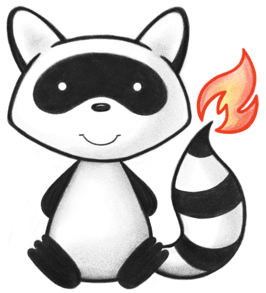
001package org.hl7.fhir.dstu2.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.instance.model.api.IBaseBundle; 046import org.hl7.fhir.exceptions.FHIRException; 047import org.hl7.fhir.utilities.Utilities; 048 049/** 050 * A container for a collection of resources. 051 */ 052@ResourceDef(name = "Bundle", profile = "http://hl7.org/fhir/Profile/Bundle") 053public class Bundle extends Resource implements IBaseBundle { 054 055 public enum BundleType { 056 /** 057 * The bundle is a document. The first resource is a Composition. 058 */ 059 DOCUMENT, 060 /** 061 * The bundle is a message. The first resource is a MessageHeader. 062 */ 063 MESSAGE, 064 /** 065 * The bundle is a transaction - intended to be processed by a server as an 066 * atomic commit. 067 */ 068 TRANSACTION, 069 /** 070 * The bundle is a transaction response. Because the response is a transaction 071 * response, the transactionhas succeeded, and all responses are error free. 072 */ 073 TRANSACTIONRESPONSE, 074 /** 075 * The bundle is a transaction - intended to be processed by a server as a group 076 * of actions. 077 */ 078 BATCH, 079 /** 080 * The bundle is a batch response. Note that as a batch, some responses may 081 * indicate failure and others success. 082 */ 083 BATCHRESPONSE, 084 /** 085 * The bundle is a list of resources from a history interaction on a server. 086 */ 087 HISTORY, 088 /** 089 * The bundle is a list of resources returned as a result of a search/query 090 * interaction, operation, or message. 091 */ 092 SEARCHSET, 093 /** 094 * The bundle is a set of resources collected into a single document for ease of 095 * distribution. 096 */ 097 COLLECTION, 098 /** 099 * added to help the parsers 100 */ 101 NULL; 102 103 public static BundleType fromCode(String codeString) throws FHIRException { 104 if (codeString == null || "".equals(codeString)) 105 return null; 106 if ("document".equals(codeString)) 107 return DOCUMENT; 108 if ("message".equals(codeString)) 109 return MESSAGE; 110 if ("transaction".equals(codeString)) 111 return TRANSACTION; 112 if ("transaction-response".equals(codeString)) 113 return TRANSACTIONRESPONSE; 114 if ("batch".equals(codeString)) 115 return BATCH; 116 if ("batch-response".equals(codeString)) 117 return BATCHRESPONSE; 118 if ("history".equals(codeString)) 119 return HISTORY; 120 if ("searchset".equals(codeString)) 121 return SEARCHSET; 122 if ("collection".equals(codeString)) 123 return COLLECTION; 124 throw new FHIRException("Unknown BundleType code '" + codeString + "'"); 125 } 126 127 public String toCode() { 128 switch (this) { 129 case DOCUMENT: 130 return "document"; 131 case MESSAGE: 132 return "message"; 133 case TRANSACTION: 134 return "transaction"; 135 case TRANSACTIONRESPONSE: 136 return "transaction-response"; 137 case BATCH: 138 return "batch"; 139 case BATCHRESPONSE: 140 return "batch-response"; 141 case HISTORY: 142 return "history"; 143 case SEARCHSET: 144 return "searchset"; 145 case COLLECTION: 146 return "collection"; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 154 public String getSystem() { 155 switch (this) { 156 case DOCUMENT: 157 return "http://hl7.org/fhir/bundle-type"; 158 case MESSAGE: 159 return "http://hl7.org/fhir/bundle-type"; 160 case TRANSACTION: 161 return "http://hl7.org/fhir/bundle-type"; 162 case TRANSACTIONRESPONSE: 163 return "http://hl7.org/fhir/bundle-type"; 164 case BATCH: 165 return "http://hl7.org/fhir/bundle-type"; 166 case BATCHRESPONSE: 167 return "http://hl7.org/fhir/bundle-type"; 168 case HISTORY: 169 return "http://hl7.org/fhir/bundle-type"; 170 case SEARCHSET: 171 return "http://hl7.org/fhir/bundle-type"; 172 case COLLECTION: 173 return "http://hl7.org/fhir/bundle-type"; 174 case NULL: 175 return null; 176 default: 177 return "?"; 178 } 179 } 180 181 public String getDefinition() { 182 switch (this) { 183 case DOCUMENT: 184 return "The bundle is a document. The first resource is a Composition."; 185 case MESSAGE: 186 return "The bundle is a message. The first resource is a MessageHeader."; 187 case TRANSACTION: 188 return "The bundle is a transaction - intended to be processed by a server as an atomic commit."; 189 case TRANSACTIONRESPONSE: 190 return "The bundle is a transaction response. Because the response is a transaction response, the transactionhas succeeded, and all responses are error free."; 191 case BATCH: 192 return "The bundle is a transaction - intended to be processed by a server as a group of actions."; 193 case BATCHRESPONSE: 194 return "The bundle is a batch response. Note that as a batch, some responses may indicate failure and others success."; 195 case HISTORY: 196 return "The bundle is a list of resources from a history interaction on a server."; 197 case SEARCHSET: 198 return "The bundle is a list of resources returned as a result of a search/query interaction, operation, or message."; 199 case COLLECTION: 200 return "The bundle is a set of resources collected into a single document for ease of distribution."; 201 case NULL: 202 return null; 203 default: 204 return "?"; 205 } 206 } 207 208 public String getDisplay() { 209 switch (this) { 210 case DOCUMENT: 211 return "Document"; 212 case MESSAGE: 213 return "Message"; 214 case TRANSACTION: 215 return "Transaction"; 216 case TRANSACTIONRESPONSE: 217 return "Transaction Response"; 218 case BATCH: 219 return "Batch"; 220 case BATCHRESPONSE: 221 return "Batch Response"; 222 case HISTORY: 223 return "History List"; 224 case SEARCHSET: 225 return "Search Results"; 226 case COLLECTION: 227 return "Collection"; 228 case NULL: 229 return null; 230 default: 231 return "?"; 232 } 233 } 234 } 235 236 public static class BundleTypeEnumFactory implements EnumFactory<BundleType> { 237 public BundleType fromCode(String codeString) throws IllegalArgumentException { 238 if (codeString == null || "".equals(codeString)) 239 if (codeString == null || "".equals(codeString)) 240 return null; 241 if ("document".equals(codeString)) 242 return BundleType.DOCUMENT; 243 if ("message".equals(codeString)) 244 return BundleType.MESSAGE; 245 if ("transaction".equals(codeString)) 246 return BundleType.TRANSACTION; 247 if ("transaction-response".equals(codeString)) 248 return BundleType.TRANSACTIONRESPONSE; 249 if ("batch".equals(codeString)) 250 return BundleType.BATCH; 251 if ("batch-response".equals(codeString)) 252 return BundleType.BATCHRESPONSE; 253 if ("history".equals(codeString)) 254 return BundleType.HISTORY; 255 if ("searchset".equals(codeString)) 256 return BundleType.SEARCHSET; 257 if ("collection".equals(codeString)) 258 return BundleType.COLLECTION; 259 throw new IllegalArgumentException("Unknown BundleType code '" + codeString + "'"); 260 } 261 262 public Enumeration<BundleType> fromType(Base code) throws FHIRException { 263 if (code == null || code.isEmpty()) 264 return null; 265 String codeString = ((PrimitiveType) code).asStringValue(); 266 if (codeString == null || "".equals(codeString)) 267 return null; 268 if ("document".equals(codeString)) 269 return new Enumeration<BundleType>(this, BundleType.DOCUMENT); 270 if ("message".equals(codeString)) 271 return new Enumeration<BundleType>(this, BundleType.MESSAGE); 272 if ("transaction".equals(codeString)) 273 return new Enumeration<BundleType>(this, BundleType.TRANSACTION); 274 if ("transaction-response".equals(codeString)) 275 return new Enumeration<BundleType>(this, BundleType.TRANSACTIONRESPONSE); 276 if ("batch".equals(codeString)) 277 return new Enumeration<BundleType>(this, BundleType.BATCH); 278 if ("batch-response".equals(codeString)) 279 return new Enumeration<BundleType>(this, BundleType.BATCHRESPONSE); 280 if ("history".equals(codeString)) 281 return new Enumeration<BundleType>(this, BundleType.HISTORY); 282 if ("searchset".equals(codeString)) 283 return new Enumeration<BundleType>(this, BundleType.SEARCHSET); 284 if ("collection".equals(codeString)) 285 return new Enumeration<BundleType>(this, BundleType.COLLECTION); 286 throw new FHIRException("Unknown BundleType code '" + codeString + "'"); 287 } 288 289 public String toCode(BundleType code) 290 { 291 if (code == BundleType.NULL) 292 return null; 293 if (code == BundleType.DOCUMENT) 294 return "document"; 295 if (code == BundleType.MESSAGE) 296 return "message"; 297 if (code == BundleType.TRANSACTION) 298 return "transaction"; 299 if (code == BundleType.TRANSACTIONRESPONSE) 300 return "transaction-response"; 301 if (code == BundleType.BATCH) 302 return "batch"; 303 if (code == BundleType.BATCHRESPONSE) 304 return "batch-response"; 305 if (code == BundleType.HISTORY) 306 return "history"; 307 if (code == BundleType.SEARCHSET) 308 return "searchset"; 309 if (code == BundleType.COLLECTION) 310 return "collection"; 311 return "?"; 312 } 313 } 314 315 public enum SearchEntryMode { 316 /** 317 * This resource matched the search specification. 318 */ 319 MATCH, 320 /** 321 * This resource is returned because it is referred to from another resource in 322 * the search set. 323 */ 324 INCLUDE, 325 /** 326 * An OperationOutcome that provides additional information about the processing 327 * of a search. 328 */ 329 OUTCOME, 330 /** 331 * added to help the parsers 332 */ 333 NULL; 334 335 public static SearchEntryMode fromCode(String codeString) throws FHIRException { 336 if (codeString == null || "".equals(codeString)) 337 return null; 338 if ("match".equals(codeString)) 339 return MATCH; 340 if ("include".equals(codeString)) 341 return INCLUDE; 342 if ("outcome".equals(codeString)) 343 return OUTCOME; 344 throw new FHIRException("Unknown SearchEntryMode code '" + codeString + "'"); 345 } 346 347 public String toCode() { 348 switch (this) { 349 case MATCH: 350 return "match"; 351 case INCLUDE: 352 return "include"; 353 case OUTCOME: 354 return "outcome"; 355 case NULL: 356 return null; 357 default: 358 return "?"; 359 } 360 } 361 362 public String getSystem() { 363 switch (this) { 364 case MATCH: 365 return "http://hl7.org/fhir/search-entry-mode"; 366 case INCLUDE: 367 return "http://hl7.org/fhir/search-entry-mode"; 368 case OUTCOME: 369 return "http://hl7.org/fhir/search-entry-mode"; 370 case NULL: 371 return null; 372 default: 373 return "?"; 374 } 375 } 376 377 public String getDefinition() { 378 switch (this) { 379 case MATCH: 380 return "This resource matched the search specification."; 381 case INCLUDE: 382 return "This resource is returned because it is referred to from another resource in the search set."; 383 case OUTCOME: 384 return "An OperationOutcome that provides additional information about the processing of a search."; 385 case NULL: 386 return null; 387 default: 388 return "?"; 389 } 390 } 391 392 public String getDisplay() { 393 switch (this) { 394 case MATCH: 395 return "Match"; 396 case INCLUDE: 397 return "Include"; 398 case OUTCOME: 399 return "Outcome"; 400 case NULL: 401 return null; 402 default: 403 return "?"; 404 } 405 } 406 } 407 408 public static class SearchEntryModeEnumFactory implements EnumFactory<SearchEntryMode> { 409 public SearchEntryMode fromCode(String codeString) throws IllegalArgumentException { 410 if (codeString == null || "".equals(codeString)) 411 if (codeString == null || "".equals(codeString)) 412 return null; 413 if ("match".equals(codeString)) 414 return SearchEntryMode.MATCH; 415 if ("include".equals(codeString)) 416 return SearchEntryMode.INCLUDE; 417 if ("outcome".equals(codeString)) 418 return SearchEntryMode.OUTCOME; 419 throw new IllegalArgumentException("Unknown SearchEntryMode code '" + codeString + "'"); 420 } 421 422 public Enumeration<SearchEntryMode> fromType(Base code) throws FHIRException { 423 if (code == null || code.isEmpty()) 424 return null; 425 String codeString = ((PrimitiveType) code).asStringValue(); 426 if (codeString == null || "".equals(codeString)) 427 return null; 428 if ("match".equals(codeString)) 429 return new Enumeration<SearchEntryMode>(this, SearchEntryMode.MATCH); 430 if ("include".equals(codeString)) 431 return new Enumeration<SearchEntryMode>(this, SearchEntryMode.INCLUDE); 432 if ("outcome".equals(codeString)) 433 return new Enumeration<SearchEntryMode>(this, SearchEntryMode.OUTCOME); 