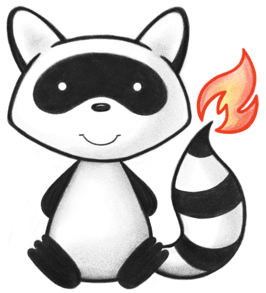
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.List; 034 035import ca.uhn.fhir.model.api.annotation.Child; 036import ca.uhn.fhir.model.api.annotation.Description; 037import ca.uhn.fhir.model.api.annotation.ResourceDef; 038import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 039import org.hl7.fhir.instance.model.api.IBaseBinary; 040import org.hl7.fhir.exceptions.FHIRException; 041 042/** 043 * A binary resource can contain any content, whether text, image, pdf, zip 044 * archive, etc. 045 */ 046@ResourceDef(name = "Binary", profile = "http://hl7.org/fhir/Profile/Binary") 047public class Binary extends BaseBinary implements IBaseBinary { 048 049 /** 050 * MimeType of the binary content represented as a standard MimeType (BCP 13). 051 */ 052 @Child(name = "contentType", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 053 @Description(shortDefinition = "MimeType of the binary content", formalDefinition = "MimeType of the binary content represented as a standard MimeType (BCP 13).") 054 protected CodeType contentType; 055 056 /** 057 * The actual content, base64 encoded. 058 */ 059 @Child(name = "content", type = { 060 Base64BinaryType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 061 @Description(shortDefinition = "The actual content", formalDefinition = "The actual content, base64 encoded.") 062 protected Base64BinaryType content; 063 064 private static final long serialVersionUID = 974764407L; 065 066 /* 067 * Constructor 068 */ 069 public Binary() { 070 super(); 071 } 072 073 /* 074 * Constructor 075 */ 076 public Binary(CodeType contentType, Base64BinaryType content) { 077 super(); 078 this.contentType = contentType; 079 this.content = content; 080 } 081 082 /** 083 * @return {@link #contentType} (MimeType of the binary content represented as a 084 * standard MimeType (BCP 13).). This is the underlying object with id, 085 * value and extensions. The accessor "getContentType" gives direct 086 * access to the value 087 */ 088 public CodeType getContentTypeElement() { 089 if (this.contentType == null) 090 if (Configuration.errorOnAutoCreate()) 091 throw new Error("Attempt to auto-create Binary.contentType"); 092 else if (Configuration.doAutoCreate()) 093 this.contentType = new CodeType(); // bb 094 return this.contentType; 095 } 096 097 public boolean hasContentTypeElement() { 098 return this.contentType != null && !this.contentType.isEmpty(); 099 } 100 101 public boolean hasContentType() { 102 return this.contentType != null && !this.contentType.isEmpty(); 103 } 104 105 /** 106 * @param value {@link #contentType} (MimeType of the binary content represented 107 * as a standard MimeType (BCP 13).). This is the underlying object 108 * with id, value and extensions. The accessor "getContentType" 109 * gives direct access to the value 110 */ 111 public Binary setContentTypeElement(CodeType value) { 112 this.contentType = value; 113 return this; 114 } 115 116 /** 117 * @return MimeType of the binary content represented as a standard MimeType 118 * (BCP 13). 119 */ 120 public String getContentType() { 121 return this.contentType == null ? null : this.contentType.getValue(); 122 } 123 124 /** 125 * @param value MimeType of the binary content represented as a standard 126 * MimeType (BCP 13). 