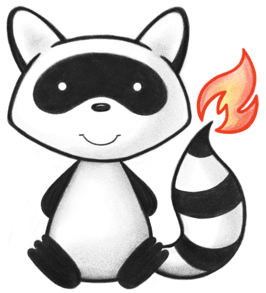
001package org.hl7.fhir.dstu2.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 045import org.hl7.fhir.exceptions.FHIRException; 046import org.hl7.fhir.utilities.Utilities; 047 048/** 049 * A provider issued list of services and products provided, or to be provided, 050 * to a patient which is provided to an insurer for payment recovery. 051 */ 052@ResourceDef(name = "Claim", profile = "http://hl7.org/fhir/Profile/Claim") 053public class Claim extends DomainResource { 054 055 public enum ClaimType { 056 /** 057 * A claim for Institution based, typically in-patient, goods and services. 058 */ 059 INSTITUTIONAL, 060 /** 061 * A claim for Oral Health (Dentist, Denturist, Hygienist) goods and services. 062 */ 063 ORAL, 064 /** 065 * A claim for Pharmacy based goods and services. 066 */ 067 PHARMACY, 068 /** 069 * A claim for Professional, typically out-patient, goods and services. 070 */ 071 PROFESSIONAL, 072 /** 073 * A claim for Vision (Ophthamologist, Optometrist and Optician) goods and 074 * services. 075 */ 076 VISION, 077 /** 078 * added to help the parsers 079 */ 080 NULL; 081 082 public static ClaimType fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("institutional".equals(codeString)) 086 return INSTITUTIONAL; 087 if ("oral".equals(codeString)) 088 return ORAL; 089 if ("pharmacy".equals(codeString)) 090 return PHARMACY; 091 if ("professional".equals(codeString)) 092 return PROFESSIONAL; 093 if ("vision".equals(codeString)) 094 return VISION; 095 throw new FHIRException("Unknown ClaimType code '" + codeString + "'"); 096 } 097 098 public String toCode() { 099 switch (this) { 100 case INSTITUTIONAL: 101 return "institutional"; 102 case ORAL: 103 return "oral"; 104 case PHARMACY: 105 return "pharmacy"; 106 case PROFESSIONAL: 107 return "professional"; 108 case VISION: 109 return "vision"; 110 case NULL: 111 return null; 112 default: 113 return "?"; 114 } 115 } 116 117 public String getSystem() { 118 switch (this) { 119 case INSTITUTIONAL: 120 return "http://hl7.org/fhir/claim-type-link"; 121 case ORAL: 122 return "http://hl7.org/fhir/claim-type-link"; 123 case PHARMACY: 124 return "http://hl7.org/fhir/claim-type-link"; 125 case PROFESSIONAL: 126 return "http://hl7.org/fhir/claim-type-link"; 127 case VISION: 128 return "http://hl7.org/fhir/claim-type-link"; 129 case NULL: 130 return null; 131 default: 132 return "?"; 133 } 134 } 135 136 public String getDefinition() { 137 switch (this) { 138 case INSTITUTIONAL: 139 return "A claim for Institution based, typically in-patient, goods and services."; 140 case ORAL: 141 return "A claim for Oral Health (Dentist, Denturist, Hygienist) goods and services."; 142 case PHARMACY: 143 return "A claim for Pharmacy based goods and services."; 144 case PROFESSIONAL: 145 return "A claim for Professional, typically out-patient, goods and services."; 146 case VISION: 147 return "A claim for Vision (Ophthamologist, Optometrist and Optician) goods and services."; 148 case NULL: 149 return null; 150 default: 151 return "?"; 152 } 153 } 154 155 public String getDisplay() { 156 switch (this) { 157 case INSTITUTIONAL: 158 return "Institutional"; 159 case ORAL: 160 return "Oral Health"; 161 case PHARMACY: 162 return "Pharmacy"; 163 case PROFESSIONAL: 164 return "Professional"; 165 case VISION: 166 return "Vision"; 167 case NULL: 168 return null; 169 default: 170 return "?"; 171 } 172 } 173 } 174 175 public static class ClaimTypeEnumFactory implements EnumFactory<ClaimType> { 176 public ClaimType fromCode(String codeString) throws IllegalArgumentException { 177 if (codeString == null || "".equals(codeString)) 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("institutional".equals(codeString)) 181 return ClaimType.INSTITUTIONAL; 182 if ("oral".equals(codeString)) 183 return ClaimType.ORAL; 184 if ("pharmacy".equals(codeString)) 185 return ClaimType.PHARMACY; 186 if ("professional".equals(codeString)) 187 return ClaimType.PROFESSIONAL; 188 if ("vision".equals(codeString)) 189 return ClaimType.VISION; 190 throw new IllegalArgumentException("Unknown ClaimType code '" + codeString + "'"); 191 } 192 193 public Enumeration<ClaimType> fromType(Base code) throws FHIRException { 194 if (code == null || code.isEmpty()) 195 return null; 196 String codeString = ((PrimitiveType) code).asStringValue(); 197 if (codeString == null || "".equals(codeString)) 198 return null; 199 if ("institutional".equals(codeString)) 200 return new Enumeration<ClaimType>(this, ClaimType.INSTITUTIONAL); 201 if ("oral".equals(codeString)) 202 return new Enumeration<ClaimType>(this, ClaimType.ORAL); 203 if ("pharmacy".equals(codeString)) 204 return new Enumeration<ClaimType>(this, ClaimType.PHARMACY); 205 if ("professional".equals(codeString)) 206 return new Enumeration<ClaimType>(this, ClaimType.PROFESSIONAL); 207 if ("vision".equals(codeString)) 208 return new Enumeration<ClaimType>(this, ClaimType.VISION); 209 throw new FHIRException("Unknown ClaimType code '" + codeString + "'"); 210 } 211 212 public String toCode(ClaimType code) 213 { 214 if (code == ClaimType.NULL) 215 return null; 216 if (code == ClaimType.INSTITUTIONAL) 217 return "institutional"; 218 if (code == ClaimType.ORAL) 219 return "oral"; 220 if (code == ClaimType.PHARMACY) 221 return "pharmacy"; 222 if (code == ClaimType.PROFESSIONAL) 223 return "professional"; 224 if (code == ClaimType.VISION) 225 return "vision"; 226 return "?"; 227 } 228 } 229 230 public enum Use { 231 /** 232 * The treatment is complete and this represents a Claim for the services. 233 */ 234 COMPLETE, 235 /** 236 * The treatment is proposed and this represents a Pre-authorization for the 237 * services. 238 */ 239 PROPOSED, 240 /** 241 * The treatment is proposed and this represents a Pre-determination for the 242 * services. 243 */ 244 EXPLORATORY, 245 /** 246 * A locally defined or otherwise resolved status. 247 */ 248 OTHER, 249 /** 250 * added to help the parsers 251 */ 252 NULL; 253 254 public static Use fromCode(String codeString) throws FHIRException { 255 if (codeString == null || "".equals(codeString)) 256 return null; 257 if ("complete".equals(codeString)) 258 return COMPLETE; 259 if ("proposed".equals(codeString)) 260 return PROPOSED; 261 if ("exploratory".equals(codeString)) 262 return EXPLORATORY; 263 if ("other".equals(codeString)) 264 return OTHER; 265 throw new FHIRException("Unknown Use code '" + codeString + "'"); 266 } 267 268 public String toCode() { 269 switch (this) { 270 case COMPLETE: 271 return "complete"; 272 case PROPOSED: 273 return "proposed"; 274 case EXPLORATORY: 275 return "exploratory"; 276 case OTHER: 277 return "other"; 278 case NULL: 279 return null; 280 default: 281 return "?"; 282 } 283 } 284 285 public String getSystem() { 286 switch (this) { 287 case COMPLETE: 288 return "http://hl7.org/fhir/claim-use-link"; 289 case PROPOSED: 290 return "http://hl7.org/fhir/claim-use-link"; 291 case EXPLORATORY: 292 return "http://hl7.org/fhir/claim-use-link"; 293 case OTHER: 294 return "http://hl7.org/fhir/claim-use-link"; 295 case NULL: 296 return null; 297 default: 298 return "?"; 299 } 300 } 301 302 public String getDefinition() { 303 switch (this) { 304 case COMPLETE: 305 return "The treatment is complete and this represents a Claim for the services."; 306 case PROPOSED: 307 return "The treatment is proposed and this represents a Pre-authorization for the services."; 308 case EXPLORATORY: 309 return "The treatment is proposed and this represents a Pre-determination for the services."; 310 case OTHER: 311 return "A locally defined or otherwise resolved status."; 312 case NULL: 313 return null; 314 default: 315 return "?"; 316 } 317 } 318 319 public String getDisplay() { 320 switch (this) { 321 case COMPLETE: 322 return "Complete"; 323 case PROPOSED: 324 return "Proposed"; 325 case EXPLORATORY: 326 return "Exploratory"; 327 case OTHER: 328 return "Other"; 329 case NULL: 330 return null; 331 default: 332 return "?"; 333 } 334 } 335 } 336 337 public static class UseEnumFactory implements EnumFactory<Use> { 338 public Use fromCode(String codeString) throws IllegalArgumentException { 339 if (codeString == null || "".equals(codeString)) 340 if (codeString == null || "".equals(codeString)) 341 return null; 342 if ("complete".equals(codeString)) 343 return Use.COMPLETE; 344 if ("proposed".equals(codeString)) 345 return Use.PROPOSED; 346 if ("exploratory".equals(codeString)) 347 return Use.EXPLORATORY; 348 if ("other".equals(codeString)) 349 return Use.OTHER; 350 throw new IllegalArgumentException("Unknown Use code '" + codeString + "'"); 351 } 352 353 public Enumeration<Use> fromType(Base code) throws FHIRException { 354 if (code == null || code.isEmpty()) 355 return null; 356 String codeString = ((PrimitiveType) code).asStringValue(); 357 if (codeString == null || "".equals(codeString)) 358 return null; 359 if ("complete".equals(codeString)) 360 return new Enumeration<Use>(this, Use.COMPLETE); 361 if ("proposed".equals(codeString)) 362 return new Enumeration<Use>(this, Use.PROPOSED); 363 if ("exploratory".equals(codeString)) 364 return new Enumeration<Use>(this, Use.EXPLORATORY); 365 if ("other".equals(codeString)) 366 return new Enumeration<Use>(this, Use.OTHER); 367 throw new FHIRException("Unknown Use code '" + codeString + "'"); 368 } 369 370 public String toCode(Use code) 371 { 372 if (code == Use.NULL) 373 return null; 374 if (code == Use.COMPLETE) 375 return "complete"; 376 if (code == Use.PROPOSED) 377 return "proposed"; 378 if (code == Use.EXPLORATORY) 379 return "exploratory"; 380 if (code == Use.OTHER) 381 return "other"; 382 return "?"; 383 } 384 } 385 386 @Block() 387 public static class PayeeComponent extends BackboneElement implements IBaseBackboneElement { 388 /** 389 * Party to be reimbursed: Subscriber, provider, other. 390 */ 391 @Child(name = "type", type = { Coding.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 392 @Description(shortDefinition = "Party to be paid any benefits payable", formalDefinition = "Party to be reimbursed: Subscriber, provider, other.") 393 protected Coding type; 394 395 /** 396 * The provider who is to be reimbursed for the claim (the party to whom any 397 * benefit is assigned). 398 */ 399 @Child(name = "provider", type = { 400 Practitioner.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 401 @Description(shortDefinition = "Provider who is the payee", formalDefinition = "The provider who is to be reimbursed for the claim (the party to whom any benefit is assigned).") 402 protected Reference provider; 403 404 /** 405 * The actual object that is the target of the reference (The provider who is to 406 * be reimbursed for the claim (the party to whom any benefit is assigned).) 407 */ 408 protected Practitioner providerTarget; 409 410 /** 411 * The organization who is to be reimbursed for the claim (the party to whom any 412 * benefit is assigned). 413 */ 414 @Child(name = "organization", type = { 415 Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 416 @Description(shortDefinition = "Organization who is the payee", formalDefinition = "The organization who is to be reimbursed for the claim (the party to whom any benefit is assigned).") 417 protected Reference organization; 418 419 /** 420 * The actual object that is the target of the reference (The organization who 421 * is to be reimbursed for the claim (the party to whom any benefit is 422 * assigned).) 423 */ 424 protected Organization organizationTarget; 425 426 /** 427 * The person other than the subscriber who is to be reimbursed for the claim 428 * (the party to whom any benefit is assigned). 429 */ 430 @Child(name = "person", type = { Patient.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 431 @Description(shortDefinition = "Other person who is the payee", formalDefinition = "The person other than the subscriber who is to be reimbursed for the claim (the party to whom any benefit is assigned).") 432 protected Reference person; 433 434 /** 435 * The actual object that is the target of the reference (The person other than 436 * the subscriber who is to be reimbursed for the claim (the party to whom any 437 * benefit is assigned).) 438 */ 439 protected Patient personTarget; 440 441 private static final long serialVersionUID = -503108488L; 442 443 /* 444 * Constructor 445 */ 446 public PayeeComponent() { 447 super(); 448 } 449 450 /** 451 * @return {@link #type} (Party to be reimbursed: Subscriber, provider, other.) 452 */ 453 public Coding getType() { 454 if (this.type == null) 455 if (Configuration.errorOnAutoCreate()) 456 throw new Error("Attempt to auto-create PayeeComponent.type"); 457 else if (Configuration.doAutoCreate()) 458 this.type = new Coding(); // cc 459 return this.type; 460 } 461 462 public boolean hasType() { 463 return this.type != null && !this.type.isEmpty(); 464 } 465 466 /** 467 * @param value {@link #type} (Party to be reimbursed: Subscriber, provider, 468 * other.) 469 */ 470 public PayeeComponent setType(Coding value) { 471 this.type = value; 472 return this; 473 } 474 475 /** 476 * @return {@link #provider} (The provider who is to be reimbursed for the claim 477 * (the party to whom any benefit is assigned).) 478 */ 479 public Reference getProvider() { 480 if (this.provider == null) 481 if (Configuration.errorOnAutoCreate()) 482 throw new Error("Attempt to auto-create PayeeComponent.provider"); 483 else if (Configuration.doAutoCreate()) 484 this.provider = new Reference(); // cc 485 return this.provider; 486 } 487 488 public boolean hasProvider() { 489 return this.provider != null && !this.provider.isEmpty(); 490 } 491 492 /** 493 * @param value {@link #provider} (The provider who is to be reimbursed for the 494 * claim (the party to whom any benefit is assigned).) 495 */ 496 public PayeeComponent setProvider(Reference value) { 497 this.provider = value; 498 return this; 499 } 500 501 /** 502 * @return {@link #provider} The actual object that is the target of the 503 * reference. The reference library doesn't populate this, but you can 504 * use it to hold the resource if you resolve it. (The provider who is 505 * to be reimbursed for the claim (the party to whom any benefit is 506 * assigned).) 507 */ 508 public Practitioner getProviderTarget() { 509 if (this.providerTarget == null) 510 if (Configuration.errorOnAutoCreate()) 511 throw new Error("Attempt to auto-create PayeeComponent.provider"); 512 else if (Configuration.doAutoCreate()) 513 this.providerTarget = new Practitioner(); // aa 514 return this.providerTarget; 515 } 516 517 /** 518 * @param value {@link #provider} The actual object that is the target of the 519 * reference. The reference library doesn't use these, but you can 520 * use it to hold the resource if you resolve it. (The provider who 521 * is to be reimbursed for the claim (the party to whom any benefit 522 * is assigned).) 523 */ 524 public PayeeComponent setProviderTarget(Practitioner value) { 525 this.providerTarget = value; 526 return this; 527 } 528 529 /** 530 * @return {@link #organization} (The organization who is to be reimbursed for 531 * the claim (the party to whom any benefit is assigned).) 532 */ 533 public Reference getOrganization() { 534 if (this.organization == null) 535 if (Configuration.errorOnAutoCreate()) 536 throw new Error("Attempt to auto-create PayeeComponent.organization"); 537 else if (Configuration.doAutoCreate()) 538 this.organization = new Reference(); // cc 539 return this.organization; 540 } 541 542 public boolean hasOrganization() { 543 return this.organization != null && !this.organization.isEmpty(); 544 } 545 546 /** 547 * @param value {@link #organization} (The organization who is to be reimbursed 548 * for the claim (the party to whom any benefit is assigned).) 549 */ 550 public PayeeComponent setOrganization(Reference value) { 551 this.organization = value; 552 return this; 553 } 554 555 /** 556 * @return {@link #organization} The actual object that is the target of the 557 * reference. The reference library doesn't populate this, but you can 558 * use it to hold the resource if you resolve it. (The organization who 559 * is to be reimbursed for the claim (the party to whom any benefit is 560 * assigned).) 561 */ 562 public Organization getOrganizationTarget() { 563 if (this.organizationTarget == null) 564 if (Configuration.errorOnAutoCreate()) 565 throw new Error("Attempt to auto-create PayeeComponent.organization"); 566 else if (Configuration.doAutoCreate()) 567 this.organizationTarget = new Organization(); // aa 568 return this.organizationTarget; 569 } 570 571 /** 572 * @param value {@link #organization} The actual object that is the target of 573 * the reference. The reference library doesn't use these, but you 574 * can use it to hold the resource if you resolve it. (The 575 * organization who is to be reimbursed for the claim (the party to 576 * whom any benefit is assigned).) 577 */ 578 public PayeeComponent setOrganizationTarget(Organization value) { 579 this.organizationTarget = value; 580 return this; 581 } 582 583 /** 584 * @return {@link #person} (The person other than the subscriber who is to be 585 * reimbursed for the claim (the party to whom any benefit is 586 * assigned).) 587 */ 588 public Reference getPerson() { 589 if (this.person == null) 590 if (Configuration.errorOnAutoCreate()) 591 throw new Error("Attempt to auto-create PayeeComponent.person"); 592 else if (Configuration.doAutoCreate()) 593 this.person = new Reference(); // cc 594 return this.person; 595 } 596 597 public boolean hasPerson() { 598 return this.person != null && !this.person.isEmpty(); 599 } 600 601 /** 602 * @param value {@link #person} (The person other than the subscriber who is to 603 * be reimbursed for the claim (the party to whom any benefit is 604 * assigned).) 605 */ 606 public PayeeComponent setPerson(Reference value) { 607 this.person = value; 608 return this; 609 } 610 611 /** 612 * @return {@link #person} The actual object that is the target of the 613 * reference. The reference library doesn't populate this, but you can 614 * use it to hold the resource if you resolve it. (The person other than 615 * the subscriber who is to be reimbursed for the claim (the party to 616 * whom any benefit is assigned).) 617 */ 618 public Patient getPersonTarget() { 619 if (this.personTarget == null) 620 if (Configuration.errorOnAutoCreate()) 621 throw new Error("Attempt to auto-create PayeeComponent.person"); 622 else if (Configuration.doAutoCreate()) 623 this.personTarget = new Patient(); // aa 624 return this.personTarget; 625 } 626 627 /** 628 * @param value {@link #person} The actual object that is the target of the 629 * reference. The reference library doesn't use these, but you can 630 * use it to hold the resource if you resolve it. (The person other 631 * than the subscriber who is to be reimbursed for the claim (the 632 * party to whom any benefit is assigned).) 633 */ 634 public PayeeComponent setPersonTarget(Patient value) { 635 this.personTarget = value; 636 return this; 637 } 638 639 protected void listChildren(List<Property> childrenList) { 640 super.listChildren(childrenList); 641 childrenList.add(new Property("type", "Coding", "Party to be reimbursed: Subscriber, provider, other.", 0, 642 java.lang.Integer.MAX_VALUE, type)); 643 childrenList.add(new Property("provider", "Reference(Practitioner)", 644 "The provider who is to be reimbursed for the claim (the party to whom any benefit is assigned).", 0, 645 java.lang.Integer.MAX_VALUE, provider)); 646 childrenList.add(new Property("organization", "Reference(Organization)", 647 "The organization who is to be reimbursed for the claim (the party to whom any benefit is assigned).", 0, 648 java.lang.Integer.MAX_VALUE, organization)); 649 childrenList.add(new Property("person", "Reference(Patient)", 650 "The person other than the subscriber who is to be reimbursed for the claim (the party to whom any benefit is assigned).", 651 0, java.lang.Integer.MAX_VALUE, person)); 652 } 653 654 @Override 655 public void setProperty(String name, Base value) throws FHIRException { 656 if (name.equals("type")) 657 this.type = castToCoding(value); // Coding 658 else if (name.equals("provider")) 659 this.provider = castToReference(value); // Reference 660 else if (name.equals("organization")) 661 this.organization = castToReference(value); // Reference 662 else if (name.equals("person")) 663 this.person = castToReference(value); // Reference 664 else 665 super.setProperty(name, value); 666 } 667 668 @Override 669 public Base addChild(String name) throws FHIRException { 670 if (name.equals("type")) { 671 this.type = new Coding(); 672 return this.type; 673 } else if (name.equals("provider")) { 674 this.provider = new Reference(); 675 return this.provider; 676 } else if (name.equals("organization")) { 677 this.organization = new Reference(); 678 return this.organization; 679 } else if (name.equals("person")) { 680 this.person = new Reference(); 681 return this.person; 682 } else 683 return super.addChild(name); 684 } 685 686 public PayeeComponent copy() { 687 PayeeComponent dst = new PayeeComponent(); 688 copyValues(dst); 689 dst.type = type == null ? null : type.copy(); 690 dst.provider = provider == null ? null : provider.copy(); 691 dst.organization = organization == null ? null : organization.copy(); 692 dst.person = person == null ? null : person.copy(); 693 return dst; 694 } 695 696 @Override 697 public boolean equalsDeep(Base other) { 698 if (!super.equalsDeep(other)) 699 return false; 700 if (!(other instanceof PayeeComponent)) 701 return false; 702 PayeeComponent o = (PayeeComponent) other; 703 return compareDeep(type, o.type, true) && compareDeep(provider, o.provider, true) 704 && compareDeep(organization, o.organization, true) && compareDeep(person, o.person, true); 705 } 706 707 @Override 708 public boolean equalsShallow(Base other) { 709 if (!super.equalsShallow(other)) 710 return false; 711 if (!(other instanceof PayeeComponent)) 712 return false; 713 PayeeComponent o = (PayeeComponent) other; 714 return true; 715 } 716 717 public boolean isEmpty() { 718 return super.isEmpty() && (type == null || type.isEmpty()) && (provider == null || provider.isEmpty()) 719 && (organization == null || organization.isEmpty()) && (person == null || person.isEmpty()); 720 } 721 722 public String fhirType() { 723 return "Claim.payee"; 724 725 } 726 727 } 728 729 @Block() 730 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 731 /** 732 * Sequence of diagnosis which serves to order and provide a link. 733 */ 734 @Child(name = "sequence", type = { 735 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 736 @Description(shortDefinition = "Sequence of diagnosis", formalDefinition = "Sequence of diagnosis which serves to order and provide a link.") 737 protected PositiveIntType sequence; 738 739 /** 740 * The diagnosis. 741 */ 742 @Child(name = "diagnosis", type = { Coding.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 743 @Description(shortDefinition = "Patient's list of diagnosis", formalDefinition = "The diagnosis.") 744 protected Coding diagnosis; 745 746 private static final long serialVersionUID = -795010186L; 747 748 /* 749 * Constructor 750 */ 751 public DiagnosisComponent() { 752 super(); 753 } 754 755 /* 756 * Constructor 757 */ 758 public DiagnosisComponent(PositiveIntType sequence, Coding diagnosis) { 759 super(); 760 this.sequence = sequence; 761 this.diagnosis = diagnosis; 762 } 763 764 /** 765 * @return {@link #sequence} (Sequence of diagnosis which serves to order and 766 * provide a link.). This is the underlying object with id, value and 767 * extensions. The accessor "getSequence" gives direct access to the 768 * value 769 */ 770 public PositiveIntType getSequenceElement() { 771 if (this.sequence == null) 772 if (Configuration.errorOnAutoCreate()) 773 throw new Error("Attempt to auto-create DiagnosisComponent.sequence"); 774 else if (Configuration.doAutoCreate()) 775 this.sequence = new PositiveIntType(); // bb 776 return this.sequence; 777 } 778 779 public boolean hasSequenceElement() { 780 return this.sequence != null && !this.sequence.isEmpty(); 781 } 782 783 public boolean hasSequence() { 784 return this.sequence != null && !this.sequence.isEmpty(); 785 } 786 787 /** 788 * @param value {@link #sequence} (Sequence of diagnosis which serves to order 789 * and provide a link.). This is the underlying object with id, 790 * value and extensions. The accessor "getSequence" gives direct 791 * access to the value 792 */ 793 public DiagnosisComponent setSequenceElement(PositiveIntType value) { 794 this.sequence = value; 795 return this; 796 } 797 798 /** 799 * @return Sequence of diagnosis which serves to order and provide a link. 800 */ 801 public int getSequence() { 802 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 803 } 804 805 /** 806 * @param value Sequence of diagnosis which serves to order and provide a link. 807 */ 808 public DiagnosisComponent setSequence(int value) { 809 if (this.sequence == null) 810 this.sequence = new PositiveIntType(); 811 this.sequence.setValue(value); 812 return this; 813 } 814 815 /** 816 * @return {@link #diagnosis} (The diagnosis.) 817 */ 818 public Coding getDiagnosis() { 819 if (this.diagnosis == null) 820 if (Configuration.errorOnAutoCreate()) 821 throw new Error("Attempt to auto-create DiagnosisComponent.diagnosis"); 822 else if (Configuration.doAutoCreate()) 823 this.diagnosis = new Coding(); // cc 824 return this.diagnosis; 825 } 826 827 public boolean hasDiagnosis() { 828 return this.diagnosis != null && !this.diagnosis.isEmpty(); 829 } 830 831 /** 832 * @param value {@link #diagnosis} (The diagnosis.) 833 */ 834 public DiagnosisComponent setDiagnosis(Coding value) { 835 this.diagnosis = value; 836 return this; 837 } 838 839 protected void listChildren(List<Property> childrenList) { 840 super.listChildren(childrenList); 841 childrenList.add(new Property("sequence", "positiveInt", 842 "Sequence of diagnosis which serves to order and provide a link.", 0, java.lang.Integer.MAX_VALUE, sequence)); 843 childrenList 844 .add(new Property("diagnosis", "Coding", "The diagnosis.", 0, java.lang.Integer.MAX_VALUE, diagnosis)); 845 } 846 847 @Override 848 public void setProperty(String name, Base value) throws FHIRException { 849 if (name.equals("sequence")) 850 this.sequence = castToPositiveInt(value); // PositiveIntType 851 else if (name.equals("diagnosis")) 852 this.diagnosis = castToCoding(value); // Coding 853 else 854 super.setProperty(name, value); 855 } 856 857 @Override 858 public Base addChild(String name) throws FHIRException { 859 if (name.equals("sequence")) { 860 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 861 } else if (name.equals("diagnosis")) { 862 this.diagnosis = new Coding(); 863 return this.diagnosis; 864 } else 865 return super.addChild(name); 866 } 867 868 public DiagnosisComponent copy() { 869 DiagnosisComponent dst = new DiagnosisComponent(); 870 copyValues(dst); 871 dst.sequence = sequence == null ? null : sequence.copy(); 872 dst.diagnosis = diagnosis == null ? null : diagnosis.copy(); 873 return dst; 874 } 875 876 @Override 877 public boolean equalsDeep(Base other) { 878 if (!super.equalsDeep(other)) 879 return false; 880 if (!(other instanceof DiagnosisComponent)) 881 return false; 882 DiagnosisComponent o = (DiagnosisComponent) other; 883 return compareDeep(sequence, o.sequence, true) && compareDeep(diagnosis, o.diagnosis, true); 884 } 885 886 @Override 887 public boolean equalsShallow(Base other) { 888 if (!super.equalsShallow(other)) 889 return false; 890 if (!(other instanceof DiagnosisComponent)) 891 return false; 892 DiagnosisComponent o = (DiagnosisComponent) other; 893 return compareValues(sequence, o.sequence, true); 894 } 895 896 public boolean isEmpty() { 897 return super.isEmpty() && (sequence == null || sequence.isEmpty()) && (diagnosis == null || diagnosis.isEmpty()); 898 } 899 900 public String fhirType() { 901 return "Claim.diagnosis"; 902 903 } 904 905 } 906 907 @Block() 908 public static class CoverageComponent extends BackboneElement implements IBaseBackboneElement { 909 /** 910 * A service line item. 911 */ 912 @Child(name = "sequence", type = { 913 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 914 @Description(shortDefinition = "Service instance identifier", formalDefinition = "A service line item.") 915 protected PositiveIntType sequence; 916 917 /** 918 * The instance number of the Coverage which is the focus for adjudication. The 919 * Coverage against which the claim is to be adjudicated. 920 */ 921 @Child(name = "focal", type = { BooleanType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 922 @Description(shortDefinition = "The focal Coverage", formalDefinition = "The instance number of the Coverage which is the focus for adjudication. The Coverage against which the claim is to be adjudicated.") 