434 throw new FHIRException("Unknown SearchEntryMode code '" + codeString + "'"); 435 } 436 437 public String toCode(SearchEntryMode code) 438 { 439 if (code == SearchEntryMode.NULL) 440 return null; 441 if (code == SearchEntryMode.MATCH) 442 return "match"; 443 if (code == SearchEntryMode.INCLUDE) 444 return "include"; 445 if (code == SearchEntryMode.OUTCOME) 446 return "outcome"; 447 return "?"; 448 } 449 } 450 451 public enum HTTPVerb { 452 /** 453 * HTTP GET 454 */ 455 GET, 456 /** 457 * HTTP POST 458 */ 459 POST, 460 /** 461 * HTTP PUT 462 */ 463 PUT, 464 /** 465 * HTTP DELETE 466 */ 467 DELETE, 468 /** 469 * added to help the parsers 470 */ 471 NULL; 472 473 public static HTTPVerb fromCode(String codeString) throws FHIRException { 474 if (codeString == null || "".equals(codeString)) 475 return null; 476 if ("GET".equals(codeString)) 477 return GET; 478 if ("POST".equals(codeString)) 479 return POST; 480 if ("PUT".equals(codeString)) 481 return PUT; 482 if ("DELETE".equals(codeString)) 483 return DELETE; 484 throw new FHIRException("Unknown HTTPVerb code '" + codeString + "'"); 485 } 486 487 public String toCode() { 488 switch (this) { 489 case GET: 490 return "GET"; 491 case POST: 492 return "POST"; 493 case PUT: 494 return "PUT"; 495 case DELETE: 496 return "DELETE"; 497 case NULL: 498 return null; 499 default: 500 return "?"; 501 } 502 } 503 504 public String getSystem() { 505 switch (this) { 506 case GET: 507 return "http://hl7.org/fhir/http-verb"; 508 case POST: 509 return "http://hl7.org/fhir/http-verb"; 510 case PUT: 511 return "http://hl7.org/fhir/http-verb"; 512 case DELETE: 513 return "http://hl7.org/fhir/http-verb"; 514 case NULL: 515 return null; 516 default: 517 return "?"; 518 } 519 } 520 521 public String getDefinition() { 522 switch (this) { 523 case GET: 524 return "HTTP GET"; 525 case POST: 526 return "HTTP POST"; 527 case PUT: 528 return "HTTP PUT"; 529 case DELETE: 530 return "HTTP DELETE"; 531 case NULL: 532 return null; 533 default: 534 return "?"; 535 } 536 } 537 538 public String getDisplay() { 539 switch (this) { 540 case GET: 541 return "GET"; 542 case POST: 543 return "POST"; 544 case PUT: 545 return "PUT"; 546 case DELETE: 547 return "DELETE"; 548 case NULL: 549 return null; 550 default: 551 return "?"; 552 } 553 } 554 } 555 556 public static class HTTPVerbEnumFactory implements EnumFactory<HTTPVerb> { 557 public HTTPVerb fromCode(String codeString) throws IllegalArgumentException { 558 if (codeString == null || "".equals(codeString)) 559 if (codeString == null || "".equals(codeString)) 560 return null; 561 if ("GET".equals(codeString)) 562 return HTTPVerb.GET; 563 if ("POST".equals(codeString)) 564 return HTTPVerb.POST; 565 if ("PUT".equals(codeString)) 566 return HTTPVerb.PUT; 567 if ("DELETE".equals(codeString)) 568 return HTTPVerb.DELETE; 569 throw new IllegalArgumentException("Unknown HTTPVerb code '" + codeString + "'"); 570 } 571 572 public Enumeration<HTTPVerb> fromType(Base code) throws FHIRException { 573 if (code == null || code.isEmpty()) 574 return null; 575 String codeString = ((PrimitiveType) code).asStringValue(); 576 if (codeString == null || "".equals(codeString)) 577 return null; 578 if ("GET".equals(codeString)) 579 return new Enumeration<HTTPVerb>(this, HTTPVerb.GET); 580 if ("POST".equals(codeString)) 581 return new Enumeration<HTTPVerb>(this, HTTPVerb.POST); 582 if ("PUT".equals(codeString)) 583 return new Enumeration<HTTPVerb>(this, HTTPVerb.PUT); 584 if ("DELETE".equals(codeString)) 585 return new Enumeration<HTTPVerb>(this, HTTPVerb.DELETE); 586 throw new FHIRException("Unknown HTTPVerb code '" + codeString + "'"); 587 } 588 589 public String toCode(HTTPVerb code) 590 { 591 if (code == HTTPVerb.NULL) 592 return null; 593 if (code == HTTPVerb.GET) 594 return "GET"; 595 if (code == HTTPVerb.POST) 596 return "POST"; 597 if (code == HTTPVerb.PUT) 598 return "PUT"; 599 if (code == HTTPVerb.DELETE) 600 return "DELETE"; 601 return "?"; 602 } 603 } 604 605 @Block() 606 public static class BundleLinkComponent extends BackboneElement implements IBaseBackboneElement { 607 /** 608 * A name which details the functional use for this link - see 609 * [[http://www.iana.org/assignments/link-relations/link-relations.xhtml]]. 610 */ 611 @Child(name = "relation", type = { 612 StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 613 @Description(shortDefinition = "http://www.iana.org/assignments/link-relations/link-relations.xhtml", formalDefinition = "A name which details the functional use for this link - see [[http://www.iana.org/assignments/link-relations/link-relations.xhtml]].") 614 protected StringType relation; 615 616 /** 617 * The reference details for the link. 618 */ 619 @Child(name = "url", type = { UriType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 620 @Description(shortDefinition = "Reference details for the link", formalDefinition = "The reference details for the link.") 621 protected UriType url; 622 623 private static final long serialVersionUID = -1010386066L; 624 625 /* 626 * Constructor 627 */ 628 public BundleLinkComponent() { 629 super(); 630 } 631 632 /* 633 * Constructor 634 */ 635 public BundleLinkComponent(StringType relation, UriType url) { 636 super(); 637 this.relation = relation; 638 this.url = url; 639 } 640 641 /** 642 * @return {@link #relation} (A name which details the functional use for this 643 * link - see 644 * [[http://www.iana.org/assignments/link-relations/link-relations.xhtml]].). 645 * This is the underlying object with id, value and extensions. The 646 * accessor "getRelation" gives direct access to the value 647 */ 648 public StringType getRelationElement() { 649 if (this.relation == null) 650 if (Configuration.errorOnAutoCreate()) 651 throw new Error("Attempt to auto-create BundleLinkComponent.relation"); 652 else if (Configuration.doAutoCreate()) 653 this.relation = new StringType(); // bb 654 return this.relation; 655 } 656 657 public boolean hasRelationElement() { 658 return this.relation != null && !this.relation.isEmpty(); 659 } 660 661 public boolean hasRelation() { 662 return this.relation != null && !this.relation.isEmpty(); 663 } 664 665 /** 666 * @param value {@link #relation} (A name which details the functional use for 667 * this link - see 668 * [[http://www.iana.org/assignments/link-relations/link-relations.xhtml]].). 669 * This is the underlying object with id, value and extensions. The 670 * accessor "getRelation" gives direct access to the value 671 */ 672 public BundleLinkComponent setRelationElement(StringType value) { 673 this.relation = value; 674 return this; 675 } 676 677 /** 678 * @return A name which details the functional use for this link - see 679 * [[http://www.iana.org/assignments/link-relations/link-relations.xhtml]]. 680 */ 681 public String getRelation() { 682 return this.relation == null ? null : this.relation.getValue(); 683 } 684 685 /** 686 * @param value A name which details the functional use for this link - see 687 * [[http://www.iana.org/assignments/link-relations/link-relations.xhtml]]. 688 */ 689 public BundleLinkComponent setRelation(String value) { 690 if (this.relation == null) 691 this.relation = new StringType(); 692 this.relation.setValue(value); 693 return this; 694 } 695 696 /** 697 * @return {@link #url} (The reference details for the link.). This is the 698 * underlying object with id, value and extensions. The accessor 699 * "getUrl" gives direct access to the value 700 */ 701 public UriType getUrlElement() { 702 if (this.url == null) 703 if (Configuration.errorOnAutoCreate()) 704 throw new Error("Attempt to auto-create BundleLinkComponent.url"); 705 else if (Configuration.doAutoCreate()) 706 this.url = new UriType(); // bb 707 return this.url; 708 } 709 710 public boolean hasUrlElement() { 711 return this.url != null && !this.url.isEmpty(); 712 } 713 714 public boolean hasUrl() { 715 return this.url != null && !this.url.isEmpty(); 716 } 717 718 /** 719 * @param value {@link #url} (The reference details for the link.). This is the 720 * underlying object with id, value and extensions. The accessor 721 * "getUrl" gives direct access to the value 722 */ 723 public BundleLinkComponent setUrlElement(UriType value) { 724 this.url = value; 725 return this; 726 } 727 728 /** 729 * @return The reference details for the link. 730 */ 731 public String getUrl() { 732 return this.url == null ? null : this.url.getValue(); 733 } 734 735 /** 736 * @param value The reference details for the link. 737 */ 738 public BundleLinkComponent setUrl(String value) { 739 if (this.url == null) 740 this.url = new UriType(); 741 this.url.setValue(value); 742 return this; 743 } 744 745 protected void listChildren(List<Property> childrenList) { 746 super.listChildren(childrenList); 747 childrenList.add(new Property("relation", "string", 748 "A name which details the functional use for this link - see [[http://www.iana.org/assignments/link-relations/link-relations.xhtml]].", 749 0, java.lang.Integer.MAX_VALUE, relation)); 750 childrenList 751 .add(new Property("url", "uri", "The reference details for the link.", 0, java.lang.Integer.MAX_VALUE, url)); 752 } 753 754 @Override 755 public void setProperty(String name, Base value) throws FHIRException { 756 if (name.equals("relation")) 757 this.relation = castToString(value); // StringType 758 else if (name.equals("url")) 759 this.url = castToUri(value); // UriType 760 else 761 super.setProperty(name, value); 762 } 763 764 @Override 765 public Base addChild(String name) throws FHIRException { 766 if (name.equals("relation")) { 767 throw new FHIRException("Cannot call addChild on a singleton property Bundle.relation"); 768 } else if (name.equals("url")) { 769 throw new FHIRException("Cannot call addChild on a singleton property Bundle.url"); 770 } else 771 return super.addChild(name); 772 } 773 774 public BundleLinkComponent copy() { 775 BundleLinkComponent dst = new BundleLinkComponent(); 776 copyValues(dst); 777 dst.relation = relation == null ? null : relation.copy(); 778 dst.url = url == null ? null : url.copy(); 779 return dst; 780 } 781 782 @Override 783 public boolean equalsDeep(Base other) { 784 if (!super.equalsDeep(other)) 785 return false; 786 if (!(other instanceof BundleLinkComponent)) 787 return false; 788 BundleLinkComponent o = (BundleLinkComponent) other; 789 return compareDeep(relation, o.relation, true) && compareDeep(url, o.url, true); 790 } 791 792 @Override 793 public boolean equalsShallow(Base other) { 794 if (!super.equalsShallow(other)) 795 return false; 796 if (!(other instanceof BundleLinkComponent)) 797 return false; 798 BundleLinkComponent o = (BundleLinkComponent) other; 799 return compareValues(relation, o.relation, true) && compareValues(url, o.url, true); 800 } 801 802 public boolean isEmpty() { 803 return super.isEmpty() && (relation == null || relation.isEmpty()) && (url == null || url.isEmpty()); 804 } 805 806 public String fhirType() { 807 return "Bundle.link"; 808 809 } 810 811 } 812 813 @Block() 814 public static class BundleEntryComponent extends BackboneElement implements IBaseBackboneElement { 815 /** 816 * A series of links that provide context to this entry. 817 */ 818 @Child(name = "link", type = { 819 BundleLinkComponent.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 820 @Description(shortDefinition = "Links related to this entry", formalDefinition = "A series of links that provide context to this entry.") 821 protected List<BundleLinkComponent> link; 822 823 /** 824 * The Absolute URL for the resource. This must be provided for all resources. 825 * The fullUrl SHALL not disagree with the id in the resource. The fullUrl is a 826 * version independent reference to the resource. 827 */ 828 @Child(name = "fullUrl", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 829 @Description(shortDefinition = "Absolute URL for resource (server address, or UUID/OID)", formalDefinition = "The Absolute URL for the resource. This must be provided for all resources. The fullUrl SHALL not disagree with the id in the resource. The fullUrl is a version independent reference to the resource.") 