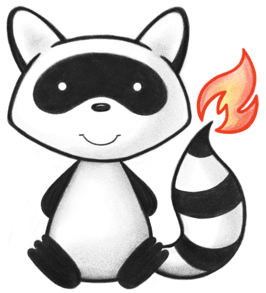
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Child; 037import ca.uhn.fhir.model.api.annotation.Description; 038import ca.uhn.fhir.model.api.annotation.ResourceDef; 039import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.utilities.Utilities; 042 043/** 044 * Record details about the anatomical location of a specimen or body part. This 045 * resource may be used when a coded concept does not provide the necessary 046 * detail needed for the use case. 047 */ 048@ResourceDef(name = "BodySite", profile = "http://hl7.org/fhir/Profile/BodySite") 049public class BodySite extends DomainResource { 050 051 /** 052 * The person to which the body site belongs. 053 */ 054 @Child(name = "patient", type = { Patient.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 055 @Description(shortDefinition = "Patient", formalDefinition = "The person to which the body site belongs.") 056 protected Reference patient; 057 058 /** 059 * The actual object that is the target of the reference (The person to which 060 * the body site belongs.) 061 */ 062 protected Patient patientTarget; 063 064 /** 065 * Identifier for this instance of the anatomical location. 066 */ 067 @Child(name = "identifier", type = { 068 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 069 @Description(shortDefinition = "Bodysite identifier", formalDefinition = "Identifier for this instance of the anatomical location.") 070 protected List<Identifier> identifier; 071 072 /** 073 * Named anatomical location - ideally coded where possible. 074 */ 075 @Child(name = "code", type = { CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 076 @Description(shortDefinition = "Named anatomical location", formalDefinition = "Named anatomical location - ideally coded where possible.") 077 protected CodeableConcept code; 078 079 /** 080 * Modifier to refine the anatomical location. These include modifiers for 081 * laterality, relative location, directionality, number, and plane. 082 */ 083 @Child(name = "modifier", type = { 084 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 085 @Description(shortDefinition = "Modification to location code", formalDefinition = "Modifier to refine the anatomical location. These include modifiers for laterality, relative location, directionality, number, and plane.") 086 protected List<CodeableConcept> modifier; 087 088 /** 089 * Description of anatomical location. 090 */ 091 @Child(name = "description", type = { 092 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 093 @Description(shortDefinition = "The Description of anatomical location", formalDefinition = "Description of anatomical location.") 094 protected StringType description; 095 096 /** 097 * Image or images used to identify a location. 098 */ 099 @Child(name = "image", type = { 100 Attachment.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 101 @Description(shortDefinition = "Attached images", formalDefinition = "Image or images used to identify a location.") 102 protected List<Attachment> image; 103 104 private static final long serialVersionUID = 1568109920L; 105 106 /* 107 * Constructor 108 */ 109 public BodySite() { 110 super(); 111 } 112 113 /* 114 * Constructor 115 */ 116 public BodySite(Reference patient) { 117 super(); 118 this.patient = patient; 119 } 120 121 /** 122 * @return {@link #patient} (The person to which the body site belongs.) 123 */ 124 public Reference getPatient() { 125 if (this.patient == null) 126 if (Configuration.errorOnAutoCreate()) 127 throw new Error("Attempt to auto-create BodySite.patient"); 128 else if (Configuration.doAutoCreate()) 129 this.patient = new Reference(); // cc 130 return this.patient; 131 } 132 133 public boolean hasPatient() { 134 return this.patient != null && !this.patient.isEmpty(); 135 } 136 137 /** 138 * @param value {@link #patient} (The person to which the body site belongs.) 