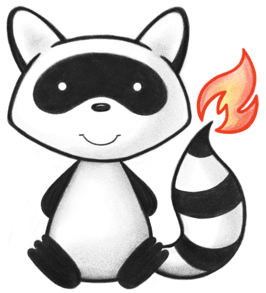
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * Describes the intention of how one or more practitioners intend to deliver 048 * care for a particular patient, group or community for a period of time, 049 * possibly limited to care for a specific condition or set of conditions. 050 */ 051@ResourceDef(name = "CarePlan", profile = "http://hl7.org/fhir/Profile/CarePlan") 052public class CarePlan extends DomainResource { 053 054 public enum CarePlanStatus { 055 /** 056 * The plan has been suggested but no commitment to it has yet been made. 057 */ 058 PROPOSED, 059 /** 060 * The plan is in development or awaiting use but is not yet intended to be 061 * acted upon. 062 */ 063 DRAFT, 064 /** 065 * The plan is intended to be followed and used as part of patient care. 066 */ 067 ACTIVE, 068 /** 069 * The plan is no longer in use and is not expected to be followed or used in 070 * patient care. 071 */ 072 COMPLETED, 073 /** 074 * The plan has been terminated prior to reaching completion (though it may have 075 * been replaced by a new plan). 076 */ 077 CANCELLED, 078 /** 079 * added to help the parsers 080 */ 081 NULL; 082 083 public static CarePlanStatus fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("proposed".equals(codeString)) 087 return PROPOSED; 088 if ("draft".equals(codeString)) 089 return DRAFT; 090 if ("active".equals(codeString)) 091 return ACTIVE; 092 if ("completed".equals(codeString)) 093 return COMPLETED; 094 if ("cancelled".equals(codeString)) 095 return CANCELLED; 096 throw new FHIRException("Unknown CarePlanStatus code '" + codeString + "'"); 097 } 098 099 public String toCode() { 100 switch (this) { 101 case PROPOSED: 102 return "proposed"; 103 case DRAFT: 104 return "draft"; 105 case ACTIVE: 106 return "active"; 107 case COMPLETED: 108 return "completed"; 109 case CANCELLED: 110 return "cancelled"; 111 case NULL: 112 return null; 113 default: 114 return "?"; 115 } 116 } 117 118 public String getSystem() { 119 switch (this) { 120 case PROPOSED: 121 return "http://hl7.org/fhir/care-plan-status"; 122 case DRAFT: 123 return "http://hl7.org/fhir/care-plan-status"; 124 case ACTIVE: 125 return "http://hl7.org/fhir/care-plan-status"; 126 case COMPLETED: 127 return "http://hl7.org/fhir/care-plan-status"; 128 case CANCELLED: 129 return "http://hl7.org/fhir/care-plan-status"; 130 case NULL: 131 return null; 132 default: 133 return "?"; 134 } 135 } 136 137 public String getDefinition() { 138 switch (this) { 139 case PROPOSED: 140 return "The plan has been suggested but no commitment to it has yet been made."; 141 case DRAFT: 142 return "The plan is in development or awaiting use but is not yet intended to be acted upon."; 143 case ACTIVE: 144 return "The plan is intended to be followed and used as part of patient care."; 145 case COMPLETED: 146 return "The plan is no longer in use and is not expected to be followed or used in patient care."; 147 case CANCELLED: 148 return "The plan has been terminated prior to reaching completion (though it may have been replaced by a new plan)."; 149 case NULL: 150 return null; 151 default: 152 return "?"; 153 } 154 } 155 156 public String getDisplay() { 157 switch (this) { 158 case PROPOSED: 159 return "Proposed"; 160 case DRAFT: 161 return "Pending"; 162 case ACTIVE: 163 return "Active"; 164 case COMPLETED: 165 return "Completed"; 166 case CANCELLED: 167 return "Cancelled"; 168 case NULL: 169 return null; 170 default: 171 return "?"; 172 } 173 } 174 } 175 176 public static class CarePlanStatusEnumFactory implements EnumFactory<CarePlanStatus> { 177 public CarePlanStatus fromCode(String codeString) throws IllegalArgumentException { 178 if (codeString == null || "".equals(codeString)) 179 if (codeString == null || "".equals(codeString)) 180 return null; 181 if ("proposed".equals(codeString)) 182 return CarePlanStatus.PROPOSED; 183 if ("draft".equals(codeString)) 184 return CarePlanStatus.DRAFT; 185 if ("active".equals(codeString)) 186 return CarePlanStatus.ACTIVE; 187 if ("completed".equals(codeString)) 188 return CarePlanStatus.COMPLETED; 189 if ("cancelled".equals(codeString)) 190 return CarePlanStatus.CANCELLED; 191 throw new IllegalArgumentException("Unknown CarePlanStatus code '" + codeString + "'"); 192 } 193 194 public Enumeration<CarePlanStatus> fromType(Base code) throws FHIRException { 195 if (code == null || code.isEmpty()) 196 return null; 197 String codeString = ((PrimitiveType) code).asStringValue(); 198 if (codeString == null || "".equals(codeString)) 199 return null; 200 if ("proposed".equals(codeString)) 201 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.PROPOSED); 202 if ("draft".equals(codeString)) 203 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.DRAFT); 204 if ("active".equals(codeString)) 205 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.ACTIVE); 206 if ("completed".equals(codeString)) 207 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.COMPLETED); 208 if ("cancelled".equals(codeString)) 209 return new Enumeration<CarePlanStatus>(this, CarePlanStatus.CANCELLED); 210 throw new FHIRException("Unknown CarePlanStatus code '" + codeString + "'"); 211 } 212 213 public String toCode(CarePlanStatus code) 214 { 215 if (code == CarePlanStatus.NULL) 216 return null; 217 if (code == CarePlanStatus.PROPOSED) 218 return "proposed"; 219 if (code == CarePlanStatus.DRAFT) 220 return "draft"; 221 if (code == CarePlanStatus.ACTIVE) 222 return "active"; 223 if (code == CarePlanStatus.COMPLETED) 224 return "completed"; 225 if (code == CarePlanStatus.CANCELLED) 226 return "cancelled"; 227 return "?"; 228 } 229 } 230 231 public enum CarePlanRelationship { 232 /** 233 * The referenced plan is considered to be part of this plan. 234 */ 235 INCLUDES, 236 /** 237 * This plan takes the places of the referenced plan. 238 */ 239 REPLACES, 240 /** 241 * This plan provides details about how to perform activities defined at a 242 * higher level by the referenced plan. 243 */ 244 FULFILLS, 245 /** 246 * added to help the parsers 247 */ 248 NULL; 249 250 public static CarePlanRelationship fromCode(String codeString) throws FHIRException { 251 if (codeString == null || "".equals(codeString)) 252 return null; 253 if ("includes".equals(codeString)) 254 return INCLUDES; 255 if ("replaces".equals(codeString)) 256 return REPLACES; 257 if ("fulfills".equals(codeString)) 258 return FULFILLS; 259 throw new FHIRException("Unknown CarePlanRelationship code '" + codeString + "'"); 260 } 261 262 public String toCode() { 263 switch (this) { 264 case INCLUDES: 265 return "includes"; 266 case REPLACES: 267 return "replaces"; 268 case FULFILLS: 269 return "fulfills"; 270 case NULL: 271 return null; 272 default: 273 return "?"; 274 } 275 } 276 277 public String getSystem() { 278 switch (this) { 279 case INCLUDES: 280 return "http://hl7.org/fhir/care-plan-relationship"; 281 case REPLACES: 282 return "http://hl7.org/fhir/care-plan-relationship"; 283 case FULFILLS: 284 return "http://hl7.org/fhir/care-plan-relationship"; 285 case NULL: 286 return null; 287 default: 288 return "?"; 289 } 290 } 291 292 public String getDefinition() { 293 switch (this) { 294 case INCLUDES: 295 return "The referenced plan is considered to be part of this plan."; 296 case REPLACES: 297 return "This plan takes the places of the referenced plan."; 298 case FULFILLS: 299 return "This plan provides details about how to perform activities defined at a higher level by the referenced plan."; 300 case NULL: 301 return null; 302 default: 303 return "?"; 304 } 305 } 306 307 public String getDisplay() { 308 switch (this) { 309 case INCLUDES: 310 return "Includes"; 311 case REPLACES: 312 return "Replaces"; 313 case FULFILLS: 314 return "Fulfills"; 315 case NULL: 316 return null; 317 default: 318 return "?"; 319 } 320 } 321 } 322 323 public static class CarePlanRelationshipEnumFactory implements EnumFactory<CarePlanRelationship> { 324 public CarePlanRelationship fromCode(String codeString) throws IllegalArgumentException { 325 if (codeString == null || "".equals(codeString)) 326 if (codeString == null || "".equals(codeString)) 327 return null; 328 if ("includes".equals(codeString)) 329 return CarePlanRelationship.INCLUDES; 330 if ("replaces".equals(codeString)) 331 return CarePlanRelationship.REPLACES; 332 if ("fulfills".equals(codeString)) 333 return CarePlanRelationship.FULFILLS; 334 throw new IllegalArgumentException("Unknown CarePlanRelationship code '" + codeString + "'"); 335 } 336 337 public Enumeration<CarePlanRelationship> fromType(Base code) throws FHIRException { 338 if (code == null || code.isEmpty()) 339 return null; 340 String codeString = ((PrimitiveType) code).asStringValue(); 341 if (codeString == null || "".equals(codeString)) 342 return null; 343 if ("includes".equals(codeString)) 344 return new Enumeration<CarePlanRelationship>(this, CarePlanRelationship.INCLUDES); 345 if ("replaces".equals(codeString)) 346 return new Enumeration<CarePlanRelationship>(this, CarePlanRelationship.REPLACES); 347 if ("fulfills".equals(codeString)) 348 return new Enumeration<CarePlanRelationship>(this, CarePlanRelationship.FULFILLS); 349 throw new FHIRException("Unknown CarePlanRelationship code '" + codeString + "'"); 350 } 351 352 public String toCode(CarePlanRelationship code) 353 { 354 if (code == CarePlanRelationship.NULL) 355 return null; 356 if (code == CarePlanRelationship.INCLUDES) 357 return "includes"; 358 if (code == CarePlanRelationship.REPLACES) 359 return "replaces"; 360 if (code == CarePlanRelationship.FULFILLS) 361 return "fulfills"; 362 return "?"; 363 } 364 } 365 366 public enum CarePlanActivityStatus { 367 /** 368 * Activity is planned but no action has yet been taken. 369 */ 370 NOTSTARTED, 371 /** 372 * Appointment or other booking has occurred but activity has not yet begun. 373 */ 374 SCHEDULED, 375 /** 376 * Activity has been started but is not yet complete. 377 */ 378 INPROGRESS, 379 /** 380 * Activity was started but has temporarily ceased with an expectation of 381 * resumption at a future time. 382 */ 383 ONHOLD, 384 /** 385 * The activities have been completed (more or less) as planned. 386 */ 387 COMPLETED, 388 /** 389 * The activities have been ended prior to completion (perhaps even before they 390 * were started). 391 */ 392 CANCELLED, 393 /** 394 * added to help the parsers 395 */ 396 NULL; 397 398 public static CarePlanActivityStatus fromCode(String codeString) throws FHIRException { 399 if (codeString == null || "".equals(codeString)) 400 return null; 401 if ("not-started".equals(codeString)) 402 return NOTSTARTED; 403 if ("scheduled".equals(codeString)) 404 return SCHEDULED; 405 if ("in-progress".equals(codeString)) 406 return INPROGRESS; 407 if ("on-hold".equals(codeString)) 408 return ONHOLD; 409 if ("completed".equals(codeString)) 410 return COMPLETED; 411 if ("cancelled".equals(codeString)) 412 return CANCELLED; 413 throw new FHIRException("Unknown CarePlanActivityStatus code '" + codeString + "'"); 414 } 415 416 public String toCode() { 417 switch (this) { 418 case NOTSTARTED: 419 return "not-started"; 420 case SCHEDULED: 421 return "scheduled"; 422 case INPROGRESS: 423 return "in-progress"; 424 case ONHOLD: 425 return "on-hold"; 426 case COMPLETED: 427 return "completed"; 428 case CANCELLED: 429 return "cancelled"; 430 case NULL: 431 return null; 432 default: 433 return "?"; 434 } 435 } 436 437 public String getSystem() { 438 switch (this) { 439 case NOTSTARTED: 440 return "http://hl7.org/fhir/care-plan-activity-status"; 441 case SCHEDULED: 442 return "http://hl7.org/fhir/care-plan-activity-status"; 443 case INPROGRESS: 444 return "http://hl7.org/fhir/care-plan-activity-status"; 445 case ONHOLD: 446 return "http://hl7.org/fhir/care-plan-activity-status"; 447 case COMPLETED: 448 return "http://hl7.org/fhir/care-plan-activity-status"; 449 case CANCELLED: 450 return "http://hl7.org/fhir/care-plan-activity-status"; 451 case NULL: 452 return null; 453 default: 454 return "?"; 455 } 456 } 457 458 public String getDefinition() { 459 switch (this) { 460 case NOTSTARTED: 461 return "Activity is planned but no action has yet been taken."; 462 case SCHEDULED: 463 return "Appointment or other booking has occurred but activity has not yet begun."; 464 case INPROGRESS: 465 return "Activity has been started but is not yet complete."; 466 case ONHOLD: 467 return "Activity was started but has temporarily ceased with an expectation of resumption at a future time."; 468 case COMPLETED: 469 return "The activities have been completed (more or less) as planned."; 470 case CANCELLED: 471 return "The activities have been ended prior to completion (perhaps even before they were started)."; 472 case NULL: 473 return null; 474 default: 475 return "?"; 476 } 477 } 478 479 public String getDisplay() { 480 switch (this) { 481 case NOTSTARTED: 482 return "Not Started"; 483 case SCHEDULED: 484 return "Scheduled"; 485 case INPROGRESS: 486 return "In Progress"; 487 case ONHOLD: 488 return "On Hold"; 489 case COMPLETED: 490 return "Completed"; 491 case CANCELLED: 492 return "Cancelled"; 493 case NULL: 494 return null; 495 default: 496 return "?"; 497 } 498 } 499 } 500 501 public static class CarePlanActivityStatusEnumFactory implements EnumFactory<CarePlanActivityStatus> { 502 public CarePlanActivityStatus fromCode(String codeString) throws IllegalArgumentException { 503 if (codeString == null || "".equals(codeString)) 504 if (codeString == null || "".equals(codeString)) 505 return null; 506 if ("not-started".equals(codeString)) 507 return CarePlanActivityStatus.NOTSTARTED; 508 if ("scheduled".equals(codeString)) 509 return CarePlanActivityStatus.SCHEDULED; 510 if ("in-progress".equals(codeString)) 511 return CarePlanActivityStatus.INPROGRESS; 512 if ("on-hold".equals(codeString)) 513 return CarePlanActivityStatus.ONHOLD; 514 if ("completed".equals(codeString)) 515 return CarePlanActivityStatus.COMPLETED; 516 if ("cancelled".equals(codeString)) 517 return CarePlanActivityStatus.CANCELLED; 518 throw new IllegalArgumentException("Unknown CarePlanActivityStatus code '" + codeString + "'"); 519 } 520 521 public Enumeration<CarePlanActivityStatus> fromType(Base code) throws FHIRException { 522 if (code == null || code.isEmpty()) 523 return null; 524 String codeString = ((PrimitiveType) code).asStringValue(); 525 if (codeString == null || "".equals(codeString)) 526 return null; 527 if ("not-started".equals(codeString)) 528 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.NOTSTARTED); 529 if ("scheduled".equals(codeString)) 530 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.SCHEDULED); 531 if ("in-progress".equals(codeString)) 532 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.INPROGRESS); 533 if ("on-hold".equals(codeString)) 534 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.ONHOLD); 535 if ("completed".equals(codeString)) 536 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.COMPLETED); 537 if ("cancelled".equals(codeString)) 538 return new Enumeration<CarePlanActivityStatus>(this, CarePlanActivityStatus.CANCELLED); 539 throw new FHIRException("Unknown CarePlanActivityStatus code '" + codeString + "'"); 540 } 541 542 public String toCode(CarePlanActivityStatus code) 543 { 544 if (code == CarePlanActivityStatus.NULL) 545 return null; 546 if (code == CarePlanActivityStatus.NOTSTARTED) 547 return "not-started"; 548 if (code == CarePlanActivityStatus.SCHEDULED) 549 return "scheduled"; 550 if (code == CarePlanActivityStatus.INPROGRESS) 551 return "in-progress"; 552 if (code == CarePlanActivityStatus.ONHOLD) 553 return "on-hold"; 554 if (code == CarePlanActivityStatus.COMPLETED) 555 return "completed"; 556 if (code == CarePlanActivityStatus.CANCELLED) 557 return "cancelled"; 558 return "?"; 559 } 560 } 561 562 @Block() 563 public static class CarePlanRelatedPlanComponent extends BackboneElement implements IBaseBackboneElement { 564 /** 565 * Identifies the type of relationship this plan has to the target plan. 566 */ 567 @Child(name = "code", type = { CodeType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 568 @Description(shortDefinition = "includes | replaces | fulfills", formalDefinition = "Identifies the type of relationship this plan has to the target plan.") 569 protected Enumeration<CarePlanRelationship> code; 570 571 /** 572 * A reference to the plan to which a relationship is asserted. 573 */ 574 @Child(name = "plan", type = { CarePlan.class }, order = 2, min = 1, max = 1, modifier = false, summary = false) 575 @Description(shortDefinition = "Plan relationship exists with", formalDefinition = "A reference to the plan to which a relationship is asserted.") 576 protected Reference plan; 577 578 /** 579 * The actual object that is the target of the reference (A reference to the 580 * plan to which a relationship is asserted.) 