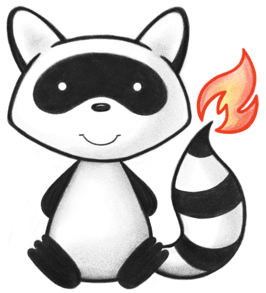
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * A record of an event made for purposes of maintaining a security log. Typical 048 * uses include detection of intrusion attempts and monitoring for inappropriate 049 * usage. 050 */ 051@ResourceDef(name = "AuditEvent", profile = "http://hl7.org/fhir/Profile/AuditEvent") 052public class AuditEvent extends DomainResource { 053 054 public enum AuditEventAction { 055 /** 056 * Create a new database object, such as placing an order. 057 */ 058 C, 059 /** 060 * Display or print data, such as a doctor census. 061 */ 062 R, 063 /** 064 * Update data, such as revise patient information. 065 */ 066 U, 067 /** 068 * Delete items, such as a doctor master file record. 069 */ 070 D, 071 /** 072 * Perform a system or application function such as log-on, program execution or 073 * use of an object's method, or perform a query/search operation. 074 */ 075 E, 076 /** 077 * added to help the parsers 078 */ 079 NULL; 080 081 public static AuditEventAction fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("C".equals(codeString)) 085 return C; 086 if ("R".equals(codeString)) 087 return R; 088 if ("U".equals(codeString)) 089 return U; 090 if ("D".equals(codeString)) 091 return D; 092 if ("E".equals(codeString)) 093 return E; 094 throw new FHIRException("Unknown AuditEventAction code '" + codeString + "'"); 095 } 096 097 public String toCode() { 098 switch (this) { 099 case C: 100 return "C"; 101 case R: 102 return "R"; 103 case U: 104 return "U"; 105 case D: 106 return "D"; 107 case E: 108 return "E"; 109 case NULL: 110 return null; 111 default: 112 return "?"; 113 } 114 } 115 116 public String getSystem() { 117 switch (this) { 118 case C: 119 return "http://hl7.org/fhir/audit-event-action"; 120 case R: 121 return "http://hl7.org/fhir/audit-event-action"; 122 case U: 123 return "http://hl7.org/fhir/audit-event-action"; 124 case D: 125 return "http://hl7.org/fhir/audit-event-action"; 126 case E: 127 return "http://hl7.org/fhir/audit-event-action"; 128 case NULL: 129 return null; 130 default: 131 return "?"; 132 } 133 } 134 135 public String getDefinition() { 136 switch (this) { 137 case C: 138 return "Create a new database object, such as placing an order."; 139 case R: 140 return "Display or print data, such as a doctor census."; 141 case U: 142 return "Update data, such as revise patient information."; 143 case D: 144 return "Delete items, such as a doctor master file record."; 145 case E: 146 return "Perform a system or application function such as log-on, program execution or use of an object's method, or perform a query/search operation."; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 154 public String getDisplay() { 155 switch (this) { 156 case C: 157 return "Create"; 158 case R: 159 return "Read/View/Print"; 160 case U: 161 return "Update"; 162 case D: 163 return "Delete"; 164 case E: 165 return "Execute"; 166 case NULL: 167 return null; 168 default: 169 return "?"; 170 } 171 } 172 } 173 174 public static class AuditEventActionEnumFactory implements EnumFactory<AuditEventAction> { 175 public AuditEventAction fromCode(String codeString) throws IllegalArgumentException { 176 if (codeString == null || "".equals(codeString)) 177 if (codeString == null || "".equals(codeString)) 178 return null; 179 if ("C".equals(codeString)) 180 return AuditEventAction.C; 181 if ("R".equals(codeString)) 182 return AuditEventAction.R; 183 if ("U".equals(codeString)) 184 return AuditEventAction.U; 185 if ("D".equals(codeString)) 186 return AuditEventAction.D; 187 if ("E".equals(codeString)) 188 return AuditEventAction.E; 189 throw new IllegalArgumentException("Unknown AuditEventAction code '" + codeString + "'"); 190 } 191 192 public Enumeration<AuditEventAction> fromType(Base code) throws FHIRException { 193 if (code == null || code.isEmpty()) 194 return null; 195 String codeString = ((PrimitiveType) code).asStringValue(); 196 if (codeString == null || "".equals(codeString)) 197 return null; 198 if ("C".equals(codeString)) 199 return new Enumeration<AuditEventAction>(this, AuditEventAction.C); 200 if ("R".equals(codeString)) 201 return new Enumeration<AuditEventAction>(this, AuditEventAction.R); 202 if ("U".equals(codeString)) 203 return new Enumeration<AuditEventAction>(this, AuditEventAction.U); 204 if ("D".equals(codeString)) 205 return new Enumeration<AuditEventAction>(this, AuditEventAction.D); 206 if ("E".equals(codeString)) 207 return new Enumeration<AuditEventAction>(this, AuditEventAction.E); 208 throw new FHIRException("Unknown AuditEventAction code '" + codeString + "'"); 209 } 210 211 public String toCode(AuditEventAction code) 212 { 213 if (code == AuditEventAction.NULL) 214 return null; 215 if (code == AuditEventAction.C) 216 return "C"; 217 if (code == AuditEventAction.R) 218 return "R"; 219 if (code == AuditEventAction.U) 220 return "U"; 221 if (code == AuditEventAction.D) 222 return "D"; 223 if (code == AuditEventAction.E) 224 return "E"; 225 return "?"; 226 } 227 } 228 229 public enum AuditEventOutcome { 230 /** 231 * The operation completed successfully (whether with warnings or not). 232 */ 233 _0, 234 /** 235 * The action was not successful due to some kind of catered for error (often 236 * equivalent to an HTTP 400 response). 237 */ 238 _4, 239 /** 240 * The action was not successful due to some kind of unexpected error (often 241 * equivalent to an HTTP 500 response). 242 */ 243 _8, 244 /** 245 * An error of such magnitude occurred that the system is no longer available 246 * for use (i.e. the system died). 247 */ 248 _12, 249 /** 250 * added to help the parsers 251 */ 252 NULL; 253 254 public static AuditEventOutcome fromCode(String codeString) throws FHIRException { 255 if (codeString == null || "".equals(codeString)) 256 return null; 257 if ("0".equals(codeString)) 258 return _0; 259 if ("4".equals(codeString)) 260 return _4; 261 if ("8".equals(codeString)) 262 return _8; 263 if ("12".equals(codeString)) 264 return _12; 265 throw new FHIRException("Unknown AuditEventOutcome code '" + codeString + "'"); 266 } 267 268 public String toCode() { 269 switch (this) { 270 case _0: 271 return "0"; 272 case _4: 273 return "4"; 274 case _8: 275 return "8"; 276 case _12: 277 return "12"; 278 case NULL: 279 return null; 280 default: 281 return "?"; 282 } 283 } 284 285 public String getSystem() { 286 switch (this) { 287 case _0: 288 return "http://hl7.org/fhir/audit-event-outcome"; 289 case _4: 290 return "http://hl7.org/fhir/audit-event-outcome"; 291 case _8: 292 return "http://hl7.org/fhir/audit-event-outcome"; 293 case _12: 294 return "http://hl7.org/fhir/audit-event-outcome"; 295 case NULL: 296 return null; 297 default: 298 return "?"; 299 } 300 } 301 302 public String getDefinition() { 303 switch (this) { 304 case _0: 305 return "The operation completed successfully (whether with warnings or not)."; 306 case _4: 307 return "The action was not successful due to some kind of catered for error (often equivalent to an HTTP 400 response)."; 308 case _8: 309 return "The action was not successful due to some kind of unexpected error (often equivalent to an HTTP 500 response)."; 310 case _12: 311 return "An error of such magnitude occurred that the system is no longer available for use (i.e. the system died)."; 312 case NULL: 313 return null; 314 default: 315 return "?"; 316 } 317 } 318 319 public String getDisplay() { 320 switch (this) { 321 case _0: 322 return "Success"; 323 case _4: 324 return "Minor failure"; 325 case _8: 326 return "Serious failure"; 327 case _12: 328 return "Major failure"; 329 case NULL: 330 return null; 331 default: 332 return "?"; 333 } 334 } 335 } 336 337 public static class AuditEventOutcomeEnumFactory implements EnumFactory<AuditEventOutcome> { 338 public AuditEventOutcome fromCode(String codeString) throws IllegalArgumentException { 339 if (codeString == null || "".equals(codeString)) 340 if (codeString == null || "".equals(codeString)) 341 return null; 342 if ("0".equals(codeString)) 343 return AuditEventOutcome._0; 344 if ("4".equals(codeString)) 345 return AuditEventOutcome._4; 346 if ("8".equals(codeString)) 347 return AuditEventOutcome._8; 348 if ("12".equals(codeString)) 349 return AuditEventOutcome._12; 350 throw new IllegalArgumentException("Unknown AuditEventOutcome code '" + codeString + "'"); 351 } 352 353 public Enumeration<AuditEventOutcome> fromType(Base code) throws FHIRException { 354 if (code == null || code.isEmpty()) 355 return null; 356 String codeString = ((PrimitiveType) code).asStringValue(); 357 if (codeString == null || "".equals(codeString)) 358 return null; 359 if ("0".equals(codeString)) 360 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome._0); 361 if ("4".equals(codeString)) 362 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome._4); 363 if ("8".equals(codeString)) 364 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome._8); 365 if ("12".equals(codeString)) 366 return new Enumeration<AuditEventOutcome>(this, AuditEventOutcome._12); 367 throw new FHIRException("Unknown AuditEventOutcome code '" + codeString + "'"); 368 } 369 370 public String toCode(AuditEventOutcome code) 371 { 372 if (code == AuditEventOutcome.NULL) 373 return null; 374 if (code == AuditEventOutcome._0) 375 return "0"; 376 if (code == AuditEventOutcome._4) 377 return "4"; 378 if (code == AuditEventOutcome._8) 379 return "8"; 380 if (code == AuditEventOutcome._12) 381 return "12"; 382 return "?"; 383 } 384 } 385 386 public enum AuditEventParticipantNetworkType { 387 /** 388 * The machine name, including DNS name. 389 */ 390 _1, 391 /** 392 * The assigned Internet Protocol (IP) address. 393 */ 394 _2, 395 /** 396 * The assigned telephone number. 397 */ 398 _3, 399 /** 400 * The assigned email address. 401 */ 402 _4, 403 /** 404 * URI (User directory, HTTP-PUT, ftp, etc.). 405 */ 406 _5, 407 /** 408 * added to help the parsers 409 */ 410 NULL; 411 412 public static AuditEventParticipantNetworkType fromCode(String codeString) throws FHIRException { 413 if (codeString == null || "".equals(codeString)) 414 return null; 415 if ("1".equals(codeString)) 416 return _1; 417 if ("2".equals(codeString)) 418 return _2; 419 if ("3".equals(codeString)) 420 return _3; 421 if ("4".equals(codeString)) 422 return _4; 423 if ("5".equals(codeString)) 424 return _5; 425 throw new FHIRException("Unknown AuditEventParticipantNetworkType code '" + codeString + "'"); 426 } 427 428 public String toCode() { 429 switch (this) { 430 case _1: 431 return "1"; 432 case _2: 433 return "2"; 434 case _3: 435 return "3"; 436 case _4: 437 return "4"; 438 case _5: 439 return "5"; 440 case NULL: 441 return null; 442 default: 443 return "?"; 444 } 445 } 446 447 public String getSystem() { 448 switch (this) { 449 case _1: 450 return "http://hl7.org/fhir/network-type"; 451 case _2: 452 return "http://hl7.org/fhir/network-type"; 453 case _3: 454 return "http://hl7.org/fhir/network-type"; 455 case _4: 456 return "http://hl7.org/fhir/network-type"; 457 case _5: 458 return "http://hl7.org/fhir/network-type"; 459 case NULL: 460 return null; 461 default: 462 return "?"; 463 } 464 } 465 466 public String getDefinition() { 467 switch (this) { 468 case _1: 469 return "The machine name, including DNS name."; 470 case _2: 471 return "The assigned Internet Protocol (IP) address."; 472 case _3: 473 return "The assigned telephone number."; 474 case _4: 475 return "The assigned email address."; 476 case _5: 477 return "URI (User directory, HTTP-PUT, ftp, etc.)."; 478 case NULL: 479 return null; 480 default: 481 return "?"; 482 } 483 } 484 485 public String getDisplay() { 486 switch (this) { 487 case _1: 488 return "Machine Name"; 489 case _2: 490 return "IP Address"; 491 case _3: 492 return "Telephone Number"; 493 case _4: 494 return "Email address"; 495 case _5: 496 return "URI"; 497 case NULL: 498 return null; 499 default: 500 return "?"; 501 } 502 } 503 } 504 505 public static class AuditEventParticipantNetworkTypeEnumFactory 506 implements EnumFactory<AuditEventParticipantNetworkType> { 507 public AuditEventParticipantNetworkType fromCode(String codeString) throws IllegalArgumentException { 508 if (codeString == null || "".equals(codeString)) 509 if (codeString == null || "".equals(codeString)) 510 return null; 511 if ("1".equals(codeString)) 512 return AuditEventParticipantNetworkType._1; 513 if ("2".equals(codeString)) 514 return AuditEventParticipantNetworkType._2; 515 if ("3".equals(codeString)) 516 return AuditEventParticipantNetworkType._3; 517 if ("4".equals(codeString)) 518 return AuditEventParticipantNetworkType._4; 519 if ("5".equals(codeString)) 520 return AuditEventParticipantNetworkType._5; 521 throw new IllegalArgumentException("Unknown AuditEventParticipantNetworkType code '" + codeString + "'"); 522 } 523 524 public Enumeration<AuditEventParticipantNetworkType> fromType(Base code) throws FHIRException { 525 if (code == null || code.isEmpty()) 526 return null; 527 String codeString = ((PrimitiveType) code).asStringValue(); 528 if (codeString == null || "".equals(codeString)) 529 return null; 530 if ("1".equals(codeString)) 531 return new Enumeration<AuditEventParticipantNetworkType>(this, AuditEventParticipantNetworkType._1); 532 if ("2".equals(codeString)) 533 return new Enumeration<AuditEventParticipantNetworkType>(this, AuditEventParticipantNetworkType._2); 534 if ("3".equals(codeString)) 535 return new Enumeration<AuditEventParticipantNetworkType>(this, AuditEventParticipantNetworkType._3); 536 if ("4".equals(codeString)) 537 return new Enumeration<AuditEventParticipantNetworkType>(this, AuditEventParticipantNetworkType._4); 538 if ("5".equals(codeString)) 539 return new Enumeration<AuditEventParticipantNetworkType>(this, AuditEventParticipantNetworkType._5); 540 throw new FHIRException("Unknown AuditEventParticipantNetworkType code '" + codeString + "'"); 541 } 542 543 public String toCode(AuditEventParticipantNetworkType code) 544 { 545 if (code == AuditEventParticipantNetworkType.NULL) 546 return null; 547 if (code == AuditEventParticipantNetworkType._1) 548 return "1"; 549 if (code == AuditEventParticipantNetworkType._2) 550 return "2"; 551 if (code == AuditEventParticipantNetworkType._3) 552 return "3"; 553 if (code == AuditEventParticipantNetworkType._4) 554 return "4"; 555 if (code == AuditEventParticipantNetworkType._5) 556 return "5"; 557 return "?"; 558 } 559 } 560 561 @Block() 562 public static class AuditEventEventComponent extends BackboneElement implements IBaseBackboneElement { 563 /** 564 * Identifier for a family of the event. For example, a menu item, program, 565 * rule, policy, function code, application name or URL. It identifies the 566 * performed function. 567 */ 568 @Child(name = "type", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 569 @Description(shortDefinition = "Type/identifier of event", formalDefinition = "Identifier for a family of the event. For example, a menu item, program, rule, policy, function code, application name or URL. It identifies the performed function.") 570 protected Coding type; 571 572 /** 573 * Identifier for the category of event. 574 */ 575 @Child(name = "subtype", type = { 576 Coding.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 577 @Description(shortDefinition = "More specific type/id for the event", formalDefinition = "Identifier for the category of event.") 578 protected List<Coding> subtype; 579 580 /** 581 * Indicator for type of action performed during the event that generated the 582 * audit. 