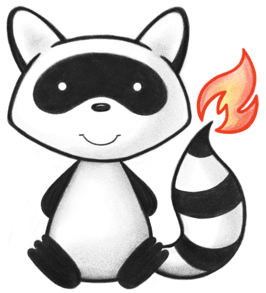
001package org.hl7.fhir.dstu2.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jul 13, 2016 05:32+1000 for FHIR v1.0.2 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import ca.uhn.fhir.model.api.annotation.Block; 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.exceptions.FHIRException; 044 045/** 046 * An occurrence of information being transmitted; e.g. an alert that was sent 047 * to a responsible provider, a public health agency was notified about a 048 * reportable condition. 049 */ 050@ResourceDef(name = "Communication", profile = "http://hl7.org/fhir/Profile/Communication") 051public class Communication extends DomainResource { 052 053 public enum CommunicationStatus { 054 /** 055 * The communication transmission is ongoing. 056 */ 057 INPROGRESS, 058 /** 059 * The message transmission is complete, i.e., delivered to the recipient's 060 * destination. 061 */ 062 COMPLETED, 063 /** 064 * The communication transmission has been held by originating system/user 065 * request. 066 */ 067 SUSPENDED, 068 /** 069 * The receiving system has declined to accept the message. 070 */ 071 REJECTED, 072 /** 073 * There was a failure in transmitting the message out. 074 */ 075 FAILED, 076 /** 077 * added to help the parsers 078 */ 079 NULL; 080 081 public static CommunicationStatus fromCode(String codeString) throws FHIRException { 082 if (codeString == null || "".equals(codeString)) 083 return null; 084 if ("in-progress".equals(codeString)) 085 return INPROGRESS; 086 if ("completed".equals(codeString)) 087 return COMPLETED; 088 if ("suspended".equals(codeString)) 089 return SUSPENDED; 090 if ("rejected".equals(codeString)) 091 return REJECTED; 092 if ("failed".equals(codeString)) 093 return FAILED; 094 throw new FHIRException("Unknown CommunicationStatus code '" + codeString + "'"); 095 } 096 097 public String toCode() { 098 switch (this) { 099 case INPROGRESS: 100 return "in-progress"; 101 case COMPLETED: 102 return "completed"; 103 case SUSPENDED: 104 return "suspended"; 105 case REJECTED: 106 return "rejected"; 107 case FAILED: 108 return "failed"; 109 case NULL: 110 return null; 111 default: 112 return "?"; 113 } 114 } 115 116 public String getSystem() { 117 switch (this) { 118 case INPROGRESS: 119 return "http://hl7.org/fhir/communication-status"; 120 case COMPLETED: 121 return "http://hl7.org/fhir/communication-status"; 122 case SUSPENDED: 123 return "http://hl7.org/fhir/communication-status"; 124 case REJECTED: 125 return "http://hl7.org/fhir/communication-status"; 126 case FAILED: 127 return "http://hl7.org/fhir/communication-status"; 128 case NULL: 129 return null; 130 default: 131 return "?"; 132 } 133 } 134 135 public String getDefinition() { 136 switch (this) { 137 case INPROGRESS: 138 return "The communication transmission is ongoing."; 139 case COMPLETED: 140 return "The message transmission is complete, i.e., delivered to the recipient's destination."; 141 case SUSPENDED: 142 return "The communication transmission has been held by originating system/user request."; 143 case REJECTED: 144 return "The receiving system has declined to accept the message."; 145 case FAILED: 146 return "There was a failure in transmitting the message out."; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 154 public String getDisplay() { 155 switch (this) { 156 case INPROGRESS: 157 return "In Progress"; 158 case COMPLETED: 159 return "Completed"; 160 case SUSPENDED: 161 return "Suspended"; 162 case REJECTED: 163 return "Rejected"; 164 case FAILED: 165 return "Failed"; 166 case NULL: 167 return null; 168 default: 169 return "?"; 170 } 171 } 172 } 173 174 public static class CommunicationStatusEnumFactory implements EnumFactory<CommunicationStatus> { 175 public CommunicationStatus fromCode(String codeString) throws IllegalArgumentException { 176 if (codeString == null || "".equals(codeString)) 177 if (codeString == null || "".equals(codeString)) 178 return null; 179 if ("in-progress".equals(codeString)) 180 return CommunicationStatus.INPROGRESS; 181 if ("completed".equals(codeString)) 182 return CommunicationStatus.COMPLETED; 183 if ("suspended".equals(codeString)) 184 return CommunicationStatus.SUSPENDED; 185 if ("rejected".equals(codeString)) 186 return CommunicationStatus.REJECTED; 187 if ("failed".equals(codeString)) 188 return CommunicationStatus.FAILED; 189 throw new IllegalArgumentException("Unknown CommunicationStatus code '" + codeString + "'"); 190 } 191 192 public Enumeration<CommunicationStatus> fromType(Base code) throws FHIRException { 193 if (code == null || code.isEmpty()) 194 return null; 195 String codeString = ((PrimitiveType) code).asStringValue(); 196 if (codeString == null || "".equals(codeString)) 197 return null; 198 if ("in-progress".equals(codeString)) 199 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.INPROGRESS); 200 if ("completed".equals(codeString)) 201 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.COMPLETED); 202 if ("suspended".equals(codeString)) 203 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.SUSPENDED); 204 if ("rejected".equals(codeString)) 205 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.REJECTED); 206 if ("failed".equals(codeString)) 207 return new Enumeration<CommunicationStatus>(this, CommunicationStatus.FAILED); 208 throw new FHIRException("Unknown CommunicationStatus code '" + codeString + "'"); 209 } 210 211 public String toCode(CommunicationStatus code) 212 { 213 if (code == CommunicationStatus.NULL) 214 return null; 215 if (code == CommunicationStatus.INPROGRESS) 216 return "in-progress"; 217 if (code == CommunicationStatus.COMPLETED) 218 return "completed"; 219 if (code == CommunicationStatus.SUSPENDED) 220 return "suspended"; 221 if (code == CommunicationStatus.REJECTED) 222 return "rejected"; 223 if (code == CommunicationStatus.FAILED) 224 return "failed"; 225 return "?"; 226 } 227 } 228 229 @Block() 230 public static class CommunicationPayloadComponent extends BackboneElement implements IBaseBackboneElement { 231 /** 232 * A communicated content (or for multi-part communications, one portion of the 233 * communication). 234 */ 235 @Child(name = "content", type = { StringType.class, 236 Attachment.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 237 @Description(shortDefinition = "Message part content", formalDefinition = "A communicated content (or for multi-part communications, one portion of the communication).") 