581 */ 582 protected CarePlan planTarget; 583 584 private static final long serialVersionUID = 1875598050L; 585 586 /* 587 * Constructor 588 */ 589 public CarePlanRelatedPlanComponent() { 590 super(); 591 } 592 593 /* 594 * Constructor 595 */ 596 public CarePlanRelatedPlanComponent(Reference plan) { 597 super(); 598 this.plan = plan; 599 } 600 601 /** 602 * @return {@link #code} (Identifies the type of relationship this plan has to 603 * the target plan.). This is the underlying object with id, value and 604 * extensions. The accessor "getCode" gives direct access to the value 605 */ 606 public Enumeration<CarePlanRelationship> getCodeElement() { 607 if (this.code == null) 608 if (Configuration.errorOnAutoCreate()) 609 throw new Error("Attempt to auto-create CarePlanRelatedPlanComponent.code"); 610 else if (Configuration.doAutoCreate()) 611 this.code = new Enumeration<CarePlanRelationship>(new CarePlanRelationshipEnumFactory()); // bb 612 return this.code; 613 } 614 615 public boolean hasCodeElement() { 616 return this.code != null && !this.code.isEmpty(); 617 } 618 619 public boolean hasCode() { 620 return this.code != null && !this.code.isEmpty(); 621 } 622 623 /** 624 * @param value {@link #code} (Identifies the type of relationship this plan has 625 * to the target plan.). This is the underlying object with id, 626 * value and extensions. The accessor "getCode" gives direct access 627 * to the value 628 */ 629 public CarePlanRelatedPlanComponent setCodeElement(Enumeration<CarePlanRelationship> value) { 630 this.code = value; 631 return this; 632 } 633 634 /** 635 * @return Identifies the type of relationship this plan has to the target plan. 636 */ 637 public CarePlanRelationship getCode() { 638 return this.code == null ? null : this.code.getValue(); 639 } 640 641 /** 642 * @param value Identifies the type of relationship this plan has to the target 643 * plan. 644 */ 645 public CarePlanRelatedPlanComponent setCode(CarePlanRelationship value) { 646 if (value == null) 647 this.code = null; 648 else { 649 if (this.code == null) 650 this.code = new Enumeration<CarePlanRelationship>(new CarePlanRelationshipEnumFactory()); 651 this.code.setValue(value); 652 } 653 return this; 654 } 655 656 /** 657 * @return {@link #plan} (A reference to the plan to which a relationship is 658 * asserted.) 659 */ 660 public Reference getPlan() { 661 if (this.plan == null) 662 if (Configuration.errorOnAutoCreate()) 663 throw new Error("Attempt to auto-create CarePlanRelatedPlanComponent.plan"); 664 else if (Configuration.doAutoCreate()) 665 this.plan = new Reference(); // cc 666 return this.plan; 667 } 668 669 public boolean hasPlan() { 670 return this.plan != null && !this.plan.isEmpty(); 671 } 672 673 /** 674 * @param value {@link #plan} (A reference to the plan to which a relationship 675 * is asserted.) 676 */ 677 public CarePlanRelatedPlanComponent setPlan(Reference value) { 678 this.plan = value; 679 return this; 680 } 681 682 /** 683 * @return {@link #plan} The actual object that is the target of the reference. 684 * The reference library doesn't populate this, but you can use it to 685 * hold the resource if you resolve it. (A reference to the plan to 686 * which a relationship is asserted.) 687 */ 688 public CarePlan getPlanTarget() { 689 if (this.planTarget == null) 690 if (Configuration.errorOnAutoCreate()) 691 throw new Error("Attempt to auto-create CarePlanRelatedPlanComponent.plan"); 692 else if (Configuration.doAutoCreate()) 693 this.planTarget = new CarePlan(); // aa 694 return this.planTarget; 695 } 696 697 /** 698 * @param value {@link #plan} The actual object that is the target of the 699 * reference. The reference library doesn't use these, but you can 700 * use it to hold the resource if you resolve it. (A reference to 701 * the plan to which a relationship is asserted.) 702 */ 703 public CarePlanRelatedPlanComponent setPlanTarget(CarePlan value) { 704 this.planTarget = value; 705 return this; 706 } 707 708 protected void listChildren(List<Property> childrenList) { 709 super.listChildren(childrenList); 710 childrenList 711 .add(new Property("code", "code", "Identifies the type of relationship this plan has to the target plan.", 0, 712 java.lang.Integer.MAX_VALUE, code)); 713 childrenList.add(new Property("plan", "Reference(CarePlan)", 714 "A reference to the plan to which a relationship is asserted.", 0, java.lang.Integer.MAX_VALUE, plan)); 715 } 716 717 @Override 718 public void setProperty(String name, Base value) throws FHIRException { 719 if (name.equals("code")) 720 this.code = new CarePlanRelationshipEnumFactory().fromType(value); // Enumeration<CarePlanRelationship> 721 else if (name.equals("plan")) 722 this.plan = castToReference(value); // Reference 723 else 724 super.setProperty(name, value); 725 } 726 727 @Override 728 public Base addChild(String name) throws FHIRException { 729 if (name.equals("code")) { 730 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.code"); 731 } else if (name.equals("plan")) { 732 this.plan = new Reference(); 733 return this.plan; 734 } else 735 return super.addChild(name); 736 } 737 738 public CarePlanRelatedPlanComponent copy() { 739 CarePlanRelatedPlanComponent dst = new CarePlanRelatedPlanComponent(); 740 copyValues(dst); 741 dst.code = code == null ? null : code.copy(); 742 dst.plan = plan == null ? null : plan.copy(); 743 return dst; 744 } 745 746 @Override 747 public boolean equalsDeep(Base other) { 748 if (!super.equalsDeep(other)) 749 return false; 750 if (!(other instanceof CarePlanRelatedPlanComponent)) 751 return false; 752 CarePlanRelatedPlanComponent o = (CarePlanRelatedPlanComponent) other; 753 return compareDeep(code, o.code, true) && compareDeep(plan, o.plan, true); 754 } 755 756 @Override 757 public boolean equalsShallow(Base other) { 758 if (!super.equalsShallow(other)) 759 return false; 760 if (!(other instanceof CarePlanRelatedPlanComponent)) 761 return false; 762 CarePlanRelatedPlanComponent o = (CarePlanRelatedPlanComponent) other; 763 return compareValues(code, o.code, true); 764 } 765 766 public boolean isEmpty() { 767 return super.isEmpty() && (code == null || code.isEmpty()) && (plan == null || plan.isEmpty()); 768 } 769 770 public String fhirType() { 771 return "CarePlan.relatedPlan"; 772 773 } 774 775 } 776 777 @Block() 778 public static class CarePlanParticipantComponent extends BackboneElement implements IBaseBackboneElement { 779 /** 780 * Indicates specific responsibility of an individual within the care plan; e.g. 781 * "Primary physician", "Team coordinator", "Caregiver", etc. 782 */ 783 @Child(name = "role", type = { 784 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 785 @Description(shortDefinition = "Type of involvement", formalDefinition = "Indicates specific responsibility of an individual within the care plan; e.g. \"Primary physician\", \"Team coordinator\", \"Caregiver\", etc.") 786 protected CodeableConcept role; 787 788 /** 789 * The specific person or organization who is participating/expected to 790 * participate in the care plan. 791 */ 792 @Child(name = "member", type = { Practitioner.class, RelatedPerson.class, Patient.class, 793 Organization.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 794 @Description(shortDefinition = "Who is involved", formalDefinition = "The specific person or organization who is participating/expected to participate in the care plan.") 795 protected Reference member; 796 797 /** 798 * The actual object that is the target of the reference (The specific person or 799 * organization who is participating/expected to participate in the care plan.) 800 */ 801 protected Resource memberTarget; 802 803 private static final long serialVersionUID = -466811117L; 804 805 /* 806 * Constructor 807 */ 808 public CarePlanParticipantComponent() { 809 super(); 810 } 811 812 /** 813 * @return {@link #role} (Indicates specific responsibility of an individual 814 * within the care plan; e.g. "Primary physician", "Team coordinator", 815 * "Caregiver", etc.) 816 */ 817 public CodeableConcept getRole() { 818 if (this.role == null) 819 if (Configuration.errorOnAutoCreate()) 820 throw new Error("Attempt to auto-create CarePlanParticipantComponent.role"); 821 else if (Configuration.doAutoCreate()) 822 this.role = new CodeableConcept(); // cc 823 return this.role; 824 } 825 826 public boolean hasRole() { 827 return this.role != null && !this.role.isEmpty(); 828 } 829 830 /** 831 * @param value {@link #role} (Indicates specific responsibility of an 832 * individual within the care plan; e.g. "Primary physician", "Team 833 * coordinator", "Caregiver", etc.) 834 */ 835 public CarePlanParticipantComponent setRole(CodeableConcept value) { 836 this.role = value; 837 return this; 838 } 839 840 /** 841 * @return {@link #member} (The specific person or organization who is 842 * participating/expected to participate in the care plan.) 843 */ 844 public Reference getMember() { 845 if (this.member == null) 846 if (Configuration.errorOnAutoCreate()) 847 throw new Error("Attempt to auto-create CarePlanParticipantComponent.member"); 848 else if (Configuration.doAutoCreate()) 849 this.member = new Reference(); // cc 850 return this.member; 851 } 852 853 public boolean hasMember() { 854 return this.member != null && !this.member.isEmpty(); 855 } 856 857 /** 858 * @param value {@link #member} (The specific person or organization who is 859 * participating/expected to participate in the care plan.) 860 */ 861 public CarePlanParticipantComponent setMember(Reference value) { 862 this.member = value; 863 return this; 864 } 865 866 /** 867 * @return {@link #member} The actual object that is the target of the 868 * reference. The reference library doesn't populate this, but you can 869 * use it to hold the resource if you resolve it. (The specific person 870 * or organization who is participating/expected to participate in the 871 * care plan.) 872 */ 873 public Resource getMemberTarget() { 874 return this.memberTarget; 875 } 876 877 /** 878 * @param value {@link #member} The actual object that is the target of the 879 * reference. The reference library doesn't use these, but you can 880 * use it to hold the resource if you resolve it. (The specific 881 * person or organization who is participating/expected to 882 * participate in the care plan.) 883 */ 884 public CarePlanParticipantComponent setMemberTarget(Resource value) { 885 this.memberTarget = value; 886 return this; 887 } 888 889 protected void listChildren(List<Property> childrenList) { 890 super.listChildren(childrenList); 891 childrenList.add(new Property("role", "CodeableConcept", 892 "Indicates specific responsibility of an individual within the care plan; e.g. \"Primary physician\", \"Team coordinator\", \"Caregiver\", etc.", 893 0, java.lang.Integer.MAX_VALUE, role)); 894 childrenList.add(new Property("member", "Reference(Practitioner|RelatedPerson|Patient|Organization)", 895 "The specific person or organization who is participating/expected to participate in the care plan.", 0, 896 java.lang.Integer.MAX_VALUE, member)); 897 } 898 899 @Override 900 public void setProperty(String name, Base value) throws FHIRException { 901 if (name.equals("role")) 902 this.role = castToCodeableConcept(value); // CodeableConcept 903 else if (name.equals("member")) 904 this.member = castToReference(value); // Reference 905 else 906 super.setProperty(name, value); 907 } 908 909 @Override 910 public Base addChild(String name) throws FHIRException { 911 if (name.equals("role")) { 912 this.role = new CodeableConcept(); 913 return this.role; 914 } else if (name.equals("member")) { 915 this.member = new Reference(); 916 return this.member; 917 } else 918 return super.addChild(name); 919 } 920 921 public CarePlanParticipantComponent copy() { 922 CarePlanParticipantComponent dst = new CarePlanParticipantComponent(); 923 copyValues(dst); 924 dst.role = role == null ? null : role.copy(); 925 dst.member = member == null ? null : member.copy(); 926 return dst; 927 } 928 929 @Override 930 public boolean equalsDeep(Base other) { 931 if (!super.equalsDeep(other)) 932 return false; 933 if (!(other instanceof CarePlanParticipantComponent)) 934 return false; 935 CarePlanParticipantComponent o = (CarePlanParticipantComponent) other; 936 return compareDeep(role, o.role, true) && compareDeep(member, o.member, true); 937 } 938 939 @Override 940 public boolean equalsShallow(Base other) { 941 if (!super.equalsShallow(other)) 942 return false; 943 if (!(other instanceof CarePlanParticipantComponent)) 944 return false; 945 CarePlanParticipantComponent o = (CarePlanParticipantComponent) other; 946 return true; 947 } 948 949 public boolean isEmpty() { 950 return super.isEmpty() && (role == null || role.isEmpty()) && (member == null || member.isEmpty()); 951 } 952 953 public String fhirType() { 954 return "CarePlan.participant"; 955 956 } 957 958 } 959 960 @Block() 961 public static class CarePlanActivityComponent extends BackboneElement implements IBaseBackboneElement { 962 /** 963 * Resources that describe follow-on actions resulting from the plan, such as 964 * drug prescriptions, encounter records, appointments, etc. 965 */ 966 @Child(name = "actionResulting", type = {}, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 967 @Description(shortDefinition = "Appointments, orders, etc.", formalDefinition = "Resources that describe follow-on actions resulting from the plan, such as drug prescriptions, encounter records, appointments, etc.") 968 protected List<Reference> actionResulting; 969 /** 970 * The actual objects that are the target of the reference (Resources that 971 * describe follow-on actions resulting from the plan, such as drug 972 * prescriptions, encounter records, appointments, etc.) 973 */ 974 protected List<Resource> actionResultingTarget; 975 976 /** 977 * Notes about the adherence/status/progress of the activity. 978 */ 979 @Child(name = "progress", type = { 980 Annotation.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 981 @Description(shortDefinition = "Comments about the activity status/progress", formalDefinition = "Notes about the adherence/status/progress of the activity.") 982 protected List<Annotation> progress; 983 984 /** 985 * The details of the proposed activity represented in a specific resource. 986 */ 987 @Child(name = "reference", type = { Appointment.class, CommunicationRequest.class, DeviceUseRequest.class, 988 DiagnosticOrder.class, MedicationOrder.class, NutritionOrder.class, Order.class, ProcedureRequest.class, 989 ProcessRequest.class, ReferralRequest.class, SupplyRequest.class, 990 VisionPrescription.class }, order = 3, min = 0, max = 1, modifier = false, summary = false) 991 @Description(shortDefinition = "Activity details defined in specific resource", formalDefinition = "The details of the proposed activity represented in a specific resource.") 992 protected Reference reference; 993 994 /** 995 * The actual object that is the target of the reference (The details of the 996 * proposed activity represented in a specific resource.) 997 */ 998 protected Resource referenceTarget; 999 1000 /** 1001 * A simple summary of a planned activity suitable for a general care plan 1002 * system (e.g. form driven) that doesn't know about specific resources such as 1003 * procedure etc. 1004 */ 1005 @Child(name = "detail", type = {}, order = 4, min = 0, max = 1, modifier = false, summary = false) 1006 @Description(shortDefinition = "In-line definition of activity", formalDefinition = "A simple summary of a planned activity suitable for a general care plan system (e.g. form driven) that doesn't know about specific resources such as procedure etc.") 1007 protected CarePlanActivityDetailComponent detail; 1008 1009 private static final long serialVersionUID = 40181608L; 1010 1011 /* 1012 * Constructor 1013 */ 1014 public CarePlanActivityComponent() { 1015 super(); 1016 } 1017 1018 /** 1019 * @return {@link #actionResulting} (Resources that describe follow-on actions 1020 * resulting from the plan, such as drug prescriptions, encounter 1021 * records, appointments, etc.) 1022 */ 1023 public List<Reference> getActionResulting() { 1024 if (this.actionResulting == null) 1025 this.actionResulting = new ArrayList<Reference>(); 1026 return this.actionResulting; 1027 } 1028 1029 public boolean hasActionResulting() { 1030 if (this.actionResulting == null) 1031 return false; 1032 for (Reference item : this.actionResulting) 1033 if (!item.isEmpty()) 1034 return true; 1035 return false; 1036 } 1037 1038 /** 1039 * @return {@link #actionResulting} (Resources that describe follow-on actions 1040 * resulting from the plan, such as drug prescriptions, encounter 1041 * records, appointments, etc.) 1042 */ 1043 // syntactic sugar 1044 public Reference addActionResulting() { // 3 1045 Reference t = new Reference(); 1046 if (this.actionResulting == null) 1047 this.actionResulting = new ArrayList<Reference>(); 1048 this.actionResulting.add(t); 1049 return t; 1050 } 1051 1052 // syntactic sugar 1053 public CarePlanActivityComponent addActionResulting(Reference t) { // 3 1054 if (t == null) 1055 return this; 1056 if (this.actionResulting == null) 1057 this.actionResulting = new ArrayList<Reference>(); 1058 this.actionResulting.add(t); 1059 return this; 1060 } 1061 1062 /** 1063 * @return {@link #actionResulting} (The actual objects that are the target of 1064 * the reference. The reference library doesn't populate this, but you 1065 * can use this to hold the resources if you resolvethemt. Resources 1066 * that describe follow-on actions resulting from the plan, such as drug 1067 * prescriptions, encounter records, appointments, etc.) 1068 */ 1069 public List<Resource> getActionResultingTarget() { 1070 if (this.actionResultingTarget == null) 1071 this.actionResultingTarget = new ArrayList<Resource>(); 1072 return this.actionResultingTarget; 1073 } 1074 1075 /** 1076 * @return {@link #progress} (Notes about the adherence/status/progress of the 1077 * activity.) 