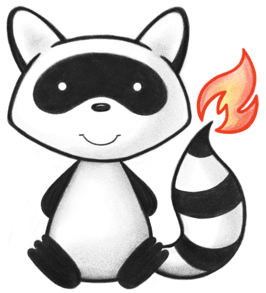
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.utilities.Utilities; 043 044/** 045 * A reply to an appointment request for a patient and/or practitioner(s), such 046 * as a confirmation or rejection. 047 */ 048@ResourceDef(name = "AppointmentResponse", profile = "http://hl7.org/fhir/Profile/AppointmentResponse") 049public class AppointmentResponse extends DomainResource { 050 051 public enum ParticipantStatus { 052 /** 053 * The appointment participant has accepted that they can attend the appointment 054 * at the time specified in the AppointmentResponse. 055 */ 056 ACCEPTED, 057 /** 058 * The appointment participant has declined the appointment. 059 */ 060 DECLINED, 061 /** 062 * The appointment participant has tentatively accepted the appointment. 063 */ 064 TENTATIVE, 065 /** 066 * The participant has in-process the appointment. 067 */ 068 INPROCESS, 069 /** 070 * The participant has completed the appointment. 071 */ 072 COMPLETED, 073 /** 074 * This is the intitial status of an appointment participant until a participant 075 * has replied. It implies that there is no commitment for the appointment. 076 */ 077 NEEDSACTION, 078 /** 079 * added to help the parsers 080 */ 081 NULL; 082 083 public static ParticipantStatus fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("accepted".equals(codeString)) 087 return ACCEPTED; 088 if ("declined".equals(codeString)) 089 return DECLINED; 090 if ("tentative".equals(codeString)) 091 return TENTATIVE; 092 if ("in-process".equals(codeString)) 093 return INPROCESS; 094 if ("completed".equals(codeString)) 095 return COMPLETED; 096 if ("needs-action".equals(codeString)) 097 return NEEDSACTION; 098 throw new FHIRException("Unknown ParticipantStatus code '" + codeString + "'"); 099 } 100 101 public String toCode() { 102 switch (this) { 103 case ACCEPTED: 104 return "accepted"; 105 case DECLINED: 106 return "declined"; 107 case TENTATIVE: 108 return "tentative"; 109 case INPROCESS: 110 return "in-process"; 111 case COMPLETED: 112 return "completed"; 113 case NEEDSACTION: 114 return "needs-action"; 115 case NULL: 116 return null; 117 default: 118 return "?"; 119 } 120 } 121 122 public String getSystem() { 123 switch (this) { 124 case ACCEPTED: 125 return "http://hl7.org/fhir/participantstatus"; 126 case DECLINED: 127 return "http://hl7.org/fhir/participantstatus"; 128 case TENTATIVE: 129 return "http://hl7.org/fhir/participantstatus"; 130 case INPROCESS: 131 return "http://hl7.org/fhir/participantstatus"; 132 case COMPLETED: 133 return "http://hl7.org/fhir/participantstatus"; 134 case NEEDSACTION: 135 return "http://hl7.org/fhir/participantstatus"; 136 case NULL: 137 return null; 138 default: 139 return "?"; 140 } 141 } 142 143 public String getDefinition() { 144 switch (this) { 145 case ACCEPTED: 146 return "The appointment participant has accepted that they can attend the appointment at the time specified in the AppointmentResponse."; 147 case DECLINED: 148 return "The appointment participant has declined the appointment."; 149 case TENTATIVE: 150 return "The appointment participant has tentatively accepted the appointment."; 151 case INPROCESS: 152 return "The participant has in-process the appointment."; 153 case COMPLETED: 154 return "The participant has completed the appointment."; 155 case NEEDSACTION: 156 return "This is the intitial status of an appointment participant until a participant has replied. It implies that there is no commitment for the appointment."; 157 case NULL: 158 return null; 159 default: 160 return "?"; 161 } 162 } 163 164 public String getDisplay() { 165 switch (this) { 166 case ACCEPTED: 167 return "Accepted"; 168 case DECLINED: 169 return "Declined"; 170 case TENTATIVE: 171 return "Tentative"; 172 case INPROCESS: 173 return "In Process"; 174 case COMPLETED: 175 return "Completed"; 176 case NEEDSACTION: 177 return "Needs Action"; 178 case NULL: 179 return null; 180 default: 181 return "?"; 182 } 183 } 184 } 185 186 public static class ParticipantStatusEnumFactory implements EnumFactory<ParticipantStatus> { 187 public ParticipantStatus fromCode(String codeString) throws IllegalArgumentException { 188 if (codeString == null || "".equals(codeString)) 189 if (codeString == null || "".equals(codeString)) 