830 protected UriType fullUrl; 831 832 /** 833 * The Resources for the entry. 834 */ 835 @Child(name = "resource", type = { Resource.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 836 @Description(shortDefinition = "A resource in the bundle", formalDefinition = "The Resources for the entry.") 837 protected Resource resource; 838 839 /** 840 * Information about the search process that lead to the creation of this entry. 841 */ 842 @Child(name = "search", type = {}, order = 4, min = 0, max = 1, modifier = false, summary = true) 843 @Description(shortDefinition = "Search related information", formalDefinition = "Information about the search process that lead to the creation of this entry.") 844 protected BundleEntrySearchComponent search; 845 846 /** 847 * Additional information about how this entry should be processed as part of a 848 * transaction. 849 */ 850 @Child(name = "request", type = {}, order = 5, min = 0, max = 1, modifier = false, summary = true) 851 @Description(shortDefinition = "Transaction Related Information", formalDefinition = "Additional information about how this entry should be processed as part of a transaction.") 852 protected BundleEntryRequestComponent request; 853 854 /** 855 * Additional information about how this entry should be processed as part of a 856 * transaction. 857 */ 858 @Child(name = "response", type = {}, order = 6, min = 0, max = 1, modifier = false, summary = true) 859 @Description(shortDefinition = "Transaction Related Information", formalDefinition = "Additional information about how this entry should be processed as part of a transaction.") 860 protected BundleEntryResponseComponent response; 861 862 private static final long serialVersionUID = 517783054L; 863 864 /* 865 * Constructor 866 */ 867 public BundleEntryComponent() { 868 super(); 869 } 870 871 /** 872 * @return {@link #link} (A series of links that provide context to this entry.) 873 */ 874 public List<BundleLinkComponent> getLink() { 875 if (this.link == null) 876 this.link = new ArrayList<BundleLinkComponent>(); 877 return this.link; 878 } 879 880 public boolean hasLink() { 881 if (this.link == null) 882 return false; 883 for (BundleLinkComponent item : this.link) 884 if (!item.isEmpty()) 885 return true; 886 return false; 887 } 888 889 /** 890 * @return {@link #link} (A series of links that provide context to this entry.) 891 */ 892 // syntactic sugar 893 public BundleLinkComponent addLink() { // 3 894 BundleLinkComponent t = new BundleLinkComponent(); 895 if (this.link == null) 896 this.link = new ArrayList<BundleLinkComponent>(); 897 this.link.add(t); 898 return t; 899 } 900 901 // syntactic sugar 902 public BundleEntryComponent addLink(BundleLinkComponent t) { // 3 903 if (t == null) 904 return this; 905 if (this.link == null) 906 this.link = new ArrayList<BundleLinkComponent>(); 907 this.link.add(t); 908 return this; 909 } 910 911 /** 912 * @return {@link #fullUrl} (The Absolute URL for the resource. This must be 913 * provided for all resources. The fullUrl SHALL not disagree with the 914 * id in the resource. The fullUrl is a version independent reference to 915 * the resource.). This is the underlying object with id, value and 916 * extensions. The accessor "getFullUrl" gives direct access to the 917 * value 918 */ 919 public UriType getFullUrlElement() { 920 if (this.fullUrl == null) 921 if (Configuration.errorOnAutoCreate()) 922 throw new Error("Attempt to auto-create BundleEntryComponent.fullUrl"); 923 else if (Configuration.doAutoCreate()) 924 this.fullUrl = new UriType(); // bb 925 return this.fullUrl; 926 } 927 928 public boolean hasFullUrlElement() { 929 return this.fullUrl != null && !this.fullUrl.isEmpty(); 930 } 931 932 public boolean hasFullUrl() { 933 return this.fullUrl != null && !this.fullUrl.isEmpty(); 934 } 935 936 /** 937 * @param value {@link #fullUrl} (The Absolute URL for the resource. This must 938 * be provided for all resources. The fullUrl SHALL not disagree 939 * with the id in the resource. The fullUrl is a version 940 * independent reference to the resource.). This is the underlying 941 * object with id, value and extensions. The accessor "getFullUrl" 942 * gives direct access to the value 943 */ 944 public BundleEntryComponent setFullUrlElement(UriType value) { 945 this.fullUrl = value; 946 return this; 947 } 948 949 /** 950 * @return The Absolute URL for the resource. This must be provided for all 951 * resources. The fullUrl SHALL not disagree with the id in the 952 * resource. The fullUrl is a version independent reference to the 953 * resource. 954 */ 955 public String getFullUrl() { 956 return this.fullUrl == null ? null : this.fullUrl.getValue(); 957 } 958 959 /** 960 * @param value The Absolute URL for the resource. This must be provided for all 961 * resources. The fullUrl SHALL not disagree with the id in the 962 * resource. The fullUrl is a version independent reference to the 963 * resource. 964 */ 965 public BundleEntryComponent setFullUrl(String value) { 966 if (Utilities.noString(value)) 967 this.fullUrl = null; 968 else { 969 if (this.fullUrl == null) 970 this.fullUrl = new UriType(); 971 this.fullUrl.setValue(value); 972 } 973 return this; 974 } 975 976 /** 977 * @return {@link #resource} (The Resources for the entry.) 978 */ 979 public Resource getResource() { 980 return this.resource; 981 } 982 983 public boolean hasResource() { 984 return this.resource != null && !this.resource.isEmpty(); 985 } 986 987 /** 988 * @param value {@link #resource} (The Resources for the entry.) 989 */ 990 public BundleEntryComponent setResource(Resource value) { 991 this.resource = value; 992 return this; 993 } 994 995 /** 996 * @return {@link #search} (Information about the search process that lead to 997 * the creation of this entry.) 998 */ 999 public BundleEntrySearchComponent getSearch() { 1000 if (this.search == null) 1001 if (Configuration.errorOnAutoCreate()) 1002 throw new Error("Attempt to auto-create BundleEntryComponent.search"); 1003 else if (Configuration.doAutoCreate()) 1004 this.search = new BundleEntrySearchComponent(); // cc 1005 return this.search; 1006 } 1007 1008 public boolean hasSearch() { 1009 return this.search != null && !this.search.isEmpty(); 1010 } 1011 1012 /** 1013 * @param value {@link #search} (Information about the search process that lead 1014 * to the creation of this entry.) 1015 */ 1016 public BundleEntryComponent setSearch(BundleEntrySearchComponent value) { 1017 this.search = value; 1018 return this; 1019 } 1020 1021 /** 1022 * @return {@link #request} (Additional information about how this entry should 1023 * be processed as part of a transaction.) 1024 */ 1025 public BundleEntryRequestComponent getRequest() { 1026 if (this.request == null) 1027 if (Configuration.errorOnAutoCreate()) 1028 throw new Error("Attempt to auto-create BundleEntryComponent.request"); 1029 else if (Configuration.doAutoCreate()) 1030 this.request = new BundleEntryRequestComponent(); // cc 1031 return this.request; 1032 } 1033 1034 public boolean hasRequest() { 1035 return this.request != null && !this.request.isEmpty(); 1036 } 1037 1038 /** 1039 * @param value {@link #request} (Additional information about how this entry 1040 * should be processed as part of a transaction.) 1041 */ 1042 public BundleEntryComponent setRequest(BundleEntryRequestComponent value) { 1043 this.request = value; 1044 return this; 1045 } 1046 1047 /** 1048 * @return {@link #response} (Additional information about how this entry should 1049 * be processed as part of a transaction.) 1050 */ 1051 public BundleEntryResponseComponent getResponse() { 1052 if (this.response == null) 1053 if (Configuration.errorOnAutoCreate()) 1054 throw new Error("Attempt to auto-create BundleEntryComponent.response"); 1055 else if (Configuration.doAutoCreate()) 1056 this.response = new BundleEntryResponseComponent(); // cc 1057 return this.response; 1058 } 1059 1060 public boolean hasResponse() { 1061 return this.response != null && !this.response.isEmpty(); 1062 } 1063 1064 /** 1065 * @param value {@link #response} (Additional information about how this entry 1066 * should be processed as part of a transaction.) 1067 */ 1068 public BundleEntryComponent setResponse(BundleEntryResponseComponent value) { 1069 this.response = value; 1070 return this; 1071 } 1072 1073 protected void listChildren(List<Property> childrenList) { 1074 super.listChildren(childrenList); 1075 childrenList.add(new Property("link", "@Bundle.link", "A series of links that provide context to this entry.", 0, 1076 java.lang.Integer.MAX_VALUE, link)); 1077 childrenList.add(new Property("fullUrl", "uri", 1078 "The Absolute URL for the resource. This must be provided for all resources. The fullUrl SHALL not disagree with the id in the resource. The fullUrl is a version independent reference to the resource.", 1079 0, java.lang.Integer.MAX_VALUE, fullUrl)); 1080 childrenList.add(new Property("resource", "Resource", "The Resources for the entry.", 0, 1081 java.lang.Integer.MAX_VALUE, resource)); 1082 childrenList.add( 1083 new Property("search", "", "Information about the search process that lead to the creation of this entry.", 0, 1084 java.lang.Integer.MAX_VALUE, search)); 1085 childrenList.add(new Property("request", "", 1086 "Additional information about how this entry should be processed as part of a transaction.", 0, 1087 java.lang.Integer.MAX_VALUE, request)); 1088 childrenList.add(new Property("response", "", 1089 "Additional information about how this entry should be processed as part of a transaction.", 0, 1090 java.lang.Integer.MAX_VALUE, response)); 1091 } 1092 1093 @Override 1094 public void setProperty(String name, Base value) throws FHIRException { 1095 if (name.equals("link")) 1096 this.getLink().add((BundleLinkComponent) value); 1097 else if (name.equals("fullUrl")) 1098 this.fullUrl = castToUri(value); // UriType 1099 else if (name.equals("resource")) 1100 this.resource = castToResource(value); // Resource 1101 else if (name.equals("search")) 1102 this.search = (BundleEntrySearchComponent) value; // BundleEntrySearchComponent 1103 else if (name.equals("request")) 1104 this.request = (BundleEntryRequestComponent) value; // BundleEntryRequestComponent 1105 else if (name.equals("response")) 1106 this.response = (BundleEntryResponseComponent) value; // BundleEntryResponseComponent 1107 else 1108 super.setProperty(name, value); 1109 } 1110 1111 @Override 1112 public Base addChild(String name) throws FHIRException { 1113 if (name.equals("link")) { 1114 return addLink(); 1115 } else if (name.equals("fullUrl")) { 1116 throw new FHIRException("Cannot call addChild on a singleton property Bundle.fullUrl"); 1117 } else if (name.equals("resource")) { 1118 throw new FHIRException("Cannot call addChild on an abstract type Bundle.resource"); 1119 } else if (name.equals("search")) { 1120 this.search = new BundleEntrySearchComponent(); 1121 return this.search; 1122 } else if (name.equals("request")) { 1123 this.request = new BundleEntryRequestComponent(); 1124 return this.request; 1125 } else if (name.equals("response")) { 1126 this.response = new BundleEntryResponseComponent(); 1127 return this.response; 1128 } else 1129 return super.addChild(name); 1130 } 1131 1132 public BundleEntryComponent copy() { 1133 BundleEntryComponent dst = new BundleEntryComponent(); 1134 copyValues(dst); 1135 if (link != null) { 1136 dst.link = new ArrayList<BundleLinkComponent>(); 1137 for (BundleLinkComponent i : link) 1138 dst.link.add(i.copy()); 1139 } 1140 ; 1141 dst.fullUrl = fullUrl == null ? null : fullUrl.copy(); 1142 dst.resource = resource == null ? null : resource.copy(); 1143 dst.search = search == null ? null : search.copy(); 1144 dst.request = request == null ? null : request.copy(); 1145 dst.response = response == null ? null : response.copy(); 1146 return dst; 1147 } 1148 1149 @Override 1150 public boolean equalsDeep(Base other) { 1151 if (!super.equalsDeep(other)) 1152 return false; 1153 if (!