1078 */ 1079 public List<Annotation> getProgress() { 1080 if (this.progress == null) 1081 this.progress = new ArrayList<Annotation>(); 1082 return this.progress; 1083 } 1084 1085 public boolean hasProgress() { 1086 if (this.progress == null) 1087 return false; 1088 for (Annotation item : this.progress) 1089 if (!item.isEmpty()) 1090 return true; 1091 return false; 1092 } 1093 1094 /** 1095 * @return {@link #progress} (Notes about the adherence/status/progress of the 1096 * activity.) 1097 */ 1098 // syntactic sugar 1099 public Annotation addProgress() { // 3 1100 Annotation t = new Annotation(); 1101 if (this.progress == null) 1102 this.progress = new ArrayList<Annotation>(); 1103 this.progress.add(t); 1104 return t; 1105 } 1106 1107 // syntactic sugar 1108 public CarePlanActivityComponent addProgress(Annotation t) { // 3 1109 if (t == null) 1110 return this; 1111 if (this.progress == null) 1112 this.progress = new ArrayList<Annotation>(); 1113 this.progress.add(t); 1114 return this; 1115 } 1116 1117 /** 1118 * @return {@link #reference} (The details of the proposed activity represented 1119 * in a specific resource.) 1120 */ 1121 public Reference getReference() { 1122 if (this.reference == null) 1123 if (Configuration.errorOnAutoCreate()) 1124 throw new Error("Attempt to auto-create CarePlanActivityComponent.reference"); 1125 else if (Configuration.doAutoCreate()) 1126 this.reference = new Reference(); // cc 1127 return this.reference; 1128 } 1129 1130 public boolean hasReference() { 1131 return this.reference != null && !this.reference.isEmpty(); 1132 } 1133 1134 /** 1135 * @param value {@link #reference} (The details of the proposed activity 1136 * represented in a specific resource.) 1137 */ 1138 public CarePlanActivityComponent setReference(Reference value) { 1139 this.reference = value; 1140 return this; 1141 } 1142 1143 /** 1144 * @return {@link #reference} The actual object that is the target of the 1145 * reference. The reference library doesn't populate this, but you can 1146 * use it to hold the resource if you resolve it. (The details of the 1147 * proposed activity represented in a specific resource.) 1148 */ 1149 public Resource getReferenceTarget() { 1150 return this.referenceTarget; 1151 } 1152 1153 /** 1154 * @param value {@link #reference} The actual object that is the target of the 1155 * reference. The reference library doesn't use these, but you can 1156 * use it to hold the resource if you resolve it. (The details of 1157 * the proposed activity represented in a specific resource.) 1158 */ 1159 public CarePlanActivityComponent setReferenceTarget(Resource value) { 1160 this.referenceTarget = value; 1161 return this; 1162 } 1163 1164 /** 1165 * @return {@link #detail} (A simple summary of a planned activity suitable for 1166 * a general care plan system (e.g. form driven) that doesn't know about 1167 * specific resources such as procedure etc.) 1168 */ 1169 public CarePlanActivityDetailComponent getDetail() { 1170 if (this.detail == null) 1171 if (Configuration.errorOnAutoCreate()) 1172 throw new Error("Attempt to auto-create CarePlanActivityComponent.detail"); 1173 else if (Configuration.doAutoCreate()) 1174 this.detail = new CarePlanActivityDetailComponent(); // cc 1175 return this.detail; 1176 } 1177 1178 public boolean hasDetail() { 1179 return this.detail != null && !this.detail.isEmpty(); 1180 } 1181 1182 /** 1183 * @param value {@link #detail} (A simple summary of a planned activity suitable 1184 * for a general care plan system (e.g. form driven) that doesn't 1185 * know about specific resources such as procedure etc.) 1186 */ 1187 public CarePlanActivityComponent setDetail(CarePlanActivityDetailComponent value) { 1188 this.detail = value; 1189 return this; 1190 } 1191 1192 protected void listChildren(List<Property> childrenList) { 1193 super.listChildren(childrenList); 1194 childrenList.add(new Property("actionResulting", "Reference(Any)", 1195 "Resources that describe follow-on actions resulting from the plan, such as drug prescriptions, encounter records, appointments, etc.", 1196 0, java.lang.Integer.MAX_VALUE, actionResulting)); 1197 childrenList.add(new Property("progress", "Annotation", 1198 "Notes about the adherence/status/progress of the activity.", 0, java.lang.Integer.MAX_VALUE, progress)); 1199 childrenList.add(new Property("reference", 1200 "Reference(Appointment|CommunicationRequest|DeviceUseRequest|DiagnosticOrder|MedicationOrder|NutritionOrder|Order|ProcedureRequest|ProcessRequest|ReferralRequest|SupplyRequest|VisionPrescription)", 1201 "The details of the proposed activity represented in a specific resource.", 0, java.lang.Integer.MAX_VALUE, 1202 reference)); 1203 childrenList.add(new Property("detail", "", 1204 "A simple summary of a planned activity suitable for a general care plan system (e.g. form driven) that doesn't know about specific resources such as procedure etc.", 1205 0, java.lang.Integer.MAX_VALUE, detail)); 1206 } 1207 1208 @Override 1209 public void setProperty(String name, Base value) throws FHIRException { 1210 if (name.equals("actionResulting")) 1211 this.getActionResulting().add(castToReference(value)); 1212 else if (name.equals("progress")) 1213 this.getProgress().add(castToAnnotation(value)); 1214 else if (name.equals("reference")) 1215 this.reference = castToReference(value); // Reference 1216 else if (name.equals("detail")) 1217 this.detail = (CarePlanActivityDetailComponent) value; // CarePlanActivityDetailComponent 1218 else 1219 super.setProperty(name, value); 1220 } 1221 1222 @Override 1223 public Base addChild(String name) throws FHIRException { 1224 if (name.equals("actionResulting")) { 1225 return addActionResulting(); 1226 } else if (name.equals("progress")) { 1227 return addProgress(); 1228 } else if (name.equals("reference")) { 1229 this.reference = new Reference(); 1230 return this.reference; 1231 } else if (name.equals("detail")) { 1232 this.detail = new CarePlanActivityDetailComponent(); 1233 return this.detail; 1234 } else 1235 return super.addChild(name); 1236 } 1237 1238 public CarePlanActivityComponent copy() { 1239 CarePlanActivityComponent dst = new CarePlanActivityComponent(); 1240 copyValues(dst); 1241 if (actionResulting != null) { 1242 dst.actionResulting = new ArrayList<Reference>(); 1243 for (Reference i : actionResulting) 1244 dst.actionResulting.add(i.copy()); 1245 } 1246 ; 1247 if (progress != null) { 1248 dst.progress = new ArrayList<Annotation>(); 1249 for (Annotation i : progress) 1250 dst.progress.add(i.copy()); 1251 } 1252 ; 1253 dst.reference = reference == null ? null : reference.copy(); 1254 dst.detail = detail == null ? null : detail.copy(); 1255 return dst; 1256 } 1257 1258 @Override 1259 public boolean equalsDeep(Base other) { 1260 if (!super.equalsDeep(other)) 1261 return false; 1262 if (!(other instanceof CarePlanActivityComponent)) 1263 return false; 1264 CarePlanActivityComponent o = (CarePlanActivityComponent) other; 1265 return compareDeep(actionResulting, o.actionResulting, true) && compareDeep(progress, o.progress, true) 1266 && compareDeep(reference, o.reference, true) && compareDeep(detail, o.detail, true); 1267 } 1268 1269 @Override 1270 public boolean equalsShallow(Base other) { 1271 if (!super.equalsShallow(other)) 1272 return false; 1273 if (!(other instanceof CarePlanActivityComponent)) 1274 return false; 1275 CarePlanActivityComponent o = (CarePlanActivityComponent) other; 1276 return true; 1277 } 1278 1279 public boolean isEmpty() { 1280 return super.isEmpty() && (actionResulting == null || actionResulting.isEmpty()) 1281 && (progress == null || progress.isEmpty()) && (reference == null || reference.isEmpty()) 1282 && (detail == null || detail.isEmpty()); 1283 } 1284 1285 public String fhirType() { 1286 return "CarePlan.activity"; 1287 1288 } 1289 1290 } 1291 1292 @Block() 1293 public static class CarePlanActivityDetailComponent extends BackboneElement implements IBaseBackboneElement { 1294 /** 1295 * High-level categorization of the type of activity in a care plan. 1296 */ 1297 @Child(name = "category", type = { 1298 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 1299 @Description(shortDefinition = "diet | drug | encounter | observation | procedure | supply | other", formalDefinition = "High-level categorization of the type of activity in a care plan.") 1300 protected CodeableConcept category; 1301 1302 /** 1303 * Detailed description of the type of planned activity; e.g. What lab test, 1304 * what procedure, what kind of encounter. 1305 */ 1306 @Child(name = "code", type = { 1307 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 1308 @Description(shortDefinition = "Detail type of activity", formalDefinition = "Detailed description of the type of planned activity; e.g. What lab test, what procedure, what kind of encounter.") 1309 protected CodeableConcept code; 1310 1311 /** 1312 * Provides the rationale that drove the inclusion of this particular activity 1313 * as part of the plan. 1314 */ 1315 @Child(name = "reasonCode", type = { 1316 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1317 @Description(shortDefinition = "Why activity should be done", formalDefinition = "Provides the rationale that drove the inclusion of this particular activity as part of the plan.") 1318 protected List<CodeableConcept> reasonCode; 1319 1320 /** 1321 * Provides the health condition(s) that drove the inclusion of this particular 1322 * activity as part of the plan. 1323 */ 1324 @Child(name = "reasonReference", type = { 1325 Condition.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1326 @Description(shortDefinition = "Condition triggering need for activity", formalDefinition = "Provides the health condition(s) that drove the inclusion of this particular activity as part of the plan.") 1327 protected List<Reference> reasonReference; 1328 /** 1329 * The actual objects that are the target of the reference (Provides the health 1330 * condition(s) that drove the inclusion of this particular activity as part of 1331 * the plan.) 1332 */ 1333 protected List<Condition> reasonReferenceTarget; 1334 1335 /** 1336 * Internal reference that identifies the goals that this activity is intended 1337 * to contribute towards meeting. 1338 */ 1339 @Child(name = "goal", type = { 1340 Goal.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1341 @Description(shortDefinition = "Goals this activity relates to", formalDefinition = "Internal reference that identifies the goals that this activity is intended to contribute towards meeting.") 1342 protected List<Reference> goal; 1343 /** 1344 * The actual objects that are the target of the reference (Internal reference 1345 * that identifies the goals that this activity is intended to contribute 1346 * towards meeting.) 1347 */ 1348 protected List<Goal> goalTarget; 1349 1350 /** 1351 * Identifies what progress is being made for the specific activity. 1352 */ 1353 @Child(name = "status", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = true, summary = false) 1354 @Description(shortDefinition = "not-started | scheduled | in-progress | on-hold | completed | cancelled", formalDefinition = "Identifies what progress is being made for the specific activity.") 1355 protected Enumeration<CarePlanActivityStatus> status; 1356 1357 /** 1358 * Provides reason why the activity isn't yet started, is on hold, was 1359 * cancelled, etc. 1360 */ 1361 @Child(name = "statusReason", type = { 1362 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 1363 @Description(shortDefinition = "Reason for current status", formalDefinition = "Provides reason why the activity isn't yet started, is on hold, was cancelled, etc.") 1364 protected CodeableConcept statusReason; 1365 1366 /** 1367 * If true, indicates that the described activity is one that must NOT be 1368 * engaged in when following the plan. 1369 */ 1370 @Child(name = "prohibited", type = { 1371 BooleanType.class }, order = 8, min = 1, max = 1, modifier = true, summary = false) 1372 @Description(shortDefinition = "Do NOT do", formalDefinition = "If true, indicates that the described activity is one that must NOT be engaged in when following the plan.") 1373 protected BooleanType prohibited; 1374 1375 /** 1376 * The period, timing or frequency upon which the described activity is to 1377 * occur. 1378 */ 1379 @Child(name = "scheduled", type = { Timing.class, Period.class, 1380 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 1381 @Description(shortDefinition = "When activity is to occur", formalDefinition = "The period, timing or frequency upon which the described activity is to occur.") 1382 protected Type scheduled; 1383 1384 /** 1385 * Identifies the facility where the activity will occur; e.g. home, hospital, 1386 * specific clinic, etc. 1387 */ 1388 @Child(name = "location", type = { 1389 Location.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 1390 @Description(shortDefinition = "Where it should happen", formalDefinition = "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.") 1391 protected Reference location; 1392 1393 /** 1394 * The actual object that is the target of the reference (Identifies the 1395 * facility where the activity will occur; e.g. home, hospital, specific clinic, 1396 * etc.) 1397 */ 1398 protected Location locationTarget; 1399 1400 /** 1401 * Identifies who's expected to be involved in the activity. 1402 */ 1403 @Child(name = "performer", type = { Practitioner.class, Organization.class, RelatedPerson.class, 1404 Patient.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 1405 @Description(shortDefinition = "Who will be responsible?", formalDefinition = "Identifies who's expected to be involved in the activity.") 1406 protected List<Reference> performer; 1407 /** 1408 * The actual objects that are the target of the reference (Identifies who's 1409 * expected to be involved in the activity.) 1410 */ 1411 protected List<Resource> performerTarget; 1412 1413 /** 1414 * Identifies the food, drug or other product to be consumed or supplied in the 1415 * activity. 1416 */ 1417 @Child(name = "product", type = { CodeableConcept.class, Medication.class, 1418 Substance.class }, order = 12, min = 0, max = 1, modifier = false, summary = false) 1419 @Description(shortDefinition = "What is to be administered/supplied", formalDefinition = "Identifies the food, drug or other product to be consumed or supplied in the activity.") 1420 protected Type product; 1421 1422 /** 1423 * Identifies the quantity expected to be consumed in a given day. 1424 */ 1425 @Child(name = "dailyAmount", type = { 1426 SimpleQuantity.class }, order = 13, min = 0, max = 1, modifier = false, summary = false) 1427 @Description(shortDefinition = "How to consume/day?", formalDefinition = "Identifies the quantity expected to be consumed in a given day.") 1428 protected SimpleQuantity dailyAmount; 1429 1430 /** 1431 * Identifies the quantity expected to be supplied, administered or consumed by 1432 * the subject. 1433 */ 1434 @Child(name = "quantity", type = { 1435 SimpleQuantity.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 1436 @Description(shortDefinition = "How much to administer/supply/consume", formalDefinition = "Identifies the quantity expected to be supplied, administered or consumed by the subject.") 1437 protected SimpleQuantity quantity; 1438 1439 /** 1440 * This provides a textual description of constraints on the intended activity 1441 * occurrence, including relation to other activities. It may also include 1442 * objectives, pre-conditions and end-conditions. Finally, it may convey 1443 * specifics about the activity such as body site, method, route, etc. 1444 */ 1445 @Child(name = "description", type = { 1446 StringType.