583 */ 584 @Child(name = "action", type = { CodeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 585 @Description(shortDefinition = "Type of action performed during the event", formalDefinition = "Indicator for type of action performed during the event that generated the audit.") 586 protected Enumeration<AuditEventAction> action; 587 588 /** 589 * The time when the event occurred on the source. 590 */ 591 @Child(name = "dateTime", type = { 592 InstantType.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 593 @Description(shortDefinition = "Time when the event occurred on source", formalDefinition = "The time when the event occurred on the source.") 594 protected InstantType dateTime; 595 596 /** 597 * Indicates whether the event succeeded or failed. 598 */ 599 @Child(name = "outcome", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 600 @Description(shortDefinition = "Whether the event succeeded or failed", formalDefinition = "Indicates whether the event succeeded or failed.") 601 protected Enumeration<AuditEventOutcome> outcome; 602 603 /** 604 * A free text description of the outcome of the event. 605 */ 606 @Child(name = "outcomeDesc", type = { 607 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 608 @Description(shortDefinition = "Description of the event outcome", formalDefinition = "A free text description of the outcome of the event.") 609 protected StringType outcomeDesc; 610 611 /** 612 * The purposeOfUse (reason) that was used during the event being recorded. 613 */ 614 @Child(name = "purposeOfEvent", type = { 615 Coding.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 616 @Description(shortDefinition = "The purposeOfUse of the event", formalDefinition = "The purposeOfUse (reason) that was used during the event being recorded.") 617 protected List<Coding> purposeOfEvent; 618 619 private static final long serialVersionUID = 1916806397L; 620 621 /* 622 * Constructor 623 */ 624 public AuditEventEventComponent() { 625 super(); 626 } 627 628 /* 629 * Constructor 630 */ 631 public AuditEventEventComponent(Coding type, InstantType dateTime) { 632 super(); 633 this.type = type; 634 this.dateTime = dateTime; 635 } 636 637 /** 638 * @return {@link #type} (Identifier for a family of the event. For example, a 639 * menu item, program, rule, policy, function code, application name or 640 * URL. It identifies the performed function.) 641 */ 642 public Coding getType() { 643 if (this.type == null) 644 if (Configuration.errorOnAutoCreate()) 645 throw new Error("Attempt to auto-create AuditEventEventComponent.type"); 646 else if (Configuration.doAutoCreate()) 647 this.type = new Coding(); // cc 648 return this.type; 649 } 650 651 public boolean hasType() { 652 return this.type != null && !this.type.isEmpty(); 653 } 654 655 /** 656 * @param value {@link #type} (Identifier for a family of the event. For 657 * example, a menu item, program, rule, policy, function code, 658 * application name or URL. It identifies the performed function.) 659 */ 660 public AuditEventEventComponent setType(Coding value) { 661 this.type = value; 662 return this; 663 } 664 665 /** 666 * @return {@link #subtype} (Identifier for the category of event.) 667 */ 668 public List<Coding> getSubtype() { 669 if (this.subtype == null) 670 this.subtype = new ArrayList<Coding>(); 671 return this.subtype; 672 } 673 674 public boolean hasSubtype() { 675 if (this.subtype == null) 676 return false; 677 for (Coding item : this.subtype) 678 if (!item.isEmpty()) 679 return true; 680 return false; 681 } 682 683 /** 684 * @return {@link #subtype} (Identifier for the category of event.) 685 */ 686 // syntactic sugar 687 public Coding addSubtype() { // 3 688 Coding t = new Coding(); 689 if (this.subtype == null) 690 this.subtype = new ArrayList<Coding>(); 691 this.subtype.add(t); 692 return t; 693 } 694 695 // syntactic sugar 696 public AuditEventEventComponent addSubtype(Coding t) { // 3 697 if (t == null) 698 return this; 699 if (this.subtype == null) 700 this.subtype = new ArrayList<Coding>(); 701 this.subtype.add(t); 702 return this; 703 } 704 705 /** 706 * @return {@link #action} (Indicator for type of action performed during the 707 * event that generated the audit.). This is the underlying object with 708 * id, value and extensions. The accessor "getAction" gives direct 709 * access to the value 710 */ 711 public Enumeration<AuditEventAction> getActionElement() { 712 if (this.action == null) 713 if (Configuration.errorOnAutoCreate()) 714 throw new Error("Attempt to auto-create AuditEventEventComponent.action"); 715 else if (Configuration.doAutoCreate()) 716 this.action = new Enumeration<AuditEventAction>(new AuditEventActionEnumFactory()); // bb 717 return this.action; 718 } 719 720 public boolean hasActionElement() { 721 return this.action != null && !this.action.isEmpty(); 722 } 723 724 public boolean hasAction() { 725 return this.action != null && !this.action.isEmpty(); 726 } 727 728 /** 729 * @param value {@link #action} (Indicator for type of action performed during 730 * the event that generated the audit.). This is the underlying 731 * object with id, value and extensions. The accessor "getAction" 732 * gives direct access to the value 733 */ 734 public AuditEventEventComponent setActionElement(Enumeration<AuditEventAction> value) { 735 this.action = value; 736 return this; 737 } 738 739 /** 740 * @return Indicator for type of action performed during the event that 741 * generated the audit. 742 */ 743 public AuditEventAction getAction() { 744 return this.action == null ? null : this.action.getValue(); 745 } 746 747 /** 748 * @param value Indicator for type of action performed during the event that 749 * generated the audit. 750 */ 751 public AuditEventEventComponent setAction(AuditEventAction value) { 752 if (value == null) 753 this.action = null; 754 else { 755 if (this.action == null) 756 this.action = new Enumeration<AuditEventAction>(new AuditEventActionEnumFactory()); 757 this.action.setValue(value); 758 } 759 return this; 760 } 761 762 /** 763 * @return {@link #dateTime} (The time when the event occurred on the source.). 764 * This is the underlying object with id, value and extensions. The 765 * accessor "getDateTime" gives direct access to the value 766 */ 767 public InstantType getDateTimeElement() { 768 if (this.dateTime == null) 769 if (Configuration.errorOnAutoCreate()) 770 throw new Error("Attempt to auto-create AuditEventEventComponent.dateTime"); 771 else if (Configuration.doAutoCreate()) 772 this.dateTime = new InstantType(); // bb 773 return this.dateTime; 774 } 775 776 public boolean hasDateTimeElement() { 777 return this.dateTime != null && !this.dateTime.isEmpty(); 778 } 779 780 public boolean hasDateTime() { 781 return this.dateTime != null && !this.dateTime.isEmpty(); 782 } 783 784 /** 785 * @param value {@link #dateTime} (The time when the event occurred on the 786 * source.). This is the underlying object with id, value and 787 * extensions. The accessor "getDateTime" gives direct access to 788 * the value 789 */ 790 public AuditEventEventComponent setDateTimeElement(InstantType value) { 791 this.dateTime = value; 792 return this; 793 } 794 795 /** 796 * @return The time when the event occurred on the source. 797 */ 798 public Date getDateTime() { 799 return this.dateTime == null ? null : this.dateTime.getValue(); 800 } 801 802 /** 803 * @param value The time when the event occurred on the source. 804 */ 805 public AuditEventEventComponent setDateTime(Date value) { 806 if (this.dateTime == null) 807 this.dateTime = new InstantType(); 808 this.dateTime.setValue(value); 809 return this; 810 } 811 812 /** 813 * @return {@link #outcome} (Indicates whether the event succeeded or failed.). 814 * This is the underlying object with id, value and extensions. The 815 * accessor "getOutcome" gives direct access to the value 816 */ 817 public Enumeration<AuditEventOutcome> getOutcomeElement() { 818 if (this.outcome == null) 819 if (Configuration.errorOnAutoCreate()) 820 throw new Error("Attempt to auto-create AuditEventEventComponent.outcome"); 821 else if (Configuration.doAutoCreate()) 822 this.outcome = new Enumeration<AuditEventOutcome>(new AuditEventOutcomeEnumFactory()); // bb 823 return this.outcome; 824 } 825 826 public boolean hasOutcomeElement() { 827 return this.outcome != null && !this.outcome.isEmpty(); 828 } 829 830 public boolean hasOutcome() { 831 return this.outcome != null && !this.outcome.isEmpty(); 832 } 833 834 /** 835 * @param value {@link #outcome} (Indicates whether the event succeeded or 836 * failed.). This is the underlying object with id, value and 837 * extensions. The accessor "getOutcome" gives direct access to the 838 * value 839 */ 840 public AuditEventEventComponent setOutcomeElement(Enumeration<AuditEventOutcome> value) { 841 this.outcome = value; 842 return this; 843 } 844 845 /** 846 * @return Indicates whether the event succeeded or failed. 847 */ 848 public AuditEventOutcome getOutcome() { 849 return this.outcome == null ? null : this.outcome.getValue(); 850 } 851 852 /** 853 * @param value Indicates whether the event succeeded or failed. 854 */ 855 public AuditEventEventComponent setOutcome(AuditEventOutcome value) { 856 if (value == null) 857 this.outcome = null; 858 else { 859 if (this.outcome == null) 860 this.outcome = new Enumeration<AuditEventOutcome>(new AuditEventOutcomeEnumFactory()); 861 this.outcome.setValue(value); 862 } 863 return this; 864 } 865 866 /** 867 * @return {@link #outcomeDesc} (A free text description of the outcome of the 868 * event.). This is the underlying object with id, value and extensions. 869 * The accessor "getOutcomeDesc" gives direct access to the value 870 */ 871 public StringType getOutcomeDescElement() { 872 if (this.outcomeDesc == null) 873 if (Configuration.errorOnAutoCreate()) 874 throw new Error("Attempt to auto-create AuditEventEventComponent.outcomeDesc"); 875 else if (Configuration.doAutoCreate()) 876 this.outcomeDesc = new StringType(); // bb 877 return this.outcomeDesc; 878 } 879 880 public boolean hasOutcomeDescElement() { 881 return this.outcomeDesc != null && !this.outcomeDesc.isEmpty(); 882 } 883 884 public boolean hasOutcomeDesc() { 885 return this.outcomeDesc != null && !this.outcomeDesc.isEmpty(); 886 } 887 888 /** 889 * @param value {@link #outcomeDesc} (A free text description of the outcome of 890 * the event.). This is the underlying object with id, value and 891 * extensions. The accessor "getOutcomeDesc" gives direct access to 892 * the value 893 */ 894 public AuditEventEventComponent setOutcomeDescElement(StringType value) { 895 this.outcomeDesc = value; 896 return this; 897 } 898 899 /** 900 * @return A free text description of the outcome of the event. 901 */ 902 public String getOutcomeDesc() { 903 return this.outcomeDesc == null ? null : this.outcomeDesc.getValue(); 904 } 905 906 /** 907 * @param value A free text description of the outcome of the event. 908 */ 909 public AuditEventEventComponent setOutcomeDesc(String value) { 910 if (Utilities.noString(value)) 911 this.outcomeDesc = null; 912 else { 913 if (this.outcomeDesc == null) 914 this.outcomeDesc = new StringType(); 915 this.outcomeDesc.setValue(value); 916 } 917 return this; 918 } 919 920 /** 921 * @return {@link #purposeOfEvent} (The purposeOfUse (reason) that was used 922 * during the event being recorded.) 923 */ 924 public List<Coding> getPurposeOfEvent() { 925 if (this.purposeOfEvent == null) 926 this.purposeOfEvent = new ArrayList<Coding>(); 927 return this.purposeOfEvent; 928 } 929 930 public boolean hasPurposeOfEvent() { 931 if (this.purposeOfEvent == null) 932 return false; 933 for (Coding item : this.purposeOfEvent) 934 if (!item.isEmpty()) 935 return true; 936 return false; 937 } 938 939 /** 940 * @return {@link #purposeOfEvent} (The purposeOfUse (reason) that was used 941 * during the event being recorded.) 942 */ 943 // syntactic sugar 944 public Coding addPurposeOfEvent() { // 3 945 Coding t = new Coding(); 946 if (this.purposeOfEvent == null) 947 this.purposeOfEvent = new ArrayList<Coding>(); 948 this.purposeOfEvent.add(t); 949 return t; 950 } 951 952 // syntactic sugar 953 public AuditEventEventComponent addPurposeOfEvent(Coding t) { // 3 954 if (t == null) 955 return this; 956 if (this.purposeOfEvent == null) 957 this.purposeOfEvent = new ArrayList<Coding>(); 958 this.purposeOfEvent.add(t); 959 return this; 960 } 961 962 protected void listChildren(List<Property> childrenList) { 963 super.listChildren(childrenList); 964 childrenList.add(new Property("type", "Coding", 965 "Identifier for a family of the event. For example, a menu item, program, rule, policy, function code, application name or URL. It identifies the performed function.", 966 0, java.lang.Integer.MAX_VALUE, type)); 967 childrenList.add(new Property("subtype", "Coding", "Identifier for the category of event.", 0, 968 java.lang.Integer.MAX_VALUE, subtype)); 969 childrenList.add(new Property("action", "code", 970 "Indicator for type of action performed during the event that generated the audit.", 0, 971 java.lang.Integer.MAX_VALUE, action)); 972 childrenList.add(new Property("dateTime", "instant", "The time when the event occurred on the source.", 0, 973 java.lang.Integer.MAX_VALUE, dateTime)); 974 childrenList.add(new Property("outcome", "code", "Indicates whether the event succeeded or failed.", 0, 975 java.lang.Integer.MAX_VALUE, outcome)); 976 childrenList.add(new Property("outcomeDesc", "string", "A free text description of the outcome of the event.", 0, 977 java.lang.Integer.MAX_VALUE, outcomeDesc)); 978 childrenList.add(new Property("purposeOfEvent", "Coding", 979 "The purposeOfUse (reason) that was used during the event being recorded.", 0, java.lang.Integer.MAX_VALUE, 980 purposeOfEvent)); 981 } 982 983 @Override 984 public void setProperty(String name, Base value) throws FHIRException { 985 if (name.equals("type")) 986 this.type = castToCoding(value); // Coding 987 else if (name.equals("subtype")) 988 this.getSubtype().add(castToCoding(value)); 989 else if (name.equals("action")) 990 this.action = new AuditEventActionEnumFactory().fromType(value); // Enumeration<AuditEventAction> 991 else if (name.equals("dateTime")) 992 this.dateTime = castToInstant(value); // InstantType 993 else if (name.equals("outcome")) 994 this.outcome = new AuditEventOutcomeEnumFactory().fromType(value); // Enumeration<AuditEventOutcome> 995 else if (name.equals("outcomeDesc")) 996 this.outcomeDesc = castToString(value); // StringType 997 else if (name.equals("purposeOfEvent")) 998 this.getPurposeOfEvent().add(castToCoding(value)); 999 else 1000 super.setProperty(name, value); 1001 } 1002 1003 @Override 1004 public Base addChild(String name) throws FHIRException { 1005 if (name.equals("type")) { 1006 this.type = new Coding(); 1007 return this.type; 1008 } else if (name.equals("subtype")) { 1009 return addSubtype(); 1010 } else if (name.equals("action")) { 1011 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.action"); 1012 } else if (name.equals("dateTime")) { 1013 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.dateTime"); 1014 } else if (name.equals("outcome")) { 1015 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.outcome"); 1016 } else if (name.equals("outcomeDesc")) { 1017 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.outcomeDesc"); 1018 } else if (name.equals("purposeOfEvent")) { 1019 return addPurposeOfEvent(); 1020 } else 1021 return super.addChild(name); 1022 } 1023 1024 public AuditEventEventComponent copy() { 1025 AuditEventEventComponent dst = new AuditEventEventComponent(); 1026 copyValues(dst); 1027 dst.type = type == null ? null : type.copy(); 1028 if (subtype != null) { 1029 dst.subtype = new ArrayList<Coding>(); 1030 for (Coding i : subtype) 1031 dst.subtype.add(i.copy()); 1032 } 1033 ; 1034 dst.action = action == null ? null : action.copy(); 1035 dst.dateTime = dateTime == null ? null : dateTime.copy(); 1036 dst.outcome = outcome == null ? null : outcome.copy(); 1037 dst.outcomeDesc = outcomeDesc == null ? null : outcomeDesc.copy(); 1038 if (purposeOfEvent != null) { 1039 dst.purposeOfEvent = new ArrayList<Coding>(); 1040 for (Coding i : purposeOfEvent) 1041 dst.purposeOfEvent.add(i.copy()); 1042 } 1043 ; 1044 return dst; 1045 } 1046 1047 @Override 1048 public boolean equalsDeep(Base other) { 1049 if (!super.equalsDeep(other)) 1050 return false; 1051 if (!