(other instanceof BundleEntryComponent)) 1154 return false; 1155 BundleEntryComponent o = (BundleEntryComponent) other; 1156 return compareDeep(link, o.link, true) && compareDeep(fullUrl, o.fullUrl, true) 1157 && compareDeep(resource, o.resource, true) && compareDeep(search, o.search, true) 1158 && compareDeep(request, o.request, true) && compareDeep(response, o.response, true); 1159 } 1160 1161 @Override 1162 public boolean equalsShallow(Base other) { 1163 if (!super.equalsShallow(other)) 1164 return false; 1165 if (!(other instanceof BundleEntryComponent)) 1166 return false; 1167 BundleEntryComponent o = (BundleEntryComponent) other; 1168 return compareValues(fullUrl, o.fullUrl, true); 1169 } 1170 1171 public boolean isEmpty() { 1172 return super.isEmpty() && (link == null || link.isEmpty()) && (fullUrl == null || fullUrl.isEmpty()) 1173 && (resource == null || resource.isEmpty()) && (search == null || search.isEmpty()) 1174 && (request == null || request.isEmpty()) && (response == null || response.isEmpty()); 1175 } 1176 1177 public String fhirType() { 1178 return "Bundle.entry"; 1179 1180 } 1181 1182 } 1183 1184 @Block() 1185 public static class BundleEntrySearchComponent extends BackboneElement implements IBaseBackboneElement { 1186 /** 1187 * Why this entry is in the result set - whether it's included as a match or 1188 * because of an _include requirement. 1189 */ 1190 @Child(name = "mode", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 1191 @Description(shortDefinition = "match | include | outcome - why this is in the result set", formalDefinition = "Why this entry is in the result set - whether it's included as a match or because of an _include requirement.") 1192 protected Enumeration<SearchEntryMode> mode; 1193 1194 /** 1195 * When searching, the server's search ranking score for the entry. 1196 */ 1197 @Child(name = "score", type = { DecimalType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1198 @Description(shortDefinition = "Search ranking (between 0 and 1)", formalDefinition = "When searching, the server's search ranking score for the entry.") 1199 protected DecimalType score; 1200 1201 private static final long serialVersionUID = 837739866L; 1202 1203 /* 1204 * Constructor 1205 */ 1206 public BundleEntrySearchComponent() { 1207 super(); 1208 } 1209 1210 /** 1211 * @return {@link #mode} (Why this entry is in the result set - whether it's 1212 * included as a match or because of an _include requirement.). This is 1213 * the underlying object with id, value and extensions. The accessor 1214 * "getMode" gives direct access to the value 1215 */ 1216 public Enumeration<SearchEntryMode> getModeElement() { 1217 if (this.mode == null) 1218 if (Configuration.errorOnAutoCreate()) 1219 throw new Error("Attempt to auto-create BundleEntrySearchComponent.mode"); 1220 else if (Configuration.doAutoCreate()) 1221 this.mode = new Enumeration<SearchEntryMode>(new SearchEntryModeEnumFactory()); // bb 1222 return this.mode; 1223 } 1224 1225 public boolean hasModeElement() { 1226 return this.mode != null && !this.mode.isEmpty(); 1227 } 1228 1229 public boolean hasMode() { 1230 return this.mode != null && !this.mode.isEmpty(); 1231 } 1232 1233 /** 1234 * @param value {@link #mode} (Why this entry is in the result set - whether 1235 * it's included as a match or because of an _include 1236 * requirement.). This is the underlying object with id, value and 1237 * extensions. The accessor "getMode" gives direct access to the 1238 * value 1239 */ 1240 public BundleEntrySearchComponent setModeElement(Enumeration<SearchEntryMode> value) { 1241 this.mode = value; 1242 return this; 1243 } 1244 1245 /** 1246 * @return Why this entry is in the result set - whether it's included as a 1247 * match or because of an _include requirement. 1248 */ 1249 public SearchEntryMode getMode() { 1250 return this.mode == null ? null : this.mode.getValue(); 1251 } 1252 1253 /** 1254 * @param value Why this entry is in the result set - whether it's included as a 1255 * match or because of an _include requirement. 1256 */ 1257 public BundleEntrySearchComponent setMode(SearchEntryMode value) { 1258 if (value == null) 1259 this.mode = null; 1260 else { 1261 if (this.mode == null) 1262 this.mode = new Enumeration<SearchEntryMode>(new SearchEntryModeEnumFactory()); 1263 this.mode.setValue(value); 1264 } 1265 return this; 1266 } 1267 1268 /** 1269 * @return {@link #score} (When searching, the server's search ranking score for 1270 * the entry.). This is the underlying object with id, value and 1271 * extensions. The accessor "getScore" gives direct access to the value 1272 */ 1273 public DecimalType getScoreElement() { 1274 if (this.score == null) 1275 if (Configuration.errorOnAutoCreate()) 1276 throw new Error("Attempt to auto-create BundleEntrySearchComponent.score"); 1277 else if (Configuration.doAutoCreate()) 1278 this.score = new DecimalType(); // bb 1279 return this.score; 1280 } 1281 1282 public boolean hasScoreElement() { 1283 return this.score != null && !this.score.isEmpty(); 1284 } 1285 1286 public boolean hasScore() { 1287 return this.score != null && !this.score.isEmpty(); 1288 } 1289 1290 /** 1291 * @param value {@link #score} (When searching, the server's search ranking 1292 * score for the entry.). This is the underlying object with id, 1293 * value and extensions. The accessor "getScore" gives direct 1294 * access to the value 1295 */ 1296 public BundleEntrySearchComponent setScoreElement(DecimalType value) { 1297 this.score = value; 1298 return this; 1299 } 1300 1301 /** 1302 * @return When searching, the server's search ranking score for the entry. 1303 */ 1304 public BigDecimal getScore() { 1305 return this.score == null ? null : this.score.getValue(); 1306 } 1307 1308 /** 1309 * @param value When searching, the server's search ranking score for the entry. 1310 */ 1311 public BundleEntrySearchComponent setScore(BigDecimal value) { 1312 if (value == null) 1313 this.score = null; 1314 else { 1315 if (this.score == null) 1316 this.score = new DecimalType(); 1317 this.score.setValue(value); 1318 } 1319 return this; 1320 } 1321 1322 protected void listChildren(List<Property> childrenList) { 1323 super.listChildren(childrenList); 1324 childrenList.add(new Property("mode", "code", 1325 "Why this entry is in the result set - whether it's included as a match or because of an _include requirement.", 1326 0, java.lang.Integer.MAX_VALUE, mode)); 1327 childrenList.add(new Property("score", "decimal", 1328 "When searching, the server's search ranking score for the entry.", 0, java.lang.Integer.MAX_VALUE, score)); 1329 } 1330 1331 @Override 1332 public void setProperty(String name, Base value) throws FHIRException { 1333 if (name.equals("mode")) 1334 this.mode = new SearchEntryModeEnumFactory().fromType(value); // Enumeration<SearchEntryMode> 1335 else if (name.equals("score")) 1336 this.score = castToDecimal(value); // DecimalType 1337 else 1338 super.setProperty(name, value); 1339 } 1340 1341 @Override 1342 public Base addChild(String name) throws FHIRException { 1343 if (name.equals("mode")) { 1344 throw new FHIRException("Cannot call addChild on a singleton property Bundle.mode"); 1345 } else if (name.equals("score")) { 1346 throw new FHIRException("Cannot call addChild on a singleton property Bundle.score"); 1347 } else 1348 return super.addChild(name); 1349 } 1350 1351 public BundleEntrySearchComponent copy() { 1352 BundleEntrySearchComponent dst = new BundleEntrySearchComponent(); 1353 copyValues(dst); 1354 dst.mode = mode == null ? null : mode.copy(); 1355 dst.score = score == null ? null : score.copy(); 1356 return dst; 1357 } 1358 1359 @Override 1360 public boolean equalsDeep(Base other) { 1361 if (!super.equalsDeep(other)) 1362 return false; 1363 if (!(other instanceof BundleEntrySearchComponent)) 1364 return false; 1365 BundleEntrySearchComponent o = (BundleEntrySearchComponent) other; 1366 return compareDeep(mode, o.mode, true) && compareDeep(score, o.score, true); 1367 } 1368 1369 @Override 1370 public boolean equalsShallow(Base other) { 1371 if (!super.equalsShallow(other)) 1372 return false; 1373 if (!(other instanceof BundleEntrySearchComponent)) 1374 return false; 1375 BundleEntrySearchComponent o = (BundleEntrySearchComponent) other; 1376 return compareValues(mode, o.mode, true) && compareValues(score, o.score, true); 1377 } 1378 1379 public boolean isEmpty() { 1380 return super.isEmpty() && (mode == null || mode.isEmpty()) && (score == null || score.isEmpty()); 1381 } 1382 1383 public String fhirType() { 1384 return "Bundle.entry.search"; 1385 1386 } 1387 1388 } 1389 1390 @Block() 1391 public static class BundleEntryRequestComponent extends BackboneElement implements IBaseBackboneElement { 1392 /** 1393 * The HTTP verb for this entry in either a update history, or a transaction/ 1394 * transaction response. 1395 */ 1396 @Child(name = "method", type = { CodeType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1397 @Description(shortDefinition = "GET | POST | PUT | DELETE", formalDefinition = "The HTTP verb for this entry in either a update history, or a transaction/ transaction response.") 1398 protected Enumeration<HTTPVerb> method; 1399 1400 /** 1401 * The URL for this entry, relative to the root (the address to which the 1402 * request is posted). 1403 */ 1404 @Child(name = "url", type = { UriType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1405 @Description(shortDefinition = "URL for HTTP equivalent of this entry", formalDefinition = "The URL for this entry, relative to the root (the address to which the request is posted).") 1406 protected UriType url; 1407 1408 /** 1409 * If the ETag values match, return a 304 Not modified status. See the API 1410 * documentation for ["Conditional Read"](http.html#cread). 1411 */ 1412 @Child(name = "ifNoneMatch", type = { 1413 StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1414 @Description(shortDefinition = "For managing cache currency", formalDefinition = "If the ETag values match, return a 304 Not modified status. See the API documentation for [\"Conditional Read\"](http.html#cread).") 1415 protected StringType ifNoneMatch; 1416 1417 /** 1418 * Only perform the operation if the last updated date matches. See the API 1419 * documentation for ["Conditional Read"](http.html#cread). 1420 */ 1421 @Child(name = "ifModifiedSince", type = { 1422 InstantType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1423 @Description(shortDefinition = "For managing update contention", formalDefinition = "Only perform the operation if the last updated date matches. See the API documentation for [\"Conditional Read\"](http.html#cread).") 1424 protected InstantType ifModifiedSince; 1425 1426 /** 1427 * Only perform the operation if the Etag value matches. For more information, 1428 * see the API section ["Managing Resource Contention"](http.html#concurrency). 1429 */ 1430 @Child(name = "ifMatch", type = { StringType.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 1431 @Description(shortDefinition = "For managing update contention", formalDefinition = "Only perform the operation if the Etag value matches. For more information, see the API section [\"Managing Resource Contention\"](http.html#concurrency).") 1432 protected StringType ifMatch; 1433 1434 /** 1435 * Instruct the server not to perform the create if a specified resource already 1436 * exists. For further information, see the API documentation for ["Conditional 1437 * Create"](http.html#ccreate). This is just the query portion of the URL - what 1438 * follows the "?" (not including the "?"). 1439 */ 1440 @Child(name = "ifNoneExist", type = { 1441 StringType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1442 @Description(shortDefinition = "For conditional creates", formalDefinition = "Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for [\"Conditional Create\"](http.html#ccreate). This is just the query portion of the URL - what follows the \"?\" (not including the \"?\").") 