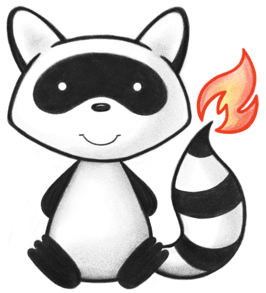
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.Date; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Child; 037import ca.uhn.fhir.model.api.annotation.DatatypeDef; 038import ca.uhn.fhir.model.api.annotation.Description; 039import org.hl7.fhir.instance.model.api.ICompositeType; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.utilities.Utilities; 042 043/** 044 * For referring to data content defined in other formats. 045 */ 046@DatatypeDef(name = "Attachment") 047public class Attachment extends Type implements ICompositeType { 048 049 /** 050 * Identifies the type of the data in the attachment and allows a method to be 051 * chosen to interpret or render the data. Includes mime type parameters such as 052 * charset where appropriate. 053 */ 054 @Child(name = "contentType", type = { CodeType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 055 @Description(shortDefinition = "Mime type of the content, with charset etc.", formalDefinition = "Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate.") 056 protected CodeType contentType; 057 058 /** 059 * The human language of the content. The value can be any valid value according 060 * to BCP 47. 061 */ 062 @Child(name = "language", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 063 @Description(shortDefinition = "Human language of the content (BCP-47)", formalDefinition = "The human language of the content. The value can be any valid value according to BCP 47.") 064 protected CodeType language; 065 066 /** 067 * The actual data of the attachment - a sequence of bytes. In XML, represented 068 * using base64. 069 */ 070 @Child(name = "data", type = { 071 Base64BinaryType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 072 @Description(shortDefinition = "Data inline, base64ed", formalDefinition = "The actual data of the attachment - a sequence of bytes. In XML, represented using base64.") 073 protected Base64BinaryType data; 074 075 /** 076 * An alternative location where the data can be accessed. 077 */ 078 @Child(name = "url", type = { UriType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 079 @Description(shortDefinition = "Uri where the data can be found", formalDefinition = "An alternative location where the data can be accessed.") 080 protected UriType url; 081 082 /** 083 * The number of bytes of data that make up this attachment. 084 */ 085 @Child(name = "size", type = { UnsignedIntType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 086 @Description(shortDefinition = "Number of bytes of content (if url provided)", formalDefinition = "The number of bytes of data that make up this attachment.") 087 protected UnsignedIntType size; 088 089 /** 090 * The calculated hash of the data using SHA-1. Represented using base64. 091 */ 092 @Child(name = "hash", type = { 093 Base64BinaryType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 094 @Description(shortDefinition = "Hash of the data (sha-1, base64ed)", formalDefinition = "The calculated hash of the data using SHA-1. Represented using base64.") 095 protected Base64BinaryType hash; 096 097 /** 098 * A label or set of text to display in place of the data. 099 */ 100 @Child(name = "title", type = { StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 101 @Description(shortDefinition = "Label to display in place of the data", formalDefinition = "A label or set of text to display in place of the data.") 102 protected StringType title; 103 104 /** 105 * The date that the attachment was first created. 106 */ 107 @Child(name = "creation", type = { 108 DateTimeType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 109 @Description(shortDefinition = "Date attachment was first created", formalDefinition = "The date that the attachment was first created.") 110 protected DateTimeType creation; 111 112 private static final long serialVersionUID = 581007080L; 113 114 /* 115 * Constructor 116 */ 117 public Attachment() { 118 super(); 119 } 120 121 /** 122 * @return {@link #contentType} (Identifies the type of the data in the 123 * attachment and allows a method to be chosen to interpret or render 124 * the data. Includes mime type parameters such as charset where 125 * appropriate.). This is the underlying object with id, value and 126 * extensions. The accessor "getContentType" gives direct access to the 127 * value 128 */ 129 public CodeType getContentTypeElement() { 130 if (this.contentType == null) 131 if (Configuration.errorOnAutoCreate()) 132 throw new Error("Attempt to auto-create Attachment.contentType"); 133 else if (Configuration.doAutoCreate()) 134 this.contentType = new CodeType(); // bb 135 return this.contentType; 136 } 137 138 public boolean hasContentTypeElement() { 139 return