923 protected BooleanType focal; 924 925 /** 926 * Reference to the program or plan identification, underwriter or payor. 927 */ 928 @Child(name = "coverage", type = { Coverage.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 929 @Description(shortDefinition = "Insurance information", formalDefinition = "Reference to the program or plan identification, underwriter or payor.") 930 protected Reference coverage; 931 932 /** 933 * The actual object that is the target of the reference (Reference to the 934 * program or plan identification, underwriter or payor.) 935 */ 936 protected Coverage coverageTarget; 937 938 /** 939 * The contract number of a business agreement which describes the terms and 940 * conditions. 941 */ 942 @Child(name = "businessArrangement", type = { 943 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 944 @Description(shortDefinition = "Business agreement", formalDefinition = "The contract number of a business agreement which describes the terms and conditions.") 945 protected StringType businessArrangement; 946 947 /** 948 * The relationship of the patient to the subscriber. 949 */ 950 @Child(name = "relationship", type = { 951 Coding.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 952 @Description(shortDefinition = "Patient relationship to subscriber", formalDefinition = "The relationship of the patient to the subscriber.") 953 protected Coding relationship; 954 955 /** 956 * A list of references from the Insurer to which these services pertain. 957 */ 958 @Child(name = "preAuthRef", type = { 959 StringType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 960 @Description(shortDefinition = "Pre-Authorization/Determination Reference", formalDefinition = "A list of references from the Insurer to which these services pertain.") 961 protected List<StringType> preAuthRef; 962 963 /** 964 * The Coverages adjudication details. 965 */ 966 @Child(name = "claimResponse", type = { 967 ClaimResponse.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 968 @Description(shortDefinition = "Adjudication results", formalDefinition = "The Coverages adjudication details.") 969 protected Reference claimResponse; 970 971 /** 972 * The actual object that is the target of the reference (The Coverages 973 * adjudication details.) 974 */ 975 protected ClaimResponse claimResponseTarget; 976 977 /** 978 * The style (standard) and version of the original material which was converted 979 * into this resource. 980 */ 981 @Child(name = "originalRuleset", type = { 982 Coding.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 983 @Description(shortDefinition = "Original version", formalDefinition = "The style (standard) and version of the original material which was converted into this resource.") 984 protected Coding originalRuleset; 985 986 private static final long serialVersionUID = 621250924L; 987 988 /* 989 * Constructor 990 */ 991 public CoverageComponent() { 992 super(); 993 } 994 995 /* 996 * Constructor 997 */ 998 public CoverageComponent(PositiveIntType sequence, BooleanType focal, Reference coverage, Coding relationship) { 999 super(); 1000 this.sequence = sequence; 1001 this.focal = focal; 1002 this.coverage = coverage; 1003 this.relationship = relationship; 1004 } 1005 1006 /** 1007 * @return {@link #sequence} (A service line item.). This is the underlying 1008 * object with id, value and extensions. The accessor "getSequence" 1009 * gives direct access to the value 1010 */ 1011 public PositiveIntType getSequenceElement() { 1012 if (this.sequence == null) 1013 if (Configuration.errorOnAutoCreate()) 1014 throw new Error("Attempt to auto-create CoverageComponent.sequence"); 1015 else if (Configuration.doAutoCreate()) 1016 this.sequence = new PositiveIntType(); // bb 1017 return this.sequence; 1018 } 1019 1020 public boolean hasSequenceElement() { 1021 return this.sequence != null && !this.sequence.isEmpty(); 1022 } 1023 1024 public boolean hasSequence() { 1025 return this.sequence != null && !this.sequence.isEmpty(); 1026 } 1027 1028 /** 1029 * @param value {@link #sequence} (A service line item.). This is the underlying 1030 * object with id, value and extensions. The accessor "getSequence" 1031 * gives direct access to the value 1032 */ 1033 public CoverageComponent setSequenceElement(PositiveIntType value) { 1034 this.sequence = value; 1035 return this; 1036 } 1037 1038 /** 1039 * @return A service line item. 1040 */ 1041 public int getSequence() { 1042 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1043 } 1044 1045 /** 1046 * @param value A service line item. 1047 */ 1048 public CoverageComponent setSequence(int value) { 1049 if (this.sequence == null) 1050 this.sequence = new PositiveIntType(); 1051 this.sequence.setValue(value); 1052 return this; 1053 } 1054 1055 /** 1056 * @return {@link #focal} (The instance number of the Coverage which is the 1057 * focus for adjudication. The Coverage against which the claim is to be 1058 * adjudicated.). This is the underlying object with id, value and 1059 * extensions. The accessor "getFocal" gives direct access to the value 1060 */ 1061 public BooleanType getFocalElement() { 1062 if (this.focal == null) 1063 if (Configuration.errorOnAutoCreate()) 1064 throw new Error("Attempt to auto-create CoverageComponent.focal"); 1065 else if (Configuration.doAutoCreate()) 1066 this.focal = new BooleanType(); // bb 1067 return this.focal; 1068 } 1069 1070 public boolean hasFocalElement() { 1071 return this.focal != null && !this.focal.isEmpty(); 1072 } 1073 1074 public boolean hasFocal() { 1075 return this.focal != null && !this.focal.isEmpty(); 1076 } 1077 1078 /** 1079 * @param value {@link #focal} (The instance number of the Coverage which is the 1080 * focus for adjudication. The Coverage against which the claim is 1081 * to be adjudicated.). This is the underlying object with id, 1082 * value and extensions. The accessor "getFocal" gives direct 1083 * access to the value 1084 */ 1085 public CoverageComponent setFocalElement(BooleanType value) { 1086 this.focal = value; 1087 return this; 1088 } 1089 1090 /** 1091 * @return The instance number of the Coverage which is the focus for 1092 * adjudication. The Coverage against which the claim is to be 1093 * adjudicated. 1094 */ 1095 public boolean getFocal() { 1096 return this.focal == null || this.focal.isEmpty() ? false : this.focal.getValue(); 1097 } 1098 1099 /** 1100 * @param value The instance number of the Coverage which is the focus for 1101 * adjudication. The Coverage against which the claim is to be 1102 * adjudicated. 1103 */ 1104 public CoverageComponent setFocal(boolean value) { 1105 if (this.focal == null) 1106 this.focal = new BooleanType(); 1107 this.focal.setValue(value); 1108 return this; 1109 } 1110 1111 /** 1112 * @return {@link #coverage} (Reference to the program or plan identification, 1113 * underwriter or payor.) 1114 */ 1115 public Reference getCoverage() { 1116 if (this.coverage == null) 1117 if (Configuration.errorOnAutoCreate()) 1118 throw new Error("Attempt to auto-create CoverageComponent.coverage"); 1119 else if (Configuration.doAutoCreate()) 1120 this.coverage = new Reference(); // cc 1121 return this.coverage; 1122 } 1123 1124 public boolean hasCoverage() { 1125 return this.coverage != null && !this.coverage.isEmpty(); 1126 } 1127 1128 /** 1129 * @param value {@link #coverage} (Reference to the program or plan 1130 * identification, underwriter or payor.) 1131 */ 1132 public CoverageComponent setCoverage(Reference value) { 1133 this.coverage = value; 1134 return this; 1135 } 1136 1137 /** 1138 * @return {@link #coverage} The actual object that is the target of the 1139 * reference. The reference library doesn't populate this, but you can 1140 * use it to hold the resource if you resolve it. (Reference to the 1141 * program or plan identification, underwriter or payor.) 1142 */ 1143 public Coverage getCoverageTarget() { 1144 if (this.coverageTarget == null) 1145 if (Configuration.errorOnAutoCreate()) 1146 throw new Error("Attempt to auto-create CoverageComponent.coverage"); 1147 else if (Configuration.doAutoCreate()) 1148 this.coverageTarget = new Coverage(); // aa 1149 return this.coverageTarget; 1150 } 1151 1152 /** 1153 * @param value {@link #coverage} The actual object that is the target of the 1154 * reference. The reference library doesn't use these, but you can 1155 * use it to hold the resource if you resolve it. (Reference to the 1156 * program or plan identification, underwriter or payor.) 1157 */ 1158 public CoverageComponent setCoverageTarget(Coverage value) { 1159 this.coverageTarget = value; 1160 return this; 1161 } 1162 1163 /** 1164 * @return {@link #businessArrangement} (The contract number of a business 1165 * agreement which describes the terms and conditions.). This is the 1166 * underlying object with id, value and extensions. The accessor 1167 * "getBusinessArrangement" gives direct access to the value 1168 */ 1169 public StringType getBusinessArrangementElement() { 1170 if (this.businessArrangement == null) 1171 if (Configuration.errorOnAutoCreate()) 1172 throw new Error("Attempt to auto-create CoverageComponent.businessArrangement"); 1173 else if (Configuration.doAutoCreate()) 1174 this.businessArrangement = new StringType(); // bb 1175 return this.businessArrangement; 1176 } 1177 1178 public boolean hasBusinessArrangementElement() { 1179 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 1180 } 1181 1182 public boolean hasBusinessArrangement() { 1183 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 1184 } 1185 1186 /** 1187 * @param value {@link #businessArrangement} (The contract number of a business 1188 * agreement which describes the terms and conditions.). This is 1189 * the underlying object with id, value and extensions. The 1190 * accessor "getBusinessArrangement" gives direct access to the 1191 * value 1192 */ 1193 public CoverageComponent setBusinessArrangementElement(StringType value) { 1194 this.businessArrangement = value; 1195 return this; 1196 } 1197 1198 /** 1199 * @return The contract number of a business agreement which describes the terms 1200 * and conditions. 1201 */ 1202 public String getBusinessArrangement() { 1203 return this.businessArrangement == null ? null : this.businessArrangement.getValue(); 1204 } 1205 1206 /** 1207 * @param value The contract number of a business agreement which describes the 1208 * terms and conditions. 1209 */ 1210 public CoverageComponent setBusinessArrangement(String value) { 1211 if (Utilities.noString(value)) 1212 this.businessArrangement = null; 1213 else { 1214 if (this.businessArrangement == null) 1215 this.businessArrangement = new StringType(); 1216 this.businessArrangement.setValue(value); 1217 } 1218 return this; 1219 } 1220 1221 /** 1222 * @return {@link #relationship} (The relationship of the patient to the 1223 * subscriber.) 1224 */ 1225 public Coding getRelationship() { 1226 if (this.relationship == null) 1227 if (Configuration.errorOnAutoCreate()) 1228 throw new Error("Attempt to auto-create CoverageComponent.relationship"); 1229 else if (Configuration.doAutoCreate()) 1230 this.relationship = new Coding(); // cc 1231 return this.relationship; 1232 } 1233 1234 public boolean hasRelationship() { 1235 return this.relationship != null && !this.relationship.isEmpty(); 1236 } 1237 1238 /** 1239 * @param value {@link #relationship} (The relationship of the patient to the 1240 * subscriber.) 1241 */ 1242 public CoverageComponent setRelationship(Coding value) { 1243 this.relationship = value; 1244 return this; 1245 } 1246 1247 /** 1248 * @return {@link #preAuthRef} (A list of references from the Insurer to which 1249 * these services pertain.) 1250 */ 1251 public List<StringType> getPreAuthRef() { 1252 if (this.preAuthRef == null) 1253 this.preAuthRef = new ArrayList<StringType>(); 1254 return this.preAuthRef; 1255 } 1256 1257 public boolean hasPreAuthRef() { 1258 if (this.preAuthRef == null) 1259 return false; 1260 for (StringType item : this.preAuthRef) 1261 if (!item.isEmpty()) 1262 return true; 1263 return false; 1264 } 1265 1266 /** 1267 * @return {@link #preAuthRef} (A list of references from the Insurer to which 1268 * these services pertain.) 1269 */ 1270 // syntactic sugar 1271 public StringType addPreAuthRefElement() {// 2 1272 StringType t = new StringType(); 1273 if (this.preAuthRef == null) 1274 this.preAuthRef = new ArrayList<StringType>(); 1275 this.preAuthRef.add(t); 1276 return t; 1277 } 1278 1279 /** 1280 * @param value {@link #preAuthRef} (A list of references from the Insurer to 1281 * which these services pertain.) 1282 */ 1283 public CoverageComponent addPreAuthRef(String value) { // 1 1284 StringType t = new StringType(); 1285 t.setValue(value); 1286 if (this.preAuthRef == null) 1287 this.preAuthRef = new ArrayList<StringType>(); 1288 this.preAuthRef.add(t); 1289 return this; 1290 } 1291 1292 /** 1293 * @param value {@link #preAuthRef} (A list of references from the Insurer to 1294 * which these services pertain.) 1295 */ 1296 public boolean hasPreAuthRef(String value) { 1297 if (this.preAuthRef == null) 1298 return false; 1299 for (StringType v : this.preAuthRef) 1300 if (v.equals(value)) // string 1301 return true; 1302 return false; 1303 } 1304 1305 /** 1306 * @return {@link #claimResponse} (The Coverages adjudication details.) 1307 */ 1308 public Reference getClaimResponse() { 1309 if (this.claimResponse == null) 1310 if (Configuration.errorOnAutoCreate()) 1311 throw new Error("Attempt to auto-create CoverageComponent.claimResponse"); 1312 else if (Configuration.doAutoCreate()) 1313 this.claimResponse = new Reference(); // cc 1314 return this.claimResponse; 1315 } 1316 1317 public boolean hasClaimResponse() { 1318 return this.claimResponse != null && !this.claimResponse.isEmpty(); 1319 } 1320 1321 /** 1322 * @param value {@link #claimResponse} (The Coverages adjudication details.) 1323 */ 1324 public CoverageComponent setClaimResponse(Reference value) { 1325 this.claimResponse = value; 1326 return this; 1327 } 1328 1329 /** 1330 * @return {@link #claimResponse} The actual object that is the target of the 1331 * reference. The reference library doesn't populate this, but you can 1332 * use it to hold the resource if you resolve it. (The Coverages 1333 * adjudication details.) 1334 */ 1335 public ClaimResponse getClaimResponseTarget() { 1336 if (this.claimResponseTarget == null) 1337 if (Configuration.errorOnAutoCreate()) 1338 throw new Error("Attempt to auto-create CoverageComponent.claimResponse"); 1339 else if (Configuration.doAutoCreate()) 1340 this.claimResponseTarget = new ClaimResponse(); // aa 1341 return this.claimResponseTarget; 1342 } 1343 1344 /** 1345 * @param value {@link #claimResponse} The actual object that is the target of 1346 * the reference. The reference library doesn't use these, but you 1347 * can use it to hold the resource if you resolve it. (The 1348 * Coverages adjudication details.) 1349 */ 1350 public CoverageComponent setClaimResponseTarget(ClaimResponse value) { 1351 this.claimResponseTarget = value; 1352 return this; 1353 } 1354 1355 /** 1356 * @return {@link #originalRuleset} (The style (standard) and version of the 1357 * original material which was converted into this resource.) 1358 */ 1359 public Coding getOriginalRuleset() { 1360 if (this.originalRuleset == null) 1361 if (Configuration.errorOnAutoCreate()) 1362 throw new Error("Attempt to auto-create CoverageComponent.originalRuleset"); 1363 else if (Configuration.doAutoCreate()) 1364 this.originalRuleset = new Coding(); // cc 1365 return this.originalRuleset; 1366 } 1367 1368 public boolean hasOriginalRuleset() { 1369 return this.originalRuleset != null && !this.originalRuleset.isEmpty(); 1370 } 1371 1372 /** 1373 * @param value {@link #originalRuleset} (The style (standard) and version of 1374 * the original material which was converted into this resource.) 1375 */ 1376 public CoverageComponent setOriginalRuleset(Coding value) { 1377 this.originalRuleset = value; 1378 return this; 1379 } 1380 1381 protected void listChildren(List<Property> childrenList) { 1382 super.listChildren(childrenList); 1383 childrenList.add( 1384 new Property("sequence", "positiveInt", "A service line item.", 0, java.lang.Integer.MAX_VALUE, sequence)); 1385 childrenList.add(new Property("focal", "boolean", 1386 "The instance number of the Coverage which is the focus for adjudication. The Coverage against which the claim is to be adjudicated.", 1387 0, java.lang.Integer.MAX_VALUE, focal)); 1388 childrenList.add(new Property("coverage", "Reference(Coverage)", 1389 "Reference to the program or plan identification, underwriter or payor.", 0, java.lang.Integer.MAX_VALUE, 1390 coverage)); 1391 childrenList.add(new Property("businessArrangement", "string", 1392 "The contract number of a business agreement which describes the terms and conditions.", 0, 1393 java.lang.Integer.MAX_VALUE, businessArrangement)); 1394 childrenList.add(new Property("relationship", "Coding", "The relationship of the patient to the subscriber.", 0, 1395 java.lang.Integer.MAX_VALUE, relationship)); 1396 childrenList.add( 1397 new Property("preAuthRef", "string", "A list of references from the Insurer to which these services pertain.", 1398 0, java.lang.Integer.MAX_VALUE, preAuthRef)); 1399 childrenList.add(new Property("claimResponse", "Reference(ClaimResponse)", "The Coverages adjudication details.", 1400 0, java.lang.Integer.MAX_VALUE, claimResponse)); 1401 childrenList.add(new Property("originalRuleset", "Coding", 1402 "The style (standard) and version of the original material which was converted into this resource.", 0, 1403 java.lang.Integer.MAX_VALUE, originalRuleset)); 1404 } 1405 1406 @Override 1407 public void setProperty(String name, Base value) throws FHIRException { 1408 if (name.equals("sequence")) 1409 this.sequence = castToPositiveInt(value); // PositiveIntType 1410 else if (name.equals("focal")) 1411 this.focal = castToBoolean(value); // BooleanType 1412 else if (name.equals("coverage")) 1413 this.coverage = castToReference(value); // Reference 1414 else if (name.equals("businessArrangement")) 1415 this.businessArrangement = castToString(value); // StringType 1416 else if (name.equals("relationship")) 1417 this.relationship = castToCoding(value); // Coding 1418 else if (name.equals("preAuthRef")) 1419 this.getPreAuthRef().add(castToString(value)); 1420 else if (name.equals("claimResponse")) 1421 this.claimResponse = castToReference(value); // Reference 1422 else if (name.equals("originalRuleset")) 1423 this.originalRuleset = castToCoding(value); // Coding 1424 else 1425 super.setProperty(name, value); 1426 } 1427 1428 @Override 1429 public Base addChild(String name) throws FHIRException { 1430 if (name.equals("sequence")) { 1431 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 1432 } else if (name.equals("focal")) { 1433 throw new FHIRException("Cannot call addChild on a singleton property Claim.focal"); 1434 } else if (name.equals("coverage")) { 1435 this.coverage = new Reference(); 1436 return this.coverage; 1437 } else if (name.equals("businessArrangement")) { 1438 throw new FHIRException("Cannot call addChild on a singleton property Claim.businessArrangement"); 1439 } else if (name.equals("relationship")) { 1440 this.relationship = new Coding(); 1441 return this.relationship; 1442 } else if (name.equals("preAuthRef")) { 1443 throw new FHIRException("Cannot call addChild on a singleton property Claim.preAuthRef"); 1444 } else if (name.equals("claimResponse")) { 1445 this.claimResponse = new Reference(); 1446 return this.claimResponse; 1447 } else if (name.equals("originalRuleset")) { 1448 this.originalRuleset = new Coding(); 1449 return this.originalRuleset; 1450 } else 1451 return super.addChild(name); 1452 } 1453 1454 public CoverageComponent copy() { 1455 CoverageComponent dst = new CoverageComponent(); 1456 copyValues(dst); 1457 dst.sequence = sequence == null ? null : sequence.copy(); 1458 dst.focal = focal == null ? null : focal.copy(); 1459 dst.coverage = coverage == null ? null : coverage.copy(); 1460 dst.businessArrangement = businessArrangement == null ? null : businessArrangement.copy(); 1461 dst.relationship = relationship == null ? null : relationship.copy(); 1462 if (preAuthRef != null) { 1463 dst.preAuthRef = new ArrayList<StringType>(); 1464 for (StringType i : preAuthRef) 1465 dst.preAuthRef.add(i.copy()); 1466 } 1467 ; 1468 dst.claimResponse = claimResponse == null ? null : claimResponse.copy(); 1469 dst.originalRuleset = originalRuleset == null ? null : originalRuleset.copy(); 1470 return dst; 1471 } 1472 1473 @Override 1474 public boolean equalsDeep(Base other) { 1475 if (!super.equalsDeep(other)) 1476 return false; 1477 if (!(other instanceof CoverageComponent)) 1478 return false; 1479 CoverageComponent o = (CoverageComponent) other; 1480 return compareDeep(sequence, o.sequence, true) && compareDeep(focal, o.focal, true) 1481 && compareDeep(coverage, o.coverage, true) && compareDeep(businessArrangement, o.businessArrangement, true) 1482 && compareDeep(relationship, o.relationship, true) && compareDeep(preAuthRef, o.preAuthRef, true) 1483 && compareDeep(claimResponse, o.claimResponse, true) && compareDeep(originalRuleset, o.originalRuleset, true); 1484 } 1485 1486 @Override 1487 public boolean equalsShallow(Base other) { 1488 if (!super.equalsShallow(other)) 1489 return false; 1490 if (!(other instanceof CoverageComponent)) 1491 return false; 1492 CoverageComponent o = (CoverageComponent) other; 1493 return compareValues(sequence, o.sequence, true) && compareValues(focal, o.focal, true) 1494 && compareValues(businessArrangement, o.businessArrangement, true) 1495 && compareValues(preAuthRef, o.preAuthRef, true); 1496 } 1497 1498 public boolean isEmpty() { 1499 return super.isEmpty() && (sequence == null || sequence.isEmpty()) && (focal == null || focal.isEmpty()) 1500 && (coverage == null || coverage.isEmpty()) && (businessArrangement == null || businessArrangement.isEmpty()) 1501 && (relationship == null || relationship.isEmpty()) && (preAuthRef == null || preAuthRef.isEmpty()) 1502 && (claimResponse == null || claimResponse.isEmpty()) 1503 && (originalRuleset == null || originalRuleset.isEmpty()); 1504 } 1505 1506 public String fhirType() { 1507 return "Claim.coverage"; 1508 1509 } 1510 1511 } 1512 1513 @Block() 1514 public static class ItemsComponent extends BackboneElement implements IBaseBackboneElement { 1515 /** 1516 * A service line number. 1517 */ 1518 @Child(name = "sequence", type = { 1519 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1520 @Description(shortDefinition = "Service instance", formalDefinition = "A service line number.") 1521 protected PositiveIntType sequence; 1522 1523 /** 1524 * The type of product or service. 1525 */ 1526 @Child(name = "type", type = { Coding.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1527 @Description(shortDefinition = "Group or type of product or service", formalDefinition = "The type of product or service.") 1528 protected Coding type; 1529 1530 /** 1531 * The practitioner who is responsible for the services rendered to the patient. 1532 */ 1533 @Child(name = "provider", type = { 1534 Practitioner.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1535 @Description(shortDefinition = "Responsible practitioner", formalDefinition = "The practitioner who is responsible for the services rendered to the patient.") 1536 protected Reference provider; 1537 1538 /** 1539 * The actual object that is the target of the reference (The practitioner who 1540 * is responsible for the services rendered to the patient.) 1541 */ 1542 protected Practitioner providerTarget; 1543 1544 /** 1545 * Diagnosis applicable for this service or product line. 1546 */ 1547 @Child(name = "diagnosisLinkId", type = { 1548 PositiveIntType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1549 @Description(shortDefinition = "Diagnosis Link", formalDefinition = "Diagnosis applicable for this service or product line.") 1550 protected List<PositiveIntType> diagnosisLinkId; 1551 1552 /** 1553 * If a grouping item then 'GROUP' otherwise it is a node therefore a code to 1554 * indicate the Professional Service or Product supplied. 1555 */ 1556 @Child(name = "service", type = { Coding.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 1557 @Description(shortDefinition = "Item Code", formalDefinition = "If a grouping item then 'GROUP' otherwise it is a node therefore a code to indicate the Professional Service or Product supplied.") 1558 protected Coding service; 1559 1560 /** 1561 * The date when the enclosed suite of services were performed or completed. 1562 */ 1563 @Child(name = "serviceDate", type = { 1564 DateType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 1565 @Description(shortDefinition = "Date of Service", formalDefinition = "The date when the enclosed suite of services were performed or completed.") 1566 protected DateType serviceDate; 1567 1568 /** 1569 * The number of repetitions of a service or product. 1570 */ 1571 @Child(name = "quantity", type = { 1572 SimpleQuantity.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 1573 @Description(shortDefinition = "Count of Products or Services", formalDefinition = "The number of repetitions of a service or product.") 1574 protected SimpleQuantity quantity; 1575 1576 /** 1577 * If the item is a node then this is the fee for the product or service, 1578 * otherwise this is the total of the fees for the children of the group. 1579 */ 1580 @Child(name = "unitPrice", type = { Money.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 1581 @Description(shortDefinition = "Fee, charge or cost per point", formalDefinition = "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.") 1582 protected Money unitPrice; 1583 1584 /** 1585 * A real number that represents a multiplier used in determining the overall 1586 * value of services delivered and/or goods received. The concept of a Factor 1587 * allows for a discount or surcharge multiplier to be applied to a monetary 1588 * amount. 1589 */ 1590 @Child(name = "factor", type = { DecimalType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 1591 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 1592 protected DecimalType factor; 1593 1594 /** 1595 * An amount that expresses the weighting (based on difficulty, cost and/or 1596 * resource intensiveness) associated with the good or service delivered. The 1597 * concept of Points allows for assignment of point values for services and/or 1598 * goods, such that a monetary amount can be assigned to each point. 1599 */ 1600 @Child(name = "points", type = { 1601 DecimalType.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 1602 @Description(shortDefinition = "Difficulty scaling factor", formalDefinition = "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the good or service delivered. The concept of Points allows for assignment of point values for services and/or goods, such that a monetary amount can be assigned to each point.") 1603 protected DecimalType points; 1604 1605 /** 1606 * The quantity times the unit price for an additional service or product or 1607 * charge. For example, the formula: unit Quantity * unit Price (Cost per Point) 1608 * * factor Number * points = net Amount. Quantity, factor and points are 1609 * assumed to be 1 if not supplied. 1610 */ 1611 @Child(name = "net", type = { Money.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 1612 @Description(shortDefinition = "Total item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.") 1613 protected Money net; 1614 1615 /** 1616 * List of Unique Device Identifiers associated with this line item. 1617 */ 1618 @Child(name = "udi", type = { Coding.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 1619 @Description(shortDefinition = "Unique Device Identifier", formalDefinition = "List of Unique Device Identifiers associated with this line item.") 1620 protected Coding udi; 1621 1622 /** 1623 * Physical service site on the patient (limb, tooth, etc.). 1624 */ 1625 @Child(name = "bodySite", type = { Coding.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 1626 @Description(shortDefinition = "Service Location", formalDefinition = "Physical service site on the patient (limb, tooth, etc.).") 1627 protected Coding bodySite; 1628 1629 /** 1630 * A region or surface of the site, e.g. limb region or tooth surface(s). 1631 */ 1632 @Child(name = "subSite", type = { 1633 Coding.class }, order = 14, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1634 @Description(shortDefinition = "Service Sub-location", formalDefinition = "A region or surface of the site, e.g. limb region or tooth surface(s).") 1635 protected List<Coding> subSite; 1636 1637 /** 1638 * Item typification or modifiers codes, e.g. for Oral whether the treatment is 1639 * cosmetic or associated with TMJ, or an appliance was lost or stolen. 