238 protected Type content; 239 240 private static final long serialVersionUID = -1763459053L; 241 242 /* 243 * Constructor 244 */ 245 public CommunicationPayloadComponent() { 246 super(); 247 } 248 249 /* 250 * Constructor 251 */ 252 public CommunicationPayloadComponent(Type content) { 253 super(); 254 this.content = content; 255 } 256 257 /** 258 * @return {@link #content} (A communicated content (or for multi-part 259 * communications, one portion of the communication).) 260 */ 261 public Type getContent() { 262 return this.content; 263 } 264 265 /** 266 * @return {@link #content} (A communicated content (or for multi-part 267 * communications, one portion of the communication).) 268 */ 269 public StringType getContentStringType() throws FHIRException { 270 if (!(this.content instanceof StringType)) 271 throw new FHIRException("Type mismatch: the type StringType was expected, but " 272 + this.content.getClass().getName() + " was encountered"); 273 return (StringType) this.content; 274 } 275 276 public boolean hasContentStringType() { 277 return this.content instanceof StringType; 278 } 279 280 /** 281 * @return {@link #content} (A communicated content (or for multi-part 282 * communications, one portion of the communication).) 283 */ 284 public Attachment getContentAttachment() throws FHIRException { 285 if (!(this.content instanceof Attachment)) 286 throw new FHIRException("Type mismatch: the type Attachment was expected, but " 287 + this.content.getClass().getName() + " was encountered"); 288 return (Attachment) this.content; 289 } 290 291 public boolean hasContentAttachment() { 292 return this.content instanceof Attachment; 293 } 294 295 /** 296 * @return {@link #content} (A communicated content (or for multi-part 297 * communications, one portion of the communication).) 298 */ 299 public Reference getContentReference() throws FHIRException { 300 if (!(this.content instanceof Reference)) 301 throw new FHIRException("Type mismatch: the type Reference was expected, but " 302 + this.content.getClass().getName() + " was encountered"); 303 return (Reference) this.content; 304 } 305 306 public boolean hasContentReference() { 307 return this.content instanceof Reference; 308 } 309 310 public boolean hasContent() { 311 return this.content != null && !this.content.isEmpty(); 312 } 313 314 /** 315 * @param value {@link #content} (A communicated content (or for multi-part 316 * communications, one portion of the communication).) 317 */ 318 public CommunicationPayloadComponent setContent(Type value) { 319 this.content = value; 320 return this; 321 } 322 323 protected void listChildren(List<Property> childrenList) { 324 super.listChildren(childrenList); 325 childrenList.add(new Property("content[x]", "string|Attachment|Reference(Any)", 326 "A communicated content (or for multi-part communications, one portion of the communication).", 0, 327 java.lang.Integer.MAX_VALUE, content)); 328 } 329 330 @Override 331 public void setProperty(String name, Base value) throws FHIRException { 332 if (name.equals("content[x]")) 333 this.content = (Type) value; // Type 334 else 335 super.setProperty(name, value); 336 } 337 338 @Override 339 public Base addChild(String name) throws FHIRException { 340 if (name.equals("contentString")) { 341 this.content = new StringType(); 342 return this.content; 343 } else if (name.equals("contentAttachment")) { 344 this.content = new Attachment(); 345 return this.content; 346 } else if (name.equals("contentReference")) { 347 this.content = new Reference(); 348 return this.content; 349 } else 350 return super.addChild(name); 351 } 352 353 public CommunicationPayloadComponent copy() { 354 CommunicationPayloadComponent dst = new CommunicationPayloadComponent(); 355 copyValues(dst); 356 dst.content = content == null ? null : content.copy(); 357 return dst; 358 } 359 360 @Override 361 public boolean equalsDeep(Base other) { 362 if (!super.equalsDeep(other)) 363 return false; 364 if (!(other instanceof CommunicationPayloadComponent)) 365 return false; 366 CommunicationPayloadComponent o = (CommunicationPayloadComponent) other; 367 return compareDeep(content, o.content, true); 368 } 369 370 @Override 371 public boolean equalsShallow(Base other) { 372 if (!super.equalsShallow(other)) 373 return false; 374 if (!(other instanceof CommunicationPayloadComponent)) 375 return false; 376 CommunicationPayloadComponent o = (CommunicationPayloadComponent) other; 377 return true; 378 } 379 380 public boolean isEmpty() { 381 return super.isEmpty() && (content == null || content.isEmpty()); 382 } 383 384 public String fhirType() { 385 return "Communication.payload"; 386 387 } 388 389 } 390 391 /** 392 * Identifiers associated with this Communication that are defined by business 393 * processes and/ or used to refer to it when a direct URL reference to the 394 * resource itself is not appropriate (e.g. in CDA documents, or in written / 395 * printed documentation). 396 */ 397 @Child(name = "identifier", type = { 398 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 399 @Description(shortDefinition = "Unique identifier", formalDefinition = "Identifiers associated with this Communication that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).") 400 protected List<Identifier> identifier; 401 402 /** 403 * The type of message conveyed such as alert, notification, reminder, 404 * instruction, etc. 405 */ 406 @Child(name = "category", type = { 407 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 408 @Description(shortDefinition = "Message category", formalDefinition = "The type of message conveyed such as alert, notification, reminder, instruction, etc.") 409 protected CodeableConcept category; 410 411 /** 412 * The entity (e.g. person, organization, clinical information system, or 413 * device) which was the source of the communication. 414 */ 415 @Child(name = "sender", type = { Device.class, Organization.class, Patient.class, Practitioner.class, 416 RelatedPerson.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 417 @Description(shortDefinition = "Message sender", formalDefinition = "The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.") 418 protected Reference sender; 419 420 /** 421 * The actual object that is the target of the reference (The entity (e.g. 422 * person, organization, clinical information system, or device) which was the 423 * source of the communication.) 