(other instanceof AuditEventEventComponent)) 1052 return false; 1053 AuditEventEventComponent o = (AuditEventEventComponent) other; 1054 return compareDeep(type, o.type, true) && compareDeep(subtype, o.subtype, true) 1055 && compareDeep(action, o.action, true) && compareDeep(dateTime, o.dateTime, true) 1056 && compareDeep(outcome, o.outcome, true) && compareDeep(outcomeDesc, o.outcomeDesc, true) 1057 && compareDeep(purposeOfEvent, o.purposeOfEvent, true); 1058 } 1059 1060 @Override 1061 public boolean equalsShallow(Base other) { 1062 if (!super.equalsShallow(other)) 1063 return false; 1064 if (!(other instanceof AuditEventEventComponent)) 1065 return false; 1066 AuditEventEventComponent o = (AuditEventEventComponent) other; 1067 return compareValues(action, o.action, true) && compareValues(dateTime, o.dateTime, true) 1068 && compareValues(outcome, o.outcome, true) && compareValues(outcomeDesc, o.outcomeDesc, true); 1069 } 1070 1071 public boolean isEmpty() { 1072 return super.isEmpty() && (type == null || type.isEmpty()) && (subtype == null || subtype.isEmpty()) 1073 && (action == null || action.isEmpty()) && (dateTime == null || dateTime.isEmpty()) 1074 && (outcome == null || outcome.isEmpty()) && (outcomeDesc == null || outcomeDesc.isEmpty()) 1075 && (purposeOfEvent == null || purposeOfEvent.isEmpty()); 1076 } 1077 1078 public String fhirType() { 1079 return "AuditEvent.event"; 1080 1081 } 1082 1083 } 1084 1085 @Block() 1086 public static class AuditEventParticipantComponent extends BackboneElement implements IBaseBackboneElement { 1087 /** 1088 * Specification of the role(s) the user plays when performing the event. 1089 * Usually the codes used in this element are local codes defined by the 1090 * role-based access control security system used in the local context. 1091 */ 1092 @Child(name = "role", type = { 1093 CodeableConcept.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1094 @Description(shortDefinition = "User roles (e.g. local RBAC codes)", formalDefinition = "Specification of the role(s) the user plays when performing the event. Usually the codes used in this element are local codes defined by the role-based access control security system used in the local context.") 1095 protected List<CodeableConcept> role; 1096 1097 /** 1098 * Direct reference to a resource that identifies the participant. 1099 */ 1100 @Child(name = "reference", type = { Practitioner.class, Organization.class, Device.class, Patient.class, 1101 RelatedPerson.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1102 @Description(shortDefinition = "Direct reference to resource", formalDefinition = "Direct reference to a resource that identifies the participant.") 1103 protected Reference reference; 1104 1105 /** 1106 * The actual object that is the target of the reference (Direct reference to a 1107 * resource that identifies the participant.) 1108 */ 1109 protected Resource referenceTarget; 1110 1111 /** 1112 * Unique identifier for the user actively participating in the event. 1113 */ 1114 @Child(name = "userId", type = { Identifier.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1115 @Description(shortDefinition = "Unique identifier for the user", formalDefinition = "Unique identifier for the user actively participating in the event.") 1116 protected Identifier userId; 1117 1118 /** 1119 * Alternative Participant Identifier. For a human, this should be a user 1120 * identifier text string from authentication system. This identifier would be 1121 * one known to a common authentication system (e.g. single sign-on), if 1122 * available. 1123 */ 1124 @Child(name = "altId", type = { StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 1125 @Description(shortDefinition = "Alternative User id e.g. authentication", formalDefinition = "Alternative Participant Identifier. For a human, this should be a user identifier text string from authentication system. This identifier would be one known to a common authentication system (e.g. single sign-on), if available.") 1126 protected StringType altId; 1127 1128 /** 1129 * Human-meaningful name for the user. 1130 */ 1131 @Child(name = "name", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 1132 @Description(shortDefinition = "Human-meaningful name for the user", formalDefinition = "Human-meaningful name for the user.") 1133 protected StringType name; 1134 1135 /** 1136 * Indicator that the user is or is not the requestor, or initiator, for the 1137 * event being audited. 1138 */ 1139 @Child(name = "requestor", type = { 1140 BooleanType.class }, order = 6, min = 1, max = 1, modifier = false, summary = false) 1141 @Description(shortDefinition = "Whether user is initiator", formalDefinition = "Indicator that the user is or is not the requestor, or initiator, for the event being audited.") 1142 protected BooleanType requestor; 1143 1144 /** 1145 * Where the event occurred. 1146 */ 1147 @Child(name = "location", type = { Location.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1148 @Description(shortDefinition = "Where", formalDefinition = "Where the event occurred.") 1149 protected Reference location; 1150 1151 /** 1152 * The actual object that is the target of the reference (Where the event 1153 * occurred.) 1154 */ 1155 protected Location locationTarget; 1156 1157 /** 1158 * The policy or plan that authorized the activity being recorded. Typically, a 1159 * single activity may have multiple applicable policies, such as patient 1160 * consent, guarantor funding, etc. The policy would also indicate the security 1161 * token used. 1162 */ 1163 @Child(name = "policy", type = { 1164 UriType.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1165 @Description(shortDefinition = "Policy that authorized event", formalDefinition = "The policy or plan that authorized the activity being recorded. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.") 1166 protected List<UriType> policy; 1167 1168 /** 1169 * Type of media involved. Used when the event is about exporting/importing onto 1170 * media. 1171 */ 1172 @Child(name = "media", type = { Coding.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1173 @Description(shortDefinition = "Type of media", formalDefinition = "Type of media involved. Used when the event is about exporting/importing onto media.") 1174 protected Coding media; 1175 1176 /** 1177 * Logical network location for application activity, if the activity has a 1178 * network location. 1179 */ 1180 @Child(name = "network", type = {}, order = 10, min = 0, max = 1, modifier = false, summary = false) 1181 @Description(shortDefinition = "Logical network location for application activity", formalDefinition = "Logical network location for application activity, if the activity has a network location.") 1182 protected AuditEventParticipantNetworkComponent network; 1183 1184 /** 1185 * The reason (purpose of use), specific to this participant, that was used 1186 * during the event being recorded. 1187 */ 1188 @Child(name = "purposeOfUse", type = { 1189 Coding.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1190 @Description(shortDefinition = "Reason given for this user", formalDefinition = "The reason (purpose of use), specific to this participant, that was used during the event being recorded.") 1191 protected List<Coding> purposeOfUse; 1192 1193 private static final long serialVersionUID = -1783296995L; 1194 1195 /* 1196 * Constructor 1197 */ 1198 public AuditEventParticipantComponent() { 1199 super(); 1200 } 1201 1202 /* 1203 * Constructor 1204 */ 1205 public AuditEventParticipantComponent(BooleanType requestor) { 1206 super(); 1207 this.requestor = requestor; 1208 } 1209 1210 /** 1211 * @return {@link #role} (Specification of the role(s) the user plays when 1212 * performing the event. Usually the codes used in this element are 1213 * local codes defined by the role-based access control security system 1214 * used in the local context.) 1215 */ 1216 public List<CodeableConcept> getRole() { 1217 if (this.role == null) 1218 this.role = new ArrayList<CodeableConcept>(); 1219 return this.role; 1220 } 1221 1222 public boolean hasRole() { 1223 if (this.role == null) 1224 return false; 1225 for (CodeableConcept item : this.role) 1226 if (!item.isEmpty()) 1227 return true; 1228 return false; 1229 } 1230 1231 /** 1232 * @return {@link #role} (Specification of the role(s) the user plays when 1233 * performing the event. Usually the codes used in this element are 1234 * local codes defined by the role-based access control security system 1235 * used in the local context.) 1236 */ 1237 // syntactic sugar 1238 public CodeableConcept addRole() { // 3 1239 CodeableConcept t = new CodeableConcept(); 1240 if (this.role == null) 1241 this.role = new ArrayList<CodeableConcept>(); 1242 this.role.add(t); 1243 return t; 1244 } 1245 1246 // syntactic sugar 1247 public AuditEventParticipantComponent addRole(CodeableConcept t) { // 3 1248 if (t == null) 1249 return this; 1250 if (this.role == null) 1251 this.role = new ArrayList<CodeableConcept>(); 1252 this.role.add(t); 1253 return this; 1254 } 1255 1256 /** 1257 * @return {@link #reference} (Direct reference to a resource that identifies 1258 * the participant.) 1259 */ 1260 public Reference getReference() { 1261 if (this.reference == null) 1262 if (Configuration.errorOnAutoCreate()) 1263 throw new Error("Attempt to auto-create AuditEventParticipantComponent.reference"); 1264 else if (Configuration.doAutoCreate()) 1265 this.reference = new Reference(); // cc 1266 return this.reference; 1267 } 1268 1269 public boolean hasReference() { 1270 return this.reference != null && !this.reference.isEmpty(); 1271 } 1272 1273 /** 1274 * @param value {@link #reference} (Direct reference to a resource that 1275 * identifies the participant.) 1276 */ 1277 public AuditEventParticipantComponent setReference(Reference value) { 1278 this.reference = value; 1279 return this; 1280 } 1281 1282 /** 1283 * @return {@link #reference} The actual object that is the target of the 1284 * reference. The reference library doesn't populate this, but you can 1285 * use it to hold the resource if you resolve it. (Direct reference to a 1286 * resource that identifies the participant.) 1287 */ 1288 public Resource getReferenceTarget() { 1289 return this.referenceTarget; 1290 } 1291 1292 /** 1293 * @param value {@link #reference} The actual object that is the target of the 1294 * reference. The reference library doesn't use these, but you can 1295 * use it to hold the resource if you resolve it. (Direct reference 1296 * to a resource that identifies the participant.) 1297 */ 1298 public AuditEventParticipantComponent setReferenceTarget(Resource value) { 1299 this.referenceTarget = value; 1300 return this; 1301 } 1302 1303 /** 1304 * @return {@link #userId} (Unique identifier for the user actively 1305 * participating in the event.) 1306 */ 1307 public Identifier getUserId() { 1308 if (this.userId == null) 1309 if (Configuration.errorOnAutoCreate()) 1310 throw new Error("Attempt to auto-create AuditEventParticipantComponent.userId"); 1311 else if (Configuration.doAutoCreate()) 1312 this.userId = new Identifier(); // cc 1313 return this.userId; 1314 } 1315 1316 public boolean hasUserId() { 1317 return this.userId != null && !this.userId.isEmpty(); 1318 } 1319 1320 /** 1321 * @param value {@link #userId} (Unique identifier for the user actively 1322 * participating in the event.) 1323 */ 1324 public AuditEventParticipantComponent setUserId(Identifier value) { 1325 this.userId = value; 1326 return this; 1327 } 1328 1329 /** 1330 * @return {@link #altId} (Alternative Participant Identifier. For a human, this 1331 * should be a user identifier text string from authentication system. 1332 * This identifier would be one known to a common authentication system 1333 * (e.g. single sign-on), if available.). This is the underlying object 1334 * with id, value and extensions. The accessor "getAltId" gives direct 1335 * access to the value 1336 */ 1337 public StringType getAltIdElement() { 1338 if (this.altId == null) 1339 if (Configuration.errorOnAutoCreate()) 1340 throw new Error("Attempt to auto-create AuditEventParticipantComponent.altId"); 1341 else if (Configuration.doAutoCreate()) 1342 this.altId = new StringType(); // bb 1343 return this.altId; 1344 } 1345 1346 public boolean hasAltIdElement() { 1347 return this.altId != null && !this.altId.isEmpty(); 1348 } 1349 1350 public boolean hasAltId() { 1351 return this.altId != null && !this.altId.isEmpty(); 1352 } 1353 1354 /** 1355 * @param value {@link #altId} (Alternative Participant Identifier. For a human, 1356 * this should be a user identifier text string from authentication 1357 * system. This identifier would be one known to a common 1358 * authentication system (e.g. single sign-on), if available.). 1359 * This is the underlying object with id, value and extensions. The 1360 * accessor "getAltId" gives direct access to the value 1361 */ 1362 public AuditEventParticipantComponent setAltIdElement(StringType value) { 1363 this.altId = value; 1364 return this; 1365 } 1366 1367 /** 1368 * @return Alternative Participant Identifier. For a human, this should be a 1369 * user identifier text string from authentication system. This 1370 * identifier would be one known to a common authentication system (e.g. 1371 * single sign-on), if available. 1372 */ 1373 public String getAltId() { 1374 return this.altId == null ? null : this.altId.getValue(); 1375 } 1376 1377 /** 1378 * @param value Alternative Participant Identifier. For a human, this should be 1379 * a user identifier text string from authentication system. This 1380 * identifier would be one known to a common authentication system 1381 * (e.g. single sign-on), if available. 