1640 */ 1641 @Child(name = "modifier", type = { 1642 Coding.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1643 @Description(shortDefinition = "Service/Product billing modifiers", formalDefinition = "Item typification or modifiers codes, e.g. for Oral whether the treatment is cosmetic or associated with TMJ, or an appliance was lost or stolen.") 1644 protected List<Coding> modifier; 1645 1646 /** 1647 * Second tier of goods and services. 1648 */ 1649 @Child(name = "detail", type = {}, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1650 @Description(shortDefinition = "Additional items", formalDefinition = "Second tier of goods and services.") 1651 protected List<DetailComponent> detail; 1652 1653 /** 1654 * The materials and placement date of prior fixed prosthesis. 1655 */ 1656 @Child(name = "prosthesis", type = {}, order = 17, min = 0, max = 1, modifier = false, summary = true) 1657 @Description(shortDefinition = "Prosthetic details", formalDefinition = "The materials and placement date of prior fixed prosthesis.") 1658 protected ProsthesisComponent prosthesis; 1659 1660 private static final long serialVersionUID = 1295830456L; 1661 1662 /* 1663 * Constructor 1664 */ 1665 public ItemsComponent() { 1666 super(); 1667 } 1668 1669 /* 1670 * Constructor 1671 */ 1672 public ItemsComponent(PositiveIntType sequence, Coding type, Coding service) { 1673 super(); 1674 this.sequence = sequence; 1675 this.type = type; 1676 this.service = service; 1677 } 1678 1679 /** 1680 * @return {@link #sequence} (A service line number.). This is the underlying 1681 * object with id, value and extensions. The accessor "getSequence" 1682 * gives direct access to the value 1683 */ 1684 public PositiveIntType getSequenceElement() { 1685 if (this.sequence == null) 1686 if (Configuration.errorOnAutoCreate()) 1687 throw new Error("Attempt to auto-create ItemsComponent.sequence"); 1688 else if (Configuration.doAutoCreate()) 1689 this.sequence = new PositiveIntType(); // bb 1690 return this.sequence; 1691 } 1692 1693 public boolean hasSequenceElement() { 1694 return this.sequence != null && !this.sequence.isEmpty(); 1695 } 1696 1697 public boolean hasSequence() { 1698 return this.sequence != null && !this.sequence.isEmpty(); 1699 } 1700 1701 /** 1702 * @param value {@link #sequence} (A service line number.). This is the 1703 * underlying object with id, value and extensions. The accessor 1704 * "getSequence" gives direct access to the value 1705 */ 1706 public ItemsComponent setSequenceElement(PositiveIntType value) { 1707 this.sequence = value; 1708 return this; 1709 } 1710 1711 /** 1712 * @return A service line number. 1713 */ 1714 public int getSequence() { 1715 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1716 } 1717 1718 /** 1719 * @param value A service line number. 1720 */ 1721 public ItemsComponent setSequence(int value) { 1722 if (this.sequence == null) 1723 this.sequence = new PositiveIntType(); 1724 this.sequence.setValue(value); 1725 return this; 1726 } 1727 1728 /** 1729 * @return {@link #type} (The type of product or service.) 1730 */ 1731 public Coding getType() { 1732 if (this.type == null) 1733 if (Configuration.errorOnAutoCreate()) 1734 throw new Error("Attempt to auto-create ItemsComponent.type"); 1735 else if (Configuration.doAutoCreate()) 1736 this.type = new Coding(); // cc 1737 return this.type; 1738 } 1739 1740 public boolean hasType() { 1741 return this.type != null && !this.type.isEmpty(); 1742 } 1743 1744 /** 1745 * @param value {@link #type} (The type of product or service.) 1746 */ 1747 public ItemsComponent setType(Coding value) { 1748 this.type = value; 1749 return this; 1750 } 1751 1752 /** 1753 * @return {@link #provider} (The practitioner who is responsible for the 1754 * services rendered to the patient.) 1755 */ 1756 public Reference getProvider() { 1757 if (this.provider == null) 1758 if (Configuration.errorOnAutoCreate()) 1759 throw new Error("Attempt to auto-create ItemsComponent.provider"); 1760 else if (Configuration.doAutoCreate()) 1761 this.provider = new Reference(); // cc 1762 return this.provider; 1763 } 1764 1765 public boolean hasProvider() { 1766 return this.provider != null && !this.provider.isEmpty(); 1767 } 1768 1769 /** 1770 * @param value {@link #provider} (The practitioner who is responsible for the 1771 * services rendered to the patient.) 1772 */ 1773 public ItemsComponent setProvider(Reference value) { 1774 this.provider = value; 1775 return this; 1776 } 1777 1778 /** 1779 * @return {@link #provider} The actual object that is the target of the 1780 * reference. The reference library doesn't populate this, but you can 1781 * use it to hold the resource if you resolve it. (The practitioner who 1782 * is responsible for the services rendered to the patient.) 1783 */ 1784 public Practitioner getProviderTarget() { 1785 if (this.providerTarget == null) 1786 if (Configuration.errorOnAutoCreate()) 1787 throw new Error("Attempt to auto-create ItemsComponent.provider"); 1788 else if (Configuration.doAutoCreate()) 1789 this.providerTarget = new Practitioner(); // aa 1790 return this.providerTarget; 1791 } 1792 1793 /** 1794 * @param value {@link #provider} The actual object that is the target of the 1795 * reference. The reference library doesn't use these, but you can 1796 * use it to hold the resource if you resolve it. (The practitioner 1797 * who is responsible for the services rendered to the patient.) 1798 */ 1799 public ItemsComponent setProviderTarget(Practitioner value) { 1800 this.providerTarget = value; 1801 return this; 1802 } 1803 1804 /** 1805 * @return {@link #diagnosisLinkId} (Diagnosis applicable for this service or 1806 * product line.) 1807 */ 1808 public List<PositiveIntType> getDiagnosisLinkId() { 1809 if (this.diagnosisLinkId == null) 1810 this.diagnosisLinkId = new ArrayList<PositiveIntType>(); 1811 return this.diagnosisLinkId; 1812 } 1813 1814 public boolean hasDiagnosisLinkId() { 1815 if (this.diagnosisLinkId == null) 1816 return false; 1817 for (PositiveIntType item : this.diagnosisLinkId) 1818 if (!item.isEmpty()) 1819 return true; 1820 return false; 1821 } 1822 1823 /** 1824 * @return {@link #diagnosisLinkId} (Diagnosis applicable for this service or 1825 * product line.) 1826 */ 1827 // syntactic sugar 1828 public PositiveIntType addDiagnosisLinkIdElement() {// 2 1829 PositiveIntType t = new PositiveIntType(); 1830 if (this.diagnosisLinkId == null) 1831 this.diagnosisLinkId = new ArrayList<PositiveIntType>(); 1832 this.diagnosisLinkId.add(t); 1833 return t; 1834 } 1835 1836 /** 1837 * @param value {@link #diagnosisLinkId} (Diagnosis applicable for this service 1838 * or product line.) 1839 */ 1840 public ItemsComponent addDiagnosisLinkId(int value) { // 1 1841 PositiveIntType t = new PositiveIntType(); 1842 t.setValue(value); 1843 if (this.diagnosisLinkId == null) 1844 this.diagnosisLinkId = new ArrayList<PositiveIntType>(); 1845 this.diagnosisLinkId.add(t); 1846 return this; 1847 } 1848 1849 /** 1850 * @param value {@link #diagnosisLinkId} (Diagnosis applicable for this service 1851 * or product line.) 1852 */ 1853 public boolean hasDiagnosisLinkId(int value) { 1854 if (this.diagnosisLinkId == null) 1855 return false; 1856 for (PositiveIntType v : this.diagnosisLinkId) 1857 if (v.equals(value)) // positiveInt 1858 return true; 1859 return false; 1860 } 1861 1862 /** 1863 * @return {@link #service} (If a grouping item then 'GROUP' otherwise it is a 1864 * node therefore a code to indicate the Professional Service or Product 1865 * supplied.) 1866 */ 1867 public Coding getService() { 1868 if (this.service == null) 1869 if (Configuration.errorOnAutoCreate()) 1870 throw new Error("Attempt to auto-create ItemsComponent.service"); 1871 else if (Configuration.doAutoCreate()) 1872 this.service = new Coding(); // cc 1873 return this.service; 1874 } 1875 1876 public boolean hasService() { 1877 return this.service != null && !this.service.isEmpty(); 1878 } 1879 1880 /** 1881 * @param value {@link #service} (If a grouping item then 'GROUP' otherwise it 1882 * is a node therefore a code to indicate the Professional Service 1883 * or Product supplied.) 1884 */ 1885 public ItemsComponent setService(Coding value) { 1886 this.service = value; 1887 return this; 1888 } 1889 1890 /** 1891 * @return {@link #serviceDate} (The date when the enclosed suite of services 1892 * were performed or completed.). This is the underlying object with id, 1893 * value and extensions. The accessor "getServiceDate" gives direct 1894 * access to the value 1895 */ 1896 public DateType getServiceDateElement() { 1897 if (this.serviceDate == null) 1898 if (Configuration.errorOnAutoCreate()) 1899 throw new Error("Attempt to auto-create ItemsComponent.serviceDate"); 1900 else if (Configuration.doAutoCreate()) 1901 this.serviceDate = new DateType(); // bb 1902 return this.serviceDate; 1903 } 1904 1905 public boolean hasServiceDateElement() { 1906 return this.serviceDate != null && !this.serviceDate.isEmpty(); 1907 } 1908 1909 public boolean hasServiceDate() { 1910 return this.serviceDate != null && !this.serviceDate.isEmpty(); 1911 } 1912 1913 /** 1914 * @param value {@link #serviceDate} (The date when the enclosed suite of 1915 * services were performed or completed.). This is the underlying 1916 * object with id, value and extensions. The accessor 1917 * "getServiceDate" gives direct access to the value 1918 */ 1919 public ItemsComponent setServiceDateElement(DateType value) { 1920 this.serviceDate = value; 1921 return this; 1922 } 1923 1924 /** 1925 * @return The date when the enclosed suite of services were performed or 1926 * completed. 1927 */ 1928 public Date getServiceDate() { 1929 return this.serviceDate == null ? null : this.serviceDate.getValue(); 1930 } 1931 1932 /** 1933 * @param value The date when the enclosed suite of services were performed or 1934 * completed. 1935 */ 1936 public ItemsComponent setServiceDate(Date value) { 1937 if (value == null) 1938 this.serviceDate = null; 1939 else { 1940 if (this.serviceDate == null) 1941 this.serviceDate = new DateType(); 1942 this.serviceDate.setValue(value); 1943 } 1944 return this; 1945 } 1946 1947 /** 1948 * @return {@link #quantity} (The number of repetitions of a service or 1949 * product.) 1950 */ 1951 public SimpleQuantity getQuantity() { 1952 if (this.quantity == null) 1953 if (Configuration.errorOnAutoCreate()) 1954 throw new Error("Attempt to auto-create ItemsComponent.quantity"); 1955 else if (Configuration.doAutoCreate()) 1956 this.quantity = new SimpleQuantity(); // cc 1957 return this.quantity; 1958 } 1959 1960 public boolean hasQuantity() { 1961 return this.quantity != null && !this.quantity.isEmpty(); 1962 } 1963 1964 /** 1965 * @param value {@link #quantity} (The number of repetitions of a service or 1966 * product.) 1967 */ 1968 public ItemsComponent setQuantity(SimpleQuantity value) { 1969 this.quantity = value; 1970 return this; 1971 } 1972 1973 /** 1974 * @return {@link #unitPrice} (If the item is a node then this is the fee for 1975 * the product or service, otherwise this is the total of the fees for 1976 * the children of the group.) 1977 */ 1978 public Money getUnitPrice() { 1979 if (this.unitPrice == null) 1980 if (Configuration.errorOnAutoCreate()) 1981 throw new Error("Attempt to auto-create ItemsComponent.unitPrice"); 1982 else if (Configuration.doAutoCreate()) 1983 this.unitPrice = new Money(); // cc 1984 return this.unitPrice; 1985 } 1986 1987 public boolean hasUnitPrice() { 1988 return this.unitPrice != null && !this.unitPrice.isEmpty(); 1989 } 1990 1991 /** 1992 * @param value {@link #unitPrice} (If the item is a node then this is the fee 1993 * for the product or service, otherwise this is the total of the 1994 * fees for the children of the group.) 1995 */ 1996 public ItemsComponent setUnitPrice(Money value) { 1997 this.unitPrice = value; 1998 return this; 1999 } 2000 2001 /** 2002 * @return {@link #factor} (A real number that represents a multiplier used in 2003 * determining the overall value of services delivered and/or goods 2004 * received. The concept of a Factor allows for a discount or surcharge 2005 * multiplier to be applied to a monetary amount.). This is the 2006 * underlying object with id, value and extensions. The accessor 2007 * "getFactor" gives direct access to the value 2008 */ 2009 public DecimalType getFactorElement() { 2010 if (this.factor == null) 2011 if (Configuration.errorOnAutoCreate()) 2012 throw new Error("Attempt to auto-create ItemsComponent.factor"); 2013 else if (Configuration.doAutoCreate()) 2014 this.factor = new DecimalType(); // bb 2015 return this.factor; 2016 } 2017 2018 public boolean hasFactorElement() { 2019 return this.factor != null && !this.factor.isEmpty(); 2020 } 2021 2022 public boolean hasFactor() { 2023 return this.factor != null && !this.factor.isEmpty(); 2024 } 2025 2026 /** 2027 * @param value {@link #factor} (A real number that represents a multiplier used 2028 * in determining the overall value of services delivered and/or 2029 * goods received. The concept of a Factor allows for a discount or 2030 * surcharge multiplier to be applied to a monetary amount.). This 2031 * is the underlying object with id, value and extensions. The 2032 * accessor "getFactor" gives direct access to the value 2033 */ 2034 public ItemsComponent setFactorElement(DecimalType value) { 2035 this.factor = value; 2036 return this; 2037 } 2038 2039 /** 2040 * @return A real number that represents a multiplier used in determining the 2041 * overall value of services delivered and/or goods received. The 2042 * concept of a Factor allows for a discount or surcharge multiplier to 2043 * be applied to a monetary amount. 2044 */ 2045 public BigDecimal getFactor() { 2046 return this.factor == null ? null : this.factor.getValue(); 2047 } 2048 2049 /** 2050 * @param value A real number that represents a multiplier used in determining 2051 * the overall value of services delivered and/or goods received. 2052 * The concept of a Factor allows for a discount or surcharge 2053 * multiplier to be applied to a monetary amount. 2054 */ 2055 public ItemsComponent setFactor(BigDecimal value) { 2056 if (value == null) 2057 this.factor = null; 2058 else { 2059 if (this.factor == null) 2060 this.factor = new DecimalType(); 2061 this.factor.setValue(value); 2062 } 2063 return this; 2064 } 2065 2066 /** 2067 * @return {@link #points} (An amount that expresses the weighting (based on 2068 * difficulty, cost and/or resource intensiveness) associated with the 2069 * good or service delivered. The concept of Points allows for 2070 * assignment of point values for services and/or goods, such that a 2071 * monetary amount can be assigned to each point.). This is the 2072 * underlying object with id, value and extensions. The accessor 2073 * "getPoints" gives direct access to the value 2074 */ 2075 public DecimalType getPointsElement() { 2076 if (this.points == null) 2077 if (Configuration.errorOnAutoCreate()) 2078 throw new Error("Attempt to auto-create ItemsComponent.points"); 2079 else if (Configuration.doAutoCreate()) 2080 this.points = new DecimalType(); // bb 2081 return this.points; 2082 } 2083 2084 public boolean hasPointsElement() { 2085 return this.points != null && !this.points.isEmpty(); 2086 } 2087 2088 public boolean hasPoints() { 2089 return this.points != null && !this.points.isEmpty(); 2090 } 2091 2092 /** 2093 * @param value {@link #points} (An amount that expresses the weighting (based 2094 * on difficulty, cost and/or resource intensiveness) associated 2095 * with the good or service delivered. The concept of Points allows 2096 * for assignment of point values for services and/or goods, such 2097 * that a monetary amount can be assigned to each point.). This is 2098 * the underlying object with id, value and extensions. The 2099 * accessor "getPoints" gives direct access to the value 2100 */ 2101 public ItemsComponent setPointsElement(DecimalType value) { 2102 this.points = value; 2103 return this; 2104 } 2105 2106 /** 2107 * @return An amount that expresses the weighting (based on difficulty, cost 2108 * and/or resource intensiveness) associated with the good or service 2109 * delivered. The concept of Points allows for assignment of point 2110 * values for services and/or goods, such that a monetary amount can be 2111 * assigned to each point. 2112 */ 2113 public BigDecimal getPoints() { 2114 return this.points == null ? null : this.points.getValue(); 2115 } 2116 2117 /** 2118 * @param value An amount that expresses the weighting (based on difficulty, 2119 * cost and/or resource intensiveness) associated with the good or 2120 * service delivered. The concept of Points allows for assignment 2121 * of point values for services and/or goods, such that a monetary 2122 * amount can be assigned to each point. 2123 */ 2124 public ItemsComponent setPoints(BigDecimal value) { 2125 if (value == null) 2126 this.points = null; 2127 else { 2128 if (this.points == null) 2129 this.points = new DecimalType(); 2130 this.points.setValue(value); 2131 } 2132 return this; 2133 } 2134 2135 /** 2136 * @return {@link #net} (The quantity times the unit price for an additional 2137 * service or product or charge. For example, the formula: unit Quantity 2138 * * unit Price (Cost per Point) * factor Number * points = net Amount. 2139 * Quantity, factor and points are assumed to be 1 if not supplied.) 2140 */ 2141 public Money getNet() { 2142 if (this.net == null) 2143 if (Configuration.errorOnAutoCreate()) 2144 throw new Error("Attempt to auto-create ItemsComponent.net"); 2145 else if (Configuration.doAutoCreate()) 2146 this.net = new Money(); // cc 2147 return this.net; 2148 } 2149 2150 public boolean hasNet() { 2151 return this.net != null && !this.net.isEmpty(); 2152 } 2153 2154 /** 2155 * @param value {@link #net} (The quantity times the unit price for an 2156 * additional service or product or charge. For example, the 2157 * formula: unit Quantity * unit Price (Cost per Point) * factor 2158 * Number * points = net Amount. Quantity, factor and points are 2159 * assumed to be 1 if not supplied.) 2160 */ 2161 public ItemsComponent setNet(Money value) { 2162 this.net = value; 2163 return this; 2164 } 2165 2166 /** 2167 * @return {@link #udi} (List of Unique Device Identifiers associated with this 2168 * line item.) 2169 */ 2170 public Coding getUdi() { 2171 if (this.udi == null) 2172 if (Configuration.errorOnAutoCreate()) 2173 throw new Error("Attempt to auto-create ItemsComponent.udi"); 2174 else if (Configuration.doAutoCreate()) 2175 this.udi = new Coding(); // cc 2176 return this.udi; 2177 } 2178 2179 public boolean hasUdi() { 2180 return this.udi != null && !this.udi.isEmpty(); 2181 } 2182 2183 /** 2184 * @param value {@link #udi} (List of Unique Device Identifiers associated with 2185 * this line item.) 2186 */ 2187 public ItemsComponent setUdi(Coding value) { 2188 this.udi = value; 2189 return this; 2190 } 2191 2192 /** 2193 * @return {@link #bodySite} (Physical service site on the patient (limb, tooth, 2194 * etc.).) 2195 */ 2196 public Coding getBodySite() { 2197 if (this.bodySite == null) 2198 if (Configuration.errorOnAutoCreate()) 2199 throw new Error("Attempt to auto-create ItemsComponent.bodySite"); 2200 else if (Configuration.doAutoCreate()) 2201 this.bodySite = new Coding(); // cc 2202 return this.bodySite; 2203 } 2204 2205 public boolean hasBodySite() { 2206 return this.bodySite != null && !this.bodySite.isEmpty(); 2207 } 2208 2209 /** 2210 * @param value {@link #bodySite} (Physical service site on the patient (limb, 2211 * tooth, etc.).) 2212 */ 2213 public ItemsComponent setBodySite(Coding value) { 2214 this.bodySite = value; 2215 return this; 2216 } 2217 2218 /** 2219 * @return {@link #subSite} (A region or surface of the site, e.g. limb region 2220 * or tooth surface(s).) 2221 */ 2222 public List<Coding> getSubSite() { 2223 if (this.subSite == null) 2224 this.subSite = new ArrayList<Coding>(); 2225 return this.subSite; 2226 } 2227 2228 public boolean hasSubSite() { 2229 if (this.subSite == null) 2230 return false; 2231 for (Coding item : this.subSite) 2232 if (!item.isEmpty()) 2233 return true; 2234 return false; 2235 } 2236 2237 /** 2238 * @return {@link #subSite} (A region or surface of the site, e.g. limb region 2239 * or tooth surface(s).) 2240 */ 2241 // syntactic sugar 2242 public Coding addSubSite() { // 3 2243 Coding t = new Coding(); 2244 if (this.subSite == null) 2245 this.subSite = new ArrayList<Coding>(); 2246 this.subSite.add(t); 2247 return t; 2248 } 2249 2250 // syntactic sugar 2251 public ItemsComponent addSubSite(Coding t) { // 3 2252 if (t == null) 2253 return this; 2254 if (this.subSite == null) 2255 this.subSite = new ArrayList<Coding>(); 2256 this.subSite.add(t); 2257 return this; 2258 } 2259 2260 /** 2261 * @return {@link #modifier} (Item typification or modifiers codes, e.g. for 2262 * Oral whether the treatment is cosmetic or associated with TMJ, or an 2263 * appliance was lost or stolen.) 2264 */ 2265 public List<Coding> getModifier() { 2266 if (this.modifier == null) 2267 this.modifier = new ArrayList<Coding>(); 2268 return this.modifier; 2269 } 2270 2271 public boolean hasModifier() { 2272 if (this.modifier == null) 2273 return false; 2274 for (Coding item : this.modifier) 2275 if (!item.isEmpty()) 2276 return true; 2277 return false; 2278 } 2279 2280 /** 2281 * @return {@link #modifier} (Item typification or modifiers codes, e.g. for 2282 * Oral whether the treatment is cosmetic or associated with TMJ, or an 2283 * appliance was lost or stolen.) 2284 */ 2285 // syntactic sugar 2286 public Coding addModifier() { // 3 2287 Coding t = new Coding(); 2288 if (this.modifier == null) 2289 this.modifier = new ArrayList<Coding>(); 2290 this.modifier.add(t); 2291 return t; 2292 } 2293 2294 // syntactic sugar 2295 public ItemsComponent addModifier(Coding t) { // 3 2296 if (t == null) 2297 return this; 2298 if (this.modifier == null) 2299 this.modifier = new ArrayList<Coding>(); 2300 this.modifier.add(t); 2301 return this; 2302 } 2303 2304 /** 2305 * @return {@link #detail} (Second tier of goods and services.) 2306 */ 2307 public List<DetailComponent> getDetail() { 2308 if (this.detail == null) 2309 this.detail = new ArrayList<DetailComponent>(); 2310 return this.detail; 2311 } 2312 2313 public boolean hasDetail() { 2314 if (this.detail == null) 2315 return false; 2316 for (DetailComponent item : this.detail) 2317 if (!item.isEmpty()) 2318 return true; 2319 return false; 2320 } 2321 2322 /** 2323 * @return {@link #detail} (Second tier of goods and services.) 2324 */ 2325 // syntactic sugar 2326 public DetailComponent addDetail() { // 3 2327 DetailComponent t = new DetailComponent(); 2328 if (this.detail == null) 2329 this.detail = new ArrayList<DetailComponent>(); 2330 this.detail.add(t); 2331 return t; 2332 } 2333 2334 // syntactic sugar 2335 public ItemsComponent addDetail(DetailComponent t) { // 3 2336 if (t == null) 2337 return this; 2338 if (this.detail == null) 2339 this.detail = new ArrayList<DetailComponent>(); 2340 this.detail.add(t); 2341 return this; 2342 } 2343 2344 /** 2345 * @return {@link #prosthesis} (The materials and placement date of prior fixed 2346 * prosthesis.) 2347 */ 2348 public ProsthesisComponent getProsthesis() { 2349 if (this.prosthesis == null) 2350 if (Configuration.errorOnAutoCreate()) 2351 throw new Error("Attempt to auto-create ItemsComponent.prosthesis"); 2352 else if (Configuration.doAutoCreate()) 2353 this.prosthesis = new ProsthesisComponent(); // cc 2354 return this.prosthesis; 2355 } 2356 2357 public boolean hasProsthesis() { 2358 return this.prosthesis != null && !this.prosthesis.isEmpty(); 2359 } 2360 2361 /** 2362 * @param value {@link #prosthesis} (The materials and placement date of prior 2363 * fixed prosthesis.) 2364 */ 2365 public ItemsComponent setProsthesis(ProsthesisComponent value) { 2366 this.prosthesis = value; 2367 return this; 2368 } 2369 2370 protected void listChildren(List<Property> childrenList) { 2371 super.listChildren(childrenList); 2372 childrenList.add( 2373 new Property("sequence", "positiveInt", "A service line number.", 0, java.lang.Integer.MAX_VALUE, sequence)); 2374 childrenList 2375 .add(new Property("type", "Coding", "The type of product or service.", 0, java.lang.Integer.MAX_VALUE, type)); 2376 childrenList.add(new Property("provider", "Reference(Practitioner)", 2377 "The practitioner who is responsible for the services rendered to the patient.", 0, 2378 java.lang.Integer.MAX_VALUE, provider)); 2379 childrenList.add(new Property("diagnosisLinkId", "positiveInt", 2380 "Diagnosis applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, diagnosisLinkId)); 2381 childrenList.add(new Property("service", "Coding", 2382 "If a grouping item then 'GROUP' otherwise it is a node therefore a code to indicate the Professional Service or Product supplied.", 2383 0, java.lang.Integer.MAX_VALUE, service)); 2384 childrenList.add(new Property("serviceDate", "date", 2385 "The date when the enclosed suite of services were performed or completed.", 0, java.lang.Integer.MAX_VALUE, 2386 serviceDate)); 2387 childrenList.