424 */ 425 protected Resource senderTarget; 426 427 /** 428 * The entity (e.g. person, organization, clinical information system, or 429 * device) which was the target of the communication. If receipts need to be 430 * tracked by individual, a separate resource instance will need to be created 431 * for each recipient. Multiple recipient communications are intended where 432 * either a receipt(s) is not tracked (e.g. a mass mail-out) or is captured in 433 * aggregate (all emails confirmed received by a particular time). 434 */ 435 @Child(name = "recipient", type = { Device.class, Organization.class, Patient.class, Practitioner.class, 436 RelatedPerson.class, 437 Group.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 438 @Description(shortDefinition = "Message recipient", formalDefinition = "The entity (e.g. person, organization, clinical information system, or device) which was the target of the communication. If receipts need to be tracked by individual, a separate resource instance will need to be created for each recipient. Multiple recipient communications are intended where either a receipt(s) is not tracked (e.g. a mass mail-out) or is captured in aggregate (all emails confirmed received by a particular time).") 439 protected List<Reference> recipient; 440 /** 441 * The actual objects that are the target of the reference (The entity (e.g. 442 * person, organization, clinical information system, or device) which was the 443 * target of the communication. If receipts need to be tracked by individual, a 444 * separate resource instance will need to be created for each recipient. 445 * Multiple recipient communications are intended where either a receipt(s) is 446 * not tracked (e.g. a mass mail-out) or is captured in aggregate (all emails 447 * confirmed received by a particular time).) 448 */ 449 protected List<Resource> recipientTarget; 450 451 /** 452 * Text, attachment(s), or resource(s) that was communicated to the recipient. 453 */ 454 @Child(name = "payload", type = {}, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 455 @Description(shortDefinition = "Message payload", formalDefinition = "Text, attachment(s), or resource(s) that was communicated to the recipient.") 456 protected List<CommunicationPayloadComponent> payload; 457 458 /** 459 * A channel that was used for this communication (e.g. email, fax). 460 */ 461 @Child(name = "medium", type = { 462 CodeableConcept.class }, order = 5, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 463 @Description(shortDefinition = "A channel of communication", formalDefinition = "A channel that was used for this communication (e.g. email, fax).") 464 protected List<CodeableConcept> medium; 465 466 /** 467 * The status of the transmission. 468 */ 469 @Child(name = "status", type = { CodeType.class }, order = 6, min = 0, max = 1, modifier = true, summary = true) 470 @Description(shortDefinition = "in-progress | completed | suspended | rejected | failed", formalDefinition = "The status of the transmission.") 471 protected Enumeration<CommunicationStatus> status; 472 473 /** 474 * The encounter within which the communication was sent. 475 */ 476 @Child(name = "encounter", type = { Encounter.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 477 @Description(shortDefinition = "Encounter leading to message", formalDefinition = "The encounter within which the communication was sent.") 478 protected Reference encounter; 479 480 /** 481 * The actual object that is the target of the reference (The encounter within 482 * which the communication was sent.) 483 */ 484 protected Encounter encounterTarget; 485 486 /** 487 * The time when this communication was sent. 488 */ 489 @Child(name = "sent", type = { DateTimeType.class }, order = 8, min = 0, max = 1, modifier = false, summary = true) 490 @Description(shortDefinition = "When sent", formalDefinition = "The time when this communication was sent.") 491 protected DateTimeType sent; 492 493 /** 494 * The time when this communication arrived at the destination. 495 */ 496 @Child(name = "received", type = { 497 DateTimeType.class }, order = 9, min = 0, max = 1, modifier = false, summary = true) 498 @Description(shortDefinition = "When received", formalDefinition = "The time when this communication arrived at the destination.") 499 protected DateTimeType received; 500 501 /** 502 * The reason or justification for the communication. 503 */ 504 @Child(name = "reason", type = { 505 CodeableConcept.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 506 @Description(shortDefinition = "Indication for message", formalDefinition = "The reason or justification for the communication.") 507 protected List<CodeableConcept> reason; 508 509 /** 510 * The patient who was the focus of this communication. 511 */ 512 @Child(name = "subject", type = { Patient.class }, order = 11, min = 0, max = 1, modifier = false, summary = true) 513 @Description(shortDefinition = "Focus of message", formalDefinition = "The patient who was the focus of this communication.") 514 protected Reference subject; 515 516 /** 517 * The actual object that is the target of the reference (The patient who was 518 * the focus of this communication.) 519 */ 520 protected Patient subjectTarget; 521 522 /** 523 * The communication request that was responsible for producing this 524 * communication. 525 */ 526 @Child(name = "requestDetail", type = { 527 CommunicationRequest.class }, order = 12, min = 0, max = 1, modifier = false, summary = true) 528 @Description(shortDefinition = "CommunicationRequest producing this message", formalDefinition = "The communication request that was responsible for producing this communication.") 529 protected Reference requestDetail; 530 531 /** 532 * The actual object that is the target of the reference (The communication 533 * request that was responsible for producing this communication.) 534 */ 535 protected CommunicationRequest requestDetailTarget; 536 537 private static final long serialVersionUID = -1654449146L; 538 539 /* 540 * Constructor 541 */ 542 public Communication() { 543 super(); 544 } 545 546 /** 547 * @return {@link #identifier} (Identifiers associated with this Communication 548 * that are defined by business processes and/ or used to refer to it 549 * when a direct URL reference to the resource itself is not appropriate 550 * (e.g. in CDA documents, or in written / printed documentation).) 