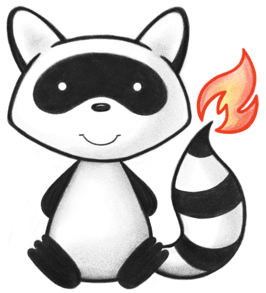
001package org.hl7.fhir.dstu2.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu2.model.Enumerations.RemittanceOutcome; 040import org.hl7.fhir.dstu2.model.Enumerations.RemittanceOutcomeEnumFactory; 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 047import org.hl7.fhir.exceptions.FHIRException; 048import org.hl7.fhir.utilities.Utilities; 049 050/** 051 * This resource provides the adjudication details from the processing of a 052 * Claim resource. 053 */ 054@ResourceDef(name = "ClaimResponse", profile = "http://hl7.org/fhir/Profile/ClaimResponse") 055public class ClaimResponse extends DomainResource { 056 057 @Block() 058 public static class ItemsComponent extends BackboneElement implements IBaseBackboneElement { 059 /** 060 * A service line number. 061 */ 062 @Child(name = "sequenceLinkId", type = { 063 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 064 @Description(shortDefinition = "Service instance", formalDefinition = "A service line number.") 065 protected PositiveIntType sequenceLinkId; 066 067 /** 068 * A list of note references to the notes provided below. 069 */ 070 @Child(name = "noteNumber", type = { 071 PositiveIntType.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 072 @Description(shortDefinition = "List of note numbers which apply", formalDefinition = "A list of note references to the notes provided below.") 073 protected List<PositiveIntType> noteNumber; 074 075 /** 076 * The adjudications results. 077 */ 078 @Child(name = "adjudication", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 079 @Description(shortDefinition = "Adjudication details", formalDefinition = "The adjudications results.") 080 protected List<ItemAdjudicationComponent> adjudication; 081 082 /** 083 * The second tier service adjudications for submitted services. 084 */ 085 @Child(name = "detail", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 086 @Description(shortDefinition = "Detail line items", formalDefinition = "The second tier service adjudications for submitted services.") 087 protected List<ItemDetailComponent> detail; 088 089 private static final long serialVersionUID = -1917866697L; 090 091 /* 092 * Constructor 093 */ 094 public ItemsComponent() { 095 super(); 096 } 097 098 /* 099 * Constructor 100 */ 101 public ItemsComponent(PositiveIntType sequenceLinkId) { 102 super(); 103 this.sequenceLinkId = sequenceLinkId; 104 } 105 106 /** 107 * @return {@link #sequenceLinkId} (A service line number.). This is the 108 * underlying object with id, value and extensions. The accessor 109 * "getSequenceLinkId" gives direct access to the value 110 */ 111 public PositiveIntType getSequenceLinkIdElement() { 112 if (this.sequenceLinkId == null) 113 if (Configuration.errorOnAutoCreate()) 114 throw new Error("Attempt to auto-create ItemsComponent.sequenceLinkId"); 115 else if (Configuration.doAutoCreate()) 116 this.sequenceLinkId = new PositiveIntType(); // bb 117 return this.sequenceLinkId; 118 } 119 120 public boolean hasSequenceLinkIdElement() { 121 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 122 } 123 124 public boolean hasSequenceLinkId() { 125 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 126 } 127 128 /** 129 * @param value {@link #sequenceLinkId} (A service line number.). This is the 130 * underlying object with id, value and extensions. The accessor 131 * "getSequenceLinkId" gives direct access to the value 132 */ 133 public ItemsComponent setSequenceLinkIdElement(PositiveIntType value) { 134 this.sequenceLinkId = value; 135 return this; 136 } 137 138 /** 139 * @return A service line number. 140 */ 141 public int getSequenceLinkId() { 142 return this.sequenceLinkId == null || this.sequenceLinkId.isEmpty() ? 0 : this.sequenceLinkId.getValue(); 143 } 144 145 /** 146 * @param value A service line number. 147 */ 148 public ItemsComponent setSequenceLinkId(int value) { 149 if (this.sequenceLinkId == null) 150 this.sequenceLinkId = new PositiveIntType(); 151 this.sequenceLinkId.setValue(value); 152 return this; 153 } 154 155 /** 156 * @return {@link #noteNumber} (A list of note references to the notes provided 157 * below.) 158 */ 159 public List<PositiveIntType> getNoteNumber() { 160 if (this.noteNumber == null) 161 this.noteNumber = new ArrayList<PositiveIntType>(); 162 return this.noteNumber; 163 } 164 165 public boolean hasNoteNumber() { 166 if (this.noteNumber == null) 167 return false; 168 for (PositiveIntType item : this.noteNumber) 169 if (!item.isEmpty()) 170 return true; 171 return false; 172 } 173 174 /** 175 * @return {@link #noteNumber} (A list of note references to the notes provided 176 * below.) 177 */ 178 // syntactic sugar 179 public PositiveIntType addNoteNumberElement() {// 2 180 PositiveIntType t = new PositiveIntType(); 181 if (this.noteNumber == null) 182 this.noteNumber = new ArrayList<PositiveIntType>(); 183 this.noteNumber.add(t); 184 return t; 185 } 186 187 /** 188 * @param value {@link #noteNumber} (A list of note references to the notes 189 * provided below.) 190 */ 191 public ItemsComponent addNoteNumber(int value) { // 1 192 PositiveIntType t = new PositiveIntType(); 193 t.setValue(value); 194 if (this.noteNumber == null) 195 this.noteNumber = new ArrayList<PositiveIntType>(); 196 this.noteNumber.add(t); 197 return this; 198 } 199 200 /** 201 * @param value {@link #noteNumber} (A list of note references to the notes 202 * provided below.) 203 */ 204 public boolean hasNoteNumber(int value) { 205 if (this.noteNumber == null) 206 return false; 207 for (PositiveIntType v : this.noteNumber) 208 if (v.equals(value)) // positiveInt 209 return true; 210 return false; 211 } 212 213 /** 214 * @return {@link #adjudication} (The adjudications results.) 215 */ 216 public List<ItemAdjudicationComponent> getAdjudication() { 217 if (this.adjudication == null) 218 this.adjudication = new ArrayList<ItemAdjudicationComponent>(); 219 return this.adjudication; 220 } 221 222 public boolean hasAdjudication() { 223 if (this.adjudication == null) 224 return false; 225 for (ItemAdjudicationComponent item : this.adjudication) 226 if (!item.isEmpty()) 227 return true; 228 return false; 229 } 230 231 /** 232 * @return {@link #adjudication} (The adjudications results.) 233 */ 234 // syntactic sugar 235 public ItemAdjudicationComponent addAdjudication() { // 3 236 ItemAdjudicationComponent t = new ItemAdjudicationComponent(); 237 if (this.adjudication == null) 238 this.adjudication = new ArrayList<ItemAdjudicationComponent>(); 239 this.adjudication.add(t); 240 return t; 241 } 242 243 // syntactic sugar 244 public ItemsComponent addAdjudication(ItemAdjudicationComponent t) { // 3 245 if (t == null) 246 return this; 247 if (this.adjudication == null) 248 this.adjudication = new ArrayList<ItemAdjudicationComponent>(); 249 this.adjudication.add(t); 250 return this; 251 } 252 253 /** 254 * @return {@link #detail} (The second tier service adjudications for submitted 255 * services.) 256 */ 257 public List<ItemDetailComponent> getDetail() { 258 if (this.detail == null) 259 this.detail = new ArrayList<ItemDetailComponent>(); 260 return this.detail; 261 } 262 263 public boolean hasDetail() { 264 if (this.detail == null) 265 return false; 266 for (ItemDetailComponent item : this.detail) 267 if (!item.isEmpty()) 268 return true; 269 return false; 270 } 271 272 /** 273 * @return {@link #detail} (The second tier service adjudications for submitted 274 * services.) 275 */ 276 // syntactic sugar 277 public ItemDetailComponent addDetail() { // 3 278 ItemDetailComponent t = new ItemDetailComponent(); 279 if (this.detail == null) 280 this.detail = new ArrayList<ItemDetailComponent>(); 281 this.detail.add(t); 282 return t; 283 } 284 285 // syntactic sugar 286 public ItemsComponent addDetail(ItemDetailComponent t) { // 3 287 if (t == null) 288 return this; 289 if (this.detail == null) 290 this.detail = new ArrayList<ItemDetailComponent>(); 291 this.detail.add(t); 292 return this; 293 } 294 295 protected void listChildren(List<Property> childrenList) { 296 super.listChildren(childrenList); 297 childrenList.add(new Property("sequenceLinkId", "positiveInt", "A service line number.", 0, 298 java.lang.Integer.MAX_VALUE, sequenceLinkId)); 299 childrenList.add(new Property("noteNumber", "positiveInt", 300 "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 301 childrenList.add( 302 new Property("adjudication", "", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 303 childrenList.add(new Property("detail", "", "The second tier service adjudications for submitted services.", 0, 304 java.lang.Integer.MAX_VALUE, detail)); 305 } 306 307 @Override 308 public void setProperty(String name, Base value) throws FHIRException { 309 if (name.equals("sequenceLinkId")) 310 this.sequenceLinkId = castToPositiveInt(value); // PositiveIntType 311 else if (name.equals("noteNumber")) 312 this.getNoteNumber().add(castToPositiveInt(value)); 313 else if (name.equals("adjudication")) 314 this.getAdjudication().add((ItemAdjudicationComponent) value); 315 else if (name.equals("detail")) 316 this.getDetail().add((ItemDetailComponent) value); 317 else 318 super.setProperty(name, value); 319 } 320 321 @Override 322 public Base addChild(String name) throws FHIRException { 323 if (name.equals("sequenceLinkId")) { 324 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.sequenceLinkId"); 325 } else if (name.equals("noteNumber")) { 326 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumber"); 327 } else if (name.equals("adjudication")) { 328 return addAdjudication(); 329 } else if (name.equals("detail")) { 330 return addDetail(); 331 } else 332 return super.addChild(name); 333 } 334 335 public ItemsComponent copy() { 336 ItemsComponent dst = new ItemsComponent(); 337 copyValues(dst); 338 dst.sequenceLinkId = sequenceLinkId == null ? null : sequenceLinkId.copy(); 339 if (noteNumber != null) { 340 dst.noteNumber = new ArrayList<PositiveIntType>(); 341 for (PositiveIntType i : noteNumber) 342 dst.noteNumber.add(i.copy()); 343 } 344 ; 345 if (adjudication != null) { 346 dst.adjudication = new ArrayList<ItemAdjudicationComponent>(); 347 for (ItemAdjudicationComponent i : adjudication) 348 dst.adjudication.add(i.copy()); 349 } 350 ; 351 if (detail != null) { 352 dst.detail = new ArrayList<ItemDetailComponent>(); 353 for (ItemDetailComponent i : detail) 354 dst.detail.add(i.copy()); 355 } 356 ; 357 return dst; 358 } 359 360 @Override 361 public boolean equalsDeep(Base other) { 362 if (!super.equalsDeep(other)) 363 return false; 364 if (!(other instanceof ItemsComponent)) 365 return false; 366 ItemsComponent o = (ItemsComponent) other; 367 return compareDeep(sequenceLinkId, o.sequenceLinkId, true) && compareDeep(noteNumber, o.noteNumber, true) 368 && compareDeep(adjudication, o.adjudication, true) && compareDeep(detail, o.detail, true); 369 } 370 371 @Override 372 public boolean equalsShallow(Base other) { 373 if (!super.equalsShallow(other)) 374 return false; 375 if (!(other instanceof ItemsComponent)) 376 return false; 377 ItemsComponent o = (ItemsComponent) other; 378 return compareValues(sequenceLinkId, o.sequenceLinkId, true) && compareValues(noteNumber, o.noteNumber, true); 379 } 380 381 public boolean isEmpty() { 382 return super.isEmpty() && (sequenceLinkId == null || sequenceLinkId.isEmpty()) 383 && (noteNumber == null || noteNumber.isEmpty()) && (adjudication == null || adjudication.isEmpty()) 384 && (detail == null || detail.isEmpty()); 385 } 386 387 public String fhirType() { 388 return "ClaimResponse.item"; 389 390 } 391 392 } 393 394 @Block() 395 public static class ItemAdjudicationComponent extends BackboneElement implements IBaseBackboneElement { 396 /** 397 * Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc. 398 */ 399 @Child(name = "code", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 400 @Description(shortDefinition = "Adjudication category such as co-pay, eligible, benefit, etc.", formalDefinition = "Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc.") 401 protected Coding code; 402 403 /** 404 * Monetary amount associated with the code. 405 */ 406 @Child(name = "amount", type = { Money.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 407 @Description(shortDefinition = "Monetary amount", formalDefinition = "Monetary amount associated with the code.") 408 protected Money amount; 409 410 /** 411 * A non-monetary value for example a percentage. Mutually exclusive to the 412 * amount element above. 413 */ 414 @Child(name = "value", type = { DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 415 @Description(shortDefinition = "Non-monetary value", formalDefinition = "A non-monetary value for example a percentage. Mutually exclusive to the amount element above.") 416 protected DecimalType value; 417 418 private static final long serialVersionUID = -949880587L; 419 420 /* 421 * Constructor 422 */ 423 public ItemAdjudicationComponent() { 424 super(); 425 } 426 427 /* 428 * Constructor 429 */ 430 public ItemAdjudicationComponent(Coding code) { 431 super(); 432 this.code = code; 433 } 434 435 /** 436 * @return {@link #code} (Code indicating: Co-Pay, deductible, eligible, 437 * benefit, tax, etc.) 438 */ 439 public Coding getCode() { 440 if (this.code == null) 441 if (Configuration.errorOnAutoCreate()) 442 throw new Error("Attempt to auto-create ItemAdjudicationComponent.code"); 443 else if (Configuration.doAutoCreate()) 444 this.code = new Coding(); // cc 445 return this.code; 446 } 447 448 public boolean hasCode() { 449 return this.code != null && !this.code.isEmpty(); 450 } 451 452 /** 453 * @param value {@link #code} (Code indicating: Co-Pay, deductible, eligible, 454 * benefit, tax, etc.) 455 */ 456 public ItemAdjudicationComponent setCode(Coding value) { 457 this.code = value; 458 return this; 459 } 460 461 /** 462 * @return {@link #amount} (Monetary amount associated with the code.) 463 */ 464 public Money getAmount() { 465 if (this.amount == null) 466 if (Configuration.errorOnAutoCreate()) 467 throw new Error("Attempt to auto-create ItemAdjudicationComponent.amount"); 468 else if (Configuration.doAutoCreate()) 469 this.amount = new Money(); // cc 470 return this.amount; 471 } 472 473 public boolean hasAmount() { 474 return this.amount != null && !this.amount.isEmpty(); 475 } 476 477 /** 478 * @param value {@link #amount} (Monetary amount associated with the code.) 479 */ 480 public ItemAdjudicationComponent setAmount(Money value) { 481 this.amount = value; 482 return this; 483 } 484 485 /** 486 * @return {@link #value} (A non-monetary value for example a percentage. 487 * Mutually exclusive to the amount element above.). This is the 488 * underlying object with id, value and extensions. The accessor 489 * "getValue" gives direct access to the value 490 */ 491 public DecimalType getValueElement() { 492 if (this.value == null) 493 if (Configuration.errorOnAutoCreate()) 494 throw new Error("Attempt to auto-create ItemAdjudicationComponent.value"); 495 else if (Configuration.doAutoCreate()) 496 this.value = new DecimalType(); // bb 497 return this.value; 498 } 499 500 public boolean hasValueElement() { 501 return this.value != null && !this.value.isEmpty(); 502 } 503 504 public boolean hasValue() { 505 return this.value != null && !this.value.isEmpty(); 506 } 507 508 /** 509 * @param value {@link #value} (A non-monetary value for example a percentage. 510 * Mutually exclusive to the amount element above.). This is the 511 * underlying object with id, value and extensions. The accessor 512 * "getValue" gives direct access to the value 513 */ 514 public ItemAdjudicationComponent setValueElement(DecimalType value) { 515 this.value = value; 516 return this; 517 } 518 519 /** 520 * @return A non-monetary value for example a percentage. Mutually exclusive to 521 * the amount element above. 522 */ 523 public BigDecimal getValue() { 524 return this.value == null ? null : this.value.getValue(); 525 } 526 527 /** 528 * @param value A non-monetary value for example a percentage. Mutually 529 * exclusive to the amount element above. 530 */ 531 public ItemAdjudicationComponent setValue(BigDecimal value) { 532 if (value == null) 533 this.value = null; 534 else { 535 if (this.value == null) 536 this.value = new DecimalType(); 537 this.value.setValue(value); 538 } 539 return this; 540 } 541 542 protected void listChildren(List<Property> childrenList) { 543 super.listChildren(childrenList); 544 childrenList.add(new Property("code", "Coding", 545 "Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc.", 0, java.lang.Integer.MAX_VALUE, code)); 546 childrenList.add(new Property("amount", "Money", "Monetary amount associated with the code.", 0, 547 java.lang.Integer.MAX_VALUE, amount)); 548 childrenList.add(new Property("value", "decimal", 549 "A non-monetary value for example a percentage. Mutually exclusive to the amount element above.", 0, 550 java.lang.Integer.MAX_VALUE, value)); 551 } 552 553 @Override 554 public void setProperty(String name, Base value) throws FHIRException { 555 if (name.equals("code")) 556 this.code = castToCoding(value); // Coding 557 else if (name.equals("amount")) 558 this.amount = castToMoney(value); // Money 559 else if (name.equals("value")) 560 this.value = castToDecimal(value); // DecimalType 561 else 562 super.setProperty(name, value); 563 } 564 565 @Override 566 public Base addChild(String name) throws FHIRException { 567 if (name.equals("code")) { 568 this.code = new Coding(); 569 return this.code; 570 } else if (name.equals("amount")) { 571 this.amount = new Money(); 572 return this.amount; 573 } else if (name.equals("value")) { 574 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.value"); 575 } else 576 return super.addChild(name); 577 } 578 579 public ItemAdjudicationComponent copy() { 580 ItemAdjudicationComponent dst = new ItemAdjudicationComponent(); 581 copyValues(dst); 582 dst.code = code == null ? null : code.copy(); 583 dst.amount = amount == null ? null : amount.copy(); 584 dst.value = value == null ? null : value.copy(); 585 return dst; 586 } 587 588 @Override 589 public boolean equalsDeep(Base other) { 590 if (!super.equalsDeep(other)) 591 return false; 592 if (!(other instanceof ItemAdjudicationComponent)) 593 return false; 594 ItemAdjudicationComponent o = (ItemAdjudicationComponent) other; 595 return compareDeep(code, o.code, true) && compareDeep(amount, o.amount, true) 596 && compareDeep(value, o.value, true); 597 } 598 599 @Override 600 public boolean equalsShallow(Base other) { 601 if (!super.equalsShallow(other)) 602 return false; 603 if (!(other instanceof ItemAdjudicationComponent)) 604 return false; 605 ItemAdjudicationComponent o = (ItemAdjudicationComponent) other; 606 return compareValues(value, o.value, true); 607 } 608 609 public boolean isEmpty() { 610 return super.isEmpty() && (code == null || code.isEmpty()) && (amount == null || amount.isEmpty()) 611 && (value == null || value.isEmpty()); 612 } 613 614 public String fhirType() { 615 return "ClaimResponse.item.adjudication"; 616 617 } 618 619 } 620 621 @Block() 622 public static class ItemDetailComponent extends BackboneElement implements IBaseBackboneElement { 623 /** 624 * A service line number. 625 */ 626 @Child(name = "sequenceLinkId", type = { 627 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 628 @Description(shortDefinition = "Service instance", formalDefinition = "A service line number.") 629 protected PositiveIntType sequenceLinkId; 630 631 /** 632 * The adjudications results. 633 */ 634 @Child(name = "adjudication", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 635 @Description(shortDefinition = "Detail adjudication", formalDefinition = "The adjudications results.") 636 protected List<DetailAdjudicationComponent> adjudication; 637 638 /** 639 * The third tier service adjudications for submitted services. 640 */ 641 @Child(name = "subDetail", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 642 @Description(shortDefinition = "Subdetail line items", formalDefinition = "The third tier service adjudications for submitted services.") 643 protected List<SubDetailComponent> subDetail; 644 645 private static final long serialVersionUID = -1751018357L; 646 647 /* 648 * Constructor 649 */ 650 public ItemDetailComponent() { 651 super(); 652 } 653 654 /* 655 * Constructor 656 */ 657 public ItemDetailComponent(PositiveIntType sequenceLinkId) { 658 super(); 659 this.sequenceLinkId = sequenceLinkId; 660 } 661 662 /** 663 * @return {@link #sequenceLinkId} (A service line number.). This is the 664 * underlying object with id, value and extensions. The accessor 665 * "getSequenceLinkId" gives direct access to the value 666 */ 667 public PositiveIntType getSequenceLinkIdElement() { 668 if (this.sequenceLinkId == null) 669 if (Configuration.errorOnAutoCreate()) 670 throw new Error("Attempt to auto-create ItemDetailComponent.sequenceLinkId"); 671 else if (Configuration.doAutoCreate()) 672 this.sequenceLinkId = new PositiveIntType(); // bb 673 return this.sequenceLinkId; 674 } 675 676 public boolean hasSequenceLinkIdElement() { 677 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 678 } 679 680 public boolean hasSequenceLinkId() { 681 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 682 } 683 684 /** 685 * @param value {@link #sequenceLinkId} (A service line number.). This is the 686 * underlying object with id, value and extensions. The accessor 687 * "getSequenceLinkId" gives direct access to the value 688 */ 689 public ItemDetailComponent setSequenceLinkIdElement(PositiveIntType value) { 690 this.sequenceLinkId = value; 691 return this; 692 } 693 694 /** 695 * @return A service line number. 696 */ 697 public int getSequenceLinkId() { 698 return this.sequenceLinkId == null || this.sequenceLinkId.isEmpty() ? 0 : this.sequenceLinkId.getValue(); 699 } 700 701 /** 702 * @param value A service line number. 703 */ 704 public ItemDetailComponent setSequenceLinkId(int value) { 705 if (this.sequenceLinkId == null) 706 this.sequenceLinkId = new PositiveIntType(); 707 this.sequenceLinkId.setValue(value); 708 return this; 709 } 710 711 /** 712 * @return {@link #adjudication} (The adjudications results.) 713 */ 714 public List<DetailAdjudicationComponent> getAdjudication() { 715 if (this.adjudication == null) 716 this.adjudication = new ArrayList<DetailAdjudicationComponent>(); 717 return this.adjudication; 718 } 719 720 public boolean hasAdjudication() { 721 if (this.adjudication == null) 722 return false; 723 for (DetailAdjudicationComponent item : this.adjudication) 724 if (!item.isEmpty()) 725 return true; 726 return false; 727 } 728 729 /** 730 * @return {@link #adjudication} (The adjudications results.) 731 */ 732 // syntactic sugar 733 public DetailAdjudicationComponent addAdjudication() { // 3 734 DetailAdjudicationComponent t = new DetailAdjudicationComponent(); 735 if (this.adjudication == null) 736 this.adjudication = new ArrayList<DetailAdjudicationComponent>(); 737 this.adjudication.add(t); 738 return t; 739 } 740 741 // syntactic sugar 742 public ItemDetailComponent addAdjudication(DetailAdjudicationComponent t) { // 3 743 if (t == null) 744 return this; 745 if (this.adjudication == null) 746 this.adjudication = new ArrayList<DetailAdjudicationComponent>(); 747 this.adjudication.add(t); 748 return this; 749 } 750 751 /** 752 * @return {@link #subDetail} (The third tier service adjudications for 753 * submitted services.) 754 */ 755 public List<SubDetailComponent> getSubDetail() { 756 if (this.subDetail == null) 757 this.subDetail = new ArrayList<SubDetailComponent>(); 758 return this.subDetail; 759 } 760 761 public boolean hasSubDetail() { 762 if (this.subDetail == null) 763 return false; 764 for (SubDetailComponent item : this.subDetail) 765 if (!item.isEmpty()) 766 return true; 767 return false; 768 } 769 770 /** 771 * @return {@link #subDetail} (The third tier service adjudications for 772 * submitted services.) 