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 2388 0, java.lang.Integer.MAX_VALUE, quantity)); 2389 childrenList.add(new Property("unitPrice", "Money", 2390 "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.", 2391 0, java.lang.Integer.MAX_VALUE, unitPrice)); 2392 childrenList.add(new Property("factor", "decimal", 2393 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 2394 0, java.lang.Integer.MAX_VALUE, factor)); 2395 childrenList.add(new Property("points", "decimal", 2396 "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the good or service delivered. The concept of Points allows for assignment of point values for services and/or goods, such that a monetary amount can be assigned to each point.", 2397 0, java.lang.Integer.MAX_VALUE, points)); 2398 childrenList.add(new Property("net", "Money", 2399 "The quantity times the unit price for an additional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 2400 0, java.lang.Integer.MAX_VALUE, net)); 2401 childrenList.add(new Property("udi", "Coding", 2402 "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 2403 childrenList.add(new Property("bodySite", "Coding", "Physical service site on the patient (limb, tooth, etc.).", 2404 0, java.lang.Integer.MAX_VALUE, bodySite)); 2405 childrenList.add( 2406 new Property("subSite", "Coding", "A region or surface of the site, e.g. limb region or tooth surface(s).", 0, 2407 java.lang.Integer.MAX_VALUE, subSite)); 2408 childrenList.add(new Property("modifier", "Coding", 2409 "Item typification or modifiers codes, e.g. for Oral whether the treatment is cosmetic or associated with TMJ, or an appliance was lost or stolen.", 2410 0, java.lang.Integer.MAX_VALUE, modifier)); 2411 childrenList.add( 2412 new Property("detail", "", "Second tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, detail)); 2413 childrenList.add(new Property("prosthesis", "", "The materials and placement date of prior fixed prosthesis.", 0, 2414 java.lang.Integer.MAX_VALUE, prosthesis)); 2415 } 2416 2417 @Override 2418 public void setProperty(String name, Base value) throws FHIRException { 2419 if (name.equals("sequence")) 2420 this.sequence = castToPositiveInt(value); // PositiveIntType 2421 else if (name.equals("type")) 2422 this.type = castToCoding(value); // Coding 2423 else if (name.equals("provider")) 2424 this.provider = castToReference(value); // Reference 2425 else if (name.equals("diagnosisLinkId")) 2426 this.getDiagnosisLinkId().add(castToPositiveInt(value)); 2427 else if (name.equals("service")) 2428 this.service = castToCoding(value); // Coding 2429 else if (name.equals("serviceDate")) 2430 this.serviceDate = castToDate(value); // DateType 2431 else if (name.equals("quantity")) 2432 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 2433 else if (name.equals("unitPrice")) 2434 this.unitPrice = castToMoney(value); // Money 2435 else if (name.equals("factor")) 2436 this.factor = castToDecimal(value); // DecimalType 2437 else if (name.equals("points")) 2438 this.points = castToDecimal(value); // DecimalType 2439 else if (name.equals("net")) 2440 this.net = castToMoney(value); // Money 2441 else if (name.equals("udi")) 2442 this.udi = castToCoding(value); // Coding 2443 else if (name.equals("bodySite")) 2444 this.bodySite = castToCoding(value); // Coding 2445 else if (name.equals("subSite")) 2446 this.getSubSite().add(castToCoding(value)); 2447 else if (name.equals("modifier")) 2448 this.getModifier().add(castToCoding(value)); 2449 else if (name.equals("detail")) 2450 this.getDetail().add((DetailComponent) value); 2451 else if (name.equals("prosthesis")) 2452 this.prosthesis = (ProsthesisComponent) value; // ProsthesisComponent 2453 else 2454 super.setProperty(name, value); 2455 } 2456 2457 @Override 2458 public Base addChild(String name) throws FHIRException { 2459 if (name.equals("sequence")) { 2460 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 2461 } else if (name.equals("type")) { 2462 this.type = new Coding(); 2463 return this.type; 2464 } else if (name.equals("provider")) { 2465 this.provider = new Reference(); 2466 return this.provider; 2467 } else if (name.equals("diagnosisLinkId")) { 2468 throw new FHIRException("Cannot call addChild on a singleton property Claim.diagnosisLinkId"); 2469 } else if (name.equals("service")) { 2470 this.service = new Coding(); 2471 return this.service; 2472 } else if (name.equals("serviceDate")) { 2473 throw new FHIRException("Cannot call addChild on a singleton property Claim.serviceDate"); 2474 } else if (name.equals("quantity")) { 2475 this.quantity = new SimpleQuantity(); 2476 return this.quantity; 2477 } else if (name.equals("unitPrice")) { 2478 this.unitPrice = new Money(); 2479 return this.unitPrice; 2480 } else if (name.equals("factor")) { 2481 throw new FHIRException("Cannot call addChild on a singleton property Claim.factor"); 2482 } else if (name.equals("points")) { 2483 throw new FHIRException("Cannot call addChild on a singleton property Claim.points"); 2484 } else if (name.equals("net")) { 2485 this.net = new Money(); 2486 return this.net; 2487 } else if (name.equals("udi")) { 2488 this.udi = new Coding(); 2489 return this.udi; 2490 } else if (name.equals("bodySite")) { 2491 this.bodySite = new Coding(); 2492 return this.bodySite; 2493 } else if (name.equals("subSite")) { 2494 return addSubSite(); 2495 } else if (name.equals("modifier")) { 2496 return addModifier(); 2497 } else if (name.equals("detail")) { 2498 return addDetail(); 2499 } else if (name.equals("prosthesis")) { 2500 this.prosthesis = new ProsthesisComponent(); 2501 return this.prosthesis; 2502 } else 2503 return super.addChild(name); 2504 } 2505 2506 public ItemsComponent copy() { 2507 ItemsComponent dst = new ItemsComponent(); 2508 copyValues(dst); 2509 dst.sequence = sequence == null ? null : sequence.copy(); 2510 dst.type = type == null ? null : type.copy(); 2511 dst.provider = provider == null ? null : provider.copy(); 2512 if (diagnosisLinkId != null) { 2513 dst.diagnosisLinkId = new ArrayList<PositiveIntType>(); 2514 for (PositiveIntType i : diagnosisLinkId) 2515 dst.diagnosisLinkId.add(i.copy()); 2516 } 2517 ; 2518 dst.service = service == null ? null : service.copy(); 2519 dst.serviceDate = serviceDate == null ? null : serviceDate.copy(); 2520 dst.quantity = quantity == null ? null : quantity.copy(); 2521 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 2522 dst.factor = factor == null ? null : factor.copy(); 2523 dst.points = points == null ? null : points.copy(); 2524 dst.net = net == null ? null : net.copy(); 2525 dst.udi = udi == null ? null : udi.copy(); 2526 dst.bodySite = bodySite == null ? null : bodySite.copy(); 2527 if (subSite != null) { 2528 dst.subSite = new ArrayList<Coding>(); 2529 for (Coding i : subSite) 2530 dst.subSite.add(i.copy()); 2531 } 2532 ; 2533 if (modifier != null) { 2534 dst.modifier = new ArrayList<Coding>(); 2535 for (Coding i : modifier) 2536 dst.modifier.add(i.copy()); 2537 } 2538 ; 2539 if (detail != null) { 2540 dst.detail = new ArrayList<DetailComponent>(); 2541 for (DetailComponent i : detail) 2542 dst.detail.add(i.copy()); 2543 } 2544 ; 2545 dst.prosthesis = prosthesis == null ? null : prosthesis.copy(); 2546 return dst; 2547 } 2548 2549 @Override 2550 public boolean equalsDeep(Base other) { 2551 if (!super.equalsDeep(other)) 2552 return false; 2553 if (!(other instanceof ItemsComponent)) 2554 return false; 2555 ItemsComponent o = (ItemsComponent) other; 2556 return compareDeep(sequence, o.sequence, true) && compareDeep(type, o.type, true) 2557 && compareDeep(provider, o.provider, true) && compareDeep(diagnosisLinkId, o.diagnosisLinkId, true) 2558 && compareDeep(service, o.service, true) && compareDeep(serviceDate, o.serviceDate, true) 2559 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 2560 && compareDeep(factor, o.factor, true) && compareDeep(points, o.points, true) && compareDeep(net, o.net, true) 2561 && compareDeep(udi, o.udi, true) && compareDeep(bodySite, o.bodySite, true) 2562 && compareDeep(subSite, o.subSite, true) && compareDeep(modifier, o.modifier, true) 2563 && compareDeep(detail, o.detail, true) && compareDeep(prosthesis, o.prosthesis, true); 2564 } 2565 2566 @Override 2567 public boolean equalsShallow(Base other) { 2568 if (!super.equalsShallow(other)) 2569 return false; 2570 if (!(other instanceof ItemsComponent)) 2571 return false; 2572 ItemsComponent o = (ItemsComponent) other; 2573 return compareValues(sequence, o.sequence, true) && compareValues(diagnosisLinkId, o.diagnosisLinkId, true) 2574 && compareValues(serviceDate, o.serviceDate, true) && compareValues(factor, o.factor, true) 2575 && compareValues(points, o.points, true); 2576 } 2577 2578 public boolean isEmpty() { 2579 return super.isEmpty() && (sequence == null || sequence.isEmpty()) && (type == null || type.isEmpty()) 2580 && (provider == null || provider.isEmpty()) && (diagnosisLinkId == null || diagnosisLinkId.isEmpty()) 2581 && (service == null || service.isEmpty()) && (serviceDate == null || serviceDate.isEmpty()) 2582 && (quantity == null || quantity.isEmpty()) && (unitPrice == null || unitPrice.isEmpty()) 2583 && (factor == null || factor.isEmpty()) && (points == null || points.isEmpty()) 2584 && (net == null || net.isEmpty()) && (udi == null || udi.isEmpty()) 2585 && (bodySite == null || bodySite.isEmpty()) && (subSite == null || subSite.isEmpty()) 2586 && (modifier == null || modifier.isEmpty()) && (detail == null || detail.isEmpty()) 2587 && (prosthesis == null || prosthesis.isEmpty()); 2588 } 2589 2590 public String fhirType() { 2591 return "Claim.item"; 2592 2593 } 2594 2595 } 2596 2597 @Block() 2598 public static class DetailComponent extends BackboneElement implements IBaseBackboneElement { 2599 /** 2600 * A service line number. 2601 */ 2602 @Child(name = "sequence", type = { 2603 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2604 @Description(shortDefinition = "Service instance", formalDefinition = "A service line number.") 2605 protected PositiveIntType sequence; 2606 2607 /** 2608 * The type of product or service. 2609 */ 2610 @Child(name = "type", type = { Coding.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 2611 @Description(shortDefinition = "Group or type of product or service", formalDefinition = "The type of product or service.") 2612 protected Coding type; 2613 2614 /** 2615 * If a grouping item then 'GROUP' otherwise it is a node therefore a code to 2616 * indicate the Professional Service or Product supplied. 2617 */ 2618 @Child(name = "service", type = { Coding.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 2619 @Description(shortDefinition = "Additional item codes", formalDefinition = "If a grouping item then 'GROUP' otherwise it is a node therefore a code to indicate the Professional Service or Product supplied.") 2620 protected Coding service; 2621 2622 /** 2623 * The number of repetitions of a service or product. 2624 */ 2625 @Child(name = "quantity", type = { 2626 SimpleQuantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 2627 @Description(shortDefinition = "Count of Products or Services", formalDefinition = "The number of repetitions of a service or product.") 2628 protected SimpleQuantity quantity; 2629 2630 /** 2631 * If the item is a node then this is the fee for the product or service, 2632 * otherwise this is the total of the fees for the children of the group. 2633 */ 2634 @Child(name = "unitPrice", type = { Money.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 2635 @Description(shortDefinition = "Fee, charge or cost per point", formalDefinition = "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.") 2636 protected Money unitPrice; 2637 2638 /** 2639 * A real number that represents a multiplier used in determining the overall 2640 * value of services delivered and/or goods received. The concept of a Factor 2641 * allows for a discount or surcharge multiplier to be applied to a monetary 2642 * amount. 2643 */ 2644 @Child(name = "factor", type = { DecimalType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 2645 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 2646 protected DecimalType factor; 2647 2648 /** 2649 * An amount that expresses the weighting (based on difficulty, cost and/or 2650 * resource intensiveness) associated with the good or service delivered. The 2651 * concept of Points allows for assignment of point values for services and/or 2652 * goods, such that a monetary amount can be assigned to each point. 2653 */ 2654 @Child(name = "points", type = { DecimalType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 2655 @Description(shortDefinition = "Difficulty scaling factor", formalDefinition = "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the good or service delivered. The concept of Points allows for assignment of point values for services and/or goods, such that a monetary amount can be assigned to each point.") 2656 protected DecimalType points; 2657 2658 /** 2659 * The quantity times the unit price for an additional service or product or 2660 * charge. For example, the formula: unit Quantity * unit Price (Cost per Point) 2661 * * factor Number * points = net Amount. Quantity, factor and points are 2662 * assumed to be 1 if not supplied. 2663 */ 2664 @Child(name = "net", type = { Money.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 2665 @Description(shortDefinition = "Total additional item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.") 2666 protected Money net; 2667 2668 /** 2669 * List of Unique Device Identifiers associated with this line item. 2670 */ 2671 @Child(name = "udi", type = { Coding.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 2672 @Description(shortDefinition = "Unique Device Identifier", formalDefinition = "List of Unique Device Identifiers associated with this line item.") 2673 protected Coding udi; 2674 2675 /** 2676 * Third tier of goods and services. 2677 */ 2678 @Child(name = "subDetail", type = {}, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2679 @Description(shortDefinition = "Additional items", formalDefinition = "Third tier of goods and services.") 2680 protected List<SubDetailComponent> subDetail; 2681 2682 private static final long serialVersionUID = 5768017L; 2683 2684 /* 2685 * Constructor 2686 */ 2687 public DetailComponent() { 2688 super(); 2689 } 2690 2691 /* 2692 * Constructor 2693 */ 2694 public DetailComponent(PositiveIntType sequence, Coding type, Coding service) { 2695 super(); 2696 this.sequence = sequence; 2697 this.type = type; 2698 this.service = service; 2699 } 2700 2701 /** 2702 * @return {@link #sequence} (A service line number.). This is the underlying 2703 * object with id, value and extensions. The accessor "getSequence" 2704 * gives direct access to the value 2705 */ 2706 public PositiveIntType getSequenceElement() { 2707 if (this.sequence == null) 2708 if (Configuration.errorOnAutoCreate()) 2709 throw new Error("Attempt to auto-create DetailComponent.sequence"); 2710 else if (Configuration.doAutoCreate()) 2711 this.sequence = new PositiveIntType(); // bb 2712 return this.sequence; 2713 } 2714 2715 public boolean hasSequenceElement() { 2716 return this.sequence != null && !this.sequence.isEmpty(); 2717 } 2718 2719 public boolean hasSequence() { 2720 return this.sequence != null && !this.sequence.isEmpty(); 2721 } 2722 2723 /** 2724 * @param value {@link #sequence} (A service line number.). This is the 2725 * underlying object with id, value and extensions. The accessor 2726 * "getSequence" gives direct access to the value 2727 */ 2728 public DetailComponent setSequenceElement(PositiveIntType value) { 2729 this.sequence = value; 2730 return this; 2731 } 2732 2733 /** 2734 * @return A service line number. 2735 */ 2736 public int getSequence() { 2737 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2738 } 2739 2740 /** 2741 * @param value A service line number. 2742 */ 2743 public DetailComponent setSequence(int value) { 2744 if (this.sequence == null) 2745 this.sequence = new PositiveIntType(); 2746 this.sequence.setValue(value); 2747 return this; 2748 } 2749 2750 /** 2751 * @return {@link #type} (The type of product or service.) 2752 */ 2753 public Coding getType() { 2754 if (this.type == null) 2755 if (Configuration.errorOnAutoCreate()) 2756 throw new Error("Attempt to auto-create DetailComponent.type"); 2757 else if (Configuration.doAutoCreate()) 2758 this.type = new Coding(); // cc 2759 return this.type; 2760 } 2761 2762 public boolean hasType() { 2763 return this.type != null && !this.type.isEmpty(); 2764 } 2765 2766 /** 2767 * @param value {@link #type} (The type of product or service.) 2768 */ 2769 public DetailComponent setType(Coding value) { 2770 this.type = value; 2771 return this; 2772 } 2773 2774 /** 2775 * @return {@link #service} (If a grouping item then 'GROUP' otherwise it is a 2776 * node therefore a code to indicate the Professional Service or Product 2777 * supplied.) 2778 */ 2779 public Coding getService() { 2780 if (this.service == null) 2781 if (Configuration.errorOnAutoCreate()) 2782 throw new Error("Attempt to auto-create DetailComponent.service"); 2783 else if (Configuration.doAutoCreate()) 2784 this.service = new Coding(); // cc 2785 return this.service; 2786 } 2787 2788 public boolean hasService() { 2789 return this.service != null && !this.service.isEmpty(); 2790 } 2791 2792 /** 2793 * @param value {@link #service} (If a grouping item then 'GROUP' otherwise it 2794 * is a node therefore a code to indicate the Professional Service 2795 * or Product supplied.) 2796 */ 2797 public DetailComponent setService(Coding value) { 2798 this.service = value; 2799 return this; 2800 } 2801 2802 /** 2803 * @return {@link #quantity} (The number of repetitions of a service or 2804 * product.) 2805 */ 2806 public SimpleQuantity getQuantity() { 2807 if (this.quantity == null) 2808 if (Configuration.errorOnAutoCreate()) 2809 throw new Error("Attempt to auto-create DetailComponent.quantity"); 2810 else if (Configuration.doAutoCreate()) 2811 this.quantity = new SimpleQuantity(); // cc 2812 return this.quantity; 2813 } 2814 2815 public boolean hasQuantity() { 2816 return this.quantity != null && !this.quantity.isEmpty(); 2817 } 2818 2819 /** 2820 * @param value {@link #quantity} (The number of repetitions of a service or 2821 * product.) 2822 */ 2823 public DetailComponent setQuantity(SimpleQuantity value) { 2824 this.quantity = value; 2825 return this; 2826 } 2827 2828 /** 2829 * @return {@link #unitPrice} (If the item is a node then this is the fee for 2830 * the product or service, otherwise this is the total of the fees for 2831 * the children of the group.) 2832 */ 2833 public Money getUnitPrice() { 2834 if (this.unitPrice == null) 2835 if (Configuration.errorOnAutoCreate()) 2836 throw new Error("Attempt to auto-create DetailComponent.unitPrice"); 2837 else if (Configuration.doAutoCreate()) 2838 this.unitPrice = new Money(); // cc 2839 return this.unitPrice; 2840 } 2841 2842 public boolean hasUnitPrice() { 2843 return this.unitPrice != null && !this.unitPrice.isEmpty(); 2844 } 2845 2846 /** 2847 * @param value {@link #unitPrice} (If the item is a node then this is the fee 2848 * for the product or service, otherwise this is the total of the 2849 * fees for the children of the group.) 2850 */ 2851 public DetailComponent setUnitPrice(Money value) { 2852 this.unitPrice = value; 2853 return this; 2854 } 2855 2856 /** 2857 * @return {@link #factor} (A real number that represents a multiplier used in 2858 * determining the overall value of services delivered and/or goods 2859 * received. The concept of a Factor allows for a discount or surcharge 2860 * multiplier to be applied to a monetary amount.). This is the 2861 * underlying object with id, value and extensions. The accessor 2862 * "getFactor" gives direct access to the value 2863 */ 2864 public DecimalType getFactorElement() { 2865 if (this.factor == null) 2866 if (Configuration.errorOnAutoCreate()) 2867 throw new Error("Attempt to auto-create DetailComponent.factor"); 2868 else if (Configuration.doAutoCreate()) 2869 this.factor = new DecimalType(); // bb 2870 return this.factor; 2871 } 2872 2873 public boolean hasFactorElement() { 2874 return this.factor != null && !this.factor.isEmpty(); 2875 } 2876 2877 public boolean hasFactor() { 2878 return this.factor != null && !this.factor.isEmpty(); 2879 } 2880 2881 /** 2882 * @param value {@link #factor} (A real number that represents a multiplier used 2883 * in determining the overall value of services delivered and/or 2884 * goods received. The concept of a Factor allows for a discount or 2885 * surcharge multiplier to be applied to a monetary amount.). This 2886 * is the underlying object with id, value and extensions. The 2887 * accessor "getFactor" gives direct access to the value 2888 */ 2889 public DetailComponent setFactorElement(DecimalType value) { 2890 this.factor = value; 2891 return this; 2892 } 2893 2894 /** 2895 * @return A real number that represents a multiplier used in determining the 2896 * overall value of services delivered and/or goods received. The 2897 * concept of a Factor allows for a discount or surcharge multiplier to 2898 * be applied to a monetary amount. 2899 */ 2900 public BigDecimal getFactor() { 2901 return this.factor == null ? null : this.factor.getValue(); 2902 } 2903 2904 /** 2905 * @param value A real number that represents a multiplier used in determining 2906 * the overall value of services delivered and/or goods received. 2907 * The concept of a Factor allows for a discount or surcharge 2908 * multiplier to be applied to a monetary amount. 2909 */ 2910 public DetailComponent setFactor(BigDecimal value) { 2911 if (value == null) 2912 this.factor = null; 2913 else { 2914 if (this.factor == null) 2915 this.factor = new DecimalType(); 2916 this.factor.setValue(value); 2917 } 2918 return this; 2919 } 2920 2921 /** 2922 * @return {@link #points} (An amount that expresses the weighting (based on 2923 * difficulty, cost and/or resource intensiveness) associated with the 2924 * good or service delivered. The concept of Points allows for 2925 * assignment of point values for services and/or goods, such that a 2926 * monetary amount can be assigned to each point.). This is the 2927 * underlying object with id, value and extensions. The accessor 2928 * "getPoints" gives direct access to the value 2929 */ 2930 public DecimalType getPointsElement() { 2931 if (this.points == null) 2932 if (Configuration.errorOnAutoCreate()) 2933 throw new Error("Attempt to auto-create DetailComponent.points"); 2934 else if (Configuration.doAutoCreate()) 2935 this.points = new DecimalType(); // bb 2936 return this.points; 2937 } 2938 2939 public boolean hasPointsElement() { 2940 return this.points != null && !this.points.isEmpty(); 2941 } 2942 2943 public boolean hasPoints() { 2944 return this.points != null && !this.points.isEmpty(); 2945 } 2946 2947 /** 2948 * @param value {@link #points} (An amount that expresses the weighting (based 2949 * on difficulty, cost and/or resource intensiveness) associated 2950 * with the good or service delivered. The concept of Points allows 2951 * for assignment of point values for services and/or goods, such 2952 * that a monetary amount can be assigned to each point.). This is 2953 * the underlying object with id, value and extensions. The 2954 * accessor "getPoints" gives direct access to the value 2955 */ 2956 public DetailComponent setPointsElement(DecimalType value) { 2957 this.points = value; 2958 return this; 2959 } 2960 2961 /** 2962 * @return An amount that expresses the weighting (based on difficulty, cost 2963 * and/or resource intensiveness) associated with the good or service 2964 * delivered. The concept of Points allows for assignment of point 2965 * values for services and/or goods, such that a monetary amount can be 2966 * assigned to each point. 2967 */ 2968 public BigDecimal getPoints() { 2969 return this.points == null ? null : this.points.getValue(); 2970 } 2971 2972 /** 2973 * @param value An amount that expresses the weighting (based on difficulty, 2974 * cost and/or resource intensiveness) associated with the good or 2975 * service delivered. The concept of Points allows for assignment 2976 * of point values for services and/or goods, such that a monetary 2977 * amount can be assigned to each point. 2978 */ 2979 public DetailComponent setPoints(BigDecimal value) { 2980 if (value == null) 2981 this.points = null; 2982 else { 2983 if (this.points == null) 2984 this.points = new DecimalType(); 2985 this.points.setValue(value); 2986 } 2987 return this; 2988 } 2989 2990 /** 2991 * @return {@link #net} (The quantity times the unit price for an additional 2992 * service or product or charge. For example, the formula: unit Quantity 2993 * * unit Price (Cost per Point) * factor Number * points = net Amount. 2994 * Quantity, factor and points are assumed to be 1 if not supplied.) 2995 */ 2996 public Money getNet() { 2997 if (this.net == null) 2998 if (Configuration.errorOnAutoCreate()) 2999 throw new Error("Attempt to auto-create DetailComponent.net"); 3000 else if (Configuration.doAutoCreate()) 3001 this.net = new Money(); // cc 3002 return this.net; 3003 } 3004 3005 public boolean hasNet() { 3006 return this.net != null && !this.net.isEmpty(); 3007 } 3008 3009 /** 3010 * @param value {@link #net} (The quantity times the unit price for an 3011 * additional service or product or charge. For example, the 3012 * formula: unit Quantity * unit Price (Cost per Point) * factor 3013 * Number * points = net Amount. Quantity, factor and points are 3014 * assumed to be 1 if not supplied.) 3015 */ 3016 public DetailComponent setNet(Money value) { 3017 this.net = value; 3018 return this; 3019 } 3020 3021 /** 3022 * @return {@link #udi} (List of Unique Device Identifiers associated with this 3023 * line item.) 3024 */ 3025 public Coding getUdi() { 3026 if (this.udi == null) 3027 if (Configuration.errorOnAutoCreate()) 3028 throw new Error("Attempt to auto-create DetailComponent.udi"); 3029 else if (Configuration.doAutoCreate()) 3030 this.udi = new Coding(); // cc 3031 return this.udi; 3032 } 3033 3034 public boolean hasUdi() { 3035 return this.udi != null && !this.udi.isEmpty(); 3036 } 3037 3038 /** 3039 * @param value {@link #udi} (List of Unique Device Identifiers associated with 3040 * this line item.) 3041 */ 3042 public DetailComponent setUdi(Coding value) { 3043 this.udi = value; 3044 return this; 3045 } 3046 3047 /** 3048 * @return {@link #subDetail} (Third tier of goods and services.) 3049 */ 3050 public List<SubDetailComponent> getSubDetail() { 3051 if (this.subDetail == null) 3052 this.subDetail = new ArrayList<SubDetailComponent>(); 3053 return this.subDetail; 3054 } 3055 3056 public boolean hasSubDetail() { 3057 if (this.subDetail == null) 3058 return false; 3059 for (SubDetailComponent item : this.subDetail) 3060 if (!item.isEmpty()) 3061 return true; 3062 return false; 3063 } 3064 3065 /** 3066 * @return {@link #subDetail} (Third tier of goods and services.) 3067 */ 3068 // syntactic sugar 3069 public SubDetailComponent addSubDetail() { // 3 3070 SubDetailComponent t = new SubDetailComponent(); 3071 if (this.subDetail == null) 3072 this.subDetail = new ArrayList<SubDetailComponent>(); 3073 this.subDetail.add(t); 3074 return t; 3075 } 3076 3077 // syntactic sugar 3078 public DetailComponent addSubDetail(SubDetailComponent t) { // 3 3079 if (t == null) 3080 return this; 3081 if (this.subDetail == null) 3082 this.subDetail = new ArrayList<SubDetailComponent>(); 3083 this.subDetail.add(t); 3084 return this; 3085 } 3086 3087 protected void listChildren(List<Property> childrenList) { 3088 super.listChildren(childrenList); 3089 childrenList.add( 3090 new Property("sequence", "positiveInt", "A service line number.", 0, java.lang.Integer.MAX_VALUE, sequence)); 3091 childrenList 3092 .add(new Property("type", "Coding", "The type of product or service.", 0, java.lang.Integer.MAX_VALUE, type)); 3093 childrenList.add(new Property("service", "Coding", 3094 "If a grouping item then 'GROUP' otherwise it is a node therefore a code to indicate the Professional Service or Product supplied.", 3095 0, java.lang.Integer.MAX_VALUE, service)); 3096 childrenList.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 3097 0, java.lang.Integer.MAX_VALUE, quantity)); 3098 childrenList.add(new Property("unitPrice", "Money", 3099 "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.", 3100 0, java.lang.Integer.MAX_VALUE, unitPrice)); 3101 childrenList.add(new Property("factor", "decimal", 3102 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 3103 0, java.lang.Integer.MAX_VALUE, factor)); 3104 childrenList.add(new Property("points", "decimal", 3105 "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the good or service delivered. The concept of Points allows for assignment of point values for services and/or goods, such that a monetary amount can be assigned to each point.", 3106 0, java.lang.Integer.MAX_VALUE, points)); 3107 childrenList.add(new Property("net", "Money", 3108 "The quantity times the unit price for an additional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 3109 0, java.lang.Integer.MAX_VALUE, net)); 3110 childrenList.add(new Property("udi", "Coding", 3111 "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 3112 childrenList.add(new Property("subDetail", "", "Third tier of goods and services.", 0, 3113 java.lang.Integer.MAX_VALUE, subDetail)); 3114 } 3115 3116 @Override 3117 public void setProperty(String name, Base value) throws FHIRException { 3118 if (name.equals("sequence")) 3119 this.sequence = castToPositiveInt(value); // PositiveIntType 3120 else if (name.equals("type")) 3121 this.type = castToCoding(value); // Coding 3122 else if (name.equals("service")) 3123 this.service = castToCoding(value); // Coding 3124 else if (name.equals("quantity")) 3125 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 3126 else if (name.equals("unitPrice")) 3127 this.unitPrice = castToMoney(value); // Money 3128 else if (name.equals("factor")) 3129 this.factor = castToDecimal(value); // DecimalType 3130 else if (name.equals("points")) 3131 this.points = castToDecimal(value); // DecimalType 3132 else if (name.equals("net")) 3133 this.net = castToMoney(value); // Money 3134 else if (name.equals("udi")) 3135 this.udi = castToCoding(value); // Coding 3136 else if (name.equals("subDetail")) 3137 this.getSubDetail().add((SubDetailComponent) value); 3138 else 3139 super.setProperty(name, value); 3140 } 3141 3142 @Override 3143 public Base addChild(String name) throws FHIRException { 3144 if (name.equals("sequence")) { 3145 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 3146 } else if (name.equals("type")) { 3147 this.type = new Coding(); 3148 return this.type; 3149 } else if (name.equals("service")) { 3150 this.service = new Coding(); 3151 return this.service; 3152 } else if (name.equals("quantity")) { 3153 this.quantity = new SimpleQuantity(); 3154 return this.quantity; 3155 } else if (name.equals("unitPrice")) { 3156 this.unitPrice = new Money(); 3157 return this.unitPrice; 3158 } else if (name.equals("factor")) { 3159 throw new FHIRException("Cannot call addChild on a singleton property Claim.factor"); 3160 } else if (name.equals("points")) { 3161 throw new FHIRException("Cannot call addChild on a singleton property Claim.points"); 3162 } else if (name.equals("net")) { 3163 this.net = new Money(); 3164 return this.net; 3165 } else if (name.equals("udi")) { 3166 this.udi = new Coding(); 3167 return this.udi; 3168 } else if (name.equals("subDetail")) { 3169 return addSubDetail(); 3170 } else 3171 return super.addChild(name); 3172 } 3173 3174 public DetailComponent copy() { 3175 DetailComponent dst = new DetailComponent(); 3176 copyValues(dst); 3177 dst.sequence = sequence == null ? null : sequence.copy(); 3178 dst.type = type == null ? null : type.copy(); 3179 dst.service = service == null ? null : service.copy(); 3180 dst.quantity = quantity == null ? null : quantity.copy(); 3181 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 3182 dst.factor = factor == null ? null : factor.copy(); 3183 dst.points = points == null ? null : points.copy(); 3184 dst.net = net == null ? null : net.copy(); 3185 dst.udi = udi == null ? null : udi.copy(); 3186 if (subDetail != null) { 3187 dst.subDetail = new ArrayList<SubDetailComponent>(); 3188 for (SubDetailComponent i : subDetail) 3189 dst.subDetail.add(i.copy()); 3190 } 3191 ; 3192 return dst; 3193 } 3194 3195 @Override 3196 public boolean equalsDeep(Base other) { 3197 if (!super.equalsDeep(other)) 3198 return false; 3199 if (!