this.contentType != null && !this.contentType.isEmpty(); 140 } 141 142 public boolean hasContentType() { 143 return this.contentType != null && !this.contentType.isEmpty(); 144 } 145 146 /** 147 * @param value {@link #contentType} (Identifies the type of the data in the 148 * attachment and allows a method to be chosen to interpret or 149 * render the data. Includes mime type parameters such as charset 150 * where appropriate.). This is the underlying object with id, 151 * value and extensions. The accessor "getContentType" gives direct 152 * access to the value 153 */ 154 public Attachment setContentTypeElement(CodeType value) { 155 this.contentType = value; 156 return this; 157 } 158 159 /** 160 * @return Identifies the type of the data in the attachment and allows a method 161 * to be chosen to interpret or render the data. Includes mime type 162 * parameters such as charset where appropriate. 163 */ 164 public String getContentType() { 165 return this.contentType == null ? null : this.contentType.getValue(); 166 } 167 168 /** 169 * @param value Identifies the type of the data in the attachment and allows a 170 * method to be chosen to interpret or render the data. Includes 171 * mime type parameters such as charset where appropriate. 172 */ 173 public Attachment setContentType(String value) { 174 if (Utilities.noString(value)) 175 this.contentType = null; 176 else { 177 if (this.contentType == null) 178 this.contentType = new CodeType(); 179 this.contentType.setValue(value); 180 } 181 return this; 182 } 183 184 /** 185 * @return {@link #language} (The human language of the content. The value can 186 * be any valid value according to BCP 47.). This is the underlying 187 * object with id, value and extensions. The accessor "getLanguage" 188 * gives direct access to the value 189 */ 190 public CodeType getLanguageElement() { 191 if (this.language == null) 192 if (Configuration.errorOnAutoCreate()) 193 throw new Error("Attempt to auto-create Attachment.language"); 194 else if (Configuration.doAutoCreate()) 195 this.language = new CodeType(); // bb 196 return this.language; 197 } 198 199 public boolean hasLanguageElement() { 200 return this.language != null && !this.language.isEmpty(); 201 } 202 203 public boolean hasLanguage() { 204 return this.language != null && !this.language.isEmpty(); 205 } 206 207 /** 208 * @param value {@link #language} (The human language of the content. The value 209 * can be any valid value according to BCP 47.). This is the 210 * underlying object with id, value and extensions. The accessor 211 * "getLanguage" gives direct access to the value 212 */ 213 public Attachment setLanguageElement(CodeType value) { 214 this.language = value; 215 return this; 216 } 217 218 /** 219 * @return The human language of the content. The value can be any valid value 220 * according to BCP 47. 221 */ 222 public String getLanguage() { 223 return this.language == null ? null : this.language.getValue(); 224 } 225 226 /** 227 * @param value The human language of the content. The value can be any valid 228 * value according to BCP 47. 229 */ 230 public Attachment setLanguage(String value) { 231 if (Utilities.noString(value)) 232 this.language = null; 233 else { 234 if (this.language == null) 235 this.language = new CodeType(); 236 this.language.setValue(value); 237 } 238 return this; 239 } 240 241 /** 242 * @return {@link #data} (The actual data of the attachment - a sequence of 243 * bytes. In XML, represented using base64.). This is the underlying 244 * object with id, value and extensions. The accessor "getData" gives 245 * direct access to the value 246 */ 247 public Base64BinaryType getDataElement() { 248 if (this.data == null) 249 if (Configuration.errorOnAutoCreate()) 250 throw new Error("Attempt to auto-create Attachment.data"); 251 else if (Configuration.doAutoCreate()) 252 this.data = new Base64BinaryType(); // bb 253 return this.data; 254 } 255 256 public boolean hasDataElement() { 257 return this.data != null && !this.data.isEmpty(); 258 } 259 260 public boolean hasData() { 261 return this.data != null && !this.data.isEmpty(); 262 } 263 264 /** 265 * @param value {@link #data} (The actual data of the attachment - a sequence of 266 * bytes. In XML, represented using base64.). This is the 267 * underlying object with id, value and extensions. The accessor 268 * "getData" gives direct access to the value 269 */ 270 public Attachment setDataElement(Base64BinaryType value) { 271 this.data = value; 272 return this; 273 } 274 275 /** 276 * @return The actual data of the attachment - a sequence of bytes. In XML, 277 * represented using base64. 278 */ 279 public byte[] getData() { 280 return this.data == null ? null : this.data.getValue(); 281 } 282 283 /** 284 * @param value The actual data of the attachment - a sequence of bytes. In XML, 285 * represented using base64. 