551 */ 552 public List<Identifier> getIdentifier() { 553 if (this.identifier == null) 554 this.identifier = new ArrayList<Identifier>(); 555 return this.identifier; 556 } 557 558 public boolean hasIdentifier() { 559 if (this.identifier == null) 560 return false; 561 for (Identifier item : this.identifier) 562 if (!item.isEmpty()) 563 return true; 564 return false; 565 } 566 567 /** 568 * @return {@link #identifier} (Identifiers associated with this Communication 569 * that are defined by business processes and/ or used to refer to it 570 * when a direct URL reference to the resource itself is not appropriate 571 * (e.g. in CDA documents, or in written / printed documentation).) 572 */ 573 // syntactic sugar 574 public Identifier addIdentifier() { // 3 575 Identifier t = new Identifier(); 576 if (this.identifier == null) 577 this.identifier = new ArrayList<Identifier>(); 578 this.identifier.add(t); 579 return t; 580 } 581 582 // syntactic sugar 583 public Communication addIdentifier(Identifier t) { // 3 584 if (t == null) 585 return this; 586 if (this.identifier == null) 587 this.identifier = new ArrayList<Identifier>(); 588 this.identifier.add(t); 589 return this; 590 } 591 592 /** 593 * @return {@link #category} (The type of message conveyed such as alert, 594 * notification, reminder, instruction, etc.) 595 */ 596 public CodeableConcept getCategory() { 597 if (this.category == null) 598 if (Configuration.errorOnAutoCreate()) 599 throw new Error("Attempt to auto-create Communication.category"); 600 else if (Configuration.doAutoCreate()) 601 this.category = new CodeableConcept(); // cc 602 return this.category; 603 } 604 605 public boolean hasCategory() { 606 return this.category != null && !this.category.isEmpty(); 607 } 608 609 /** 610 * @param value {@link #category} (The type of message conveyed such as alert, 611 * notification, reminder, instruction, etc.) 612 */ 613 public Communication setCategory(CodeableConcept value) { 614 this.category = value; 615 return this; 616 } 617 618 /** 619 * @return {@link #sender} (The entity (e.g. person, organization, clinical 620 * information system, or device) which was the source of the 621 * communication.) 622 */ 623 public Reference getSender() { 624 if (this.sender == null) 625 if (Configuration.errorOnAutoCreate()) 626 throw new Error("Attempt to auto-create Communication.sender"); 627 else if (Configuration.doAutoCreate()) 628 this.sender = new Reference(); // cc 629 return this.sender; 630 } 631 632 public boolean hasSender() { 633 return this.sender != null && !this.sender.isEmpty(); 634 } 635 636 /** 637 * @param value {@link #sender} (The entity (e.g. person, organization, clinical 638 * information system, or device) which was the source of the 639 * communication.) 640 */ 641 public Communication setSender(Reference value) { 642 this.sender = value; 643 return this; 644 } 645 646 /** 647 * @return {@link #sender} The actual object that is the target of the 648 * reference. The reference library doesn't populate this, but you can 649 * use it to hold the resource if you resolve it. (The entity (e.g. 650 * person, organization, clinical information system, or device) which 651 * was the source of the communication.) 652 */ 653 public Resource getSenderTarget() { 654 return this.senderTarget; 655 } 656 657 /** 658 * @param value {@link #sender} The actual object that is the target of the 659 * reference. The reference library doesn't use these, but you can 660 * use it to hold the resource if you resolve it. (The entity (e.g. 661 * person, organization, clinical information system, or device) 662 * which was the source of the communication.) 663 */ 664 public Communication setSenderTarget(Resource value) { 665 this.senderTarget = value; 666 return this; 667 } 668 669 /** 670 * @return {@link #recipient} (The entity (e.g. person, organization, clinical 671 * information system, or device) which was the target of the 672 * communication. If receipts need to be tracked by individual, a 673 * separate resource instance will need to be created for each 674 * recipient. Multiple recipient communications are intended where 675 * either a receipt(s) is not tracked (e.g. a mass mail-out) or is 676 * captured in aggregate (all emails confirmed received by a particular 677 * time).) 678 */ 679 public List<Reference> getRecipient() { 680 if (this.recipient == null) 681 this.recipient = new ArrayList<Reference>(); 682 return this.recipient; 683 } 684 685 public boolean hasRecipient() { 686 if (this.recipient == null) 687 return false; 688 for (Reference item : this.recipient) 689 if (!item.isEmpty()) 690 return true; 691 return false; 692 } 693 694 /** 695 * @return {@link #recipient} (The entity (e.g. person, organization, clinical 696 * information system, or device) which was the target of the 697 * communication. If receipts need to be tracked by individual, a 698 * separate resource instance will need to be created for each 699 * recipient. Multiple recipient communications are intended where 700 * either a receipt(s) is not tracked (e.g. a mass mail-out) or is 701 * captured in aggregate (all emails confirmed received by a particular 702 * time).) 703 */ 704 // syntactic sugar 705 public Reference addRecipient() { // 3 706 Reference t = new Reference(); 707 if (this.recipient == null) 708 this.recipient = new ArrayList<Reference>(); 709 this.recipient.add(t); 710 return t; 711 } 712 713 // syntactic sugar 714 public Communication addRecipient(Reference t) { // 3 715 if (t == null) 716 return this; 717 if (this.recipient == null) 718 this.recipient = new ArrayList<Reference>(); 719 this.recipient.add(t); 720 return this; 721 } 722 723 /** 724 * @return {@link #recipient} (The actual objects that are the target of the 725 * reference. The reference library doesn't populate this, but you can 726 * use this to hold the resources if you resolvethemt. The entity (e.g. 727 * person, organization, clinical information system, or device) which 728 * was the target of the communication. If receipts need to be tracked 729 * by individual, a separate resource instance will need to be created 730 * for each recipient. Multiple recipient communications are intended 731 * where either a receipt(s) is not tracked (e.g. a mass mail-out) or is 732 * captured in aggregate (all emails confirmed received by a particular 733 * time).) 734 */ 735 public List<Resource> getRecipientTarget() { 736 if (this.recipientTarget == null) 737 this.recipientTarget = new ArrayList<Resource>(); 738 return this.recipientTarget; 739 } 740 741 /** 742 * @return {@link #payload} (Text, attachment(s), or resource(s) that was 743 * communicated to the recipient.) 