(other instanceof DetailComponent)) 3200 return false; 3201 DetailComponent o = (DetailComponent) other; 3202 return compareDeep(sequence, o.sequence, true) && compareDeep(type, o.type, true) 3203 && compareDeep(service, o.service, true) && compareDeep(quantity, o.quantity, true) 3204 && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 3205 && compareDeep(points, o.points, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 3206 && compareDeep(subDetail, o.subDetail, true); 3207 } 3208 3209 @Override 3210 public boolean equalsShallow(Base other) { 3211 if (!super.equalsShallow(other)) 3212 return false; 3213 if (!(other instanceof DetailComponent)) 3214 return false; 3215 DetailComponent o = (DetailComponent) other; 3216 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true) 3217 && compareValues(points, o.points, true); 3218 } 3219 3220 public boolean isEmpty() { 3221 return super.isEmpty() && (sequence == null || sequence.isEmpty()) && (type == null || type.isEmpty()) 3222 && (service == null || service.isEmpty()) && (quantity == null || quantity.isEmpty()) 3223 && (unitPrice == null || unitPrice.isEmpty()) && (factor == null || factor.isEmpty()) 3224 && (points == null || points.isEmpty()) && (net == null || net.isEmpty()) && (udi == null || udi.isEmpty()) 3225 && (subDetail == null || subDetail.isEmpty()); 3226 } 3227 3228 public String fhirType() { 3229 return "Claim.item.detail"; 3230 3231 } 3232 3233 } 3234 3235 @Block() 3236 public static class SubDetailComponent extends BackboneElement implements IBaseBackboneElement { 3237 /** 3238 * A service line number. 3239 */ 3240 @Child(name = "sequence", type = { 3241 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 3242 @Description(shortDefinition = "Service instance", formalDefinition = "A service line number.") 3243 protected PositiveIntType sequence; 3244 3245 /** 3246 * The type of product or service. 3247 */ 3248 @Child(name = "type", type = { Coding.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 3249 @Description(shortDefinition = "Type of product or service", formalDefinition = "The type of product or service.") 3250 protected Coding type; 3251 3252 /** 3253 * The fee for an additional service or product or charge. 3254 */ 3255 @Child(name = "service", type = { Coding.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 3256 @Description(shortDefinition = "Additional item codes", formalDefinition = "The fee for an additional service or product or charge.") 3257 protected Coding service; 3258 3259 /** 3260 * The number of repetitions of a service or product. 3261 */ 3262 @Child(name = "quantity", type = { 3263 SimpleQuantity.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 3264 @Description(shortDefinition = "Count of Products or Services", formalDefinition = "The number of repetitions of a service or product.") 3265 protected SimpleQuantity quantity; 3266 3267 /** 3268 * The fee for an additional service or product or charge. 3269 */ 3270 @Child(name = "unitPrice", type = { Money.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 3271 @Description(shortDefinition = "Fee, charge or cost per point", formalDefinition = "The fee for an additional service or product or charge.") 3272 protected Money unitPrice; 3273 3274 /** 3275 * A real number that represents a multiplier used in determining the overall 3276 * value of services delivered and/or goods received. The concept of a Factor 3277 * allows for a discount or surcharge multiplier to be applied to a monetary 3278 * amount. 3279 */ 3280 @Child(name = "factor", type = { DecimalType.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 3281 @Description(shortDefinition = "Price scaling factor", formalDefinition = "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.") 3282 protected DecimalType factor; 3283 3284 /** 3285 * An amount that expresses the weighting (based on difficulty, cost and/or 3286 * resource intensiveness) associated with the good or service delivered. The 3287 * concept of Points allows for assignment of point values for services and/or 3288 * goods, such that a monetary amount can be assigned to each point. 3289 */ 3290 @Child(name = "points", type = { DecimalType.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 3291 @Description(shortDefinition = "Difficulty scaling factor", formalDefinition = "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the good or service delivered. The concept of Points allows for assignment of point values for services and/or goods, such that a monetary amount can be assigned to each point.") 3292 protected DecimalType points; 3293 3294 /** 3295 * The quantity times the unit price for an additional service or product or 3296 * charge. For example, the formula: unit Quantity * unit Price (Cost per Point) 3297 * * factor Number * points = net Amount. Quantity, factor and points are 3298 * assumed to be 1 if not supplied. 3299 */ 3300 @Child(name = "net", type = { Money.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 3301 @Description(shortDefinition = "Net additional item cost", formalDefinition = "The quantity times the unit price for an additional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.") 3302 protected Money net; 3303 3304 /** 3305 * List of Unique Device Identifiers associated with this line item. 3306 */ 3307 @Child(name = "udi", type = { Coding.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 3308 @Description(shortDefinition = "Unique Device Identifier", formalDefinition = "List of Unique Device Identifiers associated with this line item.") 3309 protected Coding udi; 3310 3311 private static final long serialVersionUID = 623567568L; 3312 3313 /* 3314 * Constructor 3315 */ 3316 public SubDetailComponent() { 3317 super(); 3318 } 3319 3320 /* 3321 * Constructor 3322 */ 3323 public SubDetailComponent(PositiveIntType sequence, Coding type, Coding service) { 3324 super(); 3325 this.sequence = sequence; 3326 this.type = type; 3327 this.service = service; 3328 } 3329 3330 /** 3331 * @return {@link #sequence} (A service line number.). This is the underlying 3332 * object with id, value and extensions. The accessor "getSequence" 3333 * gives direct access to the value 3334 */ 3335 public PositiveIntType getSequenceElement() { 3336 if (this.sequence == null) 3337 if (Configuration.errorOnAutoCreate()) 3338 throw new Error("Attempt to auto-create SubDetailComponent.sequence"); 3339 else if (Configuration.doAutoCreate()) 3340 this.sequence = new PositiveIntType(); // bb 3341 return this.sequence; 3342 } 3343 3344 public boolean hasSequenceElement() { 3345 return this.sequence != null && !this.sequence.isEmpty(); 3346 } 3347 3348 public boolean hasSequence() { 3349 return this.sequence != null && !this.sequence.isEmpty(); 3350 } 3351 3352 /** 3353 * @param value {@link #sequence} (A service line number.). This is the 3354 * underlying object with id, value and extensions. The accessor 3355 * "getSequence" gives direct access to the value 3356 */ 3357 public SubDetailComponent setSequenceElement(PositiveIntType value) { 3358 this.sequence = value; 3359 return this; 3360 } 3361 3362 /** 3363 * @return A service line number. 3364 */ 3365 public int getSequence() { 3366 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 3367 } 3368 3369 /** 3370 * @param value A service line number. 3371 */ 3372 public SubDetailComponent setSequence(int value) { 3373 if (this.sequence == null) 3374 this.sequence = new PositiveIntType(); 3375 this.sequence.setValue(value); 3376 return this; 3377 } 3378 3379 /** 3380 * @return {@link #type} (The type of product or service.) 3381 */ 3382 public Coding getType() { 3383 if (this.type == null) 3384 if (Configuration.errorOnAutoCreate()) 3385 throw new Error("Attempt to auto-create SubDetailComponent.type"); 3386 else if (Configuration.doAutoCreate()) 3387 this.type = new Coding(); // cc 3388 return this.type; 3389 } 3390 3391 public boolean hasType() { 3392 return this.type != null && !this.type.isEmpty(); 3393 } 3394 3395 /** 3396 * @param value {@link #type} (The type of product or service.) 3397 */ 3398 public SubDetailComponent setType(Coding value) { 3399 this.type = value; 3400 return this; 3401 } 3402 3403 /** 3404 * @return {@link #service} (The fee for an additional service or product or 3405 * charge.) 3406 */ 3407 public Coding getService() { 3408 if (this.service == null) 3409 if (Configuration.errorOnAutoCreate()) 3410 throw new Error("Attempt to auto-create SubDetailComponent.service"); 3411 else if (Configuration.doAutoCreate()) 3412 this.service = new Coding(); // cc 3413 return this.service; 3414 } 3415 3416 public boolean hasService() { 3417 return this.service != null && !this.service.isEmpty(); 3418 } 3419 3420 /** 3421 * @param value {@link #service} (The fee for an additional service or product 3422 * or charge.) 3423 */ 3424 public SubDetailComponent setService(Coding value) { 3425 this.service = value; 3426 return this; 3427 } 3428 3429 /** 3430 * @return {@link #quantity} (The number of repetitions of a service or 3431 * product.) 3432 */ 3433 public SimpleQuantity getQuantity() { 3434 if (this.quantity == null) 3435 if (Configuration.errorOnAutoCreate()) 3436 throw new Error("Attempt to auto-create SubDetailComponent.quantity"); 3437 else if (Configuration.doAutoCreate()) 3438 this.quantity = new SimpleQuantity(); // cc 3439 return this.quantity; 3440 } 3441 3442 public boolean hasQuantity() { 3443 return this.quantity != null && !this.quantity.isEmpty(); 3444 } 3445 3446 /** 3447 * @param value {@link #quantity} (The number of repetitions of a service or 3448 * product.) 3449 */ 3450 public SubDetailComponent setQuantity(SimpleQuantity value) { 3451 this.quantity = value; 3452 return this; 3453 } 3454 3455 /** 3456 * @return {@link #unitPrice} (The fee for an additional service or product or 3457 * charge.) 3458 */ 3459 public Money getUnitPrice() { 3460 if (this.unitPrice == null) 3461 if (Configuration.errorOnAutoCreate()) 3462 throw new Error("Attempt to auto-create SubDetailComponent.unitPrice"); 3463 else if (Configuration.doAutoCreate()) 3464 this.unitPrice = new Money(); // cc 3465 return this.unitPrice; 3466 } 3467 3468 public boolean hasUnitPrice() { 3469 return this.unitPrice != null && !this.unitPrice.isEmpty(); 3470 } 3471 3472 /** 3473 * @param value {@link #unitPrice} (The fee for an additional service or product 3474 * or charge.) 3475 */ 3476 public SubDetailComponent setUnitPrice(Money value) { 3477 this.unitPrice = value; 3478 return this; 3479 } 3480 3481 /** 3482 * @return {@link #factor} (A real number that represents a multiplier used in 3483 * determining the overall value of services delivered and/or goods 3484 * received. The concept of a Factor allows for a discount or surcharge 3485 * multiplier to be applied to a monetary amount.). This is the 3486 * underlying object with id, value and extensions. The accessor 3487 * "getFactor" gives direct access to the value 3488 */ 3489 public DecimalType getFactorElement() { 3490 if (this.factor == null) 3491 if (Configuration.errorOnAutoCreate()) 3492 throw new Error("Attempt to auto-create SubDetailComponent.factor"); 3493 else if (Configuration.doAutoCreate()) 3494 this.factor = new DecimalType(); // bb 3495 return this.factor; 3496 } 3497 3498 public boolean hasFactorElement() { 3499 return this.factor != null && !this.factor.isEmpty(); 3500 } 3501 3502 public boolean hasFactor() { 3503 return this.factor != null && !this.factor.isEmpty(); 3504 } 3505 3506 /** 3507 * @param value {@link #factor} (A real number that represents a multiplier used 3508 * in determining the overall value of services delivered and/or 3509 * goods received. The concept of a Factor allows for a discount or 3510 * surcharge multiplier to be applied to a monetary amount.). This 3511 * is the underlying object with id, value and extensions. The 3512 * accessor "getFactor" gives direct access to the value 3513 */ 3514 public SubDetailComponent setFactorElement(DecimalType value) { 3515 this.factor = value; 3516 return this; 3517 } 3518 3519 /** 3520 * @return A real number that represents a multiplier used in determining the 3521 * overall value of services delivered and/or goods received. The 3522 * concept of a Factor allows for a discount or surcharge multiplier to 3523 * be applied to a monetary amount. 3524 */ 3525 public BigDecimal getFactor() { 3526 return this.factor == null ? null : this.factor.getValue(); 3527 } 3528 3529 /** 3530 * @param value A real number that represents a multiplier used in determining 3531 * the overall value of services delivered and/or goods received. 3532 * The concept of a Factor allows for a discount or surcharge 3533 * multiplier to be applied to a monetary amount. 3534 */ 3535 public SubDetailComponent setFactor(BigDecimal value) { 3536 if (value == null) 3537 this.factor = null; 3538 else { 3539 if (this.factor == null) 3540 this.factor = new DecimalType(); 3541 this.factor.setValue(value); 3542 } 3543 return this; 3544 } 3545 3546 /** 3547 * @return {@link #points} (An amount that expresses the weighting (based on 3548 * difficulty, cost and/or resource intensiveness) associated with the 3549 * good or service delivered. The concept of Points allows for 3550 * assignment of point values for services and/or goods, such that a 3551 * monetary amount can be assigned to each point.). This is the 3552 * underlying object with id, value and extensions. The accessor 3553 * "getPoints" gives direct access to the value 3554 */ 3555 public DecimalType getPointsElement() { 3556 if (this.points == null) 3557 if (Configuration.errorOnAutoCreate()) 3558 throw new Error("Attempt to auto-create SubDetailComponent.points"); 3559 else if (Configuration.doAutoCreate()) 3560 this.points = new DecimalType(); // bb 3561 return this.points; 3562 } 3563 3564 public boolean hasPointsElement() { 3565 return this.points != null && !this.points.isEmpty(); 3566 } 3567 3568 public boolean hasPoints() { 3569 return this.points != null && !this.points.isEmpty(); 3570 } 3571 3572 /** 3573 * @param value {@link #points} (An amount that expresses the weighting (based 3574 * on difficulty, cost and/or resource intensiveness) associated 3575 * with the good or service delivered. The concept of Points allows 3576 * for assignment of point values for services and/or goods, such 3577 * that a monetary amount can be assigned to each point.). This is 3578 * the underlying object with id, value and extensions. The 3579 * accessor "getPoints" gives direct access to the value 3580 */ 3581 public SubDetailComponent setPointsElement(DecimalType value) { 3582 this.points = value; 3583 return this; 3584 } 3585 3586 /** 3587 * @return An amount that expresses the weighting (based on difficulty, cost 3588 * and/or resource intensiveness) associated with the good or service 3589 * delivered. The concept of Points allows for assignment of point 3590 * values for services and/or goods, such that a monetary amount can be 3591 * assigned to each point. 3592 */ 3593 public BigDecimal getPoints() { 3594 return this.points == null ? null : this.points.getValue(); 3595 } 3596 3597 /** 3598 * @param value An amount that expresses the weighting (based on difficulty, 3599 * cost and/or resource intensiveness) associated with the good or 3600 * service delivered. The concept of Points allows for assignment 3601 * of point values for services and/or goods, such that a monetary 3602 * amount can be assigned to each point. 3603 */ 3604 public SubDetailComponent setPoints(BigDecimal value) { 3605 if (value == null) 3606 this.points = null; 3607 else { 3608 if (this.points == null) 3609 this.points = new DecimalType(); 3610 this.points.setValue(value); 3611 } 3612 return this; 3613 } 3614 3615 /** 3616 * @return {@link #net} (The quantity times the unit price for an additional 3617 * service or product or charge. For example, the formula: unit Quantity 3618 * * unit Price (Cost per Point) * factor Number * points = net Amount. 3619 * Quantity, factor and points are assumed to be 1 if not supplied.) 3620 */ 3621 public Money getNet() { 3622 if (this.net == null) 3623 if (Configuration.errorOnAutoCreate()) 3624 throw new Error("Attempt to auto-create SubDetailComponent.net"); 3625 else if (Configuration.doAutoCreate()) 3626 this.net = new Money(); // cc 3627 return this.net; 3628 } 3629 3630 public boolean hasNet() { 3631 return this.net != null && !this.net.isEmpty(); 3632 } 3633 3634 /** 3635 * @param value {@link #net} (The quantity times the unit price for an 3636 * additional service or product or charge. For example, the 3637 * formula: unit Quantity * unit Price (Cost per Point) * factor 3638 * Number * points = net Amount. Quantity, factor and points are 3639 * assumed to be 1 if not supplied.) 3640 */ 3641 public SubDetailComponent setNet(Money value) { 3642 this.net = value; 3643 return this; 3644 } 3645 3646 /** 3647 * @return {@link #udi} (List of Unique Device Identifiers associated with this 3648 * line item.) 3649 */ 3650 public Coding getUdi() { 3651 if (this.udi == null) 3652 if (Configuration.errorOnAutoCreate()) 3653 throw new Error("Attempt to auto-create SubDetailComponent.udi"); 3654 else if (Configuration.doAutoCreate()) 3655 this.udi = new Coding(); // cc 3656 return this.udi; 3657 } 3658 3659 public boolean hasUdi() { 3660 return this.udi != null && !this.udi.isEmpty(); 3661 } 3662 3663 /** 3664 * @param value {@link #udi} (List of Unique Device Identifiers associated with 3665 * this line item.) 3666 */ 3667 public SubDetailComponent setUdi(Coding value) { 3668 this.udi = value; 3669 return this; 3670 } 3671 3672 protected void listChildren(List<Property> childrenList) { 3673 super.listChildren(childrenList); 3674 childrenList.add( 3675 new Property("sequence", "positiveInt", "A service line number.", 0, java.lang.Integer.MAX_VALUE, sequence)); 3676 childrenList 3677 .add(new Property("type", "Coding", "The type of product or service.", 0, java.lang.Integer.MAX_VALUE, type)); 3678 childrenList.add(new Property("service", "Coding", "The fee for an additional service or product or charge.", 0, 3679 java.lang.Integer.MAX_VALUE, service)); 3680 childrenList.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 3681 0, java.lang.Integer.MAX_VALUE, quantity)); 3682 childrenList.add(new Property("unitPrice", "Money", "The fee for an additional service or product or charge.", 0, 3683 java.lang.Integer.MAX_VALUE, unitPrice)); 3684 childrenList.add(new Property("factor", "decimal", 3685 "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 3686 0, java.lang.Integer.MAX_VALUE, factor)); 3687 childrenList.add(new Property("points", "decimal", 3688 "An amount that expresses the weighting (based on difficulty, cost and/or resource intensiveness) associated with the good or service delivered. The concept of Points allows for assignment of point values for services and/or goods, such that a monetary amount can be assigned to each point.", 3689 0, java.lang.Integer.MAX_VALUE, points)); 3690 childrenList.add(new Property("net", "Money", 3691 "The quantity times the unit price for an additional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 3692 0, java.lang.Integer.MAX_VALUE, net)); 3693 childrenList.add(new Property("udi", "Coding", 3694 "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 3695 } 3696 3697 @Override 3698 public void setProperty(String name, Base value) throws FHIRException { 3699 if (name.equals("sequence")) 3700 this.sequence = castToPositiveInt(value); // PositiveIntType 3701 else if (name.equals("type")) 3702 this.type = castToCoding(value); // Coding 3703 else if (name.equals("service")) 3704 this.service = castToCoding(value); // Coding 3705 else if (name.equals("quantity")) 3706 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 3707 else if (name.equals("unitPrice")) 3708 this.unitPrice = castToMoney(value); // Money 3709 else if (name.equals("factor")) 3710 this.factor = castToDecimal(value); // DecimalType 3711 else if (name.equals("points")) 3712 this.points = castToDecimal(value); // DecimalType 3713 else if (name.equals("net")) 3714 this.net = castToMoney(value); // Money 3715 else if (name.equals("udi")) 3716 this.udi = castToCoding(value); // Coding 3717 else 3718 super.setProperty(name, value); 3719 } 3720 3721 @Override 3722 public Base addChild(String name) throws FHIRException { 3723 if (name.equals("sequence")) { 3724 throw new FHIRException("Cannot call addChild on a singleton property Claim.sequence"); 3725 } else if (name.equals("type")) { 3726 this.type = new Coding(); 3727 return this.type; 3728 } else if (name.equals("service")) { 3729 this.service = new Coding(); 3730 return this.service; 3731 } else if (name.equals("quantity")) { 3732 this.quantity = new SimpleQuantity(); 3733 return this.quantity; 3734 } else if (name.equals("unitPrice")) { 3735 this.unitPrice = new Money(); 3736 return this.unitPrice; 3737 } else if (name.equals("factor")) { 3738 throw new FHIRException("Cannot call addChild on a singleton property Claim.factor"); 3739 } else if (name.equals("points")) { 3740 throw new FHIRException("Cannot call addChild on a singleton property Claim.points"); 3741 } else if (name.equals("net")) { 3742 this.net = new Money(); 3743 return this.net; 3744 } else if (name.equals("udi")) { 3745 this.udi = new Coding(); 3746 return this.udi; 3747 } else 3748 return super.addChild(name); 3749 } 3750 3751 public SubDetailComponent copy() { 3752 SubDetailComponent dst = new SubDetailComponent(); 3753 copyValues(dst); 3754 dst.sequence = sequence == null ? null : sequence.copy(); 3755 dst.type = type == null ? null : type.copy(); 3756 dst.service = service == null ? null : service.copy(); 3757 dst.quantity = quantity == null ? null : quantity.copy(); 3758 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 3759 dst.factor = factor == null ? null : factor.copy(); 3760 dst.points = points == null ? null : points.copy(); 3761 dst.net = net == null ? null : net.copy(); 3762 dst.udi = udi == null ? null : udi.copy(); 3763 return dst; 3764 } 3765 3766 @Override 3767 public boolean equalsDeep(Base other) { 3768 if (!super.equalsDeep(other)) 3769 return false; 3770 if (!(other instanceof SubDetailComponent)) 3771 return false; 3772 SubDetailComponent o = (SubDetailComponent) other; 3773 return compareDeep(sequence, o.sequence, true) && compareDeep(type, o.type, true) 3774 && compareDeep(service, o.service, true) && compareDeep(quantity, o.quantity, true) 3775 && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 3776 && compareDeep(points, o.points, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true); 3777 } 3778 3779 @Override 3780 public boolean equalsShallow(Base other) { 3781 if (!super.equalsShallow(other)) 3782 return false; 3783 if (!(other instanceof SubDetailComponent)) 3784 return false; 3785 SubDetailComponent o = (SubDetailComponent) other; 3786 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true) 3787 && compareValues(points, o.points, true); 3788 } 3789 3790 public boolean isEmpty() { 3791 return super.isEmpty() && (sequence == null || sequence.isEmpty()) && (type == null || type.isEmpty()) 3792 && (service == null || service.isEmpty()) && (quantity == null || quantity.isEmpty()) 3793 && (unitPrice == null || unitPrice.isEmpty()) && (factor == null || factor.isEmpty()) 3794 && (points == null || points.isEmpty()) && (net == null || net.isEmpty()) && (udi == null || udi.isEmpty()); 3795 } 3796 3797 public String fhirType() { 3798 return "Claim.item.detail.subDetail"; 3799 3800 } 3801 3802 } 3803 3804 @Block() 3805 public static class ProsthesisComponent extends BackboneElement implements IBaseBackboneElement { 3806 /** 3807 * Indicates whether this is the initial placement of a fixed prosthesis. 3808 */ 3809 @Child(name = "initial", type = { 3810 BooleanType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 3811 @Description(shortDefinition = "Is this the initial service", formalDefinition = "Indicates whether this is the initial placement of a fixed prosthesis.") 3812 protected BooleanType initial; 3813 3814 /** 3815 * Date of the initial placement. 3816 */ 3817 @Child(name = "priorDate", type = { DateType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 3818 @Description(shortDefinition = "Initial service Date", formalDefinition = "Date of the initial placement.") 3819 protected DateType priorDate; 3820 3821 /** 3822 * Material of the prior denture or bridge prosthesis. (Oral). 3823 */ 3824 @Child(name = "priorMaterial", type = { 3825 Coding.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 3826 @Description(shortDefinition = "Prosthetic Material", formalDefinition = "Material of the prior denture or bridge prosthesis. (Oral).") 3827 protected Coding priorMaterial; 3828 3829 private static final long serialVersionUID = 1739349641L; 3830 3831 /* 3832 * Constructor 3833 */ 3834 public ProsthesisComponent() { 3835 super(); 3836 } 3837 3838 /** 3839 * @return {@link #initial} (Indicates whether this is the initial placement of 3840 * a fixed prosthesis.). This is the underlying object with id, value 3841 * and extensions. The accessor "getInitial" gives direct access to the 3842 * value 3843 */ 3844 public BooleanType getInitialElement() { 3845 if (this.initial == null) 3846 if (Configuration.errorOnAutoCreate()) 3847 throw new Error("Attempt to auto-create ProsthesisComponent.initial"); 3848 else if (Configuration.doAutoCreate()) 3849 this.initial = new BooleanType(); // bb 3850 return this.initial; 3851 } 3852 3853 public boolean hasInitialElement() { 3854 return this.initial != null && !this.initial.isEmpty(); 3855 } 3856 3857 public boolean hasInitial() { 3858 return this.initial != null && !this.initial.isEmpty(); 3859 } 3860 3861 /** 3862 * @param value {@link #initial} (Indicates whether this is the initial 3863 * placement of a fixed prosthesis.). This is the underlying object 3864 * with id, value and extensions. The accessor "getInitial" gives 3865 * direct access to the value 3866 */ 3867 public ProsthesisComponent setInitialElement(BooleanType value) { 3868 this.initial = value; 3869 return this; 3870 } 3871 3872 /** 3873 * @return Indicates whether this is the initial placement of a fixed 3874 * prosthesis. 3875 */ 3876 public boolean getInitial() { 3877 return this.initial == null || this.initial.isEmpty() ? false : this.initial.getValue(); 3878 } 3879 3880 /** 3881 * @param value Indicates whether this is the initial placement of a fixed 3882 * prosthesis. 3883 */ 3884 public ProsthesisComponent setInitial(boolean value) { 3885 if (this.initial == null) 3886 this.initial = new BooleanType(); 3887 this.initial.setValue(value); 3888 return this; 3889 } 3890 3891 /** 3892 * @return {@link #priorDate} (Date of the initial placement.). This is the 3893 * underlying object with id, value and extensions. The accessor 3894 * "getPriorDate" gives direct access to the value 3895 */ 3896 public DateType getPriorDateElement() { 3897 if (this.priorDate == null) 3898 if (Configuration.errorOnAutoCreate()) 3899 throw new Error("Attempt to auto-create ProsthesisComponent.priorDate"); 3900 else if (Configuration.doAutoCreate()) 3901 this.priorDate = new DateType(); // bb 3902 return this.priorDate; 3903 } 3904 3905 public boolean hasPriorDateElement() { 3906 return this.priorDate != null && !this.priorDate.isEmpty(); 3907 } 3908 3909 public boolean hasPriorDate() { 3910 return this.priorDate != null && !this.priorDate.isEmpty(); 3911 } 3912 3913 /** 3914 * @param value {@link #priorDate} (Date of the initial placement.). This is the 3915 * underlying object with id, value and extensions. The accessor 3916 * "getPriorDate" gives direct access to the value 3917 */ 3918 public ProsthesisComponent setPriorDateElement(DateType value) { 3919 this.priorDate = value; 3920 return this; 3921 } 3922 3923 /** 3924 * @return Date of the initial placement. 3925 */ 3926 public Date getPriorDate() { 3927 return this.priorDate == null ? null : this.priorDate.getValue(); 3928 } 3929 3930 /** 3931 * @param value Date of the initial placement. 3932 */ 3933 public ProsthesisComponent setPriorDate(Date value) { 3934 if (value == null) 3935 this.priorDate = null; 3936 else { 3937 if (this.priorDate == null) 3938 this.priorDate = new DateType(); 3939 this.priorDate.setValue(value); 3940 } 3941 return this; 3942 } 3943 3944 /** 3945 * @return {@link #priorMaterial} (Material of the prior denture or bridge 3946 * prosthesis. (Oral).) 