286 */ 287 public Attachment setData(byte[] value) { 288 if (value == null) 289 this.data = null; 290 else { 291 if (this.data == null) 292 this.data = new Base64BinaryType(); 293 this.data.setValue(value); 294 } 295 return this; 296 } 297 298 /** 299 * @return {@link #url} (An alternative location where the data can be 300 * accessed.). This is the underlying object with id, value and 301 * extensions. The accessor "getUrl" gives direct access to the value 302 */ 303 public UriType getUrlElement() { 304 if (this.url == null) 305 if (Configuration.errorOnAutoCreate()) 306 throw new Error("Attempt to auto-create Attachment.url"); 307 else if (Configuration.doAutoCreate()) 308 this.url = new UriType(); // bb 309 return this.url; 310 } 311 312 public boolean hasUrlElement() { 313 return this.url != null && !this.url.isEmpty(); 314 } 315 316 public boolean hasUrl() { 317 return this.url != null && !this.url.isEmpty(); 318 } 319 320 /** 321 * @param value {@link #url} (An alternative location where the data can be 322 * accessed.). This is the underlying object with id, value and 323 * extensions. The accessor "getUrl" gives direct access to the 324 * value 325 */ 326 public Attachment setUrlElement(UriType value) { 327 this.url = value; 328 return this; 329 } 330 331 /** 332 * @return An alternative location where the data can be accessed. 333 */ 334 public String getUrl() { 335 return this.url == null ? null : this.url.getValue(); 336 } 337 338 /** 339 * @param value An alternative location where the data can be accessed. 340 */ 341 public Attachment setUrl(String value) { 342 if (Utilities.noString(value)) 343 this.url = null; 344 else { 345 if (this.url == null) 346 this.url = new UriType(); 347 this.url.setValue(value); 348 } 349 return this; 350 } 351 352 /** 353 * @return {@link #size} (The number of bytes of data that make up this 354 * attachment.). This is the underlying object with id, value and 355 * extensions. The accessor "getSize" gives direct access to the value 356 */ 357 public UnsignedIntType getSizeElement() { 358 if (this.size == null) 359 if (Configuration.errorOnAutoCreate()) 360 throw new Error("Attempt to auto-create Attachment.size"); 361 else if (Configuration.doAutoCreate()) 362 this.size = new UnsignedIntType(); // bb 363 return this.size; 364 } 365 366 public boolean hasSizeElement() { 367 return this.size != null && !this.size.isEmpty(); 368 } 369 370 public boolean hasSize() { 371 return this.size != null && !this.size.isEmpty(); 372 } 373 374 /** 375 * @param value {@link #size} (The number of bytes of data that make up this 376 * attachment.). This is the underlying object with id, value and 377 * extensions. The accessor "getSize" gives direct access to the 378 * value 379 */ 380 public Attachment setSizeElement(UnsignedIntType value) { 381 this.size = value; 382 return this; 383 } 384 385 /** 386 * @return The number of bytes of data that make up this attachment. 387 */ 388 public int getSize() { 389 return this.size == null || this.size.isEmpty() ? 0 : this.size.getValue(); 390 } 391 392 /** 393 * @param value The number of bytes of data that make up this attachment. 394 */ 395 public Attachment setSize(int value) { 396 if (this.size == null) 397 this.size = new UnsignedIntType(); 398 this.size.setValue(value); 399 return this; 400 } 401 402 /** 403 * @return {@link #hash} (The calculated hash of the data using SHA-1. 404 * Represented using base64.). This is the underlying object with id, 405 * value and extensions. The accessor "getHash" gives direct access to 406 * the value 407 */ 408 public Base64BinaryType getHashElement() { 409 if (this.hash == null) 410 if (Configuration.errorOnAutoCreate()) 411 throw new Error("Attempt to auto-create Attachment.hash"); 412 else if (Configuration.doAutoCreate()) 413 this.hash = new Base64BinaryType(); // bb 414 return this.hash; 415 } 416 417 public boolean hasHashElement() { 418 return this.hash != null && !this.hash.isEmpty(); 419 } 420 421 public boolean hasHash() { 422 return this.hash != null && !this.hash.isEmpty(); 423 } 424 425 /** 426 * @param value {@link #hash} (The calculated hash of the data using SHA-1. 427 * Represented using base64.). This is the underlying object with 428 * id, value and extensions. The accessor "getHash" gives direct 429 * access to the value 430 */ 431 public Attachment setHashElement(Base64BinaryType value) { 432 this.hash = value; 433 return this; 434 } 435 436 /** 437 * @return The calculated hash of the data using SHA-1. Represented using 438 * base64. 