139 */ 140 public BodySite setPatient(Reference value) { 141 this.patient = value; 142 return this; 143 } 144 145 /** 146 * @return {@link #patient} The actual object that is the target of the 147 * reference. The reference library doesn't populate this, but you can 148 * use it to hold the resource if you resolve it. (The person to which 149 * the body site belongs.) 150 */ 151 public Patient getPatientTarget() { 152 if (this.patientTarget == null) 153 if (Configuration.errorOnAutoCreate()) 154 throw new Error("Attempt to auto-create BodySite.patient"); 155 else if (Configuration.doAutoCreate()) 156 this.patientTarget = new Patient(); // aa 157 return this.patientTarget; 158 } 159 160 /** 161 * @param value {@link #patient} The actual object that is the target of the 162 * reference. The reference library doesn't use these, but you can 163 * use it to hold the resource if you resolve it. (The person to 164 * which the body site belongs.) 165 */ 166 public BodySite setPatientTarget(Patient value) { 167 this.patientTarget = value; 168 return this; 169 } 170 171 /** 172 * @return {@link #identifier} (Identifier for this instance of the anatomical 173 * location.) 174 */ 175 public List<Identifier> getIdentifier() { 176 if (this.identifier == null) 177 this.identifier = new ArrayList<Identifier>(); 178 return this.identifier; 179 } 180 181 public boolean hasIdentifier() { 182 if (this.identifier == null) 183 return false; 184 for (Identifier item : this.identifier) 185 if (!item.isEmpty()) 186 return true; 187 return false; 188 } 189 190 /** 191 * @return {@link #identifier} (Identifier for this instance of the anatomical 192 * location.) 193 */ 194 // syntactic sugar 195 public Identifier addIdentifier() { // 3 196 Identifier t = new Identifier(); 197 if (this.identifier == null) 198 this.identifier = new ArrayList<Identifier>(); 199 this.identifier.add(t); 200 return t; 201 } 202 203 // syntactic sugar 204 public BodySite addIdentifier(Identifier t) { // 3 205 if (t == null) 206 return this; 207 if (this.identifier == null) 208 this.identifier = new ArrayList<Identifier>(); 209 this.identifier.add(t); 210 return this; 211 } 212 213 /** 214 * @return {@link #code} (Named anatomical location - ideally coded where 215 * possible.) 216 */ 217 public CodeableConcept getCode() { 218 if (this.code == null) 219 if (Configuration.errorOnAutoCreate()) 220 throw new Error("Attempt to auto-create BodySite.code"); 221 else if (Configuration.doAutoCreate()) 222 this.code = new CodeableConcept(); // cc 223 return this.code; 224 } 225 226 public boolean hasCode() { 227 return this.code != null && !this.code.isEmpty(); 228 } 229 230 /** 231 * @param value {@link #code} (Named anatomical location - ideally coded where 232 * possible.) 233 */ 234 public BodySite setCode(CodeableConcept value) { 235 this.code = value; 236 return this; 237 } 238 239 /** 240 * @return {@link #modifier} (Modifier to refine the anatomical location. These 241 * include modifiers for laterality, relative location, directionality, 242 * number, and plane.) 243 */ 244 public List<CodeableConcept> getModifier() { 245 if (this.modifier == null) 246 this.modifier = new ArrayList<CodeableConcept>(); 247 return this.modifier; 248 } 249 250 public boolean hasModifier() { 251 if (this.modifier == null) 252 return false; 253 for (CodeableConcept item : this.modifier) 254 if (!item.isEmpty()) 255 return true; 256 return false; 257 } 258 259 /** 260 * @return {@link #modifier} (Modifier to refine the anatomical location. These 261 * include modifiers for laterality, relative location, directionality, 262 * number, and plane.) 