3947 */ 3948 public Coding getPriorMaterial() { 3949 if (this.priorMaterial == null) 3950 if (Configuration.errorOnAutoCreate()) 3951 throw new Error("Attempt to auto-create ProsthesisComponent.priorMaterial"); 3952 else if (Configuration.doAutoCreate()) 3953 this.priorMaterial = new Coding(); // cc 3954 return this.priorMaterial; 3955 } 3956 3957 public boolean hasPriorMaterial() { 3958 return this.priorMaterial != null && !this.priorMaterial.isEmpty(); 3959 } 3960 3961 /** 3962 * @param value {@link #priorMaterial} (Material of the prior denture or bridge 3963 * prosthesis. (Oral).) 3964 */ 3965 public ProsthesisComponent setPriorMaterial(Coding value) { 3966 this.priorMaterial = value; 3967 return this; 3968 } 3969 3970 protected void listChildren(List<Property> childrenList) { 3971 super.listChildren(childrenList); 3972 childrenList.add( 3973 new Property("initial", "boolean", "Indicates whether this is the initial placement of a fixed prosthesis.", 3974 0, java.lang.Integer.MAX_VALUE, initial)); 3975 childrenList.add(new Property("priorDate", "date", "Date of the initial placement.", 0, 3976 java.lang.Integer.MAX_VALUE, priorDate)); 3977 childrenList 3978 .add(new Property("priorMaterial", "Coding", "Material of the prior denture or bridge prosthesis. (Oral).", 0, 3979 java.lang.Integer.MAX_VALUE, priorMaterial)); 3980 } 3981 3982 @Override 3983 public void setProperty(String name, Base value) throws FHIRException { 3984 if (name.equals("initial")) 3985 this.initial = castToBoolean(value); // BooleanType 3986 else if (name.equals("priorDate")) 3987 this.priorDate = castToDate(value); // DateType 3988 else if (name.equals("priorMaterial")) 3989 this.priorMaterial = castToCoding(value); // Coding 3990 else 3991 super.setProperty(name, value); 3992 } 3993 3994 @Override 3995 public Base addChild(String name) throws FHIRException { 3996 if (name.equals("initial")) { 3997 throw new FHIRException("Cannot call addChild on a singleton property Claim.initial"); 3998 } else if (name.equals("priorDate")) { 3999 throw new FHIRException("Cannot call addChild on a singleton property Claim.priorDate"); 4000 } else if (name.equals("priorMaterial")) { 4001 this.priorMaterial = new Coding(); 4002 return this.priorMaterial; 4003 } else 4004 return super.addChild(name); 4005 } 4006 4007 public ProsthesisComponent copy() { 4008 ProsthesisComponent dst = new ProsthesisComponent(); 4009 copyValues(dst); 4010 dst.initial = initial == null ? null : initial.copy(); 4011 dst.priorDate = priorDate == null ? null : priorDate.copy(); 4012 dst.priorMaterial = priorMaterial == null ? null : priorMaterial.copy(); 4013 return dst; 4014 } 4015 4016 @Override 4017 public boolean equalsDeep(Base other) { 4018 if (!super.equalsDeep(other)) 4019 return false; 4020 if (!(other instanceof ProsthesisComponent)) 4021 return false; 4022 ProsthesisComponent o = (ProsthesisComponent) other; 4023 return compareDeep(initial, o.initial, true) && compareDeep(priorDate, o.priorDate, true) 4024 && compareDeep(priorMaterial, o.priorMaterial, true); 4025 } 4026 4027 @Override 4028 public boolean equalsShallow(Base other) { 4029 if (!super.equalsShallow(other)) 4030 return false; 4031 if (!(other instanceof ProsthesisComponent)) 4032 return false; 4033 ProsthesisComponent o = (ProsthesisComponent) other; 4034 return compareValues(initial, o.initial, true) && compareValues(priorDate, o.priorDate, true); 4035 } 4036 4037 public boolean isEmpty() { 4038 return super.isEmpty() && (initial == null || initial.isEmpty()) && (priorDate == null || priorDate.isEmpty()) 4039 && (priorMaterial == null || priorMaterial.isEmpty()); 4040 } 4041 4042 public String fhirType() { 4043 return "Claim.item.prosthesis"; 4044 4045 } 4046 4047 } 4048 4049 @Block() 4050 public static class MissingTeethComponent extends BackboneElement implements IBaseBackboneElement { 4051 /** 4052 * The code identifying which tooth is missing. 4053 */ 4054 @Child(name = "tooth", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 4055 @Description(shortDefinition = "Tooth Code", formalDefinition = "The code identifying which tooth is missing.") 4056 protected Coding tooth; 4057 4058 /** 4059 * Missing reason may be: E-extraction, O-other. 4060 */ 4061 @Child(name = "reason", type = { Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 4062 @Description(shortDefinition = "Reason for missing", formalDefinition = "Missing reason may be: E-extraction, O-other.") 4063 protected Coding reason; 4064 4065 /** 4066 * The date of the extraction either known from records or patient reported 4067 * estimate. 4068 */ 4069 @Child(name = "extractionDate", type = { 4070 DateType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 4071 @Description(shortDefinition = "Date of Extraction", formalDefinition = "The date of the extraction either known from records or patient reported estimate.") 4072 protected DateType extractionDate; 4073 4074 private static final long serialVersionUID = 352913313L; 4075 4076 /* 4077 * Constructor 4078 */ 4079 public MissingTeethComponent() { 4080 super(); 4081 } 4082 4083 /* 4084 * Constructor 4085 */ 4086 public MissingTeethComponent(Coding tooth) { 4087 super(); 4088 this.tooth = tooth; 4089 } 4090 4091 /** 4092 * @return {@link #tooth} (The code identifying which tooth is missing.) 4093 */ 4094 public Coding getTooth() { 4095 if (this.tooth == null) 4096 if (Configuration.errorOnAutoCreate()) 4097 throw new Error("Attempt to auto-create MissingTeethComponent.tooth"); 4098 else if (Configuration.doAutoCreate()) 4099 this.tooth = new Coding(); // cc 4100 return this.tooth; 4101 } 4102 4103 public boolean hasTooth() { 4104 return this.tooth != null && !this.tooth.isEmpty(); 4105 } 4106 4107 /** 4108 * @param value {@link #tooth} (The code identifying which tooth is missing.) 4109 */ 4110 public MissingTeethComponent setTooth(Coding value) { 4111 this.tooth = value; 4112 return this; 4113 } 4114 4115 /** 4116 * @return {@link #reason} (Missing reason may be: E-extraction, O-other.) 4117 */ 4118 public Coding getReason() { 4119 if (this.reason == null) 4120 if (Configuration.errorOnAutoCreate()) 4121 throw new Error("Attempt to auto-create MissingTeethComponent.reason"); 4122 else if (Configuration.doAutoCreate()) 4123 this.reason = new Coding(); // cc 4124 return this.reason; 4125 } 4126 4127 public boolean hasReason() { 4128 return this.reason != null && !this.reason.isEmpty(); 4129 } 4130 4131 /** 4132 * @param value {@link #reason} (Missing reason may be: E-extraction, O-other.) 4133 */ 4134 public MissingTeethComponent setReason(Coding value) { 4135 this.reason = value; 4136 return this; 4137 } 4138 4139 /** 4140 * @return {@link #extractionDate} (The date of the extraction either known from 4141 * records or patient reported estimate.). This is the underlying object 4142 * with id, value and extensions. The accessor "getExtractionDate" gives 4143 * direct access to the value 4144 */ 4145 public DateType getExtractionDateElement() { 4146 if (this.extractionDate == null) 4147 if (Configuration.errorOnAutoCreate()) 4148 throw new Error("Attempt to auto-create MissingTeethComponent.extractionDate"); 4149 else if (Configuration.doAutoCreate()) 4150 this.extractionDate = new DateType(); // bb 4151 return this.extractionDate; 4152 } 4153 4154 public boolean hasExtractionDateElement() { 4155 return this.extractionDate != null && !this.extractionDate.isEmpty(); 4156 } 4157 4158 public boolean hasExtractionDate() { 4159 return this.extractionDate != null && !this.extractionDate.isEmpty(); 4160 } 4161 4162 /** 4163 * @param value {@link #extractionDate} (The date of the extraction either known 4164 * from records or patient reported estimate.). This is the 4165 * underlying object with id, value and extensions. The accessor 4166 * "getExtractionDate" gives direct access to the value 4167 */ 4168 public MissingTeethComponent setExtractionDateElement(DateType value) { 4169 this.extractionDate = value; 4170 return this; 4171 } 4172 4173 /** 4174 * @return The date of the extraction either known from records or patient 4175 * reported estimate. 4176 */ 4177 public Date getExtractionDate() { 4178 return this.extractionDate == null ? null : this.extractionDate.getValue(); 4179 } 4180 4181 /** 4182 * @param value The date of the extraction either known from records or patient 4183 * reported estimate. 4184 */ 4185 public MissingTeethComponent setExtractionDate(Date value) { 4186 if (value == null) 4187 this.extractionDate = null; 4188 else { 4189 if (this.extractionDate == null) 4190 this.extractionDate = new DateType(); 4191 this.extractionDate.setValue(value); 4192 } 4193 return this; 4194 } 4195 4196 protected void listChildren(List<Property> childrenList) { 4197 super.listChildren(childrenList); 4198 childrenList.add(new Property("tooth", "Coding", "The code identifying which tooth is missing.", 0, 4199 java.lang.Integer.MAX_VALUE, tooth)); 4200 childrenList.add(new Property("reason", "Coding", "Missing reason may be: E-extraction, O-other.", 0, 4201 java.lang.Integer.MAX_VALUE, reason)); 4202 childrenList.add(new Property("extractionDate", "date", 4203 "The date of the extraction either known from records or patient reported estimate.", 0, 4204 java.lang.Integer.MAX_VALUE, extractionDate)); 4205 } 4206 4207 @Override 4208 public void setProperty(String name, Base value) throws FHIRException { 4209 if (name.equals("tooth")) 4210 this.tooth = castToCoding(value); // Coding 4211 else if (name.equals("reason")) 4212 this.reason = castToCoding(value); // Coding 4213 else if (name.equals("extractionDate")) 4214 this.extractionDate = castToDate(value); // DateType 4215 else 4216 super.setProperty(name, value); 4217 } 4218 4219 @Override 4220 public Base addChild(String name) throws FHIRException { 4221 if (name.equals("tooth")) { 4222 this.tooth = new Coding(); 4223 return this.tooth; 4224 } else if (name.equals("reason")) { 4225 this.reason = new Coding(); 4226 return this.reason; 4227 } else if (name.equals("extractionDate")) { 4228 throw new FHIRException("Cannot call addChild on a singleton property Claim.extractionDate"); 4229 } else 4230 return super.addChild(name); 4231 } 4232 4233 public MissingTeethComponent copy() { 4234 MissingTeethComponent dst = new MissingTeethComponent(); 4235 copyValues(dst); 4236 dst.tooth = tooth == null ? null : tooth.copy(); 4237 dst.reason = reason == null ? null : reason.copy(); 4238 dst.extractionDate = extractionDate == null ? null : extractionDate.copy(); 4239 return dst; 4240 } 4241 4242 @Override 4243 public boolean equalsDeep(Base other) { 4244 if (!super.equalsDeep(other)) 4245 return false; 4246 if (!(other instanceof MissingTeethComponent)) 4247 return false; 4248 MissingTeethComponent o = (MissingTeethComponent) other; 4249 return compareDeep(tooth, o.tooth, true) && compareDeep(reason, o.reason, true) 4250 && compareDeep(extractionDate, o.extractionDate, true); 4251 } 4252 4253 @Override 4254 public boolean equalsShallow(Base other) { 4255 if (!super.equalsShallow(other)) 4256 return false; 4257 if (!(other instanceof MissingTeethComponent)) 4258 return false; 4259 MissingTeethComponent o = (MissingTeethComponent) other; 4260 return compareValues(extractionDate, o.extractionDate, true); 4261 } 4262 4263 public boolean isEmpty() { 4264 return super.isEmpty() && (tooth == null || tooth.isEmpty()) && (reason == null || reason.isEmpty()) 4265 && (extractionDate == null || extractionDate.isEmpty()); 4266 } 4267 4268 public String fhirType() { 4269 return "Claim.missingTeeth"; 4270 4271 } 4272 4273 } 4274 4275 /** 4276 * The category of claim this is. 4277 */ 4278 @Child(name = "type", type = { CodeType.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 4279 @Description(shortDefinition = "institutional | oral | pharmacy | professional | vision", formalDefinition = "The category of claim this is.") 4280 protected Enumeration<ClaimType> type; 4281 4282 /** 4283 * The business identifier for the instance: invoice number, claim number, 4284 * pre-determination or pre-authorization number. 4285 */ 4286 @Child(name = "identifier", type = { 4287 Identifier.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4288 @Description(shortDefinition = "Claim number", formalDefinition = "The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.") 4289 protected List<Identifier> identifier; 4290 4291 /** 4292 * The version of the specification on which this instance relies. 4293 */ 4294 @Child(name = "ruleset", type = { Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 4295 @Description(shortDefinition = "Current specification followed", formalDefinition = "The version of the specification on which this instance relies.") 4296 protected Coding ruleset; 4297 4298 /** 4299 * The version of the specification from which the original instance was 4300 * created. 4301 */ 4302 @Child(name = "originalRuleset", type = { 4303 Coding.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 4304 @Description(shortDefinition = "Original specification followed", formalDefinition = "The version of the specification from which the original instance was created.") 4305 protected Coding originalRuleset; 4306 4307 /** 4308 * The date when the enclosed suite of services were performed or completed. 4309 */ 4310 @Child(name = "created", type = { DateTimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 4311 @Description(shortDefinition = "Creation date", formalDefinition = "The date when the enclosed suite of services were performed or completed.") 4312 protected DateTimeType created; 4313 4314 /** 4315 * Insurer Identifier, typical BIN number (6 digit). 4316 */ 4317 @Child(name = "target", type = { Organization.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 4318 @Description(shortDefinition = "Insurer", formalDefinition = "Insurer Identifier, typical BIN number (6 digit).") 4319 protected Reference target; 4320 4321 /** 4322 * The actual object that is the target of the reference (Insurer Identifier, 4323 * typical BIN number (6 digit).) 4324 */ 4325 protected Organization targetTarget; 4326 4327 /** 4328 * The provider which is responsible for the bill, claim pre-determination, 4329 * pre-authorization. 4330 */ 4331 @Child(name = "provider", type = { 4332 Practitioner.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 4333 @Description(shortDefinition = "Responsible provider", formalDefinition = "The provider which is responsible for the bill, claim pre-determination, pre-authorization.") 4334 protected Reference provider; 4335 4336 /** 4337 * The actual object that is the target of the reference (The provider which is 4338 * responsible for the bill, claim pre-determination, pre-authorization.) 4339 */ 4340 protected Practitioner providerTarget; 4341 4342 /** 4343 * The organization which is responsible for the bill, claim pre-determination, 4344 * pre-authorization. 4345 */ 4346 @Child(name = "organization", type = { 4347 Organization.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 4348 @Description(shortDefinition = "Responsible organization", formalDefinition = "The organization which is responsible for the bill, claim pre-determination, pre-authorization.") 4349 protected Reference organization; 4350 4351 /** 4352 * The actual object that is the target of the reference (The organization which 4353 * is responsible for the bill, claim pre-determination, pre-authorization.) 4354 */ 4355 protected Organization organizationTarget; 4356 4357 /** 4358 * Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory 4359 * (Pre-determination). 4360 */ 4361 @Child(name = "use", type = { CodeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 4362 @Description(shortDefinition = "complete | proposed | exploratory | other", formalDefinition = "Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory (Pre-determination).") 4363 protected Enumeration<Use> use; 4364 4365 /** 4366 * Immediate (stat), best effort (normal), deferred (deferred). 4367 */ 4368 @Child(name = "priority", type = { Coding.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 4369 @Description(shortDefinition = "Desired processing priority", formalDefinition = "Immediate (stat), best effort (normal), deferred (deferred).") 4370 protected Coding priority; 4371 4372 /** 4373 * In the case of a Pre-Determination/Pre-Authorization the provider may request 4374 * that funds in the amount of the expected Benefit be reserved ('Patient' or 4375 * 'Provider') to pay for the Benefits determined on the subsequent claim(s). 4376 * 'None' explicitly indicates no funds reserving is requested. 4377 */ 4378 @Child(name = "fundsReserve", type = { Coding.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 4379 @Description(shortDefinition = "Funds requested to be reserved", formalDefinition = "In the case of a Pre-Determination/Pre-Authorization the provider may request that funds in the amount of the expected Benefit be reserved ('Patient' or 'Provider') to pay for the Benefits determined on the subsequent claim(s). 'None' explicitly indicates no funds reserving is requested.") 4380 protected Coding fundsReserve; 4381 4382 /** 4383 * Person who created the invoice/claim/pre-determination or pre-authorization. 4384 */ 4385 @Child(name = "enterer", type = { 4386 Practitioner.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 4387 @Description(shortDefinition = "Author", formalDefinition = "Person who created the invoice/claim/pre-determination or pre-authorization.") 4388 protected Reference enterer; 4389 4390 /** 4391 * The actual object that is the target of the reference (Person who created the 4392 * invoice/claim/pre-determination or pre-authorization.) 4393 */ 4394 protected Practitioner entererTarget; 4395 4396 /** 4397 * Facility where the services were provided. 4398 */ 4399 @Child(name = "facility", type = { Location.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 4400 @Description(shortDefinition = "Servicing Facility", formalDefinition = "Facility where the services were provided.") 4401 protected Reference facility; 4402 4403 /** 4404 * The actual object that is the target of the reference (Facility where the 4405 * services were provided.) 4406 */ 4407 protected Location facilityTarget; 4408 4409 /** 4410 * Prescription to support the dispensing of Pharmacy or Vision products. 4411 */ 4412 @Child(name = "prescription", type = { MedicationOrder.class, 4413 VisionPrescription.class }, order = 13, min = 0, max = 1, modifier = false, summary = true) 4414 @Description(shortDefinition = "Prescription", formalDefinition = "Prescription to support the dispensing of Pharmacy or Vision products.") 4415 protected Reference prescription; 4416 4417 /** 4418 * The actual object that is the target of the reference (Prescription to 4419 * support the dispensing of Pharmacy or Vision products.) 4420 */ 4421 protected Resource prescriptionTarget; 4422 4423 /** 4424 * Original prescription to support the dispensing of pharmacy services, 4425 * medications or products. 4426 */ 4427 @Child(name = "originalPrescription", type = { 4428 MedicationOrder.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 4429 @Description(shortDefinition = "Original Prescription", formalDefinition = "Original prescription to support the dispensing of pharmacy services, medications or products.") 4430 protected Reference originalPrescription; 4431 4432 /** 4433 * The actual object that is the target of the reference (Original prescription 4434 * to support the dispensing of pharmacy services, medications or products.) 4435 */ 4436 protected MedicationOrder originalPrescriptionTarget; 4437 4438 /** 4439 * The party to be reimbursed for the services. 4440 */ 4441 @Child(name = "payee", type = {}, order = 15, min = 0, max = 1, modifier = false, summary = true) 4442 @Description(shortDefinition = "Payee", formalDefinition = "The party to be reimbursed for the services.") 4443 protected PayeeComponent payee; 4444 4445 /** 4446 * The referral resource which lists the date, practitioner, reason and other 4447 * supporting information. 4448 */ 4449 @Child(name = "referral", type = { 4450 ReferralRequest.class }, order = 16, min = 0, max = 1, modifier = false, summary = true) 4451 @Description(shortDefinition = "Treatment Referral", formalDefinition = "The referral resource which lists the date, practitioner, reason and other supporting information.") 4452 protected Reference referral; 4453 4454 /** 4455 * The actual object that is the target of the reference (The referral resource 4456 * which lists the date, practitioner, reason and other supporting information.) 4457 */ 4458 protected ReferralRequest referralTarget; 4459 4460 /** 4461 * Ordered list of patient diagnosis for which care is sought. 4462 */ 4463 @Child(name = "diagnosis", type = {}, order = 17, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4464 @Description(shortDefinition = "Diagnosis", formalDefinition = "Ordered list of patient diagnosis for which care is sought.") 4465 protected List<DiagnosisComponent> diagnosis; 4466 4467 /** 4468 * List of patient conditions for which care is sought. 4469 */ 4470 @Child(name = "condition", type = { 4471 Coding.class }, order = 18, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4472 @Description(shortDefinition = "List of presenting Conditions", formalDefinition = "List of patient conditions for which care is sought.") 4473 protected List<Coding> condition; 4474 4475 /** 4476 * Patient Resource. 4477 */ 4478 @Child(name = "patient", type = { Patient.class }, order = 19, min = 1, max = 1, modifier = false, summary = true) 4479 @Description(shortDefinition = "The subject of the Products and Services", formalDefinition = "Patient Resource.") 4480 protected Reference patient; 4481 4482 /** 4483 * The actual object that is the target of the reference (Patient Resource.) 4484 */ 4485 protected Patient patientTarget; 4486 4487 /** 4488 * Financial instrument by which payment information for health care. 4489 */ 4490 @Child(name = "coverage", type = {}, order = 20, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4491 @Description(shortDefinition = "Insurance or medical plan", formalDefinition = "Financial instrument by which payment information for health care.") 4492 protected List<CoverageComponent> coverage; 4493 4494 /** 4495 * Factors which may influence the applicability of coverage. 4496 */ 4497 @Child(name = "exception", type = { 4498 Coding.class }, order = 21, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4499 @Description(shortDefinition = "Eligibility exceptions", formalDefinition = "Factors which may influence the applicability of coverage.") 4500 protected List<Coding> exception; 4501 4502 /** 4503 * Name of school for over-aged dependents. 4504 */ 4505 @Child(name = "school", type = { StringType.class }, order = 22, min = 0, max = 1, modifier = false, summary = true) 4506 @Description(shortDefinition = "Name of School", formalDefinition = "Name of school for over-aged dependents.") 4507 protected StringType school; 4508 4509 /** 4510 * Date of an accident which these services are addressing. 4511 */ 4512 @Child(name = "accident", type = { DateType.class }, order = 23, min = 0, max = 1, modifier = false, summary = true) 4513 @Description(shortDefinition = "Accident Date", formalDefinition = "Date of an accident which these services are addressing.") 4514 protected DateType accident; 4515 4516 /** 4517 * Type of accident: work, auto, etc. 4518 */ 4519 @Child(name = "accidentType", type = { Coding.class }, order = 24, min = 0, max = 1, modifier = false, summary = true) 4520 @Description(shortDefinition = "Accident Type", formalDefinition = "Type of accident: work, auto, etc.") 4521 protected Coding accidentType; 4522 4523 /** 4524 * A list of intervention and exception codes which may influence the 4525 * adjudication of the claim. 4526 */ 4527 @Child(name = "interventionException", type = { 4528 Coding.class }, order = 25, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4529 @Description(shortDefinition = "Intervention and exception code (Pharma)", formalDefinition = "A list of intervention and exception codes which may influence the adjudication of the claim.") 4530 protected List<Coding> interventionException; 4531 4532 /** 4533 * First tier of goods and services. 4534 */ 4535 @Child(name = "item", type = {}, order = 26, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4536 @Description(shortDefinition = "Goods and Services", formalDefinition = "First tier of goods and services.") 4537 protected List<ItemsComponent> item; 4538 4539 /** 4540 * Code to indicate that Xrays, images, emails, documents, models or attachments 4541 * are being sent in support of this submission. 4542 */ 4543 @Child(name = "additionalMaterials", type = { 4544 Coding.class }, order = 27, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4545 @Description(shortDefinition = "Additional materials, documents, etc.", formalDefinition = "Code to indicate that Xrays, images, emails, documents, models or attachments are being sent in support of this submission.") 4546 protected List<Coding> additionalMaterials; 4547 4548 /** 4549 * A list of teeth which would be expected but are not found due to having been 4550 * previously extracted or for other reasons. 4551 */ 4552 @Child(name = "missingTeeth", type = {}, order = 28, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4553 @Description(shortDefinition = "Only if type = oral", formalDefinition = "A list of teeth which would be expected but are not found due to having been previously extracted or for other reasons.") 4554 protected List<MissingTeethComponent> missingTeeth; 4555 4556 private static final long serialVersionUID = 4272227L; 4557 4558 /* 4559 * Constructor 4560 */ 4561 public Claim() { 4562 super(); 4563 } 4564 4565 /* 4566 * Constructor 4567 */ 4568 public Claim(Enumeration<ClaimType> type, Reference patient) { 4569 super(); 4570 this.type = type; 4571 this.patient = patient; 4572 } 4573 4574 /** 4575 * @return {@link #type} (The category of claim this is.). This is the 4576 * underlying object with id, value and extensions. The accessor 4577 * "getType" gives direct access to the value 4578 */ 4579 public Enumeration<ClaimType> getTypeElement() { 4580 if (this.type == null) 4581 if (Configuration.errorOnAutoCreate()) 4582 throw new Error("Attempt to auto-create Claim.type"); 4583 else if (Configuration.doAutoCreate()) 4584 this.type = new Enumeration<ClaimType>(new ClaimTypeEnumFactory()); // bb 4585 return this.type; 4586 } 4587 4588 public boolean hasTypeElement() { 4589 return this.type != null && !this.type.isEmpty(); 4590 } 4591 4592 public boolean hasType() { 4593 return this.type != null && !this.type.isEmpty(); 4594 } 4595 4596 /** 4597 * @param value {@link #type} (The category of claim this is.). This is the 4598 * underlying object with id, value and extensions. The accessor 4599 * "getType" gives direct access to the value 4600 */ 4601 public Claim setTypeElement(Enumeration<ClaimType> value) { 4602 this.type = value; 4603 return this; 4604 } 4605 4606 /** 4607 * @return The category of claim this is. 4608 */ 4609 public ClaimType getType() { 4610 return this.type == null ? null : this.type.getValue(); 4611 } 4612 4613 /** 4614 * @param value The category of claim this is. 4615 */ 4616 public Claim setType(ClaimType value) { 4617 if (this.type == null) 4618 this.type = new Enumeration<ClaimType>(new ClaimTypeEnumFactory()); 4619 this.type.setValue(value); 4620 return this; 4621 } 4622 4623 /** 4624 * @return {@link #identifier} (The business identifier for the instance: 4625 * invoice number, claim number, pre-determination or pre-authorization 4626 * number.) 4627 */ 4628 public List<Identifier> getIdentifier() { 4629 if (this.identifier == null) 4630 this.identifier = new ArrayList<Identifier>(); 4631 return this.identifier; 4632 } 4633 4634 public boolean hasIdentifier() { 4635 if (this.identifier == null) 4636 return false; 4637 for (Identifier item : this.identifier) 4638 if (!item.isEmpty()) 4639 return true; 4640 return false; 4641 } 4642 4643 /** 4644 * @return {@link #identifier} (The business identifier for the instance: 4645 * invoice number, claim number, pre-determination or pre-authorization 4646 * number.) 4647 */ 4648 // syntactic sugar 4649 public Identifier addIdentifier() { // 3 4650 Identifier t = new Identifier(); 4651 if (this.identifier == null) 4652 this.identifier = new ArrayList<Identifier>(); 4653 this.identifier.add(t); 4654 return t; 4655 } 4656 4657 // syntactic sugar 4658 public Claim addIdentifier(Identifier t) { // 3 4659 if (t == null) 4660 return this; 4661 if (this.identifier == null) 4662 this.identifier = new ArrayList<Identifier>(); 4663 this.identifier.add(t); 4664 return this; 4665 } 4666 4667 /** 4668 * @return {@link #ruleset} (The version of the specification on which this 4669 * instance relies.) 