1443 protected StringType ifNoneExist; 1444 1445 private static final long serialVersionUID = -1349769744L; 1446 1447 /* 1448 * Constructor 1449 */ 1450 public BundleEntryRequestComponent() { 1451 super(); 1452 } 1453 1454 /* 1455 * Constructor 1456 */ 1457 public BundleEntryRequestComponent(Enumeration<HTTPVerb> method, UriType url) { 1458 super(); 1459 this.method = method; 1460 this.url = url; 1461 } 1462 1463 /** 1464 * @return {@link #method} (The HTTP verb for this entry in either a update 1465 * history, or a transaction/ transaction response.). This is the 1466 * underlying object with id, value and extensions. The accessor 1467 * "getMethod" gives direct access to the value 1468 */ 1469 public Enumeration<HTTPVerb> getMethodElement() { 1470 if (this.method == null) 1471 if (Configuration.errorOnAutoCreate()) 1472 throw new Error("Attempt to auto-create BundleEntryRequestComponent.method"); 1473 else if (Configuration.doAutoCreate()) 1474 this.method = new Enumeration<HTTPVerb>(new HTTPVerbEnumFactory()); // bb 1475 return this.method; 1476 } 1477 1478 public boolean hasMethodElement() { 1479 return this.method != null && !this.method.isEmpty(); 1480 } 1481 1482 public boolean hasMethod() { 1483 return this.method != null && !this.method.isEmpty(); 1484 } 1485 1486 /** 1487 * @param value {@link #method} (The HTTP verb for this entry in either a update 1488 * history, or a transaction/ transaction response.). This is the 1489 * underlying object with id, value and extensions. The accessor 1490 * "getMethod" gives direct access to the value 1491 */ 1492 public BundleEntryRequestComponent setMethodElement(Enumeration<HTTPVerb> value) { 1493 this.method = value; 1494 return this; 1495 } 1496 1497 /** 1498 * @return The HTTP verb for this entry in either a update history, or a 1499 * transaction/ transaction response. 1500 */ 1501 public HTTPVerb getMethod() { 1502 return this.method == null ? null : this.method.getValue(); 1503 } 1504 1505 /** 1506 * @param value The HTTP verb for this entry in either a update history, or a 1507 * transaction/ transaction response. 1508 */ 1509 public BundleEntryRequestComponent setMethod(HTTPVerb value) { 1510 if (this.method == null) 1511 this.method = new Enumeration<HTTPVerb>(new HTTPVerbEnumFactory()); 1512 this.method.setValue(value); 1513 return this; 1514 } 1515 1516 /** 1517 * @return {@link #url} (The URL for this entry, relative to the root (the 1518 * address to which the request is posted).). This is the underlying 1519 * object with id, value and extensions. The accessor "getUrl" gives 1520 * direct access to the value 1521 */ 1522 public UriType getUrlElement() { 1523 if (this.url == null) 1524 if (Configuration.errorOnAutoCreate()) 1525 throw new Error("Attempt to auto-create BundleEntryRequestComponent.url"); 1526 else if (Configuration.doAutoCreate()) 1527 this.url = new UriType(); // bb 1528 return this.url; 1529 } 1530 1531 public boolean hasUrlElement() { 1532 return this.url != null && !this.url.isEmpty(); 1533 } 1534 1535 public boolean hasUrl() { 1536 return this.url != null && !this.url.isEmpty(); 1537 } 1538 1539 /** 1540 * @param value {@link #url} (The URL for this entry, relative to the root (the 1541 * address to which the request is posted).). This is the 1542 * underlying object with id, value and extensions. The accessor 1543 * "getUrl" gives direct access to the value 1544 */ 1545 public BundleEntryRequestComponent setUrlElement(UriType value) { 1546 this.url = value; 1547 return this; 1548 } 1549 1550 /** 1551 * @return The URL for this entry, relative to the root (the address to which 1552 * the request is posted). 1553 */ 1554 public String getUrl() { 1555 return this.url == null ? null : this.url.getValue(); 1556 } 1557 1558 /** 1559 * @param value The URL for this entry, relative to the root (the address to 1560 * which the request is posted). 1561 */ 1562 public BundleEntryRequestComponent setUrl(String value) { 1563 if (this.url == null) 1564 this.url = new UriType(); 1565 this.url.setValue(value); 1566 return this; 1567 } 1568 1569 /** 1570 * @return {@link #ifNoneMatch} (If the ETag values match, return a 304 Not 1571 * modified status. See the API documentation for ["Conditional 1572 * Read"](http.html#cread).). This is the underlying object with id, 1573 * value and extensions. The accessor "getIfNoneMatch" gives direct 1574 * access to the value 1575 */ 1576 public StringType getIfNoneMatchElement() { 1577 if (this.ifNoneMatch == null) 1578 if (Configuration.errorOnAutoCreate()) 1579 throw new Error("Attempt to auto-create BundleEntryRequestComponent.ifNoneMatch"); 1580 else if (Configuration.doAutoCreate()) 1581 this.ifNoneMatch = new StringType(); // bb 1582 return this.ifNoneMatch; 1583 } 1584 1585 public boolean hasIfNoneMatchElement() { 1586 return this.ifNoneMatch != null && !this.ifNoneMatch.isEmpty(); 1587 } 1588 1589 public boolean hasIfNoneMatch() { 1590 return this.ifNoneMatch != null && !this.ifNoneMatch.isEmpty(); 1591 } 1592 1593 /** 1594 * @param value {@link #ifNoneMatch} (If the ETag values match, return a 304 Not 1595 * modified status. See the API documentation for ["Conditional 1596 * Read"](http.html#cread).). This is the underlying object with 1597 * id, value and extensions. The accessor "getIfNoneMatch" gives 1598 * direct access to the value 1599 */ 1600 public BundleEntryRequestComponent setIfNoneMatchElement(StringType value) { 1601 this.ifNoneMatch = value; 1602 return this; 1603 } 1604 1605 /** 1606 * @return If the ETag values match, return a 304 Not modified status. See the 1607 * API documentation for ["Conditional Read"](http.html#cread). 1608 */ 1609 public String getIfNoneMatch() { 1610 return this.ifNoneMatch == null ? null : this.ifNoneMatch.getValue(); 1611 } 1612 1613 /** 1614 * @param value If the ETag values match, return a 304 Not modified status. See 1615 * the API documentation for ["Conditional Read"](http.html#cread). 1616 */ 1617 public BundleEntryRequestComponent setIfNoneMatch(String value) { 1618 if (Utilities.noString(value)) 1619 this.ifNoneMatch = null; 1620 else { 1621 if (this.ifNoneMatch == null) 1622 this.ifNoneMatch = new StringType(); 1623 this.ifNoneMatch.setValue(value); 1624 } 1625 return this; 1626 } 1627 1628 /** 1629 * @return {@link #ifModifiedSince} (Only perform the operation if the last 1630 * updated date matches. See the API documentation for ["Conditional 1631 * Read"](http.html#cread).). This is the underlying object with id, 1632 * value and extensions. The accessor "getIfModifiedSince" gives direct 1633 * access to the value 1634 */ 1635 public InstantType getIfModifiedSinceElement() { 1636 if (this.ifModifiedSince == null) 1637 if (Configuration.errorOnAutoCreate()) 1638 throw new Error("Attempt to auto-create BundleEntryRequestComponent.ifModifiedSince"); 1639 else if (Configuration.doAutoCreate()) 1640 this.ifModifiedSince = new InstantType(); // bb 1641 return this.ifModifiedSince; 1642 } 1643 1644 public boolean hasIfModifiedSinceElement() { 1645 return this.ifModifiedSince != null && !this.ifModifiedSince.isEmpty(); 1646 } 1647 1648 public boolean hasIfModifiedSince() { 1649 return this.ifModifiedSince != null && !this.ifModifiedSince.isEmpty(); 1650 } 1651 1652 /** 1653 * @param value {@link #ifModifiedSince} (Only perform the operation if the last 1654 * updated date matches. See the API documentation for 1655 * ["Conditional Read"](http.html#cread).). This is the underlying 1656 * object with id, value and extensions. The accessor 1657 * "getIfModifiedSince" gives direct access to the value 1658 */ 1659 public BundleEntryRequestComponent setIfModifiedSinceElement(InstantType value) { 1660 this.ifModifiedSince = value; 1661 return this; 1662 } 1663 1664 /** 1665 * @return Only perform the operation if the last updated date matches. See the 1666 * API documentation for ["Conditional Read"](http.html#cread). 1667 */ 1668 public Date getIfModifiedSince() { 1669 return this.ifModifiedSince == null ? null : this.ifModifiedSince.getValue(); 1670 } 1671 1672 /** 1673 * @param value Only perform the operation if the last updated date matches. See 1674 * the API documentation for ["Conditional Read"](http.html#cread). 1675 */ 1676 public BundleEntryRequestComponent setIfModifiedSince(Date value) { 1677 if (value == null) 1678 this.ifModifiedSince = null; 1679 else { 1680 if (this.ifModifiedSince == null) 1681 this.ifModifiedSince = new InstantType(); 1682 this.ifModifiedSince.setValue(value); 1683 } 1684 return this; 1685 } 1686 1687 /** 1688 * @return {@link #ifMatch} (Only perform the operation if the Etag value 1689 * matches. For more information, see the API section ["Managing 1690 * Resource Contention"](http.html#concurrency).). This is the 1691 * underlying object with id, value and extensions. The accessor 1692 * "getIfMatch" gives direct access to the value 1693 */ 1694 public StringType getIfMatchElement() { 1695 if (this.ifMatch == null) 1696 if (Configuration.errorOnAutoCreate()) 1697 throw new Error("Attempt to auto-create BundleEntryRequestComponent.ifMatch"); 1698 else if (Configuration.doAutoCreate()) 1699 this.ifMatch = new StringType(); // bb 1700 return this.ifMatch; 1701 } 1702 1703 public boolean hasIfMatchElement() { 1704 return this.ifMatch != null && !this.ifMatch.isEmpty(); 1705 } 1706 1707 public boolean hasIfMatch() { 1708 return this.ifMatch != null && !this.ifMatch.isEmpty(); 1709 } 1710 1711 /** 1712 * @param value {@link #ifMatch} (Only perform the operation if the Etag value 1713 * matches. For more information, see the API section ["Managing 1714 * Resource Contention"](http.html#concurrency).). This is the 1715 * underlying object with id, value and extensions. The accessor 1716 * "getIfMatch" gives direct access to the value 1717 */ 1718 public BundleEntryRequestComponent setIfMatchElement(StringType value) { 1719 this.ifMatch = value; 1720 return this; 1721 } 1722 1723 /** 1724 * @return Only perform the operation if the Etag value matches. For more 1725 * information, see the API section ["Managing Resource 1726 * Contention"](http.html#concurrency). 1727 */ 1728 public String getIfMatch() { 1729 return this.ifMatch == null ? null : this.ifMatch.getValue(); 1730 } 1731 1732 /** 1733 * @param value Only perform the operation if the Etag value matches. For more 1734 * information, see the API section ["Managing Resource 1735 * Contention"](http.html#concurrency). 1736 */ 1737 public BundleEntryRequestComponent setIfMatch(String value) { 1738 if (Utilities.noString(value)) 1739 this.ifMatch = null; 1740 else { 1741 if (this.ifMatch == null) 1742 this.ifMatch = new StringType(); 1743 this.ifMatch.setValue(value); 1744 } 1745 return this; 1746 } 1747 1748 /** 1749 * @return {@link #ifNoneExist} (Instruct the server not to perform the create 1750 * if a specified resource already exists. For further information, see 1751 * the API documentation for ["Conditional Create"](http.html#ccreate). 1752 * This is just the query portion of the URL - what follows the "?" (not 1753 * including the "?").). This is the underlying object with id, value 1754 * and extensions. The accessor "getIfNoneExist" gives direct access to 1755 * the value 1756 */ 1757 public StringType getIfNoneExistElement() { 1758 if (this.ifNoneExist == null) 1759 if (Configuration.errorOnAutoCreate()) 1760 throw new Error("Attempt to auto-create BundleEntryRequestComponent.ifNoneExist"); 1761 else if (Configuration.doAutoCreate()) 1762 this.ifNoneExist = new StringType(); // bb 1763 return this.ifNoneExist; 1764 } 1765 1766 public boolean hasIfNoneExistElement() { 1767 return this.ifNoneExist != null && !this.ifNoneExist.isEmpty(); 1768 } 1769 1770 public boolean hasIfNoneExist() { 1771 return this.ifNoneExist != null && !this.ifNoneExist.isEmpty(); 1772 } 1773 1774 /** 1775 * @param value {@link #ifNoneExist} (Instruct the server not to perform the 1776 * create if a specified resource already exists. For further 1777 * information, see the API documentation for ["Conditional 1778 * Create"](http.html#ccreate). This is just the query portion of 1779 * the URL - what follows the "?" (not including the "?").). This 1780 * is the underlying object with id, value and extensions. The 1781 * accessor "getIfNoneExist" gives direct access to the value 1782 */ 1783 public BundleEntryRequestComponent setIfNoneExistElement(StringType value) { 1784 this.ifNoneExist = value; 1785 return this; 1786 } 1787 1788 /** 1789 * @return Instruct the server not to perform the create if a specified resource 1790 * already exists. For further information, see the API documentation 1791 * for ["Conditional Create"](http.html#ccreate). This is just the query 1792 * portion of the URL - what follows the "?" (not including the "?"). 