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 1447 @Description(shortDefinition = "Extra info describing activity to perform", formalDefinition = "This provides a textual description of constraints on the intended activity occurrence, including relation to other activities. It may also include objectives, pre-conditions and end-conditions. Finally, it may convey specifics about the activity such as body site, method, route, etc.") 1448 protected StringType description; 1449 1450 private static final long serialVersionUID = -1763965702L; 1451 1452 /* 1453 * Constructor 1454 */ 1455 public CarePlanActivityDetailComponent() { 1456 super(); 1457 } 1458 1459 /* 1460 * Constructor 1461 */ 1462 public CarePlanActivityDetailComponent(BooleanType prohibited) { 1463 super(); 1464 this.prohibited = prohibited; 1465 } 1466 1467 /** 1468 * @return {@link #category} (High-level categorization of the type of activity 1469 * in a care plan.) 1470 */ 1471 public CodeableConcept getCategory() { 1472 if (this.category == null) 1473 if (Configuration.errorOnAutoCreate()) 1474 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.category"); 1475 else if (Configuration.doAutoCreate()) 1476 this.category = new CodeableConcept(); // cc 1477 return this.category; 1478 } 1479 1480 public boolean hasCategory() { 1481 return this.category != null && !this.category.isEmpty(); 1482 } 1483 1484 /** 1485 * @param value {@link #category} (High-level categorization of the type of 1486 * activity in a care plan.) 1487 */ 1488 public CarePlanActivityDetailComponent setCategory(CodeableConcept value) { 1489 this.category = value; 1490 return this; 1491 } 1492 1493 /** 1494 * @return {@link #code} (Detailed description of the type of planned activity; 1495 * e.g. What lab test, what procedure, what kind of encounter.) 1496 */ 1497 public CodeableConcept getCode() { 1498 if (this.code == null) 1499 if (Configuration.errorOnAutoCreate()) 1500 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.code"); 1501 else if (Configuration.doAutoCreate()) 1502 this.code = new CodeableConcept(); // cc 1503 return this.code; 1504 } 1505 1506 public boolean hasCode() { 1507 return this.code != null && !this.code.isEmpty(); 1508 } 1509 1510 /** 1511 * @param value {@link #code} (Detailed description of the type of planned 1512 * activity; e.g. What lab test, what procedure, what kind of 1513 * encounter.) 1514 */ 1515 public CarePlanActivityDetailComponent setCode(CodeableConcept value) { 1516 this.code = value; 1517 return this; 1518 } 1519 1520 /** 1521 * @return {@link #reasonCode} (Provides the rationale that drove the inclusion 1522 * of this particular activity as part of the plan.) 1523 */ 1524 public List<CodeableConcept> getReasonCode() { 1525 if (this.reasonCode == null) 1526 this.reasonCode = new ArrayList<CodeableConcept>(); 1527 return this.reasonCode; 1528 } 1529 1530 public boolean hasReasonCode() { 1531 if (this.reasonCode == null) 1532 return false; 1533 for (CodeableConcept item : this.reasonCode) 1534 if (!item.isEmpty()) 1535 return true; 1536 return false; 1537 } 1538 1539 /** 1540 * @return {@link #reasonCode} (Provides the rationale that drove the inclusion 1541 * of this particular activity as part of the plan.) 1542 */ 1543 // syntactic sugar 1544 public CodeableConcept addReasonCode() { // 3 1545 CodeableConcept t = new CodeableConcept(); 1546 if (this.reasonCode == null) 1547 this.reasonCode = new ArrayList<CodeableConcept>(); 1548 this.reasonCode.add(t); 1549 return t; 1550 } 1551 1552 // syntactic sugar 1553 public CarePlanActivityDetailComponent addReasonCode(CodeableConcept t) { // 3 1554 if (t == null) 1555 return this; 1556 if (this.reasonCode == null) 1557 this.reasonCode = new ArrayList<CodeableConcept>(); 1558 this.reasonCode.add(t); 1559 return this; 1560 } 1561 1562 /** 1563 * @return {@link #reasonReference} (Provides the health condition(s) that drove 1564 * the inclusion of this particular activity as part of the plan.) 1565 */ 1566 public List<Reference> getReasonReference() { 1567 if (this.reasonReference == null) 1568 this.reasonReference = new ArrayList<Reference>(); 1569 return this.reasonReference; 1570 } 1571 1572 public boolean hasReasonReference() { 1573 if (this.reasonReference == null) 1574 return false; 1575 for (Reference item : this.reasonReference) 1576 if (!item.isEmpty()) 1577 return true; 1578 return false; 1579 } 1580 1581 /** 1582 * @return {@link #reasonReference} (Provides the health condition(s) that drove 1583 * the inclusion of this particular activity as part of the plan.) 1584 */ 1585 // syntactic sugar 1586 public Reference addReasonReference() { // 3 1587 Reference t = new Reference(); 1588 if (this.reasonReference == null) 1589 this.reasonReference = new ArrayList<Reference>(); 1590 this.reasonReference.add(t); 1591 return t; 1592 } 1593 1594 // syntactic sugar 1595 public CarePlanActivityDetailComponent addReasonReference(Reference t) { // 3 1596 if (t == null) 1597 return this; 1598 if (this.reasonReference == null) 1599 this.reasonReference = new ArrayList<Reference>(); 1600 this.reasonReference.add(t); 1601 return this; 1602 } 1603 1604 /** 1605 * @return {@link #reasonReference} (The actual objects that are the target of 1606 * the reference. The reference library doesn't populate this, but you 1607 * can use this to hold the resources if you resolvethemt. Provides the 1608 * health condition(s) that drove the inclusion of this particular 1609 * activity as part of the plan.) 1610 */ 1611 public List<Condition> getReasonReferenceTarget() { 1612 if (this.reasonReferenceTarget == null) 1613 this.reasonReferenceTarget = new ArrayList<Condition>(); 1614 return this.reasonReferenceTarget; 1615 } 1616 1617 // syntactic sugar 1618 /** 1619 * @return {@link #reasonReference} (Add an actual object that is the target of 1620 * the reference. The reference library doesn't use these, but you can 1621 * use this to hold the resources if you resolvethemt. Provides the 1622 * health condition(s) that drove the inclusion of this particular 1623 * activity as part of the plan.) 1624 */ 1625 public Condition addReasonReferenceTarget() { 1626 Condition r = new Condition(); 1627 if (this.reasonReferenceTarget == null) 1628 this.reasonReferenceTarget = new ArrayList<Condition>(); 1629 this.reasonReferenceTarget.add(r); 1630 return r; 1631 } 1632 1633 /** 1634 * @return {@link #goal} (Internal reference that identifies the goals that this 1635 * activity is intended to contribute towards meeting.) 1636 */ 1637 public List<Reference> getGoal() { 1638 if (this.goal == null) 1639 this.goal = new ArrayList<Reference>(); 1640 return this.goal; 1641 } 1642 1643 public boolean hasGoal() { 1644 if (this.goal == null) 1645 return false; 1646 for (Reference item : this.goal) 1647 if (!item.isEmpty()) 1648 return true; 1649 return false; 1650 } 1651 1652 /** 1653 * @return {@link #goal} (Internal reference that identifies the goals that this 1654 * activity is intended to contribute towards meeting.) 1655 */ 1656 // syntactic sugar 1657 public Reference addGoal() { // 3 1658 Reference t = new Reference(); 1659 if (this.goal == null) 1660 this.goal = new ArrayList<Reference>(); 1661 this.goal.add(t); 1662 return t; 1663 } 1664 1665 // syntactic sugar 1666 public CarePlanActivityDetailComponent addGoal(Reference t) { // 3 1667 if (t == null) 1668 return this; 1669 if (this.goal == null) 1670 this.goal = new ArrayList<Reference>(); 1671 this.goal.add(t); 1672 return this; 1673 } 1674 1675 /** 1676 * @return {@link #goal} (The actual objects that are the target of the 1677 * reference. The reference library doesn't populate this, but you can 1678 * use this to hold the resources if you resolvethemt. Internal 1679 * reference that identifies the goals that this activity is intended to 1680 * contribute towards meeting.) 1681 */ 1682 public List<Goal> getGoalTarget() { 1683 if (this.goalTarget == null) 1684 this.goalTarget = new ArrayList<Goal>(); 1685 return this.goalTarget; 1686 } 1687 1688 // syntactic sugar 1689 /** 1690 * @return {@link #goal} (Add an actual object that is the target of the 1691 * reference. The reference library doesn't use these, but you can use 1692 * this to hold the resources if you resolvethemt. Internal reference 1693 * that identifies the goals that this activity is intended to 1694 * contribute towards meeting.) 1695 */ 1696 public Goal addGoalTarget() { 1697 Goal r = new Goal(); 1698 if (this.goalTarget == null) 1699 this.goalTarget = new ArrayList<Goal>(); 1700 this.goalTarget.add(r); 1701 return r; 1702 } 1703 1704 /** 1705 * @return {@link #status} (Identifies what progress is being made for the 1706 * specific activity.). This is the underlying object with id, value and 1707 * extensions. The accessor "getStatus" gives direct access to the value 1708 */ 1709 public Enumeration<CarePlanActivityStatus> getStatusElement() { 1710 if (this.status == null) 1711 if (Configuration.errorOnAutoCreate()) 1712 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.status"); 1713 else if (Configuration.doAutoCreate()) 1714 this.status = new Enumeration<CarePlanActivityStatus>(new CarePlanActivityStatusEnumFactory()); // bb 1715 return this.status; 1716 } 1717 1718 public boolean hasStatusElement() { 1719 return this.status != null && !this.status.isEmpty(); 1720 } 1721 1722 public boolean hasStatus() { 1723 return this.status != null && !this.status.isEmpty(); 1724 } 1725 1726 /** 1727 * @param value {@link #status} (Identifies what progress is being made for the 1728 * specific activity.). This is the underlying object with id, 1729 * value and extensions. The accessor "getStatus" gives direct 1730 * access to the value 1731 */ 1732 public CarePlanActivityDetailComponent setStatusElement(Enumeration<CarePlanActivityStatus> value) { 1733 this.status = value; 1734 return this; 1735 } 1736 1737 /** 1738 * @return Identifies what progress is being made for the specific activity. 1739 */ 1740 public CarePlanActivityStatus getStatus() { 1741 return this.status == null ? null : this.status.getValue(); 1742 } 1743 1744 /** 1745 * @param value Identifies what progress is being made for the specific 1746 * activity. 1747 */ 1748 public CarePlanActivityDetailComponent setStatus(CarePlanActivityStatus value) { 1749 if (value == null) 1750 this.status = null; 1751 else { 1752 if (this.status == null) 1753 this.status = new Enumeration<CarePlanActivityStatus>(new CarePlanActivityStatusEnumFactory()); 1754 this.status.setValue(value); 1755 } 1756 return this; 1757 } 1758 1759 /** 1760 * @return {@link #statusReason} (Provides reason why the activity isn't yet 1761 * started, is on hold, was cancelled, etc.) 1762 */ 1763 public CodeableConcept getStatusReason() { 1764 if (this.statusReason == null) 1765 if (Configuration.errorOnAutoCreate()) 1766 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.statusReason"); 1767 else if (Configuration.doAutoCreate()) 1768 this.statusReason = new CodeableConcept(); // cc 1769 return this.statusReason; 1770 } 1771 1772 public boolean hasStatusReason() { 1773 return this.statusReason != null && !this.statusReason.isEmpty(); 1774 } 1775 1776 /** 1777 * @param value {@link #statusReason} (Provides reason why the activity isn't 1778 * yet started, is on hold, was cancelled, etc.) 1779 */ 1780 public CarePlanActivityDetailComponent setStatusReason(CodeableConcept value) { 1781 this.statusReason = value; 1782 return this; 1783 } 1784 1785 /** 1786 * @return {@link #prohibited} (If true, indicates that the described activity 1787 * is one that must NOT be engaged in when following the plan.). This is 1788 * the underlying object with id, value and extensions. The accessor 1789 * "getProhibited" gives direct access to the value 1790 */ 1791 public BooleanType getProhibitedElement() { 1792 if (this.prohibited == null) 1793 if (Configuration.errorOnAutoCreate()) 1794 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.prohibited"); 1795 else if (Configuration.doAutoCreate()) 1796 this.prohibited = new BooleanType(); // bb 1797 return this.prohibited; 1798 } 1799 1800 public boolean hasProhibitedElement() { 1801 return this.prohibited != null && !this.prohibited.isEmpty(); 1802 } 1803 1804 public boolean hasProhibited() { 1805 return this.prohibited != null && !this.prohibited.isEmpty(); 1806 } 1807 1808 /** 1809 * @param value {@link #prohibited} (If true, indicates that the described 1810 * activity is one that must NOT be engaged in when following the 1811 * plan.). This is the underlying object with id, value and 1812 * extensions. The accessor "getProhibited" gives direct access to 1813 * the value 1814 */ 1815 public CarePlanActivityDetailComponent setProhibitedElement(BooleanType value) { 1816 this.prohibited = value; 1817 return this; 1818 } 1819 1820 /** 1821 * @return If true, indicates that the described activity is one that must NOT 1822 * be engaged in when following the plan. 1823 */ 1824 public boolean getProhibited() { 1825 return this.prohibited == null || this.prohibited.isEmpty() ? false : this.prohibited.getValue(); 1826 } 1827 1828 /** 1829 * @param value If true, indicates that the described activity is one that must 1830 * NOT be engaged in when following the plan. 1831 */ 1832 public CarePlanActivityDetailComponent setProhibited(boolean value) { 1833 if (this.prohibited == null) 1834 this.prohibited = new BooleanType(); 1835 this.prohibited.setValue(value); 1836 return this; 1837 } 1838 1839 /** 1840 * @return {@link #scheduled} (The period, timing or frequency upon which the 1841 * described activity is to occur.) 1842 */ 1843 public Type getScheduled() { 1844 return this.scheduled; 1845 } 1846 1847 /** 1848 * @return {@link #scheduled} (The period, timing or frequency upon which the 1849 * described activity is to occur.) 1850 */ 1851 public Timing getScheduledTiming() throws FHIRException { 1852 if (!(this.scheduled instanceof Timing)) 1853 throw new FHIRException("Type mismatch: the type Timing was expected, but " 1854 + this.scheduled.getClass().getName() + " was encountered"); 1855 return (Timing) this.scheduled; 1856 } 1857 1858 public boolean hasScheduledTiming() { 1859 return this.scheduled instanceof Timing; 1860 } 1861 1862 /** 1863 * @return {@link #scheduled} (The period, timing or frequency upon which the 1864 * described activity is to occur.) 1865 */ 1866 public Period getScheduledPeriod() throws FHIRException { 1867 if (!(this.scheduled instanceof Period)) 1868 throw new FHIRException("Type mismatch: the type Period was expected, but " 1869 + this.scheduled.getClass().getName() + " was encountered"); 1870 return (Period) this.scheduled; 1871 } 1872 1873 public boolean hasScheduledPeriod() { 1874 return this.scheduled instanceof Period; 1875 } 1876 1877 /** 1878 * @return {@link #scheduled} (The period, timing or frequency upon which the 1879 * described activity is to occur.) 1880 */ 1881 public StringType getScheduledStringType() throws FHIRException { 1882 if (!(this.scheduled instanceof StringType)) 1883 throw new FHIRException("Type mismatch: the type StringType was expected, but " 1884 + this.scheduled.getClass().getName() + " was encountered"); 1885 return (StringType) this.scheduled; 1886 } 1887 1888 public boolean hasScheduledStringType() { 1889 return this.scheduled instanceof StringType; 1890 } 1891 1892 public boolean hasScheduled() { 1893 return this.scheduled != null && !this.scheduled.isEmpty(); 1894 } 1895 1896 /** 1897 * @param value {@link #scheduled} (The period, timing or frequency upon which 1898 * the described activity is to occur.) 1899 */ 1900 public CarePlanActivityDetailComponent setScheduled(Type value) { 1901 this.scheduled = value; 1902 return this; 1903 } 1904 1905 /** 1906 * @return {@link #location} (Identifies the facility where the activity will 1907 * occur; e.g. home, hospital, specific clinic, etc.) 1908 */ 1909 public Reference getLocation() { 1910 if (this.location == null) 1911 if (Configuration.errorOnAutoCreate()) 1912 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.location"); 1913 else if (Configuration.doAutoCreate()) 1914 this.location = new Reference(); // cc 1915 return this.location; 1916 } 1917 1918 public boolean hasLocation() { 1919 return this.location != null && !this.location.isEmpty(); 1920 } 1921 1922 /** 1923 * @param value {@link #location} (Identifies the facility where the activity 1924 * will occur; e.g. home, hospital, specific clinic, etc.) 1925 */ 1926 public CarePlanActivityDetailComponent setLocation(Reference value) { 1927 this.location = value; 1928 return this; 1929 } 1930 1931 /** 1932 * @return {@link #location} The actual object that is the target of the 1933 * reference. The reference library doesn't populate this, but you can 1934 * use it to hold the resource if you resolve it. (Identifies the 1935 * facility where the activity will occur; e.g. home, hospital, specific 1936 * clinic, etc.) 1937 */ 1938 public Location getLocationTarget() { 1939 if (this.locationTarget == null) 1940 if (Configuration.errorOnAutoCreate()) 1941 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.location"); 1942 else if (Configuration.doAutoCreate()) 1943 this.locationTarget = new Location(); // aa 1944 return this.locationTarget; 1945 } 1946 1947 /** 1948 * @param value {@link #location} The actual object that is the target of the 1949 * reference. The reference library doesn't use these, but you can 1950 * use it to hold the resource if you resolve it. (Identifies the 1951 * facility where the activity will occur; e.g. home, hospital, 1952 * specific clinic, etc.) 1953 */ 1954 public CarePlanActivityDetailComponent setLocationTarget(Location value) { 1955 this.locationTarget = value; 1956 return this; 1957 } 1958 1959 /** 1960 * @return {@link #performer} (Identifies who's expected to be involved in the 1961 * activity.) 