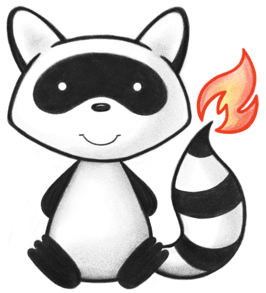
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.List; 034 035import ca.uhn.fhir.model.api.annotation.Child; 036import ca.uhn.fhir.model.api.annotation.DatatypeDef; 037import ca.uhn.fhir.model.api.annotation.Description; 038import org.hl7.fhir.instance.model.api.IBaseCoding; 039import org.hl7.fhir.instance.model.api.ICompositeType; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.utilities.Utilities; 042 043/** 044 * A reference to a code defined by a terminology system. 045 */ 046@DatatypeDef(name = "Coding") 047public class Coding extends Type implements IBaseCoding, ICompositeType { 048 049 /** 050 * The identification of the code system that defines the meaning of the symbol 051 * in the code. 052 */ 053 @Child(name = "system", type = { UriType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 054 @Description(shortDefinition = "Identity of the terminology system", formalDefinition = "The identification of the code system that defines the meaning of the symbol in the code.") 055 protected UriType system; 056 057 /** 058 * The version of the code system which was used when choosing this code. Note 059 * that a well-maintained code system does not need the version reported, 060 * because the meaning of codes is consistent across versions. However this 061 * cannot consistently be assured. and when the meaning is not guaranteed to be 062 * consistent, the version SHOULD be exchanged. 063 */ 064 @Child(name = "version", type = { StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 065 @Description(shortDefinition = "Version of the system - if relevant", formalDefinition = "The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured. and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.") 066 protected StringType version; 067 068 /** 069 * A symbol in syntax defined by the system. The symbol may be a predefined code 070 * or an expression in a syntax defined by the coding system (e.g. 071 * post-coordination). 072 */ 073 @Child(name = "code", type = { CodeType.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 074 @Description(shortDefinition = "Symbol in syntax defined by the system", formalDefinition = "A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination).") 075 protected CodeType code; 076 077 /** 078 * A representation of the meaning of the code in the system, following the 079 * rules of the system. 080 */ 081 @Child(name = "display", type = { StringType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 082 @Description(shortDefinition = "Representation defined by the system", formalDefinition = "A representation of the meaning of the code in the system, following the rules of the system.") 083 protected StringType display; 084 085 /** 086 * Indicates that this coding was chosen by a user directly - i.e. off a pick 087 * list of available items (codes or displays). 088 */ 089 @Child(name = "userSelected", type = { 090 BooleanType.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 091 @Description(shortDefinition = "If this coding was chosen directly by the user", formalDefinition = "Indicates that this coding was chosen by a user directly - i.e. off a pick list of available items (codes or displays).") 092 protected BooleanType userSelected; 093 094 private static final long serialVersionUID = -1417514061L; 095 096 /* 097 * Constructor 098 */ 099 public Coding() { 100 super(); 101 } 102 103 /** 104 * @return {@link #system} (The identification of the code system that defines 105 * the meaning of the symbol in the code.). This is the underlying 106 * object with id, value and extensions. The accessor "getSystem" gives 107 * direct access to the value 108 */ 109 public UriType getSystemElement() { 110 if (this.system == null) 111 if (Configuration.errorOnAutoCreate()) 112 throw new Error("Attempt to auto-create Coding.system"); 113 else if (Configuration.doAutoCreate()) 114 this.system = new UriType(); // bb 115 return this.system; 116 } 117 118 public boolean hasSystemElement() { 119 return this.system != null && !this.system.isEmpty(); 120 } 121 122 public boolean hasSystem() { 123 return this.system != null && !this.system.isEmpty(); 124 } 125 126 /** 127 * @param value {@link #system} (The identification of the code system that 128 * defines the meaning of the symbol in the code.). This is the 129 * underlying object with id, value and extensions. The accessor 130 * "getSystem" gives direct access to the value 131 */ 132 public Coding setSystemElement(UriType value) { 133 this.system = value; 134 return this; 135 } 136 137 /** 138 * @return The identification of the code system that defines the meaning of the 139 * symbol in the code. 140 */ 141 public String getSystem() { 142 return this.system == null ? null : this.system.getValue(); 143 } 144 145 /** 146 * @param value The identification of the code system that defines the meaning 147 * of the symbol in the code. 148 */ 149 public Coding setSystem(String value) { 150 if (Utilities.noString(value)) 151 this.system = null; 152 else { 153 if (this.system == null) 154 this.system = new UriType(); 155 this.system.setValue(value); 156 } 157 return this; 158 } 159 160 /** 161 * @return {@link #version} (The version of the code system which was used when 162 * choosing this code. Note that a well-maintained code system does not 163 * need the version reported, because the meaning of codes is consistent 164 * across versions. However this cannot consistently be assured. and 165 * when the meaning is not guaranteed to be consistent, the version 166 * SHOULD be exchanged.). This is the underlying object with id, value 167 * and extensions. The accessor "getVersion" gives direct access to the 168 * value 169 */ 170 public StringType getVersionElement() { 171 if (this.version == null) 172 if (Configuration.errorOnAutoCreate()) 173 throw new Error("Attempt to auto-create Coding.version"); 174 else if (Configuration.doAutoCreate()) 175 this.version = new StringType(); // bb 176 return this.version; 177 } 178 179 public boolean hasVersionElement() { 180 return this.version != null && !this.version.isEmpty(); 181 } 182 183 public boolean hasVersion() { 184 return this.version != null && !this.version.isEmpty(); 185 } 186 187 /** 188 * @param value {@link #version} (The version of the code system which was used 189 * when choosing this code. Note that a well-maintained code system 190 * does not need the version reported, because the meaning of codes 191 * is consistent across versions. However this cannot consistently 192 * be assured. and when the meaning is not guaranteed to be 193 * consistent, the version SHOULD be exchanged.). This is the 194 * underlying object with id, value and extensions. The accessor 195 * "getVersion" gives direct access to the value 196 */ 197 public Coding setVersionElement(StringType value) { 198 this.version = value; 199 return this; 200 } 201 202 /** 203 * @return The version of the code system which was used when choosing this 204 * code. Note that a well-maintained code system does not need the 205 * version reported, because the meaning of codes is consistent across 206 * versions. However this cannot consistently be assured. and when the 207 * meaning is not guaranteed to be consistent, the version SHOULD be 208 * exchanged. 209 */ 210 public String getVersion() { 211 return this.version == null ? null : this.version.getValue(); 212 } 213 214 /** 215 * @param value The version of the code system which was used when choosing this 216 * code. Note that a well-maintained code system does not need the 217 * version reported, because the meaning of codes is consistent 218 * across versions. However this cannot consistently be assured. 219 * and when the meaning is not guaranteed to be consistent, the 220 * version SHOULD be exchanged. 221 */ 222 public Coding setVersion(String value) { 223 if (Utilities.noString(value)) 224 this.version = null; 225 else { 226 if (this.version == null) 227 this.version = new StringType(); 228 this.version.setValue(value); 229 } 230 return this; 231 } 232 233 /** 234 * @return {@link #code} (A symbol in syntax defined by the system. The symbol 235 * may be a predefined code or an expression in a syntax defined by the 236 * coding system (e.g. post-coordination).). This is the underlying 237 * object with id, value and extensions. The accessor "getCode" gives 238 * direct access to the value 239 */ 240 public CodeType getCodeElement() { 241 if (this.code == null) 242 if (Configuration.errorOnAutoCreate()) 243 throw new Error("Attempt to auto-create Coding.code"); 244 else if (Configuration.doAutoCreate()) 245 this.code = new CodeType(); // bb 246 return this.code; 247 } 248 249 public boolean hasCodeElement() { 250 return this.code != null && !this.code.isEmpty(); 251 } 252 253 public boolean hasCode() { 254 return this.code != null && !this.code.isEmpty(); 255 } 256 257 /** 258 * @param value {@link #code} (A symbol in syntax defined by the system. The 259 * symbol may be a predefined code or an expression in a syntax 260 * defined by the coding system (e.g. post-coordination).). This is 261 * the underlying object with id, value and extensions. The 262 * accessor "getCode" gives direct access to the value 263 */ 264 public Coding setCodeElement(CodeType value) { 265 this.code = value; 266 return this; 267 } 268 269 /** 270 * @return A symbol in syntax defined by the system. The symbol may be a 271 * predefined code or an expression in a syntax defined by the coding 272 * system (e.g. post-coordination). 