1793 */ 1794 public String getIfNoneExist() { 1795 return this.ifNoneExist == null ? null : this.ifNoneExist.getValue(); 1796 } 1797 1798 /** 1799 * @param value Instruct the server not to perform the create if a specified 1800 * resource already exists. For further information, see the API 1801 * documentation for ["Conditional Create"](http.html#ccreate). 1802 * This is just the query portion of the URL - what follows the "?" 1803 * (not including the "?"). 1804 */ 1805 public BundleEntryRequestComponent setIfNoneExist(String value) { 1806 if (Utilities.noString(value)) 1807 this.ifNoneExist = null; 1808 else { 1809 if (this.ifNoneExist == null) 1810 this.ifNoneExist = new StringType(); 1811 this.ifNoneExist.setValue(value); 1812 } 1813 return this; 1814 } 1815 1816 protected void listChildren(List<Property> childrenList) { 1817 super.listChildren(childrenList); 1818 childrenList.add(new Property("method", "code", 1819 "The HTTP verb for this entry in either a update history, or a transaction/ transaction response.", 0, 1820 java.lang.Integer.MAX_VALUE, method)); 1821 childrenList.add(new Property("url", "uri", 1822 "The URL for this entry, relative to the root (the address to which the request is posted).", 0, 1823 java.lang.Integer.MAX_VALUE, url)); 1824 childrenList.add(new Property("ifNoneMatch", "string", 1825 "If the ETag values match, return a 304 Not modified status. See the API documentation for [\"Conditional Read\"](http.html#cread).", 1826 0, java.lang.Integer.MAX_VALUE, ifNoneMatch)); 1827 childrenList.add(new Property("ifModifiedSince", "instant", 1828 "Only perform the operation if the last updated date matches. See the API documentation for [\"Conditional Read\"](http.html#cread).", 1829 0, java.lang.Integer.MAX_VALUE, ifModifiedSince)); 1830 childrenList.add(new Property("ifMatch", "string", 1831 "Only perform the operation if the Etag value matches. For more information, see the API section [\"Managing Resource Contention\"](http.html#concurrency).", 1832 0, java.lang.Integer.MAX_VALUE, ifMatch)); 1833 childrenList.add(new Property("ifNoneExist", "string", 1834 "Instruct the server not to perform the create if a specified resource already exists. For further information, see the API documentation for [\"Conditional Create\"](http.html#ccreate). This is just the query portion of the URL - what follows the \"?\" (not including the \"?\").", 1835 0, java.lang.Integer.MAX_VALUE, ifNoneExist)); 1836 } 1837 1838 @Override 1839 public void setProperty(String name, Base value) throws FHIRException { 1840 if (name.equals("method")) 1841 this.method = new HTTPVerbEnumFactory().fromType(value); // Enumeration<HTTPVerb> 1842 else if (name.equals("url")) 1843 this.url = castToUri(value); // UriType 1844 else if (name.equals("ifNoneMatch")) 1845 this.ifNoneMatch = castToString(value); // StringType 1846 else if (name.equals("ifModifiedSince")) 1847 this.ifModifiedSince = castToInstant(value); // InstantType 1848 else if (name.equals("ifMatch")) 1849 this.ifMatch = castToString(value); // StringType 1850 else if (name.equals("ifNoneExist")) 1851 this.ifNoneExist = castToString(value); // StringType 1852 else 1853 super.setProperty(name, value); 1854 } 1855 1856 @Override 1857 public Base addChild(String name) throws FHIRException { 1858 if (name.equals("method")) { 1859 throw new FHIRException("Cannot call addChild on a singleton property Bundle.method"); 1860 } else if (name.equals("url")) { 1861 throw new FHIRException("Cannot call addChild on a singleton property Bundle.url"); 1862 } else if (name.equals("ifNoneMatch")) { 1863 throw new FHIRException("Cannot call addChild on a singleton property Bundle.ifNoneMatch"); 1864 } else if (name.equals("ifModifiedSince")) { 1865 throw new FHIRException("Cannot call addChild on a singleton property Bundle.ifModifiedSince"); 1866 } else if (name.equals("ifMatch")) { 1867 throw new FHIRException("Cannot call addChild on a singleton property Bundle.ifMatch"); 1868 } else if (name.equals("ifNoneExist")) { 1869 throw new FHIRException("Cannot call addChild on a singleton property Bundle.ifNoneExist"); 1870 } else 1871 return super.addChild(name); 1872 } 1873 1874 public BundleEntryRequestComponent copy() { 1875 BundleEntryRequestComponent dst = new BundleEntryRequestComponent(); 1876 copyValues(dst); 1877 dst.method = method == null ? null : method.copy(); 1878 dst.url = url == null ? null : url.copy(); 1879 dst.ifNoneMatch = ifNoneMatch == null ? null : ifNoneMatch.copy(); 1880 dst.ifModifiedSince = ifModifiedSince == null ? null : ifModifiedSince.copy(); 1881 dst.ifMatch = ifMatch == null ? null : ifMatch.copy(); 1882 dst.ifNoneExist = ifNoneExist == null ? null : ifNoneExist.copy(); 1883 return dst; 1884 } 1885 1886 @Override 1887 public boolean equalsDeep(Base other) { 1888 if (!super.equalsDeep(other)) 1889 return false; 1890 if (!(other instanceof BundleEntryRequestComponent)) 1891 return false; 1892 BundleEntryRequestComponent o = (BundleEntryRequestComponent) other; 1893 return compareDeep(method, o.method, true) && compareDeep(url, o.url, true) 1894 && compareDeep(ifNoneMatch, o.ifNoneMatch, true) && compareDeep(ifModifiedSince, o.ifModifiedSince, true) 1895 && compareDeep(ifMatch, o.ifMatch, true) && compareDeep(ifNoneExist, o.ifNoneExist, true); 1896 } 1897 1898 @Override 1899 public boolean equalsShallow(Base other) { 1900 if (!super.equalsShallow(other)) 1901 return false; 1902 if (!(other instanceof BundleEntryRequestComponent)) 1903 return false; 1904 BundleEntryRequestComponent o = (BundleEntryRequestComponent) other; 1905 return compareValues(method, o.method, true) && compareValues(url, o.url, true) 1906 && compareValues(ifNoneMatch, o.ifNoneMatch, true) && compareValues(ifModifiedSince, o.ifModifiedSince, true) 1907 && compareValues(ifMatch, o.ifMatch, true) && compareValues(ifNoneExist, o.ifNoneExist, true); 1908 } 1909 1910 public boolean isEmpty() { 1911 return super.isEmpty() && (method == null || method.isEmpty()) && (url == null || url.isEmpty()) 1912 && (ifNoneMatch == null || ifNoneMatch.isEmpty()) && (ifModifiedSince == null || ifModifiedSince.isEmpty()) 1913 && (ifMatch == null || ifMatch.isEmpty()) && (ifNoneExist == null || ifNoneExist.isEmpty()); 1914 } 1915 1916 public String fhirType() { 1917 return "Bundle.entry.request"; 1918 1919 } 1920 1921 } 1922 1923 @Block() 1924 public static class BundleEntryResponseComponent extends BackboneElement implements IBaseBackboneElement { 1925 /** 1926 * The status code returned by processing this entry. 1927 */ 1928 @Child(name = "status", type = { StringType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1929 @Description(shortDefinition = "Status return code for entry", formalDefinition = "The status code returned by processing this entry.") 1930 protected StringType status; 1931 1932 /** 1933 * The location header created by processing this operation. 1934 */ 1935 @Child(name = "location", type = { UriType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1936 @Description(shortDefinition = "The location, if the operation returns a location", formalDefinition = "The location header created by processing this operation.") 1937 protected UriType location; 1938 1939 /** 1940 * The etag for the resource, it the operation for the entry produced a 1941 * versioned resource. 1942 */ 1943 @Child(name = "etag", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1944 @Description(shortDefinition = "The etag for the resource (if relevant)", formalDefinition = "The etag for the resource, it the operation for the entry produced a versioned resource.") 1945 protected StringType etag; 1946 1947 /** 1948 * The date/time that the resource was modified on the server. 1949 */ 1950 @Child(name = "lastModified", type = { 1951 InstantType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 1952 @Description(shortDefinition = "Server's date time modified", formalDefinition = "The date/time that the resource was modified on the server.") 1953 protected InstantType lastModified; 1954 1955 private static final long serialVersionUID = -1526413234L; 1956 1957 /* 1958 * Constructor 1959 */ 1960 public BundleEntryResponseComponent() { 1961 super(); 1962 } 1963 1964 /* 1965 * Constructor 1966 */ 1967 public BundleEntryResponseComponent(StringType status) { 1968 super(); 1969 this.status = status; 1970 } 1971 1972 /** 1973 * @return {@link #status} (The status code returned by processing this entry.). 1974 * This is the underlying object with id, value and extensions. The 1975 * accessor "getStatus" gives direct access to the value 1976 */ 1977 public StringType getStatusElement() { 1978 if (this.status == null) 1979 if (Configuration.errorOnAutoCreate()) 1980 throw new Error("Attempt to auto-create BundleEntryResponseComponent.status"); 1981 else if (Configuration.doAutoCreate()) 1982 this.status = new StringType(); // bb 1983 return this.status; 1984 } 1985 1986 public boolean hasStatusElement() { 1987 return this.status != null && !this.status.isEmpty(); 1988 } 1989 1990 public boolean hasStatus() { 1991 return this.status != null && !this.status.isEmpty(); 1992 } 1993 1994 /** 1995 * @param value {@link #status} (The status code returned by processing this 1996 * entry.). This is the underlying object with id, value and 1997 * extensions. The accessor "getStatus" gives direct access to the 1998 * value 1999 */ 2000 public BundleEntryResponseComponent setStatusElement(StringType value) { 2001 this.status = value; 2002 return this; 2003 } 2004 2005 /** 2006 * @return The status code returned by processing this entry. 2007 */ 2008 public String getStatus() { 2009 return this.status == null ? null : this.status.getValue(); 2010 } 2011 2012 /** 2013 * @param value The status code returned by processing this entry. 2014 */ 2015 public BundleEntryResponseComponent setStatus(String value) { 2016 if (this.status == null) 2017 this.status = new StringType(); 2018 this.status.setValue(value); 2019 return this; 2020 } 2021 2022 /** 2023 * @return {@link #location} (The location header created by processing this 2024 * operation.). This is the underlying object with id, value and 2025 * extensions. The accessor "getLocation" gives direct access to the 2026 * value 2027 */ 2028 public UriType getLocationElement() { 2029 if (this.location == null) 2030 if (Configuration.errorOnAutoCreate()) 2031 throw new Error("Attempt to auto-create BundleEntryResponseComponent.location"); 2032 else if (Configuration.doAutoCreate()) 2033 this.location = new UriType(); // bb 2034 return this.location; 2035 } 2036 2037 public boolean hasLocationElement() { 2038 return this.location != null && !this.location.isEmpty(); 2039 } 2040 2041 public boolean hasLocation() { 2042 return this.location != null && !this.location.isEmpty(); 2043 } 2044 2045 /** 2046 * @param value {@link #location} (The location header created by processing 2047 * this operation.). This is the underlying object with id, value 2048 * and extensions. The accessor "getLocation" gives direct access 2049 * to the value 2050 */ 2051 public BundleEntryResponseComponent setLocationElement(UriType value) { 2052 this.location = value; 2053 return this; 2054 } 2055 2056 /** 2057 * @return The location header created by processing this operation. 2058 */ 2059 public String getLocation() { 2060 return this.location == null ? null : this.location.getValue(); 2061 } 2062 2063 /** 2064 * @param value The location header created by processing this operation. 