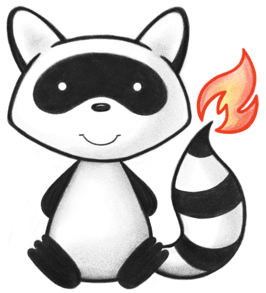
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044import org.hl7.fhir.utilities.Utilities; 045 046/** 047 * A record of a clinical assessment performed to determine what problem(s) may 048 * affect the patient and before planning the treatments or management 049 * strategies that are best to manage a patient's condition. Assessments are 050 * often 1:1 with a clinical consultation / encounter, but this varies greatly 051 * depending on the clinical workflow. This resource is called 052 * "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with 053 * the recording of assessment tools such as Apgar score. 054 */ 055@ResourceDef(name = "ClinicalImpression", profile = "http://hl7.org/fhir/Profile/ClinicalImpression") 056public class ClinicalImpression extends DomainResource { 057 058 public enum ClinicalImpressionStatus { 059 /** 060 * The assessment is still on-going and results are not yet final. 061 */ 062 INPROGRESS, 063 /** 064 * The assessment is done and the results are final. 065 */ 066 COMPLETED, 067 /** 068 * This assessment was never actually done and the record is erroneous (e.g. 069 * Wrong patient). 070 */ 071 ENTEREDINERROR, 072 /** 073 * added to help the parsers 074 */ 075 NULL; 076 077 public static ClinicalImpressionStatus fromCode(String codeString) throws FHIRException { 078 if (codeString == null || "".equals(codeString)) 079 return null; 080 if ("in-progress".equals(codeString)) 081 return INPROGRESS; 082 if ("completed".equals(codeString)) 083 return COMPLETED; 084 if ("entered-in-error".equals(codeString)) 085 return ENTEREDINERROR; 086 throw new FHIRException("Unknown ClinicalImpressionStatus code '" + codeString + "'"); 087 } 088 089 public String toCode() { 090 switch (this) { 091 case INPROGRESS: 092 return "in-progress"; 093 case COMPLETED: 094 return "completed"; 095 case ENTEREDINERROR: 096 return "entered-in-error"; 097 case NULL: 098 return null; 099 default: 100 return "?"; 101 } 102 } 103 104 public String getSystem() { 105 switch (this) { 106 case INPROGRESS: 107 return "http://hl7.org/fhir/clinical-impression-status"; 108 case COMPLETED: 109 return "http://hl7.org/fhir/clinical-impression-status"; 110 case ENTEREDINERROR: 111 return "http://hl7.org/fhir/clinical-impression-status"; 112 case NULL: 113 return null; 114 default: 115 return "?"; 116 } 117 } 118 119 public String getDefinition() { 120 switch (this) { 121 case INPROGRESS: 122 return "The assessment is still on-going and results are not yet final."; 123 case COMPLETED: 124 return "The assessment is done and the results are final."; 125 case ENTEREDINERROR: 126 return "This assessment was never actually done and the record is erroneous (e.g. Wrong patient)."; 127 case NULL: 128 return null; 129 default: 130 return "?"; 131 } 132 } 133 134 public String getDisplay() { 135 switch (this) { 136 case INPROGRESS: 137 return "In progress"; 138 case COMPLETED: 139 return "Completed"; 140 case ENTEREDINERROR: 141 return "Entered in Error"; 142 case NULL: 143 return null; 144 default: 145 return "?"; 146 } 147 } 148 } 149 150 public static class ClinicalImpressionStatusEnumFactory implements EnumFactory<ClinicalImpressionStatus> { 151 public ClinicalImpressionStatus fromCode(String codeString) throws IllegalArgumentException { 152 if (codeString == null || "".equals(codeString)) 153 if (codeString == null || "".equals(codeString)) 154 return null; 155 if ("in-progress".equals(codeString)) 156 return ClinicalImpressionStatus.INPROGRESS; 157 if ("completed".equals(codeString)) 158 return ClinicalImpressionStatus.COMPLETED; 159 if ("entered-in-error".equals(codeString)) 160 return ClinicalImpressionStatus.ENTEREDINERROR; 161 throw new IllegalArgumentException("Unknown ClinicalImpressionStatus code '" + codeString + "'"); 162 } 163 164 public Enumeration<ClinicalImpressionStatus> fromType(Base code) throws FHIRException { 165 if (code == null || code.isEmpty()) 166 return null; 167 String codeString = ((PrimitiveType) code).asStringValue(); 168 if (codeString == null || "".equals(codeString)) 169 return null; 170 if ("in-progress".equals(codeString)) 171 return new Enumeration<ClinicalImpressionStatus>(this, ClinicalImpressionStatus.INPROGRESS); 172 if ("completed".equals(codeString)) 173 return new Enumeration<ClinicalImpressionStatus>(this, ClinicalImpressionStatus.COMPLETED); 174 if ("entered-in-error".equals(codeString)) 175 return new Enumeration<ClinicalImpressionStatus>(this, ClinicalImpressionStatus.ENTEREDINERROR); 176 throw new FHIRException("Unknown ClinicalImpressionStatus code '" + codeString + "'"); 177 } 178 179 public String toCode(ClinicalImpressionStatus code) 180 { 181 if (code == ClinicalImpressionStatus.NULL) 182 return null; 183 if (code == ClinicalImpressionStatus.INPROGRESS) 184 return "in-progress"; 185 if (code == ClinicalImpressionStatus.COMPLETED) 186 return "completed"; 187 if (code == ClinicalImpressionStatus.ENTEREDINERROR) 188 return "entered-in-error"; 189 return "?"; 190 } 191 } 192 193 @Block() 194 public static class ClinicalImpressionInvestigationsComponent extends BackboneElement 195 implements IBaseBackboneElement { 196 /** 197 * A name/code for the group ("set") of investigations. Typically, this will be 198 * something like "signs", "symptoms", "clinical", "diagnostic", but the list is 199 * not constrained, and others such groups such as 200 * (exposure|family|travel|nutitirional) history may be used. 201 */ 202 @Child(name = "code", type = { 203 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 204 @Description(shortDefinition = "A name/code for the set", formalDefinition = "A name/code for the group (\"set\") of investigations. Typically, this will be something like \"signs\", \"symptoms\", \"clinical\", \"diagnostic\", but the list is not constrained, and others such groups such as (exposure|family|travel|nutitirional) history may be used.") 205 protected CodeableConcept code; 206 207 /** 208 * A record of a specific investigation that was undertaken. 209 */ 210 @Child(name = "item", type = { Observation.class, QuestionnaireResponse.class, FamilyMemberHistory.class, 211 DiagnosticReport.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 212 @Description(shortDefinition = "Record of a specific investigation", formalDefinition = "A record of a specific investigation that was undertaken.") 213 protected List<Reference> item; 214 /** 215 * The actual objects that are the target of the reference (A record of a 216 * specific investigation that was undertaken.) 217 */ 218 protected List<Resource> itemTarget; 219 220 private static final long serialVersionUID = -301363326L; 221 222 /* 223 * Constructor 224 */ 225 public ClinicalImpressionInvestigationsComponent() { 226 super(); 227 } 228 229 /* 230 * Constructor 231 */ 232 public ClinicalImpressionInvestigationsComponent(CodeableConcept code) { 233 super(); 234 this.code = code; 235 } 236 237 /** 238 * @return {@link #code} (A name/code for the group ("set") of investigations. 239 * Typically, this will be something like "signs", "symptoms", 240 * "clinical", "diagnostic", but the list is not constrained, and others 241 * such groups such as (exposure|family|travel|nutitirional) history may 242 * be used.) 243 */ 244 public CodeableConcept getCode() { 245 if (this.code == null) 246 if (Configuration.errorOnAutoCreate()) 247 throw new Error("Attempt to auto-create ClinicalImpressionInvestigationsComponent.code"); 248 else if (Configuration.doAutoCreate()) 249 this.code = new CodeableConcept(); // cc 250 return this.code; 251 } 252 253 public boolean hasCode() { 254 return this.code != null && !this.code.isEmpty(); 255 } 256 257 /** 258 * @param value {@link #code} (A name/code for the group ("set") of 259 * investigations. Typically, this will be something like "signs", 260 * "symptoms", "clinical", "diagnostic", but the list is not 261 * constrained, and others such groups such as 262 * (exposure|family|travel|nutitirional) history may be used.) 263 */ 264 public ClinicalImpressionInvestigationsComponent setCode(CodeableConcept value) { 265 this.code = value; 266 return this; 267 } 268 269 /** 270 * @return {@link #item} (A record of a specific investigation that was 271 * undertaken.) 272 */ 273 public List<Reference> getItem() { 274 if (this.item == null) 275 this.item = new ArrayList<Reference>(); 276 return this.item; 277 } 278 279 public boolean hasItem() { 280 if (this.item == null) 281 return false; 282 for (Reference item : this.item) 283 if (!item.isEmpty()) 284 return true; 285 return false; 286 } 287 288 /** 289 * @return {@link #item} (A record of a specific investigation that was 290 * undertaken.) 291 */ 292 // syntactic sugar 293 public Reference addItem() { // 3 294 Reference t = new Reference(); 295 if (this.item == null) 296 this.item = new ArrayList<Reference>(); 297 this.item.add(t); 298 return t; 299 } 300 301 // syntactic sugar 302 public ClinicalImpressionInvestigationsComponent addItem(Reference t) { // 3 303 if (t == null) 304 return this; 305 if (this.item == null) 306 this.item = new ArrayList<Reference>(); 307 this.item.add(t); 308 return this; 309 } 310 311 /** 312 * @return {@link #item} (The actual objects that are the target of the 313 * reference. The reference library doesn't populate this, but you can 314 * use this to hold the resources if you resolvethemt. A record of a 315 * specific investigation that was undertaken.) 