190 return null; 191 if ("accepted".equals(codeString)) 192 return ParticipantStatus.ACCEPTED; 193 if ("declined".equals(codeString)) 194 return ParticipantStatus.DECLINED; 195 if ("tentative".equals(codeString)) 196 return ParticipantStatus.TENTATIVE; 197 if ("in-process".equals(codeString)) 198 return ParticipantStatus.INPROCESS; 199 if ("completed".equals(codeString)) 200 return ParticipantStatus.COMPLETED; 201 if ("needs-action".equals(codeString)) 202 return ParticipantStatus.NEEDSACTION; 203 throw new IllegalArgumentException("Unknown ParticipantStatus code '" + codeString + "'"); 204 } 205 206 public Enumeration<ParticipantStatus> fromType(Base code) throws FHIRException { 207 if (code == null || code.isEmpty()) 208 return null; 209 String codeString = ((PrimitiveType) code).asStringValue(); 210 if (codeString == null || "".equals(codeString)) 211 return null; 212 if ("accepted".equals(codeString)) 213 return new Enumeration<ParticipantStatus>(this, ParticipantStatus.ACCEPTED); 214 if ("declined".equals(codeString)) 215 return new Enumeration<ParticipantStatus>(this, ParticipantStatus.DECLINED); 216 if ("tentative".equals(codeString)) 217 return new Enumeration<ParticipantStatus>(this, ParticipantStatus.TENTATIVE); 218 if ("in-process".equals(codeString)) 219 return new Enumeration<ParticipantStatus>(this, ParticipantStatus.INPROCESS); 220 if ("completed".equals(codeString)) 221 return new Enumeration<ParticipantStatus>(this, ParticipantStatus.COMPLETED); 222 if ("needs-action".equals(codeString)) 223 return new Enumeration<ParticipantStatus>(this, ParticipantStatus.NEEDSACTION); 224 throw new FHIRException("Unknown ParticipantStatus code '" + codeString + "'"); 225 } 226 227 public String toCode(ParticipantStatus code) 228 { 229 if (code == ParticipantStatus.NULL) 230 return null; 231 if (code == ParticipantStatus.ACCEPTED) 232 return "accepted"; 233 if (code == ParticipantStatus.DECLINED) 234 return "declined"; 235 if (code == ParticipantStatus.TENTATIVE) 236 return "tentative"; 237 if (code == ParticipantStatus.INPROCESS) 238 return "in-process"; 239 if (code == ParticipantStatus.COMPLETED) 240 return "completed"; 241 if (code == ParticipantStatus.NEEDSACTION) 242 return "needs-action"; 243 return "?"; 244 } 245 } 246 247 /** 248 * This records identifiers associated with this appointment response concern 249 * that are defined by business processes and/ or used to refer to it when a 250 * direct URL reference to the resource itself is not appropriate. 251 */ 252 @Child(name = "identifier", type = { 253 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 254 @Description(shortDefinition = "External Ids for this item", formalDefinition = "This records identifiers associated with this appointment response concern that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate.") 255 protected List<Identifier> identifier; 256 257 /** 258 * Appointment that this response is replying to. 259 */ 260 @Child(name = "appointment", type = { 261 Appointment.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 262 @Description(shortDefinition = "Appointment this response relates to", formalDefinition = "Appointment that this response is replying to.") 263 protected Reference appointment; 264 265 /** 266 * The actual object that is the target of the reference (Appointment that this 267 * response is replying to.) 268 */ 269 protected Appointment appointmentTarget; 270 271 /** 272 * Date/Time that the appointment is to take place, or requested new start time. 273 */ 274 @Child(name = "start", type = { InstantType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 275 @Description(shortDefinition = "Time from appointment, or requested new start time", formalDefinition = "Date/Time that the appointment is to take place, or requested new start time.") 276 protected InstantType start; 277 278 /** 279 * This may be either the same as the appointment request to confirm the details 280 * of the appointment, or alternately a new time to request a re-negotiation of 281 * the end time. 282 */ 283 @Child(name = "end", type = { InstantType.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 284 @Description(shortDefinition = "Time from appointment, or requested new end time", formalDefinition = "This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time.") 285 protected InstantType end; 286 287 /** 288 * Role of participant in the appointment. 