773 */ 774 // syntactic sugar 775 public SubDetailComponent addSubDetail() { // 3 776 SubDetailComponent t = new SubDetailComponent(); 777 if (this.subDetail == null) 778 this.subDetail = new ArrayList<SubDetailComponent>(); 779 this.subDetail.add(t); 780 return t; 781 } 782 783 // syntactic sugar 784 public ItemDetailComponent addSubDetail(SubDetailComponent t) { // 3 785 if (t == null) 786 return this; 787 if (this.subDetail == null) 788 this.subDetail = new ArrayList<SubDetailComponent>(); 789 this.subDetail.add(t); 790 return this; 791 } 792 793 protected void listChildren(List<Property> childrenList) { 794 super.listChildren(childrenList); 795 childrenList.add(new Property("sequenceLinkId", "positiveInt", "A service line number.", 0, 796 java.lang.Integer.MAX_VALUE, sequenceLinkId)); 797 childrenList.add( 798 new Property("adjudication", "", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 799 childrenList.add(new Property("subDetail", "", "The third tier service adjudications for submitted services.", 0, 800 java.lang.Integer.MAX_VALUE, subDetail)); 801 } 802 803 @Override 804 public void setProperty(String name, Base value) throws FHIRException { 805 if (name.equals("sequenceLinkId")) 806 this.sequenceLinkId = castToPositiveInt(value); // PositiveIntType 807 else if (name.equals("adjudication")) 808 this.getAdjudication().add((DetailAdjudicationComponent) value); 809 else if (name.equals("subDetail")) 810 this.getSubDetail().add((SubDetailComponent) value); 811 else 812 super.setProperty(name, value); 813 } 814 815 @Override 816 public Base addChild(String name) throws FHIRException { 817 if (name.equals("sequenceLinkId")) { 818 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.sequenceLinkId"); 819 } else if (name.equals("adjudication")) { 820 return addAdjudication(); 821 } else if (name.equals("subDetail")) { 822 return addSubDetail(); 823 } else 824 return super.addChild(name); 825 } 826 827 public ItemDetailComponent copy() { 828 ItemDetailComponent dst = new ItemDetailComponent(); 829 copyValues(dst); 830 dst.sequenceLinkId = sequenceLinkId == null ? null : sequenceLinkId.copy(); 831 if (adjudication != null) { 832 dst.adjudication = new ArrayList<DetailAdjudicationComponent>(); 833 for (DetailAdjudicationComponent i : adjudication) 834 dst.adjudication.add(i.copy()); 835 } 836 ; 837 if (subDetail != null) { 838 dst.subDetail = new ArrayList<SubDetailComponent>(); 839 for (SubDetailComponent i : subDetail) 840 dst.subDetail.add(i.copy()); 841 } 842 ; 843 return dst; 844 } 845 846 @Override 847 public boolean equalsDeep(Base other) { 848 if (!super.equalsDeep(other)) 849 return false; 850 if (!(other instanceof ItemDetailComponent)) 851 return false; 852 ItemDetailComponent o = (ItemDetailComponent) other; 853 return compareDeep(sequenceLinkId, o.sequenceLinkId, true) && compareDeep(adjudication, o.adjudication, true) 854 && compareDeep(subDetail, o.subDetail, true); 855 } 856 857 @Override 858 public boolean equalsShallow(Base other) { 859 if (!super.equalsShallow(other)) 860 return false; 861 if (!(other instanceof ItemDetailComponent)) 862 return false; 863 ItemDetailComponent o = (ItemDetailComponent) other; 864 return compareValues(sequenceLinkId, o.sequenceLinkId, true); 865 } 866 867 public boolean isEmpty() { 868 return super.isEmpty() && (sequenceLinkId == null || sequenceLinkId.isEmpty()) 869 && (adjudication == null || adjudication.isEmpty()) && (subDetail == null || subDetail.isEmpty()); 870 } 871 872 public String fhirType() { 873 return "ClaimResponse.item.detail"; 874 875 } 876 877 } 878 879 @Block() 880 public static class DetailAdjudicationComponent extends BackboneElement implements IBaseBackboneElement { 881 /** 882 * Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc. 883 */ 884 @Child(name = "code", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 885 @Description(shortDefinition = "Adjudication category such as co-pay, eligible, benefit, etc.", formalDefinition = "Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc.") 886 protected Coding code; 887 888 /** 889 * Monetary amount associated with the code. 890 */ 891 @Child(name = "amount", type = { Money.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 892 @Description(shortDefinition = "Monetary amount", formalDefinition = "Monetary amount associated with the code.") 893 protected Money amount; 894 895 /** 896 * A non-monetary value for example a percentage. Mutually exclusive to the 897 * amount element above. 898 */ 899 @Child(name = "value", type = { DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 900 @Description(shortDefinition = "Non-monetary value", formalDefinition = "A non-monetary value for example a percentage. Mutually exclusive to the amount element above.") 901 protected DecimalType value; 902 903 private static final long serialVersionUID = -949880587L; 904 905 /* 906 * Constructor 907 */ 908 public DetailAdjudicationComponent() { 909 super(); 910 } 911 912 /* 913 * Constructor 914 */ 915 public DetailAdjudicationComponent(Coding code) { 916 super(); 917 this.code = code; 918 } 919 920 /** 921 * @return {@link #code} (Code indicating: Co-Pay, deductible, eligible, 922 * benefit, tax, etc.) 923 */ 924 public Coding getCode() { 925 if (this.code == null) 926 if (Configuration.errorOnAutoCreate()) 927 throw new Error("Attempt to auto-create DetailAdjudicationComponent.code"); 928 else if (Configuration.doAutoCreate()) 929 this.code = new Coding(); // cc 930 return this.code; 931 } 932 933 public boolean hasCode() { 934 return this.code != null && !this.code.isEmpty(); 935 } 936 937 /** 938 * @param value {@link #code} (Code indicating: Co-Pay, deductible, eligible, 939 * benefit, tax, etc.) 940 */ 941 public DetailAdjudicationComponent setCode(Coding value) { 942 this.code = value; 943 return this; 944 } 945 946 /** 947 * @return {@link #amount} (Monetary amount associated with the code.) 948 */ 949 public Money getAmount() { 950 if (this.amount == null) 951 if (Configuration.errorOnAutoCreate()) 952 throw new Error("Attempt to auto-create DetailAdjudicationComponent.amount"); 953 else if (Configuration.doAutoCreate()) 954 this.amount = new Money(); // cc 955 return this.amount; 956 } 957 958 public boolean hasAmount() { 959 return this.amount != null && !this.amount.isEmpty(); 960 } 961 962 /** 963 * @param value {@link #amount} (Monetary amount associated with the code.) 964 */ 965 public DetailAdjudicationComponent setAmount(Money value) { 966 this.amount = value; 967 return this; 968 } 969 970 /** 971 * @return {@link #value} (A non-monetary value for example a percentage. 972 * Mutually exclusive to the amount element above.). This is the 973 * underlying object with id, value and extensions. The accessor 974 * "getValue" gives direct access to the value 975 */ 976 public DecimalType getValueElement() { 977 if (this.value == null) 978 if (Configuration.errorOnAutoCreate()) 979 throw new Error("Attempt to auto-create DetailAdjudicationComponent.value"); 980 else if (Configuration.doAutoCreate()) 981 this.value = new DecimalType(); // bb 982 return this.value; 983 } 984 985 public boolean hasValueElement() { 986 return this.value != null && !this.value.isEmpty(); 987 } 988 989 public boolean hasValue() { 990 return this.value != null && !this.value.isEmpty(); 991 } 992 993 /** 994 * @param value {@link #value} (A non-monetary value for example a percentage. 995 * Mutually exclusive to the amount element above.). This is the 996 * underlying object with id, value and extensions. The accessor 997 * "getValue" gives direct access to the value 998 */ 999 public DetailAdjudicationComponent setValueElement(DecimalType value) { 1000 this.value = value; 1001 return this; 1002 } 1003 1004 /** 1005 * @return A non-monetary value for example a percentage. Mutually exclusive to 1006 * the amount element above. 1007 */ 1008 public BigDecimal getValue() { 1009 return this.value == null ? null : this.value.getValue(); 1010 } 1011 1012 /** 1013 * @param value A non-monetary value for example a percentage. Mutually 1014 * exclusive to the amount element above. 1015 */ 1016 public DetailAdjudicationComponent setValue(BigDecimal value) { 1017 if (value == null) 1018 this.value = null; 1019 else { 1020 if (this.value == null) 1021 this.value = new DecimalType(); 1022 this.value.setValue(value); 1023 } 1024 return this; 1025 } 1026 1027 protected void listChildren(List<Property> childrenList) { 1028 super.listChildren(childrenList); 1029 childrenList.add(new Property("code", "Coding", 1030 "Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc.", 0, java.lang.Integer.MAX_VALUE, code)); 1031 childrenList.add(new Property("amount", "Money", "Monetary amount associated with the code.", 0, 1032 java.lang.Integer.MAX_VALUE, amount)); 1033 childrenList.add(new Property("value", "decimal", 1034 "A non-monetary value for example a percentage. Mutually exclusive to the amount element above.", 0, 1035 java.lang.Integer.MAX_VALUE, value)); 1036 } 1037 1038 @Override 1039 public void setProperty(String name, Base value) throws FHIRException { 1040 if (name.equals("code")) 1041 this.code = castToCoding(value); // Coding 1042 else if (name.equals("amount")) 1043 this.amount = castToMoney(value); // Money 1044 else if (name.equals("value")) 1045 this.value = castToDecimal(value); // DecimalType 1046 else 1047 super.setProperty(name, value); 1048 } 1049 1050 @Override 1051 public Base addChild(String name) throws FHIRException { 1052 if (name.equals("code")) { 1053 this.code = new Coding(); 1054 return this.code; 1055 } else if (name.equals("amount")) { 1056 this.amount = new Money(); 1057 return this.amount; 1058 } else if (name.equals("value")) { 1059 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.value"); 1060 } else 1061 return super.addChild(name); 1062 } 1063 1064 public DetailAdjudicationComponent copy() { 1065 DetailAdjudicationComponent dst = new DetailAdjudicationComponent(); 1066 copyValues(dst); 1067 dst.code = code == null ? null : code.copy(); 1068 dst.amount = amount == null ? null : amount.copy(); 1069 dst.value = value == null ? null : value.copy(); 1070 return dst; 1071 } 1072 1073 @Override 1074 public boolean equalsDeep(Base other) { 1075 if (!super.equalsDeep(other)) 1076 return false; 1077 if (!(other instanceof DetailAdjudicationComponent)) 1078 return false; 1079 DetailAdjudicationComponent o = (DetailAdjudicationComponent) other; 1080 return compareDeep(code, o.code, true) && compareDeep(amount, o.amount, true) 1081 && compareDeep(value, o.value, true); 1082 } 1083 1084 @Override 1085 public boolean equalsShallow(Base other) { 1086 if (!super.equalsShallow(other)) 1087 return false; 1088 if (!(other instanceof DetailAdjudicationComponent)) 1089 return false; 1090 DetailAdjudicationComponent o = (DetailAdjudicationComponent) other; 1091 return compareValues(value, o.value, true); 1092 } 1093 1094 public boolean isEmpty() { 1095 return super.isEmpty() && (code == null || code.isEmpty()) && (amount == null || amount.isEmpty()) 1096 && (value == null || value.isEmpty()); 1097 } 1098 1099 public String fhirType() { 1100 return "ClaimResponse.item.detail.adjudication"; 1101 1102 } 1103 1104 } 1105 1106 @Block() 1107 public static class SubDetailComponent extends BackboneElement implements IBaseBackboneElement { 1108 /** 1109 * A service line number. 1110 */ 1111 @Child(name = "sequenceLinkId", type = { 1112 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1113 @Description(shortDefinition = "Service instance", formalDefinition = "A service line number.") 1114 protected PositiveIntType sequenceLinkId; 1115 1116 /** 1117 * The adjudications results. 1118 */ 1119 @Child(name = "adjudication", type = {}, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1120 @Description(shortDefinition = "Subdetail adjudication", formalDefinition = "The adjudications results.") 1121 protected List<SubdetailAdjudicationComponent> adjudication; 1122 1123 private static final long serialVersionUID = 1780202110L; 1124 1125 /* 1126 * Constructor 1127 */ 1128 public SubDetailComponent() { 1129 super(); 1130 } 1131 1132 /* 1133 * Constructor 1134 */ 1135 public SubDetailComponent(PositiveIntType sequenceLinkId) { 1136 super(); 1137 this.sequenceLinkId = sequenceLinkId; 1138 } 1139 1140 /** 1141 * @return {@link #sequenceLinkId} (A service line number.). This is the 1142 * underlying object with id, value and extensions. The accessor 1143 * "getSequenceLinkId" gives direct access to the value 1144 */ 1145 public PositiveIntType getSequenceLinkIdElement() { 1146 if (this.sequenceLinkId == null) 1147 if (Configuration.errorOnAutoCreate()) 1148 throw new Error("Attempt to auto-create SubDetailComponent.sequenceLinkId"); 1149 else if (Configuration.doAutoCreate()) 1150 this.sequenceLinkId = new PositiveIntType(); // bb 1151 return this.sequenceLinkId; 1152 } 1153 1154 public boolean hasSequenceLinkIdElement() { 1155 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 1156 } 1157 1158 public boolean hasSequenceLinkId() { 1159 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 1160 } 1161 1162 /** 1163 * @param value {@link #sequenceLinkId} (A service line number.). This is the 1164 * underlying object with id, value and extensions. The accessor 1165 * "getSequenceLinkId" gives direct access to the value 1166 */ 1167 public SubDetailComponent setSequenceLinkIdElement(PositiveIntType value) { 1168 this.sequenceLinkId = value; 1169 return this; 1170 } 1171 1172 /** 1173 * @return A service line number. 1174 */ 1175 public int getSequenceLinkId() { 1176 return this.sequenceLinkId == null || this.sequenceLinkId.isEmpty() ? 0 : this.sequenceLinkId.getValue(); 1177 } 1178 1179 /** 1180 * @param value A service line number. 1181 */ 1182 public SubDetailComponent setSequenceLinkId(int value) { 1183 if (this.sequenceLinkId == null) 1184 this.sequenceLinkId = new PositiveIntType(); 1185 this.sequenceLinkId.setValue(value); 1186 return this; 1187 } 1188 1189 /** 1190 * @return {@link #adjudication} (The adjudications results.) 1191 */ 1192 public List<SubdetailAdjudicationComponent> getAdjudication() { 1193 if (this.adjudication == null) 1194 this.adjudication = new ArrayList<SubdetailAdjudicationComponent>(); 1195 return this.adjudication; 1196 } 1197 1198 public boolean hasAdjudication() { 1199 if (this.adjudication == null) 1200 return false; 1201 for (SubdetailAdjudicationComponent item : this.adjudication) 1202 if (!item.isEmpty()) 1203 return true; 1204 return false; 1205 } 1206 1207 /** 1208 * @return {@link #adjudication} (The adjudications results.) 1209 */ 1210 // syntactic sugar 1211 public SubdetailAdjudicationComponent addAdjudication() { // 3 1212 SubdetailAdjudicationComponent t = new SubdetailAdjudicationComponent(); 1213 if (this.adjudication == null) 1214 this.adjudication = new ArrayList<SubdetailAdjudicationComponent>(); 1215 this.adjudication.add(t); 1216 return t; 1217 } 1218 1219 // syntactic sugar 1220 public SubDetailComponent addAdjudication(SubdetailAdjudicationComponent t) { // 3 1221 if (t == null) 1222 return this; 1223 if (this.adjudication == null) 1224 this.adjudication = new ArrayList<SubdetailAdjudicationComponent>(); 1225 this.adjudication.add(t); 1226 return this; 1227 } 1228 1229 protected void listChildren(List<Property> childrenList) { 1230 super.listChildren(childrenList); 1231 childrenList.add(new Property("sequenceLinkId", "positiveInt", "A service line number.", 0, 1232 java.lang.Integer.MAX_VALUE, sequenceLinkId)); 1233 childrenList.add( 1234 new Property("adjudication", "", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 1235 } 1236 1237 @Override 1238 public void setProperty(String name, Base value) throws FHIRException { 1239 if (name.equals("sequenceLinkId")) 1240 this.sequenceLinkId = castToPositiveInt(value); // PositiveIntType 1241 else if (name.equals("adjudication")) 1242 this.getAdjudication().add((SubdetailAdjudicationComponent) value); 1243 else 1244 super.setProperty(name, value); 1245 } 1246 1247 @Override 1248 public Base addChild(String name) throws FHIRException { 1249 if (name.equals("sequenceLinkId")) { 1250 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.sequenceLinkId"); 1251 } else if (name.equals("adjudication")) { 1252 return addAdjudication(); 1253 } else 1254 return super.addChild(name); 1255 } 1256 1257 public SubDetailComponent copy() { 1258 SubDetailComponent dst = new SubDetailComponent(); 1259 copyValues(dst); 1260 dst.sequenceLinkId = sequenceLinkId == null ? null : sequenceLinkId.copy(); 1261 if (adjudication != null) { 1262 dst.adjudication = new ArrayList<SubdetailAdjudicationComponent>(); 1263 for (SubdetailAdjudicationComponent i : adjudication) 1264 dst.adjudication.add(i.copy()); 1265 } 1266 ; 1267 return dst; 1268 } 1269 1270 @Override 1271 public boolean equalsDeep(Base other) { 1272 if (!super.equalsDeep(other)) 1273 return false; 1274 if (!(other instanceof SubDetailComponent)) 1275 return false; 1276 SubDetailComponent o = (SubDetailComponent) other; 1277 return compareDeep(sequenceLinkId, o.sequenceLinkId, true) && compareDeep(adjudication, o.adjudication, true); 1278 } 1279 1280 @Override 1281 public boolean equalsShallow(Base other) { 1282 if (!super.equalsShallow(other)) 1283 return false; 1284 if (!(other instanceof SubDetailComponent)) 1285 return false; 1286 SubDetailComponent o = (SubDetailComponent) other; 1287 return compareValues(sequenceLinkId, o.sequenceLinkId, true); 1288 } 1289 1290 public boolean isEmpty() { 1291 return super.isEmpty() && (sequenceLinkId == null || sequenceLinkId.isEmpty()) 1292 && (adjudication == null || adjudication.isEmpty()); 1293 } 1294 1295 public String fhirType() { 1296 return "ClaimResponse.item.detail.subDetail"; 1297 1298 } 1299 1300 } 1301 1302 @Block() 1303 public static class SubdetailAdjudicationComponent extends BackboneElement implements IBaseBackboneElement { 1304 /** 1305 * Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc. 1306 */ 1307 @Child(name = "code", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1308 @Description(shortDefinition = "Adjudication category such as co-pay, eligible, benefit, etc.", formalDefinition = "Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc.") 1309 protected Coding code; 1310 1311 /** 1312 * Monetary amount associated with the code. 1313 */ 1314 @Child(name = "amount", type = { Money.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1315 @Description(shortDefinition = "Monetary amount", formalDefinition = "Monetary amount associated with the code.") 1316 protected Money amount; 1317 1318 /** 1319 * A non-monetary value for example a percentage. Mutually exclusive to the 1320 * amount element above. 1321 */ 1322 @Child(name = "value", type = { DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1323 @Description(shortDefinition = "Non-monetary value", formalDefinition = "A non-monetary value for example a percentage. Mutually exclusive to the amount element above.") 1324 protected DecimalType value; 1325 1326 private static final long serialVersionUID = -949880587L; 1327 1328 /* 1329 * Constructor 1330 */ 1331 public SubdetailAdjudicationComponent() { 1332 super(); 1333 } 1334 1335 /* 1336 * Constructor 1337 */ 1338 public SubdetailAdjudicationComponent(Coding code) { 1339 super(); 1340 this.code = code; 1341 } 1342 1343 /** 1344 * @return {@link #code} (Code indicating: Co-Pay, deductible, eligible, 1345 * benefit, tax, etc.) 1346 */ 1347 public Coding getCode() { 1348 if (this.code == null) 1349 if (Configuration.errorOnAutoCreate()) 1350 throw new Error("Attempt to auto-create SubdetailAdjudicationComponent.code"); 1351 else if (Configuration.doAutoCreate()) 1352 this.code = new Coding(); // cc 1353 return this.code; 1354 } 1355 1356 public boolean hasCode() { 1357 return this.code != null && !this.code.isEmpty(); 1358 } 1359 1360 /** 1361 * @param value {@link #code} (Code indicating: Co-Pay, deductible, eligible, 1362 * benefit, tax, etc.) 1363 */ 1364 public SubdetailAdjudicationComponent setCode(Coding value) { 1365 this.code = value; 1366 return this; 1367 } 1368 1369 /** 1370 * @return {@link #amount} (Monetary amount associated with the code.) 1371 */ 1372 public Money getAmount() { 1373 if (this.amount == null) 1374 if (Configuration.errorOnAutoCreate()) 1375 throw new Error("Attempt to auto-create SubdetailAdjudicationComponent.amount"); 1376 else if (Configuration.doAutoCreate()) 1377 this.amount = new Money(); // cc 1378 return this.amount; 1379 } 1380 1381 public boolean hasAmount() { 1382 return this.amount != null && !this.amount.isEmpty(); 1383 } 1384 1385 /** 1386 * @param value {@link #amount} (Monetary amount associated with the code.) 1387 */ 1388 public SubdetailAdjudicationComponent setAmount(Money value) { 1389 this.amount = value; 1390 return this; 1391 } 1392 1393 /** 1394 * @return {@link #value} (A non-monetary value for example a percentage. 1395 * Mutually exclusive to the amount element above.). This is the 1396 * underlying object with id, value and extensions. The accessor 1397 * "getValue" gives direct access to the value 1398 */ 1399 public DecimalType getValueElement() { 1400 if (this.value == null) 1401 if (Configuration.errorOnAutoCreate()) 1402 throw new Error("Attempt to auto-create SubdetailAdjudicationComponent.value"); 1403 else if (Configuration.doAutoCreate()) 1404 this.value = new DecimalType(); // bb 1405 return this.value; 1406 } 1407 1408 public boolean hasValueElement() { 1409 return this.value != null && !this.value.isEmpty(); 1410 } 1411 1412 public boolean hasValue() { 1413 return this.value != null && !this.value.isEmpty(); 1414 } 1415 1416 /** 1417 * @param value {@link #value} (A non-monetary value for example a percentage. 1418 * Mutually exclusive to the amount element above.). This is the 1419 * underlying object with id, value and extensions. The accessor 1420 * "getValue" gives direct access to the value 1421 */ 1422 public SubdetailAdjudicationComponent setValueElement(DecimalType value) { 1423 this.value = value; 1424 return this; 1425 } 1426 1427 /** 1428 * @return A non-monetary value for example a percentage. Mutually exclusive to 1429 * the amount element above. 1430 */ 1431 public BigDecimal getValue() { 1432 return this.value == null ? null : this.value.getValue(); 1433 } 1434 1435 /** 1436 * @param value A non-monetary value for example a percentage. Mutually 1437 * exclusive to the amount element above. 1438 */ 1439 public SubdetailAdjudicationComponent setValue(BigDecimal value) { 1440 if (value == null) 1441 this.value = null; 1442 else { 1443 if (this.value == null) 1444 this.value = new DecimalType(); 1445 this.value.setValue(value); 1446 } 1447 return this; 1448 } 1449 1450 protected void listChildren(List<Property> childrenList) { 1451 super.listChildren(childrenList); 1452 childrenList.add(new Property("code", "Coding", 1453 "Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc.", 0, java.lang.Integer.MAX_VALUE, code)); 1454 childrenList.add(new Property("amount", "Money", "Monetary amount associated with the code.", 0, 1455 java.lang.Integer.MAX_VALUE, amount)); 1456 childrenList.add(new Property("value", "decimal", 1457 "A non-monetary value for example a percentage. Mutually exclusive to the amount element above.", 0, 1458 java.lang.Integer.MAX_VALUE, value)); 1459 } 1460 1461 @Override 1462 public void setProperty(String name, Base value) throws FHIRException { 1463 if (name.equals("code")) 1464 this.code = castToCoding(value); // Coding 1465 else if (name.equals("amount")) 1466 this.amount = castToMoney(value); // Money 1467 else if (name.equals("value")) 1468 this.value = castToDecimal(value); // DecimalType 1469 else 1470 super.setProperty(name, value); 1471 } 1472 1473 @Override 1474 public Base addChild(String name) throws FHIRException { 1475 if (name.equals("code")) { 1476 this.code = new Coding(); 1477 return this.code; 1478 } else if (name.equals("amount")) { 1479 this.amount = new Money(); 1480 return this.amount; 1481 } else if (name.equals("value")) { 1482 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.value"); 1483 } else 1484 return super.addChild(name); 1485 } 1486 1487 public SubdetailAdjudicationComponent copy() { 1488 SubdetailAdjudicationComponent dst = new SubdetailAdjudicationComponent(); 1489 copyValues(dst); 1490 dst.code = code == null ? null : code.copy(); 1491 dst.amount = amount == null ? null : amount.copy(); 1492 dst.value = value == null ? null : value.copy(); 1493 return dst; 1494 } 1495 1496 @Override 1497 public boolean equalsDeep(Base other) { 1498 if (!super.equalsDeep(other)) 1499 return false; 1500 if (!