316 */ 317 public List<Resource> getItemTarget() { 318 if (this.itemTarget == null) 319 this.itemTarget = new ArrayList<Resource>(); 320 return this.itemTarget; 321 } 322 323 protected void listChildren(List<Property> childrenList) { 324 super.listChildren(childrenList); 325 childrenList.add(new Property("code", "CodeableConcept", 326 "A name/code for the group (\"set\") of investigations. Typically, this will be something like \"signs\", \"symptoms\", \"clinical\", \"diagnostic\", but the list is not constrained, and others such groups such as (exposure|family|travel|nutitirional) history may be used.", 327 0, java.lang.Integer.MAX_VALUE, code)); 328 childrenList 329 .add(new Property("item", "Reference(Observation|QuestionnaireResponse|FamilyMemberHistory|DiagnosticReport)", 330 "A record of a specific investigation that was undertaken.", 0, java.lang.Integer.MAX_VALUE, item)); 331 } 332 333 @Override 334 public void setProperty(String name, Base value) throws FHIRException { 335 if (name.equals("code")) 336 this.code = castToCodeableConcept(value); // CodeableConcept 337 else if (name.equals("item")) 338 this.getItem().add(castToReference(value)); 339 else 340 super.setProperty(name, value); 341 } 342 343 @Override 344 public Base addChild(String name) throws FHIRException { 345 if (name.equals("code")) { 346 this.code = new CodeableConcept(); 347 return this.code; 348 } else if (name.equals("item")) { 349 return addItem(); 350 } else 351 return super.addChild(name); 352 } 353 354 public ClinicalImpressionInvestigationsComponent copy() { 355 ClinicalImpressionInvestigationsComponent dst = new ClinicalImpressionInvestigationsComponent(); 356 copyValues(dst); 357 dst.code = code == null ? null : code.copy(); 358 if (item != null) { 359 dst.item = new ArrayList<Reference>(); 360 for (Reference i : item) 361 dst.item.add(i.copy()); 362 } 363 ; 364 return dst; 365 } 366 367 @Override 368 public boolean equalsDeep(Base other) { 369 if (!super.equalsDeep(other)) 370 return false; 371 if (!(other instanceof ClinicalImpressionInvestigationsComponent)) 372 return false; 373 ClinicalImpressionInvestigationsComponent o = (ClinicalImpressionInvestigationsComponent) other; 374 return compareDeep(code, o.code, true) && compareDeep(item, o.item, true); 375 } 376 377 @Override 378 public boolean equalsShallow(Base other) { 379 if (!super.equalsShallow(other)) 380 return false; 381 if (!(other instanceof ClinicalImpressionInvestigationsComponent)) 382 return false; 383 ClinicalImpressionInvestigationsComponent o = (ClinicalImpressionInvestigationsComponent) other; 384 return true; 385 } 386 387 public boolean isEmpty() { 388 return super.isEmpty() && (code == null || code.isEmpty()) && (item == null || item.isEmpty()); 389 } 390 391 public String fhirType() { 392 return "ClinicalImpression.investigations"; 393 394 } 395 396 } 397 398 @Block() 399 public static class ClinicalImpressionFindingComponent extends BackboneElement implements IBaseBackboneElement { 400 /** 401 * Specific text of code for finding or diagnosis. 402 */ 403 @Child(name = "item", type = { 404 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 405 @Description(shortDefinition = "Specific text or code for finding", formalDefinition = "Specific text of code for finding or diagnosis.") 406 protected CodeableConcept item; 407 408 /** 409 * Which investigations support finding or diagnosis. 410 */ 411 @Child(name = "cause", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 412 @Description(shortDefinition = "Which investigations support finding", formalDefinition = "Which investigations support finding or diagnosis.") 413 protected StringType cause; 414 415 private static final long serialVersionUID = -888590978L; 416 417 /* 418 * Constructor 419 */ 420 public ClinicalImpressionFindingComponent() { 421 super(); 422 } 423 424 /* 425 * Constructor 426 */ 427 public ClinicalImpressionFindingComponent(CodeableConcept item) { 428 super(); 429 this.item = item; 430 } 431 432 /** 433 * @return {@link #item} (Specific text of code for finding or diagnosis.) 434 */ 435 public CodeableConcept getItem() { 436 if (this.item == null) 437 if (Configuration.errorOnAutoCreate()) 438 throw new Error("Attempt to auto-create ClinicalImpressionFindingComponent.item"); 439 else if (Configuration.doAutoCreate()) 440 this.item = new CodeableConcept(); // cc 441 return this.item; 442 } 443 444 public boolean hasItem() { 445 return this.item != null && !this.item.isEmpty(); 446 } 447 448 /** 449 * @param value {@link #item} (Specific text of code for finding or diagnosis.) 450 */ 451 public ClinicalImpressionFindingComponent setItem(CodeableConcept value) { 452 this.item = value; 453 return this; 454 } 455 456 /** 457 * @return {@link #cause} (Which investigations support finding or diagnosis.). 458 * This is the underlying object with id, value and extensions. The 459 * accessor "getCause" gives direct access to the value 460 */ 461 public StringType getCauseElement() { 462 if (this.cause == null) 463 if (Configuration.errorOnAutoCreate()) 464 throw new Error("Attempt to auto-create ClinicalImpressionFindingComponent.cause"); 465 else if (Configuration.doAutoCreate()) 466 this.cause = new StringType(); // bb 467 return this.cause; 468 } 469 470 public boolean hasCauseElement() { 471 return this.cause != null && !this.cause.isEmpty(); 472 } 473 474 public boolean hasCause() { 475 return this.cause != null && !this.cause.isEmpty(); 476 } 477 478 /** 479 * @param value {@link #cause} (Which investigations support finding or 480 * diagnosis.). This is the underlying object with id, value and 481 * extensions. The accessor "getCause" gives direct access to the 482 * value 483 */ 484 public ClinicalImpressionFindingComponent setCauseElement(StringType value) { 485 this.cause = value; 486 return this; 487 } 488 489 /** 490 * @return Which investigations support finding or diagnosis. 491 */ 492 public String getCause() { 493 return this.cause == null ? null : this.cause.getValue(); 494 } 495 496 /** 497 * @param value Which investigations support finding or diagnosis. 498 */ 499 public ClinicalImpressionFindingComponent setCause(String value) { 500 if (Utilities.noString(value)) 501 this.cause = null; 502 else { 503 if (this.cause == null) 504 this.cause = new StringType(); 505 this.cause.setValue(value); 506 } 507 return this; 508 } 509 510 protected void listChildren(List<Property> childrenList) { 511 super.listChildren(childrenList); 512 childrenList.add(new Property("item", "CodeableConcept", "Specific text of code for finding or diagnosis.", 0, 513 java.lang.Integer.MAX_VALUE, item)); 514 childrenList.add(new Property("cause", "string", "Which investigations support finding or diagnosis.", 0, 515 java.lang.Integer.MAX_VALUE, cause)); 516 } 517 518 @Override 519 public void setProperty(String name, Base value) throws FHIRException { 520 if (name.equals("item")) 521 this.item = castToCodeableConcept(value); // CodeableConcept 522 else if (name.equals("cause")) 523 this.cause = castToString(value); // StringType 524 else 525 super.setProperty(name, value); 526 } 527 528 @Override 529 public Base addChild(String name) throws FHIRException { 530 if (name.equals("item")) { 531 this.item = new CodeableConcept(); 532 return this.item; 533 } else if (name.equals("cause")) { 534 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.cause"); 535 } else 536 return super.addChild(name); 537 } 538 539 public ClinicalImpressionFindingComponent copy() { 540 ClinicalImpressionFindingComponent dst = new ClinicalImpressionFindingComponent(); 541 copyValues(dst); 542 dst.item = item == null ? null : item.copy(); 543 dst.cause = cause == null ? null : cause.copy(); 544 return dst; 545 } 546 547 @Override 548 public boolean equalsDeep(Base other) { 549 if (!super.equalsDeep(other)) 550 return false; 551 if (!(other instanceof ClinicalImpressionFindingComponent)) 552 return false; 553 ClinicalImpressionFindingComponent o = (ClinicalImpressionFindingComponent) other; 554 return compareDeep(item, o.item, true) && compareDeep(cause, o.cause, true); 555 } 556 557 @Override 558 public boolean equalsShallow(Base other) { 559 if (!super.equalsShallow(other)) 560 return false; 561 if (!(other instanceof ClinicalImpressionFindingComponent)) 562 return false; 563 ClinicalImpressionFindingComponent o = (ClinicalImpressionFindingComponent) other; 564 return compareValues(cause, o.cause, true); 565 } 566 567 public boolean isEmpty() { 568 return super.isEmpty() && (item == null || item.isEmpty()) && (cause == null || cause.isEmpty()); 569 } 570 571 public String fhirType() { 572 return "ClinicalImpression.finding"; 573 574 } 575 576 } 577 578 @Block() 579 public static class ClinicalImpressionRuledOutComponent extends BackboneElement implements IBaseBackboneElement { 580 /** 581 * Specific text of code for diagnosis. 582 */ 583 @Child(name = "item", type = { 584 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = false) 585 @Description(shortDefinition = "Specific text of code for diagnosis", formalDefinition = "Specific text of code for diagnosis.") 586 protected CodeableConcept item; 587 588 /** 589 * Grounds for elimination. 590 */ 591 @Child(name = "reason", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 592 @Description(shortDefinition = "Grounds for elimination", formalDefinition = "Grounds for elimination.") 593 protected StringType reason; 594 595 private static final long serialVersionUID = -1001661243L; 596 597 /* 598 * Constructor 599 */ 600 public ClinicalImpressionRuledOutComponent() { 601 super(); 602 } 603 604 /* 605 * Constructor 606 */ 607 public ClinicalImpressionRuledOutComponent(CodeableConcept item) { 608 super(); 609 this.item = item; 610 } 611 612 /** 613 * @return {@link #item} (Specific text of code for diagnosis.) 614 */ 615 public CodeableConcept getItem() { 616 if (this.item == null) 617 if (Configuration.errorOnAutoCreate()) 618 throw new Error("Attempt to auto-create ClinicalImpressionRuledOutComponent.item"); 619 else if (Configuration.doAutoCreate()) 620 this.item = new CodeableConcept(); // cc 621 return this.item; 622 } 623 624 public boolean hasItem() { 625 return this.item != null && !this.item.isEmpty(); 626 } 627 628 /** 629 * @param value {@link #item} (Specific text of code for diagnosis.) 