289 */ 290 @Child(name = "participantType", type = { 291 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 292 @Description(shortDefinition = "Role of participant in the appointment", formalDefinition = "Role of participant in the appointment.") 293 protected List<CodeableConcept> participantType; 294 295 /** 296 * A Person, Location/HealthcareService or Device that is participating in the 297 * appointment. 298 */ 299 @Child(name = "actor", type = { Patient.class, Practitioner.class, RelatedPerson.class, Device.class, 300 HealthcareService.class, Location.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 301 @Description(shortDefinition = "Person, Location/HealthcareService or Device", formalDefinition = "A Person, Location/HealthcareService or Device that is participating in the appointment.") 302 protected Reference actor; 303 304 /** 305 * The actual object that is the target of the reference (A Person, 306 * Location/HealthcareService or Device that is participating in the 307 * appointment.) 308 */ 309 protected Resource actorTarget; 310 311 /** 312 * Participation status of the participant. When the status is declined or 313 * tentative if the start/end times are different to the appointment, then these 314 * times should be interpreted as a requested time change. When the status is 315 * accepted, the times can either be the time of the appointment (as a 316 * confirmation of the time) or can be empty. 317 */ 318 @Child(name = "participantStatus", type = { 319 CodeType.class }, order = 6, min = 1, max = 1, modifier = true, summary = true) 320 @Description(shortDefinition = "accepted | declined | tentative | in-process | completed | needs-action", formalDefinition = "Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty.") 321 protected Enumeration<ParticipantStatus> participantStatus; 322 323 /** 324 * Additional comments about the appointment. 325 */ 326 @Child(name = "comment", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 327 @Description(shortDefinition = "Additional comments", formalDefinition = "Additional comments about the appointment.") 328 protected StringType comment; 329 330 private static final long serialVersionUID = 248548635L; 331 332 /* 333 * Constructor 334 */ 335 public AppointmentResponse() { 336 super(); 337 } 338 339 /* 340 * Constructor 341 */ 342 public AppointmentResponse(Reference appointment, Enumeration<ParticipantStatus> participantStatus) { 343 super(); 344 this.appointment = appointment; 345 this.participantStatus = participantStatus; 346 } 347 348 /** 349 * @return {@link #identifier} (This records identifiers associated with this 350 * appointment response concern that are defined by business processes 351 * and/ or used to refer to it when a direct URL reference to the 352 * resource itself is not appropriate.) 353 */ 354 public List<Identifier> getIdentifier() { 355 if (this.identifier == null) 356 this.identifier = new ArrayList<Identifier>(); 357 return this.identifier; 358 } 359 360 public boolean hasIdentifier() { 361 if (this.identifier == null) 362 return false; 363 for (Identifier item : this.identifier) 364 if (!item.isEmpty()) 365 return true; 366 return false; 367 } 368 369 /** 370 * @return {@link #identifier} (This records identifiers associated with this 371 * appointment response concern that are defined by business processes 372 * and/ or used to refer to it when a direct URL reference to the 373 * resource itself is not appropriate.) 374 */ 375 // syntactic sugar 376 public Identifier addIdentifier() { // 3 377 Identifier t = new Identifier(); 378 if (this.identifier == null) 379 this.identifier = new ArrayList<Identifier>(); 380 this.identifier.add(t); 381 return t; 382 } 383 384 // syntactic sugar 385 public AppointmentResponse addIdentifier(Identifier t) { // 3 386 if (t == null) 387 return this; 388 if (this.identifier == null) 389 this.identifier = new ArrayList<Identifier>(); 390 this.identifier.add(t); 391 return this; 392 } 393 394 /** 395 * @return {@link #appointment} (Appointment that this response is replying to.) 396 */ 397 public Reference getAppointment() { 398 if (this.appointment == null) 399 if (Configuration.errorOnAutoCreate()) 400 throw new Error("Attempt to auto-create AppointmentResponse.appointment"); 401 else if (Configuration.doAutoCreate()) 402 this.appointment = new Reference(); // cc 403 return this.appointment; 404 } 405 406 public boolean hasAppointment() { 407 return this.appointment != null && !this.appointment.isEmpty(); 408 } 409 410 /** 411 * @param value {@link #appointment} (Appointment that this response is replying 412 * to.) 413 */ 414 public AppointmentResponse setAppointment(Reference value) { 415 this.appointment = value; 416 return this; 417 } 418 419 /** 420 * @return {@link #appointment} The actual object that is the target of the 421 * reference. The reference library doesn't populate this, but you can 422 * use it to hold the resource if you resolve it. (Appointment that this 423 * response is replying to.) 424 */ 425 public Appointment getAppointmentTarget() { 426 if (this.appointmentTarget == null) 427 if (Configuration.errorOnAutoCreate()) 428 throw new Error("Attempt to auto-create AppointmentResponse.appointment"); 429 else if (Configuration.doAutoCreate()) 430 this.appointmentTarget = new Appointment(); // aa 431 return this.appointmentTarget; 432 } 433 434 /** 435 * @param value {@link #appointment} The actual object that is the target of the 436 * reference. The reference library doesn't use these, but you can 437 * use it to hold the resource if you resolve it. (Appointment that 438 * this response is replying to.) 439 */ 440 public AppointmentResponse setAppointmentTarget(Appointment value) { 441 this.appointmentTarget = value; 442 return this; 443 } 444 445 /** 446 * @return {@link #start} (Date/Time that the appointment is to take place, or 447 * requested new start time.). This is the underlying object with id, 448 * value and extensions. The accessor "getStart" gives direct access to 449 * the value 450 */ 451 public InstantType getStartElement() { 452 if (this.start == null) 453 if (Configuration.errorOnAutoCreate()) 454 throw new Error("Attempt to auto-create AppointmentResponse.start"); 455 else if (Configuration.doAutoCreate()) 456 this.start = new InstantType(); // bb 457 return this.start; 458 } 459 460 public boolean hasStartElement() { 461 return this.start != null && !this.start.isEmpty(); 462 } 463 464 public boolean hasStart() { 465 return this.start != null && !this.start.isEmpty(); 466 } 467 468 /** 469 * @param value {@link #start} (Date/Time that the appointment is to take place, 470 * or requested new start time.). This is the underlying object 471 * with id, value and extensions. The accessor "getStart" gives 472 * direct access to the value 473 */ 474 public AppointmentResponse setStartElement(InstantType value) { 475 this.start = value; 476 return this; 477 } 478 479 /** 480 * @return Date/Time that the appointment is to take place, or requested new 481 * start time. 482 */ 483 public Date getStart() { 484 return this.start == null ? null : this.start.getValue(); 485 } 486 487 /** 488 * @param value Date/Time that the appointment is to take place, or requested 489 * new start time. 490 */ 491 public AppointmentResponse setStart(Date value) { 492 if (value == null) 493 this.start = null; 494 else { 495 if (this.start == null) 496 this.start = new InstantType(); 497 this.start.setValue(value); 498 } 499 return this; 500 } 501 502 /** 503 * @return {@link #end} (This may be either the same as the appointment request 504 * to confirm the details of the appointment, or alternately a new time 505 * to request a re-negotiation of the end time.). This is the underlying 506 * object with id, value and extensions. The accessor "getEnd" gives 507 * direct access to the value 508 */ 509 public InstantType getEndElement() { 510 if (this.end == null) 511 if (Configuration.errorOnAutoCreate()) 512 throw new Error("Attempt to auto-create AppointmentResponse.end"); 513 else if (Configuration.doAutoCreate()) 514 this.end = new InstantType(); // bb 515 return this.end; 516 } 517 518 public boolean hasEndElement() { 519 return this.end != null && !this.end.isEmpty(); 520 } 521 522 public boolean hasEnd() { 523 return this.end != null && !this.end.isEmpty(); 524 } 525 526 /** 527 * @param value {@link #end} (This may be