1382 */ 1383 public AuditEventParticipantComponent setAltId(String value) { 1384 if (Utilities.noString(value)) 1385 this.altId = null; 1386 else { 1387 if (this.altId == null) 1388 this.altId = new StringType(); 1389 this.altId.setValue(value); 1390 } 1391 return this; 1392 } 1393 1394 /** 1395 * @return {@link #name} (Human-meaningful name for the user.). This is the 1396 * underlying object with id, value and extensions. The accessor 1397 * "getName" gives direct access to the value 1398 */ 1399 public StringType getNameElement() { 1400 if (this.name == null) 1401 if (Configuration.errorOnAutoCreate()) 1402 throw new Error("Attempt to auto-create AuditEventParticipantComponent.name"); 1403 else if (Configuration.doAutoCreate()) 1404 this.name = new StringType(); // bb 1405 return this.name; 1406 } 1407 1408 public boolean hasNameElement() { 1409 return this.name != null && !this.name.isEmpty(); 1410 } 1411 1412 public boolean hasName() { 1413 return this.name != null && !this.name.isEmpty(); 1414 } 1415 1416 /** 1417 * @param value {@link #name} (Human-meaningful name for the user.). This is the 1418 * underlying object with id, value and extensions. The accessor 1419 * "getName" gives direct access to the value 1420 */ 1421 public AuditEventParticipantComponent setNameElement(StringType value) { 1422 this.name = value; 1423 return this; 1424 } 1425 1426 /** 1427 * @return Human-meaningful name for the user. 1428 */ 1429 public String getName() { 1430 return this.name == null ? null : this.name.getValue(); 1431 } 1432 1433 /** 1434 * @param value Human-meaningful name for the user. 1435 */ 1436 public AuditEventParticipantComponent setName(String value) { 1437 if (Utilities.noString(value)) 1438 this.name = null; 1439 else { 1440 if (this.name == null) 1441 this.name = new StringType(); 1442 this.name.setValue(value); 1443 } 1444 return this; 1445 } 1446 1447 /** 1448 * @return {@link #requestor} (Indicator that the user is or is not the 1449 * requestor, or initiator, for the event being audited.). This is the 1450 * underlying object with id, value and extensions. The accessor 1451 * "getRequestor" gives direct access to the value 1452 */ 1453 public BooleanType getRequestorElement() { 1454 if (this.requestor == null) 1455 if (Configuration.errorOnAutoCreate()) 1456 throw new Error("Attempt to auto-create AuditEventParticipantComponent.requestor"); 1457 else if (Configuration.doAutoCreate()) 1458 this.requestor = new BooleanType(); // bb 1459 return this.requestor; 1460 } 1461 1462 public boolean hasRequestorElement() { 1463 return this.requestor != null && !this.requestor.isEmpty(); 1464 } 1465 1466 public boolean hasRequestor() { 1467 return this.requestor != null && !this.requestor.isEmpty(); 1468 } 1469 1470 /** 1471 * @param value {@link #requestor} (Indicator that the user is or is not the 1472 * requestor, or initiator, for the event being audited.). This is 1473 * the underlying object with id, value and extensions. The 1474 * accessor "getRequestor" gives direct access to the value 1475 */ 1476 public AuditEventParticipantComponent setRequestorElement(BooleanType value) { 1477 this.requestor = value; 1478 return this; 1479 } 1480 1481 /** 1482 * @return Indicator that the user is or is not the requestor, or initiator, for 1483 * the event being audited. 1484 */ 1485 public boolean getRequestor() { 1486 return this.requestor == null || this.requestor.isEmpty() ? false : this.requestor.getValue(); 1487 } 1488 1489 /** 1490 * @param value Indicator that the user is or is not the requestor, or 1491 * initiator, for the event being audited. 1492 */ 1493 public AuditEventParticipantComponent setRequestor(boolean value) { 1494 if (this.requestor == null) 1495 this.requestor = new BooleanType(); 1496 this.requestor.setValue(value); 1497 return this; 1498 } 1499 1500 /** 1501 * @return {@link #location} (Where the event occurred.) 1502 */ 1503 public Reference getLocation() { 1504 if (this.location == null) 1505 if (Configuration.errorOnAutoCreate()) 1506 throw new Error("Attempt to auto-create AuditEventParticipantComponent.location"); 1507 else if (Configuration.doAutoCreate()) 1508 this.location = new Reference(); // cc 1509 return this.location; 1510 } 1511 1512 public boolean hasLocation() { 1513 return this.location != null && !this.location.isEmpty(); 1514 } 1515 1516 /** 1517 * @param value {@link #location} (Where the event occurred.) 1518 */ 1519 public AuditEventParticipantComponent setLocation(Reference value) { 1520 this.location = value; 1521 return this; 1522 } 1523 1524 /** 1525 * @return {@link #location} The actual object that is the target of the 1526 * reference. The reference library doesn't populate this, but you can 1527 * use it to hold the resource if you resolve it. (Where the event 1528 * occurred.) 1529 */ 1530 public Location getLocationTarget() { 1531 if (this.locationTarget == null) 1532 if (Configuration.errorOnAutoCreate()) 1533 throw new Error("Attempt to auto-create AuditEventParticipantComponent.location"); 1534 else if (Configuration.doAutoCreate()) 1535 this.locationTarget = new Location(); // aa 1536 return this.locationTarget; 1537 } 1538 1539 /** 1540 * @param value {@link #location} The actual object that is the target of the 1541 * reference. The reference library doesn't use these, but you can 1542 * use it to hold the resource if you resolve it. (Where the event 1543 * occurred.) 1544 */ 1545 public AuditEventParticipantComponent setLocationTarget(Location value) { 1546 this.locationTarget = value; 1547 return this; 1548 } 1549 1550 /** 1551 * @return {@link #policy} (The policy or plan that authorized the activity 1552 * being recorded. Typically, a single activity may have multiple 1553 * applicable policies, such as patient consent, guarantor funding, etc. 1554 * The policy would also indicate the security token used.) 1555 */ 1556 public List<UriType> getPolicy() { 1557 if (this.policy == null) 1558 this.policy = new ArrayList<UriType>(); 1559 return this.policy; 1560 } 1561 1562 public boolean hasPolicy() { 1563 if (this.policy == null) 1564 return false; 1565 for (UriType item : this.policy) 1566 if (!item.isEmpty()) 1567 return true; 1568 return false; 1569 } 1570 1571 /** 1572 * @return {@link #policy} (The policy or plan that authorized the activity 1573 * being recorded. Typically, a single activity may have multiple 1574 * applicable policies, such as patient consent, guarantor funding, etc. 1575 * The policy would also indicate the security token used.) 1576 */ 1577 // syntactic sugar 1578 public UriType addPolicyElement() {// 2 1579 UriType t = new UriType(); 1580 if (this.policy == null) 1581 this.policy = new ArrayList<UriType>(); 1582 this.policy.add(t); 1583 return t; 1584 } 1585 1586 /** 1587 * @param value {@link #policy} (The policy or plan that authorized the activity 1588 * being recorded. Typically, a single activity may have multiple 1589 * applicable policies, such as patient consent, guarantor funding, 1590 * etc. The policy would also indicate the security token used.) 1591 */ 1592 public AuditEventParticipantComponent addPolicy(String value) { // 1 1593 UriType t = new UriType(); 1594 t.setValue(value); 1595 if (this.policy == null) 1596 this.policy = new ArrayList<UriType>(); 1597 this.policy.add(t); 1598 return this; 1599 } 1600 1601 /** 1602 * @param value {@link #policy} (The policy or plan that authorized the activity 1603 * being recorded. Typically, a single activity may have multiple 1604 * applicable policies, such as patient consent, guarantor funding, 1605 * etc. The policy would also indicate the security token used.) 1606 */ 1607 public boolean hasPolicy(String value) { 1608 if (this.policy == null) 1609 return false; 1610 for (UriType v : this.policy) 1611 if (v.equals(value)) // uri 1612 return true; 1613 return false; 1614 } 1615 1616 /** 1617 * @return {@link #media} (Type of media involved. Used when the event is about 1618 * exporting/importing onto media.) 1619 */ 1620 public Coding getMedia() { 1621 if (this.media == null) 1622 if (Configuration.errorOnAutoCreate()) 1623 throw new Error("Attempt to auto-create AuditEventParticipantComponent.media"); 1624 else if (Configuration.doAutoCreate()) 1625 this.media = new Coding(); // cc 1626 return this.media; 1627 } 1628 1629 public boolean hasMedia() { 1630 return this.media != null && !this.media.isEmpty(); 1631 } 1632 1633 /** 1634 * @param value {@link #media} (Type of media involved. Used when the event is 1635 * about exporting/importing onto media.) 1636 */ 1637 public AuditEventParticipantComponent setMedia(Coding value) { 1638 this.media = value; 1639 return this; 1640 } 1641 1642 /** 1643 * @return {@link #network} (Logical network location for application activity, 1644 * if the activity has a network location.) 1645 */ 1646 public AuditEventParticipantNetworkComponent getNetwork() { 1647 if (this.network == null) 1648 if (Configuration.errorOnAutoCreate()) 1649 throw new Error("Attempt to auto-create AuditEventParticipantComponent.network"); 1650 else if (Configuration.doAutoCreate()) 1651 this.network = new AuditEventParticipantNetworkComponent(); // cc 1652 return this.network; 1653 } 1654 1655 public boolean hasNetwork() { 1656 return this.network != null && !this.network.isEmpty(); 1657 } 1658 1659 /** 1660 * @param value {@link #network} (Logical network location for application 1661 * activity, if the activity has a network location.) 1662 */ 1663 public AuditEventParticipantComponent setNetwork(AuditEventParticipantNetworkComponent value) { 1664 this.network = value; 1665 return this; 1666 } 1667 1668 /** 1669 * @return {@link #purposeOfUse} (The reason (purpose of use), specific to this 1670 * participant, that was used during the event being recorded.) 1671 */ 1672 public List<Coding> getPurposeOfUse() { 1673 if (this.purposeOfUse == null) 1674 this.purposeOfUse = new ArrayList<Coding>(); 1675 return this.purposeOfUse; 1676 } 1677 1678 public boolean hasPurposeOfUse() { 1679 if (this.purposeOfUse == null) 1680 return false; 1681 for (Coding item : this.purposeOfUse) 1682 if (!item.isEmpty()) 1683 return true; 1684 return false; 1685 } 1686 1687 /** 1688 * @return {@link #purposeOfUse} (The reason (purpose of use), specific to this 1689 * participant, that was used during the event being recorded.) 1690 */ 1691 // syntactic sugar 1692 public Coding addPurposeOfUse() { // 3 1693 Coding t = new Coding(); 1694 if (this.purposeOfUse == null) 1695 this.purposeOfUse = new ArrayList<Coding>(); 1696 this.purposeOfUse.add(t); 1697 return t; 1698 } 1699 1700 // syntactic sugar 1701 public AuditEventParticipantComponent addPurposeOfUse(Coding t) { // 3 1702 if (t == null) 1703 return this; 1704 if (this.purposeOfUse == null) 1705 this.purposeOfUse = new ArrayList<Coding>(); 1706 this.purposeOfUse.add(t); 1707 return this; 1708 } 1709 1710 protected void listChildren(List<Property> childrenList) { 1711 super.listChildren(childrenList); 1712 childrenList.add(new Property("role", "CodeableConcept", 1713 "Specification of the role(s) the user plays when performing the event. Usually the codes used in this element are local codes defined by the role-based access control security system used in the local context.", 1714 0, java.lang.Integer.MAX_VALUE, role)); 1715 childrenList.add(new Property("reference", "Reference(Practitioner|Organization|Device|Patient|RelatedPerson)", 1716 "Direct reference to a resource that identifies the participant.", 0, java.lang.Integer.MAX_VALUE, 1717 reference)); 1718 childrenList.add( 1719 new Property("userId", "Identifier", "Unique identifier for the user actively participating in the event.", 0, 1720 java.lang.Integer.MAX_VALUE, userId)); 1721 childrenList.add(new Property("altId", "string", 1722 "Alternative Participant Identifier. For a human, this should be a user identifier text string from authentication system. This identifier would be one known to a common authentication system (e.g. single sign-on), if available.", 1723 0, java.lang.Integer.MAX_VALUE, altId)); 1724 childrenList.add( 1725 new Property("name", "string", "Human-meaningful name for the user.", 0, java.lang.Integer.MAX_VALUE, name)); 1726 childrenList.add(new Property("requestor", "boolean", 1727 "Indicator that the user is or is not the requestor, or initiator, for the event being audited.", 0, 1728 java.lang.Integer.MAX_VALUE, requestor)); 1729 childrenList.add(new Property("location", "Reference(Location)", "Where the event occurred.", 0, 1730 java.lang.Integer.MAX_VALUE, location)); 1731 childrenList.add(new Property("policy", "uri", 1732 "The policy or plan that authorized the activity being recorded. Typically, a single activity may have multiple applicable policies, such as patient consent, guarantor funding, etc. The policy would also indicate the security token used.", 1733 0, java.lang.Integer.MAX_VALUE, policy)); 1734 childrenList.add(new Property("media", "Coding", 1735 "Type of media involved. Used when the event is about exporting/importing onto media.", 0, 1736 java.lang.Integer.MAX_VALUE, media)); 1737 childrenList.add(new Property("network", "", 1738 "Logical network location for application activity, if the activity has a network location.", 0, 1739 java.lang.Integer.MAX_VALUE, network)); 1740 childrenList.add(new Property("purposeOfUse", "Coding", 1741 "The reason (purpose of use), specific to this participant, that was used during the event being recorded.", 1742 0, java.lang.Integer.MAX_VALUE, purposeOfUse)); 1743 } 1744 1745 @Override 1746 public void setProperty(String name, Base value) throws FHIRException { 1747 if (name.equals("role")) 1748 this.getRole().add(castToCodeableConcept(value)); 1749 else if (name.equals("reference")) 1750 this.reference = castToReference(value); // Reference 1751 else if (name.equals("userId")) 1752 this.userId = castToIdentifier(value); // Identifier 1753 else if (name.equals("altId")) 1754 this.altId = castToString(value); // StringType 1755 else if (name.equals("name")) 1756 this.name = castToString(value); // StringType 1757 else if (name.equals("requestor")) 1758 this.requestor = castToBoolean(value); // BooleanType 1759 else if (name.equals("location")) 1760 this.location = castToReference(value); // Reference 1761 else if (name.equals("policy")) 1762 this.getPolicy().add(castToUri(value)); 1763 else if (name.equals("media")) 1764 this.media = castToCoding(value); // Coding 1765 else if (name.equals("network")) 1766 this.network = (AuditEventParticipantNetworkComponent) value; // AuditEventParticipantNetworkComponent 1767 else if (name.equals("purposeOfUse")) 1768 this.getPurposeOfUse().add(castToCoding(value)); 1769 else 1770 super.setProperty(name, value); 1771 } 1772 1773 @Override 1774 public Base addChild(String name) throws FHIRException { 1775 if (name.equals("role")) { 1776 return addRole(); 1777 } else if (name.equals("reference")) { 1778 this.reference = new Reference(); 1779 return this.reference; 1780 } else if (name.equals("userId")) { 1781 this.userId = new Identifier(); 1782 return this.userId; 1783 } else if (name.equals("altId")) { 1784 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.altId"); 1785 } else if (name.equals("name")) { 1786 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.name"); 1787 } else if (name.equals("requestor")) { 1788 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.requestor"); 1789 } else if (name.equals("location")) { 1790 this.location = new Reference(); 1791 return this.location; 1792 } else if (name.equals("policy")) { 1793 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.policy"); 1794 } else if (name.equals("media")) { 1795 this.media = new Coding(); 1796 return this.media; 1797 } else if (name.equals("network")) { 1798 this.network = new AuditEventParticipantNetworkComponent(); 1799 return this.network; 1800 } else if (name.equals("purposeOfUse")) { 1801 return addPurposeOfUse(); 1802 } else 1803 return super.addChild(name); 1804 } 1805 1806 public AuditEventParticipantComponent copy() { 1807 AuditEventParticipantComponent dst = new AuditEventParticipantComponent(); 1808 copyValues(dst); 1809 if (role != null) { 1810 dst.role = new ArrayList<CodeableConcept>(); 1811 for (CodeableConcept i : role) 1812 dst.role.add(i.copy()); 1813 } 1814 ; 1815 dst.reference = reference == null ? null : reference.copy(); 1816 dst.userId = userId == null ? null : userId.copy(); 1817 dst.altId = altId == null ? null : altId.copy(); 1818 dst.name = name == null ? null : name.copy(); 1819 dst.requestor = requestor == null ? null : requestor.copy(); 1820 dst.location = location == null ? null : location.copy(); 1821 if (policy != null) { 1822 dst.policy = new ArrayList<UriType>(); 1823 for (UriType i : policy) 1824 dst.policy.add(i.copy()); 1825 } 1826 ; 1827 dst.media = media == null ? null : media.copy(); 1828 dst.network = network == null ? null : network.copy(); 1829 if (purposeOfUse != null) { 1830 dst.purposeOfUse = new ArrayList<Coding>(); 1831 for (Coding i : purposeOfUse) 1832 dst.purposeOfUse.add(i.copy()); 1833 } 1834 ; 1835 return dst; 1836 } 1837 1838 @Override 1839 public boolean equalsDeep(Base other) { 1840 if (!super.equalsDeep(other)) 1841 return false; 1842 if (!