4670 */ 4671 public Coding getRuleset() { 4672 if (this.ruleset == null) 4673 if (Configuration.errorOnAutoCreate()) 4674 throw new Error("Attempt to auto-create Claim.ruleset"); 4675 else if (Configuration.doAutoCreate()) 4676 this.ruleset = new Coding(); // cc 4677 return this.ruleset; 4678 } 4679 4680 public boolean hasRuleset() { 4681 return this.ruleset != null && !this.ruleset.isEmpty(); 4682 } 4683 4684 /** 4685 * @param value {@link #ruleset} (The version of the specification on which this 4686 * instance relies.) 4687 */ 4688 public Claim setRuleset(Coding value) { 4689 this.ruleset = value; 4690 return this; 4691 } 4692 4693 /** 4694 * @return {@link #originalRuleset} (The version of the specification from which 4695 * the original instance was created.) 4696 */ 4697 public Coding getOriginalRuleset() { 4698 if (this.originalRuleset == null) 4699 if (Configuration.errorOnAutoCreate()) 4700 throw new Error("Attempt to auto-create Claim.originalRuleset"); 4701 else if (Configuration.doAutoCreate()) 4702 this.originalRuleset = new Coding(); // cc 4703 return this.originalRuleset; 4704 } 4705 4706 public boolean hasOriginalRuleset() { 4707 return this.originalRuleset != null && !this.originalRuleset.isEmpty(); 4708 } 4709 4710 /** 4711 * @param value {@link #originalRuleset} (The version of the specification from 4712 * which the original instance was created.) 4713 */ 4714 public Claim setOriginalRuleset(Coding value) { 4715 this.originalRuleset = value; 4716 return this; 4717 } 4718 4719 /** 4720 * @return {@link #created} (The date when the enclosed suite of services were 4721 * performed or completed.). This is the underlying object with id, 4722 * value and extensions. The accessor "getCreated" gives direct access 4723 * to the value 4724 */ 4725 public DateTimeType getCreatedElement() { 4726 if (this.created == null) 4727 if (Configuration.errorOnAutoCreate()) 4728 throw new Error("Attempt to auto-create Claim.created"); 4729 else if (Configuration.doAutoCreate()) 4730 this.created = new DateTimeType(); // bb 4731 return this.created; 4732 } 4733 4734 public boolean hasCreatedElement() { 4735 return this.created != null && !this.created.isEmpty(); 4736 } 4737 4738 public boolean hasCreated() { 4739 return this.created != null && !this.created.isEmpty(); 4740 } 4741 4742 /** 4743 * @param value {@link #created} (The date when the enclosed suite of services 4744 * were performed or completed.). This is the underlying object 4745 * with id, value and extensions. The accessor "getCreated" gives 4746 * direct access to the value 4747 */ 4748 public Claim setCreatedElement(DateTimeType value) { 4749 this.created = value; 4750 return this; 4751 } 4752 4753 /** 4754 * @return The date when the enclosed suite of services were performed or 4755 * completed. 4756 */ 4757 public Date getCreated() { 4758 return this.created == null ? null : this.created.getValue(); 4759 } 4760 4761 /** 4762 * @param value The date when the enclosed suite of services were performed or 4763 * completed. 4764 */ 4765 public Claim setCreated(Date value) { 4766 if (value == null) 4767 this.created = null; 4768 else { 4769 if (this.created == null) 4770 this.created = new DateTimeType(); 4771 this.created.setValue(value); 4772 } 4773 return this; 4774 } 4775 4776 /** 4777 * @return {@link #target} (Insurer Identifier, typical BIN number (6 digit).) 4778 */ 4779 public Reference getTarget() { 4780 if (this.target == null) 4781 if (Configuration.errorOnAutoCreate()) 4782 throw new Error("Attempt to auto-create Claim.target"); 4783 else if (Configuration.doAutoCreate()) 4784 this.target = new Reference(); // cc 4785 return this.target; 4786 } 4787 4788 public boolean hasTarget() { 4789 return this.target != null && !this.target.isEmpty(); 4790 } 4791 4792 /** 4793 * @param value {@link #target} (Insurer Identifier, typical BIN number (6 4794 * digit).) 4795 */ 4796 public Claim setTarget(Reference value) { 4797 this.target = value; 4798 return this; 4799 } 4800 4801 /** 4802 * @return {@link #target} The actual object that is the target of the 4803 * reference. The reference library doesn't populate this, but you can 4804 * use it to hold the resource if you resolve it. (Insurer Identifier, 4805 * typical BIN number (6 digit).) 4806 */ 4807 public Organization getTargetTarget() { 4808 if (this.targetTarget == null) 4809 if (Configuration.errorOnAutoCreate()) 4810 throw new Error("Attempt to auto-create Claim.target"); 4811 else if (Configuration.doAutoCreate()) 4812 this.targetTarget = new Organization(); // aa 4813 return this.targetTarget; 4814 } 4815 4816 /** 4817 * @param value {@link #target} The actual object that is the target of the 4818 * reference. The reference library doesn't use these, but you can 4819 * use it to hold the resource if you resolve it. (Insurer 4820 * Identifier, typical BIN number (6 digit).) 4821 */ 4822 public Claim setTargetTarget(Organization value) { 4823 this.targetTarget = value; 4824 return this; 4825 } 4826 4827 /** 4828 * @return {@link #provider} (The provider which is responsible for the bill, 4829 * claim pre-determination, pre-authorization.) 4830 */ 4831 public Reference getProvider() { 4832 if (this.provider == null) 4833 if (Configuration.errorOnAutoCreate()) 4834 throw new Error("Attempt to auto-create Claim.provider"); 4835 else if (Configuration.doAutoCreate()) 4836 this.provider = new Reference(); // cc 4837 return this.provider; 4838 } 4839 4840 public boolean hasProvider() { 4841 return this.provider != null && !this.provider.isEmpty(); 4842 } 4843 4844 /** 4845 * @param value {@link #provider} (The provider which is responsible for the 4846 * bill, claim pre-determination, pre-authorization.) 4847 */ 4848 public Claim setProvider(Reference value) { 4849 this.provider = value; 4850 return this; 4851 } 4852 4853 /** 4854 * @return {@link #provider} The actual object that is the target of the 4855 * reference. The reference library doesn't populate this, but you can 4856 * use it to hold the resource if you resolve it. (The provider which is 4857 * responsible for the bill, claim pre-determination, 4858 * pre-authorization.) 4859 */ 4860 public Practitioner getProviderTarget() { 4861 if (this.providerTarget == null) 4862 if (Configuration.errorOnAutoCreate()) 4863 throw new Error("Attempt to auto-create Claim.provider"); 4864 else if (Configuration.doAutoCreate()) 4865 this.providerTarget = new Practitioner(); // aa 4866 return this.providerTarget; 4867 } 4868 4869 /** 4870 * @param value {@link #provider} The actual object that is the target of the 4871 * reference. The reference library doesn't use these, but you can 4872 * use it to hold the resource if you resolve it. (The provider 4873 * which is responsible for the bill, claim pre-determination, 4874 * pre-authorization.) 4875 */ 4876 public Claim setProviderTarget(Practitioner value) { 4877 this.providerTarget = value; 4878 return this; 4879 } 4880 4881 /** 4882 * @return {@link #organization} (The organization which is responsible for the 4883 * bill, claim pre-determination, pre-authorization.) 4884 */ 4885 public Reference getOrganization() { 4886 if (this.organization == null) 4887 if (Configuration.errorOnAutoCreate()) 4888 throw new Error("Attempt to auto-create Claim.organization"); 4889 else if (Configuration.doAutoCreate()) 4890 this.organization = new Reference(); // cc 4891 return this.organization; 4892 } 4893 4894 public boolean hasOrganization() { 4895 return this.organization != null && !this.organization.isEmpty(); 4896 } 4897 4898 /** 4899 * @param value {@link #organization} (The organization which is responsible for 4900 * the bill, claim pre-determination, pre-authorization.) 4901 */ 4902 public Claim setOrganization(Reference value) { 4903 this.organization = value; 4904 return this; 4905 } 4906 4907 /** 4908 * @return {@link #organization} The actual object that is the target of the 4909 * reference. The reference library doesn't populate this, but you can 4910 * use it to hold the resource if you resolve it. (The organization 4911 * which is responsible for the bill, claim pre-determination, 4912 * pre-authorization.) 4913 */ 4914 public Organization getOrganizationTarget() { 4915 if (this.organizationTarget == null) 4916 if (Configuration.errorOnAutoCreate()) 4917 throw new Error("Attempt to auto-create Claim.organization"); 4918 else if (Configuration.doAutoCreate()) 4919 this.organizationTarget = new Organization(); // aa 4920 return this.organizationTarget; 4921 } 4922 4923 /** 4924 * @param value {@link #organization} The actual object that is the target of 4925 * the reference. The reference library doesn't use these, but you 4926 * can use it to hold the resource if you resolve it. (The 4927 * organization which is responsible for the bill, claim 4928 * pre-determination, pre-authorization.) 4929 */ 4930 public Claim setOrganizationTarget(Organization value) { 4931 this.organizationTarget = value; 4932 return this; 4933 } 4934 4935 /** 4936 * @return {@link #use} (Complete (Bill or Claim), Proposed (Pre-Authorization), 4937 * Exploratory (Pre-determination).). This is the underlying object with 4938 * id, value and extensions. The accessor "getUse" gives direct access 4939 * to the value 4940 */ 4941 public Enumeration<Use> getUseElement() { 4942 if (this.use == null) 4943 if (Configuration.errorOnAutoCreate()) 4944 throw new Error("Attempt to auto-create Claim.use"); 4945 else if (Configuration.doAutoCreate()) 4946 this.use = new Enumeration<Use>(new UseEnumFactory()); // bb 4947 return this.use; 4948 } 4949 4950 public boolean hasUseElement() { 4951 return this.use != null && !this.use.isEmpty(); 4952 } 4953 4954 public boolean hasUse() { 4955 return this.use != null && !this.use.isEmpty(); 4956 } 4957 4958 /** 4959 * @param value {@link #use} (Complete (Bill or Claim), Proposed 4960 * (Pre-Authorization), Exploratory (Pre-determination).). This is 4961 * the underlying object with id, value and extensions. The 4962 * accessor "getUse" gives direct access to the value 4963 */ 4964 public Claim setUseElement(Enumeration<Use> value) { 4965 this.use = value; 4966 return this; 4967 } 4968 4969 /** 4970 * @return Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory 4971 * (Pre-determination). 4972 */ 4973 public Use getUse() { 4974 return this.use == null ? null : this.use.getValue(); 4975 } 4976 4977 /** 4978 * @param value Complete (Bill or Claim), Proposed (Pre-Authorization), 4979 * Exploratory (Pre-determination). 4980 */ 4981 public Claim setUse(Use value) { 4982 if (value == null) 4983 this.use = null; 4984 else { 4985 if (this.use == null) 4986 this.use = new Enumeration<Use>(new UseEnumFactory()); 4987 this.use.setValue(value); 4988 } 4989 return this; 4990 } 4991 4992 /** 4993 * @return {@link #priority} (Immediate (stat), best effort (normal), deferred 4994 * (deferred).) 4995 */ 4996 public Coding getPriority() { 4997 if (this.priority == null) 4998 if (Configuration.errorOnAutoCreate()) 4999 throw new Error("Attempt to auto-create Claim.priority"); 5000 else if (Configuration.doAutoCreate()) 5001 this.priority = new Coding(); // cc 5002 return this.priority; 5003 } 5004 5005 public boolean hasPriority() { 5006 return this.priority != null && !this.priority.isEmpty(); 5007 } 5008 5009 /** 5010 * @param value {@link #priority} (Immediate (stat), best effort (normal), 5011 * deferred (deferred).) 5012 */ 5013 public Claim setPriority(Coding value) { 5014 this.priority = value; 5015 return this; 5016 } 5017 5018 /** 5019 * @return {@link #fundsReserve} (In the case of a 5020 * Pre-Determination/Pre-Authorization the provider may request that 5021 * funds in the amount of the expected Benefit be reserved ('Patient' or 5022 * 'Provider') to pay for the Benefits determined on the subsequent 5023 * claim(s). 'None' explicitly indicates no funds reserving is 5024 * requested.) 5025 */ 5026 public Coding getFundsReserve() { 5027 if (this.fundsReserve == null) 5028 if (Configuration.errorOnAutoCreate()) 5029 throw new Error("Attempt to auto-create Claim.fundsReserve"); 5030 else if (Configuration.doAutoCreate()) 5031 this.fundsReserve = new Coding(); // cc 5032 return this.fundsReserve; 5033 } 5034 5035 public boolean hasFundsReserve() { 5036 return this.fundsReserve != null && !this.fundsReserve.isEmpty(); 5037 } 5038 5039 /** 5040 * @param value {@link #fundsReserve} (In the case of a 5041 * Pre-Determination/Pre-Authorization the provider may request 5042 * that funds in the amount of the expected Benefit be reserved 5043 * ('Patient' or 'Provider') to pay for the Benefits determined on 5044 * the subsequent claim(s). 'None' explicitly indicates no funds 5045 * reserving is requested.) 5046 */ 5047 public Claim setFundsReserve(Coding value) { 5048 this.fundsReserve = value; 5049 return this; 5050 } 5051 5052 /** 5053 * @return {@link #enterer} (Person who created the 5054 * invoice/claim/pre-determination or pre-authorization.) 5055 */ 5056 public Reference getEnterer() { 5057 if (this.enterer == null) 5058 if (Configuration.errorOnAutoCreate()) 5059 throw new Error("Attempt to auto-create Claim.enterer"); 5060 else if (Configuration.doAutoCreate()) 5061 this.enterer = new Reference(); // cc 5062 return this.enterer; 5063 } 5064 5065 public boolean hasEnterer() { 5066 return this.enterer != null && !this.enterer.isEmpty(); 5067 } 5068 5069 /** 5070 * @param value {@link #enterer} (Person who created the 5071 * invoice/claim/pre-determination or pre-authorization.) 5072 */ 5073 public Claim setEnterer(Reference value) { 5074 this.enterer = value; 5075 return this; 5076 } 5077 5078 /** 5079 * @return {@link #enterer} The actual object that is the target of the 5080 * reference. The reference library doesn't populate this, but you can 5081 * use it to hold the resource if you resolve it. (Person who created 5082 * the invoice/claim/pre-determination or pre-authorization.) 5083 */ 5084 public Practitioner getEntererTarget() { 5085 if (this.entererTarget == null) 5086 if (Configuration.errorOnAutoCreate()) 5087 throw new Error("Attempt to auto-create Claim.enterer"); 5088 else if (Configuration.doAutoCreate()) 5089 this.entererTarget = new Practitioner(); // aa 5090 return this.entererTarget; 5091 } 5092 5093 /** 5094 * @param value {@link #enterer} The actual object that is the target of the 5095 * reference. The reference library doesn't use these, but you can 5096 * use it to hold the resource if you resolve it. (Person who 5097 * created the invoice/claim/pre-determination or 5098 * pre-authorization.) 5099 */ 5100 public Claim setEntererTarget(Practitioner value) { 5101 this.entererTarget = value; 5102 return this; 5103 } 5104 5105 /** 5106 * @return {@link #facility} (Facility where the services were provided.) 5107 */ 5108 public Reference getFacility() { 5109 if (this.facility == null) 5110 if (Configuration.errorOnAutoCreate()) 5111 throw new Error("Attempt to auto-create Claim.facility"); 5112 else if (Configuration.doAutoCreate()) 5113 this.facility = new Reference(); // cc 5114 return this.facility; 5115 } 5116 5117 public boolean hasFacility() { 5118 return this.facility != null && !this.facility.isEmpty(); 5119 } 5120 5121 /** 5122 * @param value {@link #facility} (Facility where the services were provided.) 5123 */ 5124 public Claim setFacility(Reference value) { 5125 this.facility = value; 5126 return this; 5127 } 5128 5129 /** 5130 * @return {@link #facility} The actual object that is the target of the 5131 * reference. The reference library doesn't populate this, but you can 5132 * use it to hold the resource if you resolve it. (Facility where the 5133 * services were provided.) 5134 */ 5135 public Location getFacilityTarget() { 5136 if (this.facilityTarget == null) 5137 if (Configuration.errorOnAutoCreate()) 5138 throw new Error("Attempt to auto-create Claim.facility"); 5139 else if (Configuration.doAutoCreate()) 5140 this.facilityTarget = new Location(); // aa 5141 return this.facilityTarget; 5142 } 5143 5144 /** 5145 * @param value {@link #facility} The actual object that is the target of the 5146 * reference. The reference library doesn't use these, but you can 5147 * use it to hold the resource if you resolve it. (Facility where 5148 * the services were provided.) 5149 */ 5150 public Claim setFacilityTarget(Location value) { 5151 this.facilityTarget = value; 5152 return this; 5153 } 5154 5155 /** 5156 * @return {@link #prescription} (Prescription to support the dispensing of 5157 * Pharmacy or Vision products.) 5158 */ 5159 public Reference getPrescription() { 5160 if (this.prescription == null) 5161 if (Configuration.errorOnAutoCreate()) 5162 throw new Error("Attempt to auto-create Claim.prescription"); 5163 else if (Configuration.doAutoCreate()) 5164 this.prescription = new Reference(); // cc 5165 return this.prescription; 5166 } 5167 5168 public boolean hasPrescription() { 5169 return this.prescription != null && !this.prescription.isEmpty(); 5170 } 5171 5172 /** 5173 * @param value {@link #prescription} (Prescription to support the dispensing of 5174 * Pharmacy or Vision products.) 5175 */ 5176 public Claim setPrescription(Reference value) { 5177 this.prescription = value; 5178 return this; 5179 } 5180 5181 /** 5182 * @return {@link #prescription} The actual object that is the target of the 5183 * reference. The reference library doesn't populate this, but you can 5184 * use it to hold the resource if you resolve it. (Prescription to 5185 * support the dispensing of Pharmacy or Vision products.) 5186 */ 5187 public Resource getPrescriptionTarget() { 5188 return this.prescriptionTarget; 5189 } 5190 5191 /** 5192 * @param value {@link #prescription} The actual object that is the target of 5193 * the reference. The reference library doesn't use these, but you 5194 * can use it to hold the resource if you resolve it. (Prescription 5195 * to support the dispensing of Pharmacy or Vision products.) 5196 */ 5197 public Claim setPrescriptionTarget(Resource value) { 5198 this.prescriptionTarget = value; 5199 return this; 5200 } 5201 5202 /** 5203 * @return {@link #originalPrescription} (Original prescription to support the 5204 * dispensing of pharmacy services, medications or products.) 5205 */ 5206 public Reference getOriginalPrescription() { 5207 if (this.originalPrescription == null) 5208 if (Configuration.errorOnAutoCreate()) 5209 throw new Error("Attempt to auto-create Claim.originalPrescription"); 5210 else if (Configuration.doAutoCreate()) 5211 this.originalPrescription = new Reference(); // cc 5212 return this.originalPrescription; 5213 } 5214 5215 public boolean hasOriginalPrescription() { 5216 return this.originalPrescription != null && !this.originalPrescription.isEmpty(); 5217 } 5218 5219 /** 5220 * @param value {@link #originalPrescription} (Original prescription to support 5221 * the dispensing of pharmacy services, medications or products.) 5222 */ 5223 public Claim setOriginalPrescription(Reference value) { 5224 this.originalPrescription = value; 5225 return this; 5226 } 5227 5228 /** 5229 * @return {@link #originalPrescription} The actual object that is the target of 5230 * the reference. The reference library doesn't populate this, but you 5231 * can use it to hold the resource if you resolve it. (Original 5232 * prescription to support the dispensing of pharmacy services, 5233 * medications or products.) 5234 */ 5235 public MedicationOrder getOriginalPrescriptionTarget() { 5236 if (this.originalPrescriptionTarget == null) 5237 if (Configuration.errorOnAutoCreate()) 5238 throw new Error("Attempt to auto-create Claim.originalPrescription"); 5239 else if (Configuration.doAutoCreate()) 5240 this.originalPrescriptionTarget = new MedicationOrder(); // aa 5241 return this.originalPrescriptionTarget; 5242 } 5243 5244 /** 5245 * @param value {@link #originalPrescription} The actual object that is the 5246 * target of the reference. The reference library doesn't use 5247 * these, but you can use it to hold the resource if you resolve 5248 * it. (Original prescription to support the dispensing of pharmacy 5249 * services, medications or products.) 5250 */ 5251 public Claim setOriginalPrescriptionTarget(MedicationOrder value) { 5252 this.originalPrescriptionTarget = value; 5253 return this; 5254 } 5255 5256 /** 5257 * @return {@link #payee} (The party to be reimbursed for the services.) 5258 */ 5259 public PayeeComponent getPayee() { 5260 if (this.payee == null) 5261 if (Configuration.errorOnAutoCreate()) 5262 throw new Error("Attempt to auto-create Claim.payee"); 5263 else if (Configuration.doAutoCreate()) 5264 this.payee = new PayeeComponent(); // cc 5265 return this.payee; 5266 } 5267 5268 public boolean hasPayee() { 5269 return this.payee != null && !this.payee.isEmpty(); 5270 } 5271 5272 /** 5273 * @param value {@link #payee} (The party to be reimbursed for the services.) 5274 */ 5275 public Claim setPayee(PayeeComponent value) { 5276 this.payee = value; 5277 return this; 5278 } 5279 5280 /** 5281 * @return {@link #referral} (The referral resource which lists the date, 5282 * practitioner, reason and other supporting information.) 5283 */ 5284 public Reference getReferral() { 5285 if (this.referral == null) 5286 if (Configuration.errorOnAutoCreate()) 5287 throw new Error("Attempt to auto-create Claim.referral"); 5288 else if (Configuration.doAutoCreate()) 5289 this.referral = new Reference(); // cc 5290 return this.referral; 5291 } 5292 5293 public boolean hasReferral() { 5294 return this.referral != null && !this.referral.isEmpty(); 5295 } 5296 5297 /** 5298 * @param value {@link #referral} (The referral resource which lists the date, 5299 * practitioner, reason and other supporting information.) 5300 */ 5301 public Claim setReferral(Reference value) { 5302 this.referral = value; 5303 return this; 5304 } 5305 5306 /** 5307 * @return {@link #referral} The actual object that is the target of the 5308 * reference. The reference library doesn't populate this, but you can 5309 * use it to hold the resource if you resolve it. (The referral resource 5310 * which lists the date, practitioner, reason and other supporting 5311 * information.) 5312 */ 5313 public ReferralRequest getReferralTarget() { 5314 if (this.referralTarget == null) 5315 if (Configuration.errorOnAutoCreate()) 5316 throw new Error("Attempt to auto-create Claim.referral"); 5317 else if (Configuration.doAutoCreate()) 5318 this.referralTarget = new ReferralRequest(); // aa 5319 return this.referralTarget; 5320 } 5321 5322 /** 5323 * @param value {@link #referral} The actual object that is the target of the 5324 * reference. The reference library doesn't use these, but you can 5325 * use it to hold the resource if you resolve it. (The referral 5326 * resource which lists the date, practitioner, reason and other 5327 * supporting information.) 5328 */ 5329 public Claim setReferralTarget(ReferralRequest value) { 5330 this.referralTarget = value; 5331 return this; 5332 } 5333 5334 /** 5335 * @return {@link #diagnosis} (Ordered list of patient diagnosis for which care 5336 * is sought.) 5337 */ 5338 public List<DiagnosisComponent> getDiagnosis() { 5339 if (this.diagnosis == null) 5340 this.diagnosis = new ArrayList<DiagnosisComponent>(); 5341 return this.diagnosis; 5342 } 5343 5344 public boolean hasDiagnosis() { 5345 if (this.diagnosis == null) 5346 return false; 5347 for (DiagnosisComponent item : this.diagnosis) 5348 if (!item.isEmpty()) 5349 return true; 5350 return false; 5351 } 5352 5353 /** 5354 * @return {@link #diagnosis} (Ordered list of patient diagnosis for which care 5355 * is sought.) 5356 */ 5357 // syntactic sugar 5358 public DiagnosisComponent addDiagnosis() { // 3 5359 DiagnosisComponent t = new DiagnosisComponent(); 5360 if (this.diagnosis == null) 5361 this.diagnosis = new ArrayList<DiagnosisComponent>(); 5362 this.diagnosis.add(t); 5363 return t; 5364 } 5365 5366 // syntactic sugar 5367 public Claim addDiagnosis(DiagnosisComponent t) { // 3 5368 if (t == null) 5369 return this; 5370 if (this.diagnosis == null) 5371 this.diagnosis = new ArrayList<DiagnosisComponent>(); 5372 this.diagnosis.add(t); 5373 return this; 5374 } 5375 5376 /** 5377 * @return {@link #condition} (List of patient conditions for which care is 5378 * sought.) 5379 */ 5380 public List<Coding> getCondition() { 5381 if (this.condition == null) 5382 this.condition = new ArrayList<Coding>(); 5383 return this.condition; 5384 } 5385 5386 public boolean hasCondition() { 5387 if (this.condition == null) 5388 return false; 5389 for (Coding item : this.condition) 5390 if (!item.isEmpty()) 5391 return true; 5392 return false; 5393 } 5394 5395 /** 5396 * @return {@link #condition} (List of patient conditions for which care is 5397 * sought.) 5398 */ 5399 // syntactic sugar 5400 public Coding addCondition() { // 3 5401 Coding t = new Coding(); 5402 if (this.condition == null) 5403 this.condition = new ArrayList<Coding>(); 5404 this.condition.add(t); 5405 return t; 5406 } 5407 5408 // syntactic sugar 5409 public Claim addCondition(Coding t) { // 3 5410 if (t == null) 5411 return this; 5412 if (this.condition == null) 5413 this.condition = new ArrayList<Coding>(); 5414 this.condition.add(t); 5415 return this; 5416 } 5417 5418 /** 5419 * @return {@link #patient} (Patient Resource.) 5420 */ 5421 public Reference getPatient() { 5422 if (this.patient == null) 5423 if (Configuration.errorOnAutoCreate()) 5424 throw new Error("Attempt to auto-create Claim.patient"); 5425 else if (Configuration.doAutoCreate()) 5426 this.patient = new Reference(); // cc 5427 return this.patient; 5428 } 5429 5430 public boolean hasPatient() { 5431 return this.patient != null && !this.patient.isEmpty(); 5432 } 5433 5434 /** 5435 * @param value {@link #patient} (Patient Resource.) 5436 */ 5437 public Claim setPatient(Reference value) { 5438 this.patient = value; 5439 return this; 5440 } 5441 5442 /** 5443 * @return {@link #patient} The actual object that is the target of the 5444 * reference. The reference library doesn't populate this, but you can 5445 * use it to hold the resource if you resolve it. (Patient Resource.) 5446 */ 5447 public Patient getPatientTarget() { 5448 if (this.patientTarget == null) 5449 if (Configuration.errorOnAutoCreate()) 5450 throw new Error("Attempt to auto-create Claim.patient"); 5451 else if (Configuration.doAutoCreate()) 5452 this.patientTarget = new Patient(); // aa 5453 return this.patientTarget; 5454 } 5455 5456 /** 5457 * @param value {@link #patient} The actual object that is the target of the 5458 * reference. The reference library doesn't use these, but you can 5459 * use it to hold the resource if you resolve it. (Patient 5460 * Resource.) 5461 */ 5462 public Claim setPatientTarget(Patient value) { 5463 this.patientTarget = value; 5464 return this; 5465 } 5466 5467 /** 5468 * @return {@link #coverage} (Financial instrument by which payment information 5469 * for health care.) 5470 */ 5471 public List<CoverageComponent> getCoverage() { 5472 if (this.coverage == null) 5473 this.coverage = new ArrayList<CoverageComponent>(); 5474 return this.coverage; 5475 } 5476 5477 public boolean hasCoverage() { 5478 if (this.coverage == null) 5479 return false; 5480 for (CoverageComponent item : this.coverage) 5481 if (!item.isEmpty()) 5482 return true; 5483 return false; 5484 } 5485 5486 /** 5487 * @return {@link #coverage} (Financial instrument by which payment information 5488 * for health care.) 5489 */ 5490 // syntactic sugar 5491 public CoverageComponent addCoverage() { // 3 5492 CoverageComponent t = new CoverageComponent(); 5493 if (this.coverage == null) 5494 this.coverage = new ArrayList<CoverageComponent>(); 5495 this.coverage.add(t); 5496 return t; 5497 } 5498 5499 // syntactic sugar 5500 public Claim addCoverage(CoverageComponent t) { // 3 5501 if (t == null) 5502 return this; 5503 if (this.coverage == null) 5504 this.coverage = new ArrayList<CoverageComponent>(); 5505 this.coverage.add(t); 5506 return this; 5507 } 5508 5509 /** 5510 * @return {@link #exception} (Factors which may influence the applicability of 5511 * coverage.) 5512 */ 5513 public List<Coding> getException() { 5514 if (this.exception == null) 5515 this.exception = new ArrayList<Coding>(); 5516 return this.exception; 5517 } 5518 5519 public boolean hasException() { 5520 if (this.exception == null) 5521 return false; 5522 for (Coding item : this.exception) 5523 if (!item.isEmpty()) 5524 return true; 5525 return false; 5526 } 5527 5528 /** 5529 * @return {@link #exception} (Factors which may influence the applicability of 5530 * coverage.) 