1962 */ 1963 public List<Reference> getPerformer() { 1964 if (this.performer == null) 1965 this.performer = new ArrayList<Reference>(); 1966 return this.performer; 1967 } 1968 1969 public boolean hasPerformer() { 1970 if (this.performer == null) 1971 return false; 1972 for (Reference item : this.performer) 1973 if (!item.isEmpty()) 1974 return true; 1975 return false; 1976 } 1977 1978 /** 1979 * @return {@link #performer} (Identifies who's expected to be involved in the 1980 * activity.) 1981 */ 1982 // syntactic sugar 1983 public Reference addPerformer() { // 3 1984 Reference t = new Reference(); 1985 if (this.performer == null) 1986 this.performer = new ArrayList<Reference>(); 1987 this.performer.add(t); 1988 return t; 1989 } 1990 1991 // syntactic sugar 1992 public CarePlanActivityDetailComponent addPerformer(Reference t) { // 3 1993 if (t == null) 1994 return this; 1995 if (this.performer == null) 1996 this.performer = new ArrayList<Reference>(); 1997 this.performer.add(t); 1998 return this; 1999 } 2000 2001 /** 2002 * @return {@link #performer} (The actual objects that are the target of the 2003 * reference. The reference library doesn't populate this, but you can 2004 * use this to hold the resources if you resolvethemt. Identifies who's 2005 * expected to be involved in the activity.) 2006 */ 2007 public List<Resource> getPerformerTarget() { 2008 if (this.performerTarget == null) 2009 this.performerTarget = new ArrayList<Resource>(); 2010 return this.performerTarget; 2011 } 2012 2013 /** 2014 * @return {@link #product} (Identifies the food, drug or other product to be 2015 * consumed or supplied in the activity.) 2016 */ 2017 public Type getProduct() { 2018 return this.product; 2019 } 2020 2021 /** 2022 * @return {@link #product} (Identifies the food, drug or other product to be 2023 * consumed or supplied in the activity.) 2024 */ 2025 public CodeableConcept getProductCodeableConcept() throws FHIRException { 2026 if (!(this.product instanceof CodeableConcept)) 2027 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 2028 + this.product.getClass().getName() + " was encountered"); 2029 return (CodeableConcept) this.product; 2030 } 2031 2032 public boolean hasProductCodeableConcept() { 2033 return this.product instanceof CodeableConcept; 2034 } 2035 2036 /** 2037 * @return {@link #product} (Identifies the food, drug or other product to be 2038 * consumed or supplied in the activity.) 2039 */ 2040 public Reference getProductReference() throws FHIRException { 2041 if (!(this.product instanceof Reference)) 2042 throw new FHIRException("Type mismatch: the type Reference was expected, but " 2043 + this.product.getClass().getName() + " was encountered"); 2044 return (Reference) this.product; 2045 } 2046 2047 public boolean hasProductReference() { 2048 return this.product instanceof Reference; 2049 } 2050 2051 public boolean hasProduct() { 2052 return this.product != null && !this.product.isEmpty(); 2053 } 2054 2055 /** 2056 * @param value {@link #product} (Identifies the food, drug or other product to 2057 * be consumed or supplied in the activity.) 2058 */ 2059 public CarePlanActivityDetailComponent setProduct(Type value) { 2060 this.product = value; 2061 return this; 2062 } 2063 2064 /** 2065 * @return {@link #dailyAmount} (Identifies the quantity expected to be consumed 2066 * in a given day.) 2067 */ 2068 public SimpleQuantity getDailyAmount() { 2069 if (this.dailyAmount == null) 2070 if (Configuration.errorOnAutoCreate()) 2071 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.dailyAmount"); 2072 else if (Configuration.doAutoCreate()) 2073 this.dailyAmount = new SimpleQuantity(); // cc 2074 return this.dailyAmount; 2075 } 2076 2077 public boolean hasDailyAmount() { 2078 return this.dailyAmount != null && !this.dailyAmount.isEmpty(); 2079 } 2080 2081 /** 2082 * @param value {@link #dailyAmount} (Identifies the quantity expected to be 2083 * consumed in a given day.) 2084 */ 2085 public CarePlanActivityDetailComponent setDailyAmount(SimpleQuantity value) { 2086 this.dailyAmount = value; 2087 return this; 2088 } 2089 2090 /** 2091 * @return {@link #quantity} (Identifies the quantity expected to be supplied, 2092 * administered or consumed by the subject.) 2093 */ 2094 public SimpleQuantity getQuantity() { 2095 if (this.quantity == null) 2096 if (Configuration.errorOnAutoCreate()) 2097 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.quantity"); 2098 else if (Configuration.doAutoCreate()) 2099 this.quantity = new SimpleQuantity(); // cc 2100 return this.quantity; 2101 } 2102 2103 public boolean hasQuantity() { 2104 return this.quantity != null && !this.quantity.isEmpty(); 2105 } 2106 2107 /** 2108 * @param value {@link #quantity} (Identifies the quantity expected to be 2109 * supplied, administered or consumed by the subject.) 2110 */ 2111 public CarePlanActivityDetailComponent setQuantity(SimpleQuantity value) { 2112 this.quantity = value; 2113 return this; 2114 } 2115 2116 /** 2117 * @return {@link #description} (This provides a textual description of 2118 * constraints on the intended activity occurrence, including relation 2119 * to other activities. It may also include objectives, pre-conditions 2120 * and end-conditions. Finally, it may convey specifics about the 2121 * activity such as body site, method, route, etc.). This is the 2122 * underlying object with id, value and extensions. The accessor 2123 * "getDescription" gives direct access to the value 2124 */ 2125 public StringType getDescriptionElement() { 2126 if (this.description == null) 2127 if (Configuration.errorOnAutoCreate()) 2128 throw new Error("Attempt to auto-create CarePlanActivityDetailComponent.description"); 2129 else if (Configuration.doAutoCreate()) 2130 this.description = new StringType(); // bb 2131 return this.description; 2132 } 2133 2134 public boolean hasDescriptionElement() { 2135 return this.description != null && !this.description.isEmpty(); 2136 } 2137 2138 public boolean hasDescription() { 2139 return this.description != null && !this.description.isEmpty(); 2140 } 2141 2142 /** 2143 * @param value {@link #description} (This provides a textual description of 2144 * constraints on the intended activity occurrence, including 2145 * relation to other activities. It may also include objectives, 2146 * pre-conditions and end-conditions. Finally, it may convey 2147 * specifics about the activity such as body site, method, route, 2148 * etc.). This is the underlying object with id, value and 2149 * extensions. The accessor "getDescription" gives direct access to 2150 * the value 2151 */ 2152 public CarePlanActivityDetailComponent setDescriptionElement(StringType value) { 2153 this.description = value; 2154 return this; 2155 } 2156 2157 /** 2158 * @return This provides a textual description of constraints on the intended 2159 * activity occurrence, including relation to other activities. It may 2160 * also include objectives, pre-conditions and end-conditions. Finally, 2161 * it may convey specifics about the activity such as body site, method, 2162 * route, etc. 2163 */ 2164 public String getDescription() { 2165 return this.description == null ? null : this.description.getValue(); 2166 } 2167 2168 /** 2169 * @param value This provides a textual description of constraints on the 2170 * intended activity occurrence, including relation to other 2171 * activities. It may also include objectives, pre-conditions and 2172 * end-conditions. Finally, it may convey specifics about the 2173 * activity such as body site, method, route, etc. 2174 */ 2175 public CarePlanActivityDetailComponent setDescription(String value) { 2176 if (Utilities.noString(value)) 2177 this.description = null; 2178 else { 2179 if (this.description == null) 2180 this.description = new StringType(); 2181 this.description.setValue(value); 2182 } 2183 return this; 2184 } 2185 2186 protected void listChildren(List<Property> childrenList) { 2187 super.listChildren(childrenList); 2188 childrenList.add(new Property("category", "CodeableConcept", 2189 "High-level categorization of the type of activity in a care plan.", 0, java.lang.Integer.MAX_VALUE, 2190 category)); 2191 childrenList.add(new Property("code", "CodeableConcept", 2192 "Detailed description of the type of planned activity; e.g. What lab test, what procedure, what kind of encounter.", 2193 0, java.lang.Integer.MAX_VALUE, code)); 2194 childrenList.add(new Property("reasonCode", "CodeableConcept", 2195 "Provides the rationale that drove the inclusion of this particular activity as part of the plan.", 0, 2196 java.lang.Integer.MAX_VALUE, reasonCode)); 2197 childrenList.add(new Property("reasonReference", "Reference(Condition)", 2198 "Provides the health condition(s) that drove the inclusion of this particular activity as part of the plan.", 2199 0, java.lang.Integer.MAX_VALUE, reasonReference)); 2200 childrenList.add(new Property("goal", "Reference(Goal)", 2201 "Internal reference that identifies the goals that this activity is intended to contribute towards meeting.", 2202 0, java.lang.Integer.MAX_VALUE, goal)); 2203 childrenList.add(new Property("status", "code", 2204 "Identifies what progress is being made for the specific activity.", 0, java.lang.Integer.MAX_VALUE, status)); 2205 childrenList.add(new Property("statusReason", "CodeableConcept", 2206 "Provides reason why the activity isn't yet started, is on hold, was cancelled, etc.", 0, 2207 java.lang.Integer.MAX_VALUE, statusReason)); 2208 childrenList.add(new Property("prohibited", "boolean", 2209 "If true, indicates that the described activity is one that must NOT be engaged in when following the plan.", 2210 0, java.lang.Integer.MAX_VALUE, prohibited)); 2211 childrenList.add(new Property("scheduled[x]", "Timing|Period|string", 2212 "The period, timing or frequency upon which the described activity is to occur.", 0, 2213 java.lang.Integer.MAX_VALUE, scheduled)); 2214 childrenList.add(new Property("location", "Reference(Location)", 2215 "Identifies the facility where the activity will occur; e.g. home, hospital, specific clinic, etc.", 0, 2216 java.lang.Integer.MAX_VALUE, location)); 2217 childrenList.add(new Property("performer", "Reference(Practitioner|Organization|RelatedPerson|Patient)", 2218 "Identifies who's expected to be involved in the activity.", 0, java.lang.Integer.MAX_VALUE, performer)); 2219 childrenList.add(new Property("product[x]", "CodeableConcept|Reference(Medication|Substance)", 2220 "Identifies the food, drug or other product to be consumed or supplied in the activity.", 0, 2221 java.lang.Integer.MAX_VALUE, product)); 2222 childrenList.add(new Property("dailyAmount", "SimpleQuantity", 2223 "Identifies the quantity expected to be consumed in a given day.", 0, java.lang.Integer.MAX_VALUE, 2224 dailyAmount)); 2225 childrenList.add(new Property("quantity", "SimpleQuantity", 2226 "Identifies the quantity expected to be supplied, administered or consumed by the subject.", 0, 2227 java.lang.Integer.MAX_VALUE, quantity)); 2228 childrenList.add(new Property("description", "string", 2229 "This provides a textual description of constraints on the intended activity occurrence, including relation to other activities. It may also include objectives, pre-conditions and end-conditions. Finally, it may convey specifics about the activity such as body site, method, route, etc.", 2230 0, java.lang.Integer.MAX_VALUE, description)); 2231 } 2232 2233 @Override 2234 public void setProperty(String name, Base value) throws FHIRException { 2235 if (name.equals("category")) 2236 this.category = castToCodeableConcept(value); // CodeableConcept 2237 else if (name.equals("code")) 2238 this.code = castToCodeableConcept(value); // CodeableConcept 2239 else if (name.equals("reasonCode")) 2240 this.getReasonCode().add(castToCodeableConcept(value)); 2241 else if (name.equals("reasonReference")) 2242 this.getReasonReference().add(castToReference(value)); 2243 else if (name.equals("goal")) 2244 this.getGoal().add(castToReference(value)); 2245 else if (name.equals("status")) 2246 this.status = new CarePlanActivityStatusEnumFactory().fromType(value); // Enumeration<CarePlanActivityStatus> 2247 else if (name.equals("statusReason")) 2248 this.statusReason = castToCodeableConcept(value); // CodeableConcept 2249 else if (name.equals("prohibited")) 2250 this.prohibited = castToBoolean(value); // BooleanType 2251 else if (name.equals("scheduled[x]")) 2252 this.scheduled = (Type) value; // Type 2253 else if (name.equals("location")) 2254 this.location = castToReference(value); // Reference 2255 else if (name.equals("performer")) 2256 this.getPerformer().add(castToReference(value)); 2257 else if (name.equals("product[x]")) 2258 this.product = (Type) value; // Type 2259 else if (name.equals("dailyAmount")) 2260 this.dailyAmount = castToSimpleQuantity(value); // SimpleQuantity 2261 else if (name.equals("quantity")) 2262 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 2263 else if (name.equals("description")) 2264 this.description = castToString(value); // StringType 2265 else 2266 super.setProperty(name, value); 2267 } 2268 2269 @Override 2270 public Base addChild(String name) throws FHIRException { 2271 if (name.equals("category")) { 2272 this.category = new CodeableConcept(); 2273 return this.category; 2274 } else if (name.equals("code")) { 2275 this.code = new CodeableConcept(); 2276 return this.code; 2277 } else if (name.equals("reasonCode")) { 2278 return addReasonCode(); 2279 } else if (name.equals("reasonReference")) { 2280 return addReasonReference(); 2281 } else if (name.equals("goal")) { 2282 return addGoal(); 2283 } else if (name.equals("status")) { 2284 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.status"); 2285 } else if (name.equals("statusReason")) { 2286 this.statusReason = new CodeableConcept(); 2287 return this.statusReason; 2288 } else if (name.equals("prohibited")) { 2289 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.prohibited"); 2290 } else if (name.equals("scheduledTiming")) { 2291 this.scheduled = new Timing(); 2292 return this.scheduled; 2293 } else if (name.equals("scheduledPeriod")) { 2294 this.scheduled = new Period(); 2295 return this.scheduled; 2296 } else if (name.equals("scheduledString")) { 2297 this.scheduled = new StringType(); 2298 return this.scheduled; 2299 } else if (name.equals("location")) { 2300 this.location = new Reference(); 2301 return this.location; 2302 } else if (name.equals("performer")) { 2303 return addPerformer(); 2304 } else if (name.equals("productCodeableConcept")) { 2305 this.product = new CodeableConcept(); 2306 return this.product; 2307 } else if (name.equals("productReference")) { 2308 this.product = new Reference(); 2309 return this.product; 2310 } else if (name.equals("dailyAmount")) { 2311 this.dailyAmount = new SimpleQuantity(); 2312 return this.dailyAmount; 2313 } else if (name.equals("quantity")) { 2314 this.quantity = new SimpleQuantity(); 2315 return this.quantity; 2316 } else if (name.equals("description")) { 2317 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.description"); 2318 } else 2319 return super.addChild(name); 2320 } 2321 2322 public CarePlanActivityDetailComponent copy() { 2323 CarePlanActivityDetailComponent dst = new CarePlanActivityDetailComponent(); 2324 copyValues(dst); 2325 dst.category = category == null ? null : category.copy(); 2326 dst.code = code == null ? null : code.copy(); 2327 if (reasonCode != null) { 2328 dst.reasonCode = new ArrayList<CodeableConcept>(); 2329 for (CodeableConcept i : reasonCode) 2330 dst.reasonCode.add(i.copy()); 2331 } 2332 ; 2333 if (reasonReference != null) { 2334 dst.reasonReference = new ArrayList<Reference>(); 2335 for (Reference i : reasonReference) 2336 dst.reasonReference.add(i.copy()); 2337 } 2338 ; 2339 if (goal != null) { 2340 dst.goal = new ArrayList<Reference>(); 2341 for (Reference i : goal) 2342 dst.goal.add(i.copy()); 2343 } 2344 ; 2345 dst.status = status == null ? null : status.copy(); 2346 dst.statusReason = statusReason == null ? null : statusReason.copy(); 2347 dst.prohibited = prohibited == null ? null : prohibited.copy(); 2348 dst.scheduled = scheduled == null ? null : scheduled.copy(); 2349 dst.location = location == null ? null : location.copy(); 2350 if (performer != null) { 2351 dst.performer = new ArrayList<Reference>(); 2352 for (Reference i : performer) 2353 dst.performer.add(i.copy()); 2354 } 2355 ; 2356 dst.product = product == null ? null : product.copy(); 2357 dst.dailyAmount = dailyAmount == null ? null : dailyAmount.copy(); 2358 dst.quantity = quantity == null ? null : quantity.copy(); 2359 dst.description = description == null ? null : description.copy(); 2360 return dst; 2361 } 2362 2363 @Override 2364 public boolean equalsDeep(Base other) { 2365 if (!super.equalsDeep(other)) 2366 return false; 2367 if (!