(other instanceof AuditEventParticipantComponent)) 1843 return false; 1844 AuditEventParticipantComponent o = (AuditEventParticipantComponent) other; 1845 return compareDeep(role, o.role, true) && compareDeep(reference, o.reference, true) 1846 && compareDeep(userId, o.userId, true) && compareDeep(altId, o.altId, true) && compareDeep(name, o.name, true) 1847 && compareDeep(requestor, o.requestor, true) && compareDeep(location, o.location, true) 1848 && compareDeep(policy, o.policy, true) && compareDeep(media, o.media, true) 1849 && compareDeep(network, o.network, true) && compareDeep(purposeOfUse, o.purposeOfUse, true); 1850 } 1851 1852 @Override 1853 public boolean equalsShallow(Base other) { 1854 if (!super.equalsShallow(other)) 1855 return false; 1856 if (!(other instanceof AuditEventParticipantComponent)) 1857 return false; 1858 AuditEventParticipantComponent o = (AuditEventParticipantComponent) other; 1859 return compareValues(altId, o.altId, true) && compareValues(name, o.name, true) 1860 && compareValues(requestor, o.requestor, true) && compareValues(policy, o.policy, true); 1861 } 1862 1863 public boolean isEmpty() { 1864 return super.isEmpty() && (role == null || role.isEmpty()) && (reference == null || reference.isEmpty()) 1865 && (userId == null || userId.isEmpty()) && (altId == null || altId.isEmpty()) 1866 && (name == null || name.isEmpty()) && (requestor == null || requestor.isEmpty()) 1867 && (location == null || location.isEmpty()) && (policy == null || policy.isEmpty()) 1868 && (media == null || media.isEmpty()) && (network == null || network.isEmpty()) 1869 && (purposeOfUse == null || purposeOfUse.isEmpty()); 1870 } 1871 1872 public String fhirType() { 1873 return "AuditEvent.participant"; 1874 1875 } 1876 1877 } 1878 1879 @Block() 1880 public static class AuditEventParticipantNetworkComponent extends BackboneElement implements IBaseBackboneElement { 1881 /** 1882 * An identifier for the network access point of the user device for the audit 1883 * event. 1884 */ 1885 @Child(name = "address", type = { 1886 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1887 @Description(shortDefinition = "Identifier for the network access point of the user device", formalDefinition = "An identifier for the network access point of the user device for the audit event.") 1888 protected StringType address; 1889 1890 /** 1891 * An identifier for the type of network access point that originated the audit 1892 * event. 1893 */ 1894 @Child(name = "type", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1895 @Description(shortDefinition = "The type of network access point", formalDefinition = "An identifier for the type of network access point that originated the audit event.") 1896 protected Enumeration<AuditEventParticipantNetworkType> type; 1897 1898 private static final long serialVersionUID = -1355220390L; 1899 1900 /* 1901 * Constructor 1902 */ 1903 public AuditEventParticipantNetworkComponent() { 1904 super(); 1905 } 1906 1907 /** 1908 * @return {@link #address} (An identifier for the network access point of the 1909 * user device for the audit event.). This is the underlying object with 1910 * id, value and extensions. The accessor "getAddress" gives direct 1911 * access to the value 1912 */ 1913 public StringType getAddressElement() { 1914 if (this.address == null) 1915 if (Configuration.errorOnAutoCreate()) 1916 throw new Error("Attempt to auto-create AuditEventParticipantNetworkComponent.address"); 1917 else if (Configuration.doAutoCreate()) 1918 this.address = new StringType(); // bb 1919 return this.address; 1920 } 1921 1922 public boolean hasAddressElement() { 1923 return this.address != null && !this.address.isEmpty(); 1924 } 1925 1926 public boolean hasAddress() { 1927 return this.address != null && !this.address.isEmpty(); 1928 } 1929 1930 /** 1931 * @param value {@link #address} (An identifier for the network access point of 1932 * the user device for the audit event.). This is the underlying 1933 * object with id, value and extensions. The accessor "getAddress" 1934 * gives direct access to the value 1935 */ 1936 public AuditEventParticipantNetworkComponent setAddressElement(StringType value) { 1937 this.address = value; 1938 return this; 1939 } 1940 1941 /** 1942 * @return An identifier for the network access point of the user device for the 1943 * audit event. 1944 */ 1945 public String getAddress() { 1946 return this.address == null ? null : this.address.getValue(); 1947 } 1948 1949 /** 1950 * @param value An identifier for the network access point of the user device 1951 * for the audit event. 1952 */ 1953 public AuditEventParticipantNetworkComponent setAddress(String value) { 1954 if (Utilities.noString(value)) 1955 this.address = null; 1956 else { 1957 if (this.address == null) 1958 this.address = new StringType(); 1959 this.address.setValue(value); 1960 } 1961 return this; 1962 } 1963 1964 /** 1965 * @return {@link #type} (An identifier for the type of network access point 1966 * that originated the audit event.). This is the underlying object with 1967 * id, value and extensions. The accessor "getType" gives direct access 1968 * to the value 1969 */ 1970 public Enumeration<AuditEventParticipantNetworkType> getTypeElement() { 1971 if (this.type == null) 1972 if (Configuration.errorOnAutoCreate()) 1973 throw new Error("Attempt to auto-create AuditEventParticipantNetworkComponent.type"); 1974 else if (Configuration.doAutoCreate()) 1975 this.type = new Enumeration<AuditEventParticipantNetworkType>( 1976 new AuditEventParticipantNetworkTypeEnumFactory()); // bb 1977 return this.type; 1978 } 1979 1980 public boolean hasTypeElement() { 1981 return this.type != null && !this.type.isEmpty(); 1982 } 1983 1984 public boolean hasType() { 1985 return this.type != null && !this.type.isEmpty(); 1986 } 1987 1988 /** 1989 * @param value {@link #type} (An identifier for the type of network access 1990 * point that originated the audit event.). This is the underlying 1991 * object with id, value and extensions. The accessor "getType" 1992 * gives direct access to the value 1993 */ 1994 public AuditEventParticipantNetworkComponent setTypeElement(Enumeration<AuditEventParticipantNetworkType> value) { 1995 this.type = value; 1996 return this; 1997 } 1998 1999 /** 2000 * @return An identifier for the type of network access point that originated 2001 * the audit event. 2002 */ 2003 public AuditEventParticipantNetworkType getType() { 2004 return this.type == null ? null : this.type.getValue(); 2005 } 2006 2007 /** 2008 * @param value An identifier for the type of network access point that 2009 * originated the audit event. 2010 */ 2011 public AuditEventParticipantNetworkComponent setType(AuditEventParticipantNetworkType value) { 2012 if (value == null) 2013 this.type = null; 2014 else { 2015 if (this.type == null) 2016 this.type = new Enumeration<AuditEventParticipantNetworkType>( 2017 new AuditEventParticipantNetworkTypeEnumFactory()); 2018 this.type.setValue(value); 2019 } 2020 return this; 2021 } 2022 2023 protected void listChildren(List<Property> childrenList) { 2024 super.listChildren(childrenList); 2025 childrenList.add(new Property("address", "string", 2026 "An identifier for the network access point of the user device for the audit event.", 0, 2027 java.lang.Integer.MAX_VALUE, address)); 2028 childrenList.add(new Property("type", "code", 2029 "An identifier for the type of network access point that originated the audit event.", 0, 2030 java.lang.Integer.MAX_VALUE, type)); 2031 } 2032 2033 @Override 2034 public void setProperty(String name, Base value) throws FHIRException { 2035 if (name.equals("address")) 2036 this.address = castToString(value); // StringType 2037 else if (name.equals("type")) 2038 this.type = new AuditEventParticipantNetworkTypeEnumFactory().fromType(value); // Enumeration<AuditEventParticipantNetworkType> 2039 else 2040 super.setProperty(name, value); 2041 } 2042 2043 @Override 2044 public Base addChild(String name) throws FHIRException { 2045 if (name.equals("address")) { 2046 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.address"); 2047 } else if (name.equals("type")) { 2048 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.type"); 2049 } else 2050 return super.addChild(name); 2051 } 2052 2053 public AuditEventParticipantNetworkComponent copy() { 2054 AuditEventParticipantNetworkComponent dst = new AuditEventParticipantNetworkComponent(); 2055 copyValues(dst); 2056 dst.address = address == null ? null : address.copy(); 2057 dst.type = type == null ? null : type.copy(); 2058 return dst; 2059 } 2060 2061 @Override 2062 public boolean equalsDeep(Base other) { 2063 if (!super.equalsDeep(other)) 2064 return false; 2065 if (!(other instanceof AuditEventParticipantNetworkComponent)) 2066 return false; 2067 AuditEventParticipantNetworkComponent o = (AuditEventParticipantNetworkComponent) other; 2068 return compareDeep(address, o.address, true) && compareDeep(type, o.type, true); 2069 } 2070 2071 @Override 2072 public boolean equalsShallow(Base other) { 2073 if (!super.equalsShallow(other)) 2074 return false; 2075 if (!(other instanceof AuditEventParticipantNetworkComponent)) 2076 return false; 2077 AuditEventParticipantNetworkComponent o = (AuditEventParticipantNetworkComponent) other; 2078 return compareValues(address, o.address, true) && compareValues(type, o.type, true); 2079 } 2080 2081 public boolean isEmpty() { 2082 return super.isEmpty() && (address == null || address.isEmpty()) && (type == null || type.isEmpty()); 2083 } 2084 2085 public String fhirType() { 2086 return "AuditEvent.participant.network"; 2087 2088 } 2089 2090 } 2091 2092 @Block() 2093 public static class AuditEventSourceComponent extends BackboneElement implements IBaseBackboneElement { 2094 /** 2095 * Logical source location within the healthcare enterprise network. For 2096 * example, a hospital or other provider location within a multi-entity provider 2097 * group. 2098 */ 2099 @Child(name = "site", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 2100 @Description(shortDefinition = "Logical source location within the enterprise", formalDefinition = "Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group.") 2101 protected StringType site; 2102 2103 /** 2104 * Identifier of the source where the event was detected. 2105 */ 2106 @Child(name = "identifier", type = { 2107 Identifier.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 2108 @Description(shortDefinition = "The identity of source detecting the event", formalDefinition = "Identifier of the source where the event was detected.") 2109 protected Identifier identifier; 2110 2111 /** 2112 * Code specifying the type of source where event originated. 2113 */ 2114 @Child(name = "type", type = { 2115 Coding.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2116 @Description(shortDefinition = "The type of source where event originated", formalDefinition = "Code specifying the type of source where event originated.") 2117 protected List<Coding> type; 2118 2119 private static final long serialVersionUID = -1562673890L; 2120 2121 /* 2122 * Constructor 2123 */ 2124 public AuditEventSourceComponent() { 2125 super(); 2126 } 2127 2128 /* 2129 * Constructor 2130 */ 2131 public AuditEventSourceComponent(Identifier identifier) { 2132 super(); 2133 this.identifier = identifier; 2134 } 2135 2136 /** 2137 * @return {@link #site} (Logical source location within the healthcare 2138 * enterprise network. For example, a hospital or other provider 2139 * location within a multi-entity provider group.). This is the 2140 * underlying object with id, value and extensions. The accessor 2141 * "getSite" gives direct access to the value 2142 */ 2143 public StringType getSiteElement() { 2144 if (this.site == null) 2145 if (Configuration.errorOnAutoCreate()) 2146 throw new Error("Attempt to auto-create AuditEventSourceComponent.site"); 2147 else if (Configuration.doAutoCreate()) 2148 this.site = new StringType(); // bb 2149 return this.site; 2150 } 2151 2152 public boolean hasSiteElement() { 2153 return this.site != null && !this.site.isEmpty(); 2154 } 2155 2156 public boolean hasSite() { 2157 return this.site != null && !this.site.isEmpty(); 2158 } 2159 2160 /** 2161 * @param value {@link #site} (Logical source location within the healthcare 2162 * enterprise network. For example, a hospital or other provider 2163 * location within a multi-entity provider group.). This is the 2164 * underlying object with id, value and extensions. The accessor 2165 * "getSite" gives direct access to the value 2166 */ 2167 public AuditEventSourceComponent setSiteElement(StringType value) { 2168 this.site = value; 2169 return this; 2170 } 2171 2172 /** 2173 * @return Logical source location within the healthcare enterprise network. For 2174 * example, a hospital or other provider location within a multi-entity 2175 * provider group. 2176 */ 2177 public String getSite() { 2178 return this.site == null ? null : this.site.getValue(); 2179 } 2180 2181 /** 2182 * @param value Logical source location within the healthcare enterprise 2183 * network. For example, a hospital or other provider location 2184 * within a multi-entity provider group. 2185 */ 2186 public AuditEventSourceComponent setSite(String value) { 2187 if (Utilities.noString(value)) 2188 this.site = null; 2189 else { 2190 if (this.site == null) 2191 this.site = new StringType(); 2192 this.site.setValue(value); 2193 } 2194 return this; 2195 } 2196 2197 /** 2198 * @return {@link #identifier} (Identifier of the source where the event was 2199 * detected.) 2200 */ 2201 public Identifier getIdentifier() { 2202 if (this.identifier == null) 2203 if (Configuration.errorOnAutoCreate()) 2204 throw new Error("Attempt to auto-create AuditEventSourceComponent.identifier"); 2205 else if (Configuration.doAutoCreate()) 2206 this.identifier = new Identifier(); // cc 2207 return this.identifier; 2208 } 2209 2210 public boolean hasIdentifier() { 2211 return this.identifier != null && !this.identifier.isEmpty(); 2212 } 2213 2214 /** 2215 * @param value {@link #identifier} (Identifier of the source where the event 2216 * was detected.) 2217 */ 2218 public AuditEventSourceComponent setIdentifier(Identifier value) { 2219 this.identifier = value; 2220 return this; 2221 } 2222 2223 /** 2224 * @return {@link #type} (Code specifying the type of source where event 2225 * originated.) 2226 */ 2227 public List<Coding> getType() { 2228 if (this.type == null) 2229 this.type = new ArrayList<Coding>(); 2230 return this.type; 2231 } 2232 2233 public boolean hasType() { 2234 if (this.type == null) 2235 return false; 2236 for (Coding item : this.type) 2237 if (!item.isEmpty()) 2238 return true; 2239 return false; 2240 } 2241 2242 /** 2243 * @return {@link #type} (Code specifying the type of source where event 2244 * originated.) 2245 */ 2246 // syntactic sugar 2247 public Coding addType() { // 3 2248 Coding t = new Coding(); 2249 if (this.type == null) 2250 this.type = new ArrayList<Coding>(); 2251 this.type.add(t); 2252 return t; 2253 } 2254 2255 // syntactic sugar 2256 public AuditEventSourceComponent addType(Coding t) { // 3 2257 if (t == null) 2258 return this; 2259 if (this.type == null) 2260 this.type = new ArrayList<Coding>(); 2261 this.type.add(t); 2262 return this; 2263 } 2264 2265 protected void listChildren(List<Property> childrenList) { 2266 super.listChildren(childrenList); 2267 childrenList.add(new Property("site", "string", 2268 "Logical source location within the healthcare enterprise network. For example, a hospital or other provider location within a multi-entity provider group.", 2269 0, java.lang.Integer.MAX_VALUE, site)); 2270 childrenList.add(new Property("identifier", "Identifier", 2271 "Identifier of the source where the event was detected.