273 */ 274 public String getCode() { 275 return this.code == null ? null : this.code.getValue(); 276 } 277 278 /** 279 * @param value A symbol in syntax defined by the system. The symbol may be a 280 * predefined code or an expression in a syntax defined by the 281 * coding system (e.g. post-coordination). 282 */ 283 public Coding setCode(String value) { 284 if (Utilities.noString(value)) 285 this.code = null; 286 else { 287 if (this.code == null) 288 this.code = new CodeType(); 289 this.code.setValue(value); 290 } 291 return this; 292 } 293 294 /** 295 * @return {@link #display} (A representation of the meaning of the code in the 296 * system, following the rules of the system.). This is the underlying 297 * object with id, value and extensions. The accessor "getDisplay" gives 298 * direct access to the value 299 */ 300 public StringType getDisplayElement() { 301 if (this.display == null) 302 if (Configuration.errorOnAutoCreate()) 303 throw new Error("Attempt to auto-create Coding.display"); 304 else if (Configuration.doAutoCreate()) 305 this.display = new StringType(); // bb 306 return this.display; 307 } 308 309 public boolean hasDisplayElement() { 310 return this.display != null && !this.display.isEmpty(); 311 } 312 313 public boolean hasDisplay() { 314 return this.display != null && !this.display.isEmpty(); 315 } 316 317 /** 318 * @param value {@link #display} (A representation of the meaning of the code in 319 * the system, following the rules of the system.). This is the 320 * underlying object with id, value and extensions. The accessor 321 * "getDisplay" gives direct access to the value 322 */ 323 public Coding setDisplayElement(StringType value) { 324 this.display = value; 325 return this; 326 } 327 328 /** 329 * @return A representation of the meaning of the code in the system, following 330 * the rules of the system. 331 */ 332 public String getDisplay() { 333 return this.display == null ? null : this.display.getValue(); 334 } 335 336 /** 337 * @param value A representation of the meaning of the code in the system, 338 * following the rules of the system. 339 */ 340 public Coding setDisplay(String value) { 341 if (Utilities.noString(value)) 342 this.display = null; 343 else { 344 if (this.display == null) 345 this.display = new StringType(); 346 this.display.setValue(value); 347 } 348 return this; 349 } 350 351 /** 352 * @return {@link #userSelected} (Indicates that this coding was chosen by a 353 * user directly - i.e. off a pick list of available items (codes or 354 * displays).). This is the underlying object with id, value and 355 * extensions. The accessor "getUserSelected" gives direct access to the 356 * value 357 */ 358 public BooleanType getUserSelectedElement() { 359 if (this.userSelected == null) 360 if (Configuration.errorOnAutoCreate()) 361 throw new Error("Attempt to auto-create Coding.userSelected"); 362 else if (Configuration.doAutoCreate()) 363 this.userSelected = new BooleanType(); // bb 364 return this.userSelected; 365 } 366 367 public boolean hasUserSelectedElement() { 368 return this.userSelected != null && !this.userSelected.isEmpty(); 369 } 370 371 public boolean hasUserSelected() { 372 return this.userSelected != null && !this.userSelected.isEmpty(); 373 } 374 375 /** 376 * @param value {@link #userSelected} (Indicates that this coding was chosen by 377 * a user directly - i.e. off a pick list of available items (codes 378 * or displays).). This is the underlying object with id, value and 379 * extensions. The accessor "getUserSelected" gives direct access 380 * to the value 381 */ 382 public Coding setUserSelectedElement(BooleanType value) { 383 this.userSelected = value; 384 return this; 385 } 386 387 /** 388 * @return Indicates that this coding was chosen by a user directly - i.e. off a 389 * pick list of available items (codes or displays). 390 */ 391 public boolean getUserSelected() { 392 return this.userSelected == null || this.userSelected.isEmpty() ? false : this.userSelected.getValue(); 393 } 394 395 /** 396 * @param value Indicates that this coding was chosen by a user directly - i.e. 397 * off a pick list of available items (codes or displays). 