(other instanceof SubdetailAdjudicationComponent)) 1501 return false; 1502 SubdetailAdjudicationComponent o = (SubdetailAdjudicationComponent) other; 1503 return compareDeep(code, o.code, true) && compareDeep(amount, o.amount, true) 1504 && compareDeep(value, o.value, true); 1505 } 1506 1507 @Override 1508 public boolean equalsShallow(Base other) { 1509 if (!super.equalsShallow(other)) 1510 return false; 1511 if (!(other instanceof SubdetailAdjudicationComponent)) 1512 return false; 1513 SubdetailAdjudicationComponent o = (SubdetailAdjudicationComponent) other; 1514 return compareValues(value, o.value, true); 1515 } 1516 1517 public boolean isEmpty() { 1518 return super.isEmpty() && (code == null || code.isEmpty()) && (amount == null || amount.isEmpty()) 1519 && (value == null || value.isEmpty()); 1520 } 1521 1522 public String fhirType() { 1523 return "ClaimResponse.item.detail.subDetail.adjudication"; 1524 1525 } 1526 1527 } 1528 1529 @Block() 1530 public static class AddedItemComponent extends BackboneElement implements IBaseBackboneElement { 1531 /** 1532 * List of input service items which this service line is intended to replace. 1533 */ 1534 @Child(name = "sequenceLinkId", type = { 1535 PositiveIntType.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1536 @Description(shortDefinition = "Service instances", formalDefinition = "List of input service items which this service line is intended to replace.") 1537 protected List<PositiveIntType> sequenceLinkId; 1538 1539 /** 1540 * A code to indicate the Professional Service or Product supplied. 1541 */ 1542 @Child(name = "service", type = { Coding.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 1543 @Description(shortDefinition = "Group, Service or Product", formalDefinition = "A code to indicate the Professional Service or Product supplied.") 1544 protected Coding service; 1545 1546 /** 1547 * The fee charged for the professional service or product.. 1548 */ 1549 @Child(name = "fee", type = { Money.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1550 @Description(shortDefinition = "Professional fee or Product charge", formalDefinition = "The fee charged for the professional service or product..") 1551 protected Money fee; 1552 1553 /** 1554 * A list of note references to the notes provided below. 1555 */ 1556 @Child(name = "noteNumberLinkId", type = { 1557 PositiveIntType.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1558 @Description(shortDefinition = "List of note numbers which apply", formalDefinition = "A list of note references to the notes provided below.") 1559 protected List<PositiveIntType> noteNumberLinkId; 1560 1561 /** 1562 * The adjudications results. 1563 */ 1564 @Child(name = "adjudication", type = {}, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1565 @Description(shortDefinition = "Added items adjudication", formalDefinition = "The adjudications results.") 1566 protected List<AddedItemAdjudicationComponent> adjudication; 1567 1568 /** 1569 * The second tier service adjudications for payor added services. 1570 */ 1571 @Child(name = "detail", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 1572 @Description(shortDefinition = "Added items details", formalDefinition = "The second tier service adjudications for payor added services.") 1573 protected List<AddedItemsDetailComponent> detail; 1574 1575 private static final long serialVersionUID = -1675935854L; 1576 1577 /* 1578 * Constructor 1579 */ 1580 public AddedItemComponent() { 1581 super(); 1582 } 1583 1584 /* 1585 * Constructor 1586 */ 1587 public AddedItemComponent(Coding service) { 1588 super(); 1589 this.service = service; 1590 } 1591 1592 /** 1593 * @return {@link #sequenceLinkId} (List of input service items which this 1594 * service line is intended to replace.) 1595 */ 1596 public List<PositiveIntType> getSequenceLinkId() { 1597 if (this.sequenceLinkId == null) 1598 this.sequenceLinkId = new ArrayList<PositiveIntType>(); 1599 return this.sequenceLinkId; 1600 } 1601 1602 public boolean hasSequenceLinkId() { 1603 if (this.sequenceLinkId == null) 1604 return false; 1605 for (PositiveIntType item : this.sequenceLinkId) 1606 if (!item.isEmpty()) 1607 return true; 1608 return false; 1609 } 1610 1611 /** 1612 * @return {@link #sequenceLinkId} (List of input service items which this 1613 * service line is intended to replace.) 1614 */ 1615 // syntactic sugar 1616 public PositiveIntType addSequenceLinkIdElement() {// 2 1617 PositiveIntType t = new PositiveIntType(); 1618 if (this.sequenceLinkId == null) 1619 this.sequenceLinkId = new ArrayList<PositiveIntType>(); 1620 this.sequenceLinkId.add(t); 1621 return t; 1622 } 1623 1624 /** 1625 * @param value {@link #sequenceLinkId} (List of input service items which this 1626 * service line is intended to replace.) 1627 */ 1628 public AddedItemComponent addSequenceLinkId(int value) { // 1 1629 PositiveIntType t = new PositiveIntType(); 1630 t.setValue(value); 1631 if (this.sequenceLinkId == null) 1632 this.sequenceLinkId = new ArrayList<PositiveIntType>(); 1633 this.sequenceLinkId.add(t); 1634 return this; 1635 } 1636 1637 /** 1638 * @param value {@link #sequenceLinkId} (List of input service items which this 1639 * service line is intended to replace.) 1640 */ 1641 public boolean hasSequenceLinkId(int value) { 1642 if (this.sequenceLinkId == null) 1643 return false; 1644 for (PositiveIntType v : this.sequenceLinkId) 1645 if (v.equals(value)) // positiveInt 1646 return true; 1647 return false; 1648 } 1649 1650 /** 1651 * @return {@link #service} (A code to indicate the Professional Service or 1652 * Product supplied.) 1653 */ 1654 public Coding getService() { 1655 if (this.service == null) 1656 if (Configuration.errorOnAutoCreate()) 1657 throw new Error("Attempt to auto-create AddedItemComponent.service"); 1658 else if (Configuration.doAutoCreate()) 1659 this.service = new Coding(); // cc 1660 return this.service; 1661 } 1662 1663 public boolean hasService() { 1664 return this.service != null && !this.service.isEmpty(); 1665 } 1666 1667 /** 1668 * @param value {@link #service} (A code to indicate the Professional Service or 1669 * Product supplied.) 1670 */ 1671 public AddedItemComponent setService(Coding value) { 1672 this.service = value; 1673 return this; 1674 } 1675 1676 /** 1677 * @return {@link #fee} (The fee charged for the professional service or 1678 * product..) 1679 */ 1680 public Money getFee() { 1681 if (this.fee == null) 1682 if (Configuration.errorOnAutoCreate()) 1683 throw new Error("Attempt to auto-create AddedItemComponent.fee"); 1684 else if (Configuration.doAutoCreate()) 1685 this.fee = new Money(); // cc 1686 return this.fee; 1687 } 1688 1689 public boolean hasFee() { 1690 return this.fee != null && !this.fee.isEmpty(); 1691 } 1692 1693 /** 1694 * @param value {@link #fee} (The fee charged for the professional service or 1695 * product..) 1696 */ 1697 public AddedItemComponent setFee(Money value) { 1698 this.fee = value; 1699 return this; 1700 } 1701 1702 /** 1703 * @return {@link #noteNumberLinkId} (A list of note references to the notes 1704 * provided below.) 1705 */ 1706 public List<PositiveIntType> getNoteNumberLinkId() { 1707 if (this.noteNumberLinkId == null) 1708 this.noteNumberLinkId = new ArrayList<PositiveIntType>(); 1709 return this.noteNumberLinkId; 1710 } 1711 1712 public boolean hasNoteNumberLinkId() { 1713 if (this.noteNumberLinkId == null) 1714 return false; 1715 for (PositiveIntType item : this.noteNumberLinkId) 1716 if (!item.isEmpty()) 1717 return true; 1718 return false; 1719 } 1720 1721 /** 1722 * @return {@link #noteNumberLinkId} (A list of note references to the notes 1723 * provided below.) 1724 */ 1725 // syntactic sugar 1726 public PositiveIntType addNoteNumberLinkIdElement() {// 2 1727 PositiveIntType t = new PositiveIntType(); 1728 if (this.noteNumberLinkId == null) 1729 this.noteNumberLinkId = new ArrayList<PositiveIntType>(); 1730 this.noteNumberLinkId.add(t); 1731 return t; 1732 } 1733 1734 /** 1735 * @param value {@link #noteNumberLinkId} (A list of note references to the 1736 * notes provided below.) 1737 */ 1738 public AddedItemComponent addNoteNumberLinkId(int value) { // 1 1739 PositiveIntType t = new PositiveIntType(); 1740 t.setValue(value); 1741 if (this.noteNumberLinkId == null) 1742 this.noteNumberLinkId = new ArrayList<PositiveIntType>(); 1743 this.noteNumberLinkId.add(t); 1744 return this; 1745 } 1746 1747 /** 1748 * @param value {@link #noteNumberLinkId} (A list of note references to the 1749 * notes provided below.) 1750 */ 1751 public boolean hasNoteNumberLinkId(int value) { 1752 if (this.noteNumberLinkId == null) 1753 return false; 1754 for (PositiveIntType v : this.noteNumberLinkId) 1755 if (v.equals(value)) // positiveInt 1756 return true; 1757 return false; 1758 } 1759 1760 /** 1761 * @return {@link #adjudication} (The adjudications results.) 1762 */ 1763 public List<AddedItemAdjudicationComponent> getAdjudication() { 1764 if (this.adjudication == null) 1765 this.adjudication = new ArrayList<AddedItemAdjudicationComponent>(); 1766 return this.adjudication; 1767 } 1768 1769 public boolean hasAdjudication() { 1770 if (this.adjudication == null) 1771 return false; 1772 for (AddedItemAdjudicationComponent item : this.adjudication) 1773 if (!item.isEmpty()) 1774 return true; 1775 return false; 1776 } 1777 1778 /** 1779 * @return {@link #adjudication} (The adjudications results.) 1780 */ 1781 // syntactic sugar 1782 public AddedItemAdjudicationComponent addAdjudication() { // 3 1783 AddedItemAdjudicationComponent t = new AddedItemAdjudicationComponent(); 1784 if (this.adjudication == null) 1785 this.adjudication = new ArrayList<AddedItemAdjudicationComponent>(); 1786 this.adjudication.add(t); 1787 return t; 1788 } 1789 1790 // syntactic sugar 1791 public AddedItemComponent addAdjudication(AddedItemAdjudicationComponent t) { // 3 1792 if (t == null) 1793 return this; 1794 if (this.adjudication == null) 1795 this.adjudication = new ArrayList<AddedItemAdjudicationComponent>(); 1796 this.adjudication.add(t); 1797 return this; 1798 } 1799 1800 /** 1801 * @return {@link #detail} (The second tier service adjudications for payor 1802 * added services.) 1803 */ 1804 public List<AddedItemsDetailComponent> getDetail() { 1805 if (this.detail == null) 1806 this.detail = new ArrayList<AddedItemsDetailComponent>(); 1807 return this.detail; 1808 } 1809 1810 public boolean hasDetail() { 1811 if (this.detail == null) 1812 return false; 1813 for (AddedItemsDetailComponent item : this.detail) 1814 if (!item.isEmpty()) 1815 return true; 1816 return false; 1817 } 1818 1819 /** 1820 * @return {@link #detail} (The second tier service adjudications for payor 1821 * added services.) 1822 */ 1823 // syntactic sugar 1824 public AddedItemsDetailComponent addDetail() { // 3 1825 AddedItemsDetailComponent t = new AddedItemsDetailComponent(); 1826 if (this.detail == null) 1827 this.detail = new ArrayList<AddedItemsDetailComponent>(); 1828 this.detail.add(t); 1829 return t; 1830 } 1831 1832 // syntactic sugar 1833 public AddedItemComponent addDetail(AddedItemsDetailComponent t) { // 3 1834 if (t == null) 1835 return this; 1836 if (this.detail == null) 1837 this.detail = new ArrayList<AddedItemsDetailComponent>(); 1838 this.detail.add(t); 1839 return this; 1840 } 1841 1842 protected void listChildren(List<Property> childrenList) { 1843 super.listChildren(childrenList); 1844 childrenList.add(new Property("sequenceLinkId", "positiveInt", 1845 "List of input service items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, 1846 sequenceLinkId)); 1847 childrenList.add(new Property("service", "Coding", 1848 "A code to indicate the Professional Service or Product supplied.", 0, java.lang.Integer.MAX_VALUE, service)); 1849 childrenList.add(new Property("fee", "Money", "The fee charged for the professional service or product..", 0, 1850 java.lang.Integer.MAX_VALUE, fee)); 1851 childrenList.add(new Property("noteNumberLinkId", "positiveInt", 1852 "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumberLinkId)); 1853 childrenList.add( 1854 new Property("adjudication", "", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 1855 childrenList.add(new Property("detail", "", "The second tier service adjudications for payor added services.", 0, 1856 java.lang.Integer.MAX_VALUE, detail)); 1857 } 1858 1859 @Override 1860 public void setProperty(String name, Base value) throws FHIRException { 1861 if (name.equals("sequenceLinkId")) 1862 this.getSequenceLinkId().add(castToPositiveInt(value)); 1863 else if (name.equals("service")) 1864 this.service = castToCoding(value); // Coding 1865 else if (name.equals("fee")) 1866 this.fee = castToMoney(value); // Money 1867 else if (name.equals("noteNumberLinkId")) 1868 this.getNoteNumberLinkId().add(castToPositiveInt(value)); 1869 else if (name.equals("adjudication")) 1870 this.getAdjudication().add((AddedItemAdjudicationComponent) value); 1871 else if (name.equals("detail")) 1872 this.getDetail().add((AddedItemsDetailComponent) value); 1873 else 1874 super.setProperty(name, value); 1875 } 1876 1877 @Override 1878 public Base addChild(String name) throws FHIRException { 1879 if (name.equals("sequenceLinkId")) { 1880 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.sequenceLinkId"); 1881 } else if (name.equals("service")) { 1882 this.service = new Coding(); 1883 return this.service; 1884 } else if (name.equals("fee")) { 1885 this.fee = new Money(); 1886 return this.fee; 1887 } else if (name.equals("noteNumberLinkId")) { 1888 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.noteNumberLinkId"); 1889 } else if (name.equals("adjudication")) { 1890 return addAdjudication(); 1891 } else if (name.equals("detail")) { 1892 return addDetail(); 1893 } else 1894 return super.addChild(name); 1895 } 1896 1897 public AddedItemComponent copy() { 1898 AddedItemComponent dst = new AddedItemComponent(); 1899 copyValues(dst); 1900 if (sequenceLinkId != null) { 1901 dst.sequenceLinkId = new ArrayList<PositiveIntType>(); 1902 for (PositiveIntType i : sequenceLinkId) 1903 dst.sequenceLinkId.add(i.copy()); 1904 } 1905 ; 1906 dst.service = service == null ? null : service.copy(); 1907 dst.fee = fee == null ? null : fee.copy(); 1908 if (noteNumberLinkId != null) { 1909 dst.noteNumberLinkId = new ArrayList<PositiveIntType>(); 1910 for (PositiveIntType i : noteNumberLinkId) 1911 dst.noteNumberLinkId.add(i.copy()); 1912 } 1913 ; 1914 if (adjudication != null) { 1915 dst.adjudication = new ArrayList<AddedItemAdjudicationComponent>(); 1916 for (AddedItemAdjudicationComponent i : adjudication) 1917 dst.adjudication.add(i.copy()); 1918 } 1919 ; 1920 if (detail != null) { 1921 dst.detail = new ArrayList<AddedItemsDetailComponent>(); 1922 for (AddedItemsDetailComponent i : detail) 1923 dst.detail.add(i.copy()); 1924 } 1925 ; 1926 return dst; 1927 } 1928 1929 @Override 1930 public boolean equalsDeep(Base other) { 1931 if (!super.equalsDeep(other)) 1932 return false; 1933 if (!(other instanceof AddedItemComponent)) 1934 return false; 1935 AddedItemComponent o = (AddedItemComponent) other; 1936 return compareDeep(sequenceLinkId, o.sequenceLinkId, true) && compareDeep(service, o.service, true) 1937 && compareDeep(fee, o.fee, true) && compareDeep(noteNumberLinkId, o.noteNumberLinkId, true) 1938 && compareDeep(adjudication, o.adjudication, true) && compareDeep(detail, o.detail, true); 1939 } 1940 1941 @Override 1942 public boolean equalsShallow(Base other) { 1943 if (!super.equalsShallow(other)) 1944 return false; 1945 if (!(other instanceof AddedItemComponent)) 1946 return false; 1947 AddedItemComponent o = (AddedItemComponent) other; 1948 return compareValues(sequenceLinkId, o.sequenceLinkId, true) 1949 && compareValues(noteNumberLinkId, o.noteNumberLinkId, true); 1950 } 1951 1952 public boolean isEmpty() { 1953 return super.isEmpty() && (sequenceLinkId == null || sequenceLinkId.isEmpty()) 1954 && (service == null || service.isEmpty()) && (fee == null || fee.isEmpty()) 1955 && (noteNumberLinkId == null || noteNumberLinkId.isEmpty()) 1956 && (adjudication == null || adjudication.isEmpty()) && (detail == null || detail.isEmpty()); 1957 } 1958 1959 public String fhirType() { 1960 return "ClaimResponse.addItem"; 1961 1962 } 1963 1964 } 1965 1966 @Block() 1967 public static class AddedItemAdjudicationComponent extends BackboneElement implements IBaseBackboneElement { 1968 /** 1969 * Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc. 1970 */ 1971 @Child(name = "code", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 1972 @Description(shortDefinition = "Adjudication category such as co-pay, eligible, benefit, etc.", formalDefinition = "Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc.") 1973 protected Coding code; 1974 1975 /** 1976 * Monetary amount associated with the code. 1977 */ 1978 @Child(name = "amount", type = { Money.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 1979 @Description(shortDefinition = "Monetary amount", formalDefinition = "Monetary amount associated with the code.") 1980 protected Money amount; 1981 1982 /** 1983 * A non-monetary value for example a percentage. Mutually exclusive to the 1984 * amount element above. 1985 */ 1986 @Child(name = "value", type = { DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 1987 @Description(shortDefinition = "Non-monetary value", formalDefinition = "A non-monetary value for example a percentage. Mutually exclusive to the amount element above.") 1988 protected DecimalType value; 1989 1990 private static final long serialVersionUID = -949880587L; 1991 1992 /* 1993 * Constructor 1994 */ 1995 public AddedItemAdjudicationComponent() { 1996 super(); 1997 } 1998 1999 /* 2000 * Constructor 2001 */ 2002 public AddedItemAdjudicationComponent(Coding code) { 2003 super(); 2004 this.code = code; 2005 } 2006 2007 /** 2008 * @return {@link #code} (Code indicating: Co-Pay, deductible, eligible, 2009 * benefit, tax, etc.) 2010 */ 2011 public Coding getCode() { 2012 if (this.code == null) 2013 if (Configuration.errorOnAutoCreate()) 2014 throw new Error("Attempt to auto-create AddedItemAdjudicationComponent.code"); 2015 else if (Configuration.doAutoCreate()) 2016 this.code = new Coding(); // cc 2017 return this.code; 2018 } 2019 2020 public boolean hasCode() { 2021 return this.code != null && !this.code.isEmpty(); 2022 } 2023 2024 /** 2025 * @param value {@link #code} (Code indicating: Co-Pay, deductible, eligible, 2026 * benefit, tax, etc.) 2027 */ 2028 public AddedItemAdjudicationComponent setCode(Coding value) { 2029 this.code = value; 2030 return this; 2031 } 2032 2033 /** 2034 * @return {@link #amount} (Monetary amount associated with the code.) 2035 */ 2036 public Money getAmount() { 2037 if (this.amount == null) 2038 if (Configuration.errorOnAutoCreate()) 2039 throw new Error("Attempt to auto-create AddedItemAdjudicationComponent.amount"); 2040 else if (Configuration.doAutoCreate()) 2041 this.amount = new Money(); // cc 2042 return this.amount; 2043 } 2044 2045 public boolean hasAmount() { 2046 return this.amount != null && !this.amount.isEmpty(); 2047 } 2048 2049 /** 2050 * @param value {@link #amount} (Monetary amount associated with the code.) 2051 */ 2052 public AddedItemAdjudicationComponent setAmount(Money value) { 2053 this.amount = value; 2054 return this; 2055 } 2056 2057 /** 2058 * @return {@link #value} (A non-monetary value for example a percentage. 2059 * Mutually exclusive to the amount element above.). This is the 2060 * underlying object with id, value and extensions. The accessor 2061 * "getValue" gives direct access to the value 2062 */ 2063 public DecimalType getValueElement() { 2064 if (this.value == null) 2065 if (Configuration.errorOnAutoCreate()) 2066 throw new Error("Attempt to auto-create AddedItemAdjudicationComponent.value"); 2067 else if (Configuration.doAutoCreate()) 2068 this.value = new DecimalType(); // bb 2069 return this.value; 2070 } 2071 2072 public boolean hasValueElement() { 2073 return this.value != null && !this.value.isEmpty(); 2074 } 2075 2076 public boolean hasValue() { 2077 return this.value != null && !this.value.isEmpty(); 2078 } 2079 2080 /** 2081 * @param value {@link #value} (A non-monetary value for example a percentage. 2082 * Mutually exclusive to the amount element above.). This is the 2083 * underlying object with id, value and extensions. The accessor 2084 * "getValue" gives direct access to the value 2085 */ 2086 public AddedItemAdjudicationComponent setValueElement(DecimalType value) { 2087 this.value = value; 2088 return this; 2089 } 2090 2091 /** 2092 * @return A non-monetary value for example a percentage. Mutually exclusive to 2093 * the amount element above. 2094 */ 2095 public BigDecimal getValue() { 2096 return this.value == null ? null : this.value.getValue(); 2097 } 2098 2099 /** 2100 * @param value A non-monetary value for example a percentage. Mutually 2101 * exclusive to the amount element above. 2102 */ 2103 public AddedItemAdjudicationComponent setValue(BigDecimal value) { 2104 if (value == null) 2105 this.value = null; 2106 else { 2107 if (this.value == null) 2108 this.value = new DecimalType(); 2109 this.value.setValue(value); 2110 } 2111 return this; 2112 } 2113 2114 protected void listChildren(List<Property> childrenList) { 2115 super.listChildren(childrenList); 2116 childrenList.add(new Property("code", "Coding", 2117 "Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc.", 0, java.lang.Integer.MAX_VALUE, code)); 2118 childrenList.add(new Property("amount", "Money", "Monetary amount associated with the code.", 0, 2119 java.lang.Integer.MAX_VALUE, amount)); 2120 childrenList.add(new Property("value", "decimal", 2121 "A non-monetary value for example a percentage. Mutually exclusive to the amount element above.", 0, 2122 java.lang.Integer.MAX_VALUE, value)); 2123 } 2124 2125 @Override 2126 public void setProperty(String name, Base value) throws FHIRException { 2127 if (name.equals("code")) 2128 this.code = castToCoding(value); // Coding 2129 else if (name.equals("amount")) 2130 this.amount = castToMoney(value); // Money 2131 else if (name.equals("value")) 2132 this.value = castToDecimal(value); // DecimalType 2133 else 2134 super.setProperty(name, value); 2135 } 2136 2137 @Override 2138 public Base addChild(String name) throws FHIRException { 2139 if (name.equals("code")) { 2140 this.code = new Coding(); 2141 return this.code; 2142 } else if (name.equals("amount")) { 2143 this.amount = new Money(); 2144 return this.amount; 2145 } else if (name.equals("value")) { 2146 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.value"); 2147 } else 2148 return super.addChild(name); 2149 } 2150 2151 public AddedItemAdjudicationComponent copy() { 2152 AddedItemAdjudicationComponent dst = new AddedItemAdjudicationComponent(); 2153 copyValues(dst); 2154 dst.code = code == null ? null : code.copy(); 2155 dst.amount = amount == null ? null : amount.copy(); 2156 dst.value = value == null ? null : value.copy(); 2157 return dst; 2158 } 2159 2160 @Override 2161 public boolean equalsDeep(Base other) { 2162 if (!super.equalsDeep(other)) 2163 return false; 2164 if (!(other instanceof AddedItemAdjudicationComponent)) 2165 return false; 2166 AddedItemAdjudicationComponent o = (AddedItemAdjudicationComponent) other; 2167 return compareDeep(code, o.code, true) && compareDeep(amount, o.amount, true) 2168 && compareDeep(value, o.value, true); 2169 } 2170 2171 @Override 2172 public boolean equalsShallow(Base other) { 2173 if (!super.equalsShallow(other)) 2174 return false; 2175 if (!