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2272 childrenList.add(new Property("type", "Coding", "Code specifying the type of source where event originated.", 0, 2273 java.lang.Integer.MAX_VALUE, type)); 2274 } 2275 2276 @Override 2277 public void setProperty(String name, Base value) throws FHIRException { 2278 if (name.equals("site")) 2279 this.site = castToString(value); // StringType 2280 else if (name.equals("identifier")) 2281 this.identifier = castToIdentifier(value); // Identifier 2282 else if (name.equals("type")) 2283 this.getType().add(castToCoding(value)); 2284 else 2285 super.setProperty(name, value); 2286 } 2287 2288 @Override 2289 public Base addChild(String name) throws FHIRException { 2290 if (name.equals("site")) { 2291 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.site"); 2292 } else if (name.equals("identifier")) { 2293 this.identifier = new Identifier(); 2294 return this.identifier; 2295 } else if (name.equals("type")) { 2296 return addType(); 2297 } else 2298 return super.addChild(name); 2299 } 2300 2301 public AuditEventSourceComponent copy() { 2302 AuditEventSourceComponent dst = new AuditEventSourceComponent(); 2303 copyValues(dst); 2304 dst.site = site == null ? null : site.copy(); 2305 dst.identifier = identifier == null ? null : identifier.copy(); 2306 if (type != null) { 2307 dst.type = new ArrayList<Coding>(); 2308 for (Coding i : type) 2309 dst.type.add(i.copy()); 2310 } 2311 ; 2312 return dst; 2313 } 2314 2315 @Override 2316 public boolean equalsDeep(Base other) { 2317 if (!super.equalsDeep(other)) 2318 return false; 2319 if (!(other instanceof AuditEventSourceComponent)) 2320 return false; 2321 AuditEventSourceComponent o = (AuditEventSourceComponent) other; 2322 return compareDeep(site, o.site, true) && compareDeep(identifier, o.identifier, true) 2323 && compareDeep(type, o.type, true); 2324 } 2325 2326 @Override 2327 public boolean equalsShallow(Base other) { 2328 if (!super.equalsShallow(other)) 2329 return false; 2330 if (!(other instanceof AuditEventSourceComponent)) 2331 return false; 2332 AuditEventSourceComponent o = (AuditEventSourceComponent) other; 2333 return compareValues(site, o.site, true); 2334 } 2335 2336 public boolean isEmpty() { 2337 return super.isEmpty() && (site == null || site.isEmpty()) && (identifier == null || identifier.isEmpty()) 2338 && (type == null || type.isEmpty()); 2339 } 2340 2341 public String fhirType() { 2342 return "AuditEvent.source"; 2343 2344 } 2345 2346 } 2347 2348 @Block() 2349 public static class AuditEventObjectComponent extends BackboneElement implements IBaseBackboneElement { 2350 /** 2351 * Identifies a specific instance of the participant object. The reference 2352 * should always be version specific. 2353 */ 2354 @Child(name = "identifier", type = { 2355 Identifier.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2356 @Description(shortDefinition = "Specific instance of object (e.g. versioned)", formalDefinition = "Identifies a specific instance of the participant object. The reference should always be version specific.") 2357 protected Identifier identifier; 2358 2359 /** 2360 * Identifies a specific instance of the participant object. The reference 2361 * should always be version specific. 2362 */ 2363 @Child(name = "reference", type = {}, order = 2, min = 0, max = 1, modifier = false, summary = true) 2364 @Description(shortDefinition = "Specific instance of resource (e.g. versioned)", formalDefinition = "Identifies a specific instance of the participant object. The reference should always be version specific.") 2365 protected Reference reference; 2366 2367 /** 2368 * The actual object that is the target of the reference (Identifies a specific 2369 * instance of the participant object. The reference should always be version 2370 * specific.) 2371 */ 2372 protected Resource referenceTarget; 2373 2374 /** 2375 * The type of the object that was involved in this audit event. 2376 */ 2377 @Child(name = "type", type = { Coding.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 2378 @Description(shortDefinition = "Type of object involved", formalDefinition = "The type of the object that was involved in this audit event.") 2379 protected Coding type; 2380 2381 /** 2382 * Code representing the functional application role of Participant Object being 2383 * audited. 2384 */ 2385 @Child(name = "role", type = { Coding.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 2386 @Description(shortDefinition = "What role the Object played", formalDefinition = "Code representing the functional application role of Participant Object being audited.") 2387 protected Coding role; 2388 2389 /** 2390 * Identifier for the data life-cycle stage for the participant object. 2391 */ 2392 @Child(name = "lifecycle", type = { Coding.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 2393 @Description(shortDefinition = "Life-cycle stage for the object", formalDefinition = "Identifier for the data life-cycle stage for the participant object.") 2394 protected Coding lifecycle; 2395 2396 /** 2397 * Denotes security labels for the identified object. 2398 */ 2399 @Child(name = "securityLabel", type = { 2400 Coding.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2401 @Description(shortDefinition = "Security labels applied to the object", formalDefinition = "Denotes security labels for the identified object.") 2402 protected List<Coding> securityLabel; 2403 2404 /** 2405 * An instance-specific descriptor of the Participant Object ID audited, such as 2406 * a person's name. 2407 */ 2408 @Child(name = "name", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 2409 @Description(shortDefinition = "Instance-specific descriptor for Object", formalDefinition = "An instance-specific descriptor of the Participant Object ID audited, such as a person's name.") 2410 protected StringType name; 2411 2412 /** 2413 * Text that describes the object in more detail. 2414 */ 2415 @Child(name = "description", type = { 2416 StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = false) 2417 @Description(shortDefinition = "Descriptive text", formalDefinition = "Text that describes the object in more detail.") 2418 protected StringType description; 2419 2420 /** 2421 * The actual query for a query-type participant object. 2422 */ 2423 @Child(name = "query", type = { 2424 Base64BinaryType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 2425 @Description(shortDefinition = "Actual query for object", formalDefinition = "The actual query for a query-type participant object.") 2426 protected Base64BinaryType query; 2427 2428 /** 2429 * Additional Information about the Object. 2430 */ 2431 @Child(name = "detail", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2432 @Description(shortDefinition = "Additional Information about the Object", formalDefinition = "Additional Information about the Object.") 2433 protected List<AuditEventObjectDetailComponent> detail; 2434 2435 private static final long serialVersionUID = 997591908L; 2436 2437 /* 2438 * Constructor 2439 */ 2440 public AuditEventObjectComponent() { 2441 super(); 2442 } 2443 2444 /** 2445 * @return {@link #identifier} (Identifies a specific instance of the 2446 * participant object. The reference should always be version specific.) 2447 */ 2448 public Identifier getIdentifier() { 2449 if (this.identifier == null) 2450 if (Configuration.errorOnAutoCreate()) 2451 throw new Error("Attempt to auto-create AuditEventObjectComponent.identifier"); 2452 else if (Configuration.doAutoCreate()) 2453 this.identifier = new Identifier(); // cc 2454 return this.identifier; 2455 } 2456 2457 public boolean hasIdentifier() { 2458 return this.identifier != null && !this.identifier.isEmpty(); 2459 } 2460 2461 /** 2462 * @param value {@link #identifier} (Identifies a specific instance of the 2463 * participant object. The reference should always be version 2464 * specific.) 2465 */ 2466 public AuditEventObjectComponent setIdentifier(Identifier value) { 2467 this.identifier = value; 2468 return this; 2469 } 2470 2471 /** 2472 * @return {@link #reference} (Identifies a specific instance of the participant 2473 * object. The reference should always be version specific.) 2474 */ 2475 public Reference getReference() { 2476 if (this.reference == null) 2477 if (Configuration.errorOnAutoCreate()) 2478 throw new Error("Attempt to auto-create AuditEventObjectComponent.reference"); 2479 else if (Configuration.doAutoCreate()) 2480 this.reference = new Reference(); // cc 2481 return this.reference; 2482 } 2483 2484 public boolean hasReference() { 2485 return this.reference != null && !this.reference.isEmpty(); 2486 } 2487 2488 /** 2489 * @param value {@link #reference} (Identifies a specific instance of the 2490 * participant object. The reference should always be version 2491 * specific.) 2492 */ 2493 public AuditEventObjectComponent setReference(Reference value) { 2494 this.reference = value; 2495 return this; 2496 } 2497 2498 /** 2499 * @return {@link #reference} The actual object that is the target of the 2500 * reference. The reference library doesn't populate this, but you can 2501 * use it to hold the resource if you resolve it. (Identifies a specific 2502 * instance of the participant object. The reference should always be 2503 * version specific.) 2504 */ 2505 public Resource getReferenceTarget() { 2506 return this.referenceTarget; 2507 } 2508 2509 /** 2510 * @param value {@link #reference} The actual object that is the target of the 2511 * reference. The reference library doesn't use these, but you can 2512 * use it to hold the resource if you resolve it. (Identifies a 2513 * specific instance of the participant object. The reference 2514 * should always be version specific.) 2515 */ 2516 public AuditEventObjectComponent setReferenceTarget(Resource value) { 2517 this.referenceTarget = value; 2518 return this; 2519 } 2520 2521 /** 2522 * @return {@link #type} (The type of the object that was involved in this audit 2523 * event.) 2524 */ 2525 public Coding getType() { 2526 if (this.type == null) 2527 if (Configuration.errorOnAutoCreate()) 2528 throw new Error("Attempt to auto-create AuditEventObjectComponent.type"); 2529 else if (Configuration.doAutoCreate()) 2530 this.type = new Coding(); // cc 2531 return this.type; 2532 } 2533 2534 public boolean hasType() { 2535 return this.type != null && !this.type.isEmpty(); 2536 } 2537 2538 /** 2539 * @param value {@link #type} (The type of the object that was involved in this 2540 * audit event.) 2541 */ 2542 public AuditEventObjectComponent setType(Coding value) { 2543 this.type = value; 2544 return this; 2545 } 2546 2547 /** 2548 * @return {@link #role} (Code representing the functional application role of 2549 * Participant Object being audited.) 2550 */ 2551 public Coding getRole() { 2552 if (this.role == null) 2553 if (Configuration.errorOnAutoCreate()) 2554 throw new Error("Attempt to auto-create AuditEventObjectComponent.role"); 2555 else if (Configuration.doAutoCreate()) 2556 this.role = new Coding(); // cc 2557 return this.role; 2558 } 2559 2560 public boolean hasRole() { 2561 return this.role != null && !this.role.isEmpty(); 2562 } 2563 2564 /** 2565 * @param value {@link #role} (Code representing the functional application role 2566 * of Participant Object being audited.) 2567 */ 2568 public AuditEventObjectComponent setRole(Coding value) { 2569 this.role = value; 2570 return this; 2571 } 2572 2573 /** 2574 * @return {@link #lifecycle} (Identifier for the data life-cycle stage for the 2575 * participant object.) 2576 */ 2577 public Coding getLifecycle() { 2578 if (this.lifecycle == null) 2579 if (Configuration.errorOnAutoCreate()) 2580 throw new Error("Attempt to auto-create AuditEventObjectComponent.lifecycle"); 2581 else if (Configuration.doAutoCreate()) 2582 this.lifecycle = new Coding(); // cc 2583 return this.lifecycle; 2584 } 2585 2586 public boolean hasLifecycle() { 2587 return this.lifecycle != null && !this.lifecycle.isEmpty(); 2588 } 2589 2590 /** 2591 * @param value {@link #lifecycle} (Identifier for the data life-cycle stage for 2592 * the participant object.) 2593 */ 2594 public AuditEventObjectComponent setLifecycle(Coding value) { 2595 this.lifecycle = value; 2596 return this; 2597 } 2598 2599 /** 2600 * @return {@link #securityLabel} (Denotes security labels for the identified 2601 * object.) 2602 */ 2603 public List<Coding> getSecurityLabel() { 2604 if (this.securityLabel == null) 2605 this.securityLabel = new ArrayList<Coding>(); 2606 return this.securityLabel; 2607 } 2608 2609 public boolean hasSecurityLabel() { 2610 if (this.securityLabel == null) 2611 return false; 2612 for (Coding item : this.securityLabel) 2613 if (!item.isEmpty()) 2614 return true; 2615 return false; 2616 } 2617 2618 /** 2619 * @return {@link #securityLabel} (Denotes security labels for the identified 2620 * object.) 2621 */ 2622 // syntactic sugar 2623 public Coding addSecurityLabel() { // 3 2624 Coding t = new Coding(); 2625 if (this.securityLabel == null) 2626 this.securityLabel = new ArrayList<Coding>(); 2627 this.securityLabel.add(t); 2628 return t; 2629 } 2630 2631 // syntactic sugar 2632 public AuditEventObjectComponent addSecurityLabel(Coding t) { // 3 2633 if (t == null) 2634 return this; 2635 if (this.securityLabel == null) 2636 this.securityLabel = new ArrayList<Coding>(); 2637 this.securityLabel.add(t); 2638 return this; 2639 } 2640 2641 /** 2642 * @return {@link #name} (An instance-specific descriptor of the Participant 2643 * Object ID audited, such as a person's name.). This is the underlying 2644 * object with id, value and extensions. The accessor "getName" gives 2645 * direct access to the value 2646 */ 2647 public StringType getNameElement() { 2648 if (this.name == null) 2649 if (Configuration.errorOnAutoCreate()) 2650 throw new Error("Attempt to auto-create AuditEventObjectComponent.name"); 2651 else if (Configuration.doAutoCreate()) 2652 this.name = new StringType(); // bb 2653 return this.name; 2654 } 2655 2656 public boolean hasNameElement() { 2657 return this.name != null && !this.name.isEmpty(); 2658 } 2659 2660 public boolean hasName() { 2661 return this.name != null && !this.name.isEmpty(); 2662 } 2663 2664 /** 2665 * @param value {@link #name} (An instance-specific descriptor of the 2666 * Participant Object ID audited, such as a person's name.). This 2667 * is the underlying object with id, value and extensions. The 2668 * accessor "getName" gives direct access to the value 2669 */ 2670 public AuditEventObjectComponent setNameElement(StringType value) { 2671 this.name = value; 2672 return this; 2673 } 2674 2675 /** 2676 * @return An instance-specific descriptor of the Participant Object ID audited, 2677 * such as a person's name. 2678 */ 2679 public String getName() { 2680 return this.name == null ? null : this.name.getValue(); 2681 } 2682 2683 /** 2684 * @param value An instance-specific descriptor of the Participant Object ID 2685 * audited, such as a person's name. 2686 */ 2687 public AuditEventObjectComponent setName(String value) { 2688 if (Utilities.noString(value)) 2689 this.name = null; 2690 else { 2691 if (this.name == null) 2692 this.name = new StringType(); 2693 this.name.setValue(value); 2694 } 2695 return this; 2696 } 2697 2698 /** 2699 * @return {@link #description} (Text that describes the object in more 2700 * detail.). This is the underlying object with id, value and 2701 * extensions. The accessor "getDescription" gives direct access to the 2702 * value 2703 */ 2704 public StringType getDescriptionElement() { 2705 if (this.description == null) 2706 if (Configuration.errorOnAutoCreate()) 2707 throw new Error("Attempt to auto-create AuditEventObjectComponent.description"); 2708 else if (Configuration.doAutoCreate()) 2709 this.description = new StringType(); // bb 2710 return this.description; 2711 } 2712 2713 public boolean hasDescriptionElement() { 2714 return this.description != null && !this.description.isEmpty(); 2715 } 2716 2717 public boolean hasDescription() { 2718 return this.description != null && !this.description.isEmpty(); 2719 } 2720 2721 /** 2722 * @param value {@link #description} (Text that describes the object in more 2723 * detail.). This is the underlying object with id, value and 2724 * extensions. The accessor "getDescription" gives direct access to 2725 * the value 2726 */ 2727 public AuditEventObjectComponent setDescriptionElement(StringType value) { 2728 this.description = value; 2729 return this; 2730 } 2731 2732 /** 2733 * @return Text that describes the object in more detail. 