(other instanceof CarePlanActivityDetailComponent)) 2368 return false; 2369 CarePlanActivityDetailComponent o = (CarePlanActivityDetailComponent) other; 2370 return compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 2371 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 2372 && compareDeep(goal, o.goal, true) && compareDeep(status, o.status, true) 2373 && compareDeep(statusReason, o.statusReason, true) && compareDeep(prohibited, o.prohibited, true) 2374 && compareDeep(scheduled, o.scheduled, true) && compareDeep(location, o.location, true) 2375 && compareDeep(performer, o.performer, true) && compareDeep(product, o.product, true) 2376 && compareDeep(dailyAmount, o.dailyAmount, true) && compareDeep(quantity, o.quantity, true) 2377 && compareDeep(description, o.description, true); 2378 } 2379 2380 @Override 2381 public boolean equalsShallow(Base other) { 2382 if (!super.equalsShallow(other)) 2383 return false; 2384 if (!(other instanceof CarePlanActivityDetailComponent)) 2385 return false; 2386 CarePlanActivityDetailComponent o = (CarePlanActivityDetailComponent) other; 2387 return compareValues(status, o.status, true) && compareValues(prohibited, o.prohibited, true) 2388 && compareValues(description, o.description, true); 2389 } 2390 2391 public boolean isEmpty() { 2392 return super.isEmpty() && (category == null || category.isEmpty()) && (code == null || code.isEmpty()) 2393 && (reasonCode == null || reasonCode.isEmpty()) && (reasonReference == null || reasonReference.isEmpty()) 2394 && (goal == null || goal.isEmpty()) && (status == null || status.isEmpty()) 2395 && (statusReason == null || statusReason.isEmpty()) && (prohibited == null || prohibited.isEmpty()) 2396 && (scheduled == null || scheduled.isEmpty()) && (location == null || location.isEmpty()) 2397 && (performer == null || performer.isEmpty()) && (product == null || product.isEmpty()) 2398 && (dailyAmount == null || dailyAmount.isEmpty()) && (quantity == null || quantity.isEmpty()) 2399 && (description == null || description.isEmpty()); 2400 } 2401 2402 public String fhirType() { 2403 return "CarePlan.activity.detail"; 2404 2405 } 2406 2407 } 2408 2409 /** 2410 * This records identifiers associated with this care plan that are defined by 2411 * business processes and/or used to refer to it when a direct URL reference to 2412 * the resource itself is not appropriate (e.g. in CDA documents, or in written 2413 * / printed documentation). 2414 */ 2415 @Child(name = "identifier", type = { 2416 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2417 @Description(shortDefinition = "External Ids for this plan", formalDefinition = "This records identifiers associated with this care plan that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).") 2418 protected List<Identifier> identifier; 2419 2420 /** 2421 * Identifies the patient or group whose intended care is described by the plan. 2422 */ 2423 @Child(name = "subject", type = { Patient.class, 2424 Group.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2425 @Description(shortDefinition = "Who care plan is for", formalDefinition = "Identifies the patient or group whose intended care is described by the plan.") 2426 protected Reference subject; 2427 2428 /** 2429 * The actual object that is the target of the reference (Identifies the patient 2430 * or group whose intended care is described by the plan.) 2431 */ 2432 protected Resource subjectTarget; 2433 2434 /** 2435 * Indicates whether the plan is currently being acted upon, represents future 2436 * intentions or is now a historical record. 2437 */ 2438 @Child(name = "status", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 2439 @Description(shortDefinition = "proposed | draft | active | completed | cancelled", formalDefinition = "Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record.") 2440 protected Enumeration<CarePlanStatus> status; 2441 2442 /** 2443 * Identifies the context in which this particular CarePlan is defined. 2444 */ 2445 @Child(name = "context", type = { Encounter.class, 2446 EpisodeOfCare.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2447 @Description(shortDefinition = "Created in context of", formalDefinition = "Identifies the context in which this particular CarePlan is defined.") 2448 protected Reference context; 2449 2450 /** 2451 * The actual object that is the target of the reference (Identifies the context 2452 * in which this particular CarePlan is defined.) 2453 */ 2454 protected Resource contextTarget; 2455 2456 /** 2457 * Indicates when the plan did (or is intended to) come into effect and end. 2458 */ 2459 @Child(name = "period", type = { Period.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2460 @Description(shortDefinition = "Time period plan covers", formalDefinition = "Indicates when the plan did (or is intended to) come into effect and end.") 2461 protected Period period; 2462 2463 /** 2464 * Identifies the individual(s) or ogranization who is responsible for the 2465 * content of the care plan. 2466 */ 2467 @Child(name = "author", type = { Patient.class, Practitioner.class, RelatedPerson.class, 2468 Organization.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2469 @Description(shortDefinition = "Who is responsible for contents of the plan", formalDefinition = "Identifies the individual(s) or ogranization who is responsible for the content of the care plan.") 2470 protected List<Reference> author; 2471 /** 2472 * The actual objects that are the target of the reference (Identifies the 2473 * individual(s) or ogranization who is responsible for the content of the care 2474 * plan.) 2475 */ 2476 protected List<Resource> authorTarget; 2477 2478 /** 2479 * Identifies the most recent date on which the plan has been revised. 2480 */ 2481 @Child(name = "modified", type = { 2482 DateTimeType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 2483 @Description(shortDefinition = "When last updated", formalDefinition = "Identifies the most recent date on which the plan has been revised.") 2484 protected DateTimeType modified; 2485 2486 /** 2487 * Identifies what "kind" of plan this is to support differentiation between 2488 * multiple co-existing plans; e.g. "Home health", "psychiatric", "asthma", 2489 * "disease management", "wellness plan", etc. 2490 */ 2491 @Child(name = "category", type = { 2492 CodeableConcept.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2493 @Description(shortDefinition = "Type of plan", formalDefinition = "Identifies what \"kind\" of plan this is to support differentiation between multiple co-existing plans; e.g. \"Home health\", \"psychiatric\", \"asthma\", \"disease management\", \"wellness plan\", etc.") 2494 protected List<CodeableConcept> category; 2495 2496 /** 2497 * A description of the scope and nature of the plan. 2498 */ 2499 @Child(name = "description", type = { 2500 StringType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 2501 @Description(shortDefinition = "Summary of nature of plan", formalDefinition = "A description of the scope and nature of the plan.") 2502 protected StringType description; 2503 2504 /** 2505 * Identifies the conditions/problems/concerns/diagnoses/etc. whose management 2506 * and/or mitigation are handled by this plan. 2507 */ 2508 @Child(name = "addresses", type = { 2509 Condition.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2510 @Description(shortDefinition = "Health issues this plan addresses", formalDefinition = "Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan.") 2511 protected List<Reference> addresses; 2512 /** 2513 * The actual objects that are the target of the reference (Identifies the 2514 * conditions/problems/concerns/diagnoses/etc. whose management and/or 2515 * mitigation are handled by this plan.) 2516 */ 2517 protected List<Condition> addressesTarget; 2518 2519 /** 2520 * Identifies portions of the patient's record that specifically influenced the 2521 * formation of the plan. These might include co-morbidities, recent procedures, 2522 * limitations, recent assessments, etc. 2523 */ 2524 @Child(name = "support", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2525 @Description(shortDefinition = "Information considered as part of plan", formalDefinition = "Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include co-morbidities, recent procedures, limitations, recent assessments, etc.") 2526 protected List<Reference> support; 2527 /** 2528 * The actual objects that are the target of the reference (Identifies portions 2529 * of the patient's record that specifically influenced the formation of the 2530 * plan. These might include co-morbidities, recent procedures, limitations, 2531 * recent assessments, etc.) 2532 */ 2533 protected List<Resource> supportTarget; 2534 2535 /** 2536 * Identifies CarePlans with some sort of formal relationship to the current 2537 * plan. 2538 */ 2539 @Child(name = "relatedPlan", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2540 @Description(shortDefinition = "Plans related to this one", formalDefinition = "Identifies CarePlans with some sort of formal relationship to the current plan.") 2541 protected List<CarePlanRelatedPlanComponent> relatedPlan; 2542 2543 /** 2544 * Identifies all people and organizations who are expected to be involved in 2545 * the care envisioned by this plan. 2546 */ 2547 @Child(name = "participant", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2548 @Description(shortDefinition = "Who's involved in plan?", formalDefinition = "Identifies all people and organizations who are expected to be involved in the care envisioned by this plan.") 2549 protected List<CarePlanParticipantComponent> participant; 2550 2551 /** 2552 * Describes the intended objective(s) of carrying out the care plan. 2553 */ 2554 @Child(name = "goal", type = { 2555 Goal.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2556 @Description(shortDefinition = "Desired outcome of plan", formalDefinition = "Describes the intended objective(s) of carrying out the care plan.") 2557 protected List<Reference> goal; 2558 /** 2559 * The actual objects that are the target of the reference (Describes the 2560 * intended objective(s) of carrying out the care plan.) 2561 */ 2562 protected List<Goal> goalTarget; 2563 2564 /** 2565 * Identifies a planned action to occur as part of the plan. For example, a 2566 * medication to be used, lab tests to perform, self-monitoring, education, etc. 2567 */ 2568 @Child(name = "activity", type = {}, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 2569 @Description(shortDefinition = "Action to occur as part of plan", formalDefinition = "Identifies a planned action to occur as part of the plan. For example, a medication to be used, lab tests to perform, self-monitoring, education, etc.") 2570 protected List<CarePlanActivityComponent> activity; 2571 2572 /** 2573 * General notes about the care plan not covered elsewhere. 2574 */ 2575 @Child(name = "note", type = { Annotation.class }, order = 15, min = 0, max = 1, modifier = false, summary = false) 2576 @Description(shortDefinition = "Comments about the plan", formalDefinition = "General notes about the care plan not covered elsewhere.") 2577 protected Annotation note; 2578 2579 private static final long serialVersionUID = -307500543L; 2580 2581 /* 2582 * Constructor 2583 */ 2584 public CarePlan() { 2585 super(); 2586 } 2587 2588 /* 2589 * Constructor 2590 */ 2591 public CarePlan(Enumeration<CarePlanStatus> status) { 2592 super(); 2593 this.status = status; 2594 } 2595 2596 /** 2597 * @return {@link #identifier} (This records identifiers associated with this 2598 * care plan that are defined by business processes and/or used to refer 2599 * to it when a direct URL reference to the resource itself is not 2600 * appropriate (e.g. in CDA documents, or in written / printed 2601 * documentation).) 2602 */ 2603 public List<Identifier> getIdentifier() { 2604 if (this.identifier == null) 2605 this.identifier = new ArrayList<Identifier>(); 2606 return this.identifier; 2607 } 2608 2609 public boolean hasIdentifier() { 2610 if (this.identifier == null) 2611 return false; 2612 for (Identifier item : this.identifier) 2613 if (!item.isEmpty()) 2614 return true; 2615 return false; 2616 } 2617 2618 /** 2619 * @return {@link #identifier} (This records identifiers associated with this 2620 * care plan that are defined by business processes and/or used to refer 2621 * to it when a direct URL reference to the resource itself is not 2622 * appropriate (e.g. in CDA documents, or in written / printed 2623 * documentation).) 2624 */ 2625 // syntactic sugar 2626 public Identifier addIdentifier() { // 3 2627 Identifier t = new Identifier(); 2628 if (this.identifier == null) 2629 this.identifier = new ArrayList<Identifier>(); 2630 this.identifier.add(t); 2631 return t; 2632 } 2633 2634 // syntactic sugar 2635 public CarePlan addIdentifier(Identifier t) { // 3 2636 if (t == null) 2637 return this; 2638 if (this.identifier == null) 2639 this.identifier = new ArrayList<Identifier>(); 2640 this.identifier.add(t); 2641 return this; 2642 } 2643 2644 /** 2645 * @return {@link #subject} (Identifies the patient or group whose intended care 2646 * is described by the plan.) 2647 */ 2648 public Reference getSubject() { 2649 if (this.subject == null) 2650 if (Configuration.errorOnAutoCreate()) 2651 throw new Error("Attempt to auto-create CarePlan.subject"); 2652 else if (Configuration.doAutoCreate()) 2653 this.subject = new Reference(); // cc 2654 return this.subject; 2655 } 2656 2657 public boolean hasSubject() { 2658 return this.subject != null && !this.subject.isEmpty(); 2659 } 2660 2661 /** 2662 * @param value {@link #subject} (Identifies the patient or group whose intended 2663 * care is described by the plan.) 2664 */ 2665 public CarePlan setSubject(Reference value) { 2666 this.subject = value; 2667 return this; 2668 } 2669 2670 /** 2671 * @return {@link #subject} The actual object that is the target of the 2672 * reference. The reference library doesn't populate this, but you can 2673 * use it to hold the resource if you resolve it. (Identifies the 2674 * patient or group whose intended care is described by the plan.) 2675 */ 2676 public Resource getSubjectTarget() { 2677 return this.subjectTarget; 2678 } 2679 2680 /** 2681 * @param value {@link #subject} The actual object that is the target of the 2682 * reference. The reference library doesn't use these, but you can 2683 * use it to hold the resource if you resolve it. (Identifies the 2684 * patient or group whose intended care is described by the plan.) 2685 */ 2686 public CarePlan setSubjectTarget(Resource value) { 2687 this.subjectTarget = value; 2688 return this; 2689 } 2690 2691 /** 2692 * @return {@link #status} (Indicates whether the plan is currently being acted 2693 * upon, represents future intentions or is now a historical record.). 2694 * This is the underlying object with id, value and extensions. The 2695 * accessor "getStatus" gives direct access to the value 2696 */ 2697 public Enumeration<CarePlanStatus> getStatusElement() { 2698 if (this.status == null) 2699 if (Configuration.errorOnAutoCreate()) 2700 throw new Error("Attempt to auto-create CarePlan.status"); 2701 else if (Configuration.doAutoCreate()) 2702 this.status = new Enumeration<CarePlanStatus>(new CarePlanStatusEnumFactory()); // bb 2703 return this.status; 2704 } 2705 2706 public boolean hasStatusElement() { 2707 return this.status != null && !this.status.isEmpty(); 2708 } 2709 2710 public boolean hasStatus() { 2711 return this.status != null && !this.status.isEmpty(); 2712 } 2713 2714 /** 2715 * @param value {@link #status} (Indicates whether the plan is currently being 2716 * acted upon, represents future intentions or is now a historical 2717 * record.). This is the underlying object with id, value and 2718 * extensions. The accessor "getStatus" gives direct access to the 2719 * value 2720 */ 2721 public CarePlan setStatusElement(Enumeration<CarePlanStatus> value) { 2722 this.status = value; 2723 return this; 2724 } 2725 2726 /** 2727 * @return Indicates whether the plan is currently being acted upon, represents 2728 * future intentions or is now a historical record. 2729 */ 2730 public CarePlanStatus getStatus() { 2731 return this.status == null ? null : this.status.getValue(); 2732 } 2733 2734 /** 2735 * @param value Indicates whether the plan is currently being acted upon, 2736 * represents future intentions or is now a historical record. 2737 */ 2738 public CarePlan setStatus(CarePlanStatus value) { 2739 if (this.status == null) 2740 this.status = new Enumeration<CarePlanStatus>(new CarePlanStatusEnumFactory()); 2741 this.status.setValue(value); 2742 return this; 2743 } 2744 2745 /** 2746 * @return {@link #context} (Identifies the context in which this particular 2747 * CarePlan is defined.) 2748 */ 2749 public Reference getContext() { 2750 if (this.context == null) 2751 if (Configuration.errorOnAutoCreate()) 2752 throw new Error("Attempt to auto-create CarePlan.context"); 2753 else if (Configuration.doAutoCreate()) 2754 this.context = new Reference(); // cc 2755 return this.context; 2756 } 2757 2758 public boolean hasContext() { 2759 return this.context != null && !this.context.isEmpty(); 2760 } 2761 2762 /** 2763 * @param value {@link #context} (Identifies the context in which this 2764 * particular CarePlan is defined.) 