either the same as the appointment 528 * request to confirm the details of the appointment, or 529 * alternately a new time to request a re-negotiation of the end 530 * time.). This is the underlying object with id, value and 531 * extensions. The accessor "getEnd" gives direct access to the 532 * value 533 */ 534 public AppointmentResponse setEndElement(InstantType value) { 535 this.end = value; 536 return this; 537 } 538 539 /** 540 * @return This may be either the same as the appointment request to confirm the 541 * details of the appointment, or alternately a new time to request a 542 * re-negotiation of the end time. 543 */ 544 public Date getEnd() { 545 return this.end == null ? null : this.end.getValue(); 546 } 547 548 /** 549 * @param value This may be either the same as the appointment request to 550 * confirm the details of the appointment, or alternately a new 551 * time to request a re-negotiation of the end time. 552 */ 553 public AppointmentResponse setEnd(Date value) { 554 if (value == null) 555 this.end = null; 556 else { 557 if (this.end == null) 558 this.end = new InstantType(); 559 this.end.setValue(value); 560 } 561 return this; 562 } 563 564 /** 565 * @return {@link #participantType} (Role of participant in the appointment.) 566 */ 567 public List<CodeableConcept> getParticipantType() { 568 if (this.participantType == null) 569 this.participantType = new ArrayList<CodeableConcept>(); 570 return this.participantType; 571 } 572 573 public boolean hasParticipantType() { 574 if (this.participantType == null) 575 return false; 576 for (CodeableConcept item : this.participantType) 577 if (!item.isEmpty()) 578 return true; 579 return false; 580 } 581 582 /** 583 * @return {@link #participantType} (Role of participant in the appointment.) 584 */ 585 // syntactic sugar 586 public CodeableConcept addParticipantType() { // 3 587 CodeableConcept t = new CodeableConcept(); 588 if (this.participantType == null) 589 this.participantType = new ArrayList<CodeableConcept>(); 590 this.participantType.add(t); 591 return t; 592 } 593 594 // syntactic sugar 595 public AppointmentResponse addParticipantType(CodeableConcept t) { // 3 596 if (t == null) 597 return this; 598 if (this.participantType == null) 599 this.participantType = new ArrayList<CodeableConcept>(); 600 this.participantType.add(t); 601 return this; 602 } 603 604 /** 605 * @return {@link #actor} (A Person, Location/HealthcareService or Device that 606 * is participating in the appointment.) 607 */ 608 public Reference getActor() { 609 if (this.actor == null) 610 if (Configuration.errorOnAutoCreate()) 611 throw new Error("Attempt to auto-create AppointmentResponse.actor"); 612 else if (Configuration.doAutoCreate()) 613 this.actor = new Reference(); // cc 614 return this.actor; 615 } 616 617 public boolean hasActor() { 618 return this.actor != null && !this.actor.isEmpty(); 619 } 620 621 /** 622 * @param value {@link #actor} (A Person, Location/HealthcareService or Device 623 * that is participating in the appointment.) 624 */ 625 public AppointmentResponse setActor(Reference value) { 626 this.actor = value; 627 return this; 628 } 629 630 /** 631 * @return {@link #actor} The actual object that is the target of the reference. 632 * The reference library doesn't populate this, but you can use it to 633 * hold the resource if you resolve it. (A Person, 634 * Location/HealthcareService or Device that is participating in the 635 * appointment.) 636 */ 637 public Resource getActorTarget() { 638 return this.actorTarget; 639 } 640 641 /** 642 * @param value {@link #actor} The actual object that is the target of the 643 * reference. The reference library doesn't use these, but you can 644 * use it to hold the resource if you resolve it. (A Person, 645 * Location/HealthcareService or Device that is participating in 646 * the appointment.) 647 */ 648 public AppointmentResponse setActorTarget(Resource value) { 649 this.actorTarget = value; 650 return this; 651 } 652 653 /** 654 * @return {@link #participantStatus} (Participation status of the participant. 