(other instanceof AddedItemAdjudicationComponent)) 2176 return false; 2177 AddedItemAdjudicationComponent o = (AddedItemAdjudicationComponent) other; 2178 return compareValues(value, o.value, true); 2179 } 2180 2181 public boolean isEmpty() { 2182 return super.isEmpty() && (code == null || code.isEmpty()) && (amount == null || amount.isEmpty()) 2183 && (value == null || value.isEmpty()); 2184 } 2185 2186 public String fhirType() { 2187 return "ClaimResponse.addItem.adjudication"; 2188 2189 } 2190 2191 } 2192 2193 @Block() 2194 public static class AddedItemsDetailComponent extends BackboneElement implements IBaseBackboneElement { 2195 /** 2196 * A code to indicate the Professional Service or Product supplied. 2197 */ 2198 @Child(name = "service", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2199 @Description(shortDefinition = "Service or Product", formalDefinition = "A code to indicate the Professional Service or Product supplied.") 2200 protected Coding service; 2201 2202 /** 2203 * The fee charged for the professional service or product.. 2204 */ 2205 @Child(name = "fee", type = { Money.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2206 @Description(shortDefinition = "Professional fee or Product charge", formalDefinition = "The fee charged for the professional service or product..") 2207 protected Money fee; 2208 2209 /** 2210 * The adjudications results. 2211 */ 2212 @Child(name = "adjudication", type = {}, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 2213 @Description(shortDefinition = "Added items detail adjudication", formalDefinition = "The adjudications results.") 2214 protected List<AddedItemDetailAdjudicationComponent> adjudication; 2215 2216 private static final long serialVersionUID = -2104242020L; 2217 2218 /* 2219 * Constructor 2220 */ 2221 public AddedItemsDetailComponent() { 2222 super(); 2223 } 2224 2225 /* 2226 * Constructor 2227 */ 2228 public AddedItemsDetailComponent(Coding service) { 2229 super(); 2230 this.service = service; 2231 } 2232 2233 /** 2234 * @return {@link #service} (A code to indicate the Professional Service or 2235 * Product supplied.) 2236 */ 2237 public Coding getService() { 2238 if (this.service == null) 2239 if (Configuration.errorOnAutoCreate()) 2240 throw new Error("Attempt to auto-create AddedItemsDetailComponent.service"); 2241 else if (Configuration.doAutoCreate()) 2242 this.service = new Coding(); // cc 2243 return this.service; 2244 } 2245 2246 public boolean hasService() { 2247 return this.service != null && !this.service.isEmpty(); 2248 } 2249 2250 /** 2251 * @param value {@link #service} (A code to indicate the Professional Service or 2252 * Product supplied.) 2253 */ 2254 public AddedItemsDetailComponent setService(Coding value) { 2255 this.service = value; 2256 return this; 2257 } 2258 2259 /** 2260 * @return {@link #fee} (The fee charged for the professional service or 2261 * product..) 2262 */ 2263 public Money getFee() { 2264 if (this.fee == null) 2265 if (Configuration.errorOnAutoCreate()) 2266 throw new Error("Attempt to auto-create AddedItemsDetailComponent.fee"); 2267 else if (Configuration.doAutoCreate()) 2268 this.fee = new Money(); // cc 2269 return this.fee; 2270 } 2271 2272 public boolean hasFee() { 2273 return this.fee != null && !this.fee.isEmpty(); 2274 } 2275 2276 /** 2277 * @param value {@link #fee} (The fee charged for the professional service or 2278 * product..) 2279 */ 2280 public AddedItemsDetailComponent setFee(Money value) { 2281 this.fee = value; 2282 return this; 2283 } 2284 2285 /** 2286 * @return {@link #adjudication} (The adjudications results.) 2287 */ 2288 public List<AddedItemDetailAdjudicationComponent> getAdjudication() { 2289 if (this.adjudication == null) 2290 this.adjudication = new ArrayList<AddedItemDetailAdjudicationComponent>(); 2291 return this.adjudication; 2292 } 2293 2294 public boolean hasAdjudication() { 2295 if (this.adjudication == null) 2296 return false; 2297 for (AddedItemDetailAdjudicationComponent item : this.adjudication) 2298 if (!item.isEmpty()) 2299 return true; 2300 return false; 2301 } 2302 2303 /** 2304 * @return {@link #adjudication} (The adjudications results.) 2305 */ 2306 // syntactic sugar 2307 public AddedItemDetailAdjudicationComponent addAdjudication() { // 3 2308 AddedItemDetailAdjudicationComponent t = new AddedItemDetailAdjudicationComponent(); 2309 if (this.adjudication == null) 2310 this.adjudication = new ArrayList<AddedItemDetailAdjudicationComponent>(); 2311 this.adjudication.add(t); 2312 return t; 2313 } 2314 2315 // syntactic sugar 2316 public AddedItemsDetailComponent addAdjudication(AddedItemDetailAdjudicationComponent t) { // 3 2317 if (t == null) 2318 return this; 2319 if (this.adjudication == null) 2320 this.adjudication = new ArrayList<AddedItemDetailAdjudicationComponent>(); 2321 this.adjudication.add(t); 2322 return this; 2323 } 2324 2325 protected void listChildren(List<Property> childrenList) { 2326 super.listChildren(childrenList); 2327 childrenList.add(new Property("service", "Coding", 2328 "A code to indicate the Professional Service or Product supplied.", 0, java.lang.Integer.MAX_VALUE, service)); 2329 childrenList.add(new Property("fee", "Money", "The fee charged for the professional service or product..", 0, 2330 java.lang.Integer.MAX_VALUE, fee)); 2331 childrenList.add( 2332 new Property("adjudication", "", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 2333 } 2334 2335 @Override 2336 public void setProperty(String name, Base value) throws FHIRException { 2337 if (name.equals("service")) 2338 this.service = castToCoding(value); // Coding 2339 else if (name.equals("fee")) 2340 this.fee = castToMoney(value); // Money 2341 else if (name.equals("adjudication")) 2342 this.getAdjudication().add((AddedItemDetailAdjudicationComponent) value); 2343 else 2344 super.setProperty(name, value); 2345 } 2346 2347 @Override 2348 public Base addChild(String name) throws FHIRException { 2349 if (name.equals("service")) { 2350 this.service = new Coding(); 2351 return this.service; 2352 } else if (name.equals("fee")) { 2353 this.fee = new Money(); 2354 return this.fee; 2355 } else if (name.equals("adjudication")) { 2356 return addAdjudication(); 2357 } else 2358 return super.addChild(name); 2359 } 2360 2361 public AddedItemsDetailComponent copy() { 2362 AddedItemsDetailComponent dst = new AddedItemsDetailComponent(); 2363 copyValues(dst); 2364 dst.service = service == null ? null : service.copy(); 2365 dst.fee = fee == null ? null : fee.copy(); 2366 if (adjudication != null) { 2367 dst.adjudication = new ArrayList<AddedItemDetailAdjudicationComponent>(); 2368 for (AddedItemDetailAdjudicationComponent i : adjudication) 2369 dst.adjudication.add(i.copy()); 2370 } 2371 ; 2372 return dst; 2373 } 2374 2375 @Override 2376 public boolean equalsDeep(Base other) { 2377 if (!super.equalsDeep(other)) 2378 return false; 2379 if (!(other instanceof AddedItemsDetailComponent)) 2380 return false; 2381 AddedItemsDetailComponent o = (AddedItemsDetailComponent) other; 2382 return compareDeep(service, o.service, true) && compareDeep(fee, o.fee, true) 2383 && compareDeep(adjudication, o.adjudication, true); 2384 } 2385 2386 @Override 2387 public boolean equalsShallow(Base other) { 2388 if (!super.equalsShallow(other)) 2389 return false; 2390 if (!(other instanceof AddedItemsDetailComponent)) 2391 return false; 2392 AddedItemsDetailComponent o = (AddedItemsDetailComponent) other; 2393 return true; 2394 } 2395 2396 public boolean isEmpty() { 2397 return super.isEmpty() && (service == null || service.isEmpty()) && (fee == null || fee.isEmpty()) 2398 && (adjudication == null || adjudication.isEmpty()); 2399 } 2400 2401 public String fhirType() { 2402 return "ClaimResponse.addItem.detail"; 2403 2404 } 2405 2406 } 2407 2408 @Block() 2409 public static class AddedItemDetailAdjudicationComponent extends BackboneElement implements IBaseBackboneElement { 2410 /** 2411 * Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc. 2412 */ 2413 @Child(name = "code", type = { Coding.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 2414 @Description(shortDefinition = "Adjudication category such as co-pay, eligible, benefit, etc.", formalDefinition = "Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc.") 2415 protected Coding code; 2416 2417 /** 2418 * Monetary amount associated with the code. 2419 */ 2420 @Child(name = "amount", type = { Money.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2421 @Description(shortDefinition = "Monetary amount", formalDefinition = "Monetary amount associated with the code.") 2422 protected Money amount; 2423 2424 /** 2425 * A non-monetary value for example a percentage. Mutually exclusive to the 2426 * amount element above. 2427 */ 2428 @Child(name = "value", type = { DecimalType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2429 @Description(shortDefinition = "Non-monetary value", formalDefinition = "A non-monetary value for example a percentage. Mutually exclusive to the amount element above.") 2430 protected DecimalType value; 2431 2432 private static final long serialVersionUID = -949880587L; 2433 2434 /* 2435 * Constructor 2436 */ 2437 public AddedItemDetailAdjudicationComponent() { 2438 super(); 2439 } 2440 2441 /* 2442 * Constructor 2443 */ 2444 public AddedItemDetailAdjudicationComponent(Coding code) { 2445 super(); 2446 this.code = code; 2447 } 2448 2449 /** 2450 * @return {@link #code} (Code indicating: Co-Pay, deductible, eligible, 2451 * benefit, tax, etc.) 2452 */ 2453 public Coding getCode() { 2454 if (this.code == null) 2455 if (Configuration.errorOnAutoCreate()) 2456 throw new Error("Attempt to auto-create AddedItemDetailAdjudicationComponent.code"); 2457 else if (Configuration.doAutoCreate()) 2458 this.code = new Coding(); // cc 2459 return this.code; 2460 } 2461 2462 public boolean hasCode() { 2463 return this.code != null && !this.code.isEmpty(); 2464 } 2465 2466 /** 2467 * @param value {@link #code} (Code indicating: Co-Pay, deductible, eligible, 2468 * benefit, tax, etc.) 2469 */ 2470 public AddedItemDetailAdjudicationComponent setCode(Coding value) { 2471 this.code = value; 2472 return this; 2473 } 2474 2475 /** 2476 * @return {@link #amount} (Monetary amount associated with the code.) 2477 */ 2478 public Money getAmount() { 2479 if (this.amount == null) 2480 if (Configuration.errorOnAutoCreate()) 2481 throw new Error("Attempt to auto-create AddedItemDetailAdjudicationComponent.amount"); 2482 else if (Configuration.doAutoCreate()) 2483 this.amount = new Money(); // cc 2484 return this.amount; 2485 } 2486 2487 public boolean hasAmount() { 2488 return this.amount != null && !this.amount.isEmpty(); 2489 } 2490 2491 /** 2492 * @param value {@link #amount} (Monetary amount associated with the code.) 2493 */ 2494 public AddedItemDetailAdjudicationComponent setAmount(Money value) { 2495 this.amount = value; 2496 return this; 2497 } 2498 2499 /** 2500 * @return {@link #value} (A non-monetary value for example a percentage. 2501 * Mutually exclusive to the amount element above.). This is the 2502 * underlying object with id, value and extensions. The accessor 2503 * "getValue" gives direct access to the value 2504 */ 2505 public DecimalType getValueElement() { 2506 if (this.value == null) 2507 if (Configuration.errorOnAutoCreate()) 2508 throw new Error("Attempt to auto-create AddedItemDetailAdjudicationComponent.value"); 2509 else if (Configuration.doAutoCreate()) 2510 this.value = new DecimalType(); // bb 2511 return this.value; 2512 } 2513 2514 public boolean hasValueElement() { 2515 return this.value != null && !this.value.isEmpty(); 2516 } 2517 2518 public boolean hasValue() { 2519 return this.value != null && !this.value.isEmpty(); 2520 } 2521 2522 /** 2523 * @param value {@link #value} (A non-monetary value for example a percentage. 2524 * Mutually exclusive to the amount element above.). This is the 2525 * underlying object with id, value and extensions. The accessor 2526 * "getValue" gives direct access to the value 2527 */ 2528 public AddedItemDetailAdjudicationComponent setValueElement(DecimalType value) { 2529 this.value = value; 2530 return this; 2531 } 2532 2533 /** 2534 * @return A non-monetary value for example a percentage. Mutually exclusive to 2535 * the amount element above. 2536 */ 2537 public BigDecimal getValue() { 2538 return this.value == null ? null : this.value.getValue(); 2539 } 2540 2541 /** 2542 * @param value A non-monetary value for example a percentage. Mutually 2543 * exclusive to the amount element above. 2544 */ 2545 public AddedItemDetailAdjudicationComponent setValue(BigDecimal value) { 2546 if (value == null) 2547 this.value = null; 2548 else { 2549 if (this.value == null) 2550 this.value = new DecimalType(); 2551 this.value.setValue(value); 2552 } 2553 return this; 2554 } 2555 2556 protected void listChildren(List<Property> childrenList) { 2557 super.listChildren(childrenList); 2558 childrenList.add(new Property("code", "Coding", 2559 "Code indicating: Co-Pay, deductible, eligible, benefit, tax, etc.", 0, java.lang.Integer.MAX_VALUE, code)); 2560 childrenList.add(new Property("amount", "Money", "Monetary amount associated with the code.", 0, 2561 java.lang.Integer.MAX_VALUE, amount)); 2562 childrenList.add(new Property("value", "decimal", 2563 "A non-monetary value for example a percentage. Mutually exclusive to the amount element above.", 0, 2564 java.lang.Integer.MAX_VALUE, value)); 2565 } 2566 2567 @Override 2568 public void setProperty(String name, Base value) throws FHIRException { 2569 if (name.equals("code")) 2570 this.code = castToCoding(value); // Coding 2571 else if (name.equals("amount")) 2572 this.amount = castToMoney(value); // Money 2573 else if (name.equals("value")) 2574 this.value = castToDecimal(value); // DecimalType 2575 else 2576 super.setProperty(name, value); 2577 } 2578 2579 @Override 2580 public Base addChild(String name) throws FHIRException { 2581 if (name.equals("code")) { 2582 this.code = new Coding(); 2583 return this.code; 2584 } else if (name.equals("amount")) { 2585 this.amount = new Money(); 2586 return this.amount; 2587 } else if (name.equals("value")) { 2588 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.value"); 2589 } else 2590 return super.addChild(name); 2591 } 2592 2593 public AddedItemDetailAdjudicationComponent copy() { 2594 AddedItemDetailAdjudicationComponent dst = new AddedItemDetailAdjudicationComponent(); 2595 copyValues(dst); 2596 dst.code = code == null ? null : code.copy(); 2597 dst.amount = amount == null ? null : amount.copy(); 2598 dst.value = value == null ? null : value.copy(); 2599 return dst; 2600 } 2601 2602 @Override 2603 public boolean equalsDeep(Base other) { 2604 if (!super.equalsDeep(other)) 2605 return false; 2606 if (!(other instanceof AddedItemDetailAdjudicationComponent)) 2607 return false; 2608 AddedItemDetailAdjudicationComponent o = (AddedItemDetailAdjudicationComponent) other; 2609 return compareDeep(code, o.code, true) && compareDeep(amount, o.amount, true) 2610 && compareDeep(value, o.value, true); 2611 } 2612 2613 @Override 2614 public boolean equalsShallow(Base other) { 2615 if (!super.equalsShallow(other)) 2616 return false; 2617 if (!(other instanceof AddedItemDetailAdjudicationComponent)) 2618 return false; 2619 AddedItemDetailAdjudicationComponent o = (AddedItemDetailAdjudicationComponent) other; 2620 return compareValues(value, o.value, true); 2621 } 2622 2623 public boolean isEmpty() { 2624 return super.isEmpty() && (code == null || code.isEmpty()) && (amount == null || amount.isEmpty()) 2625 && (value == null || value.isEmpty()); 2626 } 2627 2628 public String fhirType() { 2629 return "ClaimResponse.addItem.detail.adjudication"; 2630 2631 } 2632 2633 } 2634 2635 @Block() 2636 public static class ErrorsComponent extends BackboneElement implements IBaseBackboneElement { 2637 /** 2638 * The sequence number of the line item submitted which contains the error. This 2639 * value is omitted when the error is elsewhere. 2640 */ 2641 @Child(name = "sequenceLinkId", type = { 2642 PositiveIntType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2643 @Description(shortDefinition = "Item sequence number", formalDefinition = "The sequence number of the line item submitted which contains the error. This value is omitted when the error is elsewhere.") 2644 protected PositiveIntType sequenceLinkId; 2645 2646 /** 2647 * The sequence number of the addition within the line item submitted which 2648 * contains the error. This value is omitted when the error is not related to an 2649 * Addition. 2650 */ 2651 @Child(name = "detailSequenceLinkId", type = { 2652 PositiveIntType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2653 @Description(shortDefinition = "Detail sequence number", formalDefinition = "The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition.") 2654 protected PositiveIntType detailSequenceLinkId; 2655 2656 /** 2657 * The sequence number of the addition within the line item submitted which 2658 * contains the error. This value is omitted when the error is not related to an 2659 * Addition. 2660 */ 2661 @Child(name = "subdetailSequenceLinkId", type = { 2662 PositiveIntType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 2663 @Description(shortDefinition = "Subdetail sequence number", formalDefinition = "The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition.") 2664 protected PositiveIntType subdetailSequenceLinkId; 2665 2666 /** 2667 * An error code,froma specified code system, which details why the claim could 2668 * not be adjudicated. 2669 */ 2670 @Child(name = "code", type = { Coding.class }, order = 4, min = 1, max = 1, modifier = false, summary = true) 2671 @Description(shortDefinition = "Error code detailing processing issues", formalDefinition = "An error code,froma specified code system, which details why the claim could not be adjudicated.") 2672 protected Coding code; 2673 2674 private static final long serialVersionUID = -1893641175L; 2675 2676 /* 2677 * Constructor 2678 */ 2679 public ErrorsComponent() { 2680 super(); 2681 } 2682 2683 /* 2684 * Constructor 2685 */ 2686 public ErrorsComponent(Coding code) { 2687 super(); 2688 this.code = code; 2689 } 2690 2691 /** 2692 * @return {@link #sequenceLinkId} (The sequence number of the line item 2693 * submitted which contains the error. This value is omitted when the 2694 * error is elsewhere.). This is the underlying object with id, value 2695 * and extensions. The accessor "getSequenceLinkId" gives direct access 2696 * to the value 2697 */ 2698 public PositiveIntType getSequenceLinkIdElement() { 2699 if (this.sequenceLinkId == null) 2700 if (Configuration.errorOnAutoCreate()) 2701 throw new Error("Attempt to auto-create ErrorsComponent.sequenceLinkId"); 2702 else if (Configuration.doAutoCreate()) 2703 this.sequenceLinkId = new PositiveIntType(); // bb 2704 return this.sequenceLinkId; 2705 } 2706 2707 public boolean hasSequenceLinkIdElement() { 2708 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 2709 } 2710 2711 public boolean hasSequenceLinkId() { 2712 return this.sequenceLinkId != null && !this.sequenceLinkId.isEmpty(); 2713 } 2714 2715 /** 2716 * @param value {@link #sequenceLinkId} (The sequence number of the line item 2717 * submitted which contains the error. This value is omitted when 2718 * the error is elsewhere.). This is the underlying object with id, 2719 * value and extensions. The accessor "getSequenceLinkId" gives 2720 * direct access to the value 2721 */ 2722 public ErrorsComponent setSequenceLinkIdElement(PositiveIntType value) { 2723 this.sequenceLinkId = value; 2724 return this; 2725 } 2726 2727 /** 2728 * @return The sequence number of the line item submitted which contains the 2729 * error. This value is omitted when the error is elsewhere. 2730 */ 2731 public int getSequenceLinkId() { 2732 return this.sequenceLinkId == null || this.sequenceLinkId.isEmpty() ? 0 : this.sequenceLinkId.getValue(); 2733 } 2734 2735 /** 2736 * @param value The sequence number of the line item submitted which contains 2737 * the error. This value is omitted when the error is elsewhere. 2738 */ 2739 public ErrorsComponent setSequenceLinkId(int value) { 2740 if (this.sequenceLinkId == null) 2741 this.sequenceLinkId = new PositiveIntType(); 2742 this.sequenceLinkId.setValue(value); 2743 return this; 2744 } 2745 2746 /** 2747 * @return {@link #detailSequenceLinkId} (The sequence number of the addition 2748 * within the line item submitted which contains the error. This value 2749 * is omitted when the error is not related to an Addition.). This is 2750 * the underlying object with id, value and extensions. The accessor 2751 * "getDetailSequenceLinkId" gives direct access to the value 2752 */ 2753 public PositiveIntType getDetailSequenceLinkIdElement() { 2754 if (this.detailSequenceLinkId == null) 2755 if (Configuration.errorOnAutoCreate()) 2756 throw new Error("Attempt to auto-create ErrorsComponent.detailSequenceLinkId"); 2757 else if (Configuration.doAutoCreate()) 2758 this.detailSequenceLinkId = new PositiveIntType(); // bb 2759 return this.detailSequenceLinkId; 2760 } 2761 2762 public boolean hasDetailSequenceLinkIdElement() { 2763 return this.detailSequenceLinkId != null && !this.detailSequenceLinkId.isEmpty(); 2764 } 2765 2766 public boolean hasDetailSequenceLinkId() { 2767 return this.detailSequenceLinkId != null && !this.detailSequenceLinkId.isEmpty(); 2768 } 2769 2770 /** 2771 * @param value {@link #detailSequenceLinkId} (The sequence number of the 2772 * addition within the line item submitted which contains the 2773 * error. This value is omitted when the error is not related to an 2774 * Addition.). This is the underlying object with id, value and 2775 * extensions. The accessor "getDetailSequenceLinkId" gives direct 2776 * access to the value 2777 */ 2778 public ErrorsComponent setDetailSequenceLinkIdElement(PositiveIntType value) { 2779 this.detailSequenceLinkId = value; 2780 return this; 2781 } 2782 2783 /** 2784 * @return The sequence number of the addition within the line item submitted 2785 * which contains the error. This value is omitted when the error is not 2786 * related to an Addition. 2787 */ 2788 public int getDetailSequenceLinkId() { 2789 return this.detailSequenceLinkId == null || this.detailSequenceLinkId.isEmpty() ? 0 2790 : this.detailSequenceLinkId.getValue(); 2791 } 2792 2793 /** 2794 * @param value The sequence number of the addition within the line item 2795 * submitted which contains the error. This value is omitted when 2796 * the error is not related to an Addition. 2797 */ 2798 public ErrorsComponent setDetailSequenceLinkId(int value) { 2799 if (this.detailSequenceLinkId == null) 2800 this.detailSequenceLinkId = new PositiveIntType(); 2801 this.detailSequenceLinkId.setValue(value); 2802 return this; 2803 } 2804 2805 /** 2806 * @return {@link #subdetailSequenceLinkId} (The sequence number of the addition 2807 * within the line item submitted which contains the error. This value 2808 * is omitted when the error is not related to an Addition.). This is 2809 * the underlying object with id, value and extensions. The accessor 2810 * "getSubdetailSequenceLinkId" gives direct access to the value 2811 */ 2812 public PositiveIntType getSubdetailSequenceLinkIdElement() { 2813 if (this.subdetailSequenceLinkId == null) 2814 if (Configuration.errorOnAutoCreate()) 2815 throw new Error("Attempt to auto-create ErrorsComponent.subdetailSequenceLinkId"); 2816 else if (Configuration.doAutoCreate()) 2817 this.subdetailSequenceLinkId = new PositiveIntType(); // bb 2818 return this.subdetailSequenceLinkId; 2819 } 2820 2821 public boolean hasSubdetailSequenceLinkIdElement() { 2822 return this.subdetailSequenceLinkId != null && !this.subdetailSequenceLinkId.isEmpty(); 2823 } 2824 2825 public boolean hasSubdetailSequenceLinkId() { 2826 return this.subdetailSequenceLinkId != null && !this.subdetailSequenceLinkId.isEmpty(); 2827 } 2828 2829 /** 2830 * @param value {@link #subdetailSequenceLinkId} (The sequence number of the 2831 * addition within the line item submitted which contains the 2832 * error. This value is omitted when the error is not related to an 2833 * Addition.). This is the underlying object with id, value and 2834 * extensions. The accessor "getSubdetailSequenceLinkId" gives 2835 * direct access to the value 2836 */ 2837 public ErrorsComponent setSubdetailSequenceLinkIdElement(PositiveIntType value) { 2838 this.subdetailSequenceLinkId = value; 2839 return this; 2840 } 2841 2842 /** 2843 * @return The sequence number of the addition within the line item submitted 2844 * which contains the error. This value is omitted when the error is not 2845 * related to an Addition. 