2765 */ 2766 public CarePlan setContext(Reference value) { 2767 this.context = value; 2768 return this; 2769 } 2770 2771 /** 2772 * @return {@link #context} The actual object that is the target of the 2773 * reference. The reference library doesn't populate this, but you can 2774 * use it to hold the resource if you resolve it. (Identifies the 2775 * context in which this particular CarePlan is defined.) 2776 */ 2777 public Resource getContextTarget() { 2778 return this.contextTarget; 2779 } 2780 2781 /** 2782 * @param value {@link #context} The actual object that is the target of the 2783 * reference. The reference library doesn't use these, but you can 2784 * use it to hold the resource if you resolve it. (Identifies the 2785 * context in which this particular CarePlan is defined.) 2786 */ 2787 public CarePlan setContextTarget(Resource value) { 2788 this.contextTarget = value; 2789 return this; 2790 } 2791 2792 /** 2793 * @return {@link #period} (Indicates when the plan did (or is intended to) come 2794 * into effect and end.) 2795 */ 2796 public Period getPeriod() { 2797 if (this.period == null) 2798 if (Configuration.errorOnAutoCreate()) 2799 throw new Error("Attempt to auto-create CarePlan.period"); 2800 else if (Configuration.doAutoCreate()) 2801 this.period = new Period(); // cc 2802 return this.period; 2803 } 2804 2805 public boolean hasPeriod() { 2806 return this.period != null && !this.period.isEmpty(); 2807 } 2808 2809 /** 2810 * @param value {@link #period} (Indicates when the plan did (or is intended to) 2811 * come into effect and end.) 2812 */ 2813 public CarePlan setPeriod(Period value) { 2814 this.period = value; 2815 return this; 2816 } 2817 2818 /** 2819 * @return {@link #author} (Identifies the individual(s) or ogranization who is 2820 * responsible for the content of the care plan.) 2821 */ 2822 public List<Reference> getAuthor() { 2823 if (this.author == null) 2824 this.author = new ArrayList<Reference>(); 2825 return this.author; 2826 } 2827 2828 public boolean hasAuthor() { 2829 if (this.author == null) 2830 return false; 2831 for (Reference item : this.author) 2832 if (!item.isEmpty()) 2833 return true; 2834 return false; 2835 } 2836 2837 /** 2838 * @return {@link #author} (Identifies the individual(s) or ogranization who is 2839 * responsible for the content of the care plan.) 2840 */ 2841 // syntactic sugar 2842 public Reference addAuthor() { // 3 2843 Reference t = new Reference(); 2844 if (this.author == null) 2845 this.author = new ArrayList<Reference>(); 2846 this.author.add(t); 2847 return t; 2848 } 2849 2850 // syntactic sugar 2851 public CarePlan addAuthor(Reference t) { // 3 2852 if (t == null) 2853 return this; 2854 if (this.author == null) 2855 this.author = new ArrayList<Reference>(); 2856 this.author.add(t); 2857 return this; 2858 } 2859 2860 /** 2861 * @return {@link #author} (The actual objects that are the target of the 2862 * reference. The reference library doesn't populate this, but you can 2863 * use this to hold the resources if you resolvethemt. Identifies the 2864 * individual(s) or ogranization who is responsible for the content of 2865 * the care plan.) 2866 */ 2867 public List<Resource> getAuthorTarget() { 2868 if (this.authorTarget == null) 2869 this.authorTarget = new ArrayList<Resource>(); 2870 return this.authorTarget; 2871 } 2872 2873 /** 2874 * @return {@link #modified} (Identifies the most recent date on which the plan 2875 * has been revised.). This is the underlying object with id, value and 2876 * extensions. The accessor "getModified" gives direct access to the 2877 * value 2878 */ 2879 public DateTimeType getModifiedElement() { 2880 if (this.modified == null) 2881 if (Configuration.errorOnAutoCreate()) 2882 throw new Error("Attempt to auto-create CarePlan.modified"); 2883 else if (Configuration.doAutoCreate()) 2884 this.modified = new DateTimeType(); // bb 2885 return this.modified; 2886 } 2887 2888 public boolean hasModifiedElement() { 2889 return this.modified != null && !this.modified.isEmpty(); 2890 } 2891 2892 public boolean hasModified() { 2893 return this.modified != null && !this.modified.isEmpty(); 2894 } 2895 2896 /** 2897 * @param value {@link #modified} (Identifies the most recent date on which the 2898 * plan has been revised.). This is the underlying object with id, 2899 * value and extensions. The accessor "getModified" gives direct 2900 * access to the value 2901 */ 2902 public CarePlan setModifiedElement(DateTimeType value) { 2903 this.modified = value; 2904 return this; 2905 } 2906 2907 /** 2908 * @return Identifies the most recent date on which the plan has been revised. 2909 */ 2910 public Date getModified() { 2911 return this.modified == null ? null : this.modified.getValue(); 2912 } 2913 2914 /** 2915 * @param value Identifies the most recent date on which the plan has been 2916 * revised. 2917 */ 2918 public CarePlan setModified(Date value) { 2919 if (value == null) 2920 this.modified = null; 2921 else { 2922 if (this.modified == null) 2923 this.modified = new DateTimeType(); 2924 this.modified.setValue(value); 2925 } 2926 return this; 2927 } 2928 2929 /** 2930 * @return {@link #category} (Identifies what "kind" of plan this is to support 2931 * differentiation between multiple co-existing plans; e.g. "Home 2932 * health", "psychiatric", "asthma", "disease management", "wellness 2933 * plan", etc.) 2934 */ 2935 public List<CodeableConcept> getCategory() { 2936 if (this.category == null) 2937 this.category = new ArrayList<CodeableConcept>(); 2938 return this.category; 2939 } 2940 2941 public boolean hasCategory() { 2942 if (this.category == null) 2943 return false; 2944 for (CodeableConcept item : this.category) 2945 if (!item.isEmpty()) 2946 return true; 2947 return false; 2948 } 2949 2950 /** 2951 * @return {@link #category} (Identifies what "kind" of plan this is to support 2952 * differentiation between multiple co-existing plans; e.g. "Home 2953 * health", "psychiatric", "asthma", "disease management", "wellness 2954 * plan", etc.) 2955 */ 2956 // syntactic sugar 2957 public CodeableConcept addCategory() { // 3 2958 CodeableConcept t = new CodeableConcept(); 2959 if (this.category == null) 2960 this.category = new ArrayList<CodeableConcept>(); 2961 this.category.add(t); 2962 return t; 2963 } 2964 2965 // syntactic sugar 2966 public CarePlan addCategory(CodeableConcept t) { // 3 2967 if (t == null) 2968 return this; 2969 if (this.category == null) 2970 this.category = new ArrayList<CodeableConcept>(); 2971 this.category.add(t); 2972 return this; 2973 } 2974 2975 /** 2976 * @return {@link #description} (A description of the scope and nature of the 2977 * plan.). This is the underlying object with id, value and extensions. 2978 * The accessor "getDescription" gives direct access to the value 2979 */ 2980 public StringType getDescriptionElement() { 2981 if (this.description == null) 2982 if (Configuration.errorOnAutoCreate()) 2983 throw new Error("Attempt to auto-create CarePlan.description"); 2984 else if (Configuration.doAutoCreate()) 2985 this.description = new StringType(); // bb 2986 return this.description; 2987 } 2988 2989 public boolean hasDescriptionElement() { 2990 return this.description != null && !this.description.isEmpty(); 2991 } 2992 2993 public boolean hasDescription() { 2994 return this.description != null && !this.description.isEmpty(); 2995 } 2996 2997 /** 2998 * @param value {@link #description} (A description of the scope and nature of 2999 * the plan.). This is the underlying object with id, value and 3000 * extensions. The accessor "getDescription" gives direct access to 3001 * the value 3002 */ 3003 public CarePlan setDescriptionElement(StringType value) { 3004 this.description = value; 3005 return this; 3006 } 3007 3008 /** 3009 * @return A description of the scope and nature of the plan. 3010 */ 3011 public String getDescription() { 3012 return this.description == null ? null : this.description.getValue(); 3013 } 3014 3015 /** 3016 * @param value A description of the scope and nature of the plan. 3017 */ 3018 public CarePlan setDescription(String value) { 3019 if (Utilities.noString(value)) 3020 this.description = null; 3021 else { 3022 if (this.description == null) 3023 this.description = new StringType(); 3024 this.description.setValue(value); 3025 } 3026 return this; 3027 } 3028 3029 /** 3030 * @return {@link #addresses} (Identifies the 3031 * conditions/problems/concerns/diagnoses/etc. whose management and/or 3032 * mitigation are handled by this plan.) 3033 */ 3034 public List<Reference> getAddresses() { 3035 if (this.addresses == null) 3036 this.addresses = new ArrayList<Reference>(); 3037 return this.addresses; 3038 } 3039 3040 public boolean hasAddresses() { 3041 if (this.addresses == null) 3042 return false; 3043 for (Reference item : this.addresses) 3044 if (!item.isEmpty()) 3045 return true; 3046 return false; 3047 } 3048 3049 /** 3050 * @return {@link #addresses} (Identifies the 3051 * conditions/problems/concerns/diagnoses/etc. whose management and/or 3052 * mitigation are handled by this plan.) 3053 */ 3054 // syntactic sugar 3055 public Reference addAddresses() { // 3 3056 Reference t = new Reference(); 3057 if (this.addresses == null) 3058 this.addresses = new ArrayList<Reference>(); 3059 this.addresses.add(t); 3060 return t; 3061 } 3062 3063 // syntactic sugar 3064 public CarePlan addAddresses(Reference t) { // 3 3065 if (t == null) 3066 return this; 3067 if (this.addresses == null) 3068 this.addresses = new ArrayList<Reference>(); 3069 this.addresses.add(t); 3070 return this; 3071 } 3072 3073 /** 3074 * @return {@link #addresses} (The actual objects that are the target of the 3075 * reference. The reference library doesn't populate this, but you can 3076 * use this to hold the resources if you resolvethemt. Identifies the 3077 * conditions/problems/concerns/diagnoses/etc. whose management and/or 3078 * mitigation are handled by this plan.) 3079 */ 3080 public List<Condition> getAddressesTarget() { 3081 if (this.addressesTarget == null) 3082 this.addressesTarget = new ArrayList<Condition>(); 3083 return this.addressesTarget; 3084 } 3085 3086 // syntactic sugar 3087 /** 3088 * @return {@link #addresses} (Add an actual object that is the target of the 3089 * reference. The reference library doesn't use these, but you can use 3090 * this to hold the resources if you resolvethemt. Identifies the 3091 * conditions/problems/concerns/diagnoses/etc. whose management and/or 3092 * mitigation are handled by this plan.) 3093 */ 3094 public Condition addAddressesTarget() { 3095 Condition r = new Condition(); 3096 if (this.addressesTarget == null) 3097 this.addressesTarget = new ArrayList<Condition>(); 3098 this.addressesTarget.add(r); 3099 return r; 3100 } 3101 3102 /** 3103 * @return {@link #support} (Identifies portions of the patient's record that 3104 * specifically influenced the formation of the plan. These might 3105 * include co-morbidities, recent procedures, limitations, recent 3106 * assessments, etc.) 3107 */ 3108 public List<Reference> getSupport() { 3109 if (this.support == null) 3110 this.support = new ArrayList<Reference>(); 3111 return this.support; 3112 } 3113 3114 public boolean hasSupport() { 3115 if (this.support == null) 3116 return false; 3117 for (Reference item : this.support) 3118 if (!item.isEmpty()) 3119 return true; 3120 return false; 3121 } 3122 3123 /** 3124 * @return {@link #support} (Identifies portions of the patient's record that 3125 * specifically influenced the formation of the plan. These might 3126 * include co-morbidities, recent procedures, limitations, recent 3127 * assessments, etc.) 3128 */ 3129 // syntactic sugar 3130 public Reference addSupport() { // 3 3131 Reference t = new Reference(); 3132 if (this.support == null) 3133 this.support = new ArrayList<Reference>(); 3134 this.support.add(t); 3135 return t; 3136 } 3137 3138 // syntactic sugar 3139 public CarePlan addSupport(Reference t) { // 3 3140 if (t == null) 3141 return this; 3142 if (this.support == null) 3143 this.support = new ArrayList<Reference>(); 3144 this.support.add(t); 3145 return this; 3146 } 3147 3148 /** 3149 * @return {@link #support} (The actual objects that are the target of the 3150 * reference. The reference library doesn't populate this, but you can 3151 * use this to hold the resources if you resolvethemt. Identifies 3152 * portions of the patient's record that specifically influenced the 3153 * formation of the plan. These might include co-morbidities, recent 3154 * procedures, limitations, recent assessments, etc.) 3155 */ 3156 public List<Resource> getSupportTarget() { 3157 if (this.supportTarget == null) 3158 this.supportTarget = new ArrayList<Resource>(); 3159 return this.supportTarget; 3160 } 3161 3162 /** 3163 * @return {@link #relatedPlan} (Identifies CarePlans with some sort of formal 3164 * relationship to the current plan.) 3165 */ 3166 public List<CarePlanRelatedPlanComponent> getRelatedPlan() { 3167 if (this.relatedPlan == null) 3168 this.relatedPlan = new ArrayList<CarePlanRelatedPlanComponent>(); 3169 return this.relatedPlan; 3170 } 3171 3172 public boolean hasRelatedPlan() { 3173 if (this.relatedPlan == null) 3174 return false; 3175 for (CarePlanRelatedPlanComponent item : this.relatedPlan) 3176 if (!item.isEmpty()) 3177 return true; 3178 return false; 3179 } 3180 3181 /** 3182 * @return {@link #relatedPlan} (Identifies CarePlans with some sort of formal 3183 * relationship to the current plan.) 3184 */ 3185 // syntactic sugar 3186 public CarePlanRelatedPlanComponent addRelatedPlan() { // 3 3187 CarePlanRelatedPlanComponent t = new CarePlanRelatedPlanComponent(); 3188 if (this.relatedPlan == null) 3189 this.relatedPlan = new ArrayList<CarePlanRelatedPlanComponent>(); 3190 this.relatedPlan.add(t); 3191 return t; 3192 } 3193 3194 // syntactic sugar 3195 public CarePlan addRelatedPlan(CarePlanRelatedPlanComponent t) { // 3 3196 if (t == null) 3197 return this; 3198 if (this.relatedPlan == null) 3199 this.relatedPlan = new ArrayList<CarePlanRelatedPlanComponent>(); 3200 this.relatedPlan.add(t); 3201 return this; 3202 } 3203 3204 /** 3205 * @return {@link #participant} (Identifies all people and organizations who are 3206 * expected to be involved in the care envisioned by this plan.) 3207 */ 3208 public List<CarePlanParticipantComponent> getParticipant() { 3209 if (this.participant == null) 3210 this.participant = new ArrayList<CarePlanParticipantComponent>(); 3211 return this.participant; 3212 } 3213 3214 public boolean hasParticipant() { 3215 if (this.participant == null) 3216 return false; 3217 for (CarePlanParticipantComponent item : this.participant) 3218 if (!item.isEmpty()) 3219 return true; 3220 return false; 3221 } 3222 3223 /** 3224 * @return {@link #participant} (Identifies all people and organizations who are 3225 * expected to be involved in the care envisioned by this plan.) 3226 */ 3227 // syntactic sugar 3228 public CarePlanParticipantComponent addParticipant() { // 3 3229 CarePlanParticipantComponent t = new CarePlanParticipantComponent(); 3230 if (this.participant == null) 3231 this.participant = new ArrayList<CarePlanParticipantComponent>(); 3232 this.participant.add(t); 3233 return t; 3234 } 3235 3236 // syntactic sugar 3237 public CarePlan addParticipant(CarePlanParticipantComponent t) { // 3 3238 if (t == null) 3239 return this; 3240 if (this.participant == null) 3241 this.participant = new ArrayList<CarePlanParticipantComponent>(); 3242 this.participant.add(t); 3243 return this; 3244 } 3245 3246 /** 3247 * @return {@link #goal} (Describes the intended objective(s) of carrying out 3248 * the care plan.) 3249 */ 3250 public List<Reference> getGoal() { 3251 if (this.goal == null) 3252 this.goal = new ArrayList<Reference>(); 3253 return this.goal; 3254 } 3255 3256 public boolean hasGoal() { 3257 if (this.goal == null) 3258 return false; 3259 for (Reference item : this.goal) 3260 if (!item.isEmpty()) 3261 return true; 3262 return false; 3263 } 3264 3265 /** 3266 * @return {@link #goal} (Describes the intended objective(s) of carrying out 3267 * the care plan.) 3268 */ 3269 // syntactic sugar 3270 public Reference addGoal() { // 3 3271 Reference t = new Reference(); 3272 if (this.goal == null) 3273 this.goal = new ArrayList<Reference>(); 3274 this.goal.add(t); 3275 return t; 3276 } 3277 3278 // syntactic sugar 3279 public CarePlan addGoal(Reference t) { // 3 3280 if (t == null) 3281 return this; 3282 if (this.goal == null) 3283 this.goal = new ArrayList<Reference>(); 3284 this.goal.add(t); 3285 return this; 3286 } 3287 3288 /** 3289 * @return {@link #goal} (The actual objects that are the target of the 3290 * reference. The reference library doesn't populate this, but you can 3291 * use this to hold the resources if you resolvethemt. Describes the 3292 * intended objective(s) of carrying out the care plan.) 3293 */ 3294 public List<Goal> getGoalTarget() { 3295 if (this.goalTarget == null) 3296 this.goalTarget = new ArrayList<Goal>(); 3297 return this.goalTarget; 3298 } 3299 3300 // syntactic sugar 3301 /** 3302 * @return {@link #goal} (Add an actual object that is the target of the 3303 * reference. The reference library doesn't use these, but you can use 3304 * this to hold the resources if you resolvethemt. Describes the 3305 * intended objective(s) of carrying out the care plan.) 3306 */ 3307 public Goal addGoalTarget() { 3308 Goal r = new Goal(); 3309 if (this.goalTarget == null) 3310 this.goalTarget = new ArrayList<Goal>(); 3311 this.goalTarget.add(r); 3312 return r; 3313 } 3314 3315 /** 3316 * @return {@link #activity} (Identifies a planned action to occur as part of 3317 * the plan. For example, a medication to be used, lab tests to perform, 3318 * self-monitoring, education, etc.) 3319 */ 3320 public List<CarePlanActivityComponent> getActivity() { 3321 if (this.activity == null) 3322 this.activity = new ArrayList<CarePlanActivityComponent>(); 3323 return this.activity; 3324 } 3325 3326 public boolean hasActivity() { 3327 if (this.activity == null) 3328 return false; 3329 for (CarePlanActivityComponent item : this.activity) 3330 if (!item.isEmpty()) 3331 return true; 3332 return false; 3333 } 3334 3335 /** 3336 * @return {@link #activity} (Identifies a planned action to occur as part of 3337 * the plan. For example, a medication to be used, lab tests to perform, 3338 * self-monitoring, education, etc.) 3339 */ 3340 // syntactic sugar 3341 public CarePlanActivityComponent addActivity() { // 3 3342 CarePlanActivityComponent t = new CarePlanActivityComponent(); 3343 if (this.activity == null) 3344 this.activity = new ArrayList<CarePlanActivityComponent>(); 3345 this.activity.add(t); 3346 return t; 3347 } 3348 3349 // syntactic sugar 3350 public CarePlan addActivity(CarePlanActivityComponent t) { // 3 3351 if (t == null) 3352 return this; 3353 if (this.activity == null) 3354 this.activity = new ArrayList<CarePlanActivityComponent>(); 3355 this.activity.add(t); 3356 return this; 3357 } 3358 3359 /** 3360 * @return {@link #note} (General notes about the care plan not covered 3361 * elsewhere.) 