630 */ 631 public ClinicalImpressionRuledOutComponent setItem(CodeableConcept value) { 632 this.item = value; 633 return this; 634 } 635 636 /** 637 * @return {@link #reason} (Grounds for elimination.). This is the underlying 638 * object with id, value and extensions. The accessor "getReason" gives 639 * direct access to the value 640 */ 641 public StringType getReasonElement() { 642 if (this.reason == null) 643 if (Configuration.errorOnAutoCreate()) 644 throw new Error("Attempt to auto-create ClinicalImpressionRuledOutComponent.reason"); 645 else if (Configuration.doAutoCreate()) 646 this.reason = new StringType(); // bb 647 return this.reason; 648 } 649 650 public boolean hasReasonElement() { 651 return this.reason != null && !this.reason.isEmpty(); 652 } 653 654 public boolean hasReason() { 655 return this.reason != null && !this.reason.isEmpty(); 656 } 657 658 /** 659 * @param value {@link #reason} (Grounds for elimination.). This is the 660 * underlying object with id, value and extensions. The accessor 661 * "getReason" gives direct access to the value 662 */ 663 public ClinicalImpressionRuledOutComponent setReasonElement(StringType value) { 664 this.reason = value; 665 return this; 666 } 667 668 /** 669 * @return Grounds for elimination. 670 */ 671 public String getReason() { 672 return this.reason == null ? null : this.reason.getValue(); 673 } 674 675 /** 676 * @param value Grounds for elimination. 677 */ 678 public ClinicalImpressionRuledOutComponent setReason(String value) { 679 if (Utilities.noString(value)) 680 this.reason = null; 681 else { 682 if (this.reason == null) 683 this.reason = new StringType(); 684 this.reason.setValue(value); 685 } 686 return this; 687 } 688 689 protected void listChildren(List<Property> childrenList) { 690 super.listChildren(childrenList); 691 childrenList.add(new Property("item", "CodeableConcept", "Specific text of code for diagnosis.", 0, 692 java.lang.Integer.MAX_VALUE, item)); 693 childrenList 694 .add(new Property("reason", "string", "Grounds for elimination.", 0, java.lang.Integer.MAX_VALUE, reason)); 695 } 696 697 @Override 698 public void setProperty(String name, Base value) throws FHIRException { 699 if (name.equals("item")) 700 this.item = castToCodeableConcept(value); // CodeableConcept 701 else if (name.equals("reason")) 702 this.reason = castToString(value); // StringType 703 else 704 super.setProperty(name, value); 705 } 706 707 @Override 708 public Base addChild(String name) throws FHIRException { 709 if (name.equals("item")) { 710 this.item = new CodeableConcept(); 711 return this.item; 712 } else if (name.equals("reason")) { 713 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.reason"); 714 } else 715 return super.addChild(name); 716 } 717 718 public ClinicalImpressionRuledOutComponent copy() { 719 ClinicalImpressionRuledOutComponent dst = new ClinicalImpressionRuledOutComponent(); 720 copyValues(dst); 721 dst.item = item == null ? null : item.copy(); 722 dst.reason = reason == null ? null : reason.copy(); 723 return dst; 724 } 725 726 @Override 727 public boolean equalsDeep(Base other) { 728 if (!super.equalsDeep(other)) 729 return false; 730 if (!(other instanceof ClinicalImpressionRuledOutComponent)) 731 return false; 732 ClinicalImpressionRuledOutComponent o = (ClinicalImpressionRuledOutComponent) other; 733 return compareDeep(item, o.item, true) && compareDeep(reason, o.reason, true); 734 } 735 736 @Override 737 public boolean equalsShallow(Base other) { 738 if (!super.equalsShallow(other)) 739 return false; 740 if (!(other instanceof ClinicalImpressionRuledOutComponent)) 741 return false; 742 ClinicalImpressionRuledOutComponent o = (ClinicalImpressionRuledOutComponent) other; 743 return compareValues(reason, o.reason, true); 744 } 745 746 public boolean isEmpty() { 747 return super.isEmpty() && (item == null || item.isEmpty()) && (reason == null || reason.isEmpty()); 748 } 749 750 public String fhirType() { 751 return "ClinicalImpression.ruledOut"; 752 753 } 754 755 } 756 757 /** 758 * The patient being assessed. 759 */ 760 @Child(name = "patient", type = { Patient.class }, order = 0, min = 1, max = 1, modifier = false, summary = true) 761 @Description(shortDefinition = "The patient being assessed", formalDefinition = "The patient being assessed.") 762 protected Reference patient; 763 764 /** 765 * The actual object that is the target of the reference (The patient being 766 * assessed.) 767 */ 768 protected Patient patientTarget; 769 770 /** 771 * The clinician performing the assessment. 772 */ 773 @Child(name = "assessor", type = { 774 Practitioner.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 775 @Description(shortDefinition = "The clinician performing the assessment", formalDefinition = "The clinician performing the assessment.") 776 protected Reference assessor; 777 778 /** 779 * The actual object that is the target of the reference (The clinician 780 * performing the assessment.) 781 */ 782 protected Practitioner assessorTarget; 783 784 /** 785 * Identifies the workflow status of the assessment. 786 */ 787 @Child(name = "status", type = { CodeType.class }, order = 2, min = 1, max = 1, modifier = true, summary = true) 788 @Description(shortDefinition = "in-progress | completed | entered-in-error", formalDefinition = "Identifies the workflow status of the assessment.") 789 protected Enumeration<ClinicalImpressionStatus> status; 790 791 /** 792 * The point in time at which the assessment was concluded (not when it was 793 * recorded). 794 */ 795 @Child(name = "date", type = { DateTimeType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 796 @Description(shortDefinition = "When the assessment occurred", formalDefinition = "The point in time at which the assessment was concluded (not when it was recorded).") 797 protected DateTimeType date; 798 799 /** 800 * A summary of the context and/or cause of the assessment - why / where was it 801 * peformed, and what patient events/sstatus prompted it. 802 */ 803 @Child(name = "description", type = { 804 StringType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 805 @Description(shortDefinition = "Why/how the assessment was performed", formalDefinition = "A summary of the context and/or cause of the assessment - why / where was it peformed, and what patient events/sstatus prompted it.") 806 protected StringType description; 807 808 /** 809 * A reference to the last assesment that was conducted bon this patient. 810 * Assessments are often/usually ongoing in nature; a care provider 811 * (practitioner or team) will make new assessments on an ongoing basis as new 812 * data arises or the patient's conditions changes. 813 */ 814 @Child(name = "previous", type = { 815 ClinicalImpression.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 816 @Description(shortDefinition = "Reference to last assessment", formalDefinition = "A reference to the last assesment that was conducted bon this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.") 817 protected Reference previous; 818 819 /** 820 * The actual object that is the target of the reference (A reference to the 821 * last assesment that was conducted bon this patient. Assessments are 822 * often/usually ongoing in nature; a care provider (practitioner or team) will 823 * make new assessments on an ongoing basis as new data arises or the patient's 824 * conditions changes.) 825 */ 826 protected ClinicalImpression previousTarget; 827 828 /** 829 * This a list of the general problems/conditions for a patient. 830 */ 831 @Child(name = "problem", type = { Condition.class, 832 AllergyIntolerance.class }, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 833 @Description(shortDefinition = "General assessment of patient state", formalDefinition = "This a list of the general problems/conditions for a patient.") 834 protected List<Reference> problem; 835 /** 836 * The actual objects that are the target of the reference (This a list of the 837 * general problems/conditions for a patient.) 838 */ 839 protected List<Resource> problemTarget; 840 841 /** 842 * The request or event that necessitated this assessment. This may be a 843 * diagnosis, a Care Plan, a Request Referral, or some other resource. 844 */ 845 @Child(name = "trigger", type = { 846 CodeableConcept.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 847 @Description(shortDefinition = "Request or event that necessitated this assessment", formalDefinition = "The request or event that necessitated this assessment. This may be a diagnosis, a Care Plan, a Request Referral, or some other resource.") 848 protected Type trigger; 849 850 /** 851 * One or more sets of investigations (signs, symptions, etc.). The actual 852 * grouping of investigations vary greatly depending on the type and context of 853 * the assessment. These investigations may include data generated during the 854 * assessment process, or data previously generated and recorded that is 855 * pertinent to the outcomes. 856 */ 857 @Child(name = "investigations", type = {}, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 858 @Description(shortDefinition = "One or more sets of investigations (signs, symptions, etc.)", formalDefinition = "One or more sets of investigations (signs, symptions, etc.). The actual grouping of investigations vary greatly depending on the type and context of the assessment. These investigations may include data generated during the assessment process, or data previously generated and recorded that is pertinent to the outcomes.") 859 protected List<ClinicalImpressionInvestigationsComponent> investigations; 860 861 /** 862 * Reference to a specific published clinical protocol that was followed during 863 * this assessment, and/or that provides evidence in support of the diagnosis. 