2846 */ 2847 public int getSubdetailSequenceLinkId() { 2848 return this.subdetailSequenceLinkId == null || this.subdetailSequenceLinkId.isEmpty() ? 0 2849 : this.subdetailSequenceLinkId.getValue(); 2850 } 2851 2852 /** 2853 * @param value The sequence number of the addition within the line item 2854 * submitted which contains the error. This value is omitted when 2855 * the error is not related to an Addition. 2856 */ 2857 public ErrorsComponent setSubdetailSequenceLinkId(int value) { 2858 if (this.subdetailSequenceLinkId == null) 2859 this.subdetailSequenceLinkId = new PositiveIntType(); 2860 this.subdetailSequenceLinkId.setValue(value); 2861 return this; 2862 } 2863 2864 /** 2865 * @return {@link #code} (An error code,froma specified code system, which 2866 * details why the claim could not be adjudicated.) 2867 */ 2868 public Coding getCode() { 2869 if (this.code == null) 2870 if (Configuration.errorOnAutoCreate()) 2871 throw new Error("Attempt to auto-create ErrorsComponent.code"); 2872 else if (Configuration.doAutoCreate()) 2873 this.code = new Coding(); // cc 2874 return this.code; 2875 } 2876 2877 public boolean hasCode() { 2878 return this.code != null && !this.code.isEmpty(); 2879 } 2880 2881 /** 2882 * @param value {@link #code} (An error code,froma specified code system, which 2883 * details why the claim could not be adjudicated.) 2884 */ 2885 public ErrorsComponent setCode(Coding value) { 2886 this.code = value; 2887 return this; 2888 } 2889 2890 protected void listChildren(List<Property> childrenList) { 2891 super.listChildren(childrenList); 2892 childrenList.add(new Property("sequenceLinkId", "positiveInt", 2893 "The sequence number of the line item submitted which contains the error. This value is omitted when the error is elsewhere.", 2894 0, java.lang.Integer.MAX_VALUE, sequenceLinkId)); 2895 childrenList.add(new Property("detailSequenceLinkId", "positiveInt", 2896 "The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition.", 2897 0, java.lang.Integer.MAX_VALUE, detailSequenceLinkId)); 2898 childrenList.add(new Property("subdetailSequenceLinkId", "positiveInt", 2899 "The sequence number of the addition within the line item submitted which contains the error. This value is omitted when the error is not related to an Addition.", 2900 0, java.lang.Integer.MAX_VALUE, subdetailSequenceLinkId)); 2901 childrenList.add(new Property("code", "Coding", 2902 "An error code,froma specified code system, which details why the claim could not be adjudicated.", 0, 2903 java.lang.Integer.MAX_VALUE, code)); 2904 } 2905 2906 @Override 2907 public void setProperty(String name, Base value) throws FHIRException { 2908 if (name.equals("sequenceLinkId")) 2909 this.sequenceLinkId = castToPositiveInt(value); // PositiveIntType 2910 else if (name.equals("detailSequenceLinkId")) 2911 this.detailSequenceLinkId = castToPositiveInt(value); // PositiveIntType 2912 else if (name.equals("subdetailSequenceLinkId")) 2913 this.subdetailSequenceLinkId = castToPositiveInt(value); // PositiveIntType 2914 else if (name.equals("code")) 2915 this.code = castToCoding(value); // Coding 2916 else 2917 super.setProperty(name, value); 2918 } 2919 2920 @Override 2921 public Base addChild(String name) throws FHIRException { 2922 if (name.equals("sequenceLinkId")) { 2923 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.sequenceLinkId"); 2924 } else if (name.equals("detailSequenceLinkId")) { 2925 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.detailSequenceLinkId"); 2926 } else if (name.equals("subdetailSequenceLinkId")) { 2927 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.subdetailSequenceLinkId"); 2928 } else if (name.equals("code")) { 2929 this.code = new Coding(); 2930 return this.code; 2931 } else 2932 return super.addChild(name); 2933 } 2934 2935 public ErrorsComponent copy() { 2936 ErrorsComponent dst = new ErrorsComponent(); 2937 copyValues(dst); 2938 dst.sequenceLinkId = sequenceLinkId == null ? null : sequenceLinkId.copy(); 2939 dst.detailSequenceLinkId = detailSequenceLinkId == null ? null : detailSequenceLinkId.copy(); 2940 dst.subdetailSequenceLinkId = subdetailSequenceLinkId == null ? null : subdetailSequenceLinkId.copy(); 2941 dst.code = code == null ? null : code.copy(); 2942 return dst; 2943 } 2944 2945 @Override 2946 public boolean equalsDeep(Base other) { 2947 if (!super.equalsDeep(other)) 2948 return false; 2949 if (!(other instanceof ErrorsComponent)) 2950 return false; 2951 ErrorsComponent o = (ErrorsComponent) other; 2952 return compareDeep(sequenceLinkId, o.sequenceLinkId, true) 2953 && compareDeep(detailSequenceLinkId, o.detailSequenceLinkId, true) 2954 && compareDeep(subdetailSequenceLinkId, o.subdetailSequenceLinkId, true) && compareDeep(code, o.code, true); 2955 } 2956 2957 @Override 2958 public boolean equalsShallow(Base other) { 2959 if (!super.equalsShallow(other)) 2960 return false; 2961 if (!(other instanceof ErrorsComponent)) 2962 return false; 2963 ErrorsComponent o = (ErrorsComponent) other; 2964 return compareValues(sequenceLinkId, o.sequenceLinkId, true) 2965 && compareValues(detailSequenceLinkId, o.detailSequenceLinkId, true) 2966 && compareValues(subdetailSequenceLinkId, o.subdetailSequenceLinkId, true); 2967 } 2968 2969 public boolean isEmpty() { 2970 return super.isEmpty() && (sequenceLinkId == null || sequenceLinkId.isEmpty()) 2971 && (detailSequenceLinkId == null || detailSequenceLinkId.isEmpty()) 2972 && (subdetailSequenceLinkId == null || subdetailSequenceLinkId.isEmpty()) && (code == null || code.isEmpty()); 2973 } 2974 2975 public String fhirType() { 2976 return "ClaimResponse.error"; 2977 2978 } 2979 2980 } 2981 2982 @Block() 2983 public static class NotesComponent extends BackboneElement implements IBaseBackboneElement { 2984 /** 2985 * An integer associated with each note which may be referred to from each 2986 * service line item. 2987 */ 2988 @Child(name = "number", type = { 2989 PositiveIntType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 2990 @Description(shortDefinition = "Note Number for this note", formalDefinition = "An integer associated with each note which may be referred to from each service line item.") 2991 protected PositiveIntType number; 2992 2993 /** 2994 * The note purpose: Print/Display. 2995 */ 2996 @Child(name = "type", type = { Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 2997 @Description(shortDefinition = "display | print | printoper", formalDefinition = "The note purpose: Print/Display.") 2998 protected Coding type; 2999 3000 /** 3001 * The note text. 3002 */ 3003 @Child(name = "text", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 3004 @Description(shortDefinition = "Note explanatory text", formalDefinition = "The note text.") 3005 protected StringType text; 3006 3007 private static final long serialVersionUID = 1768923951L; 3008 3009 /* 3010 * Constructor 3011 */ 3012 public NotesComponent() { 3013 super(); 3014 } 3015 3016 /** 3017 * @return {@link #number} (An integer associated with each note which may be 3018 * referred to from each service line item.). This is the underlying 3019 * object with id, value and extensions. The accessor "getNumber" gives 3020 * direct access to the value 3021 */ 3022 public PositiveIntType getNumberElement() { 3023 if (this.number == null) 3024 if (Configuration.errorOnAutoCreate()) 3025 throw new Error("Attempt to auto-create NotesComponent.number"); 3026 else if (Configuration.doAutoCreate()) 3027 this.number = new PositiveIntType(); // bb 3028 return this.number; 3029 } 3030 3031 public boolean hasNumberElement() { 3032 return this.number != null && !this.number.isEmpty(); 3033 } 3034 3035 public boolean hasNumber() { 3036 return this.number != null && !this.number.isEmpty(); 3037 } 3038 3039 /** 3040 * @param value {@link #number} (An integer associated with each note which may 3041 * be referred to from each service line item.). This is the 3042 * underlying object with id, value and extensions. The accessor 3043 * "getNumber" gives direct access to the value 3044 */ 3045 public NotesComponent setNumberElement(PositiveIntType value) { 3046 this.number = value; 3047 return this; 3048 } 3049 3050 /** 3051 * @return An integer associated with each note which may be referred to from 3052 * each service line item. 3053 */ 3054 public int getNumber() { 3055 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 3056 } 3057 3058 /** 3059 * @param value An integer associated with each note which may be referred to 3060 * from each service line item. 3061 */ 3062 public NotesComponent setNumber(int value) { 3063 if (this.number == null) 3064 this.number = new PositiveIntType(); 3065 this.number.setValue(value); 3066 return this; 3067 } 3068 3069 /** 3070 * @return {@link #type} (The note purpose: Print/Display.) 3071 */ 3072 public Coding getType() { 3073 if (this.type == null) 3074 if (Configuration.errorOnAutoCreate()) 3075 throw new Error("Attempt to auto-create NotesComponent.type"); 3076 else if (Configuration.doAutoCreate()) 3077 this.type = new Coding(); // cc 3078 return this.type; 3079 } 3080 3081 public boolean hasType() { 3082 return this.type != null && !this.type.isEmpty(); 3083 } 3084 3085 /** 3086 * @param value {@link #type} (The note purpose: Print/Display.) 3087 */ 3088 public NotesComponent setType(Coding value) { 3089 this.type = value; 3090 return this; 3091 } 3092 3093 /** 3094 * @return {@link #text} (The note text.). This is the underlying object with 3095 * id, value and extensions. The accessor "getText" gives direct access 3096 * to the value 3097 */ 3098 public StringType getTextElement() { 3099 if (this.text == null) 3100 if (Configuration.errorOnAutoCreate()) 3101 throw new Error("Attempt to auto-create NotesComponent.text"); 3102 else if (Configuration.doAutoCreate()) 3103 this.text = new StringType(); // bb 3104 return this.text; 3105 } 3106 3107 public boolean hasTextElement() { 3108 return this.text != null && !this.text.isEmpty(); 3109 } 3110 3111 public boolean hasText() { 3112 return this.text != null && !this.text.isEmpty(); 3113 } 3114 3115 /** 3116 * @param value {@link #text} (The note text.). This is the underlying object 3117 * with id, value and extensions. The accessor "getText" gives 3118 * direct access to the value 3119 */ 3120 public NotesComponent setTextElement(StringType value) { 3121 this.text = value; 3122 return this; 3123 } 3124 3125 /** 3126 * @return The note text. 3127 */ 3128 public String getText() { 3129 return this.text == null ? null : this.text.getValue(); 3130 } 3131 3132 /** 3133 * @param value The note text. 3134 */ 3135 public NotesComponent setText(String value) { 3136 if (Utilities.noString(value)) 3137 this.text = null; 3138 else { 3139 if (this.text == null) 3140 this.text = new StringType(); 3141 this.text.setValue(value); 3142 } 3143 return this; 3144 } 3145 3146 protected void listChildren(List<Property> childrenList) { 3147 super.listChildren(childrenList); 3148 childrenList.add(new Property("number", "positiveInt", 3149 "An integer associated with each note which may be referred to from each service line item.", 0, 3150 java.lang.Integer.MAX_VALUE, number)); 3151 childrenList.add( 3152 new Property("type", "Coding", "The note purpose: Print/Display.", 0, java.lang.Integer.MAX_VALUE, type)); 3153 childrenList.add(new Property("text", "string", "The note text.", 0, java.lang.Integer.MAX_VALUE, text)); 3154 } 3155 3156 @Override 3157 public void setProperty(String name, Base value) throws FHIRException { 3158 if (name.equals("number")) 3159 this.number = castToPositiveInt(value); // PositiveIntType 3160 else if (name.equals("type")) 3161 this.type = castToCoding(value); // Coding 3162 else if (name.equals("text")) 3163 this.text = castToString(value); // StringType 3164 else 3165 super.setProperty(name, value); 3166 } 3167 3168 @Override 3169 public Base addChild(String name) throws FHIRException { 3170 if (name.equals("number")) { 3171 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.number"); 3172 } else if (name.equals("type")) { 3173 this.type = new Coding(); 3174 return this.type; 3175 } else if (name.equals("text")) { 3176 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.text"); 3177 } else 3178 return super.addChild(name); 3179 } 3180 3181 public NotesComponent copy() { 3182 NotesComponent dst = new NotesComponent(); 3183 copyValues(dst); 3184 dst.number = number == null ? null : number.copy(); 3185 dst.type = type == null ? null : type.copy(); 3186 dst.text = text == null ? null : text.copy(); 3187 return dst; 3188 } 3189 3190 @Override 3191 public boolean equalsDeep(Base other) { 3192 if (!super.equalsDeep(other)) 3193 return false; 3194 if (!(other instanceof NotesComponent)) 3195 return false; 3196 NotesComponent o = (NotesComponent) other; 3197 return compareDeep(number, o.number, true) && compareDeep(type, o.type, true) && compareDeep(text, o.text, true); 3198 } 3199 3200 @Override 3201 public boolean equalsShallow(Base other) { 3202 if (!super.equalsShallow(other)) 3203 return false; 3204 if (!(other instanceof NotesComponent)) 3205 return false; 3206 NotesComponent o = (NotesComponent) other; 3207 return compareValues(number, o.number, true) && compareValues(text, o.text, true); 3208 } 3209 3210 public boolean isEmpty() { 3211 return super.isEmpty() && (number == null || number.isEmpty()) && (type == null || type.isEmpty()) 3212 && (text == null || text.isEmpty()); 3213 } 3214 3215 public String fhirType() { 3216 return "ClaimResponse.note"; 3217 3218 } 3219 3220 } 3221 3222 @Block() 3223 public static class CoverageComponent extends BackboneElement implements IBaseBackboneElement { 3224 /** 3225 * A service line item. 3226 */ 3227 @Child(name = "sequence", type = { 3228 PositiveIntType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 3229 @Description(shortDefinition = "Service instance identifier", formalDefinition = "A service line item.") 3230 protected PositiveIntType sequence; 3231 3232 /** 3233 * The instance number of the Coverage which is the focus for adjudication. The 3234 * Coverage against which the claim is to be adjudicated. 3235 */ 3236 @Child(name = "focal", type = { BooleanType.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 3237 @Description(shortDefinition = "Is the focal Coverage", formalDefinition = "The instance number of the Coverage which is the focus for adjudication. The Coverage against which the claim is to be adjudicated.") 3238 protected BooleanType focal; 3239 3240 /** 3241 * Reference to the program or plan identification, underwriter or payor. 3242 */ 3243 @Child(name = "coverage", type = { Coverage.class }, order = 3, min = 1, max = 1, modifier = false, summary = true) 3244 @Description(shortDefinition = "Insurance information", formalDefinition = "Reference to the program or plan identification, underwriter or payor.") 3245 protected Reference coverage; 3246 3247 /** 3248 * The actual object that is the target of the reference (Reference to the 3249 * program or plan identification, underwriter or payor.) 3250 */ 3251 protected Coverage coverageTarget; 3252 3253 /** 3254 * The contract number of a business agreement which describes the terms and 3255 * conditions. 3256 */ 3257 @Child(name = "businessArrangement", type = { 3258 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 3259 @Description(shortDefinition = "Business agreement", formalDefinition = "The contract number of a business agreement which describes the terms and conditions.") 3260 protected StringType businessArrangement; 3261 3262 /** 3263 * The relationship of the patient to the subscriber. 3264 */ 3265 @Child(name = "relationship", type = { 3266 Coding.class }, order = 5, min = 1, max = 1, modifier = false, summary = true) 3267 @Description(shortDefinition = "Patient relationship to subscriber", formalDefinition = "The relationship of the patient to the subscriber.") 3268 protected Coding relationship; 3269 3270 /** 3271 * A list of references from the Insurer to which these services pertain. 3272 */ 3273 @Child(name = "preAuthRef", type = { 3274 StringType.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3275 @Description(shortDefinition = "Pre-Authorization/Determination Reference", formalDefinition = "A list of references from the Insurer to which these services pertain.") 3276 protected List<StringType> preAuthRef; 3277 3278 /** 3279 * The Coverages adjudication details. 3280 */ 3281 @Child(name = "claimResponse", type = { 3282 ClaimResponse.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 3283 @Description(shortDefinition = "Adjudication results", formalDefinition = "The Coverages adjudication details.") 3284 protected Reference claimResponse; 3285 3286 /** 3287 * The actual object that is the target of the reference (The Coverages 3288 * adjudication details.) 3289 */ 3290 protected ClaimResponse claimResponseTarget; 3291 3292 /** 3293 * The style (standard) and version of the original material which was converted 3294 * into this resource. 3295 */ 3296 @Child(name = "originalRuleset", type = { 3297 Coding.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 3298 @Description(shortDefinition = "Original version", formalDefinition = "The style (standard) and version of the original material which was converted into this resource.") 3299 protected Coding originalRuleset; 3300 3301 private static final long serialVersionUID = 621250924L; 3302 3303 /* 3304 * Constructor 3305 */ 3306 public CoverageComponent() { 3307 super(); 3308 } 3309 3310 /* 3311 * Constructor 3312 */ 3313 public CoverageComponent(PositiveIntType sequence, BooleanType focal, Reference coverage, Coding relationship) { 3314 super(); 3315 this.sequence = sequence; 3316 this.focal = focal; 3317 this.coverage = coverage; 3318 this.relationship = relationship; 3319 } 3320 3321 /** 3322 * @return {@link #sequence} (A service line item.). This is the underlying 3323 * object with id, value and extensions. The accessor "getSequence" 3324 * gives direct access to the value 3325 */ 3326 public PositiveIntType getSequenceElement() { 3327 if (this.sequence == null) 3328 if (Configuration.errorOnAutoCreate()) 3329 throw new Error("Attempt to auto-create CoverageComponent.sequence"); 3330 else if (Configuration.doAutoCreate()) 3331 this.sequence = new PositiveIntType(); // bb 3332 return this.sequence; 3333 } 3334 3335 public boolean hasSequenceElement() { 3336 return this.sequence != null && !this.sequence.isEmpty(); 3337 } 3338 3339 public boolean hasSequence() { 3340 return this.sequence != null && !this.sequence.isEmpty(); 3341 } 3342 3343 /** 3344 * @param value {@link #sequence} (A service line item.). This is the underlying 3345 * object with id, value and extensions. The accessor "getSequence" 3346 * gives direct access to the value 3347 */ 3348 public CoverageComponent setSequenceElement(PositiveIntType value) { 3349 this.sequence = value; 3350 return this; 3351 } 3352 3353 /** 3354 * @return A service line item. 3355 */ 3356 public int getSequence() { 3357 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 3358 } 3359 3360 /** 3361 * @param value A service line item. 3362 */ 3363 public CoverageComponent setSequence(int value) { 3364 if (this.sequence == null) 3365 this.sequence = new PositiveIntType(); 3366 this.sequence.setValue(value); 3367 return this; 3368 } 3369 3370 /** 3371 * @return {@link #focal} (The instance number of the Coverage which is the 3372 * focus for adjudication. The Coverage against which the claim is to be 3373 * adjudicated.). This is the underlying object with id, value and 3374 * extensions. The accessor "getFocal" gives direct access to the value 3375 */ 3376 public BooleanType getFocalElement() { 3377 if (this.focal == null) 3378 if (Configuration.errorOnAutoCreate()) 3379 throw new Error("Attempt to auto-create CoverageComponent.focal"); 3380 else if (Configuration.doAutoCreate()) 3381 this.focal = new BooleanType(); // bb 3382 return this.focal; 3383 } 3384 3385 public boolean hasFocalElement() { 3386 return this.focal != null && !this.focal.isEmpty(); 3387 } 3388 3389 public boolean hasFocal() { 3390 return this.focal != null && !this.focal.isEmpty(); 3391 } 3392 3393 /** 3394 * @param value {@link #focal} (The instance number of the Coverage which is the 3395 * focus for adjudication. The Coverage against which the claim is 3396 * to be adjudicated.). This is the underlying object with id, 3397 * value and extensions. The accessor "getFocal" gives direct 3398 * access to the value 3399 */ 3400 public CoverageComponent setFocalElement(BooleanType value) { 3401 this.focal = value; 3402 return this; 3403 } 3404 3405 /** 3406 * @return The instance number of the Coverage which is the focus for 3407 * adjudication. The Coverage against which the claim is to be 3408 * adjudicated. 3409 */ 3410 public boolean getFocal() { 3411 return this.focal == null || this.focal.isEmpty() ? false : this.focal.getValue(); 3412 } 3413 3414 /** 3415 * @param value The instance number of the Coverage which is the focus for 3416 * adjudication. The Coverage against which the claim is to be 3417 * adjudicated. 3418 */ 3419 public CoverageComponent setFocal(boolean value) { 3420 if (this.focal == null) 3421 this.focal = new BooleanType(); 3422 this.focal.setValue(value); 3423 return this; 3424 } 3425 3426 /** 3427 * @return {@link #coverage} (Reference to the program or plan identification, 3428 * underwriter or payor.) 3429 */ 3430 public Reference getCoverage() { 3431 if (this.coverage == null) 3432 if (Configuration.errorOnAutoCreate()) 3433 throw new Error("Attempt to auto-create CoverageComponent.coverage"); 3434 else if (Configuration.doAutoCreate()) 3435 this.coverage = new Reference(); // cc 3436 return this.coverage; 3437 } 3438 3439 public boolean hasCoverage() { 3440 return this.coverage != null && !this.coverage.isEmpty(); 3441 } 3442 3443 /** 3444 * @param value {@link #coverage} (Reference to the program or plan 3445 * identification, underwriter or payor.) 3446 */ 3447 public CoverageComponent setCoverage(Reference value) { 3448 this.coverage = value; 3449 return this; 3450 } 3451 3452 /** 3453 * @return {@link #coverage} The actual object that is the target of the 3454 * reference. The reference library doesn't populate this, but you can 3455 * use it to hold the resource if you resolve it. (Reference to the 3456 * program or plan identification, underwriter or payor.) 3457 */ 3458 public Coverage getCoverageTarget() { 3459 if (this.coverageTarget == null) 3460 if (Configuration.errorOnAutoCreate()) 3461 throw new Error("Attempt to auto-create CoverageComponent.coverage"); 3462 else if (Configuration.doAutoCreate()) 3463 this.coverageTarget = new Coverage(); // aa 3464 return this.coverageTarget; 3465 } 3466 3467 /** 3468 * @param value {@link #coverage} The actual object that is the target of the 3469 * reference. The reference library doesn't use these, but you can 3470 * use it to hold the resource if you resolve it. (Reference to the 3471 * program or plan identification, underwriter or payor.) 