1545 /** 1546 * @return {@link #slot} (The actual objects that are the target of the 1547 * reference. The reference library doesn't populate this, but you can 1548 * use this to hold the resources if you resolvethemt. The slot that 1549 * this appointment is filling. If provided then the schedule will not 1550 * be provided as slots are not recursive, and the start/end values MUST 1551 * be the same as from the slot.) 1552 */ 1553 public List<Slot> getSlotTarget() { 1554 if (this.slotTarget == null) 1555 this.slotTarget = new ArrayList<Slot>(); 1556 return this.slotTarget; 1557 } 1558 1559 // syntactic sugar 1560 /** 1561 * @return {@link #slot} (Add an actual object that is the target of the 1562 * reference. The reference library doesn't use these, but you can use 1563 * this to hold the resources if you resolvethemt. The slot that this 1564 * appointment is filling. If provided then the schedule will not be 1565 * provided as slots are not recursive, and the start/end values MUST be 1566 * the same as from the slot.) 1567 */ 1568 public Slot addSlotTarget() { 1569 Slot r = new Slot(); 1570 if (this.slotTarget == null) 1571 this.slotTarget = new ArrayList<Slot>(); 1572 this.slotTarget.add(r); 1573 return r; 1574 } 1575 1576 /** 1577 * @return {@link #comment} (Additional comments about the appointment.). This 1578 * is the underlying object with id, value and extensions. The accessor 1579 * "getComment" gives direct access to the value 1580 */ 1581 public StringType getCommentElement() { 1582 if (this.comment == null) 1583 if (Configuration.errorOnAutoCreate()) 1584 throw new Error("Attempt to auto-create Appointment.comment"); 1585 else if (Configuration.doAutoCreate()) 1586 this.comment = new StringType(); // bb 1587 return this.comment; 1588 } 1589 1590 public boolean hasCommentElement() { 1591 return this.comment != null && !this.comment.isEmpty(); 1592 } 1593 1594 public boolean hasComment() { 1595 return this.comment != null && !this.comment.isEmpty(); 1596 } 1597 1598 /** 1599 * @param value {@link #comment} (Additional comments about the appointment.). 1600 * This is the underlying object with id, value and extensions. The 1601 * accessor "getComment" gives direct access to the value 1602 */ 1603 public Appointment setCommentElement(StringType value) { 1604 this.comment = value; 1605 return this; 1606 } 1607 1608 /** 1609 * @return Additional comments about the appointment. 1610 */ 1611 public String getComment() { 1612 return this.comment == null ? null : this.comment.getValue(); 1613 } 1614 1615 /** 1616 * @param value Additional comments about the appointment. 1617 */ 1618 public Appointment setComment(String value) { 1619 if (Utilities.noString(value)) 1620 this.comment = null; 1621 else { 1622 if (this.comment == null) 1623 this.comment = new StringType(); 1624 this.comment.setValue(value); 1625 } 1626 return this; 1627 } 1628 1629 /** 1630 * @return {@link #participant} (List of participants involved in the 1631 * appointment.) 1632 */ 1633 public List<AppointmentParticipantComponent> getParticipant() { 1634 if (this.participant == null) 1635 this.participant = new ArrayList<AppointmentParticipantComponent>(); 1636 return this.participant; 1637 } 1638 1639 public boolean hasParticipant() { 1640 if (this.participant == null) 1641 return false; 1642 for (AppointmentParticipantComponent item : this.participant) 1643 if (!item.isEmpty()) 1644 return true; 1645 return false; 1646 } 1647 1648 /** 1649 * @return {@link #participant} (List of participants involved in the 1650 * appointment.) 1651 */ 1652 // syntactic sugar 1653 public AppointmentParticipantComponent addParticipant() { // 3 1654 AppointmentParticipantComponent t = new AppointmentParticipantComponent(); 1655 if (this.participant == null) 1656 this.participant = new ArrayList<AppointmentParticipantComponent>(); 1657 this.participant.add(t); 1658 return t; 1659 } 1660 1661 // syntactic sugar 1662 public Appointment addParticipant(AppointmentParticipantComponent t) { // 3 1663 if (t == null) 1664 return this; 1665 if (this.participant == null) 1666 this.participant = new ArrayList<AppointmentParticipantComponent>(); 1667 this.participant.add(t); 1668 return this; 1669 } 1670 1671 protected void listChildren(List<Property> childrenList) { 1672 super.listChildren(childrenList); 1673 childrenList.add(new Property("identifier", "Identifier", 1674 "This records identifiers associated with this appointment concern that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 1675 0, java.lang.Integer.MAX_VALUE, identifier)); 1676 childrenList.add(new Property("status", "code", 1677 "The overall status of the Appointment. Each of the participants has their own participation status which indicates their involvement in the process, however this status indicates the shared status.", 1678 0, java.lang.Integer.MAX_VALUE, status)); 1679 childrenList.add(new Property("type", "CodeableConcept", 1680 "The type of appointment that is being booked (This may also be associated with participants for location, and/or a HealthcareService).", 1681 0, java.lang.Integer.MAX_VALUE, type)); 1682 