439 */ 440 public byte[] getHash() { 441 return this.hash == null ? null : this.hash.getValue(); 442 } 443 444 /** 445 * @param value The calculated hash of the data using SHA-1. Represented using 446 * base64. 447 */ 448 public Attachment setHash(byte[] value) { 449 if (value == null) 450 this.hash = null; 451 else { 452 if (this.hash == null) 453 this.hash = new Base64BinaryType(); 454 this.hash.setValue(value); 455 } 456 return this; 457 } 458 459 /** 460 * @return {@link #title} (A label or set of text to display in place of the 461 * data.). This is the underlying object with id, value and extensions. 462 * The accessor "getTitle" gives direct access to the value 463 */ 464 public StringType getTitleElement() { 465 if (this.title == null) 466 if (Configuration.errorOnAutoCreate()) 467 throw new Error("Attempt to auto-create Attachment.title"); 468 else if (Configuration.doAutoCreate()) 469 this.title = new StringType(); // bb 470 return this.title; 471 } 472 473 public boolean hasTitleElement() { 474 return this.title != null && !this.title.isEmpty(); 475 } 476 477 public boolean hasTitle() { 478 return this.title != null && !this.title.isEmpty(); 479 } 480 481 /** 482 * @param value {@link #title} (A label or set of text to display in place of 483 * the data.). This is the underlying object with id, value and 484 * extensions. The accessor "getTitle" gives direct access to the 485 * value 486 */ 487 public Attachment setTitleElement(StringType value) { 488 this.title = value; 489 return this; 490 } 491 492 /** 493 * @return A label or set of text to display in place of the data. 494 */ 495 public String getTitle() { 496 return this.title == null ? null : this.title.getValue(); 497 } 498 499 /** 500 * @param value A label or set of text to display in place of the data. 501 */ 502 public Attachment setTitle(String value) { 503 if (Utilities.noString(value)) 504 this.title = null; 505 else { 506 if (this.title == null) 507 this.title = new StringType(); 508 this.title.setValue(value); 509 } 510 return this; 511 } 512 513 /** 514 * @return {@link #creation} (The date that the attachment was first created.). 515 * This is the underlying object with id, value and extensions. The 516 * accessor "getCreation" gives direct access to the value 517 */ 518 public DateTimeType getCreationElement() { 519 if (this.creation == null) 520 if (Configuration.errorOnAutoCreate()) 521 throw new Error("Attempt to auto-create Attachment.creation"); 522 else if (Configuration.doAutoCreate()) 523 this.creation = new DateTimeType(); // bb 524 return this.creation; 525 } 526 527 public boolean hasCreationElement() { 528 return this.creation != null && !this.creation.isEmpty(); 529 } 530 531 public boolean hasCreation() { 532 return this.creation != null && !this.creation.isEmpty(); 533 } 534 535 /** 536 * @param value {@link #creation} (The date that the attachment was first 537 * created.). This is the underlying object with id, value and 538 * extensions. The accessor "getCreation" gives direct access to 539 * the value 540 */ 541 public Attachment setCreationElement(DateTimeType value) { 542 this.creation = value; 543 return this; 544 } 545 546 /** 547 * @return The date that the attachment was first created. 548 */ 549 public Date getCreation() { 550 return this.creation == null ? null : this.creation.getValue(); 551 } 552 553 /** 554 * @param value The date that the attachment was first created. 555 */ 556 public Attachment setCreation(Date value) { 557 if (value == null) 558 this.creation = null; 559 else { 560 if (this.creation == null) 561 this.creation = new DateTimeType(); 562 this.creation.setValue(value); 563 } 564 return this; 565 } 566 567 protected void listChildren(List<Property> childrenList) { 568 super.listChildren(childrenList); 569 childrenList.add(new Property("contentType", "code", 570 "Identifies the type of the data in the attachment and allows a method to be chosen to interpret or render the data. Includes mime type parameters such as charset where appropriate.", 571 0, java.lang.Integer.MAX_VALUE, contentType)); 572 childrenList.add(new Property("language", "code", 573 "The human language of the content. The value can be any valid value according to BCP 47.", 0, 574 java.lang.Integer.MAX_VALUE, language)); 575 childrenList.add(new Property("data", "base64Binary", 576 "The actual data of the attachment - a sequence of bytes. In XML, represented using base64.", 0, 577 java.lang.Integer.MAX_VALUE, data)); 578 childrenList.add(new Property("url", "uri", "An alternative location where the data can be accessed.", 0, 579 java.lang.Integer.MAX_VALUE, url)); 580 childrenList.add(new Property("size", "unsignedInt", "The number of bytes of data that make up this attachment.", 0, 581 java.lang.Integer.MAX_VALUE, size)); 582 childrenList.add( 583 new