864 */ 865 @Child(name = "protocol", type = { UriType.class }, order = 9, min = 0, max = 1, modifier = false, summary = false) 866 @Description(shortDefinition = "Clinical Protocol followed", formalDefinition = "Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.") 867 protected UriType protocol; 868 869 /** 870 * A text summary of the investigations and the diagnosis. 871 */ 872 @Child(name = "summary", type = { StringType.class }, order = 10, min = 0, max = 1, modifier = false, summary = false) 873 @Description(shortDefinition = "Summary of the assessment", formalDefinition = "A text summary of the investigations and the diagnosis.") 874 protected StringType summary; 875 876 /** 877 * Specific findings or diagnoses that was considered likely or relevant to 878 * ongoing treatment. 879 */ 880 @Child(name = "finding", type = {}, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 881 @Description(shortDefinition = "Possible or likely findings and diagnoses", formalDefinition = "Specific findings or diagnoses that was considered likely or relevant to ongoing treatment.") 882 protected List<ClinicalImpressionFindingComponent> finding; 883 884 /** 885 * Diagnoses/conditions resolved since the last assessment. 886 */ 887 @Child(name = "resolved", type = { 888 CodeableConcept.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 889 @Description(shortDefinition = "Diagnoses/conditions resolved since previous assessment", formalDefinition = "Diagnoses/conditions resolved since the last assessment.") 890 protected List<CodeableConcept> resolved; 891 892 /** 893 * Diagnosis considered not possible. 894 */ 895 @Child(name = "ruledOut", type = {}, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 896 @Description(shortDefinition = "Diagnosis considered not possible", formalDefinition = "Diagnosis considered not possible.") 897 protected List<ClinicalImpressionRuledOutComponent> ruledOut; 898 899 /** 900 * Estimate of likely outcome. 901 */ 902 @Child(name = "prognosis", type = { 903 StringType.class }, order = 14, min = 0, max = 1, modifier = false, summary = false) 904 @Description(shortDefinition = "Estimate of likely outcome", formalDefinition = "Estimate of likely outcome.") 905 protected StringType prognosis; 906 907 /** 908 * Plan of action after assessment. 909 */ 910 @Child(name = "plan", type = { CarePlan.class, Appointment.class, CommunicationRequest.class, DeviceUseRequest.class, 911 DiagnosticOrder.class, MedicationOrder.class, NutritionOrder.class, Order.class, ProcedureRequest.class, 912 ProcessRequest.class, ReferralRequest.class, SupplyRequest.class, 913 VisionPrescription.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 914 @Description(shortDefinition = "Plan of action after assessment", formalDefinition = "Plan of action after assessment.") 915 protected List<Reference> plan; 916 /** 917 * The actual objects that are the target of the reference (Plan of action after 918 * assessment.) 919 */ 920 protected List<Resource> planTarget; 921 922 /** 923 * Actions taken during assessment. 924 */ 925 @Child(name = "action", type = { ReferralRequest.class, ProcedureRequest.class, Procedure.class, 926 MedicationOrder.class, DiagnosticOrder.class, NutritionOrder.class, SupplyRequest.class, 927 Appointment.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 928 @Description(shortDefinition = "Actions taken during assessment", formalDefinition = "Actions taken during assessment.") 929 protected List<Reference> action; 930 /** 931 * The actual objects that are the target of the reference (Actions taken during 932 * assessment.) 933 */ 934 protected List<Resource> actionTarget; 935 936 private static final long serialVersionUID = 1650458630L; 937 938 /* 939 * Constructor 940 */ 941 public ClinicalImpression() { 942 super(); 943 } 944 945 /* 946 * Constructor 947 */ 948 public ClinicalImpression(Reference patient, Enumeration<ClinicalImpressionStatus> status) { 949 super(); 950 this.patient = patient; 951 this.status = status; 952 } 953 954 /** 955 * @return {@link #patient} (The patient being assessed.) 956 */ 957 public Reference getPatient() { 958 if (this.patient == null) 959 if (Configuration.errorOnAutoCreate()) 960 throw new Error("Attempt to auto-create ClinicalImpression.patient"); 961 else if (Configuration.doAutoCreate()) 962 this.patient = new Reference(); // cc 963 return this.patient; 964 } 965 966 public boolean hasPatient() { 967 return this.patient != null && !this.patient.isEmpty(); 968 } 969 970 /** 971 * @param value {@link #patient} (The patient being assessed.) 972 */ 973 public ClinicalImpression setPatient(Reference value) { 974 this.patient = value; 975 return this; 976 } 977 978 /** 979 * @return {@link #patient} The actual object that is the target of the 980 * reference. The reference library doesn't populate this, but you can 981 * use it to hold the resource if you resolve it. (The patient being 982 * assessed.) 983 */ 984 public Patient getPatientTarget() { 985 if (this.patientTarget == null) 986 if (Configuration.errorOnAutoCreate()) 987 throw new Error("Attempt to auto-create ClinicalImpression.patient"); 988 else if (Configuration.doAutoCreate()) 989 this.patientTarget = new Patient(); // aa 990 return this.patientTarget; 991 } 992 993 /** 994 * @param value {@link #patient} The actual object that is the target of the 995 * reference. The reference library doesn't use these, but you can 996 * use it to hold the resource if you resolve it. (The patient 997 * being assessed.) 998 */ 999 public ClinicalImpression setPatientTarget(Patient value) { 1000 this.patientTarget = value; 1001 return this; 1002 } 1003 1004 /** 1005 * @return {@link #assessor} (The clinician performing the assessment.) 1006 */ 1007 public Reference getAssessor() { 1008 if (this.assessor == null) 1009 if (Configuration.errorOnAutoCreate()) 1010 throw new Error("Attempt to auto-create ClinicalImpression.assessor"); 1011 else if (Configuration.doAutoCreate()) 1012 this.assessor = new Reference(); // cc 1013 return this.assessor; 1014 } 1015 1016 public boolean hasAssessor() { 1017 return this.assessor != null && !this.assessor.isEmpty(); 1018 } 1019 1020 /** 1021 * @param value {@link #assessor} (The clinician performing the assessment.) 1022 */ 1023 public ClinicalImpression setAssessor(Reference value) { 1024 this.assessor = value; 1025 return this; 1026 } 1027 1028 /** 1029 * @return {@link #assessor} The actual object that is the target of the 1030 * reference. The reference library doesn't populate this, but you can 1031 * use it to hold the resource if you resolve it. (The clinician 1032 * performing the assessment.) 1033 */ 1034 public Practitioner getAssessorTarget() { 1035 if (this.assessorTarget == null) 1036 if (Configuration.errorOnAutoCreate()) 1037 throw new Error("Attempt to auto-create ClinicalImpression.assessor"); 1038 else if (Configuration.doAutoCreate()) 1039 this.assessorTarget = new Practitioner(); // aa 1040 return this.assessorTarget; 1041 } 1042 1043 /** 1044 * @param value {@link #assessor} The actual object that is the target of the 1045 * reference. The reference library doesn't use these, but you can 1046 * use it to hold the resource if you resolve it. (The clinician 1047 * performing the assessment.) 1048 */ 1049 public ClinicalImpression setAssessorTarget(Practitioner value) { 1050 this.assessorTarget = value; 1051 return this; 1052 } 1053 1054 /** 1055 * @return {@link #status} (Identifies the workflow status of the assessment.). 1056 * This is the underlying object with id, value and extensions. The 1057 * accessor "getStatus" gives direct access to the value 1058 */ 1059 public Enumeration<ClinicalImpressionStatus> getStatusElement() { 1060 if (this.status == null) 1061 if (Configuration.errorOnAutoCreate()) 1062 throw new Error("Attempt to auto-create ClinicalImpression.status"); 1063 else if (Configuration.doAutoCreate()) 1064 this.status = new Enumeration<ClinicalImpressionStatus>(new ClinicalImpressionStatusEnumFactory()); // bb 1065 return this.status; 1066 } 1067 1068 public boolean hasStatusElement() { 1069 return this.status != null && !this.status.isEmpty(); 1070 } 1071 1072 public boolean hasStatus() { 1073 return this.status != null && !this.status.isEmpty(); 1074 } 1075 1076 /** 1077 * @param value {@link #status} (Identifies the workflow status of the 1078 * assessment.). This is the underlying object with id, value and 1079 * extensions. The accessor "getStatus" gives direct access to the 1080 * value 1081 */ 1082 public ClinicalImpression setStatusElement(Enumeration<ClinicalImpressionStatus> value) { 1083 this.status = value; 1084 return this; 1085 } 1086 1087 /** 1088 * @return Identifies the workflow status of the assessment. 1089 */ 1090 public ClinicalImpressionStatus getStatus() { 1091 return this.status == null ? null : this.status.getValue(); 1092 } 1093 1094 /** 1095 * @param value Identifies the workflow status of the assessment. 1096 */ 1097 public ClinicalImpression setStatus(ClinicalImpressionStatus value) { 1098 if (this.status == null) 1099 this.status = new Enumeration<ClinicalImpressionStatus>(new ClinicalImpressionStatusEnumFactory()); 1100 this.status.setValue(value); 1101 return this; 1102 } 1103 1104 /** 1105 * @return {@link #date} (The point in time at which the assessment was 1106 * concluded (not when it was recorded).). This is the underlying object 1107 * with id, value and extensions. The accessor "getDate" gives direct 1108 * access to the value 1109 */ 1110 public DateTimeType getDateElement() { 1111 if (this.date == null) 1112 if (Configuration.errorOnAutoCreate()) 1113 throw new Error("Attempt to auto-create ClinicalImpression.date"); 1114 else if (Configuration.doAutoCreate()) 1115 this.date = new DateTimeType(); // bb 1116 return this.date; 1117 } 1118 1119 public boolean hasDateElement() { 1120 return this.date != null && !this.date.isEmpty(); 1121 } 1122 1123 public boolean hasDate() { 1124 return this.date != null && !this.date.isEmpty(); 1125 } 1126 1127 /** 1128 * @param value {@link #date} (The point in time at which the assessment was 1129 * concluded (not when it was recorded).). This is the underlying 1130 * object with id, value and extensions. The accessor "getDate" 1131 * gives direct access to the value 1132 */ 1133 public ClinicalImpression setDateElement(DateTimeType value) { 1134 this.date = value; 1135 return this; 1136 } 1137 1138 /** 1139 * @return The point in time at which the assessment was concluded (not when it 1140 * was recorded). 