3472 */ 3473 public CoverageComponent setCoverageTarget(Coverage value) { 3474 this.coverageTarget = value; 3475 return this; 3476 } 3477 3478 /** 3479 * @return {@link #businessArrangement} (The contract number of a business 3480 * agreement which describes the terms and conditions.). This is the 3481 * underlying object with id, value and extensions. The accessor 3482 * "getBusinessArrangement" gives direct access to the value 3483 */ 3484 public StringType getBusinessArrangementElement() { 3485 if (this.businessArrangement == null) 3486 if (Configuration.errorOnAutoCreate()) 3487 throw new Error("Attempt to auto-create CoverageComponent.businessArrangement"); 3488 else if (Configuration.doAutoCreate()) 3489 this.businessArrangement = new StringType(); // bb 3490 return this.businessArrangement; 3491 } 3492 3493 public boolean hasBusinessArrangementElement() { 3494 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 3495 } 3496 3497 public boolean hasBusinessArrangement() { 3498 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 3499 } 3500 3501 /** 3502 * @param value {@link #businessArrangement} (The contract number of a business 3503 * agreement which describes the terms and conditions.). This is 3504 * the underlying object with id, value and extensions. The 3505 * accessor "getBusinessArrangement" gives direct access to the 3506 * value 3507 */ 3508 public CoverageComponent setBusinessArrangementElement(StringType value) { 3509 this.businessArrangement = value; 3510 return this; 3511 } 3512 3513 /** 3514 * @return The contract number of a business agreement which describes the terms 3515 * and conditions. 3516 */ 3517 public String getBusinessArrangement() { 3518 return this.businessArrangement == null ? null : this.businessArrangement.getValue(); 3519 } 3520 3521 /** 3522 * @param value The contract number of a business agreement which describes the 3523 * terms and conditions. 3524 */ 3525 public CoverageComponent setBusinessArrangement(String value) { 3526 if (Utilities.noString(value)) 3527 this.businessArrangement = null; 3528 else { 3529 if (this.businessArrangement == null) 3530 this.businessArrangement = new StringType(); 3531 this.businessArrangement.setValue(value); 3532 } 3533 return this; 3534 } 3535 3536 /** 3537 * @return {@link #relationship} (The relationship of the patient to the 3538 * subscriber.) 3539 */ 3540 public Coding getRelationship() { 3541 if (this.relationship == null) 3542 if (Configuration.errorOnAutoCreate()) 3543 throw new Error("Attempt to auto-create CoverageComponent.relationship"); 3544 else if (Configuration.doAutoCreate()) 3545 this.relationship = new Coding(); // cc 3546 return this.relationship; 3547 } 3548 3549 public boolean hasRelationship() { 3550 return this.relationship != null && !this.relationship.isEmpty(); 3551 } 3552 3553 /** 3554 * @param value {@link #relationship} (The relationship of the patient to the 3555 * subscriber.) 3556 */ 3557 public CoverageComponent setRelationship(Coding value) { 3558 this.relationship = value; 3559 return this; 3560 } 3561 3562 /** 3563 * @return {@link #preAuthRef} (A list of references from the Insurer to which 3564 * these services pertain.) 3565 */ 3566 public List<StringType> getPreAuthRef() { 3567 if (this.preAuthRef == null) 3568 this.preAuthRef = new ArrayList<StringType>(); 3569 return this.preAuthRef; 3570 } 3571 3572 public boolean hasPreAuthRef() { 3573 if (this.preAuthRef == null) 3574 return false; 3575 for (StringType item : this.preAuthRef) 3576 if (!item.isEmpty()) 3577 return true; 3578 return false; 3579 } 3580 3581 /** 3582 * @return {@link #preAuthRef} (A list of references from the Insurer to which 3583 * these services pertain.) 3584 */ 3585 // syntactic sugar 3586 public StringType addPreAuthRefElement() {// 2 3587 StringType t = new StringType(); 3588 if (this.preAuthRef == null) 3589 this.preAuthRef = new ArrayList<StringType>(); 3590 this.preAuthRef.add(t); 3591 return t; 3592 } 3593 3594 /** 3595 * @param value {@link #preAuthRef} (A list of references from the Insurer to 3596 * which these services pertain.) 3597 */ 3598 public CoverageComponent addPreAuthRef(String value) { // 1 3599 StringType t = new StringType(); 3600 t.setValue(value); 3601 if (this.preAuthRef == null) 3602 this.preAuthRef = new ArrayList<StringType>(); 3603 this.preAuthRef.add(t); 3604 return this; 3605 } 3606 3607 /** 3608 * @param value {@link #preAuthRef} (A list of references from the Insurer to 3609 * which these services pertain.) 3610 */ 3611 public boolean hasPreAuthRef(String value) { 3612 if (this.preAuthRef == null) 3613 return false; 3614 for (StringType v : this.preAuthRef) 3615 if (v.equals(value)) // string 3616 return true; 3617 return false; 3618 } 3619 3620 /** 3621 * @return {@link #claimResponse} (The Coverages adjudication details.) 3622 */ 3623 public Reference getClaimResponse() { 3624 if (this.claimResponse == null) 3625 if (Configuration.errorOnAutoCreate()) 3626 throw new Error("Attempt to auto-create CoverageComponent.claimResponse"); 3627 else if (Configuration.doAutoCreate()) 3628 this.claimResponse = new Reference(); // cc 3629 return this.claimResponse; 3630 } 3631 3632 public boolean hasClaimResponse() { 3633 return this.claimResponse != null && !this.claimResponse.isEmpty(); 3634 } 3635 3636 /** 3637 * @param value {@link #claimResponse} (The Coverages adjudication details.) 3638 */ 3639 public CoverageComponent setClaimResponse(Reference value) { 3640 this.claimResponse = value; 3641 return this; 3642 } 3643 3644 /** 3645 * @return {@link #claimResponse} The actual object that is the target of the 3646 * reference. The reference library doesn't populate this, but you can 3647 * use it to hold the resource if you resolve it. (The Coverages 3648 * adjudication details.) 3649 */ 3650 public ClaimResponse getClaimResponseTarget() { 3651 if (this.claimResponseTarget == null) 3652 if (Configuration.errorOnAutoCreate()) 3653 throw new Error("Attempt to auto-create CoverageComponent.claimResponse"); 3654 else if (Configuration.doAutoCreate()) 3655 this.claimResponseTarget = new ClaimResponse(); // aa 3656 return this.claimResponseTarget; 3657 } 3658 3659 /** 3660 * @param value {@link #claimResponse} The actual object that is the target of 3661 * the reference. The reference library doesn't use these, but you 3662 * can use it to hold the resource if you resolve it. (The 3663 * Coverages adjudication details.) 3664 */ 3665 public CoverageComponent setClaimResponseTarget(ClaimResponse value) { 3666 this.claimResponseTarget = value; 3667 return this; 3668 } 3669 3670 /** 3671 * @return {@link #originalRuleset} (The style (standard) and version of the 3672 * original material which was converted into this resource.) 3673 */ 3674 public Coding getOriginalRuleset() { 3675 if (this.originalRuleset == null) 3676 if (Configuration.errorOnAutoCreate()) 3677 throw new Error("Attempt to auto-create CoverageComponent.originalRuleset"); 3678 else if (Configuration.doAutoCreate()) 3679 this.originalRuleset = new Coding(); // cc 3680 return this.originalRuleset; 3681 } 3682 3683 public boolean hasOriginalRuleset() { 3684 return this.originalRuleset != null && !this.originalRuleset.isEmpty(); 3685 } 3686 3687 /** 3688 * @param value {@link #originalRuleset} (The style (standard) and version of 3689 * the original material which was converted into this resource.) 3690 */ 3691 public CoverageComponent setOriginalRuleset(Coding value) { 3692 this.originalRuleset = value; 3693 return this; 3694 } 3695 3696 protected void listChildren(List<Property> childrenList) { 3697 super.listChildren(childrenList); 3698 childrenList.add( 3699 new Property("sequence", "positiveInt", "A service line item.", 0, java.lang.Integer.MAX_VALUE, sequence)); 3700 childrenList.add(new Property("focal", "boolean", 3701 "The instance number of the Coverage which is the focus for adjudication. The Coverage against which the claim is to be adjudicated.", 3702 0, java.lang.Integer.MAX_VALUE, focal)); 3703 childrenList.add(new Property("coverage", "Reference(Coverage)", 3704 "Reference to the program or plan identification, underwriter or payor.", 0, java.lang.Integer.MAX_VALUE, 3705 coverage)); 3706 childrenList.add(new Property("businessArrangement", "string", 3707 "The contract number of a business agreement which describes the terms and conditions.", 0, 3708 java.lang.Integer.MAX_VALUE, businessArrangement)); 3709 childrenList.add(new Property("relationship", "Coding", "The relationship of the patient to the subscriber.", 0, 3710 java.lang.Integer.MAX_VALUE, relationship)); 3711 childrenList.add( 3712 new Property("preAuthRef", "string", "A list of references from the Insurer to which these services pertain.", 3713 0, java.lang.Integer.MAX_VALUE, preAuthRef)); 3714 childrenList.add(new Property("claimResponse", "Reference(ClaimResponse)", "The Coverages adjudication details.", 3715 0, java.lang.Integer.MAX_VALUE, claimResponse)); 3716 childrenList.add(new Property("originalRuleset", "Coding", 3717 "The style (standard) and version of the original material which was converted into this resource.", 0, 3718 java.lang.Integer.MAX_VALUE, originalRuleset)); 3719 } 3720 3721 @Override 3722 public void setProperty(String name, Base value) throws FHIRException { 3723 if (name.equals("sequence")) 3724 this.sequence = castToPositiveInt(value); // PositiveIntType 3725 else if (name.equals("focal")) 3726 this.focal = castToBoolean(value); // BooleanType 3727 else if (name.equals("coverage")) 3728 this.coverage = castToReference(value); // Reference 3729 else if (name.equals("businessArrangement")) 3730 this.businessArrangement = castToString(value); // StringType 3731 else if (name.equals("relationship")) 3732 this.relationship = castToCoding(value); // Coding 3733 else if (name.equals("preAuthRef")) 3734 this.getPreAuthRef().add(castToString(value)); 3735 else if (name.equals("claimResponse")) 3736 this.claimResponse = castToReference(value); // Reference 3737 else if (name.equals("originalRuleset")) 3738 this.originalRuleset = castToCoding(value); // Coding 3739 else 3740 super.setProperty(name, value); 3741 } 3742 3743 @Override 3744 public Base addChild(String name) throws FHIRException { 3745 if (name.equals("sequence")) { 3746 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.sequence"); 3747 } else if (name.equals("focal")) { 3748 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.focal"); 3749 } else if (name.equals("coverage")) { 3750 this.coverage = new Reference(); 3751 return this.coverage; 3752 } else if (name.equals("businessArrangement")) { 3753 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.businessArrangement"); 3754 } else if (name.equals("relationship")) { 3755 this.relationship = new Coding(); 3756 return this.relationship; 3757 } else if (name.equals("preAuthRef")) { 3758 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.preAuthRef"); 3759 } else if (name.equals("claimResponse")) { 3760 this.claimResponse = new Reference(); 3761 return this.claimResponse; 3762 } else if (name.equals("originalRuleset")) { 3763 this.originalRuleset = new Coding(); 3764 return this.originalRuleset; 3765 } else 3766 return super.addChild(name); 3767 } 3768 3769 public CoverageComponent copy() { 3770 CoverageComponent dst = new CoverageComponent(); 3771 copyValues(dst); 3772 dst.sequence = sequence == null ? null : sequence.copy(); 3773 dst.focal = focal == null ? null : focal.copy(); 3774 dst.coverage = coverage == null ? null : coverage.copy(); 3775 dst.businessArrangement = businessArrangement == null ? null : businessArrangement.copy(); 3776 dst.relationship = relationship == null ? null : relationship.copy(); 3777 if (preAuthRef != null) { 3778 dst.preAuthRef = new ArrayList<StringType>(); 3779 for (StringType i : preAuthRef) 3780 dst.preAuthRef.add(i.copy()); 3781 } 3782 ; 3783 dst.claimResponse = claimResponse == null ? null : claimResponse.copy(); 3784 dst.originalRuleset = originalRuleset == null ? null : originalRuleset.copy(); 3785 return dst; 3786 } 3787 3788 @Override 3789 public boolean equalsDeep(Base other) { 3790 if (!super.equalsDeep(other)) 3791 return false; 3792 if (!(other instanceof CoverageComponent)) 3793 return false; 3794 CoverageComponent o = (CoverageComponent) other; 3795 return compareDeep(sequence, o.sequence, true) && compareDeep(focal, o.focal, true) 3796 && compareDeep(coverage, o.coverage, true) && compareDeep(businessArrangement, o.businessArrangement, true) 3797 && compareDeep(relationship, o.relationship, true) && compareDeep(preAuthRef, o.preAuthRef, true) 3798 && compareDeep(claimResponse, o.claimResponse, true) && compareDeep(originalRuleset, o.originalRuleset, true); 3799 } 3800 3801 @Override 3802 public boolean equalsShallow(Base other) { 3803 if (!super.equalsShallow(other)) 3804 return false; 3805 if (!(other instanceof CoverageComponent)) 3806 return false; 3807 CoverageComponent o = (CoverageComponent) other; 3808 return compareValues(sequence, o.sequence, true) && compareValues(focal, o.focal, true) 3809 && compareValues(businessArrangement, o.businessArrangement, true) 3810 && compareValues(preAuthRef, o.preAuthRef, true); 3811 } 3812 3813 public boolean isEmpty() { 3814 return super.isEmpty() && (sequence == null || sequence.isEmpty()) && (focal == null || focal.isEmpty()) 3815 && (coverage == null || coverage.isEmpty()) && (businessArrangement == null || businessArrangement.isEmpty()) 3816 && (relationship == null || relationship.isEmpty()) && (preAuthRef == null || preAuthRef.isEmpty()) 3817 && (claimResponse == null || claimResponse.isEmpty()) 3818 && (originalRuleset == null || originalRuleset.isEmpty()); 3819 } 3820 3821 public String fhirType() { 3822 return "ClaimResponse.coverage"; 3823 3824 } 3825 3826 } 3827 3828 /** 3829 * The Response business identifier. 3830 */ 3831 @Child(name = "identifier", type = { 3832 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3833 @Description(shortDefinition = "Response number", formalDefinition = "The Response business identifier.") 3834 protected List<Identifier> identifier; 3835 3836 /** 3837 * Original request resource referrence. 3838 */ 3839 @Child(name = "request", type = { Claim.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 3840 @Description(shortDefinition = "Id of resource triggering adjudication", formalDefinition = "Original request resource referrence.") 3841 protected Reference request; 3842 3843 /** 3844 * The actual object that is the target of the reference (Original request 3845 * resource referrence.) 3846 */ 3847 protected Claim requestTarget; 3848 3849 /** 3850 * The version of the style of resource contents. This should be mapped to the 3851 * allowable profiles for this and supporting resources. 3852 */ 3853 @Child(name = "ruleset", type = { Coding.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 3854 @Description(shortDefinition = "Resource version", formalDefinition = "The version of the style of resource contents. This should be mapped to the allowable profiles for this and supporting resources.") 3855 protected Coding ruleset; 3856 3857 /** 3858 * The style (standard) and version of the original material which was converted 3859 * into this resource. 3860 */ 3861 @Child(name = "originalRuleset", type = { 3862 Coding.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 3863 @Description(shortDefinition = "Original version", formalDefinition = "The style (standard) and version of the original material which was converted into this resource.") 3864 protected Coding originalRuleset; 3865 3866 /** 3867 * The date when the enclosed suite of services were performed or completed. 3868 */ 3869 @Child(name = "created", type = { DateTimeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 3870 @Description(shortDefinition = "Creation date", formalDefinition = "The date when the enclosed suite of services were performed or completed.") 3871 protected DateTimeType created; 3872 3873 /** 3874 * The Insurer who produced this adjudicated response. 3875 */ 3876 @Child(name = "organization", type = { 3877 Organization.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 3878 @Description(shortDefinition = "Insurer", formalDefinition = "The Insurer who produced this adjudicated response.") 3879 protected Reference organization; 3880 3881 /** 3882 * The actual object that is the target of the reference (The Insurer who 3883 * produced this adjudicated response.) 3884 */ 3885 protected Organization organizationTarget; 3886 3887 /** 3888 * The practitioner who is responsible for the services rendered to the patient. 3889 */ 3890 @Child(name = "requestProvider", type = { 3891 Practitioner.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 3892 @Description(shortDefinition = "Responsible practitioner", formalDefinition = "The practitioner who is responsible for the services rendered to the patient.") 3893 protected Reference requestProvider; 3894 3895 /** 3896 * The actual object that is the target of the reference (The practitioner who 3897 * is responsible for the services rendered to the patient.) 3898 */ 3899 protected Practitioner requestProviderTarget; 3900 3901 /** 3902 * The organization which is responsible for the services rendered to the 3903 * patient. 3904 */ 3905 @Child(name = "requestOrganization", type = { 3906 Organization.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 3907 @Description(shortDefinition = "Responsible organization", formalDefinition = "The organization which is responsible for the services rendered to the patient.") 3908 protected Reference requestOrganization; 3909 3910 /** 3911 * The actual object that is the target of the reference (The organization which 3912 * is responsible for the services rendered to the patient.) 3913 */ 3914 protected Organization requestOrganizationTarget; 3915 3916 /** 3917 * Transaction status: error, complete. 3918 */ 3919 @Child(name = "outcome", type = { CodeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 3920 @Description(shortDefinition = "complete | error", formalDefinition = "Transaction status: error, complete.") 3921 protected Enumeration<RemittanceOutcome> outcome; 3922 3923 /** 3924 * A description of the status of the adjudication. 3925 */ 3926 @Child(name = "disposition", type = { 3927 StringType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 3928 @Description(shortDefinition = "Disposition Message", formalDefinition = "A description of the status of the adjudication.") 3929 protected StringType disposition; 3930 3931 /** 3932 * Party to be reimbursed: Subscriber, provider, other. 3933 */ 3934 @Child(name = "payeeType", type = { Coding.class }, order = 10, min = 0, max = 1, modifier = false, summary = true) 3935 @Description(shortDefinition = "Party to be paid any benefits payable", formalDefinition = "Party to be reimbursed: Subscriber, provider, other.") 3936 protected Coding payeeType; 3937 3938 /** 3939 * The first tier service adjudications for submitted services. 3940 */ 3941 @Child(name = "item", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3942 @Description(shortDefinition = "Line items", formalDefinition = "The first tier service adjudications for submitted services.") 3943 protected List<ItemsComponent> item; 3944 3945 /** 3946 * The first tier service adjudications for payor added services. 3947 */ 3948 @Child(name = "addItem", type = {}, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3949 @Description(shortDefinition = "Insurer added line items", formalDefinition = "The first tier service adjudications for payor added services.") 3950 protected List<AddedItemComponent> addItem; 3951 3952 /** 3953 * Mutually exclusive with Services Provided (Item). 3954 */ 3955 @Child(name = "error", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 3956 @Description(shortDefinition = "Processing errors", formalDefinition = "Mutually exclusive with Services Provided (Item).") 3957 protected List<ErrorsComponent> error; 3958 3959 /** 3960 * The total cost of the services reported. 3961 */ 3962 @Child(name = "totalCost", type = { Money.class }, order = 14, min = 0, max = 1, modifier = false, summary = true) 3963 @Description(shortDefinition = "Total Cost of service from the Claim", formalDefinition = "The total cost of the services reported.") 3964 protected Money totalCost; 3965 3966 /** 3967 * The amount of deductible applied which was not allocated to any particular 3968 * service line. 3969 */ 3970 @Child(name = "unallocDeductable", type = { 3971 Money.class }, order = 15, min = 0, max = 1, modifier = false, summary = true) 3972 @Description(shortDefinition = "Unallocated deductible", formalDefinition = "The amount of deductible applied which was not allocated to any particular service line.") 3973 protected Money unallocDeductable; 3974 3975 /** 3976 * Total amount of benefit payable (Equal to sum of the Benefit amounts from all 3977 * detail lines and additions less the Unallocated Deductible). 3978 */ 3979 @Child(name = "totalBenefit", type = { Money.class }, order = 16, min = 0, max = 1, modifier = false, summary = true) 3980 @Description(shortDefinition = "Total benefit payable for the Claim", formalDefinition = "Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductible).") 3981 protected Money totalBenefit; 3982 3983 /** 3984 * Adjustment to the payment of this transaction which is not related to 3985 * adjudication of this transaction. 3986 */ 3987 @Child(name = "paymentAdjustment", type = { 3988 Money.class }, order = 17, min = 0, max = 1, modifier = false, summary = true) 3989 @Description(shortDefinition = "Payment adjustment for non-Claim issues", formalDefinition = "Adjustment to the payment of this transaction which is not related to adjudication of this transaction.") 3990 protected Money paymentAdjustment; 3991 3992 /** 3993 * Reason for the payment adjustment. 3994 */ 3995 @Child(name = "paymentAdjustmentReason", type = { 3996 Coding.class }, order = 18, min = 0, max = 1, modifier = false, summary = true) 3997 @Description(shortDefinition = "Reason for Payment adjustment", formalDefinition = "Reason for the payment adjustment.") 3998 protected Coding paymentAdjustmentReason; 3999 4000 /** 4001 * Estimated payment data. 4002 */ 4003 @Child(name = "paymentDate", type = { 4004 DateType.class }, order = 19, min = 0, max = 1, modifier = false, summary = true) 4005 @Description(shortDefinition = "Expected data of Payment", formalDefinition = "Estimated payment data.") 4006 protected DateType paymentDate; 4007 4008 /** 4009 * Payable less any payment adjustment. 4010 */ 4011 @Child(name = "paymentAmount", type = { Money.class }, order = 20, min = 0, max = 1, modifier = false, summary = true) 4012 @Description(shortDefinition = "Payment amount", formalDefinition = "Payable less any payment adjustment.") 4013 protected Money paymentAmount; 4014 4015 /** 4016 * Payment identifier. 4017 */ 4018 @Child(name = "paymentRef", type = { 4019 Identifier.class }, order = 21, min = 0, max = 1, modifier = false, summary = true) 4020 @Description(shortDefinition = "Payment identifier", formalDefinition = "Payment identifier.") 4021 protected Identifier paymentRef; 4022 4023 /** 4024 * Status of funds reservation (For provider, for Patient, None). 4025 */ 4026 @Child(name = "reserved", type = { Coding.class }, order = 22, min = 0, max = 1, modifier = false, summary = true) 4027 @Description(shortDefinition = "Funds reserved status", formalDefinition = "Status of funds reservation (For provider, for Patient, None).") 4028 protected Coding reserved; 4029 4030 /** 4031 * The form to be used for printing the content. 4032 */ 4033 @Child(name = "form", type = { Coding.class }, order = 23, min = 0, max = 1, modifier = false, summary = true) 4034 @Description(shortDefinition = "Printed Form Identifier", formalDefinition = "The form to be used for printing the content.") 4035 protected Coding form; 4036 4037 /** 4038 * Note text. 4039 */ 4040 @Child(name = "note", type = {}, order = 24, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4041 @Description(shortDefinition = "Processing notes", formalDefinition = "Note text.") 4042 protected List<NotesComponent> note; 4043 4044 /** 4045 * Financial instrument by which payment information for health care. 4046 */ 4047 @Child(name = "coverage", type = {}, order = 25, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 4048 @Description(shortDefinition = "Insurance or medical plan", formalDefinition = "Financial instrument by which payment information for health care.") 4049 protected List<CoverageComponent> coverage; 4050 4051 private static final long serialVersionUID = 2021598689L; 4052 4053 /* 4054 * Constructor 4055 */ 4056 public ClaimResponse() { 4057 super(); 4058 } 4059 4060 /** 4061 * @return {@link #identifier} (The Response business identifier.) 