744 */ 745 public List<CommunicationPayloadComponent> getPayload() { 746 if (this.payload == null) 747 this.payload = new ArrayList<CommunicationPayloadComponent>(); 748 return this.payload; 749 } 750 751 public boolean hasPayload() { 752 if (this.payload == null) 753 return false; 754 for (CommunicationPayloadComponent item : this.payload) 755 if (!item.isEmpty()) 756 return true; 757 return false; 758 } 759 760 /** 761 * @return {@link #payload} (Text, attachment(s), or resource(s) that was 762 * communicated to the recipient.) 763 */ 764 // syntactic sugar 765 public CommunicationPayloadComponent addPayload() { // 3 766 CommunicationPayloadComponent t = new CommunicationPayloadComponent(); 767 if (this.payload == null) 768 this.payload = new ArrayList<CommunicationPayloadComponent>(); 769 this.payload.add(t); 770 return t; 771 } 772 773 // syntactic sugar 774 public Communication addPayload(CommunicationPayloadComponent t) { // 3 775 if (t == null) 776 return this; 777 if (this.payload == null) 778 this.payload = new ArrayList<CommunicationPayloadComponent>(); 779 this.payload.add(t); 780 return this; 781 } 782 783 /** 784 * @return {@link #medium} (A channel that was used for this communication (e.g. 785 * email, fax).) 786 */ 787 public List<CodeableConcept> getMedium() { 788 if (this.medium == null) 789 this.medium = new ArrayList<CodeableConcept>(); 790 return this.medium; 791 } 792 793 public boolean hasMedium() { 794 if (this.medium == null) 795 return false; 796 for (CodeableConcept item : this.medium) 797 if (!item.isEmpty()) 798 return true; 799 return false; 800 } 801 802 /** 803 * @return {@link #medium} (A channel that was used for this communication (e.g. 804 * email, fax).) 805 */ 806 // syntactic sugar 807 public CodeableConcept addMedium() { // 3 808 CodeableConcept t = new CodeableConcept(); 809 if (this.medium == null) 810 this.medium = new ArrayList<CodeableConcept>(); 811 this.medium.add(t); 812 return t; 813 } 814 815 // syntactic sugar 816 public Communication addMedium(CodeableConcept t) { // 3 817 if (t == null) 818 return this; 819 if (this.medium == null) 820 this.medium = new ArrayList<CodeableConcept>(); 821 this.medium.add(t); 822 return this; 823 } 824 825 /** 826 * @return {@link #status} (The status of the transmission.). This is the 827 * underlying object with id, value and extensions. The accessor 828 * "getStatus" gives direct access to the value 829 */ 830 public Enumeration<CommunicationStatus> getStatusElement() { 831 if (this.status == null) 832 if (Configuration.errorOnAutoCreate()) 833 throw new Error("Attempt to auto-create Communication.status"); 834 else if (Configuration.doAutoCreate()) 835 this.status = new Enumeration<CommunicationStatus>(new CommunicationStatusEnumFactory()); // bb 836 return this.status; 837 } 838 839 public boolean hasStatusElement() { 840 return this.status != null && !this.status.isEmpty(); 841 } 842 843 public boolean hasStatus() { 844 return this.status != null && !this.status.isEmpty(); 845 } 846 847 /** 848 * @param value {@link #status} (The status of the transmission.). This is the 849 * underlying object with id, value and extensions. The accessor 850 * "getStatus" gives direct access to the value 851 */ 852 public Communication setStatusElement(Enumeration<CommunicationStatus> value) { 853 this.status = value; 854 return this; 855 } 856 857 /** 858 * @return The status of the transmission. 859 */ 860 public CommunicationStatus getStatus() { 861 return this.status == null ? null : this.status.getValue(); 862 } 863 864 /** 865 * @param value The status of the transmission. 866 */ 867 public Communication setStatus(CommunicationStatus value) { 868 if (value == null) 869 this.status = null; 870 else { 871 if (this.status == null) 872 this.status = new Enumeration<CommunicationStatus>(new CommunicationStatusEnumFactory()); 873 this.status.setValue(value); 874 } 875 return this; 876 } 877 878 /** 879 * @return {@link #encounter} (The encounter within which the communication was 880 * sent.) 881 */ 882 public Reference getEncounter() { 883 if (this.encounter == null) 884 if (Configuration.errorOnAutoCreate()) 885 throw new Error("Attempt to auto-create Communication.encounter"); 886 else if (Configuration.doAutoCreate()) 887 this.encounter = new Reference(); // cc 888 return this.encounter; 889 } 890 891 public boolean hasEncounter() { 892 return this.encounter != null && !this.encounter.isEmpty(); 893 } 894 895 /** 896 * @param value {@link #encounter} (The encounter within which the communication 897 * was sent.) 898 */ 899 public Communication setEncounter(Reference value) { 900 this.encounter = value; 901 return this; 902 } 903 904 /** 905 * @return {@link #encounter} The actual object that is the target of the 906 * reference. The reference library doesn't populate this, but you can 907 * use it to hold the resource if you resolve it. (The encounter within 908 * which the communication was sent.) 909 */ 910 public Encounter getEncounterTarget() { 911 if (this.encounterTarget == null) 912 if (Configuration.errorOnAutoCreate()) 913 throw new Error("Attempt to auto-create Communication.encounter"); 914 else if (Configuration.doAutoCreate()) 915 this.encounterTarget = new Encounter(); // aa 916 return this.encounterTarget; 917 } 918 919 /** 920 * @param value {@link #encounter} The actual object that is the target of the 921 * reference. The reference library doesn't use these, but you can 922 * use it to hold the resource if you resolve it. (The encounter 923 * within which the communication was sent.) 924 */ 925 public Communication setEncounterTarget(Encounter value) { 926 this.encounterTarget = value; 927 return this; 928 } 929 930 /** 931 * @return {@link #sent} (The time when this communication was sent.). This is 932 * the underlying object with id, value and extensions. The accessor 933 * "getSent" gives direct access to the value 934 */ 935 public DateTimeType getSentElement() { 936 if (this.sent == null) 937 if (Configuration.errorOnAutoCreate()) 938 throw new Error("Attempt to auto-create Communication.sent"); 939 else if (Configuration.doAutoCreate()) 940 this.sent = new DateTimeType(); // bb 941 return this.sent; 942 } 943 944 public boolean hasSentElement() { 945 return this.sent != null && !this.sent.isEmpty(); 946 } 947 948 public boolean hasSent() { 949 return this.sent != null && !this.sent.isEmpty(); 950 } 951 952 /** 953 * @param value {@link #sent} (The time when this communication was sent.). This 954 * is the underlying object with id, value and extensions. The 955 * accessor "getSent" gives direct access to the value 956 */ 957 public Communication setSentElement(DateTimeType value) { 958 this.sent = value; 959 return this; 960 } 961 962 /** 963 * @return The time when this communication was sent. 964 */ 965 public Date getSent() { 966 return this.sent == null ? null : this.sent.getValue(); 967 } 968 969 /** 970 * @param value The time when this communication was sent. 971 */ 972 public Communication setSent(Date value) { 973 if (value == null) 974 this.sent = null; 975 else { 976 if (this.sent == null) 977 this.sent = new DateTimeType(); 978 this.sent.setValue(value); 979 } 980 return this; 981 } 982 983 /** 984 * @return {@link #received} (The time when this communication arrived at the 985 * destination.). This is the underlying object with id, value and 986 * extensions. The accessor "getReceived" gives direct access to the 987 * value 988 */ 989 public DateTimeType getReceivedElement() { 990 if (this.received == null) 991 if (Configuration.errorOnAutoCreate()) 992 throw new Error("Attempt to auto-create Communication.received"); 993 else if (Configuration.doAutoCreate()) 994 this.received = new DateTimeType(); // bb 995 return this.received; 996 } 997 998 public boolean hasReceivedElement() { 999 return this.received != null && !this.received.isEmpty(); 1000 } 1001 1002 public boolean hasReceived() { 1003 return this.received != null && !this.received.isEmpty(); 1004 } 1005 1006 /** 1007 * @param value {@link #received} (The time when this communication arrived at 1008 * the destination.). This is the underlying object with id, value 1009 * and extensions. The accessor "getReceived" gives direct access 1010 * to the value 1011 */ 1012 public Communication setReceivedElement(DateTimeType value) { 1013 this.received = value; 1014 return this; 1015 } 1016 1017 /** 1018 * @return The time when this communication arrived at the destination. 1019 */ 1020 public Date getReceived() { 1021 return this.received == null ? null : this.received.getValue(); 1022 } 1023 1024 /** 1025 * @param value The time when this communication arrived at the destination. 1026 */ 1027 public Communication setReceived(Date value) { 1028 if (value == null) 1029 this.received = null; 1030 else { 1031 if (this.received == null) 1032 this.received = new DateTimeType(); 1033 this.received.setValue(value); 1034 } 1035 return this; 1036 } 1037 1038 /** 1039 * @return {@link #reason} (The reason or justification for the communication.) 1040 */ 1041 public List<CodeableConcept> getReason() { 1042 if (this.reason == null) 1043 this.reason = new ArrayList<CodeableConcept>(); 1044 return this.reason; 1045 } 1046 1047 public boolean hasReason() { 1048 if (this.reason == null) 1049 return false; 1050 for (CodeableConcept item : this.reason) 1051 if (!item.isEmpty()) 1052 return true; 1053 return false; 1054 } 1055 1056 /** 1057 * @return {@link #reason} (The reason or justification for the communication.) 1058 */ 1059 // syntactic sugar 1060 public CodeableConcept addReason() { // 3 1061 CodeableConcept t = new CodeableConcept(); 1062 if (this.reason == null) 1063 this.reason = new ArrayList<CodeableConcept>(); 1064 this.reason.add(t); 1065 return t; 1066 } 1067 1068 // syntactic sugar 1069 public Communication addReason(CodeableConcept t) { // 3 1070 if (t == null) 1071 return this; 1072 if (this.reason == null) 1073 this.reason = new ArrayList<CodeableConcept>(); 1074 this.reason.add(t); 1075 return this; 1076 } 1077 1078 /** 1079 * @return {@link #subject} (The patient who was the focus of this 1080 * communication.) 1081 */ 1082 public Reference getSubject() { 1083 if (this.subject == null) 1084 if (Configuration.errorOnAutoCreate()) 1085 throw new Error("Attempt to auto-create Communication.subject"); 1086 else if (Configuration.doAutoCreate()) 1087 this.subject = new Reference(); // cc 1088 return this.subject; 1089 } 1090 1091 public boolean hasSubject() { 1092 return this.subject != null && !this.subject.isEmpty(); 1093 } 1094 1095 /** 1096 * @param value {@link #subject} (The patient who was the focus of this 1097 * communication.) 1098 */ 1099 public Communication setSubject(Reference value) { 1100 this.subject = value; 1101 return this; 1102 } 1103 1104 /** 1105 * @return {@link #subject} The actual object that is the target of the 1106 * reference. The reference library doesn't populate this, but you can 1107 * use it to hold the resource if you resolve it. (The patient who was 1108 * the focus of this communication.) 1109 */ 1110 public Patient getSubjectTarget() { 1111 if (this.subjectTarget == null) 1112 if (Configuration.errorOnAutoCreate()) 1113 throw new Error("Attempt to auto-create Communication.subject"); 1114 else if (Configuration.doAutoCreate()) 1115 this.subjectTarget = new Patient(); // aa 1116 return this.subjectTarget; 1117 } 1118 1119 /** 1120 * @param value {@link #subject} The actual object that is the target of the 1121 * reference. The reference library doesn't use these, but you can 1122 * use it to hold the resource if you resolve it. (The patient who 1123 * was the focus of this communication.) 1124 */ 1125 public Communication setSubjectTarget(Patient value) { 1126 this.subjectTarget = value; 1127 return this; 1128 } 1129 1130 /** 1131 * @return {@link #requestDetail} (The communication request that was 1132 * responsible for producing this communication.) 1133 */ 1134 public Reference getRequestDetail() { 1135 if (this.requestDetail == null) 1136 if (Configuration.errorOnAutoCreate()) 1137 throw new Error("Attempt to auto-create Communication.requestDetail"); 1138 else if (Configuration.doAutoCreate()) 1139 this.requestDetail = new Reference(); // cc 1140 return this.requestDetail; 1141 } 1142 1143 public boolean hasRequestDetail() { 1144 return this.requestDetail != null && !this.requestDetail.isEmpty(); 1145 } 1146 1147 /** 1148 * @param value {@link #requestDetail} (The communication request that was 1149 * responsible for producing this communication.) 1150 */ 1151 public Communication setRequestDetail(Reference value) { 1152 this.requestDetail = value; 1153 return this; 1154 } 1155 1156 /** 1157 * @return {@link #requestDetail} The actual object that is the target of the 1158 * reference. The reference library doesn't populate this, but you can 1159 * use it to hold the resource if you resolve it. (The communication 1160 * request that was responsible for producing this communication.) 