655 * When the status is declined or tentative if the start/end times are 656 * different to the appointment, then these times should be interpreted 657 * as a requested time change. When the status is accepted, the times 658 * can either be the time of the appointment (as a confirmation of the 659 * time) or can be empty.). This is the underlying object with id, value 660 * and extensions. The accessor "getParticipantStatus" gives direct 661 * access to the value 662 */ 663 public Enumeration<ParticipantStatus> getParticipantStatusElement() { 664 if (this.participantStatus == null) 665 if (Configuration.errorOnAutoCreate()) 666 throw new Error("Attempt to auto-create AppointmentResponse.participantStatus"); 667 else if (Configuration.doAutoCreate()) 668 this.participantStatus = new Enumeration<ParticipantStatus>(new ParticipantStatusEnumFactory()); // bb 669 return this.participantStatus; 670 } 671 672 public boolean hasParticipantStatusElement() { 673 return this.participantStatus != null && !this.participantStatus.isEmpty(); 674 } 675 676 public boolean hasParticipantStatus() { 677 return this.participantStatus != null && !this.participantStatus.isEmpty(); 678 } 679 680 /** 681 * @param value {@link #participantStatus} (Participation status of the 682 * participant. When the status is declined or tentative if the 683 * start/end times are different to the appointment, then these 684 * times should be interpreted as a requested time change. When the 685 * status is accepted, the times can either be the time of the 686 * appointment (as a confirmation of the time) or can be empty.). 687 * This is the underlying object with id, value and extensions. The 688 * accessor "getParticipantStatus" gives direct access to the value 689 */ 690 public AppointmentResponse setParticipantStatusElement(Enumeration<ParticipantStatus> value) { 691 this.participantStatus = value; 692 return this; 693 } 694 695 /** 696 * @return Participation status of the participant. When the status is declined 697 * or tentative if the start/end times are different to the appointment, 698 * then these times should be interpreted as a requested time change. 699 * When the status is accepted, the times can either be the time of the 700 * appointment (as a confirmation of the time) or can be empty. 701 */ 702 public ParticipantStatus getParticipantStatus() { 703 return this.participantStatus == null ? null : this.participantStatus.getValue(); 704 } 705 706 /** 707 * @param value Participation status of the participant. When the status is 708 * declined or tentative if the start/end times are different to 709 * the appointment, then these times should be interpreted as a 710 * requested time change. When the status is accepted, the times 711 * can either be the time of the appointment (as a confirmation of 712 * the time) or can be empty. 713 */ 714 public AppointmentResponse setParticipantStatus(ParticipantStatus value) { 715 if (this.participantStatus == null) 716 this.participantStatus = new Enumeration<ParticipantStatus>(new ParticipantStatusEnumFactory()); 717 this.participantStatus.setValue(value); 718 return this; 719 } 720 721 /** 722 * @return {@link #comment} (Additional comments about the appointment.). This 723 * is the underlying object with id, value and extensions. The accessor 724 * "getComment" gives direct access to the value 725 */ 726 public StringType getCommentElement() { 727 if (this.comment == null) 728 if (Configuration.errorOnAutoCreate()) 729 throw new Error("Attempt to auto-create AppointmentResponse.comment"); 730 else if (Configuration.doAutoCreate()) 731 this.comment = new StringType(); // bb 732 return this.comment; 733 } 734 735 public boolean hasCommentElement() { 736 return this.comment != null && !this.comment.isEmpty(); 737 } 738 739 public boolean hasComment() { 740 return this.comment != null && !this.comment.isEmpty(); 741 } 742 743 /** 744 * @param value {@link #comment} (Additional comments about the appointment.). 745 * This is the underlying object with id, value and extensions. The 746 * accessor "getComment" gives direct access to the value 747 */ 748 public AppointmentResponse setCommentElement(StringType value) { 749 this.comment = value; 750 return this; 751 } 752 753 /** 754 * @return Additional comments about the appointment. 755 */ 756 public String getComment() { 757 return this.comment == null ? null : this.comment.getValue(); 758 } 759 760 /** 761 * @param value Additional comments about the appointment. 