2065 */ 2066 public BundleEntryResponseComponent setLocation(String value) { 2067 if (Utilities.noString(value)) 2068 this.location = null; 2069 else { 2070 if (this.location == null) 2071 this.location = new UriType(); 2072 this.location.setValue(value); 2073 } 2074 return this; 2075 } 2076 2077 /** 2078 * @return {@link #etag} (The etag for the resource, it the operation for the 2079 * entry produced a versioned resource.). This is the underlying object 2080 * with id, value and extensions. The accessor "getEtag" gives direct 2081 * access to the value 2082 */ 2083 public StringType getEtagElement() { 2084 if (this.etag == null) 2085 if (Configuration.errorOnAutoCreate()) 2086 throw new Error("Attempt to auto-create BundleEntryResponseComponent.etag"); 2087 else if (Configuration.doAutoCreate()) 2088 this.etag = new StringType(); // bb 2089 return this.etag; 2090 } 2091 2092 public boolean hasEtagElement() { 2093 return this.etag != null && !this.etag.isEmpty(); 2094 } 2095 2096 public boolean hasEtag() { 2097 return this.etag != null && !this.etag.isEmpty(); 2098 } 2099 2100 /** 2101 * @param value {@link #etag} (The etag for the resource, it the operation for 2102 * the entry produced a versioned resource.). This is the 2103 * underlying object with id, value and extensions. The accessor 2104 * "getEtag" gives direct access to the value 2105 */ 2106 public BundleEntryResponseComponent setEtagElement(StringType value) { 2107 this.etag = value; 2108 return this; 2109 } 2110 2111 /** 2112 * @return The etag for the resource, it the operation for the entry produced a 2113 * versioned resource. 2114 */ 2115 public String getEtag() { 2116 return this.etag == null ? null : this.etag.getValue(); 2117 } 2118 2119 /** 2120 * @param value The etag for the resource, it the operation for the entry 2121 * produced a versioned resource. 2122 */ 2123 public BundleEntryResponseComponent setEtag(String value) { 2124 if (Utilities.noString(value)) 2125 this.etag = null; 2126 else { 2127 if (this.etag == null) 2128 this.etag = new StringType(); 2129 this.etag.setValue(value); 2130 } 2131 return this; 2132 } 2133 2134 /** 2135 * @return {@link #lastModified} (The date/time that the resource was modified 2136 * on the server.). This is the underlying object with id, value and 2137 * extensions. The accessor "getLastModified" gives direct access to the 2138 * value 2139 */ 2140 public InstantType getLastModifiedElement() { 2141 if (this.lastModified == null) 2142 if (Configuration.errorOnAutoCreate()) 2143 throw new Error("Attempt to auto-create BundleEntryResponseComponent.lastModified"); 2144 else if (Configuration.doAutoCreate()) 2145 this.lastModified = new InstantType(); // bb 2146 return this.lastModified; 2147 } 2148 2149 public boolean hasLastModifiedElement() { 2150 return this.lastModified != null && !this.lastModified.isEmpty(); 2151 } 2152 2153 public boolean hasLastModified() { 2154 return this.lastModified != null && !this.lastModified.isEmpty(); 2155 } 2156 2157 /** 2158 * @param value {@link #lastModified} (The date/time that the resource was 2159 * modified on the server.). This is the underlying object with id, 2160 * value and extensions. The accessor "getLastModified" gives 2161 * direct access to the value 2162 */ 2163 public BundleEntryResponseComponent setLastModifiedElement(InstantType value) { 2164 this.lastModified = value; 2165 return this; 2166 } 2167 2168 /** 2169 * @return The date/time that the resource was modified on the server. 2170 */ 2171 public Date getLastModified() { 2172 return this.lastModified == null ? null : this.lastModified.getValue(); 2173 } 2174 2175 /** 2176 * @param value The date/time that the resource was modified on the server. 2177 */ 2178 public BundleEntryResponseComponent setLastModified(Date value) { 2179 if (value == null) 2180 this.lastModified = null; 2181 else { 2182 if (this.lastModified == null) 2183 this.lastModified = new InstantType(); 2184 this.lastModified.setValue(value); 2185 } 2186 return this; 2187 } 2188 2189 protected void listChildren(List<Property> childrenList) { 2190 super.listChildren(childrenList); 2191 childrenList.add(new Property("status", "string", "The status code returned by processing this entry.", 0, 2192 java.lang.Integer.MAX_VALUE, status)); 2193 childrenList.add(new Property("location", "uri", "The location header created by processing this operation.", 0, 2194 java.lang.Integer.MAX_VALUE, location)); 2195 childrenList.add(new Property("etag", "string", 2196 "The etag for the resource, it the operation for the entry produced a versioned resource.", 0, 2197 java.lang.Integer.MAX_VALUE, etag)); 2198 childrenList.add(new Property("lastModified", "instant", 2199 "The date/time that the resource was modified on the server.", 0, java.lang.Integer.MAX_VALUE, lastModified)); 2200 } 2201 2202 @Override 2203 public void setProperty(String name, Base value) throws FHIRException { 2204 if (name.equals("status")) 2205 this.status = castToString(value); // StringType 2206 else if (name.equals("location")) 2207 this.location = castToUri(value); // UriType 2208 else if (name.equals("etag")) 2209 this.etag = castToString(value); // StringType 2210 else if (name.equals("lastModified")) 2211 this.lastModified = castToInstant(value); // InstantType 2212 else 2213 super.setProperty(name, value); 2214 } 2215 2216 @Override 2217 public Base addChild(String name) throws FHIRException { 2218 if (name.equals("status")) { 2219 throw new FHIRException("Cannot call addChild on a singleton property Bundle.status"); 2220 } else if (name.equals("location")) { 2221 throw new FHIRException("Cannot call addChild on a singleton property Bundle.location"); 2222 } else if (name.equals("etag")) { 2223 throw new FHIRException("Cannot call addChild on a singleton property Bundle.etag"); 2224 } else if (name.equals("lastModified")) { 2225 throw new FHIRException("Cannot call addChild on a singleton property Bundle.lastModified"); 2226 } else 2227 return super.addChild(name); 2228 } 2229 2230 public BundleEntryResponseComponent copy() { 2231 BundleEntryResponseComponent dst = new BundleEntryResponseComponent(); 2232 copyValues(dst); 2233 dst.status = status == null ? null : status.copy(); 2234 dst.location = location == null ? null : location.copy(); 2235 dst.etag = etag == null ? null : etag.copy(); 2236 dst.lastModified = lastModified == null ? null : lastModified.copy(); 2237 return dst; 2238 } 2239 2240 @Override 2241 public boolean equalsDeep(Base other) { 2242 if (!super.equalsDeep(other)) 2243 return false; 2244 if (!(other instanceof BundleEntryResponseComponent)) 2245 return false; 2246 BundleEntryResponseComponent o = (BundleEntryResponseComponent) other; 2247 return compareDeep(status, o.status, true) && compareDeep(location, o.location, true) 2248 && compareDeep(etag, o.etag, true) && compareDeep(lastModified, o.lastModified, true); 2249 } 2250 2251 @Override 2252 public boolean equalsShallow(Base other) { 2253 if (!super.equalsShallow(other)) 2254 return false; 2255 if (!(other instanceof BundleEntryResponseComponent)) 2256 return false; 2257 BundleEntryResponseComponent o = (BundleEntryResponseComponent) other; 2258 return compareValues(status, o.status, true) && compareValues(location, o.location, true) 2259 && compareValues(etag, o.etag, true) && compareValues(lastModified, o.lastModified, true); 2260 } 2261 2262 public boolean isEmpty() { 2263 return super.isEmpty() && (status == null || status.isEmpty()) && (location == null || location.isEmpty()) 2264 && (etag == null || etag.isEmpty()) && (lastModified == null || lastModified.isEmpty()); 2265 } 2266 2267 public String fhirType() { 2268 return "Bundle.entry.response"; 2269 2270 } 2271 2272 } 2273 2274 /** 2275 * Indicates the purpose of this bundle- how it was intended to be used. 2276 */ 2277 @Child(name = "type", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 2278 @Description(shortDefinition = "document | message | transaction | transaction-response | batch | batch-response | history | searchset | collection", formalDefinition = "Indicates the purpose of this bundle- how it was intended to be used.") 2279 protected Enumeration<BundleType> type; 2280 2281 /** 2282 * If a set of search matches, this is the total number of matches for the 2283 * search (as opposed to the number of results in this bundle). 2284 */ 2285 @Child(name = "total", type = { 2286 UnsignedIntType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2287 @Description(shortDefinition = "If search, the total number of matches", formalDefinition = "If a set of search matches, this is the total number of matches for the search (as opposed to the number of results in this bundle).") 2288 protected UnsignedIntType total; 2289 2290 /** 2291 * A series of links that provide context to this bundle. 2292 */ 2293 @Child(name = "link", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2294 @Description(shortDefinition = "Links related to this Bundle", formalDefinition = "A series of links that provide context to this bundle.") 2295 protected List<BundleLinkComponent> link; 2296 2297 /** 2298 * An entry in a bundle resource - will either contain a resource, or 2299 * information about a resource (transactions and history only). 2300 */ 2301 @Child(name = "entry", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2302 @Description(shortDefinition = "Entry in the bundle - will have a resource, or information", formalDefinition = "An entry in a bundle resource - will either contain a resource, or information about a resource (transactions and history only).") 2303 protected List<BundleEntryComponent> entry; 2304 2305 /** 2306 * Digital Signature - base64 encoded. XML DigSIg or a JWT. 2307 */ 2308 @Child(name = "signature", type = { Signature.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2309 @Description(shortDefinition = "Digital Signature", formalDefinition = "Digital Signature - base64 encoded. XML DigSIg or a JWT.") 2310 protected Signature signature; 2311 2312 private static final long serialVersionUID = -2041954721L; 2313 2314 /* 2315 * Constructor 2316 */ 2317 public Bundle() { 2318 super(); 2319 } 2320 2321 /* 2322 * Constructor 2323 */ 2324 public Bundle(Enumeration<BundleType> type) { 2325 super(); 2326 this.type = type; 2327 } 2328 2329 /** 2330 * @return {@link #type} (Indicates the purpose of this bundle- how it was 2331 * intended to be used.). This is the underlying object with id, value 2332 * and extensions. The accessor "getType" gives direct access to the 2333 * value 2334 */ 2335 public Enumeration<BundleType> getTypeElement() { 2336 if (this.type == null) 2337 if (Configuration.errorOnAutoCreate()) 2338 throw new Error("Attempt to auto-create Bundle.type"); 2339 else if (Configuration.doAutoCreate()) 2340 this.type = new Enumeration<BundleType>(new BundleTypeEnumFactory()); // bb 2341 return this.type; 2342 } 2343 2344 public boolean hasTypeElement() { 2345 return this.type != null && !this.type.isEmpty(); 2346 } 2347 2348 public boolean hasType() { 2349 return this.type != null && !this.type.isEmpty(); 2350 } 2351 2352 /** 2353 * @param value {@link #type} (Indicates the purpose of this bundle- how it was 2354 * intended to be used.). This is the underlying object with id, 2355 * value and extensions. The accessor "getType" gives direct access 2356 * to the value 2357 */ 2358 public Bundle setTypeElement(Enumeration<BundleType> value) { 2359 this.type = value; 2360 return this; 2361 } 2362 2363 /** 2364 * @return Indicates the purpose of this bundle- how it was intended to be used. 2365 */ 2366 public BundleType getType() { 2367 return this.type == null ? null : this.type.getValue(); 2368 } 2369 2370 /** 2371 * @param value Indicates the purpose of this bundle- how it was intended to be 2372 * used. 2373 */ 2374 public Bundle setType(BundleType value) { 2375 if (this.type == null) 2376 this.type = new Enumeration<BundleType>(new BundleTypeEnumFactory()); 2377 this.type.setValue(value); 2378 return this; 2379 } 2380 2381 /** 2382 * @return {@link #total} (If a set of search matches, this is the total number 2383 * of matches for the search (as opposed to the number of results in 2384 * this bundle).). This is the underlying object with id, value and 2385 * extensions. The accessor "getTotal" gives direct access to the value 2386 */ 2387 public UnsignedIntType getTotalElement() { 2388 if (this.total == null) 2389 if (Configuration.errorOnAutoCreate()) 2390 throw new Error("Attempt to auto-create Bundle.total"); 2391 else if (Configuration.doAutoCreate()) 2392 this.total = new UnsignedIntType(); // bb 2393 return this.total; 2394 } 2395 2396 public boolean hasTotalElement() { 2397 return this.total != null && !this.total.isEmpty(); 2398 } 2399 2400 public boolean hasTotal() { 2401 return this.total != null && !this.total.isEmpty(); 2402 } 2403 2404 /** 2405 * @param value {@link #total} (If a set of search matches, this is the total 2406 * number of matches for the search (as opposed to the number of 2407 * results in this bundle).). This is the underlying object with 2408 * id, value and extensions. The accessor "getTotal" gives direct 2409 * access to the value 2410 */ 2411 public Bundle setTotalElement(UnsignedIntType value) { 2412 this.total = value; 2413 return this; 2414 } 2415 2416 /** 2417 * @return If a set of search matches, this is the total number of matches for 2418 * the search (as opposed to the number of results in this bundle). 