childrenList.add(new Property("reason", "CodeableConcept", 1683 "The reason that this appointment is being scheduled. This is more clinical than administrative.", 0, 1684 java.lang.Integer.MAX_VALUE, reason)); 1685 childrenList.add(new Property("priority", "unsignedInt", 1686 "The priority of the appointment. Can be used to make informed decisions if needing to re-prioritize appointments. (The iCal Standard specifies 0 as undefined, 1 as highest, 9 as lowest priority).", 1687 0, java.lang.Integer.MAX_VALUE, priority)); 1688 childrenList.add(new Property("description", "string", 1689 "The brief description of the appointment as would be shown on a subject line in a meeting request, or appointment list. Detailed or expanded information should be put in the comment field.", 1690 0, java.lang.Integer.MAX_VALUE, description)); 1691 childrenList.add(new Property("start", "instant", "Date/Time that the appointment is to take place.", 0, 1692 java.lang.Integer.MAX_VALUE, start)); 1693 childrenList.add(new Property("end", "instant", "Date/Time that the appointment is to conclude.", 0, 1694 java.lang.Integer.MAX_VALUE, end)); 1695 childrenList.add(new Property("minutesDuration", "positiveInt", 1696 "Number of minutes that the appointment is to take. This can be less than the duration between the start and end times (where actual time of appointment is only an estimate or is a planned appointment request).", 1697 0, java.lang.Integer.MAX_VALUE, minutesDuration)); 1698 childrenList.add(new Property("slot", "Reference(Slot)", 1699 "The slot that this appointment is filling. If provided then the schedule will not be provided as slots are not recursive, and the start/end values MUST be the same as from the slot.", 1700 0, java.lang.Integer.MAX_VALUE, slot)); 1701 childrenList.add(new Property("comment", "string", "Additional comments about the appointment.", 0, 1702 java.lang.Integer.MAX_VALUE, comment)); 1703 childrenList.add(new Property("participant", "", "List of participants involved in the appointment.", 0, 1704 java.lang.Integer.MAX_VALUE, participant)); 1705 } 1706 1707 @Override 1708 public void setProperty(String name, Base value) throws FHIRException { 1709 if (name.equals("identifier")) 1710 this.getIdentifier().add(castToIdentifier(value)); 1711 else if (name.equals("status")) 1712 this.status = new AppointmentStatusEnumFactory().fromType(value); // Enumeration<AppointmentStatus> 1713 else if (name.equals("type")) 1714 this.type = castToCodeableConcept(value); // CodeableConcept 1715 else if (name.equals("reason")) 1716 this.reason = castToCodeableConcept(value); // CodeableConcept 1717 else if (name.equals("priority")) 1718 this.priority = castToUnsignedInt(value); // UnsignedIntType 1719 else if (name.equals("description")) 1720 this.description = castToString(value); // StringType 1721 else if (name.equals("start")) 1722 this.start = castToInstant(value); // InstantType 1723 else if (name.equals("end")) 1724 this.end = castToInstant(value); // InstantType 1725 else if (name.equals("minutesDuration")) 1726 this.minutesDuration = castToPositiveInt(value); // PositiveIntType 1727 else if (name.equals("slot")) 1728 this.getSlot().add(castToReference(value)); 1729 else if (name.equals("comment")) 1730 this.comment = castToString(value); // StringType 1731 else if (name.equals("participant")) 1732 this.getParticipant().add((AppointmentParticipantComponent) value); 1733 else 1734 super.setProperty(name, value); 1735 } 1736 1737 @Override 1738 public Base addChild(String name) throws FHIRException { 1739 if (name.equals("identifier")) { 1740 return addIdentifier(); 1741 } else if (name.equals("status")) { 1742 throw new FHIRException("Cannot call addChild on a singleton property Appointment.status"); 1743 } else if (name.equals("type")) { 1744 this.type = new CodeableConcept(); 1745 return this.type; 1746 } else if (name.equals("reason")) { 1747 this.reason = new CodeableConcept(); 1748 return this.reason; 1749 } else if (name.equals("priority")) { 1750 throw new FHIRException("Cannot call addChild on a singleton property Appointment.priority"); 1751 } else if (name.equals("description")) { 1752 throw new FHIRException("Cannot call addChild on a singleton property Appointment.description"); 1753 } else if (name.equals("start")) { 1754 throw new FHIRException("Cannot call addChild on a singleton property Appointment.start"); 1755 } else if (name.equals("end")) { 1756 throw new FHIRException("Cannot call addChild on a singleton property Appointment.end"); 1757 } else if (name.equals("minutesDuration")) { 1758 throw new FHIRException("Cannot call addChild on a singleton property Appointment.minutesDuration"); 1759 } else if (name.equals("slot")) { 1760 return addSlot(); 1761 } else if (name.equals("comment")) { 1762 throw new FHIRException("Cannot call addChild on a singleton property Appointment.comment"); 1763 } else if (name.equals("participant")) { 1764 return addParticipant(); 1765 } else 1766 return super.addChild(name); 1767 } 1768 1769 public String fhirType() { 1770 return "Appointment"; 1771 1772 } 1773 1774 public Appointment copy() { 1775 Appointment dst = new Appointment(); 1776 copyValues(dst); 1777 if (identifier != null) { 1778 dst.identifier = new ArrayList<Identifier>(); 1779 for (Identifier i : identifier) 1780 dst.identifier.add(i.copy()); 1781 } 1782 ; 1783 dst.status = status == null ? null : status.copy(); 1784 dst.type = type == null ? null : type.copy(); 1785 dst.reason = reason == null ? null : reason.copy(); 1786 dst.priority = priority == null ? null : priority.copy(); 1787 dst.description = description == null ? null : description.copy(); 1788 dst.start = start == null ? null : start.copy(); 1789 dst.end = end == null ? null : end.copy(); 1790 dst.minutesDuration = minutesDuration == null ? null : minutesDuration.copy(); 1791 if (slot != null) { 1792 dst.slot = new ArrayList<Reference>(); 1793 for (Reference i : slot) 1794 dst.slot.add(i.copy()); 1795 } 1796 ; 1797 dst.comment = comment == null ? null : comment.copy(); 1798 if (participant != null) { 1799 dst.participant = new ArrayList<AppointmentParticipantComponent>(); 1800 for (AppointmentParticipantComponent i : participant) 1801 dst.participant.add(i.copy()); 1802 } 1803 ; 1804 return dst; 1805 } 1806 1807 protected Appointment typedCopy() { 1808 return copy(); 1809 } 1810 1811 @Override 1812 public boolean equalsDeep(Base other) { 1813 if (!super.equalsDeep(other)) 1814 return false; 1815 if (!(other instanceof Appointment)) 1816 return false; 1817 Appointment o = (Appointment) other; 1818 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) 1819 && compareDeep(type, o.type, true) && compareDeep(reason, o.reason, true) 1820 && compareDeep(priority, o.priority, true) && compareDeep(description, o.description, true) 1821 && compareDeep(start, o.start, true) && compareDeep(end, o.end, true) 1822 && compareDeep(minutesDuration, o.minutesDuration, true) && compareDeep(slot, o.slot, true) 1823 && compareDeep(comment, o.comment, true) && compareDeep(participant, o.participant, true); 1824 } 1825 1826 @Override 1827 public boolean equalsShallow(Base other) { 1828 if (!super.equalsShallow(other)) 1829 return false; 1830 if (!(other instanceof Appointment)) 1831 return false; 1832 Appointment o = (Appointment) other; 1833 return compareValues(status, o.status, true) && compareValues(priority, o.priority, true) 1834 && compareValues(description, o.description, true) && compareValues(start, o.start, true) 1835 && compareValues(end, o.end, true) && compareValues(minutesDuration, o.minutesDuration, true) 1836 && compareValues(comment, o.comment, true); 1837 } 1838 1839 public boolean isEmpty() { 1840 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (status == null || status.isEmpty()) 1841 && (type == null || type.isEmpty()) && (reason == null || reason.isEmpty()) 1842 && (priority == null || priority.isEmpty()) && (description == null || description.isEmpty()) 1843 && (start == null || start.isEmpty()) && (end == null || end.isEmpty()) 1844 && (minutesDuration == null || minutesDuration.isEmpty()) && (slot == null || slot.isEmpty()) 1845 && (comment == null || comment.isEmpty()) && (participant == null || participant.isEmpty()); 1846 } 1847 1848 @Override 1849 public ResourceType getResourceType() { 1850 return ResourceType.Appointment; 1851 } 1852 1853 @SearchParamDefinition(name = "date", path = "Appointment.start", description = "Appointment date/time.", type = "date") 1854 public static final String SP_DATE = "date"; 1855 @SearchParamDefinition(name = "actor", path = "Appointment.participant.actor", description = "Any one of the individuals participating in the appointment", type = "reference") 1856 public static final String SP_ACTOR = "actor"; 1857 @SearchParamDefinition(name = "identifier", path = "Appointment.identifier", description = "An Identifier of the Appointment", type = "token") 1858 public static final String SP_IDENTIFIER = "identifier"; 1859 @SearchParamDefinition(name = "practitioner", path = "Appointment.participant.actor", description = "One of the individuals of the appointment is this practitioner", type = "reference") 1860 public static final String SP_PRACTITIONER = "practitioner"; 1861 @SearchParamDefinition(name = "part-status", path = "Appointment.participant.status", description = "The Participation status of the subject, or other participant on the appointment. Can be used to locate participants that have not responded to meeting requests.", type = "token") 1862 public static final String SP_PARTSTATUS = "part-status"; 1863 @SearchParamDefinition(name = "patient", path = "Appointment.participant.actor", description = "One of the individuals of the appointment is this patient", type = "reference") 1864 public static final String SP_PATIENT = "patient"; 1865 @SearchParamDefinition(name = "location", path = "Appointment.participant.actor", description = "This location is listed in the participants of the appointment", type = "reference") 1866 public static final String SP_LOCATION = "location"; 1867 @SearchParamDefinition(name = "status", path = "Appointment.status", description = "The overall status of the appointment", type = "token") 1868 public static final String SP_STATUS = "status"; 1869 1870}