5531 */ 5532 // syntactic sugar 5533 public Coding addException() { // 3 5534 Coding t = new Coding(); 5535 if (this.exception == null) 5536 this.exception = new ArrayList<Coding>(); 5537 this.exception.add(t); 5538 return t; 5539 } 5540 5541 // syntactic sugar 5542 public Claim addException(Coding t) { // 3 5543 if (t == null) 5544 return this; 5545 if (this.exception == null) 5546 this.exception = new ArrayList<Coding>(); 5547 this.exception.add(t); 5548 return this; 5549 } 5550 5551 /** 5552 * @return {@link #school} (Name of school for over-aged dependents.). This is 5553 * the underlying object with id, value and extensions. The accessor 5554 * "getSchool" gives direct access to the value 5555 */ 5556 public StringType getSchoolElement() { 5557 if (this.school == null) 5558 if (Configuration.errorOnAutoCreate()) 5559 throw new Error("Attempt to auto-create Claim.school"); 5560 else if (Configuration.doAutoCreate()) 5561 this.school = new StringType(); // bb 5562 return this.school; 5563 } 5564 5565 public boolean hasSchoolElement() { 5566 return this.school != null && !this.school.isEmpty(); 5567 } 5568 5569 public boolean hasSchool() { 5570 return this.school != null && !this.school.isEmpty(); 5571 } 5572 5573 /** 5574 * @param value {@link #school} (Name of school for over-aged dependents.). This 5575 * is the underlying object with id, value and extensions. The 5576 * accessor "getSchool" gives direct access to the value 5577 */ 5578 public Claim setSchoolElement(StringType value) { 5579 this.school = value; 5580 return this; 5581 } 5582 5583 /** 5584 * @return Name of school for over-aged dependents. 5585 */ 5586 public String getSchool() { 5587 return this.school == null ? null : this.school.getValue(); 5588 } 5589 5590 /** 5591 * @param value Name of school for over-aged dependents. 5592 */ 5593 public Claim setSchool(String value) { 5594 if (Utilities.noString(value)) 5595 this.school = null; 5596 else { 5597 if (this.school == null) 5598 this.school = new StringType(); 5599 this.school.setValue(value); 5600 } 5601 return this; 5602 } 5603 5604 /** 5605 * @return {@link #accident} (Date of an accident which these services are 5606 * addressing.). This is the underlying object with id, value and 5607 * extensions. The accessor "getAccident" gives direct access to the 5608 * value 5609 */ 5610 public DateType getAccidentElement() { 5611 if (this.accident == null) 5612 if (Configuration.errorOnAutoCreate()) 5613 throw new Error("Attempt to auto-create Claim.accident"); 5614 else if (Configuration.doAutoCreate()) 5615 this.accident = new DateType(); // bb 5616 return this.accident; 5617 } 5618 5619 public boolean hasAccidentElement() { 5620 return this.accident != null && !this.accident.isEmpty(); 5621 } 5622 5623 public boolean hasAccident() { 5624 return this.accident != null && !this.accident.isEmpty(); 5625 } 5626 5627 /** 5628 * @param value {@link #accident} (Date of an accident which these services are 5629 * addressing.). This is the underlying object with id, value and 5630 * extensions. The accessor "getAccident" gives direct access to 5631 * the value 5632 */ 5633 public Claim setAccidentElement(DateType value) { 5634 this.accident = value; 5635 return this; 5636 } 5637 5638 /** 5639 * @return Date of an accident which these services are addressing. 5640 */ 5641 public Date getAccident() { 5642 return this.accident == null ? null : this.accident.getValue(); 5643 } 5644 5645 /** 5646 * @param value Date of an accident which these services are addressing. 5647 */ 5648 public Claim setAccident(Date value) { 5649 if (value == null) 5650 this.accident = null; 5651 else { 5652 if (this.accident == null) 5653 this.accident = new DateType(); 5654 this.accident.setValue(value); 5655 } 5656 return this; 5657 } 5658 5659 /** 5660 * @return {@link #accidentType} (Type of accident: work, auto, etc.) 5661 */ 5662 public Coding getAccidentType() { 5663 if (this.accidentType == null) 5664 if (Configuration.errorOnAutoCreate()) 5665 throw new Error("Attempt to auto-create Claim.accidentType"); 5666 else if (Configuration.doAutoCreate()) 5667 this.accidentType = new Coding(); // cc 5668 return this.accidentType; 5669 } 5670 5671 public boolean hasAccidentType() { 5672 return this.accidentType != null && !this.accidentType.isEmpty(); 5673 } 5674 5675 /** 5676 * @param value {@link #accidentType} (Type of accident: work, auto, etc.) 5677 */ 5678 public Claim setAccidentType(Coding value) { 5679 this.accidentType = value; 5680 return this; 5681 } 5682 5683 /** 5684 * @return {@link #interventionException} (A list of intervention and exception 5685 * codes which may influence the adjudication of the claim.) 5686 */ 5687 public List<Coding> getInterventionException() { 5688 if (this.interventionException == null) 5689 this.interventionException = new ArrayList<Coding>(); 5690 return this.interventionException; 5691 } 5692 5693 public boolean hasInterventionException() { 5694 if (this.interventionException == null) 5695 return false; 5696 for (Coding item : this.interventionException) 5697 if (!item.isEmpty()) 5698 return true; 5699 return false; 5700 } 5701 5702 /** 5703 * @return {@link #interventionException} (A list of intervention and exception 5704 * codes which may influence the adjudication of the claim.) 5705 */ 5706 // syntactic sugar 5707 public Coding addInterventionException() { // 3 5708 Coding t = new Coding(); 5709 if (this.interventionException == null) 5710 this.interventionException = new ArrayList<Coding>(); 5711 this.interventionException.add(t); 5712 return t; 5713 } 5714 5715 // syntactic sugar 5716 public Claim addInterventionException(Coding t) { // 3 5717 if (t == null) 5718 return this; 5719 if (this.interventionException == null) 5720 this.interventionException = new ArrayList<Coding>(); 5721 this.interventionException.add(t); 5722 return this; 5723 } 5724 5725 /** 5726 * @return {@link #item} (First tier of goods and services.) 5727 */ 5728 public List<ItemsComponent> getItem() { 5729 if (this.item == null) 5730 this.item = new ArrayList<ItemsComponent>(); 5731 return this.item; 5732 } 5733 5734 public boolean hasItem() { 5735 if (this.item == null) 5736 return false; 5737 for (ItemsComponent item : this.item) 5738 if (!item.isEmpty()) 5739 return true; 5740 return false; 5741 } 5742 5743 /** 5744 * @return {@link #item} (First tier of goods and services.) 5745 */ 5746 // syntactic sugar 5747 public ItemsComponent addItem() { // 3 5748 ItemsComponent t = new ItemsComponent(); 5749 if (this.item == null) 5750 this.item = new ArrayList<ItemsComponent>(); 5751 this.item.add(t); 5752 return t; 5753 } 5754 5755 // syntactic sugar 5756 public Claim addItem(ItemsComponent t) { // 3 5757 if (t == null) 5758 return this; 5759 if (this.item == null) 5760 this.item = new ArrayList<ItemsComponent>(); 5761 this.item.add(t); 5762 return this; 5763 } 5764 5765 /** 5766 * @return {@link #additionalMaterials} (Code to indicate that Xrays, images, 5767 * emails, documents, models or attachments are being sent in support of 5768 * this submission.) 5769 */ 5770 public List<Coding> getAdditionalMaterials() { 5771 if (this.additionalMaterials == null) 5772 this.additionalMaterials = new ArrayList<Coding>(); 5773 return this.additionalMaterials; 5774 } 5775 5776 public boolean hasAdditionalMaterials() { 5777 if (this.additionalMaterials == null) 5778 return false; 5779 for (Coding item : this.additionalMaterials) 5780 if (!item.isEmpty()) 5781 return true; 5782 return false; 5783 } 5784 5785 /** 5786 * @return {@link #additionalMaterials} (Code to indicate that Xrays, images, 5787 * emails, documents, models or attachments are being sent in support of 5788 * this submission.) 5789 */ 5790 // syntactic sugar 5791 public Coding addAdditionalMaterials() { // 3 5792 Coding t = new Coding(); 5793 if (this.additionalMaterials == null) 5794 this.additionalMaterials = new ArrayList<Coding>(); 5795 this.additionalMaterials.add(t); 5796 return t; 5797 } 5798 5799 // syntactic sugar 5800 public Claim addAdditionalMaterials(Coding t) { // 3 5801 if (t == null) 5802 return this; 5803 if (this.additionalMaterials == null) 5804 this.additionalMaterials = new ArrayList<Coding>(); 5805 this.additionalMaterials.add(t); 5806 return this; 5807 } 5808 5809 /** 5810 * @return {@link #missingTeeth} (A list of teeth which would be expected but 5811 * are not found due to having been previously extracted or for other 5812 * reasons.) 5813 */ 5814 public List<MissingTeethComponent> getMissingTeeth() { 5815 if (this.missingTeeth == null) 5816 this.missingTeeth = new ArrayList<MissingTeethComponent>(); 5817 return this.missingTeeth; 5818 } 5819 5820 public boolean hasMissingTeeth() { 5821 if (this.missingTeeth == null) 5822 return false; 5823 for (MissingTeethComponent item : this.missingTeeth) 5824 if (!item.isEmpty()) 5825 return true; 5826 return false; 5827 } 5828 5829 /** 5830 * @return {@link #missingTeeth} (A list of teeth which would be expected but 5831 * are not found due to having been previously extracted or for other 5832 * reasons.) 5833 */ 5834 // syntactic sugar 5835 public MissingTeethComponent addMissingTeeth() { // 3 5836 MissingTeethComponent t = new MissingTeethComponent(); 5837 if (this.missingTeeth == null) 5838 this.missingTeeth = new ArrayList<MissingTeethComponent>(); 5839 this.missingTeeth.add(t); 5840 return t; 5841 } 5842 5843 // syntactic sugar 5844 public Claim addMissingTeeth(MissingTeethComponent t) { // 3 5845 if (t == null) 5846 return this; 5847 if (this.missingTeeth == null) 5848 this.missingTeeth = new ArrayList<MissingTeethComponent>(); 5849 this.missingTeeth.add(t); 5850 return this; 5851 } 5852 5853 protected void listChildren(List<Property> childrenList) { 5854 super.listChildren(childrenList); 5855 childrenList 5856 .add(new Property("type", "code", "The category of claim this is.", 0, java.lang.Integer.MAX_VALUE, type)); 5857 childrenList.add(new Property("identifier", "Identifier", 5858 "The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.", 5859 0, java.lang.Integer.MAX_VALUE, identifier)); 5860 childrenList.add(new Property("ruleset", "Coding", 5861 "The version of the specification on which this instance relies.", 0, java.lang.Integer.MAX_VALUE, ruleset)); 5862 childrenList.add(new Property("originalRuleset", "Coding", 5863 "The version of the specification from which the original instance was created.", 0, 5864 java.lang.Integer.MAX_VALUE, originalRuleset)); 5865 childrenList.add( 5866 new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 5867 0, java.lang.Integer.MAX_VALUE, created)); 5868 childrenList.add(new Property("target", "Reference(Organization)", 5869 "Insurer Identifier, typical BIN number (6 digit).", 0, java.lang.Integer.MAX_VALUE, target)); 5870 childrenList.add(new Property("provider", "Reference(Practitioner)", 5871 "The provider which is responsible for the bill, claim pre-determination, pre-authorization.", 0, 5872 java.lang.Integer.MAX_VALUE, provider)); 5873 childrenList.add(new Property("organization", "Reference(Organization)", 5874 "The organization which is responsible for the bill, claim pre-determination, pre-authorization.", 0, 5875 java.lang.Integer.MAX_VALUE, organization)); 5876 childrenList.add(new Property("use", "code", 5877 "Complete (Bill or Claim), Proposed (Pre-Authorization), Exploratory (Pre-determination).", 0, 5878 java.lang.Integer.MAX_VALUE, use)); 5879 childrenList.add(new Property("priority", "Coding", "Immediate (stat), best effort (normal), deferred (deferred).", 5880 0, java.lang.Integer.MAX_VALUE, priority)); 5881 childrenList.add(new Property("fundsReserve", "Coding", 5882 "In the case of a Pre-Determination/Pre-Authorization the provider may request that funds in the amount of the expected Benefit be reserved ('Patient' or 'Provider') to pay for the Benefits determined on the subsequent claim(s). 'None' explicitly indicates no funds reserving is requested.", 5883 0, java.lang.Integer.MAX_VALUE, fundsReserve)); 5884 childrenList.add(new Property("enterer", "Reference(Practitioner)", 5885 "Person who created the invoice/claim/pre-determination or pre-authorization.", 0, java.lang.Integer.MAX_VALUE, 5886 enterer)); 5887 childrenList.add(new Property("facility", "Reference(Location)", "Facility where the services were provided.", 0, 5888 java.lang.Integer.MAX_VALUE, facility)); 5889 childrenList.add(new Property("prescription", "Reference(MedicationOrder|VisionPrescription)", 5890 "Prescription to support the dispensing of Pharmacy or Vision products.", 0, java.lang.Integer.MAX_VALUE, 5891 prescription)); 5892 childrenList.add(new Property("originalPrescription", "Reference(MedicationOrder)", 5893 "Original prescription to support the dispensing of pharmacy services, medications or products.", 0, 5894 java.lang.Integer.MAX_VALUE, originalPrescription)); 5895 childrenList.add(new Property("payee", "", "The party to be reimbursed for the services.", 0, 5896 java.lang.Integer.MAX_VALUE, payee)); 5897 childrenList.add(new Property("referral", "Reference(ReferralRequest)", 5898 "The referral resource which lists the date, practitioner, reason and other supporting information.", 0, 5899 java.lang.Integer.MAX_VALUE, referral)); 5900 childrenList.add(new Property("diagnosis", "", "Ordered list of patient diagnosis for which care is sought.", 0, 5901 java.lang.Integer.MAX_VALUE, diagnosis)); 5902 childrenList.add(new Property("condition", "Coding", "List of patient conditions for which care is sought.", 0, 5903 java.lang.Integer.MAX_VALUE, condition)); 5904 childrenList.add( 5905 new Property("patient", "Reference(Patient)", "Patient Resource.", 0, java.lang.Integer.MAX_VALUE, patient)); 5906 childrenList.add(new Property("coverage", "", "Financial instrument by which payment information for health care.", 5907 0, java.lang.Integer.MAX_VALUE, coverage)); 5908 childrenList.add(new Property("exception", "Coding", "Factors which may influence the applicability of coverage.", 5909 0, java.lang.Integer.MAX_VALUE, exception)); 5910 childrenList.add(new Property("school", "string", "Name of school for over-aged dependents.", 0, 5911 java.lang.Integer.MAX_VALUE, school)); 5912 childrenList.add(new Property("accident", "date", "Date of an accident which these services are addressing.", 0, 5913 java.lang.Integer.MAX_VALUE, accident)); 5914 childrenList.add(new Property("accidentType", "Coding", "Type of accident: work, auto, etc.", 0, 5915 java.lang.Integer.MAX_VALUE, accidentType)); 5916 childrenList.add(new Property("interventionException", "Coding", 5917 "A list of intervention and exception codes which may influence the adjudication of the claim.", 0, 5918 java.lang.Integer.MAX_VALUE, interventionException)); 5919 childrenList 5920 .add(new Property("item", "", "First tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, item)); 5921 childrenList.add(new Property("additionalMaterials", "Coding", 5922 "Code to indicate that Xrays, images, emails, documents, models or attachments are being sent in support of this submission.", 5923 0, java.lang.Integer.MAX_VALUE, additionalMaterials)); 5924 childrenList.add(new Property("missingTeeth", "", 5925 "A list of teeth which would be expected but are not found due to having been previously extracted or for other reasons.", 5926 0, java.lang.Integer.MAX_VALUE, missingTeeth)); 5927 } 5928 5929 @Override 5930 public void setProperty(String name, Base value) throws FHIRException { 5931 if (name.equals("type")) 5932 this.type = new ClaimTypeEnumFactory().fromType(value); // Enumeration<ClaimType> 5933 else if (name.equals("identifier")) 5934 this.getIdentifier().add(castToIdentifier(value)); 5935 else if (name.equals("ruleset")) 5936 this.ruleset = castToCoding(value); // Coding 5937 else if (name.equals("originalRuleset")) 5938 this.originalRuleset = castToCoding(value); // Coding 5939 else if (name.equals("created")) 5940 this.created = castToDateTime(value); // DateTimeType 5941 else if (name.equals("target")) 5942 this.target = castToReference(value); // Reference 5943 else if (name.equals("provider")) 5944 this.provider = castToReference(value); // Reference 5945 else if (name.equals("organization")) 5946 this.organization = castToReference(value); // Reference 5947 else if (name.equals("use")) 5948 this.use = new UseEnumFactory().fromType(value); // Enumeration<Use> 5949 else if (name.equals("priority")) 5950 this.priority = castToCoding(value); // Coding 5951 else if (name.equals("fundsReserve")) 5952 this.fundsReserve = castToCoding(value); // Coding 5953 else if (name.equals("enterer")) 5954 this.enterer = castToReference(value); // Reference 5955 else if (name.equals("facility")) 5956 this.facility = castToReference(value); // Reference 5957 else if (name.equals("prescription")) 5958 this.prescription = castToReference(value); // Reference 5959 else if (name.equals("originalPrescription")) 5960 this.originalPrescription = castToReference(value); // Reference 5961 else if (name.equals("payee")) 5962 this.payee = (PayeeComponent) value; // PayeeComponent 5963 else if (name.equals("referral")) 5964 this.referral = castToReference(value); // Reference 5965 else if (name.equals("diagnosis")) 5966 this.getDiagnosis().add((DiagnosisComponent) value); 5967 else if (name.equals("condition")) 5968 this.getCondition().add(castToCoding(value)); 5969 else if (name.equals("patient")) 5970 this.patient = castToReference(value); // Reference 5971 else if (name.equals("coverage")) 5972 this.getCoverage().add((CoverageComponent) value); 5973 else if (name.equals("exception")) 5974 this.getException().add(castToCoding(value)); 5975 else if (name.equals("school")) 5976 this.school = castToString(value); // StringType 5977 else if (name.equals("accident")) 5978 this.accident = castToDate(value); // DateType 5979 else if (name.equals("accidentType")) 5980 this.accidentType = castToCoding(value); // Coding 5981 else if (name.equals("interventionException")) 5982 this.getInterventionException().add(castToCoding(value)); 5983 else if (name.equals("item")) 5984 this.getItem().add((ItemsComponent) value); 5985 else if (name.equals("additionalMaterials")) 5986 this.getAdditionalMaterials().add(castToCoding(value)); 5987 else if (name.equals("missingTeeth")) 5988 this.getMissingTeeth().add((MissingTeethComponent) value); 5989 else 5990 super.setProperty(name, value); 5991 } 5992 5993 @Override 5994 public Base addChild(String name) throws FHIRException { 5995 if (name.equals("type")) { 5996 throw new FHIRException("Cannot call addChild on a singleton property Claim.type"); 5997 } else if (name.equals("identifier")) { 5998 return addIdentifier(); 5999 } else if (name.equals("ruleset")) { 6000 this.ruleset = new Coding(); 6001 return this.ruleset; 6002 } else if (name.equals("originalRuleset")) { 6003 this.originalRuleset = new Coding(); 6004 return this.originalRuleset; 6005 } else if (name.equals("created")) { 6006 throw new FHIRException("Cannot call addChild on a singleton property Claim.created"); 6007 } else if (name.equals("target")) { 6008 this.target = new Reference(); 6009 return this.target; 6010 } else if (name.equals("provider")) { 6011 this.provider = new Reference(); 6012 return this.provider; 6013 } else if (name.equals("organization")) { 6014 this.organization = new Reference(); 6015 return this.organization; 6016 } else if (name.equals("use")) { 6017 throw new FHIRException("Cannot call addChild on a singleton property Claim.use"); 6018 } else if (name.equals("priority")) { 6019 this.priority = new Coding(); 6020 return this.priority; 6021 } else if (name.equals("fundsReserve")) { 6022 this.fundsReserve = new Coding(); 6023 return this.fundsReserve; 6024 } else if (name.equals("enterer")) { 6025 this.enterer = new Reference(); 6026 return this.enterer; 6027 } else if (name.equals("facility")) { 6028 this.facility = new Reference(); 6029 return this.facility; 6030 } else if (name.equals("prescription")) { 6031 this.prescription = new Reference(); 6032 return this.prescription; 6033 } else if (name.equals("originalPrescription")) { 6034 this.originalPrescription = new Reference(); 6035 return this.originalPrescription; 6036 } else if (name.equals("payee")) { 6037 this.payee = new PayeeComponent(); 6038 return this.payee; 6039 } else if (name.equals("referral")) { 6040 this.referral = new Reference(); 6041 return this.referral; 6042 } else if (name.equals("diagnosis")) { 6043 return addDiagnosis(); 6044 } else if (name.equals("condition")) { 6045 return addCondition(); 6046 } else if (name.equals("patient")) { 6047 this.patient = new Reference(); 6048 return this.patient; 6049 } else if (name.equals("coverage")) { 6050 return addCoverage(); 6051 } else if (name.equals("exception")) { 6052 return addException(); 6053 } else if (name.equals("school")) { 6054 throw new FHIRException("Cannot call addChild on a singleton property Claim.school"); 6055 } else if (name.equals("accident")) { 6056 throw new FHIRException("Cannot call addChild on a singleton property Claim.accident"); 6057 } else if (name.equals("accidentType")) { 6058 this.accidentType = new Coding(); 6059 return this.accidentType; 6060 } else if (name.equals("interventionException")) { 6061 return addInterventionException(); 6062 } else if (name.equals("item")) { 6063 return addItem(); 6064 } else if (name.equals("additionalMaterials")) { 6065 return addAdditionalMaterials(); 6066 } else if (name.equals("missingTeeth")) { 6067 return addMissingTeeth(); 6068 } else 6069 return super.addChild(name); 6070 } 6071 6072 public String fhirType() { 6073 return "Claim"; 6074 6075 } 6076 6077 public Claim copy() { 6078 Claim dst = new Claim(); 6079 copyValues(dst); 6080 dst.type = type == null ? null : type.copy(); 6081 if (identifier != null) { 6082 dst.identifier = new ArrayList<Identifier>(); 6083 for (Identifier i : identifier) 6084 dst.identifier.add(i.copy()); 6085 } 6086 ; 6087 dst.ruleset = ruleset == null ? null : ruleset.copy(); 6088 dst.originalRuleset = originalRuleset == null ? null : originalRuleset.copy(); 6089 dst.created = created == null ? null : created.copy(); 6090 dst.target = target == null ? null : target.copy(); 6091 dst.provider = provider == null ? null : provider.copy(); 6092 dst.organization = organization == null ? null : organization.copy(); 6093 dst.use = use == null ? null : use.copy(); 6094 dst.priority = priority == null ? null : priority.copy(); 6095 dst.fundsReserve = fundsReserve == null ? null : fundsReserve.copy(); 6096 dst.enterer = enterer == null ? null : enterer.copy(); 6097 dst.facility = facility == null ? null : facility.copy(); 6098 dst.prescription = prescription == null ? null : prescription.copy(); 6099 dst.originalPrescription = originalPrescription == null ? null : originalPrescription.copy(); 6100 dst.payee = payee == null ? null : payee.copy(); 6101 dst.referral = referral == null ? null : referral.copy(); 6102 if (diagnosis != null) { 6103 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 6104 for (DiagnosisComponent i : diagnosis) 6105 dst.diagnosis.add(i.copy()); 6106 } 6107 ; 6108 if (condition != null) { 6109 dst.condition = new ArrayList<Coding>(); 6110 for (Coding i : condition) 6111 dst.condition.add(i.copy()); 6112 } 6113 ; 6114 dst.patient = patient == null ? null : patient.copy(); 6115 if (coverage != null) { 6116 dst.coverage = new ArrayList<CoverageComponent>(); 6117 for (CoverageComponent i : coverage) 6118 dst.coverage.add(i.copy()); 6119 } 6120 ; 6121 if (exception != null) { 6122 dst.exception = new ArrayList<Coding>(); 6123 for (Coding i : exception) 6124 dst.exception.add(i.copy()); 6125 } 6126 ; 6127 dst.school = school == null ? null : school.copy(); 6128 dst.accident = accident == null ? null : accident.copy(); 6129 dst.accidentType = accidentType == null ? null : accidentType.copy(); 6130 if (interventionException != null) { 6131 dst.interventionException = new ArrayList<Coding>(); 6132 for (Coding i : interventionException) 6133 dst.interventionException.add(i.copy()); 6134 } 6135 ; 6136 if (item != null) { 6137 dst.item = new ArrayList<ItemsComponent>(); 6138 for (ItemsComponent i : item) 6139 dst.item.add(i.copy()); 6140 } 6141 ; 6142 if (additionalMaterials != null) { 6143 dst.additionalMaterials = new ArrayList<Coding>(); 6144 for (Coding i : additionalMaterials) 6145 dst.additionalMaterials.add(i.copy()); 6146 } 6147 ; 6148 if (missingTeeth != null) { 6149 dst.missingTeeth = new ArrayList<MissingTeethComponent>(); 6150 for (MissingTeethComponent i : missingTeeth) 6151 dst.missingTeeth.add(i.copy()); 6152 } 6153 ; 6154 return dst; 6155 } 6156 6157 protected Claim typedCopy() { 6158 return copy(); 6159 } 6160 6161 @Override 6162 public boolean equalsDeep(Base other) { 6163 if (!super.equalsDeep(other)) 6164 return false; 6165 if (!(other instanceof Claim)) 6166 return false; 6167 Claim o = (Claim) other; 6168 return compareDeep(type, o.type, true) && compareDeep(identifier, o.identifier, true) 6169 && compareDeep(ruleset, o.ruleset, true) && compareDeep(originalRuleset, o.originalRuleset, true) 6170 && compareDeep(created, o.created, true) && compareDeep(target, o.target, true) 6171 && compareDeep(provider, o.provider, true) && compareDeep(organization, o.organization, true) 6172 && compareDeep(use, o.use, true) && compareDeep(priority, o.priority, true) 6173 && compareDeep(fundsReserve, o.fundsReserve, true) && compareDeep(enterer, o.enterer, true) 6174 && compareDeep(facility, o.facility, true) && compareDeep(prescription, o.prescription, true) 6175 && compareDeep(originalPrescription, o.originalPrescription, true) && compareDeep(payee, o.payee, true) 6176 && compareDeep(referral, o.referral, true) && compareDeep(diagnosis, o.diagnosis, true) 6177 && compareDeep(condition, o.condition, true) && compareDeep(patient, o.patient, true) 6178 && compareDeep(coverage, o.coverage, true) && compareDeep(exception, o.exception, true) 6179 && compareDeep(school, o.school, true) && compareDeep(accident, o.accident, true) 6180 && compareDeep(accidentType, o.accidentType, true) 6181 && compareDeep(interventionException, o.interventionException, true) && compareDeep(item, o.item, true) 6182 && compareDeep(additionalMaterials, o.additionalMaterials, true) 6183 && compareDeep(missingTeeth, o.missingTeeth, true); 6184 } 6185 6186 @Override 6187 public boolean equalsShallow(Base other) { 6188 if (!super.equalsShallow(other)) 6189 return false; 6190 if (!(other instanceof Claim)) 6191 return false; 6192 Claim o = (Claim) other; 6193 return compareValues(type, o.type, true) && compareValues(created, o.created, true) 6194 && compareValues(use, o.use, true) && compareValues(school, o.school, true) 6195 && compareValues(accident, o.accident, true); 6196 } 6197 6198 public boolean isEmpty() { 6199 return super.isEmpty() && (type == null || type.isEmpty()) && (identifier == null || identifier.isEmpty()) 6200 && (ruleset == null || ruleset.isEmpty()) && (originalRuleset == null || originalRuleset.isEmpty()) 6201 && (created == null || created.isEmpty()) && (target == null || target.isEmpty()) 6202 && (provider == null || provider.isEmpty()) && (organization == null || organization.isEmpty()) 6203 && (use == null || use.isEmpty()) && (priority == null || priority.isEmpty()) 6204 && (fundsReserve == null || fundsReserve.isEmpty()) && (enterer == null || enterer.isEmpty()) 6205 && (facility == null || facility.isEmpty()) && (prescription == null || prescription.isEmpty()) 6206 && (originalPrescription == null || originalPrescription.isEmpty()) && (payee == null || payee.isEmpty()) 6207 && (referral == null || referral.isEmpty()) && (diagnosis == null || diagnosis.isEmpty()) 6208 && (condition == null || condition.isEmpty()) && (patient == null || patient.isEmpty()) 6209 && (coverage == null || coverage.isEmpty()) && (exception == null || exception.isEmpty()) 6210 && (school == null || school.isEmpty()) && (accident == null || accident.isEmpty()) 6211 && (accidentType == null || accidentType.isEmpty()) 6212 && (interventionException == null || interventionException.isEmpty()) && (item == null || item.isEmpty()) 6213 && (additionalMaterials == null || additionalMaterials.isEmpty()) 6214 && (missingTeeth == null || missingTeeth.isEmpty()); 6215 } 6216 6217 @Override 6218 public ResourceType getResourceType() { 6219 return ResourceType.Claim; 6220 } 6221 6222 @SearchParamDefinition(name = "identifier", path = "Claim.identifier", description = "The primary identifier of the financial resource", type = "token") 6223 public static final String SP_IDENTIFIER = "identifier"; 6224 @SearchParamDefinition(name = "provider", path = "Claim.provider", description = "Provider responsible for the claim", type = "reference") 6225 public static final String SP_PROVIDER = "provider"; 6226 @SearchParamDefinition(name = "use", path = "Claim.use", description = "The kind of financial resource", type = "token") 6227 public static final String SP_USE = "use"; 6228 @SearchParamDefinition(name = "patient", path = "Claim.patient", description = "Patient", type = "reference") 6229 public static final String SP_PATIENT = "patient"; 6230 @SearchParamDefinition(name = "priority", path = "Claim.priority", description = "Processing priority requested", type = "token") 6231 public static final String SP_PRIORITY = "priority"; 6232 6233}