127 */ 128 public Binary setContentType(String value) { 129 if (this.contentType == null) 130 this.contentType = new CodeType(); 131 this.contentType.setValue(value); 132 return this; 133 } 134 135 /** 136 * @return {@link #content} (The actual content, base64 encoded.). This is the 137 * underlying object with id, value and extensions. The accessor 138 * "getContent" gives direct access to the value 139 */ 140 public Base64BinaryType getContentElement() { 141 if (this.content == null) 142 if (Configuration.errorOnAutoCreate()) 143 throw new Error("Attempt to auto-create Binary.content"); 144 else if (Configuration.doAutoCreate()) 145 this.content = new Base64BinaryType(); // bb 146 return this.content; 147 } 148 149 public boolean hasContentElement() { 150 return this.content != null && !this.content.isEmpty(); 151 } 152 153 public boolean hasContent() { 154 return this.content != null && !this.content.isEmpty(); 155 } 156 157 /** 158 * @param value {@link #content} (The actual content, base64 encoded.). This is 159 * the underlying object with id, value and extensions. The 160 * accessor "getContent" gives direct access to the value 161 */ 162 public Binary setContentElement(Base64BinaryType value) { 163 this.content = value; 164 return this; 165 } 166 167 /** 168 * @return The actual content, base64 encoded. 169 */ 170 public byte[] getContent() { 171 return this.content == null ? null : this.content.getValue(); 172 } 173 174 /** 175 * @param value The actual content, base64 encoded. 176 */ 177 public Binary setContent(byte[] value) { 178 if (this.content == null) 179 this.content = new Base64BinaryType(); 180 this.content.setValue(value); 181 return this; 182 } 183 184 protected void listChildren(List<Property> childrenList) { 185 super.listChildren(childrenList); 186 childrenList.add(new Property("contentType", "code", 187 "MimeType of the binary content represented as a standard MimeType (BCP 13).", 0, java.lang.Integer.MAX_VALUE, 188 contentType)); 189 childrenList.add(new Property("content", "base64Binary", "The actual content, base64 encoded.", 0, 190 java.lang.Integer.MAX_VALUE, content)); 191 } 192 193 @Override 194 public void setProperty(String name, Base value) throws FHIRException { 195 if (name.equals("contentType")) 196 this.contentType = castToCode(value); // CodeType 197 else if (name.equals("content")) 198 this.content = castToBase64Binary(value); // Base64BinaryType 199 else 200 super.setProperty(name, value); 201 } 202 203 @Override 204 public Base addChild(String name) throws FHIRException { 205 if (name.equals("contentType")) { 206 throw new FHIRException("Cannot call addChild on a singleton property Binary.contentType"); 207 } else if (name.equals("content")) { 208 throw new FHIRException("Cannot call addChild on a singleton property Binary.content"); 209 } else 210 return super.addChild(name); 211 } 212 213 public String fhirType() { 214 return "Binary"; 215 216 } 217 218 public Binary copy() { 219 Binary dst = new Binary(); 220 copyValues(dst); 221 dst.contentType = contentType == null ? null : contentType.copy(); 222 dst.content = content == null ? null : content.copy(); 223 return dst; 224 } 225 226 protected Binary typedCopy() { 227 return copy(); 228 } 229 230 @Override 231 public boolean equalsDeep(Base other) { 232 if (!super.equalsDeep(other)) 233 return false; 234 if (!(other instanceof Binary)) 235 return false; 236 Binary o = (Binary) other; 237 return compareDeep(contentType, o.contentType, true) && compareDeep(content, o.content, true); 238 } 239 240 @Override 241 public boolean equalsShallow(Base other) { 242 if (!super.equalsShallow(other)) 243 return false; 244 if (!(other instanceof Binary)) 245 return false; 246 Binary o = (Binary) other; 247 return compareValues(contentType, o.contentType, true) && compareValues(content, o.content, true); 248 } 249 250 public boolean isEmpty() { 251 return super.isEmpty() && (contentType == null || contentType.isEmpty()) && (content == null || content.isEmpty()); 252 } 253 254 @Override 255 public ResourceType getResourceType() { 256 return ResourceType.Binary; 257 } 258 259 @SearchParamDefinition(name = "contenttype", path = "Binary.contentType", description = "MimeType of the binary content", type = "token") 260 public static final String SP_CONTENTTYPE = "contenttype"; 261 262}