1141 */ 1142 public Date getDate() { 1143 return this.date == null ? null : this.date.getValue(); 1144 } 1145 1146 /** 1147 * @param value The point in time at which the assessment was concluded (not 1148 * when it was recorded). 1149 */ 1150 public ClinicalImpression setDate(Date value) { 1151 if (value == null) 1152 this.date = null; 1153 else { 1154 if (this.date == null) 1155 this.date = new DateTimeType(); 1156 this.date.setValue(value); 1157 } 1158 return this; 1159 } 1160 1161 /** 1162 * @return {@link #description} (A summary of the context and/or cause of the 1163 * assessment - why / where was it peformed, and what patient 1164 * events/sstatus prompted it.). This is the underlying object with id, 1165 * value and extensions. The accessor "getDescription" gives direct 1166 * access to the value 1167 */ 1168 public StringType getDescriptionElement() { 1169 if (this.description == null) 1170 if (Configuration.errorOnAutoCreate()) 1171 throw new Error("Attempt to auto-create ClinicalImpression.description"); 1172 else if (Configuration.doAutoCreate()) 1173 this.description = new StringType(); // bb 1174 return this.description; 1175 } 1176 1177 public boolean hasDescriptionElement() { 1178 return this.description != null && !this.description.isEmpty(); 1179 } 1180 1181 public boolean hasDescription() { 1182 return this.description != null && !this.description.isEmpty(); 1183 } 1184 1185 /** 1186 * @param value {@link #description} (A summary of the context and/or cause of 1187 * the assessment - why / where was it peformed, and what patient 1188 * events/sstatus prompted it.). This is the underlying object with 1189 * id, value and extensions. The accessor "getDescription" gives 1190 * direct access to the value 1191 */ 1192 public ClinicalImpression setDescriptionElement(StringType value) { 1193 this.description = value; 1194 return this; 1195 } 1196 1197 /** 1198 * @return A summary of the context and/or cause of the assessment - why / where 1199 * was it peformed, and what patient events/sstatus prompted it. 1200 */ 1201 public String getDescription() { 1202 return this.description == null ? null : this.description.getValue(); 1203 } 1204 1205 /** 1206 * @param value A summary of the context and/or cause of the assessment - why / 1207 * where was it peformed, and what patient events/sstatus prompted 1208 * it. 1209 */ 1210 public ClinicalImpression setDescription(String value) { 1211 if (Utilities.noString(value)) 1212 this.description = null; 1213 else { 1214 if (this.description == null) 1215 this.description = new StringType(); 1216 this.description.setValue(value); 1217 } 1218 return this; 1219 } 1220 1221 /** 1222 * @return {@link #previous} (A reference to the last assesment that was 1223 * conducted bon this patient. Assessments are often/usually ongoing in 1224 * nature; a care provider (practitioner or team) will make new 1225 * assessments on an ongoing basis as new data arises or the patient's 1226 * conditions changes.) 1227 */ 1228 public Reference getPrevious() { 1229 if (this.previous == null) 1230 if (Configuration.errorOnAutoCreate()) 1231 throw new Error("Attempt to auto-create ClinicalImpression.previous"); 1232 else if (Configuration.doAutoCreate()) 1233 this.previous = new Reference(); // cc 1234 return this.previous; 1235 } 1236 1237 public boolean hasPrevious() { 1238 return this.previous != null && !this.previous.isEmpty(); 1239 } 1240 1241 /** 1242 * @param value {@link #previous} (A reference to the last assesment that was 1243 * conducted bon this patient. Assessments are often/usually 1244 * ongoing in nature; a care provider (practitioner or team) will 1245 * make new assessments on an ongoing basis as new data arises or 1246 * the patient's conditions changes.) 1247 */ 1248 public ClinicalImpression setPrevious(Reference value) { 1249 this.previous = value; 1250 return this; 1251 } 1252 1253 /** 1254 * @return {@link #previous} The actual object that is the target of the 1255 * reference. The reference library doesn't populate this, but you can 1256 * use it to hold the resource if you resolve it. (A reference to the 1257 * last assesment that was conducted bon this patient. Assessments are 1258 * often/usually ongoing in nature; a care provider (practitioner or 1259 * team) will make new assessments on an ongoing basis as new data 1260 * arises or the patient's conditions changes.) 1261 */ 1262 public ClinicalImpression getPreviousTarget() { 1263 if (this.previousTarget == null) 1264 if (Configuration.errorOnAutoCreate()) 1265 throw new Error("Attempt to auto-create ClinicalImpression.previous"); 1266 else if (Configuration.doAutoCreate()) 1267 this.previousTarget = new ClinicalImpression(); // aa 1268 return this.previousTarget; 1269 } 1270 1271 /** 1272 * @param value {@link #previous} The actual object that is the target of the 1273 * reference. The reference library doesn't use these, but you can 1274 * use it to hold the resource if you resolve it. (A reference to 1275 * the last assesment that was conducted bon this patient. 1276 * Assessments are often/usually ongoing in nature; a care provider 1277 * (practitioner or team) will make new assessments on an ongoing 1278 * basis as new data arises or the patient's conditions changes.) 1279 */ 1280 public ClinicalImpression setPreviousTarget(ClinicalImpression value) { 1281 this.previousTarget = value; 1282 return this; 1283 } 1284 1285 /** 1286 * @return {@link #problem} (This a list of the general problems/conditions for 1287 * a patient.) 1288 */ 1289 public List<Reference> getProblem() { 1290 if (this.problem == null) 1291 this.problem = new ArrayList<Reference>(); 1292 return this.problem; 1293 } 1294 1295 public boolean hasProblem() { 1296 if (this.problem == null) 1297 return false; 1298 for (Reference item : this.problem) 1299 if (!item.isEmpty()) 1300 return true; 1301 return false; 1302 } 1303 1304 /** 1305 * @return {@link #problem} (This a list of the general problems/conditions for 1306 * a patient.) 1307 */ 1308 // syntactic sugar 1309 public Reference addProblem() { // 3 1310 Reference t = new Reference(); 1311 if (this.problem == null) 1312 this.problem = new ArrayList<Reference>(); 1313 this.problem.add(t); 1314 return t; 1315 } 1316 1317 // syntactic sugar 1318 public ClinicalImpression addProblem(Reference t) { // 3 1319 if (t == null) 1320 return this; 1321 if (this.problem == null) 1322 this.problem = new ArrayList<Reference>(); 1323 this.problem.add(t); 1324 return this; 1325 } 1326 1327 /** 1328 * @return {@link #problem} (The actual objects that are the target of the 1329 * reference. The reference library doesn't populate this, but you can 1330 * use this to hold the resources if you resolvethemt. This a list of 1331 * the general problems/conditions for a patient.) 1332 */ 1333 public List<Resource> getProblemTarget() { 1334 if (this.problemTarget == null) 1335 this.problemTarget = new ArrayList<Resource>(); 1336 return this.problemTarget; 1337 } 1338 1339 /** 1340 * @return {@link #trigger} (The request or event that necessitated this 1341 * assessment. This may be a diagnosis, a Care Plan, a Request Referral, 1342 * or some other resource.) 1343 */ 1344 public Type getTrigger() { 1345 return this.trigger; 1346 } 1347 1348 /** 1349 * @return {@link #trigger} (The request or event that necessitated this 1350 * assessment. This may be a diagnosis, a Care Plan, a Request Referral, 1351 * or some other resource.) 1352 */ 1353 public CodeableConcept getTriggerCodeableConcept() throws FHIRException { 1354 if (!(this.trigger instanceof CodeableConcept)) 1355 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 1356 + this.trigger.getClass().getName() + " was encountered"); 1357 return (CodeableConcept) this.trigger; 1358 } 1359 1360 public boolean hasTriggerCodeableConcept() { 1361 return this.trigger instanceof CodeableConcept; 1362 } 1363 1364 /** 1365 * @return {@link #trigger} (The request or event that necessitated this 1366 * assessment. This may be a diagnosis, a Care Plan, a Request Referral, 1367 * or some other resource.) 1368 */ 1369 public Reference getTriggerReference() throws FHIRException { 1370 if (!(this.trigger instanceof Reference)) 1371 throw new FHIRException("Type mismatch: the type Reference was expected, but " + this.trigger.getClass().getName() 1372 + " was encountered"); 1373 return (Reference) this.trigger; 1374 } 1375 1376 public boolean hasTriggerReference() { 1377 return this.trigger instanceof Reference; 1378 } 1379 1380 public boolean hasTrigger() { 1381 return this.trigger != null && !this.trigger.isEmpty(); 1382 } 1383 1384 /** 1385 * @param value {@link #trigger} (The request or event that necessitated this 1386 * assessment. This may be a diagnosis, a Care Plan, a Request 1387 * Referral, or some other resource.) 1388 */ 1389 public ClinicalImpression setTrigger(Type value) { 1390 this.trigger = value; 1391 return this; 1392 } 1393 1394 /** 1395 * @return {@link #investigations} (One or more sets of investigations (signs, 1396 * symptions, etc.). The actual grouping of investigations vary greatly 1397 * depending on the type and context of the assessment. These 1398 * investigations may include data generated during the assessment 1399 * process, or data previously generated and recorded that is pertinent 1400 * to the outcomes.) 