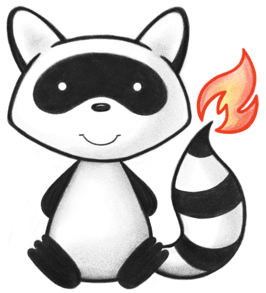
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Child; 038import ca.uhn.fhir.model.api.annotation.Description; 039import ca.uhn.fhir.model.api.annotation.ResourceDef; 040import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 041import org.hl7.fhir.exceptions.FHIRException; 042 043/** 044 * Basic is used for handling concepts not yet defined in FHIR, narrative-only 045 * resources that don't map to an existing resource, and custom resources not 046 * appropriate for inclusion in the FHIR specification. 047 */ 048@ResourceDef(name = "Basic", profile = "http://hl7.org/fhir/Profile/Basic") 049public class Basic extends DomainResource { 050 051 /** 052 * Identifier assigned to the resource for business purposes, outside the 053 * context of FHIR. 054 */ 055 @Child(name = "identifier", type = { 056 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 057 @Description(shortDefinition = "Business identifier", formalDefinition = "Identifier assigned to the resource for business purposes, outside the context of FHIR.") 058 protected List<Identifier> identifier; 059 060 /** 061 * Identifies the 'type' of resource - equivalent to the resource name for other 062 * resources. 063 */ 064 @Child(name = "code", type = { CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 065 @Description(shortDefinition = "Kind of Resource", formalDefinition = "Identifies the 'type' of resource - equivalent to the resource name for other resources.") 066 protected CodeableConcept code; 067 068 /** 069 * Identifies the patient, practitioner, device or any other resource that is 070 * the "focus" of this resource. 071 */ 072 @Child(name = "subject", type = {}, order = 2, min = 0, max = 1, modifier = false, summary = true) 073 @Description(shortDefinition = "Identifies the focus of this resource", formalDefinition = "Identifies the patient, practitioner, device or any other resource that is the \"focus\" of this resource.") 074 protected Reference subject; 075 076 /** 077 * The actual object that is the target of the reference (Identifies the 078 * patient, practitioner, device or any other resource that is the "focus" of 079 * this resource.) 080 */ 081 protected Resource subjectTarget; 082 083 /** 084 * Indicates who was responsible for creating the resource instance. 085 */ 086 @Child(name = "author", type = { Practitioner.class, Patient.class, 087 RelatedPerson.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 088 @Description(shortDefinition = "Who created", formalDefinition = "Indicates who was responsible for creating the resource instance.") 089 protected Reference author; 090 091 /** 092 * The actual object that is the target of the reference (Indicates who was 093 * responsible for creating the resource instance.) 094 */ 095 protected Resource authorTarget; 096 097 /** 098 * Identifies when the resource was first created. 099 */ 100 @Child(name = "created", type = { DateType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 101 @Description(shortDefinition = "When created", formalDefinition = "Identifies when the resource was first created.") 102 protected DateType created; 103 104 private static final long serialVersionUID = 916539354L; 105 106 /* 107 * Constructor 108 */ 109 public Basic() { 110 super(); 111 } 112 113 /* 114 * Constructor 115 */ 116 public Basic(CodeableConcept code) { 117 super(); 118 this.code = code; 119 } 120 121 /** 122 * @return {@link #identifier} (Identifier assigned to the resource for business 123 * purposes, outside the context of FHIR.) 124 */ 125 public List<Identifier> getIdentifier() { 126 if (this.identifier == null) 127 this.identifier = new ArrayList<Identifier>(); 128 return this.identifier; 129 } 130 131 public boolean hasIdentifier() { 132 if (this.identifier == null) 133 return false; 134 for (Identifier item : this.identifier) 135 if (!item.isEmpty()) 136 return true; 137 return false; 138 } 139 140 /** 141 * @return {@link #identifier} (Identifier assigned to the resource for business 142 * purposes, outside the context of FHIR.) 143 */ 144 // syntactic sugar 145 public Identifier addIdentifier() { // 3 146 Identifier t = new Identifier(); 147 if (this.identifier == null) 148 this.identifier = new ArrayList<Identifier>(); 149 this.identifier.add(t); 150 return t; 151 } 152 153 // syntactic sugar 154 public Basic addIdentifier(Identifier t) { // 3 155 if (t == null) 156 return this; 157 if (this.identifier == null) 158 this.identifier = new ArrayList<Identifier>(); 159 this.identifier.add(t); 160 return this; 161 } 162 163 /** 164 * @return {@link #code} (Identifies the 'type' of resource - equivalent to the 165 * resource name for other resources.) 166 */ 167 public CodeableConcept getCode() { 168 if (this.code == null) 169 if (Configuration.errorOnAutoCreate()) 170 throw new Error("Attempt to auto-create Basic.code"); 171 else if (Configuration.doAutoCreate()) 172 this.code = new CodeableConcept(); // cc 173 return this.code; 174 } 175 176 public boolean hasCode() { 177 return this.code != null && !this.code.isEmpty(); 178 } 179 180 /** 181 * @param value {@link #code} (Identifies the 'type' of resource - equivalent to 182 * the resource name for other resources.) 