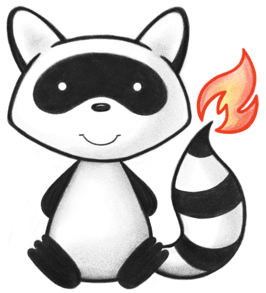
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.List; 035 036import ca.uhn.fhir.model.api.annotation.Child; 037import ca.uhn.fhir.model.api.annotation.DatatypeDef; 038import ca.uhn.fhir.model.api.annotation.Description; 039import org.hl7.fhir.instance.model.api.ICompositeType; 040import org.hl7.fhir.dstu2.model.Coding; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.utilities.Utilities; 043 044/** 045 * A concept that may be defined by a formal reference to a terminology or 046 * ontology or may be provided by text. 047 */ 048@DatatypeDef(name = "CodeableConcept") 049public class CodeableConcept extends Type implements ICompositeType { 050 051 /** 052 * A reference to a code defined by a terminology system. 053 */ 054 @Child(name = "coding", type = { 055 Coding.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 056 @Description(shortDefinition = "Code defined by a terminology system", formalDefinition = "A reference to a code defined by a terminology system.") 057 protected List<Coding> coding; 058 059 /** 060 * A human language representation of the concept as seen/selected/uttered by 061 * the user who entered the data and/or which represents the intended meaning of 062 * the user. 063 */ 064 @Child(name = "text", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 065 @Description(shortDefinition = "Plain text representation of the concept", formalDefinition = "A human language representation of the concept as seen/selected/uttered by the user who entered the data and/or which represents the intended meaning of the user.") 066 protected StringType text; 067 068 private static final long serialVersionUID = 760353246L; 069 070 /* 071 * Constructor 072 */ 073 public CodeableConcept() { 074 super(); 075 } 076 077 /** 078 * @return {@link #coding} (A reference to a code defined by a terminology 079 * system.) 080 */ 081 public List<Coding> getCoding() { 082 if (this.coding == null) 083 this.coding = new ArrayList<Coding>(); 084 return this.coding; 085 } 086 087 public boolean hasCoding() { 088 if (this.coding == null) 089 return false; 090 for (Coding item : this.coding) 091 if (!item.isEmpty()) 092 return true; 093 return false; 094 } 095 096 /** 097 * @return {@link #coding} (A reference to a code defined by a terminology 098 * system.) 099 */ 100 // syntactic sugar 101 public Coding addCoding() { // 3 102 Coding t = new Coding(); 103 if (this.coding == null) 104 this.coding = new ArrayList<Coding>(); 105 this.coding.add(t); 106 return t; 107 } 108 109 // syntactic sugar 110 public CodeableConcept addCoding(Coding t) { // 3 111 if (t == null) 112 return this; 113 if (this.coding == null) 114 this.coding = new ArrayList<Coding>(); 115 this.coding.add(t); 116 return this; 117 } 118 119 /** 120 * @return {@link #text} (A human language representation of the concept as 121 * seen/selected/uttered by the user who entered the data and/or which 122 * represents the intended meaning of the user.). This is the underlying 123 * object with id, value and extensions. The accessor "getText" gives 124 * direct access to the value 125 */ 126 public StringType getTextElement() { 127 if (this.text == null) 128 if (Configuration.errorOnAutoCreate()) 129 throw new Error("Attempt to auto-create CodeableConcept.text"); 130 else if (Configuration.doAutoCreate()) 131 this.text = new StringType(); // bb 132 return this.text; 133 } 134 135 public boolean hasTextElement() { 136 return this.text != null && !this.text.isEmpty(); 137 } 138 139 public boolean hasText() { 140 return this.text != null && !this.text.isEmpty(); 141 } 142 143 /** 144 * @param value {@link #text} (A human language representation of the concept as 145 * seen/selected/uttered by the user who entered the data and/or 146 * which represents the intended meaning of the user.). This is the 147 * underlying object with id, value and extensions. The accessor 148 * "getText" gives direct access to the value 149 */ 150 public CodeableConcept setTextElement(StringType value) { 151 this.text = value; 152 return this; 153 } 154 155 /** 156 * @return A human language representation of the concept as 157 * seen/selected/uttered by the user who entered the data and/or which 158 * represents the intended meaning of the user. 159 */ 160 public String getText() { 161 return this.text == null ? null : this.text.getValue(); 162 } 163 164 /** 165 * @param value A human language representation of the concept as 166 * seen/selected/uttered by the user who entered the data and/or 167 * which represents the intended meaning of the user. 168 */ 169 public CodeableConcept setText(String value) { 170 if (Utilities.noString(value)) 171 this.text = null; 172 else { 173 if (this.text == null) 174 this.text = new StringType(); 175 this.text.setValue(value); 176 } 177 return this; 178 } 179 180 protected void listChildren(List<Property> childrenList) { 181 super.listChildren(childrenList); 182 childrenList.add(new Property("coding", "Coding", "A reference to a code defined by a terminology system.", 0, 183 java.lang.Integer.MAX_VALUE, coding)); 184 childrenList.add(new Property("text", "string", 185 "A human language representation of the concept as seen/selected/uttered by the user who entered the data and/or which represents the intended meaning of the user.", 186 0, java.lang.Integer.MAX_VALUE, text)); 187 } 188 189 @Override 190 public void setProperty(String name, Base value) throws FHIRException { 191 if (name.equals("coding")) 192 this.getCoding().add(castToCoding(value)); 193 else if (name.equals("text")) 194 this.text = castToString(value); // StringType 195 else 196 super.setProperty(name, value); 197 } 198 199 @Override 200 public Base addChild(String name) throws FHIRException { 201 if (name.equals("coding")) { 202 return addCoding(); 203 } else if (name.equals("text")) { 204 throw new FHIRException("Cannot call addChild on a singleton property CodeableConcept.text"); 205 } else 206 return super.addChild(name); 207 } 208 209 public String fhirType() { 210 return "CodeableConcept"; 211 212 } 213 214 public CodeableConcept copy() { 215 CodeableConcept dst = new CodeableConcept(); 216 copyValues(dst); 217 if (coding != null) { 218 dst.coding = new ArrayList<Coding>(); 219 for (Coding i : coding) 220 dst.coding.add(i.copy()); 221 } 222 ; 223 dst.text = text == null ? null : text.copy(); 224 return dst; 225 } 226 227 protected CodeableConcept typedCopy() { 228 return copy(); 229 } 230 231 @Override 232 public boolean equalsDeep(Base other) { 233 if (!super.equalsDeep(other)) 234 return false; 235 if (!(other instanceof CodeableConcept)) 236 return false; 237 CodeableConcept o = (CodeableConcept) other; 238 return compareDeep(coding, o.coding, true) && compareDeep(text, o.text, true); 239 } 240 241 @Override 242 public boolean equalsShallow(Base other) { 243 if (!super.equalsShallow(other)) 244 return false; 245 if (!(other instanceof CodeableConcept)) 246 return false; 247 CodeableConcept o = (CodeableConcept) other; 248 return compareValues(text, o.text, true); 249 } 250 251 public boolean isEmpty() { 252 return super.isEmpty() && (coding == null || coding.isEmpty()) && (text == null || text.isEmpty()); 253 } 254 255 // added from java-adornments.txt: 256 257 public boolean hasCoding(String system, String code) { 258 for (Coding c : getCoding()) { 259 if (system.equals(c.getSystem()) && code.equals(c.getCode())) 260 return true; 261 } 262 return false; 263 } 264 265 public CodeableConcept(Coding code) { 266 super(); 267 addCoding(code); 268 } 269 270 // end addition 271 272}