1401 */ 1402 public List<ClinicalImpressionInvestigationsComponent> getInvestigations() { 1403 if (this.investigations == null) 1404 this.investigations = new ArrayList<ClinicalImpressionInvestigationsComponent>(); 1405 return this.investigations; 1406 } 1407 1408 public boolean hasInvestigations() { 1409 if (this.investigations == null) 1410 return false; 1411 for (ClinicalImpressionInvestigationsComponent item : this.investigations) 1412 if (!item.isEmpty()) 1413 return true; 1414 return false; 1415 } 1416 1417 /** 1418 * @return {@link #investigations} (One or more sets of investigations (signs, 1419 * symptions, etc.). The actual grouping of investigations vary greatly 1420 * depending on the type and context of the assessment. These 1421 * investigations may include data generated during the assessment 1422 * process, or data previously generated and recorded that is pertinent 1423 * to the outcomes.) 1424 */ 1425 // syntactic sugar 1426 public ClinicalImpressionInvestigationsComponent addInvestigations() { // 3 1427 ClinicalImpressionInvestigationsComponent t = new ClinicalImpressionInvestigationsComponent(); 1428 if (this.investigations == null) 1429 this.investigations = new ArrayList<ClinicalImpressionInvestigationsComponent>(); 1430 this.investigations.add(t); 1431 return t; 1432 } 1433 1434 // syntactic sugar 1435 public ClinicalImpression addInvestigations(ClinicalImpressionInvestigationsComponent t) { // 3 1436 if (t == null) 1437 return this; 1438 if (this.investigations == null) 1439 this.investigations = new ArrayList<ClinicalImpressionInvestigationsComponent>(); 1440 this.investigations.add(t); 1441 return this; 1442 } 1443 1444 /** 1445 * @return {@link #protocol} (Reference to a specific published clinical 1446 * protocol that was followed during this assessment, and/or that 1447 * provides evidence in support of the diagnosis.). This is the 1448 * underlying object with id, value and extensions. The accessor 1449 * "getProtocol" gives direct access to the value 1450 */ 1451 public UriType getProtocolElement() { 1452 if (this.protocol == null) 1453 if (Configuration.errorOnAutoCreate()) 1454 throw new Error("Attempt to auto-create ClinicalImpression.protocol"); 1455 else if (Configuration.doAutoCreate()) 1456 this.protocol = new UriType(); // bb 1457 return this.protocol; 1458 } 1459 1460 public boolean hasProtocolElement() { 1461 return this.protocol != null && !this.protocol.isEmpty(); 1462 } 1463 1464 public boolean hasProtocol() { 1465 return this.protocol != null && !this.protocol.isEmpty(); 1466 } 1467 1468 /** 1469 * @param value {@link #protocol} (Reference to a specific published clinical 1470 * protocol that was followed during this assessment, and/or that 1471 * provides evidence in support of the diagnosis.). This is the 1472 * underlying object with id, value and extensions. The accessor 1473 * "getProtocol" gives direct access to the value 1474 */ 1475 public ClinicalImpression setProtocolElement(UriType value) { 1476 this.protocol = value; 1477 return this; 1478 } 1479 1480 /** 1481 * @return Reference to a specific published clinical protocol that was followed 1482 * during this assessment, and/or that provides evidence in support of 1483 * the diagnosis. 1484 */ 1485 public String getProtocol() { 1486 return this.protocol == null ? null : this.protocol.getValue(); 1487 } 1488 1489 /** 1490 * @param value Reference to a specific published clinical protocol that was 1491 * followed during this assessment, and/or that provides evidence 1492 * in support of the diagnosis. 1493 */ 1494 public ClinicalImpression setProtocol(String value) { 1495 if (Utilities.noString(value)) 1496 this.protocol = null; 1497 else { 1498 if (this.protocol == null) 1499 this.protocol = new UriType(); 1500 this.protocol.setValue(value); 1501 } 1502 return this; 1503 } 1504 1505 /** 1506 * @return {@link #summary} (A text summary of the investigations and the 1507 * diagnosis.). This is the underlying object with id, value and 1508 * extensions. The accessor "getSummary" gives direct access to the 1509 * value 1510 */ 1511 public StringType getSummaryElement() { 1512 if (this.summary == null) 1513 if (Configuration.errorOnAutoCreate()) 1514 throw new Error("Attempt to auto-create ClinicalImpression.summary"); 1515 else if (Configuration.doAutoCreate()) 1516 this.summary = new StringType(); // bb 1517 return this.summary; 1518 } 1519 1520 public boolean hasSummaryElement() { 1521 return this.summary != null && !this.summary.isEmpty(); 1522 } 1523 1524 public boolean hasSummary() { 1525 return this.summary != null && !this.summary.isEmpty(); 1526 } 1527 1528 /** 1529 * @param value {@link #summary} (A text summary of the investigations and the 1530 * diagnosis.). This is the underlying object with id, value and 1531 * extensions. The accessor "getSummary" gives direct access to the 1532 * value 1533 */ 1534 public ClinicalImpression setSummaryElement(StringType value) { 1535 this.summary = value; 1536 return this; 1537 } 1538 1539 /** 1540 * @return A text summary of the investigations and the diagnosis. 1541 */ 1542 public String getSummary() { 1543 return this.summary == null ? null : this.summary.getValue(); 1544 } 1545 1546 /** 1547 * @param value A text summary of the investigations and the diagnosis. 1548 */ 1549 public ClinicalImpression setSummary(String value) { 1550 if (Utilities.noString(value)) 1551 this.summary = null; 1552 else { 1553 if (this.summary == null) 1554 this.summary = new StringType(); 1555 this.summary.setValue(value); 1556 } 1557 return this; 1558 } 1559 1560 /** 1561 * @return {@link #finding} (Specific findings or diagnoses that was considered 1562 * likely or relevant to ongoing treatment.) 1563 */ 1564 public List<ClinicalImpressionFindingComponent> getFinding() { 1565 if (this.finding == null) 1566 this.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 1567 return this.finding; 1568 } 1569 1570 public boolean hasFinding() { 1571 if (this.finding == null) 1572 return false; 1573 for (ClinicalImpressionFindingComponent item : this.finding) 1574 if (!item.isEmpty()) 1575 return true; 1576 return false; 1577 } 1578 1579 /** 1580 * @return {@link #finding} (Specific findings or diagnoses that was considered 1581 * likely or relevant to ongoing treatment.) 1582 */ 1583 // syntactic sugar 1584 public ClinicalImpressionFindingComponent addFinding() { // 3 1585 ClinicalImpressionFindingComponent t = new ClinicalImpressionFindingComponent(); 1586 if (this.finding == null) 1587 this.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 1588 this.finding.add(t); 1589 return t; 1590 } 1591 1592 // syntactic sugar 1593 public ClinicalImpression addFinding(ClinicalImpressionFindingComponent t) { // 3 1594 if (t == null) 1595 return this; 1596 if (this.finding == null) 1597 this.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 1598 this.finding.add(t); 1599 return this; 1600 } 1601 1602 /** 1603 * @return {@link #resolved} (Diagnoses/conditions resolved since the last 1604 * assessment.) 1605 */ 1606 public List<CodeableConcept> getResolved() { 1607 if (this.resolved == null) 1608 this.resolved = new ArrayList<CodeableConcept>(); 1609 return this.resolved; 1610 } 1611 1612 public boolean hasResolved() { 1613 if (this.resolved == null) 1614 return false; 1615 for (CodeableConcept item : this.resolved) 1616 if (!item.isEmpty()) 1617 return true; 1618 return false; 1619 } 1620 1621 /** 1622 * @return {@link #resolved} (Diagnoses/conditions resolved since the last 1623 * assessment.) 1624 */ 1625 // syntactic sugar 1626 public CodeableConcept addResolved() { // 3 1627 CodeableConcept t = new CodeableConcept(); 1628 if (this.resolved == null) 1629 this.resolved = new ArrayList<CodeableConcept>(); 1630 this.resolved.add(t); 1631 return t; 1632 } 1633 1634 // syntactic sugar 1635 public ClinicalImpression addResolved(CodeableConcept t) { // 3 1636 if (t == null) 1637 return this; 1638 if (this.resolved == null) 1639 this.resolved = new ArrayList<CodeableConcept>(); 1640 this.resolved.add(t); 1641 return this; 1642 } 1643 1644 /** 1645 * @return {@link #ruledOut} (Diagnosis considered not possible.) 1646 */ 1647 public List<ClinicalImpressionRuledOutComponent> getRuledOut() { 1648 if (this.ruledOut == null) 1649 this.ruledOut = new ArrayList<ClinicalImpressionRuledOutComponent>(); 1650 return this.ruledOut; 1651 } 1652 1653 public boolean hasRuledOut() { 1654 if (this.ruledOut == null) 1655 return false; 1656 for (ClinicalImpressionRuledOutComponent item : this.ruledOut) 1657 if (!item.isEmpty()) 1658 return true; 1659 return false; 1660 } 1661 1662 /** 1663 * @return {@link #ruledOut} (Diagnosis considered not possible.) 1664 */ 1665 // syntactic sugar 1666 public ClinicalImpressionRuledOutComponent addRuledOut() { // 3 1667 ClinicalImpressionRuledOutComponent t = new ClinicalImpressionRuledOutComponent(); 1668 if (this.ruledOut == null) 1669 this.ruledOut = new ArrayList<ClinicalImpressionRuledOutComponent>(); 1670 this.ruledOut.add(t); 1671 return t; 1672 } 1673 1674 // syntactic sugar 1675 public ClinicalImpression addRuledOut(ClinicalImpressionRuledOutComponent t) { // 3 1676 if (t == null) 1677 return this; 1678 if (this.ruledOut == null) 1679 this.ruledOut = new ArrayList<ClinicalImpressionRuledOutComponent>(); 1680 this.ruledOut.add(t); 1681 return this; 1682 } 1683 1684 /** 1685 * @return {@link #prognosis} (Estimate of likely outcome.). This is the 1686 * underlying object with id, value and extensions. The accessor 1687 * "getPrognosis" gives direct access to the value 1688 */ 1689 public StringType getPrognosisElement() { 1690 if (this.prognosis == null) 1691 if (Configuration.errorOnAutoCreate()) 1692 throw new Error("Attempt to auto-create ClinicalImpression.prognosis"); 1693 else if (Configuration.doAutoCreate()) 1694 this.prognosis = new StringType(); // bb 1695 return this.prognosis; 1696 } 1697 1698 public boolean hasPrognosisElement() { 1699 return this.prognosis != null && !this.prognosis.isEmpty(); 1700 } 1701 1702 public boolean hasPrognosis() { 1703 return this.prognosis != null && !this.prognosis.isEmpty(); 1704 } 1705 1706 /** 1707 * @param value {@link #prognosis} (Estimate of likely outcome.). This is the 1708 * underlying object with id, value and extensions. The accessor 1709 * "getPrognosis" gives direct access to the value 1710 */ 1711 public ClinicalImpression setPrognosisElement(StringType value) { 1712 this.prognosis = value; 1713 return this; 1714 } 1715 1716 /** 1717 * @return Estimate of likely outcome. 