183 */ 184 public Basic setCode(CodeableConcept value) { 185 this.code = value; 186 return this; 187 } 188 189 /** 190 * @return {@link #subject} (Identifies the patient, practitioner, device or any 191 * other resource that is the "focus" of this resource.) 192 */ 193 public Reference getSubject() { 194 if (this.subject == null) 195 if (Configuration.errorOnAutoCreate()) 196 throw new Error("Attempt to auto-create Basic.subject"); 197 else if (Configuration.doAutoCreate()) 198 this.subject = new Reference(); // cc 199 return this.subject; 200 } 201 202 public boolean hasSubject() { 203 return this.subject != null && !this.subject.isEmpty(); 204 } 205 206 /** 207 * @param value {@link #subject} (Identifies the patient, practitioner, device 208 * or any other resource that is the "focus" of this resource.) 209 */ 210 public Basic setSubject(Reference value) { 211 this.subject = value; 212 return this; 213 } 214 215 /** 216 * @return {@link #subject} The actual object that is the target of the 217 * reference. The reference library doesn't populate this, but you can 218 * use it to hold the resource if you resolve it. (Identifies the 219 * patient, practitioner, device or any other resource that is the 220 * "focus" of this resource.) 221 */ 222 public Resource getSubjectTarget() { 223 return this.subjectTarget; 224 } 225 226 /** 227 * @param value {@link #subject} The actual object that is the target of the 228 * reference. The reference library doesn't use these, but you can 229 * use it to hold the resource if you resolve it. (Identifies the 230 * patient, practitioner, device or any other resource that is the 231 * "focus" of this resource.) 232 */ 233 public Basic setSubjectTarget(Resource value) { 234 this.subjectTarget = value; 235 return this; 236 } 237 238 /** 239 * @return {@link #author} (Indicates who was responsible for creating the 240 * resource instance.) 241 */ 242 public Reference getAuthor() { 243 if (this.author == null) 244 if (Configuration.errorOnAutoCreate()) 245 throw new Error("Attempt to auto-create Basic.author"); 246 else if (Configuration.doAutoCreate()) 247 this.author = new Reference(); // cc 248 return this.author; 249 } 250 251 public boolean hasAuthor() { 252 return this.author != null && !this.author.isEmpty(); 253 } 254 255 /** 256 * @param value {@link #author} (Indicates who was responsible for creating the 257 * resource instance.) 258 */ 259 public Basic setAuthor(Reference value) { 260 this.author = value; 261 return this; 262 } 263 264 /** 265 * @return {@link #author} The actual object that is the target of the 266 * reference. The reference library doesn't populate this, but you can 267 * use it to hold the resource if you resolve it. (Indicates who was 268 * responsible for creating the resource instance.) 269 */ 270 public Resource getAuthorTarget() { 271 return this.authorTarget; 272 } 273 274 /** 275 * @param value {@link #author} The actual object that is the target of the 276 * reference. The reference library doesn't use these, but you can 277 * use it to hold the resource if you resolve it. (Indicates who 278 * was responsible for creating the resource instance.) 279 */ 280 public Basic setAuthorTarget(Resource value) { 281 this.authorTarget = value; 282 return this; 283 } 284 285 /** 286 * @return {@link #created} (Identifies when the resource was first created.). 287 * This is the underlying object with id, value and extensions. The 288 * accessor "getCreated" gives direct access to the value 289 */ 290 public DateType getCreatedElement() { 291 if (this.created == null) 292 if (Configuration.errorOnAutoCreate()) 293 throw new Error("Attempt to auto-create Basic.created"); 294 else if (Configuration.doAutoCreate()) 295 this.created = new DateType(); // bb 296 return this.created; 297 } 298 299 public boolean hasCreatedElement() { 300 return this.created != null && !this.created.isEmpty(); 301 } 302 303 public boolean hasCreated() { 304 return this.created != null && !this.created.isEmpty(); 305 } 306 307 /** 308 * @param value {@link #created} (Identifies when the resource was first 309 * created.). This is the underlying object with id, value and 310 * extensions. The accessor "getCreated" gives direct access to the 311 * value 312 */ 313 public Basic setCreatedElement(DateType value) { 314 this.created = value; 315 return this; 316 } 317 318 /** 319 * @return Identifies when the resource was first created. 320 */ 321 public Date getCreated() { 322 return this.created == null ? null : this.created.getValue(); 323 } 324 325 /** 326 * @param value Identifies when the resource was first created. 