398 */ 399 public Coding setUserSelected(boolean value) { 400 if (this.userSelected == null) 401 this.userSelected = new BooleanType(); 402 this.userSelected.setValue(value); 403 return this; 404 } 405 406 protected void listChildren(List<Property> childrenList) { 407 super.listChildren(childrenList); 408 childrenList.add(new Property("system", "uri", 409 "The identification of the code system that defines the meaning of the symbol in the code.", 0, 410 java.lang.Integer.MAX_VALUE, system)); 411 childrenList.add(new Property("version", "string", 412 "The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured. and when the meaning is not guaranteed to be consistent, the version SHOULD be exchanged.", 413 0, java.lang.Integer.MAX_VALUE, version)); 414 childrenList.add(new Property("code", "code", 415 "A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination).", 416 0, java.lang.Integer.MAX_VALUE, code)); 417 childrenList.add(new Property("display", "string", 418 "A representation of the meaning of the code in the system, following the rules of the system.", 0, 419 java.lang.Integer.MAX_VALUE, display)); 420 childrenList.add(new Property("userSelected", "boolean", 421 "Indicates that this coding was chosen by a user directly - i.e. off a pick list of available items (codes or displays).", 422 0, java.lang.Integer.MAX_VALUE, userSelected)); 423 } 424 425 @Override 426 public void setProperty(String name, Base value) throws FHIRException { 427 if (name.equals("system")) 428 this.system = castToUri(value); // UriType 429 else if (name.equals("version")) 430 this.version = castToString(value); // StringType 431 else if (name.equals("code")) 432 this.code = castToCode(value); // CodeType 433 else if (name.equals("display")) 434 this.display = castToString(value); // StringType 435 else if (name.equals("userSelected")) 436 this.userSelected = castToBoolean(value); // BooleanType 437 else 438 super.setProperty(name, value); 439 } 440 441 @Override 442 public Base addChild(String name) throws FHIRException { 443 if (name.equals("system")) { 444 throw new FHIRException("Cannot call addChild on a singleton property Coding.system"); 445 } else if (name.equals("version")) { 446 throw new FHIRException("Cannot call addChild on a singleton property Coding.version"); 447 } else if (name.equals("code")) { 448 throw new FHIRException("Cannot call addChild on a singleton property Coding.code"); 449 } else if (name.equals("display")) { 450 throw new FHIRException("Cannot call addChild on a singleton property Coding.display"); 451 } else if (name.equals("userSelected")) { 452 throw new FHIRException("Cannot call addChild on a singleton property Coding.userSelected"); 453 } else 454 return super.addChild(name); 455 } 456 457 public String fhirType() { 458 return "Coding"; 459 460 } 461 462 public Coding copy() { 463 Coding dst = new Coding(); 464 copyValues(dst); 465 dst.system = system == null ? null : system.copy(); 466 dst.version = version == null ? null : version.copy(); 467 dst.code = code == null ? null : code.copy(); 468 dst.display = display == null ? null : display.copy(); 469 dst.userSelected = userSelected == null ? null : userSelected.copy(); 470 return dst; 471 } 472 473 protected Coding typedCopy() { 474 return copy(); 475 } 476 477 @Override 478 public boolean equalsDeep(Base other) { 479 if (!super.equalsDeep(other)) 480 return false; 481 if (!(other instanceof Coding)) 482 return false; 483 Coding o = (Coding) other; 484 return compareDeep(system, o.system, true) && compareDeep(version, o.version, true) 485 && compareDeep(code, o.code, true) && compareDeep(display, o.display, true) 486 && compareDeep(userSelected, o.userSelected, true); 487 } 488 489 @Override 490 public boolean equalsShallow(Base other) { 491 if (!super.equalsShallow(other)) 492 return false; 493 if (!(other instanceof Coding)) 494 return false; 495 Coding o = (Coding) other; 496 return compareValues(system, o.system, true) && compareValues(version, o.version, true) 497 && compareValues(code, o.code, true) && compareValues(display, o.display, true) 498 && compareValues(userSelected, o.userSelected, true); 499 } 500 501 public boolean isEmpty() { 502 return super.isEmpty() && (system == null || system.isEmpty()) && (version == null || version.isEmpty()) 503 && (code == null || code.isEmpty()) && (display == null || display.isEmpty()) 504 && (userSelected == null || userSelected.isEmpty()); 505 } 506 507}