762 */ 763 public AppointmentResponse setComment(String value) { 764 if (Utilities.noString(value)) 765 this.comment = null; 766 else { 767 if (this.comment == null) 768 this.comment = new StringType(); 769 this.comment.setValue(value); 770 } 771 return this; 772 } 773 774 protected void listChildren(List<Property> childrenList) { 775 super.listChildren(childrenList); 776 childrenList.add(new Property("identifier", "Identifier", 777 "This records identifiers associated with this appointment response concern that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate.", 778 0, java.lang.Integer.MAX_VALUE, identifier)); 779 childrenList.add(new Property("appointment", "Reference(Appointment)", 780 "Appointment that this response is replying to.", 0, java.lang.Integer.MAX_VALUE, appointment)); 781 childrenList.add(new Property("start", "instant", 782 "Date/Time that the appointment is to take place, or requested new start time.", 0, java.lang.Integer.MAX_VALUE, 783 start)); 784 childrenList.add(new Property("end", "instant", 785 "This may be either the same as the appointment request to confirm the details of the appointment, or alternately a new time to request a re-negotiation of the end time.", 786 0, java.lang.Integer.MAX_VALUE, end)); 787 childrenList.add(new Property("participantType", "CodeableConcept", "Role of participant in the appointment.", 0, 788 java.lang.Integer.MAX_VALUE, participantType)); 789 childrenList 790 .add(new Property("actor", "Reference(Patient|Practitioner|RelatedPerson|Device|HealthcareService|Location)", 791 "A Person, Location/HealthcareService or Device that is participating in the appointment.", 0, 792 java.lang.Integer.MAX_VALUE, actor)); 793 childrenList.add(new Property("participantStatus", "code", 794 "Participation status of the participant. When the status is declined or tentative if the start/end times are different to the appointment, then these times should be interpreted as a requested time change. When the status is accepted, the times can either be the time of the appointment (as a confirmation of the time) or can be empty.", 795 0, java.lang.Integer.MAX_VALUE, participantStatus)); 796 childrenList.add(new Property("comment", "string", "Additional comments about the appointment.", 0, 797 java.lang.Integer.MAX_VALUE, comment)); 798 } 799 800 @Override 801 public void setProperty(String name, Base value) throws FHIRException { 802 if (name.equals("identifier")) 803 this.getIdentifier().add(castToIdentifier(value)); 804 else if (name.equals("appointment")) 805 this.appointment = castToReference(value); // Reference 806 else if (name.equals("start")) 807 this.start = castToInstant(value); // InstantType 808 else if (name.equals("end")) 809 this.end = castToInstant(value); // InstantType 810 else if (name.equals("participantType")) 811 this.getParticipantType().add(castToCodeableConcept(value)); 812 else if (name.equals("actor")) 813 this.actor = castToReference(value); // Reference 814 else if (name.equals("participantStatus")) 815 this.participantStatus = new ParticipantStatusEnumFactory().fromType(value); // Enumeration<ParticipantStatus> 816 else if (name.equals("comment")) 817 this.comment = castToString(value); // StringType 818 else 819 super.setProperty(name, value); 820 } 821 822 @Override 823 public Base addChild(String name) throws FHIRException { 824 if (name.equals("identifier")) { 825 return addIdentifier(); 826 } else if (name.equals("appointment")) { 827 this.appointment = new Reference(); 828 return this.appointment; 829 } else if (name.equals("start")) { 830 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.start"); 831 } else if (name.equals("end")) { 832 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.end"); 833 } else if (name.equals("participantType")) { 834 return addParticipantType(); 835 } else if (name.equals("actor")) { 836 this.actor = new Reference(); 837 return this.actor; 838 } else if (name.equals("participantStatus")) { 839 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.participantStatus"); 840 } else if (name.equals("comment")) { 841 throw new FHIRException("Cannot call addChild on a singleton property AppointmentResponse.comment"); 842 } else 843 return super.addChild(name); 844 } 845 846 public