2734 */ 2735 public String getDescription() { 2736 return this.description == null ? null : this.description.getValue(); 2737 } 2738 2739 /** 2740 * @param value Text that describes the object in more detail. 2741 */ 2742 public AuditEventObjectComponent setDescription(String value) { 2743 if (Utilities.noString(value)) 2744 this.description = null; 2745 else { 2746 if (this.description == null) 2747 this.description = new StringType(); 2748 this.description.setValue(value); 2749 } 2750 return this; 2751 } 2752 2753 /** 2754 * @return {@link #query} (The actual query for a query-type participant 2755 * object.). This is the underlying object with id, value and 2756 * extensions. The accessor "getQuery" gives direct access to the value 2757 */ 2758 public Base64BinaryType getQueryElement() { 2759 if (this.query == null) 2760 if (Configuration.errorOnAutoCreate()) 2761 throw new Error("Attempt to auto-create AuditEventObjectComponent.query"); 2762 else if (Configuration.doAutoCreate()) 2763 this.query = new Base64BinaryType(); // bb 2764 return this.query; 2765 } 2766 2767 public boolean hasQueryElement() { 2768 return this.query != null && !this.query.isEmpty(); 2769 } 2770 2771 public boolean hasQuery() { 2772 return this.query != null && !this.query.isEmpty(); 2773 } 2774 2775 /** 2776 * @param value {@link #query} (The actual query for a query-type participant 2777 * object.). This is the underlying object with id, value and 2778 * extensions. The accessor "getQuery" gives direct access to the 2779 * value 2780 */ 2781 public AuditEventObjectComponent setQueryElement(Base64BinaryType value) { 2782 this.query = value; 2783 return this; 2784 } 2785 2786 /** 2787 * @return The actual query for a query-type participant object. 2788 */ 2789 public byte[] getQuery() { 2790 return this.query == null ? null : this.query.getValue(); 2791 } 2792 2793 /** 2794 * @param value The actual query for a query-type participant object. 2795 */ 2796 public AuditEventObjectComponent setQuery(byte[] value) { 2797 if (value == null) 2798 this.query = null; 2799 else { 2800 if (this.query == null) 2801 this.query = new Base64BinaryType(); 2802 this.query.setValue(value); 2803 } 2804 return this; 2805 } 2806 2807 /** 2808 * @return {@link #detail} (Additional Information about the Object.) 2809 */ 2810 public List<AuditEventObjectDetailComponent> getDetail() { 2811 if (this.detail == null) 2812 this.detail = new ArrayList<AuditEventObjectDetailComponent>(); 2813 return this.detail; 2814 } 2815 2816 public boolean hasDetail() { 2817 if (this.detail == null) 2818 return false; 2819 for (AuditEventObjectDetailComponent item : this.detail) 2820 if (!item.isEmpty()) 2821 return true; 2822 return false; 2823 } 2824 2825 /** 2826 * @return {@link #detail} (Additional Information about the Object.) 2827 */ 2828 // syntactic sugar 2829 public AuditEventObjectDetailComponent addDetail() { // 3 2830 AuditEventObjectDetailComponent t = new AuditEventObjectDetailComponent(); 2831 if (this.detail == null) 2832 this.detail = new ArrayList<AuditEventObjectDetailComponent>(); 2833 this.detail.add(t); 2834 return t; 2835 } 2836 2837 // syntactic sugar 2838 public AuditEventObjectComponent addDetail(AuditEventObjectDetailComponent t) { // 3 2839 if (t == null) 2840 return this; 2841 if (this.detail == null) 2842 this.detail = new ArrayList<AuditEventObjectDetailComponent>(); 2843 this.detail.add(t); 2844 return this; 2845 } 2846 2847 protected void listChildren(List<Property> childrenList) { 2848 super.listChildren(childrenList); 2849 childrenList.add(new Property("identifier", "Identifier", 2850 "Identifies a specific instance of the participant object. The reference should always be version specific.", 2851 0, java.lang.Integer.MAX_VALUE, identifier)); 2852 childrenList.add(new Property("reference", "Reference(Any)", 2853 "Identifies a specific instance of the participant object. The reference should always be version specific.", 2854 0, java.lang.Integer.MAX_VALUE, reference)); 2855 childrenList.add(new Property("type", "Coding", "The type of the object that was involved in this audit event.", 2856 0, java.lang.Integer.MAX_VALUE, type)); 2857 childrenList.add(new Property("role", "Coding", 2858 "Code representing the functional application role of Participant Object being audited.", 0, 2859 java.lang.Integer.MAX_VALUE, role)); 2860 childrenList.add( 2861 new Property("lifecycle", "Coding", "Identifier for the data life-cycle stage for the participant object.", 0, 2862 java.lang.Integer.MAX_VALUE, lifecycle)); 2863 childrenList.add(new Property("securityLabel", "Coding", "Denotes security labels for the identified object.", 0, 2864 java.lang.Integer.MAX_VALUE, securityLabel)); 2865 childrenList.add(new Property("name", "string", 2866 "An instance-specific descriptor of the Participant Object ID audited, such as a person's name.", 0, 2867 java.lang.Integer.MAX_VALUE, name)); 2868 childrenList.add(new Property("description", "string", "Text that describes the object in more detail.", 0, 2869 java.lang.Integer.MAX_VALUE, description)); 2870 childrenList.add(new Property("query", "base64Binary", "The actual query for a query-type participant object.", 0, 2871 java.lang.Integer.MAX_VALUE, query)); 2872 childrenList.add(new Property("detail", "", "Additional Information about the Object.", 0, 2873 java.lang.Integer.MAX_VALUE, detail)); 2874 } 2875 2876 @Override 2877 public void setProperty(String name, Base value) throws FHIRException { 2878 if (name.equals("identifier")) 2879 this.identifier = castToIdentifier(value); // Identifier 2880 else if (name.equals("reference")) 2881 this.reference = castToReference(value); // Reference 2882 else if (name.equals("type")) 2883 this.type = castToCoding(value); // Coding 2884 else if (name.equals("role")) 2885 this.role = castToCoding(value); // Coding 2886 else if (name.equals("lifecycle")) 2887 this.lifecycle = castToCoding(value); // Coding 2888 else if (name.equals("securityLabel")) 2889 this.getSecurityLabel().add(castToCoding(value)); 2890 else if (name.equals("name")) 2891 this.name = castToString(value); // StringType 2892 else if (name.equals("description")) 2893 this.description = castToString(value); // StringType 2894 else if (name.equals("query")) 2895 this.query = castToBase64Binary(value); // Base64BinaryType 2896 else if (name.equals("detail")) 2897 this.getDetail().add((AuditEventObjectDetailComponent) value); 2898 else 2899 super.setProperty(name, value); 2900 } 2901 2902 @Override 2903 public Base addChild(String name) throws FHIRException { 2904 if (name.equals("identifier")) { 2905 this.identifier = new Identifier(); 2906 return this.identifier; 2907 } else if (name.equals("reference")) { 2908 this.reference = new Reference(); 2909 return this.reference; 2910 } else if (name.equals("type")) { 2911 this.type = new Coding(); 2912 return this.type; 2913 } else if (name.equals("role")) { 2914 this.role = new Coding(); 2915 return this.role; 2916 } else if (name.equals("lifecycle")) { 2917 this.lifecycle = new Coding(); 2918 return this.lifecycle; 2919 } else if (name.equals("securityLabel")) { 2920 return addSecurityLabel(); 2921 } else if (name.equals("name")) { 2922 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.name"); 2923 } else if (name.equals("description")) { 2924 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.description"); 2925 } else if (name.equals("query")) { 2926 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.query"); 2927 } else if (name.equals("detail")) { 2928 return addDetail(); 2929 } else 2930 return super.addChild(name); 2931 } 2932 2933 public AuditEventObjectComponent copy() { 2934 AuditEventObjectComponent dst = new AuditEventObjectComponent(); 2935 copyValues(dst); 2936 dst.identifier = identifier == null ? null : identifier.copy(); 2937 dst.reference = reference == null ? null : reference.copy(); 2938 dst.type = type == null ? null : type.copy(); 2939 dst.role = role == null ? null : role.copy(); 2940 dst.lifecycle = lifecycle == null ? null : lifecycle.copy(); 2941 if (securityLabel != null) { 2942 dst.securityLabel = new ArrayList<Coding>(); 2943 for (Coding i : securityLabel) 2944 dst.securityLabel.add(i.copy()); 2945 } 2946 ; 2947 dst.name = name == null ? null : name.copy(); 2948 dst.description = description == null ? null : description.copy(); 2949 dst.query = query == null ? null : query.copy(); 2950 if (detail != null) { 2951 dst.detail = new ArrayList<AuditEventObjectDetailComponent>(); 2952 for (AuditEventObjectDetailComponent i : detail) 2953 dst.detail.add(i.copy()); 2954 } 2955 ; 2956 return dst; 2957 } 2958 2959 @Override 2960 public boolean equalsDeep(Base other) { 2961 if (!super.equalsDeep(other)) 2962 return false; 2963 if (!(other instanceof AuditEventObjectComponent)) 2964 return false; 2965 AuditEventObjectComponent o = (AuditEventObjectComponent) other; 2966 return compareDeep(identifier, o.identifier, true) && compareDeep(reference, o.reference, true) 2967 && compareDeep(type, o.type, true) && compareDeep(role, o.role, true) 2968 && compareDeep(lifecycle, o.lifecycle, true) && compareDeep(securityLabel, o.securityLabel, true) 2969 && compareDeep(name, o.name, true) && compareDeep(description, o.description, true) 2970 && compareDeep(query, o.query, true) && compareDeep(detail, o.detail, true); 2971 } 2972 2973 @Override 2974 public boolean equalsShallow(Base other) { 2975 if (!super.equalsShallow(other)) 2976 return false; 2977 if (!(other instanceof AuditEventObjectComponent)) 2978 return false; 2979 AuditEventObjectComponent o = (AuditEventObjectComponent) other; 2980 return compareValues(name, o.name, true) && compareValues(description, o.description, true) 2981 && compareValues(query, o.query, true); 2982 } 2983 2984 public boolean isEmpty() { 2985 return super.isEmpty() && (identifier == null || identifier.isEmpty()) 2986 && (reference == null || reference.isEmpty()) && (type == null || type.isEmpty()) 2987 && (role == null || role.isEmpty()) && (lifecycle == null || lifecycle.isEmpty()) 2988 && (securityLabel == null || securityLabel.isEmpty()) && (name == null || name.isEmpty()) 2989 && (description == null || description.isEmpty()) && (query == null || query.isEmpty()) 2990 && (detail == null || detail.isEmpty()); 2991 } 2992 2993 public String fhirType() { 2994 return "AuditEvent.object"; 2995 2996 } 2997 2998 } 2999 3000 @Block() 3001 public static class AuditEventObjectDetailComponent extends BackboneElement implements IBaseBackboneElement { 3002 /** 3003 * Name of the property. 3004 */ 3005 @Child(name = "type", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 3006 @Description(shortDefinition = "Name of the property", formalDefinition = "Name of the property.") 3007 protected StringType type; 3008 3009 /** 3010 * Property value. 3011 */ 3012 @Child(name = "value", type = { 3013 Base64BinaryType.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 3014 @Description(shortDefinition = "Property value", formalDefinition = "Property value.") 3015 protected Base64BinaryType value; 3016 3017 private static final long serialVersionUID = 11139504L; 3018 3019 /* 3020 * Constructor 3021 */ 3022 public AuditEventObjectDetailComponent() { 3023 super(); 3024 } 3025 3026 /* 3027 * Constructor 3028 */ 3029 public AuditEventObjectDetailComponent(StringType type, Base64BinaryType value) { 3030 super(); 3031 this.type = type; 3032 this.value = value; 3033 } 3034 3035 /** 3036 * @return {@link #type} (Name of the property.). This is the underlying object 3037 * with id, value and extensions. The accessor "getType" gives direct 3038 * access to the value 3039 */ 3040 public StringType getTypeElement() { 3041 if (this.type == null) 3042 if (Configuration.errorOnAutoCreate()) 3043 throw new Error("Attempt to auto-create AuditEventObjectDetailComponent.type"); 3044 else if (Configuration.doAutoCreate()) 3045 this.type = new StringType(); // bb 3046 return this.type; 3047 } 3048 3049 public boolean hasTypeElement() { 3050 return this.type != null && !this.type.isEmpty(); 3051 } 3052 3053 public boolean hasType() { 3054 return this.type != null && !this.type.isEmpty(); 3055 } 3056 3057 /** 3058 * @param value {@link #type} (Name of the property.). This is the underlying 3059 * object with id, value and extensions. The accessor "getType" 3060 * gives direct access to the value 3061 */ 3062 public AuditEventObjectDetailComponent setTypeElement(StringType value) { 3063 this.type = value; 3064 return this; 3065 } 3066 3067 /** 3068 * @return Name of the property. 3069 */ 3070 public String getType() { 3071 return this.type == null ? null : this.type.getValue(); 3072 } 3073 3074 /** 3075 * @param value Name of the property. 3076 */ 3077 public AuditEventObjectDetailComponent setType(String value) { 3078 if (this.type == null) 3079 this.type = new StringType(); 3080 this.type.setValue(value); 3081 return this; 3082 } 3083 3084 /** 3085 * @return {@link #value} (Property value.). This is the underlying object with 3086 * id, value and extensions. The accessor "getValue" gives direct access 3087 * to the value 3088 */ 3089 public Base64BinaryType getValueElement() { 3090 if (this.value == null) 3091 if (Configuration.errorOnAutoCreate()) 3092 throw new Error("Attempt to auto-create AuditEventObjectDetailComponent.value"); 3093 else if (Configuration.doAutoCreate()) 3094 this.value = new Base64BinaryType(); // bb 3095 return this.value; 3096 } 3097 3098 public boolean hasValueElement() { 3099 return this.value != null && !this.value.isEmpty(); 3100 } 3101 3102 public boolean hasValue() { 3103 return this.value != null && !this.value.isEmpty(); 3104 } 3105 3106 /** 3107 * @param value {@link #value} (Property value.). This is the underlying object 3108 * with id, value and extensions. The accessor "getValue" gives 3109 * direct access to the value 3110 */ 3111 public AuditEventObjectDetailComponent setValueElement(Base64BinaryType value) { 3112 this.value = value; 3113 return this; 3114 } 3115 3116 /** 3117 * @return Property value. 3118 */ 3119 public byte[] getValue() { 3120 return this.value == null ? null : this.value.getValue(); 3121 } 3122 3123 /** 3124 * @param value Property value. 