2419 */ 2420 public int getTotal() { 2421 return this.total == null || this.total.isEmpty() ? 0 : this.total.getValue(); 2422 } 2423 2424 /** 2425 * @param value If a set of search matches, this is the total number of matches 2426 * for the search (as opposed to the number of results in this 2427 * bundle). 2428 */ 2429 public Bundle setTotal(int value) { 2430 if (this.total == null) 2431 this.total = new UnsignedIntType(); 2432 this.total.setValue(value); 2433 return this; 2434 } 2435 2436 /** 2437 * @return {@link #link} (A series of links that provide context to this 2438 * bundle.) 2439 */ 2440 public List<BundleLinkComponent> getLink() { 2441 if (this.link == null) 2442 this.link = new ArrayList<BundleLinkComponent>(); 2443 return this.link; 2444 } 2445 2446 public boolean hasLink() { 2447 if (this.link == null) 2448 return false; 2449 for (BundleLinkComponent item : this.link) 2450 if (!item.isEmpty()) 2451 return true; 2452 return false; 2453 } 2454 2455 /** 2456 * @return {@link #link} (A series of links that provide context to this 2457 * bundle.) 2458 */ 2459 // syntactic sugar 2460 public BundleLinkComponent addLink() { // 3 2461 BundleLinkComponent t = new BundleLinkComponent(); 2462 if (this.link == null) 2463 this.link = new ArrayList<BundleLinkComponent>(); 2464 this.link.add(t); 2465 return t; 2466 } 2467 2468 // syntactic sugar 2469 public Bundle addLink(BundleLinkComponent t) { // 3 2470 if (t == null) 2471 return this; 2472 if (this.link == null) 2473 this.link = new ArrayList<BundleLinkComponent>(); 2474 this.link.add(t); 2475 return this; 2476 } 2477 2478 /** 2479 * @return {@link #entry} (An entry in a bundle resource - will either contain a 2480 * resource, or information about a resource (transactions and history 2481 * only).) 2482 */ 2483 public List<BundleEntryComponent> getEntry() { 2484 if (this.entry == null) 2485 this.entry = new ArrayList<BundleEntryComponent>(); 2486 return this.entry; 2487 } 2488 2489 public boolean hasEntry() { 2490 if (this.entry == null) 2491 return false; 2492 for (BundleEntryComponent item : this.entry) 2493 if (!item.isEmpty()) 2494 return true; 2495 return false; 2496 } 2497 2498 /** 2499 * @return {@link #entry} (An entry in a bundle resource - will either contain a 2500 * resource, or information about a resource (transactions and history 2501 * only).) 2502 */ 2503 // syntactic sugar 2504 public BundleEntryComponent addEntry() { // 3 2505 BundleEntryComponent t = new BundleEntryComponent(); 2506 if (this.entry == null) 2507 this.entry = new ArrayList<BundleEntryComponent>(); 2508 this.entry.add(t); 2509 return t; 2510 } 2511 2512 // syntactic sugar 2513 public Bundle addEntry(BundleEntryComponent t) { // 3 2514 if (t == null) 2515 return this; 2516 if (this.entry == null) 2517 this.entry = new ArrayList<BundleEntryComponent>(); 2518 this.entry.add(t); 2519 return this; 2520 } 2521 2522 /** 2523 * @return {@link #signature} (Digital Signature - base64 encoded. XML DigSIg or 2524 * a JWT.) 2525 */ 2526 public Signature getSignature() { 2527 if (this.signature == null) 2528 if (Configuration.errorOnAutoCreate()) 2529 throw new Error("Attempt to auto-create Bundle.signature"); 2530 else if (Configuration.doAutoCreate()) 2531 this.signature = new Signature(); // cc 2532 return this.signature; 2533 } 2534 2535 public boolean hasSignature() { 2536 return this.signature != null && !this.signature.isEmpty(); 2537 } 2538 2539 /** 2540 * @param value {@link #signature} (Digital Signature - base64 encoded. XML 2541 * DigSIg or a JWT.) 2542 */ 2543 public Bundle setSignature(Signature value) { 2544 this.signature = value; 2545 return this; 2546 } 2547 2548 /** 2549 * Returns the {@link #getLink() link} which matches a given 2550 * {@link BundleLinkComponent#getRelation() relation}. If no link is found which 2551 * matches the given relation, returns <code>null</code>. If more than one link 2552 * is found which matches the given relation, returns the first matching 2553 * BundleLinkComponent. 2554 * 2555 * @param theRelation The relation, such as "next", or "self. See the constants 2556 * such as {@link IBaseBundle#LINK_SELF} and 2557 * {@link IBaseBundle#LINK_NEXT}. 2558 * @return Returns a matching BundleLinkComponent, or <code>null</code> 2559 * @see IBaseBundle#LINK_NEXT 2560 * @see IBaseBundle#LINK_PREV 2561 * @see IBaseBundle#LINK_SELF 2562 */ 2563 public BundleLinkComponent getLink(String theRelation) { 2564 org.apache.commons.lang3.Validate.notBlank(theRelation, "theRelation may not be null or empty"); 2565 for (BundleLinkComponent next : getLink()) { 2566 if (theRelation.equals(next.getRelation())) { 2567 return next; 2568 } 2569 } 2570 return null; 2571 } 2572 2573 /** 2574 * Returns the {@link #getLink() link} which matches a given 2575 * {@link BundleLinkComponent#getRelation() relation}. If no link is found which 2576 * matches the given relation, creates a new BundleLinkComponent with the given 2577 * relation and adds it to this Bundle. If more than one link is found which 2578 * matches the given relation, returns the first matching BundleLinkComponent. 2579 * 2580 * @param theRelation The relation, such as "next", or "self. See the constants 2581 * such as {@link IBaseBundle#LINK_SELF} and 2582 * {@link IBaseBundle#LINK_NEXT}. 2583 * @return Returns a matching BundleLinkComponent, or <code>null</code> 2584 * @see IBaseBundle#LINK_NEXT 2585 * @see IBaseBundle#LINK_PREV 2586 * @see IBaseBundle#LINK_SELF 2587 */ 2588 public BundleLinkComponent getLinkOrCreate(String theRelation) { 2589 org.apache.commons.lang3.Validate.notBlank(theRelation, "theRelation may not be null or empty"); 2590 for (BundleLinkComponent next : getLink()) { 2591 if (theRelation.equals(next.getRelation())) { 2592 return next; 2593 } 2594 } 2595 BundleLinkComponent retVal = new BundleLinkComponent(); 2596 retVal.setRelation(theRelation); 2597 getLink().add(retVal); 2598 return retVal; 2599 } 2600 2601 protected void listChildren(List<Property> childrenList) { 2602 super.listChildren(childrenList); 2603 childrenList.add(new Property("type", "code", 2604 "Indicates the purpose of this bundle- how it was intended to be used.", 0, java.lang.Integer.MAX_VALUE, type)); 2605 childrenList.add(new Property("total", "unsignedInt", 2606 "If a set of search matches, this is the total number of matches for the search (as opposed to the number of results in this bundle).", 2607 0, java.lang.Integer.MAX_VALUE, total)); 2608 childrenList.add(new Property("link", "", "A series of links that provide context to this bundle.", 0, 2609 java.lang.Integer.MAX_VALUE, link)); 2610 childrenList.add(new Property("entry", "", 2611 "An entry in a bundle resource - will either contain a resource, or information about a resource (transactions and history only).", 2612 0, java.lang.Integer.MAX_VALUE, entry)); 2613 childrenList.add(new Property("signature", "Signature", "Digital Signature - base64 encoded. XML DigSIg or a JWT.", 2614 0, java.lang.Integer.MAX_VALUE, signature)); 2615 } 2616 2617 @Override 2618 public void setProperty(String name, Base value) throws FHIRException { 2619 if (name.equals("type")) 2620 this.type = new BundleTypeEnumFactory().fromType(value); // Enumeration<BundleType> 2621 else if (name.equals("total")) 2622 this.total = castToUnsignedInt(value); // UnsignedIntType 2623 else if (name.equals("link")) 2624 this.getLink().add((BundleLinkComponent) value); 2625 else if (name.equals("entry")) 2626 this.getEntry().add((BundleEntryComponent) value); 2627 else if (name.equals("signature")) 2628 this.signature = castToSignature(value); // Signature 2629 else 2630 super.setProperty(name, value); 2631 } 2632 2633 @Override 2634 public Base addChild(String name) throws FHIRException { 2635 if (name.equals("type")) { 2636 throw new FHIRException("Cannot call addChild on a singleton property Bundle.type"); 2637 } else if (name.equals("total")) { 2638 throw new FHIRException("Cannot call addChild on a singleton property Bundle.total"); 2639 } else if (name.equals("link")) { 2640 return addLink(); 2641 } else if (name.equals("entry")) { 2642 return addEntry(); 2643 } else if (name.equals("signature")) { 2644 this.signature = new Signature(); 2645 return this.signature; 2646 } else 2647 return super.addChild(name); 2648 } 2649 2650 public String fhirType() { 2651 return "Bundle"; 2652 2653 } 2654 2655 public Bundle copy() { 2656 Bundle dst = new Bundle(); 2657 copyValues(dst); 2658 dst.type = type == null ? null : type.copy(); 2659 dst.total = total == null ? null : total.copy(); 2660 if (link != null) { 2661 dst.link = new ArrayList<BundleLinkComponent>(); 2662 for (BundleLinkComponent i : link) 2663 dst.link.add(i.copy()); 2664 } 2665 ; 2666 if (entry != null) { 2667 dst.entry = new ArrayList<BundleEntryComponent>(); 2668 for (BundleEntryComponent i : entry) 2669 dst.entry.add(i.copy()); 2670 } 2671 ; 2672 dst.signature = signature == null ? null : signature.copy(); 2673 return dst; 2674 } 2675 2676 protected Bundle typedCopy() { 2677 return copy(); 2678 } 2679 2680 @Override 2681 public boolean equalsDeep(Base other) { 2682 if (!super.equalsDeep(other)) 2683 return false; 2684 if (!(other instanceof Bundle)) 2685 return false; 2686 Bundle o = (Bundle) other; 2687 return compareDeep(type, o.type, true) && compareDeep(total, o.total, true) && compareDeep(link, o.link, true) 2688 && compareDeep(entry, o.entry, true) && compareDeep(signature, o.signature, true); 2689 } 2690 2691 @Override 2692 public boolean equalsShallow(Base other) { 2693 if (!super.equalsShallow(other)) 2694 return false; 2695 if (!(other instanceof Bundle)) 2696 return false; 2697 Bundle o = (Bundle) other; 2698 return compareValues(type, o.type, true) && compareValues(total, o.total, true); 2699 } 2700 2701 public boolean isEmpty() { 2702 return super.isEmpty() && (type == null || type.isEmpty()) && (total == null || total.isEmpty()) 2703 && (link == null || link.isEmpty()) && (entry == null || entry.isEmpty()) 2704 && (signature == null || signature.isEmpty()); 2705 } 2706 2707 @Override 2708 public ResourceType getResourceType() { 2709 return ResourceType.Bundle; 2710 } 2711 2712 @SearchParamDefinition(name = "composition", path = "Bundle.entry.resource.item(0)", description = "The first resource in the bundle, if the bundle type is \"document\" - this is a composition, and this parameter provides access to searches its contents", type = "reference") 2713 public static final String SP_COMPOSITION = "composition"; 2714 @SearchParamDefinition(name = "type", path = "Bundle.type", description = "document | message | transaction | transaction-response | batch | batch-response | history | searchset | collection", type = "token") 2715 public static final String SP_TYPE = "type"; 2716 @SearchParamDefinition(name = "message", path = "Bundle.entry.resource.item(0)", description = "The first resource in the bundle, if the bundle type is \"message\" - this is a message header, and this parameter provides access to search its contents", type = "reference") 2717 public static final String SP_MESSAGE = "message"; 2718 2719}