Property("hash", "base64Binary", "The calculated hash of the data using SHA-1. Represented using base64.", 584 0, java.lang.Integer.MAX_VALUE, hash)); 585 childrenList.add(new Property("title", "string", "A label or set of text to display in place of the data.", 0, 586 java.lang.Integer.MAX_VALUE, title)); 587 childrenList.add(new Property("creation", "dateTime", "The date that the attachment was first created.", 0, 588 java.lang.Integer.MAX_VALUE, creation)); 589 } 590 591 @Override 592 public void setProperty(String name, Base value) throws FHIRException { 593 if (name.equals("contentType")) 594 this.contentType = castToCode(value); // CodeType 595 else if (name.equals("language")) 596 this.language = castToCode(value); // CodeType 597 else if (name.equals("data")) 598 this.data = castToBase64Binary(value); // Base64BinaryType 599 else if (name.equals("url")) 600 this.url = castToUri(value); // UriType 601 else if (name.equals("size")) 602 this.size = castToUnsignedInt(value); // UnsignedIntType 603 else if (name.equals("hash")) 604 this.hash = castToBase64Binary(value); // Base64BinaryType 605 else if (name.equals("title")) 606 this.title = castToString(value); // StringType 607 else if (name.equals("creation")) 608 this.creation = castToDateTime(value); // DateTimeType 609 else 610 super.setProperty(name, value); 611 } 612 613 @Override 614 public Base addChild(String name) throws FHIRException { 615 if (name.equals("contentType")) { 616 throw new FHIRException("Cannot call addChild on a singleton property Attachment.contentType"); 617 } else if (name.equals("language")) { 618 throw new FHIRException("Cannot call addChild on a singleton property Attachment.language"); 619 } else if (name.equals("data")) { 620 throw new FHIRException("Cannot call addChild on a singleton property Attachment.data"); 621 } else if (name.equals("url")) { 622 throw new FHIRException("Cannot call addChild on a singleton property Attachment.url"); 623 } else if (name.equals("size")) { 624 throw new FHIRException("Cannot call addChild on a singleton property Attachment.size"); 625 } else if (name.equals("hash")) { 626 throw new FHIRException("Cannot call addChild on a singleton property Attachment.hash"); 627 } else if (name.equals("title")) { 628 throw new FHIRException("Cannot call addChild on a singleton property Attachment.title"); 629 } else if (name.equals("creation")) { 630 throw new FHIRException("Cannot call addChild on a singleton property Attachment.creation"); 631 } else 632 return super.addChild(name); 633 } 634 635 public String fhirType() { 636 return "Attachment"; 637 638 } 639 640 public Attachment copy() { 641 Attachment dst = new Attachment(); 642 copyValues(dst); 643 dst.contentType = contentType == null ? null : contentType.copy(); 644 dst.language = language == null ? null : language.copy(); 645 dst.data = data == null ? null : data.copy(); 646 dst.url = url == null ? null : url.copy(); 647 dst.size = size == null ? null : size.copy(); 648 dst.hash = hash == null ? null : hash.copy(); 649 dst.title = title == null ? null : title.copy(); 650 dst.creation = creation == null ? null : creation.copy(); 651 return dst; 652 } 653 654 protected Attachment typedCopy() { 655 return copy(); 656 } 657 658 @Override 659 public boolean equalsDeep(Base other) { 660 if (!super.equalsDeep(other)) 661 return false; 662 if (!(other instanceof Attachment)) 663 return false; 664 Attachment o = (Attachment) other; 665 return compareDeep(contentType, o.contentType, true) && compareDeep(language, o.language, true) 666 && compareDeep(data, o.data, true) && compareDeep(url, o.url, true) && compareDeep(size, o.size, true) 667 && compareDeep(hash, o.hash, true) && compareDeep(title, o.title, true) 668 && compareDeep(creation, o.creation, true); 669 } 670 671 @Override 672 public boolean equalsShallow(Base other) { 673 if (!super.equalsShallow(other)) 674 return false; 675 if (!(other instanceof Attachment)) 676 return false; 677 Attachment o = (Attachment) other; 678 return compareValues(contentType, o.contentType, true) && compareValues(language, o.language, true) 679 && compareValues(data, o.data, true) && compareValues(url, o.url, true) && compareValues(size, o.size, true) 680 && compareValues(hash, o.hash, true) && compareValues(title, o.title, true) 681 && compareValues(creation, o.creation, true); 682 } 683 684 public boolean isEmpty() { 685 return super.isEmpty() && (contentType == null || contentType.isEmpty()) && (language == null || language.isEmpty()) 686 && (data == null || data.isEmpty()) && (url == null || url.isEmpty()) && (size == null || size.isEmpty()) 687 && (hash == null || hash.isEmpty()) && (title == null || title.isEmpty()) 688 && (creation == null || creation.isEmpty()); 689 } 690 691}