4062 */ 4063 public List<Identifier> getIdentifier() { 4064 if (this.identifier == null) 4065 this.identifier = new ArrayList<Identifier>(); 4066 return this.identifier; 4067 } 4068 4069 public boolean hasIdentifier() { 4070 if (this.identifier == null) 4071 return false; 4072 for (Identifier item : this.identifier) 4073 if (!item.isEmpty()) 4074 return true; 4075 return false; 4076 } 4077 4078 /** 4079 * @return {@link #identifier} (The Response business identifier.) 4080 */ 4081 // syntactic sugar 4082 public Identifier addIdentifier() { // 3 4083 Identifier t = new Identifier(); 4084 if (this.identifier == null) 4085 this.identifier = new ArrayList<Identifier>(); 4086 this.identifier.add(t); 4087 return t; 4088 } 4089 4090 // syntactic sugar 4091 public ClaimResponse addIdentifier(Identifier t) { // 3 4092 if (t == null) 4093 return this; 4094 if (this.identifier == null) 4095 this.identifier = new ArrayList<Identifier>(); 4096 this.identifier.add(t); 4097 return this; 4098 } 4099 4100 /** 4101 * @return {@link #request} (Original request resource referrence.) 4102 */ 4103 public Reference getRequest() { 4104 if (this.request == null) 4105 if (Configuration.errorOnAutoCreate()) 4106 throw new Error("Attempt to auto-create ClaimResponse.request"); 4107 else if (Configuration.doAutoCreate()) 4108 this.request = new Reference(); // cc 4109 return this.request; 4110 } 4111 4112 public boolean hasRequest() { 4113 return this.request != null && !this.request.isEmpty(); 4114 } 4115 4116 /** 4117 * @param value {@link #request} (Original request resource referrence.) 4118 */ 4119 public ClaimResponse setRequest(Reference value) { 4120 this.request = value; 4121 return this; 4122 } 4123 4124 /** 4125 * @return {@link #request} The actual object that is the target of the 4126 * reference. The reference library doesn't populate this, but you can 4127 * use it to hold the resource if you resolve it. (Original request 4128 * resource referrence.) 4129 */ 4130 public Claim getRequestTarget() { 4131 if (this.requestTarget == null) 4132 if (Configuration.errorOnAutoCreate()) 4133 throw new Error("Attempt to auto-create ClaimResponse.request"); 4134 else if (Configuration.doAutoCreate()) 4135 this.requestTarget = new Claim(); // aa 4136 return this.requestTarget; 4137 } 4138 4139 /** 4140 * @param value {@link #request} The actual object that is the target of the 4141 * reference. The reference library doesn't use these, but you can 4142 * use it to hold the resource if you resolve it. (Original request 4143 * resource referrence.) 4144 */ 4145 public ClaimResponse setRequestTarget(Claim value) { 4146 this.requestTarget = value; 4147 return this; 4148 } 4149 4150 /** 4151 * @return {@link #ruleset} (The version of the style of resource contents. This 4152 * should be mapped to the allowable profiles for this and supporting 4153 * resources.) 4154 */ 4155 public Coding getRuleset() { 4156 if (this.ruleset == null) 4157 if (Configuration.errorOnAutoCreate()) 4158 throw new Error("Attempt to auto-create ClaimResponse.ruleset"); 4159 else if (Configuration.doAutoCreate()) 4160 this.ruleset = new Coding(); // cc 4161 return this.ruleset; 4162 } 4163 4164 public boolean hasRuleset() { 4165 return this.ruleset != null && !this.ruleset.isEmpty(); 4166 } 4167 4168 /** 4169 * @param value {@link #ruleset} (The version of the style of resource contents. 4170 * This should be mapped to the allowable profiles for this and 4171 * supporting resources.) 4172 */ 4173 public ClaimResponse setRuleset(Coding value) { 4174 this.ruleset = value; 4175 return this; 4176 } 4177 4178 /** 4179 * @return {@link #originalRuleset} (The style (standard) and version of the 4180 * original material which was converted into this resource.) 4181 */ 4182 public Coding getOriginalRuleset() { 4183 if (this.originalRuleset == null) 4184 if (Configuration.errorOnAutoCreate()) 4185 throw new Error("Attempt to auto-create ClaimResponse.originalRuleset"); 4186 else if (Configuration.doAutoCreate()) 4187 this.originalRuleset = new Coding(); // cc 4188 return this.originalRuleset; 4189 } 4190 4191 public boolean hasOriginalRuleset() { 4192 return this.originalRuleset != null && !this.originalRuleset.isEmpty(); 4193 } 4194 4195 /** 4196 * @param value {@link #originalRuleset} (The style (standard) and version of 4197 * the original material which was converted into this resource.) 4198 */ 4199 public ClaimResponse setOriginalRuleset(Coding value) { 4200 this.originalRuleset = value; 4201 return this; 4202 } 4203 4204 /** 4205 * @return {@link #created} (The date when the enclosed suite of services were 4206 * performed or completed.). This is the underlying object with id, 4207 * value and extensions. The accessor "getCreated" gives direct access 4208 * to the value 4209 */ 4210 public DateTimeType getCreatedElement() { 4211 if (this.created == null) 4212 if (Configuration.errorOnAutoCreate()) 4213 throw new Error("Attempt to auto-create ClaimResponse.created"); 4214 else if (Configuration.doAutoCreate()) 4215 this.created = new DateTimeType(); // bb 4216 return this.created; 4217 } 4218 4219 public boolean hasCreatedElement() { 4220 return this.created != null && !this.created.isEmpty(); 4221 } 4222 4223 public boolean hasCreated() { 4224 return this.created != null && !this.created.isEmpty(); 4225 } 4226 4227 /** 4228 * @param value {@link #created} (The date when the enclosed suite of services 4229 * were performed or completed.). This is the underlying object 4230 * with id, value and extensions. The accessor "getCreated" gives 4231 * direct access to the value 4232 */ 4233 public ClaimResponse setCreatedElement(DateTimeType value) { 4234 this.created = value; 4235 return this; 4236 } 4237 4238 /** 4239 * @return The date when the enclosed suite of services were performed or 4240 * completed. 4241 */ 4242 public Date getCreated() { 4243 return this.created == null ? null : this.created.getValue(); 4244 } 4245 4246 /** 4247 * @param value The date when the enclosed suite of services were performed or 4248 * completed. 4249 */ 4250 public ClaimResponse setCreated(Date value) { 4251 if (value == null) 4252 this.created = null; 4253 else { 4254 if (this.created == null) 4255 this.created = new DateTimeType(); 4256 this.created.setValue(value); 4257 } 4258 return this; 4259 } 4260 4261 /** 4262 * @return {@link #organization} (The Insurer who produced this adjudicated 4263 * response.) 4264 */ 4265 public Reference getOrganization() { 4266 if (this.organization == null) 4267 if (Configuration.errorOnAutoCreate()) 4268 throw new Error("Attempt to auto-create ClaimResponse.organization"); 4269 else if (Configuration.doAutoCreate()) 4270 this.organization = new Reference(); // cc 4271 return this.organization; 4272 } 4273 4274 public boolean hasOrganization() { 4275 return this.organization != null && !this.organization.isEmpty(); 4276 } 4277 4278 /** 4279 * @param value {@link #organization} (The Insurer who produced this adjudicated 4280 * response.) 4281 */ 4282 public ClaimResponse setOrganization(Reference value) { 4283 this.organization = value; 4284 return this; 4285 } 4286 4287 /** 4288 * @return {@link #organization} The actual object that is the target of the 4289 * reference. The reference library doesn't populate this, but you can 4290 * use it to hold the resource if you resolve it. (The Insurer who 4291 * produced this adjudicated response.) 4292 */ 4293 public Organization getOrganizationTarget() { 4294 if (this.organizationTarget == null) 4295 if (Configuration.errorOnAutoCreate()) 4296 throw new Error("Attempt to auto-create ClaimResponse.organization"); 4297 else if (Configuration.doAutoCreate()) 4298 this.organizationTarget = new Organization(); // aa 4299 return this.organizationTarget; 4300 } 4301 4302 /** 4303 * @param value {@link #organization} The actual object that is the target of 4304 * the reference. The reference library doesn't use these, but you 4305 * can use it to hold the resource if you resolve it. (The Insurer 4306 * who produced this adjudicated response.) 4307 */ 4308 public ClaimResponse setOrganizationTarget(Organization value) { 4309 this.organizationTarget = value; 4310 return this; 4311 } 4312 4313 /** 4314 * @return {@link #requestProvider} (The practitioner who is responsible for the 4315 * services rendered to the patient.) 4316 */ 4317 public Reference getRequestProvider() { 4318 if (this.requestProvider == null) 4319 if (Configuration.errorOnAutoCreate()) 4320 throw new Error("Attempt to auto-create ClaimResponse.requestProvider"); 4321 else if (Configuration.doAutoCreate()) 4322 this.requestProvider = new Reference(); // cc 4323 return this.requestProvider; 4324 } 4325 4326 public boolean hasRequestProvider() { 4327 return this.requestProvider != null && !this.requestProvider.isEmpty(); 4328 } 4329 4330 /** 4331 * @param value {@link #requestProvider} (The practitioner who is responsible 4332 * for the services rendered to the patient.) 4333 */ 4334 public ClaimResponse setRequestProvider(Reference value) { 4335 this.requestProvider = value; 4336 return this; 4337 } 4338 4339 /** 4340 * @return {@link #requestProvider} The actual object that is the target of the 4341 * reference. The reference library doesn't populate this, but you can 4342 * use it to hold the resource if you resolve it. (The practitioner who 4343 * is responsible for the services rendered to the patient.) 4344 */ 4345 public Practitioner getRequestProviderTarget() { 4346 if (this.requestProviderTarget == null) 4347 if (Configuration.errorOnAutoCreate()) 4348 throw new Error("Attempt to auto-create ClaimResponse.requestProvider"); 4349 else if (Configuration.doAutoCreate()) 4350 this.requestProviderTarget = new Practitioner(); // aa 4351 return this.requestProviderTarget; 4352 } 4353 4354 /** 4355 * @param value {@link #requestProvider} The actual object that is the target of 4356 * the reference. The reference library doesn't use these, but you 4357 * can use it to hold the resource if you resolve it. (The 4358 * practitioner who is responsible for the services rendered to the 4359 * patient.) 4360 */ 4361 public ClaimResponse setRequestProviderTarget(Practitioner value) { 4362 this.requestProviderTarget = value; 4363 return this; 4364 } 4365 4366 /** 4367 * @return {@link #requestOrganization} (The organization which is responsible 4368 * for the services rendered to the patient.) 4369 */ 4370 public Reference getRequestOrganization() { 4371 if (this.requestOrganization == null) 4372 if (Configuration.errorOnAutoCreate()) 4373 throw new Error("Attempt to auto-create ClaimResponse.requestOrganization"); 4374 else if (Configuration.doAutoCreate()) 4375 this.requestOrganization = new Reference(); // cc 4376 return this.requestOrganization; 4377 } 4378 4379 public boolean hasRequestOrganization() { 4380 return this.requestOrganization != null && !this.requestOrganization.isEmpty(); 4381 } 4382 4383 /** 4384 * @param value {@link #requestOrganization} (The organization which is 4385 * responsible for the services rendered to the patient.) 4386 */ 4387 public ClaimResponse setRequestOrganization(Reference value) { 4388 this.requestOrganization = value; 4389 return this; 4390 } 4391 4392 /** 4393 * @return {@link #requestOrganization} The actual object that is the target of 4394 * the reference. The reference library doesn't populate this, but you 4395 * can use it to hold the resource if you resolve it. (The organization 4396 * which is responsible for the services rendered to the patient.) 4397 */ 4398 public Organization getRequestOrganizationTarget() { 4399 if (this.requestOrganizationTarget == null) 4400 if (Configuration.errorOnAutoCreate()) 4401 throw new Error("Attempt to auto-create ClaimResponse.requestOrganization"); 4402 else if (Configuration.doAutoCreate()) 4403 this.requestOrganizationTarget = new Organization(); // aa 4404 return this.requestOrganizationTarget; 4405 } 4406 4407 /** 4408 * @param value {@link #requestOrganization} The actual object that is the 4409 * target of the reference. The reference library doesn't use 4410 * these, but you can use it to hold the resource if you resolve 4411 * it. (The organization which is responsible for the services 4412 * rendered to the patient.) 4413 */ 4414 public ClaimResponse setRequestOrganizationTarget(Organization value) { 4415 this.requestOrganizationTarget = value; 4416 return this; 4417 } 4418 4419 /** 4420 * @return {@link #outcome} (Transaction status: error, complete.). This is the 4421 * underlying object with id, value and extensions. The accessor 4422 * "getOutcome" gives direct access to the value 4423 */ 4424 public Enumeration<RemittanceOutcome> getOutcomeElement() { 4425 if (this.outcome == null) 4426 if (Configuration.errorOnAutoCreate()) 4427 throw new Error("Attempt to auto-create ClaimResponse.outcome"); 4428 else if (Configuration.doAutoCreate()) 4429 this.outcome = new Enumeration<RemittanceOutcome>(new RemittanceOutcomeEnumFactory()); // bb 4430 return this.outcome; 4431 } 4432 4433 public boolean hasOutcomeElement() { 4434 return this.outcome != null && !this.outcome.isEmpty(); 4435 } 4436 4437 public boolean hasOutcome() { 4438 return this.outcome != null && !this.outcome.isEmpty(); 4439 } 4440 4441 /** 4442 * @param value {@link #outcome} (Transaction status: error, complete.). This is 4443 * the underlying object with id, value and extensions. The 4444 * accessor "getOutcome" gives direct access to the value 4445 */ 4446 public ClaimResponse setOutcomeElement(Enumeration<RemittanceOutcome> value) { 4447 this.outcome = value; 4448 return this; 4449 } 4450 4451 /** 4452 * @return Transaction status: error, complete. 4453 */ 4454 public RemittanceOutcome getOutcome() { 4455 return this.outcome == null ? null : this.outcome.getValue(); 4456 } 4457 4458 /** 4459 * @param value Transaction status: error, complete. 4460 */ 4461 public ClaimResponse setOutcome(RemittanceOutcome value) { 4462 if (value == null) 4463 this.outcome = null; 4464 else { 4465 if (this.outcome == null) 4466 this.outcome = new Enumeration<RemittanceOutcome>(new RemittanceOutcomeEnumFactory()); 4467 this.outcome.setValue(value); 4468 } 4469 return this; 4470 } 4471 4472 /** 4473 * @return {@link #disposition} (A description of the status of the 4474 * adjudication.). This is the underlying object with id, value and 4475 * extensions. The accessor "getDisposition" gives direct access to the 4476 * value 4477 */ 4478 public StringType getDispositionElement() { 4479 if (this.disposition == null) 4480 if (Configuration.errorOnAutoCreate()) 4481 throw new Error("Attempt to auto-create ClaimResponse.disposition"); 4482 else if (Configuration.doAutoCreate()) 4483 this.disposition = new StringType(); // bb 4484 return this.disposition; 4485 } 4486 4487 public boolean hasDispositionElement() { 4488 return this.disposition != null && !this.disposition.isEmpty(); 4489 } 4490 4491 public boolean hasDisposition() { 4492 return this.disposition != null && !this.disposition.isEmpty(); 4493 } 4494 4495 /** 4496 * @param value {@link #disposition} (A description of the status of the 4497 * adjudication.). This is the underlying object with id, value and 4498 * extensions. The accessor "getDisposition" gives direct access to 4499 * the value 4500 */ 4501 public ClaimResponse setDispositionElement(StringType value) { 4502 this.disposition = value; 4503 return this; 4504 } 4505 4506 /** 4507 * @return A description of the status of the adjudication. 4508 */ 4509 public String getDisposition() { 4510 return this.disposition == null ? null : this.disposition.getValue(); 4511 } 4512 4513 /** 4514 * @param value A description of the status of the adjudication. 4515 */ 4516 public ClaimResponse setDisposition(String value) { 4517 if (Utilities.noString(value)) 4518 this.disposition = null; 4519 else { 4520 if (this.disposition == null) 4521 this.disposition = new StringType(); 4522 this.disposition.setValue(value); 4523 } 4524 return this; 4525 } 4526 4527 /** 4528 * @return {@link #payeeType} (Party to be reimbursed: Subscriber, provider, 4529 * other.) 4530 */ 4531 public Coding getPayeeType() { 4532 if (this.payeeType == null) 4533 if (Configuration.errorOnAutoCreate()) 4534 throw new Error("Attempt to auto-create ClaimResponse.payeeType"); 4535 else if (Configuration.doAutoCreate()) 4536 this.payeeType = new Coding(); // cc 4537 return this.payeeType; 4538 } 4539 4540 public boolean hasPayeeType() { 4541 return this.payeeType != null && !this.payeeType.isEmpty(); 4542 } 4543 4544 /** 4545 * @param value {@link #payeeType} (Party to be reimbursed: Subscriber, 4546 * provider, other.) 4547 */ 4548 public ClaimResponse setPayeeType(Coding value) { 4549 this.payeeType = value; 4550 return this; 4551 } 4552 4553 /** 4554 * @return {@link #item} (The first tier service adjudications for submitted 4555 * services.) 4556 */ 4557 public List<ItemsComponent> getItem() { 4558 if (this.item == null) 4559 this.item = new ArrayList<ItemsComponent>(); 4560 return this.item; 4561 } 4562 4563 public boolean hasItem() { 4564 if (this.item == null) 4565 return false; 4566 for (ItemsComponent item : this.item) 4567 if (!item.isEmpty()) 4568 return true; 4569 return false; 4570 } 4571 4572 /** 4573 * @return {@link #item} (The first tier service adjudications for submitted 4574 * services.) 4575 */ 4576 // syntactic sugar 4577 public ItemsComponent addItem() { // 3 4578 ItemsComponent t = new ItemsComponent(); 4579 if (this.item == null) 4580 this.item = new ArrayList<ItemsComponent>(); 4581 this.item.add(t); 4582 return t; 4583 } 4584 4585 // syntactic sugar 4586 public ClaimResponse addItem(ItemsComponent t) { // 3 4587 if (t == null) 4588 return this; 4589 if (this.item == null) 4590 this.item = new ArrayList<ItemsComponent>(); 4591 this.item.add(t); 4592 return this; 4593 } 4594 4595 /** 4596 * @return {@link #addItem} (The first tier service adjudications for payor 4597 * added services.) 4598 */ 4599 public List<AddedItemComponent> getAddItem() { 4600 if (this.addItem == null) 4601 this.addItem = new ArrayList<AddedItemComponent>(); 4602 return this.addItem; 4603 } 4604 4605 public boolean hasAddItem() { 4606 if (this.addItem == null) 4607 return false; 4608 for (AddedItemComponent item : this.addItem) 4609 if (!item.isEmpty()) 4610 return true; 4611 return false; 4612 } 4613 4614 /** 4615 * @return {@link #addItem} (The first tier service adjudications for payor 4616 * added services.) 4617 */ 4618 // syntactic sugar 4619 public AddedItemComponent addAddItem() { // 3 4620 AddedItemComponent t = new AddedItemComponent(); 4621 if (this.addItem == null) 4622 this.addItem = new ArrayList<AddedItemComponent>(); 4623 this.addItem.add(t); 4624 return t; 4625 } 4626 4627 // syntactic sugar 4628 public ClaimResponse addAddItem(AddedItemComponent t) { // 3 4629 if (t == null) 4630 return this; 4631 if (this.addItem == null) 4632 this.addItem = new ArrayList<AddedItemComponent>(); 4633 this.addItem.add(t); 4634 return this; 4635 } 4636 4637 /** 4638 * @return {@link #error} (Mutually exclusive with Services Provided (Item).) 4639 */ 4640 public List<ErrorsComponent> getError() { 4641 if (this.error == null) 4642 this.error = new ArrayList<ErrorsComponent>(); 4643 return this.error; 4644 } 4645 4646 public boolean hasError() { 4647 if (this.error == null) 4648 return false; 4649 for (ErrorsComponent item : this.error) 4650 if (!item.isEmpty()) 4651 return true; 4652 return false; 4653 } 4654 4655 /** 4656 * @return {@link #error} (Mutually exclusive with Services Provided (Item).) 4657 */ 4658 // syntactic sugar 4659 public ErrorsComponent addError() { // 3 4660 ErrorsComponent t = new ErrorsComponent(); 4661 if (this.error == null) 4662 this.error = new ArrayList<ErrorsComponent>(); 4663 this.error.add(t); 4664 return t; 4665 } 4666 4667 // syntactic sugar 4668 public ClaimResponse addError(ErrorsComponent t) { // 3 4669 if (t == null) 4670 return this; 4671 if (this.error == null) 4672 this.error = new ArrayList<ErrorsComponent>(); 4673 this.error.add(t); 4674 return this; 4675 } 4676 4677 /** 4678 * @return {@link #totalCost} (The total cost of the services reported.) 4679 */ 4680 public Money getTotalCost() { 4681 if (this.totalCost == null) 4682 if (Configuration.errorOnAutoCreate()) 4683 throw new Error("Attempt to auto-create ClaimResponse.totalCost"); 4684 else if (Configuration.doAutoCreate()) 4685 this.totalCost = new Money(); // cc 4686 return this.totalCost; 4687 } 4688 4689 public boolean hasTotalCost() { 4690 return this.totalCost != null && !this.totalCost.isEmpty(); 4691 } 4692 4693 /** 4694 * @param value {@link #totalCost} (The total cost of the services reported.) 4695 */ 4696 public ClaimResponse setTotalCost(Money value) { 4697 this.totalCost = value; 4698 return this; 4699 } 4700 4701 /** 4702 * @return {@link #unallocDeductable} (The amount of deductible applied which 4703 * was not allocated to any particular service line.) 4704 */ 4705 public Money getUnallocDeductable() { 4706 if (this.unallocDeductable == null) 4707 if (Configuration.errorOnAutoCreate()) 4708 throw new Error("Attempt to auto-create ClaimResponse.unallocDeductable"); 4709 else if (Configuration.doAutoCreate()) 4710 this.unallocDeductable = new Money(); // cc 4711 return this.unallocDeductable; 4712 } 4713 4714 public boolean hasUnallocDeductable() { 4715 return this.unallocDeductable != null && !this.unallocDeductable.isEmpty(); 4716 } 4717 4718 /** 4719 * @param value {@link #unallocDeductable} (The amount of deductible applied 4720 * which was not allocated to any particular service line.) 4721 */ 4722 public ClaimResponse setUnallocDeductable(Money value) { 4723 this.unallocDeductable = value; 4724 return this; 4725 } 4726 4727 /** 4728 * @return {@link #totalBenefit} (Total amount of benefit payable (Equal to sum 4729 * of the Benefit amounts from all detail lines and additions less the 4730 * Unallocated Deductible).) 4731 */ 4732 public Money getTotalBenefit() { 4733 if (this.totalBenefit == null) 4734 if (Configuration.errorOnAutoCreate()) 4735 throw new Error("Attempt to auto-create ClaimResponse.totalBenefit"); 4736 else if (Configuration.doAutoCreate()) 4737 this.totalBenefit = new Money(); // cc 4738 return this.totalBenefit; 4739 } 4740 4741 public boolean hasTotalBenefit() { 4742 return this.totalBenefit != null && !this.totalBenefit.isEmpty(); 4743 } 4744 4745 /** 4746 * @param value {@link #totalBenefit} (Total amount of benefit payable (Equal to 4747 * sum of the Benefit amounts from all detail lines and additions 4748 * less the Unallocated Deductible).) 4749 */ 4750 public ClaimResponse setTotalBenefit(Money value) { 4751 this.totalBenefit = value; 4752 return this; 4753 } 4754 4755 /** 4756 * @return {@link #paymentAdjustment} (Adjustment to the payment of this 4757 * transaction which is not related to adjudication of this 4758 * transaction.) 4759 */ 4760 public Money getPaymentAdjustment() { 4761 if (this.paymentAdjustment == null) 4762 if (Configuration.errorOnAutoCreate()) 4763 throw new Error("Attempt to auto-create ClaimResponse.paymentAdjustment"); 4764 else if (Configuration.doAutoCreate()) 4765 this.paymentAdjustment = new Money(); // cc 4766 return this.paymentAdjustment; 4767 } 4768 4769 public boolean hasPaymentAdjustment() { 4770 return this.paymentAdjustment != null && !this.paymentAdjustment.isEmpty(); 4771 } 4772 4773 /** 4774 * @param value {@link #paymentAdjustment} (Adjustment to the payment of this 4775 * transaction which is not related to adjudication of this 4776 * transaction.) 4777 */ 4778 public ClaimResponse setPaymentAdjustment(Money value) { 4779 this.paymentAdjustment = value; 4780 return this; 4781 } 4782 4783 /** 4784 * @return {@link #paymentAdjustmentReason} (Reason for the payment adjustment.) 