263 */ 264 // syntactic sugar 265 public CodeableConcept addModifier() { // 3 266 CodeableConcept t = new CodeableConcept(); 267 if (this.modifier == null) 268 this.modifier = new ArrayList<CodeableConcept>(); 269 this.modifier.add(t); 270 return t; 271 } 272 273 // syntactic sugar 274 public BodySite addModifier(CodeableConcept t) { // 3 275 if (t == null) 276 return this; 277 if (this.modifier == null) 278 this.modifier = new ArrayList<CodeableConcept>(); 279 this.modifier.add(t); 280 return this; 281 } 282 283 /** 284 * @return {@link #description} (Description of anatomical location.). This is 285 * the underlying object with id, value and extensions. The accessor 286 * "getDescription" gives direct access to the value 287 */ 288 public StringType getDescriptionElement() { 289 if (this.description == null) 290 if (Configuration.errorOnAutoCreate()) 291 throw new Error("Attempt to auto-create BodySite.description"); 292 else if (Configuration.doAutoCreate()) 293 this.description = new StringType(); // bb 294 return this.description; 295 } 296 297 public boolean hasDescriptionElement() { 298 return this.description != null && !this.description.isEmpty(); 299 } 300 301 public boolean hasDescription() { 302 return this.description != null && !this.description.isEmpty(); 303 } 304 305 /** 306 * @param value {@link #description} (Description of anatomical location.). This 307 * is the underlying object with id, value and extensions. The 308 * accessor "getDescription" gives direct access to the value 309 */ 310 public BodySite setDescriptionElement(StringType value) { 311 this.description = value; 312 return this; 313 } 314 315 /** 316 * @return Description of anatomical location. 317 */ 318 public String getDescription() { 319 return this.description == null ? null : this.description.getValue(); 320 } 321 322 /** 323 * @param value Description of anatomical location. 324 */ 325 public BodySite setDescription(String value) { 326 if (Utilities.noString(value)) 327 this.description = null; 328 else { 329 if (this.description == null) 330 this.description = new StringType(); 331 this.description.setValue(value); 332 } 333 return this; 334 } 335 336 /** 337 * @return {@link #image} (Image or images used to identify a location.) 338 */ 339 public List<Attachment> getImage() { 340 if (this.image == null) 341 this.image = new ArrayList<Attachment>(); 342 return this.image; 343 } 344 345 public boolean hasImage() { 346 if (this.image == null) 347 return false; 348 for (Attachment item : this.image) 349 if (!item.isEmpty()) 350 return true; 351 return false; 352 } 353 354 /** 355 * @return {@link #image} (Image or images used to identify a location.) 356 */ 357 // syntactic sugar 358 public Attachment addImage() { // 3 359 Attachment t = new Attachment(); 360 if (this.image == null) 361 this.image = new ArrayList<Attachment>(); 362 this.image.add(t); 363 return t; 364 } 365 366 // syntactic sugar 367 public BodySite addImage(Attachment t) { // 3 368 if (t == null) 369 return this; 370 if (this.image == null) 371 this.image = new ArrayList<Attachment>(); 372 this.image.add(t); 373 return this; 374 } 375 376 protected void listChildren(List<Property> childrenList) { 377 super.listChildren(childrenList); 378 childrenList.add(new Property("patient", "Reference(Patient)", "The person to which the body site belongs.", 0, 379 java.lang.Integer.MAX_VALUE, patient)); 380 childrenList.add(new Property("identifier", "Identifier", 381 "Identifier for this instance of the anatomical location.", 0, java.lang.Integer.MAX_VALUE, identifier)); 382 childrenList.add(new Property("code", "CodeableConcept", 383 "Named anatomical location - ideally coded where possible.", 0, java.lang.Integer.MAX_VALUE, code)); 384 childrenList.add(new Property("modifier", "CodeableConcept", 385 "Modifier to refine the anatomical location. These include modifiers for laterality, relative location, directionality, number, and plane.", 386 0, java.lang.Integer.MAX_VALUE, modifier)); 387 childrenList.add(new Property("description", "string", "Description of anatomical location.", 0, 388 java.lang.Integer.MAX_VALUE, description)); 389 childrenList.add(new Property("image", "Attachment", "Image or images used to identify a location.", 0, 390 java.lang.Integer.MAX_VALUE, image)); 391 } 392 393 @Override 394 public void setProperty(String name, Base value) throws FHIRException { 395 if (name.equals("patient")) 396 this.patient = castToReference(value); // Reference 397 else if (name.equals("identifier")) 398 this.getIdentifier().add(castToIdentifier(value)); 399 else if (name.equals("code")) 400 this.code = castToCodeableConcept(value); // CodeableConcept 401 else if (name.equals("modifier")) 402 this.getModifier().add(castToCodeableConcept(value)); 403 else if (name.equals("description")) 404 this.description = castToString(value); // StringType 405 else if (name.equals("image")) 406 this.getImage().add(castToAttachment(value)); 407 else 408 super.setProperty(name, value); 409 } 410 411 @Override 412 public Base addChild(String name) throws FHIRException { 413 if (name.equals("patient")) { 414 this.patient = new Reference(); 415 return this.patient; 416 } else if (name.equals("identifier")) { 417 return addIdentifier(); 418 } else if (name.equals("code")) { 419 this.code = new CodeableConcept(); 420 return this.code; 421 } else if (name.equals("modifier")) { 422 return addModifier(); 423 } else if (name.equals("description")) { 424 throw new FHIRException("Cannot call addChild on a singleton property BodySite.description"); 425 } else if (name.equals("image")) { 426 return addImage(); 427 } else 428 return super.addChild(name); 429 } 430 431 public String fhirType() { 432 return "BodySite"; 433 434 } 435 436 public BodySite copy() { 437 BodySite dst = new BodySite(); 438 copyValues(dst); 439 dst.patient = patient == null ? null : patient.copy(); 440 if (identifier != null) { 441 dst.identifier = new ArrayList<Identifier>(); 442 for (Identifier i : identifier) 443 dst.identifier.add(i.copy()); 444 } 445 ; 446 dst.code = code == null ? null : code.copy(); 447 if (modifier != null) { 448 dst.modifier = new ArrayList<CodeableConcept>(); 449 for (CodeableConcept i : modifier) 450 dst.modifier.add(i.copy()); 451 } 452 ; 453 dst.description = description == null ? null : description.copy(); 454 if (image != null) { 455 dst.image = new ArrayList<Attachment>(); 456 for (Attachment i : image) 457 dst.image.add(i.copy()); 458 } 459 ; 460 return dst; 461 } 462 463 protected BodySite typedCopy() { 464 return copy(); 465 } 466 467 @Override 468 public boolean equalsDeep(Base other) { 469 if (!super.equalsDeep(other)) 470 return false; 471 if (!(other instanceof BodySite)) 472 return false; 473 BodySite o = (BodySite) other; 474 return compareDeep(patient, o.patient, true) && compareDeep(identifier, o.identifier, true) 475 && compareDeep(code, o.code, true) && compareDeep(modifier, o.modifier, true) 476 && compareDeep(description, o.description, true) && compareDeep(image, o.image, true); 477 } 478 479 @Override 480 public boolean equalsShallow(Base other) { 481 if (!super.equalsShallow(other)) 482 return false; 483 if (!(other instanceof BodySite)) 484 return false; 485 BodySite o = (BodySite) other; 486 return compareValues(description, o.description, true); 487 } 488 489 public boolean isEmpty() { 490 return super.isEmpty() && (patient == null || patient.isEmpty()) && (identifier == null || identifier.isEmpty()) 491 && (code == null || code.isEmpty()) && (modifier == null || modifier.isEmpty()) 492 && (description == null || description.isEmpty()) && (image == null || image.isEmpty()); 493 } 494 495 @Override 496 public ResourceType getResourceType() { 497 return ResourceType.BodySite; 498 } 499 500 @SearchParamDefinition(name = "identifier", path = "BodySite.identifier", description = "Identifier for this instance of the anatomical location", type = "token") 501 public static final String SP_IDENTIFIER = "identifier"; 502 @SearchParamDefinition(name = "code", path = "BodySite.code", description = "Named anatomical location", type = "token") 503 public static final String SP_CODE = "code"; 504 @SearchParamDefinition(name = "patient", path = "BodySite.patient", description = "Patient to whom bodysite belongs", type = "reference") 505 public static final String SP_PATIENT = "patient"; 506 507}