1718 */ 1719 public String getPrognosis() { 1720 return this.prognosis == null ? null : this.prognosis.getValue(); 1721 } 1722 1723 /** 1724 * @param value Estimate of likely outcome. 1725 */ 1726 public ClinicalImpression setPrognosis(String value) { 1727 if (Utilities.noString(value)) 1728 this.prognosis = null; 1729 else { 1730 if (this.prognosis == null) 1731 this.prognosis = new StringType(); 1732 this.prognosis.setValue(value); 1733 } 1734 return this; 1735 } 1736 1737 /** 1738 * @return {@link #plan} (Plan of action after assessment.) 1739 */ 1740 public List<Reference> getPlan() { 1741 if (this.plan == null) 1742 this.plan = new ArrayList<Reference>(); 1743 return this.plan; 1744 } 1745 1746 public boolean hasPlan() { 1747 if (this.plan == null) 1748 return false; 1749 for (Reference item : this.plan) 1750 if (!item.isEmpty()) 1751 return true; 1752 return false; 1753 } 1754 1755 /** 1756 * @return {@link #plan} (Plan of action after assessment.) 1757 */ 1758 // syntactic sugar 1759 public Reference addPlan() { // 3 1760 Reference t = new Reference(); 1761 if (this.plan == null) 1762 this.plan = new ArrayList<Reference>(); 1763 this.plan.add(t); 1764 return t; 1765 } 1766 1767 // syntactic sugar 1768 public ClinicalImpression addPlan(Reference t) { // 3 1769 if (t == null) 1770 return this; 1771 if (this.plan == null) 1772 this.plan = new ArrayList<Reference>(); 1773 this.plan.add(t); 1774 return this; 1775 } 1776 1777 /** 1778 * @return {@link #plan} (The actual objects that are the target of the 1779 * reference. The reference library doesn't populate this, but you can 1780 * use this to hold the resources if you resolvethemt. Plan of action 1781 * after assessment.) 1782 */ 1783 public List<Resource> getPlanTarget() { 1784 if (this.planTarget == null) 1785 this.planTarget = new ArrayList<Resource>(); 1786 return this.planTarget; 1787 } 1788 1789 /** 1790 * @return {@link #action} (Actions taken during assessment.) 1791 */ 1792 public List<Reference> getAction() { 1793 if (this.action == null) 1794 this.action = new ArrayList<Reference>(); 1795 return this.action; 1796 } 1797 1798 public boolean hasAction() { 1799 if (this.action == null) 1800 return false; 1801 for (Reference item : this.action) 1802 if (!item.isEmpty()) 1803 return true; 1804 return false; 1805 } 1806 1807 /** 1808 * @return {@link #action} (Actions taken during assessment.) 1809 */ 1810 // syntactic sugar 1811 public Reference addAction() { // 3 1812 Reference t = new Reference(); 1813 if (this.action == null) 1814 this.action = new ArrayList<Reference>(); 1815 this.action.add(t); 1816 return t; 1817 } 1818 1819 // syntactic sugar 1820 public ClinicalImpression addAction(Reference t) { // 3 1821 if (t == null) 1822 return this; 1823 if (this.action == null) 1824 this.action = new ArrayList<Reference>(); 1825 this.action.add(t); 1826 return this; 1827 } 1828 1829 /** 1830 * @return {@link #action} (The actual objects that are the target of the 1831 * reference. The reference library doesn't populate this, but you can 1832 * use this to hold the resources if you resolvethemt. Actions taken 1833 * during assessment.) 1834 */ 1835 public List<Resource> getActionTarget() { 1836 if (this.actionTarget == null) 1837 this.actionTarget = new ArrayList<Resource>(); 1838 return this.actionTarget; 1839 } 1840 1841 protected void listChildren(List<Property> childrenList) { 1842 super.listChildren(childrenList); 1843 childrenList.add(new Property("patient", "Reference(Patient)", "The patient being assessed.", 0, 1844 java.lang.Integer.MAX_VALUE, patient)); 1845 childrenList.add(new Property("assessor", "Reference(Practitioner)", "The clinician performing the assessment.", 0, 1846 java.lang.Integer.MAX_VALUE, assessor)); 1847 childrenList.add(new Property("status", "code", "Identifies the workflow status of the assessment.", 0, 1848 java.lang.Integer.MAX_VALUE, status)); 1849 childrenList.add(new Property("date", "dateTime", 1850 "The point in time at which the assessment was concluded (not when it was recorded).", 0, 1851 java.lang.Integer.MAX_VALUE, date)); 1852 childrenList.add(new Property("description", "string", 1853 "A summary of the context and/or cause of the assessment - why / where was it peformed, and what patient events/sstatus prompted it.", 1854 0, java.lang.Integer.MAX_VALUE, description)); 1855 childrenList.add(new Property("previous", "Reference(ClinicalImpression)", 1856 "A reference to the last assesment that was conducted bon this patient. Assessments are often/usually ongoing in nature; a care provider (practitioner or team) will make new assessments on an ongoing basis as new data arises or the patient's conditions changes.", 1857 0, java.lang.Integer.MAX_VALUE, previous)); 1858 childrenList.add(new Property("problem", "Reference(Condition|AllergyIntolerance)", 1859 "This a list of the general problems/conditions for a patient.", 0, java.lang.Integer.MAX_VALUE, problem)); 1860 childrenList.add(new Property("trigger[x]", "CodeableConcept|Reference(Any)", 1861 "The request or event that necessitated this assessment. This may be a diagnosis, a Care Plan, a Request Referral, or some other resource.", 1862 0, java.lang.Integer.MAX_VALUE, trigger)); 1863 childrenList.add(new Property("investigations", "", 1864 "One or more sets of investigations (signs, symptions, etc.). The actual grouping of investigations vary greatly depending on the type and context of the assessment. These investigations may include data generated during the assessment process, or data previously generated and recorded that is pertinent to the outcomes.", 1865 0, java.lang.Integer.MAX_VALUE, investigations)); 1866 childrenList.add(new Property("protocol", "uri", 1867 "Reference to a specific published clinical protocol that was followed during this assessment, and/or that provides evidence in support of the diagnosis.", 1868 0, java.lang.Integer.MAX_VALUE, protocol)); 1869 childrenList.add(new Property("summary", "string", "A text summary of the investigations and the diagnosis.", 0, 1870 java.lang.Integer.MAX_VALUE, summary)); 1871 childrenList.add(new Property("finding", "", 1872 "Specific findings or diagnoses that was considered likely or relevant to ongoing treatment.", 0, 1873 java.lang.Integer.MAX_VALUE, finding)); 1874 childrenList.add(new Property("resolved", "CodeableConcept", 1875 "Diagnoses/conditions resolved since the last assessment.", 0, java.lang.Integer.MAX_VALUE, resolved)); 1876 childrenList.add( 1877 new Property("ruledOut", "", "Diagnosis considered not possible.", 0, java.lang.Integer.MAX_VALUE, ruledOut)); 1878 childrenList.add( 1879 new Property("prognosis", "string", "Estimate of likely outcome.", 0, java.lang.Integer.MAX_VALUE, prognosis)); 1880 childrenList.add(new Property("plan", 1881 "Reference(CarePlan|Appointment|CommunicationRequest|DeviceUseRequest|DiagnosticOrder|MedicationOrder|NutritionOrder|Order|ProcedureRequest|ProcessRequest|ReferralRequest|SupplyRequest|VisionPrescription)", 1882 "Plan of action after assessment.", 0, java.lang.Integer.MAX_VALUE, plan)); 1883 childrenList.add(new Property("action", 1884 "Reference(ReferralRequest|ProcedureRequest|Procedure|MedicationOrder|DiagnosticOrder|NutritionOrder|SupplyRequest|Appointment)", 1885 "Actions taken during assessment.", 0, java.lang.Integer.MAX_VALUE, action)); 1886 } 1887 1888 @Override 1889 public void setProperty(String name, Base value) throws FHIRException { 1890 if (name.equals("patient")) 1891 this.patient = castToReference(value); // Reference 1892 else if (name.equals("assessor")) 1893 this.assessor = castToReference(value); // Reference 1894 else if (name.equals("status")) 1895 this.status = new ClinicalImpressionStatusEnumFactory().fromType(value); // Enumeration<ClinicalImpressionStatus> 1896 else if (name.equals("date")) 1897 this.date = castToDateTime(value); // DateTimeType 1898 else if (name.equals("description")) 1899 this.description = castToString(value); // StringType 1900 else if (name.equals("previous")) 1901 this.previous = castToReference(value); // Reference 1902 else if (name.equals("problem")) 1903 this.getProblem().add(castToReference(value)); 1904 else if (name.equals("trigger[x]")) 1905 this.trigger = (Type) value; // Type 1906 else if (name.equals("investigations")) 1907 this.getInvestigations().add((ClinicalImpressionInvestigationsComponent) value); 1908 else if (name.equals("protocol")) 1909 this.protocol = castToUri(value); // UriType 1910 else if (name.equals("summary")) 1911 this.summary = castToString(value); // StringType 1912 else if (name.equals("finding")) 1913 this.getFinding().add((ClinicalImpressionFindingComponent) value); 1914 else if (name.equals("resolved")) 1915 this.getResolved().add(castToCodeableConcept(value)); 1916 else if (name.equals("ruledOut")) 1917 this.getRuledOut().add((ClinicalImpressionRuledOutComponent) value); 1918 else if (name.equals("prognosis")) 1919 this.prognosis = castToString(value); // StringType 1920 else if (name.equals("plan")) 1921 this.getPlan().add(castToReference(value)); 1922 else if (name.equals("action")) 1923 this.getAction().add(castToReference(value)); 1924 else 1925 super.setProperty(name, value); 1926 } 1927 1928 @Override 1929 public Base addChild(String name) throws FHIRException { 1930 if (name.equals("patient")) { 1931 this.patient = new Reference(); 1932 return this.patient; 1933 } else if (name.equals("assessor")) { 1934 this.assessor = new Reference(); 1935 return this.assessor; 1936 } else if (name.equals("status")) { 1937 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.status"); 1938 } else if (name.equals("date")) { 1939 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.date"); 1940 } else if (name.equals("description")) { 1941 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.description"); 1942 } else if (name.equals("previous")) { 1943 this.previous = new Reference(); 1944 return this.previous; 1945 } else if (name.equals("problem")) { 1946 return addProblem(); 1947 } else if (name.equals("triggerCodeableConcept")) { 1948 this.trigger = new CodeableConcept(); 1949 return this.trigger; 1950 } else if (name.equals("triggerReference")) { 1951 this.trigger = new Reference(); 1952 return this.trigger; 1953 } else if (name.equals("investigations")) { 1954 return addInvestigations(); 1955 } else if (name.equals("protocol")) { 1956 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.protocol"); 1957 } else if (name.equals("summary")) { 1958 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.summary"); 1959 } else if (name.equals("finding")) { 1960 return addFinding(); 1961 } else if (name.equals("resolved")) { 1962 return addResolved(); 1963 } else if (name.equals("ruledOut")) { 1964 return addRuledOut(); 1965 } else if (name.equals("prognosis")) { 1966 throw new FHIRException("Cannot call addChild on a singleton property ClinicalImpression.prognosis"); 1967 } else if (name.equals("plan")) { 1968 return addPlan(); 1969 } else if (name.equals("action")) { 1970 return addAction(); 1971 } else 1972 return super.addChild(name); 1973 } 1974 1975 public String fhirType() { 1976 return "ClinicalImpression"; 1977 1978 } 1979 1980 public ClinicalImpression copy() { 1981 ClinicalImpression dst = new ClinicalImpression(); 1982 copyValues(dst); 1983 dst.patient = patient == null ? null : patient.copy(); 1984 dst.assessor = assessor == null ? null : assessor.copy(); 1985 dst.status = status == null ? null : status.copy(); 1986 dst.date = date == null ? null : date.copy(); 1987 dst.description = description == null ? null : description.copy(); 1988 dst.previous = previous == null ? null : previous.copy(); 1989 if (problem != null) { 1990 dst.problem = new ArrayList<Reference>(); 1991 for (Reference i : problem) 1992 dst.problem.add(i.copy()); 1993 } 1994 ; 1995 dst.trigger = trigger == null ? null : trigger.copy(); 1996 if (investigations != null) { 1997 dst.investigations = new ArrayList<ClinicalImpressionInvestigationsComponent>(); 1998 for (ClinicalImpressionInvestigationsComponent i : investigations) 1999 dst.investigations.add(i.copy()); 2000 } 2001 ; 2002 dst.protocol = protocol == null ? null : protocol.copy(); 2003 dst.summary = summary == null ? null : summary.copy(); 2004 if (finding != null) { 2005 dst.finding = new ArrayList<ClinicalImpressionFindingComponent>(); 2006 for (ClinicalImpressionFindingComponent i : finding) 2007 dst.finding.add(i.copy()); 2008 } 2009 ; 2010 if (resolved != null) { 2011 dst.resolved = new ArrayList<CodeableConcept>(); 2012 for (CodeableConcept i : resolved) 2013 dst.resolved.add(i.copy()); 2014 } 2015 ; 2016 if (ruledOut != null) { 2017 dst.ruledOut = new ArrayList<ClinicalImpressionRuledOutComponent>(); 2018 for (ClinicalImpressionRuledOutComponent i : ruledOut) 2019 dst.ruledOut.add(i.copy()); 2020 } 2021 ; 2022 dst.prognosis = prognosis == null ? null : prognosis.copy(); 2023 if (plan != null) { 2024 dst.plan = new ArrayList<Reference>(); 2025 for (Reference i : plan) 2026 dst.plan.add(i.copy()); 2027 } 2028 ; 2029 if (action != null) { 2030 dst.action = new ArrayList<Reference>(); 2031 for (Reference i : action) 2032 dst.action.add(i.copy()); 2033 } 2034 ; 2035 return dst; 2036 } 2037 2038 protected ClinicalImpression typedCopy() { 2039 return copy(); 2040 } 2041 2042 @Override 2043 public boolean equalsDeep(Base other) { 2044 if (!super.equalsDeep(other)) 2045 return false; 2046 if (!(other instanceof ClinicalImpression)) 2047 return false; 2048 ClinicalImpression o = (ClinicalImpression) other; 2049 return compareDeep(patient, o.patient, true) && compareDeep(assessor, o.assessor, true) 2050 && compareDeep(status, o.status, true) && compareDeep(date, o.date, true) 2051 && compareDeep(description, o.description, true) && compareDeep(previous, o.previous, true) 2052 && compareDeep(problem, o.problem, true) && compareDeep(trigger, o.trigger, true) 2053 && compareDeep(investigations, o.investigations, true) && compareDeep(protocol, o.protocol, true) 2054 && compareDeep(summary, o.summary, true) && compareDeep(finding, o.finding, true) 2055 && compareDeep(resolved, o.resolved, true) && compareDeep(ruledOut, o.ruledOut, true) 2056 && compareDeep(prognosis, o.prognosis, true) && compareDeep(plan, o.plan, true) 2057 && compareDeep(action, o.action, true); 2058 } 2059 2060 @Override 2061 public boolean equalsShallow(Base other) { 2062 if (!super.equalsShallow(other)) 2063 return false; 2064 if (!(other instanceof ClinicalImpression)) 2065 return false; 2066 ClinicalImpression o = (ClinicalImpression) other; 2067 return compareValues(status, o.status, true) && compareValues(date, o.date, true) 2068 && compareValues(description, o.description, true) && compareValues(protocol, o.protocol, true) 2069 && compareValues(summary, o.summary, true) && compareValues(prognosis, o.prognosis, true); 2070 } 2071 2072 public boolean isEmpty() { 2073 return super.isEmpty() && (patient == null || patient.isEmpty()) && (assessor == null || assessor.isEmpty()) 2074 && (status == null || status.isEmpty()) && (date == null || date.isEmpty()) 2075 && (description == null || description.isEmpty()) && (previous == null || previous.isEmpty()) 2076 && (problem == null || problem.isEmpty()) && (trigger == null || trigger.isEmpty()) 2077 && (investigations == null || investigations.isEmpty()) && (protocol == null || protocol.isEmpty()) 2078 && (summary == null || summary.isEmpty()) && (finding == null || finding.isEmpty()) 2079 && (resolved == null || resolved.isEmpty()) && (ruledOut == null || ruledOut.isEmpty()) 2080 && (prognosis == null || prognosis.isEmpty()) && (plan == null || plan.isEmpty()) 2081 && (action == null || action.isEmpty()); 2082 } 2083 2084 @Override 2085 public ResourceType getResourceType() { 2086 return ResourceType.ClinicalImpression; 2087 } 2088 2089 @SearchParamDefinition(name = "date", path = "ClinicalImpression.date", description = "When the assessment occurred", type = "date") 2090 public static final String SP_DATE = "date"; 2091 @SearchParamDefinition(name = "previous", path = "ClinicalImpression.previous", description = "Reference to last assessment", type = "reference") 2092 public static final String SP_PREVIOUS = "previous"; 2093 @SearchParamDefinition(name = "assessor", path = "ClinicalImpression.assessor", description = "The clinician performing the assessment", type = "reference") 2094 public static final String SP_ASSESSOR = "assessor"; 2095 @SearchParamDefinition(name = "trigger", path = "ClinicalImpression.triggerReference", description = "Request or event that necessitated this assessment", type = "reference") 2096 public static final String SP_TRIGGER = "trigger"; 2097 @SearchParamDefinition(name = "finding", path = "ClinicalImpression.finding.item", description = "Specific text or code for finding", type = "token") 2098 public static final String SP_FINDING = "finding"; 2099 @SearchParamDefinition(name = "ruledout", path = "ClinicalImpression.ruledOut.item", description = "Specific text of code for diagnosis", type = "token") 2100 public static final String SP_RULEDOUT = "ruledout"; 2101 @SearchParamDefinition(name = "problem", path = "ClinicalImpression.problem", description = "General assessment of patient state", type = "reference") 2102 public static final String SP_PROBLEM = "problem"; 2103 @SearchParamDefinition(name = "patient", path = "ClinicalImpression.patient", description = "The patient being assessed", type = "reference") 2104 public static final String SP_PATIENT = "patient"; 2105 @SearchParamDefinition(name = "investigation", path = "ClinicalImpression.investigations.item", description = "Record of a specific investigation", type = "reference") 2106 public static final String SP_INVESTIGATION = "investigation"; 2107 @SearchParamDefinition(name = "action", path = "ClinicalImpression.action", description = "Actions taken during assessment", type = "reference") 2108 public static final String SP_ACTION = "action"; 2109 @SearchParamDefinition(name = "trigger-code", path = "ClinicalImpression.triggerCodeableConcept", description = "Request or event that necessitated this assessment", type = "token") 2110 public static final String SP_TRIGGERCODE = "trigger-code"; 2111 @SearchParamDefinition(name = "plan", path = "ClinicalImpression.plan", description = "Plan of action after assessment", type = "reference") 2112 public static final String SP_PLAN = "plan"; 2113 @SearchParamDefinition(name = "resolved", path = "ClinicalImpression.resolved", description = "Diagnoses/conditions resolved since previous assessment", type = "token") 2114 public static final String SP_RESOLVED = "resolved"; 2115 @SearchParamDefinition(name = "status", path = "ClinicalImpression.status", description = "in-progress | completed | entered-in-error", type = "token") 2116 public static final String SP_STATUS = "status"; 2117 2118}