4785 */ 4786 public Coding getPaymentAdjustmentReason() { 4787 if (this.paymentAdjustmentReason == null) 4788 if (Configuration.errorOnAutoCreate()) 4789 throw new Error("Attempt to auto-create ClaimResponse.paymentAdjustmentReason"); 4790 else if (Configuration.doAutoCreate()) 4791 this.paymentAdjustmentReason = new Coding(); // cc 4792 return this.paymentAdjustmentReason; 4793 } 4794 4795 public boolean hasPaymentAdjustmentReason() { 4796 return this.paymentAdjustmentReason != null && !this.paymentAdjustmentReason.isEmpty(); 4797 } 4798 4799 /** 4800 * @param value {@link #paymentAdjustmentReason} (Reason for the payment 4801 * adjustment.) 4802 */ 4803 public ClaimResponse setPaymentAdjustmentReason(Coding value) { 4804 this.paymentAdjustmentReason = value; 4805 return this; 4806 } 4807 4808 /** 4809 * @return {@link #paymentDate} (Estimated payment data.). This is the 4810 * underlying object with id, value and extensions. The accessor 4811 * "getPaymentDate" gives direct access to the value 4812 */ 4813 public DateType getPaymentDateElement() { 4814 if (this.paymentDate == null) 4815 if (Configuration.errorOnAutoCreate()) 4816 throw new Error("Attempt to auto-create ClaimResponse.paymentDate"); 4817 else if (Configuration.doAutoCreate()) 4818 this.paymentDate = new DateType(); // bb 4819 return this.paymentDate; 4820 } 4821 4822 public boolean hasPaymentDateElement() { 4823 return this.paymentDate != null && !this.paymentDate.isEmpty(); 4824 } 4825 4826 public boolean hasPaymentDate() { 4827 return this.paymentDate != null && !this.paymentDate.isEmpty(); 4828 } 4829 4830 /** 4831 * @param value {@link #paymentDate} (Estimated payment data.). This is the 4832 * underlying object with id, value and extensions. The accessor 4833 * "getPaymentDate" gives direct access to the value 4834 */ 4835 public ClaimResponse setPaymentDateElement(DateType value) { 4836 this.paymentDate = value; 4837 return this; 4838 } 4839 4840 /** 4841 * @return Estimated payment data. 4842 */ 4843 public Date getPaymentDate() { 4844 return this.paymentDate == null ? null : this.paymentDate.getValue(); 4845 } 4846 4847 /** 4848 * @param value Estimated payment data. 4849 */ 4850 public ClaimResponse setPaymentDate(Date value) { 4851 if (value == null) 4852 this.paymentDate = null; 4853 else { 4854 if (this.paymentDate == null) 4855 this.paymentDate = new DateType(); 4856 this.paymentDate.setValue(value); 4857 } 4858 return this; 4859 } 4860 4861 /** 4862 * @return {@link #paymentAmount} (Payable less any payment adjustment.) 4863 */ 4864 public Money getPaymentAmount() { 4865 if (this.paymentAmount == null) 4866 if (Configuration.errorOnAutoCreate()) 4867 throw new Error("Attempt to auto-create ClaimResponse.paymentAmount"); 4868 else if (Configuration.doAutoCreate()) 4869 this.paymentAmount = new Money(); // cc 4870 return this.paymentAmount; 4871 } 4872 4873 public boolean hasPaymentAmount() { 4874 return this.paymentAmount != null && !this.paymentAmount.isEmpty(); 4875 } 4876 4877 /** 4878 * @param value {@link #paymentAmount} (Payable less any payment adjustment.) 4879 */ 4880 public ClaimResponse setPaymentAmount(Money value) { 4881 this.paymentAmount = value; 4882 return this; 4883 } 4884 4885 /** 4886 * @return {@link #paymentRef} (Payment identifier.) 4887 */ 4888 public Identifier getPaymentRef() { 4889 if (this.paymentRef == null) 4890 if (Configuration.errorOnAutoCreate()) 4891 throw new Error("Attempt to auto-create ClaimResponse.paymentRef"); 4892 else if (Configuration.doAutoCreate()) 4893 this.paymentRef = new Identifier(); // cc 4894 return this.paymentRef; 4895 } 4896 4897 public boolean hasPaymentRef() { 4898 return this.paymentRef != null && !this.paymentRef.isEmpty(); 4899 } 4900 4901 /** 4902 * @param value {@link #paymentRef} (Payment identifier.) 4903 */ 4904 public ClaimResponse setPaymentRef(Identifier value) { 4905 this.paymentRef = value; 4906 return this; 4907 } 4908 4909 /** 4910 * @return {@link #reserved} (Status of funds reservation (For provider, for 4911 * Patient, None).) 4912 */ 4913 public Coding getReserved() { 4914 if (this.reserved == null) 4915 if (Configuration.errorOnAutoCreate()) 4916 throw new Error("Attempt to auto-create ClaimResponse.reserved"); 4917 else if (Configuration.doAutoCreate()) 4918 this.reserved = new Coding(); // cc 4919 return this.reserved; 4920 } 4921 4922 public boolean hasReserved() { 4923 return this.reserved != null && !this.reserved.isEmpty(); 4924 } 4925 4926 /** 4927 * @param value {@link #reserved} (Status of funds reservation (For provider, 4928 * for Patient, None).) 4929 */ 4930 public ClaimResponse setReserved(Coding value) { 4931 this.reserved = value; 4932 return this; 4933 } 4934 4935 /** 4936 * @return {@link #form} (The form to be used for printing the content.) 4937 */ 4938 public Coding getForm() { 4939 if (this.form == null) 4940 if (Configuration.errorOnAutoCreate()) 4941 throw new Error("Attempt to auto-create ClaimResponse.form"); 4942 else if (Configuration.doAutoCreate()) 4943 this.form = new Coding(); // cc 4944 return this.form; 4945 } 4946 4947 public boolean hasForm() { 4948 return this.form != null && !this.form.isEmpty(); 4949 } 4950 4951 /** 4952 * @param value {@link #form} (The form to be used for printing the content.) 4953 */ 4954 public ClaimResponse setForm(Coding value) { 4955 this.form = value; 4956 return this; 4957 } 4958 4959 /** 4960 * @return {@link #note} (Note text.) 4961 */ 4962 public List<NotesComponent> getNote() { 4963 if (this.note == null) 4964 this.note = new ArrayList<NotesComponent>(); 4965 return this.note; 4966 } 4967 4968 public boolean hasNote() { 4969 if (this.note == null) 4970 return false; 4971 for (NotesComponent item : this.note) 4972 if (!item.isEmpty()) 4973 return true; 4974 return false; 4975 } 4976 4977 /** 4978 * @return {@link #note} (Note text.) 4979 */ 4980 // syntactic sugar 4981 public NotesComponent addNote() { // 3 4982 NotesComponent t = new NotesComponent(); 4983 if (this.note == null) 4984 this.note = new ArrayList<NotesComponent>(); 4985 this.note.add(t); 4986 return t; 4987 } 4988 4989 // syntactic sugar 4990 public ClaimResponse addNote(NotesComponent t) { // 3 4991 if (t == null) 4992 return this; 4993 if (this.note == null) 4994 this.note = new ArrayList<NotesComponent>(); 4995 this.note.add(t); 4996 return this; 4997 } 4998 4999 /** 5000 * @return {@link #coverage} (Financial instrument by which payment information 5001 * for health care.) 5002 */ 5003 public List<CoverageComponent> getCoverage() { 5004 if (this.coverage == null) 5005 this.coverage = new ArrayList<CoverageComponent>(); 5006 return this.coverage; 5007 } 5008 5009 public boolean hasCoverage() { 5010 if (this.coverage == null) 5011 return false; 5012 for (CoverageComponent item : this.coverage) 5013 if (!item.isEmpty()) 5014 return true; 5015 return false; 5016 } 5017 5018 /** 5019 * @return {@link #coverage} (Financial instrument by which payment information 5020 * for health care.) 5021 */ 5022 // syntactic sugar 5023 public CoverageComponent addCoverage() { // 3 5024 CoverageComponent t = new CoverageComponent(); 5025 if (this.coverage == null) 5026 this.coverage = new ArrayList<CoverageComponent>(); 5027 this.coverage.add(t); 5028 return t; 5029 } 5030 5031 // syntactic sugar 5032 public ClaimResponse addCoverage(CoverageComponent t) { // 3 5033 if (t == null) 5034 return this; 5035 if (this.coverage == null) 5036 this.coverage = new ArrayList<CoverageComponent>(); 5037 this.coverage.add(t); 5038 return this; 5039 } 5040 5041 protected void listChildren(List<Property> childrenList) { 5042 super.listChildren(childrenList); 5043 childrenList.add(new Property("identifier", "Identifier", "The Response business identifier.", 0, 5044 java.lang.Integer.MAX_VALUE, identifier)); 5045 childrenList.add(new Property("request", "Reference(Claim)", "Original request resource referrence.", 0, 5046 java.lang.Integer.MAX_VALUE, request)); 5047 childrenList.add(new Property("ruleset", "Coding", 5048 "The version of the style of resource contents. This should be mapped to the allowable profiles for this and supporting resources.", 5049 0, java.lang.Integer.MAX_VALUE, ruleset)); 5050 childrenList.add(new Property("originalRuleset", "Coding", 5051 "The style (standard) and version of the original material which was converted into this resource.", 0, 5052 java.lang.Integer.MAX_VALUE, originalRuleset)); 5053 childrenList.add( 5054 new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 5055 0, java.lang.Integer.MAX_VALUE, created)); 5056 childrenList.add(new Property("organization", "Reference(Organization)", 5057 "The Insurer who produced this adjudicated response.", 0, java.lang.Integer.MAX_VALUE, organization)); 5058 childrenList.add(new Property("requestProvider", "Reference(Practitioner)", 5059 "The practitioner who is responsible for the services rendered to the patient.", 0, java.lang.Integer.MAX_VALUE, 5060 requestProvider)); 5061 childrenList.add(new Property("requestOrganization", "Reference(Organization)", 5062 "The organization which is responsible for the services rendered to the patient.", 0, 5063 java.lang.Integer.MAX_VALUE, requestOrganization)); 5064 childrenList.add(new Property("outcome", "code", "Transaction status: error, complete.", 0, 5065 java.lang.Integer.MAX_VALUE, outcome)); 5066 childrenList.add(new Property("disposition", "string", "A description of the status of the adjudication.", 0, 5067 java.lang.Integer.MAX_VALUE, disposition)); 5068 childrenList.add(new Property("payeeType", "Coding", "Party to be reimbursed: Subscriber, provider, other.", 0, 5069 java.lang.Integer.MAX_VALUE, payeeType)); 5070 childrenList.add(new Property("item", "", "The first tier service adjudications for submitted services.", 0, 5071 java.lang.Integer.MAX_VALUE, item)); 5072 childrenList.add(new Property("addItem", "", "The first tier service adjudications for payor added services.", 0, 5073 java.lang.Integer.MAX_VALUE, addItem)); 5074 childrenList.add(new Property("error", "", "Mutually exclusive with Services Provided (Item).", 0, 5075 java.lang.Integer.MAX_VALUE, error)); 5076 childrenList.add(new Property("totalCost", "Money", "The total cost of the services reported.", 0, 5077 java.lang.Integer.MAX_VALUE, totalCost)); 5078 childrenList.add(new Property("unallocDeductable", "Money", 5079 "The amount of deductible applied which was not allocated to any particular service line.", 0, 5080 java.lang.Integer.MAX_VALUE, unallocDeductable)); 5081 childrenList.add(new Property("totalBenefit", "Money", 5082 "Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductible).", 5083 0, java.lang.Integer.MAX_VALUE, totalBenefit)); 5084 childrenList.add(new Property("paymentAdjustment", "Money", 5085 "Adjustment to the payment of this transaction which is not related to adjudication of this transaction.", 0, 5086 java.lang.Integer.MAX_VALUE, paymentAdjustment)); 5087 childrenList.add(new Property("paymentAdjustmentReason", "Coding", "Reason for the payment adjustment.", 0, 5088 java.lang.Integer.MAX_VALUE, paymentAdjustmentReason)); 5089 childrenList.add( 5090 new Property("paymentDate", "date", "Estimated payment data.", 0, java.lang.Integer.MAX_VALUE, paymentDate)); 5091 childrenList.add(new Property("paymentAmount", "Money", "Payable less any payment adjustment.", 0, 5092 java.lang.Integer.MAX_VALUE, paymentAmount)); 5093 childrenList.add( 5094 new Property("paymentRef", "Identifier", "Payment identifier.", 0, java.lang.Integer.MAX_VALUE, paymentRef)); 5095 childrenList.add(new Property("reserved", "Coding", 5096 "Status of funds reservation (For provider, for Patient, None).", 0, java.lang.Integer.MAX_VALUE, reserved)); 5097 childrenList.add(new Property("form", "Coding", "The form to be used for printing the content.", 0, 5098 java.lang.Integer.MAX_VALUE, form)); 5099 childrenList.add(new Property("note", "", "Note text.", 0, java.lang.Integer.MAX_VALUE, note)); 5100 childrenList.add(new Property("coverage", "", "Financial instrument by which payment information for health care.", 5101 0, java.lang.Integer.MAX_VALUE, coverage)); 5102 } 5103 5104 @Override 5105 public void setProperty(String name, Base value) throws FHIRException { 5106 if (name.equals("identifier")) 5107 this.getIdentifier().add(castToIdentifier(value)); 5108 else if (name.equals("request")) 5109 this.request = castToReference(value); // Reference 5110 else if (name.equals("ruleset")) 5111 this.ruleset = castToCoding(value); // Coding 5112 else if (name.equals("originalRuleset")) 5113 this.originalRuleset = castToCoding(value); // Coding 5114 else if (name.equals("created")) 5115 this.created = castToDateTime(value); // DateTimeType 5116 else if (name.equals("organization")) 5117 this.organization = castToReference(value); // Reference 5118 else if (name.equals("requestProvider")) 5119 this.requestProvider = castToReference(value); // Reference 5120 else if (name.equals("requestOrganization")) 5121 this.requestOrganization = castToReference(value); // Reference 5122 else if (name.equals("outcome")) 5123 this.outcome = new RemittanceOutcomeEnumFactory().fromType(value); // Enumeration<RemittanceOutcome> 5124 else if (name.equals("disposition")) 5125 this.disposition = castToString(value); // StringType 5126 else if (name.equals("payeeType")) 5127 this.payeeType = castToCoding(value); // Coding 5128 else if (name.equals("item")) 5129 this.getItem().add((ItemsComponent) value); 5130 else if (name.equals("addItem")) 5131 this.getAddItem().add((AddedItemComponent) value); 5132 else if (name.equals("error")) 5133 this.getError().add((ErrorsComponent) value); 5134 else if (name.equals("totalCost")) 5135 this.totalCost = castToMoney(value); // Money 5136 else if (name.equals("unallocDeductable")) 5137 this.unallocDeductable = castToMoney(value); // Money 5138 else if (name.equals("totalBenefit")) 5139 this.totalBenefit = castToMoney(value); // Money 5140 else if (name.equals("paymentAdjustment")) 5141 this.paymentAdjustment = castToMoney(value); // Money 5142 else if (name.equals("paymentAdjustmentReason")) 5143 this.paymentAdjustmentReason = castToCoding(value); // Coding 5144 else if (name.equals("paymentDate")) 5145 this.paymentDate = castToDate(value); // DateType 5146 else if (name.equals("paymentAmount")) 5147 this.paymentAmount = castToMoney(value); // Money 5148 else if (name.equals("paymentRef")) 5149 this.paymentRef = castToIdentifier(value); // Identifier 5150 else if (name.equals("reserved")) 5151 this.reserved = castToCoding(value); // Coding 5152 else if (name.equals("form")) 5153 this.form = castToCoding(value); // Coding 5154 else if (name.equals("note")) 5155 this.getNote().add((NotesComponent) value); 5156 else if (name.equals("coverage")) 5157 this.getCoverage().add((CoverageComponent) value); 5158 else 5159 super.setProperty(name, value); 5160 } 5161 5162 @Override 5163 public Base addChild(String name) throws FHIRException { 5164 if (name.equals("identifier")) { 5165 return addIdentifier(); 5166 } else if (name.equals("request")) { 5167 this.request = new Reference(); 5168 return this.request; 5169 } else if (name.equals("ruleset")) { 5170 this.ruleset = new Coding(); 5171 return this.ruleset; 5172 } else if (name.equals("originalRuleset")) { 5173 this.originalRuleset = new Coding(); 5174 return this.originalRuleset; 5175 } else if (name.equals("created")) { 5176 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.created"); 5177 } else if (name.equals("organization")) { 5178 this.organization = new Reference(); 5179 return this.organization; 5180 } else if (name.equals("requestProvider")) { 5181 this.requestProvider = new Reference(); 5182 return this.requestProvider; 5183 } else if (name.equals("requestOrganization")) { 5184 this.requestOrganization = new Reference(); 5185 return this.requestOrganization; 5186 } else if (name.equals("outcome")) { 5187 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.outcome"); 5188 } else if (name.equals("disposition")) { 5189 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.disposition"); 5190 } else if (name.equals("payeeType")) { 5191 this.payeeType = new Coding(); 5192 return this.payeeType; 5193 } else if (name.equals("item")) { 5194 return addItem(); 5195 } else if (name.equals("addItem")) { 5196 return addAddItem(); 5197 } else if (name.equals("error")) { 5198 return addError(); 5199 } else if (name.equals("totalCost")) { 5200 this.totalCost = new Money(); 5201 return this.totalCost; 5202 } else if (name.equals("unallocDeductable")) { 5203 this.unallocDeductable = new Money(); 5204 return this.unallocDeductable; 5205 } else if (name.equals("totalBenefit")) { 5206 this.totalBenefit = new Money(); 5207 return this.totalBenefit; 5208 } else if (name.equals("paymentAdjustment")) { 5209 this.paymentAdjustment = new Money(); 5210 return this.paymentAdjustment; 5211 } else if (name.equals("paymentAdjustmentReason")) { 5212 this.paymentAdjustmentReason = new Coding(); 5213 return this.paymentAdjustmentReason; 5214 } else if (name.equals("paymentDate")) { 5215 throw new FHIRException("Cannot call addChild on a singleton property ClaimResponse.paymentDate"); 5216 } else if (name.equals("paymentAmount")) { 5217 this.paymentAmount = new Money(); 5218 return this.paymentAmount; 5219 } else if (name.equals("paymentRef")) { 5220 this.paymentRef = new Identifier(); 5221 return this.paymentRef; 5222 } else if (name.equals("reserved")) { 5223 this.reserved = new Coding(); 5224 return this.reserved; 5225 } else if (name.equals("form")) { 5226 this.form = new Coding(); 5227 return this.form; 5228 } else if (name.equals("note")) { 5229 return addNote(); 5230 } else if (name.equals("coverage")) { 5231 return addCoverage(); 5232 } else 5233 return super.addChild(name); 5234 } 5235 5236 public String fhirType() { 5237 return "ClaimResponse"; 5238 5239 } 5240 5241 public ClaimResponse copy() { 5242 ClaimResponse dst = new ClaimResponse(); 5243 copyValues(dst); 5244 if (identifier != null) { 5245 dst.identifier = new ArrayList<Identifier>(); 5246 for (Identifier i : identifier) 5247 dst.identifier.add(i.copy()); 5248 } 5249 ; 5250 dst.request = request == null ? null : request.copy(); 5251 dst.ruleset = ruleset == null ? null : ruleset.copy(); 5252 dst.originalRuleset = originalRuleset == null ? null : originalRuleset.copy(); 5253 dst.created = created == null ? null : created.copy(); 5254 dst.organization = organization == null ? null : organization.copy(); 5255 dst.requestProvider = requestProvider == null ? null : requestProvider.copy(); 5256 dst.requestOrganization = requestOrganization == null ? null : requestOrganization.copy(); 5257 dst.outcome = outcome == null ? null : outcome.copy(); 5258 dst.disposition = disposition == null ? null : disposition.copy(); 5259 dst.payeeType = payeeType == null ? null : payeeType.copy(); 5260 if (item != null) { 5261 dst.item = new ArrayList<ItemsComponent>(); 5262 for (ItemsComponent i : item) 5263 dst.item.add(i.copy()); 5264 } 5265 ; 5266 if (addItem != null) { 5267 dst.addItem = new ArrayList<AddedItemComponent>(); 5268 for (AddedItemComponent i : addItem) 5269 dst.addItem.add(i.copy()); 5270 } 5271 ; 5272 if (error != null) { 5273 dst.error = new ArrayList<ErrorsComponent>(); 5274 for (ErrorsComponent i : error) 5275 dst.error.add(i.copy()); 5276 } 5277 ; 5278 dst.totalCost = totalCost == null ? null : totalCost.copy(); 5279 dst.unallocDeductable = unallocDeductable == null ? null : unallocDeductable.copy(); 5280 dst.totalBenefit = totalBenefit == null ? null : totalBenefit.copy(); 5281 dst.paymentAdjustment = paymentAdjustment == null ? null : paymentAdjustment.copy(); 5282 dst.paymentAdjustmentReason = paymentAdjustmentReason == null ? null : paymentAdjustmentReason.copy(); 5283 dst.paymentDate = paymentDate == null ? null : paymentDate.copy(); 5284 dst.paymentAmount = paymentAmount == null ? null : paymentAmount.copy(); 5285 dst.paymentRef = paymentRef == null ? null : paymentRef.copy(); 5286 dst.reserved = reserved == null ? null : reserved.copy(); 5287 dst.form = form == null ? null : form.copy(); 5288 if (note != null) { 5289 dst.note = new ArrayList<NotesComponent>(); 5290 for (NotesComponent i : note) 5291 dst.note.add(i.copy()); 5292 } 5293 ; 5294 if (coverage != null) { 5295 dst.coverage = new ArrayList<CoverageComponent>(); 5296 for (CoverageComponent i : coverage) 5297 dst.coverage.add(i.copy()); 5298 } 5299 ; 5300 return dst; 5301 } 5302 5303 protected ClaimResponse typedCopy() { 5304 return copy(); 5305 } 5306 5307 @Override 5308 public boolean equalsDeep(Base other) { 5309 if (!super.equalsDeep(other)) 5310 return false; 5311 if (!(other instanceof ClaimResponse)) 5312 return false; 5313 ClaimResponse o = (ClaimResponse) other; 5314 return compareDeep(identifier, o.identifier, true) && compareDeep(request, o.request, true) 5315 && compareDeep(ruleset, o.ruleset, true) && compareDeep(originalRuleset, o.originalRuleset, true) 5316 && compareDeep(created, o.created, true) && compareDeep(organization, o.organization, true) 5317 && compareDeep(requestProvider, o.requestProvider, true) 5318 && compareDeep(requestOrganization, o.requestOrganization, true) && compareDeep(outcome, o.outcome, true) 5319 && compareDeep(disposition, o.disposition, true) && compareDeep(payeeType, o.payeeType, true) 5320 && compareDeep(item, o.item, true) && compareDeep(addItem, o.addItem, true) && compareDeep(error, o.error, true) 5321 && compareDeep(totalCost, o.totalCost, true) && compareDeep(unallocDeductable, o.unallocDeductable, true) 5322 && compareDeep(totalBenefit, o.totalBenefit, true) && compareDeep(paymentAdjustment, o.paymentAdjustment, true) 5323 && compareDeep(paymentAdjustmentReason, o.paymentAdjustmentReason, true) 5324 && compareDeep(paymentDate, o.paymentDate, true) && compareDeep(paymentAmount, o.paymentAmount, true) 5325 && compareDeep(paymentRef, o.paymentRef, true) && compareDeep(reserved, o.reserved, true) 5326 && compareDeep(form, o.form, true) && compareDeep(note, o.note, true) 5327 && compareDeep(coverage, o.coverage, true); 5328 } 5329 5330 @Override 5331 public boolean equalsShallow(Base other) { 5332 if (!super.equalsShallow(other)) 5333 return false; 5334 if (!(other instanceof ClaimResponse)) 5335 return false; 5336 ClaimResponse o = (ClaimResponse) other; 5337 return compareValues(created, o.created, true) && compareValues(outcome, o.outcome, true) 5338 && compareValues(disposition, o.disposition, true) && compareValues(paymentDate, o.paymentDate, true); 5339 } 5340 5341 public boolean isEmpty() { 5342 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (request == null || request.isEmpty()) 5343 && (ruleset == null || ruleset.isEmpty()) && (originalRuleset == null || originalRuleset.isEmpty()) 5344 && (created == null || created.isEmpty()) && (organization == null || organization.isEmpty()) 5345 && (requestProvider == null || requestProvider.isEmpty()) 5346 && (requestOrganization == null || requestOrganization.isEmpty()) && (outcome == null || outcome.isEmpty()) 5347 && (disposition == null || disposition.isEmpty()) && (payeeType == null || payeeType.isEmpty()) 5348 && (item == null || item.isEmpty()) && (addItem == null || addItem.isEmpty()) 5349 && (error == null || error.isEmpty()) && (totalCost == null || totalCost.isEmpty()) 5350 && (unallocDeductable == null || unallocDeductable.isEmpty()) 5351 && (totalBenefit == null || totalBenefit.isEmpty()) 5352 && (paymentAdjustment == null || paymentAdjustment.isEmpty()) 5353 && (paymentAdjustmentReason == null || paymentAdjustmentReason.isEmpty()) 5354 && (paymentDate == null || paymentDate.isEmpty()) && (paymentAmount == null || paymentAmount.isEmpty()) 5355 && (paymentRef == null || paymentRef.isEmpty()) && (reserved == null || reserved.isEmpty()) 5356 && (form == null || form.isEmpty()) && (note == null || note.isEmpty()) 5357 && (coverage == null || coverage.isEmpty()); 5358 } 5359 5360 @Override 5361 public ResourceType getResourceType() { 5362 return ResourceType.ClaimResponse; 5363 } 5364 5365 @SearchParamDefinition(name = "identifier", path = "ClaimResponse.identifier", description = "The identity of the insurer", type = "token") 5366 public static final String SP_IDENTIFIER = "identifier"; 5367 5368}