1161 */ 1162 public CommunicationRequest getRequestDetailTarget() { 1163 if (this.requestDetailTarget == null) 1164 if (Configuration.errorOnAutoCreate()) 1165 throw new Error("Attempt to auto-create Communication.requestDetail"); 1166 else if (Configuration.doAutoCreate()) 1167 this.requestDetailTarget = new CommunicationRequest(); // aa 1168 return this.requestDetailTarget; 1169 } 1170 1171 /** 1172 * @param value {@link #requestDetail} The actual object that is the target of 1173 * the reference. The reference library doesn't use these, but you 1174 * can use it to hold the resource if you resolve it. (The 1175 * communication request that was responsible for producing this 1176 * communication.) 1177 */ 1178 public Communication setRequestDetailTarget(CommunicationRequest value) { 1179 this.requestDetailTarget = value; 1180 return this; 1181 } 1182 1183 protected void listChildren(List<Property> childrenList) { 1184 super.listChildren(childrenList); 1185 childrenList.add(new Property("identifier", "Identifier", 1186 "Identifiers associated with this Communication that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 1187 0, java.lang.Integer.MAX_VALUE, identifier)); 1188 childrenList.add(new Property("category", "CodeableConcept", 1189 "The type of message conveyed such as alert, notification, reminder, instruction, etc.", 0, 1190 java.lang.Integer.MAX_VALUE, category)); 1191 childrenList.add(new Property("sender", "Reference(Device|Organization|Patient|Practitioner|RelatedPerson)", 1192 "The entity (e.g. person, organization, clinical information system, or device) which was the source of the communication.", 1193 0, java.lang.Integer.MAX_VALUE, sender)); 1194 childrenList.add(new Property("recipient", 1195 "Reference(Device|Organization|Patient|Practitioner|RelatedPerson|Group)", 1196 "The entity (e.g. person, organization, clinical information system, or device) which was the target of the communication. If receipts need to be tracked by individual, a separate resource instance will need to be created for each recipient. Multiple recipient communications are intended where either a receipt(s) is not tracked (e.g. a mass mail-out) or is captured in aggregate (all emails confirmed received by a particular time).", 1197 0, java.lang.Integer.MAX_VALUE, recipient)); 1198 childrenList 1199 .add(new Property("payload", "", "Text, attachment(s), or resource(s) that was communicated to the recipient.", 1200 0, java.lang.Integer.MAX_VALUE, payload)); 1201 childrenList.add(new Property("medium", "CodeableConcept", 1202 "A channel that was used for this communication (e.g. email, fax).", 0, java.lang.Integer.MAX_VALUE, medium)); 1203 childrenList 1204 .add(new Property("status", "code", "The status of the transmission.", 0, java.lang.Integer.MAX_VALUE, status)); 1205 childrenList.add(new Property("encounter", "Reference(Encounter)", 1206 "The encounter within which the communication was sent.", 0, java.lang.Integer.MAX_VALUE, encounter)); 1207 childrenList.add(new Property("sent", "dateTime", "The time when this communication was sent.", 0, 1208 java.lang.Integer.MAX_VALUE, sent)); 1209 childrenList.add(new Property("received", "dateTime", 1210 "The time when this communication arrived at the destination.", 0, java.lang.Integer.MAX_VALUE, received)); 1211 childrenList.add(new Property("reason", "CodeableConcept", "The reason or justification for the communication.", 0, 1212 java.lang.Integer.MAX_VALUE, reason)); 1213 childrenList.add(new Property("subject", "Reference(Patient)", 1214 "The patient who was the focus of this communication.", 0, java.lang.Integer.MAX_VALUE, subject)); 1215 childrenList.add(new Property("requestDetail", "Reference(CommunicationRequest)", 1216 "The communication request that was responsible for producing this communication.", 0, 1217 java.lang.Integer.MAX_VALUE, requestDetail)); 1218 } 1219 1220 @Override 1221 public void setProperty(String name, Base value) throws FHIRException { 1222 if (name.equals("identifier")) 1223 this.getIdentifier().add(castToIdentifier(value)); 1224 else if (name.equals("category")) 1225 this.category = castToCodeableConcept(value); // CodeableConcept 1226 else if (name.equals("sender")) 1227 this.sender = castToReference(value); // Reference 1228 else if (name.equals("recipient")) 1229 this.getRecipient().add(castToReference(value)); 1230 else if (name.equals("payload")) 1231 this.getPayload().add((CommunicationPayloadComponent) value); 1232 else if (name.equals("medium")) 1233 this.getMedium().add(castToCodeableConcept(value)); 1234 else if (name.equals("status")) 1235 this.status = new CommunicationStatusEnumFactory().fromType(value); // Enumeration<CommunicationStatus> 1236 else if (name.equals("encounter")) 1237 this.encounter = castToReference(value); // Reference 1238 else if (name.equals("sent")) 1239 this.sent = castToDateTime(value); // DateTimeType 1240 else if (name.equals("received")) 1241 this.received = castToDateTime(value); // DateTimeType 1242 else if (name.equals("reason")) 1243 this.getReason().add(castToCodeableConcept(value)); 1244 else if (name.equals("subject")) 1245 this.subject = castToReference(value); // Reference 1246 else if (name.equals("requestDetail")) 1247 this.requestDetail = castToReference(value); // Reference 1248 else 1249 super.setProperty(name, value); 1250 } 1251 1252 @Override 1253 public Base addChild(String name) throws FHIRException { 1254 if (name.equals("identifier")) { 1255 return addIdentifier(); 1256 } else if (name.equals("category")) { 1257 this.category = new CodeableConcept(); 1258 return this.category; 1259 } else if (name.equals("sender")) { 1260 this.sender = new Reference(); 1261 return this.sender; 1262 } else if (name.equals("recipient")) { 1263 return addRecipient(); 1264 } else if (name.equals("payload")) { 1265 return addPayload(); 1266 } else if (name.equals("medium")) { 1267 return addMedium(); 1268 } else if (name.equals("status")) { 1269 throw new FHIRException("Cannot call addChild on a singleton property Communication.status"); 1270 } else if (name.equals("encounter")) { 1271 this.encounter = new Reference(); 1272 return this.encounter; 1273 } else if (name.equals("sent")) { 1274 throw new FHIRException("Cannot call addChild on a singleton