3362 */ 3363 public Annotation getNote() { 3364 if (this.note == null) 3365 if (Configuration.errorOnAutoCreate()) 3366 throw new Error("Attempt to auto-create CarePlan.note"); 3367 else if (Configuration.doAutoCreate()) 3368 this.note = new Annotation(); // cc 3369 return this.note; 3370 } 3371 3372 public boolean hasNote() { 3373 return this.note != null && !this.note.isEmpty(); 3374 } 3375 3376 /** 3377 * @param value {@link #note} (General notes about the care plan not covered 3378 * elsewhere.) 3379 */ 3380 public CarePlan setNote(Annotation value) { 3381 this.note = value; 3382 return this; 3383 } 3384 3385 protected void listChildren(List<Property> childrenList) { 3386 super.listChildren(childrenList); 3387 childrenList.add(new Property("identifier", "Identifier", 3388 "This records identifiers associated with this care plan that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 3389 0, java.lang.Integer.MAX_VALUE, identifier)); 3390 childrenList.add(new Property("subject", "Reference(Patient|Group)", 3391 "Identifies the patient or group whose intended care is described by the plan.", 0, java.lang.Integer.MAX_VALUE, 3392 subject)); 3393 childrenList.add(new Property("status", "code", 3394 "Indicates whether the plan is currently being acted upon, represents future intentions or is now a historical record.", 3395 0, java.lang.Integer.MAX_VALUE, status)); 3396 childrenList.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", 3397 "Identifies the context in which this particular CarePlan is defined.", 0, java.lang.Integer.MAX_VALUE, 3398 context)); 3399 childrenList.add( 3400 new Property("period", "Period", "Indicates when the plan did (or is intended to) come into effect and end.", 0, 3401 java.lang.Integer.MAX_VALUE, period)); 3402 childrenList.add(new Property("author", "Reference(Patient|Practitioner|RelatedPerson|Organization)", 3403 "Identifies the individual(s) or ogranization who is responsible for the content of the care plan.", 0, 3404 java.lang.Integer.MAX_VALUE, author)); 3405 childrenList 3406 .add(new Property("modified", "dateTime", "Identifies the most recent date on which the plan has been revised.", 3407 0, java.lang.Integer.MAX_VALUE, modified)); 3408 childrenList.add(new Property("category", "CodeableConcept", 3409 "Identifies what \"kind\" of plan this is to support differentiation between multiple co-existing plans; e.g. \"Home health\", \"psychiatric\", \"asthma\", \"disease management\", \"wellness plan\", etc.", 3410 0, java.lang.Integer.MAX_VALUE, category)); 3411 childrenList.add(new Property("description", "string", "A description of the scope and nature of the plan.", 0, 3412 java.lang.Integer.MAX_VALUE, description)); 3413 childrenList.add(new Property("addresses", "Reference(Condition)", 3414 "Identifies the conditions/problems/concerns/diagnoses/etc. whose management and/or mitigation are handled by this plan.", 3415 0, java.lang.Integer.MAX_VALUE, addresses)); 3416 childrenList.add(new Property("support", "Reference(Any)", 3417 "Identifies portions of the patient's record that specifically influenced the formation of the plan. These might include co-morbidities, recent procedures, limitations, recent assessments, etc.", 3418 0, java.lang.Integer.MAX_VALUE, support)); 3419 childrenList.add(new Property("relatedPlan", "", 3420 "Identifies CarePlans with some sort of formal relationship to the current plan.", 0, 3421 java.lang.Integer.MAX_VALUE, relatedPlan)); 3422 childrenList.add(new Property("participant", "", 3423 "Identifies all people and organizations who are expected to be involved in the care envisioned by this plan.", 3424 0, java.lang.Integer.MAX_VALUE, participant)); 3425 childrenList.add(new Property("goal", "Reference(Goal)", 3426 "Describes the intended objective(s) of carrying out the care plan.", 0, java.lang.Integer.MAX_VALUE, goal)); 3427 childrenList.add(new Property("activity", "", 3428 "Identifies a planned action to occur as part of the plan. For example, a medication to be used, lab tests to perform, self-monitoring, education, etc.", 3429 0, java.lang.Integer.MAX_VALUE, activity)); 3430 childrenList.add(new Property("note", "Annotation", "General notes about the care plan not covered elsewhere.", 0, 3431 java.lang.Integer.MAX_VALUE, note)); 3432 } 3433 3434 @Override 3435 public void setProperty(String name, Base value) throws FHIRException { 3436 if (name.equals("identifier")) 3437 this.getIdentifier().add(castToIdentifier(value)); 3438 else if (name.equals("subject")) 3439 this.subject = castToReference(value); // Reference 3440 else if (name.equals("status")) 3441 this.status = new CarePlanStatusEnumFactory().fromType(value); // Enumeration<CarePlanStatus> 3442 else if (name.equals("context")) 3443 this.context = castToReference(value); // Reference 3444 else if (name.equals("period")) 3445 this.period = castToPeriod(value); // Period 3446 else if (name.equals("author")) 3447 this.getAuthor().add(castToReference(value)); 3448 else if (name.equals("modified")) 3449 this.modified = castToDateTime(value); // DateTimeType 3450 else if (name.equals("category")) 3451 this.getCategory().add(castToCodeableConcept(value)); 3452 else if (name.equals("description")) 3453 this.description = castToString(value); // StringType 3454 else if (name.equals("addresses")) 3455 this.getAddresses().add(castToReference(value)); 3456 else if (name.equals("support")) 3457 this.getSupport().add(castToReference(value)); 3458 else if (name.equals("relatedPlan")) 3459 this.getRelatedPlan().add((CarePlanRelatedPlanComponent) value); 3460 else if (name.equals("participant")) 3461 this.getParticipant().add((CarePlanParticipantComponent) value); 3462 else if (name.equals("goal")) 3463 this.getGoal().add(castToReference(value)); 3464 else if (name.equals("activity")) 3465 this.getActivity().add((CarePlanActivityComponent) value); 3466 else if (name.equals("note")) 3467 this.note = castToAnnotation(value); // Annotation 3468 else 3469 super.setProperty(name, value); 3470 } 3471 3472 @Override 3473 public Base addChild(String name) throws FHIRException { 3474 if (name.equals("identifier")) { 3475 return addIdentifier(); 3476 } else if (name.equals("subject")) { 3477 this.subject = new Reference(); 3478 return this.subject; 3479 } else if (name.equals("status")) { 3480 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.status"); 3481 } else if (name.equals("context")) { 3482 this.context = new Reference(); 3483 return this.context; 3484 } else if (name.equals("period")) { 3485 this.period = new Period(); 3486 return this.period; 3487 } else if (name.equals("author")) { 3488 return addAuthor(); 3489 } else if (name.equals("modified")) { 3490 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.modified"); 3491 } else if (name.equals("category")) { 3492 return addCategory(); 3493 } else if (name.equals("description")) { 3494 throw new FHIRException("Cannot call addChild on a singleton property CarePlan.description"); 3495 } else if (name.equals("addresses")) { 3496 return addAddresses(); 3497 } else if (name.equals("support")) { 3498 return addSupport(); 3499 } else if (name.equals("relatedPlan")) { 3500 return addRelatedPlan(); 3501 } else if (name.equals("participant")) { 3502 return addParticipant(); 3503 } else if (name.equals("goal")) { 3504 return addGoal(); 3505 } else if (name.equals("activity")) { 3506 return addActivity(); 3507 } else if (name.equals("note")) { 3508 this.note = new Annotation(); 3509 return this.note; 3510 } else 3511 return super.addChild(name); 3512 } 3513 3514 public String fhirType() { 3515 return "CarePlan"; 3516 3517 } 3518 3519 public CarePlan copy() { 3520 CarePlan dst = new CarePlan(); 3521 copyValues(dst); 3522 if (identifier != null) { 3523 dst.identifier = new ArrayList<Identifier>(); 3524 for (Identifier i : identifier) 3525 dst.identifier.add(i.copy()); 3526 } 3527 ; 3528 dst.subject = subject == null ? null : subject.copy(); 3529 dst.status = status == null ? null : status.copy(); 3530 dst.context = context == null ? null : context.copy(); 3531 dst.period = period == null ? null : period.copy(); 3532 if (author != null) { 3533 dst.author = new ArrayList<Reference>(); 3534 for (Reference i : author) 3535 dst.author.add(i.copy()); 3536 } 3537 ; 3538 dst.modified = modified == null ? null : modified.copy(); 3539 if (category != null) { 3540 dst.category = new ArrayList<CodeableConcept>(); 3541 for (CodeableConcept i : category) 3542 dst.category.add(i.copy()); 3543 } 3544 ; 3545 dst.description = description == null ? null : description.copy(); 3546 if (addresses != null) { 3547 dst.addresses = new ArrayList<Reference>(); 3548 for (Reference i : addresses) 3549 dst.addresses.add(i.copy()); 3550 } 3551 ; 3552 if (support != null) { 3553 dst.support = new ArrayList<Reference>(); 3554 for (Reference i : support) 3555 dst.support.add(i.copy()); 3556 } 3557 ; 3558 if (relatedPlan != null) { 3559 dst.relatedPlan = new ArrayList<CarePlanRelatedPlanComponent>(); 3560 for (CarePlanRelatedPlanComponent i : relatedPlan) 3561 dst.relatedPlan.add(i.copy()); 3562 } 3563 ; 3564 if (participant != null) { 3565 dst.participant = new ArrayList<CarePlanParticipantComponent>(); 3566 for (CarePlanParticipantComponent i : participant) 3567 dst.participant.add(i.copy()); 3568 } 3569 ; 3570 if (goal != null) { 3571 dst.goal = new ArrayList<Reference>(); 3572 for (Reference i : goal) 3573 dst.goal.add(i.copy()); 3574 } 3575 ; 3576 if (activity != null) { 3577 dst.activity = new ArrayList<CarePlanActivityComponent>(); 3578 for (CarePlanActivityComponent i : activity) 3579 dst.activity.add(i.copy()); 3580 } 3581 ; 3582 dst.note = note == null ? null : note.copy(); 3583 return dst; 3584 } 3585 3586 protected CarePlan typedCopy() { 3587 return copy(); 3588 } 3589 3590 @Override 3591 public boolean equalsDeep(Base other) { 3592 if (!super.equalsDeep(other)) 3593 return false; 3594 if (!(other instanceof CarePlan)) 3595 return false; 3596 CarePlan o = (CarePlan) other; 3597 return compareDeep(identifier, o.identifier, true) && compareDeep(subject, o.subject, true) 3598 && compareDeep(status, o.status, true) && compareDeep(context, o.context, true) 3599 && compareDeep(period, o.period, true) && compareDeep(author, o.author, true) 3600 && compareDeep(modified, o.modified, true) && compareDeep(category, o.category, true) 3601 && compareDeep(description, o.description, true) && compareDeep(addresses, o.addresses, true) 3602 && compareDeep(support, o.support, true) && compareDeep(relatedPlan, o.relatedPlan, true) 3603 && compareDeep(participant, o.participant, true) && compareDeep(goal, o.goal, true) 3604 && compareDeep(activity, o.activity, true) && compareDeep(note, o.note, true); 3605 } 3606 3607 @Override 3608 public boolean equalsShallow(Base other) { 3609 if (!super.equalsShallow(other)) 3610 return false; 3611 if (!(other instanceof CarePlan)) 3612 return false; 3613 CarePlan o = (CarePlan) other; 3614 return compareValues(status, o.status, true) && compareValues(modified, o.modified, true) 3615 && compareValues(description, o.description, true); 3616 } 3617 3618 public boolean isEmpty() { 3619 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (subject == null || subject.isEmpty()) 3620 && (status == null || status.isEmpty()) && (context == null || context.isEmpty()) 3621 && (period == null || period.isEmpty()) && (author == null || author.isEmpty()) 3622 && (modified == null || modified.isEmpty()) && (category == null || category.isEmpty()) 3623 && (description == null || description.isEmpty()) && (addresses == null || addresses.isEmpty()) 3624 && (support == null || support.isEmpty()) && (relatedPlan == null || relatedPlan.isEmpty()) 3625 && (participant == null || participant.isEmpty()) && (goal == null || goal.isEmpty()) 3626 && (activity == null || activity.isEmpty()) && (note == null || note.isEmpty()); 3627 } 3628 3629 @Override 3630 public ResourceType getResourceType() { 3631 return ResourceType.CarePlan; 3632 } 3633 3634 @SearchParamDefinition(name = "date", path = "CarePlan.period", description = "Time period plan covers", type = "date") 3635 public static final String SP_DATE = "date"; 3636 @SearchParamDefinition(name = "activitycode", path = "CarePlan.activity.detail.code", description = "Detail type of activity", type = "token") 3637 public static final String SP_ACTIVITYCODE = "activitycode"; 3638 @SearchParamDefinition(name = "activitydate", path = "CarePlan.activity.detail.scheduled[x]", description = "Specified date occurs within period specified by CarePlan.activity.timingSchedule", type = "date") 3639 public static final String SP_ACTIVITYDATE = "activitydate"; 3640 @SearchParamDefinition(name = "activityreference", path = "CarePlan.activity.reference", description = "Activity details defined in specific resource", type = "reference") 3641 public static final String SP_ACTIVITYREFERENCE = "activityreference"; 3642 @SearchParamDefinition(name = "performer", path = "CarePlan.activity.detail.performer", description = "Matches if the practitioner is listed as a performer in any of the \"simple\" activities. (For performers of the detailed activities, chain through the activitydetail search parameter.)", type = "reference") 3643 public static final String SP_PERFORMER = "performer"; 3644 @SearchParamDefinition(name = "goal", path = "CarePlan.goal", description = "Desired outcome of plan", type = "reference") 3645 public static final String SP_GOAL = "goal"; 3646 @SearchParamDefinition(name = "subject", path = "CarePlan.subject", description = "Who care plan is for", type = "reference") 3647 public static final String SP_SUBJECT = "subject"; 3648 @SearchParamDefinition(name = "relatedcode", path = "CarePlan.relatedPlan.code", description = "includes | replaces | fulfills", type = "token") 3649 public static final String SP_RELATEDCODE = "relatedcode"; 3650 @SearchParamDefinition(name = "participant", path = "CarePlan.participant.member", description = "Who is involved", type = "reference") 3651 public static final String SP_PARTICIPANT = "participant"; 3652 @SearchParamDefinition(name = "relatedplan", path = "CarePlan.relatedPlan.plan", description = "Plan relationship exists with", type = "reference") 3653 public static final String SP_RELATEDPLAN = "relatedplan"; 3654 @SearchParamDefinition(name = "condition", path = "CarePlan.addresses", description = "Health issues this plan addresses", type = "reference") 3655 public static final String SP_CONDITION = "condition"; 3656 @SearchParamDefinition(name = "related", path = "null", description = "A combination of the type of relationship and the related plan", type = "composite") 3657 public static final String SP_RELATED = "related"; 3658 @SearchParamDefinition(name = "patient", path = "CarePlan.subject", description = "Who care plan is for", type = "reference") 3659 public static final String SP_PATIENT = "patient"; 3660 3661}