String fhirType() { 847 return "AppointmentResponse"; 848 849 } 850 851 public AppointmentResponse copy() { 852 AppointmentResponse dst = new AppointmentResponse(); 853 copyValues(dst); 854 if (identifier != null) { 855 dst.identifier = new ArrayList<Identifier>(); 856 for (Identifier i : identifier) 857 dst.identifier.add(i.copy()); 858 } 859 ; 860 dst.appointment = appointment == null ? null : appointment.copy(); 861 dst.start = start == null ? null : start.copy(); 862 dst.end = end == null ? null : end.copy(); 863 if (participantType != null) { 864 dst.participantType = new ArrayList<CodeableConcept>(); 865 for (CodeableConcept i : participantType) 866 dst.participantType.add(i.copy()); 867 } 868 ; 869 dst.actor = actor == null ? null : actor.copy(); 870 dst.participantStatus = participantStatus == null ? null : participantStatus.copy(); 871 dst.comment = comment == null ? null : comment.copy(); 872 return dst; 873 } 874 875 protected AppointmentResponse typedCopy() { 876 return copy(); 877 } 878 879 @Override 880 public boolean equalsDeep(Base other) { 881 if (!super.equalsDeep(other)) 882 return false; 883 if (!(other instanceof AppointmentResponse)) 884 return false; 885 AppointmentResponse o = (AppointmentResponse) other; 886 return compareDeep(identifier, o.identifier, true) && compareDeep(appointment, o.appointment, true) 887 && compareDeep(start, o.start, true) && compareDeep(end, o.end, true) 888 && compareDeep(participantType, o.participantType, true) && compareDeep(actor, o.actor, true) 889 && compareDeep(participantStatus, o.participantStatus, true) && compareDeep(comment, o.comment, true); 890 } 891 892 @Override 893 public boolean equalsShallow(Base other) { 894 if (!super.equalsShallow(other)) 895 return false; 896 if (!(other instanceof AppointmentResponse)) 897 return false; 898 AppointmentResponse o = (AppointmentResponse) other; 899 return compareValues(start, o.start, true) && compareValues(end, o.end, true) 900 && compareValues(participantStatus, o.participantStatus, true) && compareValues(comment, o.comment, true); 901 } 902 903 public boolean isEmpty() { 904 return super.isEmpty() && (identifier == null || identifier.isEmpty()) 905 && (appointment == null || appointment.isEmpty()) && (start == null || start.isEmpty()) 906 && (end == null || end.isEmpty()) && (participantType == null || participantType.isEmpty()) 907 && (actor == null || actor.isEmpty()) && (participantStatus == null || participantStatus.isEmpty()) 908 && (comment == null || comment.isEmpty()); 909 } 910 911 @Override 912 public ResourceType getResourceType() { 913 return ResourceType.AppointmentResponse; 914 } 915 916 @SearchParamDefinition(name = "actor", path = "AppointmentResponse.actor", description = "The Person, Location/HealthcareService or Device that this appointment response replies for", type = "reference") 917 public static final String SP_ACTOR = "actor"; 918 @SearchParamDefinition(name = "identifier", path = "AppointmentResponse.identifier", description = "An Identifier in this appointment response", type = "token") 919 public static final String SP_IDENTIFIER = "identifier"; 920 @SearchParamDefinition(name = "practitioner", path = "AppointmentResponse.actor", description = "This Response is for this Practitioner", type = "reference") 921 public static final String SP_PRACTITIONER = "practitioner"; 922 @SearchParamDefinition(name = "part-status", path = "AppointmentResponse.participantStatus", description = "The participants acceptance status for this appointment", type = "token") 923 public static final String SP_PARTSTATUS = "part-status"; 924 @SearchParamDefinition(name = "patient", path = "AppointmentResponse.actor", description = "This Response is for this Patient", type = "reference") 925 public static final String SP_PATIENT = "patient"; 926 @SearchParamDefinition(name = "appointment", path = "AppointmentResponse.appointment", description = "The appointment that the response is attached to", type = "reference") 927 public static final String SP_APPOINTMENT = "appointment"; 928 @SearchParamDefinition(name = "location", path = "AppointmentResponse.actor", description = "This Response is for this Location", type = "reference") 929 public static final String SP_LOCATION = "location"; 930 931}