property Communication.sent"); 1275 } else if (name.equals("received")) { 1276 throw new FHIRException("Cannot call addChild on a singleton property Communication.received"); 1277 } else if (name.equals("reason")) { 1278 return addReason(); 1279 } else if (name.equals("subject")) { 1280 this.subject = new Reference(); 1281 return this.subject; 1282 } else if (name.equals("requestDetail")) { 1283 this.requestDetail = new Reference(); 1284 return this.requestDetail; 1285 } else 1286 return super.addChild(name); 1287 } 1288 1289 public String fhirType() { 1290 return "Communication"; 1291 1292 } 1293 1294 public Communication copy() { 1295 Communication dst = new Communication(); 1296 copyValues(dst); 1297 if (identifier != null) { 1298 dst.identifier = new ArrayList<Identifier>(); 1299 for (Identifier i : identifier) 1300 dst.identifier.add(i.copy()); 1301 } 1302 ; 1303 dst.category = category == null ? null : category.copy(); 1304 dst.sender = sender == null ? null : sender.copy(); 1305 if (recipient != null) { 1306 dst.recipient = new ArrayList<Reference>(); 1307 for (Reference i : recipient) 1308 dst.recipient.add(i.copy()); 1309 } 1310 ; 1311 if (payload != null) { 1312 dst.payload = new ArrayList<CommunicationPayloadComponent>(); 1313 for (CommunicationPayloadComponent i : payload) 1314 dst.payload.add(i.copy()); 1315 } 1316 ; 1317 if (medium != null) { 1318 dst.medium = new ArrayList<CodeableConcept>(); 1319 for (CodeableConcept i : medium) 1320 dst.medium.add(i.copy()); 1321 } 1322 ; 1323 dst.status = status == null ? null : status.copy(); 1324 dst.encounter = encounter == null ? null : encounter.copy(); 1325 dst.sent = sent == null ? null : sent.copy(); 1326 dst.received = received == null ? null : received.copy(); 1327 if (reason != null) { 1328 dst.reason = new ArrayList<CodeableConcept>(); 1329 for (CodeableConcept i : reason) 1330 dst.reason.add(i.copy()); 1331 } 1332 ; 1333 dst.subject = subject == null ? null : subject.copy(); 1334 dst.requestDetail = requestDetail == null ? null : requestDetail.copy(); 1335 return dst; 1336 } 1337 1338 protected Communication typedCopy() { 1339 return copy(); 1340 } 1341 1342 @Override 1343 public boolean equalsDeep(Base other) { 1344 if (!super.equalsDeep(other)) 1345 return false; 1346 if (!(other instanceof Communication)) 1347 return false; 1348 Communication o = (Communication) other; 1349 return compareDeep(identifier, o.identifier, true) && compareDeep(category, o.category, true) 1350 && compareDeep(sender, o.sender, true) && compareDeep(recipient, o.recipient, true) 1351 && compareDeep(payload, o.payload, true) && compareDeep(medium, o.medium, true) 1352 && compareDeep(status, o.status, true) && compareDeep(encounter, o.encounter, true) 1353 && compareDeep(sent, o.sent, true) && compareDeep(received, o.received, true) 1354 && compareDeep(reason, o.reason, true) && compareDeep(subject, o.subject, true) 1355 && compareDeep(requestDetail, o.requestDetail, true); 1356 } 1357 1358 @Override 1359 public boolean equalsShallow(Base other) { 1360 if (!super.equalsShallow(other)) 1361 return false; 1362 if (!(other instanceof Communication)) 1363 return false; 1364 Communication o = (Communication) other; 1365 return compareValues(status, o.status, true) && compareValues(sent, o.sent, true) 1366 && compareValues(received, o.received, true); 1367 } 1368 1369 public boolean isEmpty() { 1370 return super.isEmpty() && (identifier == null || identifier.isEmpty()) && (category == null || category.isEmpty()) 1371 && (sender == null || sender.isEmpty()) && (recipient == null || recipient.isEmpty()) 1372 && (payload == null || payload.isEmpty()) && (medium == null || medium.isEmpty()) 1373 && (status == null || status.isEmpty()) && (encounter == null || encounter.isEmpty()) 1374 && (sent == null || sent.isEmpty()) && (received == null || received.isEmpty()) 1375 && (reason == null || reason.isEmpty()) && (subject == null || subject.isEmpty()) 1376 && (requestDetail == null || requestDetail.isEmpty()); 1377 } 1378 1379 @Override 1380 public ResourceType getResourceType() { 1381 return ResourceType.Communication; 1382 } 1383 1384 @SearchParamDefinition(name = "identifier", path = "Communication.identifier", description = "Unique identifier", type = "token") 1385 public static final String SP_IDENTIFIER = "identifier"; 1386 @SearchParamDefinition(name = "request", path = "Communication.requestDetail", description = "CommunicationRequest producing this message", type = "reference") 1387 public static final String SP_REQUEST = "request"; 1388 @SearchParamDefinition(name = "sender", path = "Communication.sender", description = "Message sender", type = "reference") 1389 public static final String SP_SENDER = "sender"; 1390 @SearchParamDefinition(name = "subject", path = "Communication.subject", description = "Focus of message", type = "reference") 1391 public static final String SP_SUBJECT = "subject"; 1392 @SearchParamDefinition(name = "patient", path = "Communication.subject", description = "Focus of message", type = "reference") 1393 public static final String SP_PATIENT = "patient"; 1394 @SearchParamDefinition(name = "recipient", path = "Communication.recipient", description = "Message recipient", type = "reference") 1395 public static final String SP_RECIPIENT = "recipient"; 1396 @SearchParamDefinition(name = "received", path = "Communication.received", description = "When received", type = "date") 1397 public static final String SP_RECEIVED = "received"; 1398 @SearchParamDefinition(name = "medium", path = "Communication.medium", description = "A channel of communication", type = "token") 1399 public static final String SP_MEDIUM = "medium"; 1400 @SearchParamDefinition(name = "encounter", path = "Communication.encounter", description = "Encounter leading to message", type = "reference") 1401 public static final String SP_ENCOUNTER = "encounter"; 1402 @SearchParamDefinition(name = "category", path = "Communication.category", description = "Message category", type = "token") 1403 public static final String SP_CATEGORY = "category"; 1404 @SearchParamDefinition(name = "sent", path = "Communication.sent", description = "When sent", type = "date") 1405 public static final String SP_SENT = "sent"; 1406 @SearchParamDefinition(name = "status", path = "Communication.status", description = "in-progress | completed | suspended | rejected | failed", type = "token") 1407 public static final String SP_STATUS = "status"; 1408 1409}