327 */ 328 public Basic setCreated(Date value) { 329 if (value == null) 330 this.created = null; 331 else { 332 if (this.created == null) 333 this.created = new DateType(); 334 this.created.setValue(value); 335 } 336 return this; 337 } 338 339 protected void listChildren(List<Property> childrenList) { 340 super.listChildren(childrenList); 341 childrenList.add(new Property("identifier", "Identifier", 342 "Identifier assigned to the resource for business purposes, outside the context of FHIR.", 0, 343 java.lang.Integer.MAX_VALUE, identifier)); 344 childrenList.add(new Property("code", "CodeableConcept", 345 "Identifies the 'type' of resource - equivalent to the resource name for other resources.", 0, 346 java.lang.Integer.MAX_VALUE, code)); 347 childrenList.add(new Property("subject", "Reference(Any)", 348 "Identifies the patient, practitioner, device or any other resource that is the \"focus\" of this resource.", 0, 349 java.lang.Integer.MAX_VALUE, subject)); 350 childrenList.add(new Property("author", "Reference(Practitioner|Patient|RelatedPerson)", 351 "Indicates who was responsible for creating the resource instance.", 0, java.lang.Integer.MAX_VALUE, author)); 352 childrenList.add(new Property("created", "date", "Identifies when the resource was first created.", 0, 353 java.lang.Integer.MAX_VALUE, created)); 354 } 355 356 @Override 357 public void setProperty(String name, Base value) throws FHIRException { 358 if (name.equals("identifier")) 359 this.getIdentifier().add(castToIdentifier(value)); 360 else if (name.equals("code")) 361 this.code = castToCodeableConcept(value); // CodeableConcept 362 else if (name.equals("subject")) 363 this.subject = castToReference(value); // Reference 364 else if (name.equals("author")) 365 this.author = castToReference(value); // Reference 366 else if (name.equals("created")) 367 this.created = castToDate(value); // DateType 368 else 369 super.setProperty(name, value); 370 } 371 372 @Override 373 public Base addChild(String name) throws FHIRException { 374 if (name.equals("identifier")) { 375 return addIdentifier(); 376 } else if (name.equals("code")) { 377 this.code = new CodeableConcept(); 378 return this.code; 379 } else if (name.equals("subject")) { 380 this.subject = new Reference(); 381 return this.subject; 382 } else if (name.equals("author")) { 383 this.author = new Reference(); 384 return this.author; 385 } else if (name.equals("created")) { 386 throw new FHIRException("Cannot call addChild on a singleton property Basic.created"); 387 } else 388 return super.addChild(name); 389 } 390 391 public String fhirType() { 392 return "Basic"; 393 394 } 395 396 public Basic copy() { 397 Basic dst = new Basic(); 398 copyValues(dst); 399 if (identifier != null) { 400 dst.identifier = new ArrayList<Identifier>(); 401 for (Identifier i : identifier) 402 dst.identifier.add(i.copy()); 403 } 404 ; 405 dst.code = code == null ? null : code.copy(); 406 dst.subject = subject == null ? null : subject.copy(); 407 dst.author = author == null ? null : author.copy(); 408 dst.created = created == null ? null : created.copy(); 409 return dst; 410 } 411 412 protected Basic typedCopy() { 413 return copy(); 414 } 415 416 @Override 417 public boolean equalsDeep(Base other) { 418 if (!super.equalsDeep(other)) 419 return false; 420 if (!(other instanceof Basic)) 421 return false; 422 Basic o = (Basic) other; 423 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) 424 && compareDeep(subject, o.subject, true) && compareDeep(author, o.author, true) 425 && compareDeep(created, o.created, true); 426 } 427 428 @Override 429 public boolean equalsShallow(Base other) { 430 if (!super.equalsShallow(other)) 431 return false; 432 if (!(other instanceof Basic)) 433 return false; 434 Basic o = (Basic) other; 435 return compareValues(created, o.created, true); 436 } 437 438 public boolean isEmpty() { 439 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (code == null || code.isEmpty()) 440 && (subject == null || subject.isEmpty()) && (author == null || author.isEmpty()) 441 && (created == null || created.isEmpty()); 442 } 443 444 @Override 445 public ResourceType getResourceType() { 446 return ResourceType.Basic; 447 } 448 449 @SearchParamDefinition(name = "identifier", path = "Basic.identifier", description = "Business identifier", type = "token") 450 public static final String SP_IDENTIFIER = "identifier"; 451 @SearchParamDefinition(name = "code", path = "Basic.code", description = "Kind of Resource", type = "token") 452 public static final String SP_CODE = "code"; 453 @SearchParamDefinition(name = "subject", path = "Basic.subject", description = "Identifies the focus of this resource", type = "reference") 454 public static final String SP_SUBJECT = "subject"; 455 @SearchParamDefinition(name = "created", path = "Basic.created", description = "When created", type = "date") 456 public static final String SP_CREATED = "created"; 457 @SearchParamDefinition(name = "patient", path = "Basic.subject", description = "Identifies the focus of this resource", type = "reference") 458 public static final String SP_PATIENT = "patient"; 459 @SearchParamDefinition(name = "author", path = "Basic.author", description = "Who created", type = "reference") 460 public static final String SP_AUTHOR = "author"; 461 462}