3125 */ 3126 public AuditEventObjectDetailComponent setValue(byte[] value) { 3127 if (this.value == null) 3128 this.value = new Base64BinaryType(); 3129 this.value.setValue(value); 3130 return this; 3131 } 3132 3133 protected void listChildren(List<Property> childrenList) { 3134 super.listChildren(childrenList); 3135 childrenList.add(new Property("type", "string", "Name of the property.", 0, java.lang.Integer.MAX_VALUE, type)); 3136 childrenList.add(new Property("value", "base64Binary", "Property value.", 0, java.lang.Integer.MAX_VALUE, value)); 3137 } 3138 3139 @Override 3140 public void setProperty(String name, Base value) throws FHIRException { 3141 if (name.equals("type")) 3142 this.type = castToString(value); // StringType 3143 else if (name.equals("value")) 3144 this.value = castToBase64Binary(value); // Base64BinaryType 3145 else 3146 super.setProperty(name, value); 3147 } 3148 3149 @Override 3150 public Base addChild(String name) throws FHIRException { 3151 if (name.equals("type")) { 3152 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.type"); 3153 } else if (name.equals("value")) { 3154 throw new FHIRException("Cannot call addChild on a singleton property AuditEvent.value"); 3155 } else 3156 return super.addChild(name); 3157 } 3158 3159 public AuditEventObjectDetailComponent copy() { 3160 AuditEventObjectDetailComponent dst = new AuditEventObjectDetailComponent(); 3161 copyValues(dst); 3162 dst.type = type == null ? null : type.copy(); 3163 dst.value = value == null ? null : value.copy(); 3164 return dst; 3165 } 3166 3167 @Override 3168 public boolean equalsDeep(Base other) { 3169 if (!super.equalsDeep(other)) 3170 return false; 3171 if (!(other instanceof AuditEventObjectDetailComponent)) 3172 return false; 3173 AuditEventObjectDetailComponent o = (AuditEventObjectDetailComponent) other; 3174 return compareDeep(type, o.type, true) && compareDeep(value, o.value, true); 3175 } 3176 3177 @Override 3178 public boolean equalsShallow(Base other) { 3179 if (!super.equalsShallow(other)) 3180 return false; 3181 if (!(other instanceof AuditEventObjectDetailComponent)) 3182 return false; 3183 AuditEventObjectDetailComponent o = (AuditEventObjectDetailComponent) other; 3184 return compareValues(type, o.type, true) && compareValues(value, o.value, true); 3185 } 3186 3187 public boolean isEmpty() { 3188 return super.isEmpty() && (type == null || type.isEmpty()) && (value == null || value.isEmpty()); 3189 } 3190 3191 public String fhirType() { 3192 return "AuditEvent.object.detail"; 3193 3194 } 3195 3196 } 3197 3198 /** 3199 * Identifies the name, action type, time, and disposition of the audited event. 3200 */ 3201 @Child(name = "event", type = {}, order = 0, min = 1, max = 1, modifier = false, summary = false) 3202 @Description(shortDefinition = "What was done", formalDefinition = "Identifies the name, action type, time, and disposition of the audited event.") 3203 protected AuditEventEventComponent event; 3204 3205 /** 3206 * A person, a hardware device or software process. 3207 */ 3208 @Child(name = "participant", type = {}, order = 1, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3209 @Description(shortDefinition = "A person, a hardware device or software process", formalDefinition = "A person, a hardware device or software process.") 3210 protected List<AuditEventParticipantComponent> participant; 3211 3212 /** 3213 * Application systems and processes. 3214 */ 3215 @Child(name = "source", type = {}, order = 2, min = 1, max = 1, modifier = false, summary = false) 3216 @Description(shortDefinition = "Application systems and processes", formalDefinition = "Application systems and processes.") 3217 protected AuditEventSourceComponent source; 3218 3219 /** 3220 * Specific instances of data or objects that have been accessed. 3221 */ 3222 @Child(name = "object", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 3223 @Description(shortDefinition = "Specific instances of data or objects that have been accessed", formalDefinition = "Specific instances of data or objects that have been accessed.") 3224 protected List<AuditEventObjectComponent> object; 3225 3226 private static final long serialVersionUID = -1495151000L; 3227 3228 /* 3229 * Constructor 3230 */ 3231 public AuditEvent() { 3232 super(); 3233 } 3234 3235 /* 3236 * Constructor 3237 */ 3238 public AuditEvent(AuditEventEventComponent event, AuditEventSourceComponent source) { 3239 super(); 3240 this.event = event; 3241 this.source = source; 3242 } 3243 3244 /** 3245 * @return {@link #event} (Identifies the name, action type, time, and 3246 * disposition of the audited event.) 3247 */ 3248 public AuditEventEventComponent getEvent() { 3249 if (this.event == null) 3250 if (Configuration.errorOnAutoCreate()) 3251 throw new Error("Attempt to auto-create AuditEvent.event"); 3252 else if (Configuration.doAutoCreate()) 3253 this.event = new AuditEventEventComponent(); // cc 3254 return this.event; 3255 } 3256 3257 public boolean hasEvent() { 3258 return this.event != null && !this.event.isEmpty(); 3259 } 3260 3261 /** 3262 * @param value {@link #event} (Identifies the name, action type, time, and 3263 * disposition of the audited event.) 3264 */ 3265 public AuditEvent setEvent(AuditEventEventComponent value) { 3266 this.event = value; 3267 return this; 3268 } 3269 3270 /** 3271 * @return {@link #participant} (A person, a hardware device or software 3272 * process.) 3273 */ 3274 public List<AuditEventParticipantComponent> getParticipant() { 3275 if (this.participant == null) 3276 this.participant = new ArrayList<AuditEventParticipantComponent>(); 3277 return this.participant; 3278 } 3279 3280 public boolean hasParticipant() { 3281 if (this.participant == null) 3282 return false; 3283 for (AuditEventParticipantComponent item : this.participant) 3284 if (!item.isEmpty()) 3285 return true; 3286 return false; 3287 } 3288 3289 /** 3290 * @return {@link #participant} (A person, a hardware device or software 3291 * process.) 3292 */ 3293 // syntactic sugar 3294 public AuditEventParticipantComponent addParticipant() { // 3 3295 AuditEventParticipantComponent t = new AuditEventParticipantComponent(); 3296 if (this.participant == null) 3297 this.participant = new ArrayList<AuditEventParticipantComponent>(); 3298 this.participant.add(t); 3299 return t; 3300 } 3301 3302 // syntactic sugar 3303 public AuditEvent addParticipant(AuditEventParticipantComponent t) { // 3 3304 if (t == null) 3305 return this; 3306 if (this.participant == null) 3307 this.participant = new ArrayList<AuditEventParticipantComponent>(); 3308 this.participant.add(t); 3309 return this; 3310 } 3311 3312 /** 3313 * @return {@link #source} (Application systems and processes.) 3314 */ 3315 public AuditEventSourceComponent getSource() { 3316 if (this.source == null) 3317 if (Configuration.errorOnAutoCreate()) 3318 throw new Error("Attempt to auto-create AuditEvent.source"); 3319 else if (Configuration.doAutoCreate()) 3320 this.source = new AuditEventSourceComponent(); // cc 3321 return this.source; 3322 } 3323 3324 public boolean hasSource() { 3325 return this.source != null && !this.source.isEmpty(); 3326 } 3327 3328 /** 3329 * @param value {@link #source} (Application systems and processes.) 3330 */ 3331 public AuditEvent setSource(AuditEventSourceComponent value) { 3332 this.source = value; 3333 return this; 3334 } 3335 3336 /** 3337 * @return {@link #object} (Specific instances of data or objects that have been 3338 * accessed.) 3339 */ 3340 public List<AuditEventObjectComponent> getObject() { 3341 if (this.object == null) 3342 this.object = new ArrayList<AuditEventObjectComponent>(); 3343 return this.object; 3344 } 3345 3346 public boolean hasObject() { 3347 if (this.object == null) 3348 return false; 3349 for (AuditEventObjectComponent item : this.object) 3350 if (!item.isEmpty()) 3351 return true; 3352 return false; 3353 } 3354 3355 /** 3356 * @return {@link #object} (Specific instances of data or objects that have been 3357 * accessed.) 3358 */ 3359 // syntactic sugar 3360 public AuditEventObjectComponent addObject() { // 3 3361 AuditEventObjectComponent t = new AuditEventObjectComponent(); 3362 if (this.object == null) 3363 this.object = new ArrayList<AuditEventObjectComponent>(); 3364 this.object.add(t); 3365 return t; 3366 } 3367 3368 // syntactic sugar 3369 public AuditEvent addObject(AuditEventObjectComponent t) { // 3 3370 if (t == null) 3371 return this; 3372 if (this.object == null) 3373 this.object = new ArrayList<AuditEventObjectComponent>(); 3374 this.object.add(t); 3375 return this; 3376 } 3377 3378 protected void listChildren(List<Property> childrenList) { 3379 super.listChildren(childrenList); 3380 childrenList 3381 .add(new Property("event", "", "Identifies the name, action type, time, and disposition of the audited event.", 3382 0, java.lang.Integer.MAX_VALUE, event)); 3383 childrenList.add(new Property("participant", "", "A person, a hardware device or software process.", 0, 3384 java.lang.Integer.MAX_VALUE, participant)); 3385 childrenList 3386 .add(new Property("source", "", "Application systems and processes.", 0, java.lang.Integer.MAX_VALUE, source)); 3387 childrenList.add(new Property("object", "", "Specific instances of data or objects that have been accessed.", 0, 3388 java.lang.Integer.MAX_VALUE, object)); 3389 } 3390 3391 @Override 3392 public void setProperty(String name, Base value) throws FHIRException { 3393 if (name.equals("event")) 3394 this.event = (AuditEventEventComponent) value; // AuditEventEventComponent 3395 else if (name.equals("participant")) 3396 this.getParticipant().add((AuditEventParticipantComponent) value); 3397 else if (name.equals("source")) 3398 this.source = (AuditEventSourceComponent) value; // AuditEventSourceComponent 3399 else if (name.equals("object")) 3400 this.getObject().add((AuditEventObjectComponent) value); 3401 else 3402 super.setProperty(name, value); 3403 } 3404 3405 @Override 3406 public Base addChild(String name) throws FHIRException { 3407 if (name.equals("event")) { 3408 this.event = new AuditEventEventComponent(); 3409 return this.event; 3410 } else if (name.equals("participant")) { 3411 return addParticipant(); 3412 } else if (name.equals("source")) { 3413 this.source = new AuditEventSourceComponent(); 3414 return this.source; 3415 } else if (name.equals("object")) { 3416 return addObject(); 3417 } else 3418 return super.addChild(name); 3419 } 3420 3421 public String fhirType() { 3422 return "AuditEvent"; 3423 3424 } 3425 3426 public AuditEvent copy() { 3427 AuditEvent dst = new AuditEvent(); 3428 copyValues(dst); 3429 dst.event = event == null ? null : event.copy(); 3430 if (participant != null) { 3431 dst.participant = new ArrayList<AuditEventParticipantComponent>(); 3432 for (AuditEventParticipantComponent i : participant) 3433 dst.participant.add(i.copy()); 3434 } 3435 ; 3436 dst.source = source == null ? null : source.copy(); 3437 if (object != null) { 3438 dst.object = new ArrayList<AuditEventObjectComponent>(); 3439 for (AuditEventObjectComponent i : object) 3440 dst.object.add(i.copy()); 3441 } 3442 ; 3443 return dst; 3444 } 3445 3446 protected AuditEvent typedCopy() { 3447 return copy(); 3448 } 3449 3450 @Override 3451 public boolean equalsDeep(Base other) { 3452 if (!super.equalsDeep(other)) 3453 return false; 3454 if (!(other instanceof AuditEvent)) 3455 return false; 3456 AuditEvent o = (AuditEvent) other; 3457 return compareDeep(event, o.event, true) && compareDeep(participant, o.participant, true) 3458 && compareDeep(source, o.source, true) && compareDeep(object, o.object, true); 3459 } 3460 3461 @Override 3462 public boolean equalsShallow(Base other) { 3463 if (!super.equalsShallow(other)) 3464 return false; 3465 if (!(other instanceof AuditEvent)) 3466 return false; 3467 AuditEvent o = (AuditEvent) other; 3468 return true; 3469 } 3470 3471 public boolean isEmpty() { 3472 return super.isEmpty() && (event == null || event.isEmpty()) && (participant == null || participant.isEmpty()) 3473 && (source == null || source.isEmpty()) && (object == null || object.isEmpty()); 3474 } 3475 3476 @Override 3477 public ResourceType getResourceType() { 3478 return ResourceType.AuditEvent; 3479 } 3480 3481 @SearchParamDefinition(name = "date", path = "AuditEvent.event.dateTime", description = "Time when the event occurred on source", type = "date") 3482 public static final String SP_DATE = "date"; 3483 @SearchParamDefinition(name = "address", path = "AuditEvent.participant.network.address", description = "Identifier for the network access point of the user device", type = "token") 3484 public static final String SP_ADDRESS = "address"; 3485 @SearchParamDefinition(name = "source", path = "AuditEvent.source.identifier", description = "The identity of source detecting the event", type = "token") 3486 public static final String SP_SOURCE = "source"; 3487 @SearchParamDefinition(name = "type", path = "AuditEvent.event.type", description = "Type/identifier of event", type = "token") 3488 public static final String SP_TYPE = "type"; 3489 @SearchParamDefinition(name = "altid", path = "AuditEvent.participant.altId", description = "Alternative User id e.g. authentication", type = "token") 3490 public static final String SP_ALTID = "altid"; 3491 @SearchParamDefinition(name = "participant", path = "AuditEvent.participant.reference", description = "Direct reference to resource", type = "reference") 3492 public static final String SP_PARTICIPANT = "participant"; 3493 @SearchParamDefinition(name = "reference", path = "AuditEvent.object.reference", description = "Specific instance of resource (e.g. versioned)", type = "reference") 3494 public static final String SP_REFERENCE = "reference"; 3495 @SearchParamDefinition(name = "site", path = "AuditEvent.source.site", description = "Logical source location within the enterprise", type = "token") 3496 public static final String SP_SITE = "site"; 3497 @SearchParamDefinition(name = "subtype", path = "AuditEvent.event.subtype", description = "More specific type/id for the event", type = "token") 3498 public static final String SP_SUBTYPE = "subtype"; 3499 @SearchParamDefinition(name = "identity", path = "AuditEvent.object.identifier", description = "Specific instance of object (e.g. versioned)", type = "token") 3500 public static final String SP_IDENTITY = "identity"; 3501 @SearchParamDefinition(name = "patient", path = "AuditEvent.participant.reference | AuditEvent.object.reference", description = "Direct reference to resource", type = "reference") 3502 public static final String SP_PATIENT = "patient"; 3503 @SearchParamDefinition(name = "object-type", path = "AuditEvent.object.type", description = "Type of object involved", type = "token") 3504 public static final String SP_OBJECTTYPE = "object-type"; 3505 @SearchParamDefinition(name = "name", path = "AuditEvent.participant.name", description = "Human-meaningful name for the user", type = "string") 3506 public static final String SP_NAME = "name"; 3507 @SearchParamDefinition(name = "action", path = "AuditEvent.event.action", description = "Type of action performed during the event", type = "token") 3508 public static final String SP_ACTION = "action"; 3509 @SearchParamDefinition(name = "user", path = "AuditEvent.participant.userId", description = "Unique identifier for the user", type = "token") 3510 public static final String SP_USER = "user"; 3511 @SearchParamDefinition(name = "desc", path = "AuditEvent.object.name", description = "Instance-specific descriptor for Object", type = "string") 3512 public static final String SP_DESC = "desc"; 3513 @SearchParamDefinition(name = "policy", path = "AuditEvent.participant.policy", description = "Policy that authorized event", type = "uri") 3514 public static final String SP_POLICY = "policy"; 3515 3516}