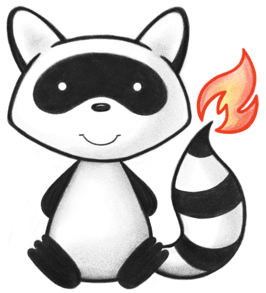
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Child; 037import ca.uhn.fhir.model.api.annotation.DatatypeDef; 038import ca.uhn.fhir.model.api.annotation.Description; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040import org.hl7.fhir.exceptions.FHIRException; 041 042/** 043 * Base definition for all elements that are defined inside a resource - but not 044 * those in a data type. 045 */ 046@DatatypeDef(name = "BackboneElement") 047public abstract class BackboneElement extends Element implements IBaseBackboneElement { 048 049 /** 050 * May be used to represent additional information that is not part of the basic 051 * definition of the element, and that modifies the understanding of the element 052 * that contains it. Usually modifier elements provide negation or 053 * qualification. In order to make the use of extensions safe and manageable, 054 * there is a strict set of governance applied to the definition and use of 055 * extensions. Though any implementer is allowed to define an extension, there 056 * is a set of requirements that SHALL be met as part of the definition of the 057 * extension. Applications processing a resource are required to check for 058 * modifier extensions. 059 */ 060 @Child(name = "modifierExtension", type = { 061 Extension.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = true, summary = false) 062 @Description(shortDefinition = "Extensions that cannot be ignored", formalDefinition = "May be used to represent additional information that is not part of the basic definition of the element, and that modifies the understanding of the element that contains it. Usually modifier elements provide negation or qualification. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.") 063 protected List<Extension> modifierExtension; 064 065 private static final long serialVersionUID = -1431673179L; 066 067 /* 068 * Constructor 069 */ 070 public BackboneElement() { 071 super(); 072 } 073 074 /** 075 * @return {@link #modifierExtension} (May be used to represent additional 076 * information that is not part of the basic definition of the element, 077 * and that modifies the understanding of the element that contains it. 078 * Usually modifier elements provide negation or qualification. In order 079 * to make the use of extensions safe and manageable, there is a strict 080 * set of governance applied to the definition and use of extensions. 081 * Though any implementer is allowed to define an extension, there is a 082 * set of requirements that SHALL be met as part of the definition of 083 * the extension. Applications processing a resource are required to 084 * check for modifier extensions.) 085 */ 086 public List<Extension> getModifierExtension() { 087 if (this.modifierExtension == null) 088 this.modifierExtension = new ArrayList<Extension>(); 089 return this.modifierExtension; 090 } 091 092 public boolean hasModifierExtension() { 093 if (this.modifierExtension == null) 094 return false; 095 for (Extension item : this.modifierExtension) 096 if (!item.isEmpty()) 097 return true; 098 return false; 099 } 100 101 /** 102 * @return {@link #modifierExtension} (May be used to represent additional 103 * information that is not part of the basic definition of the element, 104 * and that modifies the understanding of the element that contains it. 105 * Usually modifier elements provide negation or qualification. In order 106 * to make the use of extensions safe and manageable, there is a strict 107 * set of governance applied to the definition and use of extensions. 108 * Though any implementer is allowed to define an extension, there is a 109 * set of requirements that SHALL be met as part of the definition of 110 * the extension. Applications processing a resource are required to 111 * check for modifier extensions.) 112 */ 113 // syntactic sugar 114 public Extension addModifierExtension() { // 3 115 Extension t = new Extension(); 116 if (this.modifierExtension == null) 117 this.modifierExtension = new ArrayList<Extension>(); 118 this.modifierExtension.add(t); 119 return t; 120 } 121 122 // syntactic sugar 123 public BackboneElement addModifierExtension(Extension t) { // 3 124 if (t == null) 125 return this; 126 if (this.modifierExtension == null) 127 this.modifierExtension = new ArrayList<Extension>(); 128 this.modifierExtension.add(t); 129 return this; 130 } 131 132 protected void listChildren(List<Property> childrenList) { 133 childrenList.add(new Property("modifierExtension", "Extension", 134 "May be used to represent additional information that is not part of the basic definition of the element, and that modifies the understanding of the element that contains it. Usually modifier elements provide negation or qualification. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.", 135 0, java.lang.Integer.MAX_VALUE, modifierExtension)); 136 } 137 138 @Override 139 public void setProperty(String name, Base value) throws FHIRException { 140 if (name.equals("modifierExtension")) 141 this.getModifierExtension().add(castToExtension(value)); 142 else 143 super.setProperty(name, value); 144 } 145 146 @Override 147 public Base addChild(String name) throws FHIRException { 148 if (name.equals("modifierExtension")) { 149 return addModifierExtension(); 150 } else 151 return super.addChild(name); 152 } 153 154 public String fhirType() { 155 return "BackboneElement"; 156 157 } 158 159 public abstract BackboneElement copy(); 160 161 public void copyValues(BackboneElement dst) { 162 super.copyValues(dst); 163 if (modifierExtension != null) { 164 dst.modifierExtension = new ArrayList<Extension>(); 165 for (Extension i : modifierExtension) 166 dst.modifierExtension.add(i.copy()); 167 } 168 ; 169 } 170 171 @Override 172 public boolean equalsDeep(Base other) { 173 if (!super.equalsDeep(other)) 174 return false; 175 if (!(other instanceof BackboneElement)) 176 return false; 177 BackboneElement o = (BackboneElement) other; 178 return compareDeep(modifierExtension, o.modifierExtension, true); 179 } 180 181 @Override 182 public boolean equalsShallow(Base other) { 183 if (!super.equalsShallow(other)) 184 return false; 185 if (!(other instanceof BackboneElement)) 186 return false; 187 BackboneElement o = (BackboneElement) other; 188 return true; 189 } 190 191 public boolean isEmpty() { 192 return super.isEmpty() && (modifierExtension == null || modifierExtension.isEmpty()); 193 } 194 195}